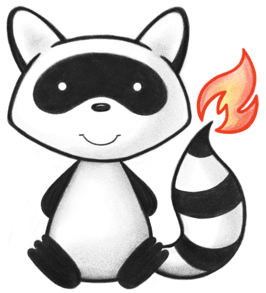
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a healthcare consumer?s choices or choices made on their behalf by a third party, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 052 */ 053@ResourceDef(name="Consent", profile="http://hl7.org/fhir/StructureDefinition/Consent") 054public class Consent extends DomainResource { 055 056 public enum ConsentState { 057 /** 058 * The consent is in development or awaiting use but is not yet intended to be acted upon. 059 */ 060 DRAFT, 061 /** 062 * The consent is to be followed and enforced. 063 */ 064 ACTIVE, 065 /** 066 * The consent is terminated or replaced. 067 */ 068 INACTIVE, 069 /** 070 * The consent development has been terminated prior to completion. 071 */ 072 NOTDONE, 073 /** 074 * The consent was created wrongly (e.g. wrong patient) and should be ignored. 075 */ 076 ENTEREDINERROR, 077 /** 078 * The resource is in an indeterminate state. 079 */ 080 UNKNOWN, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static ConsentState fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("draft".equals(codeString)) 089 return DRAFT; 090 if ("active".equals(codeString)) 091 return ACTIVE; 092 if ("inactive".equals(codeString)) 093 return INACTIVE; 094 if ("not-done".equals(codeString)) 095 return NOTDONE; 096 if ("entered-in-error".equals(codeString)) 097 return ENTEREDINERROR; 098 if ("unknown".equals(codeString)) 099 return UNKNOWN; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case DRAFT: return "draft"; 108 case ACTIVE: return "active"; 109 case INACTIVE: return "inactive"; 110 case NOTDONE: return "not-done"; 111 case ENTEREDINERROR: return "entered-in-error"; 112 case UNKNOWN: return "unknown"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getSystem() { 118 switch (this) { 119 case DRAFT: return "http://hl7.org/fhir/consent-state-codes"; 120 case ACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 121 case INACTIVE: return "http://hl7.org/fhir/consent-state-codes"; 122 case NOTDONE: return "http://hl7.org/fhir/consent-state-codes"; 123 case ENTEREDINERROR: return "http://hl7.org/fhir/consent-state-codes"; 124 case UNKNOWN: return "http://hl7.org/fhir/consent-state-codes"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDefinition() { 130 switch (this) { 131 case DRAFT: return "The consent is in development or awaiting use but is not yet intended to be acted upon."; 132 case ACTIVE: return "The consent is to be followed and enforced."; 133 case INACTIVE: return "The consent is terminated or replaced."; 134 case NOTDONE: return "The consent development has been terminated prior to completion."; 135 case ENTEREDINERROR: return "The consent was created wrongly (e.g. wrong patient) and should be ignored."; 136 case UNKNOWN: return "The resource is in an indeterminate state."; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getDisplay() { 142 switch (this) { 143 case DRAFT: return "Pending"; 144 case ACTIVE: return "Active"; 145 case INACTIVE: return "Inactive"; 146 case NOTDONE: return "Abandoned"; 147 case ENTEREDINERROR: return "Entered in Error"; 148 case UNKNOWN: return "Unknown"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 } 154 155 public static class ConsentStateEnumFactory implements EnumFactory<ConsentState> { 156 public ConsentState fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("draft".equals(codeString)) 161 return ConsentState.DRAFT; 162 if ("active".equals(codeString)) 163 return ConsentState.ACTIVE; 164 if ("inactive".equals(codeString)) 165 return ConsentState.INACTIVE; 166 if ("not-done".equals(codeString)) 167 return ConsentState.NOTDONE; 168 if ("entered-in-error".equals(codeString)) 169 return ConsentState.ENTEREDINERROR; 170 if ("unknown".equals(codeString)) 171 return ConsentState.UNKNOWN; 172 throw new IllegalArgumentException("Unknown ConsentState code '"+codeString+"'"); 173 } 174 public Enumeration<ConsentState> fromType(PrimitiveType<?> code) throws FHIRException { 175 if (code == null) 176 return null; 177 if (code.isEmpty()) 178 return new Enumeration<ConsentState>(this, ConsentState.NULL, code); 179 String codeString = ((PrimitiveType) code).asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return new Enumeration<ConsentState>(this, ConsentState.NULL, code); 182 if ("draft".equals(codeString)) 183 return new Enumeration<ConsentState>(this, ConsentState.DRAFT, code); 184 if ("active".equals(codeString)) 185 return new Enumeration<ConsentState>(this, ConsentState.ACTIVE, code); 186 if ("inactive".equals(codeString)) 187 return new Enumeration<ConsentState>(this, ConsentState.INACTIVE, code); 188 if ("not-done".equals(codeString)) 189 return new Enumeration<ConsentState>(this, ConsentState.NOTDONE, code); 190 if ("entered-in-error".equals(codeString)) 191 return new Enumeration<ConsentState>(this, ConsentState.ENTEREDINERROR, code); 192 if ("unknown".equals(codeString)) 193 return new Enumeration<ConsentState>(this, ConsentState.UNKNOWN, code); 194 throw new FHIRException("Unknown ConsentState code '"+codeString+"'"); 195 } 196 public String toCode(ConsentState code) { 197 if (code == ConsentState.DRAFT) 198 return "draft"; 199 if (code == ConsentState.ACTIVE) 200 return "active"; 201 if (code == ConsentState.INACTIVE) 202 return "inactive"; 203 if (code == ConsentState.NOTDONE) 204 return "not-done"; 205 if (code == ConsentState.ENTEREDINERROR) 206 return "entered-in-error"; 207 if (code == ConsentState.UNKNOWN) 208 return "unknown"; 209 return "?"; 210 } 211 public String toSystem(ConsentState code) { 212 return code.getSystem(); 213 } 214 } 215 216 @Block() 217 public static class ConsentPolicyBasisComponent extends BackboneElement implements IBaseBackboneElement { 218 /** 219 * A Reference that identifies the policy the organization will enforce for this Consent. 220 */ 221 @Child(name = "reference", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Reference backing policy resource", formalDefinition="A Reference that identifies the policy the organization will enforce for this Consent." ) 223 protected Reference reference; 224 225 /** 226 * A URL that links to a computable version of the policy the organization will enforce for this Consent. 227 */ 228 @Child(name = "url", type = {UrlType.class}, order=2, min=0, max=1, modifier=false, summary=false) 229 @Description(shortDefinition="URL to a computable backing policy", formalDefinition="A URL that links to a computable version of the policy the organization will enforce for this Consent." ) 230 protected UrlType url; 231 232 private static final long serialVersionUID = 252506182L; 233 234 /** 235 * Constructor 236 */ 237 public ConsentPolicyBasisComponent() { 238 super(); 239 } 240 241 /** 242 * @return {@link #reference} (A Reference that identifies the policy the organization will enforce for this Consent.) 243 */ 244 public Reference getReference() { 245 if (this.reference == null) 246 if (Configuration.errorOnAutoCreate()) 247 throw new Error("Attempt to auto-create ConsentPolicyBasisComponent.reference"); 248 else if (Configuration.doAutoCreate()) 249 this.reference = new Reference(); // cc 250 return this.reference; 251 } 252 253 public boolean hasReference() { 254 return this.reference != null && !this.reference.isEmpty(); 255 } 256 257 /** 258 * @param value {@link #reference} (A Reference that identifies the policy the organization will enforce for this Consent.) 259 */ 260 public ConsentPolicyBasisComponent setReference(Reference value) { 261 this.reference = value; 262 return this; 263 } 264 265 /** 266 * @return {@link #url} (A URL that links to a computable version of the policy the organization will enforce for this Consent.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 267 */ 268 public UrlType getUrlElement() { 269 if (this.url == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create ConsentPolicyBasisComponent.url"); 272 else if (Configuration.doAutoCreate()) 273 this.url = new UrlType(); // bb 274 return this.url; 275 } 276 277 public boolean hasUrlElement() { 278 return this.url != null && !this.url.isEmpty(); 279 } 280 281 public boolean hasUrl() { 282 return this.url != null && !this.url.isEmpty(); 283 } 284 285 /** 286 * @param value {@link #url} (A URL that links to a computable version of the policy the organization will enforce for this Consent.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 287 */ 288 public ConsentPolicyBasisComponent setUrlElement(UrlType value) { 289 this.url = value; 290 return this; 291 } 292 293 /** 294 * @return A URL that links to a computable version of the policy the organization will enforce for this Consent. 295 */ 296 public String getUrl() { 297 return this.url == null ? null : this.url.getValue(); 298 } 299 300 /** 301 * @param value A URL that links to a computable version of the policy the organization will enforce for this Consent. 302 */ 303 public ConsentPolicyBasisComponent setUrl(String value) { 304 if (Utilities.noString(value)) 305 this.url = null; 306 else { 307 if (this.url == null) 308 this.url = new UrlType(); 309 this.url.setValue(value); 310 } 311 return this; 312 } 313 314 protected void listChildren(List<Property> children) { 315 super.listChildren(children); 316 children.add(new Property("reference", "Reference(Any)", "A Reference that identifies the policy the organization will enforce for this Consent.", 0, 1, reference)); 317 children.add(new Property("url", "url", "A URL that links to a computable version of the policy the organization will enforce for this Consent.", 0, 1, url)); 318 } 319 320 @Override 321 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 322 switch (_hash) { 323 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A Reference that identifies the policy the organization will enforce for this Consent.", 0, 1, reference); 324 case 116079: /*url*/ return new Property("url", "url", "A URL that links to a computable version of the policy the organization will enforce for this Consent.", 0, 1, url); 325 default: return super.getNamedProperty(_hash, _name, _checkValid); 326 } 327 328 } 329 330 @Override 331 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 332 switch (hash) { 333 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 334 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 335 default: return super.getProperty(hash, name, checkValid); 336 } 337 338 } 339 340 @Override 341 public Base setProperty(int hash, String name, Base value) throws FHIRException { 342 switch (hash) { 343 case -925155509: // reference 344 this.reference = TypeConvertor.castToReference(value); // Reference 345 return value; 346 case 116079: // url 347 this.url = TypeConvertor.castToUrl(value); // UrlType 348 return value; 349 default: return super.setProperty(hash, name, value); 350 } 351 352 } 353 354 @Override 355 public Base setProperty(String name, Base value) throws FHIRException { 356 if (name.equals("reference")) { 357 this.reference = TypeConvertor.castToReference(value); // Reference 358 } else if (name.equals("url")) { 359 this.url = TypeConvertor.castToUrl(value); // UrlType 360 } else 361 return super.setProperty(name, value); 362 return value; 363 } 364 365 @Override 366 public void removeChild(String name, Base value) throws FHIRException { 367 if (name.equals("reference")) { 368 this.reference = null; 369 } else if (name.equals("url")) { 370 this.url = null; 371 } else 372 super.removeChild(name, value); 373 374 } 375 376 @Override 377 public Base makeProperty(int hash, String name) throws FHIRException { 378 switch (hash) { 379 case -925155509: return getReference(); 380 case 116079: return getUrlElement(); 381 default: return super.makeProperty(hash, name); 382 } 383 384 } 385 386 @Override 387 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 388 switch (hash) { 389 case -925155509: /*reference*/ return new String[] {"Reference"}; 390 case 116079: /*url*/ return new String[] {"url"}; 391 default: return super.getTypesForProperty(hash, name); 392 } 393 394 } 395 396 @Override 397 public Base addChild(String name) throws FHIRException { 398 if (name.equals("reference")) { 399 this.reference = new Reference(); 400 return this.reference; 401 } 402 else if (name.equals("url")) { 403 throw new FHIRException("Cannot call addChild on a singleton property Consent.policyBasis.url"); 404 } 405 else 406 return super.addChild(name); 407 } 408 409 public ConsentPolicyBasisComponent copy() { 410 ConsentPolicyBasisComponent dst = new ConsentPolicyBasisComponent(); 411 copyValues(dst); 412 return dst; 413 } 414 415 public void copyValues(ConsentPolicyBasisComponent dst) { 416 super.copyValues(dst); 417 dst.reference = reference == null ? null : reference.copy(); 418 dst.url = url == null ? null : url.copy(); 419 } 420 421 @Override 422 public boolean equalsDeep(Base other_) { 423 if (!super.equalsDeep(other_)) 424 return false; 425 if (!(other_ instanceof ConsentPolicyBasisComponent)) 426 return false; 427 ConsentPolicyBasisComponent o = (ConsentPolicyBasisComponent) other_; 428 return compareDeep(reference, o.reference, true) && compareDeep(url, o.url, true); 429 } 430 431 @Override 432 public boolean equalsShallow(Base other_) { 433 if (!super.equalsShallow(other_)) 434 return false; 435 if (!(other_ instanceof ConsentPolicyBasisComponent)) 436 return false; 437 ConsentPolicyBasisComponent o = (ConsentPolicyBasisComponent) other_; 438 return compareValues(url, o.url, true); 439 } 440 441 public boolean isEmpty() { 442 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, url); 443 } 444 445 public String fhirType() { 446 return "Consent.policyBasis"; 447 448 } 449 450 } 451 452 @Block() 453 public static class ConsentVerificationComponent extends BackboneElement implements IBaseBackboneElement { 454 /** 455 * Has the instruction been verified. 456 */ 457 @Child(name = "verified", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=true) 458 @Description(shortDefinition="Has been verified", formalDefinition="Has the instruction been verified." ) 459 protected BooleanType verified; 460 461 /** 462 * Extensible list of verification type starting with verification and re-validation. 463 */ 464 @Child(name = "verificationType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 465 @Description(shortDefinition="Business case of verification", formalDefinition="Extensible list of verification type starting with verification and re-validation." ) 466 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-verification") 467 protected CodeableConcept verificationType; 468 469 /** 470 * The person who conducted the verification/validation of the Grantor decision. 471 */ 472 @Child(name = "verifiedBy", type = {Organization.class, Practitioner.class, PractitionerRole.class}, order=3, min=0, max=1, modifier=false, summary=false) 473 @Description(shortDefinition="Person conducting verification", formalDefinition="The person who conducted the verification/validation of the Grantor decision." ) 474 protected Reference verifiedBy; 475 476 /** 477 * Who verified the instruction (Patient, Relative or other Authorized Person). 478 */ 479 @Child(name = "verifiedWith", type = {Patient.class, RelatedPerson.class}, order=4, min=0, max=1, modifier=false, summary=false) 480 @Description(shortDefinition="Person who verified", formalDefinition="Who verified the instruction (Patient, Relative or other Authorized Person)." ) 481 protected Reference verifiedWith; 482 483 /** 484 * Date(s) verification was collected. 485 */ 486 @Child(name = "verificationDate", type = {DateTimeType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 487 @Description(shortDefinition="When consent verified", formalDefinition="Date(s) verification was collected." ) 488 protected List<DateTimeType> verificationDate; 489 490 private static final long serialVersionUID = -1266157329L; 491 492 /** 493 * Constructor 494 */ 495 public ConsentVerificationComponent() { 496 super(); 497 } 498 499 /** 500 * Constructor 501 */ 502 public ConsentVerificationComponent(boolean verified) { 503 super(); 504 this.setVerified(verified); 505 } 506 507 /** 508 * @return {@link #verified} (Has the instruction been verified.). This is the underlying object with id, value and extensions. The accessor "getVerified" gives direct access to the value 509 */ 510 public BooleanType getVerifiedElement() { 511 if (this.verified == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create ConsentVerificationComponent.verified"); 514 else if (Configuration.doAutoCreate()) 515 this.verified = new BooleanType(); // bb 516 return this.verified; 517 } 518 519 public boolean hasVerifiedElement() { 520 return this.verified != null && !this.verified.isEmpty(); 521 } 522 523 public boolean hasVerified() { 524 return this.verified != null && !this.verified.isEmpty(); 525 } 526 527 /** 528 * @param value {@link #verified} (Has the instruction been verified.). This is the underlying object with id, value and extensions. The accessor "getVerified" gives direct access to the value 529 */ 530 public ConsentVerificationComponent setVerifiedElement(BooleanType value) { 531 this.verified = value; 532 return this; 533 } 534 535 /** 536 * @return Has the instruction been verified. 537 */ 538 public boolean getVerified() { 539 return this.verified == null || this.verified.isEmpty() ? false : this.verified.getValue(); 540 } 541 542 /** 543 * @param value Has the instruction been verified. 544 */ 545 public ConsentVerificationComponent setVerified(boolean value) { 546 if (this.verified == null) 547 this.verified = new BooleanType(); 548 this.verified.setValue(value); 549 return this; 550 } 551 552 /** 553 * @return {@link #verificationType} (Extensible list of verification type starting with verification and re-validation.) 554 */ 555 public CodeableConcept getVerificationType() { 556 if (this.verificationType == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create ConsentVerificationComponent.verificationType"); 559 else if (Configuration.doAutoCreate()) 560 this.verificationType = new CodeableConcept(); // cc 561 return this.verificationType; 562 } 563 564 public boolean hasVerificationType() { 565 return this.verificationType != null && !this.verificationType.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #verificationType} (Extensible list of verification type starting with verification and re-validation.) 570 */ 571 public ConsentVerificationComponent setVerificationType(CodeableConcept value) { 572 this.verificationType = value; 573 return this; 574 } 575 576 /** 577 * @return {@link #verifiedBy} (The person who conducted the verification/validation of the Grantor decision.) 578 */ 579 public Reference getVerifiedBy() { 580 if (this.verifiedBy == null) 581 if (Configuration.errorOnAutoCreate()) 582 throw new Error("Attempt to auto-create ConsentVerificationComponent.verifiedBy"); 583 else if (Configuration.doAutoCreate()) 584 this.verifiedBy = new Reference(); // cc 585 return this.verifiedBy; 586 } 587 588 public boolean hasVerifiedBy() { 589 return this.verifiedBy != null && !this.verifiedBy.isEmpty(); 590 } 591 592 /** 593 * @param value {@link #verifiedBy} (The person who conducted the verification/validation of the Grantor decision.) 594 */ 595 public ConsentVerificationComponent setVerifiedBy(Reference value) { 596 this.verifiedBy = value; 597 return this; 598 } 599 600 /** 601 * @return {@link #verifiedWith} (Who verified the instruction (Patient, Relative or other Authorized Person).) 602 */ 603 public Reference getVerifiedWith() { 604 if (this.verifiedWith == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create ConsentVerificationComponent.verifiedWith"); 607 else if (Configuration.doAutoCreate()) 608 this.verifiedWith = new Reference(); // cc 609 return this.verifiedWith; 610 } 611 612 public boolean hasVerifiedWith() { 613 return this.verifiedWith != null && !this.verifiedWith.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #verifiedWith} (Who verified the instruction (Patient, Relative or other Authorized Person).) 618 */ 619 public ConsentVerificationComponent setVerifiedWith(Reference value) { 620 this.verifiedWith = value; 621 return this; 622 } 623 624 /** 625 * @return {@link #verificationDate} (Date(s) verification was collected.) 626 */ 627 public List<DateTimeType> getVerificationDate() { 628 if (this.verificationDate == null) 629 this.verificationDate = new ArrayList<DateTimeType>(); 630 return this.verificationDate; 631 } 632 633 /** 634 * @return Returns a reference to <code>this</code> for easy method chaining 635 */ 636 public ConsentVerificationComponent setVerificationDate(List<DateTimeType> theVerificationDate) { 637 this.verificationDate = theVerificationDate; 638 return this; 639 } 640 641 public boolean hasVerificationDate() { 642 if (this.verificationDate == null) 643 return false; 644 for (DateTimeType item : this.verificationDate) 645 if (!item.isEmpty()) 646 return true; 647 return false; 648 } 649 650 /** 651 * @return {@link #verificationDate} (Date(s) verification was collected.) 652 */ 653 public DateTimeType addVerificationDateElement() {//2 654 DateTimeType t = new DateTimeType(); 655 if (this.verificationDate == null) 656 this.verificationDate = new ArrayList<DateTimeType>(); 657 this.verificationDate.add(t); 658 return t; 659 } 660 661 /** 662 * @param value {@link #verificationDate} (Date(s) verification was collected.) 663 */ 664 public ConsentVerificationComponent addVerificationDate(Date value) { //1 665 DateTimeType t = new DateTimeType(); 666 t.setValue(value); 667 if (this.verificationDate == null) 668 this.verificationDate = new ArrayList<DateTimeType>(); 669 this.verificationDate.add(t); 670 return this; 671 } 672 673 /** 674 * @param value {@link #verificationDate} (Date(s) verification was collected.) 