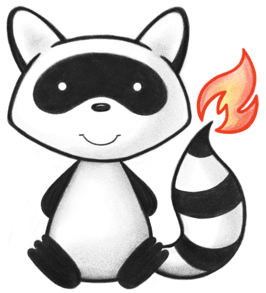
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 051 */ 052@ResourceDef(name="Condition", profile="http://hl7.org/fhir/StructureDefinition/Condition") 053public class Condition extends DomainResource { 054 055 @Block() 056 public static class ConditionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Distinguishes the type of involvement of the actor in the activities related to the condition. 059 */ 060 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="Type of involvement", formalDefinition="Distinguishes the type of involvement of the actor in the activities related to the condition." ) 062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 063 protected CodeableConcept function; 064 065 /** 066 * Indicates who or what participated in the activities related to the condition. 067 */ 068 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Device.class, Organization.class, CareTeam.class}, order=2, min=1, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Who or what participated in the activities related to the condition", formalDefinition="Indicates who or what participated in the activities related to the condition." ) 070 protected Reference actor; 071 072 private static final long serialVersionUID = -576943815L; 073 074 /** 075 * Constructor 076 */ 077 public ConditionParticipantComponent() { 078 super(); 079 } 080 081 /** 082 * Constructor 083 */ 084 public ConditionParticipantComponent(Reference actor) { 085 super(); 086 this.setActor(actor); 087 } 088 089 /** 090 * @return {@link #function} (Distinguishes the type of involvement of the actor in the activities related to the condition.) 091 */ 092 public CodeableConcept getFunction() { 093 if (this.function == null) 094 if (Configuration.errorOnAutoCreate()) 095 throw new Error("Attempt to auto-create ConditionParticipantComponent.function"); 096 else if (Configuration.doAutoCreate()) 097 this.function = new CodeableConcept(); // cc 098 return this.function; 099 } 100 101 public boolean hasFunction() { 102 return this.function != null && !this.function.isEmpty(); 103 } 104 105 /** 106 * @param value {@link #function} (Distinguishes the type of involvement of the actor in the activities related to the condition.) 107 */ 108 public ConditionParticipantComponent setFunction(CodeableConcept value) { 109 this.function = value; 110 return this; 111 } 112 113 /** 114 * @return {@link #actor} (Indicates who or what participated in the activities related to the condition.) 115 */ 116 public Reference getActor() { 117 if (this.actor == null) 118 if (Configuration.errorOnAutoCreate()) 119 throw new Error("Attempt to auto-create ConditionParticipantComponent.actor"); 120 else if (Configuration.doAutoCreate()) 121 this.actor = new Reference(); // cc 122 return this.actor; 123 } 124 125 public boolean hasActor() { 126 return this.actor != null && !this.actor.isEmpty(); 127 } 128 129 /** 130 * @param value {@link #actor} (Indicates who or what participated in the activities related to the condition.) 131 */ 132 public ConditionParticipantComponent setActor(Reference value) { 133 this.actor = value; 134 return this; 135 } 136 137 protected void listChildren(List<Property> children) { 138 super.listChildren(children); 139 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the activities related to the condition.", 0, 1, function)); 140 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device|Organization|CareTeam)", "Indicates who or what participated in the activities related to the condition.", 0, 1, actor)); 141 } 142 143 @Override 144 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 145 switch (_hash) { 146 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the activities related to the condition.", 0, 1, function); 147 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device|Organization|CareTeam)", "Indicates who or what participated in the activities related to the condition.", 0, 1, actor); 148 default: return super.getNamedProperty(_hash, _name, _checkValid); 149 } 150 151 } 152 153 @Override 154 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 155 switch (hash) { 156 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 157 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 158 default: return super.getProperty(hash, name, checkValid); 159 } 160 161 } 162 163 @Override 164 public Base setProperty(int hash, String name, Base value) throws FHIRException { 165 switch (hash) { 166 case 1380938712: // function 167 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 168 return value; 169 case 92645877: // actor 170 this.actor = TypeConvertor.castToReference(value); // Reference 171 return value; 172 default: return super.setProperty(hash, name, value); 173 } 174 175 } 176 177 @Override 178 public Base setProperty(String name, Base value) throws FHIRException { 179 if (name.equals("function")) { 180 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 181 } else if (name.equals("actor")) { 182 this.actor = TypeConvertor.castToReference(value); // Reference 183 } else 184 return super.setProperty(name, value); 185 return value; 186 } 187 188 @Override 189 public void removeChild(String name, Base value) throws FHIRException { 190 if (name.equals("function")) { 191 this.function = null; 192 } else if (name.equals("actor")) { 193 this.actor = null; 194 } else 195 super.removeChild(name, value); 196 197 } 198 199 @Override 200 public Base makeProperty(int hash, String name) throws FHIRException { 201 switch (hash) { 202 case 1380938712: return getFunction(); 203 case 92645877: return getActor(); 204 default: return super.makeProperty(hash, name); 205 } 206 207 } 208 209 @Override 210 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 211 switch (hash) { 212 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 213 case 92645877: /*actor*/ return new String[] {"Reference"}; 214 default: return super.getTypesForProperty(hash, name); 215 } 216 217 } 218 219 @Override 220 public Base addChild(String name) throws FHIRException { 221 if (name.equals("function")) { 222 this.function = new CodeableConcept(); 223 return this.function; 224 } 225 else if (name.equals("actor")) { 226 this.actor = new Reference(); 227 return this.actor; 228 } 229 else 230 return super.addChild(name); 231 } 232 233 public ConditionParticipantComponent copy() { 234 ConditionParticipantComponent dst = new ConditionParticipantComponent(); 235 copyValues(dst); 236 return dst; 237 } 238 239 public void copyValues(ConditionParticipantComponent dst) { 240 super.copyValues(dst); 241 dst.function = function == null ? null : function.copy(); 242 dst.actor = actor == null ? null : actor.copy(); 243 } 244 245 @Override 246 public boolean equalsDeep(Base other_) { 247 if (!super.equalsDeep(other_)) 248 return false; 249 if (!(other_ instanceof ConditionParticipantComponent)) 250 return false; 251 ConditionParticipantComponent o = (ConditionParticipantComponent) other_; 252 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 253 } 254 255 @Override 256 public boolean equalsShallow(Base other_) { 257 if (!super.equalsShallow(other_)) 258 return false; 259 if (!(other_ instanceof ConditionParticipantComponent)) 260 return false; 261 ConditionParticipantComponent o = (ConditionParticipantComponent) other_; 262 return true; 263 } 264 265 public boolean isEmpty() { 266 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 267 } 268 269 public String fhirType() { 270 return "Condition.participant"; 271 272 } 273 274 } 275 276 @Block() 277 public static class ConditionStageComponent extends BackboneElement implements IBaseBackboneElement { 278 /** 279 * A simple summary of the stage such as "Stage 3" or "Early Onset". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease. 280 */ 281 @Child(name = "summary", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 282 @Description(shortDefinition="Simple summary (disease specific)", formalDefinition="A simple summary of the stage such as \"Stage 3\" or \"Early Onset\". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease." ) 283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-stage") 284 protected CodeableConcept summary; 285 286 /** 287 * Reference to a formal record of the evidence on which the staging assessment is based. 288 */ 289 @Child(name = "assessment", type = {ClinicalImpression.class, DiagnosticReport.class, Observation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 290 @Description(shortDefinition="Formal record of assessment", formalDefinition="Reference to a formal record of the evidence on which the staging assessment is based." ) 291 protected List<Reference> assessment; 292 293 /** 294 * The kind of staging, such as pathological or clinical staging. 295 */ 296 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 297 @Description(shortDefinition="Kind of staging", formalDefinition="The kind of staging, such as pathological or clinical staging." ) 298 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-stage-type") 299 protected CodeableConcept type; 300 301 private static final long serialVersionUID = -394541797L; 302 303 /** 304 * Constructor 305 */ 306 public ConditionStageComponent() { 307 super(); 308 } 309 310 /** 311 * @return {@link #summary} (A simple summary of the stage such as "Stage 3" or "Early Onset". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease.) 312 */ 313 public CodeableConcept getSummary() { 314 if (this.summary == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create ConditionStageComponent.summary"); 317 else if (Configuration.doAutoCreate()) 318 this.summary = new CodeableConcept(); // cc 319 return this.summary; 320 } 321 322 public boolean hasSummary() { 323 return this.summary != null && !this.summary.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #summary} (A simple summary of the stage such as "Stage 3" or "Early Onset". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease.) 328 */ 329 public ConditionStageComponent setSummary(CodeableConcept value) { 330 this.summary = value; 331 return this; 332 } 333 334 /** 335 * @return {@link #assessment} (Reference to a formal record of the evidence on which the staging assessment is based.) 336 */ 337 public List<Reference> getAssessment() { 338 if (this.assessment == null) 339 this.assessment = new ArrayList<Reference>(); 340 return this.assessment; 341 } 342 343 /** 344 * @return Returns a reference to <code>this</code> for easy method chaining 345 */ 346 public ConditionStageComponent setAssessment(List<Reference> theAssessment) { 347 this.assessment = theAssessment; 348 return this; 349 } 350 351 public boolean hasAssessment() { 352 if (this.assessment == null) 353 return false; 354 for (Reference item : this.assessment) 355 if (!item.isEmpty()) 356 return true; 357 return false; 358 } 359 360 public Reference addAssessment() { //3 361 Reference t = new Reference(); 362 if (this.assessment == null) 363 this.assessment = new ArrayList<Reference>(); 364 this.assessment.add(t); 365 return t; 366 } 367 368 public ConditionStageComponent addAssessment(Reference t) { //3 369 if (t == null) 370 return this; 371 if (this.assessment == null) 372 this.assessment = new ArrayList<Reference>(); 373 this.assessment.add(t); 374 return this; 375 } 376 377 /** 378 * @return The first repetition of repeating field {@link #assessment}, creating it if it does not already exist {3} 379 */ 380 public Reference getAssessmentFirstRep() { 381 if (getAssessment().isEmpty()) { 382 addAssessment(); 383 } 384 return getAssessment().get(0); 385 } 386 387 /** 388 * @return {@link #type} (The kind of staging, such as pathological or clinical staging.) 389 */ 390 public CodeableConcept getType() { 391 if (this.type == null) 392 if (Configuration.errorOnAutoCreate()) 393 throw new Error("Attempt to auto-create ConditionStageComponent.type"); 394 else if (Configuration.doAutoCreate()) 395 this.type = new CodeableConcept(); // cc 396 return this.type; 397 } 398 399 public boolean hasType() { 400 return this.type != null && !this.type.isEmpty(); 401 } 402 403 /** 404 * @param value {@link #type} (The kind of staging, such as pathological or clinical staging.) 405 */ 406 public ConditionStageComponent setType(CodeableConcept value) { 407 this.type = value; 408 return this; 409 } 410 411 protected void listChildren(List<Property> children) { 412 super.listChildren(children); 413 children.add(new Property("summary", "CodeableConcept", "A simple summary of the stage such as \"Stage 3\" or \"Early Onset\". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease.", 0, 1, summary)); 414 children.add(new Property("assessment", "Reference(ClinicalImpression|DiagnosticReport|Observation)", "Reference to a formal record of the evidence on which the staging assessment is based.", 0, java.lang.Integer.MAX_VALUE, assessment)); 415 children.add(new Property("type", "CodeableConcept", "The kind of staging, such as pathological or clinical staging.", 0, 1, type)); 416 } 417 418 @Override 419 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 420 switch (_hash) { 421 case -1857640538: /*summary*/ return new Property("summary", "CodeableConcept", "A simple summary of the stage such as \"Stage 3\" or \"Early Onset\". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease.", 0, 1, summary); 422 case 2119382722: /*assessment*/ return new Property("assessment", "Reference(ClinicalImpression|DiagnosticReport|Observation)", "Reference to a formal record of the evidence on which the staging assessment is based.", 0, java.lang.Integer.MAX_VALUE, assessment); 423 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of staging, such as pathological or clinical staging.", 0, 1, type); 424 default: return super.getNamedProperty(_hash, _name, _checkValid); 425 } 426 427 } 428 429 @Override 430 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 431 switch (hash) { 432 case -1857640538: /*summary*/ return this.summary == null ? new Base[0] : new Base[] {this.summary}; // CodeableConcept 433 case 2119382722: /*assessment*/ return this.assessment == null ? new Base[0] : this.assessment.toArray(new Base[this.assessment.size()]); // Reference 434 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 435 default: return super.getProperty(hash, name, checkValid); 436 } 437 438 } 439 440 @Override 441 public Base setProperty(int hash, String name, Base value) throws FHIRException { 442 switch (hash) { 443 case -1857640538: // summary 444 this.summary = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 445 return value; 446 case 2119382722: // assessment 447 this.getAssessment().add(TypeConvertor.castToReference(value)); // Reference 448 return value; 449 case 3575610: // type 450 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 451 return value; 452 default: return super.setProperty(hash, name, value); 453 } 454 455 } 456 457 @Override 458 public Base setProperty(String name, Base value) throws FHIRException { 459 if (name.equals("summary")) { 460 this.summary = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 461 } else if (name.equals("assessment")) { 462 this.getAssessment().add(TypeConvertor.castToReference(value)); 463 } else if (name.equals("type")) { 464 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 465 } else 466 return super.setProperty(name, value); 467 return value; 468 } 469 470 @Override 471 public void removeChild(String name, Base value) throws FHIRException { 472 if (name.equals("summary")) { 473 this.summary = null; 474 } else if (name.equals("assessment")) { 475 this.getAssessment().remove(value); 476 } else if (name.equals("type")) { 477 this.type = null; 478 } else 479 super.removeChild(name, value); 480 481 } 482 483 @Override 484 public Base makeProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case -1857640538: return getSummary(); 487 case 2119382722: return addAssessment(); 488 case 3575610: return getType(); 489 default: return super.makeProperty(hash, name); 490 } 491 492 } 493 494 @Override 495 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 496 switch (hash) { 497 case -1857640538: /*summary*/ return new String[] {"CodeableConcept"}; 498 case 2119382722: /*assessment*/ return new String[] {"Reference"}; 499 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 500 default: return super.getTypesForProperty(hash, name); 501 } 502 503 } 504 505 @Override 506 public Base addChild(String name) throws FHIRException { 507 if (name.equals("summary")) { 508 this.summary = new CodeableConcept(); 509 return this.summary; 510 } 511 else if (name.equals("assessment")) { 512 return addAssessment(); 513 } 514 else if (name.equals("type")) { 515 this.type = new CodeableConcept(); 516 return this.type; 517 } 518 else 519 return super.addChild(name); 520 } 521 522 public ConditionStageComponent copy() { 523 ConditionStageComponent dst = new ConditionStageComponent(); 524 copyValues(dst); 525 return dst; 526 } 527 528 public void copyValues(ConditionStageComponent dst) { 529 super.copyValues(dst); 530 dst.summary = summary == null ? null : summary.copy(); 531 if (assessment != null) { 532 dst.assessment = new ArrayList<Reference>(); 533 for (Reference i : assessment) 534 dst.assessment.add(i.copy()); 535 }; 536 dst.type = type == null ? null : type.copy(); 537 } 538 539 @Override 540 public boolean equalsDeep(Base other_) { 541 if (!super.equalsDeep(other_)) 542 return false; 543 if (!(other_ instanceof ConditionStageComponent)) 544 return false; 545 ConditionStageComponent o = (ConditionStageComponent) other_; 546 return compareDeep(summary, o.summary, true) && compareDeep(assessment, o.assessment, true) && compareDeep(type, o.type, true) 547 ; 548 } 549 550 @Override 551 public boolean equalsShallow(Base other_) { 552 if (!super.equalsShallow(other_)) 553 return false; 554 if (!(other_ instanceof ConditionStageComponent)) 555 return false; 556 ConditionStageComponent o = (ConditionStageComponent) other_; 557 return true; 558 } 559 560 public boolean isEmpty() { 561 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(summary, assessment, type 562 ); 563 } 564 565 public String fhirType() { 566 return "Condition.stage"; 567 568 } 569 570 } 571 572 /** 573 * Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 574 */ 575 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 576 @Description(shortDefinition="External Ids for this condition", formalDefinition="Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 577 protected List<Identifier> identifier; 578 579 /** 580 * The clinical status of the condition. 581 */ 582 @Child(name = "clinicalStatus", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=true, summary=true) 583 @Description(shortDefinition="active | recurrence | relapse | inactive | remission | resolved | unknown", formalDefinition="The clinical status of the condition." ) 584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-clinical") 585 protected CodeableConcept clinicalStatus; 586 587 /** 588 * The verification status to support the clinical status of the condition. The verification status pertains to the condition, itself, not to any specific condition attribute. 589 */ 590 @Child(name = "verificationStatus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=true, summary=true) 591 @Description(shortDefinition="unconfirmed | provisional | differential | confirmed | refuted | entered-in-error", formalDefinition="The verification status to support the clinical status of the condition. The verification status pertains to the condition, itself, not to any specific condition attribute." ) 592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-ver-status") 593 protected CodeableConcept verificationStatus; 594 595 /** 596 * A category assigned to the condition. 597 */ 598 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 599 @Description(shortDefinition="problem-list-item | encounter-diagnosis", formalDefinition="A category assigned to the condition." ) 600 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-category") 601 protected List<CodeableConcept> category; 602 603 /** 604 * A subjective assessment of the severity of the condition as evaluated by the clinician. 605 */ 606 @Child(name = "severity", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 607 @Description(shortDefinition="Subjective severity of condition", formalDefinition="A subjective assessment of the severity of the condition as evaluated by the clinician." ) 608 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-severity") 609 protected CodeableConcept severity; 610 611 /** 612 * Identification of the condition, problem or diagnosis. 613 */ 614 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 615 @Description(shortDefinition="Identification of the condition, problem or diagnosis", formalDefinition="Identification of the condition, problem or diagnosis." ) 616 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 617 protected CodeableConcept code; 618 619 /** 620 * The anatomical location where this condition manifests itself. 621 */ 622 @Child(name = "bodySite", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 623 @Description(shortDefinition="Anatomical location, if relevant", formalDefinition="The anatomical location where this condition manifests itself." ) 624 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 625 protected List<CodeableConcept> bodySite; 626 627 /** 628 * Indicates the patient or group who the condition record is associated with. 629 */ 630 @Child(name = "subject", type = {Patient.class, Group.class}, order=7, min=1, max=1, modifier=false, summary=true) 631 @Description(shortDefinition="Who has the condition?", formalDefinition="Indicates the patient or group who the condition record is associated with." ) 632 protected Reference subject; 633 634 /** 635 * The Encounter during which this Condition was created or to which the creation of this record is tightly associated. 636 */ 637 @Child(name = "encounter", type = {Encounter.class}, order=8, min=0, max=1, modifier=false, summary=true) 638 @Description(shortDefinition="The Encounter during which this Condition was created", formalDefinition="The Encounter during which this Condition was created or to which the creation of this record is tightly associated." ) 639 protected Reference encounter; 640 641 /** 642 * Estimated or actual date or date-time the condition began, in the opinion of the clinician. 643 */ 644 @Child(name = "onset", type = {DateTimeType.class, Age.class, Period.class, Range.class, StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 645 @Description(shortDefinition="Estimated or actual date, date-time, or age", formalDefinition="Estimated or actual date or date-time the condition began, in the opinion of the clinician." ) 646 protected DataType onset; 647 648 /** 649 * The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate. 650 */ 651 @Child(name = "abatement", type = {DateTimeType.class, Age.class, Period.class, Range.class, StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 652 @Description(shortDefinition="When in resolution/remission", formalDefinition="The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate." ) 653 protected DataType abatement; 654 655 /** 656 * The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date. 657 */ 658 @Child(name = "recordedDate", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 659 @Description(shortDefinition="Date condition was first recorded", formalDefinition="The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date." ) 660 protected DateTimeType recordedDate; 661 662 /** 663 * Indicates who or what participated in the activities related to the condition and how they were involved. 664 */ 665 @Child(name = "participant", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 666 @Description(shortDefinition="Who or what participated in the activities related to the condition and how they were involved", formalDefinition="Indicates who or what participated in the activities related to the condition and how they were involved." ) 667 protected List<ConditionParticipantComponent> participant; 668 669 /** 670 * A simple summary of the stage such as "Stage 3" or "Early Onset". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease. 671 */ 672 @Child(name = "stage", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 673 @Description(shortDefinition="Stage/grade, usually assessed formally", formalDefinition="A simple summary of the stage such as \"Stage 3\" or \"Early Onset\". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease." ) 674 protected List<ConditionStageComponent> stage; 675 676 /** 677 * Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition. 678 */ 679 @Child(name = "evidence", type = {CodeableReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 680 @Description(shortDefinition="Supporting evidence for the verification status", formalDefinition="Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition." ) 681 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 682 protected List<CodeableReference> evidence; 683 684 /** 685 * Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis. 686 */ 687 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 688 @Description(shortDefinition="Additional information about the Condition", formalDefinition="Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis." ) 689 protected List<Annotation> note; 690 691 private static final long serialVersionUID = -610903427L; 692 693 /** 694 * Constructor 695 */ 696 public Condition() { 697 super(); 698 } 699 700 /** 701 * Constructor 702 */ 703 public Condition(CodeableConcept clinicalStatus, Reference subject) { 704 super(); 705 this.setClinicalStatus(clinicalStatus); 706 this.setSubject(subject); 707 } 708 709 /** 710 * @return {@link #identifier} (Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 711 */ 712 public List<Identifier> getIdentifier() { 713 if (this.identifier == null) 714 this.identifier = new ArrayList<Identifier>(); 715 return this.identifier; 716 } 717 718 /** 719 * @return Returns a reference to <code>this</code> for easy method chaining 720 */ 721 public Condition setIdentifier(List<Identifier> theIdentifier) { 722 this.identifier = theIdentifier; 723 return this; 724 } 725 726 public boolean hasIdentifier() { 727 if (this.identifier == null) 728 return false; 729 for (Identifier item : this.identifier) 730 if (!item.isEmpty()) 731 return true; 732 return false; 733 } 734 735 public Identifier addIdentifier() { //3 736 Identifier t = new Identifier(); 737 if (this.identifier == null) 738 this.identifier = new ArrayList<Identifier>(); 739 this.identifier.add(t); 740 return t; 741 } 742 743 public Condition addIdentifier(Identifier t) { //3 744 if (t == null) 745 return this; 746 if (this.identifier == null) 747 this.identifier = new ArrayList<Identifier>(); 748 this.identifier.add(t); 749 return this; 750 } 751 752 /** 753 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 754 */ 755 public Identifier getIdentifierFirstRep() { 756 if (getIdentifier().isEmpty()) { 757 addIdentifier(); 758 } 759 return getIdentifier().get(0); 760 } 761 762 /** 763 * @return {@link #clinicalStatus} (The clinical status of the condition.) 764 */ 765 public CodeableConcept getClinicalStatus() { 766 if (this.clinicalStatus == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create Condition.clinicalStatus"); 769 else if (Configuration.doAutoCreate()) 770 this.clinicalStatus = new CodeableConcept(); // cc 771 return this.clinicalStatus; 772 } 773 774 public boolean hasClinicalStatus() { 775 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 776 } 777 778 /** 779 * @param value {@link #clinicalStatus} (The clinical status of the condition.) 780 */ 781 public Condition setClinicalStatus(CodeableConcept value) { 782 this.clinicalStatus = value; 783 return this; 784 } 785 786 /** 787 * @return {@link #verificationStatus} (The verification status to support the clinical status of the condition. The verification status pertains to the condition, itself, not to any specific condition attribute.) 788 */ 789 public CodeableConcept getVerificationStatus() { 790 if (this.verificationStatus == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create Condition.verificationStatus"); 793 else if (Configuration.doAutoCreate()) 794 this.verificationStatus = new CodeableConcept(); // cc 795 return this.verificationStatus; 796 } 797 798 public boolean hasVerificationStatus() { 799 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 800 } 801 802 /** 803 * @param value {@link #verificationStatus} (The verification status to support the clinical status of the condition. The verification status pertains to the condition, itself, not to any specific condition attribute.) 