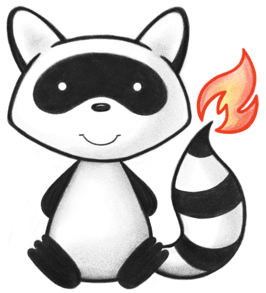
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A definition of a condition and information relevant to managing it. 052 */ 053@ResourceDef(name="ConditionDefinition", profile="http://hl7.org/fhir/StructureDefinition/ConditionDefinition") 054public class ConditionDefinition extends MetadataResource { 055 056 public enum ConditionPreconditionType { 057 /** 058 * The observation is very sensitive for the condition, but may also indicate other conditions. 059 */ 060 SENSITIVE, 061 /** 062 * The observation is very specific for this condition, but not particularly sensitive. 063 */ 064 SPECIFIC, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static ConditionPreconditionType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("sensitive".equals(codeString)) 073 return SENSITIVE; 074 if ("specific".equals(codeString)) 075 return SPECIFIC; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown ConditionPreconditionType code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case SENSITIVE: return "sensitive"; 084 case SPECIFIC: return "specific"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case SENSITIVE: return "http://hl7.org/fhir/condition-precondition-type"; 092 case SPECIFIC: return "http://hl7.org/fhir/condition-precondition-type"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case SENSITIVE: return "The observation is very sensitive for the condition, but may also indicate other conditions."; 100 case SPECIFIC: return "The observation is very specific for this condition, but not particularly sensitive."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case SENSITIVE: return "Sensitive"; 108 case SPECIFIC: return "Specific"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class ConditionPreconditionTypeEnumFactory implements EnumFactory<ConditionPreconditionType> { 116 public ConditionPreconditionType fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("sensitive".equals(codeString)) 121 return ConditionPreconditionType.SENSITIVE; 122 if ("specific".equals(codeString)) 123 return ConditionPreconditionType.SPECIFIC; 124 throw new IllegalArgumentException("Unknown ConditionPreconditionType code '"+codeString+"'"); 125 } 126 public Enumeration<ConditionPreconditionType> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.NULL, code); 134 if ("sensitive".equals(codeString)) 135 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.SENSITIVE, code); 136 if ("specific".equals(codeString)) 137 return new Enumeration<ConditionPreconditionType>(this, ConditionPreconditionType.SPECIFIC, code); 138 throw new FHIRException("Unknown ConditionPreconditionType code '"+codeString+"'"); 139 } 140 public String toCode(ConditionPreconditionType code) { 141 if (code == ConditionPreconditionType.NULL) 142 return null; 143 if (code == ConditionPreconditionType.SENSITIVE) 144 return "sensitive"; 145 if (code == ConditionPreconditionType.SPECIFIC) 146 return "specific"; 147 return "?"; 148 } 149 public String toSystem(ConditionPreconditionType code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum ConditionQuestionnairePurpose { 155 /** 156 * A pre-admit questionnaire. 157 */ 158 PREADMIT, 159 /** 160 * A questionnaire that helps with diferential diagnosis. 161 */ 162 DIFFDIAGNOSIS, 163 /** 164 * A questionnaire to check on outcomes for the patient. 165 */ 166 OUTCOME, 167 /** 168 * added to help the parsers with the generic types 169 */ 170 NULL; 171 public static ConditionQuestionnairePurpose fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("preadmit".equals(codeString)) 175 return PREADMIT; 176 if ("diff-diagnosis".equals(codeString)) 177 return DIFFDIAGNOSIS; 178 if ("outcome".equals(codeString)) 179 return OUTCOME; 180 if (Configuration.isAcceptInvalidEnums()) 181 return null; 182 else 183 throw new FHIRException("Unknown ConditionQuestionnairePurpose code '"+codeString+"'"); 184 } 185 public String toCode() { 186 switch (this) { 187 case PREADMIT: return "preadmit"; 188 case DIFFDIAGNOSIS: return "diff-diagnosis"; 189 case OUTCOME: return "outcome"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case PREADMIT: return "http://hl7.org/fhir/condition-questionnaire-purpose"; 197 case DIFFDIAGNOSIS: return "http://hl7.org/fhir/condition-questionnaire-purpose"; 198 case OUTCOME: return "http://hl7.org/fhir/condition-questionnaire-purpose"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getDefinition() { 204 switch (this) { 205 case PREADMIT: return "A pre-admit questionnaire."; 206 case DIFFDIAGNOSIS: return "A questionnaire that helps with diferential diagnosis."; 207 case OUTCOME: return "A questionnaire to check on outcomes for the patient."; 208 case NULL: return null; 209 default: return "?"; 210 } 211 } 212 public String getDisplay() { 213 switch (this) { 214 case PREADMIT: return "Pre-admit"; 215 case DIFFDIAGNOSIS: return "Diff Diagnosis"; 216 case OUTCOME: return "Outcome"; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 } 222 223 public static class ConditionQuestionnairePurposeEnumFactory implements EnumFactory<ConditionQuestionnairePurpose> { 224 public ConditionQuestionnairePurpose fromCode(String codeString) throws IllegalArgumentException { 225 if (codeString == null || "".equals(codeString)) 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("preadmit".equals(codeString)) 229 return ConditionQuestionnairePurpose.PREADMIT; 230 if ("diff-diagnosis".equals(codeString)) 231 return ConditionQuestionnairePurpose.DIFFDIAGNOSIS; 232 if ("outcome".equals(codeString)) 233 return ConditionQuestionnairePurpose.OUTCOME; 234 throw new IllegalArgumentException("Unknown ConditionQuestionnairePurpose code '"+codeString+"'"); 235 } 236 public Enumeration<ConditionQuestionnairePurpose> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.NULL, code); 241 String codeString = ((PrimitiveType) code).asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.NULL, code); 244 if ("preadmit".equals(codeString)) 245 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.PREADMIT, code); 246 if ("diff-diagnosis".equals(codeString)) 247 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.DIFFDIAGNOSIS, code); 248 if ("outcome".equals(codeString)) 249 return new Enumeration<ConditionQuestionnairePurpose>(this, ConditionQuestionnairePurpose.OUTCOME, code); 250 throw new FHIRException("Unknown ConditionQuestionnairePurpose code '"+codeString+"'"); 251 } 252 public String toCode(ConditionQuestionnairePurpose code) { 253 if (code == ConditionQuestionnairePurpose.NULL) 254 return null; 255 if (code == ConditionQuestionnairePurpose.PREADMIT) 256 return "preadmit"; 257 if (code == ConditionQuestionnairePurpose.DIFFDIAGNOSIS) 258 return "diff-diagnosis"; 259 if (code == ConditionQuestionnairePurpose.OUTCOME) 260 return "outcome"; 261 return "?"; 262 } 263 public String toSystem(ConditionQuestionnairePurpose code) { 264 return code.getSystem(); 265 } 266 } 267 268 @Block() 269 public static class ConditionDefinitionObservationComponent extends BackboneElement implements IBaseBackboneElement { 270 /** 271 * Category that is relevant. 272 */ 273 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 274 @Description(shortDefinition="Category that is relevant", formalDefinition="Category that is relevant." ) 275 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-category") 276 protected CodeableConcept category; 277 278 /** 279 * Code for relevant Observation. 280 */ 281 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 282 @Description(shortDefinition="Code for relevant Observation", formalDefinition="Code for relevant Observation." ) 283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 284 protected CodeableConcept code; 285 286 private static final long serialVersionUID = -1433986479L; 287 288 /** 289 * Constructor 290 */ 291 public ConditionDefinitionObservationComponent() { 292 super(); 293 } 294 295 /** 296 * @return {@link #category} (Category that is relevant.) 297 */ 298 public CodeableConcept getCategory() { 299 if (this.category == null) 300 if (Configuration.errorOnAutoCreate()) 301 throw new Error("Attempt to auto-create ConditionDefinitionObservationComponent.category"); 302 else if (Configuration.doAutoCreate()) 303 this.category = new CodeableConcept(); // cc 304 return this.category; 305 } 306 307 public boolean hasCategory() { 308 return this.category != null && !this.category.isEmpty(); 309 } 310 311 /** 312 * @param value {@link #category} (Category that is relevant.) 313 */ 314 public ConditionDefinitionObservationComponent setCategory(CodeableConcept value) { 315 this.category = value; 316 return this; 317 } 318 319 /** 320 * @return {@link #code} (Code for relevant Observation.) 321 */ 322 public CodeableConcept getCode() { 323 if (this.code == null) 324 if (Configuration.errorOnAutoCreate()) 325 throw new Error("Attempt to auto-create ConditionDefinitionObservationComponent.code"); 326 else if (Configuration.doAutoCreate()) 327 this.code = new CodeableConcept(); // cc 328 return this.code; 329 } 330 331 public boolean hasCode() { 332 return this.code != null && !this.code.isEmpty(); 333 } 334 335 /** 336 * @param value {@link #code} (Code for relevant Observation.) 337 */ 338 public ConditionDefinitionObservationComponent setCode(CodeableConcept value) { 339 this.code = value; 340 return this; 341 } 342 343 protected void listChildren(List<Property> children) { 344 super.listChildren(children); 345 children.add(new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category)); 346 children.add(new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code)); 347 } 348 349 @Override 350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 351 switch (_hash) { 352 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category); 353 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code); 354 default: return super.getNamedProperty(_hash, _name, _checkValid); 355 } 356 357 } 358 359 @Override 360 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 361 switch (hash) { 362 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 363 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 364 default: return super.getProperty(hash, name, checkValid); 365 } 366 367 } 368 369 @Override 370 public Base setProperty(int hash, String name, Base value) throws FHIRException { 371 switch (hash) { 372 case 50511102: // category 373 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 374 return value; 375 case 3059181: // code 376 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 377 return value; 378 default: return super.setProperty(hash, name, value); 379 } 380 381 } 382 383 @Override 384 public Base setProperty(String name, Base value) throws FHIRException { 385 if (name.equals("category")) { 386 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 387 } else if (name.equals("code")) { 388 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 389 } else 390 return super.setProperty(name, value); 391 return value; 392 } 393 394 @Override 395 public void removeChild(String name, Base value) throws FHIRException { 396 if (name.equals("category")) { 397 this.category = null; 398 } else if (name.equals("code")) { 399 this.code = null; 400 } else 401 super.removeChild(name, value); 402 403 } 404 405 @Override 406 public Base makeProperty(int hash, String name) throws FHIRException { 407 switch (hash) { 408 case 50511102: return getCategory(); 409 case 3059181: return getCode(); 410 default: return super.makeProperty(hash, name); 411 } 412 413 } 414 415 @Override 416 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 417 switch (hash) { 418 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 419 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 420 default: return super.getTypesForProperty(hash, name); 421 } 422 423 } 424 425 @Override 426 public Base addChild(String name) throws FHIRException { 427 if (name.equals("category")) { 428 this.category = new CodeableConcept(); 429 return this.category; 430 } 431 else if (name.equals("code")) { 432 this.code = new CodeableConcept(); 433 return this.code; 434 } 435 else 436 return super.addChild(name); 437 } 438 439 public ConditionDefinitionObservationComponent copy() { 440 ConditionDefinitionObservationComponent dst = new ConditionDefinitionObservationComponent(); 441 copyValues(dst); 442 return dst; 443 } 444 445 public void copyValues(ConditionDefinitionObservationComponent dst) { 446 super.copyValues(dst); 447 dst.category = category == null ? null : category.copy(); 448 dst.code = code == null ? null : code.copy(); 449 } 450 451 @Override 452 public boolean equalsDeep(Base other_) { 453 if (!super.equalsDeep(other_)) 454 return false; 455 if (!(other_ instanceof ConditionDefinitionObservationComponent)) 456 return false; 457 ConditionDefinitionObservationComponent o = (ConditionDefinitionObservationComponent) other_; 458 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true); 459 } 460 461 @Override 462 public boolean equalsShallow(Base other_) { 463 if (!super.equalsShallow(other_)) 464 return false; 465 if (!(other_ instanceof ConditionDefinitionObservationComponent)) 466 return false; 467 ConditionDefinitionObservationComponent o = (ConditionDefinitionObservationComponent) other_; 468 return true; 469 } 470 471 public boolean isEmpty() { 472 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, code); 473 } 474 475 public String fhirType() { 476 return "ConditionDefinition.observation"; 477 478 } 479 480 } 481 482 @Block() 483 public static class ConditionDefinitionMedicationComponent extends BackboneElement implements IBaseBackboneElement { 484 /** 485 * Category that is relevant. 486 */ 487 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 488 @Description(shortDefinition="Category that is relevant", formalDefinition="Category that is relevant." ) 489 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/medicationrequest-category") 490 protected CodeableConcept category; 491 492 /** 493 * Code for relevant Medication. 494 */ 495 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 496 @Description(shortDefinition="Code for relevant Medication", formalDefinition="Code for relevant Medication." ) 497 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 498 protected CodeableConcept code; 499 500 private static final long serialVersionUID = -1433986479L; 501 502 /** 503 * Constructor 504 */ 505 public ConditionDefinitionMedicationComponent() { 506 super(); 507 } 508 509 /** 510 * @return {@link #category} (Category that is relevant.) 511 */ 512 public CodeableConcept getCategory() { 513 if (this.category == null) 514 if (Configuration.errorOnAutoCreate()) 515 throw new Error("Attempt to auto-create ConditionDefinitionMedicationComponent.category"); 516 else if (Configuration.doAutoCreate()) 517 this.category = new CodeableConcept(); // cc 518 return this.category; 519 } 520 521 public boolean hasCategory() { 522 return this.category != null && !this.category.isEmpty(); 523 } 524 525 /** 526 * @param value {@link #category} (Category that is relevant.) 527 */ 528 public ConditionDefinitionMedicationComponent setCategory(CodeableConcept value) { 529 this.category = value; 530 return this; 531 } 532 533 /** 534 * @return {@link #code} (Code for relevant Medication.) 535 */ 536 public CodeableConcept getCode() { 537 if (this.code == null) 538 if (Configuration.errorOnAutoCreate()) 539 throw new Error("Attempt to auto-create ConditionDefinitionMedicationComponent.code"); 540 else if (Configuration.doAutoCreate()) 541 this.code = new CodeableConcept(); // cc 542 return this.code; 543 } 544 545 public boolean hasCode() { 546 return this.code != null && !this.code.isEmpty(); 547 } 548 549 /** 550 * @param value {@link #code} (Code for relevant Medication.) 551 */ 552 public ConditionDefinitionMedicationComponent setCode(CodeableConcept value) { 553 this.code = value; 554 return this; 555 } 556 557 protected void listChildren(List<Property> children) { 558 super.listChildren(children); 559 children.add(new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category)); 560 children.add(new Property("code", "CodeableConcept", "Code for relevant Medication.", 0, 1, code)); 561 } 562 563 @Override 564 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 565 switch (_hash) { 566 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category that is relevant.", 0, 1, category); 567 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for relevant Medication.", 0, 1, code); 568 default: return super.getNamedProperty(_hash, _name, _checkValid); 569 } 570 571 } 572 573 @Override 574 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 575 switch (hash) { 576 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 577 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 578 default: return super.getProperty(hash, name, checkValid); 579 } 580 581 } 582 583 @Override 584 public Base setProperty(int hash, String name, Base value) throws FHIRException { 585 switch (hash) { 586 case 50511102: // category 587 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 588 return value; 589 case 3059181: // code 590 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 591 return value; 592 default: return super.setProperty(hash, name, value); 593 } 594 595 } 596 597 @Override 598 public Base setProperty(String name, Base value) throws FHIRException { 599 if (name.equals("category")) { 600 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 601 } else if (name.equals("code")) { 602 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 603 } else 604 return super.setProperty(name, value); 605 return value; 606 } 607 608 @Override 609 public void removeChild(String name, Base value) throws FHIRException { 610 if (name.equals("category")) { 611 this.category = null; 612 } else if (name.equals("code")) { 613 this.code = null; 614 } else 615 super.removeChild(name, value); 616 617 } 618 619 @Override 620 public Base makeProperty(int hash, String name) throws FHIRException { 621 switch (hash) { 622 case 50511102: return getCategory(); 623 case 3059181: return getCode(); 624 default: return super.makeProperty(hash, name); 625 } 626 627 } 628 629 @Override 630 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 631 switch (hash) { 632 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 633 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 634 default: return super.getTypesForProperty(hash, name); 635 } 636 637 } 638 639 @Override 640 public Base addChild(String name) throws FHIRException { 641 if (name.equals("category")) { 642 this.category = new CodeableConcept(); 643 return this.category; 644 } 645 else if (name.equals("code")) { 646 this.code = new CodeableConcept(); 647 return this.code; 648 } 649 else 650 return super.addChild(name); 651 } 652 653 public ConditionDefinitionMedicationComponent copy() { 654 ConditionDefinitionMedicationComponent dst = new ConditionDefinitionMedicationComponent(); 655 copyValues(dst); 656 return dst; 657 } 658 659 public void copyValues(ConditionDefinitionMedicationComponent dst) { 660 super.copyValues(dst); 661 dst.category = category == null ? null : category.copy(); 662 dst.code = code == null ? null : code.copy(); 663 } 664 665 @Override 666 public boolean equalsDeep(Base other_) { 667 if (!super.equalsDeep(other_)) 668 return false; 669 if (!(other_ instanceof ConditionDefinitionMedicationComponent)) 670 return false; 671 ConditionDefinitionMedicationComponent o = (ConditionDefinitionMedicationComponent) other_; 672 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true); 673 } 674 675 @Override 676 public boolean equalsShallow(Base other_) { 677 if (!super.equalsShallow(other_)) 678 return false; 679 if (!(other_ instanceof ConditionDefinitionMedicationComponent)) 680 return false; 681 ConditionDefinitionMedicationComponent o = (ConditionDefinitionMedicationComponent) other_; 682 return true; 683 } 684 685 public boolean isEmpty() { 686 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, code); 687 } 688 689 public String fhirType() { 690 return "ConditionDefinition.medication"; 691 692 } 693 694 } 695 696 @Block() 697 public static class ConditionDefinitionPreconditionComponent extends BackboneElement implements IBaseBackboneElement { 698 /** 699 * Kind of pre-condition. 700 */ 701 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 702 @Description(shortDefinition="sensitive | specific", formalDefinition="Kind of pre-condition." ) 703 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-precondition-type") 704 protected Enumeration<ConditionPreconditionType> type; 705 706 /** 707 * Code for relevant Observation. 708 */ 709 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 710 @Description(shortDefinition="Code for relevant Observation", formalDefinition="Code for relevant Observation." ) 711 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 712 protected CodeableConcept code; 713 714 /** 715 * Value of Observation. 716 */ 717 @Child(name = "value", type = {CodeableConcept.class, Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 718 @Description(shortDefinition="Value of Observation", formalDefinition="Value of Observation." ) 719 protected DataType value; 720 721 private static final long serialVersionUID = -1210333235L; 722 723 /** 724 * Constructor 725 */ 726 public ConditionDefinitionPreconditionComponent() { 727 super(); 728 } 729 730 /** 731 * Constructor 732 */ 733 public ConditionDefinitionPreconditionComponent(ConditionPreconditionType type, CodeableConcept code) { 734 super(); 735 this.setType(type); 736 this.setCode(code); 737 } 738 739 /** 740 * @return {@link #type} (Kind of pre-condition.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 741 */ 742 public Enumeration<ConditionPreconditionType> getTypeElement() { 743 if (this.type == null) 744 if (Configuration.errorOnAutoCreate()) 745 throw new Error("Attempt to auto-create ConditionDefinitionPreconditionComponent.type"); 746 else if (Configuration.doAutoCreate()) 747 this.type = new Enumeration<ConditionPreconditionType>(new ConditionPreconditionTypeEnumFactory()); // bb 748 return this.type; 749 } 750 751 public boolean hasTypeElement() { 752 return this.type != null && !this.type.isEmpty(); 753 } 754 755 public boolean hasType() { 756 return this.type != null && !this.type.isEmpty(); 757 } 758 759 /** 760 * @param value {@link #type} (Kind of pre-condition.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 761 */ 762 public ConditionDefinitionPreconditionComponent setTypeElement(Enumeration<ConditionPreconditionType> value) { 763 this.type = value; 764 return this; 765 } 766 767 /** 768 * @return Kind of pre-condition. 769 */ 770 public ConditionPreconditionType getType() { 771 return this.type == null ? null : this.type.getValue(); 772 } 773 774 /** 775 * @param value Kind of pre-condition. 776 */ 777 public ConditionDefinitionPreconditionComponent setType(ConditionPreconditionType value) { 778 if (this.type == null) 779 this.type = new Enumeration<ConditionPreconditionType>(new ConditionPreconditionTypeEnumFactory()); 780 this.type.setValue(value); 781 return this; 782 } 783 784 /** 785 * @return {@link #code} (Code for relevant Observation.) 786 */ 787 public CodeableConcept getCode() { 788 if (this.code == null) 789 if (Configuration.errorOnAutoCreate()) 790 throw new Error("Attempt to auto-create ConditionDefinitionPreconditionComponent.code"); 791 else if (Configuration.doAutoCreate()) 792 this.code = new CodeableConcept(); // cc 793 return this.code; 794 } 795 796 public boolean hasCode() { 797 return this.code != null && !this.code.isEmpty(); 798 } 799 800 /** 801 * @param value {@link #code} (Code for relevant Observation.) 802 */ 803 public ConditionDefinitionPreconditionComponent setCode(CodeableConcept value) { 804 this.code = value; 805 return this; 806 } 807 808 /** 809 * @return {@link #value} (Value of Observation.) 810 */ 811 public DataType getValue() { 812 return this.value; 813 } 814 815 /** 816 * @return {@link #value} (Value of Observation.) 817 */ 818 public CodeableConcept getValueCodeableConcept() throws FHIRException { 819 if (this.value == null) 820 this.value = new CodeableConcept(); 821 if (!(this.value instanceof CodeableConcept)) 822 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 823 return (CodeableConcept) this.value; 824 } 825 826 public boolean hasValueCodeableConcept() { 827 return this != null && this.value instanceof CodeableConcept; 828 } 829 830 /** 831 * @return {@link #value} (Value of Observation.) 832 */ 833 public Quantity getValueQuantity() throws FHIRException { 834 if (this.value == null) 835 this.value = new Quantity(); 836 if (!(this.value instanceof Quantity)) 837 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 838 return (Quantity) this.value; 839 } 840 841 public boolean hasValueQuantity() { 842 return this != null && this.value instanceof Quantity; 843 } 844 845 public boolean hasValue() { 846 return this.value != null && !this.value.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #value} (Value of Observation.) 