675 */ 676 public boolean hasVerificationDate(Date value) { 677 if (this.verificationDate == null) 678 return false; 679 for (DateTimeType v : this.verificationDate) 680 if (v.getValue().equals(value)) // dateTime 681 return true; 682 return false; 683 } 684 685 protected void listChildren(List<Property> children) { 686 super.listChildren(children); 687 children.add(new Property("verified", "boolean", "Has the instruction been verified.", 0, 1, verified)); 688 children.add(new Property("verificationType", "CodeableConcept", "Extensible list of verification type starting with verification and re-validation.", 0, 1, verificationType)); 689 children.add(new Property("verifiedBy", "Reference(Organization|Practitioner|PractitionerRole)", "The person who conducted the verification/validation of the Grantor decision.", 0, 1, verifiedBy)); 690 children.add(new Property("verifiedWith", "Reference(Patient|RelatedPerson)", "Who verified the instruction (Patient, Relative or other Authorized Person).", 0, 1, verifiedWith)); 691 children.add(new Property("verificationDate", "dateTime", "Date(s) verification was collected.", 0, java.lang.Integer.MAX_VALUE, verificationDate)); 692 } 693 694 @Override 695 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 696 switch (_hash) { 697 case -1994383672: /*verified*/ return new Property("verified", "boolean", "Has the instruction been verified.", 0, 1, verified); 698 case 642733045: /*verificationType*/ return new Property("verificationType", "CodeableConcept", "Extensible list of verification type starting with verification and re-validation.", 0, 1, verificationType); 699 case -1047292609: /*verifiedBy*/ return new Property("verifiedBy", "Reference(Organization|Practitioner|PractitionerRole)", "The person who conducted the verification/validation of the Grantor decision.", 0, 1, verifiedBy); 700 case -1425236050: /*verifiedWith*/ return new Property("verifiedWith", "Reference(Patient|RelatedPerson)", "Who verified the instruction (Patient, Relative or other Authorized Person).", 0, 1, verifiedWith); 701 case 642233449: /*verificationDate*/ return new Property("verificationDate", "dateTime", "Date(s) verification was collected.", 0, java.lang.Integer.MAX_VALUE, verificationDate); 702 default: return super.getNamedProperty(_hash, _name, _checkValid); 703 } 704 705 } 706 707 @Override 708 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 709 switch (hash) { 710 case -1994383672: /*verified*/ return this.verified == null ? new Base[0] : new Base[] {this.verified}; // BooleanType 711 case 642733045: /*verificationType*/ return this.verificationType == null ? new Base[0] : new Base[] {this.verificationType}; // CodeableConcept 712 case -1047292609: /*verifiedBy*/ return this.verifiedBy == null ? new Base[0] : new Base[] {this.verifiedBy}; // Reference 713 case -1425236050: /*verifiedWith*/ return this.verifiedWith == null ? new Base[0] : new Base[] {this.verifiedWith}; // Reference 714 case 642233449: /*verificationDate*/ return this.verificationDate == null ? new Base[0] : this.verificationDate.toArray(new Base[this.verificationDate.size()]); // DateTimeType 715 default: return super.getProperty(hash, name, checkValid); 716 } 717 718 } 719 720 @Override 721 public Base setProperty(int hash, String name, Base value) throws FHIRException { 722 switch (hash) { 723 case -1994383672: // verified 724 this.verified = TypeConvertor.castToBoolean(value); // BooleanType 725 return value; 726 case 642733045: // verificationType 727 this.verificationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 728 return value; 729 case -1047292609: // verifiedBy 730 this.verifiedBy = TypeConvertor.castToReference(value); // Reference 731 return value; 732 case -1425236050: // verifiedWith 733 this.verifiedWith = TypeConvertor.castToReference(value); // Reference 734 return value; 735 case 642233449: // verificationDate 736 this.getVerificationDate().add(TypeConvertor.castToDateTime(value)); // DateTimeType 737 return value; 738 default: return super.setProperty(hash, name, value); 739 } 740 741 } 742 743 @Override 744 public Base setProperty(String name, Base value) throws FHIRException { 745 if (name.equals("verified")) { 746 this.verified = TypeConvertor.castToBoolean(value); // BooleanType 747 } else if (name.equals("verificationType")) { 748 this.verificationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 749 } else if (name.equals("verifiedBy")) { 750 this.verifiedBy = TypeConvertor.castToReference(value); // Reference 751 } else if (name.equals("verifiedWith")) { 752 this.verifiedWith = TypeConvertor.castToReference(value); // Reference 753 } else if (name.equals("verificationDate")) { 754 this.getVerificationDate().add(TypeConvertor.castToDateTime(value)); 755 } else 756 return super.setProperty(name, value); 757 return value; 758 } 759 760 @Override 761 public void removeChild(String name, Base value) throws FHIRException { 762 if (name.equals("verified")) { 763 this.verified = null; 764 } else if (name.equals("verificationType")) { 765 this.verificationType = null; 766 } else if (name.equals("verifiedBy")) { 767 this.verifiedBy = null; 768 } else if (name.equals("verifiedWith")) { 769 this.verifiedWith = null; 770 } else if (name.equals("verificationDate")) { 771 this.getVerificationDate().remove(value); 772 } else 773 super.removeChild(name, value); 774 775 } 776 777 @Override 778 public Base makeProperty(int hash, String name) throws FHIRException { 779 switch (hash) { 780 case -1994383672: return getVerifiedElement(); 781 case 642733045: return getVerificationType(); 782 case -1047292609: return getVerifiedBy(); 783 case -1425236050: return getVerifiedWith(); 784 case 642233449: return addVerificationDateElement(); 785 default: return super.makeProperty(hash, name); 786 } 787 788 } 789 790 @Override 791 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 792 switch (hash) { 793 case -1994383672: /*verified*/ return new String[] {"boolean"}; 794 case 642733045: /*verificationType*/ return new String[] {"CodeableConcept"}; 795 case -1047292609: /*verifiedBy*/ return new String[] {"Reference"}; 796 case -1425236050: /*verifiedWith*/ return new String[] {"Reference"}; 797 case 642233449: /*verificationDate*/ return new String[] {"dateTime"}; 798 default: return super.getTypesForProperty(hash, name); 799 } 800 801 } 802 803 @Override 804 public Base addChild(String name) throws FHIRException { 805 if (name.equals("verified")) { 806 throw new FHIRException("Cannot call addChild on a singleton property Consent.verification.verified"); 807 } 808 else if (name.equals("verificationType")) { 809 this.verificationType = new CodeableConcept(); 810 return this.verificationType; 811 } 812 else if (name.equals("verifiedBy")) { 813 this.verifiedBy = new Reference(); 814 return this.verifiedBy; 815 } 816 else if (name.equals("verifiedWith")) { 817 this.verifiedWith = new Reference(); 818 return this.verifiedWith; 819 } 820 else if (name.equals("verificationDate")) { 821 throw new FHIRException("Cannot call addChild on a singleton property Consent.verification.verificationDate"); 822 } 823 else 824 return super.addChild(name); 825 } 826 827 public ConsentVerificationComponent copy() { 828 ConsentVerificationComponent dst = new ConsentVerificationComponent(); 829 copyValues(dst); 830 return dst; 831 } 832 833 public void copyValues(ConsentVerificationComponent dst) { 834 super.copyValues(dst); 835 dst.verified = verified == null ? null : verified.copy(); 836 dst.verificationType = verificationType == null ? null : verificationType.copy(); 837 dst.verifiedBy = verifiedBy == null ? null : verifiedBy.copy(); 838 dst.verifiedWith = verifiedWith == null ? null : verifiedWith.copy(); 839 if (verificationDate != null) { 840 dst.verificationDate = new ArrayList<DateTimeType>(); 841 for (DateTimeType i : verificationDate) 842 dst.verificationDate.add(i.copy()); 843 }; 844 } 845 846 @Override 847 public boolean equalsDeep(Base other_) { 848 if (!super.equalsDeep(other_)) 849 return false; 850 if (!(other_ instanceof ConsentVerificationComponent)) 851 return false; 852 ConsentVerificationComponent o = (ConsentVerificationComponent) other_; 853 return compareDeep(verified, o.verified, true) && compareDeep(verificationType, o.verificationType, true) 854 && compareDeep(verifiedBy, o.verifiedBy, true) && compareDeep(verifiedWith, o.verifiedWith, true) 855 && compareDeep(verificationDate, o.verificationDate, true); 856 } 857 858 @Override 859 public boolean equalsShallow(Base other_) { 860 if (!super.equalsShallow(other_)) 861 return false; 862 if (!(other_ instanceof ConsentVerificationComponent)) 863 return false; 864 ConsentVerificationComponent o = (ConsentVerificationComponent) other_; 865 return compareValues(verified, o.verified, true) && compareValues(verificationDate, o.verificationDate, true) 866 ; 867 } 868 869 public boolean isEmpty() { 870 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(verified, verificationType 871 , verifiedBy, verifiedWith, verificationDate); 872 } 873 874 public String fhirType() { 875 return "Consent.verification"; 876 877 } 878 879 } 880 881 @Block() 882 public static class ProvisionComponent extends BackboneElement implements IBaseBackboneElement { 883 /** 884 * Timeframe for this provision. 885 */ 886 @Child(name = "period", type = {Period.class}, order=1, min=0, max=1, modifier=false, summary=true) 887 @Description(shortDefinition="Timeframe for this provision", formalDefinition="Timeframe for this provision." ) 888 protected Period period; 889 890 /** 891 * Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 892 */ 893 @Child(name = "actor", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 894 @Description(shortDefinition="Who|what controlled by this provision (or group, by role)", formalDefinition="Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 895 protected List<ProvisionActorComponent> actor; 896 897 /** 898 * Actions controlled by this provision. 899 */ 900 @Child(name = "action", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 901 @Description(shortDefinition="Actions controlled by this provision", formalDefinition="Actions controlled by this provision." ) 902 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-action") 903 protected List<CodeableConcept> action; 904 905 /** 906 * A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception. 907 */ 908 @Child(name = "securityLabel", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 909 @Description(shortDefinition="Security Labels that define affected resources", formalDefinition="A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception." ) 910 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-label-examples") 911 protected List<Coding> securityLabel; 912 913 /** 914 * The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision. 915 */ 916 @Child(name = "purpose", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 917 @Description(shortDefinition="Context of activities covered by this provision", formalDefinition="The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision." ) 918 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 919 protected List<Coding> purpose; 920 921 /** 922 * The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to. 923 */ 924 @Child(name = "documentType", type = {Coding.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 925 @Description(shortDefinition="e.g. Resource Type, Profile, CDA, etc", formalDefinition="The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to." ) 926 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-class") 927 protected List<Coding> documentType; 928 929 /** 930 * The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to. 931 */ 932 @Child(name = "resourceType", type = {Coding.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 933 @Description(shortDefinition="e.g. Resource Type, Profile, etc", formalDefinition="The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to." ) 934 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 935 protected List<Coding> resourceType; 936 937 /** 938 * If this code is found in an instance, then the provision applies. 939 */ 940 @Child(name = "code", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 941 @Description(shortDefinition="e.g. LOINC or SNOMED CT code, etc. in the content", formalDefinition="If this code is found in an instance, then the provision applies." ) 942 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-code") 943 protected List<CodeableConcept> code; 944 945 /** 946 * Clinical or Operational Relevant period of time that bounds the data controlled by this provision. 947 */ 948 @Child(name = "dataPeriod", type = {Period.class}, order=9, min=0, max=1, modifier=false, summary=true) 949 @Description(shortDefinition="Timeframe for data controlled by this provision", formalDefinition="Clinical or Operational Relevant period of time that bounds the data controlled by this provision." ) 950 protected Period dataPeriod; 951 952 /** 953 * The resources controlled by this provision if specific resources are referenced. 954 */ 955 @Child(name = "data", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 956 @Description(shortDefinition="Data controlled by this provision", formalDefinition="The resources controlled by this provision if specific resources are referenced." ) 957 protected List<ProvisionDataComponent> data; 958 959 /** 960 * A computable (FHIRPath or other) definition of what is controlled by this consent. 961 */ 962 @Child(name = "expression", type = {Expression.class}, order=11, min=0, max=1, modifier=false, summary=false) 963 @Description(shortDefinition="A computable expression of the consent", formalDefinition="A computable (FHIRPath or other) definition of what is controlled by this consent." ) 964 protected Expression expression; 965 966 /** 967 * Provisions which provide exceptions to the base provision or subprovisions. 968 */ 969 @Child(name = "provision", type = {ProvisionComponent.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 970 @Description(shortDefinition="Nested Exception Provisions", formalDefinition="Provisions which provide exceptions to the base provision or subprovisions." ) 971 protected List<ProvisionComponent> provision; 972 973 private static final long serialVersionUID = -587666915L; 974 975 /** 976 * Constructor 977 */ 978 public ProvisionComponent() { 979 super(); 980 } 981 982 /** 983 * @return {@link #period} (Timeframe for this provision.) 984 */ 985 public Period getPeriod() { 986 if (this.period == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create ProvisionComponent.period"); 989 else if (Configuration.doAutoCreate()) 990 this.period = new Period(); // cc 991 return this.period; 992 } 993 994 public boolean hasPeriod() { 995 return this.period != null && !this.period.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #period} (Timeframe for this provision.) 1000 */ 1001 public ProvisionComponent setPeriod(Period value) { 1002 this.period = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #actor} (Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1008 */ 1009 public List<ProvisionActorComponent> getActor() { 1010 if (this.actor == null) 1011 this.actor = new ArrayList<ProvisionActorComponent>(); 1012 return this.actor; 1013 } 1014 1015 /** 1016 * @return Returns a reference to <code>this</code> for easy method chaining 1017 */ 1018 public ProvisionComponent setActor(List<ProvisionActorComponent> theActor) { 1019 this.actor = theActor; 1020 return this; 1021 } 1022 1023 public boolean hasActor() { 1024 if (this.actor == null) 1025 return false; 1026 for (ProvisionActorComponent item : this.actor) 1027 if (!item.isEmpty()) 1028 return true; 1029 return false; 1030 } 1031 1032 public ProvisionActorComponent addActor() { //3 1033 ProvisionActorComponent t = new ProvisionActorComponent(); 1034 if (this.actor == null) 1035 this.actor = new ArrayList<ProvisionActorComponent>(); 1036 this.actor.add(t); 1037 return t; 1038 } 1039 1040 public ProvisionComponent addActor(ProvisionActorComponent t) { //3 1041 if (t == null) 1042 return this; 1043 if (this.actor == null) 1044 this.actor = new ArrayList<ProvisionActorComponent>(); 1045 this.actor.add(t); 1046 return this; 1047 } 1048 1049 /** 1050 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist {3} 1051 */ 1052 public ProvisionActorComponent getActorFirstRep() { 1053 if (getActor().isEmpty()) { 1054 addActor(); 1055 } 1056 return getActor().get(0); 1057 } 1058 1059 /** 1060 * @return {@link #action} (Actions controlled by this provision.) 1061 */ 1062 public List<CodeableConcept> getAction() { 1063 if (this.action == null) 1064 this.action = new ArrayList<CodeableConcept>(); 1065 return this.action; 1066 } 1067 1068 /** 1069 * @return Returns a reference to <code>this</code> for easy method chaining 1070 */ 1071 public ProvisionComponent setAction(List<CodeableConcept> theAction) { 1072 this.action = theAction; 1073 return this; 1074 } 1075 1076 public boolean hasAction() { 1077 if (this.action == null) 1078 return false; 1079 for (CodeableConcept item : this.action) 1080 if (!item.isEmpty()) 1081 return true; 1082 return false; 1083 } 1084 1085 public CodeableConcept addAction() { //3 1086 CodeableConcept t = new CodeableConcept(); 1087 if (this.action == null) 1088 this.action = new ArrayList<CodeableConcept>(); 1089 this.action.add(t); 1090 return t; 1091 } 1092 1093 public ProvisionComponent addAction(CodeableConcept t) { //3 1094 if (t == null) 1095 return this; 1096 if (this.action == null) 1097 this.action = new ArrayList<CodeableConcept>(); 1098 this.action.add(t); 1099 return this; 1100 } 1101 1102 /** 1103 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 1104 */ 1105 public CodeableConcept getActionFirstRep() { 1106 if (getAction().isEmpty()) { 1107 addAction(); 1108 } 1109 return getAction().get(0); 1110 } 1111 1112 /** 1113 * @return {@link #securityLabel} (A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.) 1114 */ 1115 public List<Coding> getSecurityLabel() { 1116 if (this.securityLabel == null) 1117 this.securityLabel = new ArrayList<Coding>(); 1118 return this.securityLabel; 1119 } 1120 1121 /** 1122 * @return Returns a reference to <code>this</code> for easy method chaining 1123 */ 1124 public ProvisionComponent setSecurityLabel(List<Coding> theSecurityLabel) { 1125 this.securityLabel = theSecurityLabel; 1126 return this; 1127 } 1128 1129 public boolean hasSecurityLabel() { 1130 if (this.securityLabel == null) 1131 return false; 1132 for (Coding item : this.securityLabel) 1133 if (!item.isEmpty()) 1134 return true; 1135 return false; 1136 } 1137 1138 public Coding addSecurityLabel() { //3 1139 Coding t = new Coding(); 1140 if (this.securityLabel == null) 1141 this.securityLabel = new ArrayList<Coding>(); 1142 this.securityLabel.add(t); 1143 return t; 1144 } 1145 1146 public ProvisionComponent addSecurityLabel(Coding t) { //3 1147 if (t == null) 1148 return this; 1149 if (this.securityLabel == null) 1150 this.securityLabel = new ArrayList<Coding>(); 1151 this.securityLabel.add(t); 1152 return this; 1153 } 1154 1155 /** 1156 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist {3} 1157 */ 1158 public Coding getSecurityLabelFirstRep() { 1159 if (getSecurityLabel().isEmpty()) { 1160 addSecurityLabel(); 1161 } 1162 return getSecurityLabel().get(0); 1163 } 1164 1165 /** 1166 * @return {@link #purpose} (The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision.) 1167 */ 1168 public List<Coding> getPurpose() { 1169 if (this.purpose == null) 1170 this.purpose = new ArrayList<Coding>(); 1171 return this.purpose; 1172 } 1173 1174 /** 1175 * @return Returns a reference to <code>this</code> for easy method chaining 1176 */ 1177 public ProvisionComponent setPurpose(List<Coding> thePurpose) { 1178 this.purpose = thePurpose; 1179 return this; 1180 } 1181 1182 public boolean hasPurpose() { 1183 if (this.purpose == null) 1184 return false; 1185 for (Coding item : this.purpose) 1186 if (!item.isEmpty()) 1187 return true; 1188 return false; 1189 } 1190 1191 public Coding addPurpose() { //3 1192 Coding t = new Coding(); 1193 if (this.purpose == null) 1194 this.purpose = new ArrayList<Coding>(); 1195 this.purpose.add(t); 1196 return t; 1197 } 1198 1199 public ProvisionComponent addPurpose(Coding t) { //3 1200 if (t == null) 1201 return this; 1202 if (this.purpose == null) 1203 this.purpose = new ArrayList<Coding>(); 1204 this.purpose.add(t); 1205 return this; 1206 } 1207 1208 /** 1209 * @return The first repetition of repeating field {@link #purpose}, creating it if it does not already exist {3} 1210 */ 1211 public Coding getPurposeFirstRep() { 1212 if (getPurpose().isEmpty()) { 1213 addPurpose(); 1214 } 1215 return getPurpose().get(0); 1216 } 1217 1218 /** 1219 * @return {@link #documentType} (The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to.) 1220 */ 1221 public List<Coding> getDocumentType() { 1222 if (this.documentType == null) 1223 this.documentType = new ArrayList<Coding>(); 1224 return this.documentType; 1225 } 1226 1227 /** 1228 * @return Returns a reference to <code>this</code> for easy method chaining 1229 */ 1230 public ProvisionComponent setDocumentType(List<Coding> theDocumentType) { 1231 this.documentType = theDocumentType; 1232 return this; 1233 } 1234 1235 public boolean hasDocumentType() { 1236 if (this.documentType == null) 1237 return false; 1238 for (Coding item : this.documentType) 1239 if (!item.isEmpty()) 1240 return true; 1241 return false; 1242 } 1243 1244 public Coding addDocumentType() { //3 1245 Coding t = new Coding(); 1246 if (this.documentType == null) 1247 this.documentType = new ArrayList<Coding>(); 1248 this.documentType.add(t); 1249 return t; 1250 } 1251 1252 public ProvisionComponent addDocumentType(Coding t) { //3 1253 if (t == null) 1254 return this; 1255 if (this.documentType == null) 1256 this.documentType = new ArrayList<Coding>(); 1257 this.documentType.add(t); 1258 return this; 1259 } 1260 1261 /** 1262 * @return The first repetition of repeating field {@link #documentType}, creating it if it does not already exist {3} 1263 */ 1264 public Coding getDocumentTypeFirstRep() { 1265 if (getDocumentType().isEmpty()) { 1266 addDocumentType(); 1267 } 1268 return getDocumentType().get(0); 1269 } 1270 1271 /** 1272 * @return {@link #resourceType} (The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to.) 1273 */ 1274 public List<Coding> getResourceType() { 1275 if (this.resourceType == null) 1276 this.resourceType = new ArrayList<Coding>(); 1277 return this.resourceType; 1278 } 1279 1280 /** 1281 * @return Returns a reference to <code>this</code> for easy method chaining 1282 */ 1283 public ProvisionComponent setResourceType(List<Coding> theResourceType) { 1284 this.resourceType = theResourceType; 1285 return this; 1286 } 1287 1288 public boolean hasResourceType() { 1289 if (this.resourceType == null) 1290 return false; 1291 for (Coding item : this.resourceType) 1292 if (!item.isEmpty()) 1293 return true; 1294 return false; 1295 } 1296 1297 public Coding addResourceType() { //3 1298 Coding t = new Coding(); 1299 if (this.resourceType == null) 1300 this.resourceType = new ArrayList<Coding>(); 1301 this.resourceType.add(t); 1302 return t; 1303 } 1304 1305 public ProvisionComponent addResourceType(Coding t) { //3 1306 if (t == null) 1307 return this; 1308 if (this.resourceType == null) 1309 this.resourceType = new ArrayList<Coding>(); 1310 this.resourceType.add(t); 1311 return this; 1312 } 1313 1314 /** 1315 * @return The first repetition of repeating field {@link #resourceType}, creating it if it does not already exist {3} 1316 */ 1317 public Coding getResourceTypeFirstRep() { 1318 if (getResourceType().isEmpty()) { 1319 addResourceType(); 1320 } 1321 return getResourceType().get(0); 1322 } 1323 1324 /** 1325 * @return {@link #code} (If this code is found in an instance, then the provision applies.) 