804 */ 805 public Condition setVerificationStatus(CodeableConcept value) { 806 this.verificationStatus = value; 807 return this; 808 } 809 810 /** 811 * @return {@link #category} (A category assigned to the condition.) 812 */ 813 public List<CodeableConcept> getCategory() { 814 if (this.category == null) 815 this.category = new ArrayList<CodeableConcept>(); 816 return this.category; 817 } 818 819 /** 820 * @return Returns a reference to <code>this</code> for easy method chaining 821 */ 822 public Condition setCategory(List<CodeableConcept> theCategory) { 823 this.category = theCategory; 824 return this; 825 } 826 827 public boolean hasCategory() { 828 if (this.category == null) 829 return false; 830 for (CodeableConcept item : this.category) 831 if (!item.isEmpty()) 832 return true; 833 return false; 834 } 835 836 public CodeableConcept addCategory() { //3 837 CodeableConcept t = new CodeableConcept(); 838 if (this.category == null) 839 this.category = new ArrayList<CodeableConcept>(); 840 this.category.add(t); 841 return t; 842 } 843 844 public Condition addCategory(CodeableConcept t) { //3 845 if (t == null) 846 return this; 847 if (this.category == null) 848 this.category = new ArrayList<CodeableConcept>(); 849 this.category.add(t); 850 return this; 851 } 852 853 /** 854 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 855 */ 856 public CodeableConcept getCategoryFirstRep() { 857 if (getCategory().isEmpty()) { 858 addCategory(); 859 } 860 return getCategory().get(0); 861 } 862 863 /** 864 * @return {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 865 */ 866 public CodeableConcept getSeverity() { 867 if (this.severity == null) 868 if (Configuration.errorOnAutoCreate()) 869 throw new Error("Attempt to auto-create Condition.severity"); 870 else if (Configuration.doAutoCreate()) 871 this.severity = new CodeableConcept(); // cc 872 return this.severity; 873 } 874 875 public boolean hasSeverity() { 876 return this.severity != null && !this.severity.isEmpty(); 877 } 878 879 /** 880 * @param value {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 881 */ 882 public Condition setSeverity(CodeableConcept value) { 883 this.severity = value; 884 return this; 885 } 886 887 /** 888 * @return {@link #code} (Identification of the condition, problem or diagnosis.) 889 */ 890 public CodeableConcept getCode() { 891 if (this.code == null) 892 if (Configuration.errorOnAutoCreate()) 893 throw new Error("Attempt to auto-create Condition.code"); 894 else if (Configuration.doAutoCreate()) 895 this.code = new CodeableConcept(); // cc 896 return this.code; 897 } 898 899 public boolean hasCode() { 900 return this.code != null && !this.code.isEmpty(); 901 } 902 903 /** 904 * @param value {@link #code} (Identification of the condition, problem or diagnosis.) 905 */ 906 public Condition setCode(CodeableConcept value) { 907 this.code = value; 908 return this; 909 } 910 911 /** 912 * @return {@link #bodySite} (The anatomical location where this condition manifests itself.) 913 */ 914 public List<CodeableConcept> getBodySite() { 915 if (this.bodySite == null) 916 this.bodySite = new ArrayList<CodeableConcept>(); 917 return this.bodySite; 918 } 919 920 /** 921 * @return Returns a reference to <code>this</code> for easy method chaining 922 */ 923 public Condition setBodySite(List<CodeableConcept> theBodySite) { 924 this.bodySite = theBodySite; 925 return this; 926 } 927 928 public boolean hasBodySite() { 929 if (this.bodySite == null) 930 return false; 931 for (CodeableConcept item : this.bodySite) 932 if (!item.isEmpty()) 933 return true; 934 return false; 935 } 936 937 public CodeableConcept addBodySite() { //3 938 CodeableConcept t = new CodeableConcept(); 939 if (this.bodySite == null) 940 this.bodySite = new ArrayList<CodeableConcept>(); 941 this.bodySite.add(t); 942 return t; 943 } 944 945 public Condition addBodySite(CodeableConcept t) { //3 946 if (t == null) 947 return this; 948 if (this.bodySite == null) 949 this.bodySite = new ArrayList<CodeableConcept>(); 950 this.bodySite.add(t); 951 return this; 952 } 953 954 /** 955 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 956 */ 957 public CodeableConcept getBodySiteFirstRep() { 958 if (getBodySite().isEmpty()) { 959 addBodySite(); 960 } 961 return getBodySite().get(0); 962 } 963 964 /** 965 * @return {@link #subject} (Indicates the patient or group who the condition record is associated with.) 966 */ 967 public Reference getSubject() { 968 if (this.subject == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create Condition.subject"); 971 else if (Configuration.doAutoCreate()) 972 this.subject = new Reference(); // cc 973 return this.subject; 974 } 975 976 public boolean hasSubject() { 977 return this.subject != null && !this.subject.isEmpty(); 978 } 979 980 /** 981 * @param value {@link #subject} (Indicates the patient or group who the condition record is associated with.) 982 */ 983 public Condition setSubject(Reference value) { 984 this.subject = value; 985 return this; 986 } 987 988 /** 989 * @return {@link #encounter} (The Encounter during which this Condition was created or to which the creation of this record is tightly associated.) 990 */ 991 public Reference getEncounter() { 992 if (this.encounter == null) 993 if (Configuration.errorOnAutoCreate()) 994 throw new Error("Attempt to auto-create Condition.encounter"); 995 else if (Configuration.doAutoCreate()) 996 this.encounter = new Reference(); // cc 997 return this.encounter; 998 } 999 1000 public boolean hasEncounter() { 1001 return this.encounter != null && !this.encounter.isEmpty(); 1002 } 1003 1004 /** 1005 * @param value {@link #encounter} (The Encounter during which this Condition was created or to which the creation of this record is tightly associated.) 1006 */ 1007 public Condition setEncounter(Reference value) { 1008 this.encounter = value; 1009 return this; 1010 } 1011 1012 /** 1013 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1014 */ 1015 public DataType getOnset() { 1016 return this.onset; 1017 } 1018 1019 /** 1020 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1021 */ 1022 public DateTimeType getOnsetDateTimeType() throws FHIRException { 1023 if (this.onset == null) 1024 this.onset = new DateTimeType(); 1025 if (!(this.onset instanceof DateTimeType)) 1026 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1027 return (DateTimeType) this.onset; 1028 } 1029 1030 public boolean hasOnsetDateTimeType() { 1031 return this.onset instanceof DateTimeType; 1032 } 1033 1034 /** 1035 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1036 */ 1037 public Age getOnsetAge() throws FHIRException { 1038 if (this.onset == null) 1039 this.onset = new Age(); 1040 if (!(this.onset instanceof Age)) 1041 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.onset.getClass().getName()+" was encountered"); 1042 return (Age) this.onset; 1043 } 1044 1045 public boolean hasOnsetAge() { 1046 return this.onset instanceof Age; 1047 } 1048 1049 /** 1050 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1051 */ 1052 public Period getOnsetPeriod() throws FHIRException { 1053 if (this.onset == null) 1054 this.onset = new Period(); 1055 if (!(this.onset instanceof Period)) 1056 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.onset.getClass().getName()+" was encountered"); 1057 return (Period) this.onset; 1058 } 1059 1060 public boolean hasOnsetPeriod() { 1061 return this.onset instanceof Period; 1062 } 1063 1064 /** 1065 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1066 */ 1067 public Range getOnsetRange() throws FHIRException { 1068 if (this.onset == null) 1069 this.onset = new Range(); 1070 if (!(this.onset instanceof Range)) 1071 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.onset.getClass().getName()+" was encountered"); 1072 return (Range) this.onset; 1073 } 1074 1075 public boolean hasOnsetRange() { 1076 return this.onset instanceof Range; 1077 } 1078 1079 /** 1080 * @return {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1081 */ 1082 public StringType getOnsetStringType() throws FHIRException { 1083 if (this.onset == null) 1084 this.onset = new StringType(); 1085 if (!(this.onset instanceof StringType)) 1086 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.onset.getClass().getName()+" was encountered"); 1087 return (StringType) this.onset; 1088 } 1089 1090 public boolean hasOnsetStringType() { 1091 return this.onset instanceof StringType; 1092 } 1093 1094 public boolean hasOnset() { 1095 return this.onset != null && !this.onset.isEmpty(); 1096 } 1097 1098 /** 1099 * @param value {@link #onset} (Estimated or actual date or date-time the condition began, in the opinion of the clinician.) 1100 */ 1101 public Condition setOnset(DataType value) { 1102 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period || value instanceof Range || value instanceof StringType)) 1103 throw new FHIRException("Not the right type for Condition.onset[x]: "+value.fhirType()); 1104 this.onset = value; 1105 return this; 1106 } 1107 1108 /** 1109 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.) 1110 */ 1111 public DataType getAbatement() { 1112 return this.abatement; 1113 } 1114 1115 /** 1116 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.) 1117 */ 1118 public DateTimeType getAbatementDateTimeType() throws FHIRException { 1119 if (this.abatement == null) 1120 this.abatement = new DateTimeType(); 1121 if (!(this.abatement instanceof DateTimeType)) 1122 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1123 return (DateTimeType) this.abatement; 1124 } 1125 1126 public boolean hasAbatementDateTimeType() { 1127 return this.abatement instanceof DateTimeType; 1128 } 1129 1130 /** 1131 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.) 1132 */ 1133 public Age getAbatementAge() throws FHIRException { 1134 if (this.abatement == null) 1135 this.abatement = new Age(); 1136 if (!(this.abatement instanceof Age)) 1137 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1138 return (Age) this.abatement; 1139 } 1140 1141 public boolean hasAbatementAge() { 1142 return this.abatement instanceof Age; 1143 } 1144 1145 /** 1146 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.) 1147 */ 1148 public Period getAbatementPeriod() throws FHIRException { 1149 if (this.abatement == null) 1150 this.abatement = new Period(); 1151 if (!(this.abatement instanceof Period)) 1152 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1153 return (Period) this.abatement; 1154 } 1155 1156 public boolean hasAbatementPeriod() { 1157 return this.abatement instanceof Period; 1158 } 1159 1160 /** 1161 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.) 1162 */ 1163 public Range getAbatementRange() throws FHIRException { 1164 if (this.abatement == null) 1165 this.abatement = new Range(); 1166 if (!(this.abatement instanceof Range)) 1167 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1168 return (Range) this.abatement; 1169 } 1170 1171 public boolean hasAbatementRange() { 1172 return this.abatement instanceof Range; 1173 } 1174 1175 /** 1176 * @return {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.) 1177 */ 1178 public StringType getAbatementStringType() throws FHIRException { 1179 if (this.abatement == null) 1180 this.abatement = new StringType(); 1181 if (!(this.abatement instanceof StringType)) 1182 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.abatement.getClass().getName()+" was encountered"); 1183 return (StringType) this.abatement; 1184 } 1185 1186 public boolean hasAbatementStringType() { 1187 return this.abatement instanceof StringType; 1188 } 1189 1190 public boolean hasAbatement() { 1191 return this.abatement != null && !this.abatement.isEmpty(); 1192 } 1193 1194 /** 1195 * @param value {@link #abatement} (The date or estimated date that the condition resolved or went into remission. This is called "abatement" because of the many overloaded connotations associated with "remission" or "resolution" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.) 1196 */ 1197 public Condition setAbatement(DataType value) { 1198 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period || value instanceof Range || value instanceof StringType)) 1199 throw new FHIRException("Not the right type for Condition.abatement[x]: "+value.fhirType()); 1200 this.abatement = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #recordedDate} (The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 1206 */ 1207 public DateTimeType getRecordedDateElement() { 1208 if (this.recordedDate == null) 1209 if (Configuration.errorOnAutoCreate()) 1210 throw new Error("Attempt to auto-create Condition.recordedDate"); 1211 else if (Configuration.doAutoCreate()) 1212 this.recordedDate = new DateTimeType(); // bb 1213 return this.recordedDate; 1214 } 1215 1216 public boolean hasRecordedDateElement() { 1217 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1218 } 1219 1220 public boolean hasRecordedDate() { 1221 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #recordedDate} (The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date.). This is the underlying object with id, value and extensions. The accessor "getRecordedDate" gives direct access to the value 1226 */ 1227 public Condition setRecordedDateElement(DateTimeType value) { 1228 this.recordedDate = value; 1229 return this; 1230 } 1231 1232 /** 1233 * @return The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date. 1234 */ 1235 public Date getRecordedDate() { 1236 return this.recordedDate == null ? null : this.recordedDate.getValue(); 1237 } 1238 1239 /** 1240 * @param value The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date. 1241 */ 1242 public Condition setRecordedDate(Date value) { 1243 if (value == null) 1244 this.recordedDate = null; 1245 else { 1246 if (this.recordedDate == null) 1247 this.recordedDate = new DateTimeType(); 1248 this.recordedDate.setValue(value); 1249 } 1250 return this; 1251 } 1252 1253 /** 1254 * @return {@link #participant} (Indicates who or what participated in the activities related to the condition and how they were involved.) 