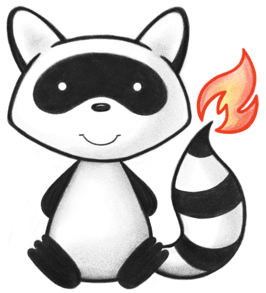
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement. 053 */ 054@ResourceDef(name="Contract", profile="http://hl7.org/fhir/StructureDefinition/Contract") 055public class Contract extends DomainResource { 056 057 public enum ContractResourcePublicationStatusCodes { 058 /** 059 * Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced. 060 */ 061 AMENDED, 062 /** 063 * Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced. 064 */ 065 APPENDED, 066 /** 067 * Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted. 068 */ 069 CANCELLED, 070 /** 071 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended. 072 */ 073 DISPUTED, 074 /** 075 * Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status. 076 */ 077 ENTEREDINERROR, 078 /** 079 * Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active. 080 */ 081 EXECUTABLE, 082 /** 083 * Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed. 084 */ 085 EXECUTED, 086 /** 087 * Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 088 */ 089 NEGOTIABLE, 090 /** 091 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new. 092 */ 093 OFFERED, 094 /** 095 * Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended. 096 */ 097 POLICY, 098 /** 099 * Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled. 100 */ 101 REJECTED, 102 /** 103 * Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded. 104 */ 105 RENEWED, 106 /** 107 * A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified. 108 */ 109 REVOKED, 110 /** 111 * Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated. 112 */ 113 RESOLVED, 114 /** 115 * Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted. 116 */ 117 TERMINATED, 118 /** 119 * added to help the parsers with the generic types 120 */ 121 NULL; 122 public static ContractResourcePublicationStatusCodes fromCode(String codeString) throws FHIRException { 123 if (codeString == null || "".equals(codeString)) 124 return null; 125 if ("amended".equals(codeString)) 126 return AMENDED; 127 if ("appended".equals(codeString)) 128 return APPENDED; 129 if ("cancelled".equals(codeString)) 130 return CANCELLED; 131 if ("disputed".equals(codeString)) 132 return DISPUTED; 133 if ("entered-in-error".equals(codeString)) 134 return ENTEREDINERROR; 135 if ("executable".equals(codeString)) 136 return EXECUTABLE; 137 if ("executed".equals(codeString)) 138 return EXECUTED; 139 if ("negotiable".equals(codeString)) 140 return NEGOTIABLE; 141 if ("offered".equals(codeString)) 142 return OFFERED; 143 if ("policy".equals(codeString)) 144 return POLICY; 145 if ("rejected".equals(codeString)) 146 return REJECTED; 147 if ("renewed".equals(codeString)) 148 return RENEWED; 149 if ("revoked".equals(codeString)) 150 return REVOKED; 151 if ("resolved".equals(codeString)) 152 return RESOLVED; 153 if ("terminated".equals(codeString)) 154 return TERMINATED; 155 if (Configuration.isAcceptInvalidEnums()) 156 return null; 157 else 158 throw new FHIRException("Unknown ContractResourcePublicationStatusCodes code '"+codeString+"'"); 159 } 160 public String toCode() { 161 switch (this) { 162 case AMENDED: return "amended"; 163 case APPENDED: return "appended"; 164 case CANCELLED: return "cancelled"; 165 case DISPUTED: return "disputed"; 166 case ENTEREDINERROR: return "entered-in-error"; 167 case EXECUTABLE: return "executable"; 168 case EXECUTED: return "executed"; 169 case NEGOTIABLE: return "negotiable"; 170 case OFFERED: return "offered"; 171 case POLICY: return "policy"; 172 case REJECTED: return "rejected"; 173 case RENEWED: return "renewed"; 174 case REVOKED: return "revoked"; 175 case RESOLVED: return "resolved"; 176 case TERMINATED: return "terminated"; 177 case NULL: return null; 178 default: return "?"; 179 } 180 } 181 public String getSystem() { 182 switch (this) { 183 case AMENDED: return "http://hl7.org/fhir/contract-publicationstatus"; 184 case APPENDED: return "http://hl7.org/fhir/contract-publicationstatus"; 185 case CANCELLED: return "http://hl7.org/fhir/contract-publicationstatus"; 186 case DISPUTED: return "http://hl7.org/fhir/contract-publicationstatus"; 187 case ENTEREDINERROR: return "http://hl7.org/fhir/contract-publicationstatus"; 188 case EXECUTABLE: return "http://hl7.org/fhir/contract-publicationstatus"; 189 case EXECUTED: return "http://hl7.org/fhir/contract-publicationstatus"; 190 case NEGOTIABLE: return "http://hl7.org/fhir/contract-publicationstatus"; 191 case OFFERED: return "http://hl7.org/fhir/contract-publicationstatus"; 192 case POLICY: return "http://hl7.org/fhir/contract-publicationstatus"; 193 case REJECTED: return "http://hl7.org/fhir/contract-publicationstatus"; 194 case RENEWED: return "http://hl7.org/fhir/contract-publicationstatus"; 195 case REVOKED: return "http://hl7.org/fhir/contract-publicationstatus"; 196 case RESOLVED: return "http://hl7.org/fhir/contract-publicationstatus"; 197 case TERMINATED: return "http://hl7.org/fhir/contract-publicationstatus"; 198 case NULL: return null; 199 default: return "?"; 200 } 201 } 202 public String getDefinition() { 203 switch (this) { 204 case AMENDED: return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 205 case APPENDED: return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 206 case CANCELLED: return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 207 case DISPUTED: return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 208 case ENTEREDINERROR: return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 209 case EXECUTABLE: return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 210 case EXECUTED: return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 211 case NEGOTIABLE: return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 212 case OFFERED: return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 213 case POLICY: return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 214 case REJECTED: return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 215 case RENEWED: return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 216 case REVOKED: return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 217 case RESOLVED: return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 218 case TERMINATED: return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 219 case NULL: return null; 220 default: return "?"; 221 } 222 } 223 public String getDisplay() { 224 switch (this) { 225 case AMENDED: return "Amended"; 226 case APPENDED: return "Appended"; 227 case CANCELLED: return "Cancelled"; 228 case DISPUTED: return "Disputed"; 229 case ENTEREDINERROR: return "Entered in Error"; 230 case EXECUTABLE: return "Executable"; 231 case EXECUTED: return "Executed"; 232 case NEGOTIABLE: return "Negotiable"; 233 case OFFERED: return "Offered"; 234 case POLICY: return "Policy"; 235 case REJECTED: return "Rejected"; 236 case RENEWED: return "Renewed"; 237 case REVOKED: return "Revoked"; 238 case RESOLVED: return "Resolved"; 239 case TERMINATED: return "Terminated"; 240 case NULL: return null; 241 default: return "?"; 242 } 243 } 244 } 245 246 public static class ContractResourcePublicationStatusCodesEnumFactory implements EnumFactory<ContractResourcePublicationStatusCodes> { 247 public ContractResourcePublicationStatusCodes fromCode(String codeString) throws IllegalArgumentException { 248 if (codeString == null || "".equals(codeString)) 249 if (codeString == null || "".equals(codeString)) 250 return null; 251 if ("amended".equals(codeString)) 252 return ContractResourcePublicationStatusCodes.AMENDED; 253 if ("appended".equals(codeString)) 254 return ContractResourcePublicationStatusCodes.APPENDED; 255 if ("cancelled".equals(codeString)) 256 return ContractResourcePublicationStatusCodes.CANCELLED; 257 if ("disputed".equals(codeString)) 258 return ContractResourcePublicationStatusCodes.DISPUTED; 259 if ("entered-in-error".equals(codeString)) 260 return ContractResourcePublicationStatusCodes.ENTEREDINERROR; 261 if ("executable".equals(codeString)) 262 return ContractResourcePublicationStatusCodes.EXECUTABLE; 263 if ("executed".equals(codeString)) 264 return ContractResourcePublicationStatusCodes.EXECUTED; 265 if ("negotiable".equals(codeString)) 266 return ContractResourcePublicationStatusCodes.NEGOTIABLE; 267 if ("offered".equals(codeString)) 268 return ContractResourcePublicationStatusCodes.OFFERED; 269 if ("policy".equals(codeString)) 270 return ContractResourcePublicationStatusCodes.POLICY; 271 if ("rejected".equals(codeString)) 272 return ContractResourcePublicationStatusCodes.REJECTED; 273 if ("renewed".equals(codeString)) 274 return ContractResourcePublicationStatusCodes.RENEWED; 275 if ("revoked".equals(codeString)) 276 return ContractResourcePublicationStatusCodes.REVOKED; 277 if ("resolved".equals(codeString)) 278 return ContractResourcePublicationStatusCodes.RESOLVED; 279 if ("terminated".equals(codeString)) 280 return ContractResourcePublicationStatusCodes.TERMINATED; 281 throw new IllegalArgumentException("Unknown ContractResourcePublicationStatusCodes code '"+codeString+"'"); 282 } 283 public Enumeration<ContractResourcePublicationStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 284 if (code == null) 285 return null; 286 if (code.isEmpty()) 287 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.NULL, code); 288 String codeString = ((PrimitiveType) code).asStringValue(); 289 if (codeString == null || "".equals(codeString)) 290 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.NULL, code); 291 if ("amended".equals(codeString)) 292 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.AMENDED, code); 293 if ("appended".equals(codeString)) 294 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.APPENDED, code); 295 if ("cancelled".equals(codeString)) 296 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.CANCELLED, code); 297 if ("disputed".equals(codeString)) 298 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.DISPUTED, code); 299 if ("entered-in-error".equals(codeString)) 300 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.ENTEREDINERROR, code); 301 if ("executable".equals(codeString)) 302 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.EXECUTABLE, code); 303 if ("executed".equals(codeString)) 304 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.EXECUTED, code); 305 if ("negotiable".equals(codeString)) 306 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.NEGOTIABLE, code); 307 if ("offered".equals(codeString)) 308 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.OFFERED, code); 309 if ("policy".equals(codeString)) 310 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.POLICY, code); 311 if ("rejected".equals(codeString)) 312 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.REJECTED, code); 313 if ("renewed".equals(codeString)) 314 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.RENEWED, code); 315 if ("revoked".equals(codeString)) 316 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.REVOKED, code); 317 if ("resolved".equals(codeString)) 318 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.RESOLVED, code); 319 if ("terminated".equals(codeString)) 320 return new Enumeration<ContractResourcePublicationStatusCodes>(this, ContractResourcePublicationStatusCodes.TERMINATED, code); 321 throw new FHIRException("Unknown ContractResourcePublicationStatusCodes code '"+codeString+"'"); 322 } 323 public String toCode(ContractResourcePublicationStatusCodes code) { 324 if (code == ContractResourcePublicationStatusCodes.NULL) 325 return null; 326 if (code == ContractResourcePublicationStatusCodes.AMENDED) 327 return "amended"; 328 if (code == ContractResourcePublicationStatusCodes.APPENDED) 329 return "appended"; 330 if (code == ContractResourcePublicationStatusCodes.CANCELLED) 331 return "cancelled"; 332 if (code == ContractResourcePublicationStatusCodes.DISPUTED) 333 return "disputed"; 334 if (code == ContractResourcePublicationStatusCodes.ENTEREDINERROR) 335 return "entered-in-error"; 336 if (code == ContractResourcePublicationStatusCodes.EXECUTABLE) 337 return "executable"; 338 if (code == ContractResourcePublicationStatusCodes.EXECUTED) 339 return "executed"; 340 if (code == ContractResourcePublicationStatusCodes.NEGOTIABLE) 341 return "negotiable"; 342 if (code == ContractResourcePublicationStatusCodes.OFFERED) 343 return "offered"; 344 if (code == ContractResourcePublicationStatusCodes.POLICY) 345 return "policy"; 346 if (code == ContractResourcePublicationStatusCodes.REJECTED) 347 return "rejected"; 348 if (code == ContractResourcePublicationStatusCodes.RENEWED) 349 return "renewed"; 350 if (code == ContractResourcePublicationStatusCodes.REVOKED) 351 return "revoked"; 352 if (code == ContractResourcePublicationStatusCodes.RESOLVED) 353 return "resolved"; 354 if (code == ContractResourcePublicationStatusCodes.TERMINATED) 355 return "terminated"; 356 return "?"; 357 } 358 public String toSystem(ContractResourcePublicationStatusCodes code) { 359 return code.getSystem(); 360 } 361 } 362 363 public enum ContractResourceStatusCodes { 364 /** 365 * Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced. 366 */ 367 AMENDED, 368 /** 369 * Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced. 370 */ 371 APPENDED, 372 /** 373 * Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted. 374 */ 375 CANCELLED, 376 /** 377 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended. 378 */ 379 DISPUTED, 380 /** 381 * Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status. 382 */ 383 ENTEREDINERROR, 384 /** 385 * Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active. 386 */ 387 EXECUTABLE, 388 /** 389 * Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed. 390 */ 391 EXECUTED, 392 /** 393 * Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 394 */ 395 NEGOTIABLE, 396 /** 397 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new. 398 */ 399 OFFERED, 400 /** 401 * Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended. 402 */ 403 POLICY, 404 /** 405 * Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled. 406 */ 407 REJECTED, 408 /** 409 * Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded. 410 */ 411 RENEWED, 412 /** 413 * A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified. 414 */ 415 REVOKED, 416 /** 417 * Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated. 418 */ 419 RESOLVED, 420 /** 421 * Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted. 422 */ 423 TERMINATED, 424 /** 425 * added to help the parsers with the generic types 426 */ 427 NULL; 428 public static ContractResourceStatusCodes fromCode(String codeString) throws FHIRException { 429 if (codeString == null || "".equals(codeString)) 430 return null; 431 if ("amended".equals(codeString)) 432 return AMENDED; 433 if ("appended".equals(codeString)) 434 return APPENDED; 435 if ("cancelled".equals(codeString)) 436 return CANCELLED; 437 if ("disputed".equals(codeString)) 438 return DISPUTED; 439 if ("entered-in-error".equals(codeString)) 440 return ENTEREDINERROR; 441 if ("executable".equals(codeString)) 442 return EXECUTABLE; 443 if ("executed".equals(codeString)) 444 return EXECUTED; 445 if ("negotiable".equals(codeString)) 446 return NEGOTIABLE; 447 if ("offered".equals(codeString)) 448 return OFFERED; 449 if ("policy".equals(codeString)) 450 return POLICY; 451 if ("rejected".equals(codeString)) 452 return REJECTED; 453 if ("renewed".equals(codeString)) 454 return RENEWED; 455 if ("revoked".equals(codeString)) 456 return REVOKED; 457 if ("resolved".equals(codeString)) 458 return RESOLVED; 459 if ("terminated".equals(codeString)) 460 return TERMINATED; 461 if (Configuration.isAcceptInvalidEnums()) 462 return null; 463 else 464 throw new FHIRException("Unknown ContractResourceStatusCodes code '"+codeString+"'"); 465 } 466 public String toCode() { 467 switch (this) { 468 case AMENDED: return "amended"; 469 case APPENDED: return "appended"; 470 case CANCELLED: return "cancelled"; 471 case DISPUTED: return "disputed"; 472 case ENTEREDINERROR: return "entered-in-error"; 473 case EXECUTABLE: return "executable"; 474 case EXECUTED: return "executed"; 475 case NEGOTIABLE: return "negotiable"; 476 case OFFERED: return "offered"; 477 case POLICY: return "policy"; 478 case REJECTED: return "rejected"; 479 case RENEWED: return "renewed"; 480 case REVOKED: return "revoked"; 481 case RESOLVED: return "resolved"; 482 case TERMINATED: return "terminated"; 483 case NULL: return null; 484 default: return "?"; 485 } 486 } 487 public String getSystem() { 488 switch (this) { 489 case AMENDED: return "http://hl7.org/fhir/contract-status"; 490 case APPENDED: return "http://hl7.org/fhir/contract-status"; 491 case CANCELLED: return "http://hl7.org/fhir/contract-status"; 492 case DISPUTED: return "http://hl7.org/fhir/contract-status"; 493 case ENTEREDINERROR: return "http://hl7.org/fhir/contract-status"; 494 case EXECUTABLE: return "http://hl7.org/fhir/contract-status"; 495 case EXECUTED: return "http://hl7.org/fhir/contract-status"; 496 case NEGOTIABLE: return "http://hl7.org/fhir/contract-status"; 497 case OFFERED: return "http://hl7.org/fhir/contract-status"; 498 case POLICY: return "http://hl7.org/fhir/contract-status"; 499 case REJECTED: return "http://hl7.org/fhir/contract-status"; 500 case RENEWED: return "http://hl7.org/fhir/contract-status"; 501 case REVOKED: return "http://hl7.org/fhir/contract-status"; 502 case RESOLVED: return "http://hl7.org/fhir/contract-status"; 503 case TERMINATED: return "http://hl7.org/fhir/contract-status"; 504 case NULL: return null; 505 default: return "?"; 506 } 507 } 508 public String getDefinition() { 509 switch (this) { 510 case AMENDED: return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 511 case APPENDED: return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 512 case CANCELLED: return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 513 case DISPUTED: return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 514 case ENTEREDINERROR: return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 515 case EXECUTABLE: return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 516 case EXECUTED: return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 517 case NEGOTIABLE: return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 518 case OFFERED: return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 519 case POLICY: return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 520 case REJECTED: return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 521 case RENEWED: return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 522 case REVOKED: return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 523 case RESOLVED: return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 524 case TERMINATED: return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 525 case NULL: return null; 526 default: return "?"; 527 } 528 } 529 public String getDisplay() { 530 switch (this) { 531 case AMENDED: return "Amended"; 532 case APPENDED: return "Appended"; 533 case CANCELLED: return "Cancelled"; 534 case DISPUTED: return "Disputed"; 535 case ENTEREDINERROR: return "Entered in Error"; 536 case EXECUTABLE: return "Executable"; 537 case EXECUTED: return "Executed"; 538 case NEGOTIABLE: return "Negotiable"; 539 case OFFERED: return "Offered"; 540 case POLICY: return "Policy"; 541 case REJECTED: return "Rejected"; 542 case RENEWED: return "Renewed"; 543 case REVOKED: return "Revoked"; 544 case RESOLVED: return "Resolved"; 545 case TERMINATED: return "Terminated"; 546 case NULL: return null; 547 default: return "?"; 548 } 549 } 550 } 551 552 public static class ContractResourceStatusCodesEnumFactory implements EnumFactory<ContractResourceStatusCodes> { 553 public ContractResourceStatusCodes fromCode(String codeString) throws IllegalArgumentException { 554 if (codeString == null || "".equals(codeString)) 555 if (codeString == null || "".equals(codeString)) 556 return null; 557 if ("amended".equals(codeString)) 558 return ContractResourceStatusCodes.AMENDED; 559 if ("appended".equals(codeString)) 560 return ContractResourceStatusCodes.APPENDED; 561 if ("cancelled".equals(codeString)) 562 return ContractResourceStatusCodes.CANCELLED; 563 if ("disputed".equals(codeString)) 564 return ContractResourceStatusCodes.DISPUTED; 565 if ("entered-in-error".equals(codeString)) 566 return ContractResourceStatusCodes.ENTEREDINERROR; 567 if ("executable".equals(codeString)) 568 return ContractResourceStatusCodes.EXECUTABLE; 569 if ("executed".equals(codeString)) 570 return ContractResourceStatusCodes.EXECUTED; 571 if ("negotiable".equals(codeString)) 572 return ContractResourceStatusCodes.NEGOTIABLE; 573 if ("offered".equals(codeString)) 574 return ContractResourceStatusCodes.OFFERED; 575 if ("policy".equals(codeString)) 576 return ContractResourceStatusCodes.POLICY; 577 if ("rejected".equals(codeString)) 578 return ContractResourceStatusCodes.REJECTED; 579 if ("renewed".equals(codeString)) 580 return ContractResourceStatusCodes.RENEWED; 581 if ("revoked".equals(codeString)) 582 return ContractResourceStatusCodes.REVOKED; 583 if ("resolved".equals(codeString)) 584 return ContractResourceStatusCodes.RESOLVED; 585 if ("terminated".equals(codeString)) 586 return ContractResourceStatusCodes.TERMINATED; 587 throw new IllegalArgumentException("Unknown ContractResourceStatusCodes code '"+codeString+"'"); 588 } 589 public Enumeration<ContractResourceStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 590 if (code == null) 591 return null; 592 if (code.isEmpty()) 593 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.NULL, code); 594 String codeString = ((PrimitiveType) code).asStringValue(); 595 if (codeString == null || "".equals(codeString)) 596 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.NULL, code); 597 if ("amended".equals(codeString)) 598 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.AMENDED, code); 599 if ("appended".equals(codeString)) 600 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.APPENDED, code); 601 if ("cancelled".equals(codeString)) 602 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.CANCELLED, code); 603 if ("disputed".equals(codeString)) 604 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.DISPUTED, code); 605 if ("entered-in-error".equals(codeString)) 606 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.ENTEREDINERROR, code); 607 if ("executable".equals(codeString)) 608 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.EXECUTABLE, code); 609 if ("executed".equals(codeString)) 610 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.EXECUTED, code); 611 if ("negotiable".equals(codeString)) 612 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.NEGOTIABLE, code); 613 if ("offered".equals(codeString)) 614 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.OFFERED, code); 615 if ("policy".equals(codeString)) 616 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.POLICY, code); 617 if ("rejected".equals(codeString)) 618 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.REJECTED, code); 619 if ("renewed".equals(codeString)) 620 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.RENEWED, code); 621 if ("revoked".equals(codeString)) 622 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.REVOKED, code); 623 if ("resolved".equals(codeString)) 624 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.RESOLVED, code); 625 if ("terminated".equals(codeString)) 626 return new Enumeration<ContractResourceStatusCodes>(this, ContractResourceStatusCodes.TERMINATED, code); 627 throw new FHIRException("Unknown ContractResourceStatusCodes code '"+codeString+"'"); 628 } 629 public String toCode(ContractResourceStatusCodes code) { 630 if (code == ContractResourceStatusCodes.NULL) 631 return null; 632 if (code == ContractResourceStatusCodes.AMENDED) 633 return "amended"; 634 if (code == ContractResourceStatusCodes.APPENDED) 635 return "appended"; 636 if (code == ContractResourceStatusCodes.CANCELLED) 637 return "cancelled"; 638 if (code == ContractResourceStatusCodes.DISPUTED) 639 return "disputed"; 640 if (code == ContractResourceStatusCodes.ENTEREDINERROR) 641 return "entered-in-error"; 642 if (code == ContractResourceStatusCodes.EXECUTABLE) 643 return "executable"; 644 if (code == ContractResourceStatusCodes.EXECUTED) 645 return "executed"; 646 if (code == ContractResourceStatusCodes.NEGOTIABLE) 647 return "negotiable"; 648 if (code == ContractResourceStatusCodes.OFFERED) 649 return "offered"; 650 if (code == ContractResourceStatusCodes.POLICY) 651 return "policy"; 652 if (code == ContractResourceStatusCodes.REJECTED) 653 return "rejected"; 654 if (code == ContractResourceStatusCodes.RENEWED) 655 return "renewed"; 656 if (code == ContractResourceStatusCodes.REVOKED) 657 return "revoked"; 658 if (code == ContractResourceStatusCodes.RESOLVED) 659 return "resolved"; 660 if (code == ContractResourceStatusCodes.TERMINATED) 661 return "terminated"; 662 return "?"; 663 } 664 public String toSystem(ContractResourceStatusCodes code) { 665 return code.getSystem(); 666 } 667 } 668 669 @Block() 670 public static class ContentDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 671 /** 672 * Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation. 673 */ 674 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 675 @Description(shortDefinition="Content structure and use", formalDefinition="Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation." ) 676 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-definition-type") 677 protected CodeableConcept type; 678 679 /** 680 * Detailed Precusory content type. 681 */ 682 @Child(name = "subType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 683 @Description(shortDefinition="Detailed Content Type Definition", formalDefinition="Detailed Precusory content type." ) 684 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-definition-subtype") 685 protected CodeableConcept subType; 686 687 /** 688 * The individual or organization that published the Contract precursor content. 689 */ 690 @Child(name = "publisher", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 691 @Description(shortDefinition="Publisher Entity", formalDefinition="The individual or organization that published the Contract precursor content." ) 692 protected Reference publisher; 693 694 /** 695 * The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes. 696 */ 697 @Child(name = "publicationDate", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 698 @Description(shortDefinition="When published", formalDefinition="The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes." ) 699 protected DateTimeType publicationDate; 700 701 /** 702 * amended | appended | cancelled | disputed | entered-in-error | executable +. 703 */ 704 @Child(name = "publicationStatus", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=false) 705 @Description(shortDefinition="amended | appended | cancelled | disputed | entered-in-error | executable +", formalDefinition="amended | appended | cancelled | disputed | entered-in-error | executable +." ) 706 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-publicationstatus") 707 protected Enumeration<ContractResourcePublicationStatusCodes> publicationStatus; 708 709 /** 710 * A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content. 711 */ 712 @Child(name = "copyright", type = {MarkdownType.class}, order=6, min=0, max=1, modifier=false, summary=false) 713 @Description(shortDefinition="Publication Ownership", formalDefinition="A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content." ) 714 protected MarkdownType copyright; 715 716 private static final long serialVersionUID = 306178803L; 717 718 /** 719 * Constructor 720 */ 721 public ContentDefinitionComponent() { 722 super(); 723 } 724 725 /** 726 * Constructor 727 */ 728 public ContentDefinitionComponent(CodeableConcept type, ContractResourcePublicationStatusCodes publicationStatus) { 729 super(); 730 this.setType(type); 731 this.setPublicationStatus(publicationStatus); 732 } 733 734 /** 735 * @return {@link #type} (Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.) 736 */ 737 public CodeableConcept getType() { 738 if (this.type == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create ContentDefinitionComponent.type"); 741 else if (Configuration.doAutoCreate()) 742 this.type = new CodeableConcept(); // cc 743 return this.type; 744 } 745 746 public boolean hasType() { 747 return this.type != null && !this.type.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #type} (Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.) 752 */ 753 public ContentDefinitionComponent setType(CodeableConcept value) { 754 this.type = value; 755 return this; 756 } 757 758 /** 759 * @return {@link #subType} (Detailed Precusory content type.) 760 */ 761 public CodeableConcept getSubType() { 762 if (this.subType == null) 763 if (Configuration.errorOnAutoCreate()) 764 throw new Error("Attempt to auto-create ContentDefinitionComponent.subType"); 765 else if (Configuration.doAutoCreate()) 766 this.subType = new CodeableConcept(); // cc 767 return this.subType; 768 } 769 770 public boolean hasSubType() { 771 return this.subType != null && !this.subType.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #subType} (Detailed Precusory content type.) 776 */ 777 public ContentDefinitionComponent setSubType(CodeableConcept value) { 778 this.subType = value; 779 return this; 780 } 781 782 /** 783 * @return {@link #publisher} (The individual or organization that published the Contract precursor content.) 784 */ 785 public Reference getPublisher() { 786 if (this.publisher == null) 787 if (Configuration.errorOnAutoCreate()) 788 throw new Error("Attempt to auto-create ContentDefinitionComponent.publisher"); 789 else if (Configuration.doAutoCreate()) 790 this.publisher = new Reference(); // cc 791 return this.publisher; 792 } 793 794 public boolean hasPublisher() { 795 return this.publisher != null && !this.publisher.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #publisher} (The individual or organization that published the Contract precursor content.) 