1326 */ 1327 public List<CodeableConcept> getCode() { 1328 if (this.code == null) 1329 this.code = new ArrayList<CodeableConcept>(); 1330 return this.code; 1331 } 1332 1333 /** 1334 * @return Returns a reference to <code>this</code> for easy method chaining 1335 */ 1336 public ProvisionComponent setCode(List<CodeableConcept> theCode) { 1337 this.code = theCode; 1338 return this; 1339 } 1340 1341 public boolean hasCode() { 1342 if (this.code == null) 1343 return false; 1344 for (CodeableConcept item : this.code) 1345 if (!item.isEmpty()) 1346 return true; 1347 return false; 1348 } 1349 1350 public CodeableConcept addCode() { //3 1351 CodeableConcept t = new CodeableConcept(); 1352 if (this.code == null) 1353 this.code = new ArrayList<CodeableConcept>(); 1354 this.code.add(t); 1355 return t; 1356 } 1357 1358 public ProvisionComponent addCode(CodeableConcept t) { //3 1359 if (t == null) 1360 return this; 1361 if (this.code == null) 1362 this.code = new ArrayList<CodeableConcept>(); 1363 this.code.add(t); 1364 return this; 1365 } 1366 1367 /** 1368 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 1369 */ 1370 public CodeableConcept getCodeFirstRep() { 1371 if (getCode().isEmpty()) { 1372 addCode(); 1373 } 1374 return getCode().get(0); 1375 } 1376 1377 /** 1378 * @return {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this provision.) 1379 */ 1380 public Period getDataPeriod() { 1381 if (this.dataPeriod == null) 1382 if (Configuration.errorOnAutoCreate()) 1383 throw new Error("Attempt to auto-create ProvisionComponent.dataPeriod"); 1384 else if (Configuration.doAutoCreate()) 1385 this.dataPeriod = new Period(); // cc 1386 return this.dataPeriod; 1387 } 1388 1389 public boolean hasDataPeriod() { 1390 return this.dataPeriod != null && !this.dataPeriod.isEmpty(); 1391 } 1392 1393 /** 1394 * @param value {@link #dataPeriod} (Clinical or Operational Relevant period of time that bounds the data controlled by this provision.) 1395 */ 1396 public ProvisionComponent setDataPeriod(Period value) { 1397 this.dataPeriod = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #data} (The resources controlled by this provision if specific resources are referenced.) 1403 */ 1404 public List<ProvisionDataComponent> getData() { 1405 if (this.data == null) 1406 this.data = new ArrayList<ProvisionDataComponent>(); 1407 return this.data; 1408 } 1409 1410 /** 1411 * @return Returns a reference to <code>this</code> for easy method chaining 1412 */ 1413 public ProvisionComponent setData(List<ProvisionDataComponent> theData) { 1414 this.data = theData; 1415 return this; 1416 } 1417 1418 public boolean hasData() { 1419 if (this.data == null) 1420 return false; 1421 for (ProvisionDataComponent item : this.data) 1422 if (!item.isEmpty()) 1423 return true; 1424 return false; 1425 } 1426 1427 public ProvisionDataComponent addData() { //3 1428 ProvisionDataComponent t = new ProvisionDataComponent(); 1429 if (this.data == null) 1430 this.data = new ArrayList<ProvisionDataComponent>(); 1431 this.data.add(t); 1432 return t; 1433 } 1434 1435 public ProvisionComponent addData(ProvisionDataComponent t) { //3 1436 if (t == null) 1437 return this; 1438 if (this.data == null) 1439 this.data = new ArrayList<ProvisionDataComponent>(); 1440 this.data.add(t); 1441 return this; 1442 } 1443 1444 /** 1445 * @return The first repetition of repeating field {@link #data}, creating it if it does not already exist {3} 1446 */ 1447 public ProvisionDataComponent getDataFirstRep() { 1448 if (getData().isEmpty()) { 1449 addData(); 1450 } 1451 return getData().get(0); 1452 } 1453 1454 /** 1455 * @return {@link #expression} (A computable (FHIRPath or other) definition of what is controlled by this consent.) 1456 */ 1457 public Expression getExpression() { 1458 if (this.expression == null) 1459 if (Configuration.errorOnAutoCreate()) 1460 throw new Error("Attempt to auto-create ProvisionComponent.expression"); 1461 else if (Configuration.doAutoCreate()) 1462 this.expression = new Expression(); // cc 1463 return this.expression; 1464 } 1465 1466 public boolean hasExpression() { 1467 return this.expression != null && !this.expression.isEmpty(); 1468 } 1469 1470 /** 1471 * @param value {@link #expression} (A computable (FHIRPath or other) definition of what is controlled by this consent.) 1472 */ 1473 public ProvisionComponent setExpression(Expression value) { 1474 this.expression = value; 1475 return this; 1476 } 1477 1478 /** 1479 * @return {@link #provision} (Provisions which provide exceptions to the base provision or subprovisions.) 1480 */ 1481 public List<ProvisionComponent> getProvision() { 1482 if (this.provision == null) 1483 this.provision = new ArrayList<ProvisionComponent>(); 1484 return this.provision; 1485 } 1486 1487 /** 1488 * @return Returns a reference to <code>this</code> for easy method chaining 1489 */ 1490 public ProvisionComponent setProvision(List<ProvisionComponent> theProvision) { 1491 this.provision = theProvision; 1492 return this; 1493 } 1494 1495 public boolean hasProvision() { 1496 if (this.provision == null) 1497 return false; 1498 for (ProvisionComponent item : this.provision) 1499 if (!item.isEmpty()) 1500 return true; 1501 return false; 1502 } 1503 1504 public ProvisionComponent addProvision() { //3 1505 ProvisionComponent t = new ProvisionComponent(); 1506 if (this.provision == null) 1507 this.provision = new ArrayList<ProvisionComponent>(); 1508 this.provision.add(t); 1509 return t; 1510 } 1511 1512 public ProvisionComponent addProvision(ProvisionComponent t) { //3 1513 if (t == null) 1514 return this; 1515 if (this.provision == null) 1516 this.provision = new ArrayList<ProvisionComponent>(); 1517 this.provision.add(t); 1518 return this; 1519 } 1520 1521 /** 1522 * @return The first repetition of repeating field {@link #provision}, creating it if it does not already exist {3} 1523 */ 1524 public ProvisionComponent getProvisionFirstRep() { 1525 if (getProvision().isEmpty()) { 1526 addProvision(); 1527 } 1528 return getProvision().get(0); 1529 } 1530 1531 protected void listChildren(List<Property> children) { 1532 super.listChildren(children); 1533 children.add(new Property("period", "Period", "Timeframe for this provision.", 0, 1, period)); 1534 children.add(new Property("actor", "", "Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor)); 1535 children.add(new Property("action", "CodeableConcept", "Actions controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, action)); 1536 children.add(new Property("securityLabel", "Coding", "A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 1537 children.add(new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, purpose)); 1538 children.add(new Property("documentType", "Coding", "The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, documentType)); 1539 children.add(new Property("resourceType", "Coding", "The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, resourceType)); 1540 children.add(new Property("code", "CodeableConcept", "If this code is found in an instance, then the provision applies.", 0, java.lang.Integer.MAX_VALUE, code)); 1541 children.add(new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this provision.", 0, 1, dataPeriod)); 1542 children.add(new Property("data", "", "The resources controlled by this provision if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data)); 1543 children.add(new Property("expression", "Expression", "A computable (FHIRPath or other) definition of what is controlled by this consent.", 0, 1, expression)); 1544 children.add(new Property("provision", "@Consent.provision", "Provisions which provide exceptions to the base provision or subprovisions.", 0, java.lang.Integer.MAX_VALUE, provision)); 1545 } 1546 1547 @Override 1548 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1549 switch (_hash) { 1550 case -991726143: /*period*/ return new Property("period", "Period", "Timeframe for this provision.", 0, 1, period); 1551 case 92645877: /*actor*/ return new Property("actor", "", "Who or what is controlled by this provision. Use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, java.lang.Integer.MAX_VALUE, actor); 1552 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Actions controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, action); 1553 case -722296940: /*securityLabel*/ return new Property("securityLabel", "Coding", "A security label, comprised of 0..* security label fields (Privacy tags), which define which resources are controlled by this exception.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 1554 case -220463842: /*purpose*/ return new Property("purpose", "Coding", "The context of the activities a user is taking - why the user is accessing the data - that are controlled by this provision.", 0, java.lang.Integer.MAX_VALUE, purpose); 1555 case -1473196299: /*documentType*/ return new Property("documentType", "Coding", "The documentType(s) covered by this provision. The type can be a CDA document, or some other type that indicates what sort of information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, documentType); 1556 case -384364440: /*resourceType*/ return new Property("resourceType", "Coding", "The resourceType(s) covered by this provision. The type can be a FHIR resource type or a profile on a type that indicates what information the consent relates to.", 0, java.lang.Integer.MAX_VALUE, resourceType); 1557 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "If this code is found in an instance, then the provision applies.", 0, java.lang.Integer.MAX_VALUE, code); 1558 case 1177250315: /*dataPeriod*/ return new Property("dataPeriod", "Period", "Clinical or Operational Relevant period of time that bounds the data controlled by this provision.", 0, 1, dataPeriod); 1559 case 3076010: /*data*/ return new Property("data", "", "The resources controlled by this provision if specific resources are referenced.", 0, java.lang.Integer.MAX_VALUE, data); 1560 case -1795452264: /*expression*/ return new Property("expression", "Expression", "A computable (FHIRPath or other) definition of what is controlled by this consent.", 0, 1, expression); 1561 case -547120939: /*provision*/ return new Property("provision", "@Consent.provision", "Provisions which provide exceptions to the base provision or subprovisions.", 0, java.lang.Integer.MAX_VALUE, provision); 1562 default: return super.getNamedProperty(_hash, _name, _checkValid); 1563 } 1564 1565 } 1566 1567 @Override 1568 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1569 switch (hash) { 1570 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1571 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ProvisionActorComponent 1572 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // CodeableConcept 1573 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // Coding 1574 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Coding 1575 case -1473196299: /*documentType*/ return this.documentType == null ? new Base[0] : this.documentType.toArray(new Base[this.documentType.size()]); // Coding 1576 case -384364440: /*resourceType*/ return this.resourceType == null ? new Base[0] : this.resourceType.toArray(new Base[this.resourceType.size()]); // Coding 1577 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1578 case 1177250315: /*dataPeriod*/ return this.dataPeriod == null ? new Base[0] : new Base[] {this.dataPeriod}; // Period 1579 case 3076010: /*data*/ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // ProvisionDataComponent 1580 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 1581 case -547120939: /*provision*/ return this.provision == null ? new Base[0] : this.provision.toArray(new Base[this.provision.size()]); // ProvisionComponent 1582 default: return super.getProperty(hash, name, checkValid); 1583 } 1584 1585 } 1586 1587 @Override 1588 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1589 switch (hash) { 1590 case -991726143: // period 1591 this.period = TypeConvertor.castToPeriod(value); // Period 1592 return value; 1593 case 92645877: // actor 1594 this.getActor().add((ProvisionActorComponent) value); // ProvisionActorComponent 1595 return value; 1596 case -1422950858: // action 1597 this.getAction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1598 return value; 1599 case -722296940: // securityLabel 1600 this.getSecurityLabel().add(TypeConvertor.castToCoding(value)); // Coding 1601 return value; 1602 case -220463842: // purpose 1603 this.getPurpose().add(TypeConvertor.castToCoding(value)); // Coding 1604 return value; 1605 case -1473196299: // documentType 1606 this.getDocumentType().add(TypeConvertor.castToCoding(value)); // Coding 1607 return value; 1608 case -384364440: // resourceType 1609 this.getResourceType().add(TypeConvertor.castToCoding(value)); // Coding 1610 return value; 1611 case 3059181: // code 1612 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1613 return value; 1614 case 1177250315: // dataPeriod 1615 this.dataPeriod = TypeConvertor.castToPeriod(value); // Period 1616 return value; 1617 case 3076010: // data 1618 this.getData().add((ProvisionDataComponent) value); // ProvisionDataComponent 1619 return value; 1620 case -1795452264: // expression 1621 this.expression = TypeConvertor.castToExpression(value); // Expression 1622 return value; 1623 case -547120939: // provision 1624 this.getProvision().add((ProvisionComponent) value); // ProvisionComponent 1625 return value; 1626 default: return super.setProperty(hash, name, value); 1627 } 1628 1629 } 1630 1631 @Override 1632 public Base setProperty(String name, Base value) throws FHIRException { 1633 if (name.equals("period")) { 1634 this.period = TypeConvertor.castToPeriod(value); // Period 1635 } else if (name.equals("actor")) { 1636 this.getActor().add((ProvisionActorComponent) value); 1637 } else if (name.equals("action")) { 1638 this.getAction().add(TypeConvertor.castToCodeableConcept(value)); 1639 } else if (name.equals("securityLabel")) { 1640 this.getSecurityLabel().add(TypeConvertor.castToCoding(value)); 1641 } else if (name.equals("purpose")) { 1642 this.getPurpose().add(TypeConvertor.castToCoding(value)); 1643 } else if (name.equals("documentType")) { 1644 this.getDocumentType().add(TypeConvertor.castToCoding(value)); 1645 } else if (name.equals("resourceType")) { 1646 this.getResourceType().add(TypeConvertor.castToCoding(value)); 1647 } else if (name.equals("code")) { 1648 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 1649 } else if (name.equals("dataPeriod")) { 1650 this.dataPeriod = TypeConvertor.castToPeriod(value); // Period 1651 } else if (name.equals("data")) { 1652 this.getData().add((ProvisionDataComponent) value); 1653 } else if (name.equals("expression")) { 1654 this.expression = TypeConvertor.castToExpression(value); // Expression 1655 } else if (name.equals("provision")) { 1656 this.getProvision().add((ProvisionComponent) value); 1657 } else 1658 return super.setProperty(name, value); 1659 return value; 1660 } 1661 1662 @Override 1663 public void removeChild(String name, Base value) throws FHIRException { 1664 if (name.equals("period")) { 1665 this.period = null; 1666 } else if (name.equals("actor")) { 1667 this.getActor().remove((ProvisionActorComponent) value); 1668 } else if (name.equals("action")) { 1669 this.getAction().remove(value); 1670 } else if (name.equals("securityLabel")) { 1671 this.getSecurityLabel().remove(value); 1672 } else if (name.equals("purpose")) { 1673 this.getPurpose().remove(value); 1674 } else if (name.equals("documentType")) { 1675 this.getDocumentType().remove(value); 1676 } else if (name.equals("resourceType")) { 1677 this.getResourceType().remove(value); 1678 } else if (name.equals("code")) { 1679 this.getCode().remove(value); 1680 } else if (name.equals("dataPeriod")) { 1681 this.dataPeriod = null; 1682 } else if (name.equals("data")) { 1683 this.getData().remove((ProvisionDataComponent) value); 1684 } else if (name.equals("expression")) { 1685 this.expression = null; 1686 } else if (name.equals("provision")) { 1687 this.getProvision().remove((ProvisionComponent) value); 1688 } else 1689 super.removeChild(name, value); 1690 1691 } 1692 1693 @Override 1694 public Base makeProperty(int hash, String name) throws FHIRException { 1695 switch (hash) { 1696 case -991726143: return getPeriod(); 1697 case 92645877: return addActor(); 1698 case -1422950858: return addAction(); 1699 case -722296940: return addSecurityLabel(); 1700 case -220463842: return addPurpose(); 1701 case -1473196299: return addDocumentType(); 1702 case -384364440: return addResourceType(); 1703 case 3059181: return addCode(); 1704 case 1177250315: return getDataPeriod(); 1705 case 3076010: return addData(); 1706 case -1795452264: return getExpression(); 1707 case -547120939: return addProvision(); 1708 default: return super.makeProperty(hash, name); 1709 } 1710 1711 } 1712 1713 @Override 1714 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1715 switch (hash) { 1716 case -991726143: /*period*/ return new String[] {"Period"}; 1717 case 92645877: /*actor*/ return new String[] {}; 1718 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 1719 case -722296940: /*securityLabel*/ return new String[] {"Coding"}; 1720 case -220463842: /*purpose*/ return new String[] {"Coding"}; 1721 case -1473196299: /*documentType*/ return new String[] {"Coding"}; 1722 case -384364440: /*resourceType*/ return new String[] {"Coding"}; 1723 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1724 case 1177250315: /*dataPeriod*/ return new String[] {"Period"}; 1725 case 3076010: /*data*/ return new String[] {}; 1726 case -1795452264: /*expression*/ return new String[] {"Expression"}; 1727 case -547120939: /*provision*/ return new String[] {"@Consent.provision"}; 1728 default: return super.getTypesForProperty(hash, name); 1729 } 1730 1731 } 1732 1733 @Override 1734 public Base addChild(String name) throws FHIRException { 1735 if (name.equals("period")) { 1736 this.period = new Period(); 1737 return this.period; 1738 } 1739 else if (name.equals("actor")) { 1740 return addActor(); 1741 } 1742 else if (name.equals("action")) { 1743 return addAction(); 1744 } 1745 else if (name.equals("securityLabel")) { 1746 return addSecurityLabel(); 1747 } 1748 else if (name.equals("purpose")) { 1749 return addPurpose(); 1750 } 1751 else if (name.equals("documentType")) { 1752 return addDocumentType(); 1753 } 1754 else if (name.equals("resourceType")) { 1755 return addResourceType(); 1756 } 1757 else if (name.equals("code")) { 1758 return addCode(); 1759 } 1760 else if (name.equals("dataPeriod")) { 1761 this.dataPeriod = new Period(); 1762 return this.dataPeriod; 1763 } 1764 else if (name.equals("data")) { 1765 return addData(); 1766 } 1767 else if (name.equals("expression")) { 1768 this.expression = new Expression(); 1769 return this.expression; 1770 } 1771 else if (name.equals("provision")) { 1772 return addProvision(); 1773 } 1774 else 1775 return super.addChild(name); 1776 } 1777 1778 public ProvisionComponent copy() { 1779 ProvisionComponent dst = new ProvisionComponent(); 1780 copyValues(dst); 1781 return dst; 1782 } 1783 1784 public void copyValues(ProvisionComponent dst) { 1785 super.copyValues(dst); 1786 dst.period = period == null ? null : period.copy(); 1787 if (actor != null) { 1788 dst.actor = new ArrayList<ProvisionActorComponent>(); 1789 for (ProvisionActorComponent i : actor) 1790 dst.actor.add(i.copy()); 1791 }; 1792 if (action != null) { 1793 dst.action = new ArrayList<CodeableConcept>(); 1794 for (CodeableConcept i : action) 1795 dst.action.add(i.copy()); 1796 }; 1797 if (securityLabel != null) { 1798 dst.securityLabel = new ArrayList<Coding>(); 1799 for (Coding i : securityLabel) 1800 dst.securityLabel.add(i.copy()); 1801 }; 1802 if (purpose != null) { 1803 dst.purpose = new ArrayList<Coding>(); 1804 for (Coding i : purpose) 1805 dst.purpose.add(i.copy()); 1806 }; 1807 if (documentType != null) { 1808 dst.documentType = new ArrayList<Coding>(); 1809 for (Coding i : documentType) 1810 dst.documentType.add(i.copy()); 1811 }; 1812 if (resourceType != null) { 1813 dst.resourceType = new ArrayList<Coding>(); 1814 for (Coding i : resourceType) 1815 dst.resourceType.add(i.copy()); 1816 }; 1817 if (code != null) { 1818 dst.code = new ArrayList<CodeableConcept>(); 1819 for (CodeableConcept i : code) 1820 dst.code.add(i.copy()); 1821 }; 1822 dst.dataPeriod = dataPeriod == null ? null : dataPeriod.copy(); 1823 if (data != null) { 1824 dst.data = new ArrayList<ProvisionDataComponent>(); 1825 for (ProvisionDataComponent i : data) 1826 dst.data.add(i.copy()); 1827 }; 1828 dst.expression = expression == null ? null : expression.copy(); 1829 if (provision != null) { 1830 dst.provision = new ArrayList<ProvisionComponent>(); 1831 for (ProvisionComponent i : provision) 1832 dst.provision.add(i.copy()); 1833 }; 1834 } 1835 1836 @Override 1837 public boolean equalsDeep(Base other_) { 1838 if (!super.equalsDeep(other_)) 1839 return false; 1840 if (!(other_ instanceof ProvisionComponent)) 1841 return false; 1842 ProvisionComponent o = (ProvisionComponent) other_; 1843 return compareDeep(period, o.period, true) && compareDeep(actor, o.actor, true) && compareDeep(action, o.action, true) 1844 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(purpose, o.purpose, true) && compareDeep(documentType, o.documentType, true) 1845 && compareDeep(resourceType, o.resourceType, true) && compareDeep(code, o.code, true) && compareDeep(dataPeriod, o.dataPeriod, true) 1846 && compareDeep(data, o.data, true) && compareDeep(expression, o.expression, true) && compareDeep(provision, o.provision, true) 1847 ; 1848 } 1849 1850 @Override 1851 public boolean equalsShallow(Base other_) { 1852 if (!super.equalsShallow(other_)) 1853 return false; 1854 if (!