1255 */ 1256 public List<ConditionParticipantComponent> getParticipant() { 1257 if (this.participant == null) 1258 this.participant = new ArrayList<ConditionParticipantComponent>(); 1259 return this.participant; 1260 } 1261 1262 /** 1263 * @return Returns a reference to <code>this</code> for easy method chaining 1264 */ 1265 public Condition setParticipant(List<ConditionParticipantComponent> theParticipant) { 1266 this.participant = theParticipant; 1267 return this; 1268 } 1269 1270 public boolean hasParticipant() { 1271 if (this.participant == null) 1272 return false; 1273 for (ConditionParticipantComponent item : this.participant) 1274 if (!item.isEmpty()) 1275 return true; 1276 return false; 1277 } 1278 1279 public ConditionParticipantComponent addParticipant() { //3 1280 ConditionParticipantComponent t = new ConditionParticipantComponent(); 1281 if (this.participant == null) 1282 this.participant = new ArrayList<ConditionParticipantComponent>(); 1283 this.participant.add(t); 1284 return t; 1285 } 1286 1287 public Condition addParticipant(ConditionParticipantComponent t) { //3 1288 if (t == null) 1289 return this; 1290 if (this.participant == null) 1291 this.participant = new ArrayList<ConditionParticipantComponent>(); 1292 this.participant.add(t); 1293 return this; 1294 } 1295 1296 /** 1297 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 1298 */ 1299 public ConditionParticipantComponent getParticipantFirstRep() { 1300 if (getParticipant().isEmpty()) { 1301 addParticipant(); 1302 } 1303 return getParticipant().get(0); 1304 } 1305 1306 /** 1307 * @return {@link #stage} (A simple summary of the stage such as "Stage 3" or "Early Onset". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease.) 1308 */ 1309 public List<ConditionStageComponent> getStage() { 1310 if (this.stage == null) 1311 this.stage = new ArrayList<ConditionStageComponent>(); 1312 return this.stage; 1313 } 1314 1315 /** 1316 * @return Returns a reference to <code>this</code> for easy method chaining 1317 */ 1318 public Condition setStage(List<ConditionStageComponent> theStage) { 1319 this.stage = theStage; 1320 return this; 1321 } 1322 1323 public boolean hasStage() { 1324 if (this.stage == null) 1325 return false; 1326 for (ConditionStageComponent item : this.stage) 1327 if (!item.isEmpty()) 1328 return true; 1329 return false; 1330 } 1331 1332 public ConditionStageComponent addStage() { //3 1333 ConditionStageComponent t = new ConditionStageComponent(); 1334 if (this.stage == null) 1335 this.stage = new ArrayList<ConditionStageComponent>(); 1336 this.stage.add(t); 1337 return t; 1338 } 1339 1340 public Condition addStage(ConditionStageComponent t) { //3 1341 if (t == null) 1342 return this; 1343 if (this.stage == null) 1344 this.stage = new ArrayList<ConditionStageComponent>(); 1345 this.stage.add(t); 1346 return this; 1347 } 1348 1349 /** 1350 * @return The first repetition of repeating field {@link #stage}, creating it if it does not already exist {3} 1351 */ 1352 public ConditionStageComponent getStageFirstRep() { 1353 if (getStage().isEmpty()) { 1354 addStage(); 1355 } 1356 return getStage().get(0); 1357 } 1358 1359 /** 1360 * @return {@link #evidence} (Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition.) 1361 */ 1362 public List<CodeableReference> getEvidence() { 1363 if (this.evidence == null) 1364 this.evidence = new ArrayList<CodeableReference>(); 1365 return this.evidence; 1366 } 1367 1368 /** 1369 * @return Returns a reference to <code>this</code> for easy method chaining 1370 */ 1371 public Condition setEvidence(List<CodeableReference> theEvidence) { 1372 this.evidence = theEvidence; 1373 return this; 1374 } 1375 1376 public boolean hasEvidence() { 1377 if (this.evidence == null) 1378 return false; 1379 for (CodeableReference item : this.evidence) 1380 if (!item.isEmpty()) 1381 return true; 1382 return false; 1383 } 1384 1385 public CodeableReference addEvidence() { //3 1386 CodeableReference t = new CodeableReference(); 1387 if (this.evidence == null) 1388 this.evidence = new ArrayList<CodeableReference>(); 1389 this.evidence.add(t); 1390 return t; 1391 } 1392 1393 public Condition addEvidence(CodeableReference t) { //3 1394 if (t == null) 1395 return this; 1396 if (this.evidence == null) 1397 this.evidence = new ArrayList<CodeableReference>(); 1398 this.evidence.add(t); 1399 return this; 1400 } 1401 1402 /** 1403 * @return The first repetition of repeating field {@link #evidence}, creating it if it does not already exist {3} 1404 */ 1405 public CodeableReference getEvidenceFirstRep() { 1406 if (getEvidence().isEmpty()) { 1407 addEvidence(); 1408 } 1409 return getEvidence().get(0); 1410 } 1411 1412 /** 1413 * @return {@link #note} (Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.) 1414 */ 1415 public List<Annotation> getNote() { 1416 if (this.note == null) 1417 this.note = new ArrayList<Annotation>(); 1418 return this.note; 1419 } 1420 1421 /** 1422 * @return Returns a reference to <code>this</code> for easy method chaining 1423 */ 1424 public Condition setNote(List<Annotation> theNote) { 1425 this.note = theNote; 1426 return this; 1427 } 1428 1429 public boolean hasNote() { 1430 if (this.note == null) 1431 return false; 1432 for (Annotation item : this.note) 1433 if (!item.isEmpty()) 1434 return true; 1435 return false; 1436 } 1437 1438 public Annotation addNote() { //3 1439 Annotation t = new Annotation(); 1440 if (this.note == null) 1441 this.note = new ArrayList<Annotation>(); 1442 this.note.add(t); 1443 return t; 1444 } 1445 1446 public Condition addNote(Annotation t) { //3 1447 if (t == null) 1448 return this; 1449 if (this.note == null) 1450 this.note = new ArrayList<Annotation>(); 1451 this.note.add(t); 1452 return this; 1453 } 1454 1455 /** 1456 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1457 */ 1458 public Annotation getNoteFirstRep() { 1459 if (getNote().isEmpty()) { 1460 addNote(); 1461 } 1462 return getNote().get(0); 1463 } 1464 1465 protected void listChildren(List<Property> children) { 1466 super.listChildren(children); 1467 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1468 children.add(new Property("clinicalStatus", "CodeableConcept", "The clinical status of the condition.", 0, 1, clinicalStatus)); 1469 children.add(new Property("verificationStatus", "CodeableConcept", "The verification status to support the clinical status of the condition. The verification status pertains to the condition, itself, not to any specific condition attribute.", 0, 1, verificationStatus)); 1470 children.add(new Property("category", "CodeableConcept", "A category assigned to the condition.", 0, java.lang.Integer.MAX_VALUE, category)); 1471 children.add(new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity)); 1472 children.add(new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code)); 1473 children.add(new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 1474 children.add(new Property("subject", "Reference(Patient|Group)", "Indicates the patient or group who the condition record is associated with.", 0, 1, subject)); 1475 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter during which this Condition was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 1476 children.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset)); 1477 children.add(new Property("abatement[x]", "dateTime|Age|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement)); 1478 children.add(new Property("recordedDate", "dateTime", "The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date.", 0, 1, recordedDate)); 1479 children.add(new Property("participant", "", "Indicates who or what participated in the activities related to the condition and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant)); 1480 children.add(new Property("stage", "", "A simple summary of the stage such as \"Stage 3\" or \"Early Onset\". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease.", 0, java.lang.Integer.MAX_VALUE, stage)); 1481 children.add(new Property("evidence", "CodeableReference(Any)", "Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition.", 0, java.lang.Integer.MAX_VALUE, evidence)); 1482 children.add(new Property("note", "Annotation", "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.", 0, java.lang.Integer.MAX_VALUE, note)); 1483 } 1484 1485 @Override 1486 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1487 switch (_hash) { 1488 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1489 case -462853915: /*clinicalStatus*/ return new Property("clinicalStatus", "CodeableConcept", "The clinical status of the condition.", 0, 1, clinicalStatus); 1490 case -842509843: /*verificationStatus*/ return new Property("verificationStatus", "CodeableConcept", "The verification status to support the clinical status of the condition. The verification status pertains to the condition, itself, not to any specific condition attribute.", 0, 1, verificationStatus); 1491 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A category assigned to the condition.", 0, java.lang.Integer.MAX_VALUE, category); 1492 case 1478300413: /*severity*/ return new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity); 1493 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code); 1494 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, java.lang.Integer.MAX_VALUE, bodySite); 1495 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "Indicates the patient or group who the condition record is associated with.", 0, 1, subject); 1496 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter during which this Condition was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 1497 case -1886216323: /*onset[x]*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1498 case 105901603: /*onset*/ return new Property("onset[x]", "dateTime|Age|Period|Range|string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1499 case -1701663010: /*onsetDateTime*/ return new Property("onset[x]", "dateTime", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1500 case -1886241828: /*onsetAge*/ return new Property("onset[x]", "Age", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1501 case -1545082428: /*onsetPeriod*/ return new Property("onset[x]", "Period", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1502 case -186664742: /*onsetRange*/ return new Property("onset[x]", "Range", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1503 case -1445342188: /*onsetString*/ return new Property("onset[x]", "string", "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1504 case -584196495: /*abatement[x]*/ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement); 1505 case -921554001: /*abatement*/ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement); 1506 case 44869738: /*abatementDateTime*/ return new Property("abatement[x]", "dateTime", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement); 1507 case -584222000: /*abatementAge*/ return new Property("abatement[x]", "Age", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement); 1508 case -922036656: /*abatementPeriod*/ return new Property("abatement[x]", "Period", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement); 1509 case 1218906830: /*abatementRange*/ return new Property("abatement[x]", "Range", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement); 1510 case -822296416: /*abatementString*/ return new Property("abatement[x]", "string", "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Some conditions, such as chronic conditions, are never really resolved, but they can abate.", 0, 1, abatement); 1511 case -1952893826: /*recordedDate*/ return new Property("recordedDate", "dateTime", "The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date.", 0, 1, recordedDate); 1512 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what participated in the activities related to the condition and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant); 1513 case 109757182: /*stage*/ return new Property("stage", "", "A simple summary of the stage such as \"Stage 3\" or \"Early Onset\". The determination of the stage is disease-specific, such as cancer, retinopathy of prematurity, kidney diseases, Alzheimer's, or Parkinson disease.", 0, java.lang.Integer.MAX_VALUE, stage); 1514 case 382967383: /*evidence*/ return new Property("evidence", "CodeableReference(Any)", "Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition.", 0, java.lang.Integer.MAX_VALUE, evidence); 1515 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.", 0, java.lang.Integer.MAX_VALUE, note); 1516 default: return super.getNamedProperty(_hash, _name, _checkValid); 1517 } 1518 1519 } 1520 1521 @Override 1522 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1523 switch (hash) { 1524 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1525 case -462853915: /*clinicalStatus*/ return this.clinicalStatus == null ? new Base[0] : new Base[] {this.clinicalStatus}; // CodeableConcept 1526 case -842509843: /*verificationStatus*/ return this.verificationStatus == null ? new Base[0] : new Base[] {this.verificationStatus}; // CodeableConcept 1527 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1528 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // CodeableConcept 1529 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1530 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 1531 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1532 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1533 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // DataType 1534 case -921554001: /*abatement*/ return this.abatement == null ? new Base[0] : new Base[] {this.abatement}; // DataType 1535 case -1952893826: /*recordedDate*/ return this.recordedDate == null ? new Base[0] : new Base[] {this.recordedDate}; // DateTimeType 1536 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // ConditionParticipantComponent 1537 case 109757182: /*stage*/ return this.stage == null ? new Base[0] : this.stage.toArray(new Base[this.stage.size()]); // ConditionStageComponent 1538 case 382967383: /*evidence*/ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // CodeableReference 1539 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1540 default: return super.getProperty(hash, name, checkValid); 1541 } 1542 1543 } 1544 1545 @Override 1546 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1547 switch (hash) { 1548 case -1618432855: // identifier 1549 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1550 return value; 1551 case -462853915: // clinicalStatus 1552 this.clinicalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1553 return value; 1554 case -842509843: // verificationStatus 1555 this.verificationStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1556 return value; 1557 case 50511102: // category 1558 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1559 return value; 1560 case 1478300413: // severity 1561 this.severity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1562 return value; 1563 case 3059181: // code 1564 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1565 return value; 1566 case 1702620169: // bodySite 1567 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1568 return value; 1569 case -1867885268: // subject 1570 this.subject = TypeConvertor.castToReference(value); // Reference 1571 return value; 1572 case 1524132147: // encounter 1573 this.encounter = TypeConvertor.castToReference(value); // Reference 1574 return value; 1575 case 105901603: // onset 1576 this.onset = TypeConvertor.castToType(value); // DataType 1577 return value; 1578 case -921554001: // abatement 1579 this.abatement = TypeConvertor.castToType(value); // DataType 1580 return value; 1581 case -1952893826: // recordedDate 1582 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 1583 return value; 1584 case 767422259: // participant 1585 this.getParticipant().add((ConditionParticipantComponent) value); // ConditionParticipantComponent 1586 return value; 1587 case 109757182: // stage 1588 this.getStage().add((ConditionStageComponent) value); // ConditionStageComponent 1589 return value; 1590 case 382967383: // evidence 1591 this.getEvidence().