800 */ 801 public ContentDefinitionComponent setPublisher(Reference value) { 802 this.publisher = value; 803 return this; 804 } 805 806 /** 807 * @return {@link #publicationDate} (The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.). This is the underlying object with id, value and extensions. The accessor "getPublicationDate" gives direct access to the value 808 */ 809 public DateTimeType getPublicationDateElement() { 810 if (this.publicationDate == null) 811 if (Configuration.errorOnAutoCreate()) 812 throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationDate"); 813 else if (Configuration.doAutoCreate()) 814 this.publicationDate = new DateTimeType(); // bb 815 return this.publicationDate; 816 } 817 818 public boolean hasPublicationDateElement() { 819 return this.publicationDate != null && !this.publicationDate.isEmpty(); 820 } 821 822 public boolean hasPublicationDate() { 823 return this.publicationDate != null && !this.publicationDate.isEmpty(); 824 } 825 826 /** 827 * @param value {@link #publicationDate} (The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.). This is the underlying object with id, value and extensions. The accessor "getPublicationDate" gives direct access to the value 828 */ 829 public ContentDefinitionComponent setPublicationDateElement(DateTimeType value) { 830 this.publicationDate = value; 831 return this; 832 } 833 834 /** 835 * @return The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes. 836 */ 837 public Date getPublicationDate() { 838 return this.publicationDate == null ? null : this.publicationDate.getValue(); 839 } 840 841 /** 842 * @param value The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes. 843 */ 844 public ContentDefinitionComponent setPublicationDate(Date value) { 845 if (value == null) 846 this.publicationDate = null; 847 else { 848 if (this.publicationDate == null) 849 this.publicationDate = new DateTimeType(); 850 this.publicationDate.setValue(value); 851 } 852 return this; 853 } 854 855 /** 856 * @return {@link #publicationStatus} (amended | appended | cancelled | disputed | entered-in-error | executable +.). This is the underlying object with id, value and extensions. The accessor "getPublicationStatus" gives direct access to the value 857 */ 858 public Enumeration<ContractResourcePublicationStatusCodes> getPublicationStatusElement() { 859 if (this.publicationStatus == null) 860 if (Configuration.errorOnAutoCreate()) 861 throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationStatus"); 862 else if (Configuration.doAutoCreate()) 863 this.publicationStatus = new Enumeration<ContractResourcePublicationStatusCodes>(new ContractResourcePublicationStatusCodesEnumFactory()); // bb 864 return this.publicationStatus; 865 } 866 867 public boolean hasPublicationStatusElement() { 868 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 869 } 870 871 public boolean hasPublicationStatus() { 872 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 873 } 874 875 /** 876 * @param value {@link #publicationStatus} (amended | appended | cancelled | disputed | entered-in-error | executable +.). This is the underlying object with id, value and extensions. The accessor "getPublicationStatus" gives direct access to the value 877 */ 878 public ContentDefinitionComponent setPublicationStatusElement(Enumeration<ContractResourcePublicationStatusCodes> value) { 879 this.publicationStatus = value; 880 return this; 881 } 882 883 /** 884 * @return amended | appended | cancelled | disputed | entered-in-error | executable +. 885 */ 886 public ContractResourcePublicationStatusCodes getPublicationStatus() { 887 return this.publicationStatus == null ? null : this.publicationStatus.getValue(); 888 } 889 890 /** 891 * @param value amended | appended | cancelled | disputed | entered-in-error | executable +. 892 */ 893 public ContentDefinitionComponent setPublicationStatus(ContractResourcePublicationStatusCodes value) { 894 if (this.publicationStatus == null) 895 this.publicationStatus = new Enumeration<ContractResourcePublicationStatusCodes>(new ContractResourcePublicationStatusCodesEnumFactory()); 896 this.publicationStatus.setValue(value); 897 return this; 898 } 899 900 /** 901 * @return {@link #copyright} (A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 902 */ 903 public MarkdownType getCopyrightElement() { 904 if (this.copyright == null) 905 if (Configuration.errorOnAutoCreate()) 906 throw new Error("Attempt to auto-create ContentDefinitionComponent.copyright"); 907 else if (Configuration.doAutoCreate()) 908 this.copyright = new MarkdownType(); // bb 909 return this.copyright; 910 } 911 912 public boolean hasCopyrightElement() { 913 return this.copyright != null && !this.copyright.isEmpty(); 914 } 915 916 public boolean hasCopyright() { 917 return this.copyright != null && !this.copyright.isEmpty(); 918 } 919 920 /** 921 * @param value {@link #copyright} (A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 922 */ 923 public ContentDefinitionComponent setCopyrightElement(MarkdownType value) { 924 this.copyright = value; 925 return this; 926 } 927 928 /** 929 * @return A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content. 930 */ 931 public String getCopyright() { 932 return this.copyright == null ? null : this.copyright.getValue(); 933 } 934 935 /** 936 * @param value A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content. 937 */ 938 public ContentDefinitionComponent setCopyright(String value) { 939 if (Utilities.noString(value)) 940 this.copyright = null; 941 else { 942 if (this.copyright == null) 943 this.copyright = new MarkdownType(); 944 this.copyright.setValue(value); 945 } 946 return this; 947 } 948 949 protected void listChildren(List<Property> children) { 950 super.listChildren(children); 951 children.add(new Property("type", "CodeableConcept", "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.", 0, 1, type)); 952 children.add(new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1, subType)); 953 children.add(new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization that published the Contract precursor content.", 0, 1, publisher)); 954 children.add(new Property("publicationDate", "dateTime", "The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.", 0, 1, publicationDate)); 955 children.add(new Property("publicationStatus", "code", "amended | appended | cancelled | disputed | entered-in-error | executable +.", 0, 1, publicationStatus)); 956 children.add(new Property("copyright", "markdown", "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.", 0, 1, copyright)); 957 } 958 959 @Override 960 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 961 switch (_hash) { 962 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.", 0, 1, type); 963 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1, subType); 964 case 1447404028: /*publisher*/ return new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization that published the Contract precursor content.", 0, 1, publisher); 965 case 1470566394: /*publicationDate*/ return new Property("publicationDate", "dateTime", "The date (and optionally time) when the contract was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.", 0, 1, publicationDate); 966 case 616500542: /*publicationStatus*/ return new Property("publicationStatus", "code", "amended | appended | cancelled | disputed | entered-in-error | executable +.", 0, 1, publicationStatus); 967 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.", 0, 1, copyright); 968 default: return super.getNamedProperty(_hash, _name, _checkValid); 969 } 970 971 } 972 973 @Override 974 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 975 switch (hash) { 976 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 977 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 978 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // Reference 979 case 1470566394: /*publicationDate*/ return this.publicationDate == null ? new Base[0] : new Base[] {this.publicationDate}; // DateTimeType 980 case 616500542: /*publicationStatus*/ return this.publicationStatus == null ? new Base[0] : new Base[] {this.publicationStatus}; // Enumeration<ContractResourcePublicationStatusCodes> 981 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 982 default: return super.getProperty(hash, name, checkValid); 983 } 984 985 } 986 987 @Override 988 public Base setProperty(int hash, String name, Base value) throws FHIRException { 989 switch (hash) { 990 case 3575610: // type 991 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 992 return value; 993 case -1868521062: // subType 994 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 995 return value; 996 case 1447404028: // publisher 997 this.publisher = TypeConvertor.castToReference(value); // Reference 998 return value; 999 case 1470566394: // publicationDate 1000 this.publicationDate = TypeConvertor.castToDateTime(value); // DateTimeType 1001 return value; 1002 case 616500542: // publicationStatus 1003 value = new ContractResourcePublicationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1004 this.publicationStatus = (Enumeration) value; // Enumeration<ContractResourcePublicationStatusCodes> 1005 return value; 1006 case 1522889671: // copyright 1007 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 1008 return value; 1009 default: return super.setProperty(hash, name, value); 1010 } 1011 1012 } 1013 1014 @Override 1015 public Base setProperty(String name, Base value) throws FHIRException { 1016 if (name.equals("type")) { 1017 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1018 } else if (name.equals("subType")) { 1019 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1020 } else if (name.equals("publisher")) { 1021 this.publisher = TypeConvertor.castToReference(value); // Reference 1022 } else if (name.equals("publicationDate")) { 1023 this.publicationDate = TypeConvertor.castToDateTime(value); // DateTimeType 1024 } else if (name.equals("publicationStatus")) { 1025 value = new ContractResourcePublicationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1026 this.publicationStatus = (Enumeration) value; // Enumeration<ContractResourcePublicationStatusCodes> 1027 } else if (name.equals("copyright")) { 1028 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 1029 } else 1030 return super.setProperty(name, value); 1031 return value; 1032 } 1033 1034 @Override 1035 public void removeChild(String name, Base value) throws FHIRException { 1036 if (name.equals("type")) { 1037 this.type = null; 1038 } else if (name.equals("subType")) { 1039 this.subType = null; 1040 } else if (name.equals("publisher")) { 1041 this.publisher = null; 1042 } else if (name.equals("publicationDate")) { 1043 this.publicationDate = null; 1044 } else if (name.equals("publicationStatus")) { 1045 value = new ContractResourcePublicationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1046 this.publicationStatus = (Enumeration) value; // Enumeration<ContractResourcePublicationStatusCodes> 1047 } else if (name.equals("copyright")) { 1048 this.copyright = null; 1049 } else 1050 super.removeChild(name, value); 1051 1052 } 1053 1054 @Override 1055 public Base makeProperty(int hash, String name) throws FHIRException { 1056 switch (hash) { 1057 case 3575610: return getType(); 1058 case -1868521062: return getSubType(); 1059 case 1447404028: return getPublisher(); 1060 case 1470566394: return getPublicationDateElement(); 1061 case 616500542: return getPublicationStatusElement(); 1062 case 1522889671: return getCopyrightElement(); 1063 default: return super.makeProperty(hash, name); 1064 } 1065 1066 } 1067 1068 @Override 1069 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1070 switch (hash) { 1071 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1072 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 1073 case 1447404028: /*publisher*/ return new String[] {"Reference"}; 1074 case 1470566394: /*publicationDate*/ return new String[] {"dateTime"}; 1075 case 616500542: /*publicationStatus*/ return new String[] {"code"}; 1076 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 1077 default: return super.getTypesForProperty(hash, name); 1078 } 1079 1080 } 1081 1082 @Override 1083 public Base addChild(String name) throws FHIRException { 1084 if (name.equals("type")) { 1085 this.type = new CodeableConcept(); 1086 return this.type; 1087 } 1088 else if (name.equals("subType")) { 1089 this.subType = new CodeableConcept(); 1090 return this.subType; 1091 } 1092 else if (name.equals("publisher")) { 1093 this.publisher = new Reference(); 1094 return this.publisher; 1095 } 1096 else if (name.equals("publicationDate")) { 1097 throw new FHIRException("Cannot call addChild on a singleton property Contract.contentDefinition.publicationDate"); 1098 } 1099 else if (name.equals("publicationStatus")) { 1100 throw new FHIRException("Cannot call addChild on a singleton property Contract.contentDefinition.publicationStatus"); 1101 } 1102 else if (name.equals("copyright")) { 1103 throw new FHIRException("Cannot call addChild on a singleton property Contract.contentDefinition.copyright"); 1104 } 1105 else 1106 return super.addChild(name); 1107 } 1108 1109 public ContentDefinitionComponent copy() { 1110 ContentDefinitionComponent dst = new ContentDefinitionComponent(); 1111 copyValues(dst); 1112 return dst; 1113 } 1114 1115 public void copyValues(ContentDefinitionComponent dst) { 1116 super.copyValues(dst); 1117 dst.type = type == null ? null : type.copy(); 1118 dst.subType = subType == null ? null : subType.copy(); 1119 dst.publisher = publisher == null ? null : publisher.copy(); 1120 dst.publicationDate = publicationDate == null ? null : publicationDate.copy(); 1121 dst.publicationStatus = publicationStatus == null ? null : publicationStatus.copy(); 1122 dst.copyright = copyright == null ? null : copyright.copy(); 1123 } 1124 1125 @Override 1126 public boolean equalsDeep(Base other_) { 1127 if (!super.equalsDeep(other_)) 1128 return false; 1129 if (!(other_ instanceof ContentDefinitionComponent)) 1130 return false; 1131 ContentDefinitionComponent o = (ContentDefinitionComponent) other_; 1132 return compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(publisher, o.publisher, true) 1133 && compareDeep(publicationDate, o.publicationDate, true) && compareDeep(publicationStatus, o.publicationStatus, true) 1134 && compareDeep(copyright, o.copyright, true); 1135 } 1136 1137 @Override 1138 public boolean equalsShallow(Base other_) { 1139 if (!super.equalsShallow(other_)) 1140 return false; 1141 if (!(other_ instanceof ContentDefinitionComponent)) 1142 return false; 1143 ContentDefinitionComponent o = (ContentDefinitionComponent) other_; 1144 return compareValues(publicationDate, o.publicationDate, true) && compareValues(publicationStatus, o.publicationStatus, true) 1145 && compareValues(copyright, o.copyright, true); 1146 } 1147 1148 public boolean isEmpty() { 1149 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subType, publisher 1150 , publicationDate, publicationStatus, copyright); 1151 } 1152 1153 public String fhirType() { 1154 return "Contract.contentDefinition"; 1155 1156 } 1157 1158 } 1159 1160 @Block() 1161 public static class TermComponent extends BackboneElement implements IBaseBackboneElement { 1162 /** 1163 * Unique identifier for this particular Contract Provision. 1164 */ 1165 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1166 @Description(shortDefinition="Contract Term Number", formalDefinition="Unique identifier for this particular Contract Provision." ) 1167 protected Identifier identifier; 1168 1169 /** 1170 * When this Contract Provision was issued. 1171 */ 1172 @Child(name = "issued", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1173 @Description(shortDefinition="Contract Term Issue Date Time", formalDefinition="When this Contract Provision was issued." ) 1174 protected DateTimeType issued; 1175 1176 /** 1177 * Relevant time or time-period when this Contract Provision is applicable. 1178 */ 1179 @Child(name = "applies", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 1180 @Description(shortDefinition="Contract Term Effective Time", formalDefinition="Relevant time or time-period when this Contract Provision is applicable." ) 1181 protected Period applies; 1182 1183 /** 1184 * The entity that the term applies to. 1185 */ 1186 @Child(name = "topic", type = {CodeableConcept.class, Reference.class}, order=4, min=0, max=1, modifier=false, summary=false) 1187 @Description(shortDefinition="Term Concern", formalDefinition="The entity that the term applies to." ) 1188 protected DataType topic; 1189 1190 /** 1191 * A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time. 1192 */ 1193 @Child(name = "type", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1194 @Description(shortDefinition="Contract Term Type or Form", formalDefinition="A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time." ) 1195 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-term-type") 1196 protected CodeableConcept type; 1197 1198 /** 1199 * A specialized legal clause or condition based on overarching contract type. 1200 */ 1201 @Child(name = "subType", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1202 @Description(shortDefinition="Contract Term Type specific classification", formalDefinition="A specialized legal clause or condition based on overarching contract type." ) 1203 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-term-subtype") 1204 protected CodeableConcept subType; 1205 1206 /** 1207 * Statement of a provision in a policy or a contract. 1208 */ 1209 @Child(name = "text", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1210 @Description(shortDefinition="Term Statement", formalDefinition="Statement of a provision in a policy or a contract." ) 1211 protected StringType text; 1212 1213 /** 1214 * Security labels that protect the handling of information about the term and its elements, which may be specifically identified. 1215 */ 1216 @Child(name = "securityLabel", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1217 @Description(shortDefinition="Protection for the Term", formalDefinition="Security labels that protect the handling of information about the term and its elements, which may be specifically identified." ) 1218 protected List<SecurityLabelComponent> securityLabel; 1219 1220 /** 1221 * The matter of concern in the context of this provision of the agrement. 1222 */ 1223 @Child(name = "offer", type = {}, order=9, min=1, max=1, modifier=false, summary=false) 1224 @Description(shortDefinition="Context of the Contract term", formalDefinition="The matter of concern in the context of this provision of the agrement." ) 1225 protected ContractOfferComponent offer; 1226 1227 /** 1228 * Contract Term Asset List. 1229 */ 1230 @Child(name = "asset", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1231 @Description(shortDefinition="Contract Term Asset List", formalDefinition="Contract Term Asset List." ) 1232 protected List<ContractAssetComponent> asset; 1233 1234 /** 1235 * An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place. 1236 */ 1237 @Child(name = "action", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1238 @Description(shortDefinition="Entity being ascribed responsibility", formalDefinition="An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place." ) 1239 protected List<ActionComponent> action; 1240 1241 /** 1242 * Nested group of Contract Provisions. 1243 */ 1244 @Child(name = "group", type = {TermComponent.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1245 @Description(shortDefinition="Nested Contract Term Group", formalDefinition="Nested group of Contract Provisions." ) 1246 protected List<TermComponent> group; 1247 1248 private static final long serialVersionUID = -1647037544L; 1249 1250 /** 1251 * Constructor 1252 */ 1253 public TermComponent() { 1254 super(); 1255 } 1256 1257 /** 1258 * Constructor 1259 */ 1260 public TermComponent(ContractOfferComponent offer) { 1261 super(); 1262 this.setOffer(offer); 1263 } 1264 1265 /** 1266 * @return {@link #identifier} (Unique identifier for this particular Contract Provision.) 1267 */ 1268 public Identifier getIdentifier() { 1269 if (this.identifier == null) 1270 if (Configuration.errorOnAutoCreate()) 1271 throw new Error("Attempt to auto-create TermComponent.identifier"); 1272 else if (Configuration.doAutoCreate()) 1273 this.identifier = new Identifier(); // cc 1274 return this.identifier; 1275 } 1276 1277 public boolean hasIdentifier() { 1278 return this.identifier != null && !this.identifier.isEmpty(); 1279 } 1280 1281 /** 1282 * @param value {@link #identifier} (Unique identifier for this particular Contract Provision.) 1283 */ 1284 public TermComponent setIdentifier(Identifier value) { 1285 this.identifier = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return {@link #issued} (When this Contract Provision was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1291 */ 1292 public DateTimeType getIssuedElement() { 1293 if (this.issued == null) 1294 if (Configuration.errorOnAutoCreate()) 1295 throw new Error("Attempt to auto-create TermComponent.issued"); 1296 else if (Configuration.doAutoCreate()) 1297 this.issued = new DateTimeType(); // bb 1298 return this.issued; 1299 } 1300 1301 public boolean hasIssuedElement() { 1302 return this.issued != null && !this.issued.isEmpty(); 1303 } 1304 1305 public boolean hasIssued() { 1306 return this.issued != null && !this.issued.isEmpty(); 1307 } 1308 1309 /** 1310 * @param value {@link #issued} (When this Contract Provision was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1311 */ 1312 public TermComponent setIssuedElement(DateTimeType value) { 1313 this.issued = value; 1314 return this; 1315 } 1316 1317 /** 1318 * @return When this Contract Provision was issued. 1319 */ 1320 public Date getIssued() { 1321 return this.issued == null ? null : this.issued.getValue(); 1322 } 1323 1324 /** 1325 * @param value When this Contract Provision was issued. 1326 */ 1327 public TermComponent setIssued(Date value) { 1328 if (value == null) 1329 this.issued = null; 1330 else { 1331 if (this.issued == null) 1332 this.issued = new DateTimeType(); 1333 this.issued.setValue(value); 1334 } 1335 return this; 1336 } 1337 1338 /** 1339 * @return {@link #applies} (Relevant time or time-period when this Contract Provision is applicable.) 1340 */ 1341 public Period getApplies() { 1342 if (this.applies == null) 1343 if (Configuration.errorOnAutoCreate()) 1344 throw new Error("Attempt to auto-create TermComponent.applies"); 1345 else if (Configuration.doAutoCreate()) 1346 this.applies = new Period(); // cc 1347 return this.applies; 1348 } 1349 1350 public boolean hasApplies() { 1351 return this.applies != null && !this.applies.isEmpty(); 1352 } 1353 1354 /** 1355 * @param value {@link #applies} (Relevant time or time-period when this Contract Provision is applicable.) 1356 */ 1357 public TermComponent setApplies(Period value) { 1358 this.applies = value; 1359 return this; 1360 } 1361 1362 /** 1363 * @return {@link #topic} (The entity that the term applies to.) 1364 */ 1365 public DataType getTopic() { 1366 return this.topic; 1367 } 1368 1369 /** 1370 * @return {@link #topic} (The entity that the term applies to.) 1371 */ 1372 public CodeableConcept getTopicCodeableConcept() throws FHIRException { 1373 if (this.topic == null) 1374 this.topic = new CodeableConcept(); 1375 if (!(this.topic instanceof CodeableConcept)) 1376 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.topic.getClass().getName()+" was encountered"); 1377 return (CodeableConcept) this.topic; 1378 } 1379 1380 public boolean hasTopicCodeableConcept() { 1381 return this != null && this.topic instanceof CodeableConcept; 1382 } 1383 1384 /** 1385 * @return {@link #topic} (The entity that the term applies to.) 1386 */ 1387 public Reference getTopicReference() throws FHIRException { 1388 if (this.topic == null) 1389 this.topic = new Reference(); 1390 if (!(this.topic instanceof Reference)) 1391 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.topic.getClass().getName()+" was encountered"); 1392 return (Reference) this.topic; 1393 } 1394 1395 public boolean hasTopicReference() { 1396 return this != null && this.topic instanceof Reference; 1397 } 1398 1399 public boolean hasTopic() { 1400 return this.topic != null && !this.topic.isEmpty(); 1401 } 1402 1403 /** 1404 * @param value {@link #topic} (The entity that the term applies to.) 1405 */ 1406 public TermComponent setTopic(DataType value) { 1407 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1408 throw new FHIRException("Not the right type for Contract.term.topic[x]: "+value.fhirType()); 1409 this.topic = value; 1410 return this; 1411 } 1412 1413 /** 1414 * @return {@link #type} (A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.) 1415 */ 1416 public CodeableConcept getType() { 1417 if (this.type == null) 1418 if (Configuration.errorOnAutoCreate()) 1419 throw new Error("Attempt to auto-create TermComponent.type"); 1420 else if (Configuration.doAutoCreate()) 1421 this.type = new CodeableConcept(); // cc 1422 return this.type; 1423 } 1424 1425 public boolean hasType() { 1426 return this.type != null && !this.type.isEmpty(); 1427 } 1428 1429 /** 1430 * @param value {@link #type} (A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.) 1431 */ 1432 public TermComponent setType(CodeableConcept value) { 1433 this.type = value; 1434 return this; 1435 } 1436 1437 /** 1438 * @return {@link #subType} (A specialized legal clause or condition based on overarching contract type.) 1439 */ 1440 public CodeableConcept getSubType() { 1441 if (this.subType == null) 1442 if (Configuration.errorOnAutoCreate()) 1443 throw new Error("Attempt to auto-create TermComponent.subType"); 1444 else if (Configuration.doAutoCreate()) 1445 this.subType = new CodeableConcept(); // cc 1446 return this.subType; 1447 } 1448 1449 public boolean hasSubType() { 1450 return this.subType != null && !this.subType.isEmpty(); 1451 } 1452 1453 /** 1454 * @param value {@link #subType} (A specialized legal clause or condition based on overarching contract type.) 1455 */ 1456 public TermComponent setSubType(CodeableConcept value) { 1457 this.subType = value; 1458 return this; 1459 } 1460 1461 /** 1462 * @return {@link #text} (Statement of a provision in a policy or a contract.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 1463 */ 1464 public StringType getTextElement() { 1465 if (this.text == null) 1466 if (Configuration.errorOnAutoCreate()) 1467 throw new Error("Attempt to auto-create TermComponent.text"); 1468 else if (Configuration.doAutoCreate()) 1469 this.text = new StringType(); // bb 1470 return this.text; 1471 } 1472 1473 public boolean hasTextElement() { 1474 return this.text != null && !this.text.isEmpty(); 1475 } 1476 1477 public boolean hasText() { 1478 return this.text != null && !this.text.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #text} (Statement of a provision in a policy or a contract.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 1483 */ 1484 public TermComponent setTextElement(StringType value) { 1485 this.text = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return Statement of a provision in a policy or a contract. 1491 */ 1492 public String getText() { 1493 return this.text == null ? null : this.text.getValue(); 1494 } 1495 1496 /** 1497 * @param value Statement of a provision in a policy or a contract. 1498 */ 1499 public TermComponent setText(String value) { 1500 if (Utilities.noString(value)) 1501 this.text = null; 1502 else { 1503 if (this.text == null) 1504 this.text = new StringType(); 1505 this.text.setValue(value); 1506 } 1507 return this; 1508 } 1509 1510 /** 1511 * @return {@link #securityLabel} (Security labels that protect the handling of information about the term and its elements, which may be specifically identified.) 1512 */ 1513 public List<SecurityLabelComponent> getSecurityLabel() { 1514 if (this.securityLabel == null) 1515 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1516 return this.securityLabel; 1517 } 1518 1519 /** 1520 * @return Returns a reference to <code>this</code> for easy method chaining 1521 */ 1522 public TermComponent setSecurityLabel(List<SecurityLabelComponent> theSecurityLabel) { 1523 this.securityLabel = theSecurityLabel; 1524 return this; 1525 } 1526 1527 public boolean hasSecurityLabel() { 1528 if (this.securityLabel == null) 1529 return false; 1530 for (SecurityLabelComponent item : this.securityLabel) 1531 if (!item.isEmpty()) 1532 return true; 1533 return false; 1534 } 1535 1536 public SecurityLabelComponent addSecurityLabel() { //3 1537 SecurityLabelComponent t = new SecurityLabelComponent(); 1538 if (this.securityLabel == null) 1539 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1540 this.securityLabel.add(t); 1541 return t; 1542 } 1543 1544 public TermComponent addSecurityLabel(SecurityLabelComponent t) { //3 1545 if (t == null) 1546 return this; 1547 if (this.securityLabel == null) 1548 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1549 this.securityLabel.add(t); 1550 return this; 1551 } 1552 1553 /** 1554 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist {3} 1555 */ 1556 public SecurityLabelComponent getSecurityLabelFirstRep() { 1557 if (getSecurityLabel().isEmpty()) { 1558 addSecurityLabel(); 1559 } 1560 return getSecurityLabel().get(0); 1561 } 1562 1563 /** 1564 * @return {@link #offer} (The matter of concern in the context of this provision of the agrement.) 1565 */ 1566 public ContractOfferComponent getOffer() { 1567 if (this.offer == null) 1568 if (Configuration.errorOnAutoCreate()) 1569 throw new Error("Attempt to auto-create TermComponent.offer"); 1570 else if (Configuration.doAutoCreate()) 1571 this.offer = new ContractOfferComponent(); // cc 1572 return this.offer; 1573 } 1574 1575 public boolean hasOffer() { 1576 return this.offer != null && !this.offer.isEmpty(); 1577 } 1578 1579 /** 1580 * @param value {@link #offer} (The matter of concern in the context of this provision of the agrement.) 1581 */ 1582 public TermComponent setOffer(ContractOfferComponent value) { 1583 this.offer = value; 1584 return this; 1585 } 1586 1587 /** 1588 * @return {@link #asset} (Contract Term Asset List.) 1589 */ 1590 public List<ContractAssetComponent> getAsset() { 1591 if (this.asset == null) 1592 this.asset = new ArrayList<ContractAssetComponent>(); 1593 return this.asset; 1594 } 1595 1596 /** 1597 * @return Returns a reference to <code>this</code> for easy method chaining 1598 */ 1599 public TermComponent setAsset(List<ContractAssetComponent> theAsset) { 1600 this.asset = theAsset; 1601 return this; 1602 } 1603 1604 public boolean hasAsset() { 1605 if (this.asset == null) 1606 return false; 1607 for (ContractAssetComponent item : this.asset) 1608 if (!item.isEmpty()) 1609 return true; 1610 return false; 1611 } 1612 1613 public ContractAssetComponent addAsset() { //3 1614 ContractAssetComponent t = new ContractAssetComponent(); 1615 if (this.asset == null) 1616 this.asset = new ArrayList<ContractAssetComponent>(); 1617 this.asset.add(t); 1618 return t; 1619 } 1620 1621 public TermComponent addAsset(ContractAssetComponent t) { //3 1622 if (t == null) 1623 return this; 1624 if (this.asset == null) 1625 this.asset = new ArrayList<ContractAssetComponent>(); 1626 this.asset.add(t); 1627 return this; 1628 } 1629 1630 /** 1631 * @return The first repetition of repeating field {@link #asset}, creating it if it does not already exist {3} 1632 */ 1633 public ContractAssetComponent getAssetFirstRep() { 1634 if (getAsset().isEmpty()) { 1635 addAsset(); 1636 } 1637 return getAsset().get(0); 1638 } 1639 1640 /** 1641 * @return {@link #action} (An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.) 