(other_ instanceof ProvisionComponent)) 1855 return false; 1856 ProvisionComponent o = (ProvisionComponent) other_; 1857 return true; 1858 } 1859 1860 public boolean isEmpty() { 1861 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(period, actor, action, securityLabel 1862 , purpose, documentType, resourceType, code, dataPeriod, data, expression, provision 1863 ); 1864 } 1865 1866 public String fhirType() { 1867 return "Consent.provision"; 1868 1869 } 1870 1871 } 1872 1873 @Block() 1874 public static class ProvisionActorComponent extends BackboneElement implements IBaseBackboneElement { 1875 /** 1876 * How the individual is involved in the resources content that is described in the exception. 1877 */ 1878 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1879 @Description(shortDefinition="How the actor is involved", formalDefinition="How the individual is involved in the resources content that is described in the exception." ) 1880 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 1881 protected CodeableConcept role; 1882 1883 /** 1884 * The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers'). 1885 */ 1886 @Child(name = "reference", type = {Device.class, Group.class, CareTeam.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, PractitionerRole.class}, order=2, min=0, max=1, modifier=false, summary=false) 1887 @Description(shortDefinition="Resource for the actor (or group, by role)", formalDefinition="The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers')." ) 1888 protected Reference reference; 1889 1890 private static final long serialVersionUID = -1992921787L; 1891 1892 /** 1893 * Constructor 1894 */ 1895 public ProvisionActorComponent() { 1896 super(); 1897 } 1898 1899 /** 1900 * @return {@link #role} (How the individual is involved in the resources content that is described in the exception.) 1901 */ 1902 public CodeableConcept getRole() { 1903 if (this.role == null) 1904 if (Configuration.errorOnAutoCreate()) 1905 throw new Error("Attempt to auto-create ProvisionActorComponent.role"); 1906 else if (Configuration.doAutoCreate()) 1907 this.role = new CodeableConcept(); // cc 1908 return this.role; 1909 } 1910 1911 public boolean hasRole() { 1912 return this.role != null && !this.role.isEmpty(); 1913 } 1914 1915 /** 1916 * @param value {@link #role} (How the individual is involved in the resources content that is described in the exception.) 1917 */ 1918 public ProvisionActorComponent setRole(CodeableConcept value) { 1919 this.role = value; 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #reference} (The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1925 */ 1926 public Reference getReference() { 1927 if (this.reference == null) 1928 if (Configuration.errorOnAutoCreate()) 1929 throw new Error("Attempt to auto-create ProvisionActorComponent.reference"); 1930 else if (Configuration.doAutoCreate()) 1931 this.reference = new Reference(); // cc 1932 return this.reference; 1933 } 1934 1935 public boolean hasReference() { 1936 return this.reference != null && !this.reference.isEmpty(); 1937 } 1938 1939 /** 1940 * @param value {@link #reference} (The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').) 1941 */ 1942 public ProvisionActorComponent setReference(Reference value) { 1943 this.reference = value; 1944 return this; 1945 } 1946 1947 protected void listChildren(List<Property> children) { 1948 super.listChildren(children); 1949 children.add(new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role)); 1950 children.add(new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference)); 1951 } 1952 1953 @Override 1954 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1955 switch (_hash) { 1956 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "How the individual is involved in the resources content that is described in the exception.", 0, 1, role); 1957 case -925155509: /*reference*/ return new Property("reference", "Reference(Device|Group|CareTeam|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The resource that identifies the actor. To identify actors by type, use group to identify a set of actors by some property they share (e.g. 'admitting officers').", 0, 1, reference); 1958 default: return super.getNamedProperty(_hash, _name, _checkValid); 1959 } 1960 1961 } 1962 1963 @Override 1964 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1965 switch (hash) { 1966 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1967 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 1968 default: return super.getProperty(hash, name, checkValid); 1969 } 1970 1971 } 1972 1973 @Override 1974 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1975 switch (hash) { 1976 case 3506294: // role 1977 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1978 return value; 1979 case -925155509: // reference 1980 this.reference = TypeConvertor.castToReference(value); // Reference 1981 return value; 1982 default: return super.setProperty(hash, name, value); 1983 } 1984 1985 } 1986 1987 @Override 1988 public Base setProperty(String name, Base value) throws FHIRException { 1989 if (name.equals("role")) { 1990 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1991 } else if (name.equals("reference")) { 1992 this.reference = TypeConvertor.castToReference(value); // Reference 1993 } else 1994 return super.setProperty(name, value); 1995 return value; 1996 } 1997 1998 @Override 1999 public void removeChild(String name, Base value) throws FHIRException { 2000 if (name.equals("role")) { 2001 this.role = null; 2002 } else if (name.equals("reference")) { 2003 this.reference = null; 2004 } else 2005 super.removeChild(name, value); 2006 2007 } 2008 2009 @Override 2010 public Base makeProperty(int hash, String name) throws FHIRException { 2011 switch (hash) { 2012 case 3506294: return getRole(); 2013 case -925155509: return getReference(); 2014 default: return super.makeProperty(hash, name); 2015 } 2016 2017 } 2018 2019 @Override 2020 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2021 switch (hash) { 2022 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2023 case -925155509: /*reference*/ return new String[] {"Reference"}; 2024 default: return super.getTypesForProperty(hash, name); 2025 } 2026 2027 } 2028 2029 @Override 2030 public Base addChild(String name) throws FHIRException { 2031 if (name.equals("role")) { 2032 this.role = new CodeableConcept(); 2033 return this.role; 2034 } 2035 else if (name.equals("reference")) { 2036 this.reference = new Reference(); 2037 return this.reference; 2038 } 2039 else 2040 return super.addChild(name); 2041 } 2042 2043 public ProvisionActorComponent copy() { 2044 ProvisionActorComponent dst = new ProvisionActorComponent(); 2045 copyValues(dst); 2046 return dst; 2047 } 2048 2049 public void copyValues(ProvisionActorComponent dst) { 2050 super.copyValues(dst); 2051 dst.role = role == null ? null : role.copy(); 2052 dst.reference = reference == null ? null : reference.copy(); 2053 } 2054 2055 @Override 2056 public boolean equalsDeep(Base other_) { 2057 if (!super.equalsDeep(other_)) 2058 return false; 2059 if (!(other_ instanceof ProvisionActorComponent)) 2060 return false; 2061 ProvisionActorComponent o = (ProvisionActorComponent) other_; 2062 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true); 2063 } 2064 2065 @Override 2066 public boolean equalsShallow(Base other_) { 2067 if (!super.equalsShallow(other_)) 2068 return false; 2069 if (!(other_ instanceof ProvisionActorComponent)) 2070 return false; 2071 ProvisionActorComponent o = (ProvisionActorComponent) other_; 2072 return true; 2073 } 2074 2075 public boolean isEmpty() { 2076 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, reference); 2077 } 2078 2079 public String fhirType() { 2080 return "Consent.provision.actor"; 2081 2082 } 2083 2084 } 2085 2086 @Block() 2087 public static class ProvisionDataComponent extends BackboneElement implements IBaseBackboneElement { 2088 /** 2089 * How the resource reference is interpreted when testing consent restrictions. 2090 */ 2091 @Child(name = "meaning", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2092 @Description(shortDefinition="instance | related | dependents | authoredby", formalDefinition="How the resource reference is interpreted when testing consent restrictions." ) 2093 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-data-meaning") 2094 protected Enumeration<ConsentDataMeaning> meaning; 2095 2096 /** 2097 * A reference to a specific resource that defines which resources are covered by this consent. 2098 */ 2099 @Child(name = "reference", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 2100 @Description(shortDefinition="The actual data reference", formalDefinition="A reference to a specific resource that defines which resources are covered by this consent." ) 2101 protected Reference reference; 2102 2103 private static final long serialVersionUID = 1735979153L; 2104 2105 /** 2106 * Constructor 2107 */ 2108 public ProvisionDataComponent() { 2109 super(); 2110 } 2111 2112 /** 2113 * Constructor 2114 */ 2115 public ProvisionDataComponent(ConsentDataMeaning meaning, Reference reference) { 2116 super(); 2117 this.setMeaning(meaning); 2118 this.setReference(reference); 2119 } 2120 2121 /** 2122 * @return {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2123 */ 2124 public Enumeration<ConsentDataMeaning> getMeaningElement() { 2125 if (this.meaning == null) 2126 if (Configuration.errorOnAutoCreate()) 2127 throw new Error("Attempt to auto-create ProvisionDataComponent.meaning"); 2128 else if (Configuration.doAutoCreate()) 2129 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); // bb 2130 return this.meaning; 2131 } 2132 2133 public boolean hasMeaningElement() { 2134 return this.meaning != null && !this.meaning.isEmpty(); 2135 } 2136 2137 public boolean hasMeaning() { 2138 return this.meaning != null && !this.meaning.isEmpty(); 2139 } 2140 2141 /** 2142 * @param value {@link #meaning} (How the resource reference is interpreted when testing consent restrictions.). This is the underlying object with id, value and extensions. The accessor "getMeaning" gives direct access to the value 2143 */ 2144 public ProvisionDataComponent setMeaningElement(Enumeration<ConsentDataMeaning> value) { 2145 this.meaning = value; 2146 return this; 2147 } 2148 2149 /** 2150 * @return How the resource reference is interpreted when testing consent restrictions. 2151 */ 2152 public ConsentDataMeaning getMeaning() { 2153 return this.meaning == null ? null : this.meaning.getValue(); 2154 } 2155 2156 /** 2157 * @param value How the resource reference is interpreted when testing consent restrictions. 2158 */ 2159 public ProvisionDataComponent setMeaning(ConsentDataMeaning value) { 2160 if (this.meaning == null) 2161 this.meaning = new Enumeration<ConsentDataMeaning>(new ConsentDataMeaningEnumFactory()); 2162 this.meaning.setValue(value); 2163 return this; 2164 } 2165 2166 /** 2167 * @return {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2168 */ 2169 public Reference getReference() { 2170 if (this.reference == null) 2171 if (Configuration.errorOnAutoCreate()) 2172 throw new Error("Attempt to auto-create ProvisionDataComponent.reference"); 2173 else if (Configuration.doAutoCreate()) 2174 this.reference = new Reference(); // cc 2175 return this.reference; 2176 } 2177 2178 public boolean hasReference() { 2179 return this.reference != null && !this.reference.isEmpty(); 2180 } 2181 2182 /** 2183 * @param value {@link #reference} (A reference to a specific resource that defines which resources are covered by this consent.) 2184 */ 2185 public ProvisionDataComponent setReference(Reference value) { 2186 this.reference = value; 2187 return this; 2188 } 2189 2190 protected void listChildren(List<Property> children) { 2191 super.listChildren(children); 2192 children.add(new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning)); 2193 children.add(new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference)); 2194 } 2195 2196 @Override 2197 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2198 switch (_hash) { 2199 case 938160637: /*meaning*/ return new Property("meaning", "code", "How the resource reference is interpreted when testing consent restrictions.", 0, 1, meaning); 2200 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "A reference to a specific resource that defines which resources are covered by this consent.", 0, 1, reference); 2201 default: return super.getNamedProperty(_hash, _name, _checkValid); 2202 } 2203 2204 } 2205 2206 @Override 2207 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2208 switch (hash) { 2209 case 938160637: /*meaning*/ return this.meaning == null ? new Base[0] : new Base[] {this.meaning}; // Enumeration<ConsentDataMeaning> 2210 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2211 default: return super.getProperty(hash, name, checkValid); 2212 } 2213 2214 } 2215 2216 @Override 2217 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2218 switch (hash) { 2219 case 938160637: // meaning 2220 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2221 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2222 return value; 2223 case -925155509: // reference 2224 this.reference = TypeConvertor.castToReference(value); // Reference 2225 return value; 2226 default: return super.setProperty(hash, name, value); 2227 } 2228 2229 } 2230 2231 @Override 2232 public Base setProperty(String name, Base value) throws FHIRException { 2233 if (name.equals("meaning")) { 2234 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2235 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2236 } else if (name.equals("reference")) { 2237 this.reference = TypeConvertor.castToReference(value); // Reference 2238 } else 2239 return super.setProperty(name, value); 2240 return value; 2241 } 2242 2243 @Override 2244 public void removeChild(String name, Base value) throws FHIRException { 2245 if (name.equals("meaning")) { 2246 value = new ConsentDataMeaningEnumFactory().fromType(TypeConvertor.castToCode(value)); 2247 this.meaning = (Enumeration) value; // Enumeration<ConsentDataMeaning> 2248 } else if (name.equals("reference")) { 2249 this.reference = null; 2250 } else 2251 super.removeChild(name, value); 2252 2253 } 2254 2255 @Override 2256 public Base makeProperty(int hash, String name) throws FHIRException { 2257 switch (hash) { 2258 case 938160637: return getMeaningElement(); 2259 case -925155509: return getReference(); 2260 default: return super.makeProperty(hash, name); 2261 } 2262 2263 } 2264 2265 @Override 2266 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2267 switch (hash) { 2268 case 938160637: /*meaning*/ return new String[] {"code"}; 2269 case -925155509: /*reference*/ return new String[] {"Reference"}; 2270 default: return super.getTypesForProperty(hash, name); 2271 } 2272 2273 } 2274 2275 @Override 2276 public Base addChild(String name) throws FHIRException { 2277 if (name.equals("meaning")) { 2278 throw new FHIRException("Cannot call addChild on a singleton property Consent.provision.data.meaning"); 2279 } 2280 else if (name.equals("reference")) { 2281 this.reference = new Reference(); 2282 return this.reference; 2283 } 2284 else 2285 return super.addChild(name); 2286 } 2287 2288 public ProvisionDataComponent copy() { 2289 ProvisionDataComponent dst = new ProvisionDataComponent(); 2290 copyValues(dst); 2291 return dst; 2292 } 2293 2294 public void copyValues(ProvisionDataComponent dst) { 2295 super.copyValues(dst); 2296 dst.meaning = meaning == null ? null : meaning.copy(); 2297 dst.reference = reference == null ? null : reference.copy(); 2298 } 2299 2300 @Override 2301 public boolean equalsDeep(Base other_) { 2302 if (!super.equalsDeep(other_)) 2303 return false; 2304 if (!(other_ instanceof ProvisionDataComponent)) 2305 return false; 2306 ProvisionDataComponent o = (ProvisionDataComponent) other_; 2307 return compareDeep(meaning, o.meaning, true) && compareDeep(reference, o.reference, true); 2308 } 2309 2310 @Override 2311 public boolean equalsShallow(Base other_) { 2312 if (!super.equalsShallow(other_)) 2313 return false; 2314 if (!(other_ instanceof ProvisionDataComponent)) 2315 return false; 2316 ProvisionDataComponent o = (ProvisionDataComponent) other_; 2317 return compareValues(meaning, o.meaning, true); 2318 } 2319 2320 public boolean isEmpty() { 2321 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(meaning, reference); 2322 } 2323 2324 public String fhirType() { 2325 return "Consent.provision.data"; 2326 2327 } 2328 2329 } 2330 2331 /** 2332 * Unique identifier for this copy of the Consent Statement. 2333 */ 2334 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2335 @Description(shortDefinition="Identifier for this record (external references)", formalDefinition="Unique identifier for this copy of the Consent Statement." ) 2336 protected List<Identifier> identifier; 2337 2338 /** 2339 * Indicates the current state of this Consent resource. 2340 */ 2341 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2342 @Description(shortDefinition="draft | active | inactive | not-done | entered-in-error | unknown", formalDefinition="Indicates the current state of this Consent resource." ) 2343 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-state-codes") 2344 protected Enumeration<ConsentState> status; 2345 2346 /** 2347 * A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements. 2348 */ 2349 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2350 @Description(shortDefinition="Classification of the consent statement - for indexing/retrieval", formalDefinition="A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements." ) 2351 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-category") 2352 protected List<CodeableConcept> category; 2353 2354 /** 2355 * The patient/healthcare practitioner or group of persons to whom this consent applies. 2356 */ 2357 @Child(name = "subject", type = {Patient.class, Practitioner.class, Group.class}, order=3, min=0, max=1, modifier=false, summary=true) 2358 @Description(shortDefinition="Who the consent applies to", formalDefinition="The patient/healthcare practitioner or group of persons to whom this consent applies." ) 2359 protected Reference subject; 2360 2361 /** 2362 * Date the consent instance was agreed to. 2363 */ 2364 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2365 @Description(shortDefinition="Fully executed date of the consent", formalDefinition="Date the consent instance was agreed to." ) 2366 protected DateType date; 2367 2368 /** 2369 * Effective period for this Consent Resource and all provisions unless specified in that provision. 2370 */ 2371 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 2372 @Description(shortDefinition="Effective period for this Consent", formalDefinition="Effective period for this Consent Resource and all provisions unless specified in that provision." ) 2373 protected Period period; 2374 2375 /** 2376 * The entity responsible for granting the rights listed in a Consent Directive. 2377 */ 2378 @Child(name = "grantor", type = {CareTeam.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, PractitionerRole.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2379 @Description(shortDefinition="Who is granting rights according to the policy and rules", formalDefinition="The entity responsible for granting the rights listed in a Consent Directive." ) 2380 protected List<Reference> grantor; 2381 2382 /** 2383 * The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions. 2384 */ 2385 @Child(name = "grantee", type = {CareTeam.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class, PractitionerRole.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2386 @Description(shortDefinition="Who is agreeing to the policy and rules", formalDefinition="The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions." ) 2387 protected List<Reference> grantee; 2388 2389 /** 2390 * The actor that manages the consent through its lifecycle. 2391 */ 2392 @Child(name = "manager", type = {HealthcareService.class, Organization.class, Patient.class, Practitioner.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2393 @Description(shortDefinition="Consent workflow management", formalDefinition="The actor that manages the consent through its lifecycle." ) 2394 protected List<Reference> manager; 2395 2396 /** 2397 * The actor that controls/enforces the access according to the consent. 2398 */ 2399 @Child(name = "controller", type = {HealthcareService.class, Organization.class, Patient.class, Practitioner.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2400 @Description(shortDefinition="Consent Enforcer", formalDefinition="The actor that controls/enforces the access according to the consent." ) 2401 protected List<Reference> controller; 2402 2403 /** 2404 * The source on which this consent statement is based. The source might be a scanned original paper form. 2405 */ 2406 @Child(name = "sourceAttachment", type = {Attachment.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2407 @Description(shortDefinition="Source from which this consent is taken", formalDefinition="The source on which this consent statement is based. The source might be a scanned original paper form." ) 2408 protected List<Attachment> sourceAttachment; 2409 2410 /** 2411 * A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document. 2412 */ 2413 @Child(name = "sourceReference", type = {Consent.class, DocumentReference.class, Contract.class, QuestionnaireResponse.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2414 @Description(shortDefinition="Source from which this consent is taken", formalDefinition="A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document." ) 2415 protected List<Reference> sourceReference; 2416 2417 /** 2418 * A set of codes that indicate the regulatory basis (if any) that this consent supports. 