851 */ 852 public ConditionDefinitionPreconditionComponent setValue(DataType value) { 853 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity)) 854 throw new FHIRException("Not the right type for ConditionDefinition.precondition.value[x]: "+value.fhirType()); 855 this.value = value; 856 return this; 857 } 858 859 protected void listChildren(List<Property> children) { 860 super.listChildren(children); 861 children.add(new Property("type", "code", "Kind of pre-condition.", 0, 1, type)); 862 children.add(new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code)); 863 children.add(new Property("value[x]", "CodeableConcept|Quantity", "Value of Observation.", 0, 1, value)); 864 } 865 866 @Override 867 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 868 switch (_hash) { 869 case 3575610: /*type*/ return new Property("type", "code", "Kind of pre-condition.", 0, 1, type); 870 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code for relevant Observation.", 0, 1, code); 871 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity", "Value of Observation.", 0, 1, value); 872 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity", "Value of Observation.", 0, 1, value); 873 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Value of Observation.", 0, 1, value); 874 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Value of Observation.", 0, 1, value); 875 default: return super.getNamedProperty(_hash, _name, _checkValid); 876 } 877 878 } 879 880 @Override 881 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 882 switch (hash) { 883 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ConditionPreconditionType> 884 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 885 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 886 default: return super.getProperty(hash, name, checkValid); 887 } 888 889 } 890 891 @Override 892 public Base setProperty(int hash, String name, Base value) throws FHIRException { 893 switch (hash) { 894 case 3575610: // type 895 value = new ConditionPreconditionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 896 this.type = (Enumeration) value; // Enumeration<ConditionPreconditionType> 897 return value; 898 case 3059181: // code 899 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 900 return value; 901 case 111972721: // value 902 this.value = TypeConvertor.castToType(value); // DataType 903 return value; 904 default: return super.setProperty(hash, name, value); 905 } 906 907 } 908 909 @Override 910 public Base setProperty(String name, Base value) throws FHIRException { 911 if (name.equals("type")) { 912 value = new ConditionPreconditionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 913 this.type = (Enumeration) value; // Enumeration<ConditionPreconditionType> 914 } else if (name.equals("code")) { 915 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 916 } else if (name.equals("value[x]")) { 917 this.value = TypeConvertor.castToType(value); // DataType 918 } else 919 return super.setProperty(name, value); 920 return value; 921 } 922 923 @Override 924 public void removeChild(String name, Base value) throws FHIRException { 925 if (name.equals("type")) { 926 value = new ConditionPreconditionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 927 this.type = (Enumeration) value; // Enumeration<ConditionPreconditionType> 928 } else if (name.equals("code")) { 929 this.code = null; 930 } else if (name.equals("value[x]")) { 931 this.value = null; 932 } else 933 super.removeChild(name, value); 934 935 } 936 937 @Override 938 public Base makeProperty(int hash, String name) throws FHIRException { 939 switch (hash) { 940 case 3575610: return getTypeElement(); 941 case 3059181: return getCode(); 942 case -1410166417: return getValue(); 943 case 111972721: return getValue(); 944 default: return super.makeProperty(hash, name); 945 } 946 947 } 948 949 @Override 950 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 951 switch (hash) { 952 case 3575610: /*type*/ return new String[] {"code"}; 953 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 954 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity"}; 955 default: return super.getTypesForProperty(hash, name); 956 } 957 958 } 959 960 @Override 961 public Base addChild(String name) throws FHIRException { 962 if (name.equals("type")) { 963 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.precondition.type"); 964 } 965 else if (name.equals("code")) { 966 this.code = new CodeableConcept(); 967 return this.code; 968 } 969 else if (name.equals("valueCodeableConcept")) { 970 this.value = new CodeableConcept(); 971 return this.value; 972 } 973 else if (name.equals("valueQuantity")) { 974 this.value = new Quantity(); 975 return this.value; 976 } 977 else 978 return super.addChild(name); 979 } 980 981 public ConditionDefinitionPreconditionComponent copy() { 982 ConditionDefinitionPreconditionComponent dst = new ConditionDefinitionPreconditionComponent(); 983 copyValues(dst); 984 return dst; 985 } 986 987 public void copyValues(ConditionDefinitionPreconditionComponent dst) { 988 super.copyValues(dst); 989 dst.type = type == null ? null : type.copy(); 990 dst.code = code == null ? null : code.copy(); 991 dst.value = value == null ? null : value.copy(); 992 } 993 994 @Override 995 public boolean equalsDeep(Base other_) { 996 if (!super.equalsDeep(other_)) 997 return false; 998 if (!(other_ instanceof ConditionDefinitionPreconditionComponent)) 999 return false; 1000 ConditionDefinitionPreconditionComponent o = (ConditionDefinitionPreconditionComponent) other_; 1001 return compareDeep(type, o.type, true) && compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 1002 ; 1003 } 1004 1005 @Override 1006 public boolean equalsShallow(Base other_) { 1007 if (!super.equalsShallow(other_)) 1008 return false; 1009 if (!(other_ instanceof ConditionDefinitionPreconditionComponent)) 1010 return false; 1011 ConditionDefinitionPreconditionComponent o = (ConditionDefinitionPreconditionComponent) other_; 1012 return compareValues(type, o.type, true); 1013 } 1014 1015 public boolean isEmpty() { 1016 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, code, value); 1017 } 1018 1019 public String fhirType() { 1020 return "ConditionDefinition.precondition"; 1021 1022 } 1023 1024 } 1025 1026 @Block() 1027 public static class ConditionDefinitionQuestionnaireComponent extends BackboneElement implements IBaseBackboneElement { 1028 /** 1029 * Use of the questionnaire. 1030 */ 1031 @Child(name = "purpose", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1032 @Description(shortDefinition="preadmit | diff-diagnosis | outcome", formalDefinition="Use of the questionnaire." ) 1033 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-questionnaire-purpose") 1034 protected Enumeration<ConditionQuestionnairePurpose> purpose; 1035 1036 /** 1037 * Specific Questionnaire. 1038 */ 1039 @Child(name = "reference", type = {Questionnaire.class}, order=2, min=1, max=1, modifier=false, summary=false) 1040 @Description(shortDefinition="Specific Questionnaire", formalDefinition="Specific Questionnaire." ) 1041 protected Reference reference; 1042 1043 private static final long serialVersionUID = -1791379681L; 1044 1045 /** 1046 * Constructor 1047 */ 1048 public ConditionDefinitionQuestionnaireComponent() { 1049 super(); 1050 } 1051 1052 /** 1053 * Constructor 1054 */ 1055 public ConditionDefinitionQuestionnaireComponent(ConditionQuestionnairePurpose purpose, Reference reference) { 1056 super(); 1057 this.setPurpose(purpose); 1058 this.setReference(reference); 1059 } 1060 1061 /** 1062 * @return {@link #purpose} (Use of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1063 */ 1064 public Enumeration<ConditionQuestionnairePurpose> getPurposeElement() { 1065 if (this.purpose == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error("Attempt to auto-create ConditionDefinitionQuestionnaireComponent.purpose"); 1068 else if (Configuration.doAutoCreate()) 1069 this.purpose = new Enumeration<ConditionQuestionnairePurpose>(new ConditionQuestionnairePurposeEnumFactory()); // bb 1070 return this.purpose; 1071 } 1072 1073 public boolean hasPurposeElement() { 1074 return this.purpose != null && !this.purpose.isEmpty(); 1075 } 1076 1077 public boolean hasPurpose() { 1078 return this.purpose != null && !this.purpose.isEmpty(); 1079 } 1080 1081 /** 1082 * @param value {@link #purpose} (Use of the questionnaire.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1083 */ 1084 public ConditionDefinitionQuestionnaireComponent setPurposeElement(Enumeration<ConditionQuestionnairePurpose> value) { 1085 this.purpose = value; 1086 return this; 1087 } 1088 1089 /** 1090 * @return Use of the questionnaire. 1091 */ 1092 public ConditionQuestionnairePurpose getPurpose() { 1093 return this.purpose == null ? null : this.purpose.getValue(); 1094 } 1095 1096 /** 1097 * @param value Use of the questionnaire. 1098 */ 1099 public ConditionDefinitionQuestionnaireComponent setPurpose(ConditionQuestionnairePurpose value) { 1100 if (this.purpose == null) 1101 this.purpose = new Enumeration<ConditionQuestionnairePurpose>(new ConditionQuestionnairePurposeEnumFactory()); 1102 this.purpose.setValue(value); 1103 return this; 1104 } 1105 1106 /** 1107 * @return {@link #reference} (Specific Questionnaire.) 1108 */ 1109 public Reference getReference() { 1110 if (this.reference == null) 1111 if (Configuration.errorOnAutoCreate()) 1112 throw new Error("Attempt to auto-create ConditionDefinitionQuestionnaireComponent.reference"); 1113 else if (Configuration.doAutoCreate()) 1114 this.reference = new Reference(); // cc 1115 return this.reference; 1116 } 1117 1118 public boolean hasReference() { 1119 return this.reference != null && !this.reference.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #reference} (Specific Questionnaire.) 1124 */ 1125 public ConditionDefinitionQuestionnaireComponent setReference(Reference value) { 1126 this.reference = value; 1127 return this; 1128 } 1129 1130 protected void listChildren(List<Property> children) { 1131 super.listChildren(children); 1132 children.add(new Property("purpose", "code", "Use of the questionnaire.", 0, 1, purpose)); 1133 children.add(new Property("reference", "Reference(Questionnaire)", "Specific Questionnaire.", 0, 1, reference)); 1134 } 1135 1136 @Override 1137 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1138 switch (_hash) { 1139 case -220463842: /*purpose*/ return new Property("purpose", "code", "Use of the questionnaire.", 0, 1, purpose); 1140 case -925155509: /*reference*/ return new Property("reference", "Reference(Questionnaire)", "Specific Questionnaire.", 0, 1, reference); 1141 default: return super.getNamedProperty(_hash, _name, _checkValid); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1148 switch (hash) { 1149 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // Enumeration<ConditionQuestionnairePurpose> 1150 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1151 default: return super.getProperty(hash, name, checkValid); 1152 } 1153 1154 } 1155 1156 @Override 1157 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1158 switch (hash) { 1159 case -220463842: // purpose 1160 value = new ConditionQuestionnairePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1161 this.purpose = (Enumeration) value; // Enumeration<ConditionQuestionnairePurpose> 1162 return value; 1163 case -925155509: // reference 1164 this.reference = TypeConvertor.castToReference(value); // Reference 1165 return value; 1166 default: return super.setProperty(hash, name, value); 1167 } 1168 1169 } 1170 1171 @Override 1172 public Base setProperty(String name, Base value) throws FHIRException { 1173 if (name.equals("purpose")) { 1174 value = new ConditionQuestionnairePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1175 this.purpose = (Enumeration) value; // Enumeration<ConditionQuestionnairePurpose> 1176 } else if (name.equals("reference")) { 1177 this.reference = TypeConvertor.castToReference(value); // Reference 1178 } else 1179 return super.setProperty(name, value); 1180 return value; 1181 } 1182 1183 @Override 1184 public void removeChild(String name, Base value) throws FHIRException { 1185 if (name.equals("purpose")) { 1186 value = new ConditionQuestionnairePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1187 this.purpose = (Enumeration) value; // Enumeration<ConditionQuestionnairePurpose> 1188 } else if (name.equals("reference")) { 1189 this.reference = null; 1190 } else 1191 super.removeChild(name, value); 1192 1193 } 1194 1195 @Override 1196 public Base makeProperty(int hash, String name) throws FHIRException { 1197 switch (hash) { 1198 case -220463842: return getPurposeElement(); 1199 case -925155509: return getReference(); 1200 default: return super.makeProperty(hash, name); 1201 } 1202 1203 } 1204 1205 @Override 1206 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1207 switch (hash) { 1208 case -220463842: /*purpose*/ return new String[] {"code"}; 1209 case -925155509: /*reference*/ return new String[] {"Reference"}; 1210 default: return super.getTypesForProperty(hash, name); 1211 } 1212 1213 } 1214 1215 @Override 1216 public Base addChild(String name) throws FHIRException { 1217 if (name.equals("purpose")) { 1218 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.questionnaire.purpose"); 1219 } 1220 else if (name.equals("reference")) { 1221 this.reference = new Reference(); 1222 return this.reference; 1223 } 1224 else 1225 return super.addChild(name); 1226 } 1227 1228 public ConditionDefinitionQuestionnaireComponent copy() { 1229 ConditionDefinitionQuestionnaireComponent dst = new ConditionDefinitionQuestionnaireComponent(); 1230 copyValues(dst); 1231 return dst; 1232 } 1233 1234 public void copyValues(ConditionDefinitionQuestionnaireComponent dst) { 1235 super.copyValues(dst); 1236 dst.purpose = purpose == null ? null : purpose.copy(); 1237 dst.reference = reference == null ? null : reference.copy(); 1238 } 1239 1240 @Override 1241 public boolean equalsDeep(Base other_) { 1242 if (!super.equalsDeep(other_)) 1243 return false; 1244 if (!(other_ instanceof ConditionDefinitionQuestionnaireComponent)) 1245 return false; 1246 ConditionDefinitionQuestionnaireComponent o = (ConditionDefinitionQuestionnaireComponent) other_; 1247 return compareDeep(purpose, o.purpose, true) && compareDeep(reference, o.reference, true); 1248 } 1249 1250 @Override 1251 public boolean equalsShallow(Base other_) { 1252 if (!super.equalsShallow(other_)) 1253 return false; 1254 if (!(other_ instanceof ConditionDefinitionQuestionnaireComponent)) 1255 return false; 1256 ConditionDefinitionQuestionnaireComponent o = (ConditionDefinitionQuestionnaireComponent) other_; 1257 return compareValues(purpose, o.purpose, true); 1258 } 1259 1260 public boolean isEmpty() { 1261 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, reference); 1262 } 1263 1264 public String fhirType() { 1265 return "ConditionDefinition.questionnaire"; 1266 1267 } 1268 1269 } 1270 1271 @Block() 1272 public static class ConditionDefinitionPlanComponent extends BackboneElement implements IBaseBackboneElement { 1273 /** 1274 * Use for the plan. 1275 */ 1276 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1277 @Description(shortDefinition="Use for the plan", formalDefinition="Use for the plan." ) 1278 protected CodeableConcept role; 1279 1280 /** 1281 * The actual plan. 1282 */ 1283 @Child(name = "reference", type = {PlanDefinition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1284 @Description(shortDefinition="The actual plan", formalDefinition="The actual plan." ) 1285 protected Reference reference; 1286 1287 private static final long serialVersionUID = -1992921787L; 1288 1289 /** 1290 * Constructor 1291 */ 1292 public ConditionDefinitionPlanComponent() { 1293 super(); 1294 } 1295 1296 /** 1297 * Constructor 1298 */ 1299 public ConditionDefinitionPlanComponent(Reference reference) { 1300 super(); 1301 this.setReference(reference); 1302 } 1303 1304 /** 1305 * @return {@link #role} (Use for the plan.) 1306 */ 1307 public CodeableConcept getRole() { 1308 if (this.role == null) 1309 if (Configuration.errorOnAutoCreate()) 1310 throw new Error("Attempt to auto-create ConditionDefinitionPlanComponent.role"); 1311 else if (Configuration.doAutoCreate()) 1312 this.role = new CodeableConcept(); // cc 1313 return this.role; 1314 } 1315 1316 public boolean hasRole() { 1317 return this.role != null && !this.role.isEmpty(); 1318 } 1319 1320 /** 1321 * @param value {@link #role} (Use for the plan.) 1322 */ 1323 public ConditionDefinitionPlanComponent setRole(CodeableConcept value) { 1324 this.role = value; 1325 return this; 1326 } 1327 1328 /** 1329 * @return {@link #reference} (The actual plan.) 1330 */ 1331 public Reference getReference() { 1332 if (this.reference == null) 1333 if (Configuration.errorOnAutoCreate()) 1334 throw new Error("Attempt to auto-create ConditionDefinitionPlanComponent.reference"); 1335 else if (Configuration.doAutoCreate()) 1336 this.reference = new Reference(); // cc 1337 return this.reference; 1338 } 1339 1340 public boolean hasReference() { 1341 return this.reference != null && !this.reference.isEmpty(); 1342 } 1343 1344 /** 1345 * @param value {@link #reference} (The actual plan.) 1346 */ 1347 public ConditionDefinitionPlanComponent setReference(Reference value) { 1348 this.reference = value; 1349 return this; 1350 } 1351 1352 protected void listChildren(List<Property> children) { 1353 super.listChildren(children); 1354 children.add(new Property("role", "CodeableConcept", "Use for the plan.", 0, 1, role)); 1355 children.add(new Property("reference", "Reference(PlanDefinition)", "The actual plan.", 0, 1, reference)); 1356 } 1357 1358 @Override 1359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1360 switch (_hash) { 1361 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Use for the plan.", 0, 1, role); 1362 case -925155509: /*reference*/ return new Property("reference", "Reference(PlanDefinition)", "The actual plan.", 0, 1, reference); 1363 default: return super.getNamedProperty(_hash, _name, _checkValid); 1364 } 1365 1366 } 1367 1368 @Override 1369 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1370 switch (hash) { 1371 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1372 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1373 default: return super.getProperty(hash, name, checkValid); 1374 } 1375 1376 } 1377 1378 @Override 1379 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1380 switch (hash) { 1381 case 3506294: // role 1382 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1383 return value; 1384 case -925155509: // reference 1385 this.reference = TypeConvertor.castToReference(value); // Reference 1386 return value; 1387 default: return super.setProperty(hash, name, value); 1388 } 1389 1390 } 1391 1392 @Override 1393 public Base setProperty(String name, Base value) throws FHIRException { 1394 if (name.equals("role")) { 1395 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1396 } else if (name.equals("reference")) { 1397 this.reference = TypeConvertor.castToReference(value); // Reference 1398 } else 1399 return super.setProperty(name, value); 1400 return value; 1401 } 1402 1403 @Override 1404 public void removeChild(String name, Base value) throws FHIRException { 1405 if (name.equals("role")) { 1406 this.role = null; 1407 } else if (name.equals("reference")) { 1408 this.reference = null; 1409 } else 1410 super.removeChild(name, value); 1411 1412 } 1413 1414 @Override 1415 public Base makeProperty(int hash, String name) throws FHIRException { 1416 switch (hash) { 1417 case 3506294: return getRole(); 1418 case -925155509: return getReference(); 1419 default: return super.makeProperty(hash, name); 1420 } 1421 1422 } 1423 1424 @Override 1425 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1426 switch (hash) { 1427 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1428 case -925155509: /*reference*/ return new String[] {"Reference"}; 1429 default: return super.getTypesForProperty(hash, name); 1430 } 1431 1432 } 1433 1434 @Override 1435 public Base addChild(String name) throws FHIRException { 1436 if (name.equals("role")) { 1437 this.role = new CodeableConcept(); 1438 return this.role; 1439 } 1440 else if (name.equals("reference")) { 1441 this.reference = new Reference(); 1442 return this.reference; 1443 } 1444 else 1445 return super.addChild(name); 1446 } 1447 1448 public ConditionDefinitionPlanComponent copy() { 1449 ConditionDefinitionPlanComponent dst = new ConditionDefinitionPlanComponent(); 1450 copyValues(dst); 1451 return dst; 1452 } 1453 1454 public void copyValues(ConditionDefinitionPlanComponent dst) { 1455 super.copyValues(dst); 1456 dst.role = role == null ? null : role.copy(); 1457 dst.reference = reference == null ? null : reference.copy(); 1458 } 1459 1460 @Override 1461 public boolean equalsDeep(Base other_) { 1462 if (!super.equalsDeep(other_)) 1463 return false; 1464 if (!(other_ instanceof ConditionDefinitionPlanComponent)) 1465 return false; 1466 ConditionDefinitionPlanComponent o = (ConditionDefinitionPlanComponent) other_; 1467 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 1468 } 1469 1470 @Override 1471 public boolean equalsShallow(Base other_) { 1472 if (!super.equalsShallow(other_)) 1473 return false; 1474 if (!(other_ instanceof ConditionDefinitionPlanComponent)) 1475 return false; 1476 ConditionDefinitionPlanComponent o = (ConditionDefinitionPlanComponent) other_; 1477 return true; 1478 } 1479 1480 public boolean isEmpty() { 1481 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 1482 } 1483 1484 public String fhirType() { 1485 return "ConditionDefinition.plan"; 1486 1487 } 1488 1489 } 1490 1491 /** 1492 * An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers. 1493 */ 1494 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1495 @Description(shortDefinition="Canonical identifier for this condition definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers." ) 1496 protected UriType url; 1497 1498 /** 1499 * A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 1500 */ 1501 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1502 @Description(shortDefinition="Additional identifier for the condition definition", formalDefinition="A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1503 protected List<Identifier> identifier; 1504 1505 /** 1506 * The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1507 */ 1508 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1509 @Description(shortDefinition="Business version of the condition definition", formalDefinition="The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 1510 protected StringType version; 1511 1512 /** 1513 * Indicates the mechanism used to compare versions to determine which is more current. 1514 */ 1515 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1516 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1517 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1518 protected DataType versionAlgorithm; 1519 1520 /** 1521 * A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1522 */ 1523 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1524 @Description(shortDefinition="Name for this condition definition (computer friendly)", formalDefinition="A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1525 protected StringType name; 1526 1527 /** 1528 * A short, descriptive, user-friendly title for the condition definition. 1529 */ 1530 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1531 @Description(shortDefinition="Name for this condition definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the condition definition." ) 1532 protected StringType title; 1533 1534 /** 1535 * An explanatory or alternate title for the event definition giving additional information about its content. 1536 */ 1537 @Child(name = "subtitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1538 @Description(shortDefinition="Subordinate title of the event definition", formalDefinition="An explanatory or alternate title for the event definition giving additional information about its content." ) 1539 protected StringType subtitle; 1540 1541 /** 1542 * The status of this condition definition. Enables tracking the life-cycle of the content. 1543 */ 1544 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 1545 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this condition definition. Enables tracking the life-cycle of the content." ) 1546 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1547 protected Enumeration<PublicationStatus> status; 1548 1549 /** 1550 * A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1551 */ 1552 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1553 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1554 protected BooleanType experimental; 1555 1556 /** 1557 * The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes. 1558 */ 1559 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1560 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes." ) 1561 protected DateTimeType date; 1562 1563 /** 1564 * The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition. 1565 */ 1566 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1567 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition." ) 1568 protected StringType publisher; 1569 1570 /** 1571 * Contact details to assist a user in finding and communicating with the publisher. 1572 */ 1573 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1574 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1575 protected List<ContactDetail> contact; 1576 1577 /** 1578 * A free text natural language description of the condition definition from a consumer's perspective. 1579 */ 1580 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1581 @Description(shortDefinition="Natural language description of the condition definition", formalDefinition="A free text natural language description of the condition definition from a consumer's perspective." ) 1582 protected MarkdownType description; 1583 1584 /** 1585 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances. 1586 */ 1587 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1588 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances." ) 1589 protected List<UsageContext> useContext; 1590 1591 /** 1592 * A legal or geographic region in which the condition definition is intended to be used. 1593 */ 1594 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1595 @Description(shortDefinition="Intended jurisdiction for condition definition (if applicable)", formalDefinition="A legal or geographic region in which the condition definition is intended to be used." ) 1596 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1597 protected List<CodeableConcept> jurisdiction; 1598 1599 /** 1600 * Identification of the condition, problem or diagnosis. 1601 */ 1602 @Child(name = "code", type = {CodeableConcept.class}, order=15, min=1, max=1, modifier=false, summary=true) 1603 @Description(shortDefinition="Identification of the condition, problem or diagnosis", formalDefinition="Identification of the condition, problem or diagnosis." ) 1604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 1605 protected CodeableConcept code; 1606 1607 /** 1608 * A subjective assessment of the severity of the condition as evaluated by the clinician. 1609 */ 1610 @Child(name = "severity", type = {CodeableConcept.class}, order=16, min=0, max=1, modifier=false, summary=true) 1611 @Description(shortDefinition="Subjective severity of condition", formalDefinition="A subjective assessment of the severity of the condition as evaluated by the clinician." ) 1612 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-severity") 1613 protected CodeableConcept severity; 1614 1615 /** 1616 * The anatomical location where this condition manifests itself. 1617 */ 1618 @Child(name = "bodySite", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=true) 1619 @Description(shortDefinition="Anatomical location, if relevant", formalDefinition="The anatomical location where this condition manifests itself." ) 1620 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1621 protected CodeableConcept bodySite; 1622 1623 /** 1624 * Clinical stage or grade of a condition. May include formal severity assessments. 1625 */ 1626 @Child(name = "stage", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=true) 1627 @Description(shortDefinition="Stage/grade, usually assessed formally", formalDefinition="Clinical stage or grade of a condition. May include formal severity assessments." ) 1628 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-stage") 1629 protected CodeableConcept stage; 1630 1631 /** 1632 * Whether Severity is appropriate to collect for this condition. 