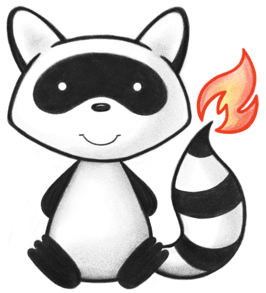
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A definition of a condition and information relevant to managing it. 052 */ 053@ResourceDef(name="ConditionDefinition", profile="http://hl7.org/fhir/StructureDefinition/ConditionDefinition") 054public class ConditionDefinition extends MetadataResource { 055 056 public enum ConditionPreconditionType { 057 /** 058 * The observation is very sensitive for the condition, but may also indicate other conditions. 059 */ 060 SENSITIVE, 061 /** 062 * The observation is very specific for this condition, but not particularly sensitive. 063 */ 064 SPECIFIC, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static ConditionPreconditionType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("sensitive".equals(codeString)) 073 return SENSITIVE; 074 if ("specific".equals(codeString)) 075 return SPECIFIC; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown ConditionPreconditionType code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case SENSITIVE: return "sensitive"; 084 case SPECIFIC: return "specific"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case SENSITIVE: return "http://hl7.org/fhir/condition-precondition-type"; 092 case SPECIFIC: return "http://hl7.org/fhir/condition-precondition-type"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case SENSITIVE: return "The observation is very sensitive for the condition, but may also indicate other conditions."; 100 case SPECIFIC: return "The observation is very specific for this condition, but not particularly sensitive."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case SENSITIVE: return "Sensitive"; 108 case SPECIFIC: return "Specific"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class ConditionPreconditionTypeEnumFactory implements EnumFactory<ConditionPreconditionType> { 116 public ConditionPreconditionType fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("sensitive".equals(codeString)) 121 return ConditionPreconditionType.SENSITIVE; 122 if ("specific".equals(codeString)) 123 return ConditionPreconditionType.SPECIFIC; 124 throw new IllegalArgumentException("Unknown ConditionPreconditionType code '"+codeString+"'"); 125 } 126 public Enumeration<ConditionPreconditionType> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.NULL, code); 134 if ("sensitive".equals(codeString)) 135 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.SENSITIVE, code); 136 if ("specific".equals(codeString)) 137 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.SPECIFIC, code); 138 throw new FHIRException("Unknown ConditionPreconditionType code '"+codeString+"'"); 139 } 140 public String toCode(ConditionPreconditionType code) { 141 if (code == ConditionPreconditionType.SENSITIVE) 142 return "sensitive"; 143 if (code == ConditionPreconditionType.SPECIFIC) 144 return "specific"; 145 return "?"; 146 } 147 public String toSystem(ConditionPreconditionType code) { 148 return code.getSystem(); 149 } 150 } 151 152 public enum ConditionQuestionnairePurpose { 153 /** 154 * A pre-admit questionnaire. 155 */ 156 PREADMIT, 157 /** 158 * A questionnaire that helps with diferential diagnosis. 159 */ 160 DIFFDIAGNOSIS, 161 /** 162 * A questionnaire to check on outcomes for the patient. 163 */ 164 OUTCOME, 165 /** 166 * added to help the parsers with the generic types 167 */ 168 NULL; 169 public static ConditionQuestionnairePurpose fromCode(String codeString) throws FHIRException { 170 if (codeString == null || "".equals(codeString)) 171 return null; 172 if ("preadmit".equals(codeString)) 173 return PREADMIT; 174 if ("diff-diagnosis".equals(codeString)) 175 return DIFFDIAGNOSIS; 176 if ("outcome".equals(codeString)) 177 return OUTCOME; 178 if (Configuration.isAcceptInvalidEnums()) 179 return null; 180 else 181 throw new FHIRException("Unknown ConditionQuestionnairePurpose code '"+codeString+"'"); 182 } 183 public String toCode() { 184 switch (this) { 185 case PREADMIT: return "preadmit"; 186 case DIFFDIAGNOSIS: return "diff-diagnosis"; 187 case OUTCOME: return "outcome"; 188 case NULL: return null; 189 default: return "?"; 190 } 191 } 192 public String getSystem() { 193 switch (this) { 194 case PREADMIT: return "http://hl7.org/fhir/condition-questionnaire-purpose"; 195 case DIFFDIAGNOSIS: return "http://hl7.org/fhir/condition-questionnaire-purpose"; 196 case OUTCOME: return "http://hl7.org/fhir/condition-questionnaire-purpose"; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 public String getDefinition() { 202 switch (this) { 203 case PREADMIT: return "A pre-admit questionnaire."; 204 case DIFFDIAGNOSIS: return "A questionnaire that helps with diferential diagnosis."; 205 case OUTCOME: return "A questionnaire to check on outcomes for the patient."; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 public String getDisplay() { 211 switch (this) { 212 case PREADMIT: return "Pre-admit"; 213 case DIFFDIAGNOSIS: return "Diff Diagnosis"; 214 case OUTCOME: return "Outcome"; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 } 220 221 public static class ConditionQuestionnairePurposeEnumFactory implements EnumFactory<ConditionQuestionnairePurpose> { 222 public ConditionQuestionnairePurpose fromCode(String codeString) throws IllegalArgumentException { 223 if (codeString == null || "".equals(codeString)) 224 if (codeString == null || "".equals(codeString)) 225 return null; 226 if ("preadmit".equals(codeString)) 227 return ConditionQuestionnairePurpose.PREADMIT; 228 if ("diff-diagnosis".equals(codeString)) 229 return ConditionQuestionnairePurpose.DIFFDIAGNOSIS; 230 if ("outcome".equals(codeString)) 231 return ConditionQuestionnairePurpose.OUTCOME; 232 throw new IllegalArgumentException("Unknown ConditionQuestionnairePurpose code '"+codeString+"'"); 233 } 234 public Enumeration<ConditionQuestionnairePurpose> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.NULL, code); 239 String codeString = ((PrimitiveType) code).asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.NULL, code); 242 if ("preadmit".equals(codeString)) 243 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.PREADMIT, code); 244 if ("diff-diagnosis".equals(codeString)) 245 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.DIFFDIAGNOSIS, code); 246 if ("outcome".equals(codeString)) 247 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.OUTCOME, code); 248 throw new FHIRException("Unknown ConditionQuestionnairePurpose code '"+codeString+"'"); 249 } 250 public String toCode(ConditionQuestionnairePurpose code) { 251 if (code == ConditionQuestionnairePurpose.PREADMIT) 252 return "preadmit"; 253 if (code == ConditionQuestionnairePurpose.DIFFDIAGNOSIS) 254 return "diff-diagnosis"; 255 if (code == ConditionQuestionnairePurpose.OUTCOME) 256 return "outcome"; 257 return "?"; 258 } 259 public String toSystem(ConditionQuestionnairePurpose code) { 260 return code.getSystem(); 261 } 262 } 263 264 @Block() 265 public static class ConditionDefinitionObservationComponent extends BackboneElement implements IBaseBackboneElement { 266 /** 267 * Category that is relevant. 268 */ 269 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 270 @Description(shortDefinition="Category that is relevant", formalDefinition="Category that is relevant." ) 271 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-category") 272 protected CodeableConcept category; 273 274 /** 275 * Code for relevant Observation. 276 */ 277 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 278 @Description(shortDefinition="Code for relevant Observation", formalDefinition="Code for relevant Observation." ) 279 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 280 protected CodeableConcept code; 281 282 private static final long serialVersionUID = -1433986479L; 283 284 /** 285 * Constructor 286 */ 287 public ConditionDefinitionObservationComponent() { 288 super(); 289 } 290 291 /** 292 * @return {@link #category} (Category that is relevant.) 293 */ 294 public CodeableConcept getCategory() { 295 if (this.category == null) 296 if (Configuration.errorOnAutoCreate()) 297 throw new Error("Attempt to auto-create ConditionDefinitionObservationComponent.category"); 298 else if (Configuration.doAutoCreate()) 299 this.category = new CodeableConcept(); // cc 300 return this.category; 301 } 302 303 public boolean hasCategory() { 304 return this.category != null && !this.category.isEmpty(); 305 } 306 307 /** 308 * @param value {@link #category} (Category that is relevant.) 309 */ 310 public ConditionDefinitionObservationComponent setCategory(CodeableConcept value) { 311 this.category = value; 312 return this; 313 } 314 315 /** 316 * @return {@link #code} (Code for relevant Observation.) 317 */ 318 public CodeableConcept getCode() { 319 if (this.code == null) 320 if (Configuration.errorOnAutoCreate()) 321 throw new Error("Attempt to auto-create ConditionDefinitionObservationComponent.code"); 322 else if (Configuration.doAutoCreate()) 323 this.code = new CodeableConcept(); // cc 324 return this.code; 325 } 326 327 public boolean hasCode() { 328 return this.code != null && !this.code.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #code} (Code for relevant Observation.) 333 */ 334 public ConditionDefinitionObservationComponent setCode(CodeableConcept value) { 335 this.code = value; 336 return this; 337 } 338 339 protected void listChildren(List<Property> children) { 340 super.listChildren(children); 341 children.add(new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category)); 342 children.add(new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code)); 343 } 344 345 @Override 346 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 347 switch (_hash) { 348 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category); 349 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code); 350 default: return super.getNamedProperty(_hash, _name, _checkValid); 351 } 352 353 } 354 355 @Override 356 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 357 switch (hash) { 358 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 359 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 360 default: return super.getProperty(hash, name, checkValid); 361 } 362 363 } 364 365 @Override 366 public Base setProperty(int hash, String name, Base value) throws FHIRException { 367 switch (hash) { 368 case 50511102: // category 369 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 370 return value; 371 case 3059181: // code 372 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 373 return value; 374 default: return super.setProperty(hash, name, value); 375 } 376 377 } 378 379 @Override 380 public Base setProperty(String name, Base value) throws FHIRException { 381 if (name.equals("category")) { 382 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 383 } else if (name.equals("code")) { 384 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 385 } else 386 return super.setProperty(name, value); 387 return value; 388 } 389 390 @Override 391 public void removeChild(String name, Base value) throws FHIRException { 392 if (name.equals("category")) { 393 this.category = null; 394 } else if (name.equals("code")) { 395 this.code = null; 396 } else 397 super.removeChild(name, value); 398 399 } 400 401 @Override 402 public Base makeProperty(int hash, String name) throws FHIRException { 403 switch (hash) { 404 case 50511102: return getCategory(); 405 case 3059181: return getCode(); 406 default: return super.makeProperty(hash, name); 407 } 408 409 } 410 411 @Override 412 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 413 switch (hash) { 414 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 415 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 416 default: return super.getTypesForProperty(hash, name); 417 } 418 419 } 420 421 @Override 422 public Base addChild(String name) throws FHIRException { 423 if (name.equals("category")) { 424 this.category = new CodeableConcept(); 425 return this.category; 426 } 427 else if (name.equals("code")) { 428 this.code = new CodeableConcept(); 429 return this.code; 430 } 431 else 432 return super.addChild(name); 433 } 434 435 public ConditionDefinitionObservationComponent copy() { 436 ConditionDefinitionObservationComponent dst = new ConditionDefinitionObservationComponent(); 437 copyValues(dst); 438 return dst; 439 } 440 441 public void copyValues(ConditionDefinitionObservationComponent dst) { 442 super.copyValues(dst); 443 dst.category = category == null ? null : category.copy(); 444 dst.code = code == null ? null : code.copy(); 445 } 446 447 @Override 448 public boolean equalsDeep(Base other_) { 449 if (!super.equalsDeep(other_)) 450 return false; 451 if (!(other_ instanceof ConditionDefinitionObservationComponent)) 452 return false; 453 ConditionDefinitionObservationComponent o = (ConditionDefinitionObservationComponent) other_; 454 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true); 455 } 456 457 @Override 458 public boolean equalsShallow(Base other_) { 459 if (!super.equalsShallow(other_)) 460 return false; 461 if (!(other_ instanceof ConditionDefinitionObservationComponent)) 462 return false; 463 ConditionDefinitionObservationComponent o = (ConditionDefinitionObservationComponent) other_; 464 return true; 465 } 466 467 public boolean isEmpty() { 468 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, code); 469 } 470 471 public String fhirType() { 472 return "ConditionDefinition.observation"; 473 474 } 475 476 } 477 478 @Block() 479 public static class ConditionDefinitionMedicationComponent extends BackboneElement implements IBaseBackboneElement { 480 /** 481 * Category that is relevant. 482 */ 483 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 484 @Description(shortDefinition="Category that is relevant", formalDefinition="Category that is relevant." ) 485 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/medicationrequest-category") 486 protected CodeableConcept category; 487 488 /** 489 * Code for relevant Medication. 490 */ 491 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 492 @Description(shortDefinition="Code for relevant Medication", formalDefinition="Code for relevant Medication." ) 493 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 494 protected CodeableConcept code; 495 496 private static final long serialVersionUID = -1433986479L; 497 498 /** 499 * Constructor 500 */ 501 public ConditionDefinitionMedicationComponent() { 502 super(); 503 } 504 505 /** 506 * @return {@link #category} (Category that is relevant.) 507 */ 508 public CodeableConcept getCategory() { 509 if (this.category == null) 510 if (Configuration.errorOnAutoCreate()) 511 throw new Error("Attempt to auto-create ConditionDefinitionMedicationComponent.category"); 512 else if (Configuration.doAutoCreate()) 513 this.category = new CodeableConcept(); // cc 514 return this.category; 515 } 516 517 public boolean hasCategory() { 518 return this.category != null && !this.category.isEmpty(); 519 } 520 521 /** 522 * @param value {@link #category} (Category that is relevant.) 523 */ 524 public ConditionDefinitionMedicationComponent setCategory(CodeableConcept value) { 525 this.category = value; 526 return this; 527 } 528 529 /** 530 * @return {@link #code} (Code for relevant Medication.) 531 */ 532 public CodeableConcept getCode() { 533 if (this.code == null) 534 if (Configuration.errorOnAutoCreate()) 535 throw new Error("Attempt to auto-create ConditionDefinitionMedicationComponent.code"); 536 else if (Configuration.doAutoCreate()) 537 this.code = new CodeableConcept(); // cc 538 return this.code; 539 } 540 541 public boolean hasCode() { 542 return this.code != null && !this.code.isEmpty(); 543 } 544 545 /** 546 * @param value {@link #code} (Code for relevant Medication.) 547 */ 548 public ConditionDefinitionMedicationComponent setCode(CodeableConcept value) { 549 this.code = value; 550 return this; 551 } 552 553 protected void listChildren(List<Property> children) { 554 super.listChildren(children); 555 children.add(new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category)); 556 children.add(new Property("code", "CodeableConcept", "Code for relevant Medication.", 0, 1, code)); 557 } 558 559 @Override 560 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 561 switch (_hash) { 562 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category); 563 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for relevant Medication.", 0, 1, code); 564 default: return super.getNamedProperty(_hash, _name, _checkValid); 565 } 566 567 } 568 569 @Override 570 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 571 switch (hash) { 572 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 573 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 574 default: return super.getProperty(hash, name, checkValid); 575 } 576 577 } 578 579 @Override 580 public Base setProperty(int hash, String name, Base value) throws FHIRException { 581 switch (hash) { 582 case 50511102: // category 583 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 584 return value; 585 case 3059181: // code 586 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 587 return value; 588 default: return super.setProperty(hash, name, value); 589 } 590 591 } 592 593 @Override 594 public Base setProperty(String name, Base value) throws FHIRException { 595 if (name.equals("category")) { 596 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 597 } else if (name.equals("code")) { 598 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 599 } else 600 return super.setProperty(name, value); 601 return value; 602 } 603 604 @Override 605 public void removeChild(String name, Base value) throws FHIRException { 606 if (name.equals("category")) { 607 this.category = null; 608 } else if (name.equals("code")) { 609 this.code = null; 610 } else 611 super.removeChild(name, value); 612 613 } 614 615 @Override 616 public Base makeProperty(int hash, String name) throws FHIRException { 617 switch (hash) { 618 case 50511102: return getCategory(); 619 case 3059181: return getCode(); 620 default: return super.makeProperty(hash, name); 621 } 622 623 } 624 625 @Override 626 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 627 switch (hash) { 628 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 629 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 630 default: return super.getTypesForProperty(hash, name); 631 } 632 633 } 634 635 @Override 636 public Base addChild(String name) throws FHIRException { 637 if (name.equals("category")) { 638 this.category = new CodeableConcept(); 639 return this.category; 640 } 641 else if (name.equals("code")) { 642 this.code = new CodeableConcept(); 643 return this.code; 644 } 645 else 646 return super.addChild(name); 647 } 648 649 public ConditionDefinitionMedicationComponent copy() { 650 ConditionDefinitionMedicationComponent dst = new ConditionDefinitionMedicationComponent(); 651 copyValues(dst); 652 return dst; 653 } 654 655 public void copyValues(ConditionDefinitionMedicationComponent dst) { 656 super.copyValues(dst); 657 dst.category = category == null ? null : category.copy(); 658 dst.code = code == null ? null : code.copy(); 659 } 660 661 @Override 662 public boolean equalsDeep(Base other_) { 663 if (!super.equalsDeep(other_)) 664 return false; 665 if (!(other_ instanceof ConditionDefinitionMedicationComponent)) 666 return false; 667 ConditionDefinitionMedicationComponent o = (ConditionDefinitionMedicationComponent) other_; 668 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true); 669 } 670 671 @Override 672 public boolean equalsShallow(Base other_) { 673 if (!super.equalsShallow(other_)) 674 return false; 675 if (!(other_ instanceof ConditionDefinitionMedicationComponent)) 676 return false; 677 ConditionDefinitionMedicationComponent o = (ConditionDefinitionMedicationComponent) other_; 678 return true; 679 } 680 681 public boolean isEmpty() { 682 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, code); 683 } 684 685 public String fhirType() { 686 return "ConditionDefinition.medication"; 687 688 } 689 690 } 691 692 @Block() 693 public static class ConditionDefinitionPreconditionComponent extends BackboneElement implements IBaseBackboneElement { 694 /** 695 * Kind of pre-condition. 696 */ 697 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 698 @Description(shortDefinition="sensitive | specific", formalDefinition="Kind of pre-condition." ) 699 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-precondition-type") 700 protected Enumeration<ConditionPreconditionType> type; 701 702 /** 703 * Code for relevant Observation. 704 */ 705 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 706 @Description(shortDefinition="Code for relevant Observation", formalDefinition="Code for relevant Observation." ) 707 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 708 protected CodeableConcept code; 709 710 /** 711 * Value of Observation. 712 */ 713 @Child(name = "value", type = {CodeableConcept.class, Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 714 @Description(shortDefinition="Value of Observation", formalDefinition="Value of Observation." ) 715 protected DataType value; 716 717 private static final long serialVersionUID = -1210333235L; 718 719 /** 720 * Constructor 721 */ 722 public ConditionDefinitionPreconditionComponent() { 723 super(); 724 } 725 726 /** 727 * Constructor 728 */ 729 public ConditionDefinitionPreconditionComponent(ConditionPreconditionType type, CodeableConcept code) { 730 super(); 731 this.setType(type); 732 this.setCode(code); 733 } 734 735 /** 736 * @return {@link #type} (Kind of pre-condition.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 737 */ 738 public Enumeration<ConditionPreconditionType> getTypeElement() { 739 if (this.type == null) 740 if (Configuration.errorOnAutoCreate()) 741 throw new Error("Attempt to auto-create ConditionDefinitionPreconditionComponent.type"); 742 else if (Configuration.doAutoCreate()) 743 this.type = new Enumeration<ConditionPreconditionType>(new ConditionPreconditionTypeEnumFactory()); // bb 744 return this.type; 745 } 746 747 public boolean hasTypeElement() { 748 return this.type != null && !this.type.isEmpty(); 749 } 750 751 public boolean hasType() { 752 return this.type != null && !this.type.isEmpty(); 753 } 754 755 /** 756 * @param value {@link #type} (Kind of pre-condition.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 757 */ 758 public ConditionDefinitionPreconditionComponent setTypeElement(Enumeration<ConditionPreconditionType> value) { 759 this.type = value; 760 return this; 761 } 762 763 /** 764 * @return Kind of pre-condition. 765 */ 766 public ConditionPreconditionType getType() { 767 return this.type == null ? null : this.type.getValue(); 768 } 769 770 /** 771 * @param value Kind of pre-condition. 772 */ 773 public ConditionDefinitionPreconditionComponent setType(ConditionPreconditionType value) { 774 if (this.type == null) 775 this.type = new Enumeration<ConditionPreconditionType>(new ConditionPreconditionTypeEnumFactory()); 776 this.type.setValue(value); 777 return this; 778 } 779 780 /** 781 * @return {@link #code} (Code for relevant Observation.) 782 */ 783 public CodeableConcept getCode() { 784 if (this.code == null) 785 if (Configuration.errorOnAutoCreate()) 786 throw new Error("Attempt to auto-create ConditionDefinitionPreconditionComponent.code"); 787 else if (Configuration.doAutoCreate()) 788 this.code = new CodeableConcept(); // cc 789 return this.code; 790 } 791 792 public boolean hasCode() { 793 return this.code != null && !this.code.isEmpty(); 794 } 795 796 /** 797 * @param value {@link #code} (Code for relevant Observation.) 798 */ 799 public ConditionDefinitionPreconditionComponent setCode(CodeableConcept value) { 800 this.code = value; 801 return this; 802 } 803 804 /** 805 * @return {@link #value} (Value of Observation.) 806 */ 807 public DataType getValue() { 808 return this.value; 809 } 810 811 /** 812 * @return {@link #value} (Value of Observation.) 813 */ 814 public CodeableConcept getValueCodeableConcept() throws FHIRException { 815 if (this.value == null) 816 this.value = new CodeableConcept(); 817 if (!(this.value instanceof CodeableConcept)) 818 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 819 return (CodeableConcept) this.value; 820 } 821 822 public boolean hasValueCodeableConcept() { 823 return this != null && this.value instanceof CodeableConcept; 824 } 825 826 /** 827 * @return {@link #value} (Value of Observation.) 828 */ 829 public Quantity getValueQuantity() throws FHIRException { 830 if (this.value == null) 831 this.value = new Quantity(); 832 if (!(this.value instanceof Quantity)) 833 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 834 return (Quantity) this.value; 835 } 836 837 public boolean hasValueQuantity() { 838 return this != null && this.value instanceof Quantity; 839 } 840 841 public boolean hasValue() { 842 return this.value != null && !this.value.isEmpty(); 843 } 844 845 /** 846 * @param value {@link #value} (Value of Observation.) 