2419 */ 2420 @Child(name = "regulatoryBasis", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2421 @Description(shortDefinition="Regulations establishing base Consent", formalDefinition="A set of codes that indicate the regulatory basis (if any) that this consent supports." ) 2422 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-policy") 2423 protected List<CodeableConcept> regulatoryBasis; 2424 2425 /** 2426 * A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form. 2427 */ 2428 @Child(name = "policyBasis", type = {}, order=13, min=0, max=1, modifier=false, summary=false) 2429 @Description(shortDefinition="Computable version of the backing policy", formalDefinition="A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form." ) 2430 protected ConsentPolicyBasisComponent policyBasis; 2431 2432 /** 2433 * A Reference to the human readable policy explaining the basis for the Consent. 2434 */ 2435 @Child(name = "policyText", type = {DocumentReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2436 @Description(shortDefinition="Human Readable Policy", formalDefinition="A Reference to the human readable policy explaining the basis for the Consent." ) 2437 protected List<Reference> policyText; 2438 2439 /** 2440 * Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person. 2441 */ 2442 @Child(name = "verification", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2443 @Description(shortDefinition="Consent Verified by patient or family", formalDefinition="Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person." ) 2444 protected List<ConsentVerificationComponent> verification; 2445 2446 /** 2447 * Action to take - permit or deny - as default. 2448 */ 2449 @Child(name = "decision", type = {CodeType.class}, order=16, min=0, max=1, modifier=true, summary=true) 2450 @Description(shortDefinition="deny | permit", formalDefinition="Action to take - permit or deny - as default." ) 2451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-provision-type") 2452 protected Enumeration<ConsentProvisionType> decision; 2453 2454 /** 2455 * An exception to the base policy of this consent. An exception can be an addition or removal of access permissions. 2456 */ 2457 @Child(name = "provision", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2458 @Description(shortDefinition="Constraints to the base Consent.policyRule/Consent.policy", formalDefinition="An exception to the base policy of this consent. An exception can be an addition or removal of access permissions." ) 2459 protected List<ProvisionComponent> provision; 2460 2461 private static final long serialVersionUID = -1133978742L; 2462 2463 /** 2464 * Constructor 2465 */ 2466 public Consent() { 2467 super(); 2468 } 2469 2470 /** 2471 * Constructor 2472 */ 2473 public Consent(ConsentState status) { 2474 super(); 2475 this.setStatus(status); 2476 } 2477 2478 /** 2479 * @return {@link #identifier} (Unique identifier for this copy of the Consent Statement.) 2480 */ 2481 public List<Identifier> getIdentifier() { 2482 if (this.identifier == null) 2483 this.identifier = new ArrayList<Identifier>(); 2484 return this.identifier; 2485 } 2486 2487 /** 2488 * @return Returns a reference to <code>this</code> for easy method chaining 2489 */ 2490 public Consent setIdentifier(List<Identifier> theIdentifier) { 2491 this.identifier = theIdentifier; 2492 return this; 2493 } 2494 2495 public boolean hasIdentifier() { 2496 if (this.identifier == null) 2497 return false; 2498 for (Identifier item : this.identifier) 2499 if (!item.isEmpty()) 2500 return true; 2501 return false; 2502 } 2503 2504 public Identifier addIdentifier() { //3 2505 Identifier t = new Identifier(); 2506 if (this.identifier == null) 2507 this.identifier = new ArrayList<Identifier>(); 2508 this.identifier.add(t); 2509 return t; 2510 } 2511 2512 public Consent addIdentifier(Identifier t) { //3 2513 if (t == null) 2514 return this; 2515 if (this.identifier == null) 2516 this.identifier = new ArrayList<Identifier>(); 2517 this.identifier.add(t); 2518 return this; 2519 } 2520 2521 /** 2522 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2523 */ 2524 public Identifier getIdentifierFirstRep() { 2525 if (getIdentifier().isEmpty()) { 2526 addIdentifier(); 2527 } 2528 return getIdentifier().get(0); 2529 } 2530 2531 /** 2532 * @return {@link #status} (Indicates the current state of this Consent resource.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2533 */ 2534 public Enumeration<ConsentState> getStatusElement() { 2535 if (this.status == null) 2536 if (Configuration.errorOnAutoCreate()) 2537 throw new Error("Attempt to auto-create Consent.status"); 2538 else if (Configuration.doAutoCreate()) 2539 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); // bb 2540 return this.status; 2541 } 2542 2543 public boolean hasStatusElement() { 2544 return this.status != null && !this.status.isEmpty(); 2545 } 2546 2547 public boolean hasStatus() { 2548 return this.status != null && !this.status.isEmpty(); 2549 } 2550 2551 /** 2552 * @param value {@link #status} (Indicates the current state of this Consent resource.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2553 */ 2554 public Consent setStatusElement(Enumeration<ConsentState> value) { 2555 this.status = value; 2556 return this; 2557 } 2558 2559 /** 2560 * @return Indicates the current state of this Consent resource. 2561 */ 2562 public ConsentState getStatus() { 2563 return this.status == null ? null : this.status.getValue(); 2564 } 2565 2566 /** 2567 * @param value Indicates the current state of this Consent resource. 2568 */ 2569 public Consent setStatus(ConsentState value) { 2570 if (this.status == null) 2571 this.status = new Enumeration<ConsentState>(new ConsentStateEnumFactory()); 2572 this.status.setValue(value); 2573 return this; 2574 } 2575 2576 /** 2577 * @return {@link #category} (A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.) 2578 */ 2579 public List<CodeableConcept> getCategory() { 2580 if (this.category == null) 2581 this.category = new ArrayList<CodeableConcept>(); 2582 return this.category; 2583 } 2584 2585 /** 2586 * @return Returns a reference to <code>this</code> for easy method chaining 2587 */ 2588 public Consent setCategory(List<CodeableConcept> theCategory) { 2589 this.category = theCategory; 2590 return this; 2591 } 2592 2593 public boolean hasCategory() { 2594 if (this.category == null) 2595 return false; 2596 for (CodeableConcept item : this.category) 2597 if (!item.isEmpty()) 2598 return true; 2599 return false; 2600 } 2601 2602 public CodeableConcept addCategory() { //3 2603 CodeableConcept t = new CodeableConcept(); 2604 if (this.category == null) 2605 this.category = new ArrayList<CodeableConcept>(); 2606 this.category.add(t); 2607 return t; 2608 } 2609 2610 public Consent addCategory(CodeableConcept t) { //3 2611 if (t == null) 2612 return this; 2613 if (this.category == null) 2614 this.category = new ArrayList<CodeableConcept>(); 2615 this.category.add(t); 2616 return this; 2617 } 2618 2619 /** 2620 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2621 */ 2622 public CodeableConcept getCategoryFirstRep() { 2623 if (getCategory().isEmpty()) { 2624 addCategory(); 2625 } 2626 return getCategory().get(0); 2627 } 2628 2629 /** 2630 * @return {@link #subject} (The patient/healthcare practitioner or group of persons to whom this consent applies.) 2631 */ 2632 public Reference getSubject() { 2633 if (this.subject == null) 2634 if (Configuration.errorOnAutoCreate()) 2635 throw new Error("Attempt to auto-create Consent.subject"); 2636 else if (Configuration.doAutoCreate()) 2637 this.subject = new Reference(); // cc 2638 return this.subject; 2639 } 2640 2641 public boolean hasSubject() { 2642 return this.subject != null && !this.subject.isEmpty(); 2643 } 2644 2645 /** 2646 * @param value {@link #subject} (The patient/healthcare practitioner or group of persons to whom this consent applies.) 2647 */ 2648 public Consent setSubject(Reference value) { 2649 this.subject = value; 2650 return this; 2651 } 2652 2653 /** 2654 * @return {@link #date} (Date the consent instance was agreed to.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2655 */ 2656 public DateType getDateElement() { 2657 if (this.date == null) 2658 if (Configuration.errorOnAutoCreate()) 2659 throw new Error("Attempt to auto-create Consent.date"); 2660 else if (Configuration.doAutoCreate()) 2661 this.date = new DateType(); // bb 2662 return this.date; 2663 } 2664 2665 public boolean hasDateElement() { 2666 return this.date != null && !this.date.isEmpty(); 2667 } 2668 2669 public boolean hasDate() { 2670 return this.date != null && !this.date.isEmpty(); 2671 } 2672 2673 /** 2674 * @param value {@link #date} (Date the consent instance was agreed to.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2675 */ 2676 public Consent setDateElement(DateType value) { 2677 this.date = value; 2678 return this; 2679 } 2680 2681 /** 2682 * @return Date the consent instance was agreed to. 2683 */ 2684 public Date getDate() { 2685 return this.date == null ? null : this.date.getValue(); 2686 } 2687 2688 /** 2689 * @param value Date the consent instance was agreed to. 2690 */ 2691 public Consent setDate(Date value) { 2692 if (value == null) 2693 this.date = null; 2694 else { 2695 if (this.date == null) 2696 this.date = new DateType(); 2697 this.date.setValue(value); 2698 } 2699 return this; 2700 } 2701 2702 /** 2703 * @return {@link #period} (Effective period for this Consent Resource and all provisions unless specified in that provision.) 2704 */ 2705 public Period getPeriod() { 2706 if (this.period == null) 2707 if (Configuration.errorOnAutoCreate()) 2708 throw new Error("Attempt to auto-create Consent.period"); 2709 else if (Configuration.doAutoCreate()) 2710 this.period = new Period(); // cc 2711 return this.period; 2712 } 2713 2714 public boolean hasPeriod() { 2715 return this.period != null && !this.period.isEmpty(); 2716 } 2717 2718 /** 2719 * @param value {@link #period} (Effective period for this Consent Resource and all provisions unless specified in that provision.) 2720 */ 2721 public Consent setPeriod(Period value) { 2722 this.period = value; 2723 return this; 2724 } 2725 2726 /** 2727 * @return {@link #grantor} (The entity responsible for granting the rights listed in a Consent Directive.) 2728 */ 2729 public List<Reference> getGrantor() { 2730 if (this.grantor == null) 2731 this.grantor = new ArrayList<Reference>(); 2732 return this.grantor; 2733 } 2734 2735 /** 2736 * @return Returns a reference to <code>this</code> for easy method chaining 2737 */ 2738 public Consent setGrantor(List<Reference> theGrantor) { 2739 this.grantor = theGrantor; 2740 return this; 2741 } 2742 2743 public boolean hasGrantor() { 2744 if (this.grantor == null) 2745 return false; 2746 for (Reference item : this.grantor) 2747 if (!item.isEmpty()) 2748 return true; 2749 return false; 2750 } 2751 2752 public Reference addGrantor() { //3 2753 Reference t = new Reference(); 2754 if (this.grantor == null) 2755 this.grantor = new ArrayList<Reference>(); 2756 this.grantor.add(t); 2757 return t; 2758 } 2759 2760 public Consent addGrantor(Reference t) { //3 2761 if (t == null) 2762 return this; 2763 if (this.grantor == null) 2764 this.grantor = new ArrayList<Reference>(); 2765 this.grantor.add(t); 2766 return this; 2767 } 2768 2769 /** 2770 * @return The first repetition of repeating field {@link #grantor}, creating it if it does not already exist {3} 2771 */ 2772 public Reference getGrantorFirstRep() { 2773 if (getGrantor().isEmpty()) { 2774 addGrantor(); 2775 } 2776 return getGrantor().get(0); 2777 } 2778 2779 /** 2780 * @return {@link #grantee} (The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.) 2781 */ 2782 public List<Reference> getGrantee() { 2783 if (this.grantee == null) 2784 this.grantee = new ArrayList<Reference>(); 2785 return this.grantee; 2786 } 2787 2788 /** 2789 * @return Returns a reference to <code>this</code> for easy method chaining 2790 */ 2791 public Consent setGrantee(List<Reference> theGrantee) { 2792 this.grantee = theGrantee; 2793 return this; 2794 } 2795 2796 public boolean hasGrantee() { 2797 if (this.grantee == null) 2798 return false; 2799 for (Reference item : this.grantee) 2800 if (!item.isEmpty()) 2801 return true; 2802 return false; 2803 } 2804 2805 public Reference addGrantee() { //3 2806 Reference t = new Reference(); 2807 if (this.grantee == null) 2808 this.grantee = new ArrayList<Reference>(); 2809 this.grantee.add(t); 2810 return t; 2811 } 2812 2813 public Consent addGrantee(Reference t) { //3 2814 if (t == null) 2815 return this; 2816 if (this.grantee == null) 2817 this.grantee = new ArrayList<Reference>(); 2818 this.grantee.add(t); 2819 return this; 2820 } 2821 2822 /** 2823 * @return The first repetition of repeating field {@link #grantee}, creating it if it does not already exist {3} 2824 */ 2825 public Reference getGranteeFirstRep() { 2826 if (getGrantee().isEmpty()) { 2827 addGrantee(); 2828 } 2829 return getGrantee().get(0); 2830 } 2831 2832 /** 2833 * @return {@link #manager} (The actor that manages the consent through its lifecycle.) 2834 */ 2835 public List<Reference> getManager() { 2836 if (this.manager == null) 2837 this.manager = new ArrayList<Reference>(); 2838 return this.manager; 2839 } 2840 2841 /** 2842 * @return Returns a reference to <code>this</code> for easy method chaining 2843 */ 2844 public Consent setManager(List<Reference> theManager) { 2845 this.manager = theManager; 2846 return this; 2847 } 2848 2849 public boolean hasManager() { 2850 if (this.manager == null) 2851 return false; 2852 for (Reference item : this.manager) 2853 if (!item.isEmpty()) 2854 return true; 2855 return false; 2856 } 2857 2858 public Reference addManager() { //3 2859 Reference t = new Reference(); 2860 if (this.manager == null) 2861 this.manager = new ArrayList<Reference>(); 2862 this.manager.add(t); 2863 return t; 2864 } 2865 2866 public Consent addManager(Reference t) { //3 2867 if (t == null) 2868 return this; 2869 if (this.manager == null) 2870 this.manager = new ArrayList<Reference>(); 2871 this.manager.add(t); 2872 return this; 2873 } 2874 2875 /** 2876 * @return The first repetition of repeating field {@link #manager}, creating it if it does not already exist {3} 2877 */ 2878 public Reference getManagerFirstRep() { 2879 if (getManager().isEmpty()) { 2880 addManager(); 2881 } 2882 return getManager().get(0); 2883 } 2884 2885 /** 2886 * @return {@link #controller} (The actor that controls/enforces the access according to the consent.) 2887 */ 2888 public List<Reference> getController() { 2889 if (this.controller == null) 2890 this.controller = new ArrayList<Reference>(); 2891 return this.controller; 2892 } 2893 2894 /** 2895 * @return Returns a reference to <code>this</code> for easy method chaining 2896 */ 2897 public Consent setController(List<Reference> theController) { 2898 this.controller = theController; 2899 return this; 2900 } 2901 2902 public boolean hasController() { 2903 if (this.controller == null) 2904 return false; 2905 for (Reference item : this.controller) 2906 if (!item.isEmpty()) 2907 return true; 2908 return false; 2909 } 2910 2911 public Reference addController() { //3 2912 Reference t = new Reference(); 2913 if (this.controller == null) 2914 this.controller = new ArrayList<Reference>(); 2915 this.controller.add(t); 2916 return t; 2917 } 2918 2919 public Consent addController(Reference t) { //3 2920 if (t == null) 2921 return this; 2922 if (this.controller == null) 2923 this.controller = new ArrayList<Reference>(); 2924 this.controller.add(t); 2925 return this; 2926 } 2927 2928 /** 2929 * @return The first repetition of repeating field {@link #controller}, creating it if it does not already exist {3} 2930 */ 2931 public Reference getControllerFirstRep() { 2932 if (getController().isEmpty()) { 2933 addController(); 2934 } 2935 return getController().get(0); 2936 } 2937 2938 /** 2939 * @return {@link #sourceAttachment} (The source on which this consent statement is based. The source might be a scanned original paper form.) 2940 */ 2941 public List<Attachment> getSourceAttachment() { 2942 if (this.sourceAttachment == null) 2943 this.sourceAttachment = new ArrayList<Attachment>(); 2944 return this.sourceAttachment; 2945 } 2946 2947 /** 2948 * @return Returns a reference to <code>this</code> for easy method chaining 2949 */ 2950 public Consent setSourceAttachment(List<Attachment> theSourceAttachment) { 2951 this.sourceAttachment = theSourceAttachment; 2952 return this; 2953 } 2954 2955 public boolean hasSourceAttachment() { 2956 if (this.sourceAttachment == null) 2957 return false; 2958 for (Attachment item : this.sourceAttachment) 2959 if (!item.isEmpty()) 2960 return true; 2961 return false; 2962 } 2963 2964 public Attachment addSourceAttachment() { //3 2965 Attachment t = new Attachment(); 2966 if (this.sourceAttachment == null) 2967 this.sourceAttachment = new ArrayList<Attachment>(); 2968 this.sourceAttachment.add(t); 2969 return t; 2970 } 2971 2972 public Consent addSourceAttachment(Attachment t) { //3 2973 if (t == null) 2974 return this; 2975 if (this.sourceAttachment == null) 2976 this.sourceAttachment = new ArrayList<Attachment>(); 2977 this.sourceAttachment.add(t); 2978 return this; 2979 } 2980 2981 /** 2982 * @return The first repetition of repeating field {@link #sourceAttachment}, creating it if it does not already exist {3} 2983 */ 2984 public Attachment getSourceAttachmentFirstRep() { 2985 if (getSourceAttachment().isEmpty()) { 2986 addSourceAttachment(); 2987 } 2988 return getSourceAttachment().get(0); 2989 } 2990 2991 /** 2992 * @return {@link #sourceReference} (A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.) 2993 */ 2994 public List<Reference> getSourceReference() { 2995 if (this.sourceReference == null) 2996 this.sourceReference = new ArrayList<Reference>(); 2997 return this.sourceReference; 2998 } 2999 3000 /** 3001 * @return Returns a reference to <code>this</code> for easy method chaining 3002 */ 3003 public Consent setSourceReference(List<Reference> theSourceReference) { 3004 this.sourceReference = theSourceReference; 3005 return this; 3006 } 3007 3008 public boolean hasSourceReference() { 3009 if (this.sourceReference == null) 3010 return false; 3011 for (Reference item : this.sourceReference) 3012 if (!item.isEmpty()) 3013 return true; 3014 return false; 3015 } 3016 3017 public Reference addSourceReference() { //3 3018 Reference t = new Reference(); 3019 if (this.sourceReference == null) 3020 this.sourceReference = new ArrayList<Reference>(); 3021 this.sourceReference.add(t); 3022 return t; 3023 } 3024 3025 public Consent addSourceReference(Reference t) { //3 3026 if (t == null) 3027 return this; 3028 if (this.sourceReference == null) 3029 this.sourceReference = new ArrayList<Reference>(); 3030 this.sourceReference.add(t); 3031 return this; 3032 } 3033 3034 /** 3035 * @return The first repetition of repeating field {@link #sourceReference}, creating it if it does not already exist {3} 3036 */ 3037 public Reference getSourceReferenceFirstRep() { 3038 if (getSourceReference().isEmpty()) { 3039 addSourceReference(); 3040 } 3041 return getSourceReference().get(0); 3042 } 3043 3044 /** 3045 * @return {@link #regulatoryBasis} (A set of codes that indicate the regulatory basis (if any) that this consent supports.) 3046 */ 3047 public List<CodeableConcept> getRegulatoryBasis() { 3048 if (this.regulatoryBasis == null) 3049 this.regulatoryBasis = new ArrayList<CodeableConcept>(); 3050 return this.regulatoryBasis; 3051 } 3052 3053 /** 3054 * @return Returns a reference to <code>this</code> for easy method chaining 3055 */ 3056 public Consent setRegulatoryBasis(List<CodeableConcept> theRegulatoryBasis) { 3057 this.regulatoryBasis = theRegulatoryBasis; 3058 return this; 3059 } 3060 3061 public boolean hasRegulatoryBasis() { 3062 if (this.regulatoryBasis == null) 3063 return false; 3064 for (CodeableConcept item : this.regulatoryBasis) 3065 if (!item.isEmpty()) 3066 return true; 3067 return false; 3068 } 3069 3070 public CodeableConcept addRegulatoryBasis() { //3 3071 CodeableConcept t = new CodeableConcept(); 3072 if (this.regulatoryBasis == null) 3073 this.regulatoryBasis = new ArrayList<CodeableConcept>(); 3074 this.regulatoryBasis.add(t); 3075 return t; 3076 } 3077 3078 public Consent addRegulatoryBasis(CodeableConcept t) { //3 3079 if (t == null) 3080 return this; 3081 if (this.regulatoryBasis == null) 3082 this.regulatoryBasis = new ArrayList<CodeableConcept>(); 3083 this.regulatoryBasis.add(t); 3084 return this; 3085 } 3086 3087 /** 3088 * @return The first repetition of repeating field {@link #regulatoryBasis}, creating it if it does not already exist {3} 3089 */ 3090 public CodeableConcept getRegulatoryBasisFirstRep() { 3091 if (getRegulatoryBasis().isEmpty()) { 3092 addRegulatoryBasis(); 3093 } 3094 return getRegulatoryBasis().get(0); 3095 } 3096 3097 /** 3098 * @return {@link #policyBasis} (A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.) 3099 */ 3100 public ConsentPolicyBasisComponent getPolicyBasis() { 3101 if (this.policyBasis == null) 3102 if (Configuration.errorOnAutoCreate()) 3103 throw new Error("Attempt to auto-create Consent.policyBasis"); 3104 else if (Configuration.doAutoCreate()) 3105 this.policyBasis = new ConsentPolicyBasisComponent(); // cc 3106 return this.policyBasis; 3107 } 3108 3109 public boolean hasPolicyBasis() { 3110 return this.policyBasis != null && !this.policyBasis.isEmpty(); 3111 } 3112 3113 /** 3114 * @param value {@link #policyBasis} (A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.) 3115 */ 3116 public Consent setPolicyBasis(ConsentPolicyBasisComponent value) { 3117 this.policyBasis = value; 3118 return this; 3119 } 3120 3121 /** 3122 * @return {@link #policyText} (A Reference to the human readable policy explaining the basis for the Consent.) 3123 */ 3124 public List<Reference> getPolicyText() { 3125 if (this.policyText == null) 3126 this.policyText = new ArrayList<Reference>(); 3127 return this.policyText; 3128 } 3129 3130 /** 3131 * @return Returns a reference to <code>this</code> for easy method chaining 3132 */ 3133 public Consent setPolicyText(List<Reference> thePolicyText) { 3134 this.policyText = thePolicyText; 3135 return this; 3136 } 3137 3138 public boolean hasPolicyText() { 3139 if (this.policyText == null) 3140 return false; 3141 for (Reference item : this.policyText) 3142 if (!item.isEmpty()) 3143 return true; 3144 return false; 3145 } 3146 3147 public Reference addPolicyText() { //3 3148 Reference t = new Reference(); 3149 if (this.policyText == null) 3150 this.policyText = new ArrayList<Reference>(); 3151 this.policyText.add(t); 3152 return t; 3153 } 3154 3155 public Consent addPolicyText(Reference t) { //3 3156 if (t == null) 3157 return this; 3158 if (this.policyText == null) 3159 this.policyText = new ArrayList<Reference>(); 3160 this.policyText.add(t); 3161 return this; 3162 } 3163 3164 /** 3165 * @return The first repetition of repeating field {@link #policyText}, creating it if it does not already exist {3} 3166 */ 3167 public Reference getPolicyTextFirstRep() { 3168 if (getPolicyText().isEmpty()) { 3169 addPolicyText(); 3170 } 3171 return getPolicyText().get(0); 3172 } 3173 3174 /** 3175 * @return {@link #verification} (Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person.) 