1633 */ 1634 @Child(name = "hasSeverity", type = {BooleanType.class}, order=19, min=0, max=1, modifier=false, summary=false) 1635 @Description(shortDefinition="Whether Severity is appropriate", formalDefinition="Whether Severity is appropriate to collect for this condition." ) 1636 protected BooleanType hasSeverity; 1637 1638 /** 1639 * Whether bodySite is appropriate to collect for this condition. 1640 */ 1641 @Child(name = "hasBodySite", type = {BooleanType.class}, order=20, min=0, max=1, modifier=false, summary=false) 1642 @Description(shortDefinition="Whether bodySite is appropriate", formalDefinition="Whether bodySite is appropriate to collect for this condition." ) 1643 protected BooleanType hasBodySite; 1644 1645 /** 1646 * Whether stage is appropriate to collect for this condition. 1647 */ 1648 @Child(name = "hasStage", type = {BooleanType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1649 @Description(shortDefinition="Whether stage is appropriate", formalDefinition="Whether stage is appropriate to collect for this condition." ) 1650 protected BooleanType hasStage; 1651 1652 /** 1653 * Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers. 1654 */ 1655 @Child(name = "definition", type = {UriType.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1656 @Description(shortDefinition="Formal Definition for the condition", formalDefinition="Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers." ) 1657 protected List<UriType> definition; 1658 1659 /** 1660 * Observations particularly relevant to this condition. 1661 */ 1662 @Child(name = "observation", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1663 @Description(shortDefinition="Observations particularly relevant to this condition", formalDefinition="Observations particularly relevant to this condition." ) 1664 protected List<ConditionDefinitionObservationComponent> observation; 1665 1666 /** 1667 * Medications particularly relevant for this condition. 1668 */ 1669 @Child(name = "medication", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1670 @Description(shortDefinition="Medications particularly relevant for this condition", formalDefinition="Medications particularly relevant for this condition." ) 1671 protected List<ConditionDefinitionMedicationComponent> medication; 1672 1673 /** 1674 * An observation that suggests that this condition applies. 1675 */ 1676 @Child(name = "precondition", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1677 @Description(shortDefinition="Observation that suggets this condition", formalDefinition="An observation that suggests that this condition applies." ) 1678 protected List<ConditionDefinitionPreconditionComponent> precondition; 1679 1680 /** 1681 * Appropriate team for this condition. 1682 */ 1683 @Child(name = "team", type = {CareTeam.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1684 @Description(shortDefinition="Appropriate team for this condition", formalDefinition="Appropriate team for this condition." ) 1685 protected List<Reference> team; 1686 1687 /** 1688 * Questionnaire for this condition. 1689 */ 1690 @Child(name = "questionnaire", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1691 @Description(shortDefinition="Questionnaire for this condition", formalDefinition="Questionnaire for this condition." ) 1692 protected List<ConditionDefinitionQuestionnaireComponent> questionnaire; 1693 1694 /** 1695 * Plan that is appropriate. 1696 */ 1697 @Child(name = "plan", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1698 @Description(shortDefinition="Plan that is appropriate", formalDefinition="Plan that is appropriate." ) 1699 protected List<ConditionDefinitionPlanComponent> plan; 1700 1701 private static final long serialVersionUID = 1055220331L; 1702 1703 /** 1704 * Constructor 1705 */ 1706 public ConditionDefinition() { 1707 super(); 1708 } 1709 1710 /** 1711 * Constructor 1712 */ 1713 public ConditionDefinition(PublicationStatus status, CodeableConcept code) { 1714 super(); 1715 this.setStatus(status); 1716 this.setCode(code); 1717 } 1718 1719 /** 1720 * @return {@link #url} (An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1721 */ 1722 public UriType getUrlElement() { 1723 if (this.url == null) 1724 if (Configuration.errorOnAutoCreate()) 1725 throw new Error("Attempt to auto-create ConditionDefinition.url"); 1726 else if (Configuration.doAutoCreate()) 1727 this.url = new UriType(); // bb 1728 return this.url; 1729 } 1730 1731 public boolean hasUrlElement() { 1732 return this.url != null && !this.url.isEmpty(); 1733 } 1734 1735 public boolean hasUrl() { 1736 return this.url != null && !this.url.isEmpty(); 1737 } 1738 1739 /** 1740 * @param value {@link #url} (An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1741 */ 1742 public ConditionDefinition setUrlElement(UriType value) { 1743 this.url = value; 1744 return this; 1745 } 1746 1747 /** 1748 * @return An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers. 1749 */ 1750 public String getUrl() { 1751 return this.url == null ? null : this.url.getValue(); 1752 } 1753 1754 /** 1755 * @param value An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers. 1756 */ 1757 public ConditionDefinition setUrl(String value) { 1758 if (Utilities.noString(value)) 1759 this.url = null; 1760 else { 1761 if (this.url == null) 1762 this.url = new UriType(); 1763 this.url.setValue(value); 1764 } 1765 return this; 1766 } 1767 1768 /** 1769 * @return {@link #identifier} (A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1770 */ 1771 public List<Identifier> getIdentifier() { 1772 if (this.identifier == null) 1773 this.identifier = new ArrayList<Identifier>(); 1774 return this.identifier; 1775 } 1776 1777 /** 1778 * @return Returns a reference to <code>this</code> for easy method chaining 1779 */ 1780 public ConditionDefinition setIdentifier(List<Identifier> theIdentifier) { 1781 this.identifier = theIdentifier; 1782 return this; 1783 } 1784 1785 public boolean hasIdentifier() { 1786 if (this.identifier == null) 1787 return false; 1788 for (Identifier item : this.identifier) 1789 if (!item.isEmpty()) 1790 return true; 1791 return false; 1792 } 1793 1794 public Identifier addIdentifier() { //3 1795 Identifier t = new Identifier(); 1796 if (this.identifier == null) 1797 this.identifier = new ArrayList<Identifier>(); 1798 this.identifier.add(t); 1799 return t; 1800 } 1801 1802 public ConditionDefinition addIdentifier(Identifier t) { //3 1803 if (t == null) 1804 return this; 1805 if (this.identifier == null) 1806 this.identifier = new ArrayList<Identifier>(); 1807 this.identifier.add(t); 1808 return this; 1809 } 1810 1811 /** 1812 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1813 */ 1814 public Identifier getIdentifierFirstRep() { 1815 if (getIdentifier().isEmpty()) { 1816 addIdentifier(); 1817 } 1818 return getIdentifier().get(0); 1819 } 1820 1821 /** 1822 * @return {@link #version} (The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1823 */ 1824 public StringType getVersionElement() { 1825 if (this.version == null) 1826 if (Configuration.errorOnAutoCreate()) 1827 throw new Error("Attempt to auto-create ConditionDefinition.version"); 1828 else if (Configuration.doAutoCreate()) 1829 this.version = new StringType(); // bb 1830 return this.version; 1831 } 1832 1833 public boolean hasVersionElement() { 1834 return this.version != null && !this.version.isEmpty(); 1835 } 1836 1837 public boolean hasVersion() { 1838 return this.version != null && !this.version.isEmpty(); 1839 } 1840 1841 /** 1842 * @param value {@link #version} (The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1843 */ 1844 public ConditionDefinition setVersionElement(StringType value) { 1845 this.version = value; 1846 return this; 1847 } 1848 1849 /** 1850 * @return The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1851 */ 1852 public String getVersion() { 1853 return this.version == null ? null : this.version.getValue(); 1854 } 1855 1856 /** 1857 * @param value The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1858 */ 1859 public ConditionDefinition setVersion(String value) { 1860 if (Utilities.noString(value)) 1861 this.version = null; 1862 else { 1863 if (this.version == null) 1864 this.version = new StringType(); 1865 this.version.setValue(value); 1866 } 1867 return this; 1868 } 1869 1870 /** 1871 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1872 */ 1873 public DataType getVersionAlgorithm() { 1874 return this.versionAlgorithm; 1875 } 1876 1877 /** 1878 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1879 */ 1880 public StringType getVersionAlgorithmStringType() throws FHIRException { 1881 if (this.versionAlgorithm == null) 1882 this.versionAlgorithm = new StringType(); 1883 if (!(this.versionAlgorithm instanceof StringType)) 1884 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1885 return (StringType) this.versionAlgorithm; 1886 } 1887 1888 public boolean hasVersionAlgorithmStringType() { 1889 return this != null && this.versionAlgorithm instanceof StringType; 1890 } 1891 1892 /** 1893 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1894 */ 1895 public Coding getVersionAlgorithmCoding() throws FHIRException { 1896 if (this.versionAlgorithm == null) 1897 this.versionAlgorithm = new Coding(); 1898 if (!(this.versionAlgorithm instanceof Coding)) 1899 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1900 return (Coding) this.versionAlgorithm; 1901 } 1902 1903 public boolean hasVersionAlgorithmCoding() { 1904 return this != null && this.versionAlgorithm instanceof Coding; 1905 } 1906 1907 public boolean hasVersionAlgorithm() { 1908 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1909 } 1910 1911 /** 1912 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1913 */ 1914 public ConditionDefinition setVersionAlgorithm(DataType value) { 1915 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1916 throw new FHIRException("Not the right type for ConditionDefinition.versionAlgorithm[x]: "+value.fhirType()); 1917 this.versionAlgorithm = value; 1918 return this; 1919 } 1920 1921 /** 1922 * @return {@link #name} (A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1923 */ 1924 public StringType getNameElement() { 1925 if (this.name == null) 1926 if (Configuration.errorOnAutoCreate()) 1927 throw new Error("Attempt to auto-create ConditionDefinition.name"); 1928 else if (Configuration.doAutoCreate()) 1929 this.name = new StringType(); // bb 1930 return this.name; 1931 } 1932 1933 public boolean hasNameElement() { 1934 return this.name != null && !this.name.isEmpty(); 1935 } 1936 1937 public boolean hasName() { 1938 return this.name != null && !this.name.isEmpty(); 1939 } 1940 1941 /** 1942 * @param value {@link #name} (A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1943 */ 1944 public ConditionDefinition setNameElement(StringType value) { 1945 this.name = value; 1946 return this; 1947 } 1948 1949 /** 1950 * @return A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1951 */ 1952 public String getName() { 1953 return this.name == null ? null : this.name.getValue(); 1954 } 1955 1956 /** 1957 * @param value A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1958 */ 1959 public ConditionDefinition setName(String value) { 1960 if (Utilities.noString(value)) 1961 this.name = null; 1962 else { 1963 if (this.name == null) 1964 this.name = new StringType(); 1965 this.name.setValue(value); 1966 } 1967 return this; 1968 } 1969 1970 /** 1971 * @return {@link #title} (A short, descriptive, user-friendly title for the condition definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1972 */ 1973 public StringType getTitleElement() { 1974 if (this.title == null) 1975 if (Configuration.errorOnAutoCreate()) 1976 throw new Error("Attempt to auto-create ConditionDefinition.title"); 1977 else if (Configuration.doAutoCreate()) 1978 this.title = new StringType(); // bb 1979 return this.title; 1980 } 1981 1982 public boolean hasTitleElement() { 1983 return this.title != null && !this.title.isEmpty(); 1984 } 1985 1986 public boolean hasTitle() { 1987 return this.title != null && !this.title.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #title} (A short, descriptive, user-friendly title for the condition definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1992 */ 1993 public ConditionDefinition setTitleElement(StringType value) { 1994 this.title = value; 1995 return this; 1996 } 1997 1998 /** 1999 * @return A short, descriptive, user-friendly title for the condition definition. 2000 */ 2001 public String getTitle() { 2002 return this.title == null ? null : this.title.getValue(); 2003 } 2004 2005 /** 2006 * @param value A short, descriptive, user-friendly title for the condition definition. 2007 */ 2008 public ConditionDefinition setTitle(String value) { 2009 if (Utilities.noString(value)) 2010 this.title = null; 2011 else { 2012 if (this.title == null) 2013 this.title = new StringType(); 2014 this.title.setValue(value); 2015 } 2016 return this; 2017 } 2018 2019 /** 2020 * @return {@link #subtitle} (An explanatory or alternate title for the event definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 2021 */ 2022 public StringType getSubtitleElement() { 2023 if (this.subtitle == null) 2024 if (Configuration.errorOnAutoCreate()) 2025 throw new Error("Attempt to auto-create ConditionDefinition.subtitle"); 2026 else if (Configuration.doAutoCreate()) 2027 this.subtitle = new StringType(); // bb 2028 return this.subtitle; 2029 } 2030 2031 public boolean hasSubtitleElement() { 2032 return this.subtitle != null && !this.subtitle.isEmpty(); 2033 } 2034 2035 public boolean hasSubtitle() { 2036 return this.subtitle != null && !this.subtitle.isEmpty(); 2037 } 2038 2039 /** 2040 * @param value {@link #subtitle} (An explanatory or alternate title for the event definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 2041 */ 2042 public ConditionDefinition setSubtitleElement(StringType value) { 2043 this.subtitle = value; 2044 return this; 2045 } 2046 2047 /** 2048 * @return An explanatory or alternate title for the event definition giving additional information about its content. 2049 */ 2050 public String getSubtitle() { 2051 return this.subtitle == null ? null : this.subtitle.getValue(); 2052 } 2053 2054 /** 2055 * @param value An explanatory or alternate title for the event definition giving additional information about its content. 2056 */ 2057 public ConditionDefinition setSubtitle(String value) { 2058 if (Utilities.noString(value)) 2059 this.subtitle = null; 2060 else { 2061 if (this.subtitle == null) 2062 this.subtitle = new StringType(); 2063 this.subtitle.setValue(value); 2064 } 2065 return this; 2066 } 2067 2068 /** 2069 * @return {@link #status} (The status of this condition definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2070 */ 2071 public Enumeration<PublicationStatus> getStatusElement() { 2072 if (this.status == null) 2073 if (Configuration.errorOnAutoCreate()) 2074 throw new Error("Attempt to auto-create ConditionDefinition.status"); 2075 else if (Configuration.doAutoCreate()) 2076 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2077 return this.status; 2078 } 2079 2080 public boolean hasStatusElement() { 2081 return this.status != null && !this.status.isEmpty(); 2082 } 2083 2084 public boolean hasStatus() { 2085 return this.status != null && !this.status.isEmpty(); 2086 } 2087 2088 /** 2089 * @param value {@link #status} (The status of this condition definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2090 */ 2091 public ConditionDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2092 this.status = value; 2093 return this; 2094 } 2095 2096 /** 2097 * @return The status of this condition definition. Enables tracking the life-cycle of the content. 2098 */ 2099 public PublicationStatus getStatus() { 2100 return this.status == null ? null : this.status.getValue(); 2101 } 2102 2103 /** 2104 * @param value The status of this condition definition. Enables tracking the life-cycle of the content. 2105 */ 2106 public ConditionDefinition setStatus(PublicationStatus value) { 2107 if (this.status == null) 2108 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2109 this.status.setValue(value); 2110 return this; 2111 } 2112 2113 /** 2114 * @return {@link #experimental} (A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2115 */ 2116 public BooleanType getExperimentalElement() { 2117 if (this.experimental == null) 2118 if (Configuration.errorOnAutoCreate()) 2119 throw new Error("Attempt to auto-create ConditionDefinition.experimental"); 2120 else if (Configuration.doAutoCreate()) 2121 this.experimental = new BooleanType(); // bb 2122 return this.experimental; 2123 } 2124 2125 public boolean hasExperimentalElement() { 2126 return this.experimental != null && !this.experimental.isEmpty(); 2127 } 2128 2129 public boolean hasExperimental() { 2130 return this.experimental != null && !this.experimental.isEmpty(); 2131 } 2132 2133 /** 2134 * @param value {@link #experimental} (A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2135 */ 2136 public ConditionDefinition setExperimentalElement(BooleanType value) { 2137 this.experimental = value; 2138 return this; 2139 } 2140 2141 /** 2142 * @return A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2143 */ 2144 public boolean getExperimental() { 2145 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2146 } 2147 2148 /** 2149 * @param value A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2150 */ 2151 public ConditionDefinition setExperimental(boolean value) { 2152 if (this.experimental == null) 2153 this.experimental = new BooleanType(); 2154 this.experimental.setValue(value); 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #date} (The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2160 */ 2161 public DateTimeType getDateElement() { 2162 if (this.date == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create ConditionDefinition.date"); 2165 else if (Configuration.doAutoCreate()) 2166 this.date = new DateTimeType(); // bb 2167 return this.date; 2168 } 2169 2170 public boolean hasDateElement() { 2171 return this.date != null && !this.date.isEmpty(); 2172 } 2173 2174 public boolean hasDate() { 2175 return this.date != null && !this.date.isEmpty(); 2176 } 2177 2178 /** 2179 * @param value {@link #date} (The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2180 */ 2181 public ConditionDefinition setDateElement(DateTimeType value) { 2182 this.date = value; 2183 return this; 2184 } 2185 2186 /** 2187 * @return The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes. 2188 */ 2189 public Date getDate() { 2190 return this.date == null ? null : this.date.getValue(); 2191 } 2192 2193 /** 2194 * @param value The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes. 2195 */ 2196 public ConditionDefinition setDate(Date value) { 2197 if (value == null) 2198 this.date = null; 2199 else { 2200 if (this.date == null) 2201 this.date = new DateTimeType(); 2202 this.date.setValue(value); 2203 } 2204 return this; 2205 } 2206 2207 /** 2208 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2209 */ 2210 public StringType getPublisherElement() { 2211 if (this.publisher == null) 2212 if (Configuration.errorOnAutoCreate()) 2213 throw new Error("Attempt to auto-create ConditionDefinition.publisher"); 2214 else if (Configuration.doAutoCreate()) 2215 this.publisher = new StringType(); // bb 2216 return this.publisher; 2217 } 2218 2219 public boolean hasPublisherElement() { 2220 return this.publisher != null && !this.publisher.isEmpty(); 2221 } 2222 2223 public boolean hasPublisher() { 2224 return this.publisher != null && !this.publisher.isEmpty(); 2225 } 2226 2227 /** 2228 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2229 */ 2230 public ConditionDefinition setPublisherElement(StringType value) { 2231 this.publisher = value; 2232 return this; 2233 } 2234 2235 /** 2236 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition. 2237 */ 2238 public String getPublisher() { 2239 return this.publisher == null ? null : this.publisher.getValue(); 2240 } 2241 2242 /** 2243 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition. 2244 */ 2245 public ConditionDefinition setPublisher(String value) { 2246 if (Utilities.noString(value)) 2247 this.publisher = null; 2248 else { 2249 if (this.publisher == null) 2250 this.publisher = new StringType(); 2251 this.publisher.setValue(value); 2252 } 2253 return this; 2254 } 2255 2256 /** 2257 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2258 */ 2259 public List<ContactDetail> getContact() { 2260 if (this.contact == null) 2261 this.contact = new ArrayList<ContactDetail>(); 2262 return this.contact; 2263 } 2264 2265 /** 2266 * @return Returns a reference to <code>this</code> for easy method chaining 2267 */ 2268 public ConditionDefinition setContact(List<ContactDetail> theContact) { 2269 this.contact = theContact; 2270 return this; 2271 } 2272 2273 public boolean hasContact() { 2274 if (this.contact == null) 2275 return false; 2276 for (ContactDetail item : this.contact) 2277 if (!item.isEmpty()) 2278 return true; 2279 return false; 2280 } 2281 2282 public ContactDetail addContact() { //3 2283 ContactDetail t = new ContactDetail(); 2284 if (this.contact == null) 2285 this.contact = new ArrayList<ContactDetail>(); 2286 this.contact.add(t); 2287 return t; 2288 } 2289 2290 public ConditionDefinition addContact(ContactDetail t) { //3 2291 if (t == null) 2292 return this; 2293 if (this.contact == null) 2294 this.contact = new ArrayList<ContactDetail>(); 2295 this.contact.add(t); 2296 return this; 2297 } 2298 2299 /** 2300 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2301 */ 2302 public ContactDetail getContactFirstRep() { 2303 if (getContact().isEmpty()) { 2304 addContact(); 2305 } 2306 return getContact().get(0); 2307 } 2308 2309 /** 2310 * @return {@link #description} (A free text natural language description of the condition definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2311 */ 2312 public MarkdownType getDescriptionElement() { 2313 if (this.description == null) 2314 if (Configuration.errorOnAutoCreate()) 2315 throw new Error("Attempt to auto-create ConditionDefinition.description"); 2316 else if (Configuration.doAutoCreate()) 2317 this.description = new MarkdownType(); // bb 2318 return this.description; 2319 } 2320 2321 public boolean hasDescriptionElement() { 2322 return this.description != null && !this.description.isEmpty(); 2323 } 2324 2325 public boolean hasDescription() { 2326 return this.description != null && !this.description.isEmpty(); 2327 } 2328 2329 /** 2330 * @param value {@link #description} (A free text natural language description of the condition definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2331 */ 2332 public ConditionDefinition setDescriptionElement(MarkdownType value) { 2333 this.description = value; 2334 return this; 2335 } 2336 2337 /** 2338 * @return A free text natural language description of the condition definition from a consumer's perspective. 2339 */ 2340 public String getDescription() { 2341 return this.description == null ? null : this.description.getValue(); 2342 } 2343 2344 /** 2345 * @param value A free text natural language description of the condition definition from a consumer's perspective. 2346 */ 2347 public ConditionDefinition setDescription(String value) { 2348 if (Utilities.noString(value)) 2349 this.description = null; 2350 else { 2351 if (this.description == null) 2352 this.description = new MarkdownType(); 2353 this.description.setValue(value); 2354 } 2355 return this; 2356 } 2357 2358 /** 2359 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances.) 2360 */ 2361 public List<UsageContext> getUseContext() { 2362 if (this.useContext == null) 2363 this.useContext = new ArrayList<UsageContext>(); 2364 return this.useContext; 2365 } 2366 2367 /** 2368 * @return Returns a reference to <code>this</code> for easy method chaining 2369 */ 2370 public ConditionDefinition setUseContext(List<UsageContext> theUseContext) { 2371 this.useContext = theUseContext; 2372 return this; 2373 } 2374 2375 public boolean hasUseContext() { 2376 if (this.useContext == null) 2377 return false; 2378 for (UsageContext item : this.useContext) 2379 if (!item.isEmpty()) 2380 return true; 2381 return false; 2382 } 2383 2384 public UsageContext addUseContext() { //3 2385 UsageContext t = new UsageContext(); 2386 if (this.useContext == null) 2387 this.useContext = new ArrayList<UsageContext>(); 2388 this.useContext.add(t); 2389 return t; 2390 } 2391 2392 public ConditionDefinition addUseContext(UsageContext t) { //3 2393 if (t == null) 2394 return this; 2395 if (this.useContext == null) 2396 this.useContext = new ArrayList<UsageContext>(); 2397 this.useContext.add(t); 2398 return this; 2399 } 2400 2401 /** 2402 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2403 */ 2404 public UsageContext getUseContextFirstRep() { 2405 if (getUseContext().isEmpty()) { 2406 addUseContext(); 2407 } 2408 return getUseContext().get(0); 2409 } 2410 2411 /** 2412 * @return {@link #jurisdiction} (A legal or geographic region in which the condition definition is intended to be used.) 2413 */ 2414 public List<CodeableConcept> getJurisdiction() { 2415 if (this.jurisdiction == null) 2416 this.jurisdiction = new ArrayList<CodeableConcept>(); 2417 return this.jurisdiction; 2418 } 2419 2420 /** 2421 * @return Returns a reference to <code>this</code> for easy method chaining 2422 */ 2423 public ConditionDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2424 this.jurisdiction = theJurisdiction; 2425 return this; 2426 } 2427 2428 public boolean hasJurisdiction() { 2429 if (this.jurisdiction == null) 2430 return false; 2431 for (CodeableConcept item : this.jurisdiction) 2432 if (!item.isEmpty()) 2433 return true; 2434 return false; 2435 } 2436 2437 public CodeableConcept addJurisdiction() { //3 2438 CodeableConcept t = new CodeableConcept(); 2439 if (this.jurisdiction == null) 2440 this.jurisdiction = new ArrayList<CodeableConcept>(); 2441 this.jurisdiction.add(t); 2442 return t; 2443 } 2444 2445 public ConditionDefinition addJurisdiction(CodeableConcept t) { //3 2446 if (t == null) 2447 return this; 2448 if (this.jurisdiction == null) 2449 this.jurisdiction = new ArrayList<CodeableConcept>(); 2450 this.jurisdiction.add(t); 2451 return this; 2452 } 2453 2454 /** 2455 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2456 */ 2457 public CodeableConcept getJurisdictionFirstRep() { 2458 if (getJurisdiction().isEmpty()) { 2459 addJurisdiction(); 2460 } 2461 return getJurisdiction().get(0); 2462 } 2463 2464 /** 2465 * @return {@link #code} (Identification of the condition, problem or diagnosis.) 2466 */ 2467 public CodeableConcept getCode() { 2468 if (this.code == null) 2469 if (Configuration.errorOnAutoCreate()) 2470 throw new Error("Attempt to auto-create ConditionDefinition.code"); 2471 else if (Configuration.doAutoCreate()) 2472 this.code = new CodeableConcept(); // cc 2473 return this.code; 2474 } 2475 2476 public boolean hasCode() { 2477 return this.code != null && !this.code.isEmpty(); 2478 } 2479 2480 /** 2481 * @param value {@link #code} (Identification of the condition, problem or diagnosis.) 