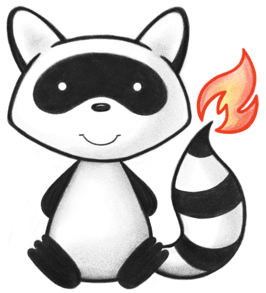
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a healthcare consumer?s choices or choices made on their behalf by a third party, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 052 */ 053@ResourceDef(name="Consent", profile="http://hl7.org/fhir/StructureDefinition/Consent") 054public class Consent extends DomainResource { 055 056 public enum ConsentState { 057 /** 058 * The consent is in development or awaiting use but is not yet intended to be acted upon. 059 */ 060 DRAFT, 061 /** 062 * The consent is to be followed and enforced. 063 */ 064 ACTIVE, 065 /** 066 * The consent is terminated or replaced. 067 */ 068 INACTIVE, 069 /** 070 * The consent development has been terminated prior to completion. 071 */ 072 NOTDONE, 073 /** 074 * The consent was created wrongly (e.g. wrong patient) and should be ignored. 075 */ 076 ENTEREDINERROR, 077 /** 078 * The resource is in an indeterminate state. 079 */ 080 UNKNOWN, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static ConsentState fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("draft".equals(codeString)) 089 return DRAFT; 090 if ("active".equals(codeString)) 091 return ACTIVE; 092 if ("inactive".equals(codeString)) 093 return INACTIVE; 094 if ("not-done".equals(codeString)) 095 return NOTDONE; 096 if ("entered-in-error".equals(codeString)) 097 return ENTEREDINERROR; 098 if ("unknown".equals(codeString)) 099 return UNKNOWN; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case DRAFT: return "draft"; 108 case ACTIVE: return "active"; 109 case INACTIVE: return "inactive"; 110 case NOTDONE: return "not-done"; 111 case ENTEREDINERROR: return "entered-in-error"; 112 case UNKNOWN: return "unknown"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getSystem() { 118 switch (this) { 119 case DRAFT: return "http://hl7.org/fhir/consent-state-codes"; 120 case ACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 121 case INACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 122 case NOTDONE: return "http://hl7.org/fhir/consent-state-codes"; 123 case ENTEREDINERROR: return "http://hl7.org/fhir/consent-state-codes"; 124 case UNKNOWN: return "http://hl7.org/fhir/consent-state-codes"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDefinition() { 130 switch (this) { 131 case DRAFT: return "The consent is in development or awaiting use but is not yet intended to be acted upon."; 132 case ACTIVE: return "The consent is to be followed and enforced."; 133 case INACTIVE: return "The consent is terminated or replaced."; 134 case NOTDONE: return "The consent development has been terminated prior to completion."; 135 case ENTEREDINERROR: return "The consent was created wrongly (e.g. wrong patient) and should be ignored."; 136 case UNKNOWN: return "The resource is in an indeterminate state."; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getDisplay() { 142 switch (this) { 143 case DRAFT: return "Pending"; 144 case ACTIVE: return "Active"; 145 case INACTIVE: return "Inactive"; 146 case NOTDONE: return "Abandoned"; 147 case ENTEREDINERROR: return "Entered in Error"; 148 case UNKNOWN: return "Unknown"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 } 154 155 public static class ConsentStateEnumFactory implements EnumFactory<ConsentState> { 156 public ConsentState fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("draft".equals(codeString)) 161 return ConsentState.DRAFT; 162 if ("active".equals(codeString)) 163 return ConsentState.ACTIVE; 164 if ("inactive".equals(codeString)) 165 return ConsentState.INACTIVE; 166 if ("not-done".equals(codeString)) 167 return ConsentState.NOTDONE; 168 if ("entered-in-error".equals(codeString)) 169 return ConsentState.ENTEREDINERROR; 170 if ("unknown".equals(codeString)) 171 return ConsentState.UNKNOWN; 172 throw new IllegalArgumentException("Unknown ConsentState code '"+codeString+"'"); 173 } 174 public Enumeration<ConsentState> fromType(PrimitiveType<?> code) throws FHIRException { 175 if (code == null) 176 return null; 177 if (code.isEmpty()) 178 return new Enumeration<ConsentState>(this, ConsentState.NULL, code); 179 String codeString = ((PrimitiveType) code).asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return new Enumeration<ConsentState>(this, ConsentState.NULL, code); 182 if ("draft".equals(codeString)) 183 return new Enumeration<ConsentState>(this, ConsentState.DRAFT, code); 184 if ("active".equals(codeString)) 185 return new Enumeration<ConsentState>(this, ConsentState.ACTIVE, code); 186 if ("inactive".equals(codeString)) 187 return new Enumeration<ConsentState>(this, ConsentState.INACTIVE, code); 188 if ("not-done".equals(codeString)) 189 return new Enumeration<ConsentState>(this, ConsentState.NOTDONE, code); 190 if ("entered-in-error".equals(codeString)) 191 return new Enumeration<ConsentState>(this, ConsentState.ENTEREDINERROR, code); 192 if ("unknown".equals(codeString)) 193 return new Enumeration<ConsentState>(this, ConsentState.UNKNOWN, code); 194 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 195 } 196 public String toCode(ConsentState code) { 197 if (code == ConsentState.NULL) 198 return null; 199 if (code == ConsentState.DRAFT) 200 return "draft"; 201 if (code == ConsentState.ACTIVE) 202 return "active"; 203 if (code == ConsentState.INACTIVE) 204 return "inactive"; 205 if (code == ConsentState.NOTDONE) 206 return "not-done"; 207 if (code == ConsentState.ENTEREDINERROR) 208 return "entered-in-error"; 209 if (code == ConsentState.UNKNOWN) 210 return "unknown"; 211 return "?"; 212 } 213 public String toSystem(ConsentState code) { 214 return code.getSystem(); 215 } 216 } 217 218 @Block() 219 public static class ConsentPolicyBasisComponent extends BackboneElement implements IBaseBackboneElement { 220 /** 221 * A Reference that identifies the policy the organization will enforce for this Consent. 222 */ 223 @Child(name = "reference", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 224 @Description(shortDefinition="Reference backing policy resource", formalDefinition="A Reference that identifies the policy the organization will enforce for this Consent." ) 225 protected Reference reference; 226 227 /** 228 * A URL that links to a computable version of the policy the organization will enforce for this Consent. 229 */ 230 @Child(name = "url", type = {UrlType.class}, order=2, min=0, max=1, modifier=false, summary=false) 231 @Description(shortDefinition="URL to a computable backing policy", formalDefinition="A URL that links to a computable version of the policy the organization will enforce for this Consent." ) 232 protected UrlType url; 233 234 private static final long serialVersionUID = 252506182L; 235 236 /** 237 * Constructor 238 */ 239 public ConsentPolicyBasisComponent() { 240 super(); 241 } 242 243 /** 244 * @return {@link #reference} (A Reference that identifies the policy the organization will enforce for this Consent.) 245 */ 246 public Reference getReference() { 247 if (this.reference == null) 248 if (Configuration.errorOnAutoCreate()) 249 throw new Error("Attempt to auto-create ConsentPolicyBasisComponent.reference"); 250 else if (Configuration.doAutoCreate()) 251 this.reference = new Reference(); // cc 252 return this.reference; 253 } 254 255 public boolean hasReference() { 256 return this.reference != null && !this.reference.isEmpty(); 257 } 258 259 /** 260 * @param value {@link #reference} (A Reference that identifies the policy the organization will enforce for this Consent.) 261 */ 262 public ConsentPolicyBasisComponent setReference(Reference value) { 263 this.reference = value; 264 return this; 265 } 266 267 /** 268 * @return {@link #url} (A URL that links to a computable version of the policy the organization will enforce for this Consent.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 269 */ 270 public UrlType getUrlElement() { 271 if (this.url == null) 272 if (Configuration.errorOnAutoCreate()) 273 throw new Error("Attempt to auto-create ConsentPolicyBasisComponent.url"); 274 else if (Configuration.doAutoCreate()) 275 this.url = new UrlType(); // bb 276 return this.url; 277 } 278 279 public boolean hasUrlElement() { 280 return this.url != null && !this.url.isEmpty(); 281 } 282 283 public boolean hasUrl() { 284 return this.url != null && !this.url.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #url} (A URL that links to a computable version of the policy the organization will enforce for this Consent.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 289 */ 290 public ConsentPolicyBasisComponent setUrlElement(UrlType value) { 291 this.url = value; 292 return this; 293 } 294 295 /** 296 * @return A URL that links to a computable version of the policy the organization will enforce for this Consent. 297 */ 298 public String getUrl() { 299 return this.url == null ? null : this.url.getValue(); 300 } 301 302 /** 303 * @param value A URL that links to a computable version of the policy the organization will enforce for this Consent. 304 */ 305 public ConsentPolicyBasisComponent setUrl(String value) { 306 if (Utilities.noString(value)) 307 this.url = null; 308 else { 309 if (this.url == null) 310 this.url = new UrlType(); 311 this.url.setValue(value); 312 } 313 return this; 314 } 315 316 protected void listChildren(List<Property> children) { 317 super.listChildren(children); 318 children.add(new Property("reference", "Reference(Any)", "A Reference that identifies the policy the organization will enforce for this Consent.", 0, 1, reference)); 319 children.add(new Property("url", "url", "A URL that links to a computable version of the policy the organization will enforce for this Consent.", 0, 1, url)); 320 } 321 322 @Override 323 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 324 switch (_hash) { 325 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A Reference that identifies the policy the organization will enforce for this Consent.", 0, 1, reference); 326 case 116079: /*url*/ return new Property("url", "url", "A URL that links to a computable version of the policy the organization will enforce for this Consent.", 0, 1, url); 327 default: return super.getNamedProperty(_hash, _name, _checkValid); 328 } 329 330 } 331 332 @Override 333 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 334 switch (hash) { 335 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 336 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 337 default: return super.getProperty(hash, name, checkValid); 338 } 339 340 } 341 342 @Override 343 public Base setProperty(int hash, String name, Base value) throws FHIRException { 344 switch (hash) { 345 case -925155509: // reference 346 this.reference = TypeConvertor.castToReference(value); // Reference 347 return value; 348 case 116079: // url 349 this.url = TypeConvertor.castToUrl(value); // UrlType 350 return value; 351 default: return super.setProperty(hash, name, value); 352 } 353 354 } 355 356 @Override 357 public Base setProperty(String name, Base value) throws FHIRException { 358 if (name.equals("reference")) { 359 this.reference = TypeConvertor.castToReference(value); // Reference 360 } else if (name.equals("url")) { 361 this.url = TypeConvertor.castToUrl(value); // UrlType 362 } else 363 return super.setProperty(name, value); 364 return value; 365 } 366 367 @Override 368 public void removeChild(String name, Base value) throws FHIRException { 369 if (name.equals("reference")) { 370 this.reference = null; 371 } else if (name.equals("url")) { 372 this.url = null; 373 } else 374 super.removeChild(name, value); 375 376 } 377 378 @Override 379 public Base makeProperty(int hash, String name) throws FHIRException { 380 switch (hash) { 381 case -925155509: return getReference(); 382 case 116079: return getUrlElement(); 383 default: return super.makeProperty(hash, name); 384 } 385 386 } 387 388 @Override 389 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 390 switch (hash) { 391 case -925155509: /*reference*/ return new String[] {"Reference"}; 392 case 116079: /*url*/ return new String[] {"url"}; 393 default: return super.getTypesForProperty(hash, name); 394 } 395 396 } 397 398 @Override 399 public Base addChild(String name) throws FHIRException { 400 if (name.equals("reference")) { 401 this.reference = new Reference(); 402 return this.reference; 403 } 404 else if (name.equals("url")) { 405 throw new FHIRException("Cannot call addChild on a singleton property Consent.policyBasis.url"); 406 } 407 else 408 return super.addChild(name); 409 } 410 411 public ConsentPolicyBasisComponent copy() { 412 ConsentPolicyBasisComponent dst = new ConsentPolicyBasisComponent(); 413 copyValues(dst); 414 return dst; 415 } 416 417 public void copyValues(ConsentPolicyBasisComponent dst) { 418 super.copyValues(dst); 419 dst.reference = reference == null ? null : reference.copy(); 420 dst.url = url == null ? null : url.copy(); 421 } 422 423 @Override 424 public boolean equalsDeep(Base other_) { 425 if (!super.equalsDeep(other_)) 426 return false; 427 if (!(other_ instanceof ConsentPolicyBasisComponent)) 428 return false; 429 ConsentPolicyBasisComponent o = (ConsentPolicyBasisComponent) other_; 430 return compareDeep(reference, o.reference, true) && compareDeep(url, o.url, true); 431 } 432 433 @Override 434 public boolean equalsShallow(Base other_) { 435 if (!super.equalsShallow(other_)) 436 return false; 437 if (!(other_ instanceof ConsentPolicyBasisComponent)) 438 return false; 439 ConsentPolicyBasisComponent o = (ConsentPolicyBasisComponent) other_; 440 return compareValues(url, o.url, true); 441 } 442 443 public boolean isEmpty() { 444 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, url); 445 } 446 447 public String fhirType() { 448 return "Consent.policyBasis"; 449 450 } 451 452 } 453 454 @Block() 455 public static class ConsentVerificationComponent extends BackboneElement implements IBaseBackboneElement { 456 /** 457 * Has the instruction been verified. 458 */ 459 @Child(name = "verified", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=true) 460 @Description(shortDefinition="Has been verified", formalDefinition="Has the instruction been verified." ) 461 protected BooleanType verified; 462 463 /** 464 * Extensible list of verification type starting with verification and re-validation. 465 */ 466 @Child(name = "verificationType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 467 @Description(shortDefinition="Business case of verification", formalDefinition="Extensible list of verification type starting with verification and re-validation." ) 468 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-verification") 469 protected CodeableConcept verificationType; 470 471 /** 472 * The person who conducted the verification/validation of the Grantor decision. 473 */ 474 @Child(name = "verifiedBy", type = {Organization.class, Practitioner.class, PractitionerRole.class}, order=3, min=0, max=1, modifier=false, summary=false) 475 @Description(shortDefinition="Person conducting verification", formalDefinition="The person who conducted the verification/validation of the Grantor decision." ) 476 protected Reference verifiedBy; 477 478 /** 479 * Who verified the instruction (Patient, Relative or other Authorized Person). 480 */ 481 @Child(name = "verifiedWith", type = {Patient.class, RelatedPerson.class}, order=4, min=0, max=1, modifier=false, summary=false) 482 @Description(shortDefinition="Person who verified", formalDefinition="Who verified the instruction (Patient, Relative or other Authorized Person)." ) 483 protected Reference verifiedWith; 484 485 /** 486 * Date(s) verification was collected. 487 */ 488 @Child(name = "verificationDate", type = {DateTimeType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 489 @Description(shortDefinition="When consent verified", formalDefinition="Date(s) verification was collected." ) 490 protected List<DateTimeType> verificationDate; 491 492 private static final long serialVersionUID = -1266157329L; 493 494 /** 495 * Constructor 496 */ 497 public ConsentVerificationComponent() { 498 super(); 499 } 500 501 /** 502 * Constructor 503 */ 504 public ConsentVerificationComponent(boolean verified) { 505 super(); 506 this.setVerified(verified); 507 } 508 509 /** 510 * @return {@link #verified} (Has the instruction been verified.). This is the underlying object with id, value and extensions. The accessor "getVerified" gives direct access to the value 511 */ 512 public BooleanType getVerifiedElement() { 513 if (this.verified == null) 514 if (Configuration.errorOnAutoCreate()) 515 throw new Error("Attempt to auto-create ConsentVerificationComponent.verified"); 516 else if (Configuration.doAutoCreate()) 517 this.verified = new BooleanType(); // bb 518 return this.verified; 519 } 520 521 public boolean hasVerifiedElement() { 522 return this.verified != null && !this.verified.isEmpty(); 523 } 524 525 public boolean hasVerified() { 526 return this.verified != null && !this.verified.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #verified} (Has the instruction been verified.). This is the underlying object with id, value and extensions. The accessor "getVerified" gives direct access to the value 531 */ 532 public ConsentVerificationComponent setVerifiedElement(BooleanType value) { 533 this.verified = value; 534 return this; 535 } 536 537 /** 538 * @return Has the instruction been verified. 539 */ 540 public boolean getVerified() { 541 return this.verified == null || this.verified.isEmpty() ? false : this.verified.getValue(); 542 } 543 544 /** 545 * @param value Has the instruction been verified. 546 */ 547 public ConsentVerificationComponent setVerified(boolean value) { 548 if (this.verified == null) 549 this.verified = new BooleanType(); 550 this.verified.setValue(value); 551 return this; 552 } 553 554 /** 555 * @return {@link #verificationType} (Extensible list of verification type starting with verification and re-validation.) 556 */ 557 public CodeableConcept getVerificationType() { 558 if (this.verificationType == null) 559 if (Configuration.errorOnAutoCreate()) 560 throw new Error("Attempt to auto-create ConsentVerificationComponent.verificationType"); 561 else if (Configuration.doAutoCreate()) 562 this.verificationType = new CodeableConcept(); // cc 563 return this.verificationType; 564 } 565 566 public boolean hasVerificationType() { 567 return this.verificationType != null && !this.verificationType.isEmpty(); 568 } 569 570 /** 571 * @param value {@link #verificationType} (Extensible list of verification type starting with verification and re-validation.) 572 */ 573 public ConsentVerificationComponent setVerificationType(CodeableConcept value) { 574 this.verificationType = value; 575 return this; 576 } 577 578 /** 579 * @return {@link #verifiedBy} (The person who conducted the verification/validation of the Grantor decision.) 580 */ 581 public Reference getVerifiedBy() { 582 if (this.verifiedBy == null) 583 if (Configuration.errorOnAutoCreate()) 584 throw new Error("Attempt to auto-create ConsentVerificationComponent.verifiedBy"); 585 else if (Configuration.doAutoCreate()) 586 this.verifiedBy = new Reference(); // cc 587 return this.verifiedBy; 588 } 589 590 public boolean hasVerifiedBy() { 591 return this.verifiedBy != null && !this.verifiedBy.isEmpty(); 592 } 593 594 /** 595 * @param value {@link #verifiedBy} (The person who conducted the verification/validation of the Grantor decision.) 596 */ 597 public ConsentVerificationComponent setVerifiedBy(Reference value) { 598 this.verifiedBy = value; 599 return this; 600 } 601 602 /** 603 * @return {@link #verifiedWith} (Who verified the instruction (Patient, Relative or other Authorized Person).) 604 */ 605 public Reference getVerifiedWith() { 606 if (this.verifiedWith == null) 607 if (Configuration.errorOnAutoCreate()) 608 throw new Error("Attempt to auto-create ConsentVerificationComponent.verifiedWith"); 609 else if (Configuration.doAutoCreate()) 610 this.verifiedWith = new Reference(); // cc 611 return this.verifiedWith; 612 } 613 614 public boolean hasVerifiedWith() { 615 return this.verifiedWith != null && !this.verifiedWith.isEmpty(); 616 } 617 618 /** 619 * @param value {@link #verifiedWith} (Who verified the instruction (Patient, Relative or other Authorized Person).) 620 */ 621 public ConsentVerificationComponent setVerifiedWith(Reference value) { 622 this.verifiedWith = value; 623 return this; 624 } 625 626 /** 627 * @return {@link #verificationDate} (Date(s) verification was collected.) 628 */ 629 public List<DateTimeType> getVerificationDate() { 630 if (this.verificationDate == null) 631 this.verificationDate = new ArrayList<DateTimeType>(); 632 return this.verificationDate; 633 } 634 635 /** 636 * @return Returns a reference to <code>this</code> for easy method chaining 637 */ 638 public ConsentVerificationComponent setVerificationDate(List<DateTimeType> theVerificationDate) { 639 this.verificationDate = theVerificationDate; 640 return this; 641 } 642 643 public boolean hasVerificationDate() { 644 if (this.verificationDate == null) 645 return false; 646 for (DateTimeType item : this.verificationDate) 647 if (!item.isEmpty()) 648 return true; 649 return false; 650 } 651 652 /** 653 * @return {@link #verificationDate} (Date(s) verification was collected.) 654 */ 655 public DateTimeType addVerificationDateElement() {//2 656 DateTimeType t = new DateTimeType(); 657 if (this.verificationDate == null) 658 this.verificationDate = new ArrayList<DateTimeType>(); 659 this.verificationDate.add(t); 660 return t; 661 } 662 663 /** 664 * @param value {@link #verificationDate} (Date(s) verification was collected.) 665 */ 666 public ConsentVerificationComponent addVerificationDate(Date value) { //1 667 DateTimeType t = new DateTimeType(); 668 t.setValue(value); 669 if (this.verificationDate == null) 670 this.verificationDate = new ArrayList<DateTimeType>(); 671 this.verificationDate.add(t); 672 return this; 673 } 674 675 /** 676 * @param value {@link #verificationDate} (Date(s) verification was collected.) 