3176 */ 3177 public List<ConsentVerificationComponent> getVerification() { 3178 if (this.verification == null) 3179 this.verification = new ArrayList<ConsentVerificationComponent>(); 3180 return this.verification; 3181 } 3182 3183 /** 3184 * @return Returns a reference to <code>this</code> for easy method chaining 3185 */ 3186 public Consent setVerification(List<ConsentVerificationComponent> theVerification) { 3187 this.verification = theVerification; 3188 return this; 3189 } 3190 3191 public boolean hasVerification() { 3192 if (this.verification == null) 3193 return false; 3194 for (ConsentVerificationComponent item : this.verification) 3195 if (!item.isEmpty()) 3196 return true; 3197 return false; 3198 } 3199 3200 public ConsentVerificationComponent addVerification() { //3 3201 ConsentVerificationComponent t = new ConsentVerificationComponent(); 3202 if (this.verification == null) 3203 this.verification = new ArrayList<ConsentVerificationComponent>(); 3204 this.verification.add(t); 3205 return t; 3206 } 3207 3208 public Consent addVerification(ConsentVerificationComponent t) { //3 3209 if (t == null) 3210 return this; 3211 if (this.verification == null) 3212 this.verification = new ArrayList<ConsentVerificationComponent>(); 3213 this.verification.add(t); 3214 return this; 3215 } 3216 3217 /** 3218 * @return The first repetition of repeating field {@link #verification}, creating it if it does not already exist {3} 3219 */ 3220 public ConsentVerificationComponent getVerificationFirstRep() { 3221 if (getVerification().isEmpty()) { 3222 addVerification(); 3223 } 3224 return getVerification().get(0); 3225 } 3226 3227 /** 3228 * @return {@link #decision} (Action to take - permit or deny - as default.). This is the underlying object with id, value and extensions. The accessor "getDecision" gives direct access to the value 3229 */ 3230 public Enumeration<ConsentProvisionType> getDecisionElement() { 3231 if (this.decision == null) 3232 if (Configuration.errorOnAutoCreate()) 3233 throw new Error("Attempt to auto-create Consent.decision"); 3234 else if (Configuration.doAutoCreate()) 3235 this.decision = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); // bb 3236 return this.decision; 3237 } 3238 3239 public boolean hasDecisionElement() { 3240 return this.decision != null && !this.decision.isEmpty(); 3241 } 3242 3243 public boolean hasDecision() { 3244 return this.decision != null && !this.decision.isEmpty(); 3245 } 3246 3247 /** 3248 * @param value {@link #decision} (Action to take - permit or deny - as default.). This is the underlying object with id, value and extensions. The accessor "getDecision" gives direct access to the value 3249 */ 3250 public Consent setDecisionElement(Enumeration<ConsentProvisionType> value) { 3251 this.decision = value; 3252 return this; 3253 } 3254 3255 /** 3256 * @return Action to take - permit or deny - as default. 3257 */ 3258 public ConsentProvisionType getDecision() { 3259 return this.decision == null ? null : this.decision.getValue(); 3260 } 3261 3262 /** 3263 * @param value Action to take - permit or deny - as default. 3264 */ 3265 public Consent setDecision(ConsentProvisionType value) { 3266 if (value == null) 3267 this.decision = null; 3268 else { 3269 if (this.decision == null) 3270 this.decision = new Enumeration<ConsentProvisionType>(new ConsentProvisionTypeEnumFactory()); 3271 this.decision.setValue(value); 3272 } 3273 return this; 3274 } 3275 3276 /** 3277 * @return {@link #provision} (An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.) 3278 */ 3279 public List<ProvisionComponent> getProvision() { 3280 if (this.provision == null) 3281 this.provision = new ArrayList<ProvisionComponent>(); 3282 return this.provision; 3283 } 3284 3285 /** 3286 * @return Returns a reference to <code>this</code> for easy method chaining 3287 */ 3288 public Consent setProvision(List<ProvisionComponent> theProvision) { 3289 this.provision = theProvision; 3290 return this; 3291 } 3292 3293 public boolean hasProvision() { 3294 if (this.provision == null) 3295 return false; 3296 for (ProvisionComponent item : this.provision) 3297 if (!item.isEmpty()) 3298 return true; 3299 return false; 3300 } 3301 3302 public ProvisionComponent addProvision() { //3 3303 ProvisionComponent t = new ProvisionComponent(); 3304 if (this.provision == null) 3305 this.provision = new ArrayList<ProvisionComponent>(); 3306 this.provision.add(t); 3307 return t; 3308 } 3309 3310 public Consent addProvision(ProvisionComponent t) { //3 3311 if (t == null) 3312 return this; 3313 if (this.provision == null) 3314 this.provision = new ArrayList<ProvisionComponent>(); 3315 this.provision.add(t); 3316 return this; 3317 } 3318 3319 /** 3320 * @return The first repetition of repeating field {@link #provision}, creating it if it does not already exist {3} 3321 */ 3322 public ProvisionComponent getProvisionFirstRep() { 3323 if (getProvision().isEmpty()) { 3324 addProvision(); 3325 } 3326 return getProvision().get(0); 3327 } 3328 3329 protected void listChildren(List<Property> children) { 3330 super.listChildren(children); 3331 children.add(new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3332 children.add(new Property("status", "code", "Indicates the current state of this Consent resource.", 0, 1, status)); 3333 children.add(new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category)); 3334 children.add(new Property("subject", "Reference(Patient|Practitioner|Group)", "The patient/healthcare practitioner or group of persons to whom this consent applies.", 0, 1, subject)); 3335 children.add(new Property("date", "date", "Date the consent instance was agreed to.", 0, 1, date)); 3336 children.add(new Property("period", "Period", "Effective period for this Consent Resource and all provisions unless specified in that provision.", 0, 1, period)); 3337 children.add(new Property("grantor", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for granting the rights listed in a Consent Directive.", 0, java.lang.Integer.MAX_VALUE, grantor)); 3338 children.add(new Property("grantee", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, grantee)); 3339 children.add(new Property("manager", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that manages the consent through its lifecycle.", 0, java.lang.Integer.MAX_VALUE, manager)); 3340 children.add(new Property("controller", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that controls/enforces the access according to the consent.", 0, java.lang.Integer.MAX_VALUE, controller)); 3341 children.add(new Property("sourceAttachment", "Attachment", "The source on which this consent statement is based. The source might be a scanned original paper form.", 0, java.lang.Integer.MAX_VALUE, sourceAttachment)); 3342 children.add(new Property("sourceReference", "Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, java.lang.Integer.MAX_VALUE, sourceReference)); 3343 children.add(new Property("regulatoryBasis", "CodeableConcept", "A set of codes that indicate the regulatory basis (if any) that this consent supports.", 0, java.lang.Integer.MAX_VALUE, regulatoryBasis)); 3344 children.add(new Property("policyBasis", "", "A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.", 0, 1, policyBasis)); 3345 children.add(new Property("policyText", "Reference(DocumentReference)", "A Reference to the human readable policy explaining the basis for the Consent.", 0, java.lang.Integer.MAX_VALUE, policyText)); 3346 children.add(new Property("verification", "", "Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person.", 0, java.lang.Integer.MAX_VALUE, verification)); 3347 children.add(new Property("decision", "code", "Action to take - permit or deny - as default.", 0, 1, decision)); 3348 children.add(new Property("provision", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, provision)); 3349 } 3350 3351 @Override 3352 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3353 switch (_hash) { 3354 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this copy of the Consent Statement.", 0, java.lang.Integer.MAX_VALUE, identifier); 3355 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of this Consent resource.", 0, 1, status); 3356 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A classification of the type of consents found in the statement. This element supports indexing and retrieval of consent statements.", 0, java.lang.Integer.MAX_VALUE, category); 3357 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Practitioner|Group)", "The patient/healthcare practitioner or group of persons to whom this consent applies.", 0, 1, subject); 3358 case 3076014: /*date*/ return new Property("date", "date", "Date the consent instance was agreed to.", 0, 1, date); 3359 case -991726143: /*period*/ return new Property("period", "Period", "Effective period for this Consent Resource and all provisions unless specified in that provision.", 0, 1, period); 3360 case 280295423: /*grantor*/ return new Property("grantor", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for granting the rights listed in a Consent Directive.", 0, java.lang.Integer.MAX_VALUE, grantor); 3361 case 280295100: /*grantee*/ return new Property("grantee", "Reference(CareTeam|HealthcareService|Organization|Patient|Practitioner|RelatedPerson|PractitionerRole)", "The entity responsible for complying with the Consent Directive, including any obligations or limitations on authorizations and enforcement of prohibitions.", 0, java.lang.Integer.MAX_VALUE, grantee); 3362 case 835260333: /*manager*/ return new Property("manager", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that manages the consent through its lifecycle.", 0, java.lang.Integer.MAX_VALUE, manager); 3363 case 637428636: /*controller*/ return new Property("controller", "Reference(HealthcareService|Organization|Patient|Practitioner)", "The actor that controls/enforces the access according to the consent.", 0, java.lang.Integer.MAX_VALUE, controller); 3364 case 1964406686: /*sourceAttachment*/ return new Property("sourceAttachment", "Attachment", "The source on which this consent statement is based. The source might be a scanned original paper form.", 0, java.lang.Integer.MAX_VALUE, sourceAttachment); 3365 case -244259472: /*sourceReference*/ return new Property("sourceReference", "Reference(Consent|DocumentReference|Contract|QuestionnaireResponse)", "A reference to a consent that links back to such a source, a reference to a document repository (e.g. XDS) that stores the original consent document.", 0, java.lang.Integer.MAX_VALUE, sourceReference); 3366 case -1780301690: /*regulatoryBasis*/ return new Property("regulatoryBasis", "CodeableConcept", "A set of codes that indicate the regulatory basis (if any) that this consent supports.", 0, java.lang.Integer.MAX_VALUE, regulatoryBasis); 3367 case 2138287660: /*policyBasis*/ return new Property("policyBasis", "", "A Reference or URL used to uniquely identify the policy the organization will enforce for this Consent. This Reference or URL should be specific to the version of the policy and should be dereferencable to a computable policy of some form.", 0, 1, policyBasis); 3368 case 1593537919: /*policyText*/ return new Property("policyText", "Reference(DocumentReference)", "A Reference to the human readable policy explaining the basis for the Consent.", 0, java.lang.Integer.MAX_VALUE, policyText); 3369 case -1484401125: /*verification*/ return new Property("verification", "", "Whether a treatment instruction (e.g. artificial respiration: yes or no) was verified with the patient, his/her family or another authorized person.", 0, java.lang.Integer.MAX_VALUE, verification); 3370 case 565719004: /*decision*/ return new Property("decision", "code", "Action to take - permit or deny - as default.", 0, 1, decision); 3371 case -547120939: /*provision*/ return new Property("provision", "", "An exception to the base policy of this consent. An exception can be an addition or removal of access permissions.", 0, java.lang.Integer.MAX_VALUE, provision); 3372 default: return super.getNamedProperty(_hash, _name, _checkValid); 3373 } 3374 3375 } 3376 3377 @Override 3378 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3379 switch (hash) { 3380 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3381 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ConsentState> 3382 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3383 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3384 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3385 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3386 case 280295423: /*grantor*/ return this.grantor == null ? new Base[0] : this.grantor.toArray(new Base[this.grantor.size()]); // Reference 3387 case 280295100: /*grantee*/ return this.grantee == null ? new Base[0] : this.grantee.toArray(new Base[this.grantee.size()]); // Reference 3388 case 835260333: /*manager*/ return this.manager == null ? new Base[0] : this.manager.toArray(new Base[this.manager.size()]); // Reference 3389 case 637428636: /*controller*/ return this.controller == null ? new Base[0] : this.controller.toArray(new Base[this.controller.size()]); // Reference 3390 case 1964406686: /*sourceAttachment*/ return this.sourceAttachment == null ? new Base[0] : this.sourceAttachment.toArray(new Base[this.sourceAttachment.size()]); // Attachment 3391 case -244259472: /*sourceReference*/ return this.sourceReference == null ? new Base[0] : this.sourceReference.toArray(new Base[this.sourceReference.size()]); // Reference 3392 case -1780301690: /*regulatoryBasis*/ return this.regulatoryBasis == null ? new Base[0] : this.regulatoryBasis.toArray(new Base[this.regulatoryBasis.size()]); // CodeableConcept 3393 case 2138287660: /*policyBasis*/ return this.policyBasis == null ? new Base[0] : new Base[] {this.policyBasis}; // ConsentPolicyBasisComponent 3394 case 1593537919: /*policyText*/ return this.policyText == null ? new Base[0] : this.policyText.toArray(new Base[this.policyText.size()]); // Reference 3395 case -1484401125: /*verification*/ return this.verification == null ? new Base[0] : this.verification.toArray(new Base[this.verification.size()]); // ConsentVerificationComponent 3396 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // Enumeration<ConsentProvisionType> 3397 case -547120939: /*provision*/ return this.provision == null ? new Base[0] : this.provision.toArray(new Base[this.provision.size()]); // ProvisionComponent 3398 default: return super.getProperty(hash, name, checkValid); 3399 } 3400 3401 } 3402 3403 @Override 3404 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3405 switch (hash) { 3406 case -1618432855: // identifier 3407 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3408 return value; 3409 case -892481550: // status 3410 value = new ConsentStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 3411 this.status = (Enumeration) value; // Enumeration<ConsentState> 3412 return value; 3413 case 50511102: // category 3414 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3415 return value; 3416 case -1867885268: // subject 3417 this.subject = TypeConvertor.castToReference(value); // Reference 3418 return value; 3419 case 3076014: // date 3420 this.date = TypeConvertor.castToDate(value); // DateType 3421 return value; 3422 case -991726143: // period 3423 this.period = TypeConvertor.castToPeriod(value); // Period 3424 return value; 3425 case 280295423: // grantor 3426 this.getGrantor().add(TypeConvertor.castToReference(value)); // Reference 3427 return value; 3428 case 280295100: // grantee 3429 this.getGrantee().add(TypeConvertor.castToReference(value)); // Reference 3430 return value; 3431 case 835260333: // manager 3432 this.getManager().add(TypeConvertor.castToReference(value)); // Reference 3433 return value; 3434 case 637428636: // controller 3435 this.getController().add(TypeConvertor.castToReference(value)); // Reference 3436 return value; 3437 case 1964406686: // sourceAttachment 3438 this.getSourceAttachment().add(TypeConvertor.castToAttachment(value)); // Attachment 3439 return value; 3440 case -244259472: // sourceReference 3441 this.getSourceReference().add(TypeConvertor.castToReference(value)); // Reference 3442 return value; 3443 case -1780301690: // regulatoryBasis 3444 this.getRegulatoryBasis().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3445 return value; 3446 case 2138287660: // policyBasis 3447 this.policyBasis = (ConsentPolicyBasisComponent) value; // ConsentPolicyBasisComponent 3448 return value; 3449 case 1593537919: // policyText 3450 this.getPolicyText().add(TypeConvertor.castToReference(value)); // Reference 3451 return value; 3452 case -1484401125: // verification 3453 this.getVerification().add((ConsentVerificationComponent) value); // ConsentVerificationComponent 3454 return value; 3455 case 565719004: // decision 3456 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3457 this.decision = (Enumeration) value; // Enumeration<ConsentProvisionType> 3458 return value; 3459 case -547120939: // provision 3460 this.getProvision().add((ProvisionComponent) value); // ProvisionComponent 3461 return value; 3462 default: return super.setProperty(hash, name, value); 3463 } 3464 3465 } 3466 3467 @Override 3468 public Base setProperty(String name, Base value) throws FHIRException { 3469 if (name.equals("identifier")) { 3470 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3471 } else if (name.equals("status")) { 3472 value = new ConsentStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 3473 this.status = (Enumeration) value; // Enumeration<ConsentState> 3474 } else if (name.equals("category")) { 3475 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3476 } else if (name.equals("subject")) { 3477 this.subject = TypeConvertor.castToReference(value); // Reference 3478 } else if (name.equals("date")) { 3479 this.date = TypeConvertor.castToDate(value); // DateType 3480 } else if (name.equals("period")) { 3481 this.period = TypeConvertor.castToPeriod(value); // Period 3482 } else if (name.equals("grantor")) { 3483 this.getGrantor().add(TypeConvertor.castToReference(value)); 3484 } else if (name.equals("grantee")) { 3485 this.getGrantee().add(TypeConvertor.castToReference(value)); 3486 } else if (name.equals("manager")) { 3487 this.getManager().add(TypeConvertor.castToReference(value)); 3488 } else if (name.equals("controller")) { 3489 this.getController().add(TypeConvertor.castToReference(value)); 3490 } else if (name.equals("sourceAttachment")) { 3491 this.getSourceAttachment().add(TypeConvertor.castToAttachment(value)); 3492 } else if (name.equals("sourceReference")) { 3493 this.getSourceReference().add(TypeConvertor.castToReference(value)); 3494 } else if (name.equals("regulatoryBasis")) { 3495 this.getRegulatoryBasis().add(TypeConvertor.castToCodeableConcept(value)); 3496 } else if (name.equals("policyBasis")) { 3497 this.policyBasis = (ConsentPolicyBasisComponent) value; // ConsentPolicyBasisComponent 3498 } else if (name.equals("policyText")) { 3499 this.getPolicyText().add(TypeConvertor.castToReference(value)); 3500 } else if (name.equals("verification")) { 3501 this.getVerification().add((ConsentVerificationComponent) value); 3502 } else if (name.equals("decision")) { 3503 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3504 this.decision = (Enumeration) value; // Enumeration<ConsentProvisionType> 3505 } else if (name.equals("provision")) { 3506 this.getProvision().add((ProvisionComponent) value); 3507 } else 3508 return super.setProperty(name, value); 3509 return value; 3510 } 3511 3512 @Override 3513 public void removeChild(String name, Base value) throws FHIRException { 3514 if (name.equals("identifier")) { 3515 this.getIdentifier().remove(value); 3516 } else if (name.equals("status")) { 3517 value = new ConsentStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 3518 this.status = (Enumeration) value; // Enumeration<ConsentState> 3519 } else if (name.equals("category")) { 3520 this.getCategory().remove(value); 3521 } else if (name.equals("subject")) { 3522 this.subject = null; 3523 } else if (name.equals("date")) { 3524 this.date = null; 3525 } else if (name.equals("period")) { 3526 this.period = null; 3527 } else if (name.equals("grantor")) { 3528 this.getGrantor().remove(value); 3529 } else if (name.equals("grantee")) { 3530 this.getGrantee().remove(value); 3531 } else if (name.equals("manager")) { 3532 this.getManager().remove(value); 3533 } else if (name.equals("controller")) { 3534 this.getController().remove(value); 3535 } else if (name.equals("sourceAttachment")) { 3536 this.getSourceAttachment().remove(value); 3537 } else if (name.equals("sourceReference")) { 3538 this.getSourceReference().remove(value); 3539 } else if (name.equals("regulatoryBasis")) { 3540 this.getRegulatoryBasis().remove(value); 3541 } else if (name.equals("policyBasis")) { 3542 this.policyBasis = (ConsentPolicyBasisComponent) value; // ConsentPolicyBasisComponent 3543 } else if (name.equals("policyText")) { 3544 this.getPolicyText().remove(value); 3545 } else if (name.equals("verification")) { 3546 this.getVerification().remove((ConsentVerificationComponent) value); 3547 } else if (name.equals("decision")) { 3548 value = new ConsentProvisionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3549 this.decision = (Enumeration) value; // Enumeration<ConsentProvisionType> 3550 } else if (name.equals("provision")) { 3551 this.getProvision().remove((ProvisionComponent) value); 3552 } else 3553 super.removeChild(name, value); 3554 3555 } 3556 3557 @Override 3558 public Base makeProperty(int hash, String name) throws FHIRException { 3559 switch (hash) { 3560 case -1618432855: return addIdentifier(); 3561 case -892481550: return getStatusElement(); 3562 case 50511102: return addCategory(); 3563 case -1867885268: return getSubject(); 3564 case 3076014: return getDateElement(); 3565 case -991726143: return getPeriod(); 3566 case 280295423: return addGrantor(); 3567 