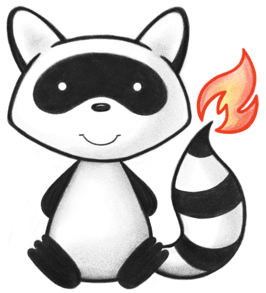
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * ContactPoint Type: Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 050 */ 051@DatatypeDef(name="ContactPoint") 052public class ContactPoint extends DataType implements ICompositeType { 053 054 public enum ContactPointSystem { 055 /** 056 * The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required. 057 */ 058 PHONE, 059 /** 060 * The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required. 061 */ 062 FAX, 063 /** 064 * The value is an email address. 065 */ 066 EMAIL, 067 /** 068 * The value is a pager number. These may be local pager numbers that are only usable on a particular pager system. 069 */ 070 PAGER, 071 /** 072 * A contact that is not a phone, fax, pager or email address and is expressed as a URL. This is intended for various institutional or personal contacts including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for email addresses. 073 */ 074 URL, 075 /** 076 * A contact that can be used for sending a sms message (e.g. mobile phones, some landlines). 077 */ 078 SMS, 079 /** 080 * A contact that is not a phone, fax, page or email address and is not expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) Extensions may be used to distinguish \"other\" contact types. 081 */ 082 OTHER, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static ContactPointSystem fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("phone".equals(codeString)) 091 return PHONE; 092 if ("fax".equals(codeString)) 093 return FAX; 094 if ("email".equals(codeString)) 095 return EMAIL; 096 if ("pager".equals(codeString)) 097 return PAGER; 098 if ("url".equals(codeString)) 099 return URL; 100 if ("sms".equals(codeString)) 101 return SMS; 102 if ("other".equals(codeString)) 103 return OTHER; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown ContactPointSystem code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case PHONE: return "phone"; 112 case FAX: return "fax"; 113 case EMAIL: return "email"; 114 case PAGER: return "pager"; 115 case URL: return "url"; 116 case SMS: return "sms"; 117 case OTHER: return "other"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case PHONE: return "http://hl7.org/fhir/contact-point-system"; 125 case FAX: return "http://hl7.org/fhir/contact-point-system"; 126 case EMAIL: return "http://hl7.org/fhir/contact-point-system"; 127 case PAGER: return "http://hl7.org/fhir/contact-point-system"; 128 case URL: return "http://hl7.org/fhir/contact-point-system"; 129 case SMS: return "http://hl7.org/fhir/contact-point-system"; 130 case OTHER: return "http://hl7.org/fhir/contact-point-system"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case PHONE: return "The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 138 case FAX: return "The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 139 case EMAIL: return "The value is an email address."; 140 case PAGER: return "The value is a pager number. These may be local pager numbers that are only usable on a particular pager system."; 141 case URL: return "A contact that is not a phone, fax, pager or email address and is expressed as a URL. This is intended for various institutional or personal contacts including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for email addresses."; 142 case SMS: return "A contact that can be used for sending a sms message (e.g. mobile phones, some landlines)."; 143 case OTHER: return "A contact that is not a phone, fax, page or email address and is not expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) Extensions may be used to distinguish \"other\" contact types."; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case PHONE: return "Phone"; 151 case FAX: return "Fax"; 152 case EMAIL: return "Email"; 153 case PAGER: return "Pager"; 154 case URL: return "URL"; 155 case SMS: return "SMS"; 156 case OTHER: return "Other"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class ContactPointSystemEnumFactory implements EnumFactory<ContactPointSystem> { 164 public ContactPointSystem fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("phone".equals(codeString)) 169 return ContactPointSystem.PHONE; 170 if ("fax".equals(codeString)) 171 return ContactPointSystem.FAX; 172 if ("email".equals(codeString)) 173 return ContactPointSystem.EMAIL; 174 if ("pager".equals(codeString)) 175 return ContactPointSystem.PAGER; 176 if ("url".equals(codeString)) 177 return ContactPointSystem.URL; 178 if ("sms".equals(codeString)) 179 return ContactPointSystem.SMS; 180 if ("other".equals(codeString)) 181 return ContactPointSystem.OTHER; 182 throw new IllegalArgumentException("Unknown