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1592 return value; 1593 case 3387378: // note 1594 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1595 return value; 1596 default: return super.setProperty(hash, name, value); 1597 } 1598 1599 } 1600 1601 @Override 1602 public Base setProperty(String name, Base value) throws FHIRException { 1603 if (name.equals("identifier")) { 1604 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1605 } else if (name.equals("clinicalStatus")) { 1606 this.clinicalStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1607 } else if (name.equals("verificationStatus")) { 1608 this.verificationStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1609 } else if (name.equals("category")) { 1610 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1611 } else if (name.equals("severity")) { 1612 this.severity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1613 } else if (name.equals("code")) { 1614 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1615 } else if (name.equals("bodySite")) { 1616 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); 1617 } else if (name.equals("subject")) { 1618 this.subject = TypeConvertor.castToReference(value); // Reference 1619 } else if (name.equals("encounter")) { 1620 this.encounter = TypeConvertor.castToReference(value); // Reference 1621 } else if (name.equals("onset[x]")) { 1622 this.onset = TypeConvertor.castToType(value); // DataType 1623 } else if (name.equals("abatement[x]")) { 1624 this.abatement = TypeConvertor.castToType(value); // DataType 1625 } else if (name.equals("recordedDate")) { 1626 this.recordedDate = TypeConvertor.castToDateTime(value); // DateTimeType 1627 } else if (name.equals("participant")) { 1628 this.getParticipant().add((ConditionParticipantComponent) value); 1629 } else if (name.equals("stage")) { 1630 this.getStage().add((ConditionStageComponent) value); 1631 } else if (name.equals("evidence")) { 1632 this.getEvidence().add(TypeConvertor.castToCodeableReference(value)); 1633 } else if (name.equals("note")) { 1634 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1635 } else 1636 return super.setProperty(name, value); 1637 return value; 1638 } 1639 1640 @Override 1641 public void removeChild(String name, Base value) throws FHIRException { 1642 if (name.equals("identifier")) { 1643 this.getIdentifier().remove(value); 1644 } else if (name.equals("clinicalStatus")) { 1645 this.clinicalStatus = null; 1646 } else if (name.equals("verificationStatus")) { 1647 this.verificationStatus = null; 1648 } else if (name.equals("category")) { 1649 this.getCategory().remove(value); 1650 } else if (name.equals("severity")) { 1651 this.severity = null; 1652 } else if (name.equals("code")) { 1653 this.code = null; 1654 } else if (name.equals("bodySite")) { 1655 this.getBodySite().remove(value); 1656 } else if (name.equals("subject")) { 1657 this.subject = null; 1658 } else if (name.equals("encounter")) { 1659 this.encounter = null; 1660 } else if (name.equals("onset[x]")) { 1661 this.onset = null; 1662 } else if (name.equals("abatement[x]")) { 1663 this.abatement = null; 1664 } else if (name.equals("recordedDate")) { 1665 this.recordedDate = null; 1666 } else if (name.equals("participant")) { 1667 this.getParticipant().remove((ConditionParticipantComponent) value); 1668 } else if (name.equals("stage")) { 1669 this.getStage().remove((ConditionStageComponent) value); 1670 } else if (name.equals("evidence")) { 1671 this.getEvidence().remove(value); 1672 } else if (name.equals("note")) { 1673 this.getNote().remove(value); 1674 } else 1675 super.removeChild(name, value); 1676 1677 } 1678 1679 @Override 1680 public Base makeProperty(int hash, String name) throws FHIRException { 1681 switch (hash) { 1682 case -1618432855: return addIdentifier(); 1683 case -462853915: return getClinicalStatus(); 1684 case -842509843: return getVerificationStatus(); 1685 case 50511102: return addCategory(); 1686 case 1478300413: return getSeverity(); 1687 case 3059181: return getCode(); 1688 case 1702620169: return addBodySite(); 1689 case -1867885268: return getSubject(); 1690 case 1524132147: return getEncounter(); 1691 case -1886216323: return getOnset(); 1692 case 105901603: return getOnset(); 1693 case -584196495: return getAbatement(); 1694 case -921554001: return getAbatement(); 1695 case -1952893826: return getRecordedDateElement(); 1696 case 767422259: return addParticipant(); 1697 case 109757182: return addStage(); 1698 case 382967383: return addEvidence(); 1699 case 3387378: return addNote(); 1700 default: return super.makeProperty(hash, name); 1701 } 1702 1703 } 1704 1705 @Override 1706 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1707 switch (hash) { 1708 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1709 case -462853915: /*clinicalStatus*/ return new String[] {"CodeableConcept"}; 1710 case -842509843: /*verificationStatus*/ return new String[] {"CodeableConcept"}; 1711 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1712 case 1478300413: /*severity*/ return new String[] {"CodeableConcept"}; 1713 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1714 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 1715 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1716 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1717 case 105901603: /*onset*/ return new String[] {"dateTime", "Age", "Period", "Range", "string"}; 1718 case -921554001: /*abatement*/ return new String[] {"dateTime", "Age", "Period", "Range", "string"}; 1719 case -1952893826: /*recordedDate*/ return new String[] {"dateTime"}; 1720 case 767422259: /*participant*/ return new String[] {}; 1721 case 109757182: /*stage*/ return new String[] {}; 1722 case 382967383: /*evidence*/ return new String[] {"CodeableReference"}; 1723 case 3387378: /*note*/ return new String[] {"Annotation"}; 1724 default: return super.getTypesForProperty(hash, name); 1725 } 1726 1727 } 1728 1729 @Override 1730 public Base addChild(String name) throws FHIRException { 1731 if (name.equals("identifier")) { 1732 return addIdentifier(); 1733 } 1734 else if (name.equals("clinicalStatus")) { 1735 this.clinicalStatus = new CodeableConcept(); 1736 return this.clinicalStatus; 1737 } 1738 else if (name.equals("verificationStatus")) { 1739 this.verificationStatus = new CodeableConcept(); 1740 return this.verificationStatus; 1741 } 1742 else if (name.equals("category")) { 1743 return addCategory(); 1744 } 1745 else if (name.equals("severity")) { 1746 this.severity = new CodeableConcept(); 1747 return this.severity; 1748 } 1749 else if (name.equals("code")) { 1750 this.code = new CodeableConcept(); 1751 return this.code; 1752 } 1753 else if (name.equals("bodySite")) { 1754 return addBodySite(); 1755 } 1756 else if (name.equals("subject")) { 1757 this.subject = new Reference(); 1758 return this.subject; 1759 } 1760 else if (name.equals("encounter")) { 1761 this.encounter = new Reference(); 1762 return this.encounter; 1763 } 1764 else if (name.equals("onsetDateTime")) { 1765 this.onset = new DateTimeType(); 1766 return this.onset; 1767 } 1768 else if (name.equals("onsetAge")) { 1769 this.onset = new Age(); 1770 return this.onset; 1771 } 1772 else if (name.equals("onsetPeriod")) { 1773 this.onset = new Period(); 1774 return this.onset; 1775 } 1776 else if (name.equals("onsetRange")) { 1777 this.onset = new Range(); 1778 return this.onset; 1779 } 1780 else if (name.equals("onsetString")) { 1781 this.onset = new StringType(); 1782 return this.onset; 1783 } 1784 else if (name.equals("abatementDateTime")) { 1785 this.abatement = new DateTimeType(); 1786 return this.abatement; 1787 } 1788 else if (name.equals("abatementAge")) { 1789 this.abatement = new Age(); 1790 return this.abatement; 1791 } 1792 else if (name.equals("abatementPeriod")) { 1793 this.abatement = new Period(); 1794 return this.abatement; 1795 } 1796 else if (name.equals("abatementRange")) { 1797 this.abatement = new Range(); 1798 return this.abatement; 1799 } 1800 else if (name.equals("abatementString")) { 1801 this.abatement = new StringType(); 1802 return this.abatement; 1803 } 1804 else if (name.equals("recordedDate")) { 1805 throw new FHIRException("Cannot call addChild on a singleton property Condition.recordedDate"); 1806 } 1807 else if (name.equals("participant")) { 1808 return addParticipant(); 1809 } 1810 else if (name.equals("stage")) { 1811 return addStage(); 1812 } 1813 else if (name.equals("evidence")) { 1814 return addEvidence(); 1815 } 1816 else if (name.equals("note")) { 1817 return addNote(); 1818 } 1819 else 1820 return super.addChild(name); 1821 } 1822 1823 public String fhirType() { 1824 return "Condition"; 1825 1826 } 1827 1828 public Condition copy() { 1829 Condition dst = new Condition(); 1830 copyValues(dst); 1831 return dst; 1832 } 1833 1834 public void copyValues(Condition dst) { 1835 super.copyValues(dst); 1836 if (identifier != null) { 1837 dst.identifier = new ArrayList<Identifier>(); 1838 for (Identifier i : identifier) 1839 dst.identifier.add(i.copy()); 1840 }; 1841 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 1842 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 1843 if (category != null) { 1844 dst.category = new ArrayList<CodeableConcept>(); 1845 for (CodeableConcept i : category) 1846 dst.category.add(i.copy()); 1847 }; 1848 dst.severity = severity == null ? null : severity.copy(); 1849 dst.code = code == null ? null : code.copy(); 1850 if (bodySite != null) { 1851 dst.bodySite = new ArrayList<CodeableConcept>(); 1852 for (CodeableConcept i : bodySite) 1853 dst.bodySite.add(i.copy()); 1854 }; 1855 dst.subject = subject == null ? null : subject.copy(); 1856 dst.encounter = encounter == null ? null : encounter.copy(); 1857 dst.onset = onset == null ? null : onset.copy(); 1858 dst.abatement = abatement == null ? null : abatement.copy(); 1859 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 1860 if (participant != null) { 1861 dst.participant = new ArrayList<ConditionParticipantComponent>(); 1862 for (ConditionParticipantComponent i : participant) 1863 dst.participant.add(i.copy()); 1864 }; 1865 if (stage != null) { 1866 dst.stage = new ArrayList<ConditionStageComponent>(); 1867 for (ConditionStageComponent i : stage) 1868 dst.stage.add(i.copy()); 1869 }; 1870 if (evidence != null) { 1871 dst.evidence = new ArrayList<CodeableReference>(); 1872 for (CodeableReference i : evidence) 1873 dst.evidence.add(i.copy()); 1874 }; 1875 if (note != null) { 1876 dst.note = new ArrayList<Annotation>(); 1877 for (Annotation i : note) 1878 dst.note.add(i.copy()); 1879 }; 1880 } 1881 1882 protected Condition typedCopy() { 1883 return copy(); 1884 } 1885 1886 @Override 1887 public boolean equalsDeep(Base other_) { 1888 if (!super.equalsDeep(other_)) 1889 return false; 1890 if (!(other_ instanceof Condition)) 1891 return false; 1892 Condition o = (Condition) other_; 1893 return compareDeep(identifier, o.identifier, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 1894 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(category, o.category, true) 1895 && compareDeep(severity, o.severity, true) && compareDeep(code, o.code, true) && compareDeep(bodySite, o.bodySite, true) 1896 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) && compareDeep(onset, o.onset, true) 1897 && compareDeep(abatement, o.abatement, true) && compareDeep(recordedDate, o.recordedDate, true) 1898 && compareDeep(participant, o.participant, true) && compareDeep(stage, o.stage, true) && compareDeep(evidence, o.evidence, true) 1899 && compareDeep(note, o.note, true); 1900 } 1901 1902 @Override 1903 public boolean equalsShallow(Base other_) { 1904 if (!super.equalsShallow(other_)) 1905 return false; 1906 if (!