1642 */ 1643 public List<ActionComponent> getAction() { 1644 if (this.action == null) 1645 this.action = new ArrayList<ActionComponent>(); 1646 return this.action; 1647 } 1648 1649 /** 1650 * @return Returns a reference to <code>this</code> for easy method chaining 1651 */ 1652 public TermComponent setAction(List<ActionComponent> theAction) { 1653 this.action = theAction; 1654 return this; 1655 } 1656 1657 public boolean hasAction() { 1658 if (this.action == null) 1659 return false; 1660 for (ActionComponent item : this.action) 1661 if (!item.isEmpty()) 1662 return true; 1663 return false; 1664 } 1665 1666 public ActionComponent addAction() { //3 1667 ActionComponent t = new ActionComponent(); 1668 if (this.action == null) 1669 this.action = new ArrayList<ActionComponent>(); 1670 this.action.add(t); 1671 return t; 1672 } 1673 1674 public TermComponent addAction(ActionComponent t) { //3 1675 if (t == null) 1676 return this; 1677 if (this.action == null) 1678 this.action = new ArrayList<ActionComponent>(); 1679 this.action.add(t); 1680 return this; 1681 } 1682 1683 /** 1684 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 1685 */ 1686 public ActionComponent getActionFirstRep() { 1687 if (getAction().isEmpty()) { 1688 addAction(); 1689 } 1690 return getAction().get(0); 1691 } 1692 1693 /** 1694 * @return {@link #group} (Nested group of Contract Provisions.) 1695 */ 1696 public List<TermComponent> getGroup() { 1697 if (this.group == null) 1698 this.group = new ArrayList<TermComponent>(); 1699 return this.group; 1700 } 1701 1702 /** 1703 * @return Returns a reference to <code>this</code> for easy method chaining 1704 */ 1705 public TermComponent setGroup(List<TermComponent> theGroup) { 1706 this.group = theGroup; 1707 return this; 1708 } 1709 1710 public boolean hasGroup() { 1711 if (this.group == null) 1712 return false; 1713 for (TermComponent item : this.group) 1714 if (!item.isEmpty()) 1715 return true; 1716 return false; 1717 } 1718 1719 public TermComponent addGroup() { //3 1720 TermComponent t = new TermComponent(); 1721 if (this.group == null) 1722 this.group = new ArrayList<TermComponent>(); 1723 this.group.add(t); 1724 return t; 1725 } 1726 1727 public TermComponent addGroup(TermComponent t) { //3 1728 if (t == null) 1729 return this; 1730 if (this.group == null) 1731 this.group = new ArrayList<TermComponent>(); 1732 this.group.add(t); 1733 return this; 1734 } 1735 1736 /** 1737 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist {3} 1738 */ 1739 public TermComponent getGroupFirstRep() { 1740 if (getGroup().isEmpty()) { 1741 addGroup(); 1742 } 1743 return getGroup().get(0); 1744 } 1745 1746 protected void listChildren(List<Property> children) { 1747 super.listChildren(children); 1748 children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 0, 1, identifier)); 1749 children.add(new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, issued)); 1750 children.add(new Property("applies", "Period", "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies)); 1751 children.add(new Property("topic[x]", "CodeableConcept|Reference(Any)", "The entity that the term applies to.", 0, 1, topic)); 1752 children.add(new Property("type", "CodeableConcept", "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.", 0, 1, type)); 1753 children.add(new Property("subType", "CodeableConcept", "A specialized legal clause or condition based on overarching contract type.", 0, 1, subType)); 1754 children.add(new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1, text)); 1755 children.add(new Property("securityLabel", "", "Security labels that protect the handling of information about the term and its elements, which may be specifically identified.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 1756 children.add(new Property("offer", "", "The matter of concern in the context of this provision of the agrement.", 0, 1, offer)); 1757 children.add(new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE, asset)); 1758 children.add(new Property("action", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, action)); 1759 children.add(new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, java.lang.Integer.MAX_VALUE, group)); 1760 } 1761 1762 @Override 1763 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1764 switch (_hash) { 1765 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 0, 1, identifier); 1766 case -1179159893: /*issued*/ return new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, issued); 1767 case -793235316: /*applies*/ return new Property("applies", "Period", "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies); 1768 case -957295375: /*topic[x]*/ return new Property("topic[x]", "CodeableConcept|Reference(Any)", "The entity that the term applies to.", 0, 1, topic); 1769 case 110546223: /*topic*/ return new Property("topic[x]", "CodeableConcept|Reference(Any)", "The entity that the term applies to.", 0, 1, topic); 1770 case 777778802: /*topicCodeableConcept*/ return new Property("topic[x]", "CodeableConcept", "The entity that the term applies to.", 0, 1, topic); 1771 case -343345444: /*topicReference*/ return new Property("topic[x]", "Reference(Any)", "The entity that the term applies to.", 0, 1, topic); 1772 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.", 0, 1, type); 1773 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A specialized legal clause or condition based on overarching contract type.", 0, 1, subType); 1774 case 3556653: /*text*/ return new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1, text); 1775 case -722296940: /*securityLabel*/ return new Property("securityLabel", "", "Security labels that protect the handling of information about the term and its elements, which may be specifically identified.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 1776 case 105650780: /*offer*/ return new Property("offer", "", "The matter of concern in the context of this provision of the agrement.", 0, 1, offer); 1777 case 93121264: /*asset*/ return new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE, asset); 1778 case -1422950858: /*action*/ return new Property("action", "", "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 0, java.lang.Integer.MAX_VALUE, action); 1779 case 98629247: /*group*/ return new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, java.lang.Integer.MAX_VALUE, group); 1780 default: return super.getNamedProperty(_hash, _name, _checkValid); 1781 } 1782 1783 } 1784 1785 @Override 1786 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1787 switch (hash) { 1788 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1789 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // DateTimeType 1790 case -793235316: /*applies*/ return this.applies == null ? new Base[0] : new Base[] {this.applies}; // Period 1791 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : new Base[] {this.topic}; // DataType 1792 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1793 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 1794 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 1795 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // SecurityLabelComponent 1796 case 105650780: /*offer*/ return this.offer == null ? new Base[0] : new Base[] {this.offer}; // ContractOfferComponent 1797 case 93121264: /*asset*/ return this.asset == null ? new Base[0] : this.asset.toArray(new Base[this.asset.size()]); // ContractAssetComponent 1798 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // ActionComponent 1799 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // TermComponent 1800 default: return super.getProperty(hash, name, checkValid); 1801 } 1802 1803 } 1804 1805 @Override 1806 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1807 switch (hash) { 1808 case -1618432855: // identifier 1809 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1810 return value; 1811 case -1179159893: // issued 1812 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 1813 return value; 1814 case -793235316: // applies 1815 this.applies = TypeConvertor.castToPeriod(value); // Period 1816 return value; 1817 case 110546223: // topic 1818 this.topic = TypeConvertor.castToType(value); // DataType 1819 return value; 1820 case 3575610: // type 1821 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1822 return value; 1823 case -1868521062: // subType 1824 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1825 return value; 1826 case 3556653: // text 1827 this.text = TypeConvertor.castToString(value); // StringType 1828 return value; 1829 case -722296940: // securityLabel 1830 this.getSecurityLabel().add((SecurityLabelComponent) value); // SecurityLabelComponent 1831 return value; 1832 case 105650780: // offer 1833 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 1834 return value; 1835 case 93121264: // asset 1836 this.getAsset().add((ContractAssetComponent) value); // ContractAssetComponent 1837 return value; 1838 case -1422950858: // action 1839 this.getAction().add((ActionComponent) value); // ActionComponent 1840 return value; 1841 case 98629247: // group 1842 this.getGroup().add((TermComponent) value); // TermComponent 1843 return value; 1844 default: return super.setProperty(hash, name, value); 1845 } 1846 1847 } 1848 1849 @Override 1850 public Base setProperty(String name, Base value) throws FHIRException { 1851 if (name.equals("identifier")) { 1852 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1853 } else if (name.equals("issued")) { 1854 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 1855 } else if (name.equals("applies")) { 1856 this.applies = TypeConvertor.castToPeriod(value); // Period 1857 } else if (name.equals("topic[x]")) { 1858 this.topic = TypeConvertor.castToType(value); // DataType 1859 } else if (name.equals("type")) { 1860 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1861 } else if (name.equals("subType")) { 1862 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1863 } else if (name.equals("text")) { 1864 this.text = TypeConvertor.castToString(value); // StringType 1865 } else if (name.equals("securityLabel")) { 1866 this.getSecurityLabel().add((SecurityLabelComponent) value); 1867 } else if (name.equals("offer")) { 1868 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 1869 } else if (name.equals("asset")) { 1870 this.getAsset().add((ContractAssetComponent) value); 1871 } else if (name.equals("action")) { 1872 this.getAction().add((ActionComponent) value); 1873 } else if (name.equals("group")) { 1874 this.getGroup().add((TermComponent) value); 1875 } else 1876 return super.setProperty(name, value); 1877 return value; 1878 } 1879 1880 @Override 1881 public void removeChild(String name, Base value) throws FHIRException { 1882 if (name.equals("identifier")) { 1883 this.identifier = null; 1884 } else if (name.equals("issued")) { 1885 this.issued = null; 1886 } else if (name.equals("applies")) { 1887 this.applies = null; 1888 } else if (name.equals("topic[x]")) { 1889 this.topic = null; 1890 } else if (name.equals("type")) { 1891 this.type = null; 1892 } else if (name.equals("subType")) { 1893 this.subType = null; 1894 } else if (name.equals("text")) { 1895 this.text = null; 1896 } else if (name.equals("securityLabel")) { 1897 this.getSecurityLabel().remove((SecurityLabelComponent) value); 1898 } else if (name.equals("offer")) { 1899 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 1900 } else if (name.equals("asset")) { 1901 this.getAsset().remove((ContractAssetComponent) value); 1902 } else if (name.equals("action")) { 1903 this.getAction().remove((ActionComponent) value); 1904 } else if (name.equals("group")) { 1905 this.getGroup().remove((TermComponent) value); 1906 } else 1907 super.removeChild(name, value); 1908 1909 } 1910 1911 @Override 1912 public Base makeProperty(int hash, String name) throws FHIRException { 1913 switch (hash) { 1914 case -1618432855: return getIdentifier(); 1915 case -1179159893: return getIssuedElement(); 1916 case -793235316: return getApplies(); 1917 case -957295375: return getTopic(); 1918 case 110546223: return getTopic(); 1919 case 3575610: return getType(); 1920 case -1868521062: return getSubType(); 1921 case 3556653: return getTextElement(); 1922 case -722296940: return addSecurityLabel(); 1923 case 105650780: return getOffer(); 1924 case 93121264: return addAsset(); 1925 case -1422950858: return addAction(); 1926 case 98629247: return addGroup(); 1927 default: return super.makeProperty(hash, name); 1928 } 1929 1930 } 1931 1932 @Override 1933 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1934 switch (hash) { 1935 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1936 case -1179159893: /*issued*/ return new String[] {"dateTime"}; 1937 case -793235316: /*applies*/ return new String[] {"Period"}; 1938 case 110546223: /*topic*/ return new String[] {"CodeableConcept", "Reference"}; 1939 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1940 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 1941 case 3556653: /*text*/ return new String[] {"string"}; 1942 case -722296940: /*securityLabel*/ return new String[] {}; 1943 case 105650780: /*offer*/ return new String[] {}; 1944 case 93121264: /*asset*/ return new String[] {}; 1945 case -1422950858: /*action*/ return new String[] {}; 1946 case 98629247: /*group*/ return new String[] {"@Contract.term"}; 1947 default: return super.getTypesForProperty(hash, name); 1948 } 1949 1950 } 1951 1952 @Override 1953 public Base addChild(String name) throws FHIRException { 1954 if (name.equals("identifier")) { 1955 this.identifier = new Identifier(); 1956 return this.identifier; 1957 } 1958 else if (name.equals("issued")) { 1959 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.issued"); 1960 } 1961 else if (name.equals("applies")) { 1962 this.applies = new Period(); 1963 return this.applies; 1964 } 1965 else if (name.equals("topicCodeableConcept")) { 1966 this.topic = new CodeableConcept(); 1967 return this.topic; 1968 } 1969 else if (name.equals("topicReference")) { 1970 this.topic = new Reference(); 1971 return this.topic; 1972 } 1973 else if (name.equals("type")) { 1974 this.type = new CodeableConcept(); 1975 return this.type; 1976 } 1977 else if (name.equals("subType")) { 1978 this.subType = new CodeableConcept(); 1979 return this.subType; 1980 } 1981 else if (name.equals("text")) { 1982 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.text"); 1983 } 1984 else if (name.equals("securityLabel")) { 1985 return addSecurityLabel(); 1986 } 1987 else if (name.equals("offer")) { 1988 this.offer = new ContractOfferComponent(); 1989 return this.offer; 1990 } 1991 else if (name.equals("asset")) { 1992 return addAsset(); 1993 } 1994 else if (name.equals("action")) { 1995 return addAction(); 1996 } 1997 else if (name.equals("group")) { 1998 return addGroup(); 1999 } 2000 else 2001 return super.addChild(name); 2002 } 2003 2004 public TermComponent copy() { 2005 TermComponent dst = new TermComponent(); 2006 copyValues(dst); 2007 return dst; 2008 } 2009 2010 public void copyValues(TermComponent dst) { 2011 super.copyValues(dst); 2012 dst.identifier = identifier == null ? null : identifier.copy(); 2013 dst.issued = issued == null ? null : issued.copy(); 2014 dst.applies = applies == null ? null : applies.copy(); 2015 dst.topic = topic == null ? null : topic.copy(); 2016 dst.type = type == null ? null : type.copy(); 2017 dst.subType = subType == null ? null : subType.copy(); 2018 dst.text = text == null ? null : text.copy(); 2019 if (securityLabel != null) { 2020 dst.securityLabel = new ArrayList<SecurityLabelComponent>(); 2021 for (SecurityLabelComponent i : securityLabel) 2022 dst.securityLabel.add(i.copy()); 2023 }; 2024 dst.offer = offer == null ? null : offer.copy(); 2025 if (asset != null) { 2026 dst.asset = new ArrayList<ContractAssetComponent>(); 2027 for (ContractAssetComponent i : asset) 2028 dst.asset.add(i.copy()); 2029 }; 2030 if (action != null) { 2031 dst.action = new ArrayList<ActionComponent>(); 2032 for (ActionComponent i : action) 2033 dst.action.add(i.copy()); 2034 }; 2035 if (group != null) { 2036 dst.group = new ArrayList<TermComponent>(); 2037 for (TermComponent i : group) 2038 dst.group.add(i.copy()); 2039 }; 2040 } 2041 2042 @Override 2043 public boolean equalsDeep(Base other_) { 2044 if (!super.equalsDeep(other_)) 2045 return false; 2046 if (!(other_ instanceof TermComponent)) 2047 return false; 2048 TermComponent o = (TermComponent) other_; 2049 return compareDeep(identifier, o.identifier, true) && compareDeep(issued, o.issued, true) && compareDeep(applies, o.applies, true) 2050 && compareDeep(topic, o.topic, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 2051 && compareDeep(text, o.text, true) && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(offer, o.offer, true) 2052 && compareDeep(asset, o.asset, true) && compareDeep(action, o.action, true) && compareDeep(group, o.group, true) 2053 ; 2054 } 2055 2056 @Override 2057 public boolean equalsShallow(Base other_) { 2058 if (!super.equalsShallow(other_)) 2059 return false; 2060 if (!(other_ instanceof TermComponent)) 2061 return false; 2062 TermComponent o = (TermComponent) other_; 2063 return compareValues(issued, o.issued, true) && compareValues(text, o.text, true); 2064 } 2065 2066 public boolean isEmpty() { 2067 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, issued, applies 2068 , topic, type, subType, text, securityLabel, offer, asset, action, group); 2069 } 2070 2071 public String fhirType() { 2072 return "Contract.term"; 2073 2074 } 2075 2076 } 2077 2078 @Block() 2079 public static class SecurityLabelComponent extends BackboneElement implements IBaseBackboneElement { 2080 /** 2081 * Number used to link this term or term element to the applicable Security Label. 2082 */ 2083 @Child(name = "number", type = {UnsignedIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2084 @Description(shortDefinition="Link to Security Labels", formalDefinition="Number used to link this term or term element to the applicable Security Label." ) 2085 protected List<UnsignedIntType> number; 2086 2087 /** 2088 * Security label privacy tag that specifies the level of confidentiality protection required for this term and/or term elements. 2089 */ 2090 @Child(name = "classification", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=false) 2091 @Description(shortDefinition="Confidentiality Protection", formalDefinition="Security label privacy tag that specifies the level of confidentiality protection required for this term and/or term elements." ) 2092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-security-classification") 2093 protected Coding classification; 2094 2095 /** 2096 * Security label privacy tag that specifies the applicable privacy and security policies governing this term and/or term elements. 2097 */ 2098 @Child(name = "category", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2099 @Description(shortDefinition="Applicable Policy", formalDefinition="Security label privacy tag that specifies the applicable privacy and security policies governing this term and/or term elements." ) 2100 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-security-category") 2101 protected List<Coding> category; 2102 2103 /** 2104 * Security label privacy tag that specifies the manner in which term and/or term elements are to be protected. 2105 */ 2106 @Child(name = "control", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2107 @Description(shortDefinition="Handling Instructions", formalDefinition="Security label privacy tag that specifies the manner in which term and/or term elements are to be protected." ) 2108 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-security-control") 2109 protected List<Coding> control; 2110 2111 private static final long serialVersionUID = 788281758L; 2112 2113 /** 2114 * Constructor 2115 */ 2116 public SecurityLabelComponent() { 2117 super(); 2118 } 2119 2120 /** 2121 * Constructor 2122 */ 2123 public SecurityLabelComponent(Coding classification) { 2124 super(); 2125 this.setClassification(classification); 2126 } 2127 2128 /** 2129 * @return {@link #number} (Number used to link this term or term element to the applicable Security Label.) 2130 */ 2131 public List<UnsignedIntType> getNumber() { 2132 if (this.number == null) 2133 this.number = new ArrayList<UnsignedIntType>(); 2134 return this.number; 2135 } 2136 2137 /** 2138 * @return Returns a reference to <code>this</code> for easy method chaining 2139 */ 2140 public SecurityLabelComponent setNumber(List<UnsignedIntType> theNumber) { 2141 this.number = theNumber; 2142 return this; 2143 } 2144 2145 public boolean hasNumber() { 2146 if (this.number == null) 2147 return false; 2148 for (UnsignedIntType item : this.number) 2149 if (!item.isEmpty()) 2150 return true; 2151 return false; 2152 } 2153 2154 /** 2155 * @return {@link #number} (Number used to link this term or term element to the applicable Security Label.) 2156 */ 2157 public UnsignedIntType addNumberElement() {//2 2158 UnsignedIntType t = new UnsignedIntType(); 2159 if (this.number == null) 2160 this.number = new ArrayList<UnsignedIntType>(); 2161 this.number.add(t); 2162 return t; 2163 } 2164 2165 /** 2166 * @param value {@link #number} (Number used to link this term or term element to the applicable Security Label.) 2167 */ 2168 public SecurityLabelComponent addNumber(int value) { //1 2169 UnsignedIntType t = new UnsignedIntType(); 2170 t.setValue(value); 2171 if (this.number == null) 2172 this.number = new ArrayList<UnsignedIntType>(); 2173 this.number.add(t); 2174 return this; 2175 } 2176 2177 /** 2178 * @param value {@link #number} (Number used to link this term or term element to the applicable Security Label.) 2179 */ 2180 public boolean hasNumber(int value) { 2181 if (this.number == null) 2182 return false; 2183 for (UnsignedIntType v : this.number) 2184 if (v.getValue().equals(value)) // unsignedInt 2185 return true; 2186 return false; 2187 } 2188 2189 /** 2190 * @return {@link #classification} (Security label privacy tag that specifies the level of confidentiality protection required for this term and/or term elements.) 2191 */ 2192 public Coding getClassification() { 2193 if (this.classification == null) 2194 if (Configuration.errorOnAutoCreate()) 2195 throw new Error("Attempt to auto-create SecurityLabelComponent.classification"); 2196 else if (Configuration.doAutoCreate()) 2197 this.classification = new Coding(); // cc 2198 return this.classification; 2199 } 2200 2201 public boolean hasClassification() { 2202 return this.classification != null && !this.classification.isEmpty(); 2203 } 2204 2205 /** 2206 * @param value {@link #classification} (Security label privacy tag that specifies the level of confidentiality protection required for this term and/or term elements.) 2207 */ 2208 public SecurityLabelComponent setClassification(Coding value) { 2209 this.classification = value; 2210 return this; 2211 } 2212 2213 /** 2214 * @return {@link #category} (Security label privacy tag that specifies the applicable privacy and security policies governing this term and/or term elements.) 2215 */ 2216 public List<Coding> getCategory() { 2217 if (this.category == null) 2218 this.category = new ArrayList<Coding>(); 2219 return this.category; 2220 } 2221 2222 /** 2223 * @return Returns a reference to <code>this</code> for easy method chaining 2224 */ 2225 public SecurityLabelComponent setCategory(List<Coding> theCategory) { 2226 this.category = theCategory; 2227 return this; 2228 } 2229 2230 public boolean hasCategory() { 2231 if (this.category == null) 2232 return false; 2233 for (Coding item : this.category) 2234 if (!item.isEmpty()) 2235 return true; 2236 return false; 2237 } 2238 2239 public Coding addCategory() { //3 2240 Coding t = new Coding(); 2241 if (this.category == null) 2242 this.category = new ArrayList<Coding>(); 2243 this.category.add(t); 2244 return t; 2245 } 2246 2247 public SecurityLabelComponent addCategory(Coding t) { //3 2248 if (t == null) 2249 return this; 2250 if (this.category == null) 2251 this.category = new ArrayList<Coding>(); 2252 this.category.add(t); 2253 return this; 2254 } 2255 2256 /** 2257 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2258 */ 2259 public Coding getCategoryFirstRep() { 2260 if (getCategory().isEmpty()) { 2261 addCategory(); 2262 } 2263 return getCategory().get(0); 2264 } 2265 2266 /** 2267 * @return {@link #control} (Security label privacy tag that specifies the manner in which term and/or term elements are to be protected.) 2268 */ 2269 public List<Coding> getControl() { 2270 if (this.control == null) 2271 this.control = new ArrayList<Coding>(); 2272 return this.control; 2273 } 2274 2275 /** 2276 * @return Returns a reference to <code>this</code> for easy method chaining 2277 */ 2278 public SecurityLabelComponent setControl(List<Coding> theControl) { 2279 this.control = theControl; 2280 return this; 2281 } 2282 2283 public boolean hasControl() { 2284 if (this.control == null) 2285 return false; 2286 for (Coding item : this.control) 2287 if (!item.isEmpty()) 2288 return true; 2289 return false; 2290 } 2291 2292 public Coding addControl() { //3 2293 Coding t = new Coding(); 2294 if (this.control == null) 2295 this.control = new ArrayList<Coding>(); 2296 this.control.add(t); 2297 return t; 2298 } 2299 2300 public SecurityLabelComponent addControl(Coding t) { //3 2301 if (t == null) 2302 return this; 2303 if (this.control == null) 2304 this.control = new ArrayList<Coding>(); 2305 this.control.add(t); 2306 return this; 2307 } 2308 2309 /** 2310 * @return The first repetition of repeating field {@link #control}, creating it if it does not already exist {3} 2311 */ 2312 public Coding getControlFirstRep() { 2313 if (getControl().isEmpty()) { 2314 addControl(); 2315 } 2316 return getControl().get(0); 2317 } 2318 2319 protected void listChildren(List<Property> children) { 2320 super.listChildren(children); 2321 children.add(new Property("number", "unsignedInt", "Number used to link this term or term element to the applicable Security Label.", 0, java.lang.Integer.MAX_VALUE, number)); 2322 children.add(new Property("classification", "Coding", "Security label privacy tag that specifies the level of confidentiality protection required for this term and/or term elements.", 0, 1, classification)); 2323 children.add(new Property("category", "Coding", "Security label privacy tag that specifies the applicable privacy and security policies governing this term and/or term elements.", 0, java.lang.Integer.MAX_VALUE, category)); 2324 children.add(new Property("control", "Coding", "Security label privacy tag that specifies the manner in which term and/or term elements are to be protected.", 0, java.lang.Integer.MAX_VALUE, control)); 2325 } 2326 2327 @Override 2328 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2329 switch (_hash) { 2330 case -1034364087: /*number*/ return new Property("number", "unsignedInt", "Number used to link this term or term element to the applicable Security Label.", 0, java.lang.Integer.MAX_VALUE, number); 2331 case 382350310: /*classification*/ return new Property("classification", "Coding", "Security label privacy tag that specifies the level of confidentiality protection required for this term and/or term elements.", 0, 1, classification); 2332 case 50511102: /*category*/ return new Property("category", "Coding", "Security label privacy tag that specifies the applicable privacy and security policies governing this term and/or term elements.", 0, java.lang.Integer.MAX_VALUE, category); 2333 case 951543133: /*control*/ return new Property("control", "Coding", "Security label privacy tag that specifies the manner in which term and/or term elements are to be protected.", 0, java.lang.Integer.MAX_VALUE, control); 2334 default: return super.getNamedProperty(_hash, _name, _checkValid); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2341 switch (hash) { 2342 case -1034364087: /*number*/ return this.number == null ? new Base[0] : this.number.toArray(new Base[this.number.size()]); // UnsignedIntType 2343 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : new Base[] {this.classification}; // Coding 2344 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // Coding 2345 case 951543133: /*control*/ return this.control == null ? new Base[0] : this.control.toArray(new Base[this.control.size()]); // Coding 2346 default: return super.getProperty(hash, name, checkValid); 2347 } 2348 2349 } 2350 2351 @Override 2352 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2353 switch (hash) { 2354 case -1034364087: // number 2355 this.getNumber().add(TypeConvertor.castToUnsignedInt(value)); // UnsignedIntType 2356 return value; 2357 case 382350310: // classification 2358 this.classification = TypeConvertor.castToCoding(value); // Coding 2359 return value; 2360 case 50511102: // category 2361 this.getCategory().add(TypeConvertor.castToCoding(value)); // Coding 2362 return value; 2363 case 951543133: // control 2364 this.getControl().add(TypeConvertor.castToCoding(value)); // Coding 2365 return value; 2366 default: return super.setProperty(hash, name, value); 2367 } 2368 2369 } 2370 2371 @Override 2372 public Base setProperty(String name, Base value) throws FHIRException { 2373 if (name.equals("number")) { 2374 this.getNumber().add(TypeConvertor.castToUnsignedInt(value)); 2375 } else if (name.equals("classification")) { 2376 this.classification = TypeConvertor.castToCoding(value); // Coding 2377 } else if (name.equals("category")) { 2378 this.getCategory().add(TypeConvertor.castToCoding(value)); 2379 } else if (name.equals("control")) { 2380 this.getControl().add(TypeConvertor.castToCoding(value)); 2381 } else 2382 return super.setProperty(name, value); 2383 return value; 2384 } 2385 2386 @Override 2387 public void removeChild(String name, Base value) throws FHIRException { 2388 if (name.equals("number")) { 2389 this.getNumber().remove(value); 2390 } else if (name.equals("classification")) { 2391 this.classification = null; 2392 } else if (name.equals("category")) { 2393 this.getCategory().remove(value); 2394 } else if (name.equals("control")) { 2395 this.getControl().remove(value); 2396 } else 2397 super.removeChild(name, value); 2398 2399 } 2400 2401 @Override 2402 public Base makeProperty(int hash, String name) throws FHIRException { 2403 switch (hash) { 2404 case -1034364087: return addNumberElement(); 2405 case 382350310: return getClassification(); 2406 case 50511102: return addCategory(); 2407 case 951543133: return addControl(); 2408 default: return super.makeProperty(hash, name); 2409 } 2410 2411 } 2412 2413 @Override 2414 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2415 switch (hash) { 2416 case -1034364087: /*number*/ return new String[] {"unsignedInt"}; 2417 case 382350310: /*classification*/ return new String[] {"Coding"}; 2418 case 50511102: /*category*/ return new String[] {"Coding"}; 2419 case 951543133: /*control*/ return new String[] {"Coding"}; 2420 default: return super.getTypesForProperty(hash, name); 2421 } 2422 2423 } 2424 2425 @Override 2426 public Base addChild(String name) throws FHIRException { 2427 if (name.equals("number")) { 2428 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.securityLabel.number"); 2429 } 2430 else if (name.equals("classification")) { 2431 this.classification = new Coding(); 2432 return this.classification; 2433 } 2434 else if (name.equals("category")) { 2435 return addCategory(); 2436 } 2437 else if (name.equals("control")) { 2438 return addControl(); 2439 } 2440 else 2441 return super.addChild(name); 2442 } 2443 2444 public SecurityLabelComponent copy() { 2445 SecurityLabelComponent dst = new SecurityLabelComponent(); 2446 copyValues(dst); 2447 return dst; 2448 } 2449 2450 public void copyValues(SecurityLabelComponent dst) { 2451 super.copyValues(dst); 2452 if (number != null) { 2453 dst.number = new ArrayList<UnsignedIntType>(); 2454 for (UnsignedIntType i : number) 2455 dst.number.add(i.copy()); 2456 }; 2457 dst.classification = classification == null ? null : classification.copy(); 2458 if (category != null) { 2459 dst.category = new ArrayList<Coding>(); 2460 for (Coding i : category) 2461 dst.category.add(i.copy()); 2462 }; 2463 if (control != null) { 2464 dst.control = new ArrayList<Coding>(); 2465 for (Coding i : control) 2466 dst.control.add(i.copy()); 2467 }; 2468 } 2469 2470 @Override 2471 public boolean equalsDeep(Base other_) { 2472 if (!super.equalsDeep(other_)) 2473 return false; 2474 if (!