2482 */ 2483 public ConditionDefinition setCode(CodeableConcept value) { 2484 this.code = value; 2485 return this; 2486 } 2487 2488 /** 2489 * @return {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 2490 */ 2491 public CodeableConcept getSeverity() { 2492 if (this.severity == null) 2493 if (Configuration.errorOnAutoCreate()) 2494 throw new Error("Attempt to auto-create ConditionDefinition.severity"); 2495 else if (Configuration.doAutoCreate()) 2496 this.severity = new CodeableConcept(); // cc 2497 return this.severity; 2498 } 2499 2500 public boolean hasSeverity() { 2501 return this.severity != null && !this.severity.isEmpty(); 2502 } 2503 2504 /** 2505 * @param value {@link #severity} (A subjective assessment of the severity of the condition as evaluated by the clinician.) 2506 */ 2507 public ConditionDefinition setSeverity(CodeableConcept value) { 2508 this.severity = value; 2509 return this; 2510 } 2511 2512 /** 2513 * @return {@link #bodySite} (The anatomical location where this condition manifests itself.) 2514 */ 2515 public CodeableConcept getBodySite() { 2516 if (this.bodySite == null) 2517 if (Configuration.errorOnAutoCreate()) 2518 throw new Error("Attempt to auto-create ConditionDefinition.bodySite"); 2519 else if (Configuration.doAutoCreate()) 2520 this.bodySite = new CodeableConcept(); // cc 2521 return this.bodySite; 2522 } 2523 2524 public boolean hasBodySite() { 2525 return this.bodySite != null && !this.bodySite.isEmpty(); 2526 } 2527 2528 /** 2529 * @param value {@link #bodySite} (The anatomical location where this condition manifests itself.) 2530 */ 2531 public ConditionDefinition setBodySite(CodeableConcept value) { 2532 this.bodySite = value; 2533 return this; 2534 } 2535 2536 /** 2537 * @return {@link #stage} (Clinical stage or grade of a condition. May include formal severity assessments.) 2538 */ 2539 public CodeableConcept getStage() { 2540 if (this.stage == null) 2541 if (Configuration.errorOnAutoCreate()) 2542 throw new Error("Attempt to auto-create ConditionDefinition.stage"); 2543 else if (Configuration.doAutoCreate()) 2544 this.stage = new CodeableConcept(); // cc 2545 return this.stage; 2546 } 2547 2548 public boolean hasStage() { 2549 return this.stage != null && !this.stage.isEmpty(); 2550 } 2551 2552 /** 2553 * @param value {@link #stage} (Clinical stage or grade of a condition. May include formal severity assessments.) 2554 */ 2555 public ConditionDefinition setStage(CodeableConcept value) { 2556 this.stage = value; 2557 return this; 2558 } 2559 2560 /** 2561 * @return {@link #hasSeverity} (Whether Severity is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasSeverity" gives direct access to the value 2562 */ 2563 public BooleanType getHasSeverityElement() { 2564 if (this.hasSeverity == null) 2565 if (Configuration.errorOnAutoCreate()) 2566 throw new Error("Attempt to auto-create ConditionDefinition.hasSeverity"); 2567 else if (Configuration.doAutoCreate()) 2568 this.hasSeverity = new BooleanType(); // bb 2569 return this.hasSeverity; 2570 } 2571 2572 public boolean hasHasSeverityElement() { 2573 return this.hasSeverity != null && !this.hasSeverity.isEmpty(); 2574 } 2575 2576 public boolean hasHasSeverity() { 2577 return this.hasSeverity != null && !this.hasSeverity.isEmpty(); 2578 } 2579 2580 /** 2581 * @param value {@link #hasSeverity} (Whether Severity is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasSeverity" gives direct access to the value 2582 */ 2583 public ConditionDefinition setHasSeverityElement(BooleanType value) { 2584 this.hasSeverity = value; 2585 return this; 2586 } 2587 2588 /** 2589 * @return Whether Severity is appropriate to collect for this condition. 2590 */ 2591 public boolean getHasSeverity() { 2592 return this.hasSeverity == null || this.hasSeverity.isEmpty() ? false : this.hasSeverity.getValue(); 2593 } 2594 2595 /** 2596 * @param value Whether Severity is appropriate to collect for this condition. 2597 */ 2598 public ConditionDefinition setHasSeverity(boolean value) { 2599 if (this.hasSeverity == null) 2600 this.hasSeverity = new BooleanType(); 2601 this.hasSeverity.setValue(value); 2602 return this; 2603 } 2604 2605 /** 2606 * @return {@link #hasBodySite} (Whether bodySite is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasBodySite" gives direct access to the value 2607 */ 2608 public BooleanType getHasBodySiteElement() { 2609 if (this.hasBodySite == null) 2610 if (Configuration.errorOnAutoCreate()) 2611 throw new Error("Attempt to auto-create ConditionDefinition.hasBodySite"); 2612 else if (Configuration.doAutoCreate()) 2613 this.hasBodySite = new BooleanType(); // bb 2614 return this.hasBodySite; 2615 } 2616 2617 public boolean hasHasBodySiteElement() { 2618 return this.hasBodySite != null && !this.hasBodySite.isEmpty(); 2619 } 2620 2621 public boolean hasHasBodySite() { 2622 return this.hasBodySite != null && !this.hasBodySite.isEmpty(); 2623 } 2624 2625 /** 2626 * @param value {@link #hasBodySite} (Whether bodySite is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasBodySite" gives direct access to the value 2627 */ 2628 public ConditionDefinition setHasBodySiteElement(BooleanType value) { 2629 this.hasBodySite = value; 2630 return this; 2631 } 2632 2633 /** 2634 * @return Whether bodySite is appropriate to collect for this condition. 2635 */ 2636 public boolean getHasBodySite() { 2637 return this.hasBodySite == null || this.hasBodySite.isEmpty() ? false : this.hasBodySite.getValue(); 2638 } 2639 2640 /** 2641 * @param value Whether bodySite is appropriate to collect for this condition. 2642 */ 2643 public ConditionDefinition setHasBodySite(boolean value) { 2644 if (this.hasBodySite == null) 2645 this.hasBodySite = new BooleanType(); 2646 this.hasBodySite.setValue(value); 2647 return this; 2648 } 2649 2650 /** 2651 * @return {@link #hasStage} (Whether stage is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasStage" gives direct access to the value 2652 */ 2653 public BooleanType getHasStageElement() { 2654 if (this.hasStage == null) 2655 if (Configuration.errorOnAutoCreate()) 2656 throw new Error("Attempt to auto-create ConditionDefinition.hasStage"); 2657 else if (Configuration.doAutoCreate()) 2658 this.hasStage = new BooleanType(); // bb 2659 return this.hasStage; 2660 } 2661 2662 public boolean hasHasStageElement() { 2663 return this.hasStage != null && !this.hasStage.isEmpty(); 2664 } 2665 2666 public boolean hasHasStage() { 2667 return this.hasStage != null && !this.hasStage.isEmpty(); 2668 } 2669 2670 /** 2671 * @param value {@link #hasStage} (Whether stage is appropriate to collect for this condition.). This is the underlying object with id, value and extensions. The accessor "getHasStage" gives direct access to the value 2672 */ 2673 public ConditionDefinition setHasStageElement(BooleanType value) { 2674 this.hasStage = value; 2675 return this; 2676 } 2677 2678 /** 2679 * @return Whether stage is appropriate to collect for this condition. 2680 */ 2681 public boolean getHasStage() { 2682 return this.hasStage == null || this.hasStage.isEmpty() ? false : this.hasStage.getValue(); 2683 } 2684 2685 /** 2686 * @param value Whether stage is appropriate to collect for this condition. 2687 */ 2688 public ConditionDefinition setHasStage(boolean value) { 2689 if (this.hasStage == null) 2690 this.hasStage = new BooleanType(); 2691 this.hasStage.setValue(value); 2692 return this; 2693 } 2694 2695 /** 2696 * @return {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2697 */ 2698 public List<UriType> getDefinition() { 2699 if (this.definition == null) 2700 this.definition = new ArrayList<UriType>(); 2701 return this.definition; 2702 } 2703 2704 /** 2705 * @return Returns a reference to <code>this</code> for easy method chaining 2706 */ 2707 public ConditionDefinition setDefinition(List<UriType> theDefinition) { 2708 this.definition = theDefinition; 2709 return this; 2710 } 2711 2712 public boolean hasDefinition() { 2713 if (this.definition == null) 2714 return false; 2715 for (UriType item : this.definition) 2716 if (!item.isEmpty()) 2717 return true; 2718 return false; 2719 } 2720 2721 /** 2722 * @return {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2723 */ 2724 public UriType addDefinitionElement() {//2 2725 UriType t = new UriType(); 2726 if (this.definition == null) 2727 this.definition = new ArrayList<UriType>(); 2728 this.definition.add(t); 2729 return t; 2730 } 2731 2732 /** 2733 * @param value {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2734 */ 2735 public ConditionDefinition addDefinition(String value) { //1 2736 UriType t = new UriType(); 2737 t.setValue(value); 2738 if (this.definition == null) 2739 this.definition = new ArrayList<UriType>(); 2740 this.definition.add(t); 2741 return this; 2742 } 2743 2744 /** 2745 * @param value {@link #definition} (Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.) 2746 */ 2747 public boolean hasDefinition(String value) { 2748 if (this.definition == null) 2749 return false; 2750 for (UriType v : this.definition) 2751 if (v.getValue().equals(value)) // uri 2752 return true; 2753 return false; 2754 } 2755 2756 /** 2757 * @return {@link #observation} (Observations particularly relevant to this condition.) 2758 */ 2759 public List<ConditionDefinitionObservationComponent> getObservation() { 2760 if (this.observation == null) 2761 this.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 2762 return this.observation; 2763 } 2764 2765 /** 2766 * @return Returns a reference to <code>this</code> for easy method chaining 2767 */ 2768 public ConditionDefinition setObservation(List<ConditionDefinitionObservationComponent> theObservation) { 2769 this.observation = theObservation; 2770 return this; 2771 } 2772 2773 public boolean hasObservation() { 2774 if (this.observation == null) 2775 return false; 2776 for (ConditionDefinitionObservationComponent item : this.observation) 2777 if (!item.isEmpty()) 2778 return true; 2779 return false; 2780 } 2781 2782 public ConditionDefinitionObservationComponent addObservation() { //3 2783 ConditionDefinitionObservationComponent t = new ConditionDefinitionObservationComponent(); 2784 if (this.observation == null) 2785 this.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 2786 this.observation.add(t); 2787 return t; 2788 } 2789 2790 public ConditionDefinition addObservation(ConditionDefinitionObservationComponent t) { //3 2791 if (t == null) 2792 return this; 2793 if (this.observation == null) 2794 this.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 2795 this.observation.add(t); 2796 return this; 2797 } 2798 2799 /** 2800 * @return The first repetition of repeating field {@link #observation}, creating it if it does not already exist {3} 2801 */ 2802 public ConditionDefinitionObservationComponent getObservationFirstRep() { 2803 if (getObservation().isEmpty()) { 2804 addObservation(); 2805 } 2806 return getObservation().get(0); 2807 } 2808 2809 /** 2810 * @return {@link #medication} (Medications particularly relevant for this condition.) 2811 */ 2812 public List<ConditionDefinitionMedicationComponent> getMedication() { 2813 if (this.medication == null) 2814 this.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 2815 return this.medication; 2816 } 2817 2818 /** 2819 * @return Returns a reference to <code>this</code> for easy method chaining 2820 */ 2821 public ConditionDefinition setMedication(List<ConditionDefinitionMedicationComponent> theMedication) { 2822 this.medication = theMedication; 2823 return this; 2824 } 2825 2826 public boolean hasMedication() { 2827 if (this.medication == null) 2828 return false; 2829 for (ConditionDefinitionMedicationComponent item : this.medication) 2830 if (!item.isEmpty()) 2831 return true; 2832 return false; 2833 } 2834 2835 public ConditionDefinitionMedicationComponent addMedication() { //3 2836 ConditionDefinitionMedicationComponent t = new ConditionDefinitionMedicationComponent(); 2837 if (this.medication == null) 2838 this.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 2839 this.medication.add(t); 2840 return t; 2841 } 2842 2843 public ConditionDefinition addMedication(ConditionDefinitionMedicationComponent t) { //3 2844 if (t == null) 2845 return this; 2846 if (this.medication == null) 2847 this.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 2848 this.medication.add(t); 2849 return this; 2850 } 2851 2852 /** 2853 * @return The first repetition of repeating field {@link #medication}, creating it if it does not already exist {3} 2854 */ 2855 public ConditionDefinitionMedicationComponent getMedicationFirstRep() { 2856 if (getMedication().isEmpty()) { 2857 addMedication(); 2858 } 2859 return getMedication().get(0); 2860 } 2861 2862 /** 2863 * @return {@link #precondition} (An observation that suggests that this condition applies.) 2864 */ 2865 public List<ConditionDefinitionPreconditionComponent> getPrecondition() { 2866 if (this.precondition == null) 2867 this.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 2868 return this.precondition; 2869 } 2870 2871 /** 2872 * @return Returns a reference to <code>this</code> for easy method chaining 2873 */ 2874 public ConditionDefinition setPrecondition(List<ConditionDefinitionPreconditionComponent> thePrecondition) { 2875 this.precondition = thePrecondition; 2876 return this; 2877 } 2878 2879 public boolean hasPrecondition() { 2880 if (this.precondition == null) 2881 return false; 2882 for (ConditionDefinitionPreconditionComponent item : this.precondition) 2883 if (!item.isEmpty()) 2884 return true; 2885 return false; 2886 } 2887 2888 public ConditionDefinitionPreconditionComponent addPrecondition() { //3 2889 ConditionDefinitionPreconditionComponent t = new ConditionDefinitionPreconditionComponent(); 2890 if (this.precondition == null) 2891 this.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 2892 this.precondition.add(t); 2893 return t; 2894 } 2895 2896 public ConditionDefinition addPrecondition(ConditionDefinitionPreconditionComponent t) { //3 2897 if (t == null) 2898 return this; 2899 if (this.precondition == null) 2900 this.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 2901 this.precondition.add(t); 2902 return this; 2903 } 2904 2905 /** 2906 * @return The first repetition of repeating field {@link #precondition}, creating it if it does not already exist {3} 2907 */ 2908 public ConditionDefinitionPreconditionComponent getPreconditionFirstRep() { 2909 if (getPrecondition().isEmpty()) { 2910 addPrecondition(); 2911 } 2912 return getPrecondition().get(0); 2913 } 2914 2915 /** 2916 * @return {@link #team} (Appropriate team for this condition.) 2917 */ 2918 public List<Reference> getTeam() { 2919 if (this.team == null) 2920 this.team = new ArrayList<Reference>(); 2921 return this.team; 2922 } 2923 2924 /** 2925 * @return Returns a reference to <code>this</code> for easy method chaining 2926 */ 2927 public ConditionDefinition setTeam(List<Reference> theTeam) { 2928 this.team = theTeam; 2929 return this; 2930 } 2931 2932 public boolean hasTeam() { 2933 if (this.team == null) 2934 return false; 2935 for (Reference item : this.team) 2936 if (!item.isEmpty()) 2937 return true; 2938 return false; 2939 } 2940 2941 public Reference addTeam() { //3 2942 Reference t = new Reference(); 2943 if (this.team == null) 2944 this.team = new ArrayList<Reference>(); 2945 this.team.add(t); 2946 return t; 2947 } 2948 2949 public ConditionDefinition addTeam(Reference t) { //3 2950 if (t == null) 2951 return this; 2952 if (this.team == null) 2953 this.team = new ArrayList<Reference>(); 2954 this.team.add(t); 2955 return this; 2956 } 2957 2958 /** 2959 * @return The first repetition of repeating field {@link #team}, creating it if it does not already exist {3} 2960 */ 2961 public Reference getTeamFirstRep() { 2962 if (getTeam().isEmpty()) { 2963 addTeam(); 2964 } 2965 return getTeam().get(0); 2966 } 2967 2968 /** 2969 * @return {@link #questionnaire} (Questionnaire for this condition.) 2970 */ 2971 public List<ConditionDefinitionQuestionnaireComponent> getQuestionnaire() { 2972 if (this.questionnaire == null) 2973 this.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 2974 return this.questionnaire; 2975 } 2976 2977 /** 2978 * @return Returns a reference to <code>this</code> for easy method chaining 2979 */ 2980 public ConditionDefinition setQuestionnaire(List<ConditionDefinitionQuestionnaireComponent> theQuestionnaire) { 2981 this.questionnaire = theQuestionnaire; 2982 return this; 2983 } 2984 2985 public boolean hasQuestionnaire() { 2986 if (this.questionnaire == null) 2987 return false; 2988 for (ConditionDefinitionQuestionnaireComponent item : this.questionnaire) 2989 if (!item.isEmpty()) 2990 return true; 2991 return false; 2992 } 2993 2994 public ConditionDefinitionQuestionnaireComponent addQuestionnaire() { //3 2995 ConditionDefinitionQuestionnaireComponent t = new ConditionDefinitionQuestionnaireComponent(); 2996 if (this.questionnaire == null) 2997 this.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 2998 this.questionnaire.add(t); 2999 return t; 3000 } 3001 3002 public ConditionDefinition addQuestionnaire(ConditionDefinitionQuestionnaireComponent t) { //3 3003 if (t == null) 3004 return this; 3005 if (this.questionnaire == null) 3006 this.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 3007 this.questionnaire.add(t); 3008 return this; 3009 } 3010 3011 /** 3012 * @return The first repetition of repeating field {@link #questionnaire}, creating it if it does not already exist {3} 3013 */ 3014 public ConditionDefinitionQuestionnaireComponent getQuestionnaireFirstRep() { 3015 if (getQuestionnaire().isEmpty()) { 3016 addQuestionnaire(); 3017 } 3018 return getQuestionnaire().get(0); 3019 } 3020 3021 /** 3022 * @return {@link #plan} (Plan that is appropriate.) 3023 */ 3024 public List<ConditionDefinitionPlanComponent> getPlan() { 3025 if (this.plan == null) 3026 this.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 3027 return this.plan; 3028 } 3029 3030 /** 3031 * @return Returns a reference to <code>this</code> for easy method chaining 3032 */ 3033 public ConditionDefinition setPlan(List<ConditionDefinitionPlanComponent> thePlan) { 3034 this.plan = thePlan; 3035 return this; 3036 } 3037 3038 public boolean hasPlan() { 3039 if (this.plan == null) 3040 return false; 3041 for (ConditionDefinitionPlanComponent item : this.plan) 3042 if (!item.isEmpty()) 3043 return true; 3044 return false; 3045 } 3046 3047 public ConditionDefinitionPlanComponent addPlan() { //3 3048 ConditionDefinitionPlanComponent t = new ConditionDefinitionPlanComponent(); 3049 if (this.plan == null) 3050 this.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 3051 this.plan.add(t); 3052 return t; 3053 } 3054 3055 public ConditionDefinition addPlan(ConditionDefinitionPlanComponent t) { //3 3056 if (t == null) 3057 return this; 3058 if (this.plan == null) 3059 this.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 3060 this.plan.add(t); 3061 return this; 3062 } 3063 3064 /** 3065 * @return The first repetition of repeating field {@link #plan}, creating it if it does not already exist {3} 3066 */ 3067 public ConditionDefinitionPlanComponent getPlanFirstRep() { 3068 if (getPlan().isEmpty()) { 3069 addPlan(); 3070 } 3071 return getPlan().get(0); 3072 } 3073 3074 /** 3075 * not supported on this implementation 3076 */ 3077 @Override 3078 public int getPurposeMax() { 3079 return 0; 3080 } 3081 /** 3082 * @return {@link #purpose} (Explanation of why this condition definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3083 */ 3084 public MarkdownType getPurposeElement() { 3085 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3086 } 3087 3088 public boolean hasPurposeElement() { 3089 return false; 3090 } 3091 public boolean hasPurpose() { 3092 return false; 3093 } 3094 3095 /** 3096 * @param value {@link #purpose} (Explanation of why this condition definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3097 */ 3098 public ConditionDefinition setPurposeElement(MarkdownType value) { 3099 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3100 } 3101 public String getPurpose() { 3102 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3103 } 3104 /** 3105 * @param value Explanation of why this condition definition is needed and why it has been designed as it has. 3106 */ 3107 public ConditionDefinition setPurpose(String value) { 3108 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"purpose\""); 3109 } 3110 /** 3111 * not supported on this implementation 3112 */ 3113 @Override 3114 public int getCopyrightMax() { 3115 return 0; 3116 } 3117 /** 3118 * @return {@link #copyright} (A copyright statement relating to the condition definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3119 */ 3120 public MarkdownType getCopyrightElement() { 3121 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3122 } 3123 3124 public boolean hasCopyrightElement() { 3125 return false; 3126 } 3127 public boolean hasCopyright() { 3128 return false; 3129 } 3130 3131 /** 3132 * @param value {@link #copyright} (A copyright statement relating to the condition definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the condition definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3133 */ 3134 public ConditionDefinition setCopyrightElement(MarkdownType value) { 3135 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3136 } 3137 public String getCopyright() { 3138 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3139 } 3140 /** 3141 * @param value A copyright statement relating to the condition definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the condition definition. 3142 */ 3143 public ConditionDefinition setCopyright(String value) { 3144 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyright\""); 3145 } 3146 /** 3147 * not supported on this implementation 3148 */ 3149 @Override 3150 public int getCopyrightLabelMax() { 3151 return 0; 3152 } 3153 /** 3154 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3155 */ 3156 public StringType getCopyrightLabelElement() { 3157 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3158 } 3159 3160 public boolean hasCopyrightLabelElement() { 3161 return false; 3162 } 3163 public boolean hasCopyrightLabel() { 3164 return false; 3165 } 3166 3167 /** 3168 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3169 */ 3170 public ConditionDefinition setCopyrightLabelElement(StringType value) { 3171 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3172 } 3173 public String getCopyrightLabel() { 3174 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3175 } 3176 /** 3177 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3178 */ 3179 public ConditionDefinition setCopyrightLabel(String value) { 3180 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"copyrightLabel\""); 3181 } 3182 /** 3183 * not supported on this implementation 3184 */ 3185 @Override 3186 public int getApprovalDateMax() { 3187 return 0; 3188 } 3189 /** 3190 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3191 */ 3192 public DateType getApprovalDateElement() { 3193 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3194 } 3195 3196 public boolean hasApprovalDateElement() { 3197 return false; 3198 } 3199 public boolean hasApprovalDate() { 3200 return false; 3201 } 3202 3203 /** 3204 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3205 */ 3206 public ConditionDefinition setApprovalDateElement(DateType value) { 3207 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3208 } 3209 public Date getApprovalDate() { 3210 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3211 } 3212 /** 3213 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3214 */ 3215 public ConditionDefinition setApprovalDate(Date value) { 3216 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"approvalDate\""); 3217 } 3218 /** 3219 * not supported on this implementation 3220 */ 3221 @Override 3222 public int getLastReviewDateMax() { 3223 return 0; 3224 } 3225 /** 3226 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3227 */ 3228 public DateType getLastReviewDateElement() { 3229 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3230 } 3231 3232 public boolean hasLastReviewDateElement() { 3233 return false; 3234 } 3235 public boolean hasLastReviewDate() { 3236 return false; 3237 } 3238 3239 /** 3240 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3241 */ 3242 public ConditionDefinition setLastReviewDateElement(DateType value) { 3243 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3244 } 3245 public Date getLastReviewDate() { 3246 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3247 } 3248 /** 3249 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3250 */ 3251 public ConditionDefinition setLastReviewDate(Date value) { 3252 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"lastReviewDate\""); 3253 } 3254 /** 3255 * not supported on this implementation 3256 */ 3257 @Override 3258 public int getEffectivePeriodMax() { 3259 return 0; 3260 } 3261 /** 3262 * @return {@link #effectivePeriod} (The period during which the condition definition content was or is planned to be in active use.) 3263 */ 3264 public Period getEffectivePeriod() { 3265 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"effectivePeriod\""); 3266 } 3267 public boolean hasEffectivePeriod() { 3268 return false; 3269 } 3270 /** 3271 * @param value {@link #effectivePeriod} (The period during which the condition definition content was or is planned to be in active use.) 3272 */ 3273 public ConditionDefinition setEffectivePeriod(Period value) { 3274 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"effectivePeriod\""); 3275 } 3276 3277 /** 3278 * not supported on this implementation 3279 */ 3280 @Override 3281 public int getTopicMax() { 3282 return 0; 3283 } 3284 /** 3285 * @return {@link #topic} (Descriptive topics related to the content of the condition definition. Topics provide a high-level categorization as well as keywords for the condition definition that can be useful for filtering and searching.) 3286 */ 3287 public List<CodeableConcept> getTopic() { 3288 return new ArrayList<>(); 3289 } 3290 /** 3291 * @return Returns a reference to <code>this</code> for easy method chaining 3292 */ 3293 public ConditionDefinition setTopic(List<CodeableConcept> theTopic) { 3294 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3295 } 3296 public boolean hasTopic() { 3297 return false; 3298 } 3299 3300 public CodeableConcept addTopic() { //3 3301 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3302 } 3303 public ConditionDefinition addTopic(CodeableConcept t) { //3 3304 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3305 } 3306 /** 3307 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 3308 */ 3309 public CodeableConcept getTopicFirstRep() { 3310 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"topic\""); 3311 } 3312 /** 3313 * not supported on this implementation 3314 */ 3315 @Override 3316 public int getAuthorMax() { 3317 return 0; 3318 } 3319 /** 3320 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the condition definition.) 