677 */ 678 public boolean hasVerificationDate(Date value) { 679 if (this.verificationDate == null) 680 return false; 681 for (DateTimeType v : this.verificationDate) 682 if (v.getValue().equals(value)) // dateTime 683 return true; 684 return false; 685 } 686 687 protected void listChildren(List<Property> children) { 688 super.listChildren(children); 689 children.add(new Property("verified", "boolean", "Has the instruction been verified.", 0, 1, verified)); 690 children.add(new Property("verificationType", "CodeableConcept", "Extensible list of verification type starting with verification and re-validation.", 0, 1, verificationType)); 691 children.add(new Property("verifiedBy", "Reference(Organization|Practitioner|PractitionerRole)", "The person who conducted the verification/validation of the Grantor decision.", 0, 1, verifiedBy)); 692 children.add(new Property("verifiedWith", "Reference(Patient|RelatedPerson)", "Who verified the instruction (Patient, Relative or other Authorized Person).", 0, 1, verifiedWith)); 693 children.add(new Property("verificationDate", "dateTime", "Date(s) verification was collected.", 0, java.lang.Integer.MAX_VALUE, verificationDate)); 694 } 695 696 @Override 697 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 698 switch (_hash) { 699 case -1994383672: /*verified*/ return new Property("verified", "boolean", "Has the instruction been verified.", 0, 1, verified); 700 case 642733045: /*verificationType*/ return new Property("verificationType", "CodeableConcept", "Extensible list of verification type starting with verification and re-validation.", 0, 1, verificationType); 701 case -1047292609: /*verifiedBy*/ return new Property("verifiedBy", "Reference(Organization|Practitioner|PractitionerRole)", "The person who conducted the verification/validation of the Grantor decision.", 0, 1, verifiedBy); 702 case -1425236050: /*verifiedWith*/ return new Property("verifiedWith", "Reference(Patient|RelatedPerson)", "Who verified the instruction (Patient, Relative or other Authorized Person).", 0, 1, verifiedWith); 703 case 642233449: /*verificationDate*/ return new Property("verificationDate", "dateTime", "Date(s) verification was collected.", 0, java.lang.Integer.MAX_VALUE, verificationDate); 704 default: return super.getNamedProperty(_hash, _name, _checkValid); 705 } 706 707 } 708 709 @Override 710 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 711 switch (hash) { 712 case -1994383672: /*verified*/ return this.verified == null ? new Base[0] : new Base[] {this.verified}; // BooleanType 713 case 642733045: /*verificationType*/ return this.verificationType == null ? new Base[0] : new Base[] {this.verificationType}; // CodeableConcept 714 case -1047292609: /*verifiedBy*/ return this.verifiedBy == null ? new Base[0] : new Base[] {this.verifiedBy}; // Reference 715 case -1425236050: /*verifiedWith*/ return this.verifiedWith == null ? new Base[0] : new Base[] {this.verifiedWith}; // Reference 716 case 642233449: /*verificationDate*/ return this.verificationDate == null ? new Base[0] : this.verificationDate.toArray(new Base[this.verificationDate.size()]); // DateTimeType 717 default: return super.getProperty(hash, name, checkValid); 718 } 719 720 } 721 722 @Override 723 public Base setProperty(int hash, String name, Base value) throws FHIRException { 724 switch (hash) { 725 case -1994383672: // verified 726 this.verified = TypeConvertor.castToBoolean(value); // BooleanType 727 return value; 728 case 642733045: // verificationType 729 this.verificationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 730 return value; 731 case -1047292609: // verifiedBy 732 this.verifiedBy = TypeConvertor.castToReference(value); // Reference 733 return value; 734 case -1425236050: // verifiedWith 735 this.verifiedWith = TypeConvertor.castToReference(value); // Reference 736 return value; 737 case 642233449: // verificationDate 738 this.getVerificationDate().add(TypeConvertor.castToDateTime(value)); // DateTimeType 739 return value; 740 default: return super.setProperty(hash, name, value); 741 } 742 743 } 744 745 @Override 746 public Base setProperty(String name, Base value) throws FHIRException { 747 if (name.equals("verified")) { 748 this.verified = TypeConvertor.castToBoolean(value); // BooleanType 749 } else if (name.equals("verificationType")) { 750 this.verificationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 751 } else if (name.equals("verifiedBy")) { 752 this.verifiedBy = TypeConvertor.castToReference(value); // Reference 753 } else if (name.equals("verifiedWith")) { 754 this.verifiedWith = TypeConvertor.castToReference(value); // Reference 755 } else if (name.equals("verificationDate")) { 756 this.getVerificationDate().add(TypeConvertor.castToDateTime(value)); 757 } else 758 return super.setProperty(name, value); 759 return value; 760 } 761 762 @Override 763 public void removeChild(String name, Base value) throws FHIRException { 764 if (name.equals("verified")) { 765 this.verified = null; 766 } else if (name.equals("verificationType")) { 767 this.verificationType = null; 768 } else if (name.equals("verifiedBy")) { 769 this.verifiedBy = null; 770 } else if (name.equals("verifiedWith")) { 771 this.verifiedWith = null; 772 } else if (name.equals("verificationDate")) { 773 this.getVerificationDate().remove(value); 774 } else 775 super.removeChild(name, value); 776 777 } 778 779 @Override 780 public Base makeProperty(int hash, String name) throws FHIRException { 781 switch (hash) { 782 case -1994383672: return getVerifiedElement(); 783 case 642733045: return getVerificationType(); 784 case -1047292609: return getVerifiedBy(); 785 case -1425236050: return getVerifiedWith(); 786 case 642233449: return addVerificationDateElement(); 787 default: return super.makeProperty(hash, name); 788 } 789 790 } 791 792 @Override 793 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 794 switch (hash) { 795 case -1994383672: /*verified*/ return new String[] {"boolean"}; 796 case 642733045: /*verificationType*/ return new String[] {"CodeableConcept"}; 797 case -1047292609: /*verifiedBy*/ return new String[] {"Reference"}; 798 case -1425236050: /*verifiedWith*/ return new String[] {"Reference"}; 799 case 642233449: /*verificationDate*/ return new String[] {"dateTime"}; 800 default: return super.getTypesForProperty(hash, name); 801 } 802 803 } 804 805 @Override 806 public Base addChild(String name) throws FHIRException { 807 if (name.equals("verified")) { 808 throw new FHIRException("Cannot call addChild on a singleton property Consent.verification.verified"); 809 } 810 else if (name.equals("verificationType")) { 811 this.verificationType = new CodeableConcept(); 812 return this.verificationType; 813 } 814 else if (name.equals("verifiedBy")) { 815 this.verifiedBy = new Reference(); 816 return this.verifiedBy; 817 } 818 else if (name.equals("verifiedWith")) { 819 this.verifiedWith = new Reference(); 820 return this.verifiedWith; 821 } 822 else if (name.equals("verificationDate")) { 823 throw new FHIRException("Cannot call addChild on a singleton property Consent.verification.verificationDate"); 824 } 825 else 826 return super.addChild(name); 827 } 828 829 public ConsentVerificationComponent copy() { 830 ConsentVerificationComponent dst = new ConsentVerificationComponent(); 831 copyValues(dst); 832 return dst; 833 } 834 835 public void copyValues(ConsentVerificationComponent dst) { 836 super.copyValues(dst); 837 dst.verified = verified == null ? null : verified.copy(); 838 dst.verificationType = verificationType == null ? null : verificationType.copy(); 839 dst.verifiedBy = verifiedBy == null ? null : verifiedBy.copy(); 840 dst.verifiedWith = verifiedWith == null ? null : verifiedWith.copy(); 841 if (verificationDate != null) { 842 dst.verificationDate = new ArrayList<DateTimeType>(); 843 for (DateTimeType i : verificationDate) 844 dst.verificationDate.add(i.copy()); 845 }; 846 } 847 848 @Override 849 public boolean equalsDeep(Base other_) { 850 if (!super.equalsDeep(other_)) 851 return false; 852 if (!(other_ instanceof ConsentVerificationComponent)) 853 return false; 854 ConsentVerificationComponent o = (ConsentVerificationComponent) other_; 855 return compareDeep(verified, o.verified, true) && compareDeep(verificationType, o.verificationType, true) 856 && compareDeep(verifiedBy, o.verifiedBy, true) && compareDeep(verifiedWith, o.verifiedWith, true) 857 && compareDeep(verificationDate, o.verificationDate, true); 858 } 859 860 @Override 861 public boolean equalsShallow(Base other_) { 862 if (!super.equalsShallow(other_)) 863 return false; 864 if (!(other_ instanceof ConsentVerificationComponent)) 865 return false; 866 ConsentVerificationComponent o = (ConsentVerificationComponent) other_; 867 return compareValues(verified, o.verified, true) && compareValues(verificationDate, o.verificationDate, true) 868 ; 869 } 870 871 public boolean isEmpty() { 872 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(verified, verificationType 873 , verifiedBy, verifiedWith, verificationDate); 874 } 875 876 public String fhirType() { 877 return "Consent.verification"; 878 879 } 880 881 } 882 883 @Block() 884 public static class ProvisionComponent extends BackboneElement implements IBaseBackboneElement { 885 /** 886 * Timeframe for this provision. 887 */ 888 @Child(name = "period", type = {Period.class}, order=1, min=0, max=1, modifier=false, summary=true) 889 @Description(shortDefinition="Timeframe for this provision", formalDefinition="Timeframe for this provision." ) 890 protected Period period; 891 892 /** 893 * Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 894 */ 895 @Child(name = "actor", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 896 @Description(shortDefinition="Who|what controlled by this provision (or group, by role)", formalDefinition="Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 897 protected List<ProvisionActorComponent> actor; 898 899 /** 900 * Actions controlled by this provision. 901 */ 902 @Child(name = "action", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 903 @Description(shortDefinition="Actions controlled by this provision", formalDefinition="Actions controlled by this provision." ) 904 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-action") 905 protected List<CodeableConcept> action; 906 907 /** 908 * A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception. 909 */ 910 @Child(name = "securityLabel", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 911 @Description(shortDefinition="Security Labels that define affected resources", formalDefinition="A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception." ) 912 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-label-examples") 913 protected List<Coding> securityLabel; 914 915 /** 916 * The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision. 917 */ 918 @Child(name = "purpose", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 919 @Description(shortDefinition="Context of activities covered by this provision", formalDefinition="The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision." ) 920 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 921 protected List<Coding> purpose; 922 923 /** 924 * The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to. 925 */ 926 @Child(name = "documentType", type = {Coding.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 927 @Description(shortDefinition="e.g. Resource Type, Profile, CDA, etc", formalDefinition="The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to." ) 928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-class") 929 protected List<Coding> documentType; 930 931 /** 932 * The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to. 933 */ 934 @Child(name = "resourceType", type = {Coding.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 935 @Description(shortDefinition="e.g. Resource Type, Profile, etc", formalDefinition="The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to." ) 936 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 937 protected List<Coding> resourceType; 938 939 /** 940 * If this code is found in an instance, then the provision applies. 941 */ 942 @Child(name = "code", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 943 @Description(shortDefinition="e.g. LOINC or SNOMED CT code, etc. in the content", formalDefinition="If this code is found in an instance, then the provision applies." ) 944 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-code") 945 protected List<CodeableConcept> code; 946 947 /** 948 * Clinical or Operational Relevant period of time that bounds the data controlled by this provision. 949 */ 950 @Child(name = "dataPeriod", type = {Period.class}, order=9, min=0, max=1, modifier=false, summary=true) 951 @Description(shortDefinition="Timeframe for data controlled by this provision", formalDefinition="Clinical or Operational Relevant period of time that bounds the data controlled by this provision." ) 952 protected Period dataPeriod; 953 954 /** 955 * The resources controlled by this provision if specific resources are referenced. 956 */ 957 @Child(name = "data", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 958 @Description(shortDefinition="Data controlled by this provision", formalDefinition="The resources controlled by this provision if specific resources are referenced." ) 959 protected List<ProvisionDataComponent> data; 960 961 /** 962 * A computable (FHIRPath or other) definition of what is controlled by this consent. 963 */ 964 @Child(name = "expression", type = {Expression.class}, order=11, min=0, max=1, modifier=false, summary=false) 965 @Description(shortDefinition="A computable expression of the consent", formalDefinition="A computable (FHIRPath or other) definition of what is controlled by this consent." ) 966 protected Expression expression; 967 968 /** 969 * Provisions which provide exceptions to the base provision or subprovisions. 970 */ 971 @Child(name = "provision", type = {ProvisionComponent.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 972 @Description(shortDefinition="Nested Exception Provisions", formalDefinition="Provisions which provide exceptions to the base provision or subprovisions." ) 973 protected List<ProvisionComponent> provision; 974 975 private static final long serialVersionUID = -587666915L; 976 977 /** 978 * Constructor 979 */ 980 public ProvisionComponent() { 981 super(); 982 } 983 984 /** 985 * @return {@link #period} (Timeframe for this provision.) 986 */ 987 public Period getPeriod() { 988 if (this.period == null) 989 if (Configuration.errorOnAutoCreate()) 990 throw new Error("Attempt to auto-create ProvisionComponent.period"); 991 else if (Configuration.doAutoCreate()) 992 this.period = new Period(); // cc 993 return this.period; 994 } 995 996 public boolean hasPeriod() { 997 return this.period != null && !this.period.isEmpty(); 998 } 999 1000 /** 1001 * @param value {@link #period} (Timeframe for this provision.) 1002 */ 1003 public ProvisionComponent setPeriod(Period value) { 1004 this.period = value; 1005 return this; 1006 } 1007 1008 /** 1009 * @return {@link #actor} (Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1010 */ 1011 public List<ProvisionActorComponent> getActor() { 1012 if (this.actor == null) 1013 this.actor = new ArrayList<ProvisionActorComponent>(); 1014 return this.actor; 1015 } 1016 1017 /** 1018 * @return Returns a reference to <code>this</code> for easy method chaining 1019 */ 1020 public ProvisionComponent setActor(List<ProvisionActorComponent> theActor) { 1021 this.actor = theActor; 1022 return this; 1023 } 1024 1025 public boolean hasActor() { 1026 if (this.actor == null) 1027 return false; 1028 for (ProvisionActorComponent item : this.actor) 1029 if (!item.isEmpty()) 1030 return true; 1031 return false; 1032 } 1033 1034 public ProvisionActorComponent addActor() { //3 1035 ProvisionActorComponent t = new ProvisionActorComponent(); 1036 if (this.actor == null) 1037 this.actor = new ArrayList<ProvisionActorComponent>(); 1038 this.actor.add(t); 1039 return t; 1040 } 1041 1042 public ProvisionComponent addActor(ProvisionActorComponent t) { //3 1043 if (t == null) 1044 return this; 1045 if (this.actor == null) 1046 this.actor = new ArrayList<ProvisionActorComponent>(); 1047 this.actor.add(t); 1048 return this; 1049 } 1050 1051 /** 1052 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist {3} 1053 */ 1054 public ProvisionActorComponent getActorFirstRep() { 1055 if (getActor().isEmpty()) { 1056 addActor(); 1057 } 1058 return getActor().get(0); 1059 } 1060 1061 /** 1062 * @return {@link #action} (Actions controlled by this provision.) 1063 */ 1064 public List<CodeableConcept> getAction() { 1065 if (this.action == null) 1066 this.action = new ArrayList<CodeableConcept>(); 1067 return this.action; 1068 } 1069 1070 /** 1071 * @return Returns a reference to <code>this</code> for easy method chaining 1072 */ 1073 public ProvisionComponent setAction(List<CodeableConcept> theAction) { 1074 this.action = theAction; 1075 return this; 1076 } 1077 1078 public boolean hasAction() { 1079 if (this.action == null) 1080 return false; 1081 for (CodeableConcept item : this.action) 1082 if (!item.isEmpty()) 1083 return true; 1084 return false; 1085 } 1086 1087 public CodeableConcept addAction() { //3 1088 CodeableConcept t = new CodeableConcept(); 1089 if (this.action == null) 1090 this.action = new ArrayList<CodeableConcept>(); 1091 this.action.add(t); 1092 return t; 1093 } 1094 1095 public ProvisionComponent addAction(CodeableConcept t) { //3 1096 if (t == null) 1097 return this; 1098 if (this.action == null) 1099 this.action = new ArrayList<CodeableConcept>(); 1100 this.action.add(t); 1101 return this; 1102 } 1103 1104 /** 1105 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 1106 */ 1107 public CodeableConcept getActionFirstRep() { 1108 if (getAction().isEmpty()) { 1109 addAction(); 1110 } 1111 return getAction().get(0); 1112 } 1113 1114 /** 1115 * @return {@link #securityLabel} (A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.) 1116 */ 1117 public List<Coding> getSecurityLabel() { 1118 if (this.securityLabel == null) 1119 this.securityLabel = new ArrayList<Coding>(); 1120 return this.securityLabel; 1121 } 1122 1123 /** 1124 * @return Returns a reference to <code>this</code> for easy method chaining 1125 */ 1126 public ProvisionComponent setSecurityLabel(List<Coding> theSecurityLabel) { 1127 this.securityLabel = theSecurityLabel; 1128 return this; 1129 } 1130 1131 public boolean hasSecurityLabel() { 1132 if (this.securityLabel == null) 1133 return false; 1134 for (Coding item : this.securityLabel) 1135 if (!item.isEmpty()) 1136 return true; 1137 return false; 1138 } 1139 1140 public Coding addSecurityLabel() { //3 1141 Coding t = new Coding(); 1142 if (this.securityLabel == null) 1143 this.securityLabel = new ArrayList<Coding>(); 1144 this.securityLabel.add(t); 1145 return t; 1146 } 1147 1148 public ProvisionComponent addSecurityLabel(Coding t) { //3 1149 if (t == null) 1150 return this; 1151 if (this.securityLabel == null) 1152 this.securityLabel = new ArrayList<Coding>(); 1153 this.securityLabel.add(t); 1154 return this; 1155 } 1156 1157 /** 1158 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist {3} 1159 */ 1160 public Coding getSecurityLabelFirstRep() { 1161 if (getSecurityLabel().isEmpty()) { 1162 addSecurityLabel(); 1163 } 1164 return getSecurityLabel().get(0); 1165 } 1166 1167 /** 1168 * @return {@link #purpose} (The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision.) 1169 */ 1170 public List<Coding> getPurpose() { 1171 if (this.purpose == null) 1172 this.purpose = new ArrayList<Coding>(); 1173 return this.purpose; 1174 } 1175 1176 /** 1177 * @return Returns a reference to <code>this</code> for easy method chaining 1178 */ 1179 public ProvisionComponent setPurpose(List<Coding> thePurpose) { 1180 this.purpose = thePurpose; 1181 return this; 1182 } 1183 1184 public boolean hasPurpose() { 1185 if (this.purpose == null) 1186 return false; 1187 for (Coding item : this.purpose) 1188 if (!item.isEmpty()) 1189 return true; 1190 return false; 1191 } 1192 1193 public Coding addPurpose() { //3 1194 Coding t = new Coding(); 1195 if (this.purpose == null) 1196 this.purpose = new ArrayList<Coding>(); 1197 this.purpose.add(t); 1198 return t; 1199 } 1200 1201 public ProvisionComponent addPurpose(Coding t) { //3 1202 if (t == null) 1203 return this; 1204 if (this.purpose == null) 1205 this.purpose = new ArrayList<Coding>(); 1206 this.purpose.add(t); 1207 return this; 1208 } 1209 1210 /** 1211 * @return The first repetition of repeating field {@link #purpose}, creating it if it does not already exist {3} 1212 */ 1213 public Coding getPurposeFirstRep() { 1214 if (getPurpose().isEmpty()) { 1215 addPurpose(); 1216 } 1217 return getPurpose().get(0); 1218 } 1219 1220 /** 1221 * @return {@link #documentType} (The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to.) 1222 */ 1223 public List<Coding> getDocumentType() { 1224 if (this.documentType == null) 1225 this.documentType = new ArrayList<Coding>(); 1226 return this.documentType; 1227 } 1228 1229 /** 1230 * @return Returns a reference to <code>this</code> for easy method chaining 1231 */ 1232 public ProvisionComponent setDocumentType(List<Coding> theDocumentType) { 1233 this.documentType = theDocumentType; 1234 return this; 1235 } 1236 1237 public boolean hasDocumentType() { 1238 if (this.documentType == null) 1239 return false; 1240 for (Coding item : this.documentType) 1241 if (!item.isEmpty()) 1242 return true; 1243 return false; 1244 } 1245 1246 public Coding addDocumentType() { //3 1247 Coding t = new Coding(); 1248 if (this.documentType == null) 1249 this.documentType = new ArrayList<Coding>(); 1250 this.documentType.add(t); 1251 return t; 1252 } 1253 1254 public ProvisionComponent addDocumentType(Coding t) { //3 1255 if (t == null) 1256 return this; 1257 if (this.documentType == null) 1258 this.documentType = new ArrayList<Coding>(); 1259 this.documentType.add(t); 1260 return this; 1261 } 1262 1263 /** 1264 * @return The first repetition of repeating field {@link #documentType}, creating it if it does not already exist {3} 1265 */ 1266 public Coding getDocumentTypeFirstRep() { 1267 if (getDocumentType().isEmpty()) { 1268 addDocumentType(); 1269 } 1270 return getDocumentType().get(0); 1271 } 1272 1273 /** 1274 * @return {@link #resourceType} (The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to.) 1275 */ 1276 public List<Coding> getResourceType() { 1277 if (this.resourceType == null) 1278 this.resourceType = new ArrayList<Coding>(); 1279 return this.resourceType; 1280 } 1281 1282 /** 1283 * @return Returns a reference to <code>this</code> for easy method chaining 1284 */ 1285 public ProvisionComponent setResourceType(List<Coding> theResourceType) { 1286 this.resourceType = theResourceType; 1287 return this; 1288 } 1289 1290 public boolean hasResourceType() { 1291 if (this.resourceType == null) 1292 return false; 1293 for (Coding item : this.resourceType) 1294 if (!item.isEmpty()) 1295 return true; 1296 return false; 1297 } 1298 1299 public Coding addResourceType() { //3 1300 Coding t = new Coding(); 1301 if (this.resourceType == null) 1302 this.resourceType = new ArrayList<Coding>(); 1303 this.resourceType.add(t); 1304 return t; 1305 } 1306 1307 public ProvisionComponent addResourceType(Coding t) { //3 1308 if (t == null) 1309 return this; 1310 if (this.resourceType == null) 1311 this.resourceType = new ArrayList<Coding>(); 1312 this.resourceType.add(t); 1313 return this; 1314 } 1315 1316 /** 1317 * @return The first repetition of repeating field {@link #resourceType}, creating it if it does not already exist {3} 1318 */ 1319 public Coding getResourceTypeFirstRep() { 1320 if (getResourceType().isEmpty()) { 1321 addResourceType(); 1322 } 1323 return getResourceType().get(0); 1324 } 1325 1326 /** 1327 * @return {@link #code} (If this code is found in an instance, then the provision applies.) 