847 */ 848 public ConditionDefinitionPreconditionComponent setValue(DataType value) { 849 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity)) 850 throw new FHIRException("Not the right type for ConditionDefinition.precondition.value[x]: "+value.fhirType()); 851 this.value = value; 852 return this; 853 } 854 855 protected void listChildren(List<Property> children) { 856 super.listChildren(children); 857 children.add(new Property("type", "code", "Kind of pre-condition.", 0, 1, type)); 858 children.add(new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code)); 859 children.add(new Property("value[x]", "CodeableConcept|Quantity", "Value of Observation.", 0, 1, value)); 860 } 861 862 @Override 863 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 864 switch (_hash) { 865 case 3575610: /*type*/ return new Property("type", "code", "Kind of pre-condition.", 0, 1, type); 866 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code); 867 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity", "Value of Observation.", 0, 1, value); 868 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity", "Value of Observation.", 0, 1, value); 869 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Value of Observation.", 0, 1, value); 870 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Value of Observation.", 0, 1, value); 871 default: return super.getNamedProperty(_hash, _name, _checkValid); 872 } 873 874 } 875 876 @Override 877 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 878 switch (hash) { 879 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ConditionPreconditionType> 880 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 881 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 882 default: return super.getProperty(hash, name, checkValid); 883 } 884 885 } 886 887 @Override 888 public Base setProperty(int hash, String name, Base value) throws FHIRException { 889 switch (hash) { 890 case 3575610: // type 891 value = new ConditionPreconditionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 892 this.type = (Enumeration) value; // Enumeration<ConditionPreconditionType> 893 return value; 894 case 3059181: // code 895 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 896 return value; 897 case 111972721: // value 898 this.value = TypeConvertor.castToType(value); // DataType 899 return value; 900 default: return super.setProperty(hash, name, value); 901 } 902 903 } 904 905 @Override 906 public Base setProperty(String name, Base value) throws FHIRException { 907 if (name.equals("type")) { 908 value = new ConditionPreconditionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 909 this.type = (Enumeration) value; // Enumeration<ConditionPreconditionType> 910 } else if (name.equals("code")) { 911 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 912 } else if (name.equals("value[x]")) { 913 this.value = TypeConvertor.castToType(value); // DataType 914 } else 915 return super.setProperty(name, value); 916 return value; 917 } 918 919 @Override 920 public void removeChild(String name, Base value) throws FHIRException { 921 if (name.equals("type")) { 922 value = new ConditionPreconditionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 923 this.type = (Enumeration) value; // Enumeration<ConditionPreconditionType> 924 } else if (name.equals("code")) { 925 this.code = null; 926 } else if (name.equals("value[x]")) { 927 this.value = null; 928 } else 929 super.removeChild(name, value); 930 931 } 932 933 @Override 934 public Base makeProperty(int hash, String name) throws FHIRException { 935 switch (hash) { 936 case 3575610: return getTypeElement(); 937 case 3059181: return getCode(); 938 case -1410166417: return getValue(); 939 case 111972721: return getValue(); 940 default: return super.makeProperty(hash, name); 941 } 942 943 } 944 945 @Override 946 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 947 switch (hash) { 948 case 3575610: /*type*/ return new String[] {"code"}; 949 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 950 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity"}; 951 default: return super.getTypesForProperty(hash, name); 952 } 953 954 } 955 956 @Override 957 public Base addChild(String name) throws FHIRException { 958 if (name.equals("type")) { 959 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.precondition.type"); 960 } 961 else if (name.equals("code")) { 962 this.code = new CodeableConcept(); 963 return this.code; 964 } 965 else if (name.equals("valueCodeableConcept")) { 966 this.value = new CodeableConcept(); 967 return this.value; 968 } 969 else if (name.equals("valueQuantity")) { 970 this.value = new Quantity(); 971 return this.value; 972 } 973 else 974 return super.addChild(name); 975 } 976 977 public ConditionDefinitionPreconditionComponent copy() { 978 ConditionDefinitionPreconditionComponent dst = new ConditionDefinitionPreconditionComponent(); 979 copyValues(dst); 980 return dst; 981 } 982 983 public void copyValues(ConditionDefinitionPreconditionComponent dst) { 984 super.copyValues(dst); 985 dst.type = type == null ? null : type.copy(); 986 dst.code = code == null ? null : code.copy(); 987 dst.value = value == null ? null : value.copy(); 988 } 989 990 @Override 991 public boolean equalsDeep(Base other_) { 992 if (!super.equalsDeep(other_)) 993 return false; 994 if (!(other_ instanceof ConditionDefinitionPreconditionComponent)) 995 return false; 996 ConditionDefinitionPreconditionComponent o = (ConditionDefinitionPreconditionComponent) other_; 997 return compareDeep(type, o.type, true) && compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 998 ; 999 } 1000 1001 @Override 1002 public boolean equalsShallow(Base other_) { 1003 if (!super.equalsShallow(other_)) 1004 return false; 1005 if (!(other_ instanceof ConditionDefinitionPreconditionComponent)) 1006 return false; 1007 ConditionDefinitionPreconditionComponent o = (ConditionDefinitionPreconditionComponent) other_; 1008 return compareValues(type, o.type, true); 1009 } 1010 1011 public boolean isEmpty() { 1012 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, code, value); 1013 } 1014 1015 public String fhirType() { 1016 return "ConditionDefinition.precondition"; 1017 1018 } 1019 1020 } 1021 1022 @Block() 1023 public static class ConditionDefinitionQuestionnaireComponent extends BackboneElement implements IBaseBackboneElement { 1024 /** 1025 * Use of the questionnaire. 1026 */ 1027 @Child(name = "purpose", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1028 @Description(shortDefinition="preadmit | diff-diagnosis | outcome", formalDefinition="Use of the questionnaire." ) 1029 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-questionnaire-purpose") 1030 protected Enumeration<ConditionQuestionnairePurpose> purpose; 1031 1032 /** 1033 * Specific Questionnaire. 1034 */ 1035 @Child(name = "reference", type = {Questionnaire.class}, order=2, min=1, max=1, modifier=false, summary=false) 1036 @Description(shortDefinition="Specific Questionnaire", formalDefinition="Specific Questionnaire." ) 1037 protected Reference reference; 1038 1039 private static final long serialVersionUID = -1791379681L; 1040 1041 /** 1042 * Constructor 1043 */ 1044 public ConditionDefinitionQuestionnaireComponent() { 1045 super(); 1046 } 1047 1048 /** 1049 * Constructor 1050 */ 1051 public ConditionDefinitionQuestionnaireComponent(ConditionQuestionnairePurpose purpose, Reference reference) { 1052 super(); 1053 this.setPurpose(purpose); 1054 this.setReference(reference); 1055 } 1056 1057 /** 1058 * @return {@link #purpose} (Use of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1059 */ 1060 public Enumeration<ConditionQuestionnairePurpose> getPurposeElement() { 1061 if (this.purpose == null) 1062 if (Configuration.errorOnAutoCreate()) 1063 throw new Error("Attempt to auto-create ConditionDefinitionQuestionnaireComponent.purpose"); 1064 else if (Configuration.doAutoCreate()) 1065 this.purpose = new Enumeration<ConditionQuestionnairePurpose>(new ConditionQuestionnairePurposeEnumFactory()); // bb 1066 return this.purpose; 1067 } 1068 1069 public boolean hasPurposeElement() { 1070 return this.purpose != null && !this.purpose.isEmpty(); 1071 } 1072 1073 public boolean hasPurpose() { 1074 return this.purpose != null && !this.purpose.isEmpty(); 1075 } 1076 1077 /** 1078 * @param value {@link #purpose} (Use of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1079 */ 1080 public ConditionDefinitionQuestionnaireComponent setPurposeElement(Enumeration<ConditionQuestionnairePurpose> value) { 1081 this.purpose = value; 1082 return this; 1083 } 1084 1085 /** 1086 * @return Use of the questionnaire. 1087 */ 1088 public ConditionQuestionnairePurpose getPurpose() { 1089 return this.purpose == null ? null : this.purpose.getValue(); 1090 } 1091 1092 /** 1093 * @param value Use of the questionnaire. 1094 */ 1095 public ConditionDefinitionQuestionnaireComponent setPurpose(ConditionQuestionnairePurpose value) { 1096 if (this.purpose == null) 1097 this.purpose = new Enumeration<ConditionQuestionnairePurpose>(new ConditionQuestionnairePurposeEnumFactory()); 1098 this.purpose.setValue(value); 1099 return this; 1100 } 1101 1102 /** 1103 * @return {@link #reference} (Specific Questionnaire.) 1104 */ 1105 public Reference getReference() { 1106 if (this.reference == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create ConditionDefinitionQuestionnaireComponent.reference"); 1109 else if (Configuration.doAutoCreate()) 1110 this.reference = new Reference(); // cc 1111 return this.reference; 1112 } 1113 1114 public boolean hasReference() { 1115 return this.reference != null && !this.reference.isEmpty(); 1116 } 1117 1118 /** 1119 * @param value {@link #reference} (Specific Questionnaire.) 1120 */ 1121 public ConditionDefinitionQuestionnaireComponent setReference(Reference value) { 1122 this.reference = value; 1123 return this; 1124 } 1125 1126 protected void listChildren(List<Property> children) { 1127 super.listChildren(children); 1128 children.add(new Property("purpose", "code", "Use of the questionnaire.", 0, 1, purpose)); 1129 children.add(new Property("reference", "Reference(Questionnaire)", "Specific Questionnaire.", 0, 1, reference)); 1130 } 1131 1132 @Override 1133 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1134 switch (_hash) { 1135 case -220463842: /*purpose*/ return new Property("purpose", "code", "Use of the questionnaire.", 0, 1, purpose); 1136 case -925155509: /*reference*/ return new Property("reference", "Reference(Questionnaire)", "Specific Questionnaire.", 0, 1, reference); 1137 default: return super.getNamedProperty(_hash, _name, _checkValid); 1138 } 1139 1140 } 1141 1142 @Override 1143 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1144 switch (hash) { 1145 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // Enumeration<ConditionQuestionnairePurpose> 1146 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1147 default: return super.getProperty(hash, name, checkValid); 1148 } 1149 1150 } 1151 1152 @Override 1153 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1154 switch (hash) { 1155 case -220463842: // purpose 1156 value = new ConditionQuestionnairePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1157 this.purpose = (Enumeration) value; // Enumeration<ConditionQuestionnairePurpose> 1158 return value; 1159 case -925155509: // reference 1160 this.reference = TypeConvertor.castToReference(value); // Reference 1161 return value; 1162 default: return super.setProperty(hash, name, value); 1163 } 1164 1165 } 1166 1167 @Override 1168 public Base setProperty(String name, Base value) throws FHIRException { 1169 if (name.equals("purpose")) { 1170 value = new ConditionQuestionnairePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1171 this.purpose = (Enumeration) value; // Enumeration<ConditionQuestionnairePurpose> 1172 } else if (name.equals("reference")) { 1173 this.reference = TypeConvertor.castToReference(value); // Reference 1174 } else 1175 return super.setProperty(name, value); 1176 return value; 1177 } 1178 1179 @Override 1180 public void removeChild(String name, Base value) throws FHIRException { 1181 if (name.equals("purpose")) { 1182 value = new ConditionQuestionnairePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1183 this.purpose = (Enumeration) value; // Enumeration<ConditionQuestionnairePurpose> 1184 } else if (name.equals("reference")) { 1185 this.reference = null; 1186 } else 1187 super.removeChild(name, value); 1188 1189 } 1190 1191 @Override 1192 public Base makeProperty(int hash, String name) throws FHIRException { 1193 switch (hash) { 1194 case -220463842: return getPurposeElement(); 1195 case -925155509: return getReference(); 1196 default: return super.makeProperty(hash, name); 1197 } 1198 1199 } 1200 1201 @Override 1202 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1203 switch (hash) { 1204 case -220463842: /*purpose*/ return new String[] {"code"}; 1205 case -925155509: /*reference*/ return new String[] {"Reference"}; 1206 default: return super.getTypesForProperty(hash, name); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base addChild(String name) throws FHIRException { 1213 if (name.equals("purpose")) { 1214 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.questionnaire.purpose"); 1215 } 1216 else if (name.equals("reference")) { 1217 this.reference = new Reference(); 1218 return this.reference; 1219 } 1220 else 1221 return super.addChild(name); 1222 } 1223 1224 public ConditionDefinitionQuestionnaireComponent copy() { 1225 ConditionDefinitionQuestionnaireComponent dst = new ConditionDefinitionQuestionnaireComponent(); 1226 copyValues(dst); 1227 return dst; 1228 } 1229 1230 public void copyValues(ConditionDefinitionQuestionnaireComponent dst) { 1231 super.copyValues(dst); 1232 dst.purpose = purpose == null ? null : purpose.copy(); 1233 dst.reference = reference == null ? null : reference.copy(); 1234 } 1235 1236 @Override 1237 public boolean equalsDeep(Base other_) { 1238 if (!super.equalsDeep(other_)) 1239 return false; 1240 if (!(other_ instanceof ConditionDefinitionQuestionnaireComponent)) 1241 return false; 1242 ConditionDefinitionQuestionnaireComponent o = (ConditionDefinitionQuestionnaireComponent) other_; 1243 return compareDeep(purpose, o.purpose, true) && compareDeep(reference, o.reference, true); 1244 } 1245 1246 @Override 1247 public boolean equalsShallow(Base other_) { 1248 if (!super.equalsShallow(other_)) 1249 return false; 1250 if (!(other_ instanceof ConditionDefinitionQuestionnaireComponent)) 1251 return false; 1252 ConditionDefinitionQuestionnaireComponent o = (ConditionDefinitionQuestionnaireComponent) other_; 1253 return compareValues(purpose, o.purpose, true); 1254 } 1255 1256 public boolean isEmpty() { 1257 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, reference); 1258 } 1259 1260 public String fhirType() { 1261 return "ConditionDefinition.questionnaire"; 1262 1263 } 1264 1265 } 1266 1267 @Block() 1268 public static class ConditionDefinitionPlanComponent extends BackboneElement implements IBaseBackboneElement { 1269 /** 1270 * Use for the plan. 1271 */ 1272 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1273 @Description(shortDefinition="Use for the plan", formalDefinition="Use for the plan." ) 1274 protected CodeableConcept role; 1275 1276 /** 1277 * The actual plan. 1278 */ 1279 @Child(name = "reference", type = {PlanDefinition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1280 @Description(shortDefinition="The actual plan", formalDefinition="The actual plan." ) 1281 protected Reference reference; 1282 1283 private static final long serialVersionUID = -1992921787L; 1284 1285 /** 1286 * Constructor 1287 */ 1288 public ConditionDefinitionPlanComponent() { 1289 super(); 1290 } 1291 1292 /** 1293 * Constructor 1294 */ 1295 public ConditionDefinitionPlanComponent(Reference reference) { 1296 super(); 1297 this.setReference(reference); 1298 } 1299 1300 /** 1301 * @return {@link #role} (Use for the plan.) 1302 */ 1303 public CodeableConcept getRole() { 1304 if (this.role == null) 1305 if (Configuration.errorOnAutoCreate()) 1306 throw new Error("Attempt to auto-create ConditionDefinitionPlanComponent.role"); 1307 else if (Configuration.doAutoCreate()) 1308 this.role = new CodeableConcept(); // cc 1309 return this.role; 1310 } 1311 1312 public boolean hasRole() { 1313 return this.role != null && !this.role.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #role} (Use for the plan.) 1318 */ 1319 public ConditionDefinitionPlanComponent setRole(CodeableConcept value) { 1320 this.role = value; 1321 return this; 1322 } 1323 1324 /** 1325 * @return {@link #reference} (The actual plan.) 1326 */ 1327 public Reference getReference() { 1328 if (this.reference == null) 1329 if (Configuration.errorOnAutoCreate()) 1330 throw new Error("Attempt to auto-create ConditionDefinitionPlanComponent.reference"); 1331 else if (Configuration.doAutoCreate()) 1332 this.reference = new Reference(); // cc 1333 return this.reference; 1334 } 1335 1336 public boolean hasReference() { 1337 return this.reference != null && !this.reference.isEmpty(); 1338 } 1339 1340 /** 1341 * @param value {@link #reference} (The actual plan.) 1342 */ 1343 public ConditionDefinitionPlanComponent setReference(Reference value) { 1344 this.reference = value; 1345 return this; 1346 } 1347 1348 protected void listChildren(List<Property> children) { 1349 super.listChildren(children); 1350 children.add(new Property("role", "CodeableConcept", "Use for the plan.", 0, 1, role)); 1351 children.add(new Property("reference", "Reference(PlanDefinition)", "The actual plan.", 0, 1, reference)); 1352 } 1353 1354 @Override 1355 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1356 switch (_hash) { 1357 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Use for the plan.", 0, 1, role); 1358 case -925155509: /*reference*/ return new Property("reference", "Reference(PlanDefinition)", "The actual plan.", 0, 1, reference); 1359 default: return super.getNamedProperty(_hash, _name, _checkValid); 1360 } 1361 1362 } 1363 1364 @Override 1365 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1366 switch (hash) { 1367 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1368 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1369 default: return super.getProperty(hash, name, checkValid); 1370 } 1371 1372 } 1373 1374 @Override 1375 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1376 switch (hash) { 1377 case 3506294: // role 1378 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1379 return value; 1380 case -925155509: // reference 1381 this.reference = TypeConvertor.castToReference(value); // Reference 1382 return value; 1383 default: return super.setProperty(hash, name, value); 1384 } 1385 1386 } 1387 1388 @Override 1389 public Base setProperty(String name, Base value) throws FHIRException { 1390 if (name.equals("role")) { 1391 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1392 } else if (name.equals("reference")) { 1393 this.reference = TypeConvertor.castToReference(value); // Reference 1394 } else 1395 return super.setProperty(name, value); 1396 return value; 1397 } 1398 1399 @Override 1400 public void removeChild(String name, Base value) throws FHIRException { 1401 if (name.equals("role")) { 1402 this.role = null; 1403 } else if (name.equals("reference")) { 1404 this.reference = null; 1405 } else 1406 super.removeChild(name, value); 1407 1408 } 1409 1410 @Override 1411 public Base makeProperty(int hash, String name) throws FHIRException { 1412 switch (hash) { 1413 case 3506294: return getRole(); 1414 case -925155509: return getReference(); 1415 default: return super.makeProperty(hash, name); 1416 } 1417 1418 } 1419 1420 @Override 1421 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1422 switch (hash) { 1423 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1424 case -925155509: /*reference*/ return new String[] {"Reference"}; 1425 default: return super.getTypesForProperty(hash, name); 1426 } 1427 1428 } 1429 1430 @Override 1431 public Base addChild(String name) throws FHIRException { 1432 if (name.equals("role")) { 1433 this.role = new CodeableConcept(); 1434 return this.role; 1435 } 1436 else if (name.equals("reference")) { 1437 this.reference = new Reference(); 1438 return this.reference; 1439 } 1440 else 1441 return super.addChild(name); 1442 } 1443 1444 public ConditionDefinitionPlanComponent copy() { 1445 ConditionDefinitionPlanComponent dst = new ConditionDefinitionPlanComponent(); 1446 copyValues(dst); 1447 return dst; 1448 } 1449 1450 public void copyValues(ConditionDefinitionPlanComponent dst) { 1451 super.copyValues(dst); 1452 dst.role = role == null ? null : role.copy(); 1453 dst.reference = reference == null ? null : reference.copy(); 1454 } 1455 1456 @Override 1457 public boolean equalsDeep(Base other_) { 1458 if (!super.equalsDeep(other_)) 1459 return false; 1460 if (!(other_ instanceof ConditionDefinitionPlanComponent)) 1461 return false; 1462 ConditionDefinitionPlanComponent o = (ConditionDefinitionPlanComponent) other_; 1463 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 1464 } 1465 1466 @Override 1467 public boolean equalsShallow(Base other_) { 1468 if (!super.equalsShallow(other_)) 1469 return false; 1470 if (!(other_ instanceof ConditionDefinitionPlanComponent)) 1471 return false; 1472 ConditionDefinitionPlanComponent o = (ConditionDefinitionPlanComponent) other_; 1473 return true; 1474 } 1475 1476 public boolean isEmpty() { 1477 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 1478 } 1479 1480 public String fhirType() { 1481 return "ConditionDefinition.plan"; 1482 1483 } 1484 1485 } 1486 1487 /** 1488 * An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers. 1489 */ 1490 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1491 @Description(shortDefinition="Canonical identifier for this condition definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers." ) 1492 protected UriType url; 1493 1494 /** 1495 * A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 1496 */ 1497 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1498 @Description(shortDefinition="Additional identifier for the condition definition", formalDefinition="A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1499 protected List<Identifier> identifier; 1500 1501 /** 1502 * The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1503 */ 1504 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1505 @Description(shortDefinition="Business version of the condition definition", formalDefinition="The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 1506 protected StringType version; 1507 1508 /** 1509 * Indicates the mechanism used to compare versions to determine which is more current. 1510 */ 1511 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1512 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1513 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1514 protected DataType versionAlgorithm; 1515 1516 /** 1517 * A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1518 */ 1519 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1520 @Description(shortDefinition="Name for this condition definition (computer friendly)", formalDefinition="A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1521 protected StringType name; 1522 1523 /** 1524 * A short, descriptive, user-friendly title for the condition definition. 1525 */ 1526 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1527 @Description(shortDefinition="Name for this condition definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the condition definition." ) 1528 protected StringType title; 1529 1530 /** 1531 * An explanatory or alternate title for the event definition giving additional information about its content. 1532 */ 1533 @Child(name = "subtitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1534 @Description(shortDefinition="Subordinate title of the event definition", formalDefinition="An explanatory or alternate title for the event definition giving additional information about its content." ) 1535 protected StringType subtitle; 1536 1537 /** 1538 * The status of this condition definition. Enables tracking the life-cycle of the content. 1539 */ 1540 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 1541 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this condition definition. Enables tracking the life-cycle of the content." ) 1542 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1543 protected Enumeration<PublicationStatus> status; 1544 1545 /** 1546 * A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1547 */ 1548 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1549 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1550 protected BooleanType experimental; 1551 1552 /** 1553 * The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes. 1554 */ 1555 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1556 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes." ) 1557 protected DateTimeType date; 1558 1559 /** 1560 * The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition. 1561 */ 1562 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1563 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition." ) 1564 protected StringType publisher; 1565 1566 /** 1567 * Contact details to assist a user in finding and communicating with the publisher. 1568 */ 1569 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1570 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1571 protected List<ContactDetail> contact; 1572 1573 /** 1574 * A free text natural language description of the condition definition from a consumer's perspective. 1575 */ 1576 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1577 @Description(shortDefinition="Natural language description of the condition definition", formalDefinition="A free text natural language description of the condition definition from a consumer's perspective." ) 1578 protected MarkdownType description; 1579 1580 /** 1581 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances. 1582 */ 1583 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1584 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances." ) 1585 protected List<UsageContext> useContext; 1586 1587 /** 1588 * A legal or geographic region in which the condition definition is intended to be used. 1589 */ 1590 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1591 @Description(shortDefinition="Intended jurisdiction for condition definition (if applicable)", formalDefinition="A legal or geographic region in which the condition definition is intended to be used." ) 1592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1593 protected List<CodeableConcept> jurisdiction; 1594 1595 /** 1596 * Identification of the condition, problem or diagnosis. 1597 */ 1598 @Child(name = "code", type = {CodeableConcept.class}, order=15, min=1, max=1, modifier=false, summary=true) 1599 @Description(shortDefinition="Identification of the condition, problem or diagnosis", formalDefinition="Identification of the condition, problem or diagnosis." ) 1600 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 1601 protected CodeableConcept code; 1602 1603 /** 1604 * A subjective assessment of the severity of the condition as evaluated by the clinician. 1605 */ 1606 @Child(name = "severity", type = {CodeableConcept.class}, order=16, min=0, max=1, modifier=false, summary=true) 1607 @Description(shortDefinition="Subjective severity of condition", formalDefinition="A subjective assessment of the severity of the condition as evaluated by the clinician." ) 1608 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-severity") 1609 protected CodeableConcept severity; 1610 1611 /** 1612 * The anatomical location where this condition manifests itself. 1613 */ 1614 @Child(name = "bodySite", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=true) 1615 @Description(shortDefinition="Anatomical location, if relevant", formalDefinition="The anatomical location where this condition manifests itself." ) 1616 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1617 protected CodeableConcept bodySite; 1618 1619 /** 1620 * Clinical stage or grade of a condition. May include formal severity assessments. 