3321 */ 3322 public List<ContactDetail> getAuthor() { 3323 return new ArrayList<>(); 3324 } 3325 /** 3326 * @return Returns a reference to <code>this</code> for easy method chaining 3327 */ 3328 public ConditionDefinition setAuthor(List<ContactDetail> theAuthor) { 3329 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3330 } 3331 public boolean hasAuthor() { 3332 return false; 3333 } 3334 3335 public ContactDetail addAuthor() { //3 3336 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3337 } 3338 public ConditionDefinition addAuthor(ContactDetail t) { //3 3339 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3340 } 3341 /** 3342 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {2} 3343 */ 3344 public ContactDetail getAuthorFirstRep() { 3345 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"author\""); 3346 } 3347 /** 3348 * not supported on this implementation 3349 */ 3350 @Override 3351 public int getEditorMax() { 3352 return 0; 3353 } 3354 /** 3355 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the condition definition.) 3356 */ 3357 public List<ContactDetail> getEditor() { 3358 return new ArrayList<>(); 3359 } 3360 /** 3361 * @return Returns a reference to <code>this</code> for easy method chaining 3362 */ 3363 public ConditionDefinition setEditor(List<ContactDetail> theEditor) { 3364 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3365 } 3366 public boolean hasEditor() { 3367 return false; 3368 } 3369 3370 public ContactDetail addEditor() { //3 3371 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3372 } 3373 public ConditionDefinition addEditor(ContactDetail t) { //3 3374 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3375 } 3376 /** 3377 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {2} 3378 */ 3379 public ContactDetail getEditorFirstRep() { 3380 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"editor\""); 3381 } 3382 /** 3383 * not supported on this implementation 3384 */ 3385 @Override 3386 public int getReviewerMax() { 3387 return 0; 3388 } 3389 /** 3390 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the condition definition.) 3391 */ 3392 public List<ContactDetail> getReviewer() { 3393 return new ArrayList<>(); 3394 } 3395 /** 3396 * @return Returns a reference to <code>this</code> for easy method chaining 3397 */ 3398 public ConditionDefinition setReviewer(List<ContactDetail> theReviewer) { 3399 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3400 } 3401 public boolean hasReviewer() { 3402 return false; 3403 } 3404 3405 public ContactDetail addReviewer() { //3 3406 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3407 } 3408 public ConditionDefinition addReviewer(ContactDetail t) { //3 3409 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3410 } 3411 /** 3412 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {2} 3413 */ 3414 public ContactDetail getReviewerFirstRep() { 3415 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"reviewer\""); 3416 } 3417 /** 3418 * not supported on this implementation 3419 */ 3420 @Override 3421 public int getEndorserMax() { 3422 return 0; 3423 } 3424 /** 3425 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the condition definition for use in some setting.) 3426 */ 3427 public List<ContactDetail> getEndorser() { 3428 return new ArrayList<>(); 3429 } 3430 /** 3431 * @return Returns a reference to <code>this</code> for easy method chaining 3432 */ 3433 public ConditionDefinition setEndorser(List<ContactDetail> theEndorser) { 3434 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3435 } 3436 public boolean hasEndorser() { 3437 return false; 3438 } 3439 3440 public ContactDetail addEndorser() { //3 3441 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3442 } 3443 public ConditionDefinition addEndorser(ContactDetail t) { //3 3444 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3445 } 3446 /** 3447 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {2} 3448 */ 3449 public ContactDetail getEndorserFirstRep() { 3450 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"endorser\""); 3451 } 3452 /** 3453 * not supported on this implementation 3454 */ 3455 @Override 3456 public int getRelatedArtifactMax() { 3457 return 0; 3458 } 3459 /** 3460 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, dependencies, bibliographic references, and predecessor and successor artifacts.) 3461 */ 3462 public List<RelatedArtifact> getRelatedArtifact() { 3463 return new ArrayList<>(); 3464 } 3465 /** 3466 * @return Returns a reference to <code>this</code> for easy method chaining 3467 */ 3468 public ConditionDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3469 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3470 } 3471 public boolean hasRelatedArtifact() { 3472 return false; 3473 } 3474 3475 public RelatedArtifact addRelatedArtifact() { //3 3476 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3477 } 3478 public ConditionDefinition addRelatedArtifact(RelatedArtifact t) { //3 3479 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3480 } 3481 /** 3482 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {2} 3483 */ 3484 public RelatedArtifact getRelatedArtifactFirstRep() { 3485 throw new Error("The resource type \"ConditionDefinition\" does not implement the property \"relatedArtifact\""); 3486 } 3487 protected void listChildren(List<Property> children) { 3488 super.listChildren(children); 3489 children.add(new Property("url", "uri", "An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.", 0, 1, url)); 3490 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3491 children.add(new Property("version", "string", "The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3492 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3493 children.add(new Property("name", "string", "A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3494 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the condition definition.", 0, 1, title)); 3495 children.add(new Property("subtitle", "string", "An explanatory or alternate title for the event definition giving additional information about its content.", 0, 1, subtitle)); 3496 children.add(new Property("status", "code", "The status of this condition definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3497 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 3498 children.add(new Property("date", "dateTime", "The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.", 0, 1, date)); 3499 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.", 0, 1, publisher)); 3500 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3501 children.add(new Property("description", "markdown", "A free text natural language description of the condition definition from a consumer's perspective.", 0, 1, description)); 3502 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3503 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the condition definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3504 children.add(new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code)); 3505 children.add(new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity)); 3506 children.add(new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, 1, bodySite)); 3507 children.add(new Property("stage", "CodeableConcept", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1, stage)); 3508 children.add(new Property("hasSeverity", "boolean", "Whether Severity is appropriate to collect for this condition.", 0, 1, hasSeverity)); 3509 children.add(new Property("hasBodySite", "boolean", "Whether bodySite is appropriate to collect for this condition.", 0, 1, hasBodySite)); 3510 children.add(new Property("hasStage", "boolean", "Whether stage is appropriate to collect for this condition.", 0, 1, hasStage)); 3511 children.add(new Property("definition", "uri", "Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.", 0, java.lang.Integer.MAX_VALUE, definition)); 3512 children.add(new Property("observation", "", "Observations particularly relevant to this condition.", 0, java.lang.Integer.MAX_VALUE, observation)); 3513 children.add(new Property("medication", "", "Medications particularly relevant for this condition.", 0, java.lang.Integer.MAX_VALUE, medication)); 3514 children.add(new Property("precondition", "", "An observation that suggests that this condition applies.", 0, java.lang.Integer.MAX_VALUE, precondition)); 3515 children.add(new Property("team", "Reference(CareTeam)", "Appropriate team for this condition.", 0, java.lang.Integer.MAX_VALUE, team)); 3516 children.add(new Property("questionnaire", "", "Questionnaire for this condition.", 0, java.lang.Integer.MAX_VALUE, questionnaire)); 3517 children.add(new Property("plan", "", "Plan that is appropriate.", 0, java.lang.Integer.MAX_VALUE, plan)); 3518 } 3519 3520 @Override 3521 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3522 switch (_hash) { 3523 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this condition definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this condition definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the condition definition is stored on different servers.", 0, 1, url); 3524 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this condition definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3525 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the condition definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the condition definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3526 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3527 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3528 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3529 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3530 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the condition definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3531 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the condition definition.", 0, 1, title); 3532 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "An explanatory or alternate title for the event definition giving additional information about its content.", 0, 1, subtitle); 3533 case -892481550: /*status*/ return new Property("status", "code", "The status of this condition definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3534 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this condition definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 3535 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the condition definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the condition definition changes.", 0, 1, date); 3536 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the condition definition.", 0, 1, publisher); 3537 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3538 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the condition definition from a consumer's perspective.", 0, 1, description); 3539 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate condition definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3540 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the condition definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3541 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code); 3542 case 1478300413: /*severity*/ return new Property("severity", "CodeableConcept", "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity); 3543 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "The anatomical location where this condition manifests itself.", 0, 1, bodySite); 3544 case 109757182: /*stage*/ return new Property("stage", "CodeableConcept", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1, stage); 3545 case 57790391: /*hasSeverity*/ return new Property("hasSeverity", "boolean", "Whether Severity is appropriate to collect for this condition.", 0, 1, hasSeverity); 3546 case 282110147: /*hasBodySite*/ return new Property("hasBodySite", "boolean", "Whether bodySite is appropriate to collect for this condition.", 0, 1, hasBodySite); 3547 case 129749124: /*hasStage*/ return new Property("hasStage", "boolean", "Whether stage is appropriate to collect for this condition.", 0, 1, hasStage); 3548 case -1014418093: /*definition*/ return new Property("definition", "uri", "Formal definitions of the condition. These may be references to ontologies, published clinical protocols or research papers.", 0, java.lang.Integer.MAX_VALUE, definition); 3549 case 122345516: /*observation*/ return new Property("observation", "", "Observations particularly relevant to this condition.", 0, java.lang.Integer.MAX_VALUE, observation); 3550 case 1998965455: /*medication*/ return new Property("medication", "", "Medications particularly relevant for this condition.", 0, java.lang.Integer.MAX_VALUE, medication); 3551 case -650968616: /*precondition*/ return new Property("precondition", "", "An observation that suggests that this condition applies.", 0, java.lang.Integer.MAX_VALUE, precondition); 3552 case 3555933: /*team*/ return new Property("team", "Reference(CareTeam)", "Appropriate team for this condition.", 0, java.lang.Integer.MAX_VALUE, team); 3553 case -1017049693: /*questionnaire*/ return new Property("questionnaire", "", "Questionnaire for this condition.", 0, java.lang.Integer.MAX_VALUE, questionnaire); 3554 case 3443497: /*plan*/ return new Property("plan", "", "Plan that is appropriate.", 0, java.lang.Integer.MAX_VALUE, plan); 3555 default: return super.getNamedProperty(_hash, _name, _checkValid); 3556 } 3557 3558 } 3559 3560 @Override 3561 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3562 switch (hash) { 3563 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3564 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3565 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3566 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3567 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3568 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3569 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 3570 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3571 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3572 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3573 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3574 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3575 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3576 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3577 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3578 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3579 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // CodeableConcept 3580 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 3581 case 109757182: /*stage*/ return this.stage == null ? new Base[0] : new Base[] {this.stage}; // CodeableConcept 3582 case 57790391: /*hasSeverity*/ return this.hasSeverity == null ? new Base[0] : new Base[] {this.hasSeverity}; // BooleanType 3583 case 282110147: /*hasBodySite*/ return this.hasBodySite == null ? new Base[0] : new Base[] {this.hasBodySite}; // BooleanType 3584 case 129749124: /*hasStage*/ return this.hasStage == null ? new Base[0] : new Base[] {this.hasStage}; // BooleanType 3585 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // UriType 3586 case 122345516: /*observation*/ return this.observation == null ? new Base[0] : this.observation.toArray(new Base[this.observation.size()]); // ConditionDefinitionObservationComponent 3587 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : this.medication.toArray(new Base[this.medication.size()]); // ConditionDefinitionMedicationComponent 3588 case -650968616: /*precondition*/ return this.precondition == null ? new Base[0] : this.precondition.toArray(new Base[this.precondition.size()]); // ConditionDefinitionPreconditionComponent 3589 case 3555933: /*team*/ return this.team == null ? new Base[0] : this.team.toArray(new Base[this.team.size()]); // Reference 3590 case -1017049693: /*questionnaire*/ return this.questionnaire == null ? new Base[0] : this.questionnaire.toArray(new Base[this.questionnaire.size()]); // ConditionDefinitionQuestionnaireComponent 3591 case 3443497: /*plan*/ return this.plan == null ? new Base[0] : this.plan.toArray(new Base[this.plan.size()]); // ConditionDefinitionPlanComponent 3592 default: return super.getProperty(hash, name, checkValid); 3593 } 3594 3595 } 3596 3597 @Override 3598 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3599 switch (hash) { 3600 case 116079: // url 3601 this.url = TypeConvertor.castToUri(value); // UriType 3602 return value; 3603 case -1618432855: // identifier 3604 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3605 return value; 3606 case 351608024: // version 3607 this.version = TypeConvertor.castToString(value); // StringType 3608 return value; 3609 case 1508158071: // versionAlgorithm 3610 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3611 return value; 3612 case 3373707: // name 3613 this.name = TypeConvertor.castToString(value); // StringType 3614 return value; 3615 case 110371416: // title 3616 this.title = TypeConvertor.castToString(value); // StringType 3617 return value; 3618 case -2060497896: // subtitle 3619 this.subtitle = TypeConvertor.castToString(value); // StringType 3620 return value; 3621 case -892481550: // status 3622 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3623 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3624 return value; 3625 case -404562712: // experimental 3626 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3627 return value; 3628 case 3076014: // date 3629 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3630 return value; 3631 case 1447404028: // publisher 3632 this.publisher = TypeConvertor.castToString(value); // StringType 3633 return value; 3634 case 951526432: // contact 3635 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3636 return value; 3637 case -1724546052: // description 3638 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3639 return value; 3640 case -669707736: // useContext 3641 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3642 return value; 3643 case -507075711: // jurisdiction 3644 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3645 return value; 3646 case 3059181: // code 3647 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3648 return value; 3649 case 1478300413: // severity 3650 this.severity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3651 return value; 3652 case 1702620169: // bodySite 3653 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3654 return value; 3655 case 109757182: // stage 3656 this.stage = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3657 return value; 3658 case 57790391: // hasSeverity 3659 this.hasSeverity = TypeConvertor.castToBoolean(value); // BooleanType 3660 return value; 3661 case 282110147: // hasBodySite 3662 this.hasBodySite = TypeConvertor.castToBoolean(value); // BooleanType 3663 return value; 3664 case 129749124: // hasStage 3665 this.hasStage = TypeConvertor.castToBoolean(value); // BooleanType 3666 return value; 3667 case -1014418093: // definition 3668 this.getDefinition().add(TypeConvertor.castToUri(value)); // UriType 3669 return value; 3670 case 122345516: // observation 3671 this.getObservation().add((ConditionDefinitionObservationComponent) value); // ConditionDefinitionObservationComponent 3672 return value; 3673 case 1998965455: // medication 3674 this.getMedication().add((ConditionDefinitionMedicationComponent) value); // ConditionDefinitionMedicationComponent 3675 return value; 3676 case -650968616: // precondition 3677 this.getPrecondition().add((ConditionDefinitionPreconditionComponent) value); // ConditionDefinitionPreconditionComponent 3678 return value; 3679 case 3555933: // team 3680 this.getTeam().add(TypeConvertor.castToReference(value)); // Reference 3681 return value; 3682 case -1017049693: // questionnaire 3683 this.getQuestionnaire().add((ConditionDefinitionQuestionnaireComponent) value); // ConditionDefinitionQuestionnaireComponent 3684 return value; 3685 case 3443497: // plan 3686 this.getPlan().add((ConditionDefinitionPlanComponent) value); // ConditionDefinitionPlanComponent 3687 return value; 3688 default: return super.setProperty(hash, name, value); 3689 } 3690 3691 } 3692 3693 @Override 3694 public Base setProperty(String name, Base value) throws FHIRException { 3695 if (name.equals("url")) { 3696 this.url = TypeConvertor.castToUri(value); // UriType 3697 } else if (name.equals("identifier")) { 3698 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3699 } else if (name.equals("version")) { 3700 this.version = TypeConvertor.castToString(value); // StringType 3701 } else if (name.equals("versionAlgorithm[x]")) { 3702 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3703 } else if (name.equals("name")) { 3704 this.name = TypeConvertor.castToString(value); // StringType 3705 } else if (name.equals("title")) { 3706 this.title = TypeConvertor.castToString(value); // StringType 3707 } else if (name.equals("subtitle")) { 3708 this.subtitle = TypeConvertor.castToString(value); // StringType 3709 } else if (name.equals("status")) { 3710 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3711 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3712 } else if (name.equals("experimental")) { 3713 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3714 } else if (name.equals("date")) { 3715 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3716 } else if (name.equals("publisher")) { 3717 this.publisher = TypeConvertor.castToString(value); // StringType 3718 } else if (name.equals("contact")) { 3719 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3720 } else if (name.equals("description")) { 3721 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3722 } else if (name.equals("useContext")) { 3723 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 3724 } else if (name.equals("jurisdiction")) { 3725 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3726 } else if (name.equals("code")) { 3727 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3728 } else if (name.equals("severity")) { 3729 this.severity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3730 } else if (name.equals("bodySite")) { 3731 this.bodySite = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3732 } else if (name.equals("stage")) { 3733 this.stage = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3734 } else if (name.equals("hasSeverity")) { 3735 this.hasSeverity = TypeConvertor.castToBoolean(value); // BooleanType 3736 } else if (name.equals("hasBodySite")) { 3737 this.hasBodySite = TypeConvertor.castToBoolean(value); // BooleanType 3738 } else if (name.equals("hasStage")) { 3739 this.hasStage = TypeConvertor.castToBoolean(value); // BooleanType 3740 } else if (name.equals("definition")) { 3741 this.getDefinition().add(TypeConvertor.castToUri(value)); 3742 } else if (name.equals("observation")) { 3743 this.getObservation().add((ConditionDefinitionObservationComponent) value); 3744 } else if (name.equals("medication")) { 3745 this.getMedication().add((ConditionDefinitionMedicationComponent) value); 3746 } else if (name.equals("precondition")) { 3747 this.getPrecondition().add((ConditionDefinitionPreconditionComponent) value); 3748 } else if (name.equals("team")) { 3749 this.getTeam().add(TypeConvertor.castToReference(value)); 3750 } else if (name.equals("questionnaire")) { 3751 this.getQuestionnaire().add((ConditionDefinitionQuestionnaireComponent) value); 3752 } else if (name.equals("plan")) { 3753 this.getPlan().add((ConditionDefinitionPlanComponent) value); 3754 } else 3755 return super.setProperty(name, value); 3756 return value; 3757 } 3758 3759 @Override 3760 public void removeChild(String name, Base value) throws FHIRException { 3761 if (name.equals("url")) { 3762 this.url = null; 3763 } else if (name.equals("identifier")) { 3764 this.getIdentifier().remove(value); 3765 } else if (name.equals("version")) { 3766 this.version = null; 3767 } else if (name.equals("versionAlgorithm[x]")) { 3768 this.versionAlgorithm = null; 3769 } else if (name.equals("name")) { 3770 this.name = null; 3771 } else if (name.equals("title")) { 3772 this.title = null; 3773 } else if (name.equals("subtitle")) { 3774 this.subtitle = null; 3775 } else if (name.equals("status")) { 3776 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3777 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3778 } else if (name.equals("experimental")) { 3779 this.experimental = null; 3780 } else if (name.equals("date")) { 3781 this.date = null; 3782 } else if (name.equals("publisher")) { 3783 this.publisher = null; 3784 } else if (name.equals("contact")) { 3785 this.getContact().remove(value); 3786 } else if (name.equals("description")) { 3787 this.description = null; 3788 } else if (name.equals("useContext")) { 3789 this.getUseContext().remove(value); 3790 } else if (name.equals("jurisdiction")) { 3791 this.getJurisdiction().remove(value); 3792 } else if (name.equals("code")) { 3793 this.code = null; 3794 } else if (name.equals("severity")) { 3795 this.severity = null; 3796 } else if (name.equals("bodySite")) { 3797 this.bodySite = null; 3798 } else if (name.equals("stage")) { 3799 this.stage = null; 3800 } else if (name.equals("hasSeverity")) { 3801 this.hasSeverity = null; 3802 } else if (name.equals("hasBodySite")) { 3803 this.hasBodySite = null; 3804 } else if (name.equals("hasStage")) { 3805 this.hasStage = null; 3806 } else if (name.equals("definition")) { 3807 this.getDefinition().remove(value); 3808 } else if (name.equals("observation")) { 3809 this.getObservation().remove((ConditionDefinitionObservationComponent) value); 3810 } else if (name.equals("medication")) { 3811 this.getMedication().remove((ConditionDefinitionMedicationComponent) value); 3812 } else if (name.equals("precondition")) { 3813 this.getPrecondition().remove((ConditionDefinitionPreconditionComponent) value); 3814 } else if (name.equals("team")) { 3815 this.getTeam().remove(value); 3816 } else if (name.equals("questionnaire")) { 3817 this.getQuestionnaire().remove((ConditionDefinitionQuestionnaireComponent) value); 3818 } else if (name.equals("plan")) { 3819 this.getPlan().remove((ConditionDefinitionPlanComponent) value); 3820 } else 3821 super.removeChild(name, value); 3822 3823 } 3824 3825 @Override 3826 public Base makeProperty(int hash, String name) throws FHIRException { 3827 switch (hash) { 3828 case 116079: return getUrlElement(); 3829 case -1618432855: return addIdentifier(); 3830 case 351608024: return getVersionElement(); 3831 case -115699031: return getVersionAlgorithm(); 3832 case 1508158071: return