(other_ instanceof SecurityLabelComponent)) 2475 return false; 2476 SecurityLabelComponent o = (SecurityLabelComponent) other_; 2477 return compareDeep(number, o.number, true) && compareDeep(classification, o.classification, true) 2478 && compareDeep(category, o.category, true) && compareDeep(control, o.control, true); 2479 } 2480 2481 @Override 2482 public boolean equalsShallow(Base other_) { 2483 if (!super.equalsShallow(other_)) 2484 return false; 2485 if (!(other_ instanceof SecurityLabelComponent)) 2486 return false; 2487 SecurityLabelComponent o = (SecurityLabelComponent) other_; 2488 return compareValues(number, o.number, true); 2489 } 2490 2491 public boolean isEmpty() { 2492 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, classification, category 2493 , control); 2494 } 2495 2496 public String fhirType() { 2497 return "Contract.term.securityLabel"; 2498 2499 } 2500 2501 } 2502 2503 @Block() 2504 public static class ContractOfferComponent extends BackboneElement implements IBaseBackboneElement { 2505 /** 2506 * Unique identifier for this particular Contract Provision. 2507 */ 2508 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2509 @Description(shortDefinition="Offer business ID", formalDefinition="Unique identifier for this particular Contract Provision." ) 2510 protected List<Identifier> identifier; 2511 2512 /** 2513 * Offer Recipient. 2514 */ 2515 @Child(name = "party", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2516 @Description(shortDefinition="Offer Recipient", formalDefinition="Offer Recipient." ) 2517 protected List<ContractPartyComponent> party; 2518 2519 /** 2520 * The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30). 2521 */ 2522 @Child(name = "topic", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=true) 2523 @Description(shortDefinition="Negotiable offer asset", formalDefinition="The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30)." ) 2524 protected Reference topic; 2525 2526 /** 2527 * Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit. 2528 */ 2529 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 2530 @Description(shortDefinition="Contract Offer Type or Form", formalDefinition="Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit." ) 2531 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-term-type") 2532 protected CodeableConcept type; 2533 2534 /** 2535 * Type of choice made by accepting party with respect to an offer made by an offeror/ grantee. 2536 */ 2537 @Child(name = "decision", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 2538 @Description(shortDefinition="Accepting party choice", formalDefinition="Type of choice made by accepting party with respect to an offer made by an offeror/ grantee." ) 2539 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActConsentDirective") 2540 protected CodeableConcept decision; 2541 2542 /** 2543 * How the decision about a Contract was conveyed. 2544 */ 2545 @Child(name = "decisionMode", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2546 @Description(shortDefinition="How decision is conveyed", formalDefinition="How the decision about a Contract was conveyed." ) 2547 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-decision-mode") 2548 protected List<CodeableConcept> decisionMode; 2549 2550 /** 2551 * Response to offer text. 2552 */ 2553 @Child(name = "answer", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2554 @Description(shortDefinition="Response to offer text", formalDefinition="Response to offer text." ) 2555 protected List<AnswerComponent> answer; 2556 2557 /** 2558 * Human readable form of this Contract Offer. 2559 */ 2560 @Child(name = "text", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2561 @Description(shortDefinition="Human readable offer text", formalDefinition="Human readable form of this Contract Offer." ) 2562 protected StringType text; 2563 2564 /** 2565 * The id of the clause or question text of the offer in the referenced questionnaire/response. 2566 */ 2567 @Child(name = "linkId", type = {StringType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2568 @Description(shortDefinition="Pointer to text", formalDefinition="The id of the clause or question text of the offer in the referenced questionnaire/response." ) 2569 protected List<StringType> linkId; 2570 2571 /** 2572 * Security labels that protects the offer. 2573 */ 2574 @Child(name = "securityLabelNumber", type = {UnsignedIntType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2575 @Description(shortDefinition="Offer restriction numbers", formalDefinition="Security labels that protects the offer." ) 2576 protected List<UnsignedIntType> securityLabelNumber; 2577 2578 private static final long serialVersionUID = -852140711L; 2579 2580 /** 2581 * Constructor 2582 */ 2583 public ContractOfferComponent() { 2584 super(); 2585 } 2586 2587 /** 2588 * @return {@link #identifier} (Unique identifier for this particular Contract Provision.) 2589 */ 2590 public List<Identifier> getIdentifier() { 2591 if (this.identifier == null) 2592 this.identifier = new ArrayList<Identifier>(); 2593 return this.identifier; 2594 } 2595 2596 /** 2597 * @return Returns a reference to <code>this</code> for easy method chaining 2598 */ 2599 public ContractOfferComponent setIdentifier(List<Identifier> theIdentifier) { 2600 this.identifier = theIdentifier; 2601 return this; 2602 } 2603 2604 public boolean hasIdentifier() { 2605 if (this.identifier == null) 2606 return false; 2607 for (Identifier item : this.identifier) 2608 if (!item.isEmpty()) 2609 return true; 2610 return false; 2611 } 2612 2613 public Identifier addIdentifier() { //3 2614 Identifier t = new Identifier(); 2615 if (this.identifier == null) 2616 this.identifier = new ArrayList<Identifier>(); 2617 this.identifier.add(t); 2618 return t; 2619 } 2620 2621 public ContractOfferComponent addIdentifier(Identifier t) { //3 2622 if (t == null) 2623 return this; 2624 if (this.identifier == null) 2625 this.identifier = new ArrayList<Identifier>(); 2626 this.identifier.add(t); 2627 return this; 2628 } 2629 2630 /** 2631 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2632 */ 2633 public Identifier getIdentifierFirstRep() { 2634 if (getIdentifier().isEmpty()) { 2635 addIdentifier(); 2636 } 2637 return getIdentifier().get(0); 2638 } 2639 2640 /** 2641 * @return {@link #party} (Offer Recipient.) 2642 */ 2643 public List<ContractPartyComponent> getParty() { 2644 if (this.party == null) 2645 this.party = new ArrayList<ContractPartyComponent>(); 2646 return this.party; 2647 } 2648 2649 /** 2650 * @return Returns a reference to <code>this</code> for easy method chaining 2651 */ 2652 public ContractOfferComponent setParty(List<ContractPartyComponent> theParty) { 2653 this.party = theParty; 2654 return this; 2655 } 2656 2657 public boolean hasParty() { 2658 if (this.party == null) 2659 return false; 2660 for (ContractPartyComponent item : this.party) 2661 if (!item.isEmpty()) 2662 return true; 2663 return false; 2664 } 2665 2666 public ContractPartyComponent addParty() { //3 2667 ContractPartyComponent t = new ContractPartyComponent(); 2668 if (this.party == null) 2669 this.party = new ArrayList<ContractPartyComponent>(); 2670 this.party.add(t); 2671 return t; 2672 } 2673 2674 public ContractOfferComponent addParty(ContractPartyComponent t) { //3 2675 if (t == null) 2676 return this; 2677 if (this.party == null) 2678 this.party = new ArrayList<ContractPartyComponent>(); 2679 this.party.add(t); 2680 return this; 2681 } 2682 2683 /** 2684 * @return The first repetition of repeating field {@link #party}, creating it if it does not already exist {3} 2685 */ 2686 public ContractPartyComponent getPartyFirstRep() { 2687 if (getParty().isEmpty()) { 2688 addParty(); 2689 } 2690 return getParty().get(0); 2691 } 2692 2693 /** 2694 * @return {@link #topic} (The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).) 2695 */ 2696 public Reference getTopic() { 2697 if (this.topic == null) 2698 if (Configuration.errorOnAutoCreate()) 2699 throw new Error("Attempt to auto-create ContractOfferComponent.topic"); 2700 else if (Configuration.doAutoCreate()) 2701 this.topic = new Reference(); // cc 2702 return this.topic; 2703 } 2704 2705 public boolean hasTopic() { 2706 return this.topic != null && !this.topic.isEmpty(); 2707 } 2708 2709 /** 2710 * @param value {@link #topic} (The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).) 2711 */ 2712 public ContractOfferComponent setTopic(Reference value) { 2713 this.topic = value; 2714 return this; 2715 } 2716 2717 /** 2718 * @return {@link #type} (Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.) 2719 */ 2720 public CodeableConcept getType() { 2721 if (this.type == null) 2722 if (Configuration.errorOnAutoCreate()) 2723 throw new Error("Attempt to auto-create ContractOfferComponent.type"); 2724 else if (Configuration.doAutoCreate()) 2725 this.type = new CodeableConcept(); // cc 2726 return this.type; 2727 } 2728 2729 public boolean hasType() { 2730 return this.type != null && !this.type.isEmpty(); 2731 } 2732 2733 /** 2734 * @param value {@link #type} (Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.) 2735 */ 2736 public ContractOfferComponent setType(CodeableConcept value) { 2737 this.type = value; 2738 return this; 2739 } 2740 2741 /** 2742 * @return {@link #decision} (Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.) 2743 */ 2744 public CodeableConcept getDecision() { 2745 if (this.decision == null) 2746 if (Configuration.errorOnAutoCreate()) 2747 throw new Error("Attempt to auto-create ContractOfferComponent.decision"); 2748 else if (Configuration.doAutoCreate()) 2749 this.decision = new CodeableConcept(); // cc 2750 return this.decision; 2751 } 2752 2753 public boolean hasDecision() { 2754 return this.decision != null && !this.decision.isEmpty(); 2755 } 2756 2757 /** 2758 * @param value {@link #decision} (Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.) 2759 */ 2760 public ContractOfferComponent setDecision(CodeableConcept value) { 2761 this.decision = value; 2762 return this; 2763 } 2764 2765 /** 2766 * @return {@link #decisionMode} (How the decision about a Contract was conveyed.) 2767 */ 2768 public List<CodeableConcept> getDecisionMode() { 2769 if (this.decisionMode == null) 2770 this.decisionMode = new ArrayList<CodeableConcept>(); 2771 return this.decisionMode; 2772 } 2773 2774 /** 2775 * @return Returns a reference to <code>this</code> for easy method chaining 2776 */ 2777 public ContractOfferComponent setDecisionMode(List<CodeableConcept> theDecisionMode) { 2778 this.decisionMode = theDecisionMode; 2779 return this; 2780 } 2781 2782 public boolean hasDecisionMode() { 2783 if (this.decisionMode == null) 2784 return false; 2785 for (CodeableConcept item : this.decisionMode) 2786 if (!item.isEmpty()) 2787 return true; 2788 return false; 2789 } 2790 2791 public CodeableConcept addDecisionMode() { //3 2792 CodeableConcept t = new CodeableConcept(); 2793 if (this.decisionMode == null) 2794 this.decisionMode = new ArrayList<CodeableConcept>(); 2795 this.decisionMode.add(t); 2796 return t; 2797 } 2798 2799 public ContractOfferComponent addDecisionMode(CodeableConcept t) { //3 2800 if (t == null) 2801 return this; 2802 if (this.decisionMode == null) 2803 this.decisionMode = new ArrayList<CodeableConcept>(); 2804 this.decisionMode.add(t); 2805 return this; 2806 } 2807 2808 /** 2809 * @return The first repetition of repeating field {@link #decisionMode}, creating it if it does not already exist {3} 2810 */ 2811 public CodeableConcept getDecisionModeFirstRep() { 2812 if (getDecisionMode().isEmpty()) { 2813 addDecisionMode(); 2814 } 2815 return getDecisionMode().get(0); 2816 } 2817 2818 /** 2819 * @return {@link #answer} (Response to offer text.) 2820 */ 2821 public List<AnswerComponent> getAnswer() { 2822 if (this.answer == null) 2823 this.answer = new ArrayList<AnswerComponent>(); 2824 return this.answer; 2825 } 2826 2827 /** 2828 * @return Returns a reference to <code>this</code> for easy method chaining 2829 */ 2830 public ContractOfferComponent setAnswer(List<AnswerComponent> theAnswer) { 2831 this.answer = theAnswer; 2832 return this; 2833 } 2834 2835 public boolean hasAnswer() { 2836 if (this.answer == null) 2837 return false; 2838 for (AnswerComponent item : this.answer) 2839 if (!item.isEmpty()) 2840 return true; 2841 return false; 2842 } 2843 2844 public AnswerComponent addAnswer() { //3 2845 AnswerComponent t = new AnswerComponent(); 2846 if (this.answer == null) 2847 this.answer = new ArrayList<AnswerComponent>(); 2848 this.answer.add(t); 2849 return t; 2850 } 2851 2852 public ContractOfferComponent addAnswer(AnswerComponent t) { //3 2853 if (t == null) 2854 return this; 2855 if (this.answer == null) 2856 this.answer = new ArrayList<AnswerComponent>(); 2857 this.answer.add(t); 2858 return this; 2859 } 2860 2861 /** 2862 * @return The first repetition of repeating field {@link #answer}, creating it if it does not already exist {3} 2863 */ 2864 public AnswerComponent getAnswerFirstRep() { 2865 if (getAnswer().isEmpty()) { 2866 addAnswer(); 2867 } 2868 return getAnswer().get(0); 2869 } 2870 2871 /** 2872 * @return {@link #text} (Human readable form of this Contract Offer.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 2873 */ 2874 public StringType getTextElement() { 2875 if (this.text == null) 2876 if (Configuration.errorOnAutoCreate()) 2877 throw new Error("Attempt to auto-create ContractOfferComponent.text"); 2878 else if (Configuration.doAutoCreate()) 2879 this.text = new StringType(); // bb 2880 return this.text; 2881 } 2882 2883 public boolean hasTextElement() { 2884 return this.text != null && !this.text.isEmpty(); 2885 } 2886 2887 public boolean hasText() { 2888 return this.text != null && !this.text.isEmpty(); 2889 } 2890 2891 /** 2892 * @param value {@link #text} (Human readable form of this Contract Offer.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 2893 */ 2894 public ContractOfferComponent setTextElement(StringType value) { 2895 this.text = value; 2896 return this; 2897 } 2898 2899 /** 2900 * @return Human readable form of this Contract Offer. 2901 */ 2902 public String getText() { 2903 return this.text == null ? null : this.text.getValue(); 2904 } 2905 2906 /** 2907 * @param value Human readable form of this Contract Offer. 2908 */ 2909 public ContractOfferComponent setText(String value) { 2910 if (Utilities.noString(value)) 2911 this.text = null; 2912 else { 2913 if (this.text == null) 2914 this.text = new StringType(); 2915 this.text.setValue(value); 2916 } 2917 return this; 2918 } 2919 2920 /** 2921 * @return {@link #linkId} (The id of the clause or question text of the offer in the referenced questionnaire/response.) 2922 */ 2923 public List<StringType> getLinkId() { 2924 if (this.linkId == null) 2925 this.linkId = new ArrayList<StringType>(); 2926 return this.linkId; 2927 } 2928 2929 /** 2930 * @return Returns a reference to <code>this</code> for easy method chaining 2931 */ 2932 public ContractOfferComponent setLinkId(List<StringType> theLinkId) { 2933 this.linkId = theLinkId; 2934 return this; 2935 } 2936 2937 public boolean hasLinkId() { 2938 if (this.linkId == null) 2939 return false; 2940 for (StringType item : this.linkId) 2941 if (!item.isEmpty()) 2942 return true; 2943 return false; 2944 } 2945 2946 /** 2947 * @return {@link #linkId} (The id of the clause or question text of the offer in the referenced questionnaire/response.) 2948 */ 2949 public StringType addLinkIdElement() {//2 2950 StringType t = new StringType(); 2951 if (this.linkId == null) 2952 this.linkId = new ArrayList<StringType>(); 2953 this.linkId.add(t); 2954 return t; 2955 } 2956 2957 /** 2958 * @param value {@link #linkId} (The id of the clause or question text of the offer in the referenced questionnaire/response.) 2959 */ 2960 public ContractOfferComponent addLinkId(String value) { //1 2961 StringType t = new StringType(); 2962 t.setValue(value); 2963 if (this.linkId == null) 2964 this.linkId = new ArrayList<StringType>(); 2965 this.linkId.add(t); 2966 return this; 2967 } 2968 2969 /** 2970 * @param value {@link #linkId} (The id of the clause or question text of the offer in the referenced questionnaire/response.) 2971 */ 2972 public boolean hasLinkId(String value) { 2973 if (this.linkId == null) 2974 return false; 2975 for (StringType v : this.linkId) 2976 if (v.getValue().equals(value)) // string 2977 return true; 2978 return false; 2979 } 2980 2981 /** 2982 * @return {@link #securityLabelNumber} (Security labels that protects the offer.) 2983 */ 2984 public List<UnsignedIntType> getSecurityLabelNumber() { 2985 if (this.securityLabelNumber == null) 2986 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 2987 return this.securityLabelNumber; 2988 } 2989 2990 /** 2991 * @return Returns a reference to <code>this</code> for easy method chaining 2992 */ 2993 public ContractOfferComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 2994 this.securityLabelNumber = theSecurityLabelNumber; 2995 return this; 2996 } 2997 2998 public boolean hasSecurityLabelNumber() { 2999 if (this.securityLabelNumber == null) 3000 return false; 3001 for (UnsignedIntType item : this.securityLabelNumber) 3002 if (!item.isEmpty()) 3003 return true; 3004 return false; 3005 } 3006 3007 /** 3008 * @return {@link #securityLabelNumber} (Security labels that protects the offer.) 3009 */ 3010 public UnsignedIntType addSecurityLabelNumberElement() {//2 3011 UnsignedIntType t = new UnsignedIntType(); 3012 if (this.securityLabelNumber == null) 3013 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3014 this.securityLabelNumber.add(t); 3015 return t; 3016 } 3017 3018 /** 3019 * @param value {@link #securityLabelNumber} (Security labels that protects the offer.) 3020 */ 3021 public ContractOfferComponent addSecurityLabelNumber(int value) { //1 3022 UnsignedIntType t = new UnsignedIntType(); 3023 t.setValue(value); 3024 if (this.securityLabelNumber == null) 3025 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3026 this.securityLabelNumber.add(t); 3027 return this; 3028 } 3029 3030 /** 3031 * @param value {@link #securityLabelNumber} (Security labels that protects the offer.) 3032 */ 3033 public boolean hasSecurityLabelNumber(int value) { 3034 if (this.securityLabelNumber == null) 3035 return false; 3036 for (UnsignedIntType v : this.securityLabelNumber) 3037 if (v.getValue().equals(value)) // unsignedInt 3038 return true; 3039 return false; 3040 } 3041 3042 protected void listChildren(List<Property> children) { 3043 super.listChildren(children); 3044 children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3045 children.add(new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party)); 3046 children.add(new Property("topic", "Reference(Any)", "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).", 0, 1, topic)); 3047 children.add(new Property("type", "CodeableConcept", "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 0, 1, type)); 3048 children.add(new Property("decision", "CodeableConcept", "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1, decision)); 3049 children.add(new Property("decisionMode", "CodeableConcept", "How the decision about a Contract was conveyed.", 0, java.lang.Integer.MAX_VALUE, decisionMode)); 3050 children.add(new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE, answer)); 3051 children.add(new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text)); 3052 children.add(new Property("linkId", "string", "The id of the clause or question text of the offer in the referenced questionnaire/response.", 0, java.lang.Integer.MAX_VALUE, linkId)); 3053 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the offer.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber)); 3054 } 3055 3056 @Override 3057 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3058 switch (_hash) { 3059 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 0, java.lang.Integer.MAX_VALUE, identifier); 3060 case 106437350: /*party*/ return new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party); 3061 case 110546223: /*topic*/ return new Property("topic", "Reference(Any)", "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).", 0, 1, topic); 3062 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 0, 1, type); 3063 case 565719004: /*decision*/ return new Property("decision", "CodeableConcept", "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1, decision); 3064 case 675909535: /*decisionMode*/ return new Property("decisionMode", "CodeableConcept", "How the decision about a Contract was conveyed.", 0, java.lang.Integer.MAX_VALUE, decisionMode); 3065 case -1412808770: /*answer*/ return new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE, answer); 3066 case 3556653: /*text*/ return new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text); 3067 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The id of the clause or question text of the offer in the referenced questionnaire/response.", 0, java.lang.Integer.MAX_VALUE, linkId); 3068 case -149460995: /*securityLabelNumber*/ return new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the offer.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 3069 default: return super.getNamedProperty(_hash, _name, _checkValid); 3070 } 3071 3072 } 3073 3074 @Override 3075 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3076 switch (hash) { 3077 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3078 case 106437350: /*party*/ return this.party == null ? new Base[0] : this.party.toArray(new Base[this.party.size()]); // ContractPartyComponent 3079 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : new Base[] {this.topic}; // Reference 3080 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3081 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // CodeableConcept 3082 case 675909535: /*decisionMode*/ return this.decisionMode == null ? new Base[0] : this.decisionMode.toArray(new Base[this.decisionMode.size()]); // CodeableConcept 3083 case -1412808770: /*answer*/ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent 3084 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 3085 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 3086 case -149460995: /*securityLabelNumber*/ return this.securityLabelNumber == null ? new Base[0] : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 3087 default: return super.getProperty(hash, name, checkValid); 3088 } 3089 3090 } 3091 3092 @Override 3093 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3094 switch (hash) { 3095 case -1618432855: // identifier 3096 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3097 return value; 3098 case 106437350: // party 3099 this.getParty().add((ContractPartyComponent) value); // ContractPartyComponent 3100 return value; 3101 case 110546223: // topic 3102 this.topic = TypeConvertor.castToReference(value); // Reference 3103 return value; 3104 case 3575610: // type 3105 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3106 return value; 3107 case 565719004: // decision 3108 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3109 return value; 3110 case 675909535: // decisionMode 3111 this.getDecisionMode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3112 return value; 3113 case -1412808770: // answer 3114 this.getAnswer().add((AnswerComponent) value); // AnswerComponent 3115 return value; 3116 case 3556653: // text 3117 this.text = TypeConvertor.castToString(value); // StringType 3118 return value; 3119 case -1102667083: // linkId 3120 this.getLinkId().add(TypeConvertor.castToString(value)); // StringType 3121 return value; 3122 case -149460995: // securityLabelNumber 3123 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); // UnsignedIntType 3124 return value; 3125 default: return super.setProperty(hash, name, value); 3126 } 3127 3128 } 3129 3130 @Override 3131 public Base setProperty(String name, Base value) throws FHIRException { 3132 if (name.equals("identifier")) { 3133 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3134 } else if (name.equals("party")) { 3135 this.getParty().add((ContractPartyComponent) value); 3136 } else if (name.equals("topic")) { 3137 this.topic = TypeConvertor.castToReference(value); // Reference 3138 } else if (name.equals("type")) { 3139 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3140 } else if (name.equals("decision")) { 3141 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3142 } else if (name.equals("decisionMode")) { 3143 this.getDecisionMode().add(TypeConvertor.castToCodeableConcept(value)); 3144 } else if (name.equals("answer")) { 3145 this.getAnswer().add((AnswerComponent) value); 3146 } else if (name.equals("text")) { 3147 this.text = TypeConvertor.castToString(value); // StringType 3148 } else if (name.equals("linkId")) { 3149 this.getLinkId().add(TypeConvertor.castToString(value)); 3150 } else if (name.equals("securityLabelNumber")) { 3151 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); 3152 } else 3153 return super.setProperty(name, value); 3154 return value; 3155 } 3156 3157 @Override 3158 public void removeChild(String name, Base value) throws FHIRException { 3159 if (name.equals("identifier")) { 3160 this.getIdentifier().remove(value); 3161 } else if (name.equals("party")) { 3162 this.getParty().remove((ContractPartyComponent) value); 3163 } else if (name.equals("topic")) { 3164 this.topic = null; 3165 } else if (name.equals("type")) { 3166 this.type = null; 3167 } else if (name.equals("decision")) { 3168 this.decision = null; 3169 } else if (name.equals("decisionMode")) { 3170 this.getDecisionMode().remove(value); 3171 } else if (name.equals("answer")) { 3172 this.getAnswer().remove((AnswerComponent) value); 3173 } else if (name.equals("text")) { 3174 this.text = null; 3175 } else if (name.equals("linkId")) { 3176 this.getLinkId().remove(value); 3177 } else if (name.equals("securityLabelNumber")) { 3178 this.getSecurityLabelNumber().remove(value); 3179 } else 3180 super.removeChild(name, value); 3181 3182 } 3183 3184 @Override 3185 public Base makeProperty(int hash, String name) throws FHIRException { 3186 switch (hash) { 3187 case -1618432855: return addIdentifier(); 3188 case 106437350: return addParty(); 3189 case 110546223: return getTopic(); 3190 case 3575610: return getType(); 3191 case 565719004: return getDecision(); 3192 case 675909535: return addDecisionMode(); 3193 case -1412808770: return addAnswer(); 3194 case 3556653: return getTextElement(); 3195 case -1102667083: return addLinkIdElement(); 3196 case -149460995: return addSecurityLabelNumberElement(); 3197 default: return super.makeProperty(hash, name); 3198 } 3199 3200 } 3201 3202 @Override 3203 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3204 switch (hash) { 3205 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3206 case 106437350: /*party*/ return new String[] {}; 3207 case 110546223: /*topic*/ return new String[] {"Reference"}; 3208 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3209 case 565719004: /*decision*/ return new String[] {"CodeableConcept"}; 3210 case 675909535: /*decisionMode*/ return new String[] {"CodeableConcept"}; 3211 case -1412808770: /*answer*/ return new String[] {}; 3212 case 3556653: /*text*/ return new String[] {"string"}; 3213 case -1102667083: /*linkId*/ return new String[] {"string"}; 3214 case -149460995: /*securityLabelNumber*/ return new String[] {"unsignedInt"}; 3215 default: return super.getTypesForProperty(hash, name); 3216 } 3217 3218 } 3219 3220 @Override 3221 public Base addChild(String name) throws FHIRException { 3222 if (name.equals("identifier")) { 3223 return addIdentifier(); 3224 } 3225 else if (name.equals("party")) { 3226 return addParty(); 3227 } 3228 else if (name.equals("topic")) { 3229 this.topic = new Reference(); 3230 return this.topic; 3231 } 3232 else if (name.equals("type")) { 3233 this.type = new CodeableConcept(); 3234 return this.type; 3235 } 3236 else if (name.equals("decision")) { 3237 this.decision = new CodeableConcept(); 3238 return this.decision; 3239 } 3240 else if (name.equals("decisionMode")) { 3241 return addDecisionMode(); 3242 } 3243 else if (name.equals("answer")) { 3244 return addAnswer(); 3245 } 3246 else if (name.equals("text")) { 3247 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.offer.text"); 3248 } 3249 else if (name.equals("linkId")) { 3250 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.offer.linkId"); 3251 } 3252 else if (name.equals("securityLabelNumber")) { 3253 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.offer.securityLabelNumber"); 3254 } 3255 else 3256 return super.addChild(name); 3257 } 3258 3259 public ContractOfferComponent copy() { 3260 ContractOfferComponent dst = new ContractOfferComponent(); 3261 copyValues(dst); 3262 return dst; 3263 } 3264 3265 public void copyValues(ContractOfferComponent dst) { 3266 super.copyValues(dst); 3267 if (identifier != null) { 3268 dst.identifier = new ArrayList<Identifier>(); 3269 for (Identifier i : identifier) 3270 dst.identifier.add(i.copy()); 3271 }; 3272 if (party != null) { 3273 dst.party = new ArrayList<ContractPartyComponent>(); 3274 for (ContractPartyComponent i : party) 3275 dst.party.add(i.copy()); 3276 }; 3277 dst.topic = topic == null ? null : topic.copy(); 3278 dst.type = type == null ? null : type.copy(); 3279 dst.decision = decision == null ? null : decision.copy(); 3280 if (decisionMode != null) { 3281 dst.decisionMode = new ArrayList<CodeableConcept>(); 3282 for (CodeableConcept i : decisionMode) 3283 dst.decisionMode.add(i.copy()); 3284 }; 3285 if (answer != null) { 3286 dst.answer = new ArrayList<AnswerComponent>(); 3287 for (AnswerComponent i : answer) 3288 dst.answer.add(i.copy()); 3289 }; 3290 dst.text = text == null ? null : text.copy(); 3291 if (linkId != null) { 3292 dst.linkId = new ArrayList<StringType>(); 3293 for (StringType i : linkId) 3294 dst.linkId.add(i.copy()); 3295 }; 3296 if (securityLabelNumber != null) { 3297 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3298 for (UnsignedIntType i : securityLabelNumber) 3299 dst.securityLabelNumber.add(i.copy()); 3300 }; 3301 } 3302 3303 @Override 3304 public boolean equalsDeep(Base other_) { 3305 if (!super.equalsDeep(other_)) 3306 return false; 3307 if (!