ContactPointSystem code '"+codeString+"'"); 183 } 184 public Enumeration<ContactPointSystem> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.NULL, code); 189 String codeString = ((PrimitiveType) code).asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.NULL, code); 192 if ("phone".equals(codeString)) 193 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PHONE, code); 194 if ("fax".equals(codeString)) 195 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.FAX, code); 196 if ("email".equals(codeString)) 197 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.EMAIL, code); 198 if ("pager".equals(codeString)) 199 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PAGER, code); 200 if ("url".equals(codeString)) 201 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.URL, code); 202 if ("sms".equals(codeString)) 203 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.SMS, code); 204 if ("other".equals(codeString)) 205 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.OTHER, code); 206 throw new FHIRException("Unknown ContactPointSystem code '"+codeString+"'"); 207 } 208 public String toCode(ContactPointSystem code) { 209 if (code == ContactPointSystem.NULL) 210 return null; 211 if (code == ContactPointSystem.PHONE) 212 return "phone"; 213 if (code == ContactPointSystem.FAX) 214 return "fax"; 215 if (code == ContactPointSystem.EMAIL) 216 return "email"; 217 if (code == ContactPointSystem.PAGER) 218 return "pager"; 219 if (code == ContactPointSystem.URL) 220 return "url"; 221 if (code == ContactPointSystem.SMS) 222 return "sms"; 223 if (code == ContactPointSystem.OTHER) 224 return "other"; 225 return "?"; 226 } 227 public String toSystem(ContactPointSystem code) { 228 return code.getSystem(); 229 } 230 } 231 232 public enum ContactPointUse { 233 /** 234 * A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available. 235 */ 236 HOME, 237 /** 238 * An office contact point. First choice for business related contacts during business hours. 239 */ 240 WORK, 241 /** 242 * A temporary contact point. The period can provide more detailed information. 243 */ 244 TEMP, 245 /** 246 * This contact point is no longer in use (or was never correct, but retained for records). 247 */ 248 OLD, 249 /** 250 * A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business. 251 */ 252 MOBILE, 253 /** 254 * added to help the parsers with the generic types 255 */ 256 NULL; 257 public static ContactPointUse fromCode(String codeString) throws FHIRException { 258 if (codeString == null || "".equals(codeString)) 259 return null; 260 if ("home".equals(codeString)) 261 return HOME; 262 if ("work".equals(codeString)) 263 return WORK; 264 if ("temp".equals(codeString)) 265 return TEMP; 266 if ("old".equals(codeString)) 267 return OLD; 268 if ("mobile".equals(codeString)) 269 return MOBILE; 270 if (Configuration.isAcceptInvalidEnums()) 271 return null; 272 else 273 throw new FHIRException("Unknown ContactPointUse code '"+codeString+"'"); 274 } 275 public String toCode() { 276 switch (this) { 277 case HOME: return "home"; 278 case WORK: return "work"; 279 case TEMP: return "temp"; 280 case OLD: return "old"; 281 case MOBILE: return "mobile"; 282 case NULL: return null; 283 default: return "?"; 284 } 285 } 286 public String getSystem() { 287 switch (this) { 288 case HOME: return "http://hl7.org/fhir/contact-point-use"; 289 case WORK: return "http://hl7.org/fhir/contact-point-use"; 290 case TEMP: return "http://hl7.org/fhir/contact-point-use"; 291 case OLD: return "http://hl7.org/fhir/contact-point-use"; 292 case MOBILE: return "http://hl7.org/fhir/contact-point-use"; 293 case NULL: return null; 294 default: return "?"; 295 } 296 } 297 public String getDefinition() { 298 switch (this) { 299 case HOME: return "A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available."; 300 case WORK: return "An office contact point. First choice for business related contacts during business hours."; 301 case TEMP: return "A temporary contact point. The period can provide more detailed information."; 302 case OLD: return "This contact point is no longer in use (or was never correct, but retained for records)."; 303 case MOBILE: return "A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business."; 304 case NULL: return null; 305 default: return "?"; 306 } 307 } 308 public String getDisplay() { 309 switch (this) { 310 case HOME: return "Home"; 311 case WORK: return "Work"; 312 case TEMP: return "Temp"; 313 case OLD: return "Old"; 314 case MOBILE: return "Mobile"; 315 case NULL: return null; 316 default: return "?"; 317 } 318 } 319 } 320 321 public static class ContactPointUseEnumFactory implements EnumFactory<ContactPointUse> { 