1621 */ 1622 @Child(name = "stage", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=true) 1623 @Description(shortDefinition="Stage/grade, usually assessed formally", formalDefinition="Clinical stage or grade of a condition. May include formal severity assessments." ) 1624 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-stage") 1625 protected CodeableConcept stage; 1626 1627 /** 1628 * Whether Severity is appropriate to collect for this condition. 1629 */ 1630 @Child(name = "hasSeverity", type = {BooleanType.class}, order=19, min=0, max=1, modifier=false, summary=false) 1631 @Description(shortDefinition="Whether Severity is appropriate", formalDefinition="Whether Severity is appropriate to collect for this condition." ) 1632 protected BooleanType hasSeverity; 1633 1634 /** 1635 * Whether bodySite is appropriate to collect for this condition. 1636 */ 1637 @Child(name = "hasBodySite", type = {BooleanType.class}, order=20, min=0, max=1, modifier=false, summary=false) 1638 @Description(shortDefinition="Whether bodySite is appropriate", formalDefinition="Whether bodySite is appropriate to collect for this condition." ) 1639 protected BooleanType hasBodySite; 1640 1641 /** 1642 * Whether stage is appropriate to collect for this condition. 1643 */ 1644 @Child(name = "hasStage", type = {BooleanType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1645 @Description(shortDefinition="Whether stage is appropriate", formalDefinition="Whether stage is appropriate to collect for this condition." ) 1646 protected BooleanType hasStage; 1647 1648 /** 1649 * Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers. 1650 */ 1651 @Child(name = "definition", type = {UriType.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1652 @Description(shortDefinition="Formal Definition for the condition", formalDefinition="Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers." ) 1653 protected List<UriType> definition; 1654 1655 /** 1656 * Observations particularly relevant to this condition. 1657 */ 1658 @Child(name = "observation", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1659 @Description(shortDefinition="Observations particularly relevant to this condition", formalDefinition="Observations particularly relevant to this condition." ) 1660 protected List<ConditionDefinitionObservationComponent> observation; 1661 1662 /** 1663 * Medications particularly relevant for this condition. 1664 */ 1665 @Child(name = "medication", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1666 @Description(shortDefinition="Medications particularly relevant for this condition", formalDefinition="Medications particularly relevant for this condition." ) 1667 protected List<ConditionDefinitionMedicationComponent> medication; 1668 1669 /** 1670 * An observation that suggests that this condition applies. 1671 */ 1672 @Child(name = "precondition", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1673 @Description(shortDefinition="Observation that suggets this condition", formalDefinition="An observation that suggests that this condition applies." ) 1674 protected List<ConditionDefinitionPreconditionComponent> precondition; 1675 1676 /** 1677 * Appropriate team for this condition. 1678 */ 1679 @Child(name = "team", type = {CareTeam.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1680 @Description(shortDefinition="Appropriate team for this condition", formalDefinition="Appropriate team for this condition." ) 1681 protected List<Reference> team; 1682 1683 /** 1684 * Questionnaire for this condition. 1685 */ 1686 @Child(name = "questionnaire", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1687 @Description(shortDefinition="Questionnaire for this condition", formalDefinition="Questionnaire for this condition." ) 1688 protected List<ConditionDefinitionQuestionnaireComponent> questionnaire; 1689 1690 /** 1691 * Plan that is appropriate. 1692 */ 1693 @Child(name = "plan", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1694 @Description(shortDefinition="Plan that is appropriate", formalDefinition="Plan that is appropriate." ) 1695 protected List<ConditionDefinitionPlanComponent> plan; 1696 1697 private static final long serialVersionUID = 1055220331L; 1698 1699 /** 1700 * Constructor 1701 */ 1702 public ConditionDefinition() { 1703 super(); 1704 } 1705 1706 /** 1707 * Constructor 1708 */ 1709 public ConditionDefinition(PublicationStatus status, CodeableConcept code) { 1710 super(); 1711 this.setStatus(status); 1712 this.setCode(code); 1713 } 1714 1715 /** 1716 * @return {@link #url} (An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1717 */ 1718 public UriType getUrlElement() { 1719 if (this.url == null) 1720 if (Configuration.errorOnAutoCreate()) 1721 throw new Error("Attempt to auto-create ConditionDefinition.url"); 1722 else if (Configuration.doAutoCreate()) 1723 this.url = new UriType(); // bb 1724 return this.url; 1725 } 1726 1727 public boolean hasUrlElement() { 1728 return this.url != null && !this.url.isEmpty(); 1729 } 1730 1731 public boolean hasUrl() { 1732 return this.url != null && !this.url.isEmpty(); 1733 } 1734 1735 /** 1736 * @param value {@link #url} (An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1737 */ 1738 public ConditionDefinition setUrlElement(UriType value) { 1739 this.url = value; 1740 return this; 1741 } 1742 1743 /** 1744 * @return An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers. 1745 */ 1746 public String getUrl() { 1747 return this.url == null ? null : this.url.getValue(); 1748 } 1749 1750 /** 1751 * @param value An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers. 1752 */ 1753 public ConditionDefinition setUrl(String value) { 1754 if (Utilities.noString(value)) 1755 this.url = null; 1756 else { 1757 if (this.url == null) 1758 this.url = new UriType(); 1759 this.url.setValue(value); 1760 } 1761 return this; 1762 } 1763 1764 /** 1765 * @return {@link #identifier} (A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1766 */ 1767 public List<Identifier> getIdentifier() { 1768 if (this.identifier == null) 1769 this.identifier = new ArrayList<Identifier>(); 1770 return this.identifier; 1771 } 1772 1773 /** 1774 * @return Returns a reference to <code>this</code> for easy method chaining 1775 */ 1776 public ConditionDefinition setIdentifier(List<Identifier> theIdentifier) { 1777 this.identifier = theIdentifier; 1778 return this; 1779 } 1780 1781 public boolean hasIdentifier() { 1782 if (this.identifier == null) 1783 return false; 1784 for (Identifier item : this.identifier) 1785 if (!item.isEmpty()) 1786 return true; 1787 return false; 1788 } 1789 1790 public Identifier addIdentifier() { //3 1791 Identifier t = new Identifier(); 1792 if (this.identifier == null) 1793 this.identifier = new ArrayList<Identifier>(); 1794 this.identifier.add(t); 1795 return t; 1796 } 1797 1798 public ConditionDefinition addIdentifier(Identifier t) { //3 1799 if (t == null) 1800 return this; 1801 if (this.identifier == null) 1802 this.identifier = new ArrayList<Identifier>(); 1803 this.identifier.add(t); 1804 return this; 1805 } 1806 1807 /** 1808 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1809 */ 1810 public Identifier getIdentifierFirstRep() { 1811 if (getIdentifier().isEmpty()) { 1812 addIdentifier(); 1813 } 1814 return getIdentifier().get(0); 1815 } 1816 1817 /** 1818 * @return {@link #version} (The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1819 */ 1820 public StringType getVersionElement() { 1821 if (this.version == null) 1822 if (Configuration.errorOnAutoCreate()) 1823 throw new Error("Attempt to auto-create ConditionDefinition.version"); 1824 else if (Configuration.doAutoCreate()) 1825 this.version = new StringType(); // bb 1826 return this.version; 1827 } 1828 1829 public boolean hasVersionElement() { 1830 return this.version != null && !this.version.isEmpty(); 1831 } 1832 1833 public boolean hasVersion() { 1834 return this.version != null && !this.version.isEmpty(); 1835 } 1836 1837 /** 1838 * @param value {@link #version} (The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1839 */ 1840 public ConditionDefinition setVersionElement(StringType value) { 1841 this.version = value; 1842 return this; 1843 } 1844 1845 /** 1846 * @return The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1847 */ 1848 public String getVersion() { 1849 return this.version == null ? null : this.version.getValue(); 1850 } 1851 1852 /** 1853 * @param value The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1854 */ 1855 public ConditionDefinition setVersion(String value) { 1856 if (Utilities.noString(value)) 1857 this.version = null; 1858 else { 1859 if (this.version == null) 1860 this.version = new StringType(); 1861 this.version.setValue(value); 1862 } 1863 return this; 1864 } 1865 1866 /** 1867 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1868 */ 1869 public DataType getVersionAlgorithm() { 1870 return this.versionAlgorithm; 1871 } 1872 1873 /** 1874 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1875 */ 1876 public StringType getVersionAlgorithmStringType() throws FHIRException { 1877 if (this.versionAlgorithm == null) 1878 this.versionAlgorithm = new StringType(); 1879 if (!(this.versionAlgorithm instanceof StringType)) 1880 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1881 return (StringType) this.versionAlgorithm; 1882 } 1883 1884 public boolean hasVersionAlgorithmStringType() { 1885 return this != null && this.versionAlgorithm instanceof StringType; 1886 } 1887 1888 /** 1889 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1890 */ 1891 public Coding getVersionAlgorithmCoding() throws FHIRException { 1892 if (this.versionAlgorithm == null) 1893 this.versionAlgorithm = new Coding(); 1894 if (!(this.versionAlgorithm instanceof Coding)) 1895 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1896 return (Coding) this.versionAlgorithm; 1897 } 1898 1899 public boolean hasVersionAlgorithmCoding() { 1900 return this != null && this.versionAlgorithm instanceof Coding; 1901 } 1902 1903 public boolean hasVersionAlgorithm() { 1904 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1905 } 1906 1907 /** 1908 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1909 */ 1910 public ConditionDefinition setVersionAlgorithm(DataType value) { 1911 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1912 throw new FHIRException("Not the right type for ConditionDefinition.versionAlgorithm[x]: "+value.fhirType()); 1913 this.versionAlgorithm = value; 1914 return this; 1915 } 1916 1917 /** 1918 * @return {@link #name} (A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1919 */ 1920 public StringType getNameElement() { 1921 if (this.name == null) 1922 if (Configuration.errorOnAutoCreate()) 1923 throw new Error("Attempt to auto-create ConditionDefinition.name"); 1924 else if (Configuration.doAutoCreate()) 1925 this.name = new StringType(); // bb 1926 return this.name; 1927 } 1928 1929 public boolean hasNameElement() { 1930 return this.name != null && !this.name.isEmpty(); 1931 } 1932 1933 public boolean hasName() { 1934 return this.name != null && !this.name.isEmpty(); 1935 } 1936 1937 /** 1938 * @param value {@link #name} (A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1939 */ 1940 public ConditionDefinition setNameElement(StringType value) { 1941 this.name = value; 1942 return this; 1943 } 1944 1945 /** 1946 * @return A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1947 */ 1948 public String getName() { 1949 return this.name == null ? null : this.name.getValue(); 1950 } 1951 1952 /** 1953 * @param value A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1954 */ 1955 public ConditionDefinition setName(String value) { 1956 if (Utilities.noString(value)) 1957 this.name = null; 1958 else { 1959 if (this.name == null) 1960 this.name = new StringType(); 1961 this.name.setValue(value); 1962 } 1963 return this; 1964 } 1965 1966 /** 1967 * @return {@link #title} (A short, descriptive, user-friendly title for the condition definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1968 */ 1969 public StringType getTitleElement() { 1970 if (this.title == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create ConditionDefinition.title"); 1973 else if (Configuration.doAutoCreate()) 1974 this.title = new StringType(); // bb 1975 return this.title; 1976 } 1977 1978 public boolean hasTitleElement() { 1979 return this.title != null && !this.title.isEmpty(); 1980 } 1981 1982 public boolean hasTitle() { 1983 return this.title != null && !this.title.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #title} (A short, descriptive, user-friendly title for the condition definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1988 */ 1989 public ConditionDefinition setTitleElement(StringType value) { 1990 this.title = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return A short, descriptive, user-friendly title for the condition definition. 1996 */ 1997 public String getTitle() { 1998 return this.title == null ? null : this.title.getValue(); 1999 } 2000 2001 /** 2002 * @param value A short, descriptive, user-friendly title for the condition definition. 2003 */ 2004 public ConditionDefinition setTitle(String value) { 2005 if (Utilities.noString(value)) 2006 this.title = null; 2007 else { 2008 if (this.title == null) 2009 this.title = new StringType(); 2010 this.title.setValue(value); 2011 } 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #subtitle} (An explanatory or alternate title for the event definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 2017 */ 2018 public StringType getSubtitleElement() { 2019 if (this.subtitle == null) 2020 if (Configuration.errorOnAutoCreate()) 2021 throw new Error("Attempt to auto-create ConditionDefinition.subtitle"); 2022 else if (Configuration.doAutoCreate()) 2023 this.subtitle = new StringType(); // bb 2024 return this.subtitle; 2025 } 2026 2027 public boolean hasSubtitleElement() { 2028 return this.subtitle != null && !this.subtitle.isEmpty(); 2029 } 2030 2031 public boolean hasSubtitle() { 2032 return this.subtitle != null && !this.subtitle.isEmpty(); 2033 } 2034 2035 /** 2036 * @param value {@link #subtitle} (An explanatory or alternate title for the event definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 2037 */ 2038 public ConditionDefinition setSubtitleElement(StringType value) { 2039 this.subtitle = value; 2040 return this; 2041 } 2042 2043 /** 2044 * @return An explanatory or alternate title for the event definition giving additional information about its content. 2045 */ 2046 public String getSubtitle() { 2047 return this.subtitle == null ? null : this.subtitle.getValue(); 2048 } 2049 2050 /** 2051 * @param value An explanatory or alternate title for the event definition giving additional information about its content. 2052 */ 2053 public ConditionDefinition setSubtitle(String value) { 2054 if (Utilities.noString(value)) 2055 this.subtitle = null; 2056 else { 2057 if (this.subtitle == null) 2058 this.subtitle = new StringType(); 2059 this.subtitle.setValue(value); 2060 } 2061 return this; 2062 } 2063 2064 /** 2065 * @return {@link #status} (The status of this condition definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2066 */ 2067 public Enumeration<PublicationStatus> getStatusElement() { 2068 if (this.status == null) 2069 if (Configuration.errorOnAutoCreate()) 2070 throw new Error("Attempt to auto-create ConditionDefinition.status"); 2071 else if (Configuration.doAutoCreate()) 2072 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2073 return this.status; 2074 } 2075 2076 public boolean hasStatusElement() { 2077 return this.status != null && !this.status.isEmpty(); 2078 } 2079 2080 public boolean hasStatus() { 2081 return this.status != null && !this.status.isEmpty(); 2082 } 2083 2084 /** 2085 * @param value {@link #status} (The status of this condition definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2086 */ 2087 public ConditionDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2088 this.status = value; 2089 return this; 2090 } 2091 2092 /** 2093 * @return The status of this condition definition. Enables tracking the life-cycle of the content. 2094 */ 2095 public PublicationStatus getStatus() { 2096 return this.status == null ? null : this.status.getValue(); 2097 } 2098 2099 /** 2100 * @param value The status of this condition definition. Enables tracking the life-cycle of the content. 2101 */ 2102 public ConditionDefinition setStatus(PublicationStatus value) { 2103 if (this.status == null) 2104 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2105 this.status.setValue(value); 2106 return this; 2107 } 2108 2109 /** 2110 * @return {@link #experimental} (A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2111 */ 2112 public BooleanType getExperimentalElement() { 2113 if (this.experimental == null) 2114 if (Configuration.errorOnAutoCreate()) 2115 throw new Error("Attempt to auto-create ConditionDefinition.experimental"); 2116 else if (Configuration.doAutoCreate()) 2117 this.experimental = new BooleanType(); // bb 2118 return this.experimental; 2119 } 2120 2121 public boolean hasExperimentalElement() { 2122 return this.experimental != null && !this.experimental.isEmpty(); 2123 } 2124 2125 public boolean hasExperimental() { 2126 return this.experimental != null && !this.experimental.isEmpty(); 2127 } 2128 2129 /** 2130 * @param value {@link #experimental} (A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2131 */ 2132 public ConditionDefinition setExperimentalElement(BooleanType value) { 2133 this.experimental = value; 2134 return this; 2135 } 2136 2137 /** 2138 * @return A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2139 */ 2140 public boolean getExperimental() { 2141 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2142 } 2143 2144 /** 2145 * @param value A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2146 */ 2147 public ConditionDefinition setExperimental(boolean value) { 2148 if (this.experimental == null) 2149 this.experimental = new BooleanType(); 2150 this.experimental.setValue(value); 2151 return this; 2152 } 2153 2154 /** 2155 * @return {@link #date} (The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2156 */ 2157 public DateTimeType getDateElement() { 2158 if (this.date == null) 2159 if (Configuration.errorOnAutoCreate()) 2160 throw new Error("Attempt to auto-create ConditionDefinition.date"); 2161 else if (Configuration.doAutoCreate()) 2162 this.date = new DateTimeType(); // bb 2163 return this.date; 2164 } 2165 2166 public boolean hasDateElement() { 2167 return this.date != null && !this.date.isEmpty(); 2168 } 2169 2170 public boolean hasDate() { 2171 return this.date != null && !this.date.isEmpty(); 2172 } 2173 2174 /** 2175 * @param value {@link #date} (The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2176 */ 2177 public ConditionDefinition setDateElement(DateTimeType value) { 2178 this.date = value; 2179 return this; 2180 } 2181 2182 /** 2183 * @return The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes. 2184 */ 2185 public Date getDate() { 2186 return this.date == null ? null : this.date.getValue(); 2187 } 2188 2189 /** 2190 * @param value The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes. 2191 */ 2192 public ConditionDefinition setDate(Date value) { 2193 if (value == null) 2194 this.date = null; 2195 else { 2196 if (this.date == null) 2197 this.date = new DateTimeType(); 2198 this.date.setValue(value); 2199 } 2200 return this; 2201 } 2202 2203 /** 2204 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2205 */ 2206 public StringType getPublisherElement() { 2207 if (this.publisher == null) 2208 if (Configuration.errorOnAutoCreate()) 2209 throw new Error("Attempt to auto-create ConditionDefinition.publisher"); 2210 else if (Configuration.doAutoCreate()) 2211 this.publisher = new StringType(); // bb 2212 return this.publisher; 2213 } 2214 2215 public boolean hasPublisherElement() { 2216 return this.publisher != null && !this.publisher.isEmpty(); 2217 } 2218 2219 public boolean hasPublisher() { 2220 return this.publisher != null && !this.publisher.isEmpty(); 2221 } 2222 2223 /** 2224 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2225 */ 2226 public ConditionDefinition setPublisherElement(StringType value) { 2227 this.publisher = value; 2228 return this; 2229 } 2230 2231 /** 2232 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition. 2233 */ 2234 public String getPublisher() { 2235 return this.publisher == null ? null : this.publisher.getValue(); 2236 } 2237 2238 /** 2239 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition. 2240 */ 2241 public ConditionDefinition setPublisher(String value) { 2242 if (Utilities.noString(value)) 2243 this.publisher = null; 2244 else { 2245 if (this.publisher == null) 2246 this.publisher = new StringType(); 2247 this.publisher.setValue(value); 2248 } 2249 return this; 2250 } 2251 2252 /** 2253 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2254 */ 2255 public List<ContactDetail> getContact() { 2256 if (this.contact == null) 2257 this.contact = new ArrayList<ContactDetail>(); 2258 return this.contact; 2259 } 2260 2261 /** 2262 * @return Returns a reference to <code>this</code> for easy method chaining 2263 */ 2264 public ConditionDefinition setContact(List<ContactDetail> theContact) { 2265 this.contact = theContact; 2266 return this; 2267 } 2268 2269 public boolean hasContact() { 2270 if (this.contact == null) 2271 return false; 2272 for (ContactDetail item : this.contact) 2273 if (!item.isEmpty()) 2274 return true; 2275 return false; 2276 } 2277 2278 public ContactDetail addContact() { //3 2279 ContactDetail t = new ContactDetail(); 2280 if (this.contact == null) 2281 this.contact = new ArrayList<ContactDetail>(); 2282 this.contact.add(t); 2283 return t; 2284 } 2285 2286 public ConditionDefinition addContact(ContactDetail t) { //3 2287 if (t == null) 2288 return this; 2289 if (this.contact == null) 2290 this.contact = new ArrayList<ContactDetail>(); 2291 this.contact.add(t); 2292 return this; 2293 } 2294 2295 /** 2296 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2297 */ 2298 public ContactDetail getContactFirstRep() { 2299 if (getContact().isEmpty()) { 2300 addContact(); 2301 } 2302 return getContact().get(0); 2303 } 2304 2305 /** 2306 * @return {@link #description} (A free text natural language description of the condition definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2307 */ 2308 public MarkdownType getDescriptionElement() { 2309 if (this.description == null) 2310 if (Configuration.errorOnAutoCreate()) 2311 throw new Error("Attempt to auto-create ConditionDefinition.description"); 2312 else if (Configuration.doAutoCreate()) 2313 this.description = new MarkdownType(); // bb 2314 return this.description; 2315 } 2316 2317 public boolean hasDescriptionElement() { 2318 return this.description != null && !this.description.isEmpty(); 2319 } 2320 2321 public boolean hasDescription() { 2322 return this.description != null && !this.description.isEmpty(); 2323 } 2324 2325 /** 2326 * @param value {@link #description} (A free text natural language description of the condition definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2327 */ 2328 public ConditionDefinition setDescriptionElement(MarkdownType value) { 2329 this.description = value; 2330 return this; 2331 } 2332 2333 /** 2334 * @return A free text natural language description of the condition definition from a consumer's perspective. 2335 */ 2336 public String getDescription() { 2337 return this.description == null ? null : this.description.getValue(); 2338 } 2339 2340 /** 2341 * @param value A free text natural language description of the condition definition from a consumer's perspective. 2342 */ 2343 public ConditionDefinition setDescription(String value) { 2344 if (Utilities.noString(value)) 2345 this.description = null; 2346 else { 2347 if (this.description == null) 2348 this.description = new MarkdownType(); 2349 this.description.setValue(value); 2350 } 2351 return this; 2352 } 2353 2354 /** 2355 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances.) 2356 */ 2357 public List<UsageContext> getUseContext() { 2358 if (this.useContext == null) 2359 this.useContext = new ArrayList<UsageContext>(); 2360 return this.useContext; 2361 } 2362 2363 /** 2364 * @return Returns a reference to <code>this</code> for easy method chaining 2365 */ 2366 public ConditionDefinition setUseContext(List<UsageContext> theUseContext) { 2367 this.useContext = theUseContext; 2368 return this; 2369 } 2370 2371 public boolean hasUseContext() { 2372 if (this.useContext == null) 2373 return false; 2374 for (UsageContext item : this.useContext) 2375 if (!item.isEmpty()) 2376 return true; 2377 return false; 2378 } 2379 2380 public UsageContext addUseContext() { //3 2381 UsageContext t = new UsageContext(); 2382 if (this.useContext == null) 2383 this.useContext = new ArrayList<UsageContext>(); 2384 this.useContext.add(t); 2385 return t; 2386 } 2387 2388 public ConditionDefinition addUseContext(UsageContext t) { //3 2389 if (t == null) 2390 return this; 2391 if (this.useContext == null) 2392 this.useContext = new ArrayList<UsageContext>(); 2393 this.useContext.add(t); 2394 return this; 2395 } 2396 2397 /** 2398 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2399 */ 2400 public UsageContext getUseContextFirstRep() { 2401 if (getUseContext().isEmpty()) { 2402 addUseContext(); 2403 } 2404 return getUseContext().get(0); 2405 } 2406 2407 /** 2408 * @return {@link #jurisdiction} (A legal or geographic region in which the condition definition is intended to be used.) 2409 */ 2410 public List<CodeableConcept> getJurisdiction() { 2411 if (this.jurisdiction == null) 2412 this.jurisdiction = new ArrayList<CodeableConcept>(); 2413 return this.jurisdiction; 2414 } 2415 2416 /** 2417 * @return Returns a reference to <code>this</code> for easy method chaining 2418 */ 2419 public ConditionDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2420 this.jurisdiction = theJurisdiction; 2421 return this; 2422 } 2423 2424 public boolean hasJurisdiction() { 2425 if (this.jurisdiction == null) 2426 return false; 2427 for (CodeableConcept item : this.jurisdiction) 2428 if (!item.isEmpty()) 2429 return true; 2430 return false; 2431 } 2432 2433 public CodeableConcept addJurisdiction() { //3 2434 CodeableConcept t = new CodeableConcept(); 2435 if (this.jurisdiction == null) 2436 this.jurisdiction = new ArrayList<CodeableConcept>(); 2437 this.jurisdiction.add(t); 2438 return t; 2439 } 2440 2441 public ConditionDefinition addJurisdiction(CodeableConcept t) { //3 2442 if (t == null) 2443 return this; 2444 if (this.jurisdiction == null) 2445 this.jurisdiction = new ArrayList<CodeableConcept>(); 2446 this.jurisdiction.add(t); 2447 return this; 2448 } 2449 2450 /** 2451 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2452 */ 2453 public CodeableConcept getJurisdictionFirstRep() { 2454 if (getJurisdiction().isEmpty()) { 2455 addJurisdiction(); 2456 } 2457 return getJurisdiction().get(0); 2458 } 2459 2460 /** 2461 * @return {@link #code} (Identification of the condition, problem or diagnosis.) 