(other_ instanceof ContractOfferComponent)) 3308 return false; 3309 ContractOfferComponent o = (ContractOfferComponent) other_; 3310 return compareDeep(identifier, o.identifier, true) && compareDeep(party, o.party, true) && compareDeep(topic, o.topic, true) 3311 && compareDeep(type, o.type, true) && compareDeep(decision, o.decision, true) && compareDeep(decisionMode, o.decisionMode, true) 3312 && compareDeep(answer, o.answer, true) && compareDeep(text, o.text, true) && compareDeep(linkId, o.linkId, true) 3313 && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 3314 } 3315 3316 @Override 3317 public boolean equalsShallow(Base other_) { 3318 if (!super.equalsShallow(other_)) 3319 return false; 3320 if (!(other_ instanceof ContractOfferComponent)) 3321 return false; 3322 ContractOfferComponent o = (ContractOfferComponent) other_; 3323 return compareValues(text, o.text, true) && compareValues(linkId, o.linkId, true) && compareValues(securityLabelNumber, o.securityLabelNumber, true) 3324 ; 3325 } 3326 3327 public boolean isEmpty() { 3328 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, party, topic 3329 , type, decision, decisionMode, answer, text, linkId, securityLabelNumber); 3330 } 3331 3332 public String fhirType() { 3333 return "Contract.term.offer"; 3334 3335 } 3336 3337 } 3338 3339 @Block() 3340 public static class ContractPartyComponent extends BackboneElement implements IBaseBackboneElement { 3341 /** 3342 * Participant in the offer. 3343 */ 3344 @Child(name = "reference", type = {Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Device.class, Group.class, Organization.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3345 @Description(shortDefinition="Referenced entity", formalDefinition="Participant in the offer." ) 3346 protected List<Reference> reference; 3347 3348 /** 3349 * How the party participates in the offer. 3350 */ 3351 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 3352 @Description(shortDefinition="Participant engagement type", formalDefinition="How the party participates in the offer." ) 3353 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-party-role") 3354 protected CodeableConcept role; 3355 3356 private static final long serialVersionUID = -1599592477L; 3357 3358 /** 3359 * Constructor 3360 */ 3361 public ContractPartyComponent() { 3362 super(); 3363 } 3364 3365 /** 3366 * Constructor 3367 */ 3368 public ContractPartyComponent(Reference reference, CodeableConcept role) { 3369 super(); 3370 this.addReference(reference); 3371 this.setRole(role); 3372 } 3373 3374 /** 3375 * @return {@link #reference} (Participant in the offer.) 3376 */ 3377 public List<Reference> getReference() { 3378 if (this.reference == null) 3379 this.reference = new ArrayList<Reference>(); 3380 return this.reference; 3381 } 3382 3383 /** 3384 * @return Returns a reference to <code>this</code> for easy method chaining 3385 */ 3386 public ContractPartyComponent setReference(List<Reference> theReference) { 3387 this.reference = theReference; 3388 return this; 3389 } 3390 3391 public boolean hasReference() { 3392 if (this.reference == null) 3393 return false; 3394 for (Reference item : this.reference) 3395 if (!item.isEmpty()) 3396 return true; 3397 return false; 3398 } 3399 3400 public Reference addReference() { //3 3401 Reference t = new Reference(); 3402 if (this.reference == null) 3403 this.reference = new ArrayList<Reference>(); 3404 this.reference.add(t); 3405 return t; 3406 } 3407 3408 public ContractPartyComponent addReference(Reference t) { //3 3409 if (t == null) 3410 return this; 3411 if (this.reference == null) 3412 this.reference = new ArrayList<Reference>(); 3413 this.reference.add(t); 3414 return this; 3415 } 3416 3417 /** 3418 * @return The first repetition of repeating field {@link #reference}, creating it if it does not already exist {3} 3419 */ 3420 public Reference getReferenceFirstRep() { 3421 if (getReference().isEmpty()) { 3422 addReference(); 3423 } 3424 return getReference().get(0); 3425 } 3426 3427 /** 3428 * @return {@link #role} (How the party participates in the offer.) 3429 */ 3430 public CodeableConcept getRole() { 3431 if (this.role == null) 3432 if (Configuration.errorOnAutoCreate()) 3433 throw new Error("Attempt to auto-create ContractPartyComponent.role"); 3434 else if (Configuration.doAutoCreate()) 3435 this.role = new CodeableConcept(); // cc 3436 return this.role; 3437 } 3438 3439 public boolean hasRole() { 3440 return this.role != null && !this.role.isEmpty(); 3441 } 3442 3443 /** 3444 * @param value {@link #role} (How the party participates in the offer.) 3445 */ 3446 public ContractPartyComponent setRole(CodeableConcept value) { 3447 this.role = value; 3448 return this; 3449 } 3450 3451 protected void listChildren(List<Property> children) { 3452 super.listChildren(children); 3453 children.add(new Property("reference", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", "Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference)); 3454 children.add(new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1, role)); 3455 } 3456 3457 @Override 3458 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3459 switch (_hash) { 3460 case -925155509: /*reference*/ return new Property("reference", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", "Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference); 3461 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1, role); 3462 default: return super.getNamedProperty(_hash, _name, _checkValid); 3463 } 3464 3465 } 3466 3467 @Override 3468 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3469 switch (hash) { 3470 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // Reference 3471 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 3472 default: return super.getProperty(hash, name, checkValid); 3473 } 3474 3475 } 3476 3477 @Override 3478 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3479 switch (hash) { 3480 case -925155509: // reference 3481 this.getReference().add(TypeConvertor.castToReference(value)); // Reference 3482 return value; 3483 case 3506294: // role 3484 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3485 return value; 3486 default: return super.setProperty(hash, name, value); 3487 } 3488 3489 } 3490 3491 @Override 3492 public Base setProperty(String name, Base value) throws FHIRException { 3493 if (name.equals("reference")) { 3494 this.getReference().add(TypeConvertor.castToReference(value)); 3495 } else if (name.equals("role")) { 3496 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3497 } else 3498 return super.setProperty(name, value); 3499 return value; 3500 } 3501 3502 @Override 3503 public void removeChild(String name, Base value) throws FHIRException { 3504 if (name.equals("reference")) { 3505 this.getReference().remove(value); 3506 } else if (name.equals("role")) { 3507 this.role = null; 3508 } else 3509 super.removeChild(name, value); 3510 3511 } 3512 3513 @Override 3514 public Base makeProperty(int hash, String name) throws FHIRException { 3515 switch (hash) { 3516 case -925155509: return addReference(); 3517 case 3506294: return getRole(); 3518 default: return super.makeProperty(hash, name); 3519 } 3520 3521 } 3522 3523 @Override 3524 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3525 switch (hash) { 3526 case -925155509: /*reference*/ return new String[] {"Reference"}; 3527 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 3528 default: return super.getTypesForProperty(hash, name); 3529 } 3530 3531 } 3532 3533 @Override 3534 public Base addChild(String name) throws FHIRException { 3535 if (name.equals("reference")) { 3536 return addReference(); 3537 } 3538 else if (name.equals("role")) { 3539 this.role = new CodeableConcept(); 3540 return this.role; 3541 } 3542 else 3543 return super.addChild(name); 3544 } 3545 3546 public ContractPartyComponent copy() { 3547 ContractPartyComponent dst = new ContractPartyComponent(); 3548 copyValues(dst); 3549 return dst; 3550 } 3551 3552 public void copyValues(ContractPartyComponent dst) { 3553 super.copyValues(dst); 3554 if (reference != null) { 3555 dst.reference = new ArrayList<Reference>(); 3556 for (Reference i : reference) 3557 dst.reference.add(i.copy()); 3558 }; 3559 dst.role = role == null ? null : role.copy(); 3560 } 3561 3562 @Override 3563 public boolean equalsDeep(Base other_) { 3564 if (!super.equalsDeep(other_)) 3565 return false; 3566 if (!(other_ instanceof ContractPartyComponent)) 3567 return false; 3568 ContractPartyComponent o = (ContractPartyComponent) other_; 3569 return compareDeep(reference, o.reference, true) && compareDeep(role, o.role, true); 3570 } 3571 3572 @Override 3573 public boolean equalsShallow(Base other_) { 3574 if (!super.equalsShallow(other_)) 3575 return false; 3576 if (!(other_ instanceof ContractPartyComponent)) 3577 return false; 3578 ContractPartyComponent o = (ContractPartyComponent) other_; 3579 return true; 3580 } 3581 3582 public boolean isEmpty() { 3583 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, role); 3584 } 3585 3586 public String fhirType() { 3587 return "Contract.term.offer.party"; 3588 3589 } 3590 3591 } 3592 3593 @Block() 3594 public static class AnswerComponent extends BackboneElement implements IBaseBackboneElement { 3595 /** 3596 * Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research. 3597 */ 3598 @Child(name = "value", type = {BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, Quantity.class, Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 3599 @Description(shortDefinition="The actual answer response", formalDefinition="Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research." ) 3600 protected DataType value; 3601 3602 private static final long serialVersionUID = -1135414639L; 3603 3604 /** 3605 * Constructor 3606 */ 3607 public AnswerComponent() { 3608 super(); 3609 } 3610 3611 /** 3612 * Constructor 3613 */ 3614 public AnswerComponent(DataType value) { 3615 super(); 3616 this.setValue(value); 3617 } 3618 3619 /** 3620 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3621 */ 3622 public DataType getValue() { 3623 return this.value; 3624 } 3625 3626 /** 3627 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3628 */ 3629 public BooleanType getValueBooleanType() throws FHIRException { 3630 if (this.value == null) 3631 this.value = new BooleanType(); 3632 if (!(this.value instanceof BooleanType)) 3633 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 3634 return (BooleanType) this.value; 3635 } 3636 3637 public boolean hasValueBooleanType() { 3638 return this != null && this.value instanceof BooleanType; 3639 } 3640 3641 /** 3642 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3643 */ 3644 public DecimalType getValueDecimalType() throws FHIRException { 3645 if (this.value == null) 3646 this.value = new DecimalType(); 3647 if (!(this.value instanceof DecimalType)) 3648 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 3649 return (DecimalType) this.value; 3650 } 3651 3652 public boolean hasValueDecimalType() { 3653 return this != null && this.value instanceof DecimalType; 3654 } 3655 3656 /** 3657 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3658 */ 3659 public IntegerType getValueIntegerType() throws FHIRException { 3660 if (this.value == null) 3661 this.value = new IntegerType(); 3662 if (!(this.value instanceof IntegerType)) 3663 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 3664 return (IntegerType) this.value; 3665 } 3666 3667 public boolean hasValueIntegerType() { 3668 return this != null && this.value instanceof IntegerType; 3669 } 3670 3671 /** 3672 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3673 */ 3674 public DateType getValueDateType() throws FHIRException { 3675 if (this.value == null) 3676 this.value = new DateType(); 3677 if (!(this.value instanceof DateType)) 3678 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 3679 return (DateType) this.value; 3680 } 3681 3682 public boolean hasValueDateType() { 3683 return this != null && this.value instanceof DateType; 3684 } 3685 3686 /** 3687 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3688 */ 3689 public DateTimeType getValueDateTimeType() throws FHIRException { 3690 if (this.value == null) 3691 this.value = new DateTimeType(); 3692 if (!(this.value instanceof DateTimeType)) 3693 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3694 return (DateTimeType) this.value; 3695 } 3696 3697 public boolean hasValueDateTimeType() { 3698 return this != null && this.value instanceof DateTimeType; 3699 } 3700 3701 /** 3702 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3703 */ 3704 public TimeType getValueTimeType() throws FHIRException { 3705 if (this.value == null) 3706 this.value = new TimeType(); 3707 if (!(this.value instanceof TimeType)) 3708 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3709 return (TimeType) this.value; 3710 } 3711 3712 public boolean hasValueTimeType() { 3713 return this != null && this.value instanceof TimeType; 3714 } 3715 3716 /** 3717 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3718 */ 3719 public StringType getValueStringType() throws FHIRException { 3720 if (this.value == null) 3721 this.value = new StringType(); 3722 if (!(this.value instanceof StringType)) 3723 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 3724 return (StringType) this.value; 3725 } 3726 3727 public boolean hasValueStringType() { 3728 return this != null && this.value instanceof StringType; 3729 } 3730 3731 /** 3732 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3733 */ 3734 public UriType getValueUriType() throws FHIRException { 3735 if (this.value == null) 3736 this.value = new UriType(); 3737 if (!(this.value instanceof UriType)) 3738 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 3739 return (UriType) this.value; 3740 } 3741 3742 public boolean hasValueUriType() { 3743 return this != null && this.value instanceof UriType; 3744 } 3745 3746 /** 3747 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3748 */ 3749 public Attachment getValueAttachment() throws FHIRException { 3750 if (this.value == null) 3751 this.value = new Attachment(); 3752 if (!(this.value instanceof Attachment)) 3753 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 3754 return (Attachment) this.value; 3755 } 3756 3757 public boolean hasValueAttachment() { 3758 return this != null && this.value instanceof Attachment; 3759 } 3760 3761 /** 3762 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3763 */ 3764 public Coding getValueCoding() throws FHIRException { 3765 if (this.value == null) 3766 this.value = new Coding(); 3767 if (!(this.value instanceof Coding)) 3768 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 3769 return (Coding) this.value; 3770 } 3771 3772 public boolean hasValueCoding() { 3773 return this != null && this.value instanceof Coding; 3774 } 3775 3776 /** 3777 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3778 */ 3779 public Quantity getValueQuantity() throws FHIRException { 3780 if (this.value == null) 3781 this.value = new Quantity(); 3782 if (!(this.value instanceof Quantity)) 3783 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3784 return (Quantity) this.value; 3785 } 3786 3787 public boolean hasValueQuantity() { 3788 return this != null && this.value instanceof Quantity; 3789 } 3790 3791 /** 3792 * @return {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3793 */ 3794 public Reference getValueReference() throws FHIRException { 3795 if (this.value == null) 3796 this.value = new Reference(); 3797 if (!(this.value instanceof Reference)) 3798 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 3799 return (Reference) this.value; 3800 } 3801 3802 public boolean hasValueReference() { 3803 return this != null && this.value instanceof Reference; 3804 } 3805 3806 public boolean hasValue() { 3807 return this.value != null && !this.value.isEmpty(); 3808 } 3809 3810 /** 3811 * @param value {@link #value} (Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.) 3812 */ 3813 public AnswerComponent setValue(DataType value) { 3814 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType || value instanceof StringType || value instanceof UriType || value instanceof Attachment || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 3815 throw new FHIRException("Not the right type for Contract.term.offer.answer.value[x]: "+value.fhirType()); 3816 this.value = value; 3817 return this; 3818 } 3819 3820 protected void listChildren(List<Property> children) { 3821 super.listChildren(children); 3822 children.add(new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value)); 3823 } 3824 3825 @Override 3826 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3827 switch (_hash) { 3828 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3829 case 111972721: /*value*/ return new Property("value[x]", "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3830 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3831 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3832 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3833 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3834 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3835 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3836 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3837 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3838 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3839 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3840 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3841 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warranty duration, or whether biospecimen may be used for further research.", 0, 1, value); 3842 default: return super.getNamedProperty(_hash, _name, _checkValid); 3843 } 3844 3845 } 3846 3847 @Override 3848 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3849 switch (hash) { 3850 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3851 default: return super.getProperty(hash, name, checkValid); 3852 } 3853 3854 } 3855 3856 @Override 3857 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3858 switch (hash) { 3859 case 111972721: // value 3860 this.value = TypeConvertor.castToType(value); // DataType 3861 return value; 3862 default: return super.setProperty(hash, name, value); 3863 } 3864 3865 } 3866 3867 @Override 3868 public Base setProperty(String name, Base value) throws FHIRException { 3869 if (name.equals("value[x]")) { 3870 this.value = TypeConvertor.castToType(value); // DataType 3871 } else 3872 return super.setProperty(name, value); 3873 return value; 3874 } 3875 3876 @Override 3877 public void removeChild(String name, Base value) throws FHIRException { 3878 if (name.equals("value[x]")) { 3879 this.value = null; 3880 } else 3881 super.removeChild(name, value); 3882 3883 } 3884 3885 @Override 3886 public Base makeProperty(int hash, String name) throws FHIRException { 3887 switch (hash) { 3888 case -1410166417: return getValue(); 3889 case 111972721: return getValue(); 3890 default: return super.makeProperty(hash, name); 3891 } 3892 3893 } 3894 3895 @Override 3896 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3897 switch (hash) { 3898 case 111972721: /*value*/ return new String[] {"boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", "Attachment", "Coding", "Quantity", "Reference"}; 3899 default: return super.getTypesForProperty(hash, name); 3900 } 3901 3902 } 3903 3904 @Override 3905 public Base addChild(String name) throws FHIRException { 3906 if (name.equals("valueBoolean")) { 3907 this.value = new BooleanType(); 3908 return this.value; 3909 } 3910 else if (name.equals("valueDecimal")) { 3911 this.value = new DecimalType(); 3912 return this.value; 3913 } 3914 else if (name.equals("valueInteger")) { 3915 this.value = new IntegerType(); 3916 return this.value; 3917 } 3918 else if (name.equals("valueDate")) { 3919 this.value = new DateType(); 3920 return this.value; 3921 } 3922 else if (name.equals("valueDateTime")) { 3923 this.value = new DateTimeType(); 3924 return this.value; 3925 } 3926 else if (name.equals("valueTime")) { 3927 this.value = new TimeType(); 3928 return this.value; 3929 } 3930 else if (name.equals("valueString")) { 3931 this.value = new StringType(); 3932 return this.value; 3933 } 3934 else if (name.equals("valueUri")) { 3935 this.value = new UriType(); 3936 return this.value; 3937 } 3938 else if (name.equals("valueAttachment")) { 3939 this.value = new Attachment(); 3940 return this.value; 3941 } 3942 else if (name.equals("valueCoding")) { 3943 this.value = new Coding(); 3944 return this.value; 3945 } 3946 else if (name.equals("valueQuantity")) { 3947 this.value = new Quantity(); 3948 return this.value; 3949 } 3950 else if (name.equals("valueReference")) { 3951 this.value = new Reference(); 3952 return this.value; 3953 } 3954 else 3955 return super.addChild(name); 3956 } 3957 3958 public AnswerComponent copy() { 3959 AnswerComponent dst = new AnswerComponent(); 3960 copyValues(dst); 3961 return dst; 3962 } 3963 3964 public void copyValues(AnswerComponent dst) { 3965 super.copyValues(dst); 3966 dst.value = value == null ? null : value.copy(); 3967 } 3968 3969 @Override 3970 public boolean equalsDeep(Base other_) { 3971 if (!super.equalsDeep(other_)) 3972 return false; 3973 if (!(other_ instanceof AnswerComponent)) 3974 return false; 3975 AnswerComponent o = (AnswerComponent) other_; 3976 return compareDeep(value, o.value, true); 3977 } 3978 3979 @Override 3980 public boolean equalsShallow(Base other_) { 3981 if (!super.equalsShallow(other_)) 3982 return false; 3983 if (!(other_ instanceof AnswerComponent)) 3984 return false; 3985 AnswerComponent o = (AnswerComponent) other_; 3986 return true; 3987 } 3988 3989 public boolean isEmpty() { 3990 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 3991 } 3992 3993 public String fhirType() { 3994 return "Contract.term.offer.answer"; 3995 3996 } 3997 3998 } 3999 4000 @Block() 4001 public static class ContractAssetComponent extends BackboneElement implements IBaseBackboneElement { 4002 /** 4003 * Differentiates the kind of the asset . 4004 */ 4005 @Child(name = "scope", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 4006 @Description(shortDefinition="Range of asset", formalDefinition="Differentiates the kind of the asset ." ) 4007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-assetscope") 4008 protected CodeableConcept scope; 4009 4010 /** 4011 * Target entity type about which the term may be concerned. 4012 */ 4013 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4014 @Description(shortDefinition="Asset category", formalDefinition="Target entity type about which the term may be concerned." ) 4015 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-assettype") 4016 protected List<CodeableConcept> type; 4017 4018 /** 4019 * Associated entities. 4020 */ 4021 @Child(name = "typeReference", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4022 @Description(shortDefinition="Associated entities", formalDefinition="Associated entities." ) 4023 protected List<Reference> typeReference; 4024 4025 /** 4026 * May be a subtype or part of an offered asset. 4027 */ 4028 @Child(name = "subtype", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4029 @Description(shortDefinition="Asset sub-category", formalDefinition="May be a subtype or part of an offered asset." ) 4030 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-assetsubtype") 4031 protected List<CodeableConcept> subtype; 4032 4033 /** 4034 * Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree. 4035 */ 4036 @Child(name = "relationship", type = {Coding.class}, order=5, min=0, max=1, modifier=false, summary=false) 4037 @Description(shortDefinition="Kinship of the asset", formalDefinition="Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree." ) 4038 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/consent-content-class") 4039 protected Coding relationship; 4040 4041 /** 4042 * Circumstance of the asset. 4043 */ 4044 @Child(name = "context", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4045 @Description(shortDefinition="Circumstance of the asset", formalDefinition="Circumstance of the asset." ) 4046 protected List<AssetContextComponent> context; 4047 4048 /** 4049 * Description of the quality and completeness of the asset that may be a factor in its valuation. 4050 */ 4051 @Child(name = "condition", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 4052 @Description(shortDefinition="Quality desctiption of asset", formalDefinition="Description of the quality and completeness of the asset that may be a factor in its valuation." ) 4053 protected StringType condition; 4054 4055 /** 4056 * Type of Asset availability for use or ownership. 4057 */ 4058 @Child(name = "periodType", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4059 @Description(shortDefinition="Asset availability types", formalDefinition="Type of Asset availability for use or ownership." ) 4060 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/asset-availability") 4061 protected List<CodeableConcept> periodType; 4062 4063 /** 4064 * Asset relevant contractual time period. 4065 */ 4066 @Child(name = "period", type = {Period.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4067 @Description(shortDefinition="Time period of the asset", formalDefinition="Asset relevant contractual time period." ) 4068 protected List<Period> period; 4069 4070 /** 4071 * Time period of asset use. 4072 */ 4073 @Child(name = "usePeriod", type = {Period.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4074 @Description(shortDefinition="Time period", formalDefinition="Time period of asset use." ) 4075 protected List<Period> usePeriod; 4076 4077 /** 4078 * Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract. 4079 */ 4080 @Child(name = "text", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 4081 @Description(shortDefinition="Asset clause or question text", formalDefinition="Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract." ) 4082 protected StringType text; 4083 4084 /** 4085 * Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse. 4086 */ 4087 @Child(name = "linkId", type = {StringType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4088 @Description(shortDefinition="Pointer to asset text", formalDefinition="Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse." ) 4089 protected List<StringType> linkId; 4090 4091 /** 4092 * Response to assets. 4093 */ 4094 @Child(name = "answer", type = {AnswerComponent.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4095 @Description(shortDefinition="Response to assets", formalDefinition="Response to assets." ) 4096 protected List<AnswerComponent> answer; 4097 4098 /** 4099 * Security labels that protects the asset. 4100 */ 4101 @Child(name = "securityLabelNumber", type = {UnsignedIntType.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4102 @Description(shortDefinition="Asset restriction numbers", formalDefinition="Security labels that protects the asset." ) 4103 protected List<UnsignedIntType> securityLabelNumber; 4104 4105 /** 4106 * Contract Valued Item List. 4107 */ 4108 @Child(name = "valuedItem", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4109 @Description(shortDefinition="Contract Valued Item List", formalDefinition="Contract Valued Item List." ) 4110 protected List<ValuedItemComponent> valuedItem; 4111 4112 private static final long serialVersionUID = -581986730L; 4113 4114 /** 4115 * Constructor 4116 */ 4117 public ContractAssetComponent() { 4118 super(); 4119 } 4120 4121 /** 4122 * @return {@link #scope} (Differentiates the kind of the asset .) 4123 */ 4124 public CodeableConcept getScope() { 4125 if (this.scope == null) 4126 if (Configuration.errorOnAutoCreate()) 4127 throw new Error("Attempt to auto-create ContractAssetComponent.scope"); 4128 else if (Configuration.doAutoCreate()) 4129 this.scope = new CodeableConcept(); // cc 4130 return this.scope; 4131 } 4132 4133 public boolean hasScope() { 4134 return this.scope != null && !this.scope.isEmpty(); 4135 } 4136 4137 /** 4138 * @param value {@link #scope} (Differentiates the kind of the asset .) 4139 */ 4140 public ContractAssetComponent setScope(CodeableConcept value) { 4141 this.scope = value; 4142 return this; 4143 } 4144 4145 /** 4146 * @return {@link #type} (Target entity type about which the term may be concerned.) 4147 */ 4148 public List<CodeableConcept> getType() { 4149 if (this.type == null) 4150 this.type = new ArrayList<CodeableConcept>(); 4151 return this.type; 4152 } 4153 4154 /** 4155 * @return Returns a reference to <code>this</code> for easy method chaining 4156 */ 4157 public ContractAssetComponent setType(List<CodeableConcept> theType) { 4158 this.type = theType; 4159 return this; 4160 } 4161 4162 public boolean hasType() { 4163 if (this.type == null) 4164 return false; 4165 for (CodeableConcept item : this.type) 4166 if (!item.isEmpty()) 4167 return true; 4168 return false; 4169 } 4170 4171 public CodeableConcept addType() { //3 4172 CodeableConcept t = new CodeableConcept(); 4173 if (this.type == null) 4174 this.type = new ArrayList<CodeableConcept>(); 4175 this.type.add(t); 4176 return t; 4177 } 4178 4179 public ContractAssetComponent addType(CodeableConcept t) { //3 4180 if (t == null) 4181 return this; 4182 if (this.type == null) 4183 this.type = new ArrayList<CodeableConcept>(); 4184 this.type.add(t); 4185 return this; 4186 } 4187 4188 /** 4189 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 4190 */ 4191 public CodeableConcept getTypeFirstRep() { 4192 if (getType().isEmpty()) { 4193 addType(); 4194 } 4195 return getType().get(0); 4196 } 4197 4198 /** 4199 * @return {@link #typeReference} (Associated entities.) 