1328 */ 1329 public List<CodeableConcept> getCode() { 1330 if (this.code == null) 1331 this.code = new ArrayList<CodeableConcept>(); 1332 return this.code; 1333 } 1334 1335 /** 1336 * @return Returns a reference to <code>this</code> for easy method chaining 1337 */ 1338 public ProvisionComponent setCode(List<CodeableConcept> theCode) { 1339 this.code = theCode; 1340 return this; 1341 } 1342 1343 public boolean hasCode() { 1344 if (this.code == null) 1345 return false; 1346 for (CodeableConcept item : this.code) 1347 if (!item.isEmpty()) 1348 return true; 1349 return false; 1350 } 1351 1352 public CodeableConcept addCode() { //3 1353 CodeableConcept t = new CodeableConcept(); 1354 if (this.code == null) 1355 this.code = new ArrayList<CodeableConcept>(); 1356 this.code.add(t); 1357 return t; 1358 } 1359 1360 public ProvisionComponent addCode(CodeableConcept t) { //3 1361 if (t == null) 1362 return this; 1363 if (this.code == null) 1364 this.code = new ArrayList<CodeableConcept>(); 1365 this.code.add(t); 1366 return this; 1367 } 1368 1369 /** 1370 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 1371 */ 1372 public CodeableConcept getCodeFirstRep() { 1373 if (getCode().isEmpty()) { 1374 addCode(); 1375 } 1376 return getCode().get(0); 1377 } 1378 1379 /** 1380 * @return {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this provision.) 1381 */ 1382 public Period getDataPeriod() { 1383 if (this.dataPeriod == null) 1384 if (Configuration.errorOnAutoCreate()) 1385 throw new Error("Attempt to auto-create ProvisionComponent.dataPeriod"); 1386 else if (Configuration.doAutoCreate()) 1387 this.dataPeriod = new Period(); // cc 1388 return this.dataPeriod; 1389 } 1390 1391 public boolean hasDataPeriod() { 1392 return this.dataPeriod != null && !this.dataPeriod.isEmpty(); 1393 } 1394 1395 /** 1396 * @param value {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this provision.) 1397 */ 1398 public ProvisionComponent setDataPeriod(Period value) { 1399 this.dataPeriod = value; 1400 return this; 1401 } 1402 1403 /** 1404 * @return {@link #data} (The resources controlled by this provision if specific resources are referenced.) 1405 */ 1406 public List<ProvisionDataComponent> getData() { 1407 if (this.data == null) 1408 this.data = new ArrayList<ProvisionDataComponent>(); 1409 return this.data; 1410 } 1411 1412 /** 1413 * @return Returns a reference to <code>this</code> for easy method chaining 1414 */ 1415 public ProvisionComponent setData(List<ProvisionDataComponent> theData) { 1416 this.data = theData; 1417 return this; 1418 } 1419 1420 public boolean hasData() { 1421 if (this.data == null) 1422 return false; 1423 for (ProvisionDataComponent item : this.data) 1424 if (!item.isEmpty()) 1425 return true; 1426 return false; 1427 } 1428 1429 public ProvisionDataComponent addData() { //3 1430 ProvisionDataComponent t = new ProvisionDataComponent(); 1431 if (this.data == null) 1432 this.data = new ArrayList<ProvisionDataComponent>(); 1433 this.data.add(t); 1434 return t; 1435 } 1436 1437 public ProvisionComponent addData(ProvisionDataComponent t) { //3 1438 if (t == null) 1439 return this; 1440 if (this.data == null) 1441 this.data = new ArrayList<ProvisionDataComponent>(); 1442 this.data.add(t); 1443 return this; 1444 } 1445 1446 /** 1447 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist {3} 1448 */ 1449 public ProvisionDataComponent getDataFirstRep() { 1450 if (getData().isEmpty()) { 1451 addData(); 1452 } 1453 return getData().get(0); 1454 } 1455 1456 /** 1457 * @return {@link #expression} (A computable (FHIRPath or other) definition of what is controlled by this consent.) 1458 */ 1459 public Expression getExpression() { 1460 if (this.expression == null) 1461 if (Configuration.errorOnAutoCreate()) 1462 throw new Error("Attempt to auto-create ProvisionComponent.expression"); 1463 else if (Configuration.doAutoCreate()) 1464 this.expression = new Expression(); // cc 1465 return this.expression; 1466 } 1467 1468 public boolean hasExpression() { 1469 return this.expression != null && !this.expression.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #expression} (A computable (FHIRPath or other) definition of what is controlled by this consent.) 1474 */ 1475 public ProvisionComponent setExpression(Expression value) { 1476 this.expression = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return {@link #provision} (Provisions which provide exceptions to the base provision or subprovisions.) 1482 */ 1483 public List<ProvisionComponent> getProvision() { 1484 if (this.provision == null) 1485 this.provision = new ArrayList<ProvisionComponent>(); 1486 return this.provision; 1487 } 1488 1489 /** 1490 * @return Returns a reference to <code>this</code> for easy method chaining 1491 */ 1492 public ProvisionComponent setProvision(List<ProvisionComponent> theProvision) { 1493 this.provision = theProvision; 1494 return this; 1495 } 1496 1497 public boolean hasProvision() { 1498 if (this.provision == null) 1499 return false; 1500 for (ProvisionComponent item : this.provision) 1501 if (!item.isEmpty()) 1502 return true; 1503 return false; 1504 } 1505 1506 public ProvisionComponent addProvision() { //3 1507 ProvisionComponent t = new ProvisionComponent(); 1508 if (this.provision == null) 1509 this.provision = new ArrayList<ProvisionComponent>(); 1510 this.provision.add(t); 1511 return t; 1512 } 1513 1514 public ProvisionComponent addProvision(ProvisionComponent t) { //3 1515 if (t == null) 1516 return this; 1517 if (this.provision == null) 1518 this.provision = new ArrayList<ProvisionComponent>(); 1519 this.provision.add(t); 1520 return this; 1521 } 1522 1523 /** 1524 * @return The first repetition of repeating field {@link #provision}, creating it if it does not already exist {3} 1525 */ 1526 public ProvisionComponent getProvisionFirstRep() { 1527 if (getProvision().isEmpty()) { 1528 addProvision(); 1529 } 1530 return getProvision().get(0); 1531 } 1532 1533 protected void listChildren(List<Property> children) { 1534 super.listChildren(children); 1535 children.add(new Property("period", "Period", "Timeframe for this provision.", 0, 1, period)); 1536 children.add(new Property("actor", "", "Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor)); 1537 children.add(new Property("action", "CodeableConcept", "Actions controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, action)); 1538 children.add(new Property("securityLabel", "Coding", "A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 1539 children.add(new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, purpose)); 1540 children.add(new Property("documentType", "Coding", "The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, documentType)); 1541 children.add(new Property("resourceType", "Coding", "The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, resourceType)); 1542 children.add(new Property("code", "CodeableConcept", "If this code is found in an instance, then the provision applies.", 0, java.lang.Integer.MAX_VALUE, code)); 1543 children.add(new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this provision.", 0, 1, dataPeriod)); 1544 children.add(new Property("data", "", "The resources controlled by this provision if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data)); 1545 children.add(new Property("expression", "Expression", "A computable (FHIRPath or other) definition of what is controlled by this consent.", 0, 1, expression)); 1546 children.add(new Property("provision", "@Consent.provision", "Provisions which provide exceptions to the base provision or subprovisions.", 0, java.lang.Integer.MAX_VALUE, provision)); 1547 } 1548 1549 @Override 1550 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1551 switch (_hash) { 1552 case -991726143: /*period*/ return new Property("period", "Period", "Timeframe for this provision.", 0, 1, period); 1553 case 92645877: /*actor*/ return new Property("actor", "", "Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor); 1554 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Actions controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, action); 1555 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 1556 case -220463842: /*purpose*/ return new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, purpose); 1557 case -1473196299: /*documentType*/ return new Property("documentType", "Coding", "The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, documentType); 1558 case -384364440: /*resourceType*/ return new Property("resourceType", "Coding", "The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, resourceType); 1559 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "If this code is found in an instance, then the provision applies.", 0, java.lang.Integer.MAX_VALUE, code); 1560 case 1177250315: /*dataPeriod*/ return new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this provision.", 0, 1, dataPeriod); 1561 case 3076010: /*data*/ return new Property("data", "", "The resources controlled by this provision if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data); 1562 case -1795452264: /*expression*/ return new Property("expression", "Expression", "A computable (FHIRPath or other) definition of what is controlled by this consent.", 0, 1, expression); 1563 case -547120939: /*provision*/ return new Property("provision", "@Consent.provision", "Provisions which provide exceptions to the base provision or subprovisions.", 0, java.lang.Integer.MAX_VALUE, provision); 1564 default: return super.getNamedProperty(_hash, _name, _checkValid); 1565 } 1566 1567 } 1568 1569 @Override 1570 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1571 switch (hash) { 1572 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1573 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ProvisionActorComponent 1574 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 1575 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 1576 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Coding 1577 case -1473196299: /*documentType*/ return this.documentType == null ? new Base[0] : this.documentType.toArray(new Base[this.documentType.size()]); // Coding 1578 case -384364440: /*resourceType*/ return this.resourceType == null ? new Base[0] : this.resourceType.toArray(new Base[this.resourceType.size()]); // Coding 1579 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1580 case 1177250315: /*dataPeriod*/ return this.dataPeriod == null ? new Base[0] : new Base[] {this.dataPeriod}; // Period 1581 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // ProvisionDataComponent 1582 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 1583 case -547120939: /*provision*/ return this.provision == null ? new Base[0] : this.provision.toArray(new Base[this.provision.size()]); // ProvisionComponent 1584 default: return super.getProperty(hash, name, checkValid); 1585 } 1586 1587 } 1588 1589 @Override 1590 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1591 switch (hash) { 1592 case -991726143: // period 1593 this.period = TypeConvertor.castToPeriod(value); // Period 1594 return value; 1595 case 92645877: // actor 1596 this.getActor().add((ProvisionActorComponent) value); // ProvisionActorComponent 1597 return value; 1598 case -1422950858: // action 1599 this.getAction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1600 return value; 1601 case -722296940: // securityLabel 1602 this.getSecurityLabel().add(TypeConvertor.castToCoding(value)); // Coding 1603 return value; 1604 case -220463842: // purpose 1605 this.getPurpose().add(TypeConvertor.castToCoding(value)); // Coding 1606 return value; 1607 case -1473196299: // documentType 1608 this.getDocumentType().add(TypeConvertor.castToCoding(value)); // Coding 1609 return value; 1610 case -384364440: // resourceType 1611 this.getResourceType().add(TypeConvertor.castToCoding(value)); // Coding 1612 return value; 1613 case 3059181: // code 1614 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1615 return value; 1616 case 1177250315: // dataPeriod 1617 this.dataPeriod = TypeConvertor.castToPeriod(value); // Period 1618 return value; 1619 case 3076010: // data 1620 this.getData().add((ProvisionDataComponent) value); // ProvisionDataComponent 1621 return value; 1622 case -1795452264: // expression 1623 this.expression = TypeConvertor.castToExpression(value); // Expression 1624 return value; 1625 case -547120939: // provision 1626 this.getProvision().add((ProvisionComponent) value); // ProvisionComponent 1627 return value; 1628 default: return super.setProperty(hash, name, value); 1629 } 1630 1631 } 1632 1633 @Override 1634 public Base setProperty(String name, Base value) throws FHIRException { 1635 if (name.equals("period")) { 1636 this.period = TypeConvertor.castToPeriod(value); // Period 1637 } else if (name.equals("actor")) { 1638 this.getActor().add((ProvisionActorComponent) value); 1639 } else if (name.equals("action")) { 1640 this.getAction().add(TypeConvertor.castToCodeableConcept(value)); 1641 } else if (name.equals("securityLabel")) { 1642 this.getSecurityLabel().add(TypeConvertor.castToCoding(value)); 1643 } else if (name.equals("purpose")) { 1644 this.getPurpose().add(TypeConvertor.castToCoding(value)); 1645 } else if (name.equals("documentType")) { 1646 this.getDocumentType().add(TypeConvertor.castToCoding(value)); 1647 } else if (name.equals("resourceType")) { 1648 this.getResourceType().add(TypeConvertor.castToCoding(value)); 1649 } else if (name.equals("code")) { 1650 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 1651 } else if (name.equals("dataPeriod")) { 1652 this.dataPeriod = TypeConvertor.castToPeriod(value); // Period 1653 } else if (name.equals("data")) { 1654 this.getData().add((ProvisionDataComponent) value); 1655 } else if (name.equals("expression")) { 1656 this.expression = TypeConvertor.castToExpression(value); // Expression 1657 } else if (name.equals("provision")) { 1658 this.getProvision().add((ProvisionComponent) value); 1659 } else 1660 return super.setProperty(name, value); 1661 return value; 1662 } 1663 1664 @Override 1665 public void removeChild(String name, Base value) throws FHIRException { 1666 if (name.equals("period")) { 1667 this.period = null; 1668 } else if (name.equals("actor")) { 1669 this.getActor().remove((ProvisionActorComponent) value); 1670 } else if (name.equals("action")) { 1671 this.getAction().remove(value); 1672 } else if (name.equals("securityLabel")) { 1673 this.getSecurityLabel().remove(value); 1674 } else if (name.equals("purpose")) { 1675 this.getPurpose().remove(value); 1676 } else if (name.equals("documentType")) { 1677 this.getDocumentType().remove(value); 1678 } else if (name.equals("resourceType")) { 1679 this.getResourceType().remove(value); 1680 } else if (name.equals("code")) { 1681 this.getCode().remove(value); 1682 } else if (name.equals("dataPeriod")) { 1683 this.dataPeriod = null; 1684 } else if (name.equals("data")) { 1685 this.getData().remove((ProvisionDataComponent) value); 1686 } else if (name.equals("expression")) { 1687 this.expression = null; 1688 } else if (name.equals("provision")) { 1689 this.getProvision().remove((ProvisionComponent) value); 1690 } else 1691 super.removeChild(name, value); 1692 1693 } 1694 1695 @Override 1696 public Base makeProperty(int hash, String name) throws FHIRException { 1697 switch (hash) { 1698 case -991726143: return getPeriod(); 1699 case 92645877: return addActor(); 1700 case -1422950858: return addAction(); 1701 case -722296940: return addSecurityLabel(); 1702 case -220463842: return addPurpose(); 1703 case -1473196299: return addDocumentType(); 1704 case -384364440: return addResourceType(); 1705 case 3059181: return addCode(); 1706 case 1177250315: return getDataPeriod(); 1707 case 3076010: return addData(); 1708 case -1795452264: return getExpression(); 1709 case -547120939: return addProvision(); 1710 default: return super.makeProperty(hash, name); 1711 } 1712 1713 } 1714 1715 @Override 1716 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1717 switch (hash) { 1718 case -991726143: /*period*/ return new String[] {"Period"}; 1719 case 92645877: /*actor*/ return new String[] {}; 1720 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 1721 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 1722 case -220463842: /*purpose*/ return new String[] {"Coding"}; 1723 case -1473196299: /*documentType*/ return new String[] {"Coding"}; 1724 case -384364440: /*resourceType*/ return new String[] {"Coding"}; 1725 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1726 case 1177250315: /*dataPeriod*/ return new String[] {"Period"}; 1727 case 3076010: /*data*/ return new String[] {}; 1728 case -1795452264: /*expression*/ return new String[] {"Expression"}; 1729 case -547120939: /*provision*/ return new String[] {"@Consent.provision"}; 1730 default: return super.getTypesForProperty(hash, name); 1731 } 1732 1733 } 1734 1735 @Override 1736 public Base addChild(String name) throws FHIRException { 1737 if (name.equals("period")) { 1738 this.period = new Period(); 1739 return this.period; 1740 } 1741 else if (name.equals("actor")) { 1742 return addActor(); 1743 } 1744 else if (name.equals("action")) { 1745 return addAction(); 1746 } 1747 else if (name.equals("securityLabel")) { 1748 return addSecurityLabel(); 1749 } 1750 else if (name.equals("purpose")) { 1751 return addPurpose(); 1752 } 1753 else if (name.equals("documentType")) { 1754 return addDocumentType(); 1755 } 1756 else if (name.equals("resourceType")) { 1757 return addResourceType(); 1758 } 1759 else if (name.equals("code")) { 1760 return addCode(); 1761 } 1762 else if (name.equals("dataPeriod")) { 1763 this.dataPeriod = new Period(); 1764 return this.dataPeriod; 1765 } 1766 else if (name.equals("data")) { 1767 return addData(); 1768 } 1769 else if (name.equals("expression")) { 1770 this.expression = new Expression(); 1771 return this.expression; 1772 } 1773 else if (name.equals("provision")) { 1774 return addProvision(); 1775 } 1776 else 1777 return super.addChild(name); 1778 } 1779 1780 public ProvisionComponent copy() { 1781 ProvisionComponent dst = new ProvisionComponent(); 1782 copyValues(dst); 1783 return dst; 1784 } 1785 1786 public void copyValues(ProvisionComponent dst) { 1787 super.copyValues(dst); 1788 dst.period = period == null ? null : period.copy(); 1789 if (actor != null) { 1790 dst.actor = new ArrayList<ProvisionActorComponent>(); 1791 for (ProvisionActorComponent i : actor) 1792 dst.actor.add(i.copy()); 1793 }; 1794 if (action != null) { 1795 dst.action = new ArrayList<CodeableConcept>(); 1796 for (CodeableConcept i : action) 1797 dst.action.add(i.copy()); 1798 }; 1799 if (securityLabel != null) { 1800 dst.securityLabel = new ArrayList<Coding>(); 1801 for (Coding i : securityLabel) 1802 dst.securityLabel.add(i.copy()); 1803 }; 1804 if (purpose != null) { 1805 dst.purpose = new ArrayList<Coding>(); 1806 for (Coding i : purpose) 1807 dst.purpose.add(i.copy()); 1808 }; 1809 if (documentType != null) { 1810 dst.documentType = new ArrayList<Coding>(); 1811 for (Coding i : documentType) 1812 dst.documentType.add(i.copy()); 1813 }; 1814 if (resourceType != null) { 1815 dst.resourceType = new ArrayList<Coding>(); 1816 for (Coding i : resourceType) 1817 dst.resourceType.add(i.copy()); 1818 }; 1819 if (code != null) { 1820 dst.code = new ArrayList<CodeableConcept>(); 1821 for (CodeableConcept i : code) 1822 dst.code.add(i.copy()); 1823 }; 1824 dst.dataPeriod = dataPeriod == null ? null : dataPeriod.copy(); 1825 if (data != null) { 1826 dst.data = new ArrayList<ProvisionDataComponent>(); 1827 for (ProvisionDataComponent i : data) 1828 dst.data.add(i.copy()); 1829 }; 1830 dst.expression = expression == null ? null : expression.copy(); 1831 if (provision != null) { 1832 dst.provision = new ArrayList<ProvisionComponent>(); 1833 for (ProvisionComponent i : provision) 1834 dst.provision.add(i.copy()); 1835 }; 1836 } 1837 1838 @Override 1839 public boolean equalsDeep(Base other_) { 1840 if (!super.equalsDeep(other_)) 1841 return false; 1842 if (!