case 280295100: return addGrantee(); 3568 case 835260333: return addManager(); 3569 case 637428636: return addController(); 3570 case 1964406686: return addSourceAttachment(); 3571 case -244259472: return addSourceReference(); 3572 case -1780301690: return addRegulatoryBasis(); 3573 case 2138287660: return getPolicyBasis(); 3574 case 1593537919: return addPolicyText(); 3575 case -1484401125: return addVerification(); 3576 case 565719004: return getDecisionElement(); 3577 case -547120939: return addProvision(); 3578 default: return super.makeProperty(hash, name); 3579 } 3580 3581 } 3582 3583 @Override 3584 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3585 switch (hash) { 3586 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3587 case -892481550: /*status*/ return new String[] {"code"}; 3588 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3589 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3590 case 3076014: /*date*/ return new String[] {"date"}; 3591 case -991726143: /*period*/ return new String[] {"Period"}; 3592 case 280295423: /*grantor*/ return new String[] {"Reference"}; 3593 case 280295100: /*grantee*/ return new String[] {"Reference"}; 3594 case 835260333: /*manager*/ return new String[] {"Reference"}; 3595 case 637428636: /*controller*/ return new String[] {"Reference"}; 3596 case 1964406686: /*sourceAttachment*/ return new String[] {"Attachment"}; 3597 case -244259472: /*sourceReference*/ return new String[] {"Reference"}; 3598 case -1780301690: /*regulatoryBasis*/ return new String[] {"CodeableConcept"}; 3599 case 2138287660: /*policyBasis*/ return new String[] {}; 3600 case 1593537919: /*policyText*/ return new String[] {"Reference"}; 3601 case -1484401125: /*verification*/ return new String[] {}; 3602 case 565719004: /*decision*/ return new String[] {"code"}; 3603 case -547120939: /*provision*/ return new String[] {}; 3604 default: return super.getTypesForProperty(hash, name); 3605 } 3606 3607 } 3608 3609 @Override 3610 public Base addChild(String name) throws FHIRException { 3611 if (name.equals("identifier")) { 3612 return addIdentifier(); 3613 } 3614 else if (name.equals("status")) { 3615 throw new FHIRException("Cannot call addChild on a singleton property Consent.status"); 3616 } 3617 else if (name.equals("category")) { 3618 return addCategory(); 3619 } 3620 else if (name.equals("subject")) { 3621 this.subject = new Reference(); 3622 return this.subject; 3623 } 3624 else if (name.equals("date")) { 3625 throw new FHIRException("Cannot call addChild on a singleton property Consent.date"); 3626 } 3627 else if (name.equals("period")) { 3628 this.period = new Period(); 3629 return this.period; 3630 } 3631 else if (name.equals("grantor")) { 3632 return addGrantor(); 3633 } 3634 else if (name.equals("grantee")) { 3635 return addGrantee(); 3636 } 3637 else if (name.equals("manager")) { 3638 return addManager(); 3639 } 3640 else if (name.equals("controller")) { 3641 return addController(); 3642 } 3643 else if (name.equals("sourceAttachment")) { 3644 return addSourceAttachment(); 3645 } 3646 else if (name.equals("sourceReference")) { 3647 return addSourceReference(); 3648 } 3649 else if (name.equals("regulatoryBasis")) { 3650 return addRegulatoryBasis(); 3651 } 3652 else if (name.equals("policyBasis")) { 3653 this.policyBasis = new ConsentPolicyBasisComponent(); 3654 return this.policyBasis; 3655 } 3656 else if (name.equals("policyText")) { 3657 return addPolicyText(); 3658 } 3659 else if (name.equals("verification")) { 3660 return addVerification(); 3661 } 3662 else if (name.equals("decision")) { 3663 throw new FHIRException("Cannot call addChild on a singleton property Consent.decision"); 3664 } 3665 else if (name.equals("provision")) { 3666 return addProvision(); 3667 } 3668 else 3669 return super.addChild(name); 3670 } 3671 3672 public String fhirType() { 3673 return "Consent"; 3674 3675 } 3676 3677 public Consent copy() { 3678 Consent dst = new Consent(); 3679 copyValues(dst); 3680 return dst; 3681 } 3682 3683 public void copyValues(Consent dst) { 3684 super.copyValues(dst); 3685 if (identifier != null) { 3686 dst.identifier = new ArrayList<Identifier>(); 3687 for (Identifier i : identifier) 3688 dst.identifier.add(i.copy()); 3689 }; 3690 dst.status = status == null ? null : status.copy(); 3691 if (category != null) { 3692 dst.category = new ArrayList<CodeableConcept>(); 3693 for (CodeableConcept i : category) 3694 dst.category.add(i.copy()); 3695 }; 3696 dst.subject = subject == null ? null : subject.copy(); 3697 dst.date = date == null ? null : date.copy(); 3698 dst.period = period == null ? null : period.copy(); 3699 if (grantor != null) { 3700 dst.grantor = new ArrayList<Reference>(); 3701 for (Reference i : grantor) 3702 dst.grantor.add(i.copy()); 3703 }; 3704 if (grantee != null) { 3705 dst.grantee = new ArrayList<Reference>(); 3706 for (Reference i : grantee) 3707 dst.grantee.add(i.copy()); 3708 }; 3709 if (manager != null) { 3710 dst.manager = new ArrayList<Reference>(); 3711 for (Reference i : manager) 3712 dst.manager.add(i.copy()); 3713 }; 3714 if (controller != null) { 3715 dst.controller = new ArrayList<Reference>(); 3716 for (Reference i : controller) 3717 dst.controller.add(i.copy()); 3718 }; 3719 if (sourceAttachment != null) { 3720 dst.sourceAttachment = new ArrayList<Attachment>(); 3721 for (Attachment i : sourceAttachment) 3722 dst.sourceAttachment.add(i.copy()); 3723 }; 3724 if (sourceReference != null) { 3725 dst.sourceReference = new ArrayList<Reference>(); 3726 for (Reference i : sourceReference) 3727 dst.sourceReference.add(i.copy()); 3728 }; 3729 if (regulatoryBasis != null) { 3730 dst.regulatoryBasis = new ArrayList<CodeableConcept>(); 3731 for (CodeableConcept i : regulatoryBasis) 3732 dst.regulatoryBasis.add(i.copy()); 3733 }; 3734 dst.policyBasis = policyBasis == null ? null : policyBasis.copy(); 3735 if (policyText != null) { 3736 dst.policyText = new ArrayList<Reference>(); 3737 for (Reference i : policyText) 3738 dst.policyText.add(i.copy()); 3739 }; 3740 if (verification != null) { 3741 dst.verification = new ArrayList<ConsentVerificationComponent>(); 3742 for (ConsentVerificationComponent i : verification) 3743 dst.verification.add(i.copy()); 3744 }; 3745 dst.decision = decision == null ? null : decision.copy(); 3746 if (provision != null) { 3747 dst.provision = new ArrayList<ProvisionComponent>(); 3748 for (ProvisionComponent i : provision) 3749 dst.provision.add(i.copy()); 3750 }; 3751 } 3752 3753 protected Consent typedCopy() { 3754 return copy(); 3755 } 3756 3757 @Override 3758 public boolean equalsDeep(Base other_) { 3759 if (!super.equalsDeep(other_)) 3760 return false; 3761 if (!(other_ instanceof Consent)) 3762 return false; 3763 Consent o = (Consent) other_; 3764 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 3765 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) && compareDeep(period, o.period, true) 3766 && compareDeep(grantor, o.grantor, true) && compareDeep(grantee, o.grantee, true) && compareDeep(manager, o.manager, true) 3767 && compareDeep(controller, o.controller, true) && compareDeep(sourceAttachment, o.sourceAttachment, true) 3768 && compareDeep(sourceReference, o.sourceReference, true) && compareDeep(regulatoryBasis, o.regulatoryBasis, true) 3769 && compareDeep(policyBasis, o.policyBasis, true) && compareDeep(policyText, o.policyText, true) 3770 && compareDeep(verification, o.verification, true) && compareDeep(decision, o.decision, true) && compareDeep(provision, o.provision, true) 3771 ; 3772 } 3773 3774 @Override 3775 public boolean equalsShallow(Base other_) { 3776 if (!super.equalsShallow(other_)) 3777 return false; 3778 if (!(other_ instanceof Consent)) 3779 return false; 3780 Consent o = (Consent) other_; 3781 return compareValues(status, o.status, true) && compareValues(date, o.date, true) && compareValues(decision, o.decision, true) 3782 ; 3783 } 3784 3785 public boolean isEmpty() { 3786 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 3787 , subject, date, period, grantor, grantee, manager, controller, sourceAttachment 3788 , sourceReference, regulatoryBasis, policyBasis, policyText, verification, decision 3789 , provision); 3790 } 3791 3792 @Override 3793 public ResourceType getResourceType() { 3794 return ResourceType.Consent; 3795 } 3796 3797 /** 3798 * Search parameter: <b>action</b> 3799 * <p> 3800 * Description: <b>Actions controlled by this rule</b><br> 3801 * Type: <b>token</b><br> 3802 * Path: <b>Consent.provision.action</b><br> 3803 * </p> 3804 */ 3805 @SearchParamDefinition(name="action", path="Consent.provision.action", description="Actions controlled by this rule", type="token" ) 3806 public static final String SP_ACTION = "action"; 3807 /** 3808 * <b>Fluent Client</b> search parameter constant for <b>action</b> 3809 * <p> 3810 * Description: <b>Actions controlled by this rule</b><br> 3811 * Type: <b>token</b><br> 3812 * Path: <b>Consent.provision.action</b><br> 3813 * </p> 3814 */ 3815 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTION); 3816 3817 /** 3818 * Search parameter: <b>actor</b> 3819 * <p> 3820 * Description: <b>Resource for the actor (or group, by role)</b><br> 3821 * Type: <b>reference</b><br> 3822 * Path: <b>Consent.provision.actor.reference</b><br> 3823 * </p> 3824 */ 3825 @SearchParamDefinition(name="actor", path="Consent.provision.actor.reference", description="Resource for the actor (or group, by role)", type="reference", target={CareTeam.class, Device.class, Group.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3826 public static final String SP_ACTOR = "actor"; 3827 /** 3828 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 3829 * <p> 3830 * Description: <b>Resource for the actor (or group, by role)</b><br> 3831 * Type: <b>reference</b><br> 3832 * Path: <b>Consent.provision.actor.reference</b><br> 3833 * </p> 3834 */ 3835 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 3836 3837/** 3838 * Constant for fluent queries to be used to add include statements. Specifies 3839 * the path value of "<b>Consent:actor</b>". 3840 */ 3841 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Consent:actor").toLocked(); 3842 3843 /** 3844 * Search parameter: <b>category</b> 3845 * <p> 3846 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 3847 * Type: <b>token</b><br> 3848 * Path: <b>Consent.category</b><br> 3849 * </p> 3850 */ 3851 @SearchParamDefinition(name="category", path="Consent.category", description="Classification of the consent statement - for indexing/retrieval", type="token" ) 3852 public static final String SP_CATEGORY = "category"; 3853 /** 3854 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3855 * <p> 3856 * Description: <b>Classification of the consent statement - for indexing/retrieval</b><br> 3857 * Type: <b>token</b><br> 3858 * Path: <b>Consent.category</b><br> 3859 * </p> 3860 */ 3861 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3862 3863 /** 3864 * Search parameter: <b>controller</b> 3865 * <p> 3866 * Description: <b>Consent Enforcer</b><br> 3867 * Type: <b>reference</b><br> 3868 * Path: <b>Consent.controller</b><br> 3869 * </p> 3870 */ 3871 @SearchParamDefinition(name="controller", path="Consent.controller", description="Consent Enforcer", type="reference", target={HealthcareService.class, Organization.class, Patient.class, Practitioner.class } ) 3872 public static final String SP_CONTROLLER = "controller"; 3873 /** 3874 * <b>Fluent Client</b> search parameter constant for <b>controller</b> 3875 * <p> 3876 * Description: <b>Consent Enforcer</b><br> 3877 * Type: <b>reference</b><br> 3878 * Path: <b>Consent.controller</b><br> 3879 * </p> 3880 */ 3881 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTROLLER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTROLLER); 3882 3883/** 3884 * Constant for fluent queries to be used to add include statements. Specifies 3885 * the path value of "<b>Consent:controller</b>". 3886 */ 3887 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTROLLER = new ca.uhn.fhir.model.api.Include("Consent:controller").toLocked(); 3888 3889 /** 3890 * Search parameter: <b>data</b> 3891 * <p> 3892 * Description: <b>The actual data reference</b><br> 3893 * Type: <b>reference</b><br> 3894 * Path: <b>Consent.provision.data.reference</b><br> 3895 * </p> 3896 */ 3897 @SearchParamDefinition(name="data", path="Consent.provision.data.reference", description="The actual data reference", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3898 public static final String SP_DATA = "data"; 3899 /** 3900 * <b>Fluent Client</b> search parameter constant for <b>data</b> 3901 * <p> 3902 * Description: <b>The actual data reference</b><br> 3903 * Type: <b>reference</b><br> 3904 * Path: <b>Consent.provision.data.reference</b><br> 3905 * </p> 3906 */ 3907 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DATA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DATA); 3908 3909/** 3910 * Constant for fluent queries to be used to add include statements. Specifies 3911 * the path value of "<b>Consent:data</b>". 3912 */ 3913 public static final ca.uhn.fhir.model.api.Include INCLUDE_DATA = new ca.uhn.fhir.model.api.Include("Consent:data").toLocked(); 3914 3915 /** 3916 * Search parameter: <b>grantee</b> 3917 * <p> 3918 * Description: <b>Who is agreeing to the policy and rules</b><br> 3919 * Type: <b>reference</b><br> 3920 * Path: <b>Consent.grantee</b><br> 3921 * </p> 3922 */ 3923 @SearchParamDefinition(name="grantee", path="Consent.grantee", description="Who is agreeing to the policy and rules", type="reference", target={CareTeam.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3924 public static final String SP_GRANTEE = "grantee"; 3925 /** 3926 * <b>Fluent Client</b> search parameter constant for <b>grantee</b> 3927 * <p> 3928 * Description: <b>Who is agreeing to the policy and rules</b><br> 3929 * Type: <b>reference</b><br> 3930 * Path: <b>Consent.grantee</b><br> 3931 * </p> 3932 */ 3933 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GRANTEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GRANTEE); 3934 3935/** 3936 * Constant for fluent queries to be used to add include statements. Specifies 3937 * the path value of "<b>Consent:grantee</b>". 3938 */ 3939 public static final ca.uhn.fhir.model.api.Include INCLUDE_GRANTEE = new ca.uhn.fhir.model.api.Include("Consent:grantee").toLocked(); 3940 3941 /** 3942 * Search parameter: <b>manager</b> 3943 * <p> 3944 * Description: <b>Consent workflow management</b><br> 3945 * Type: <b>reference</b><br> 3946 * Path: <b>Consent.manager</b><br> 3947 * </p> 3948 */ 3949 @SearchParamDefinition(name="manager", path="Consent.manager", description="Consent workflow management", type="reference", target={HealthcareService.class, Organization.class, Patient.class, Practitioner.class } ) 3950 public static final String SP_MANAGER = "manager"; 3951 /** 3952 * <b>Fluent Client</b> search parameter constant for <b>manager</b> 3953 * <p> 3954 * Description: <b>Consent workflow management</b><br> 3955 * Type: <b>reference</b><br> 3956 * Path: <b>Consent.manager</b><br> 3957 * </p> 3958 */ 3959 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANAGER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANAGER); 3960 3961/** 3962 * Constant for fluent queries to be used to add include statements. Specifies 3963 * the path value of "<b>Consent:manager</b>". 3964 */ 3965 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANAGER = new ca.uhn.fhir.model.api.Include("Consent:manager").toLocked(); 3966 3967 /** 3968 * Search parameter: <b>period</b> 3969 * <p> 3970 * Description: <b>Timeframe for this rule</b><br> 3971 * Type: <b>date</b><br> 3972 * Path: <b>Consent.provision.period</b><br> 3973 * </p> 3974 */ 3975 @SearchParamDefinition(name="period", path="Consent.provision.period", description="Timeframe for this rule", type="date" ) 3976 public static final String SP_PERIOD = "period"; 3977 /** 3978 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3979 * <p> 3980 * Description: <b>Timeframe for this rule</b><br> 3981 * Type: <b>date</b><br> 3982 * Path: <b>Consent.provision.period</b><br> 3983 * </p> 3984 */ 3985 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 3986 3987 /** 3988 * Search parameter: <b>purpose</b> 3989 * <p> 3990 * Description: <b>Context of activities covered by this rule</b><br> 3991 * Type: <b>token</b><br> 3992 * Path: <b>Consent.provision.purpose</b><br> 3993 * </p> 3994 */ 3995 @SearchParamDefinition(name="purpose", path="Consent.provision.purpose", description="Context of activities covered by this rule", type="token" ) 3996 public static final String SP_PURPOSE = "purpose"; 3997 /** 3998 * <b>Fluent Client</b> search parameter constant for <b>purpose</b> 3999 * <p> 4000 * Description: <b>Context of activities covered by this rule</b><br> 4001 * Type: <b>token</b><br> 4002 * Path: <b>Consent.provision.purpose</b><br> 4003 * </p> 4004 */ 4005 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PURPOSE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PURPOSE); 4006 4007 /** 4008 * Search parameter: <b>security-label</b> 4009 * <p> 4010 * Description: <b>Security Labels that define affected resources</b><br> 4011 * Type: <b>token</b><br> 4012 * Path: <b>Consent.provision.securityLabel</b><br> 4013 * </p> 4014 */ 4015 @SearchParamDefinition(name="security-label", path="Consent.provision.securityLabel", description="Security Labels that define affected resources", type="token" ) 4016 public static final String SP_SECURITY_LABEL = "security-label"; 4017 /** 4018 * <b>Fluent Client</b> search parameter constant for <b>security-label</b> 4019 * <p> 4020 * Description: <b>Security Labels that define affected resources</b><br> 4021 * Type: <b>token</b><br> 4022 * Path: <b>Consent.provision.securityLabel</b><br> 4023 * </p> 4024 */ 4025 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_LABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITY_LABEL); 4026 4027 /** 4028 * Search parameter: <b>source-reference</b> 4029 * <p> 4030 * Description: <b>Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse</b><br> 4031 * Type: <b>reference</b><br> 4032 * Path: <b>Consent.sourceReference</b><br> 4033 * </p> 4034 */ 4035 @SearchParamDefinition(name="source-reference", path="Consent.sourceReference", description="Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse", type="reference", target={Consent.class, Contract.class, DocumentReference.class, QuestionnaireResponse.class } ) 4036 public static final String SP_SOURCE_REFERENCE = "source-reference"; 4037 /** 4038 * <b>Fluent Client</b> search parameter constant for <b>source-reference</b> 4039 * <p> 4040 * Description: <b>Search by reference to a Consent, DocumentReference, Contract or QuestionnaireResponse</b><br> 4041 * Type: <b>reference</b><br> 4042 * Path: <b>Consent.sourceReference</b><br> 4043 * </p> 4044 */ 4045 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE_REFERENCE); 4046 4047/** 4048 * Constant for fluent queries to be used to add include statements. Specifies 4049 * the path value of "<b>Consent:source-reference</b>". 4050 */ 4051 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE_REFERENCE = new ca.uhn.fhir.model.api.Include("Consent:source-reference").toLocked(); 4052 4053 /** 4054 * Search parameter: <b>status</b> 4055 * <p> 4056 * Description: <b>draft | active | inactive | entered-in-error | unknown</b><br> 4057 * Type: <b>token</b><br> 4058 * Path: <b>Consent.status</b><br> 4059 * </p> 4060 */ 4061 @SearchParamDefinition(name="status", path="Consent.status", description="draft | active | inactive | entered-in-error | unknown", type="token" ) 4062 public static final String SP_STATUS = "status"; 4063 /** 4064 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4065 * <p> 4066 * Description: <b>draft | active | inactive | entered-in-error | unknown</b><br> 4067 * Type: <b>token</b><br> 4068 * Path: <b>Consent.status</b><br> 4069 * </p> 4070 */ 4071 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4072 4073 /** 4074 * Search parameter: <b>subject</b> 4075 * <p> 4076 * Description: <b>Who the consent applies to</b><br> 4077 * Type: <b>reference</b><br> 4078 * Path: <b>Consent.subject</b><br> 4079 * </p> 4080 */ 4081 @SearchParamDefinition(name="subject", path="Consent.subject", description="Who the consent applies to", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class, Practitioner.class } ) 4082 public static final String SP_SUBJECT = "subject"; 4083 /** 4084 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4085 * <p> 4086 * Description: <b>Who the consent applies to</b><br> 4087 * Type: <b>reference</b><br> 4088 * Path: <b>Consent.subject</b><br> 4089 * </p> 4090 */ 4091 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4092 4093/** 4094 * Constant for fluent queries to be used to add include statements. Specifies 4095 * the path value of "<b>Consent:subject</b>". 4096 */ 4097 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Consent:subject").toLocked(); 4098 4099 /** 4100 * Search parameter: <b>verified-date</b> 4101 * <p> 4102 * Description: <b>When consent verified</b><br> 4103 * Type: <b>date</b><br> 4104 * Path: <b>Consent.verification.verificationDate</b><br> 4105 * </p> 4106 */ 4107 @SearchParamDefinition(name="verified-date", path="Consent.verification.verificationDate", description="When consent verified", type="date" ) 4108 public static final String SP_VERIFIED_DATE = "verified-date"; 4109 /** 4110 * <b>Fluent Client</b> search parameter constant for <b>verified-date</b> 4111 * <p> 4112 * Description: <b>When consent verified</b><br> 4113 * Type: <b>date</b><br> 4114 * Path: <b>Consent.verification.verificationDate</b><br> 4115 * </p> 4116 */ 4117 public static final ca.uhn.fhir.rest.gclient.DateClientParam VERIFIED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_VERIFIED_DATE); 4118 4119 /** 4120 * Search parameter: <b>verified</b> 4121 * <p> 4122 * Description: <b>Has been verified</b><br> 4123 * Type: <b>token</b><br> 4124 * Path: <b>Consent.verification.verified</b><br> 4125 * </p> 4126 */ 4127 @SearchParamDefinition(name="verified", path="Consent.verification.verified", description="Has been verified", type="token" ) 4128 public static final String SP_VERIFIED = "verified"; 4129 /** 4130 * <b>Fluent Client</b> search parameter constant for <b>verified</b> 4131 * <p> 4132 * Description: <b>Has been verified</b><br> 4133 * Type: <b>token</b><br> 4134 * Path: <b>Consent.verification.verified</b><br> 4135 * </p> 4136 */ 4137 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFIED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERIFIED); 4138 4139 /** 4140 * Search parameter: <b>date</b> 4141 * <p> 4142 * Description: <b>Multiple Resources: 4143 4144* [AdverseEvent](adverseevent.html): When the event occurred 4145* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4146* [Appointment](appointment.html): Appointment date/time. 4147* [AuditEvent](auditevent.html): Time when the event was recorded 4148* [CarePlan](careplan.html): Time period plan covers 4149* [CareTeam](careteam.html): A date within the coverage time period. 