(other_ instanceof Condition)) 1907 return false; 1908 Condition o = (Condition) other_; 1909 return compareValues(recordedDate, o.recordedDate, true); 1910 } 1911 1912 public boolean isEmpty() { 1913 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, clinicalStatus 1914 , verificationStatus, category, severity, code, bodySite, subject, encounter, onset 1915 , abatement, recordedDate, participant, stage, evidence, note); 1916 } 1917 1918 @Override 1919 public ResourceType getResourceType() { 1920 return ResourceType.Condition; 1921 } 1922 1923 /** 1924 * Search parameter: <b>abatement-age</b> 1925 * <p> 1926 * Description: <b>Abatement as age or age range</b><br> 1927 * Type: <b>quantity</b><br> 1928 * Path: <b>Condition.abatement.ofType(Age) | Condition.abatement.ofType(Range)</b><br> 1929 * </p> 1930 */ 1931 @SearchParamDefinition(name="abatement-age", path="Condition.abatement.ofType(Age) | Condition.abatement.ofType(Range)", description="Abatement as age or age range", type="quantity" ) 1932 public static final String SP_ABATEMENT_AGE = "abatement-age"; 1933 /** 1934 * <b>Fluent Client</b> search parameter constant for <b>abatement-age</b> 1935 * <p> 1936 * Description: <b>Abatement as age or age range</b><br> 1937 * Type: <b>quantity</b><br> 1938 * Path: <b>Condition.abatement.ofType(Age) | Condition.abatement.ofType(Range)</b><br> 1939 * </p> 1940 */ 1941 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam ABATEMENT_AGE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_ABATEMENT_AGE); 1942 1943 /** 1944 * Search parameter: <b>abatement-date</b> 1945 * <p> 1946 * Description: <b>Date-related abatements (dateTime and period)</b><br> 1947 * Type: <b>date</b><br> 1948 * Path: <b>Condition.abatement.ofType(dateTime) | Condition.abatement.ofType(Period)</b><br> 1949 * </p> 1950 */ 1951 @SearchParamDefinition(name="abatement-date", path="Condition.abatement.ofType(dateTime) | Condition.abatement.ofType(Period)", description="Date-related abatements (dateTime and period)", type="date" ) 1952 public static final String SP_ABATEMENT_DATE = "abatement-date"; 1953 /** 1954 * <b>Fluent Client</b> search parameter constant for <b>abatement-date</b> 1955 * <p> 1956 * Description: <b>Date-related abatements (dateTime and period)</b><br> 1957 * Type: <b>date</b><br> 1958 * Path: <b>Condition.abatement.ofType(dateTime) | Condition.abatement.ofType(Period)</b><br> 1959 * </p> 1960 */ 1961 public static final ca.uhn.fhir.rest.gclient.DateClientParam ABATEMENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ABATEMENT_DATE); 1962 1963 /** 1964 * Search parameter: <b>abatement-string</b> 1965 * <p> 1966 * Description: <b>Abatement as a string</b><br> 1967 * Type: <b>string</b><br> 1968 * Path: <b>Condition.abatement.ofType(string)</b><br> 1969 * </p> 1970 */ 1971 @SearchParamDefinition(name="abatement-string", path="Condition.abatement.ofType(string)", description="Abatement as a string", type="string" ) 1972 public static final String SP_ABATEMENT_STRING = "abatement-string"; 1973 /** 1974 * <b>Fluent Client</b> search parameter constant for <b>abatement-string</b> 1975 * <p> 1976 * Description: <b>Abatement as a string</b><br> 1977 * Type: <b>string</b><br> 1978 * Path: <b>Condition.abatement.ofType(string)</b><br> 1979 * </p> 1980 */ 1981 public static final ca.uhn.fhir.rest.gclient.StringClientParam ABATEMENT_STRING = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ABATEMENT_STRING); 1982 1983 /** 1984 * Search parameter: <b>body-site</b> 1985 * <p> 1986 * Description: <b>Anatomical location, if relevant</b><br> 1987 * Type: <b>token</b><br> 1988 * Path: <b>Condition.bodySite</b><br> 1989 * </p> 1990 */ 1991 @SearchParamDefinition(name="body-site", path="Condition.bodySite", description="Anatomical location, if relevant", type="token" ) 1992 public static final String SP_BODY_SITE = "body-site"; 1993 /** 1994 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 1995 * <p> 1996 * Description: <b>Anatomical location, if relevant</b><br> 1997 * Type: <b>token</b><br> 1998 * Path: <b>Condition.bodySite</b><br> 1999 * </p> 2000 */ 2001 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 2002 2003 /** 2004 * Search parameter: <b>category</b> 2005 * <p> 2006 * Description: <b>The category of the condition</b><br> 2007 * Type: <b>token</b><br> 2008 * Path: <b>Condition.category</b><br> 2009 * </p> 2010 */ 2011 @SearchParamDefinition(name="category", path="Condition.category", description="The category of the condition", type="token" ) 2012 public static final String SP_CATEGORY = "category"; 2013 /** 2014 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2015 * <p> 2016 * Description: <b>The category of the condition</b><br> 2017 * Type: <b>token</b><br> 2018 * Path: <b>Condition.category</b><br> 2019 * </p> 2020 */ 2021 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2022 2023 /** 2024 * Search parameter: <b>clinical-status</b> 2025 * <p> 2026 * Description: <b>The clinical status of the condition</b><br> 2027 * Type: <b>token</b><br> 2028 * Path: <b>Condition.clinicalStatus</b><br> 2029 * </p> 2030 */ 2031 @SearchParamDefinition(name="clinical-status", path="Condition.clinicalStatus", description="The clinical status of the condition", type="token" ) 2032 public static final String SP_CLINICAL_STATUS = "clinical-status"; 2033 /** 2034 * <b>Fluent Client</b> search parameter constant for <b>clinical-status</b> 2035 * <p> 2036 * Description: <b>The clinical status of the condition</b><br> 2037 * Type: <b>token</b><br> 2038 * Path: <b>Condition.clinicalStatus</b><br> 2039 * </p> 2040 */ 2041 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLINICAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLINICAL_STATUS); 2042 2043 /** 2044 * Search parameter: <b>evidence-detail</b> 2045 * <p> 2046 * Description: <b>Supporting information found elsewhere</b><br> 2047 * Type: <b>reference</b><br> 2048 * Path: <b>Condition.evidence.reference</b><br> 2049 * </p> 2050 */ 2051 @SearchParamDefinition(name="evidence-detail", path="Condition.evidence.reference", description="Supporting information found elsewhere", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2052 public static final String SP_EVIDENCE_DETAIL = "evidence-detail"; 2053 /** 2054 * <b>Fluent Client</b> search parameter constant for <b>evidence-detail</b> 2055 * <p> 2056 * Description: <b>Supporting information found elsewhere</b><br> 2057 * Type: <b>reference</b><br> 2058 * Path: <b>Condition.evidence.reference</b><br> 2059 * </p> 2060 */ 2061 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EVIDENCE_DETAIL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EVIDENCE_DETAIL); 2062 2063/** 2064 * Constant for fluent queries to be used to add include statements. Specifies 2065 * the path value of "<b>Condition:evidence-detail</b>". 2066 */ 2067 public static final ca.uhn.fhir.model.api.Include INCLUDE_EVIDENCE_DETAIL = new ca.uhn.fhir.model.api.Include("Condition:evidence-detail").toLocked(); 2068 2069 /** 2070 * Search parameter: <b>evidence</b> 2071 * <p> 2072 * Description: <b>Manifestation/symptom</b><br> 2073 * Type: <b>token</b><br> 2074 * Path: <b>Condition.evidence.concept</b><br> 2075 * </p> 2076 */ 2077 @SearchParamDefinition(name="evidence", path="Condition.evidence.concept", description="Manifestation/symptom", type="token" ) 2078 public static final String SP_EVIDENCE = "evidence"; 2079 /** 2080 * <b>Fluent Client</b> search parameter constant for <b>evidence</b> 2081 * <p> 2082 * Description: <b>Manifestation/symptom</b><br> 2083 * Type: <b>token</b><br> 2084 * Path: <b>Condition.evidence.concept</b><br> 2085 * </p> 2086 */ 2087 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVIDENCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVIDENCE); 2088 2089 /** 2090 * Search parameter: <b>onset-age</b> 2091 * <p> 2092 * Description: <b>Onsets as age or age range</b><br> 2093 * Type: <b>quantity</b><br> 2094 * Path: <b>Condition.onset.ofType(Age) | Condition.onset.ofType(Range)</b><br> 2095 * </p> 2096 */ 2097 @SearchParamDefinition(name="onset-age", path="Condition.onset.ofType(Age) | Condition.onset.ofType(Range)", description="Onsets as age or age range", type="quantity" ) 2098 public static final String SP_ONSET_AGE = "onset-age"; 2099 /** 2100 * <b>Fluent Client</b> search parameter constant for <b>onset-age</b> 2101 * <p> 2102 * Description: <b>Onsets as age or age range</b><br> 2103 * Type: <b>quantity</b><br> 2104 * Path: <b>Condition.onset.ofType(Age) | Condition.onset.ofType(Range)</b><br> 2105 * </p> 2106 */ 2107 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam ONSET_AGE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_ONSET_AGE); 2108 2109 /** 2110 * Search parameter: <b>onset-date</b> 2111 * <p> 2112 * Description: <b>Date related onsets (dateTime and Period)</b><br> 2113 * Type: <b>date</b><br> 2114 * Path: <b>Condition.onset.ofType(dateTime) | Condition.onset.ofType(Period)</b><br> 2115 * </p> 2116 */ 2117 @SearchParamDefinition(name="onset-date", path="Condition.onset.ofType(dateTime) | Condition.onset.ofType(Period)", description="Date related onsets (dateTime and Period)", type="date" ) 2118 public static final String SP_ONSET_DATE = "onset-date"; 2119 /** 2120 * <b>Fluent Client</b> search parameter constant for <b>onset-date</b> 2121 * <p> 2122 * Description: <b>Date related onsets (dateTime and Period)</b><br> 2123 * Type: <b>date</b><br> 2124 * Path: <b>Condition.onset.ofType(dateTime) | Condition.onset.ofType(Period)</b><br> 2125 * </p> 2126 */ 2127 public static final ca.uhn.fhir.rest.gclient.DateClientParam ONSET_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ONSET_DATE); 2128 2129 /** 2130 * Search parameter: <b>onset-info</b> 2131 * <p> 2132 * Description: <b>Onsets as a string</b><br> 2133 * Type: <b>string</b><br> 2134 * Path: <b>Condition.onset.ofType(string)</b><br> 2135 * </p> 2136 */ 2137 @SearchParamDefinition(name="onset-info", path="Condition.onset.ofType(string)", description="Onsets as a string", type="string" ) 2138 public static final String SP_ONSET_INFO = "onset-info"; 2139 /** 2140 * <b>Fluent Client</b> search parameter constant for <b>onset-info</b> 2141 * <p> 2142 * Description: <b>Onsets as a string</b><br> 2143 * Type: <b>string</b><br> 2144 * Path: <b>Condition.onset.ofType(string)</b><br> 2145 * </p> 2146 */ 2147 public static final ca.uhn.fhir.rest.gclient.StringClientParam ONSET_INFO = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ONSET_INFO); 2148 2149 /** 2150 * Search parameter: <b>participant-actor</b> 2151 * <p> 2152 * Description: <b>Who or what participated in the activities related to the condition</b><br> 2153 * Type: <b>reference</b><br> 2154 * Path: <b>Condition.participant.actor</b><br> 2155 * </p> 2156 */ 2157 @SearchParamDefinition(name="participant-actor", path="Condition.participant.actor", description="Who or what participated in the activities related to the condition", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2158 public static final String SP_PARTICIPANT_ACTOR = "participant-actor"; 2159 /** 2160 * <b>Fluent Client</b> search parameter constant for <b>participant-actor</b> 2161 * <p> 2162 * Description: <b>Who or what participated in the activities related to the condition</b><br> 2163 * Type: <b>reference</b><br> 2164 * Path: <b>Condition.participant.actor</b><br> 2165 * </p> 2166 */ 2167 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT_ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT_ACTOR); 2168 2169/** 2170 * Constant for fluent queries to be used to add include statements. Specifies 2171 * the path value of "<b>Condition:participant-actor</b>". 2172 */ 2173 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT_ACTOR = new ca.uhn.fhir.model.api.Include("Condition:participant-actor").toLocked(); 2174 2175 /** 2176 * Search parameter: <b>participant-function</b> 2177 * <p> 2178 * Description: <b>Type of involvement of the actor in the activities related to the condition</b><br> 2179 * Type: <b>token</b><br> 2180 * Path: <b>Condition.participant.function</b><br> 2181 * </p> 2182 */ 2183 @SearchParamDefinition(name="participant-function", path="Condition.participant.function", description="Type of involvement of the actor in the activities related to the condition", type="token" ) 2184 public static final String SP_PARTICIPANT_FUNCTION = "participant-function"; 2185 /** 2186 * <b>Fluent Client</b> search parameter constant for <b>participant-function</b> 2187 * <p> 2188 * Description: <b>Type of involvement of the actor in the activities related to the condition</b><br> 2189 * Type: <b>token</b><br> 2190 * Path: <b>Condition.participant.function</b><br> 2191 * </p> 2192 */ 2193 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_FUNCTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PARTICIPANT_FUNCTION); 2194 2195 /** 2196 * Search parameter: <b>recorded-date</b> 2197 * <p> 2198 * Description: <b>Date record was first recorded</b><br> 2199 * Type: <b>date</b><br> 2200 * Path: <b>Condition.recordedDate</b><br> 2201 * </p> 2202 */ 2203 @SearchParamDefinition(name="recorded-date", path="Condition.recordedDate", description="Date record was first recorded", type="date" ) 2204 public static final String SP_RECORDED_DATE = "recorded-date"; 2205 /** 2206 * <b>Fluent Client</b> search parameter constant for <b>recorded-date</b> 2207 * <p> 2208 * Description: <b>Date record was first recorded</b><br> 2209 * Type: <b>date</b><br> 2210 * Path: <b>Condition.recordedDate</b><br> 2211 * </p> 2212 */ 2213 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECORDED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_RECORDED_DATE); 2214 2215 /** 2216 * Search parameter: <b>severity</b> 2217 * <p> 2218 * Description: <b>The severity of the condition</b><br> 2219 * Type: <b>token</b><br> 2220 * Path: <b>Condition.severity</b><br> 2221 * </p> 2222 */ 2223 @SearchParamDefinition(name="severity", path="Condition.severity", description="The severity of the condition", type="token" ) 2224 public static final String SP_SEVERITY = "severity"; 2225 /** 2226 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2227 * <p> 2228 * Description: <b>The severity of the condition</b><br> 2229 * Type: <b>token</b><br> 2230 * Path: <b>Condition.severity</b><br> 2231 * </p> 2232 */ 2233 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SEVERITY); 2234 2235 /** 2236 * Search parameter: <b>stage</b> 2237 * <p> 2238 * Description: <b>Simple summary (disease specific)</b><br> 2239 * Type: <b>token</b><br> 2240 * Path: <b>Condition.stage.summary</b><br> 2241 * </p> 2242 */ 2243 @SearchParamDefinition(name="stage", path="Condition.stage.summary", description="Simple summary (disease specific)", type="token" ) 2244 public static final String SP_STAGE = "stage"; 2245 /** 2246 * <b>Fluent Client</b> search parameter constant for <b>stage</b> 2247 * <p> 2248 * Description: <b>Simple summary (disease specific)</b><br> 2249 * Type: <b>token</b><br> 2250 * Path: <b>Condition.stage.summary</b><br> 2251 * </p> 2252 */ 2253 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STAGE); 2254 2255 /** 2256 * Search parameter: <b>subject</b> 2257 * <p> 2258 * Description: <b>Who has the condition?