(other_ instanceof ProvisionComponent)) 1843 return false; 1844 ProvisionComponent o = (ProvisionComponent) other_; 1845 return compareDeep(period, o.period, true) && compareDeep(actor, o.actor, true) && compareDeep(action, o.action, true) 1846 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(purpose, o.purpose, true) && compareDeep(documentType, o.documentType, true) 1847 && compareDeep(resourceType, o.resourceType, true) && compareDeep(code, o.code, true) && compareDeep(dataPeriod, o.dataPeriod, true) 1848 && compareDeep(data, o.data, true) && compareDeep(expression, o.expression, true) && compareDeep(provision, o.provision, true) 1849 ; 1850 } 1851 1852 @Override 1853 public boolean equalsShallow(Base other_) { 1854 if (!super.equalsShallow(other_)) 1855 return false; 1856 if (!(other_ instanceof ProvisionComponent)) 1857 return false; 1858 ProvisionComponent o = (ProvisionComponent) other_; 1859 return true; 1860 } 1861 1862 public boolean isEmpty() { 1863 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(period, actor, action, securityLabel 1864 , purpose, documentType, resourceType, code, dataPeriod, data, expression, provision 1865 ); 1866 } 1867 1868 public String fhirType() { 1869 return "Consent.provision"; 1870 1871 } 1872 1873 } 1874 1875 @Block() 1876 public static class ProvisionActorComponent extends BackboneElement implements IBaseBackboneElement { 1877 /** 1878 * How the individual is involved in the resources content that is described in the exception. 1879 */ 1880 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1881 @Description(shortDefinition="How the actor is involved", formalDefinition="How the individual is involved in the resources content that is described in the exception." ) 1882 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 1883 protected CodeableConcept role; 1884 1885 /** 1886 * The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 1887 */ 1888 @Child(name = "reference", type = {Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, PractitionerRole.class}, order=2, min=0, max=1, modifier=false, summary=false) 1889 @Description(shortDefinition="Resource for the actor (or group, by role)", formalDefinition="The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 1890 protected Reference reference; 1891 1892 private static final long serialVersionUID = -1992921787L; 1893 1894 /** 1895 * Constructor 1896 */ 1897 public ProvisionActorComponent() { 1898 super(); 1899 } 1900 1901 /** 1902 * @return {@link #role} (How the individual is involved in the resources content that is described in the exception.) 1903 */ 1904 public CodeableConcept getRole() { 1905 if (this.role == null) 1906 if (Configuration.errorOnAutoCreate()) 1907 throw new Error("Attempt to auto-create ProvisionActorComponent.role"); 1908 else if (Configuration.doAutoCreate()) 1909 this.role = new CodeableConcept(); // cc 1910 return this.role; 1911 } 1912 1913 public boolean hasRole() { 1914 return this.role != null && !this.role.isEmpty(); 1915 } 1916 1917 /** 1918 * @param value {@link #role} (How the individual is involved in the resources content that is described in the exception.) 1919 */ 1920 public ProvisionActorComponent setRole(CodeableConcept value) { 1921 this.role = value; 1922 return this; 1923 } 1924 1925 /** 1926 * @return {@link #reference} (The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1927 */ 1928 public Reference getReference() { 1929 if (this.reference == null) 1930 if (Configuration.errorOnAutoCreate()) 1931 throw new Error("Attempt to auto-create ProvisionActorComponent.reference"); 1932 else if (Configuration.doAutoCreate()) 1933 this.reference = new Reference(); // cc 1934 return this.reference; 1935 } 1936 1937 public boolean hasReference() { 1938 return this.reference != null && !this.reference.isEmpty(); 1939 } 1940 1941 /** 1942 * @param value {@link #reference} (The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1943 */ 1944 public ProvisionActorComponent setReference(Reference value) { 1945 this.reference = value; 1946 return this; 1947 } 1948 1949 protected void listChildren(List<Property> children) { 1950 super.listChildren(children); 1951 children.add(new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role)); 1952 children.add(new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference)); 1953 } 1954 1955 @Override 1956 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1957 switch (_hash) { 1958 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role); 1959 case -925155509: /*reference*/ return new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference); 1960 default: return super.getNamedProperty(_hash, _name, _checkValid); 1961 } 1962 1963 } 1964 1965 @Override 1966 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1967 switch (hash) { 1968 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1969 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1970 default: return super.getProperty(hash, name, checkValid); 1971 } 1972 1973 } 1974 1975 @Override 1976 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1977 switch (hash) { 1978 case 3506294: // role 1979 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1980 return value; 1981 case -925155509: // reference 1982 this.reference = TypeConvertor.castToReference(value); // Reference 1983 return value; 1984 default: return super.setProperty(hash, name, value); 1985 } 1986 1987 } 1988 1989 @Override 1990 public Base setProperty(String name, Base value) throws FHIRException { 1991 if (name.equals("role")) { 1992 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1993 } else if (name.equals("reference")) { 1994 this.reference = TypeConvertor.castToReference(value); // Reference 1995 } else 1996 return super.setProperty(name, value); 1997 return value; 1998 } 1999 2000 @Override 2001 public void removeChild(String name, Base value) throws FHIRException { 2002 if (name.equals("role")) { 2003 this.role = null; 2004 } else if (name.equals("reference")) { 2005 this.reference = null; 2006 } else 2007 super.removeChild(name, value); 2008 2009 } 2010 2011 @Override 2012 public Base makeProperty(int hash, String name) throws FHIRException { 2013 switch (hash) { 2014 case 3506294: return getRole(); 2015 case -925155509: return getReference(); 2016 default: return super.makeProperty(hash, name); 2017 } 2018 2019 } 2020 2021 @Override 2022 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2023 switch (hash) { 2024 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2025 case -925155509: /*reference*/ return new String[] {"Reference"}; 2026 default: return super.getTypesForProperty(hash, name); 2027 } 2028 2029 } 2030 2031 @Override 2032 public Base addChild(String name) throws FHIRException { 2033 if (name.equals("role")) { 2034 this.role = new CodeableConcept(); 2035 return this.role; 2036 } 2037 else if (name.equals("reference")) { 2038 this.reference = new Reference(); 2039 return this.reference; 2040 } 2041 else 2042 return super.addChild(name); 2043 } 2044 2045 public ProvisionActorComponent copy() { 2046 ProvisionActorComponent dst = new ProvisionActorComponent(); 2047 copyValues(dst); 2048 return dst; 2049 } 2050 2051 public void copyValues(ProvisionActorComponent dst) { 2052 super.copyValues(dst); 2053 dst.role = role == null ? null : role.copy(); 2054 dst.reference = reference == null ? null : reference.copy(); 2055 } 2056 2057 @Override 2058 public boolean equalsDeep(Base other_) { 2059 if (!super.equalsDeep(other_)) 2060 return false; 2061 if (!(other_ instanceof ProvisionActorComponent)) 2062 return false; 2063 ProvisionActorComponent o = (ProvisionActorComponent) other_; 2064 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 2065 } 2066 2067 @Override 2068 public boolean equalsShallow(Base other_) { 2069 if (!super.equalsShallow(other_)) 2070 return false; 2071 if (!(other_ instanceof ProvisionActorComponent)) 2072 return false; 2073 ProvisionActorComponent o = (ProvisionActorComponent) other_; 2074 return true; 2075 } 2076 2077 public boolean isEmpty() { 2078 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 2079 } 2080 2081 public String fhirType() { 2082 return "Consent.provision.actor"; 2083 2084 } 2085 2086 } 2087 2088 @Block() 2089 public static class ProvisionDataComponent extends BackboneElement implements IBaseBackboneElement { 2090 /** 2091 * How the resource reference is interpreted when testing consent restrictions. 2092 */ 2093 @Child(name = "meaning", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2094 @Description(shortDefinition="instance | related | dependents | authoredby", formalDefinition="How the resource reference is interpreted when testing consent restrictions." ) 2095 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-data-meaning") 2096 protected Enumeration<ConsentDataMeaning> meaning; 2097 2098 /** 2099 * A reference to a specific resource that defines which resources are covered by this consent. 2100 */ 2101 @Child(name = "reference", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 2102 @Description(shortDefinition="The actual data reference", formalDefinition="A reference to a specific resource that defines which resources are covered by this consent." ) 2103 protected Reference reference; 2104 2105 private static final long serialVersionUID = 1735979153L; 2106 2107 /** 2108 * Constructor 2109 */ 2110 public ProvisionDataComponent() { 2111 super(); 2112 } 2113 2114 /** 2115 * Constructor 2116 */ 2117 public ProvisionDataComponent(ConsentDataMeaning meaning, Reference reference) { 2118 super(); 2119 this.setMeaning(meaning); 2120 this.setReference(reference); 2121 } 2122 2123 /** 2124 * @return {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2125 */ 2126 public Enumeration<ConsentDataMeaning> getMeaningElement() { 2127 if (this.meaning == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create ProvisionDataComponent.meaning"); 2130 else if (Configuration.doAutoCreate()) 2131 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 2132 return this.meaning; 2133 } 2134 2135 public boolean hasMeaningElement() { 2136 return this.meaning != null && !this.meaning.isEmpty(); 2137 } 2138 2139 public boolean hasMeaning() { 2140 return this.meaning != null && !this.meaning.isEmpty(); 2141 } 2142 2143 /** 2144 * @param value {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2145 */ 2146 public ProvisionDataComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 2147 this.meaning = value; 2148 return this; 2149 } 2150 2151 /** 2152 * @return How the resource reference is interpreted when testing consent restrictions. 2153 */ 2154 public ConsentDataMeaning getMeaning() { 2155 return this.meaning == null ? null : this.meaning.getValue(); 2156 } 2157 2158 /** 2159 * @param value How the resource reference is interpreted when testing consent restrictions. 2160 */ 2161 public ProvisionDataComponent setMeaning(ConsentDataMeaning value) { 2162 if (this.meaning == null) 2163 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 2164 this.meaning.setValue(value); 2165 return this; 2166 } 2167 2168 /** 2169 * @return {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2170 */ 2171 public Reference getReference() { 2172 if (this.reference == null) 2173 if (Configuration.errorOnAutoCreate()) 2174 throw new Error("Attempt to auto-create ProvisionDataComponent.reference"); 2175 else if (Configuration.doAutoCreate()) 2176 this.reference = new Reference(); // cc 2177 return this.reference; 2178 } 2179 2180 public boolean hasReference() { 2181 return this.reference != null && !this.reference.isEmpty(); 2182 } 2183 2184 /** 2185 * @param value {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2186 */ 2187 public ProvisionDataComponent setReference(Reference value) { 2188 this.reference = value; 2189 return this; 2190 } 2191 2192 protected void listChildren(List<Property> children) { 2193 super.listChildren(children); 2194 children.add(new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 2195 children.add(new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference)); 2196 } 2197 2198 @Override 2199 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2200 switch (_hash) { 2201 case 938160637: /*meaning*/ return new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 2202 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference); 2203 default: return super.getNamedProperty(_hash, _name, _checkValid); 2204 } 2205 2206 } 2207 2208 @Override 2209 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2210 switch (hash) { 2211 case 938160637: /*meaning*/ return this.meaning == null ? new Base[0] : new Base[] {this.meaning}; // Enumeration<ConsentDataMeaning> 2212 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2213 default: return super.getProperty(hash, name, checkValid); 2214 } 2215 2216 } 2217 2218 @Override 2219 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2220 switch (hash) { 2221 case 938160637: // meaning 2222 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2223 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2224 return value; 2225 case -925155509: // reference 2226 this.reference = TypeConvertor.castToReference(value); // Reference 2227 return value; 2228 default: return super.setProperty(hash, name, value); 2229 } 2230 2231 } 2232 2233 @Override 2234 public Base setProperty(String name, Base value) throws FHIRException { 2235 if (name.equals("meaning")) { 2236 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2237 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2238 } else if (name.equals("reference")) { 2239 this.reference = TypeConvertor.castToReference(value); // Reference 2240 } else 2241 return super.setProperty(name, value); 2242 return value; 2243 } 2244 2245 @Override 2246 public void removeChild(String name, Base value) throws FHIRException { 2247 if (name.equals("meaning")) { 2248 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2249 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2250 } else if (name.equals("reference")) { 2251 this.reference = null; 2252 } else 2253 super.removeChild(name, value); 2254 2255 } 2256 2257 @Override 2258 public Base makeProperty(int hash, String name) throws FHIRException { 2259 switch (hash) { 2260 case 938160637: return getMeaningElement(); 2261 case -925155509: return getReference(); 2262 default: return super.makeProperty(hash, name); 2263 } 2264 2265 } 2266 2267 @Override 2268 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2269 switch (hash) { 2270 case 938160637: /*meaning*/ return new String[] {"code"}; 2271 case -925155509: /*reference*/ return new String[] {"Reference"}; 2272 default: return super.getTypesForProperty(hash, name); 2273 } 2274 2275 } 2276 2277 @Override 2278 public Base addChild(String name) throws FHIRException { 2279 if (name.equals("meaning")) { 2280 throw new FHIRException("Cannot call addChild on a singleton property Consent.provision.data.meaning"); 2281 } 2282 else if (name.equals("reference")) { 2283 this.reference = new Reference(); 2284 return this.reference; 2285 } 2286 else 2287 return super.addChild(name); 2288 } 2289 2290 public ProvisionDataComponent copy() { 2291 ProvisionDataComponent dst = new ProvisionDataComponent(); 2292 copyValues(dst); 2293 return dst; 2294 } 2295 2296 public void copyValues(ProvisionDataComponent dst) { 2297 super.copyValues(dst); 2298 dst.meaning = meaning == null ? null : meaning.copy(); 2299 dst.reference = reference == null ? null : reference.copy(); 2300 } 2301 2302 @Override 2303 public boolean equalsDeep(Base other_) { 2304 if (!super.equalsDeep(other_)) 2305 return false; 2306 if (!(other_ instanceof ProvisionDataComponent)) 2307 return false; 2308 ProvisionDataComponent o = (ProvisionDataComponent) other_; 2309 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 2310 } 2311 2312 @Override 2313 public boolean equalsShallow(Base other_) { 2314 if (!super.equalsShallow(other_)) 2315 return false; 2316 if (!(other_ instanceof ProvisionDataComponent)) 2317 return false; 2318 ProvisionDataComponent o = (ProvisionDataComponent) other_; 2319 return compareValues(meaning, o.meaning, true); 2320 } 2321 2322 public boolean isEmpty() { 2323 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 2324 } 2325 2326 public String fhirType() { 2327 return "Consent.provision.data"; 2328 2329 } 2330 2331 } 2332 2333 /** 2334 * Unique identifier for this copy of the Consent Statement. 2335 */ 2336 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2337 @Description(shortDefinition="Identifier for this record (external references)", formalDefinition="Unique identifier for this copy of the Consent Statement." ) 2338 protected List<Identifier> identifier; 2339 2340 /** 2341 * Indicates the current state of this Consent resource. 2342 */ 2343 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2344 @Description(shortDefinition="draft | active | inactive | not-done | entered-in-error | unknown", formalDefinition="Indicates the current state of this Consent resource." ) 2345 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-state-codes") 2346 protected Enumeration<ConsentState> status; 2347 2348 /** 2349 * A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements. 2350 */ 2351 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2352 @Description(shortDefinition="Classification of the consent statement - for indexing/retrieval", formalDefinition="A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements." ) 2353 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-category") 2354 protected List<CodeableConcept> category; 2355 2356 /** 2357 * The patient/healthcare practitioner or group of persons to whom this consent applies. 2358 */ 2359 @Child(name = "subject", type = {Patient.class, Practitioner.class, Group.class}, order=3, min=0, max=1, modifier=false, summary=true) 2360 @Description(shortDefinition="Who the consent applies to", formalDefinition="The patient/healthcare practitioner or group of persons to whom this consent applies." ) 2361 protected Reference subject; 2362 2363 /** 2364 * Date the consent instance was agreed to. 2365 */ 2366 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2367 @Description(shortDefinition="Fully executed date of the consent", formalDefinition="Date the consent instance was agreed to." ) 2368 protected DateType date; 2369 2370 /** 2371 * Effective period for this Consent Resource and all provisions unless specified in that provision. 2372 */ 2373 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 2374 @Description(shortDefinition="Effective period for this Consent", formalDefinition="Effective period for this Consent Resource and all provisions unless specified in that provision." ) 2375 protected Period period; 2376 2377 /** 2378 * The entity responsible for granting the rights listed in a Consent Directive. 2379 */ 2380 @Child(name = "grantor", type = {CareTeam.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, PractitionerRole.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2381 @Description(shortDefinition="Who is granting rights according to the policy and rules", formalDefinition="The entity responsible for granting the rights listed in a Consent Directive." ) 2382 protected List<Reference> grantor; 2383 2384 /** 2385 * The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions. 2386 */ 2387 @Child(name = "grantee", type = {CareTeam.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, PractitionerRole.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2388 @Description(shortDefinition="Who is agreeing to the policy and rules", formalDefinition="The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions." ) 2389 protected List<Reference> grantee; 2390 2391 /** 2392 * The actor that manages the consent through its lifecycle. 2393 */ 2394 @Child(name = "manager", type = {HealthcareService.class, Organization.class, Patient.class, Practitioner.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2395 @Description(shortDefinition="Consent workflow management", formalDefinition="The actor that manages the consent through its lifecycle." ) 2396 protected List<Reference> manager; 2397 2398 /** 2399 * The actor that controls/enforces the access according to the consent. 2400 */ 2401 @Child(name = "controller", type = {HealthcareService.class, Organization.class, Patient.class, Practitioner.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2402 @Description(shortDefinition="Consent Enforcer", formalDefinition="The actor that controls/enforces the access according to the consent." ) 2403 protected List<Reference> controller; 2404 2405 /** 2406 * The source on which this consent statement is based. The source might be a scanned original paper form. 2407 */ 2408 @Child(name = "sourceAttachment", type = {Attachment.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2409 @Description(shortDefinition="Source from which this consent is taken", formalDefinition="The source on which this consent statement is based. The source might be a scanned original paper form." ) 2410 protected List<Attachment> sourceAttachment; 2411 2412 /** 2413 * A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document. 2414 */ 2415 @Child(name = "sourceReference", type = {Consent.class, DocumentReference.class, Contract.class, QuestionnaireResponse.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2416 @Description(shortDefinition="Source from which this consent is taken", formalDefinition="A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document." ) 2417 protected List<Reference> sourceReference; 2418 2419 /** 2420 * A set of codes that indicate the regulatory basis (if any) that this consent supports. 2421 */ 2422 @Child(name = "regulatoryBasis", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2423 @Description(shortDefinition="Regulations establishing base Consent", formalDefinition="A set of codes that indicate the regulatory basis (if any) that this consent supports." ) 2424 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-policy") 2425 protected List<CodeableConcept> regulatoryBasis; 2426 2427 /** 2428 * A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form. 2429 */ 2430 @Child(name = "policyBasis", type = {}, order=13, min=0, max=1, modifier=false, summary=false) 2431 @Description(shortDefinition="Computable version of the backing policy", formalDefinition="A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form." ) 2432 protected ConsentPolicyBasisComponent policyBasis; 2433 2434 /** 2435 * A Reference to the human readable policy explaining the basis for the Consent. 