2462 */ 2463 public CodeableConcept getCode() { 2464 if (this.code == null) 2465 if (Configuration.errorOnAutoCreate()) 2466 throw new Error("Attempt to auto-create ConditionDefinition.code"); 2467 else if (Configuration.doAutoCreate()) 2468 this.code = new CodeableConcept(); // cc 2469 return this.code; 2470 } 2471 2472 public boolean hasCode() { 2473 return this.code != null && !this.code.isEmpty(); 2474 } 2475 2476 /** 2477 * @param value {@link #code} (Identification of the condition, problem or diagnosis.) 2478 */ 2479 public ConditionDefinition setCode(CodeableConcept value) { 2480 this.code = value; 2481 return this; 2482 } 2483 2484 /** 2485 * @return {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 2486 */ 2487 public CodeableConcept getSeverity() { 2488 if (this.severity == null) 2489 if (Configuration.errorOnAutoCreate()) 2490 throw new Error("Attempt to auto-create ConditionDefinition.severity"); 2491 else if (Configuration.doAutoCreate()) 2492 this.severity = new CodeableConcept(); // cc 2493 return this.severity; 2494 } 2495 2496 public boolean hasSeverity() { 2497 return this.severity != null && !this.severity.isEmpty(); 2498 } 2499 2500 /** 2501 * @param value {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 2502 */ 2503 public ConditionDefinition setSeverity(CodeableConcept value) { 2504 this.severity = value; 2505 return this; 2506 } 2507 2508 /** 2509 * @return {@link #bodySite} (The anatomical location where this condition manifests itself.) 2510 */ 2511 public CodeableConcept getBodySite() { 2512 if (this.bodySite == null) 2513 if (Configuration.errorOnAutoCreate()) 2514 throw new Error("Attempt to auto-create ConditionDefinition.bodySite"); 2515 else if (Configuration.doAutoCreate()) 2516 this.bodySite = new CodeableConcept(); // cc 2517 return this.bodySite; 2518 } 2519 2520 public boolean hasBodySite() { 2521 return this.bodySite != null && !this.bodySite.isEmpty(); 2522 } 2523 2524 /** 2525 * @param value {@link #bodySite} (The anatomical location where this condition manifests itself.) 2526 */ 2527 public ConditionDefinition setBodySite(CodeableConcept value) { 2528 this.bodySite = value; 2529 return this; 2530 } 2531 2532 /** 2533 * @return {@link #stage} (Clinical stage or grade of a condition. May include formal severity assessments.) 2534 */ 2535 public CodeableConcept getStage() { 2536 if (this.stage == null) 2537 if (Configuration.errorOnAutoCreate()) 2538 throw new Error("Attempt to auto-create ConditionDefinition.stage"); 2539 else if (Configuration.doAutoCreate()) 2540 this.stage = new CodeableConcept(); // cc 2541 return this.stage; 2542 } 2543 2544 public boolean hasStage() { 2545 return this.stage != null && !this.stage.isEmpty(); 2546 } 2547 2548 /** 2549 * @param value {@link #stage} (Clinical stage or grade of a condition. May include formal severity assessments.) 2550 */ 2551 public ConditionDefinition setStage(CodeableConcept value) { 2552 this.stage = value; 2553 return this; 2554 } 2555 2556 /** 2557 * @return {@link #hasSeverity} (Whether Severity is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasSeverity" gives direct access to the value 2558 */ 2559 public BooleanType getHasSeverityElement() { 2560 if (this.hasSeverity == null) 2561 if (Configuration.errorOnAutoCreate()) 2562 throw new Error("Attempt to auto-create ConditionDefinition.hasSeverity"); 2563 else if (Configuration.doAutoCreate()) 2564 this.hasSeverity = new BooleanType(); // bb 2565 return this.hasSeverity; 2566 } 2567 2568 public boolean hasHasSeverityElement() { 2569 return this.hasSeverity != null && !this.hasSeverity.isEmpty(); 2570 } 2571 2572 public boolean hasHasSeverity() { 2573 return this.hasSeverity != null && !this.hasSeverity.isEmpty(); 2574 } 2575 2576 /** 2577 * @param value {@link #hasSeverity} (Whether Severity is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasSeverity" gives direct access to the value 2578 */ 2579 public ConditionDefinition setHasSeverityElement(BooleanType value) { 2580 this.hasSeverity = value; 2581 return this; 2582 } 2583 2584 /** 2585 * @return Whether Severity is appropriate to collect for this condition. 2586 */ 2587 public boolean getHasSeverity() { 2588 return this.hasSeverity == null || this.hasSeverity.isEmpty() ? false : this.hasSeverity.getValue(); 2589 } 2590 2591 /** 2592 * @param value Whether Severity is appropriate to collect for this condition. 2593 */ 2594 public ConditionDefinition setHasSeverity(boolean value) { 2595 if (this.hasSeverity == null) 2596 this.hasSeverity = new BooleanType(); 2597 this.hasSeverity.setValue(value); 2598 return this; 2599 } 2600 2601 /** 2602 * @return {@link #hasBodySite} (Whether bodySite is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasBodySite" gives direct access to the value 2603 */ 2604 public BooleanType getHasBodySiteElement() { 2605 if (this.hasBodySite == null) 2606 if (Configuration.errorOnAutoCreate()) 2607 throw new Error("Attempt to auto-create ConditionDefinition.hasBodySite"); 2608 else if (Configuration.doAutoCreate()) 2609 this.hasBodySite = new BooleanType(); // bb 2610 return this.hasBodySite; 2611 } 2612 2613 public boolean hasHasBodySiteElement() { 2614 return this.hasBodySite != null && !this.hasBodySite.isEmpty(); 2615 } 2616 2617 public boolean hasHasBodySite() { 2618 return this.hasBodySite != null && !this.hasBodySite.isEmpty(); 2619 } 2620 2621 /** 2622 * @param value {@link #hasBodySite} (Whether bodySite is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasBodySite" gives direct access to the value 2623 */ 2624 public ConditionDefinition setHasBodySiteElement(BooleanType value) { 2625 this.hasBodySite = value; 2626 return this; 2627 } 2628 2629 /** 2630 * @return Whether bodySite is appropriate to collect for this condition. 2631 */ 2632 public boolean getHasBodySite() { 2633 return this.hasBodySite == null || this.hasBodySite.isEmpty() ? false : this.hasBodySite.getValue(); 2634 } 2635 2636 /** 2637 * @param value Whether bodySite is appropriate to collect for this condition. 2638 */ 2639 public ConditionDefinition setHasBodySite(boolean value) { 2640 if (this.hasBodySite == null) 2641 this.hasBodySite = new BooleanType(); 2642 this.hasBodySite.setValue(value); 2643 return this; 2644 } 2645 2646 /** 2647 * @return {@link #hasStage} (Whether stage is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasStage" gives direct access to the value 2648 */ 2649 public BooleanType getHasStageElement() { 2650 if (this.hasStage == null) 2651 if (Configuration.errorOnAutoCreate()) 2652 throw new Error("Attempt to auto-create ConditionDefinition.hasStage"); 2653 else if (Configuration.doAutoCreate()) 2654 this.hasStage = new BooleanType(); // bb 2655 return this.hasStage; 2656 } 2657 2658 public boolean hasHasStageElement() { 2659 return this.hasStage != null && !this.hasStage.isEmpty(); 2660 } 2661 2662 public boolean hasHasStage() { 2663 return this.hasStage != null && !this.hasStage.isEmpty(); 2664 } 2665 2666 /** 2667 * @param value {@link #hasStage} (Whether stage is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasStage" gives direct access to the value 2668 */ 2669 public ConditionDefinition setHasStageElement(BooleanType value) { 2670 this.hasStage = value; 2671 return this; 2672 } 2673 2674 /** 2675 * @return Whether stage is appropriate to collect for this condition. 2676 */ 2677 public boolean getHasStage() { 2678 return this.hasStage == null || this.hasStage.isEmpty() ? false : this.hasStage.getValue(); 2679 } 2680 2681 /** 2682 * @param value Whether stage is appropriate to collect for this condition. 2683 */ 2684 public ConditionDefinition setHasStage(boolean value) { 2685 if (this.hasStage == null) 2686 this.hasStage = new BooleanType(); 2687 this.hasStage.setValue(value); 2688 return this; 2689 } 2690 2691 /** 2692 * @return {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2693 */ 2694 public List<UriType> getDefinition() { 2695 if (this.definition == null) 2696 this.definition = new ArrayList<UriType>(); 2697 return this.definition; 2698 } 2699 2700 /** 2701 * @return Returns a reference to <code>this</code> for easy method chaining 2702 */ 2703 public ConditionDefinition setDefinition(List<UriType> theDefinition) { 2704 this.definition = theDefinition; 2705 return this; 2706 } 2707 2708 public boolean hasDefinition() { 2709 if (this.definition == null) 2710 return false; 2711 for (UriType item : this.definition) 2712 if (!item.isEmpty()) 2713 return true; 2714 return false; 2715 } 2716 2717 /** 2718 * @return {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2719 */ 2720 public UriType addDefinitionElement() {//2 2721 UriType t = new UriType(); 2722 if (this.definition == null) 2723 this.definition = new ArrayList<UriType>(); 2724 this.definition.add(t); 2725 return t; 2726 } 2727 2728 /** 2729 * @param value {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2730 */ 2731 public ConditionDefinition addDefinition(String value) { //1 2732 UriType t = new UriType(); 2733 t.setValue(value); 2734 if (this.definition == null) 2735 this.definition = new ArrayList<UriType>(); 2736 this.definition.add(t); 2737 return this; 2738 } 2739 2740 /** 2741 * @param value {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2742 */ 2743 public boolean hasDefinition(String value) { 2744 if (this.definition == null) 2745 return false; 2746 for (UriType v : this.definition) 2747 if (v.getValue().equals(value)) // uri 2748 return true; 2749 return false; 2750 } 2751 2752 /** 2753 * @return {@link #observation} (Observations particularly relevant to this condition.) 2754 */ 2755 public List<ConditionDefinitionObservationComponent> getObservation() { 2756 if (this.observation == null) 2757 this.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 2758 return this.observation; 2759 } 2760 2761 /** 2762 * @return Returns a reference to <code>this</code> for easy method chaining 2763 */ 2764 public ConditionDefinition setObservation(List<ConditionDefinitionObservationComponent> theObservation) { 2765 this.observation = theObservation; 2766 return this; 2767 } 2768 2769 public boolean hasObservation() { 2770 if (this.observation == null) 2771 return false; 2772 for (ConditionDefinitionObservationComponent item : this.observation) 2773 if (!item.isEmpty()) 2774 return true; 2775 return false; 2776 } 2777 2778 public ConditionDefinitionObservationComponent addObservation() { //3 2779 ConditionDefinitionObservationComponent t = new ConditionDefinitionObservationComponent(); 2780 if (this.observation == null) 2781 this.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 2782 this.observation.add(t); 2783 return t; 2784 } 2785 2786 public ConditionDefinition addObservation(ConditionDefinitionObservationComponent t) { //3 2787 if (t == null) 2788 return this; 2789 if (this.observation == null) 2790 this.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 2791 this.observation.add(t); 2792 return this; 2793 } 2794 2795 /** 2796 * @return The first repetition of repeating field {@link #observation}, creating it if it does not already exist {3} 2797 */ 2798 public ConditionDefinitionObservationComponent getObservationFirstRep() { 2799 if (getObservation().isEmpty()) { 2800 addObservation(); 2801 } 2802 return getObservation().get(0); 2803 } 2804 2805 /** 2806 * @return {@link #medication} (Medications particularly relevant for this condition.) 2807 */ 2808 public List<ConditionDefinitionMedicationComponent> getMedication() { 2809 if (this.medication == null) 2810 this.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 2811 return this.medication; 2812 } 2813 2814 /** 2815 * @return Returns a reference to <code>this</code> for easy method chaining 2816 */ 2817 public ConditionDefinition setMedication(List<ConditionDefinitionMedicationComponent> theMedication) { 2818 this.medication = theMedication; 2819 return this; 2820 } 2821 2822 public boolean hasMedication() { 2823 if (this.medication == null) 2824 return false; 2825 for (ConditionDefinitionMedicationComponent item : this.medication) 2826 if (!item.isEmpty()) 2827 return true; 2828 return false; 2829 } 2830 2831 public ConditionDefinitionMedicationComponent addMedication() { //3 2832 ConditionDefinitionMedicationComponent t = new ConditionDefinitionMedicationComponent(); 2833 if (this.medication == null) 2834 this.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 2835 this.medication.add(t); 2836 return t; 2837 } 2838 2839 public ConditionDefinition addMedication(ConditionDefinitionMedicationComponent t) { //3 2840 if (t == null) 2841 return this; 2842 if (this.medication == null) 2843 this.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 2844 this.medication.add(t); 2845 return this; 2846 } 2847 2848 /** 2849 * @return The first repetition of repeating field {@link #medication}, creating it if it does not already exist {3} 2850 */ 2851 public ConditionDefinitionMedicationComponent getMedicationFirstRep() { 2852 if (getMedication().isEmpty()) { 2853 addMedication(); 2854 } 2855 return getMedication().get(0); 2856 } 2857 2858 /** 2859 * @return {@link #precondition} (An observation that suggests that this condition applies.) 2860 */ 2861 public List<ConditionDefinitionPreconditionComponent> getPrecondition() { 2862 if (this.precondition == null) 2863 this.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 2864 return this.precondition; 2865 } 2866 2867 /** 2868 * @return Returns a reference to <code>this</code> for easy method chaining 2869 */ 2870 public ConditionDefinition setPrecondition(List<ConditionDefinitionPreconditionComponent> thePrecondition) { 2871 this.precondition = thePrecondition; 2872 return this; 2873 } 2874 2875 public boolean hasPrecondition() { 2876 if (this.precondition == null) 2877 return false; 2878 for (ConditionDefinitionPreconditionComponent item : this.precondition) 2879 if (!item.isEmpty()) 2880 return true; 2881 return false; 2882 } 2883 2884 public ConditionDefinitionPreconditionComponent addPrecondition() { //3 2885 ConditionDefinitionPreconditionComponent t = new ConditionDefinitionPreconditionComponent(); 2886 if (this.precondition == null) 2887 this.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 2888 this.precondition.add(t); 2889 return t; 2890 } 2891 2892 public ConditionDefinition addPrecondition(ConditionDefinitionPreconditionComponent t) { //3 2893 if (t == null) 2894 return this; 2895 if (this.precondition == null) 2896 this.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 2897 this.precondition.add(t); 2898 return this; 2899 } 2900 2901 /** 2902 * @return The first repetition of repeating field {@link #precondition}, creating it if it does not already exist {3} 2903 */ 2904 public ConditionDefinitionPreconditionComponent getPreconditionFirstRep() { 2905 if (getPrecondition().isEmpty()) { 2906 addPrecondition(); 2907 } 2908 return getPrecondition().get(0); 2909 } 2910 2911 /** 2912 * @return {@link #team} (Appropriate team for this condition.) 2913 */ 2914 public List<Reference> getTeam() { 2915 if (this.team == null) 2916 this.team = new ArrayList<Reference>(); 2917 return this.team; 2918 } 2919 2920 /** 2921 * @return Returns a reference to <code>this</code> for easy method chaining 2922 */ 2923 public ConditionDefinition setTeam(List<Reference> theTeam) { 2924 this.team = theTeam; 2925 return this; 2926 } 2927 2928 public boolean hasTeam() { 2929 if (this.team == null) 2930 return false; 2931 for (Reference item : this.team) 2932 if (!item.isEmpty()) 2933 return true; 2934 return false; 2935 } 2936 2937 public Reference addTeam() { //3 2938 Reference t = new Reference(); 2939 if (this.team == null) 2940 this.team = new ArrayList<Reference>(); 2941 this.team.add(t); 2942 return t; 2943 } 2944 2945 public ConditionDefinition addTeam(Reference t) { //3 2946 if (t == null) 2947 return this; 2948 if (this.team == null) 2949 this.team = new ArrayList<Reference>(); 2950 this.team.add(t); 2951 return this; 2952 } 2953 2954 /** 2955 * @return The first repetition of repeating field {@link #team}, creating it if it does not already exist {3} 2956 */ 2957 public Reference getTeamFirstRep() { 2958 if (getTeam().isEmpty()) { 2959 addTeam(); 2960 } 2961 return getTeam().get(0); 2962 } 2963 2964 /** 2965 * @return {@link #questionnaire} (Questionnaire for this condition.) 2966 */ 2967 public List<ConditionDefinitionQuestionnaireComponent> getQuestionnaire() { 2968 if (this.questionnaire == null) 2969 this.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 2970 return this.questionnaire; 2971 } 2972 2973 /** 2974 * @return Returns a reference to <code>this</code> for easy method chaining 2975 */ 2976 public ConditionDefinition setQuestionnaire(List<ConditionDefinitionQuestionnaireComponent> theQuestionnaire) { 2977 this.questionnaire = theQuestionnaire; 2978 return this; 2979 } 2980 2981 public boolean hasQuestionnaire() { 2982 if (this.questionnaire == null) 2983 return false; 2984 for (ConditionDefinitionQuestionnaireComponent item : this.questionnaire) 2985 if (!item.isEmpty()) 2986 return true; 2987 return false; 2988 } 2989 2990 public ConditionDefinitionQuestionnaireComponent addQuestionnaire() { //3 2991 ConditionDefinitionQuestionnaireComponent t = new ConditionDefinitionQuestionnaireComponent(); 2992 if (this.questionnaire == null) 2993 this.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 2994 this.questionnaire.add(t); 2995 return t; 2996 } 2997 2998 public ConditionDefinition addQuestionnaire(ConditionDefinitionQuestionnaireComponent t) { //3 2999 if (t == null) 3000 return this; 3001 if (this.questionnaire == null) 3002 this.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 3003 this.questionnaire.add(t); 3004 return this; 3005 } 3006 3007 /** 3008 * @return The first repetition of repeating field {@link #questionnaire}, creating it if it does not already exist {3} 3009 */ 3010 public ConditionDefinitionQuestionnaireComponent getQuestionnaireFirstRep() { 3011 if (getQuestionnaire().isEmpty()) { 3012 addQuestionnaire(); 3013 } 3014 return getQuestionnaire().get(0); 3015 } 3016 3017 /** 3018 * @return {@link #plan} (Plan that is appropriate.) 3019 */ 3020 public List<ConditionDefinitionPlanComponent> getPlan() { 3021 if (this.plan == null) 3022 this.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 3023 return this.plan; 3024 } 3025 3026 /** 3027 * @return Returns a reference to <code>this</code> for easy method chaining 3028 */ 3029 public ConditionDefinition setPlan(List<ConditionDefinitionPlanComponent> thePlan) { 3030 this.plan = thePlan; 3031 return this; 3032 } 3033 3034 public boolean hasPlan() { 3035 if (this.plan == null) 3036 return false; 3037 for (ConditionDefinitionPlanComponent item : this.plan) 3038 if (!item.isEmpty()) 3039 return true; 3040 return false; 3041 } 3042 3043 public ConditionDefinitionPlanComponent addPlan() { //3 3044 ConditionDefinitionPlanComponent t = new ConditionDefinitionPlanComponent(); 3045 if (this.plan == null) 3046 this.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 3047 this.plan.add(t); 3048 return t; 3049 } 3050 3051 public ConditionDefinition addPlan(ConditionDefinitionPlanComponent t) { //3 3052 if (t == null) 3053 return this; 3054 if (this.plan == null) 3055 this.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 3056 this.plan.add(t); 3057 return this; 3058 } 3059 3060 /** 3061 * @return The first repetition of repeating field {@link #plan}, creating it if it does not already exist {3} 3062 */ 3063 public ConditionDefinitionPlanComponent getPlanFirstRep() { 3064 if (getPlan().isEmpty()) { 3065 addPlan(); 3066 } 3067 return getPlan().get(0); 3068 } 3069 3070 /** 3071 * not supported on this implementation 3072 */ 3073 @Override 3074 public int getPurposeMax() { 3075 return 0; 3076 } 3077 /** 3078 * @return {@link #purpose} (Explanation of why this condition definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3079 */ 3080 public MarkdownType getPurposeElement() { 3081 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3082 } 3083 3084 public boolean hasPurposeElement() { 3085 return false; 3086 } 3087 public boolean hasPurpose() { 3088 return false; 3089 } 3090 3091 /** 3092 * @param value {@link #purpose} (Explanation of why this condition definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3093 */ 3094 public ConditionDefinition setPurposeElement(MarkdownType value) { 3095 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3096 } 3097 public String getPurpose() { 3098 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3099 } 3100 /** 3101 * @param value Explanation of why this condition definition is needed and why it has been designed as it has. 3102 */ 3103 public ConditionDefinition setPurpose(String value) { 3104 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3105 } 3106 /** 3107 * not supported on this implementation 3108 */ 3109 @Override 3110 public int getCopyrightMax() { 3111 return 0; 3112 } 3113 /** 3114 * @return {@link #copyright} (A copyright statement relating to the condition definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3115 */ 3116 public MarkdownType getCopyrightElement() { 3117 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3118 } 3119 3120 public boolean hasCopyrightElement() { 3121 return false; 3122 } 3123 public boolean hasCopyright() { 3124 return false; 3125 } 3126 3127 /** 3128 * @param value {@link #copyright} (A copyright statement relating to the condition definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3129 */ 3130 public ConditionDefinition setCopyrightElement(MarkdownType value) { 3131 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3132 } 3133 public String getCopyright() { 3134 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3135 } 3136 /** 3137 * @param value A copyright statement relating to the condition definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the condition definition. 3138 */ 3139 public ConditionDefinition setCopyright(String value) { 3140 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3141 } 3142 /** 3143 * not supported on this implementation 3144 */ 3145 @Override 3146 public int getCopyrightLabelMax() { 3147 return 0; 3148 } 3149 /** 3150 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3151 */ 3152 public StringType getCopyrightLabelElement() { 3153 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3154 } 3155 3156 public boolean hasCopyrightLabelElement() { 3157 return false; 3158 } 3159 public boolean hasCopyrightLabel() { 3160 return false; 3161 } 3162 3163 /** 3164 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3165 */ 3166 public ConditionDefinition setCopyrightLabelElement(StringType value) { 3167 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3168 } 3169 public String getCopyrightLabel() { 3170 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3171 } 3172 /** 3173 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3174 */ 3175 public ConditionDefinition setCopyrightLabel(String value) { 3176 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3177 } 3178 /** 3179 * not supported on this implementation 3180 */ 3181 @Override 3182 public int getApprovalDateMax() { 3183 return 0; 3184 } 3185 /** 3186 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3187 */ 3188 public DateType getApprovalDateElement() { 3189 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3190 } 3191 3192 public boolean hasApprovalDateElement() { 3193 return false; 3194 } 3195 public boolean hasApprovalDate() { 3196 return false; 3197 } 3198 3199 /** 3200 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3201 */ 3202 public ConditionDefinition setApprovalDateElement(DateType value) { 3203 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3204 } 3205 public Date getApprovalDate() { 3206 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3207 } 3208 /** 3209 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3210 */ 3211 public ConditionDefinition setApprovalDate(Date value) { 3212 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3213 } 3214 /** 3215 * not supported on this implementation 3216 */ 3217 @Override 3218 public int getLastReviewDateMax() { 3219 return 0; 3220 } 3221 /** 3222 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3223 */ 3224 public DateType getLastReviewDateElement() { 3225 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3226 } 3227 3228 public boolean hasLastReviewDateElement() { 3229 return false; 3230 } 3231 public boolean hasLastReviewDate() { 3232 return false; 3233 } 3234 3235 /** 3236 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3237 */ 3238 public ConditionDefinition setLastReviewDateElement(DateType value) { 3239 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3240 } 3241 public Date getLastReviewDate() { 3242 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3243 } 3244 /** 3245 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3246 */ 3247 public ConditionDefinition setLastReviewDate(Date value) { 3248 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3249 } 3250 /** 3251 * not supported on this implementation 3252 */ 3253 @Override 3254 public int getEffectivePeriodMax() { 3255 return 0; 3256 } 3257 /** 3258 * @return {@link #effectivePeriod} (The period during which the condition definition content was or is planned to be in active use.) 3259 */ 3260 public Period getEffectivePeriod() { 3261 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"effectivePeriod\""); 3262 } 3263 public boolean hasEffectivePeriod() { 3264 return false; 3265 } 3266 /** 3267 * @param value {@link #effectivePeriod} (The period during which the condition definition content was or is planned to be in active use.) 3268 */ 3269 public ConditionDefinition setEffectivePeriod(Period value) { 3270 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"effectivePeriod\""); 3271 } 3272 3273 /** 3274 * not supported on this implementation 3275 */ 3276 @Override 3277 public int getTopicMax() { 3278 return 0; 3279 } 3280 /** 3281 * @return {@link #topic} (Descriptive topics related to the content of the condition definition. Topics provide a high-level categorization as well as keywords for the condition definition that can be useful for filtering and searching.) 