getVersionAlgorithm(); 3833 case 3373707: return getNameElement(); 3834 case 110371416: return getTitleElement(); 3835 case -2060497896: return getSubtitleElement(); 3836 case -892481550: return getStatusElement(); 3837 case -404562712: return getExperimentalElement(); 3838 case 3076014: return getDateElement(); 3839 case 1447404028: return getPublisherElement(); 3840 case 951526432: return addContact(); 3841 case -1724546052: return getDescriptionElement(); 3842 case -669707736: return addUseContext(); 3843 case -507075711: return addJurisdiction(); 3844 case 3059181: return getCode(); 3845 case 1478300413: return getSeverity(); 3846 case 1702620169: return getBodySite(); 3847 case 109757182: return getStage(); 3848 case 57790391: return getHasSeverityElement(); 3849 case 282110147: return getHasBodySiteElement(); 3850 case 129749124: return getHasStageElement(); 3851 case -1014418093: return addDefinitionElement(); 3852 case 122345516: return addObservation(); 3853 case 1998965455: return addMedication(); 3854 case -650968616: return addPrecondition(); 3855 case 3555933: return addTeam(); 3856 case -1017049693: return addQuestionnaire(); 3857 case 3443497: return addPlan(); 3858 default: return super.makeProperty(hash, name); 3859 } 3860 3861 } 3862 3863 @Override 3864 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3865 switch (hash) { 3866 case 116079: /*url*/ return new String[] {"uri"}; 3867 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3868 case 351608024: /*version*/ return new String[] {"string"}; 3869 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3870 case 3373707: /*name*/ return new String[] {"string"}; 3871 case 110371416: /*title*/ return new String[] {"string"}; 3872 case -2060497896: /*subtitle*/ return new String[] {"string"}; 3873 case -892481550: /*status*/ return new String[] {"code"}; 3874 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3875 case 3076014: /*date*/ return new String[] {"dateTime"}; 3876 case 1447404028: /*publisher*/ return new String[] {"string"}; 3877 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3878 case -1724546052: /*description*/ return new String[] {"markdown"}; 3879 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3880 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3881 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3882 case 1478300413: /*severity*/ return new String[] {"CodeableConcept"}; 3883 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 3884 case 109757182: /*stage*/ return new String[] {"CodeableConcept"}; 3885 case 57790391: /*hasSeverity*/ return new String[] {"boolean"}; 3886 case 282110147: /*hasBodySite*/ return new String[] {"boolean"}; 3887 case 129749124: /*hasStage*/ return new String[] {"boolean"}; 3888 case -1014418093: /*definition*/ return new String[] {"uri"}; 3889 case 122345516: /*observation*/ return new String[] {}; 3890 case 1998965455: /*medication*/ return new String[] {}; 3891 case -650968616: /*precondition*/ return new String[] {}; 3892 case 3555933: /*team*/ return new String[] {"Reference"}; 3893 case -1017049693: /*questionnaire*/ return new String[] {}; 3894 case 3443497: /*plan*/ return new String[] {}; 3895 default: return super.getTypesForProperty(hash, name); 3896 } 3897 3898 } 3899 3900 @Override 3901 public Base addChild(String name) throws FHIRException { 3902 if (name.equals("url")) { 3903 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.url"); 3904 } 3905 else if (name.equals("identifier")) { 3906 return addIdentifier(); 3907 } 3908 else if (name.equals("version")) { 3909 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.version"); 3910 } 3911 else if (name.equals("versionAlgorithmString")) { 3912 this.versionAlgorithm = new StringType(); 3913 return this.versionAlgorithm; 3914 } 3915 else if (name.equals("versionAlgorithmCoding")) { 3916 this.versionAlgorithm = new Coding(); 3917 return this.versionAlgorithm; 3918 } 3919 else if (name.equals("name")) { 3920 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.name"); 3921 } 3922 else if (name.equals("title")) { 3923 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.title"); 3924 } 3925 else if (name.equals("subtitle")) { 3926 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.subtitle"); 3927 } 3928 else if (name.equals("status")) { 3929 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.status"); 3930 } 3931 else if (name.equals("experimental")) { 3932 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.experimental"); 3933 } 3934 else if (name.equals("date")) { 3935 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.date"); 3936 } 3937 else if (name.equals("publisher")) { 3938 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.publisher"); 3939 } 3940 else if (name.equals("contact")) { 3941 return addContact(); 3942 } 3943 else if (name.equals("description")) { 3944 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.description"); 3945 } 3946 else if (name.equals("useContext")) { 3947 return addUseContext(); 3948 } 3949 else if (name.equals("jurisdiction")) { 3950 return addJurisdiction(); 3951 } 3952 else if (name.equals("code")) { 3953 this.code = new CodeableConcept(); 3954 return this.code; 3955 } 3956 else if (name.equals("severity")) { 3957 this.severity = new CodeableConcept(); 3958 return this.severity; 3959 } 3960 else if (name.equals("bodySite")) { 3961 this.bodySite = new CodeableConcept(); 3962 return this.bodySite; 3963 } 3964 else if (name.equals("stage")) { 3965 this.stage = new CodeableConcept(); 3966 return this.stage; 3967 } 3968 else if (name.equals("hasSeverity")) { 3969 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.hasSeverity"); 3970 } 3971 else if (name.equals("hasBodySite")) { 3972 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.hasBodySite"); 3973 } 3974 else if (name.equals("hasStage")) { 3975 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.hasStage"); 3976 } 3977 else if (name.equals("definition")) { 3978 throw new FHIRException("Cannot call addChild on a singleton property ConditionDefinition.definition"); 3979 } 3980 else if (name.equals("observation")) { 3981 return addObservation(); 3982 } 3983 else if (name.equals("medication")) { 3984 return addMedication(); 3985 } 3986 else if (name.equals("precondition")) { 3987 return addPrecondition(); 3988 } 3989 else if (name.equals("team")) { 3990 return addTeam(); 3991 } 3992 else if (name.equals("questionnaire")) { 3993 return addQuestionnaire(); 3994 } 3995 else if (name.equals("plan")) { 3996 return addPlan(); 3997 } 3998 else 3999 return super.addChild(name); 4000 } 4001 4002 public String fhirType() { 4003 return "ConditionDefinition"; 4004 4005 } 4006 4007 public ConditionDefinition copy() { 4008 ConditionDefinition dst = new ConditionDefinition(); 4009 copyValues(dst); 4010 return dst; 4011 } 4012 4013 public void copyValues(ConditionDefinition dst) { 4014 super.copyValues(dst); 4015 dst.url = url == null ? null : url.copy(); 4016 if (identifier != null) { 4017 dst.identifier = new ArrayList<Identifier>(); 4018 for (Identifier i : identifier) 4019 dst.identifier.add(i.copy()); 4020 }; 4021 dst.version = version == null ? null : version.copy(); 4022 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4023 dst.name = name == null ? null : name.copy(); 4024 dst.title = title == null ? null : title.copy(); 4025 dst.subtitle = subtitle == null ? null : subtitle.copy(); 4026 dst.status = status == null ? null : status.copy(); 4027 dst.experimental = experimental == null ? null : experimental.copy(); 4028 dst.date = date == null ? null : date.copy(); 4029 dst.publisher = publisher == null ? null : publisher.copy(); 4030 if (contact != null) { 4031 dst.contact = new ArrayList<ContactDetail>(); 4032 for (ContactDetail i : contact) 4033 dst.contact.add(i.copy()); 4034 }; 4035 dst.description = description == null ? null : description.copy(); 4036 if (useContext != null) { 4037 dst.useContext = new ArrayList<UsageContext>(); 4038 for (UsageContext i : useContext) 4039 dst.useContext.add(i.copy()); 4040 }; 4041 if (jurisdiction != null) { 4042 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4043 for (CodeableConcept i : jurisdiction) 4044 dst.jurisdiction.add(i.copy()); 4045 }; 4046 dst.code = code == null ? null : code.copy(); 4047 dst.severity = severity == null ? null : severity.copy(); 4048 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4049 dst.stage = stage == null ? null : stage.copy(); 4050 dst.hasSeverity = hasSeverity == null ? null : hasSeverity.copy(); 4051 dst.hasBodySite = hasBodySite == null ? null : hasBodySite.copy(); 4052 dst.hasStage = hasStage == null ? null : hasStage.copy(); 4053 if (definition != null) { 4054 dst.definition = new ArrayList<UriType>(); 4055 for (UriType i : definition) 4056 dst.definition.add(i.copy()); 4057 }; 4058 if (observation != null) { 4059 dst.observation = new ArrayList<ConditionDefinitionObservationComponent>(); 4060 for (ConditionDefinitionObservationComponent i : observation) 4061 dst.observation.add(i.copy()); 4062 }; 4063 if (medication != null) { 4064 dst.medication = new ArrayList<ConditionDefinitionMedicationComponent>(); 4065 for (ConditionDefinitionMedicationComponent i : medication) 4066 dst.medication.add(i.copy()); 4067 }; 4068 if (precondition != null) { 4069 dst.precondition = new ArrayList<ConditionDefinitionPreconditionComponent>(); 4070 for (ConditionDefinitionPreconditionComponent i : precondition) 4071 dst.precondition.add(i.copy()); 4072 }; 4073 if (team != null) { 4074 dst.team = new ArrayList<Reference>(); 4075 for (Reference i : team) 4076 dst.team.add(i.copy()); 4077 }; 4078 if (questionnaire != null) { 4079 dst.questionnaire = new ArrayList<ConditionDefinitionQuestionnaireComponent>(); 4080 for (ConditionDefinitionQuestionnaireComponent i : questionnaire) 4081 dst.questionnaire.add(i.copy()); 4082 }; 4083 if (plan != null) { 4084 dst.plan = new ArrayList<ConditionDefinitionPlanComponent>(); 4085 for (ConditionDefinitionPlanComponent i : plan) 4086 dst.plan.add(i.copy()); 4087 }; 4088 } 4089 4090 protected ConditionDefinition typedCopy() { 4091 return copy(); 4092 } 4093 4094 @Override 4095 public boolean equalsDeep(Base other_) { 4096 if (!super.equalsDeep(other_)) 4097 return false; 4098 if (!(other_ instanceof ConditionDefinition)) 4099 return false; 4100 ConditionDefinition o = (ConditionDefinition) other_; 4101 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4102 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4103 && compareDeep(subtitle, o.subtitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 4104 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 4105 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 4106 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(code, o.code, true) && compareDeep(severity, o.severity, true) 4107 && compareDeep(bodySite, o.bodySite, true) && compareDeep(stage, o.stage, true) && compareDeep(hasSeverity, o.hasSeverity, true) 4108 && compareDeep(hasBodySite, o.hasBodySite, true) && compareDeep(hasStage, o.hasStage, true) && compareDeep(definition, o.definition, true) 4109 && compareDeep(observation, o.observation, true) && compareDeep(medication, o.medication, true) 4110 && compareDeep(precondition, o.precondition, true) && compareDeep(team, o.team, true) && compareDeep(questionnaire, o.questionnaire, true) 4111 && compareDeep(plan, o.plan, true); 4112 } 4113 4114 @Override 4115 public boolean equalsShallow(Base other_) { 4116 if (!super.equalsShallow(other_)) 4117 return false; 4118 if (!(other_ instanceof ConditionDefinition)) 4119 return false; 4120 ConditionDefinition o = (ConditionDefinition) other_; 4121 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4122 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) && compareValues(status, o.status, true) 4123 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 4124 && compareValues(description, o.description, true) && compareValues(hasSeverity, o.hasSeverity, true) 4125 && compareValues(hasBodySite, o.hasBodySite, true) && compareValues(hasStage, o.hasStage, true) && compareValues(definition, o.definition, true) 4126 ; 4127 } 4128 4129 public boolean isEmpty() { 4130 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4131 , versionAlgorithm, name, title, subtitle, status, experimental, date, publisher 4132 , contact, description, useContext, jurisdiction, code, severity, bodySite, stage 4133 , hasSeverity, hasBodySite, hasStage, definition, observation, medication, precondition 4134 , team, questionnaire, plan); 4135 } 4136 4137 @Override 4138 public ResourceType getResourceType() { 4139 return ResourceType.ConditionDefinition; 4140 } 4141 4142 /** 4143 * Search parameter: <b>context-quantity</b> 4144 * <p> 4145 * Description: <b>Multiple Resources: 4146 4147* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4148* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4149* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4150* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4151* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4152* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4153* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4154* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4155* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4156* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4157* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4158* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4159* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4160* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4161* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4162* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4163* [Library](library.html): A quantity- or range-valued use context assigned to the library 4164* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4165* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4166* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4167* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4168* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4169* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4170* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4171* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4172* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4173* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4174* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4175* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4176* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4177</b><br> 4178 * Type: <b>quantity</b><br> 4179 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4180 * </p> 4181 */ 4182 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 4183 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4184 /** 4185 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4186 * <p> 4187 * Description: <b>Multiple Resources: 4188 4189* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4190* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4191* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4192* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4193* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4194* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4195* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4196* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4197* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4198* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4199* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4200* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4201* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4202* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4203* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4204* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4205* [Library](library.html): A quantity- or range-valued use context assigned to the library 4206* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4207* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4208* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4209* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4210* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4211* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4212* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4213* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4214* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4215* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4216* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4217* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4218* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4219</b><br> 4220 * Type: <b>quantity</b><br> 4221 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4222 * </p> 4223 */ 4224 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 4225 4226 /** 4227 * Search parameter: <b>context-type-quantity</b> 4228 * <p> 4229 * Description: <b>Multiple Resources: 4230 4231* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4232* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4233* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4234* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4235* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4236* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4237* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4238* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4239* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4240* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4241* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4242* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4243* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4244* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4245* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4246* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4247* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4248* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4249* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4250* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4251* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4252* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4253* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4254* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4255* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4256* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4257* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4258* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4259* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4260* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4261</b><br> 4262 * Type: <b>composite</b><br> 4263 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4264 * </p> 4265 */ 4266 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 4267 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4268 /** 4269 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 4270 * <p> 4271 * Description: <b>Multiple Resources: 4272 4273* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4274* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4275* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4276* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4277* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4278* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4279* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4280* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4281* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4282* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4283* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4284* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4285* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4286* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4287* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4288* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4289* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4290* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4291* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4292* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4293* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4294* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4295* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4296* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4297* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4298* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4299* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4300* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4301* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4302* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4303</b><br> 4304 * Type: <b>composite</b><br> 4305 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4306 * </p> 4307 */ 4308 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 4309 4310 /** 4311 * Search parameter: <b>context-type-value</b> 4312 * <p> 4313 * Description: <b>Multiple Resources: 4314 4315* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4316* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4317* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4318* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4319* [Citation](citation.html): A use context type and value assigned to the citation 4320* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4321* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4322* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4323* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4324* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4325* [Evidence](evidence.html): A use context type and value assigned to the evidence 4326* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4327* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4328* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4329* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4330* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4331* [Library](library.html): A use context type and value assigned to the library 4332* [Measure](measure.html): A use context type and value assigned to the measure 4333* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4334* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4335* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4336* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4337* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4338* [Requirements](requirements.html): A use context type and value assigned to the requirements 4339* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4340* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4341* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4342* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4343* [TestScript](testscript.html): A use context type and value assigned to the test script 4344* [ValueSet](valueset.html): A use context type and value assigned to the value set 4345</b><br> 4346 * Type: <b>composite</b><br> 4347 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4348 * </p> 4349 */ 4350 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 4351 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4352 /** 4353 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4354 * <p> 4355 * Description: <b>Multiple Resources: 4356 4357* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4358* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4359* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4360* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4361* [Citation](citation.html): A use context type and value assigned to the citation 4362* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4363* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4364* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4365* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4366* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4367* [Evidence](evidence.html): A use context type and value assigned to the evidence 4368* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4369* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4370* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4371* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4372* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4373* [Library](library.html): A use context type and value assigned to the library 4374* [Measure](measure.html): A use context type and value assigned to the measure 4375* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4376* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4377* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4378* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4379* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4380* [Requirements](requirements.html): A use context type and value assigned to the requirements 4381* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4382* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4383* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4384* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4385* [TestScript](testscript.html): A use context type and value assigned to the test script 4386* [ValueSet](valueset.html): A use context type and value assigned to the value set 4387</b><br> 4388 * Type: <b>composite</b><br> 4389 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4390 * </p> 4391 */ 4392 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 4393 4394 /** 4395 * Search parameter: <b>context-type</b> 4396 * <p> 4397 * Description: <b>Multiple Resources: 4398 4399* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4400* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4401* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4402* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4403* [Citation](citation.html): A type of use context assigned to the citation 4404* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4405* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4406* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4407* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4408* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4409* [Evidence](evidence.html): A type of use context assigned to the evidence 4410* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4411* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4412* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4413* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4414* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4415* [Library](library.html): A type of use context assigned to the library 4416* [Measure](measure.html): A type of use context assigned to the measure 