2436 */ 2437 @Child(name = "policyText", type = {DocumentReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2438 @Description(shortDefinition="Human Readable Policy", formalDefinition="A Reference to the human readable policy explaining the basis for the Consent." ) 2439 protected List<Reference> policyText; 2440 2441 /** 2442 * Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person. 2443 */ 2444 @Child(name = "verification", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2445 @Description(shortDefinition="Consent Verified by patient or family", formalDefinition="Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person." ) 2446 protected List<ConsentVerificationComponent> verification; 2447 2448 /** 2449 * Action to take - permit or deny - as default. 2450 */ 2451 @Child(name = "decision", type = {CodeType.class}, order=16, min=0, max=1, modifier=true, summary=true) 2452 @Description(shortDefinition="deny | permit", formalDefinition="Action to take - permit or deny - as default." ) 2453 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-provision-type") 2454 protected Enumeration<ConsentProvisionType> decision; 2455 2456 /** 2457 * An exception to the base policy of this consent. An exception can be an addition or removal of access permissions. 2458 */ 2459 @Child(name = "provision", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2460 @Description(shortDefinition="Constraints to the base Consent.policyRule/Consent.policy", formalDefinition="An exception to the base policy of this consent. An exception can be an addition or removal of access permissions." ) 2461 protected List<ProvisionComponent> provision; 2462 2463 private static final long serialVersionUID = -1133978742L; 2464 2465 /** 2466 * Constructor 2467 */ 2468 public Consent() { 2469 super(); 2470 } 2471 2472 /** 2473 * Constructor 2474 */ 2475 public Consent(ConsentState status) { 2476 super(); 2477 this.setStatus(status); 2478 } 2479 2480 /** 2481 * @return {@link #identifier} (Unique identifier for this copy of the Consent Statement.) 2482 */ 2483 public List<Identifier> getIdentifier() { 2484 if (this.identifier == null) 2485 this.identifier = new ArrayList<Identifier>(); 2486 return this.identifier; 2487 } 2488 2489 /** 2490 * @return Returns a reference to <code>this</code> for easy method chaining 2491 */ 2492 public Consent setIdentifier(List<Identifier> theIdentifier) { 2493 this.identifier = theIdentifier; 2494 return this; 2495 } 2496 2497 public boolean hasIdentifier() { 2498 if (this.identifier == null) 2499 return false; 2500 for (Identifier item : this.identifier) 2501 if (!item.isEmpty()) 2502 return true; 2503 return false; 2504 } 2505 2506 public Identifier addIdentifier() { //3 2507 Identifier t = new Identifier(); 2508 if (this.identifier == null) 2509 this.identifier = new ArrayList<Identifier>(); 2510 this.identifier.add(t); 2511 return t; 2512 } 2513 2514 public Consent addIdentifier(Identifier t) { //3 2515 if (t == null) 2516 return this; 2517 if (this.identifier == null) 2518 this.identifier = new ArrayList<Identifier>(); 2519 this.identifier.add(t); 2520 return this; 2521 } 2522 2523 /** 2524 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2525 */ 2526 public Identifier getIdentifierFirstRep() { 2527 if (getIdentifier().isEmpty()) { 2528 addIdentifier(); 2529 } 2530 return getIdentifier().get(0); 2531 } 2532 2533 /** 2534 * @return {@link #status} (Indicates the current state of this Consent resource.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2535 */ 2536 public Enumeration<ConsentState> getStatusElement() { 2537 if (this.status == null) 2538 if (Configuration.errorOnAutoCreate()) 2539 throw new Error("Attempt to auto-create Consent.status"); 2540 else if (Configuration.doAutoCreate()) 2541 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); // bb 2542 return this.status; 2543 } 2544 2545 public boolean hasStatusElement() { 2546 return this.status != null && !this.status.isEmpty(); 2547 } 2548 2549 public boolean hasStatus() { 2550 return this.status != null && !this.status.isEmpty(); 2551 } 2552 2553 /** 2554 * @param value {@link #status} (Indicates the current state of this Consent resource.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2555 */ 2556 public Consent setStatusElement(Enumeration<ConsentState> value) { 2557 this.status = value; 2558 return this; 2559 } 2560 2561 /** 2562 * @return Indicates the current state of this Consent resource. 2563 */ 2564 public ConsentState getStatus() { 2565 return this.status == null ? null : this.status.getValue(); 2566 } 2567 2568 /** 2569 * @param value Indicates the current state of this Consent resource. 2570 */ 2571 public Consent setStatus(ConsentState value) { 2572 if (this.status == null) 2573 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); 2574 this.status.setValue(value); 2575 return this; 2576 } 2577 2578 /** 2579 * @return {@link #category} (A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.) 2580 */ 2581 public List<CodeableConcept> getCategory() { 2582 if (this.category == null) 2583 this.category = new ArrayList<CodeableConcept>(); 2584 return this.category; 2585 } 2586 2587 /** 2588 * @return Returns a reference to <code>this</code> for easy method chaining 2589 */ 2590 public Consent setCategory(List<CodeableConcept> theCategory) { 2591 this.category = theCategory; 2592 return this; 2593 } 2594 2595 public boolean hasCategory() { 2596 if (this.category == null) 2597 return false; 2598 for (CodeableConcept item : this.category) 2599 if (!item.isEmpty()) 2600 return true; 2601 return false; 2602 } 2603 2604 public CodeableConcept addCategory() { //3 2605 CodeableConcept t = new CodeableConcept(); 2606 if (this.category == null) 2607 this.category = new ArrayList<CodeableConcept>(); 2608 this.category.add(t); 2609 return t; 2610 } 2611 2612 public Consent addCategory(CodeableConcept t) { //3 2613 if (t == null) 2614 return this; 2615 if (this.category == null) 2616 this.category = new ArrayList<CodeableConcept>(); 2617 this.category.add(t); 2618 return this; 2619 } 2620 2621 /** 2622 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2623 */ 2624 public CodeableConcept getCategoryFirstRep() { 2625 if (getCategory().isEmpty()) { 2626 addCategory(); 2627 } 2628 return getCategory().get(0); 2629 } 2630 2631 /** 2632 * @return {@link #subject} (The patient/healthcare practitioner or group of persons to whom this consent applies.) 2633 */ 2634 public Reference getSubject() { 2635 if (this.subject == null) 2636 if (Configuration.errorOnAutoCreate()) 2637 throw new Error("Attempt to auto-create Consent.subject"); 2638 else if (Configuration.doAutoCreate()) 2639 this.subject = new Reference(); // cc 2640 return this.subject; 2641 } 2642 2643 public boolean hasSubject() { 2644 return this.subject != null && !this.subject.isEmpty(); 2645 } 2646 2647 /** 2648 * @param value {@link #subject} (The patient/healthcare practitioner or group of persons to whom this consent applies.) 2649 */ 2650 public Consent setSubject(Reference value) { 2651 this.subject = value; 2652 return this; 2653 } 2654 2655 /** 2656 * @return {@link #date} (Date the consent instance was agreed to.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2657 */ 2658 public DateType getDateElement() { 2659 if (this.date == null) 2660 if (Configuration.errorOnAutoCreate()) 2661 throw new Error("Attempt to auto-create Consent.date"); 2662 else if (Configuration.doAutoCreate()) 2663 this.date = new DateType(); // bb 2664 return this.date; 2665 } 2666 2667 public boolean hasDateElement() { 2668 return this.date != null && !this.date.isEmpty(); 2669 } 2670 2671 public boolean hasDate() { 2672 return this.date != null && !this.date.isEmpty(); 2673 } 2674 2675 /** 2676 * @param value {@link #date} (Date the consent instance was agreed to.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2677 */ 2678 public Consent setDateElement(DateType value) { 2679 this.date = value; 2680 return this; 2681 } 2682 2683 /** 2684 * @return Date the consent instance was agreed to. 2685 */ 2686 public Date getDate() { 2687 return this.date == null ? null : this.date.getValue(); 2688 } 2689 2690 /** 2691 * @param value Date the consent instance was agreed to. 2692 */ 2693 public Consent setDate(Date value) { 2694 if (value == null) 2695 this.date = null; 2696 else { 2697 if (this.date == null) 2698 this.date = new DateType(); 2699 this.date.setValue(value); 2700 } 2701 return this; 2702 } 2703 2704 /** 2705 * @return {@link #period} (Effective period for this Consent Resource and all provisions unless specified in that provision.) 2706 */ 2707 public Period getPeriod() { 2708 if (this.period == null) 2709 if (Configuration.errorOnAutoCreate()) 2710 throw new Error("Attempt to auto-create Consent.period"); 2711 else if (Configuration.doAutoCreate()) 2712 this.period = new Period(); // cc 2713 return this.period; 2714 } 2715 2716 public boolean hasPeriod() { 2717 return this.period != null && !this.period.isEmpty(); 2718 } 2719 2720 /** 2721 * @param value {@link #period} (Effective period for this Consent Resource and all provisions unless specified in that provision.) 2722 */ 2723 public Consent setPeriod(Period value) { 2724 this.period = value; 2725 return this; 2726 } 2727 2728 /** 2729 * @return {@link #grantor} (The entity responsible for granting the rights listed in a Consent Directive.) 2730 */ 2731 public List<Reference> getGrantor() { 2732 if (this.grantor == null) 2733 this.grantor = new ArrayList<Reference>(); 2734 return this.grantor; 2735 } 2736 2737 /** 2738 * @return Returns a reference to <code>this</code> for easy method chaining 2739 */ 2740 public Consent setGrantor(List<Reference> theGrantor) { 2741 this.grantor = theGrantor; 2742 return this; 2743 } 2744 2745 public boolean hasGrantor() { 2746 if (this.grantor == null) 2747 return false; 2748 for (Reference item : this.grantor) 2749 if (!item.isEmpty()) 2750 return true; 2751 return false; 2752 } 2753 2754 public Reference addGrantor() { //3 2755 Reference t = new Reference(); 2756 if (this.grantor == null) 2757 this.grantor = new ArrayList<Reference>(); 2758 this.grantor.add(t); 2759 return t; 2760 } 2761 2762 public Consent addGrantor(Reference t) { //3 2763 if (t == null) 2764 return this; 2765 if (this.grantor == null) 2766 this.grantor = new ArrayList<Reference>(); 2767 this.grantor.add(t); 2768 return this; 2769 } 2770 2771 /** 2772 * @return The first repetition of repeating field {@link #grantor}, creating it if it does not already exist {3} 2773 */ 2774 public Reference getGrantorFirstRep() { 2775 if (getGrantor().isEmpty()) { 2776 addGrantor(); 2777 } 2778 return getGrantor().get(0); 2779 } 2780 2781 /** 2782 * @return {@link #grantee} (The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.) 2783 */ 2784 public List<Reference> getGrantee() { 2785 if (this.grantee == null) 2786 this.grantee = new ArrayList<Reference>(); 2787 return this.grantee; 2788 } 2789 2790 /** 2791 * @return Returns a reference to <code>this</code> for easy method chaining 2792 */ 2793 public Consent setGrantee(List<Reference> theGrantee) { 2794 this.grantee = theGrantee; 2795 return this; 2796 } 2797 2798 public boolean hasGrantee() { 2799 if (this.grantee == null) 2800 return false; 2801 for (Reference item : this.grantee) 2802 if (!item.isEmpty()) 2803 return true; 2804 return false; 2805 } 2806 2807 public Reference addGrantee() { //3 2808 Reference t = new Reference(); 2809 if (this.grantee == null) 2810 this.grantee = new ArrayList<Reference>(); 2811 this.grantee.add(t); 2812 return t; 2813 } 2814 2815 public Consent addGrantee(Reference t) { //3 2816 if (t == null) 2817 return this; 2818 if (this.grantee == null) 2819 this.grantee = new ArrayList<Reference>(); 2820 this.grantee.add(t); 2821 return this; 2822 } 2823 2824 /** 2825 * @return The first repetition of repeating field {@link #grantee}, creating it if it does not already exist {3} 2826 */ 2827 public Reference getGranteeFirstRep() { 2828 if (getGrantee().isEmpty()) { 2829 addGrantee(); 2830 } 2831 return getGrantee().get(0); 2832 } 2833 2834 /** 2835 * @return {@link #manager} (The actor that manages the consent through its lifecycle.) 2836 */ 2837 public List<Reference> getManager() { 2838 if (this.manager == null) 2839 this.manager = new ArrayList<Reference>(); 2840 return this.manager; 2841 } 2842 2843 /** 2844 * @return Returns a reference to <code>this</code> for easy method chaining 2845 */ 2846 public Consent setManager(List<Reference> theManager) { 2847 this.manager = theManager; 2848 return this; 2849 } 2850 2851 public boolean hasManager() { 2852 if (this.manager == null) 2853 return false; 2854 for (Reference item : this.manager) 2855 if (!item.isEmpty()) 2856 return true; 2857 return false; 2858 } 2859 2860 public Reference addManager() { //3 2861 Reference t = new Reference(); 2862 if (this.manager == null) 2863 this.manager = new ArrayList<Reference>(); 2864 this.manager.add(t); 2865 return t; 2866 } 2867 2868 public Consent addManager(Reference t) { //3 2869 if (t == null) 2870 return this; 2871 if (this.manager == null) 2872 this.manager = new ArrayList<Reference>(); 2873 this.manager.add(t); 2874 return this; 2875 } 2876 2877 /** 2878 * @return The first repetition of repeating field {@link #manager}, creating it if it does not already exist {3} 2879 */ 2880 public Reference getManagerFirstRep() { 2881 if (getManager().isEmpty()) { 2882 addManager(); 2883 } 2884 return getManager().get(0); 2885 } 2886 2887 /** 2888 * @return {@link #controller} (The actor that controls/enforces the access according to the consent.) 2889 */ 2890 public List<Reference> getController() { 2891 if (this.controller == null) 2892 this.controller = new ArrayList<Reference>(); 2893 return this.controller; 2894 } 2895 2896 /** 2897 * @return Returns a reference to <code>this</code> for easy method chaining 2898 */ 2899 public Consent setController(List<Reference> theController) { 2900 this.controller = theController; 2901 return this; 2902 } 2903 2904 public boolean hasController() { 2905 if (this.controller == null) 2906 return false; 2907 for (Reference item : this.controller) 2908 if (!item.isEmpty()) 2909 return true; 2910 return false; 2911 } 2912 2913 public Reference addController() { //3 2914 Reference t = new Reference(); 2915 if (this.controller == null) 2916 this.controller = new ArrayList<Reference>(); 2917 this.controller.add(t); 2918 return t; 2919 } 2920 2921 public Consent addController(Reference t) { //3 2922 if (t == null) 2923 return this; 2924 if (this.controller == null) 2925 this.controller = new ArrayList<Reference>(); 2926 this.controller.add(t); 2927 return this; 2928 } 2929 2930 /** 2931 * @return The first repetition of repeating field {@link #controller}, creating it if it does not already exist {3} 2932 */ 2933 public Reference getControllerFirstRep() { 2934 if (getController().isEmpty()) { 2935 addController(); 2936 } 2937 return getController().get(0); 2938 } 2939 2940 /** 2941 * @return {@link #sourceAttachment} (The source on which this consent statement is based. The source might be a scanned original paper form.) 2942 */ 2943 public List<Attachment> getSourceAttachment() { 2944 if (this.sourceAttachment == null) 2945 this.sourceAttachment = new ArrayList<Attachment>(); 2946 return this.sourceAttachment; 2947 } 2948 2949 /** 2950 * @return Returns a reference to <code>this</code> for easy method chaining 2951 */ 2952 public Consent setSourceAttachment(List<Attachment> theSourceAttachment) { 2953 this.sourceAttachment = theSourceAttachment; 2954 return this; 2955 } 2956 2957 public boolean hasSourceAttachment() { 2958 if (this.sourceAttachment == null) 2959 return false; 2960 for (Attachment item : this.sourceAttachment) 2961 if (!item.isEmpty()) 2962 return true; 2963 return false; 2964 } 2965 2966 public Attachment addSourceAttachment() { //3 2967 Attachment t = new Attachment(); 2968 if (this.sourceAttachment == null) 2969 this.sourceAttachment = new ArrayList<Attachment>(); 2970 this.sourceAttachment.add(t); 2971 return t; 2972 } 2973 2974 public Consent addSourceAttachment(Attachment t) { //3 2975 if (t == null) 2976 return this; 2977 if (this.sourceAttachment == null) 2978 this.sourceAttachment = new ArrayList<Attachment>(); 2979 this.sourceAttachment.add(t); 2980 return this; 2981 } 2982 2983 /** 2984 * @return The first repetition of repeating field {@link #sourceAttachment}, creating it if it does not already exist {3} 2985 */ 2986 public Attachment getSourceAttachmentFirstRep() { 2987 if (getSourceAttachment().isEmpty()) { 2988 addSourceAttachment(); 2989 } 2990 return getSourceAttachment().get(0); 2991 } 2992 2993 /** 2994 * @return {@link #sourceReference} (A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 2995 */ 2996 public List<Reference> getSourceReference() { 2997 if (this.sourceReference == null) 2998 this.sourceReference = new ArrayList<Reference>(); 2999 return this.sourceReference; 3000 } 3001 3002 /** 3003 * @return Returns a reference to <code>this</code> for easy method chaining 3004 */ 3005 public Consent setSourceReference(List<Reference> theSourceReference) { 3006 this.sourceReference = theSourceReference; 3007 return this; 3008 } 3009 3010 public boolean hasSourceReference() { 3011 if (this.sourceReference == null) 3012 return false; 3013 for (Reference item : this.sourceReference) 3014 if (!item.isEmpty()) 3015 return true; 3016 return false; 3017 } 3018 3019 public Reference addSourceReference() { //3 3020 Reference t = new Reference(); 3021 if (this.sourceReference == null) 3022 this.sourceReference = new ArrayList<Reference>(); 3023 this.sourceReference.add(t); 3024 return t; 3025 } 3026 3027 public Consent addSourceReference(Reference t) { //3 3028 if (t == null) 3029 return this; 3030 if (this.sourceReference == null) 3031 this.sourceReference = new ArrayList<Reference>(); 3032 this.sourceReference.add(t); 3033 return this; 3034 } 3035 3036 /** 3037 * @return The first repetition of repeating field {@link #sourceReference}, creating it if it does not already exist {3} 3038 */ 3039 public Reference getSourceReferenceFirstRep() { 3040 if (getSourceReference().isEmpty()) { 3041 addSourceReference(); 3042 } 3043 return getSourceReference().get(0); 3044 } 3045 3046 /** 3047 * @return {@link #regulatoryBasis} (A set of codes that indicate the regulatory basis (if any) that this consent supports.) 3048 */ 3049 public List<CodeableConcept> getRegulatoryBasis() { 3050 if (this.regulatoryBasis == null) 3051 this.regulatoryBasis = new ArrayList<CodeableConcept>(); 3052 return this.regulatoryBasis; 3053 } 3054 3055 /** 3056 * @return Returns a reference to <code>this</code> for easy method chaining 3057 */ 3058 public Consent setRegulatoryBasis(List<CodeableConcept> theRegulatoryBasis) { 3059 this.regulatoryBasis = theRegulatoryBasis; 3060 return this; 3061 } 3062 3063 public boolean hasRegulatoryBasis() { 3064 if (this.regulatoryBasis == null) 3065 return false; 3066 for (CodeableConcept item : this.regulatoryBasis) 3067 if (!item.isEmpty()) 3068 return true; 3069 return false; 3070 } 3071 3072 public CodeableConcept addRegulatoryBasis() { //3 3073 CodeableConcept t = new CodeableConcept(); 3074 if (this.regulatoryBasis == null) 3075 this.regulatoryBasis = new ArrayList<CodeableConcept>(); 3076 this.regulatoryBasis.add(t); 3077 return t; 3078 } 3079 3080 public Consent addRegulatoryBasis(CodeableConcept t) { //3 3081 if (t == null) 3082 return this; 3083 if (this.regulatoryBasis == null) 3084 this.regulatoryBasis = new ArrayList<CodeableConcept>(); 3085 this.regulatoryBasis.add(t); 3086 return this; 3087 } 3088 3089 /** 3090 * @return The first repetition of repeating field {@link #regulatoryBasis}, creating it if it does not already exist {3} 3091 */ 3092 public CodeableConcept getRegulatoryBasisFirstRep() { 3093 if (getRegulatoryBasis().isEmpty()) { 3094 addRegulatoryBasis(); 3095 } 3096 return getRegulatoryBasis().get(0); 3097 } 3098 3099 /** 3100 * @return {@link #policyBasis} (A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.) 3101 */ 3102 public ConsentPolicyBasisComponent getPolicyBasis() { 3103 if (this.policyBasis == null) 3104 if (Configuration.errorOnAutoCreate()) 3105 throw new Error("Attempt to auto-create Consent.policyBasis"); 3106 else if (Configuration.doAutoCreate()) 3107 this.policyBasis = new ConsentPolicyBasisComponent(); // cc 3108 return this.policyBasis; 3109 } 3110 3111 public boolean hasPolicyBasis() { 3112 return this.policyBasis != null && !this.policyBasis.isEmpty(); 3113 } 3114 3115 /** 3116 * @param value {@link #policyBasis} (A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.) 3117 */ 3118 public Consent setPolicyBasis(ConsentPolicyBasisComponent value) { 3119 this.policyBasis = value; 3120 return this; 3121 } 3122 3123 /** 3124 * @return {@link #policyText} (A Reference to the human readable policy explaining the basis for the Consent.) 3125 */ 3126 public List<Reference> getPolicyText() { 3127 if (this.policyText == null) 3128 this.policyText = new ArrayList<Reference>(); 3129 return this.policyText; 3130 } 3131 3132 /** 3133 * @return Returns a reference to <code>this</code> for easy method chaining 3134 */ 3135 public Consent setPolicyText(List<Reference> thePolicyText) { 3136 this.policyText = thePolicyText; 3137 return this; 3138 } 3139 3140 public boolean hasPolicyText() { 3141 if (this.policyText == null) 3142 return false; 3143 for (Reference item : this.policyText) 3144 if (!item.isEmpty()) 3145 return true; 3146 return false; 3147 } 3148 3149 public Reference addPolicyText() { //3 3150 Reference t = new Reference(); 3151 if (this.policyText == null) 3152 this.policyText = new ArrayList<Reference>(); 3153 this.policyText.add(t); 3154 return t; 3155 } 3156 3157 public Consent addPolicyText(Reference t) { //3 3158 if (t == null) 3159 return this; 3160 if (this.policyText == null) 3161 this.policyText = new ArrayList<Reference>(); 3162 this.policyText.add(t); 3163 return this; 3164 } 3165 3166 /** 3167 * @return The first repetition of repeating field {@link #policyText}, creating it if it does not already exist {3} 3168 */ 3169 public Reference getPolicyTextFirstRep() { 3170 if (getPolicyText().isEmpty()) { 3171 addPolicyText(); 3172 } 3173 return getPolicyText().get(0); 3174 } 3175 3176 /** 3177 * @return {@link #verification} (Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person.) 