322 public ContactPointUse fromCode(String codeString) throws IllegalArgumentException { 323 if (codeString == null || "".equals(codeString)) 324 if (codeString == null || "".equals(codeString)) 325 return null; 326 if ("home".equals(codeString)) 327 return ContactPointUse.HOME; 328 if ("work".equals(codeString)) 329 return ContactPointUse.WORK; 330 if ("temp".equals(codeString)) 331 return ContactPointUse.TEMP; 332 if ("old".equals(codeString)) 333 return ContactPointUse.OLD; 334 if ("mobile".equals(codeString)) 335 return ContactPointUse.MOBILE; 336 throw new IllegalArgumentException("Unknown ContactPointUse code '"+codeString+"'"); 337 } 338 public Enumeration<ContactPointUse> fromType(PrimitiveType<?> code) throws FHIRException { 339 if (code == null) 340 return null; 341 if (code.isEmpty()) 342 return new Enumeration<ContactPointUse>(this, ContactPointUse.NULL, code); 343 String codeString = ((PrimitiveType) code).asStringValue(); 344 if (codeString == null || "".equals(codeString)) 345 return new Enumeration<ContactPointUse>(this, ContactPointUse.NULL, code); 346 if ("home".equals(codeString)) 347 return new Enumeration<ContactPointUse>(this, ContactPointUse.HOME, code); 348 if ("work".equals(codeString)) 349 return new Enumeration<ContactPointUse>(this, ContactPointUse.WORK, code); 350 if ("temp".equals(codeString)) 351 return new Enumeration<ContactPointUse>(this, ContactPointUse.TEMP, code); 352 if ("old".equals(codeString)) 353 return new Enumeration<ContactPointUse>(this, ContactPointUse.OLD, code); 354 if ("mobile".equals(codeString)) 355 return new Enumeration<ContactPointUse>(this, ContactPointUse.MOBILE, code); 356 throw new FHIRException("Unknown ContactPointUse code '"+codeString+"'"); 357 } 358 public String toCode(ContactPointUse code) { 359 if (code == ContactPointUse.NULL) 360 return null; 361 if (code == ContactPointUse.HOME) 362 return "home"; 363 if (code == ContactPointUse.WORK) 364 return "work"; 365 if (code == ContactPointUse.TEMP) 366 return "temp"; 367 if (code == ContactPointUse.OLD) 368 return "old"; 369 if (code == ContactPointUse.MOBILE) 370 return "mobile"; 371 return "?"; 372 } 373 public String toSystem(ContactPointUse code) { 374 return code.getSystem(); 375 } 376 } 377 378 /** 379 * Telecommunications form for contact point - what communications system is required to make use of the contact. 380 */ 381 @Child(name = "system", type = {CodeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 382 @Description(shortDefinition="phone | fax | email | pager | url | sms | other", formalDefinition="Telecommunications form for contact point - what communications system is required to make use of the contact." ) 383 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contact-point-system") 384 protected Enumeration<ContactPointSystem> system; 385 386 /** 387 * The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 388 */ 389 @Child(name = "value", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 390 @Description(shortDefinition="The actual contact point details", formalDefinition="The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address)." ) 391 protected StringType value; 392 393 /** 394 * Identifies the purpose for the contact point. 395 */ 396 @Child(name = "use", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 397 @Description(shortDefinition="home | work | temp | old | mobile - purpose of this contact point", formalDefinition="Identifies the purpose for the contact point." ) 398 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contact-point-use") 399 protected Enumeration<ContactPointUse> use; 400 401 /** 402 * Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values. 403 */ 404 @Child(name = "rank", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=true) 405 @Description(shortDefinition="Specify preferred order of use (1 = highest)", formalDefinition="Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values." ) 406 protected PositiveIntType rank; 407 408 /** 409 * Time period when the contact point was/is in use. 410 */ 411 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 412 @Description(shortDefinition="Time period when the contact point was/is in use", formalDefinition="Time period when the contact point was/is in use." ) 413 protected Period period; 414 415 private static final long serialVersionUID = 1509610874L; 416 417 /** 418 * Constructor 419 */ 420 public ContactPoint() { 421 super(); 422 } 423 424 /** 425 * @return {@link #system} (Telecommunications form for contact point - what communications system is required to make use of the contact.