3282 */ 3283 public List<CodeableConcept> getTopic() { 3284 return new ArrayList<>(); 3285 } 3286 /** 3287 * @return Returns a reference to <code>this</code> for easy method chaining 3288 */ 3289 public ConditionDefinition setTopic(List<CodeableConcept> theTopic) { 3290 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3291 } 3292 public boolean hasTopic() { 3293 return false; 3294 } 3295 3296 public CodeableConcept addTopic() { //3 3297 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3298 } 3299 public ConditionDefinition addTopic(CodeableConcept t) { //3 3300 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3301 } 3302 /** 3303 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 3304 */ 3305 public CodeableConcept getTopicFirstRep() { 3306 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3307 } 3308 /** 3309 * not supported on this implementation 3310 */ 3311 @Override 3312 public int getAuthorMax() { 3313 return 0; 3314 } 3315 /** 3316 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the condition definition.) 3317 */ 3318 public List<ContactDetail> getAuthor() { 3319 return new ArrayList<>(); 3320 } 3321 /** 3322 * @return Returns a reference to <code>this</code> for easy method chaining 3323 */ 3324 public ConditionDefinition setAuthor(List<ContactDetail> theAuthor) { 3325 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3326 } 3327 public boolean hasAuthor() { 3328 return false; 3329 } 3330 3331 public ContactDetail addAuthor() { //3 3332 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3333 } 3334 public ConditionDefinition addAuthor(ContactDetail t) { //3 3335 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3336 } 3337 /** 3338 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {2} 3339 */ 3340 public ContactDetail getAuthorFirstRep() { 3341 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3342 } 3343 /** 3344 * not supported on this implementation 3345 */ 3346 @Override 3347 public int getEditorMax() { 3348 return 0; 3349 } 3350 /** 3351 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the condition definition.) 3352 */ 3353 public List<ContactDetail> getEditor() { 3354 return new ArrayList<>(); 3355 } 3356 /** 3357 * @return Returns a reference to <code>this</code> for easy method chaining 3358 */ 3359 public ConditionDefinition setEditor(List<ContactDetail> theEditor) { 3360 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3361 } 3362 public boolean hasEditor() { 3363 return false; 3364 } 3365 3366 public ContactDetail addEditor() { //3 3367 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3368 } 3369 public ConditionDefinition addEditor(ContactDetail t) { //3 3370 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3371 } 3372 /** 3373 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {2} 3374 */ 3375 public ContactDetail getEditorFirstRep() { 3376 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3377 } 3378 /** 3379 * not supported on this implementation 3380 */ 3381 @Override 3382 public int getReviewerMax() { 3383 return 0; 3384 } 3385 /** 3386 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the condition definition.) 3387 */ 3388 public List<ContactDetail> getReviewer() { 3389 return new ArrayList<>(); 3390 } 3391 /** 3392 * @return Returns a reference to <code>this</code> for easy method chaining 3393 */ 3394 public ConditionDefinition setReviewer(List<ContactDetail> theReviewer) { 3395 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3396 } 3397 public boolean hasReviewer() { 3398 return false; 3399 } 3400 3401 public ContactDetail addReviewer() { //3 3402 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3403 } 3404 public ConditionDefinition addReviewer(ContactDetail t) { //3 3405 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3406 } 3407 /** 3408 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {2} 3409 */ 3410 public ContactDetail getReviewerFirstRep() { 3411 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3412 } 3413 /** 3414 * not supported on this implementation 3415 */ 3416 @Override 3417 public int getEndorserMax() { 3418 return 0; 3419 } 3420 /** 3421 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the condition definition for use in some setting.) 3422 */ 3423 public List<ContactDetail> getEndorser() { 3424 return new ArrayList<>(); 3425 } 3426 /** 3427 * @return Returns a reference to <code>this</code> for easy method chaining 3428 */ 3429 public ConditionDefinition setEndorser(List<ContactDetail> theEndorser) { 3430 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3431 } 3432 public boolean hasEndorser() { 3433 return false; 3434 } 3435 3436 public ContactDetail addEndorser() { //3 3437 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3438 } 3439 public ConditionDefinition addEndorser(ContactDetail t) { //3 3440 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3441 } 3442 /** 3443 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {2} 3444 */ 3445 public ContactDetail getEndorserFirstRep() { 3446 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3447 } 3448 /** 3449 * not supported on this implementation 3450 */ 3451 @Override 3452 public int getRelatedArtifactMax() { 3453 return 0; 3454 } 3455 /** 3456 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 3457 */ 3458 public List<RelatedArtifact> getRelatedArtifact() { 3459 return new ArrayList<>(); 3460 } 3461 /** 3462 * @return Returns a reference to <code>this</code> for easy method chaining 3463 */ 3464 public ConditionDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3465 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3466 } 3467 public boolean hasRelatedArtifact() { 3468 return false; 3469 } 3470 3471 public RelatedArtifact addRelatedArtifact() { //3 3472 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3473 } 3474 public ConditionDefinition addRelatedArtifact(RelatedArtifact t) { //3 3475 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3476 } 3477 /** 3478 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {2} 3479 */ 3480 public RelatedArtifact getRelatedArtifactFirstRep() { 3481 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3482 } 3483 protected void listChildren(List<Property> children) { 3484 super.listChildren(children); 3485 children.add(new Property("url", "uri", "An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.", 0, 1, url)); 3486 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3487 children.add(new Property("version", "string", "The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3488 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3489 children.add(new Property("name", "string", "A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3490 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the condition definition.", 0, 1, title)); 3491 children.add(new Property("subtitle", "string", "An explanatory or alternate title for the event definition giving additional information about its content.", 0, 1, subtitle)); 3492 children.add(new Property("status", "code", "The status of this condition definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3493 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 3494 children.add(new Property("date", "dateTime", "The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.", 0, 1, date)); 3495 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.", 0, 1, publisher)); 3496 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3497 children.add(new Property("description", "markdown", "A free text natural language description of the condition definition from a consumer's perspective.", 0, 1, description)); 3498 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3499 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the condition definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3500 children.add(new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code)); 3501 children.add(new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity)); 3502 children.add(new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, 1, bodySite)); 3503 children.add(new Property("stage", "CodeableConcept", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1, stage)); 3504 children.add(new Property("hasSeverity", "boolean", "Whether Severity is appropriate to collect for this condition.", 0, 1, hasSeverity)); 3505 children.add(new Property("hasBodySite", "boolean", "Whether bodySite is appropriate to collect for this condition.", 0, 1, hasBodySite)); 3506 children.add(new Property("hasStage", "boolean", "Whether stage is appropriate to collect for this condition.", 0, 1, hasStage)); 3507 children.add(new Property("definition", "uri", "Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.", 0, java.lang.Integer.MAX_VALUE, definition)); 3508 children.add(new Property("observation", "", "Observations particularly relevant to this condition.", 0, java.lang.Integer.MAX_VALUE, observation)); 3509 children.add(new Property("medication", "", "Medications particularly relevant for this condition.", 0, java.lang.Integer.MAX_VALUE, medication)); 3510 children.add(new Property("precondition", "", "An observation that suggests that this condition applies.", 0, java.lang.Integer.MAX_VALUE, precondition)); 3511 children.add(new Property("team", "Reference(CareTeam)", "Appropriate team for this condition.", 0, java.lang.Integer.MAX_VALUE, team)); 3512 children.add(new Property("questionnaire", "", "Questionnaire for this condition.", 0, java.lang.Integer.MAX_VALUE, questionnaire)); 3513 children.add(new Property("plan", "", "Plan that is appropriate.", 0, java.lang.Integer.MAX_VALUE, plan)); 3514 } 3515 3516 @Override 3517 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3518 switch (_hash) { 3519 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.", 0, 1, url); 3520 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3521 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3522 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3523 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3524 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3525 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3526 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3527 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the condition definition.", 0, 1, title); 3528 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "An explanatory or alternate title for the event definition giving additional information about its content.", 0, 1, subtitle); 3529 case -892481550: /*status*/ return new Property("status", "code", "The status of this condition definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3530 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 3531 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.", 0, 1, date); 3532 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.", 0, 1, publisher); 3533 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3534 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the condition definition from a consumer's perspective.", 0, 1, description); 3535 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3536 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the condition definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3537 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code); 3538 case 1478300413: /*severity*/ return new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity); 3539 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, 1, bodySite); 3540 case 109757182: /*stage*/ return new Property("stage", "CodeableConcept", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1, stage); 3541 case 57790391: /*hasSeverity*/ return new Property("hasSeverity", "boolean", "Whether Severity is appropriate to collect for this condition.", 0, 1, hasSeverity); 3542 case 282110147: /*hasBodySite*/ return new Property("hasBodySite", "boolean", "Whether bodySite is appropriate to collect for this condition.", 0, 1, hasBodySite); 3543 case 129749124: /*hasStage*/ return new Property("hasStage", "boolean", "Whether stage is appropriate to collect for this condition.", 0, 1, hasStage); 3544 case -1014418093: /*definition*/ return new Property("definition", "uri", "Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.", 0, java.lang.Integer.MAX_VALUE, definition); 3545 case 122345516: /*observation*/ return new Property("observation", "", "Observations particularly relevant to this condition.", 0, java.lang.Integer.MAX_VALUE, observation); 3546 case 1998965455: /*medication*/ return new Property("medication", "", "Medications particularly relevant for this condition.", 0, java.lang.Integer.MAX_VALUE, medication); 3547 case -650968616: /*precondition*/ return new Property("precondition", "", "An observation that suggests that this condition applies.", 0, java.lang.Integer.MAX_VALUE, precondition); 3548 case 3555933: /*team*/ return new Property("team", "Reference(CareTeam)", "Appropriate team for this condition.", 0, java.lang.Integer.MAX_VALUE, team); 3549 case -1017049693: /*questionnaire*/ return new Property("questionnaire", "", "Questionnaire for this condition.", 0, java.lang.Integer.MAX_VALUE, questionnaire); 3550 case 3443497: /*plan*/ return new Property("plan", "", "Plan that is appropriate.", 0, java.lang.Integer.MAX_VALUE, plan); 3551 default: return super.getNamedProperty(_hash, _name, _checkValid); 3552 } 3553 3554 } 3555 3556 @Override 3557 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3558 switch (hash) { 3559 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3560 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3561 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3562 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3563 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3564 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3565 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 3566 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3567 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3568 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3569 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3570 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3571 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3572 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3573 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3574 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3575 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // CodeableConcept 3576 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 3577 case 109757182: /*stage*/ return this.stage == null ? new Base[0] : new Base[] {this.stage}; // CodeableConcept 3578 case 57790391: /*hasSeverity*/ return this.hasSeverity == null ? new Base[0] : new Base[] {this.hasSeverity}; // BooleanType 3579 case 282110147: /*hasBodySite*/ return this.hasBodySite == null ? new Base[0] : new Base[] {this.hasBodySite}; // BooleanType 3580 case 129749124: /*hasStage*/ return this.hasStage == null ? new Base[0] : new Base[] {this.hasStage}; // BooleanType 3581 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // UriType 3582 case 122345516: /*observation*/ return this.observation == null ? new Base[0] : this.observation.toArray(new Base[this.observation.size()]); // ConditionDefinitionObservationComponent 3583 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : this.medication.toArray(new Base[this.medication.size()]); // ConditionDefinitionMedicationComponent 3584 case -650968616: /*precondition*/ return this.precondition == null ? new Base[0] : this.precondition.toArray(new Base[this.precondition.size()]); // ConditionDefinitionPreconditionComponent 3585 case 3555933: /*team*/ return this.team == null ? new Base[0] : this.team.toArray(new Base[this.team.size()]); // Reference 3586 case -1017049693: /*questionnaire*/ return this.questionnaire == null ? new Base[0] : this.questionnaire.toArray(new Base[this.questionnaire.size()]); // ConditionDefinitionQuestionnaireComponent 3587 case 3443497: /*plan*/ return this.plan == null ? new Base[0] : this.plan.toArray(new Base[this.plan.size()]); // ConditionDefinitionPlanComponent 3588 default: return super.getProperty(hash, name, checkValid); 3589 } 3590 3591 } 3592 3593 @Override 3594 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3595 switch (hash) { 3596 case 116079: // url 3597 this.url = TypeConvertor.castToUri(value); // UriType 3598 return value; 3599 case -1618432855: // identifier 3600 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3601 return value; 3602 case 351608024: // version 3603 this.version = TypeConvertor.castToString(value); // StringType 3604 return value; 3605 case 1508158071: // versionAlgorithm 3606 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3607 return value; 3608 case 3373707: // name 3609 this.name = TypeConvertor.castToString(value); // StringType 3610 return value; 3611 case 110371416: // title 3612 this.title = TypeConvertor.castToString(value); // StringType 3613 return value; 3614 case -2060497896: // subtitle 3615 this.subtitle = TypeConvertor.castToString(value); // StringType 3616 return value; 3617 case -892481550: // status 3618 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3619 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3620 return value; 3621 case -404562712: // experimental 3622 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3623 return value; 3624 case 3076014: // date 3625 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3626 return value; 3627 case 1447404028: // publisher 3628 this.publisher = TypeConvertor.castToString(value); // StringType 3629 return value; 3630 case 951526432: // contact 3631 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3632 return value; 3633 case -1724546052: // description 3634 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3635 return value; 3636 case -669707736: // useContext 3637 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3638 return value; 3639 case -507075711: // jurisdiction 3640 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3641 return value; 3642 case 3059181: // code 3643 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3644 return value; 3645 case 1478300413: // severity 3646 this.severity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3647 return value; 3648 case 1702620169: // bodySite 3649 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3650 return value; 3651 case 109757182: // stage 3652 this.stage = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3653 return value; 3654 case 57790391: // hasSeverity 3655 this.hasSeverity = TypeConvertor.castToBoolean(value); // BooleanType 3656 return value; 3657 case 282110147: // hasBodySite 3658 this.hasBodySite = TypeConvertor.castToBoolean(value); // BooleanType 3659 return value; 3660 case 129749124: // hasStage 3661 this.hasStage = TypeConvertor.castToBoolean(value); // BooleanType 3662 return value; 3663 case -1014418093: // definition 3664 this.getDefinition().add(TypeConvertor.castToUri(value)); // UriType 3665 return value; 3666 case 122345516: // observation 3667 this.getObservation().add((ConditionDefinitionObservationComponent) value); // ConditionDefinitionObservationComponent 3668 return value; 3669 case 1998965455: // medication 3670 this.getMedication().add((ConditionDefinitionMedicationComponent) value); // ConditionDefinitionMedicationComponent 3671 return value; 3672 case -650968616: // precondition 3673 this.getPrecondition().add((ConditionDefinitionPreconditionComponent) value); // ConditionDefinitionPreconditionComponent 3674 return value; 3675 case 3555933: // team 3676 this.getTeam().add(TypeConvertor.castToReference(value)); // Reference 3677 return value; 3678 case -1017049693: // questionnaire 3679 this.getQuestionnaire().add((ConditionDefinitionQuestionnaireComponent) value); // ConditionDefinitionQuestionnaireComponent 3680 return value; 3681 case 3443497: // plan 3682 this.getPlan().add((ConditionDefinitionPlanComponent) value); // ConditionDefinitionPlanComponent 3683 return value; 3684 default: return super.setProperty(hash, name, value); 3685 } 3686 3687 } 3688 3689 @Override 3690 public Base setProperty(String name, Base value) throws FHIRException { 3691 if (name.equals("url")) { 3692 this.url = TypeConvertor.castToUri(value); // UriType 3693 } else if (name.equals("identifier")) { 3694 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3695 } else if (name.equals("version")) { 3696 this.version = TypeConvertor.castToString(value); // StringType 3697 } else if (name.equals("versionAlgorithm[x]")) { 3698 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3699 } else if (name.equals("name")) { 3700 this.name = TypeConvertor.castToString(value); // StringType 3701 } else if (name.equals("title")) { 3702 this.title = TypeConvertor.castToString(value); // StringType 3703 } else if (name.equals("subtitle")) { 3704 this.subtitle = TypeConvertor.castToString(value); // StringType 3705 } else if (name.equals("status")) { 3706 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3707 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3708 } else if (name.equals("experimental")) { 3709 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3710 } else if (name.equals("date")) { 3711 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3712 } else if (name.equals("publisher")) { 3713 this.publisher = TypeConvertor.castToString(value); // StringType 3714 } else if (name.equals("contact")) { 3715 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3716 } else if (name.equals("description")) { 3717 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3718 } else if (name.equals("useContext")) { 3719 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 3720 } else if (name.equals("jurisdiction")) { 3721 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3722 } else if (name.equals("code")) { 3723 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3724 } else if (name.equals("severity")) { 3725 this.severity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3726 } else if (name.equals("bodySite")) { 3727 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3728 } else if (name.equals("stage")) { 3729 this.stage = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3730 } else if (name.equals("hasSeverity")) { 3731 this.hasSeverity = TypeConvertor.castToBoolean(value); // BooleanType 3732 } else if (name.equals("hasBodySite")) { 3733 this.hasBodySite = TypeConvertor.castToBoolean(value); // BooleanType 3734 } else if (name.equals("hasStage")) { 3735 this.hasStage = TypeConvertor.castToBoolean(value); // BooleanType 3736 } else if (name.equals("definition")) { 3737 this.getDefinition().add(TypeConvertor.castToUri(value)); 3738 } else if (name.equals("observation")) { 3739 this.getObservation().add((ConditionDefinitionObservationComponent) value); 3740 } else if (name.equals("medication")) { 3741 this.getMedication().add((ConditionDefinitionMedicationComponent) value); 3742 } else if (name.equals("precondition")) { 3743 this.getPrecondition().add((ConditionDefinitionPreconditionComponent) value); 3744 } else if (name.equals("team")) { 3745 this.getTeam().add(TypeConvertor.castToReference(value)); 3746 } else if (name.equals("questionnaire")) { 3747 this.getQuestionnaire().add((ConditionDefinitionQuestionnaireComponent) value); 3748 } else if (name.equals("plan")) { 3749 this.getPlan().add((ConditionDefinitionPlanComponent) value); 3750 } else 3751 return super.setProperty(name, value); 3752 return value; 3753 } 3754 3755 @Override 3756 public void removeChild(String name, Base value) throws FHIRException { 3757 if (name.equals("url")) { 3758 this.url = null; 3759 } else if (name.equals("identifier")) { 3760 this.getIdentifier().remove(value); 3761 } else if (name.equals("version")) { 3762 this.version = null; 3763 } else if (name.equals("versionAlgorithm[x]")) { 3764 this.versionAlgorithm = null; 3765 } else if (name.equals("name")) { 3766 this.name = null; 3767 } else if (name.equals("title")) { 3768 this.title = null; 3769 } else if (name.equals("subtitle")) { 3770 this.subtitle = null; 3771 } else if (name.equals("status")) { 3772 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3773 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3774 } else if (name.equals("experimental")) { 3775 this.experimental = null; 3776 } else if (name.equals("date")) { 3777 this.date = null; 3778 } else if (name.equals("publisher")) { 3779 this.publisher = null; 3780 } else if (name.equals("contact")) { 3781 this.getContact().remove(value); 3782 } else if (name.equals("description")) { 3783 this.description = null; 3784 } else if (name.equals("useContext")) { 3785 this.getUseContext().remove(value); 3786 } else if (name.equals("jurisdiction")) { 3787 this.getJurisdiction().remove(value); 3788 } else if (name.equals("code")) { 3789 this.code = null; 3790 } else if (name.equals("severity")) { 3791 this.severity = null; 3792 } else if (name.equals("bodySite")) { 3793 this.bodySite = null; 3794 } else if (name.equals("stage")) { 3795 this.stage = null; 3796 } else if (name.equals("hasSeverity")) { 3797 this.hasSeverity = null; 3798 } else if (name.equals("hasBodySite")) { 3799 this.hasBodySite = null; 3800 } else if (name.equals("hasStage")) { 3801 this.hasStage = null; 3802 } else if (name.equals("definition")) { 3803 this.getDefinition().remove(value); 3804 } else if (name.equals("observation")) { 3805 this.getObservation().remove((ConditionDefinitionObservationComponent) value); 3806 } else if (name.equals("medication")) { 3807 this.getMedication().remove((ConditionDefinitionMedicationComponent) value); 3808 } else if (name.equals("precondition")) { 3809 this.getPrecondition().remove((ConditionDefinitionPreconditionComponent) value); 3810 } else if (name.equals("team")) { 3811 this.getTeam().remove(value); 3812 } else if (name.equals("questionnaire")) { 3813 this.getQuestionnaire().remove((ConditionDefinitionQuestionnaireComponent) value); 3814 } else if (name.equals("plan")) { 3815 this.getPlan().remove((ConditionDefinitionPlanComponent) value); 3816 } else 3817 super.removeChild(name, value); 3818 3819 } 3820 3821 @Override 3822 public Base makeProperty(int hash, String name) throws FHIRException { 3823 switch (hash) { 3824 case 116079: return getUrlElement(); 3825 case -1618432855: return addIdentifier(); 3826 case 351608024: return getVersionElement(); 3827 case -115699031: return getVersionAlgorithm(); 3828 case 1508158071: return getVersionAlgorithm(); 3829 case 3373707: return getNameElement(); 3830 case 110371416: return getTitleElement(); 3831 case -2060497896: return getSubtitleElement(); 3832 case -892481550: return getStatusElement(); 3833 case -404562712: return getExperimentalElement(); 3834 case 3076014: return getDateElement(); 3835 case 1447404028: return getPublisherElement(); 3836 case 951526432: return addContact(); 3837 case -1724546052: return getDescriptionElement(); 3838 case -669707736: return addUseContext(); 3839 case -507075711: return addJurisdiction(); 3840 case 3059181: return getCode(); 3841 case 1478300413: return getSeverity(); 3842 case 1702620169: return getBodySite(); 3843 case 109757182: return getStage(); 3844 case 57790391: return getHasSeverityElement(); 3845 case 282110147: return getHasBodySiteElement(); 3846 case 129749124: return getHasStageElement(); 3847 case -1014418093: return addDefinitionElement(); 3848 case 122345516: return addObservation(); 3849 case 1998965455: return addMedication(); 3850 case -650968616: return addPrecondition(); 3851 case 3555933: return addTeam(); 3852 case -1017049693: return addQuestionnaire(); 3853 case 3443497: return addPlan(); 3854 default: return super.makeProperty(hash, name); 3855 } 3856 3857 } 3858 3859 @Override 3860 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3861 switch (hash) { 3862 case 116079: /*url*/ return new String[] {"uri"}; 3863 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3864 case 351608024: /*version*/ return new String[] {"string"}; 3865 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3866 case 3373707: /*name*/ return new String[] {"string"}; 3867 case 110371416: /*title*/ return new String[] {"string"}; 3868 case -2060497896: /*subtitle*/ return new String[] {"string"}; 3869 case -892481550: /*status*/ return new String[] {"code"}; 3870 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3871 case 3076014: /*date*/ return new String[] {"dateTime"}; 3872 case 1447404028: /*publisher*/ return new String[] {"string"}; 3873 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3874 case -1724546052: /*description*/ return new String[] {"markdown"}; 3875 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3876 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3877 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3878 case 1478300413: /*severity*/ return new String[] {"CodeableConcept"}; 3879 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 3880 case 109757182: /*stage*/ return new String[] {"CodeableConcept"}; 3881 case 57790391: /*hasSeverity*/ return new String[] {"boolean"}; 3882 case 282110147: /*hasBodySite*/ return new String[] {"boolean"}; 3883 case 129749124: /*hasStage*/ return new String[] {"boolean"}; 3884 case -1014418093: /*definition*/ return new String[] {"uri"}; 3885 case 122345516: /*observation*/ return new String[] {}; 3886 case 1998965455: /*medication*/ return new String[] {}; 3887 case -650968616: /*precondition*/ return new String[] {}; 3888 case 3555933: /*team*/ return new String[] {"Reference"}; 3889 case -1017049693: /*questionnaire*/ return new String[] {}; 3890 case 3443497: /*plan*/ return new String[] {}; 3891 default: return super.getTypesForProperty(hash, name); 3892 } 3893 3894 } 3895 3896 @Override 3897 public Base addChild(String name) throws FHIRException { 3898 if (name.equals("url")) { 3899 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.url"); 3900 } 3901 else if (name.equals("identifier")) { 3902 return addIdentifier(); 3903 } 3904 else if (name.equals("version")) { 3905 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.version"); 3906 } 3907 else if (name.equals("versionAlgorithmString")) { 3908 this.versionAlgorithm = new StringType(); 3909 return this.versionAlgorithm; 3910 } 3911 else if (name.equals("versionAlgorithmCoding")) { 3912 this.versionAlgorithm = new Coding(); 3913 return this.versionAlgorithm; 3914 } 3915 else if (name.equals("name")) { 3916 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.name"); 3917 } 3918 else if (name.equals("title")) { 3919 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.title"); 3920 } 3921 else if (name.equals("subtitle")) { 3922 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.subtitle"); 3923 } 3924 else if (name.equals("status")) { 3925 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.status"); 3926 } 3927 else if (name.equals("experimental")) { 3928 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.experimental"); 3929 } 3930 else if (name.equals("date")) { 3931 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.date"); 3932 } 3933 else if (name.equals("publisher")) { 3934 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.publisher"); 3935 } 3936 else if (name.equals("contact")) { 3937 return addContact(); 3938 } 3939 else if (name.equals("description")) { 3940 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.description"); 3941 } 3942 else if (name.equals("useContext")) { 3943 return addUseContext(); 3944 } 3945 else if (name.equals("jurisdiction")) { 3946 return addJurisdiction(); 3947 } 3948 else if (name.equals("code")) { 3949 this.code = new CodeableConcept(); 3950 return this.code; 3951 } 3952 else if (name.equals("severity")) { 3953 this.severity = new CodeableConcept(); 3954 return this.severity; 3955 } 3956 else if (name.equals("bodySite")) { 3957 this.bodySite = new CodeableConcept(); 3958 return this.bodySite; 3959 } 3960 else if (name.equals("stage")) { 3961 this.stage = new CodeableConcept(); 3962 return this.stage; 3963 } 3964 else if (name.equals("hasSeverity")) { 3965 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.hasSeverity"); 3966 } 3967 else if (name.equals("hasBodySite")) { 3968 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.hasBodySite"); 3969 } 3970 else if (name.equals("hasStage")) { 3971 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.hasStage"); 3972 } 3973 else if (name.equals("definition")) { 3974 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.definition"); 3975 } 3976 else if (name.equals("observation")) { 3977 return addObservation(); 3978 } 3979 else if (name.equals("medication")) { 3980 return addMedication(); 3981 } 3982 else if (name.equals("precondition")) { 3983 return addPrecondition(); 3984 } 3985 else if (name.equals("team")) { 3986 return addTeam(); 3987 } 3988 else if (name.equals("questionnaire")) { 3989 return addQuestionnaire(); 3990 } 3991 else if (name.equals("plan")) { 3992 return addPlan(); 3993 } 3994 else 3995 return super.addChild(name); 3996 } 3997 3998 public String fhirType() { 3999 return "ConditionDefinition"; 4000 4001 } 4002 4003 public ConditionDefinition copy() { 4004 ConditionDefinition dst = new ConditionDefinition(); 4005 copyValues(dst); 4006 return dst; 4007 } 4008 4009 public void copyValues(ConditionDefinition dst) { 4010 super.copyValues(dst); 4011 dst.url = url == null ? null : url.copy(); 4012 if (identifier != null) { 4013 dst.identifier = new ArrayList<Identifier>(); 4014 for (Identifier i : identifier) 4015 dst.identifier.add(i.copy()); 4016 }; 4017 dst.version = version == null ? null : version.copy(); 4018 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4019 dst.name = name == null ? null : name.copy(); 4020 dst.title = title == null ? null : title.copy(); 4021 dst.subtitle = subtitle == null ? null : subtitle.copy(); 4022 dst.status = status == null ? null : status.copy(); 4023 dst.experimental = experimental == null ? null : experimental.copy(); 4024 dst.date = date == null ? null : date.copy(); 4025 dst.publisher = publisher == null ? null : publisher.copy(); 4026 if (contact != null) { 4027 dst.contact = new ArrayList<ContactDetail>(); 4028 for (ContactDetail i : contact) 4029 dst.contact.add(i.copy()); 4030 }; 4031 dst.description = description == null ? null : description.copy(); 4032 if (useContext != null) { 4033 dst.useContext = new ArrayList<UsageContext>(); 4034 for (UsageContext i : useContext) 4035 dst.useContext.add(i.copy()); 4036 }; 4037 if (jurisdiction != null) { 4038 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4039 for (CodeableConcept i : jurisdiction) 4040 dst.jurisdiction.add(i.copy()); 4041 }; 4042 dst.code = code == null ? null : code.copy(); 4043 dst.severity = severity == null ? null : severity.copy(); 4044 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4045 dst.stage = stage == null ? null : stage.copy(); 4046 dst.hasSeverity = hasSeverity == null ? null : hasSeverity.copy(); 4047 dst.hasBodySite = hasBodySite == null ? null : hasBodySite.copy(); 4048 dst.hasStage = hasStage == null ? null : hasStage.copy(); 4049 if (definition != null) { 4050 dst.definition = new ArrayList<UriType>(); 4051 for (UriType i : definition) 4052 dst.definition.add(i.copy()); 4053 }; 4054 if (observation != null) { 4055 dst.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 4056 for (ConditionDefinitionObservationComponent i : observation) 4057 dst.observation.add(i.copy()); 4058 }; 4059 if (medication != null) { 4060 dst.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 4061 for (ConditionDefinitionMedicationComponent i : medication) 4062 dst.medication.add(i.copy()); 4063 }; 4064 if (precondition != null) { 4065 dst.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 4066 for (ConditionDefinitionPreconditionComponent i : precondition) 4067 dst.precondition.add(i.copy()); 4068 }; 4069 if (team != null) { 4070 dst.team = new ArrayList<Reference>(); 4071 for (Reference i : team) 4072 dst.team.add(i.copy()); 4073 }; 4074 if (questionnaire != null) { 4075 dst.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 4076 for (ConditionDefinitionQuestionnaireComponent i : questionnaire) 4077 dst.questionnaire.add(i.copy()); 4078 }; 4079 if (plan != null) { 4080 dst.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 4081 for (ConditionDefinitionPlanComponent i : plan) 4082 dst.plan.add(i.copy()); 4083 }; 4084 } 4085 4086 protected ConditionDefinition typedCopy() { 4087 return copy(); 4088 } 4089 4090 @Override 4091 public boolean equalsDeep(Base other_) { 4092 if (!super.equalsDeep(other_)) 4093 return false; 4094 if (!(other_ instanceof ConditionDefinition)) 4095 return false; 4096 ConditionDefinition o = (ConditionDefinition) other_; 4097 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4098 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4099 && compareDeep(subtitle, o.subtitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 4100 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 4101 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 4102 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(code, o.code, true) && compareDeep(severity, o.severity, true) 4103 && compareDeep(bodySite, o.bodySite, true) && compareDeep(stage, o.stage, true) && compareDeep(hasSeverity, o.hasSeverity, true) 4104 && compareDeep(hasBodySite, o.hasBodySite, true) && compareDeep(hasStage, o.hasStage, true) && compareDeep(definition, o.definition, true) 4105 && compareDeep(observation, o.observation, true) && compareDeep(medication, o.medication, true) 4106 && compareDeep(precondition, o.precondition, true) && compareDeep(team, o.team, true) && compareDeep(questionnaire, o.questionnaire, true) 4107 && compareDeep(plan, o.plan, true); 4108 } 4109 4110 @Override 4111 public boolean equalsShallow(Base other_) { 4112 if (!super.equalsShallow(other_)) 4113 return false; 4114 if (!(other_ instanceof ConditionDefinition)) 4115 return false; 4116 ConditionDefinition o = (ConditionDefinition) other_; 4117 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4118 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) && compareValues(status, o.status, true) 4119 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 4120 && compareValues(description, o.description, true) && compareValues(hasSeverity, o.hasSeverity, true) 4121 && compareValues(hasBodySite, o.hasBodySite, true) && compareValues(hasStage, o.hasStage, true) && compareValues(definition, o.definition, true) 4122 ; 4123 } 4124 4125 public boolean isEmpty() { 4126 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4127 , versionAlgorithm, name, title, subtitle, status, experimental, date, publisher 4128 , contact, description, useContext, jurisdiction, code, severity, bodySite, stage 4129 , hasSeverity, hasBodySite, hasStage, definition, observation, medication, precondition 4130 , team, questionnaire, plan); 4131 } 4132 4133 @Override 4134 public ResourceType getResourceType() { 4135 return ResourceType.ConditionDefinition; 4136 } 4137 4138 /** 4139 * Search parameter: <b>context-quantity</b> 4140 * <p> 4141 * Description: <b>Multiple Resources: 4142 4143* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4144* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4145* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4146* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4147* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4148* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4149* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4150* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4151* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4152* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4153* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4154* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4155* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4156* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4157* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4158* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4159* [Library](library.html): A quantity- or range-valued use context assigned to the library 4160* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4161* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4162* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4163* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4164* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4165* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4166* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4167* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4168* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4169* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4170* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4171* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4172* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4173</b><br> 4174 * Type: <b>quantity</b><br> 4175 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4176 * </p> 4177 */ 4178 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 4179 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4180 /** 4181 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4182 * <p> 4183 * Description: <b>Multiple Resources: 4184 4185* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4186* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4187* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4188* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4189* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4190* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4191* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4192* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4193* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4194* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4195* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4196* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4197* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4198* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4199* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4200* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4201* [Library](library.html): A quantity- or range-valued use context assigned to the library 4202* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4203* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4204* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4205* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4206* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4207* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4208* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4209* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4210* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4211* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4212* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4213* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4214* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4215</b><br> 4216 * Type: <b>quantity</b><br> 4217 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4218 * </p> 4219 */ 4220 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 4221 4222 /** 4223 * Search parameter: <b>context-type-quantity</b> 4224 * <p> 4225 * Description: <b>Multiple Resources: 4226 4227* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4228* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4229* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4230* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4231* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4232* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4233* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4234* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4235* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4236* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4237* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4238* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4239* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4240* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4241* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4242* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4243* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4244* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4245* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4246* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4247* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4248* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4249* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4250* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4251* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4252* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4253* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4254* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4255* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4256* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4257</b><br> 4258 * Type: <b>composite</b><br> 4259 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4260 * </p> 4261 */ 4262 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 4263 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4264 /** 4265 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 4266 * <p> 4267 * Description: <b>Multiple Resources: 4268 4269* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4270* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4271* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4272* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4273* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4274* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4275* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4276* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4277* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4278* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4279* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4280* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4281* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4282* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4283* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4284* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4285* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4286* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4287* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4288* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4289* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4290* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4291* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4292* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4293* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4294* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4295* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4296* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4297* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4298* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4299</b><br> 4300 * Type: <b>composite</b><br> 4301 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4302 * </p> 4303 */ 4304 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 4305 4306 /** 4307 * Search parameter: <b>context-type-value</b> 4308 * <p> 4309 * Description: <b>Multiple Resources: 4310 4311* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4312* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4313* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4314* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4315* [Citation](citation.html): A use context type and value assigned to the citation 4316* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4317* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4318* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4319* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4320* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4321* [Evidence](evidence.html): A use context type and value assigned to the evidence 4322* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4323* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4324* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4325* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4326* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4327* [Library](library.html): A use context type and value assigned to the library 4328* [Measure](measure.html): A use context type and value assigned to the measure 4329* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4330* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4331* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4332* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4333* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4334* [Requirements](requirements.html): A use context type and value assigned to the requirements 4335* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4336* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4337* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4338* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4339* [TestScript](testscript.html): A use context type and value assigned to the test script 4340* [ValueSet](valueset.html): A use context type and value assigned to the value set 4341</b><br> 4342 * Type: <b>composite</b><br> 4343 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4344 * </p> 4345 */ 4346 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 4347 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4348 /** 4349 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4350 * <p> 4351 * Description: <b>Multiple Resources: 4352 4353* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4354* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4355* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4356* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4357* [Citation](citation.html): A use context type and value assigned to the citation 4358* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4359* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4360* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4361* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4362* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4363* [Evidence](evidence.html): A use context type and value assigned to the evidence 4364* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4365* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4366* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4367* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4368* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4369* [Library](library.html): A use context type and value assigned to the library 4370* [Measure](measure.html): A use context type and value assigned to the measure 4371* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4372* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4373* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4374* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4375* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4376* [Requirements](requirements.html): A use context type and value assigned to the requirements 4377* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4378* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4379* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4380* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4381* [TestScript](testscript.html): A use context type and value assigned to the test script 4382* [ValueSet](valueset.html): A use context type and value assigned to the value set 4383</b><br> 4384 * Type: <b>composite</b><br> 4385 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4386 * </p> 4387 */ 4388 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 4389 4390 /** 4391 * Search parameter: <b>context-type</b> 4392 * <p> 4393 * Description: <b>Multiple Resources: 4394 4395* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4396* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4397* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4398* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4399* [Citation](citation.html): A type of use context assigned to the citation 4400* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4401* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4402* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4403* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4404* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4405* [Evidence](evidence.html): A type of use context assigned to the evidence 4406* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4407* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4408* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4409* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4410* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4411* [Library](library.html): A type of use context assigned to the library 4412* [Measure](measure.html): A type of use context assigned to the measure 4413* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4414* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4415* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4416* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4417* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4418* [Requirements](requirements.html): A type of use context assigned to the requirements 4419* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4420* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4421* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4422* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4423* [TestScript](testscript.html): A