3178 */ 3179 public List<ConsentVerificationComponent> getVerification() { 3180 if (this.verification == null) 3181 this.verification = new ArrayList<ConsentVerificationComponent>(); 3182 return this.verification; 3183 } 3184 3185 /** 3186 * @return Returns a reference to <code>this</code> for easy method chaining 3187 */ 3188 public Consent setVerification(List<ConsentVerificationComponent> theVerification) { 3189 this.verification = theVerification; 3190 return this; 3191 } 3192 3193 public boolean hasVerification() { 3194 if (this.verification == null) 3195 return false; 3196 for (ConsentVerificationComponent item : this.verification) 3197 if (!item.isEmpty()) 3198 return true; 3199 return false; 3200 } 3201 3202 public ConsentVerificationComponent addVerification() { //3 3203 ConsentVerificationComponent t = new ConsentVerificationComponent(); 3204 if (this.verification == null) 3205 this.verification = new ArrayList<ConsentVerificationComponent>(); 3206 this.verification.add(t); 3207 return t; 3208 } 3209 3210 public Consent addVerification(ConsentVerificationComponent t) { //3 3211 if (t == null) 3212 return this; 3213 if (this.verification == null) 3214 this.verification = new ArrayList<ConsentVerificationComponent>(); 3215 this.verification.add(t); 3216 return this; 3217 } 3218 3219 /** 3220 * @return The first repetition of repeating field {@link #verification}, creating it if it does not already exist {3} 3221 */ 3222 public ConsentVerificationComponent getVerificationFirstRep() { 3223 if (getVerification().isEmpty()) { 3224 addVerification(); 3225 } 3226 return getVerification().get(0); 3227 } 3228 3229 /** 3230 * @return {@link #decision} (Action to take - permit or deny - as default.). This is the underlying object with id, value and extensions. The accessor "getDecision" gives direct access to the value 3231 */ 3232 public Enumeration<ConsentProvisionType> getDecisionElement() { 3233 if (this.decision == null) 3234 if (Configuration.errorOnAutoCreate()) 3235 throw new Error("Attempt to auto-create Consent.decision"); 3236 else if (Configuration.doAutoCreate()) 3237 this.decision = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); // bb 3238 return this.decision; 3239 } 3240 3241 public boolean hasDecisionElement() { 3242 return this.decision != null && !this.decision.isEmpty(); 3243 } 3244 3245 public boolean hasDecision() { 3246 return this.decision != null && !this.decision.isEmpty(); 3247 } 3248 3249 /** 3250 * @param value {@link #decision} (Action to take - permit or deny - as default.). This is the underlying object with id, value and extensions. The accessor "getDecision" gives direct access to the value 3251 */ 3252 public Consent setDecisionElement(Enumeration<ConsentProvisionType> value) { 3253 this.decision = value; 3254 return this; 3255 } 3256 3257 /** 3258 * @return Action to take - permit or deny - as default. 3259 */ 3260 public ConsentProvisionType getDecision() { 3261 return this.decision == null ? null : this.decision.getValue(); 3262 } 3263 3264 /** 3265 * @param value Action to take - permit or deny - as default. 3266 */ 3267 public Consent setDecision(ConsentProvisionType value) { 3268 if (value == null) 3269 this.decision = null; 3270 else { 3271 if (this.decision == null) 3272 this.decision = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); 3273 this.decision.setValue(value); 3274 } 3275 return this; 3276 } 3277 3278 /** 3279 * @return {@link #provision} (An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.) 3280 */ 3281 public List<ProvisionComponent> getProvision() { 3282 if (this.provision == null) 3283 this.provision = new ArrayList<ProvisionComponent>(); 3284 return this.provision; 3285 } 3286 3287 /** 3288 * @return Returns a reference to <code>this</code> for easy method chaining 3289 */ 3290 public Consent setProvision(List<ProvisionComponent> theProvision) { 3291 this.provision = theProvision; 3292 return this; 3293 } 3294 3295 public boolean hasProvision() { 3296 if (this.provision == null) 3297 return false; 3298 for (ProvisionComponent item : this.provision) 3299 if (!item.isEmpty()) 3300 return true; 3301 return false; 3302 } 3303 3304 public ProvisionComponent addProvision() { //3 3305 ProvisionComponent t = new ProvisionComponent(); 3306 if (this.provision == null) 3307 this.provision = new ArrayList<ProvisionComponent>(); 3308 this.provision.add(t); 3309 return t; 3310 } 3311 3312 public Consent addProvision(ProvisionComponent t) { //3 3313 if (t == null) 3314 return this; 3315 if (this.provision == null) 3316 this.provision = new ArrayList<ProvisionComponent>(); 3317 this.provision.add(t); 3318 return this; 3319 } 3320 3321 /** 3322 * @return The first repetition of repeating field {@link #provision}, creating it if it does not already exist {3} 3323 */ 3324 public ProvisionComponent getProvisionFirstRep() { 3325 if (getProvision().isEmpty()) { 3326 addProvision(); 3327 } 3328 return getProvision().get(0); 3329 } 3330 3331 protected void listChildren(List<Property> children) { 3332 super.listChildren(children); 3333 children.add(new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3334 children.add(new Property("status", "code", "Indicates the current state of this Consent resource.", 0, 1, status)); 3335 children.add(new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category)); 3336 children.add(new Property("subject", "Reference(Patient|Practitioner|Group)", "The patient/healthcare practitioner or group of persons to whom this consent applies.", 0, 1, subject)); 3337 children.add(new Property("date", "date", "Date the consent instance was agreed to.", 0, 1, date)); 3338 children.add(new Property("period", "Period", "Effective period for this Consent Resource and all provisions unless specified in that provision.", 0, 1, period)); 3339 children.add(new Property("grantor", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for granting the rights listed in a Consent Directive.", 0, java.lang.Integer.MAX_VALUE, grantor)); 3340 children.add(new Property("grantee", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, grantee)); 3341 children.add(new Property("manager", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that manages the consent through its lifecycle.", 0, java.lang.Integer.MAX_VALUE, manager)); 3342 children.add(new Property("controller", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that controls/enforces the access according to the consent.", 0, java.lang.Integer.MAX_VALUE, controller)); 3343 children.add(new Property("sourceAttachment", "Attachment", "The source on which this consent statement is based. The source might be a scanned original paper form.", 0, java.lang.Integer.MAX_VALUE, sourceAttachment)); 3344 children.add(new Property("sourceReference", "Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, java.lang.Integer.MAX_VALUE, sourceReference)); 3345 children.add(new Property("regulatoryBasis", "CodeableConcept", "A set of codes that indicate the regulatory basis (if any) that this consent supports.", 0, java.lang.Integer.MAX_VALUE, regulatoryBasis)); 3346 children.add(new Property("policyBasis", "", "A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.", 0, 1, policyBasis)); 3347 children.add(new Property("policyText", "Reference(DocumentReference)", "A Reference to the human readable policy explaining the basis for the Consent.", 0, java.lang.Integer.MAX_VALUE, policyText)); 3348 children.add(new Property("verification", "", "Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person.", 0, java.lang.Integer.MAX_VALUE, verification)); 3349 children.add(new Property("decision", "code", "Action to take - permit or deny - as default.", 0, 1, decision)); 3350 children.add(new Property("provision", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, provision)); 3351 } 3352 3353 @Override 3354 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3355 switch (_hash) { 3356 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, java.lang.Integer.MAX_VALUE, identifier); 3357 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of this Consent resource.", 0, 1, status); 3358 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category); 3359 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Practitioner|Group)", "The patient/healthcare practitioner or group of persons to whom this consent applies.", 0, 1, subject); 3360 case 3076014: /*date*/ return new Property("date", "date", "Date the consent instance was agreed to.", 0, 1, date); 3361 case -991726143: /*period*/ return new Property("period", "Period", "Effective period for this Consent Resource and all provisions unless specified in that provision.", 0, 1, period); 3362 case 280295423: /*grantor*/ return new Property("grantor", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for granting the rights listed in a Consent Directive.", 0, java.lang.Integer.MAX_VALUE, grantor); 3363 case 280295100: /*grantee*/ return new Property("grantee", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, grantee); 3364 case 835260333: /*manager*/ return new Property("manager", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that manages the consent through its lifecycle.", 0, java.lang.Integer.MAX_VALUE, manager); 3365 case 637428636: /*controller*/ return new Property("controller", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that controls/enforces the access according to the consent.", 0, java.lang.Integer.MAX_VALUE, controller); 3366 case 1964406686: /*sourceAttachment*/ return new Property("sourceAttachment", "Attachment", "The source on which this consent statement is based. The source might be a scanned original paper form.", 0, java.lang.Integer.MAX_VALUE, sourceAttachment); 3367 case -244259472: /*sourceReference*/ return new Property("sourceReference", "Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, java.lang.Integer.MAX_VALUE, sourceReference); 3368 case -1780301690: /*regulatoryBasis*/ return new Property("regulatoryBasis", "CodeableConcept", "A set of codes that indicate the regulatory basis (if any) that this consent supports.", 0, java.lang.Integer.MAX_VALUE, regulatoryBasis); 3369 case 2138287660: /*policyBasis*/ return new Property("policyBasis", "", "A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.", 0, 1, policyBasis); 3370 case 1593537919: /*policyText*/ return new Property("policyText", "Reference(DocumentReference)", "A Reference to the human readable policy explaining the basis for the Consent.", 0, java.lang.Integer.MAX_VALUE, policyText); 3371 case -1484401125: /*verification*/ return new Property("verification", "", "Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person.", 0, java.lang.Integer.MAX_VALUE, verification); 3372 case 565719004: /*decision*/ return new Property("decision", "code", "Action to take - permit or deny - as default.", 0, 1, decision); 3373 case -547120939: /*provision*/ return new Property("provision", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, provision); 3374 default: return super.getNamedProperty(_hash, _name, _checkValid); 3375 } 3376 3377 } 3378 3379 @Override 3380 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3381 switch (hash) { 3382 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3383 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ConsentState> 3384 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3385 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3386 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3387 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3388 case 280295423: /*grantor*/ return this.grantor == null ? new Base[0] : this.grantor.toArray(new Base[this.grantor.size()]); // Reference 3389 case 280295100: /*grantee*/ return this.grantee == null ? new Base[0] : this.grantee.toArray(new Base[this.grantee.size()]); // Reference 3390 case 835260333: /*manager*/ return this.manager == null ? new Base[0] : this.manager.toArray(new Base[this.manager.size()]); // Reference 3391 case 637428636: /*controller*/ return this.controller == null ? new Base[0] : this.controller.toArray(new Base[this.controller.size()]); // Reference 3392 case 1964406686: /*sourceAttachment*/ return this.sourceAttachment == null ? new Base[0] : this.sourceAttachment.toArray(new Base[this.sourceAttachment.size()]); // Attachment 3393 case -244259472: /*sourceReference*/ return this.sourceReference == null ? new Base[0] : this.sourceReference.toArray(new Base[this.sourceReference.size()]); // Reference 3394 case -1780301690: /*regulatoryBasis*/ return this.regulatoryBasis == null ? new Base[0] : this.regulatoryBasis.toArray(new Base[this.regulatoryBasis.size()]); // CodeableConcept 3395 case 2138287660: /*policyBasis*/ return this.policyBasis == null ? new Base[0] : new Base[] {this.policyBasis}; // ConsentPolicyBasisComponent 3396 case 1593537919: /*policyText*/ return this.policyText == null ? new Base[0] : this.policyText.toArray(new Base[this.policyText.size()]); // Reference 3397 case -1484401125: /*verification*/ return this.verification == null ? new Base[0] : this.verification.toArray(new Base[this.verification.size()]); // ConsentVerificationComponent 3398 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // Enumeration<ConsentProvisionType> 3399 case -547120939: /*provision*/ return this.provision == null ? new Base[0] : this.provision.toArray(new Base[this.provision.size()]); // ProvisionComponent 3400 default: return super.getProperty(hash, name, checkValid); 3401 } 3402 3403 } 3404 3405 @Override 3406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3407 switch (hash) { 3408 case -1618432855: // identifier 3409 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3410 return value; 3411 case -892481550: // status 3412 value = new ConsentStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 3413 this.status = (Enumeration) value; // Enumeration<ConsentState> 3414 return value; 3415 case 50511102: // category 3416 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3417 return value; 3418 case -1867885268: // subject 3419 this.subject = TypeConvertor.castToReference(value); // Reference 3420 return value; 3421 case 3076014: // date 3422 this.date = TypeConvertor.castToDate(value); // DateType 3423 return value; 3424 case -991726143: // period 3425 this.period = TypeConvertor.castToPeriod(value); // Period 3426 return value; 3427 case 280295423: // grantor 3428 this.getGrantor().add(TypeConvertor.castToReference(value)); // Reference 3429 return value; 3430 case 280295100: // grantee 3431 this.getGrantee().add(TypeConvertor.castToReference(value)); // Reference 3432 return value; 3433 case 835260333: // manager 3434 this.getManager().add(TypeConvertor.castToReference(value)); // Reference 3435 return value; 3436 case 637428636: // controller 3437 this.getController().add(TypeConvertor.castToReference(value)); // Reference 3438 return value; 3439 case 1964406686: // sourceAttachment 3440 this.getSourceAttachment().add(TypeConvertor.castToAttachment(value)); // Attachment 3441 return value; 3442 case -244259472: // sourceReference 3443 this.getSourceReference().add(TypeConvertor.castToReference(value)); // Reference 3444 return value; 3445 case -1780301690: // regulatoryBasis 3446 this.getRegulatoryBasis().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3447 return value; 3448 case 2138287660: // policyBasis 3449 this.policyBasis = (ConsentPolicyBasisComponent) value; // ConsentPolicyBasisComponent 3450 return value; 3451 case 1593537919: // policyText 3452 this.getPolicyText().add(TypeConvertor.castToReference(value)); // Reference 3453 return value; 3454 case -1484401125: // verification 3455 this.getVerification().add((ConsentVerificationComponent) value); // ConsentVerificationComponent 3456 return value; 3457 case 565719004: // decision 3458 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3459 this.decision = (Enumeration) value; // Enumeration<ConsentProvisionType> 3460 return value; 3461 case -547120939: // provision 3462 this.getProvision().add((ProvisionComponent) value); // ProvisionComponent 3463 return value; 3464 default: return super.setProperty(hash, name, value); 3465 } 3466 3467 } 3468 3469 @Override 3470 public Base setProperty(String name, Base value) throws FHIRException { 3471 if (name.equals("identifier")) { 3472 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3473 } else if (name.equals("status")) { 3474 value = new ConsentStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 3475 this.status = (Enumeration) value; // Enumeration<ConsentState> 3476 } else if (name.equals("category")) { 3477 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3478 } else if (name.equals("subject")) { 3479 this.subject = TypeConvertor.castToReference(value); // Reference 3480 } else if (name.equals("date")) { 3481 this.date = TypeConvertor.castToDate(value); // DateType 3482 } else if (name.equals("period")) { 3483 this.period = TypeConvertor.castToPeriod(value); // Period 3484 } else if (name.equals("grantor")) { 3485 this.getGrantor().add(TypeConvertor.castToReference(value)); 3486 } else if (name.equals("grantee")) { 3487 this.getGrantee().add(TypeConvertor.castToReference(value)); 3488 } else if (name.equals("manager")) { 3489 this.getManager().add(TypeConvertor.castToReference(value)); 3490 } else if (name.equals("controller")) { 3491 this.getController().add(TypeConvertor.castToReference(value)); 3492 } else if (name.equals("sourceAttachment")) { 3493 this.getSourceAttachment().add(TypeConvertor.castToAttachment(value)); 3494 } else if (name.equals("sourceReference")) { 3495 this.getSourceReference().add(TypeConvertor.castToReference(value)); 3496 } else if (name.equals("regulatoryBasis")) { 3497 this.getRegulatoryBasis().add(TypeConvertor.castToCodeableConcept(value)); 3498 } else if (name.equals("policyBasis")) { 3499 this.policyBasis = (ConsentPolicyBasisComponent) value; // ConsentPolicyBasisComponent 3500 } else if (name.equals("policyText")) { 3501 this.getPolicyText().add(TypeConvertor.castToReference(value)); 3502 } else if (name.equals("verification")) { 3503 this.getVerification().add((ConsentVerificationComponent) value); 3504 } else if (name.equals("decision")) { 3505 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3506 this.decision = (Enumeration) value; // Enumeration<ConsentProvisionType> 3507 } else if (name.equals("provision")) { 3508 this.getProvision().add((ProvisionComponent) value); 3509 } else 3510 return super.setProperty(name, value); 3511 return value; 3512 } 3513 3514 @Override 3515 public void removeChild(String name, Base value) throws FHIRException { 3516 if (name.equals("identifier")) { 3517 this.getIdentifier().remove(value); 3518 } else if (name.equals("status")) { 3519 value = new ConsentStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 3520 this.status = (Enumeration) value; // Enumeration<ConsentState> 3521 } else if (name.equals("category")) { 3522 this.getCategory().remove(value); 3523 } else if (name.equals("subject")) { 3524 this.subject = null; 3525 } else if (name.equals("date")) { 3526 this.date = null; 3527 } else if (name.equals("period")) { 3528 this.period = null; 3529 } else if (name.equals("grantor")) { 3530 this.getGrantor().remove(value); 3531 } else if (name.equals("grantee")) { 3532 this.getGrantee().remove(value); 3533 } else if (name.equals("manager")) { 3534 this.getManager().remove(value); 3535 } else if (name.equals("controller")) { 3536 this.getController().remove(value); 3537 } else if (name.equals("sourceAttachment")) { 3538 this.getSourceAttachment().remove(value); 3539 } else if (name.equals("sourceReference")) { 3540 this.getSourceReference().remove(value); 3541 } else if (name.equals("regulatoryBasis")) { 3542 this.getRegulatoryBasis().remove(value); 3543 } else if (name.equals("policyBasis")) { 3544 this.policyBasis = (ConsentPolicyBasisComponent) value; // ConsentPolicyBasisComponent 3545 } else if (name.equals("policyText")) { 3546 this.getPolicyText().remove(value); 3547 } else if (name.equals("verification")) { 3548 this.getVerification().remove((ConsentVerificationComponent) value); 3549 } else if (name.equals("decision")) { 3550 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3551 this.decision = (Enumeration) value; // Enumeration<ConsentProvisionType> 3552 } else if (name.equals("provision")) { 3553 this.getProvision().remove((ProvisionComponent) value); 3554 } else 3555 super.removeChild(name, value); 3556 3557 } 3558 3559 @Override 3560 public Base makeProperty(int hash, String name) throws FHIRException { 3561 switch (hash) { 3562 case -1618432855: return addIdentifier(); 3563 case -892481550: return getStatusElement(); 3564 case 50511102: return addCategory(); 3565 case -1867885268: return getSubject(); 3566 case 3076014: return getDateElement(); 3567 case -991726143: return getPeriod(); 3568 case 280295423: return addGrantor(); 3569 case 280295100: return addGrantee(); 3570 case 835260333: return addManager(); 3571 case 637428636: return addController(); 3572 case 1964406686: return addSourceAttachment(); 3573 case -244259472: return addSourceReference(); 3574 case -1780301690: return addRegulatoryBasis(); 3575 case 2138287660: return getPolicyBasis(); 3576 case 1593537919: return addPolicyText(); 3577 case -1484401125: return addVerification(); 3578 case 565719004: return getDecisionElement(); 3579 case -547120939: return addProvision(); 3580 default: return super.makeProperty(hash, name); 3581 } 3582 3583 } 3584 3585 @Override 3586 