</b><br> 2259 * Type: <b>reference</b><br> 2260 * Path: <b>Condition.subject</b><br> 2261 * </p> 2262 */ 2263 @SearchParamDefinition(name="subject", path="Condition.subject", description="Who has the condition?", type="reference", target={Group.class, Patient.class } ) 2264 public static final String SP_SUBJECT = "subject"; 2265 /** 2266 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2267 * <p> 2268 * Description: <b>Who has the condition?</b><br> 2269 * Type: <b>reference</b><br> 2270 * Path: <b>Condition.subject</b><br> 2271 * </p> 2272 */ 2273 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2274 2275/** 2276 * Constant for fluent queries to be used to add include statements. Specifies 2277 * the path value of "<b>Condition:subject</b>". 2278 */ 2279 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Condition:subject").toLocked(); 2280 2281 /** 2282 * Search parameter: <b>verification-status</b> 2283 * <p> 2284 * Description: <b>unconfirmed | provisional | differential | confirmed | refuted | entered-in-error</b><br> 2285 * Type: <b>token</b><br> 2286 * Path: <b>Condition.verificationStatus</b><br> 2287 * </p> 2288 */ 2289 @SearchParamDefinition(name="verification-status", path="Condition.verificationStatus", description="unconfirmed | provisional | differential | confirmed | refuted | entered-in-error", type="token" ) 2290 public static final String SP_VERIFICATION_STATUS = "verification-status"; 2291 /** 2292 * <b>Fluent Client</b> search parameter constant for <b>verification-status</b> 2293 * <p> 2294 * Description: <b>unconfirmed | provisional | differential | confirmed | refuted | entered-in-error</b><br> 2295 * Type: <b>token</b><br> 2296 * Path: <b>Condition.verificationStatus</b><br> 2297 * </p> 2298 */ 2299 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFICATION_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERIFICATION_STATUS); 2300 2301 /** 2302 * Search parameter: <b>code</b> 2303 * <p> 2304 * Description: <b>Multiple Resources: 2305 2306* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2307* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2308* [AuditEvent](auditevent.html): More specific code for the event 2309* [Basic](basic.html): Kind of Resource 2310* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2311* [Condition](condition.html): Code for the condition 2312* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2313* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2314* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2315* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2316* [ImagingSelection](imagingselection.html): The imaging selection status 2317* [List](list.html): What the purpose of this list is 2318* [Medication](medication.html): Returns medications for a specific code 2319* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2320* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2321* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2322* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2323* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2324* [Observation](observation.html): The code of the observation type 2325* [Procedure](procedure.html): A code to identify a procedure 2326* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2327* [Task](task.html): Search by task code 2328</b><br> 2329 * Type: <b>token</b><br> 2330 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2331 * </p> 2332 */ 2333 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2334 public static final String SP_CODE = "code"; 2335 /** 2336 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2337 * <p> 2338 * Description: <b>Multiple Resources: 2339 2340* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2341* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2342* [AuditEvent](auditevent.html): More specific code for the event 2343* [Basic](basic.html): Kind of Resource 2344* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2345* [Condition](condition.html): Code for the condition 2346* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2347* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2348* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2349* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2350* [ImagingSelection](imagingselection.html): The imaging selection status 2351* [List](list.html): What the purpose of this list is 2352* [Medication](medication.html): Returns medications for a specific code 2353* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2354* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2355* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2356* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2357* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2358* [Observation](observation.html): The code of the observation type 2359* [Procedure](procedure.html): A code to identify a procedure 2360* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2361* [Task](task.html): Search by task code 2362</b><br> 2363 * Type: <b>token</b><br> 2364 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2365 * </p> 2366 */ 2367 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2368 2369 /** 2370 * Search parameter: <b>encounter</b> 2371 * <p> 2372 * Description: <b>Multiple Resources: 2373 2374* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2375* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2376* [ChargeItem](chargeitem.html): Encounter associated with event 2377* [Claim](claim.html): Encounters associated with a billed line item 2378* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2379* [Communication](communication.html): The Encounter during which this Communication was created 2380* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2381* [Composition](composition.html): Context of the Composition 2382* [Condition](condition.html): The Encounter during which this Condition was created 2383* [DeviceRequest](devicerequest.html): Encounter during which request was created 2384* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2385* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2386* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2387* [Flag](flag.html): Alert relevant during encounter 2388* [ImagingStudy](imagingstudy.html): The context of the study 2389* [List](list.html): Context in which list created 2390* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2391* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2392* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2393* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2394* [Observation](observation.html): Encounter related to the observation 2395* [Procedure](procedure.html): The Encounter during which this Procedure was created 2396* [Provenance](provenance.html): Encounter related to the Provenance 2397* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2398* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2399* [RiskAssessment](riskassessment.html): Where was assessment performed? 2400* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2401* [Task](task.html): Search by encounter 2402* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2403</b><br> 2404 * Type: <b>reference</b><br> 2405 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2406 * </p> 2407 */ 2408 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2409 public static final String SP_ENCOUNTER = "encounter"; 2410 /** 2411 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2412 * <p> 2413 * Description: <b>Multiple Resources: 2414 2415* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2416* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2417* [ChargeItem](chargeitem.html): Encounter associated with event 2418* [Claim](claim.html): Encounters associated with a billed line item 2419* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2420* [Communication](communication.html): The Encounter during which this Communication was created 2421* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2422* [Composition](composition.html): Context of the Composition 2423* [Condition](condition.html): The Encounter during which this Condition was created 2424* [DeviceRequest](devicerequest.html): Encounter during which request was created 2425* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2426* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2427* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2428* [Flag](flag.html): Alert relevant during encounter 2429* [ImagingStudy](imagingstudy.html): The context of the study 2430* [List](list.html): Context in which list created 2431* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2432* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2433* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2434* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2435* [Observation](observation.html): Encounter related to the observation 2436* [Procedure](procedure.html): The Encounter during which this Procedure was created 2437* [Provenance](provenance.html): Encounter related to the Provenance 2438* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2439* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2440* [RiskAssessment](riskassessment.html): Where was assessment performed? 2441* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2442* [Task](task.html): Search by encounter 2443* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2444</b><br> 2445 * Type: <b>reference</b><br> 2446 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2447 * </p> 2448 */ 2449 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2450 2451/** 2452 * Constant for fluent queries to be used to add include statements. Specifies 2453 * the path value of "<b>Condition:encounter</b>". 2454 */ 2455 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Condition:encounter").toLocked(); 2456 2457 /** 2458 * Search parameter: <b>identifier</b> 2459 * <p> 2460 * Description: <b>Multiple Resources: 2461 2462* [Account](account.html): Account number 2463* [AdverseEvent](adverseevent.html): Business identifier for the event 2464* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2465* [Appointment](appointment.html): An Identifier of the Appointment 2466* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2467* [Basic](basic.html): Business identifier 2468* [BodyStructure](bodystructure.html): Bodystructure identifier 2469* [CarePlan](careplan.html): External Ids for this plan 2470* [CareTeam](careteam.html): External Ids for this team 2471* [ChargeItem](chargeitem.html): Business Identifier for item 2472* [Claim](claim.html): The primary identifier of the financial resource 2473* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2474* [ClinicalImpression](clinicalimpression.html): Business identifier 2475* [Communication](communication.html): Unique identifier 2476* [CommunicationRequest](communicationrequest.html): Unique identifier 2477* [Composition](composition.html): Version-independent identifier for the Composition 2478* [Condition](condition.html): A unique identifier of the condition record 2479* [Consent](consent.html): Identifier for this record (external references) 2480* [Contract](contract.html): The identity of the contract 2481* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2482* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2483* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2484* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2485* [DeviceRequest](devicerequest.html): Business identifier for request/order 2486* [DeviceUsage](deviceusage.html): Search by identifier 2487* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2488* [DocumentReference](documentreference.html): Identifier of the attachment binary 2489* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2490* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2491* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2492* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2493* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2494* [Flag](flag.html): Business identifier 2495* [Goal](goal.html): External Ids for this goal 2496* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2497* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2498* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2499* [Immunization](immunization.html): Business identifier 2500* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2501* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2502* [Invoice](invoice.html): Business Identifier for item 2503* [List](list.html): Business identifier 2504* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2505* [Medication](medication.html): Returns medications with this external identifier 2506* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2507* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2508* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2509* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2510* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2511* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2512* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2513* [Observation](observation.html): The unique id for a particular observation 2514* [Person](person.html): A person Identifier 2515* [Procedure](procedure.html): A unique identifier for a procedure 2516* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2517* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2518* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2519* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2520* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2521* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2522* [Specimen](specimen.html): The