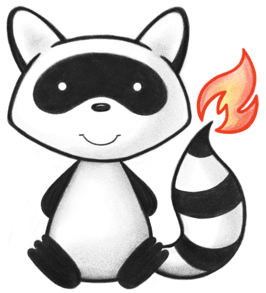
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem; 049/** 050 * ContactDetail Type: Specifies contact information for a person or organization. 051 */ 052@DatatypeDef(name="ContactDetail") 053public class ContactDetail extends DataType implements ICompositeType { 054 055 /** 056 * The name of an individual to contact. 057 */ 058 @Child(name = "name", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Name of an individual to contact", formalDefinition="The name of an individual to contact." ) 060 protected StringType name; 061 062 /** 063 * The contact details for the individual (if a name was provided) or the organization. 064 */ 065 @Child(name = "telecom", type = {ContactPoint.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 066 @Description(shortDefinition="Contact details for individual or organization", formalDefinition="The contact details for the individual (if a name was provided) or the organization." ) 067 protected List<ContactPoint> telecom; 068 069 private static final long serialVersionUID = 816838773L; 070 071 /** 072 * Constructor 073 */ 074 public ContactDetail() { 075 super(); 076 } 077 078 /** 079 * @return {@link #name} (The name of an individual to contact.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 080 */ 081 public StringType getNameElement() { 082 if (this.name == null) 083 if (Configuration.errorOnAutoCreate()) 084 throw new Error("Attempt to auto-create ContactDetail.name"); 085 else if (Configuration.doAutoCreate()) 086 this.name = new StringType(); // bb 087 return this.name; 088 } 089 090 public boolean hasNameElement() { 091 return this.name != null && !this.name.isEmpty(); 092 } 093 094 public boolean hasName() { 095 return this.name != null && !this.name.isEmpty(); 096 } 097 098 /** 099 * @param value {@link #name} (The name of an individual to contact.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 100 */ 101 public ContactDetail setNameElement(StringType value) { 102 this.name = value; 103 return this; 104 } 105 106 /** 107 * @return The name of an individual to contact. 108 */ 109 public String getName() { 110 return this.name == null ? null : this.name.getValue(); 111 } 112 113 /** 114 * @param value The name of an individual to contact. 115 */ 116 public ContactDetail setName(String value) { 117 if (Utilities.noString(value)) 118 this.name = null; 119 else { 120 if (this.name == null) 121 this.name = new StringType(); 122 this.name.setValue(value); 123 } 124 return this; 125 } 126 127 /** 128 * @return {@link #telecom} (The contact details for the individual (if a name was provided) or the organization.) 129 */ 130 public List<ContactPoint> getTelecom() { 131 if (this.telecom == null) 132 this.telecom = new ArrayList<ContactPoint>(); 133 return this.telecom; 134 } 135 136 /** 137 * @return Returns a reference to <code>this</code> for easy method chaining 138 */ 139 public ContactDetail setTelecom(List<ContactPoint> theTelecom) { 140 this.telecom = theTelecom; 141 return this; 142 } 143 144 public boolean hasTelecom() { 145 if (this.telecom == null) 146 return false; 147 for (ContactPoint item : this.telecom) 148 if (!item.isEmpty()) 149 return true; 150 return false; 151 } 152 153 public ContactPoint addTelecom() { //3 154 ContactPoint t = new ContactPoint(); 155 if (this.telecom == null) 156 this.telecom = new ArrayList<ContactPoint>(); 157 this.telecom.add(t); 158 return t; 159 } 160 161 public ContactDetail addTelecom(ContactPoint t) { //3 162 if (t == null) 163 return this; 164 if (this.telecom == null) 165 this.telecom = new ArrayList<ContactPoint>(); 166 this.telecom.add(t); 167 return this; 168 } 169 170 /** 171 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 172 */ 173 public ContactPoint getTelecomFirstRep() { 174 if (getTelecom().isEmpty()) { 175 addTelecom(); 176 } 177 return getTelecom().get(0); 178 } 179 180 protected void listChildren(List<Property> children) { 181 super.listChildren(children); 182 children.add(new Property("name", "string", "The name of an individual to contact.", 0, 1, name)); 183 children.add(new Property("telecom", "ContactPoint", "The contact details for the individual (if a name was provided) or the organization.", 0, java.lang.Integer.MAX_VALUE, telecom)); 184 } 185 186 @Override 187 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 188 switch (_hash) { 189 case 3373707: /*name*/ return new Property("name", "string", "The