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3587 switch (hash) { 3588 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3589 case -892481550: /*status*/ return new String[] {"code"}; 3590 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3591 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3592 case 3076014: /*date*/ return new String[] {"date"}; 3593 case -991726143: /*period*/ return new String[] {"Period"}; 3594 case 280295423: /*grantor*/ return new String[] {"Reference"}; 3595 case 280295100: /*grantee*/ return new String[] {"Reference"}; 3596 case 835260333: /*manager*/ return new String[] {"Reference"}; 3597 case 637428636: /*controller*/ return new String[] {"Reference"}; 3598 case 1964406686: /*sourceAttachment*/ return new String[] {"Attachment"}; 3599 case -244259472: /*sourceReference*/ return new String[] {"Reference"}; 3600 case -1780301690: /*regulatoryBasis*/ return new String[] {"CodeableConcept"}; 3601 case 2138287660: /*policyBasis*/ return new String[] {}; 3602 case 1593537919: /*policyText*/ return new String[] {"Reference"}; 3603 case -1484401125: /*verification*/ return new String[] {}; 3604 case 565719004: /*decision*/ return new String[] {"code"}; 3605 case -547120939: /*provision*/ return new String[] {}; 3606 default: return super.getTypesForProperty(hash, name); 3607 } 3608 3609 } 3610 3611 @Override 3612 public Base addChild(String name) throws FHIRException { 3613 if (name.equals("identifier")) { 3614 return addIdentifier(); 3615 } 3616 else if (name.equals("status")) { 3617 throw new FHIRException("Cannot call addChild on a singleton property Consent.status"); 3618 } 3619 else if (name.equals("category")) { 3620 return addCategory(); 3621 } 3622 else if (name.equals("subject")) { 3623 this.subject = new Reference(); 3624 return this.subject; 3625 } 3626 else if (name.equals("date")) { 3627 throw new FHIRException("Cannot call addChild on a singleton property Consent.date"); 3628 } 3629 else if (name.equals("period")) { 3630 this.period = new Period(); 3631 return this.period; 3632 } 3633 else if (name.equals("grantor")) { 3634 return addGrantor(); 3635 } 3636 else if (name.equals("grantee")) { 3637 return addGrantee(); 3638 } 3639 else if (name.equals("manager")) { 3640 return addManager(); 3641 } 3642 else if (name.equals("controller")) { 3643 return addController(); 3644 } 3645 else if (name.equals("sourceAttachment")) { 3646 return addSourceAttachment(); 3647 } 3648 else if (name.equals("sourceReference")) { 3649 return addSourceReference(); 3650 } 3651 else if (name.equals("regulatoryBasis")) { 3652 return addRegulatoryBasis(); 3653 } 3654 else if (name.equals("policyBasis")) { 3655 this.policyBasis = new ConsentPolicyBasisComponent(); 3656 return this.policyBasis; 3657 } 3658 else if (name.equals("policyText")) { 3659 return addPolicyText(); 3660 } 3661 else if (name.equals("verification")) { 3662 return addVerification(); 3663 } 3664 else if (name.equals("decision")) { 3665 throw new FHIRException("Cannot call addChild on a singleton property Consent.decision"); 3666 } 3667 else if (name.equals("provision")) { 3668 return addProvision(); 3669 } 3670 else 3671 return super.addChild(name); 3672 } 3673 3674 public String fhirType() { 3675 return "Consent"; 3676 3677 } 3678 3679 public Consent copy() { 3680 Consent dst = new Consent(); 3681 copyValues(dst); 3682 return dst; 3683 } 3684 3685 public void copyValues(Consent dst) { 3686 super.copyValues(dst); 3687 if (identifier != null) { 3688 dst.identifier = new ArrayList<Identifier>(); 3689 for (Identifier i : identifier) 3690 dst.identifier.add(i.copy()); 3691 }; 3692 dst.status = status == null ? null : status.copy(); 3693 if (category != null) { 3694 dst.category = new ArrayList<CodeableConcept>(); 3695 for (CodeableConcept i : category) 3696 dst.category.add(i.copy()); 3697 }; 3698 dst.subject = subject == null ? null : subject.copy(); 3699 dst.date = date == null ? null : date.copy(); 3700 dst.period = period == null ? null : period.copy(); 3701 if (grantor != null) { 3702 dst.grantor = new ArrayList<Reference>(); 3703 for (Reference i : grantor) 3704 dst.grantor.add(i.copy()); 3705 }; 3706 if (grantee != null) { 3707 dst.grantee = new ArrayList<Reference>(); 3708 for (Reference i : grantee) 3709 dst.grantee.add(i.copy()); 3710 }; 3711 if (manager != null) { 3712 dst.manager = new ArrayList<Reference>(); 3713 for (Reference i : manager) 3714 dst.manager.add(i.copy()); 3715 }; 3716 if (controller != null) { 3717 dst.controller = new ArrayList<Reference>(); 3718 for (Reference i : controller) 3719 dst.controller.add(i.copy()); 3720 }; 3721 if (sourceAttachment != null) { 3722 dst.sourceAttachment = new ArrayList<Attachment>(); 3723 for (Attachment i : sourceAttachment) 3724 dst.sourceAttachment.add(i.copy()); 3725 }; 3726 if (sourceReference != null) { 3727 dst.sourceReference = new ArrayList<Reference>(); 3728 for (Reference i : sourceReference) 3729 dst.sourceReference.add(i.copy()); 3730 }; 3731 if (regulatoryBasis != null) { 3732 dst.regulatoryBasis = new ArrayList<CodeableConcept>(); 3733 for (CodeableConcept i : regulatoryBasis) 3734 dst.regulatoryBasis.add(i.copy()); 3735 }; 3736 dst.policyBasis = policyBasis == null ? null : policyBasis.copy(); 3737 if (policyText != null) { 3738 dst.policyText = new ArrayList<Reference>(); 3739 for (Reference i : policyText) 3740 dst.policyText.add(i.copy()); 3741 }; 3742 if (verification != null) { 3743 dst.verification = new ArrayList<ConsentVerificationComponent>(); 3744 for (ConsentVerificationComponent i : verification) 3745 dst.verification.add(i.copy()); 3746 }; 3747 dst.decision = decision == null ? null : decision.copy(); 3748 if (provision != null) { 3749 dst.provision = new ArrayList<ProvisionComponent>(); 3750 for (ProvisionComponent i : provision) 3751 dst.provision.add(i.copy()); 3752 }; 3753 } 3754 3755 protected Consent typedCopy() { 3756 return copy(); 3757 } 3758 3759 @Override 3760 public boolean equalsDeep(Base other_) { 3761 if (!super.equalsDeep(other_)) 3762 return false; 3763 if (!(other_ instanceof Consent)) 3764 return false; 3765 Consent o = (Consent) other_; 3766 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 3767 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) && compareDeep(period, o.period, true) 3768 && compareDeep(grantor, o.grantor, true) && compareDeep(grantee, o.grantee, true) && compareDeep(manager, o.manager, true) 3769 && compareDeep(controller, o.controller, true) && compareDeep(sourceAttachment, o.sourceAttachment, true) 3770 && compareDeep(sourceReference, o.sourceReference, true) && compareDeep(regulatoryBasis, o.regulatoryBasis, true) 3771 && compareDeep(policyBasis, o.policyBasis, true) && compareDeep(policyText, o.policyText, true) 3772 && compareDeep(verification, o.verification, true) && compareDeep(decision, o.decision, true) && compareDeep(provision, o.provision, true) 3773 ; 3774 } 3775 3776 @Override 3777 public boolean equalsShallow(Base other_) { 3778 if (!super.equalsShallow(other_)) 3779 return false; 3780 if (!(other_ instanceof Consent)) 3781 return false; 3782 Consent o = (Consent) other_; 3783 return compareValues(status, o.status, true) && compareValues(date, o.date, true) && compareValues(decision, o.decision, true) 3784 ; 3785 } 3786 3787 public boolean isEmpty() { 3788 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 3789 , subject, date, period, grantor, grantee, manager, controller, sourceAttachment 3790 , sourceReference, regulatoryBasis, policyBasis, policyText, verification, decision 3791 , provision); 3792 } 3793 3794 @Override 3795 public ResourceType getResourceType() { 3796 return ResourceType.Consent; 3797 } 3798 3799 /** 3800 * Search parameter: <b>action</b> 3801 * <p> 3802 * Description: <b>Actions controlled by this rule</b><br> 3803 * Type: <b>token</b><br> 3804 * Path: <b>Consent.provision.action</b><br> 3805 * </p> 3806 */ 3807 @SearchParamDefinition(name="action", path="Consent.provision.action", description="Actions controlled by this rule", type="token" ) 3808 public static final String SP_ACTION = "action"; 3809 /** 3810 * <b>Fluent Client</b> search parameter constant for <b>action</b> 3811 * <p> 3812 * Description: <b>Actions controlled by this rule</b><br> 3813 * Type: <b>token</b><br> 3814 * Path: <b>Consent.provision.action</b><br> 3815 * </p> 3816 */ 3817 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTION); 3818 3819 /** 3820 * Search parameter: <b>actor</b> 3821 * <p> 3822 * Description: <b>Resource for the actor (or group, by role)</b><br> 3823 * Type: <b>reference</b><br> 3824 * Path: <b>Consent.provision.actor.reference</b><br> 3825 * </p> 3826 */ 3827 @SearchParamDefinition(name="actor", path="Consent.provision.actor.reference", description="Resource for the actor (or group, by role)", type="reference", target={CareTeam.class, Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3828 public static final String SP_ACTOR = "actor"; 3829 /** 3830 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 3831 * <p> 3832 * Description: <b>Resource for the actor (or group, by role)</b><br> 3833 * Type: <b>reference</b><br> 3834 * Path: <b>Consent.provision.actor.reference</b><br> 3835 * </p> 3836 */ 3837 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 3838 3839/** 3840 * Constant for fluent queries to be used to add include statements. Specifies 3841 * the path value of "<b>Consent:actor</b>". 3842 */ 3843 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Consent:actor").toLocked(); 3844 3845 /** 3846 * Search parameter: <b>category</b> 3847 * <p> 3848 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 3849 * Type: <b>token</b><br> 3850 * Path: <b>Consent.category</b><br> 3851 * </p> 3852 */ 3853 @SearchParamDefinition(name="category", path="Consent.category", description="Classification of the consent statement - for indexing/retrieval", type="token" ) 3854 public static final String SP_CATEGORY = "category"; 3855 /** 3856 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3857 * <p> 3858 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 3859 * Type: <b>token</b><br> 3860 * Path: <b>Consent.category</b><br> 3861 * </p> 3862 */ 3863 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3864 3865 /** 3866 * Search parameter: <b>controller</b> 3867 * <p> 3868 * Description: <b>Consent Enforcer</b><br> 3869 * Type: <b>reference</b><br> 3870 * Path: <b>Consent.controller</b><br> 3871 * </p> 3872 */ 3873 @SearchParamDefinition(name="controller", path="Consent.controller", description="Consent Enforcer", type="reference", target={HealthcareService.class, Organization.class, Patient.class, Practitioner.class } ) 3874 public static final String SP_CONTROLLER = "controller"; 3875 /** 3876 * <b>Fluent Client</b> search parameter constant for <b>controller</b> 3877 * <p> 3878 * Description: <b>Consent Enforcer</b><br> 3879 * Type: <b>reference</b><br> 3880 * Path: <b>Consent.controller</b><br> 3881 * </p> 3882 */ 3883 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTROLLER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTROLLER); 3884 3885/** 3886 * Constant for fluent queries to be used to add include statements. Specifies 3887 * the path value of "<b>Consent:controller</b>". 3888 */ 3889 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTROLLER = new ca.uhn.fhir.model.api.Include("Consent:controller").toLocked(); 3890 3891 /** 3892 * Search parameter: <b>data</b> 3893 * <p> 3894 * Description: <b>The actual data reference</b><br> 3895 * Type: <b>reference</b><br> 3896 * Path: <b>Consent.provision.data.reference</b><br> 3897 * </p> 3898 */ 3899 @SearchParamDefinition(name="data", path="Consent.provision.data.reference", description="The actual data reference", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3900 public static final String SP_DATA = "data"; 3901 /** 3902 * <b>Fluent Client</b> search parameter constant for <b>data</b> 3903 * <p> 3904 * Description: <b>The actual data reference</b><br> 3905 * Type: <b>reference</b><br> 3906 * Path: <b>Consent.provision.data.reference</b><br> 3907 * </p> 3908 */ 3909 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DATA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DATA); 3910 3911/** 3912 * Constant for fluent queries to be used to add include statements. Specifies 3913 * the path value of "<b>Consent:data</b>". 3914 */ 3915 public static final ca.uhn.fhir.model.api.Include INCLUDE_DATA = new ca.uhn.fhir.model.api.Include("Consent:data").toLocked(); 3916 3917 /** 3918 * Search parameter: <b>grantee</b> 3919 * <p> 3920 * Description: <b>Who is agreeing to the policy and rules</b><br> 3921 * Type: <b>reference</b><br> 3922 * Path: <b>Consent.grantee</b><br> 3923 * </p> 3924 */ 3925 @SearchParamDefinition(name="grantee", path="Consent.grantee", description="Who is agreeing to the policy and rules", type="reference", target={CareTeam.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3926 public static final String SP_GRANTEE = "grantee"; 3927 /** 3928 * <b>Fluent Client</b> search parameter constant for <b>grantee</b> 3929 * <p> 3930 * Description: <b>Who is agreeing to the policy and rules</b><br> 3931 * Type: <b>reference</b><br> 3932 * Path: <b>Consent.grantee</b><br> 3933 * </p> 3934 */ 3935 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GRANTEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GRANTEE); 3936 3937/** 3938 * Constant for fluent queries to be used to add include statements. Specifies 3939 * the path value of "<b>Consent:grantee</b>". 3940 */ 3941 public static final ca.uhn.fhir.model.api.Include INCLUDE_GRANTEE = new ca.uhn.fhir.model.api.Include("Consent:grantee").toLocked(); 3942 3943 /** 3944 * Search parameter: <b>manager</b> 3945 * <p> 3946 * Description: <b>Consent workflow management</b><br> 3947 * Type: <b>reference</b><br> 3948 * Path: <b>Consent.manager</b><br> 3949 * </p> 3950 */ 3951 @SearchParamDefinition(name="manager", path="Consent.manager", description="Consent workflow management", type="reference", target={HealthcareService.class, Organization.class, Patient.class, Practitioner.class } ) 3952 public static final String SP_MANAGER = "manager"; 3953 /** 3954 * <b>Fluent Client</b> search parameter constant for <b>manager</b> 3955 * <p> 3956 * Description: <b>Consent workflow management</b><br> 3957 * Type: <b>reference</b><br> 3958 * Path: <b>Consent.manager</b><br> 3959 * </p> 3960 */ 3961 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANAGER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANAGER); 3962 3963/** 3964 * Constant for fluent queries to be used to add include statements. Specifies 3965 * the path value of "<b>Consent:manager</b>". 3966 */ 3967 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANAGER = new ca.uhn.fhir.model.api.Include("Consent:manager").toLocked(); 3968 3969 /** 3970 * Search parameter: <b>period</b> 3971 * <p> 3972 * Description: <b>Timeframe for this rule</b><br> 3973 * Type: <b>date</b><br> 3974 * Path: <b>Consent.provision.period</b><br> 3975 * </p> 3976 */ 3977 @SearchParamDefinition(name="period", path="Consent.provision.period", description="Timeframe for this rule", type="date" ) 3978 public static final String SP_PERIOD = "period"; 3979 /** 3980 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3981 * <p> 3982 * Description: <b>Timeframe for this rule</b><br> 3983 * Type: <b>date</b><br> 3984 * Path: <b>Consent.provision.period</b><br> 3985 * </p> 3986 */ 3987 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 3988 3989 /** 3990 * Search parameter: <b>purpose</b> 3991 * <p> 3992 * Description: <b>Context of activities covered by this rule</b><br> 3993 * Type: <b>token</b><br> 3994 * Path: <b>Consent.provision.purpose</b><br> 3995 * </p> 3996 */ 3997 @SearchParamDefinition(name="purpose", path="Consent.provision.purpose", description="Context of activities covered by this rule", type="token" ) 3998 public static final String SP_PURPOSE = "purpose"; 3999 /** 4000 * <b>Fluent Client</b> search parameter constant for <b>purpose</b> 4001 * <p> 4002 * Description: <b>Context of activities covered by this rule</b><br> 4003 * Type: <b>token</b><br> 4004 * Path: <b>Consent.provision.purpose</b><br> 4005 * </p> 4006 */ 4007 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PURPOSE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PURPOSE); 4008 4009 /** 4010 * Search parameter: <b>security-label</b> 4011 * <p> 4012 * Description: <b>Security Labels that define affected resources</b><br> 4013 * Type: <b>token</b><br> 4014 * Path: <b>Consent.provision.securityLabel</b><br> 4015 * </p> 4016 */ 4017 @SearchParamDefinition(name="security-label", path="Consent.provision.securityLabel", description="Security Labels that define affected resources", type="token" ) 4018 public static final String SP_SECURITY_LABEL = "security-label"; 4019 /** 4020 * <b>Fluent Client</b> search parameter constant for <b>security-label</b> 4021 * <p> 4022 * Description: <b>Security Labels that define affected resources</b><br> 4023 * Type: <b>token</b><br> 4024 * Path: <b>Consent.provision.securityLabel</b><br> 4025 * </p> 4026 */ 4027 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_LABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITY_LABEL); 4028 4029 /** 4030 * Search parameter: <b>source-reference</b> 4031 * <p> 4032 * Description: <b>Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse</b><br> 4033 * Type: <b>reference</b><br> 4034 * Path: <b>Consent.sourceReference</b><br> 4035 * </p> 4036 */ 4037 @SearchParamDefinition(name="source-reference", path="Consent.sourceReference", description="Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse", type="reference", target={Consent.class, Contract.class, DocumentReference.class, QuestionnaireResponse.class } ) 4038 public static final String SP_SOURCE_REFERENCE = "source-reference"; 4039 /** 4040 * <b>Fluent Client</b> search parameter constant for <b>source-reference</b> 4041 * <p> 4042 * Description: <b>Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse</b><br> 4043 * Type: <b>reference</b><br> 4044 * Path: <b>Consent.sourceReference</b><br> 4045 * </p> 4046 */ 4047 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE_REFERENCE); 4048 4049/** 4050 * Constant for fluent queries to be used to add include statements. Specifies 4051 * the path value of "<b>Consent:source-reference</b>". 4052 */ 4053 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_REFERENCE = new ca.uhn.fhir.model.api.Include("Consent:source-reference").toLocked(); 4054 4055 /** 4056 * Search parameter: <b>status</b> 4057 * <p> 4058 * Description: <b>draft | active | inactive | entered-in-error | unknown</b><br> 4059 * Type: <b>token</b><br> 4060 * Path: <b>Consent.status</b><br> 4061 * </p> 4062 */ 4063 @SearchParamDefinition(name="status", path="Consent.status", description="draft | active | inactive | entered-in-error | unknown", type="token" ) 4064 public static final String SP_STATUS = "status"; 4065 /** 4066 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4067 * <p> 4068 * Description: <b>draft | active | inactive | entered-in-error | unknown</b><br> 4069 * Type: <b>token</b><br> 4070 * Path: <b>Consent.status</b><br> 4071 * </p> 4072 */ 4073 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4074 4075 /** 4076 * Search parameter: <b>subject</b> 4077 * <p> 4078 * Description: <b>Who the consent applies to</b><br> 4079 * Type: <b>reference</b><br> 4080 * Path: <b>Consent.subject</b><br> 4081 * </p> 4082 */ 4083 @SearchParamDefinition(name="subject", path="Consent.subject", description="Who the consent applies to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class, Practitioner.class } ) 4084 public static final String SP_SUBJECT = "subject"; 4085 /** 4086 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4087 * <p> 4088 * Description: <b>Who the consent applies to</b><br> 4089 * Type: <b>reference</b><br> 4090 * Path: <b>Consent.subject</b><br> 4091 * </p> 4092 */ 4093 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4094 4095/** 4096 * Constant for fluent queries to be used to add include statements. Specifies 4097 * the path value of "<b>Consent:subject</b>". 4098 */ 4099 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Consent:subject").toLocked(); 4100 4101 /** 4102 * Search parameter: <b>verified-date</b> 4103 * <p> 4104 * Description: <b>When consent verified</b><br> 4105 * Type: <b>date</b><br> 4106 * Path: <b>Consent.verification.verificationDate</b><br> 4107 * </p> 4108 */ 4109 @SearchParamDefinition(name="verified-date", path="Consent.verification.verificationDate", description="When consent verified", type="date" ) 4110 public static final String SP_VERIFIED_DATE = "verified-date"; 4111 /** 4112 * <b>Fluent Client</b> search parameter constant for <b>verified-date</b> 4113 * <p> 4114 * Description: <b>When consent verified</b><br> 4115 * Type: <b>date</b><br> 4116 * Path: <b>Consent.verification.verificationDate</b><br> 4117 * </p> 4118 */ 4119 public static final ca.uhn.fhir.rest.gclient.DateClientParam VERIFIED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_VERIFIED_DATE); 4120 4121 /** 4122 * Search parameter: <b>verified</b> 4123 * <p> 4124 * Description: <b>Has been verified</b><br> 4125 * Type: <b>token</b><br> 4126 * Path: <b>Consent.verification.verified</b><br> 4127 * </p> 4128 */ 4129 @SearchParamDefinition(name="verified", path="Consent.verification.verified", description="Has been verified", type="token" ) 4130 public static final String SP_VERIFIED = "verified"; 4131 /** 4132 * <b>Fluent Client</b> search parameter constant for <b>verified</b> 4133 * <p> 4134 * Description: <b>Has been verified</b><br> 4135 * Type: <b>token</b><br> 4136 * Path: <b>Consent.verification.verified</b><br> 4137 * </p> 4138 */ 4139 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFIED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERIFIED); 4140 4141 /** 4142 * Search parameter: <b>date</b> 4143 * <p> 4144 * Description: <b>Multiple Resources: 4145 4146* [AdverseEvent](adverseevent.html): When the event occurred 4147* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4148* [Appointment](appointment.html): Appointment date/time. 4149* [AuditEvent](auditevent.html): Time when the event was recorded 4150* [CarePlan](careplan.html): Time period plan covers 4151* [CareTeam](careteam.html): A date within the coverage time period. 