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 426 */ 427 public Enumeration<ContactPointSystem> getSystemElement() { 428 if (this.system == null) 429 if (Configuration.errorOnAutoCreate()) 430 throw new Error("Attempt to auto-create ContactPoint.system"); 431 else if (Configuration.doAutoCreate()) 432 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); // bb 433 return this.system; 434 } 435 436 public boolean hasSystemElement() { 437 return this.system != null && !this.system.isEmpty(); 438 } 439 440 public boolean hasSystem() { 441 return this.system != null && !this.system.isEmpty(); 442 } 443 444 /** 445 * @param value {@link #system} (Telecommunications form for contact point - what communications system is required to make use of the contact.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 446 */ 447 public ContactPoint setSystemElement(Enumeration<ContactPointSystem> value) { 448 this.system = value; 449 return this; 450 } 451 452 /** 453 * @return Telecommunications form for contact point - what communications system is required to make use of the contact. 454 */ 455 public ContactPointSystem getSystem() { 456 return this.system == null ? null : this.system.getValue(); 457 } 458 459 /** 460 * @param value Telecommunications form for contact point - what communications system is required to make use of the contact. 461 */ 462 public ContactPoint setSystem(ContactPointSystem value) { 463 if (value == null) 464 this.system = null; 465 else { 466 if (this.system == null) 467 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); 468 this.system.setValue(value); 469 } 470 return this; 471 } 472 473 /** 474 * @return {@link #value} (The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 475 */ 476 public StringType getValueElement() { 477 if (this.value == null) 478 if (Configuration.errorOnAutoCreate()) 479 throw new Error("Attempt to auto-create ContactPoint.value"); 480 else if (Configuration.doAutoCreate()) 481 this.value = new StringType(); // bb 482 return this.value; 483 } 484 485 public boolean hasValueElement() { 486 return this.value != null && !this.value.isEmpty(); 487 } 488 489 public boolean hasValue() { 490 return this.value != null && !this.value.isEmpty(); 491 } 492 493 /** 494 * @param value {@link #value} (The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 495 */ 496 public ContactPoint setValueElement(StringType value) { 497 this.value = value; 498 return this; 499 } 500 501 /** 502 * @return The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 503 */ 504 public String getValue() { 505 return this.value == null ? null : this.value.getValue(); 506 } 507 508 /** 509 * @param value The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 510 */ 511 public ContactPoint setValue(String value) { 512 if (Utilities.noString(value)) 513 this.value = null; 514 else { 515 if (this.value == null) 516 this.value = new StringType(); 517 this.value.setValue(value); 518 } 519 return this; 520 } 521 522 /** 523 * @return {@link #use} (Identifies the purpose for the contact point.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 524 */ 525 public Enumeration<ContactPointUse> getUseElement() { 526 if (this.use == null) 527 if (Configuration.errorOnAutoCreate()) 528 throw new Error("Attempt to auto-create ContactPoint.use"); 529 else if (Configuration.doAutoCreate()) 530 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); // bb 531 return this.use; 532 } 533 534 public boolean hasUseElement() { 535 return this.use != null && !this.use.isEmpty(); 536 } 537 538 public boolean hasUse() { 539 return this.use != null && !this.use.isEmpty(); 540 } 541 542 /** 543 * @param value {@link #use} (Identifies the purpose for the contact point.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 544 */ 545 public ContactPoint setUseElement(Enumeration<ContactPointUse> value) { 546 this.use = value; 547 return this; 548 } 549 550 /** 551 * @return Identifies the purpose for the contact point. 552 */ 553 public ContactPointUse getUse() { 554 return this.use == null ? null : this.use.getValue(); 555 } 556 557 /** 558 * @param value Identifies the purpose for the contact point. 