name of an individual to contact.", 0, 1, name); 190 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "The contact details for the individual (if a name was provided) or the organization.", 0, java.lang.Integer.MAX_VALUE, telecom); 191 default: return super.getNamedProperty(_hash, _name, _checkValid); 192 } 193 194 } 195 196 @Override 197 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 198 switch (hash) { 199 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 200 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 201 default: return super.getProperty(hash, name, checkValid); 202 } 203 204 } 205 206 @Override 207 public Base setProperty(int hash, String name, Base value) throws FHIRException { 208 switch (hash) { 209 case 3373707: // name 210 this.name = TypeConvertor.castToString(value); // StringType 211 return value; 212 case -1429363305: // telecom 213 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 214 return value; 215 default: return super.setProperty(hash, name, value); 216 } 217 218 } 219 220 @Override 221 public Base setProperty(String name, Base value) throws FHIRException { 222 if (name.equals("name")) { 223 this.name = TypeConvertor.castToString(value); // StringType 224 } else if (name.equals("telecom")) { 225 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 226 } else 227 return super.setProperty(name, value); 228 return value; 229 } 230 231 @Override 232 public void removeChild(String name, Base value) throws FHIRException { 233 if (name.equals("name")) { 234 this.name = null; 235 } else if (name.equals("telecom")) { 236 this.getTelecom().remove(value); 237 } else 238 super.removeChild(name, value); 239 240 } 241 242 @Override 243 public Base makeProperty(int hash, String name) throws FHIRException { 244 switch (hash) { 245 case 3373707: return getNameElement(); 246 case -1429363305: return addTelecom(); 247 default: return super.makeProperty(hash, name); 248 } 249 250 } 251 252 @Override 253 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 254 switch (hash) { 255 case 3373707: /*name*/ return new String[] {"string"}; 256 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 257 default: return super.getTypesForProperty(hash, name); 258 } 259 260 } 261 262 @Override 263 public Base addChild(String name) throws FHIRException { 264 if (name.equals("name")) { 265 throw new FHIRException("Cannot call addChild on a singleton property ContactDetail.name"); 266 } 267 else if (name.equals("telecom")) { 268 return addTelecom(); 269 } 270 else 271 return super.addChild(name); 272 } 273 274 public String fhirType() { 275 return "ContactDetail"; 276 277 } 278 279 public ContactDetail copy() { 280 ContactDetail dst = new ContactDetail(); 281 copyValues(dst); 282 return dst; 283 } 284 285 public void copyValues(ContactDetail dst) { 286 super.copyValues(dst); 287 dst.name = name == null ? null : name.copy(); 288 if (telecom != null) { 289 dst.telecom = new ArrayList<ContactPoint>(); 290 for (ContactPoint i : telecom) 291 dst.telecom.add(i.copy()); 292 }; 293 } 294 295 protected ContactDetail typedCopy() { 296 return copy(); 297 } 298 299 @Override 300 public boolean equalsDeep(Base other_) { 301 if (!super.equalsDeep(other_)) 302 return false; 303 if (!(other_ instanceof ContactDetail)) 304 return false; 305 ContactDetail o = (ContactDetail) other_; 306 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 307 } 308 309 @Override 310 public boolean equalsShallow(Base other_) { 311 if (!super.equalsShallow(other_)) 312 return false; 313 if (!(other_ instanceof ContactDetail)) 314 return false; 315 ContactDetail o = (ContactDetail) other_; 316 return compareValues(name, o.name, true); 317 } 318 319 public boolean isEmpty() { 320 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, telecom); 321 } 322 323// Manual code (from Configuration.txt): 324 public ContactPoint getEmail() { 325 for (ContactPoint cp : getTelecom()) { 326 if (cp.getSystem() == ContactPointSystem.EMAIL) { 327 return cp; 328 } 329 } 330 return null; 331 } 332 333 public ContactPoint getPhone() { 334 for (ContactPoint cp : getTelecom()) { 335 if (cp.getSystem() == ContactPointSystem.PHONE) { 336 return cp; 337 } 338 } 339 return null; 340 } 341 342 public ContactPoint getUrl() { 343 for (ContactPoint cp : getTelecom()) { 344 if (cp.getSystem() == ContactPointSystem.URL) { 345 return cp; 346 } 347 } 348 return null; 349 } 350 351// end addition 352 353} 354