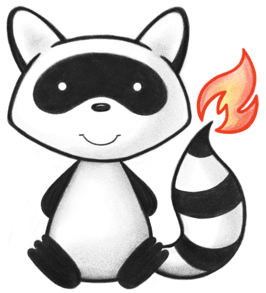
001package org.hl7.fhir.r5.model; 002 003 004 005 006/* 007Copyright (c) 2011+, HL7, Inc. 008All rights reserved. 009 010Redistribution and use in source and binary forms, with or without modification, 011are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035/** 036 * This class is created to help implementers deal with a change to 037 * the API that was made between versions 0.81 and 0.9 038 * 039 * The change is the behaviour of the .getX() where the cardinality of 040 * x is 0..1 or 1..1. Before the change, these routines would return 041 * null if the object had not previously been assigned, and after the ' 042 * change, they will automatically create the object if it had not 043 * been assigned (unless the object is polymorphic, in which case, 044 * only the type specific getters create the object) 045 * 046 * When making the transition from the old style to the new style, 047 * the main change is that when testing for presence or abssense 048 * of the element, instead of doing one of these two: 049 * 050 * if (obj.getProperty() == null) 051 * if (obj.getProperty() != null) 052 * 053 * you instead do 054 * 055 * if (!obj.hasProperty()) 056 * if (obj.hasProperty()) 057 * 058 * or else one of these two: 059 * 060 * if (obj.getProperty().isEmpty()) 061 * if (!obj.getProperty().isEmpty()) 062 * 063 * The only way to sort this out is by finding all these things 064 * in the code, and changing them. 065 * 066 * To help with that, you can set the status field of this class 067 * to change how this API behaves. Note: the status value is tied 068 * to the way that you program. The intent of this class is to 069 * help make developers the transiition to status = 0. The old 070 * behaviour is status = 2. To make the transition, set the 071 * status code to 1. This way, any time a .getX routine needs 072 * to automatically create an object, an exception will be 073 * raised instead. The expected use of this is: 074 * - set status = 1 075 * - test your code (all paths) 076 * - when an exception happens, change the code to use .hasX() or .isEmpty() 077 * - when all execution paths don't raise an exception, set status = 0 078 * - start programming to the new style. 079 * 080 * You can set status = 2 and leave it like that, but this is not 081 * compatible with the utilities and validation code, nor with the 082 * HAPI code. So everyone shoul make this transition 083 * 084 * This is a difficult change to make to an API. Most users should engage with it 085 * as they migrate from DSTU1 to DSTU2, at the same time as they encounter 086 * other changes. This change was made in order to align the two java reference 087 * implementations on a common object model, which is an important outcome that 088 * justifies making this change to implementers (sorry for any pain caused) 089 * 090 * @author Grahame 091 * 092 */ 093public class Configuration { 094 095 private static int status = 0; 096 // 0: auto-create 097 // 1: error 098 // 2: return null 099 100 private static boolean acceptInvalidEnums; 101 102 public static boolean errorOnAutoCreate() { 103 return status == 1; 104 } 105 106 107 public static boolean doAutoCreate() { 108 return status == 0; 109 } 110 111 112 public static boolean isAcceptInvalidEnums() { 113 return acceptInvalidEnums; 114 } 115 116 117 public static void setAcceptInvalidEnums(boolean value) { 118 acceptInvalidEnums = value; 119 } 120 121 122}