4150* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4151* [Composition](composition.html): Composition editing time 4152* [Consent](consent.html): When consent was agreed to 4153* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4154* [DocumentReference](documentreference.html): When this document reference was created 4155* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4156* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4157* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4158* [Flag](flag.html): Time period when flag is active 4159* [Immunization](immunization.html): Vaccination (non)-Administration Date 4160* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4161* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4162* [Invoice](invoice.html): Invoice date / posting date 4163* [List](list.html): When the list was prepared 4164* [MeasureReport](measurereport.html): The date of the measure report 4165* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4166* [Observation](observation.html): Clinically relevant time/time-period for observation 4167* [Procedure](procedure.html): When the procedure occurred or is occurring 4168* [ResearchSubject](researchsubject.html): Start and end of participation 4169* [RiskAssessment](riskassessment.html): When was assessment made? 4170* [SupplyRequest](supplyrequest.html): When the request was made 4171</b><br> 4172 * Type: <b>date</b><br> 4173 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4174 * </p> 4175 */ 4176 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4177 public static final String SP_DATE = "date"; 4178 /** 4179 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4180 * <p> 4181 * Description: <b>Multiple Resources: 4182 4183* [AdverseEvent](adverseevent.html): When the event occurred 4184* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4185* [Appointment](appointment.html): Appointment date/time. 4186* [AuditEvent](auditevent.html): Time when the event was recorded 4187* [CarePlan](careplan.html): Time period plan covers 4188* [CareTeam](careteam.html): A date within the coverage time period. 4189* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4190* [Composition](composition.html): Composition editing time 4191* [Consent](consent.html): When consent was agreed to 4192* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4193* [DocumentReference](documentreference.html): When this document reference was created 4194* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4195* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4196* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4197* [Flag](flag.html): Time period when flag is active 4198* [Immunization](immunization.html): Vaccination (non)-Administration Date 4199* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4200* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4201* [Invoice](invoice.html): Invoice date / posting date 4202* [List](list.html): When the list was prepared 4203* [MeasureReport](measurereport.html): The date of the measure report 4204* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4205* [Observation](observation.html): Clinically relevant time/time-period for observation 4206* [Procedure](procedure.html): When the procedure occurred or is occurring 4207* [ResearchSubject](researchsubject.html): Start and end of participation 4208* [RiskAssessment](riskassessment.html): When was assessment made? 4209* [SupplyRequest](supplyrequest.html): When the request was made 4210</b><br> 4211 * Type: <b>date</b><br> 4212 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4213 * </p> 4214 */ 4215 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4216 4217 /** 4218 * Search parameter: <b>identifier</b> 4219 * <p> 4220 * Description: <b>Multiple Resources: 4221 4222* [Account](account.html): Account number 4223* [AdverseEvent](adverseevent.html): Business identifier for the event 4224* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4225* [Appointment](appointment.html): An Identifier of the Appointment 4226* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4227* [Basic](basic.html): Business identifier 4228* [BodyStructure](bodystructure.html): Bodystructure identifier 4229* [CarePlan](careplan.html): External Ids for this plan 4230* [CareTeam](careteam.html): External Ids for this team 4231* [ChargeItem](chargeitem.html): Business Identifier for item 4232* [Claim](claim.html): The primary identifier of the financial resource 4233* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4234* [ClinicalImpression](clinicalimpression.html): Business identifier 4235* [Communication](communication.html): Unique identifier 4236* [CommunicationRequest](communicationrequest.html): Unique identifier 4237* [Composition](composition.html): Version-independent identifier for the Composition 4238* [Condition](condition.html): A unique identifier of the condition record 4239* [Consent](consent.html): Identifier for this record (external references) 4240* [Contract](contract.html): The identity of the contract 4241* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4242* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4243* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4244* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4245* [DeviceRequest](devicerequest.html): Business identifier for request/order 4246* [DeviceUsage](deviceusage.html): Search by identifier 4247* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4248* [DocumentReference](documentreference.html): Identifier of the attachment binary 4249* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4250* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4251* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4252* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4253* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4254* [Flag](flag.html): Business identifier 4255* [Goal](goal.html): External Ids for this goal 4256* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4257* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4258* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4259* [Immunization](immunization.html): Business identifier 4260* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4261* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4262* [Invoice](invoice.html): Business Identifier for item 4263* [List](list.html): Business identifier 4264* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4265* [Medication](medication.html): Returns medications with this external identifier 4266* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4267* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4268* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4269* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4270* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4271* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4272* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4273* [Observation](observation.html): The unique id for a particular observation 4274* [Person](person.html): A person Identifier 4275* [Procedure](procedure.html): A unique identifier for a procedure 4276* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4277* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4278* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4279* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4280* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4281* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4282* [Specimen](specimen.html): The unique identifier associated with the specimen 4283* [SupplyDelivery](supplydelivery.html): External identifier 4284* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4285* [Task](task.html): Search for a task instance by its business identifier 4286* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4287</b><br> 4288 * Type: <b>token</b><br> 4289 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4290 * </p> 4291 */ 4292 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4293 public static final String SP_IDENTIFIER = "identifier"; 4294 /** 4295 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4296 * <p> 4297 * Description: <b>Multiple Resources: 4298 4299* [Account](account.html): Account number 4300* [AdverseEvent](adverseevent.html): Business identifier for the event 4301* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4302* [Appointment](appointment.html): An Identifier of the Appointment 4303* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4304* [Basic](basic.html): Business identifier 4305* [BodyStructure](bodystructure.html): Bodystructure identifier 4306* [CarePlan](careplan.html): External Ids for this plan 4307* [CareTeam](careteam.html): External Ids for this team 4308* [ChargeItem](chargeitem.html): Business Identifier for item 4309* [Claim](claim.html): The primary identifier of the financial resource 4310* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4311* [ClinicalImpression](clinicalimpression.html): Business identifier 4312* [Communication](communication.html): Unique identifier 4313* [CommunicationRequest](communicationrequest.html): Unique identifier 4314* [Composition](composition.html): Version-independent identifier for the Composition 4315* [Condition](condition.html): A unique identifier of the condition record 4316* [Consent](consent.html): Identifier for this record (external references) 4317* [Contract](contract.html): The identity of the contract 4318* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4319* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4320* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4321* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4322* [DeviceRequest](devicerequest.html): Business identifier for request/order 4323* [DeviceUsage](deviceusage.html): Search by identifier 4324* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4325* [DocumentReference](documentreference.html): Identifier of the attachment binary 4326* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4327* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4328* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4329* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4330* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4331* [Flag](flag.html): Business identifier 4332* [Goal](goal.html): External Ids for this goal 4333* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4334* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4335* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4336* [Immunization](immunization.html): Business identifier 4337* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4338* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4339* [Invoice](invoice.html): Business Identifier for item 4340* [List](list.html): Business identifier 4341* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4342* [Medication](medication.html): Returns medications with this external identifier 4343* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4344* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4345* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4346* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4347* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4348* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4349* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4350* [Observation](observation.html): The unique id for a particular observation 4351* [Person](person.html): A person Identifier 4352* [Procedure](procedure.html): A unique identifier for a procedure 4353* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4354* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4355* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4356* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4357* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4358* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4359* [Specimen](specimen.html): The unique identifier associated with the specimen 4360* [SupplyDelivery](supplydelivery.html): External identifier 4361* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4362* [Task](task.html): Search for a task instance by its business identifier 4363* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4364</b><br> 4365 * Type: <b>token</b><br> 4366 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4367 * </p> 4368 */ 4369 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4370 4371 /** 4372 * Search parameter: <b>patient</b> 4373 * <p> 4374 * Description: <b>Multiple Resources: 4375 4376* [Account](account.html): The entity that caused the expenses 4377* [AdverseEvent](adverseevent.html): Subject impacted by event 4378* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4379* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4380* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4381* [AuditEvent](auditevent.html): Where the activity involved patient data 4382* [Basic](basic.html): Identifies the focus of this resource 4383* [BodyStructure](bodystructure.html): Who this is about 4384* [CarePlan](careplan.html): Who the care plan is for 4385* [CareTeam](careteam.html): Who care team is for 4386* [ChargeItem](chargeitem.html): Individual service was done for/to 4387* [Claim](claim.html): Patient receiving the products or services 4388* [ClaimResponse](claimresponse.html): The subject of care 4389* [ClinicalImpression](clinicalimpression.html): Patient assessed 4390* [Communication](communication.html): Focus of message 4391* [CommunicationRequest](communicationrequest.html): Focus of message 4392* [Composition](composition.html): Who and/or what the composition is about 4393* [Condition](condition.html): Who has the condition? 4394* [Consent](consent.html): Who the consent applies to 4395* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4396* [Coverage](coverage.html): Retrieve coverages for a patient 4397* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4398* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4399* [DetectedIssue](detectedissue.html): Associated patient 4400* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4401* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4402* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4403* [DocumentReference](documentreference.html): Who/what is the subject of the document 4404* [Encounter](encounter.html): The patient present at the encounter 4405* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4406* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4407* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4408* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4409* [Flag](flag.html): The identity of a subject to list flags for 4410* [Goal](goal.html): Who this goal is intended for 4411* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4412* [ImagingSelection](imagingselection.html): Who the study is about 4413* [ImagingStudy](imagingstudy.html): Who the study is about 4414* [Immunization](immunization.html): The patient for the vaccination record 4415* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4416* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4417* [Invoice](invoice.html): Recipient(s) of goods and services 4418* [List](list.html): If all resources have the same subject 4419* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4420* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4421* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4422* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4423* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4424* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4425* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4426* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4427* [Observation](observation.html): The subject that the observation is about (if patient) 4428* [Person](person.html): The Person links to this Patient 4429* [Procedure](procedure.html): Search by subject - a patient 4430* [Provenance](provenance.html): Where the activity involved patient data 4431* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4432* [RelatedPerson](relatedperson.html): The patient this related person is related to 4433* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4434* [ResearchSubject](researchsubject.html): Who or what is part of study 4435* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4436* [ServiceRequest](servicerequest.html): Search by subject - a patient 4437* [Specimen](specimen.html): The patient the specimen comes from 4438* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4439* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4440* [Task](task.html): Search by patient 4441* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4442</b><br> 4443 * Type: <b>reference</b><br> 4444 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4445 * </p> 4446 */ 4447 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4448 public static final String SP_PATIENT = "patient"; 4449 /** 4450 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4451 * <p> 4452 * Description: <b>Multiple Resources: 4453 4454* [Account](account.html): The entity that caused the expenses 4455* [AdverseEvent](adverseevent.html): Subject impacted by event 4456* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4457* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4458* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4459* [AuditEvent](auditevent.html): Where the activity involved patient data 4460* [Basic](basic.html): Identifies the focus of this resource 4461* [BodyStructure](bodystructure.html): Who this is about 4462* [CarePlan](careplan.html): Who the care plan is for 4463* [CareTeam](careteam.html): Who care team is for 4464* [ChargeItem](chargeitem.html): Individual service was done for/to 4465* [Claim](claim.html): Patient receiving the products or services 4466* [ClaimResponse](claimresponse.html): The subject of care 4467* [ClinicalImpression](clinicalimpression.html): Patient assessed 4468* [Communication](communication.html): Focus of message 4469* [CommunicationRequest](communicationrequest.html): Focus of message 4470* [Composition](composition.html): Who and/or what the composition is about 4471* [Condition](condition.html): Who has the condition? 4472* [Consent](consent.html): Who the consent applies to 4473* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4474* [Coverage](coverage.html): Retrieve coverages for a patient 4475* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4476* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4477* [DetectedIssue](detectedissue.html): Associated patient 4478* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4479* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4480* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4481* [DocumentReference](documentreference.html): Who/what is the subject of the document 4482* [Encounter](encounter.html): The patient present at the encounter 4483* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4484* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4485* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4486* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4487* [Flag](flag.html): The identity of a subject to list flags for 4488* [Goal](goal.html): Who this goal is intended for 4489* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4490* [ImagingSelection](imagingselection.html): Who the study is about 4491* [ImagingStudy](imagingstudy.html): Who the study is about 4492* [Immunization](immunization.html): The patient for the vaccination record 4493* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4494* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4495* [Invoice](invoice.html): Recipient(s) of goods and services 4496* [List](list.html): If all resources have the same subject 4497* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4498* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4499* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4500* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4501* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4502* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4503* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4504* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4505* [Observation](observation.html): The subject that the observation is about (if patient) 4506* [Person](person.html): The Person links to this Patient 4507* [Procedure](procedure.html): Search by subject - a patient 4508* [Provenance](provenance.html): Where the activity involved patient data 4509* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4510* [RelatedPerson](relatedperson.html): The patient this related person is related to 4511* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4512* [ResearchSubject](researchsubject.html): Who or what is part of study 4513* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4514* [ServiceRequest](servicerequest.html): Search by subject - a patient 4515* [Specimen](specimen.html): The patient the specimen comes from 4516* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4517* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4518* [Task](task.html): Search by patient 4519* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4520</b><br> 4521 * Type: <b>reference</b><br> 4522 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4523 * </p> 4524 */ 4525 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4526 4527/** 4528 * Constant for fluent queries to be used to add include statements. Specifies 4529 * the path value of "<b>Consent:patient</b>". 4530 */ 4531 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Consent:patient").toLocked(); 4532 4533 4534} 4535