4200 */ 4201 public List<Reference> getTypeReference() { 4202 if (this.typeReference == null) 4203 this.typeReference = new ArrayList<Reference>(); 4204 return this.typeReference; 4205 } 4206 4207 /** 4208 * @return Returns a reference to <code>this</code> for easy method chaining 4209 */ 4210 public ContractAssetComponent setTypeReference(List<Reference> theTypeReference) { 4211 this.typeReference = theTypeReference; 4212 return this; 4213 } 4214 4215 public boolean hasTypeReference() { 4216 if (this.typeReference == null) 4217 return false; 4218 for (Reference item : this.typeReference) 4219 if (!item.isEmpty()) 4220 return true; 4221 return false; 4222 } 4223 4224 public Reference addTypeReference() { //3 4225 Reference t = new Reference(); 4226 if (this.typeReference == null) 4227 this.typeReference = new ArrayList<Reference>(); 4228 this.typeReference.add(t); 4229 return t; 4230 } 4231 4232 public ContractAssetComponent addTypeReference(Reference t) { //3 4233 if (t == null) 4234 return this; 4235 if (this.typeReference == null) 4236 this.typeReference = new ArrayList<Reference>(); 4237 this.typeReference.add(t); 4238 return this; 4239 } 4240 4241 /** 4242 * @return The first repetition of repeating field {@link #typeReference}, creating it if it does not already exist {3} 4243 */ 4244 public Reference getTypeReferenceFirstRep() { 4245 if (getTypeReference().isEmpty()) { 4246 addTypeReference(); 4247 } 4248 return getTypeReference().get(0); 4249 } 4250 4251 /** 4252 * @return {@link #subtype} (May be a subtype or part of an offered asset.) 4253 */ 4254 public List<CodeableConcept> getSubtype() { 4255 if (this.subtype == null) 4256 this.subtype = new ArrayList<CodeableConcept>(); 4257 return this.subtype; 4258 } 4259 4260 /** 4261 * @return Returns a reference to <code>this</code> for easy method chaining 4262 */ 4263 public ContractAssetComponent setSubtype(List<CodeableConcept> theSubtype) { 4264 this.subtype = theSubtype; 4265 return this; 4266 } 4267 4268 public boolean hasSubtype() { 4269 if (this.subtype == null) 4270 return false; 4271 for (CodeableConcept item : this.subtype) 4272 if (!item.isEmpty()) 4273 return true; 4274 return false; 4275 } 4276 4277 public CodeableConcept addSubtype() { //3 4278 CodeableConcept t = new CodeableConcept(); 4279 if (this.subtype == null) 4280 this.subtype = new ArrayList<CodeableConcept>(); 4281 this.subtype.add(t); 4282 return t; 4283 } 4284 4285 public ContractAssetComponent addSubtype(CodeableConcept t) { //3 4286 if (t == null) 4287 return this; 4288 if (this.subtype == null) 4289 this.subtype = new ArrayList<CodeableConcept>(); 4290 this.subtype.add(t); 4291 return this; 4292 } 4293 4294 /** 4295 * @return The first repetition of repeating field {@link #subtype}, creating it if it does not already exist {3} 4296 */ 4297 public CodeableConcept getSubtypeFirstRep() { 4298 if (getSubtype().isEmpty()) { 4299 addSubtype(); 4300 } 4301 return getSubtype().get(0); 4302 } 4303 4304 /** 4305 * @return {@link #relationship} (Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.) 4306 */ 4307 public Coding getRelationship() { 4308 if (this.relationship == null) 4309 if (Configuration.errorOnAutoCreate()) 4310 throw new Error("Attempt to auto-create ContractAssetComponent.relationship"); 4311 else if (Configuration.doAutoCreate()) 4312 this.relationship = new Coding(); // cc 4313 return this.relationship; 4314 } 4315 4316 public boolean hasRelationship() { 4317 return this.relationship != null && !this.relationship.isEmpty(); 4318 } 4319 4320 /** 4321 * @param value {@link #relationship} (Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.) 4322 */ 4323 public ContractAssetComponent setRelationship(Coding value) { 4324 this.relationship = value; 4325 return this; 4326 } 4327 4328 /** 4329 * @return {@link #context} (Circumstance of the asset.) 4330 */ 4331 public List<AssetContextComponent> getContext() { 4332 if (this.context == null) 4333 this.context = new ArrayList<AssetContextComponent>(); 4334 return this.context; 4335 } 4336 4337 /** 4338 * @return Returns a reference to <code>this</code> for easy method chaining 4339 */ 4340 public ContractAssetComponent setContext(List<AssetContextComponent> theContext) { 4341 this.context = theContext; 4342 return this; 4343 } 4344 4345 public boolean hasContext() { 4346 if (this.context == null) 4347 return false; 4348 for (AssetContextComponent item : this.context) 4349 if (!item.isEmpty()) 4350 return true; 4351 return false; 4352 } 4353 4354 public AssetContextComponent addContext() { //3 4355 AssetContextComponent t = new AssetContextComponent(); 4356 if (this.context == null) 4357 this.context = new ArrayList<AssetContextComponent>(); 4358 this.context.add(t); 4359 return t; 4360 } 4361 4362 public ContractAssetComponent addContext(AssetContextComponent t) { //3 4363 if (t == null) 4364 return this; 4365 if (this.context == null) 4366 this.context = new ArrayList<AssetContextComponent>(); 4367 this.context.add(t); 4368 return this; 4369 } 4370 4371 /** 4372 * @return The first repetition of repeating field {@link #context}, creating it if it does not already exist {3} 4373 */ 4374 public AssetContextComponent getContextFirstRep() { 4375 if (getContext().isEmpty()) { 4376 addContext(); 4377 } 4378 return getContext().get(0); 4379 } 4380 4381 /** 4382 * @return {@link #condition} (Description of the quality and completeness of the asset that may be a factor in its valuation.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 4383 */ 4384 public StringType getConditionElement() { 4385 if (this.condition == null) 4386 if (Configuration.errorOnAutoCreate()) 4387 throw new Error("Attempt to auto-create ContractAssetComponent.condition"); 4388 else if (Configuration.doAutoCreate()) 4389 this.condition = new StringType(); // bb 4390 return this.condition; 4391 } 4392 4393 public boolean hasConditionElement() { 4394 return this.condition != null && !this.condition.isEmpty(); 4395 } 4396 4397 public boolean hasCondition() { 4398 return this.condition != null && !this.condition.isEmpty(); 4399 } 4400 4401 /** 4402 * @param value {@link #condition} (Description of the quality and completeness of the asset that may be a factor in its valuation.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 4403 */ 4404 public ContractAssetComponent setConditionElement(StringType value) { 4405 this.condition = value; 4406 return this; 4407 } 4408 4409 /** 4410 * @return Description of the quality and completeness of the asset that may be a factor in its valuation. 4411 */ 4412 public String getCondition() { 4413 return this.condition == null ? null : this.condition.getValue(); 4414 } 4415 4416 /** 4417 * @param value Description of the quality and completeness of the asset that may be a factor in its valuation. 4418 */ 4419 public ContractAssetComponent setCondition(String value) { 4420 if (Utilities.noString(value)) 4421 this.condition = null; 4422 else { 4423 if (this.condition == null) 4424 this.condition = new StringType(); 4425 this.condition.setValue(value); 4426 } 4427 return this; 4428 } 4429 4430 /** 4431 * @return {@link #periodType} (Type of Asset availability for use or ownership.) 4432 */ 4433 public List<CodeableConcept> getPeriodType() { 4434 if (this.periodType == null) 4435 this.periodType = new ArrayList<CodeableConcept>(); 4436 return this.periodType; 4437 } 4438 4439 /** 4440 * @return Returns a reference to <code>this</code> for easy method chaining 4441 */ 4442 public ContractAssetComponent setPeriodType(List<CodeableConcept> thePeriodType) { 4443 this.periodType = thePeriodType; 4444 return this; 4445 } 4446 4447 public boolean hasPeriodType() { 4448 if (this.periodType == null) 4449 return false; 4450 for (CodeableConcept item : this.periodType) 4451 if (!item.isEmpty()) 4452 return true; 4453 return false; 4454 } 4455 4456 public CodeableConcept addPeriodType() { //3 4457 CodeableConcept t = new CodeableConcept(); 4458 if (this.periodType == null) 4459 this.periodType = new ArrayList<CodeableConcept>(); 4460 this.periodType.add(t); 4461 return t; 4462 } 4463 4464 public ContractAssetComponent addPeriodType(CodeableConcept t) { //3 4465 if (t == null) 4466 return this; 4467 if (this.periodType == null) 4468 this.periodType = new ArrayList<CodeableConcept>(); 4469 this.periodType.add(t); 4470 return this; 4471 } 4472 4473 /** 4474 * @return The first repetition of repeating field {@link #periodType}, creating it if it does not already exist {3} 4475 */ 4476 public CodeableConcept getPeriodTypeFirstRep() { 4477 if (getPeriodType().isEmpty()) { 4478 addPeriodType(); 4479 } 4480 return getPeriodType().get(0); 4481 } 4482 4483 /** 4484 * @return {@link #period} (Asset relevant contractual time period.) 4485 */ 4486 public List<Period> getPeriod() { 4487 if (this.period == null) 4488 this.period = new ArrayList<Period>(); 4489 return this.period; 4490 } 4491 4492 /** 4493 * @return Returns a reference to <code>this</code> for easy method chaining 4494 */ 4495 public ContractAssetComponent setPeriod(List<Period> thePeriod) { 4496 this.period = thePeriod; 4497 return this; 4498 } 4499 4500 public boolean hasPeriod() { 4501 if (this.period == null) 4502 return false; 4503 for (Period item : this.period) 4504 if (!item.isEmpty()) 4505 return true; 4506 return false; 4507 } 4508 4509 public Period addPeriod() { //3 4510 Period t = new Period(); 4511 if (this.period == null) 4512 this.period = new ArrayList<Period>(); 4513 this.period.add(t); 4514 return t; 4515 } 4516 4517 public ContractAssetComponent addPeriod(Period t) { //3 4518 if (t == null) 4519 return this; 4520 if (this.period == null) 4521 this.period = new ArrayList<Period>(); 4522 this.period.add(t); 4523 return this; 4524 } 4525 4526 /** 4527 * @return The first repetition of repeating field {@link #period}, creating it if it does not already exist {3} 4528 */ 4529 public Period getPeriodFirstRep() { 4530 if (getPeriod().isEmpty()) { 4531 addPeriod(); 4532 } 4533 return getPeriod().get(0); 4534 } 4535 4536 /** 4537 * @return {@link #usePeriod} (Time period of asset use.) 4538 */ 4539 public List<Period> getUsePeriod() { 4540 if (this.usePeriod == null) 4541 this.usePeriod = new ArrayList<Period>(); 4542 return this.usePeriod; 4543 } 4544 4545 /** 4546 * @return Returns a reference to <code>this</code> for easy method chaining 4547 */ 4548 public ContractAssetComponent setUsePeriod(List<Period> theUsePeriod) { 4549 this.usePeriod = theUsePeriod; 4550 return this; 4551 } 4552 4553 public boolean hasUsePeriod() { 4554 if (this.usePeriod == null) 4555 return false; 4556 for (Period item : this.usePeriod) 4557 if (!item.isEmpty()) 4558 return true; 4559 return false; 4560 } 4561 4562 public Period addUsePeriod() { //3 4563 Period t = new Period(); 4564 if (this.usePeriod == null) 4565 this.usePeriod = new ArrayList<Period>(); 4566 this.usePeriod.add(t); 4567 return t; 4568 } 4569 4570 public ContractAssetComponent addUsePeriod(Period t) { //3 4571 if (t == null) 4572 return this; 4573 if (this.usePeriod == null) 4574 this.usePeriod = new ArrayList<Period>(); 4575 this.usePeriod.add(t); 4576 return this; 4577 } 4578 4579 /** 4580 * @return The first repetition of repeating field {@link #usePeriod}, creating it if it does not already exist {3} 4581 */ 4582 public Period getUsePeriodFirstRep() { 4583 if (getUsePeriod().isEmpty()) { 4584 addUsePeriod(); 4585 } 4586 return getUsePeriod().get(0); 4587 } 4588 4589 /** 4590 * @return {@link #text} (Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 4591 */ 4592 public StringType getTextElement() { 4593 if (this.text == null) 4594 if (Configuration.errorOnAutoCreate()) 4595 throw new Error("Attempt to auto-create ContractAssetComponent.text"); 4596 else if (Configuration.doAutoCreate()) 4597 this.text = new StringType(); // bb 4598 return this.text; 4599 } 4600 4601 public boolean hasTextElement() { 4602 return this.text != null && !this.text.isEmpty(); 4603 } 4604 4605 public boolean hasText() { 4606 return this.text != null && !this.text.isEmpty(); 4607 } 4608 4609 /** 4610 * @param value {@link #text} (Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 4611 */ 4612 public ContractAssetComponent setTextElement(StringType value) { 4613 this.text = value; 4614 return this; 4615 } 4616 4617 /** 4618 * @return Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract. 4619 */ 4620 public String getText() { 4621 return this.text == null ? null : this.text.getValue(); 4622 } 4623 4624 /** 4625 * @param value Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract. 4626 */ 4627 public ContractAssetComponent setText(String value) { 4628 if (Utilities.noString(value)) 4629 this.text = null; 4630 else { 4631 if (this.text == null) 4632 this.text = new StringType(); 4633 this.text.setValue(value); 4634 } 4635 return this; 4636 } 4637 4638 /** 4639 * @return {@link #linkId} (Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.) 4640 */ 4641 public List<StringType> getLinkId() { 4642 if (this.linkId == null) 4643 this.linkId = new ArrayList<StringType>(); 4644 return this.linkId; 4645 } 4646 4647 /** 4648 * @return Returns a reference to <code>this</code> for easy method chaining 4649 */ 4650 public ContractAssetComponent setLinkId(List<StringType> theLinkId) { 4651 this.linkId = theLinkId; 4652 return this; 4653 } 4654 4655 public boolean hasLinkId() { 4656 if (this.linkId == null) 4657 return false; 4658 for (StringType item : this.linkId) 4659 if (!item.isEmpty()) 4660 return true; 4661 return false; 4662 } 4663 4664 /** 4665 * @return {@link #linkId} (Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.) 4666 */ 4667 public StringType addLinkIdElement() {//2 4668 StringType t = new StringType(); 4669 if (this.linkId == null) 4670 this.linkId = new ArrayList<StringType>(); 4671 this.linkId.add(t); 4672 return t; 4673 } 4674 4675 /** 4676 * @param value {@link #linkId} (Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.) 4677 */ 4678 public ContractAssetComponent addLinkId(String value) { //1 4679 StringType t = new StringType(); 4680 t.setValue(value); 4681 if (this.linkId == null) 4682 this.linkId = new ArrayList<StringType>(); 4683 this.linkId.add(t); 4684 return this; 4685 } 4686 4687 /** 4688 * @param value {@link #linkId} (Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.) 4689 */ 4690 public boolean hasLinkId(String value) { 4691 if (this.linkId == null) 4692 return false; 4693 for (StringType v : this.linkId) 4694 if (v.getValue().equals(value)) // string 4695 return true; 4696 return false; 4697 } 4698 4699 /** 4700 * @return {@link #answer} (Response to assets.) 4701 */ 4702 public List<AnswerComponent> getAnswer() { 4703 if (this.answer == null) 4704 this.answer = new ArrayList<AnswerComponent>(); 4705 return this.answer; 4706 } 4707 4708 /** 4709 * @return Returns a reference to <code>this</code> for easy method chaining 4710 */ 4711 public ContractAssetComponent setAnswer(List<AnswerComponent> theAnswer) { 4712 this.answer = theAnswer; 4713 return this; 4714 } 4715 4716 public boolean hasAnswer() { 4717 if (this.answer == null) 4718 return false; 4719 for (AnswerComponent item : this.answer) 4720 if (!item.isEmpty()) 4721 return true; 4722 return false; 4723 } 4724 4725 public AnswerComponent addAnswer() { //3 4726 AnswerComponent t = new AnswerComponent(); 4727 if (this.answer == null) 4728 this.answer = new ArrayList<AnswerComponent>(); 4729 this.answer.add(t); 4730 return t; 4731 } 4732 4733 public ContractAssetComponent addAnswer(AnswerComponent t) { //3 4734 if (t == null) 4735 return this; 4736 if (this.answer == null) 4737 this.answer = new ArrayList<AnswerComponent>(); 4738 this.answer.add(t); 4739 return this; 4740 } 4741 4742 /** 4743 * @return The first repetition of repeating field {@link #answer}, creating it if it does not already exist {3} 4744 */ 4745 public AnswerComponent getAnswerFirstRep() { 4746 if (getAnswer().isEmpty()) { 4747 addAnswer(); 4748 } 4749 return getAnswer().get(0); 4750 } 4751 4752 /** 4753 * @return {@link #securityLabelNumber} (Security labels that protects the asset.) 4754 */ 4755 public List<UnsignedIntType> getSecurityLabelNumber() { 4756 if (this.securityLabelNumber == null) 4757 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 4758 return this.securityLabelNumber; 4759 } 4760 4761 /** 4762 * @return Returns a reference to <code>this</code> for easy method chaining 4763 */ 4764 public ContractAssetComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 4765 this.securityLabelNumber = theSecurityLabelNumber; 4766 return this; 4767 } 4768 4769 public boolean hasSecurityLabelNumber() { 4770 if (this.securityLabelNumber == null) 4771 return false; 4772 for (UnsignedIntType item : this.securityLabelNumber) 4773 if (!item.isEmpty()) 4774 return true; 4775 return false; 4776 } 4777 4778 /** 4779 * @return {@link #securityLabelNumber} (Security labels that protects the asset.) 4780 */ 4781 public UnsignedIntType addSecurityLabelNumberElement() {//2 4782 UnsignedIntType t = new UnsignedIntType(); 4783 if (this.securityLabelNumber == null) 4784 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 4785 this.securityLabelNumber.add(t); 4786 return t; 4787 } 4788 4789 /** 4790 * @param value {@link #securityLabelNumber} (Security labels that protects the asset.) 4791 */ 4792 public ContractAssetComponent addSecurityLabelNumber(int value) { //1 4793 UnsignedIntType t = new UnsignedIntType(); 4794 t.setValue(value); 4795 if (this.securityLabelNumber == null) 4796 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 4797 this.securityLabelNumber.add(t); 4798 return this; 4799 } 4800 4801 /** 4802 * @param value {@link #securityLabelNumber} (Security labels that protects the asset.) 4803 */ 4804 public boolean hasSecurityLabelNumber(int value) { 4805 if (this.securityLabelNumber == null) 4806 return false; 4807 for (UnsignedIntType v : this.securityLabelNumber) 4808 if (v.getValue().equals(value)) // unsignedInt 4809 return true; 4810 return false; 4811 } 4812 4813 /** 4814 * @return {@link #valuedItem} (Contract Valued Item List.) 4815 */ 4816 public List<ValuedItemComponent> getValuedItem() { 4817 if (this.valuedItem == null) 4818 this.valuedItem = new ArrayList<ValuedItemComponent>(); 4819 return this.valuedItem; 4820 } 4821 4822 /** 4823 * @return Returns a reference to <code>this</code> for easy method chaining 4824 */ 4825 public ContractAssetComponent setValuedItem(List<ValuedItemComponent> theValuedItem) { 4826 this.valuedItem = theValuedItem; 4827 return this; 4828 } 4829 4830 public boolean hasValuedItem() { 4831 if (this.valuedItem == null) 4832 return false; 4833 for (ValuedItemComponent item : this.valuedItem) 4834 if (!item.isEmpty()) 4835 return true; 4836 return false; 4837 } 4838 4839 public ValuedItemComponent addValuedItem() { //3 4840 ValuedItemComponent t = new ValuedItemComponent(); 4841 if (this.valuedItem == null) 4842 this.valuedItem = new ArrayList<ValuedItemComponent>(); 4843 this.valuedItem.add(t); 4844 return t; 4845 } 4846 4847 public ContractAssetComponent addValuedItem(ValuedItemComponent t) { //3 4848 if (t == null) 4849 return this; 4850 if (this.valuedItem == null) 4851 this.valuedItem = new ArrayList<ValuedItemComponent>(); 4852 this.valuedItem.add(t); 4853 return this; 4854 } 4855 4856 /** 4857 * @return The first repetition of repeating field {@link #valuedItem}, creating it if it does not already exist {3} 4858 */ 4859 public ValuedItemComponent getValuedItemFirstRep() { 4860 if (getValuedItem().isEmpty()) { 4861 addValuedItem(); 4862 } 4863 return getValuedItem().get(0); 4864 } 4865 4866 protected void listChildren(List<Property> children) { 4867 super.listChildren(children); 4868 children.add(new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1, scope)); 4869 children.add(new Property("type", "CodeableConcept", "Target entity type about which the term may be concerned.", 0, java.lang.Integer.MAX_VALUE, type)); 4870 children.add(new Property("typeReference", "Reference(Any)", "Associated entities.", 0, java.lang.Integer.MAX_VALUE, typeReference)); 4871 children.add(new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.", 0, java.lang.Integer.MAX_VALUE, subtype)); 4872 children.add(new Property("relationship", "Coding", "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.", 0, 1, relationship)); 4873 children.add(new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE, context)); 4874 children.add(new Property("condition", "string", "Description of the quality and completeness of the asset that may be a factor in its valuation.", 0, 1, condition)); 4875 children.add(new Property("periodType", "CodeableConcept", "Type of Asset availability for use or ownership.", 0, java.lang.Integer.MAX_VALUE, periodType)); 4876 children.add(new Property("period", "Period", "Asset relevant contractual time period.", 0, java.lang.Integer.MAX_VALUE, period)); 4877 children.add(new Property("usePeriod", "Period", "Time period of asset use.", 0, java.lang.Integer.MAX_VALUE, usePeriod)); 4878 children.add(new Property("text", "string", "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.", 0, 1, text)); 4879 children.add(new Property("linkId", "string", "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, linkId)); 4880 children.add(new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0, java.lang.Integer.MAX_VALUE, answer)); 4881 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the asset.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber)); 4882 children.add(new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem)); 4883 } 4884 4885 @Override 4886 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4887 switch (_hash) { 4888 case 109264468: /*scope*/ return new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1, scope); 4889 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Target entity type about which the term may be concerned.", 0, java.lang.Integer.MAX_VALUE, type); 4890 case 2074825009: /*typeReference*/ return new Property("typeReference", "Reference(Any)", "Associated entities.", 0, java.lang.Integer.MAX_VALUE, typeReference); 4891 case -1867567750: /*subtype*/ return new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.", 0, java.lang.Integer.MAX_VALUE, subtype); 4892 case -261851592: /*relationship*/ return new Property("relationship", "Coding", "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.", 0, 1, relationship); 4893 case 951530927: /*context*/ return new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE, context); 4894 case -861311717: /*condition*/ return new Property("condition", "string", "Description of the quality and completeness of the asset that may be a factor in its valuation.", 0, 1, condition); 4895 case 384348315: /*periodType*/ return new Property("periodType", "CodeableConcept", "Type of Asset availability for use or ownership.", 0, java.lang.Integer.MAX_VALUE, periodType); 4896 case -991726143: /*period*/ return new Property("period", "Period", "Asset relevant contractual time period.", 0, java.lang.Integer.MAX_VALUE, period); 4897 case -628382168: /*usePeriod*/ return new Property("usePeriod", "Period", "Time period of asset use.", 0, java.lang.Integer.MAX_VALUE, usePeriod); 4898 case 3556653: /*text*/ return new Property("text", "string", "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.", 0, 1, text); 4899 case -1102667083: /*linkId*/ return new Property("linkId", "string", "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, linkId); 4900 case -1412808770: /*answer*/ return new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0, java.lang.Integer.MAX_VALUE, answer); 4901 case -149460995: /*securityLabelNumber*/ return new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the asset.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 4902 case 2046675654: /*valuedItem*/ return new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem); 4903 default: return super.getNamedProperty(_hash, _name, _checkValid); 4904 } 4905 4906 } 4907 4908 @Override 4909 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4910 switch (hash) { 4911 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : new Base[] {this.scope}; // CodeableConcept 4912 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 4913 case 2074825009: /*typeReference*/ return this.typeReference == null ? new Base[0] : this.typeReference.toArray(new Base[this.typeReference.size()]); // Reference 4914 case -1867567750: /*subtype*/ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // CodeableConcept 4915 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Coding 4916 case 951530927: /*context*/ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // AssetContextComponent 4917 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // StringType 4918 case 384348315: /*periodType*/ return this.periodType == null ? new Base[0] : this.periodType.toArray(new Base[this.periodType.size()]); // CodeableConcept 4919 case -991726143: /*period*/ return this.period == null ? new Base[0] : this.period.toArray(new Base[this.period.size()]); // Period 4920 case -628382168: /*usePeriod*/ return this.usePeriod == null ? new Base[0] : this.usePeriod.toArray(new Base[this.usePeriod.size()]); // Period 4921 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 4922 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 4923 case -1412808770: /*answer*/ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent 4924 case -149460995: /*securityLabelNumber*/ return this.securityLabelNumber == null ? new Base[0] : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 4925 case 2046675654: /*valuedItem*/ return this.valuedItem == null ? new Base[0] : this.valuedItem.toArray(new Base[this.valuedItem.size()]); // ValuedItemComponent 4926 default: return super.getProperty(hash, name, checkValid); 4927 } 4928 4929 } 4930 4931 @Override 4932 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4933 switch (hash) { 4934 case 109264468: // scope 4935 this.scope = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4936 return value; 4937 case 3575610: // type 4938 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4939 return value; 4940 case 2074825009: // typeReference 4941 this.getTypeReference().add(TypeConvertor.castToReference(value)); // Reference 4942 return value; 4943 case -1867567750: // subtype 4944 this.getSubtype().