559 */ 560 public ContactPoint setUse(ContactPointUse value) { 561 if (value == null) 562 this.use = null; 563 else { 564 if (this.use == null) 565 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); 566 this.use.setValue(value); 567 } 568 return this; 569 } 570 571 /** 572 * @return {@link #rank} (Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 573 */ 574 public PositiveIntType getRankElement() { 575 if (this.rank == null) 576 if (Configuration.errorOnAutoCreate()) 577 throw new Error("Attempt to auto-create ContactPoint.rank"); 578 else if (Configuration.doAutoCreate()) 579 this.rank = new PositiveIntType(); // bb 580 return this.rank; 581 } 582 583 public boolean hasRankElement() { 584 return this.rank != null && !this.rank.isEmpty(); 585 } 586 587 public boolean hasRank() { 588 return this.rank != null && !this.rank.isEmpty(); 589 } 590 591 /** 592 * @param value {@link #rank} (Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 593 */ 594 public ContactPoint setRankElement(PositiveIntType value) { 595 this.rank = value; 596 return this; 597 } 598 599 /** 600 * @return Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values. 601 */ 602 public int getRank() { 603 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 604 } 605 606 /** 607 * @param value Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values. 608 */ 609 public ContactPoint setRank(int value) { 610 if (this.rank == null) 611 this.rank = new PositiveIntType(); 612 this.rank.setValue(value); 613 return this; 614 } 615 616 /** 617 * @return {@link #period} (Time period when the contact point was/is in use.) 618 */ 619 public Period getPeriod() { 620 if (this.period == null) 621 if (Configuration.errorOnAutoCreate()) 622 throw new Error("Attempt to auto-create ContactPoint.period"); 623 else if (Configuration.doAutoCreate()) 624 this.period = new Period(); // cc 625 return this.period; 626 } 627 628 public boolean hasPeriod() { 629 return this.period != null && !this.period.isEmpty(); 630 } 631 632 /** 633 * @param value {@link #period} (Time period when the contact point was/is in use.) 634 */ 635 public ContactPoint setPeriod(Period value) { 636 this.period = value; 637 return this; 638 } 639 640 protected void listChildren(List<Property> children) { 641 super.listChildren(children); 642 children.add(new Property("system", "code", "Telecommunications form for contact point - what communications system is required to make use of the contact.", 0, 1, system)); 643 children.add(new Property("value", "string", "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 0, 1, value)); 644 children.add(new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use)); 645 children.add(new Property("rank", "positiveInt", "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.", 0, 1, rank)); 646 children.add(new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, period)); 647 } 648 649 @Override 650 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 651 switch (_hash) { 652 case -887328209: /*system*/ return new Property("system", "code", "Telecommunications form for contact point - what communications system is required to make use of the contact.", 0, 1, system); 653 case 111972721: /*value*/ return new Property("value", "string", "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 0, 1, value); 654 case 116103: /*use*/ return new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use); 655 case 3492908: /*rank*/ return new Property("rank", "positiveInt", "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.", 0, 1, rank); 656 case -991726143: /*period*/ return new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, period); 657 default: return super.getNamedProperty(_hash, _name, _checkValid); 658 } 659 660 } 661 662 @Override 663 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 664 switch (hash) { 665 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // Enumeration<ContactPointSystem> 666 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 667 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<ContactPointUse> 668 case 3492908: /*rank*/ return this.rank == null ? new Base[0] : new Base[] {this.rank}; // PositiveIntType 669 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 670 default: return super.getProperty(hash, name, checkValid); 671 } 672 673 } 674 675 @Override 676 public Base setProperty(int hash, String name, Base value) throws FHIRException { 677 switch (hash) { 678 case -887328209: // system 679 value = new ContactPointSystemEnumFactory().fromType(TypeConvertor.castToCode(value)); 680 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 681 return value; 682 case 111972721: // value 683 this.value = TypeConvertor.castToString(value); // StringType 684 return value; 685 case 116103: // use 686 value = new ContactPointUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 687 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 688 return value; 689 case 3492908: // rank 690 this.rank = TypeConvertor.castToPositiveInt(value); // PositiveIntType 691 return value; 692 case -991726143: // period 693 this.period = TypeConvertor.castToPeriod(value); // Period 694 return value; 695 default: return super.setProperty(hash, name, value); 696 } 697 698 } 699 700 @Override 701 public Base setProperty(String name, Base value) throws FHIRException { 702 if (name.equals("system")) { 703 value = new ContactPointSystemEnumFactory().fromType(TypeConvertor.castToCode(value)); 704 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 705 } else if (name.equals("value")) { 706 this.value = TypeConvertor.castToString(value); // StringType 707 } else if (name.equals("use")) { 708 value = new ContactPointUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 709 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 710 } else if (name.equals("rank")) { 711 this.rank = TypeConvertor.castToPositiveInt(value); // PositiveIntType 712 } else if (name.equals("period")) { 713 this.period = TypeConvertor.castToPeriod(value); // Period 714 } else 715 return super.setProperty(name, value); 716 return value; 717 } 718 719 @Override 720 public void removeChild(String name, Base value) throws FHIRException { 721 if (name.equals("system")) { 722 value = new ContactPointSystemEnumFactory().fromType(TypeConvertor.castToCode(value)); 723 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 724 } else if (name.equals("value")) { 725 this.value = null; 726 } else if (name.equals("use")) { 727 value = new ContactPointUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 728 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 729 } else if (name.equals("rank")) { 730 this.rank = null; 731 } else if (name.equals("period")) { 732 this.period = null; 733 } else 734 super.removeChild(name, value); 735 736 } 737 738 @Override 739 public Base makeProperty(int hash, String name) throws FHIRException { 740 switch (hash) { 741 case -887328209: return getSystemElement(); 742 case 111972721: return getValueElement(); 743 case 116103: return getUseElement(); 744 case 3492908: return getRankElement(); 745 case -991726143: return getPeriod(); 746 default: return super.makeProperty(hash, name); 747 } 748 749 } 750 751 @Override 752 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 753 switch (hash) { 754 case -887328209: /*system*/ return new String[] {"code"}; 755 case 111972721: /*value*/ return new String[] {"string"}; 756 case 116103: /*use*/ return new String[] {"code"}; 757 case 3492908: /*rank*/ return new String[] {"positiveInt"}; 758 case -991726143: /*period*/ return new String[] {"Period"}; 759 default: return super.getTypesForProperty(hash, name); 760 } 761 762 } 763 764 @Override 765 public Base addChild(String name) throws FHIRException { 766 if (name.equals("system")) { 767 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.system"); 768 } 769 else if (name.equals("value")) { 770 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.value"); 771 } 772 else if (name.equals("use")) { 773 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.use"); 774 } 775 else if (name.equals("rank")) { 776 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.rank"); 777 } 778 else if (name.equals("period")) { 779 this.period = new Period(); 780 return this.period; 781 } 782 else 783 return super.addChild(name); 784 } 785 786 public String fhirType() { 787 return "ContactPoint"; 788 789 } 790 791 public ContactPoint copy() { 792 ContactPoint dst = new ContactPoint(); 793 copyValues(dst); 794 return dst; 795 } 796 797 public void copyValues(ContactPoint dst) { 798 super.copyValues(dst); 799 dst.system = system == null ? null : system.copy(); 800 dst.value = value == null ? null : value.copy(); 801 dst.use = use == null ? null : use.copy(); 802 dst.rank = rank == null ? null : rank.copy(); 803 dst.period = period == null ? null : period.copy(); 804 } 805 806 protected ContactPoint typedCopy() { 807 return copy(); 808 } 809 810 @Override 811 public boolean equalsDeep(Base other_) { 812 if (!super.equalsDeep(other_)) 813 return false; 814 if (!(other_ instanceof ContactPoint)) 815 return false; 816 ContactPoint o = (ContactPoint) other_; 817 return compareDeep(system, o.system, true) && compareDeep(value, o.value, true) && compareDeep(use, o.use, true) 818 && compareDeep(rank, o.rank, true) && compareDeep(period, o.period, true); 819 } 820 821 @Override 822 public boolean equalsShallow(Base other_) { 823 if (!super.equalsShallow(other_)) 824 return false; 825 if (!(other_ instanceof ContactPoint)) 826 return false; 827 ContactPoint o = (ContactPoint) other_; 828 return compareValues(system, o.system, true) && compareValues(value, o.value, true) && compareValues(use, o.use, true) 829 && compareValues(rank, o.rank, true); 830 } 831 832 public boolean isEmpty() { 833 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, value, use, rank 834 , period); 835 } 836 837 838} 839