4417* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4418* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4419* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4420* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4421* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4422* [Requirements](requirements.html): A type of use context assigned to the requirements 4423* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4424* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4425* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4426* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4427* [TestScript](testscript.html): A type of use context assigned to the test script 4428* [ValueSet](valueset.html): A type of use context assigned to the value set 4429</b><br> 4430 * Type: <b>token</b><br> 4431 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4432 * </p> 4433 */ 4434 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 4435 public static final String SP_CONTEXT_TYPE = "context-type"; 4436 /** 4437 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4438 * <p> 4439 * Description: <b>Multiple Resources: 4440 4441* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4442* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4443* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4444* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4445* [Citation](citation.html): A type of use context assigned to the citation 4446* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4447* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4448* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4449* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4450* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4451* [Evidence](evidence.html): A type of use context assigned to the evidence 4452* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4453* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4454* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4455* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4456* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4457* [Library](library.html): A type of use context assigned to the library 4458* [Measure](measure.html): A type of use context assigned to the measure 4459* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4460* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4461* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4462* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4463* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4464* [Requirements](requirements.html): A type of use context assigned to the requirements 4465* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4466* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4467* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4468* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4469* [TestScript](testscript.html): A type of use context assigned to the test script 4470* [ValueSet](valueset.html): A type of use context assigned to the value set 4471</b><br> 4472 * Type: <b>token</b><br> 4473 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4474 * </p> 4475 */ 4476 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 4477 4478 /** 4479 * Search parameter: <b>context</b> 4480 * <p> 4481 * Description: <b>Multiple Resources: 4482 4483* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4484* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4485* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4486* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4487* [Citation](citation.html): A use context assigned to the citation 4488* [CodeSystem](codesystem.html): A use context assigned to the code system 4489* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4490* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4491* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4492* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4493* [Evidence](evidence.html): A use context assigned to the evidence 4494* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4495* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4496* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4497* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4498* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4499* [Library](library.html): A use context assigned to the library 4500* [Measure](measure.html): A use context assigned to the measure 4501* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4502* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4503* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4504* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4505* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4506* [Requirements](requirements.html): A use context assigned to the requirements 4507* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4508* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4509* [StructureMap](structuremap.html): A use context assigned to the structure map 4510* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4511* [TestScript](testscript.html): A use context assigned to the test script 4512* [ValueSet](valueset.html): A use context assigned to the value set 4513</b><br> 4514 * Type: <b>token</b><br> 4515 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4516 * </p> 4517 */ 4518 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 4519 public static final String SP_CONTEXT = "context"; 4520 /** 4521 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4522 * <p> 4523 * Description: <b>Multiple Resources: 4524 4525* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4526* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4527* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4528* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4529* [Citation](citation.html): A use context assigned to the citation 4530* [CodeSystem](codesystem.html): A use context assigned to the code system 4531* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4532* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4533* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4534* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4535* [Evidence](evidence.html): A use context assigned to the evidence 4536* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4537* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4538* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4539* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4540* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4541* [Library](library.html): A use context assigned to the library 4542* [Measure](measure.html): A use context assigned to the measure 4543* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4544* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4545* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4546* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4547* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4548* [Requirements](requirements.html): A use context assigned to the requirements 4549* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4550* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4551* [StructureMap](structuremap.html): A use context assigned to the structure map 4552* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4553* [TestScript](testscript.html): A use context assigned to the test script 4554* [ValueSet](valueset.html): A use context assigned to the value set 4555</b><br> 4556 * Type: <b>token</b><br> 4557 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4558 * </p> 4559 */ 4560 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4561 4562 /** 4563 * Search parameter: <b>date</b> 4564 * <p> 4565 * Description: <b>Multiple Resources: 4566 4567* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4568* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4569* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4570* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4571* [Citation](citation.html): The citation publication date 4572* [CodeSystem](codesystem.html): The code system publication date 4573* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4574* [ConceptMap](conceptmap.html): The concept map publication date 4575* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4576* [EventDefinition](eventdefinition.html): The event definition publication date 4577* [Evidence](evidence.html): The evidence publication date 4578* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4579* [ExampleScenario](examplescenario.html): The example scenario publication date 4580* [GraphDefinition](graphdefinition.html): The graph definition publication date 4581* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4582* [Library](library.html): The library publication date 4583* [Measure](measure.html): The measure publication date 4584* [MessageDefinition](messagedefinition.html): The message definition publication date 4585* [NamingSystem](namingsystem.html): The naming system publication date 4586* [OperationDefinition](operationdefinition.html): The operation definition publication date 4587* [PlanDefinition](plandefinition.html): The plan definition publication date 4588* [Questionnaire](questionnaire.html): The questionnaire publication date 4589* [Requirements](requirements.html): The requirements publication date 4590* [SearchParameter](searchparameter.html): The search parameter publication date 4591* [StructureDefinition](structuredefinition.html): The structure definition publication date 4592* [StructureMap](structuremap.html): The structure map publication date 4593* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4594* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4595* [TestScript](testscript.html): The test script publication date 4596* [ValueSet](valueset.html): The value set publication date 4597</b><br> 4598 * Type: <b>date</b><br> 4599 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4600 * </p> 4601 */ 4602 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 4603 public static final String SP_DATE = "date"; 4604 /** 4605 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4606 * <p> 4607 * Description: <b>Multiple Resources: 4608 4609* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4610* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4611* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4612* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4613* [Citation](citation.html): The citation publication date 4614* [CodeSystem](codesystem.html): The code system publication date 4615* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4616* [ConceptMap](conceptmap.html): The concept map publication date 4617* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4618* [EventDefinition](eventdefinition.html): The event definition publication date 4619* [Evidence](evidence.html): The evidence publication date 4620* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4621* [ExampleScenario](examplescenario.html): The example scenario publication date 4622* [GraphDefinition](graphdefinition.html): The graph definition publication date 4623* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4624* [Library](library.html): The library publication date 4625* [Measure](measure.html): The measure publication date 4626* [MessageDefinition](messagedefinition.html): The message definition publication date 4627* [NamingSystem](namingsystem.html): The naming system publication date 4628* [OperationDefinition](operationdefinition.html): The operation definition publication date 4629* [PlanDefinition](plandefinition.html): The plan definition publication date 4630* [Questionnaire](questionnaire.html): The questionnaire publication date 4631* [Requirements](requirements.html): The requirements publication date 4632* [SearchParameter](searchparameter.html): The search parameter publication date 4633* [StructureDefinition](structuredefinition.html): The structure definition publication date 4634* [StructureMap](structuremap.html): The structure map publication date 4635* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4636* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4637* [TestScript](testscript.html): The test script publication date 4638* [ValueSet](valueset.html): The value set publication date 4639</b><br> 4640 * Type: <b>date</b><br> 4641 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4642 * </p> 4643 */ 4644 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4645 4646 /** 4647 * Search parameter: <b>description</b> 4648 * <p> 4649 * Description: <b>Multiple Resources: 4650 4651* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4652* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4653* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4654* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4655* [Citation](citation.html): The description of the citation 4656* [CodeSystem](codesystem.html): The description of the code system 4657* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4658* [ConceptMap](conceptmap.html): The description of the concept map 4659* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4660* [EventDefinition](eventdefinition.html): The description of the event definition 4661* [Evidence](evidence.html): The description of the evidence 4662* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4663* [GraphDefinition](graphdefinition.html): The description of the graph definition 4664* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4665* [Library](library.html): The description of the library 4666* [Measure](measure.html): The description of the measure 4667* [MessageDefinition](messagedefinition.html): The description of the message definition 4668* [NamingSystem](namingsystem.html): The description of the naming system 4669* [OperationDefinition](operationdefinition.html): The description of the operation definition 4670* [PlanDefinition](plandefinition.html): The description of the plan definition 4671* [Questionnaire](questionnaire.html): The description of the questionnaire 4672* [Requirements](requirements.html): The description of the requirements 4673* [SearchParameter](searchparameter.html): The description of the search parameter 4674* [StructureDefinition](structuredefinition.html): The description of the structure definition 4675* [StructureMap](structuremap.html): The description of the structure map 4676* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4677* [TestScript](testscript.html): The description of the test script 4678* [ValueSet](valueset.html): The description of the value set 4679</b><br> 4680 * Type: <b>string</b><br> 4681 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4682 * </p> 4683 */ 4684 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 4685 public static final String SP_DESCRIPTION = "description"; 4686 /** 4687 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4688 * <p> 4689 * Description: <b>Multiple Resources: 4690 4691* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4692* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4693* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4694* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4695* [Citation](citation.html): The description of the citation 4696* [CodeSystem](codesystem.html): The description of the code system 4697* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4698* [ConceptMap](conceptmap.html): The description of the concept map 4699* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4700* [EventDefinition](eventdefinition.html): The description of the event definition 4701* [Evidence](evidence.html): The description of the evidence 4702* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4703* [GraphDefinition](graphdefinition.html): The description of the graph definition 4704* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4705* [Library](library.html): The description of the library 4706* [Measure](measure.html): The description of the measure 4707* [MessageDefinition](messagedefinition.html): The description of the message definition 4708* [NamingSystem](namingsystem.html): The description of the naming system 4709* [OperationDefinition](operationdefinition.html): The description of the operation definition 4710* [PlanDefinition](plandefinition.html): The description of the plan definition 4711* [Questionnaire](questionnaire.html): The description of the questionnaire 4712* [Requirements](requirements.html): The description of the requirements 4713* [SearchParameter](searchparameter.html): The description of the search parameter 4714* [StructureDefinition](structuredefinition.html): The description of the structure definition 4715* [StructureMap](structuremap.html): The description of the structure map 4716* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4717* [TestScript](testscript.html): The description of the test script 4718* [ValueSet](valueset.html): The description of the value set 4719</b><br> 4720 * Type: <b>string</b><br> 4721 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4722 * </p> 4723 */ 4724 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4725 4726 /** 4727 * Search parameter: <b>identifier</b> 4728 * <p> 4729 * Description: <b>Multiple Resources: 4730 4731* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4732* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4733* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4734* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4735* [Citation](citation.html): External identifier for the citation 4736* [CodeSystem](codesystem.html): External identifier for the code system 4737* [ConceptMap](conceptmap.html): External identifier for the concept map 4738* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4739* [EventDefinition](eventdefinition.html): External identifier for the event definition 4740* [Evidence](evidence.html): External identifier for the evidence 4741* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4742* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4743* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4744* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4745* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4746* [Library](library.html): External identifier for the library 4747* [Measure](measure.html): External identifier for the measure 4748* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4749* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4750* [NamingSystem](namingsystem.html): External identifier for the naming system 4751* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4752* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4753* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4754* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4755* [Requirements](requirements.html): External identifier for the requirements 4756* [SearchParameter](searchparameter.html): External identifier for the search parameter 4757* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4758* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4759* [StructureMap](structuremap.html): External identifier for the structure map 4760* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4761* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4762* [TestPlan](testplan.html): An identifier for the test plan 4763* [TestScript](testscript.html): External identifier for the test script 4764* [ValueSet](valueset.html): External identifier for the value set 4765</b><br> 4766 * Type: <b>token</b><br> 4767 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4768 * </p> 4769 */ 4770 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4771 public static final String SP_IDENTIFIER = "identifier"; 4772 /** 4773 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4774 * <p> 4775 * Description: <b>Multiple Resources: 4776 4777* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4778* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4779* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4780* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4781* [Citation](citation.html): External identifier for the citation 4782* [CodeSystem](codesystem.html): External identifier for the code system 4783* [ConceptMap](conceptmap.html): External identifier for the concept map 4784* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4785* [EventDefinition](eventdefinition.html): External identifier for the event definition 4786* [Evidence](evidence.html): External identifier for the evidence 4787* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4788* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4789* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4790* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4791* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4792* [Library](library.html): External identifier for the library 4793* [Measure](measure.html): External identifier for the measure 4794* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4795* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4796* [NamingSystem](namingsystem.html): External identifier for the naming system 4797* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4798* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4799* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4800* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4801* [Requirements](requirements.html): External identifier for the requirements 4802* [SearchParameter](searchparameter.html): External identifier for the search parameter 4803* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4804* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4805* [StructureMap](structuremap.html): External identifier for the structure map 4806* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4807* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4808* [TestPlan](testplan.html): An identifier for the test plan 4809* [TestScript](testscript.html): External identifier for the test script 4810* [ValueSet](valueset.html): External identifier for the value set 4811</b><br> 4812 * Type: <b>token</b><br> 4813 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4814 * </p> 4815 */ 4816 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4817 4818 /** 4819 * Search parameter: <b>jurisdiction</b> 4820 * <p> 4821 * Description: <b>Multiple Resources: 4822 4823* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4824* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4825* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4826* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4827* [Citation](citation.html): Intended jurisdiction for the citation 4828* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4829* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4830* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4831* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4832* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4833* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4834* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4835* [Library](library.html): Intended jurisdiction for the library 4836* [Measure](measure.html): Intended jurisdiction for the measure 4837* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4838* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4839* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4840* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4841* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4842* [Requirements](requirements.html): Intended jurisdiction for the requirements 4843* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4844* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4845* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4846* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4847* [TestScript](testscript.html): Intended jurisdiction for the test script 4848* [ValueSet](valueset.html): Intended jurisdiction for the value set 4849</b><br> 4850 * Type: <b>token</b><br> 4851 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4852 * </p> 4853 */ 4854 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 4855 public static final String SP_JURISDICTION = "jurisdiction"; 4856 /** 4857 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4858 * <p> 4859 * Description: <b>Multiple Resources: 4860 4861* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4862* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4863* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4864* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4865* [Citation](citation.html): Intended jurisdiction for the citation 4866* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4867* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4868* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4869* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4870* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4871* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4872* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4873* [Library](library.html): Intended jurisdiction for the library 4874* [Measure](measure.html): Intended jurisdiction for the measure 4875* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4876* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4877* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4878* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4879* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4880* [Requirements](requirements.html): Intended jurisdiction for the requirements 4881* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4882* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4883* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4884* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4885* [TestScript](testscript.html): Intended jurisdiction for the test script 4886* [ValueSet](valueset.html): Intended jurisdiction for the value set 4887</b><br> 4888 * Type: <b>token</b><br> 4889 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4890 * </p> 4891 */ 4892 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4893 4894 /** 4895 * Search parameter: <b>name</b> 4896 * <p> 4897 * Description: <b>Multiple Resources: 4898 4899* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4900* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4901* [Citation](citation.html): Computationally friendly name of the citation 4902* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4903* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4904* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4905* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4906* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4907* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4908* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4909* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4910* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4911* [Library](library.html): Computationally