type of use context assigned to the test script 4424* [ValueSet](valueset.html): A type of use context assigned to the value set 4425</b><br> 4426 * Type: <b>token</b><br> 4427 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4428 * </p> 4429 */ 4430 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 4431 public static final String SP_CONTEXT_TYPE = "context-type"; 4432 /** 4433 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4434 * <p> 4435 * Description: <b>Multiple Resources: 4436 4437* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4438* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4439* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4440* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4441* [Citation](citation.html): A type of use context assigned to the citation 4442* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4443* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4444* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4445* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4446* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4447* [Evidence](evidence.html): A type of use context assigned to the evidence 4448* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4449* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4450* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4451* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4452* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4453* [Library](library.html): A type of use context assigned to the library 4454* [Measure](measure.html): A type of use context assigned to the measure 4455* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4456* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4457* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4458* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4459* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4460* [Requirements](requirements.html): A type of use context assigned to the requirements 4461* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4462* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4463* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4464* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4465* [TestScript](testscript.html): A type of use context assigned to the test script 4466* [ValueSet](valueset.html): A type of use context assigned to the value set 4467</b><br> 4468 * Type: <b>token</b><br> 4469 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4470 * </p> 4471 */ 4472 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 4473 4474 /** 4475 * Search parameter: <b>context</b> 4476 * <p> 4477 * Description: <b>Multiple Resources: 4478 4479* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4480* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4481* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4482* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4483* [Citation](citation.html): A use context assigned to the citation 4484* [CodeSystem](codesystem.html): A use context assigned to the code system 4485* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4486* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4487* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4488* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4489* [Evidence](evidence.html): A use context assigned to the evidence 4490* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4491* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4492* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4493* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4494* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4495* [Library](library.html): A use context assigned to the library 4496* [Measure](measure.html): A use context assigned to the measure 4497* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4498* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4499* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4500* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4501* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4502* [Requirements](requirements.html): A use context assigned to the requirements 4503* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4504* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4505* [StructureMap](structuremap.html): A use context assigned to the structure map 4506* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4507* [TestScript](testscript.html): A use context assigned to the test script 4508* [ValueSet](valueset.html): A use context assigned to the value set 4509</b><br> 4510 * Type: <b>token</b><br> 4511 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4512 * </p> 4513 */ 4514 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 4515 public static final String SP_CONTEXT = "context"; 4516 /** 4517 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4518 * <p> 4519 * Description: <b>Multiple Resources: 4520 4521* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4522* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4523* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4524* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4525* [Citation](citation.html): A use context assigned to the citation 4526* [CodeSystem](codesystem.html): A use context assigned to the code system 4527* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4528* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4529* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4530* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4531* [Evidence](evidence.html): A use context assigned to the evidence 4532* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4533* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4534* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4535* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4536* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4537* [Library](library.html): A use context assigned to the library 4538* [Measure](measure.html): A use context assigned to the measure 4539* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4540* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4541* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4542* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4543* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4544* [Requirements](requirements.html): A use context assigned to the requirements 4545* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4546* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4547* [StructureMap](structuremap.html): A use context assigned to the structure map 4548* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4549* [TestScript](testscript.html): A use context assigned to the test script 4550* [ValueSet](valueset.html): A use context assigned to the value set 4551</b><br> 4552 * Type: <b>token</b><br> 4553 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4554 * </p> 4555 */ 4556 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4557 4558 /** 4559 * Search parameter: <b>date</b> 4560 * <p> 4561 * Description: <b>Multiple Resources: 4562 4563* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4564* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4565* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4566* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4567* [Citation](citation.html): The citation publication date 4568* [CodeSystem](codesystem.html): The code system publication date 4569* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4570* [ConceptMap](conceptmap.html): The concept map publication date 4571* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4572* [EventDefinition](eventdefinition.html): The event definition publication date 4573* [Evidence](evidence.html): The evidence publication date 4574* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4575* [ExampleScenario](examplescenario.html): The example scenario publication date 4576* [GraphDefinition](graphdefinition.html): The graph definition publication date 4577* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4578* [Library](library.html): The library publication date 4579* [Measure](measure.html): The measure publication date 4580* [MessageDefinition](messagedefinition.html): The message definition publication date 4581* [NamingSystem](namingsystem.html): The naming system publication date 4582* [OperationDefinition](operationdefinition.html): The operation definition publication date 4583* [PlanDefinition](plandefinition.html): The plan definition publication date 4584* [Questionnaire](questionnaire.html): The questionnaire publication date 4585* [Requirements](requirements.html): The requirements publication date 4586* [SearchParameter](searchparameter.html): The search parameter publication date 4587* [StructureDefinition](structuredefinition.html): The structure definition publication date 4588* [StructureMap](structuremap.html): The structure map publication date 4589* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4590* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4591* [TestScript](testscript.html): The test script publication date 4592* [ValueSet](valueset.html): The value set publication date 4593</b><br> 4594 * Type: <b>date</b><br> 4595 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4596 * </p> 4597 */ 4598 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 4599 public static final String SP_DATE = "date"; 4600 /** 4601 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4602 * <p> 4603 * Description: <b>Multiple Resources: 4604 4605* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4606* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4607* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4608* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4609* [Citation](citation.html): The citation publication date 4610* [CodeSystem](codesystem.html): The code system publication date 4611* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4612* [ConceptMap](conceptmap.html): The concept map publication date 4613* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4614* [EventDefinition](eventdefinition.html): The event definition publication date 4615* [Evidence](evidence.html): The evidence publication date 4616* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4617* [ExampleScenario](examplescenario.html): The example scenario publication date 4618* [GraphDefinition](graphdefinition.html): The graph definition publication date 4619* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4620* [Library](library.html): The library publication date 4621* [Measure](measure.html): The measure publication date 4622* [MessageDefinition](messagedefinition.html): The message definition publication date 4623* [NamingSystem](namingsystem.html): The naming system publication date 4624* [OperationDefinition](operationdefinition.html): The operation definition publication date 4625* [PlanDefinition](plandefinition.html): The plan definition publication date 4626* [Questionnaire](questionnaire.html): The questionnaire publication date 4627* [Requirements](requirements.html): The requirements publication date 4628* [SearchParameter](searchparameter.html): The search parameter publication date 4629* [StructureDefinition](structuredefinition.html): The structure definition publication date 4630* [StructureMap](structuremap.html): The structure map publication date 4631* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4632* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4633* [TestScript](testscript.html): The test script publication date 4634* [ValueSet](valueset.html): The value set publication date 4635</b><br> 4636 * Type: <b>date</b><br> 4637 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4638 * </p> 4639 */ 4640 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4641 4642 /** 4643 * Search parameter: <b>description</b> 4644 * <p> 4645 * Description: <b>Multiple Resources: 4646 4647* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4648* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4649* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4650* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4651* [Citation](citation.html): The description of the citation 4652* [CodeSystem](codesystem.html): The description of the code system 4653* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4654* [ConceptMap](conceptmap.html): The description of the concept map 4655* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4656* [EventDefinition](eventdefinition.html): The description of the event definition 4657* [Evidence](evidence.html): The description of the evidence 4658* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4659* [GraphDefinition](graphdefinition.html): The description of the graph definition 4660* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4661* [Library](library.html): The description of the library 4662* [Measure](measure.html): The description of the measure 4663* [MessageDefinition](messagedefinition.html): The description of the message definition 4664* [NamingSystem](namingsystem.html): The description of the naming system 4665* [OperationDefinition](operationdefinition.html): The description of the operation definition 4666* [PlanDefinition](plandefinition.html): The description of the plan definition 4667* [Questionnaire](questionnaire.html): The description of the questionnaire 4668* [Requirements](requirements.html): The description of the requirements 4669* [SearchParameter](searchparameter.html): The description of the search parameter 4670* [StructureDefinition](structuredefinition.html): The description of the structure definition 4671* [StructureMap](structuremap.html): The description of the structure map 4672* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4673* [TestScript](testscript.html): The description of the test script 4674* [ValueSet](valueset.html): The description of the value set 4675</b><br> 4676 * Type: <b>string</b><br> 4677 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4678 * </p> 4679 */ 4680 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 4681 public static final String SP_DESCRIPTION = "description"; 4682 /** 4683 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4684 * <p> 4685 * Description: <b>Multiple Resources: 4686 4687* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4688* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4689* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4690* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4691* [Citation](citation.html): The description of the citation 4692* [CodeSystem](codesystem.html): The description of the code system 4693* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4694* [ConceptMap](conceptmap.html): The description of the concept map 4695* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4696* [EventDefinition](eventdefinition.html): The description of the event definition 4697* [Evidence](evidence.html): The description of the evidence 4698* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4699* [GraphDefinition](graphdefinition.html): The description of the graph definition 4700* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4701* [Library](library.html): The description of the library 4702* [Measure](measure.html): The description of the measure 4703* [MessageDefinition](messagedefinition.html): The description of the message definition 4704* [NamingSystem](namingsystem.html): The description of the naming system 4705* [OperationDefinition](operationdefinition.html): The description of the operation definition 4706* [PlanDefinition](plandefinition.html): The description of the plan definition 4707* [Questionnaire](questionnaire.html): The description of the questionnaire 4708* [Requirements](requirements.html): The description of the requirements 4709* [SearchParameter](searchparameter.html): The description of the search parameter 4710* [StructureDefinition](structuredefinition.html): The description of the structure definition 4711* [StructureMap](structuremap.html): The description of the structure map 4712* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4713* [TestScript](testscript.html): The description of the test script 4714* [ValueSet](valueset.html): The description of the value set 4715</b><br> 4716 * Type: <b>string</b><br> 4717 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4718 * </p> 4719 */ 4720 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4721 4722 /** 4723 * Search parameter: <b>identifier</b> 4724 * <p> 4725 * Description: <b>Multiple Resources: 4726 4727* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4728* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4729* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4730* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4731* [Citation](citation.html): External identifier for the citation 4732* [CodeSystem](codesystem.html): External identifier for the code system 4733* [ConceptMap](conceptmap.html): External identifier for the concept map 4734* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4735* [EventDefinition](eventdefinition.html): External identifier for the event definition 4736* [Evidence](evidence.html): External identifier for the evidence 4737* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4738* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4739* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4740* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4741* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4742* [Library](library.html): External identifier for the library 4743* [Measure](measure.html): External identifier for the measure 4744* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4745* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4746* [NamingSystem](namingsystem.html): External identifier for the naming system 4747* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4748* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4749* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4750* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4751* [Requirements](requirements.html): External identifier for the requirements 4752* [SearchParameter](searchparameter.html): External identifier for the search parameter 4753* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4754* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4755* [StructureMap](structuremap.html): External identifier for the structure map 4756* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4757* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4758* [TestPlan](testplan.html): An identifier for the test plan 4759* [TestScript](testscript.html): External identifier for the test script 4760* [ValueSet](valueset.html): External identifier for the value set 4761</b><br> 4762 * Type: <b>token</b><br> 4763 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4764 * </p> 4765 */ 4766 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4767 public static final String SP_IDENTIFIER = "identifier"; 4768 /** 4769 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4770 * <p> 4771 * Description: <b>Multiple Resources: 4772 4773* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4774* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4775* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4776* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4777* [Citation](citation.html): External identifier for the citation 4778* [CodeSystem](codesystem.html): External identifier for the code system 4779* [ConceptMap](conceptmap.html): External identifier for the concept map 4780* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4781* [EventDefinition](eventdefinition.html): External identifier for the event definition 4782* [Evidence](evidence.html): External identifier for the evidence 4783* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4784* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4785* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4786* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4787* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4788* [Library](library.html): External identifier for the library 4789* [Measure](measure.html): External identifier for the measure 4790* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4791* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4792* [NamingSystem](namingsystem.html): External identifier for the naming system 4793* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4794* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4795* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4796* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4797* [Requirements](requirements.html): External identifier for the requirements 4798* [SearchParameter](searchparameter.html): External identifier for the search parameter 4799* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4800* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4801* [StructureMap](structuremap.html): External identifier for the structure map 4802* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4803* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4804* [TestPlan](testplan.html): An identifier for the test plan 4805* [TestScript](testscript.html): External identifier for the test script 4806* [ValueSet](valueset.html): External identifier for the value set 4807</b><br> 4808 * Type: <b>token</b><br> 4809 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4810 * </p> 4811 */ 4812 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4813 4814 /** 4815 * Search parameter: <b>jurisdiction</b> 4816 * <p> 4817 * Description: <b>Multiple Resources: 4818 4819* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4820* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4821* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4822* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4823* [Citation](citation.html): Intended jurisdiction for the citation 4824* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4825* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4826* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4827* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4828* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4829* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4830* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4831* [Library](library.html): Intended jurisdiction for the library 4832* [Measure](measure.html): Intended jurisdiction for the measure 4833* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4834* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4835* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4836* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4837* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4838* [Requirements](requirements.html): Intended jurisdiction for the requirements 4839* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4840* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4841* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4842* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4843* [TestScript](testscript.html): Intended jurisdiction for the test script 4844* [ValueSet](valueset.html): Intended jurisdiction for the value set 4845</b><br> 4846 * Type: <b>token</b><br> 4847 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4848 * </p> 4849 */ 4850 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 4851 public static final String SP_JURISDICTION = "jurisdiction"; 4852 /** 4853 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4854 * <p> 4855 * Description: <b>Multiple Resources: 4856 4857* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4858* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4859* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4860* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4861* [Citation](citation.html): Intended jurisdiction for the citation 4862* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4863* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4864* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4865* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4866* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4867* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4868* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4869* [Library](library.html): Intended jurisdiction for the library 4870* [Measure](measure.html): Intended jurisdiction for the measure 4871* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4872* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4873* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4874* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4875* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4876* [Requirements](requirements.html): Intended jurisdiction for the requirements 4877* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4878* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4879* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4880* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4881* [TestScript](testscript.html): Intended jurisdiction for the test script 4882* [ValueSet](valueset.html): Intended jurisdiction for the value set 4883</b><br> 4884 * Type: <b>token</b><br> 4885 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4886 * </p> 4887 */ 4888 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4889 4890 /** 4891 * Search parameter: <b>name</b> 4892 * <p> 4893 * Description: <b>Multiple Resources: 4894 4895* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4896* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4897* [Citation](citation.html): Computationally friendly name of the citation 4898* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4899* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4900* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4901* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4902* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4903* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4904* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4905* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4906* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4907* [Library](library.html): Computationally friendly name of the library 4908* [Measure](measure.html): Computationally friendly name of the measure 