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4945 return value; 4946 case -261851592: // relationship 4947 this.relationship = TypeConvertor.castToCoding(value); // Coding 4948 return value; 4949 case 951530927: // context 4950 this.getContext().add((AssetContextComponent) value); // AssetContextComponent 4951 return value; 4952 case -861311717: // condition 4953 this.condition = TypeConvertor.castToString(value); // StringType 4954 return value; 4955 case 384348315: // periodType 4956 this.getPeriodType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4957 return value; 4958 case -991726143: // period 4959 this.getPeriod().add(TypeConvertor.castToPeriod(value)); // Period 4960 return value; 4961 case -628382168: // usePeriod 4962 this.getUsePeriod().add(TypeConvertor.castToPeriod(value)); // Period 4963 return value; 4964 case 3556653: // text 4965 this.text = TypeConvertor.castToString(value); // StringType 4966 return value; 4967 case -1102667083: // linkId 4968 this.getLinkId().add(TypeConvertor.castToString(value)); // StringType 4969 return value; 4970 case -1412808770: // answer 4971 this.getAnswer().add((AnswerComponent) value); // AnswerComponent 4972 return value; 4973 case -149460995: // securityLabelNumber 4974 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); // UnsignedIntType 4975 return value; 4976 case 2046675654: // valuedItem 4977 this.getValuedItem().add((ValuedItemComponent) value); // ValuedItemComponent 4978 return value; 4979 default: return super.setProperty(hash, name, value); 4980 } 4981 4982 } 4983 4984 @Override 4985 public Base setProperty(String name, Base value) throws FHIRException { 4986 if (name.equals("scope")) { 4987 this.scope = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4988 } else if (name.equals("type")) { 4989 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 4990 } else if (name.equals("typeReference")) { 4991 this.getTypeReference().add(TypeConvertor.castToReference(value)); 4992 } else if (name.equals("subtype")) { 4993 this.getSubtype().add(TypeConvertor.castToCodeableConcept(value)); 4994 } else if (name.equals("relationship")) { 4995 this.relationship = TypeConvertor.castToCoding(value); // Coding 4996 } else if (name.equals("context")) { 4997 this.getContext().add((AssetContextComponent) value); 4998 } else if (name.equals("condition")) { 4999 this.condition = TypeConvertor.castToString(value); // StringType 5000 } else if (name.equals("periodType")) { 5001 this.getPeriodType().add(TypeConvertor.castToCodeableConcept(value)); 5002 } else if (name.equals("period")) { 5003 this.getPeriod().add(TypeConvertor.castToPeriod(value)); 5004 } else if (name.equals("usePeriod")) { 5005 this.getUsePeriod().add(TypeConvertor.castToPeriod(value)); 5006 } else if (name.equals("text")) { 5007 this.text = TypeConvertor.castToString(value); // StringType 5008 } else if (name.equals("linkId")) { 5009 this.getLinkId().add(TypeConvertor.castToString(value)); 5010 } else if (name.equals("answer")) { 5011 this.getAnswer().add((AnswerComponent) value); 5012 } else if (name.equals("securityLabelNumber")) { 5013 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); 5014 } else if (name.equals("valuedItem")) { 5015 this.getValuedItem().add((ValuedItemComponent) value); 5016 } else 5017 return super.setProperty(name, value); 5018 return value; 5019 } 5020 5021 @Override 5022 public void removeChild(String name, Base value) throws FHIRException { 5023 if (name.equals("scope")) { 5024 this.scope = null; 5025 } else if (name.equals("type")) { 5026 this.getType().remove(value); 5027 } else if (name.equals("typeReference")) { 5028 this.getTypeReference().remove(value); 5029 } else if (name.equals("subtype")) { 5030 this.getSubtype().remove(value); 5031 } else if (name.equals("relationship")) { 5032 this.relationship = null; 5033 } else if (name.equals("context")) { 5034 this.getContext().remove((AssetContextComponent) value); 5035 } else if (name.equals("condition")) { 5036 this.condition = null; 5037 } else if (name.equals("periodType")) { 5038 this.getPeriodType().remove(value); 5039 } else if (name.equals("period")) { 5040 this.getPeriod().remove(value); 5041 } else if (name.equals("usePeriod")) { 5042 this.getUsePeriod().remove(value); 5043 } else if (name.equals("text")) { 5044 this.text = null; 5045 } else if (name.equals("linkId")) { 5046 this.getLinkId().remove(value); 5047 } else if (name.equals("answer")) { 5048 this.getAnswer().remove((AnswerComponent) value); 5049 } else if (name.equals("securityLabelNumber")) { 5050 this.getSecurityLabelNumber().remove(value); 5051 } else if (name.equals("valuedItem")) { 5052 this.getValuedItem().remove((ValuedItemComponent) value); 5053 } else 5054 super.removeChild(name, value); 5055 5056 } 5057 5058 @Override 5059 public Base makeProperty(int hash, String name) throws FHIRException { 5060 switch (hash) { 5061 case 109264468: return getScope(); 5062 case 3575610: return addType(); 5063 case 2074825009: return addTypeReference(); 5064 case -1867567750: return addSubtype(); 5065 case -261851592: return getRelationship(); 5066 case 951530927: return addContext(); 5067 case -861311717: return getConditionElement(); 5068 case 384348315: return addPeriodType(); 5069 case -991726143: return addPeriod(); 5070 case -628382168: return addUsePeriod(); 5071 case 3556653: return getTextElement(); 5072 case -1102667083: return addLinkIdElement(); 5073 case -1412808770: return addAnswer(); 5074 case -149460995: return addSecurityLabelNumberElement(); 5075 case 2046675654: return addValuedItem(); 5076 default: return super.makeProperty(hash, name); 5077 } 5078 5079 } 5080 5081 @Override 5082 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5083 switch (hash) { 5084 case 109264468: /*scope*/ return new String[] {"CodeableConcept"}; 5085 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 5086 case 2074825009: /*typeReference*/ return new String[] {"Reference"}; 5087 case -1867567750: /*subtype*/ return new String[] {"CodeableConcept"}; 5088 case -261851592: /*relationship*/ return new String[] {"Coding"}; 5089 case 951530927: /*context*/ return new String[] {}; 5090 case -861311717: /*condition*/ return new String[] {"string"}; 5091 case 384348315: /*periodType*/ return new String[] {"CodeableConcept"}; 5092 case -991726143: /*period*/ return new String[] {"Period"}; 5093 case -628382168: /*usePeriod*/ return new String[] {"Period"}; 5094 case 3556653: /*text*/ return new String[] {"string"}; 5095 case -1102667083: /*linkId*/ return new String[] {"string"}; 5096 case -1412808770: /*answer*/ return new String[] {"@Contract.term.offer.answer"}; 5097 case -149460995: /*securityLabelNumber*/ return new String[] {"unsignedInt"}; 5098 case 2046675654: /*valuedItem*/ return new String[] {}; 5099 default: return super.getTypesForProperty(hash, name); 5100 } 5101 5102 } 5103 5104 @Override 5105 public Base addChild(String name) throws FHIRException { 5106 if (name.equals("scope")) { 5107 this.scope = new CodeableConcept(); 5108 return this.scope; 5109 } 5110 else if (name.equals("type")) { 5111 return addType(); 5112 } 5113 else if (name.equals("typeReference")) { 5114 return addTypeReference(); 5115 } 5116 else if (name.equals("subtype")) { 5117 return addSubtype(); 5118 } 5119 else if (name.equals("relationship")) { 5120 this.relationship = new Coding(); 5121 return this.relationship; 5122 } 5123 else if (name.equals("context")) { 5124 return addContext(); 5125 } 5126 else if (name.equals("condition")) { 5127 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.condition"); 5128 } 5129 else if (name.equals("periodType")) { 5130 return addPeriodType(); 5131 } 5132 else if (name.equals("period")) { 5133 return addPeriod(); 5134 } 5135 else if (name.equals("usePeriod")) { 5136 return addUsePeriod(); 5137 } 5138 else if (name.equals("text")) { 5139 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.text"); 5140 } 5141 else if (name.equals("linkId")) { 5142 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.linkId"); 5143 } 5144 else if (name.equals("answer")) { 5145 return addAnswer(); 5146 } 5147 else if (name.equals("securityLabelNumber")) { 5148 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.securityLabelNumber"); 5149 } 5150 else if (name.equals("valuedItem")) { 5151 return addValuedItem(); 5152 } 5153 else 5154 return super.addChild(name); 5155 } 5156 5157 public ContractAssetComponent copy() { 5158 ContractAssetComponent dst = new ContractAssetComponent(); 5159 copyValues(dst); 5160 return dst; 5161 } 5162 5163 public void copyValues(ContractAssetComponent dst) { 5164 super.copyValues(dst); 5165 dst.scope = scope == null ? null : scope.copy(); 5166 if (type != null) { 5167 dst.type = new ArrayList<CodeableConcept>(); 5168 for (CodeableConcept i : type) 5169 dst.type.add(i.copy()); 5170 }; 5171 if (typeReference != null) { 5172 dst.typeReference = new ArrayList<Reference>(); 5173 for (Reference i : typeReference) 5174 dst.typeReference.add(i.copy()); 5175 }; 5176 if (subtype != null) { 5177 dst.subtype = new ArrayList<CodeableConcept>(); 5178 for (CodeableConcept i : subtype) 5179 dst.subtype.add(i.copy()); 5180 }; 5181 dst.relationship = relationship == null ? null : relationship.copy(); 5182 if (context != null) { 5183 dst.context = new ArrayList<AssetContextComponent>(); 5184 for (AssetContextComponent i : context) 5185 dst.context.add(i.copy()); 5186 }; 5187 dst.condition = condition == null ? null : condition.copy(); 5188 if (periodType != null) { 5189 dst.periodType = new ArrayList<CodeableConcept>(); 5190 for (CodeableConcept i : periodType) 5191 dst.periodType.add(i.copy()); 5192 }; 5193 if (period != null) { 5194 dst.period = new ArrayList<Period>(); 5195 for (Period i : period) 5196 dst.period.add(i.copy()); 5197 }; 5198 if (usePeriod != null) { 5199 dst.usePeriod = new ArrayList<Period>(); 5200 for (Period i : usePeriod) 5201 dst.usePeriod.add(i.copy()); 5202 }; 5203 dst.text = text == null ? null : text.copy(); 5204 if (linkId != null) { 5205 dst.linkId = new ArrayList<StringType>(); 5206 for (StringType i : linkId) 5207 dst.linkId.add(i.copy()); 5208 }; 5209 if (answer != null) { 5210 dst.answer = new ArrayList<AnswerComponent>(); 5211 for (AnswerComponent i : answer) 5212 dst.answer.add(i.copy()); 5213 }; 5214 if (securityLabelNumber != null) { 5215 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 5216 for (UnsignedIntType i : securityLabelNumber) 5217 dst.securityLabelNumber.add(i.copy()); 5218 }; 5219 if (valuedItem != null) { 5220 dst.valuedItem = new ArrayList<ValuedItemComponent>(); 5221 for (ValuedItemComponent i : valuedItem) 5222 dst.valuedItem.add(i.copy()); 5223 }; 5224 } 5225 5226 @Override 5227 public boolean equalsDeep(Base other_) { 5228 if (!super.equalsDeep(other_)) 5229 return false; 5230 if (!(other_ instanceof ContractAssetComponent)) 5231 return false; 5232 ContractAssetComponent o = (ContractAssetComponent) other_; 5233 return compareDeep(scope, o.scope, true) && compareDeep(type, o.type, true) && compareDeep(typeReference, o.typeReference, true) 5234 && compareDeep(subtype, o.subtype, true) && compareDeep(relationship, o.relationship, true) && compareDeep(context, o.context, true) 5235 && compareDeep(condition, o.condition, true) && compareDeep(periodType, o.periodType, true) && compareDeep(period, o.period, true) 5236 && compareDeep(usePeriod, o.usePeriod, true) && compareDeep(text, o.text, true) && compareDeep(linkId, o.linkId, true) 5237 && compareDeep(answer, o.answer, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true) 5238 && compareDeep(valuedItem, o.valuedItem, true); 5239 } 5240 5241 @Override 5242 public boolean equalsShallow(Base other_) { 5243 if (!super.equalsShallow(other_)) 5244 return false; 5245 if (!(other_ instanceof ContractAssetComponent)) 5246 return false; 5247 ContractAssetComponent o = (ContractAssetComponent) other_; 5248 return compareValues(condition, o.condition, true) && compareValues(text, o.text, true) && compareValues(linkId, o.linkId, true) 5249 && compareValues(securityLabelNumber, o.securityLabelNumber, true); 5250 } 5251 5252 public boolean isEmpty() { 5253 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(scope, type, typeReference 5254 , subtype, relationship, context, condition, periodType, period, usePeriod, text 5255 , linkId, answer, securityLabelNumber, valuedItem); 5256 } 5257 5258 public String fhirType() { 5259 return "Contract.term.asset"; 5260 5261 } 5262 5263 } 5264 5265 @Block() 5266 public static class AssetContextComponent extends BackboneElement implements IBaseBackboneElement { 5267 /** 5268 * Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction. 5269 */ 5270 @Child(name = "reference", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 5271 @Description(shortDefinition="Creator,custodian or owner", formalDefinition="Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction." ) 5272 protected Reference reference; 5273 5274 /** 5275 * Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location. 5276 */ 5277 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5278 @Description(shortDefinition="Codeable asset context", formalDefinition="Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location." ) 5279 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-assetcontext") 5280 protected List<CodeableConcept> code; 5281 5282 /** 5283 * Context description. 5284 */ 5285 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5286 @Description(shortDefinition="Context description", formalDefinition="Context description." ) 5287 protected StringType text; 5288 5289 private static final long serialVersionUID = -388598648L; 5290 5291 /** 5292 * Constructor 5293 */ 5294 public AssetContextComponent() { 5295 super(); 5296 } 5297 5298 /** 5299 * @return {@link #reference} (Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.) 5300 */ 5301 public Reference getReference() { 5302 if (this.reference == null) 5303 if (Configuration.errorOnAutoCreate()) 5304 throw new Error("Attempt to auto-create AssetContextComponent.reference"); 5305 else if (Configuration.doAutoCreate()) 5306 this.reference = new Reference(); // cc 5307 return this.reference; 5308 } 5309 5310 public boolean hasReference() { 5311 return this.reference != null && !this.reference.isEmpty(); 5312 } 5313 5314 /** 5315 * @param value {@link #reference} (Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.) 5316 */ 5317 public AssetContextComponent setReference(Reference value) { 5318 this.reference = value; 5319 return this; 5320 } 5321 5322 /** 5323 * @return {@link #code} (Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.) 5324 */ 5325 public List<CodeableConcept> getCode() { 5326 if (this.code == null) 5327 this.code = new ArrayList<CodeableConcept>(); 5328 return this.code; 5329 } 5330 5331 /** 5332 * @return Returns a reference to <code>this</code> for easy method chaining 5333 */ 5334 public AssetContextComponent setCode(List<CodeableConcept> theCode) { 5335 this.code = theCode; 5336 return this; 5337 } 5338 5339 public boolean hasCode() { 5340 if (this.code == null) 5341 return false; 5342 for (CodeableConcept item : this.code) 5343 if (!item.isEmpty()) 5344 return true; 5345 return false; 5346 } 5347 5348 public CodeableConcept addCode() { //3 5349 CodeableConcept t = new CodeableConcept(); 5350 if (this.code == null) 5351 this.code = new ArrayList<CodeableConcept>(); 5352 this.code.add(t); 5353 return t; 5354 } 5355 5356 public AssetContextComponent addCode(CodeableConcept t) { //3 5357 if (t == null) 5358 return this; 5359 if (this.code == null) 5360 this.code = new ArrayList<CodeableConcept>(); 5361 this.code.add(t); 5362 return this; 5363 } 5364 5365 /** 5366 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 5367 */ 5368 public CodeableConcept getCodeFirstRep() { 5369 if (getCode().isEmpty()) { 5370 addCode(); 5371 } 5372 return getCode().get(0); 5373 } 5374 5375 /** 5376 * @return {@link #text} (Context description.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 5377 */ 5378 public StringType getTextElement() { 5379 if (this.text == null) 5380 if (Configuration.errorOnAutoCreate()) 5381 throw new Error("Attempt to auto-create AssetContextComponent.text"); 5382 else if (Configuration.doAutoCreate()) 5383 this.text = new StringType(); // bb 5384 return this.text; 5385 } 5386 5387 public boolean hasTextElement() { 5388 return this.text != null && !this.text.isEmpty(); 5389 } 5390 5391 public boolean hasText() { 5392 return this.text != null && !this.text.isEmpty(); 5393 } 5394 5395 /** 5396 * @param value {@link #text} (Context description.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 5397 */ 5398 public AssetContextComponent setTextElement(StringType value) { 5399 this.text = value; 5400 return this; 5401 } 5402 5403 /** 5404 * @return Context description. 5405 */ 5406 public String getText() { 5407 return this.text == null ? null : this.text.getValue(); 5408 } 5409 5410 /** 5411 * @param value Context description. 5412 */ 5413 public AssetContextComponent setText(String value) { 5414 if (Utilities.noString(value)) 5415 this.text = null; 5416 else { 5417 if (this.text == null) 5418 this.text = new StringType(); 5419 this.text.setValue(value); 5420 } 5421 return this; 5422 } 5423 5424 protected void listChildren(List<Property> children) { 5425 super.listChildren(children); 5426 children.add(new Property("reference", "Reference(Any)", "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.", 0, 1, reference)); 5427 children.add(new Property("code", "CodeableConcept", "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.", 0, java.lang.Integer.MAX_VALUE, code)); 5428 children.add(new Property("text", "string", "Context description.", 0, 1, text)); 5429 } 5430 5431 @Override 5432 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5433 switch (_hash) { 5434 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.", 0, 1, reference); 5435 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.", 0, java.lang.Integer.MAX_VALUE, code); 5436 case 3556653: /*text*/ return new Property("text", "string", "Context description.", 0, 1, text); 5437 default: return super.getNamedProperty(_hash, _name, _checkValid); 5438 } 5439 5440 } 5441 5442 @Override 5443 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5444 switch (hash) { 5445 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 5446 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 5447 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 5448 default: return super.getProperty(hash, name, checkValid); 5449 } 5450 5451 } 5452 5453 @Override 5454 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5455 switch (hash) { 5456 case -925155509: // reference 5457 this.reference = TypeConvertor.castToReference(value); // Reference 5458 return value; 5459 case 3059181: // code 5460 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5461 return value; 5462 case 3556653: // text 5463 this.text = TypeConvertor.castToString(value); // StringType 5464 return value; 5465 default: return super.setProperty(hash, name, value); 5466 } 5467 5468 } 5469 5470 @Override 5471 public Base setProperty(String name, Base value) throws FHIRException { 5472 if (name.equals("reference")) { 5473 this.reference = TypeConvertor.castToReference(value); // Reference 5474 } else if (name.equals("code")) { 5475 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 5476 } else if (name.equals("text")) { 5477 this.text = TypeConvertor.castToString(value); // StringType 5478 } else 5479 return super.setProperty(name, value); 5480 return value; 5481 } 5482 5483 @Override 5484 public void removeChild(String name, Base value) throws FHIRException { 5485 if (name.equals("reference")) { 5486 this.reference = null; 5487 } else if (name.equals("code")) { 5488 this.getCode().remove(value); 5489 } else if (name.equals("text")) { 5490 this.text = null; 5491 } else 5492 super.removeChild(name, value); 5493 5494 } 5495 5496 @Override 5497 public Base makeProperty(int hash, String name) throws FHIRException { 5498 switch (hash) { 5499 case -925155509: return getReference(); 5500 case 3059181: return addCode(); 5501 case 3556653: return getTextElement(); 5502 default: return super.makeProperty(hash, name); 5503 } 5504 5505 } 5506 5507 @Override 5508 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5509 switch (hash) { 5510 case -925155509: /*reference*/ return new String[] {"Reference"}; 5511 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 5512 case 3556653: /*text*/ return new String[] {"string"}; 5513 default: return super.getTypesForProperty(hash, name); 5514 } 5515 5516 } 5517 5518 @Override 5519 public Base addChild(String name) throws FHIRException { 5520 if (name.equals("reference")) { 5521 this.reference = new Reference(); 5522 return this.reference; 5523 } 5524 else if (name.equals("code")) { 5525 return addCode(); 5526 } 5527 else if (name.equals("text")) { 5528 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.context.text"); 5529 } 5530 else 5531 return super.addChild(name); 5532 } 5533 5534 public AssetContextComponent copy() { 5535 AssetContextComponent dst = new AssetContextComponent(); 5536 copyValues(dst); 5537 return dst; 5538 } 5539 5540 public void copyValues(AssetContextComponent dst) { 5541 super.copyValues(dst); 5542 dst.reference = reference == null ? null : reference.copy(); 5543 if (code != null) { 5544 dst.code = new ArrayList<CodeableConcept>(); 5545 for (CodeableConcept i : code) 5546 dst.code.add(i.copy()); 5547 }; 5548 dst.text = text == null ? null : text.copy(); 5549 } 5550 5551 @Override 5552 public boolean equalsDeep(Base other_) { 5553 if (!super.equalsDeep(other_)) 5554 return false; 5555 if (!(other_ instanceof AssetContextComponent)) 5556 return false; 5557 AssetContextComponent o = (AssetContextComponent) other_; 5558 return compareDeep(reference, o.reference, true) && compareDeep(code, o.code, true) && compareDeep(text, o.text, true) 5559 ; 5560 } 5561 5562 @Override 5563 public boolean equalsShallow(Base other_) { 5564 if (!super.equalsShallow(other_)) 5565 return false; 5566 if (!(other_ instanceof AssetContextComponent)) 5567 return false; 5568 AssetContextComponent o = (AssetContextComponent) other_; 5569 return compareValues(text, o.text, true); 5570 } 5571 5572 public boolean isEmpty() { 5573 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, code, text); 5574 } 5575 5576 public String fhirType() { 5577 return "Contract.term.asset.context"; 5578 5579 } 5580 5581 } 5582 5583 @Block() 5584 public static class ValuedItemComponent extends BackboneElement implements IBaseBackboneElement { 5585 /** 5586 * Specific type of Contract Valued Item that may be priced. 5587 */ 5588 @Child(name = "entity", type = {CodeableConcept.class, Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 5589 @Description(shortDefinition="Contract Valued Item Type", formalDefinition="Specific type of Contract Valued Item that may be priced." ) 5590 protected DataType entity; 5591 5592 /** 5593 * Identifies a Contract Valued Item instance. 5594 */ 5595 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 5596 @Description(shortDefinition="Contract Valued Item Number", formalDefinition="Identifies a Contract Valued Item instance." ) 5597 protected Identifier identifier; 5598 5599 /** 5600 * Indicates the time during which this Contract ValuedItem information is effective. 5601 */ 5602 @Child(name = "effectiveTime", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5603 @Description(shortDefinition="Contract Valued Item Effective Tiem", formalDefinition="Indicates the time during which this Contract ValuedItem information is effective." ) 5604 protected DateTimeType effectiveTime; 5605 5606 /** 5607 * Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances. 5608 */ 5609 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 5610 @Description(shortDefinition="Count of Contract Valued Items", formalDefinition="Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances." ) 5611 protected Quantity quantity; 5612 5613 /** 5614 * A Contract Valued Item unit valuation measure. 5615 */ 5616 @Child(name = "unitPrice", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 5617 @Description(shortDefinition="Contract Valued Item fee, charge, or cost", formalDefinition="A Contract Valued Item unit valuation measure." ) 5618 protected Money unitPrice; 5619 5620 /** 5621 * A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5622 */ 5623 @Child(name = "factor", type = {DecimalType.class}, order=6, min=0, max=1, modifier=false, summary=false) 5624 @Description(shortDefinition="Contract Valued Item Price Scaling Factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5625 protected DecimalType factor; 5626 5627 /** 5628 * An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 5629 */ 5630 @Child(name = "points", type = {DecimalType.class}, order=7, min=0, max=1, modifier=false, summary=false) 5631 @Description(shortDefinition="Contract Valued Item Difficulty Scaling Factor", formalDefinition="An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point." ) 5632 protected DecimalType points; 5633 5634 /** 5635 * Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 5636 */ 5637 @Child(name = "net", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 5638 @Description(shortDefinition="Total Contract Valued Item Value", formalDefinition="Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 5639 protected Money net; 5640 5641 /** 5642 * Terms of valuation. 5643 */ 5644 @Child(name = "payment", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5645 @Description(shortDefinition="Terms of valuation", formalDefinition="Terms of valuation." ) 5646 protected StringType payment; 5647 5648 /** 5649 * When payment is due. 5650 */ 5651 @Child(name = "paymentDate", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 5652 @Description(shortDefinition="When payment is due", formalDefinition="When payment is due." ) 5653 protected DateTimeType paymentDate; 5654 5655 /** 5656 * Who will make payment. 5657 */ 5658 @Child(name = "responsible", type = {Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=11, min=0, max=1, modifier=false, summary=false) 5659 @Description(shortDefinition="Who will make payment", formalDefinition="Who will make payment." ) 5660 protected Reference responsible; 5661 5662 /** 5663 * Who will receive payment. 5664 */ 5665 @Child(name = "recipient", type = {Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=12, min=0, max=1, modifier=false, summary=false) 5666 @Description(shortDefinition="Who will receive payment", formalDefinition="Who will receive payment." ) 5667 protected Reference recipient; 5668 5669 /** 5670 * Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse. 5671 */ 5672 @Child(name = "linkId", type = {StringType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5673 @Description(shortDefinition="Pointer to specific item", formalDefinition="Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse." ) 5674 protected List<StringType> linkId; 5675 5676 /** 5677 * A set of security labels that define which terms are controlled by this condition. 5678 */ 5679 @Child(name = "securityLabelNumber", type = {UnsignedIntType.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5680 @Description(shortDefinition="Security Labels that define affected terms", formalDefinition="A set of security labels that define which terms are controlled by this condition." ) 5681 protected List<UnsignedIntType> securityLabelNumber; 5682 5683 private static final long serialVersionUID = 915998998L; 5684 5685 /** 5686 * Constructor 5687 */ 5688 public ValuedItemComponent() { 5689 super(); 5690 } 5691 5692 /** 5693 * @return {@link #entity} (Specific type of Contract Valued Item that may be priced.) 5694 */ 5695 public DataType getEntity() { 5696 return this.entity; 5697 } 5698 5699 /** 5700 * @return {@link #entity} (Specific type of Contract Valued Item that may be priced.) 5701 */ 5702 public CodeableConcept getEntityCodeableConcept() throws FHIRException { 5703 if (this.entity == null) 5704 this.entity = new CodeableConcept(); 5705 if (!(this.entity instanceof CodeableConcept)) 5706 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.entity.getClass().getName()+" was encountered"); 5707 return (CodeableConcept) this.entity; 5708 } 5709 5710 public boolean hasEntityCodeableConcept() { 5711 return this != null && this.entity instanceof CodeableConcept; 5712 } 5713 5714 /** 5715 * @return {@link #entity} (Specific type of Contract Valued Item that may be priced.) 5716 */ 5717 public Reference getEntityReference() throws FHIRException { 5718 if (this.entity == null) 5719 this.entity = new Reference(); 5720 if (!(this.entity instanceof Reference)) 5721 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.entity.getClass().getName()+" was encountered"); 5722 return (Reference) this.entity; 5723 } 5724 5725 public boolean hasEntityReference() { 5726 return this != null && this.entity instanceof Reference; 5727 } 5728 5729 public boolean hasEntity() { 5730 return this.entity != null && !this.entity.isEmpty(); 5731 } 5732 5733 /** 5734 * @param value {@link #entity} (Specific type of Contract Valued Item that may be priced.) 5735 */ 5736 public ValuedItemComponent setEntity(DataType value) { 5737 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 5738 throw new FHIRException("Not the right type for Contract.term.asset.valuedItem.entity[x]: "+value.fhirType()); 5739 this.entity = value; 5740 return this; 5741 } 5742 5743 /** 5744 * @return {@link #identifier} (Identifies a Contract Valued Item instance.) 5745 */ 5746 public Identifier getIdentifier() { 5747 if (this.identifier == null) 5748 if (Configuration.errorOnAutoCreate()) 5749 throw new Error("Attempt to auto-create ValuedItemComponent.identifier"); 5750 else if (Configuration.doAutoCreate()) 5751 this.identifier = new Identifier(); // cc 5752 return this.identifier; 5753 } 5754 5755 public boolean hasIdentifier() { 5756 return this.identifier != null && !this.identifier.isEmpty(); 5757 } 5758 5759 /** 5760 * @param value {@link #identifier} (Identifies a Contract Valued Item instance.) 5761 */ 5762 public ValuedItemComponent setIdentifier(Identifier value) { 5763 this.identifier = value; 5764 return this; 5765 } 5766 5767 /** 5768 * @return {@link #effectiveTime} (Indicates the time during which this Contract ValuedItem information is effective.). This is the underlying object with id, value and extensions. The accessor "getEffectiveTime" gives direct access to the value 5769 */ 5770 public DateTimeType getEffectiveTimeElement() { 5771 if (this.effectiveTime == null) 5772 if (Configuration.errorOnAutoCreate()) 5773 throw new Error("Attempt to auto-create ValuedItemComponent.effectiveTime"); 5774 else if (Configuration.doAutoCreate()) 5775 this.effectiveTime = new DateTimeType(); // bb 5776 return this.effectiveTime; 5777 } 5778 5779 public boolean hasEffectiveTimeElement() { 5780 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 5781 } 5782 5783 public boolean hasEffectiveTime() { 5784 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 5785 } 5786 5787 /** 5788 * @param value {@link #effectiveTime} (Indicates the time during which this Contract ValuedItem information is effective.). This is the underlying object with id, value and extensions. The accessor "getEffectiveTime" gives direct access to the value 5789 */ 5790 public ValuedItemComponent setEffectiveTimeElement(DateTimeType value) { 5791 this.effectiveTime = value; 5792 return this; 5793 } 5794 5795 /** 5796 * @return Indicates the time during which this Contract ValuedItem information is effective. 5797 */ 5798 public Date getEffectiveTime() { 5799 return this.effectiveTime == null ? null : this.effectiveTime.getValue(); 5800 } 5801 5802 /** 5803 * @param value Indicates the time during which this Contract ValuedItem information is effective. 5804 */ 5805 public ValuedItemComponent setEffectiveTime(Date value) { 5806 if (value == null) 5807 this.effectiveTime = null; 5808 else { 5809 if (this.effectiveTime == null) 5810 this.effectiveTime = new DateTimeType(); 5811 this.effectiveTime.setValue(value); 5812 } 5813 return this; 5814 } 5815 5816 /** 5817 * @return {@link #quantity} (Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.) 5818 */ 5819 public Quantity getQuantity() { 5820 if (this.quantity == null) 5821 if (Configuration.errorOnAutoCreate()) 5822 throw new Error("Attempt to auto-create ValuedItemComponent.quantity"); 5823 else if (Configuration.doAutoCreate()) 5824 this.quantity = new Quantity(); // cc 5825 return this.quantity; 5826 } 5827 5828 public boolean hasQuantity() { 5829 return this.quantity != null && !this.quantity.isEmpty(); 5830 } 5831 5832 /** 5833 * @param value {@link #quantity} (Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.) 5834 */ 5835 public ValuedItemComponent setQuantity(Quantity value) { 5836 this.quantity = value; 5837 return this; 5838 } 5839 5840 /** 5841 * @return {@link #unitPrice} (A Contract Valued Item unit valuation measure.) 5842 */ 5843 public Money getUnitPrice() { 5844 if (this.unitPrice == null) 5845 if (Configuration.errorOnAutoCreate()) 5846 throw new Error("Attempt to auto-create ValuedItemComponent.unitPrice"); 5847 else if (Configuration.doAutoCreate()) 5848 this.unitPrice = new Money(); // cc 5849 return this.unitPrice; 5850 } 5851 5852 public boolean hasUnitPrice() { 5853 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5854 } 5855 5856 /** 5857 * @param value {@link #unitPrice} (A Contract Valued Item unit valuation measure.) 