friendly name of the library 4912* [Measure](measure.html): Computationally friendly name of the measure 4913* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4914* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4915* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4916* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4917* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4918* [Requirements](requirements.html): Computationally friendly name of the requirements 4919* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4920* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4921* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4922* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4923* [TestScript](testscript.html): Computationally friendly name of the test script 4924* [ValueSet](valueset.html): Computationally friendly name of the value set 4925</b><br> 4926 * Type: <b>string</b><br> 4927 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4928 * </p> 4929 */ 4930 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 4931 public static final String SP_NAME = "name"; 4932 /** 4933 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4934 * <p> 4935 * Description: <b>Multiple Resources: 4936 4937* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4938* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4939* [Citation](citation.html): Computationally friendly name of the citation 4940* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4941* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4942* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4943* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4944* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4945* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4946* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4947* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4948* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4949* [Library](library.html): Computationally friendly name of the library 4950* [Measure](measure.html): Computationally friendly name of the measure 4951* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4952* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4953* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4954* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4955* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4956* [Requirements](requirements.html): Computationally friendly name of the requirements 4957* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4958* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4959* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4960* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4961* [TestScript](testscript.html): Computationally friendly name of the test script 4962* [ValueSet](valueset.html): Computationally friendly name of the value set 4963</b><br> 4964 * Type: <b>string</b><br> 4965 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4966 * </p> 4967 */ 4968 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4969 4970 /** 4971 * Search parameter: <b>publisher</b> 4972 * <p> 4973 * Description: <b>Multiple Resources: 4974 4975* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4976* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4977* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4978* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4979* [Citation](citation.html): Name of the publisher of the citation 4980* [CodeSystem](codesystem.html): Name of the publisher of the code system 4981* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4982* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4983* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4984* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4985* [Evidence](evidence.html): Name of the publisher of the evidence 4986* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4987* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4988* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4989* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4990* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4991* [Library](library.html): Name of the publisher of the library 4992* [Measure](measure.html): Name of the publisher of the measure 4993* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4994* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4995* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4996* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4997* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4998* [Requirements](requirements.html): Name of the publisher of the requirements 4999* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5000* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5001* [StructureMap](structuremap.html): Name of the publisher of the structure map 5002* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5003* [TestScript](testscript.html): Name of the publisher of the test script 5004* [ValueSet](valueset.html): Name of the publisher of the value set 5005</b><br> 5006 * Type: <b>string</b><br> 5007 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5008 * </p> 5009 */ 5010 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 5011 public static final String SP_PUBLISHER = "publisher"; 5012 /** 5013 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5014 * <p> 5015 * Description: <b>Multiple Resources: 5016 5017* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5018* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5019* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5020* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5021* [Citation](citation.html): Name of the publisher of the citation 5022* [CodeSystem](codesystem.html): Name of the publisher of the code system 5023* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5024* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5025* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5026* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5027* [Evidence](evidence.html): Name of the publisher of the evidence 5028* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5029* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5030* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5031* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5032* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5033* [Library](library.html): Name of the publisher of the library 5034* [Measure](measure.html): Name of the publisher of the measure 5035* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5036* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5037* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5038* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5039* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5040* [Requirements](requirements.html): Name of the publisher of the requirements 5041* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5042* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5043* [StructureMap](structuremap.html): Name of the publisher of the structure map 5044* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5045* [TestScript](testscript.html): Name of the publisher of the test script 5046* [ValueSet](valueset.html): Name of the publisher of the value set 5047</b><br> 5048 * Type: <b>string</b><br> 5049 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5050 * </p> 5051 */ 5052 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5053 5054 /** 5055 * Search parameter: <b>status</b> 5056 * <p> 5057 * Description: <b>Multiple Resources: 5058 5059* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5060* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5061* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5062* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5063* [Citation](citation.html): The current status of the citation 5064* [CodeSystem](codesystem.html): The current status of the code system 5065* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5066* [ConceptMap](conceptmap.html): The current status of the concept map 5067* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5068* [EventDefinition](eventdefinition.html): The current status of the event definition 5069* [Evidence](evidence.html): The current status of the evidence 5070* [EvidenceReport](evidencereport.html): The current status of the evidence report 5071* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5072* [ExampleScenario](examplescenario.html): The current status of the example scenario 5073* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5074* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5075* [Library](library.html): The current status of the library 5076* [Measure](measure.html): The current status of the measure 5077* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5078* [MessageDefinition](messagedefinition.html): The current status of the message definition 5079* [NamingSystem](namingsystem.html): The current status of the naming system 5080* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5081* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5082* [PlanDefinition](plandefinition.html): The current status of the plan definition 5083* [Questionnaire](questionnaire.html): The current status of the questionnaire 5084* [Requirements](requirements.html): The current status of the requirements 5085* [SearchParameter](searchparameter.html): The current status of the search parameter 5086* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5087* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5088* [StructureMap](structuremap.html): The current status of the structure map 5089* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5090* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5091* [TestPlan](testplan.html): The current status of the test plan 5092* [TestScript](testscript.html): The current status of the test script 5093* [ValueSet](valueset.html): The current status of the value set 5094</b><br> 5095 * Type: <b>token</b><br> 5096 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5097 * </p> 5098 */ 5099 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 5100 public static final String SP_STATUS = "status"; 5101 /** 5102 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5103 * <p> 5104 * Description: <b>Multiple Resources: 5105 5106* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5107* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5108* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5109* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5110* [Citation](citation.html): The current status of the citation 5111* [CodeSystem](codesystem.html): The current status of the code system 5112* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5113* [ConceptMap](conceptmap.html): The current status of the concept map 5114* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5115* [EventDefinition](eventdefinition.html): The current status of the event definition 5116* [Evidence](evidence.html): The current status of the evidence 5117* [EvidenceReport](evidencereport.html): The current status of the evidence report 5118* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5119* [ExampleScenario](examplescenario.html): The current status of the example scenario 5120* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5121* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5122* [Library](library.html): The current status of the library 5123* [Measure](measure.html): The current status of the measure 5124* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5125* [MessageDefinition](messagedefinition.html): The current status of the message definition 5126* [NamingSystem](namingsystem.html): The current status of the naming system 5127* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5128* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5129* [PlanDefinition](plandefinition.html): The current status of the plan definition 5130* [Questionnaire](questionnaire.html): The current status of the questionnaire 5131* [Requirements](requirements.html): The current status of the requirements 5132* [SearchParameter](searchparameter.html): The current status of the search parameter 5133* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5134* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5135* [StructureMap](structuremap.html): The current status of the structure map 5136* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5137* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5138* [TestPlan](testplan.html): The current status of the test plan 5139* [TestScript](testscript.html): The current status of the test script 5140* [ValueSet](valueset.html): The current status of the value set 5141</b><br> 5142 * Type: <b>token</b><br> 5143 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5144 * </p> 5145 */ 5146 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5147 5148 /** 5149 * Search parameter: <b>title</b> 5150 * <p> 5151 * Description: <b>Multiple Resources: 5152 5153* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 5154* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 5155* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 5156* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 5157* [Citation](citation.html): The human-friendly name of the citation 5158* [CodeSystem](codesystem.html): The human-friendly name of the code system 5159* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 5160* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 5161* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 5162* [Evidence](evidence.html): The human-friendly name of the evidence 5163* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 5164* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 5165* [Library](library.html): The human-friendly name of the library 5166* [Measure](measure.html): The human-friendly name of the measure 5167* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 5168* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 5169* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 5170* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 5171* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 5172* [Requirements](requirements.html): The human-friendly name of the requirements 5173* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 5174* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 5175* [StructureMap](structuremap.html): The human-friendly name of the structure map 5176* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 5177* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 5178* [TestScript](testscript.html): The human-friendly name of the test script 5179* [ValueSet](valueset.html): The human-friendly name of the value set 5180</b><br> 5181 * Type: <b>string</b><br> 5182 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 5183 * </p> 5184 */ 5185 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 5186 public static final String SP_TITLE = "title"; 5187 /** 5188 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5189 * <p> 5190 * Description: <b>Multiple Resources: 5191 5192* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 5193* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 5194* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 5195* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 5196* [Citation](citation.html): The human-friendly name of the citation 5197* [CodeSystem](codesystem.html): The human-friendly name of the code system 5198* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 5199* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 5200* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 5201* [Evidence](evidence.html): The human-friendly name of the evidence 5202* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 5203* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 5204* [Library](library.html): The human-friendly name of the library 5205* [Measure](measure.html): The human-friendly name of the measure 5206* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 5207* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 5208* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 5209* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 5210* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 5211* [Requirements](requirements.html): The human-friendly name of the requirements 5212* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 5213* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 5214* [StructureMap](structuremap.html): The human-friendly name of the structure map 5215* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 5216* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 5217* [TestScript](testscript.html): The human-friendly name of the test script 5218* [ValueSet](valueset.html): The human-friendly name of the value set 5219</b><br> 5220 * Type: <b>string</b><br> 5221 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 5222 * </p> 5223 */ 5224 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 5225 5226 /** 5227 * Search parameter: <b>url</b> 5228 * <p> 5229 * Description: <b>Multiple Resources: 5230 5231* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5232* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5233* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5234* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5235* [Citation](citation.html): The uri that identifies the citation 5236* [CodeSystem](codesystem.html): The uri that identifies the code system 5237* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5238* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5239* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5240* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5241* [Evidence](evidence.html): The uri that identifies the evidence 5242* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5243* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5244* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5245* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5246* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5247* [Library](library.html): The uri that identifies the library 5248* [Measure](measure.html): The uri that identifies the measure 5249* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5250* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5251* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5252* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5253* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5254* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5255* [Requirements](requirements.html): The uri that identifies the requirements 5256* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5257* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5258* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5259* [StructureMap](structuremap.html): The uri that identifies the structure map 5260* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5261* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5262* [TestPlan](testplan.html): The uri that identifies the test plan 5263* [TestScript](testscript.html): The uri that identifies the test script 5264* [ValueSet](valueset.html): The uri that identifies the value set 5265</b><br> 5266 * Type: <b>uri</b><br> 5267 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5268 * </p> 5269 */ 5270 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 5271 public static final String SP_URL = "url"; 5272 /** 5273 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5274 * <p> 5275 * Description: <b>Multiple Resources: 5276 5277* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5278* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5279* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5280* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5281* [Citation](citation.html): The uri that identifies the citation 5282* [CodeSystem](codesystem.html): The uri that identifies the code system 5283* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5284* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5285* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5286* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5287* [Evidence](evidence.html): The uri that identifies the evidence 5288* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5289* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5290* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5291* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5292* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5293* [Library](library.html): The uri that identifies the library 5294* [Measure](measure.html): The uri that identifies the measure 5295* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5296* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5297* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5298* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5299* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5300* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5301* [Requirements](requirements.html): The uri that identifies the requirements 5302* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5303* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5304* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5305* [StructureMap](structuremap.html): The uri that identifies the structure map 5306* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5307* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5308* [TestPlan](testplan.html): The uri that identifies the test plan 5309* [TestScript](testscript.html): The uri that identifies the test script 5310* [ValueSet](valueset.html): The uri that identifies the value set 5311</b><br> 5312 * Type: <b>uri</b><br> 5313 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5314 * </p> 5315 */ 5316 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5317 5318 /** 5319 * Search parameter: <b>version</b> 5320 * <p> 5321 * Description: <b>Multiple Resources: 5322 5323* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5324* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5325* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5326* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5327* [Citation](citation.html): The business version of the citation 5328* [CodeSystem](codesystem.html): The business version of the code system 5329* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5330* [ConceptMap](conceptmap.html): The business version of the concept map 5331* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5332* [EventDefinition](eventdefinition.html): The business version of the event definition 5333* [Evidence](evidence.html): The business version of the evidence 5334* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5335* [ExampleScenario](examplescenario.html): The business version of the example scenario 5336* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5337* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5338* [Library](library.html): The business version of the library 5339* [Measure](measure.html): The business version of the measure 5340* [MessageDefinition](messagedefinition.html): The business version of the message definition 5341* [NamingSystem](namingsystem.html): The business version of the naming system 5342* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5343* [PlanDefinition](plandefinition.html): The business version of the plan definition 5344* [Questionnaire](questionnaire.html): The business version of the questionnaire 5345* [Requirements](requirements.html): The business version of the requirements 5346* [SearchParameter](searchparameter.html): The business version of the search parameter 5347* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5348* [StructureMap](structuremap.html): The business version of the structure map 5349* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5350* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5351* [TestScript](testscript.html): The business version of the test script 5352* [ValueSet](valueset.html): The business version of the value set 5353</b><br> 5354 * Type: <b>token</b><br> 5355 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5356 * </p> 5357 */ 5358 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 5359 public static final String SP_VERSION = "version"; 5360 /** 5361 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5362 * <p> 5363 * Description: <b>Multiple Resources: 5364 5365* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5366* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5367* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5368* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5369* [Citation](citation.html): The business version of the citation 5370* [CodeSystem](codesystem.html): The business version of the code system 5371* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5372* [ConceptMap](conceptmap.html): The business version of the concept map 5373* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5374* [EventDefinition](eventdefinition.html): The business version of the event definition 5375* [Evidence](evidence.html): The business version of the evidence 5376* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5377* [ExampleScenario](examplescenario.html): The business version of the example scenario 5378* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5379* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5380* [Library](library.html): The business version of the library 5381* [Measure](measure.html): The business version of the measure 5382* [MessageDefinition](messagedefinition.html): The business version of the message definition 5383* [NamingSystem](namingsystem.html): The business version of the naming system 5384* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5385* [PlanDefinition](plandefinition.html): The business version of the plan definition 5386* [Questionnaire](questionnaire.html): The business version of the questionnaire 5387* [Requirements](requirements.html): The business version of the requirements 5388* [SearchParameter](searchparameter.html): The business version of the search parameter 5389* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5390* [StructureMap](structuremap.html): The business version of the structure map 5391* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5392* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5393* [TestScript](testscript.html): The business version of the test script 5394* [ValueSet](valueset.html): The business version of the value set 5395</b><br> 5396 * Type: <b>token</b><br> 5397 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5398 * </p> 5399 */ 5400 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5401 5402 5403} 5404