4152* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4153* [Composition](composition.html): Composition editing time 4154* [Consent](consent.html): When consent was agreed to 4155* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4156* [DocumentReference](documentreference.html): When this document reference was created 4157* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4158* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4159* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4160* [Flag](flag.html): Time period when flag is active 4161* [Immunization](immunization.html): Vaccination (non)-Administration Date 4162* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4163* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4164* [Invoice](invoice.html): Invoice date / posting date 4165* [List](list.html): When the list was prepared 4166* [MeasureReport](measurereport.html): The date of the measure report 4167* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4168* [Observation](observation.html): Clinically relevant time/time-period for observation 4169* [Procedure](procedure.html): When the procedure occurred or is occurring 4170* [ResearchSubject](researchsubject.html): Start and end of participation 4171* [RiskAssessment](riskassessment.html): When was assessment made? 4172* [SupplyRequest](supplyrequest.html): When the request was made 4173</b><br> 4174 * Type: <b>date</b><br> 4175 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4176 * </p> 4177 */ 4178 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4179 public static final String SP_DATE = "date"; 4180 /** 4181 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4182 * <p> 4183 * Description: <b>Multiple Resources: 4184 4185* [AdverseEvent](adverseevent.html): When the event occurred 4186* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4187* [Appointment](appointment.html): Appointment date/time. 4188* [AuditEvent](auditevent.html): Time when the event was recorded 4189* [CarePlan](careplan.html): Time period plan covers 4190* [CareTeam](careteam.html): A date within the coverage time period. 4191* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4192* [Composition](composition.html): Composition editing time 4193* [Consent](consent.html): When consent was agreed to 4194* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4195* [DocumentReference](documentreference.html): When this document reference was created 4196* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4197* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4198* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4199* [Flag](flag.html): Time period when flag is active 4200* [Immunization](immunization.html): Vaccination (non)-Administration Date 4201* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4202* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4203* [Invoice](invoice.html): Invoice date / posting date 4204* [List](list.html): When the list was prepared 4205* [MeasureReport](measurereport.html): The date of the measure report 4206* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4207* [Observation](observation.html): Clinically relevant time/time-period for observation 4208* [Procedure](procedure.html): When the procedure occurred or is occurring 4209* [ResearchSubject](researchsubject.html): Start and end of participation 4210* [RiskAssessment](riskassessment.html): When was assessment made? 4211* [SupplyRequest](supplyrequest.html): When the request was made 4212</b><br> 4213 * Type: <b>date</b><br> 4214 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4215 * </p> 4216 */ 4217 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4218 4219 /** 4220 * Search parameter: <b>identifier</b> 4221 * <p> 4222 * Description: <b>Multiple Resources: 4223 4224* [Account](account.html): Account number 4225* [AdverseEvent](adverseevent.html): Business identifier for the event 4226* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4227* [Appointment](appointment.html): An Identifier of the Appointment 4228* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4229* [Basic](basic.html): Business identifier 4230* [BodyStructure](bodystructure.html): Bodystructure identifier 4231* [CarePlan](careplan.html): External Ids for this plan 4232* [CareTeam](careteam.html): External Ids for this team 4233* [ChargeItem](chargeitem.html): Business Identifier for item 4234* [Claim](claim.html): The primary identifier of the financial resource 4235* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4236* [ClinicalImpression](clinicalimpression.html): Business identifier 4237* [Communication](communication.html): Unique identifier 4238* [CommunicationRequest](communicationrequest.html): Unique identifier 4239* [Composition](composition.html): Version-independent identifier for the Composition 4240* [Condition](condition.html): A unique identifier of the condition record 4241* [Consent](consent.html): Identifier for this record (external references) 4242* [Contract](contract.html): The identity of the contract 4243* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4244* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4245* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4246* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4247* [DeviceRequest](devicerequest.html): Business identifier for request/order 4248* [DeviceUsage](deviceusage.html): Search by identifier 4249* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4250* [DocumentReference](documentreference.html): Identifier of the attachment binary 4251* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4252* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4253* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4254* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4255* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4256* [Flag](flag.html): Business identifier 4257* [Goal](goal.html): External Ids for this goal 4258* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4259* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4260* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4261* [Immunization](immunization.html): Business identifier 4262* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4263* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4264* [Invoice](invoice.html): Business Identifier for item 4265* [List](list.html): Business identifier 4266* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4267* [Medication](medication.html): Returns medications with this external identifier 4268* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4269* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4270* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4271* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4272* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4273* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4274* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4275* [Observation](observation.html): The unique id for a particular observation 4276* [Person](person.html): A person Identifier 4277* [Procedure](procedure.html): A unique identifier for a procedure 4278* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4279* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4280* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4281* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4282* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4283* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4284* [Specimen](specimen.html): The unique identifier associated with the specimen 4285* [SupplyDelivery](supplydelivery.html): External identifier 4286* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4287* [Task](task.html): Search for a task instance by its business identifier 4288* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4289</b><br> 4290 * Type: <b>token</b><br> 4291 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4292 * </p> 4293 */ 4294 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4295 public static final String SP_IDENTIFIER = "identifier"; 4296 /** 4297 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4298 * <p> 4299 * Description: <b>Multiple Resources: 4300 4301* [Account](account.html): Account number 4302* [AdverseEvent](adverseevent.html): Business identifier for the event 4303* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4304* [Appointment](appointment.html): An Identifier of the Appointment 4305* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4306* [Basic](basic.html): Business identifier 4307* [BodyStructure](bodystructure.html): Bodystructure identifier 4308* [CarePlan](careplan.html): External Ids for this plan 4309* [CareTeam](careteam.html): External Ids for this team 4310* [ChargeItem](chargeitem.html): Business Identifier for item 4311* [Claim](claim.html): The primary identifier of the financial resource 4312* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4313* [ClinicalImpression](clinicalimpression.html): Business identifier 4314* [Communication](communication.html): Unique identifier 4315* [CommunicationRequest](communicationrequest.html): Unique identifier 4316* [Composition](composition.html): Version-independent identifier for the Composition 4317* [Condition](condition.html): A unique identifier of the condition record 4318* [Consent](consent.html): Identifier for this record (external references) 4319* [Contract](contract.html): The identity of the contract 4320* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4321* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4322* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4323* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4324* [DeviceRequest](devicerequest.html): Business identifier for request/order 4325* [DeviceUsage](deviceusage.html): Search by identifier 4326* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4327* [DocumentReference](documentreference.html): Identifier of the attachment binary 4328* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4329* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4330* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4331* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4332* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4333* [Flag](flag.html): Business identifier 4334* [Goal](goal.html): External Ids for this goal 4335* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4336* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4337* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4338* [Immunization](immunization.html): Business identifier 4339* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4340* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4341* [Invoice](invoice.html): Business Identifier for item 4342* [List](list.html): Business identifier 4343* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4344* [Medication](medication.html): Returns medications with this external identifier 4345* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4346* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4347* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4348* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4349* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4350* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4351* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4352* [Observation](observation.html): The unique id for a particular observation 4353* [Person](person.html): A person Identifier 4354* [Procedure](procedure.html): A unique identifier for a procedure 4355* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4356* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4357* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4358* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4359* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4360* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4361* [Specimen](specimen.html): The unique identifier associated with the specimen 4362* [SupplyDelivery](supplydelivery.html): External identifier 4363* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4364* [Task](task.html): Search for a task instance by its business identifier 4365* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4366</b><br> 4367 * Type: <b>token</b><br> 4368 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4369 * </p> 4370 */ 4371 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4372 4373 /** 4374 * Search parameter: <b>patient</b> 4375 * <p> 4376 * Description: <b>Multiple Resources: 4377 4378* [Account](account.html): The entity that caused the expenses 4379* [AdverseEvent](adverseevent.html): Subject impacted by event 4380* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4381* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4382* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4383* [AuditEvent](auditevent.html): Where the activity involved patient data 4384* [Basic](basic.html): Identifies the focus of this resource 4385* [BodyStructure](bodystructure.html): Who this is about 4386* [CarePlan](careplan.html): Who the care plan is for 4387* [CareTeam](careteam.html): Who care team is for 4388* [ChargeItem](chargeitem.html): Individual service was done for/to 4389* [Claim](claim.html): Patient receiving the products or services 4390* [ClaimResponse](claimresponse.html): The subject of care 4391* [ClinicalImpression](clinicalimpression.html): Patient assessed 4392* [Communication](communication.html): Focus of message 4393* [CommunicationRequest](communicationrequest.html): Focus of message 4394* [Composition](composition.html): Who and/or what the composition is about 4395* [Condition](condition.html): Who has the condition? 4396* [Consent](consent.html): Who the consent applies to 4397* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4398* [Coverage](coverage.html): Retrieve coverages for a patient 4399* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4400* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4401* [DetectedIssue](detectedissue.html): Associated patient 4402* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4403* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4404* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4405* [DocumentReference](documentreference.html): Who/what is the subject of the document 4406* [Encounter](encounter.html): The patient present at the encounter 4407* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4408* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4409* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4410* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4411* [Flag](flag.html): The identity of a subject to list flags for 4412* [Goal](goal.html): Who this goal is intended for 4413* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4414* [ImagingSelection](imagingselection.html): Who the study is about 4415* [ImagingStudy](imagingstudy.html): Who the study is about 4416* [Immunization](immunization.html): The patient for the vaccination record 4417* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4418* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4419* [Invoice](invoice.html): Recipient(s) of goods and services 4420* [List](list.html): If all resources have the same subject 4421* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4422* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4423* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4424* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4425* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4426* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4427* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4428* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4429* [Observation](observation.html): The subject that the observation is about (if patient) 4430* [Person](person.html): The Person links to this Patient 4431* [Procedure](procedure.html): Search by subject - a patient 4432* [Provenance](provenance.html): Where the activity involved patient data 4433* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4434* [RelatedPerson](relatedperson.html): The patient this related person is related to 4435* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4436* [ResearchSubject](researchsubject.html): Who or what is part of study 4437* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4438* [ServiceRequest](servicerequest.html): Search by subject - a patient 4439* [Specimen](specimen.html): The patient the specimen comes from 4440* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4441* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4442* [Task](task.html): Search by patient 4443* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4444</b><br> 4445 * Type: <b>reference</b><br> 4446 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4447 * </p> 4448 */ 4449 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4450 public static final String SP_PATIENT = "patient"; 4451 /** 4452 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4453 * <p> 4454 * Description: <b>Multiple Resources: 4455 4456* [Account](account.html): The entity that caused the expenses 4457* [AdverseEvent](adverseevent.html): Subject impacted by event 4458* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4459* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4460* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4461* [AuditEvent](auditevent.html): Where the activity involved patient data 4462* [Basic](basic.html): Identifies the focus of this resource 4463* [BodyStructure](bodystructure.html): Who this is about 4464* [CarePlan](careplan.html): Who the care plan is for 4465* [CareTeam](careteam.html): Who care team is for 4466* [ChargeItem](chargeitem.html): Individual service was done for/to 4467* [Claim](claim.html): Patient receiving the products or services 4468* [ClaimResponse](claimresponse.html): The subject of care 4469* [ClinicalImpression](clinicalimpression.html): Patient assessed 4470* [Communication](communication.html): Focus of message 4471* [CommunicationRequest](communicationrequest.html): Focus of message 4472* [Composition](composition.html): Who and/or what the composition is about 4473* [Condition](condition.html): Who has the condition? 4474* [Consent](consent.html): Who the consent applies to 4475* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4476* [Coverage](coverage.html): Retrieve coverages for a patient 4477* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4478* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4479* [DetectedIssue](detectedissue.html): Associated patient 4480* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4481* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4482* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4483* [DocumentReference](documentreference.html): Who/what is the subject of the document 4484* [Encounter](encounter.html): The patient present at the encounter 4485* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4486* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4487* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4488* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4489* [Flag](flag.html): The identity of a subject to list flags for 4490* [Goal](goal.html): Who this goal is intended for 4491* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4492* [ImagingSelection](imagingselection.html): Who the study is about 4493* [ImagingStudy](imagingstudy.html): Who the study is about 4494* [Immunization](immunization.html): The patient for the vaccination record 4495* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4496* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4497* [Invoice](invoice.html): Recipient(s) of goods and services 4498* [List](list.html): If all resources have the same subject 4499* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4500* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4501* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4502* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4503* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4504* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4505* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4506* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4507* [Observation](observation.html): The subject that the observation is about (if patient) 4508* [Person](person.html): The Person links to this Patient 4509* [Procedure](procedure.html): Search by subject - a patient 4510* [Provenance](provenance.html): Where the activity involved patient data 4511* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4512* [RelatedPerson](relatedperson.html): The patient this related person is related to 4513* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4514* [ResearchSubject](researchsubject.html): Who or what is part of study 4515* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4516* [ServiceRequest](servicerequest.html): Search by subject - a patient 4517* [Specimen](specimen.html): The patient the specimen comes from 4518* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4519* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4520* [Task](task.html): Search by patient 4521* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4522</b><br> 4523 * Type: <b>reference</b><br> 4524 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4525 * </p> 4526 */ 4527 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4528 4529/** 4530 * Constant for fluent queries to be used to add include statements. Specifies 4531 * the path value of "<b>Consent:patient</b>". 4532 */ 4533 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Consent:patient").toLocked(); 4534 4535 4536} 4537