4909* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4910* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4911* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4912* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4913* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4914* [Requirements](requirements.html): Computationally friendly name of the requirements 4915* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4916* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4917* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4918* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4919* [TestScript](testscript.html): Computationally friendly name of the test script 4920* [ValueSet](valueset.html): Computationally friendly name of the value set 4921</b><br> 4922 * Type: <b>string</b><br> 4923 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4924 * </p> 4925 */ 4926 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 4927 public static final String SP_NAME = "name"; 4928 /** 4929 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4930 * <p> 4931 * Description: <b>Multiple Resources: 4932 4933* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4934* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4935* [Citation](citation.html): Computationally friendly name of the citation 4936* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4937* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4938* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4939* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4940* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4941* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4942* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4943* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4944* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4945* [Library](library.html): Computationally friendly name of the library 4946* [Measure](measure.html): Computationally friendly name of the measure 4947* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4948* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4949* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4950* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4951* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4952* [Requirements](requirements.html): Computationally friendly name of the requirements 4953* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4954* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4955* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4956* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4957* [TestScript](testscript.html): Computationally friendly name of the test script 4958* [ValueSet](valueset.html): Computationally friendly name of the value set 4959</b><br> 4960 * Type: <b>string</b><br> 4961 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4962 * </p> 4963 */ 4964 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4965 4966 /** 4967 * Search parameter: <b>publisher</b> 4968 * <p> 4969 * Description: <b>Multiple Resources: 4970 4971* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4972* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4973* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4974* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4975* [Citation](citation.html): Name of the publisher of the citation 4976* [CodeSystem](codesystem.html): Name of the publisher of the code system 4977* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4978* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4979* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4980* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4981* [Evidence](evidence.html): Name of the publisher of the evidence 4982* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4983* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4984* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4985* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4986* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4987* [Library](library.html): Name of the publisher of the library 4988* [Measure](measure.html): Name of the publisher of the measure 4989* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4990* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4991* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4992* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4993* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4994* [Requirements](requirements.html): Name of the publisher of the requirements 4995* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4996* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4997* [StructureMap](structuremap.html): Name of the publisher of the structure map 4998* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4999* [TestScript](testscript.html): Name of the publisher of the test script 5000* [ValueSet](valueset.html): Name of the publisher of the value set 5001</b><br> 5002 * Type: <b>string</b><br> 5003 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5004 * </p> 5005 */ 5006 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 5007 public static final String SP_PUBLISHER = "publisher"; 5008 /** 5009 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5010 * <p> 5011 * Description: <b>Multiple Resources: 5012 5013* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5014* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5015* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5016* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5017* [Citation](citation.html): Name of the publisher of the citation 5018* [CodeSystem](codesystem.html): Name of the publisher of the code system 5019* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5020* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5021* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5022* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5023* [Evidence](evidence.html): Name of the publisher of the evidence 5024* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5025* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5026* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5027* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5028* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5029* [Library](library.html): Name of the publisher of the library 5030* [Measure](measure.html): Name of the publisher of the measure 5031* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5032* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5033* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5034* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5035* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5036* [Requirements](requirements.html): Name of the publisher of the requirements 5037* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5038* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5039* [StructureMap](structuremap.html): Name of the publisher of the structure map 5040* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5041* [TestScript](testscript.html): Name of the publisher of the test script 5042* [ValueSet](valueset.html): Name of the publisher of the value set 5043</b><br> 5044 * Type: <b>string</b><br> 5045 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5046 * </p> 5047 */ 5048 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5049 5050 /** 5051 * Search parameter: <b>status</b> 5052 * <p> 5053 * Description: <b>Multiple Resources: 5054 5055* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5056* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5057* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5058* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5059* [Citation](citation.html): The current status of the citation 5060* [CodeSystem](codesystem.html): The current status of the code system 5061* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5062* [ConceptMap](conceptmap.html): The current status of the concept map 5063* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5064* [EventDefinition](eventdefinition.html): The current status of the event definition 5065* [Evidence](evidence.html): The current status of the evidence 5066* [EvidenceReport](evidencereport.html): The current status of the evidence report 5067* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5068* [ExampleScenario](examplescenario.html): The current status of the example scenario 5069* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5070* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5071* [Library](library.html): The current status of the library 5072* [Measure](measure.html): The current status of the measure 5073* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5074* [MessageDefinition](messagedefinition.html): The current status of the message definition 5075* [NamingSystem](namingsystem.html): The current status of the naming system 5076* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5077* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5078* [PlanDefinition](plandefinition.html): The current status of the plan definition 5079* [Questionnaire](questionnaire.html): The current status of the questionnaire 5080* [Requirements](requirements.html): The current status of the requirements 5081* [SearchParameter](searchparameter.html): The current status of the search parameter 5082* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5083* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5084* [StructureMap](structuremap.html): The current status of the structure map 5085* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5086* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5087* [TestPlan](testplan.html): The current status of the test plan 5088* [TestScript](testscript.html): The current status of the test script 5089* [ValueSet](valueset.html): The current status of the value set 5090</b><br> 5091 * Type: <b>token</b><br> 5092 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5093 * </p> 5094 */ 5095 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 5096 public static final String SP_STATUS = "status"; 5097 /** 5098 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5099 * <p> 5100 * Description: <b>Multiple Resources: 5101 5102* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5103* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5104* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5105* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5106* [Citation](citation.html): The current status of the citation 5107* [CodeSystem](codesystem.html): The current status of the code system 5108* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5109* [ConceptMap](conceptmap.html): The current status of the concept map 5110* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5111* [EventDefinition](eventdefinition.html): The current status of the event definition 5112* [Evidence](evidence.html): The current status of the evidence 5113* [EvidenceReport](evidencereport.html): The current status of the evidence report 5114* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5115* [ExampleScenario](examplescenario.html): The current status of the example scenario 5116* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5117* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5118* [Library](library.html): The current status of the library 5119* [Measure](measure.html): The current status of the measure 5120* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5121* [MessageDefinition](messagedefinition.html): The current status of the message definition 5122* [NamingSystem](namingsystem.html): The current status of the naming system 5123* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5124* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5125* [PlanDefinition](plandefinition.html): The current status of the plan definition 5126* [Questionnaire](questionnaire.html): The current status of the questionnaire 5127* [Requirements](requirements.html): The current status of the requirements 5128* [SearchParameter](searchparameter.html): The current status of the search parameter 5129* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5130* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5131* [StructureMap](structuremap.html): The current status of the structure map 5132* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5133* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5134* [TestPlan](testplan.html): The current status of the test plan 5135* [TestScript](testscript.html): The current status of the test script 5136* [ValueSet](valueset.html): The current status of the value set 5137</b><br> 5138 * Type: <b>token</b><br> 5139 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5140 * </p> 5141 */ 5142 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5143 5144 /** 5145 * Search parameter: <b>title</b> 5146 * <p> 5147 * Description: <b>Multiple Resources: 5148 5149* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 5150* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 5151* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 5152* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 5153* [Citation](citation.html): The human-friendly name of the citation 5154* [CodeSystem](codesystem.html): The human-friendly name of the code system 5155* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 5156* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 5157* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 5158* [Evidence](evidence.html): The human-friendly name of the evidence 5159* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 5160* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 5161* [Library](library.html): The human-friendly name of the library 5162* [Measure](measure.html): The human-friendly name of the measure 5163* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 5164* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 5165* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 5166* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 5167* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 5168* [Requirements](requirements.html): The human-friendly name of the requirements 5169* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 5170* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 5171* [StructureMap](structuremap.html): The human-friendly name of the structure map 5172* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 5173* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 5174* [TestScript](testscript.html): The human-friendly name of the test script 5175* [ValueSet](valueset.html): The human-friendly name of the value set 5176</b><br> 5177 * Type: <b>string</b><br> 5178 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 5179 * </p> 5180 */ 5181 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 5182 public static final String SP_TITLE = "title"; 5183 /** 5184 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5185 * <p> 5186 * Description: <b>Multiple Resources: 5187 5188* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 5189* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 5190* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 5191* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 5192* [Citation](citation.html): The human-friendly name of the citation 5193* [CodeSystem](codesystem.html): The human-friendly name of the code system 5194* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 5195* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 5196* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 5197* [Evidence](evidence.html): The human-friendly name of the evidence 5198* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 5199* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 5200* [Library](library.html): The human-friendly name of the library 5201* [Measure](measure.html): The human-friendly name of the measure 5202* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 5203* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 5204* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 5205* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 5206* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 5207* [Requirements](requirements.html): The human-friendly name of the requirements 5208* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 5209* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 5210* [StructureMap](structuremap.html): The human-friendly name of the structure map 5211* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 5212* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 5213* [TestScript](testscript.html): The human-friendly name of the test script 5214* [ValueSet](valueset.html): The human-friendly name of the value set 5215</b><br> 5216 * Type: <b>string</b><br> 5217 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 5218 * </p> 5219 */ 5220 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 5221 5222 /** 5223 * Search parameter: <b>url</b> 5224 * <p> 5225 * Description: <b>Multiple Resources: 5226 5227* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5228* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5229* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5230* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5231* [Citation](citation.html): The uri that identifies the citation 5232* [CodeSystem](codesystem.html): The uri that identifies the code system 5233* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5234* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5235* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5236* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5237* [Evidence](evidence.html): The uri that identifies the evidence 5238* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5239* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5240* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5241* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5242* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5243* [Library](library.html): The uri that identifies the library 5244* [Measure](measure.html): The uri that identifies the measure 5245* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5246* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5247* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5248* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5249* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5250* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5251* [Requirements](requirements.html): The uri that identifies the requirements 5252* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5253* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5254* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5255* [StructureMap](structuremap.html): The uri that identifies the structure map 5256* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5257* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5258* [TestPlan](testplan.html): The uri that identifies the test plan 5259* [TestScript](testscript.html): The uri that identifies the test script 5260* [ValueSet](valueset.html): The uri that identifies the value set 5261</b><br> 5262 * Type: <b>uri</b><br> 5263 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5264 * </p> 5265 */ 5266 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 5267 public static final String SP_URL = "url"; 5268 /** 5269 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5270 * <p> 5271 * Description: <b>Multiple Resources: 5272 5273* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5274* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5275* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5276* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5277* [Citation](citation.html): The uri that identifies the citation 5278* [CodeSystem](codesystem.html): The uri that identifies the code system 5279* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5280* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5281* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5282* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5283* [Evidence](evidence.html): The uri that identifies the evidence 5284* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5285* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5286* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5287* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5288* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5289* [Library](library.html): The uri that identifies the library 5290* [Measure](measure.html): The uri that identifies the measure 5291* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5292* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5293* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5294* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5295* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5296* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5297* [Requirements](requirements.html): The uri that identifies the requirements 5298* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5299* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5300* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5301* [StructureMap](structuremap.html): The uri that identifies the structure map 5302* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5303* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5304* [TestPlan](testplan.html): The uri that identifies the test plan 5305* [TestScript](testscript.html): The uri that identifies the test script 5306* [ValueSet](valueset.html): The uri that identifies the value set 5307</b><br> 5308 * Type: <b>uri</b><br> 5309 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5310 * </p> 5311 */ 5312 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5313 5314 /** 5315 * Search parameter: <b>version</b> 5316 * <p> 5317 * Description: <b>Multiple Resources: 5318 5319* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5320* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5321* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5322* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5323* [Citation](citation.html): The business version of the citation 5324* [CodeSystem](codesystem.html): The business version of the code system 5325* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5326* [ConceptMap](conceptmap.html): The business version of the concept map 5327* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5328* [EventDefinition](eventdefinition.html): The business version of the event definition 5329* [Evidence](evidence.html): The business version of the evidence 5330* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5331* [ExampleScenario](examplescenario.html): The business version of the example scenario 5332* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5333* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5334* [Library](library.html): The business version of the library 5335* [Measure](measure.html): The business version of the measure 5336* [MessageDefinition](messagedefinition.html): The business version of the message definition 5337* [NamingSystem](namingsystem.html): The business version of the naming system 5338* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5339* [PlanDefinition](plandefinition.html): The business version of the plan definition 5340* [Questionnaire](questionnaire.html): The business version of the questionnaire 5341* [Requirements](requirements.html): The business version of the requirements 5342* [SearchParameter](searchparameter.html): The business version of the search parameter 5343* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5344* [StructureMap](structuremap.html): The business version of the structure map 5345* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5346* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5347* [TestScript](testscript.html): The business version of the test script 5348* [ValueSet](valueset.html): The business version of the value set 5349</b><br> 5350 * Type: <b>token</b><br> 5351 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5352 * </p> 5353 */ 5354 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 5355 public static final String SP_VERSION = "version"; 5356 /** 5357 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5358 * <p> 5359 * Description: <b>Multiple Resources: 5360 5361* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5362* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5363* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5364* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5365* [Citation](citation.html): The business version of the citation 5366* [CodeSystem](codesystem.html): The business version of the code system 5367* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5368* [ConceptMap](conceptmap.html): The business version of the concept map 5369* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5370* [EventDefinition](eventdefinition.html): The business version of the event definition 5371* [Evidence](evidence.html): The business version of the evidence 5372* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5373* [ExampleScenario](examplescenario.html): The business version of the example scenario 5374* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5375* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5376* [Library](library.html): The business version of the library 5377* [Measure](measure.html): The business version of the measure 5378* [MessageDefinition](messagedefinition.html): The business version of the message definition 5379* [NamingSystem](namingsystem.html): The business version of the naming system 5380* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5381* [PlanDefinition](plandefinition.html): The business version of the plan definition 5382* [Questionnaire](questionnaire.html): The business version of the questionnaire 5383* [Requirements](requirements.html): The business version of the requirements 5384* [SearchParameter](searchparameter.html): The business version of the search parameter 5385* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5386* [StructureMap](structuremap.html): The business version of the structure map 5387* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5388* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5389* [TestScript](testscript.html): The business version of the test script 5390* [ValueSet](valueset.html): The business version of the value set 5391</b><br> 5392 * Type: <b>token</b><br> 5393 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5394 * </p> 5395 */ 5396 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5397 5398 5399} 5400