unique identifier associated with the specimen 2523* [SupplyDelivery](supplydelivery.html): External identifier 2524* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2525* [Task](task.html): Search for a task instance by its business identifier 2526* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2527</b><br> 2528 * Type: <b>token</b><br> 2529 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2530 * </p> 2531 */ 2532 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2533 public static final String SP_IDENTIFIER = "identifier"; 2534 /** 2535 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2536 * <p> 2537 * Description: <b>Multiple Resources: 2538 2539* [Account](account.html): Account number 2540* [AdverseEvent](adverseevent.html): Business identifier for the event 2541* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2542* [Appointment](appointment.html): An Identifier of the Appointment 2543* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2544* [Basic](basic.html): Business identifier 2545* [BodyStructure](bodystructure.html): Bodystructure identifier 2546* [CarePlan](careplan.html): External Ids for this plan 2547* [CareTeam](careteam.html): External Ids for this team 2548* [ChargeItem](chargeitem.html): Business Identifier for item 2549* [Claim](claim.html): The primary identifier of the financial resource 2550* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2551* [ClinicalImpression](clinicalimpression.html): Business identifier 2552* [Communication](communication.html): Unique identifier 2553* [CommunicationRequest](communicationrequest.html): Unique identifier 2554* [Composition](composition.html): Version-independent identifier for the Composition 2555* [Condition](condition.html): A unique identifier of the condition record 2556* [Consent](consent.html): Identifier for this record (external references) 2557* [Contract](contract.html): The identity of the contract 2558* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2559* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2560* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2561* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2562* [DeviceRequest](devicerequest.html): Business identifier for request/order 2563* [DeviceUsage](deviceusage.html): Search by identifier 2564* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2565* [DocumentReference](documentreference.html): Identifier of the attachment binary 2566* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2567* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2568* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2569* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2570* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2571* [Flag](flag.html): Business identifier 2572* [Goal](goal.html): External Ids for this goal 2573* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2574* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2575* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2576* [Immunization](immunization.html): Business identifier 2577* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2578* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2579* [Invoice](invoice.html): Business Identifier for item 2580* [List](list.html): Business identifier 2581* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2582* [Medication](medication.html): Returns medications with this external identifier 2583* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2584* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2585* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2586* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2587* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2588* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2589* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2590* [Observation](observation.html): The unique id for a particular observation 2591* [Person](person.html): A person Identifier 2592* [Procedure](procedure.html): A unique identifier for a procedure 2593* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2594* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2595* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2596* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2597* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2598* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2599* [Specimen](specimen.html): The unique identifier associated with the specimen 2600* [SupplyDelivery](supplydelivery.html): External identifier 2601* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2602* [Task](task.html): Search for a task instance by its business identifier 2603* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2604</b><br> 2605 * Type: <b>token</b><br> 2606 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2607 * </p> 2608 */ 2609 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2610 2611 /** 2612 * Search parameter: <b>patient</b> 2613 * <p> 2614 * Description: <b>Multiple Resources: 2615 2616* [Account](account.html): The entity that caused the expenses 2617* [AdverseEvent](adverseevent.html): Subject impacted by event 2618* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2619* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2620* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2621* [AuditEvent](auditevent.html): Where the activity involved patient data 2622* [Basic](basic.html): Identifies the focus of this resource 2623* [BodyStructure](bodystructure.html): Who this is about 2624* [CarePlan](careplan.html): Who the care plan is for 2625* [CareTeam](careteam.html): Who care team is for 2626* [ChargeItem](chargeitem.html): Individual service was done for/to 2627* [Claim](claim.html): Patient receiving the products or services 2628* [ClaimResponse](claimresponse.html): The subject of care 2629* [ClinicalImpression](clinicalimpression.html): Patient assessed 2630* [Communication](communication.html): Focus of message 2631* [CommunicationRequest](communicationrequest.html): Focus of message 2632* [Composition](composition.html): Who and/or what the composition is about 2633* [Condition](condition.html): Who has the condition? 2634* [Consent](consent.html): Who the consent applies to 2635* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2636* [Coverage](coverage.html): Retrieve coverages for a patient 2637* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2638* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2639* [DetectedIssue](detectedissue.html): Associated patient 2640* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2641* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2642* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2643* [DocumentReference](documentreference.html): Who/what is the subject of the document 2644* [Encounter](encounter.html): The patient present at the encounter 2645* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2646* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2647* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2648* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2649* [Flag](flag.html): The identity of a subject to list flags for 2650* [Goal](goal.html): Who this goal is intended for 2651* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2652* [ImagingSelection](imagingselection.html): Who the study is about 2653* [ImagingStudy](imagingstudy.html): Who the study is about 2654* [Immunization](immunization.html): The patient for the vaccination record 2655* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2656* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2657* [Invoice](invoice.html): Recipient(s) of goods and services 2658* [List](list.html): If all resources have the same subject 2659* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2660* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2661* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2662* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2663* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2664* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2665* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2666* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2667* [Observation](observation.html): The subject that the observation is about (if patient) 2668* [Person](person.html): The Person links to this Patient 2669* [Procedure](procedure.html): Search by subject - a patient 2670* [Provenance](provenance.html): Where the activity involved patient data 2671* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2672* [RelatedPerson](relatedperson.html): The patient this related person is related to 2673* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2674* [ResearchSubject](researchsubject.html): Who or what is part of study 2675* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2676* [ServiceRequest](servicerequest.html): Search by subject - a patient 2677* [Specimen](specimen.html): The patient the specimen comes from 2678* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2679* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2680* [Task](task.html): Search by patient 2681* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2682</b><br> 2683 * Type: <b>reference</b><br> 2684 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2685 * </p> 2686 */ 2687 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2688 public static final String SP_PATIENT = "patient"; 2689 /** 2690 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2691 * <p> 2692 * Description: <b>Multiple Resources: 2693 2694* [Account](account.html): The entity that caused the expenses 2695* [AdverseEvent](adverseevent.html): Subject impacted by event 2696* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2697* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2698* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2699* [AuditEvent](auditevent.html): Where the activity involved patient data 2700* [Basic](basic.html): Identifies the focus of this resource 2701* [BodyStructure](bodystructure.html): Who this is about 2702* [CarePlan](careplan.html): Who the care plan is for 2703* [CareTeam](careteam.html): Who care team is for 2704* [ChargeItem](chargeitem.html): Individual service was done for/to 2705* [Claim](claim.html): Patient receiving the products or services 2706* [ClaimResponse](claimresponse.html): The subject of care 2707* [ClinicalImpression](clinicalimpression.html): Patient assessed 2708* [Communication](communication.html): Focus of message 2709* [CommunicationRequest](communicationrequest.html): Focus of message 2710* [Composition](composition.html): Who and/or what the composition is about 2711* [Condition](condition.html): Who has the condition? 2712* [Consent](consent.html): Who the consent applies to 2713* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2714* [Coverage](coverage.html): Retrieve coverages for a patient 2715* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2716* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2717* [DetectedIssue](detectedissue.html): Associated patient 2718* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2719* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2720* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2721* [DocumentReference](documentreference.html): Who/what is the subject of the document 2722* [Encounter](encounter.html): The patient present at the encounter 2723* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2724* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2725* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2726* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2727* [Flag](flag.html): The identity of a subject to list flags for 2728* [Goal](goal.html): Who this goal is intended for 2729* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2730* [ImagingSelection](imagingselection.html): Who the study is about 2731* [ImagingStudy](imagingstudy.html): Who the study is about 2732* [Immunization](immunization.html): The patient for the vaccination record 2733* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2734* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2735* [Invoice](invoice.html): Recipient(s) of goods and services 2736* [List](list.html): If all resources have the same subject 2737* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2738* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2739* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2740* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2741* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2742* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2743* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2744* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2745* [Observation](observation.html): The subject that the observation is about (if patient) 2746* [Person](person.html): The Person links to this Patient 2747* [Procedure](procedure.html): Search by subject - a patient 2748* [Provenance](provenance.html): Where the activity involved patient data 2749* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2750* [RelatedPerson](relatedperson.html): The patient this related person is related to 2751* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2752* [ResearchSubject](researchsubject.html): Who or what is part of study 2753* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2754* [ServiceRequest](servicerequest.html): Search by subject - a patient 2755* [Specimen](specimen.html): The patient the specimen comes from 2756* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2757* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2758* [Task](task.html): Search by patient 2759* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2760</b><br> 2761 * Type: <b>reference</b><br> 2762 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2763 * </p> 2764 */ 2765 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2766 2767/** 2768 * Constant for fluent queries to be used to add include statements. Specifies 2769 * the path value of "<b>Condition:patient</b>". 2770 */ 2771 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Condition:patient").toLocked(); 2772 2773 2774} 2775