5858 */ 5859 public ValuedItemComponent setUnitPrice(Money value) { 5860 this.unitPrice = value; 5861 return this; 5862 } 5863 5864 /** 5865 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5866 */ 5867 public DecimalType getFactorElement() { 5868 if (this.factor == null) 5869 if (Configuration.errorOnAutoCreate()) 5870 throw new Error("Attempt to auto-create ValuedItemComponent.factor"); 5871 else if (Configuration.doAutoCreate()) 5872 this.factor = new DecimalType(); // bb 5873 return this.factor; 5874 } 5875 5876 public boolean hasFactorElement() { 5877 return this.factor != null && !this.factor.isEmpty(); 5878 } 5879 5880 public boolean hasFactor() { 5881 return this.factor != null && !this.factor.isEmpty(); 5882 } 5883 5884 /** 5885 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5886 */ 5887 public ValuedItemComponent setFactorElement(DecimalType value) { 5888 this.factor = value; 5889 return this; 5890 } 5891 5892 /** 5893 * @return A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5894 */ 5895 public BigDecimal getFactor() { 5896 return this.factor == null ? null : this.factor.getValue(); 5897 } 5898 5899 /** 5900 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5901 */ 5902 public ValuedItemComponent setFactor(BigDecimal value) { 5903 if (value == null) 5904 this.factor = null; 5905 else { 5906 if (this.factor == null) 5907 this.factor = new DecimalType(); 5908 this.factor.setValue(value); 5909 } 5910 return this; 5911 } 5912 5913 /** 5914 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5915 */ 5916 public ValuedItemComponent setFactor(long value) { 5917 this.factor = new DecimalType(); 5918 this.factor.setValue(value); 5919 return this; 5920 } 5921 5922 /** 5923 * @param value A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5924 */ 5925 public ValuedItemComponent setFactor(double value) { 5926 this.factor = new DecimalType(); 5927 this.factor.setValue(value); 5928 return this; 5929 } 5930 5931 /** 5932 * @return {@link #points} (An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.). This is the underlying object with id, value and extensions. The accessor "getPoints" gives direct access to the value 5933 */ 5934 public DecimalType getPointsElement() { 5935 if (this.points == null) 5936 if (Configuration.errorOnAutoCreate()) 5937 throw new Error("Attempt to auto-create ValuedItemComponent.points"); 5938 else if (Configuration.doAutoCreate()) 5939 this.points = new DecimalType(); // bb 5940 return this.points; 5941 } 5942 5943 public boolean hasPointsElement() { 5944 return this.points != null && !this.points.isEmpty(); 5945 } 5946 5947 public boolean hasPoints() { 5948 return this.points != null && !this.points.isEmpty(); 5949 } 5950 5951 /** 5952 * @param value {@link #points} (An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.). This is the underlying object with id, value and extensions. The accessor "getPoints" gives direct access to the value 5953 */ 5954 public ValuedItemComponent setPointsElement(DecimalType value) { 5955 this.points = value; 5956 return this; 5957 } 5958 5959 /** 5960 * @return An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 5961 */ 5962 public BigDecimal getPoints() { 5963 return this.points == null ? null : this.points.getValue(); 5964 } 5965 5966 /** 5967 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 5968 */ 5969 public ValuedItemComponent setPoints(BigDecimal value) { 5970 if (value == null) 5971 this.points = null; 5972 else { 5973 if (this.points == null) 5974 this.points = new DecimalType(); 5975 this.points.setValue(value); 5976 } 5977 return this; 5978 } 5979 5980 /** 5981 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 5982 */ 5983 public ValuedItemComponent setPoints(long value) { 5984 this.points = new DecimalType(); 5985 this.points.setValue(value); 5986 return this; 5987 } 5988 5989 /** 5990 * @param value An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point. 5991 */ 5992 public ValuedItemComponent setPoints(double value) { 5993 this.points = new DecimalType(); 5994 this.points.setValue(value); 5995 return this; 5996 } 5997 5998 /** 5999 * @return {@link #net} (Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6000 */ 6001 public Money getNet() { 6002 if (this.net == null) 6003 if (Configuration.errorOnAutoCreate()) 6004 throw new Error("Attempt to auto-create ValuedItemComponent.net"); 6005 else if (Configuration.doAutoCreate()) 6006 this.net = new Money(); // cc 6007 return this.net; 6008 } 6009 6010 public boolean hasNet() { 6011 return this.net != null && !this.net.isEmpty(); 6012 } 6013 6014 /** 6015 * @param value {@link #net} (Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6016 */ 6017 public ValuedItemComponent setNet(Money value) { 6018 this.net = value; 6019 return this; 6020 } 6021 6022 /** 6023 * @return {@link #payment} (Terms of valuation.). This is the underlying object with id, value and extensions. The accessor "getPayment" gives direct access to the value 6024 */ 6025 public StringType getPaymentElement() { 6026 if (this.payment == null) 6027 if (Configuration.errorOnAutoCreate()) 6028 throw new Error("Attempt to auto-create ValuedItemComponent.payment"); 6029 else if (Configuration.doAutoCreate()) 6030 this.payment = new StringType(); // bb 6031 return this.payment; 6032 } 6033 6034 public boolean hasPaymentElement() { 6035 return this.payment != null && !this.payment.isEmpty(); 6036 } 6037 6038 public boolean hasPayment() { 6039 return this.payment != null && !this.payment.isEmpty(); 6040 } 6041 6042 /** 6043 * @param value {@link #payment} (Terms of valuation.). This is the underlying object with id, value and extensions. The accessor "getPayment" gives direct access to the value 6044 */ 6045 public ValuedItemComponent setPaymentElement(StringType value) { 6046 this.payment = value; 6047 return this; 6048 } 6049 6050 /** 6051 * @return Terms of valuation. 6052 */ 6053 public String getPayment() { 6054 return this.payment == null ? null : this.payment.getValue(); 6055 } 6056 6057 /** 6058 * @param value Terms of valuation. 6059 */ 6060 public ValuedItemComponent setPayment(String value) { 6061 if (Utilities.noString(value)) 6062 this.payment = null; 6063 else { 6064 if (this.payment == null) 6065 this.payment = new StringType(); 6066 this.payment.setValue(value); 6067 } 6068 return this; 6069 } 6070 6071 /** 6072 * @return {@link #paymentDate} (When payment is due.). This is the underlying object with id, value and extensions. The accessor "getPaymentDate" gives direct access to the value 6073 */ 6074 public DateTimeType getPaymentDateElement() { 6075 if (this.paymentDate == null) 6076 if (Configuration.errorOnAutoCreate()) 6077 throw new Error("Attempt to auto-create ValuedItemComponent.paymentDate"); 6078 else if (Configuration.doAutoCreate()) 6079 this.paymentDate = new DateTimeType(); // bb 6080 return this.paymentDate; 6081 } 6082 6083 public boolean hasPaymentDateElement() { 6084 return this.paymentDate != null && !this.paymentDate.isEmpty(); 6085 } 6086 6087 public boolean hasPaymentDate() { 6088 return this.paymentDate != null && !this.paymentDate.isEmpty(); 6089 } 6090 6091 /** 6092 * @param value {@link #paymentDate} (When payment is due.). This is the underlying object with id, value and extensions. The accessor "getPaymentDate" gives direct access to the value 6093 */ 6094 public ValuedItemComponent setPaymentDateElement(DateTimeType value) { 6095 this.paymentDate = value; 6096 return this; 6097 } 6098 6099 /** 6100 * @return When payment is due. 6101 */ 6102 public Date getPaymentDate() { 6103 return this.paymentDate == null ? null : this.paymentDate.getValue(); 6104 } 6105 6106 /** 6107 * @param value When payment is due. 6108 */ 6109 public ValuedItemComponent setPaymentDate(Date value) { 6110 if (value == null) 6111 this.paymentDate = null; 6112 else { 6113 if (this.paymentDate == null) 6114 this.paymentDate = new DateTimeType(); 6115 this.paymentDate.setValue(value); 6116 } 6117 return this; 6118 } 6119 6120 /** 6121 * @return {@link #responsible} (Who will make payment.) 6122 */ 6123 public Reference getResponsible() { 6124 if (this.responsible == null) 6125 if (Configuration.errorOnAutoCreate()) 6126 throw new Error("Attempt to auto-create ValuedItemComponent.responsible"); 6127 else if (Configuration.doAutoCreate()) 6128 this.responsible = new Reference(); // cc 6129 return this.responsible; 6130 } 6131 6132 public boolean hasResponsible() { 6133 return this.responsible != null && !this.responsible.isEmpty(); 6134 } 6135 6136 /** 6137 * @param value {@link #responsible} (Who will make payment.) 6138 */ 6139 public ValuedItemComponent setResponsible(Reference value) { 6140 this.responsible = value; 6141 return this; 6142 } 6143 6144 /** 6145 * @return {@link #recipient} (Who will receive payment.) 6146 */ 6147 public Reference getRecipient() { 6148 if (this.recipient == null) 6149 if (Configuration.errorOnAutoCreate()) 6150 throw new Error("Attempt to auto-create ValuedItemComponent.recipient"); 6151 else if (Configuration.doAutoCreate()) 6152 this.recipient = new Reference(); // cc 6153 return this.recipient; 6154 } 6155 6156 public boolean hasRecipient() { 6157 return this.recipient != null && !this.recipient.isEmpty(); 6158 } 6159 6160 /** 6161 * @param value {@link #recipient} (Who will receive payment.) 6162 */ 6163 public ValuedItemComponent setRecipient(Reference value) { 6164 this.recipient = value; 6165 return this; 6166 } 6167 6168 /** 6169 * @return {@link #linkId} (Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.) 6170 */ 6171 public List<StringType> getLinkId() { 6172 if (this.linkId == null) 6173 this.linkId = new ArrayList<StringType>(); 6174 return this.linkId; 6175 } 6176 6177 /** 6178 * @return Returns a reference to <code>this</code> for easy method chaining 6179 */ 6180 public ValuedItemComponent setLinkId(List<StringType> theLinkId) { 6181 this.linkId = theLinkId; 6182 return this; 6183 } 6184 6185 public boolean hasLinkId() { 6186 if (this.linkId == null) 6187 return false; 6188 for (StringType item : this.linkId) 6189 if (!item.isEmpty()) 6190 return true; 6191 return false; 6192 } 6193 6194 /** 6195 * @return {@link #linkId} (Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.) 6196 */ 6197 public StringType addLinkIdElement() {//2 6198 StringType t = new StringType(); 6199 if (this.linkId == null) 6200 this.linkId = new ArrayList<StringType>(); 6201 this.linkId.add(t); 6202 return t; 6203 } 6204 6205 /** 6206 * @param value {@link #linkId} (Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.) 6207 */ 6208 public ValuedItemComponent addLinkId(String value) { //1 6209 StringType t = new StringType(); 6210 t.setValue(value); 6211 if (this.linkId == null) 6212 this.linkId = new ArrayList<StringType>(); 6213 this.linkId.add(t); 6214 return this; 6215 } 6216 6217 /** 6218 * @param value {@link #linkId} (Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.) 6219 */ 6220 public boolean hasLinkId(String value) { 6221 if (this.linkId == null) 6222 return false; 6223 for (StringType v : this.linkId) 6224 if (v.getValue().equals(value)) // string 6225 return true; 6226 return false; 6227 } 6228 6229 /** 6230 * @return {@link #securityLabelNumber} (A set of security labels that define which terms are controlled by this condition.) 6231 */ 6232 public List<UnsignedIntType> getSecurityLabelNumber() { 6233 if (this.securityLabelNumber == null) 6234 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 6235 return this.securityLabelNumber; 6236 } 6237 6238 /** 6239 * @return Returns a reference to <code>this</code> for easy method chaining 6240 */ 6241 public ValuedItemComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 6242 this.securityLabelNumber = theSecurityLabelNumber; 6243 return this; 6244 } 6245 6246 public boolean hasSecurityLabelNumber() { 6247 if (this.securityLabelNumber == null) 6248 return false; 6249 for (UnsignedIntType item : this.securityLabelNumber) 6250 if (!item.isEmpty()) 6251 return true; 6252 return false; 6253 } 6254 6255 /** 6256 * @return {@link #securityLabelNumber} (A set of security labels that define which terms are controlled by this condition.) 6257 */ 6258 public UnsignedIntType addSecurityLabelNumberElement() {//2 6259 UnsignedIntType t = new UnsignedIntType(); 6260 if (this.securityLabelNumber == null) 6261 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 6262 this.securityLabelNumber.add(t); 6263 return t; 6264 } 6265 6266 /** 6267 * @param value {@link #securityLabelNumber} (A set of security labels that define which terms are controlled by this condition.) 6268 */ 6269 public ValuedItemComponent addSecurityLabelNumber(int value) { //1 6270 UnsignedIntType t = new UnsignedIntType(); 6271 t.setValue(value); 6272 if (this.securityLabelNumber == null) 6273 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 6274 this.securityLabelNumber.add(t); 6275 return this; 6276 } 6277 6278 /** 6279 * @param value {@link #securityLabelNumber} (A set of security labels that define which terms are controlled by this condition.) 6280 */ 6281 public boolean hasSecurityLabelNumber(int value) { 6282 if (this.securityLabelNumber == null) 6283 return false; 6284 for (UnsignedIntType v : this.securityLabelNumber) 6285 if (v.getValue().equals(value)) // unsignedInt 6286 return true; 6287 return false; 6288 } 6289 6290 protected void listChildren(List<Property> children) { 6291 super.listChildren(children); 6292 children.add(new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity)); 6293 children.add(new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 0, 1, identifier)); 6294 children.add(new Property("effectiveTime", "dateTime", "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime)); 6295 children.add(new Property("quantity", "Quantity", "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 0, 1, quantity)); 6296 children.add(new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 1, unitPrice)); 6297 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6298 children.add(new Property("points", "decimal", "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 0, 1, points)); 6299 children.add(new Property("net", "Money", "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 6300 children.add(new Property("payment", "string", "Terms of valuation.", 0, 1, payment)); 6301 children.add(new Property("paymentDate", "dateTime", "When payment is due.", 0, 1, paymentDate)); 6302 children.add(new Property("responsible", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will make payment.", 0, 1, responsible)); 6303 children.add(new Property("recipient", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will receive payment.", 0, 1, recipient)); 6304 children.add(new Property("linkId", "string", "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, linkId)); 6305 children.add(new Property("securityLabelNumber", "unsignedInt", "A set of security labels that define which terms are controlled by this condition.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber)); 6306 } 6307 6308 @Override 6309 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6310 switch (_hash) { 6311 case -740568643: /*entity[x]*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 6312 case -1298275357: /*entity*/ return new Property("entity[x]", "CodeableConcept|Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 6313 case 924197182: /*entityCodeableConcept*/ return new Property("entity[x]", "CodeableConcept", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 6314 case -356635992: /*entityReference*/ return new Property("entity[x]", "Reference(Any)", "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 6315 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 0, 1, identifier); 6316 case -929905388: /*effectiveTime*/ return new Property("effectiveTime", "dateTime", "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime); 6317 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 0, 1, quantity); 6318 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 1, unitPrice); 6319 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6320 case -982754077: /*points*/ return new Property("points", "decimal", "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 0, 1, points); 6321 case 108957: /*net*/ return new Property("net", "Money", "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 6322 case -786681338: /*payment*/ return new Property("payment", "string", "Terms of valuation.", 0, 1, payment); 6323 case -1540873516: /*paymentDate*/ return new Property("paymentDate", "dateTime", "When payment is due.", 0, 1, paymentDate); 6324 case 1847674614: /*responsible*/ return new Property("responsible", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will make payment.", 0, 1, responsible); 6325 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will receive payment.", 0, 1, recipient); 6326 case -1102667083: /*linkId*/ return new Property("linkId", "string", "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, linkId); 6327 case -149460995: /*securityLabelNumber*/ return new Property("securityLabelNumber", "unsignedInt", "A set of security labels that define which terms are controlled by this condition.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 6328 default: return super.getNamedProperty(_hash, _name, _checkValid); 6329 } 6330 6331 } 6332 6333 @Override 6334 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6335 switch (hash) { 6336 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : new Base[] {this.entity}; // DataType 6337 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 6338 case -929905388: /*effectiveTime*/ return this.effectiveTime == null ? new Base[0] : new Base[] {this.effectiveTime}; // DateTimeType 6339 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 6340 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6341 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6342 case -982754077: /*points*/ return this.points == null ? new Base[0] : new Base[] {this.points}; // DecimalType 6343 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6344 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // StringType 6345 case -1540873516: /*paymentDate*/ return this.paymentDate == null ? new Base[0] : new Base[] {this.paymentDate}; // DateTimeType 6346 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // Reference 6347 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : new Base[] {this.recipient}; // Reference 6348 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 6349 case -149460995: /*securityLabelNumber*/ return this.securityLabelNumber == null ? new Base[0] : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 6350 default: return super.getProperty(hash, name, checkValid); 6351 } 6352 6353 } 6354 6355 @Override 6356 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6357 switch (hash) { 6358 case -1298275357: // entity 6359 this.entity = TypeConvertor.castToType(value); // DataType 6360 return value; 6361 case -1618432855: // identifier 6362 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 6363 return value; 6364 case -929905388: // effectiveTime 6365 this.effectiveTime = TypeConvertor.castToDateTime(value); // DateTimeType 6366 return value; 6367 case -1285004149: // quantity 6368 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6369 return value; 6370 case -486196699: // unitPrice 6371 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6372 return value; 6373 case -1282148017: // factor 6374 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6375 return value; 6376 case -982754077: // points 6377 this.points = TypeConvertor.castToDecimal(value); // DecimalType 6378 return value; 6379 case 108957: // net 6380 this.net = TypeConvertor.castToMoney(value); // Money 6381 return value; 6382 case -786681338: // payment 6383 this.payment = TypeConvertor.castToString(value); // StringType 6384 return value; 6385 case -1540873516: // paymentDate 6386 this.paymentDate = TypeConvertor.castToDateTime(value); // DateTimeType 6387 return value; 6388 case 1847674614: // responsible 6389 this.responsible = TypeConvertor.castToReference(value); // Reference 6390 return value; 6391 case 820081177: // recipient 6392 this.recipient = TypeConvertor.castToReference(value); // Reference 6393 return value; 6394 case -1102667083: // linkId 6395 this.getLinkId().add(TypeConvertor.castToString(value)); // StringType 6396 return value; 6397 case -149460995: // securityLabelNumber 6398 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); // UnsignedIntType 6399 return value; 6400 default: return super.setProperty(hash, name, value); 6401 } 6402 6403 } 6404 6405 @Override 6406 public Base setProperty(String name, Base value) throws FHIRException { 6407 if (name.equals("entity[x]")) { 6408 this.entity = TypeConvertor.castToType(value); // DataType 6409 } else if (name.equals("identifier")) { 6410 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 6411 } else if (name.equals("effectiveTime")) { 6412 this.effectiveTime = TypeConvertor.castToDateTime(value); // DateTimeType 6413 } else if (name.equals("quantity")) { 6414 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6415 } else if (name.equals("unitPrice")) { 6416 this.unitPrice = TypeConvertor.castToMoney(value); // Money 6417 } else if (name.equals("factor")) { 6418 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 6419 } else if (name.equals("points")) { 6420 this.points = TypeConvertor.castToDecimal(value); // DecimalType 6421 } else if (name.equals("net")) { 6422 this.net = TypeConvertor.castToMoney(value); // Money 6423 } else if (name.equals("payment")) { 6424 this.payment = TypeConvertor.castToString(value); // StringType 6425 } else if (name.equals("paymentDate")) { 6426 this.paymentDate = TypeConvertor.castToDateTime(value); // DateTimeType 6427 } else if (name.equals("responsible")) { 6428 this.responsible = TypeConvertor.castToReference(value); // Reference 6429 } else if (name.equals("recipient")) { 6430 this.recipient = TypeConvertor.castToReference(value); // Reference 6431 } else if (name.equals("linkId")) { 6432 this.getLinkId().add(TypeConvertor.castToString(value)); 6433 } else if (name.equals("securityLabelNumber")) { 6434 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); 6435 } else 6436 return super.setProperty(name, value); 6437 return value; 6438 } 6439 6440 @Override 6441 public void removeChild(String name, Base value) throws FHIRException { 6442 if (name.equals("entity[x]")) { 6443 this.entity = null; 6444 } else if (name.equals("identifier")) { 6445 this.identifier = null; 6446 } else if (name.equals("effectiveTime")) { 6447 this.effectiveTime = null; 6448 } else if (name.equals("quantity")) { 6449 this.quantity = null; 6450 } else if (name.equals("unitPrice")) { 6451 this.unitPrice = null; 6452 } else if (name.equals("factor")) { 6453 this.factor = null; 6454 } else if (name.equals("points")) { 6455 this.points = null; 6456 } else if (name.equals("net")) { 6457 this.net = null; 6458 } else if (name.equals("payment")) { 6459 this.payment = null; 6460 } else if (name.equals("paymentDate")) { 6461 this.paymentDate = null; 6462 } else if (name.equals("responsible")) { 6463 this.responsible = null; 6464 } else if (name.equals("recipient")) { 6465 this.recipient = null; 6466 } else if (name.equals("linkId")) { 6467 this.getLinkId().remove(value); 6468 } else if (name.equals("securityLabelNumber")) { 6469 this.getSecurityLabelNumber().remove(value); 6470 } else 6471 super.removeChild(name, value); 6472 6473 } 6474 6475 @Override 6476 public Base makeProperty(int hash, String name) throws FHIRException { 6477 switch (hash) { 6478 case -740568643: return getEntity(); 6479 case -1298275357: return getEntity(); 6480 case -1618432855: return getIdentifier(); 6481 case -929905388: return getEffectiveTimeElement(); 6482 case -1285004149: return getQuantity(); 6483 case -486196699: return getUnitPrice(); 6484 case -1282148017: return getFactorElement(); 6485 case -982754077: return getPointsElement(); 6486 case 108957: return getNet(); 6487 case -786681338: return getPaymentElement(); 6488 case -1540873516: return getPaymentDateElement(); 6489 case 1847674614: return getResponsible(); 6490 case 820081177: return getRecipient(); 6491 case -1102667083: return addLinkIdElement(); 6492 case -149460995: return addSecurityLabelNumberElement(); 6493 default: return super.makeProperty(hash, name); 6494 } 6495 6496 } 6497 6498 @Override 6499 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6500 switch (hash) { 6501 case -1298275357: /*entity*/ return new String[] {"CodeableConcept", "Reference"}; 6502 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6503 case -929905388: /*effectiveTime*/ return new String[] {"dateTime"}; 6504 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 6505 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6506 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6507 case -982754077: /*points*/ return new String[] {"decimal"}; 6508 case 108957: /*net*/ return new String[] {"Money"}; 6509 case -786681338: /*payment*/ return new String[] {"string"}; 6510 case -1540873516: /*paymentDate*/ return new String[] {"dateTime"}; 6511 case 1847674614: /*responsible*/ return new String[] {"Reference"}; 6512 case 820081177: /*recipient*/ return new String[] {"Reference"}; 6513 case -1102667083: /*linkId*/ return new String[] {"string"}; 6514 case -149460995: /*securityLabelNumber*/ return new String[] {"unsignedInt"}; 6515 default: return super.getTypesForProperty(hash, name); 6516 } 6517 6518 } 6519 6520 @Override 6521 public Base addChild(String name) throws FHIRException { 6522 if (name.equals("entityCodeableConcept")) { 6523 this.entity = new CodeableConcept(); 6524 return this.entity; 6525 } 6526 else if (name.equals("entityReference")) { 6527 this.entity = new Reference(); 6528 return this.entity; 6529 } 6530 else if (name.equals("identifier")) { 6531 this.identifier = new Identifier(); 6532 return this.identifier; 6533 } 6534 else if (name.equals("effectiveTime")) { 6535 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.valuedItem.effectiveTime"); 6536 } 6537 else if (name.equals("quantity")) { 6538 this.quantity = new Quantity(); 6539 return this.quantity; 6540 } 6541 else if (name.equals("unitPrice")) { 6542 this.unitPrice = new Money(); 6543 return this.unitPrice; 6544 } 6545 else if (name.equals("factor")) { 6546 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.valuedItem.factor"); 6547 } 6548 else if (name.equals("points")) { 6549 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.valuedItem.points"); 6550 } 6551 else if (name.equals("net")) { 6552 this.net = new Money(); 6553 return this.net; 6554 } 6555 else if (name.equals("payment")) { 6556 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.valuedItem.payment"); 6557 } 6558 else if (name.equals("paymentDate")) { 6559 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.valuedItem.paymentDate"); 6560 } 6561 else if (name.equals("responsible")) { 6562 this.responsible = new Reference(); 6563 return this.responsible; 6564 } 6565 else if (name.equals("recipient")) { 6566 this.recipient = new Reference(); 6567 return this.recipient; 6568 } 6569 else if (name.equals("linkId")) { 6570 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.valuedItem.linkId"); 6571 } 6572 else if (name.equals("securityLabelNumber")) { 6573 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.asset.valuedItem.securityLabelNumber"); 6574 } 6575 else 6576 return super.addChild(name); 6577 } 6578 6579 public ValuedItemComponent copy() { 6580 ValuedItemComponent dst = new ValuedItemComponent(); 6581 copyValues(dst); 6582 return dst; 6583 } 6584 6585 public void copyValues(ValuedItemComponent dst) { 6586 super.copyValues(dst); 6587 dst.entity = entity == null ? null : entity.copy(); 6588 dst.identifier = identifier == null ? null : identifier.copy(); 6589 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 6590 dst.quantity = quantity == null ? null : quantity.copy(); 6591 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6592 dst.factor = factor == null ? null : factor.copy(); 6593 dst.points = points == null ? null : points.copy(); 6594 dst.net = net == null ? null : net.copy(); 6595 dst.payment = payment == null ? null : payment.copy(); 6596 dst.paymentDate = paymentDate == null ? null : paymentDate.copy(); 6597 dst.responsible = responsible == null ? null : responsible.copy(); 6598 dst.recipient = recipient == null ? null : recipient.copy(); 6599 if (linkId != null) { 6600 dst.linkId = new ArrayList<StringType>(); 6601 for (StringType i : linkId) 6602 dst.linkId.add(i.copy()); 6603 }; 6604 if (securityLabelNumber != null) { 6605 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 6606 for (UnsignedIntType i : securityLabelNumber) 6607 dst.securityLabelNumber.add(i.copy()); 6608 }; 6609 } 6610 6611 @Override 6612 public boolean equalsDeep(Base other_) { 6613 if (!super.equalsDeep(other_)) 6614 return false; 6615 if (!(other_ instanceof ValuedItemComponent)) 6616 return false; 6617 ValuedItemComponent o = (ValuedItemComponent) other_; 6618 return compareDeep(entity, o.entity, true) && compareDeep(identifier, o.identifier, true) && compareDeep(effectiveTime, o.effectiveTime, true) 6619 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 6620 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true) && compareDeep(payment, o.payment, true) 6621 && compareDeep(paymentDate, o.paymentDate, true) && compareDeep(responsible, o.responsible, true) 6622 && compareDeep(recipient, o.recipient, true) && compareDeep(linkId, o.linkId, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true) 6623 ; 6624 } 6625 6626 @Override 6627 public boolean equalsShallow(Base other_) { 6628 if (!super.equalsShallow(other_)) 6629 return false; 6630 if (!(other_ instanceof ValuedItemComponent)) 6631 return false; 6632 ValuedItemComponent o = (ValuedItemComponent) other_; 6633 return compareValues(effectiveTime, o.effectiveTime, true) && compareValues(factor, o.factor, true) 6634 && compareValues(points, o.points, true) && compareValues(payment, o.payment, true) && compareValues(paymentDate, o.paymentDate, true) 6635 && compareValues(linkId, o.linkId, true) && compareValues(securityLabelNumber, o.securityLabelNumber, true) 6636 ; 6637 } 6638 6639 public boolean isEmpty() { 6640 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, identifier, effectiveTime 6641 , quantity, unitPrice, factor, points, net, payment, paymentDate, responsible 6642 , recipient, linkId, securityLabelNumber); 6643 } 6644 6645 public String fhirType() { 6646 return "Contract.term.asset.valuedItem"; 6647 6648 } 6649 6650 } 6651 6652 @Block() 6653 public static class ActionComponent extends BackboneElement implements IBaseBackboneElement { 6654 /** 6655 * True if the term prohibits the action. 6656 */ 6657 @Child(name = "doNotPerform", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=false) 6658 @Description(shortDefinition="True if the term prohibits the action", formalDefinition="True if the term prohibits the action." ) 6659 protected BooleanType doNotPerform; 6660 6661 /** 6662 * Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term. 6663 */ 6664 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 6665 @Description(shortDefinition="Type or form of the action", formalDefinition="Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term." ) 6666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-action") 6667 protected CodeableConcept type; 6668 6669 /** 6670 * Entity of the action. 6671 */ 6672 @Child(name = "subject", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6673 @Description(shortDefinition="Entity of the action", formalDefinition="Entity of the action." ) 6674 protected List<ActionSubjectComponent> subject; 6675 6676 /** 6677 * Reason or purpose for the action stipulated by this Contract Provision. 6678 */ 6679 @Child(name = "intent", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=false) 6680 @Description(shortDefinition="Purpose for the Contract Term Action", formalDefinition="Reason or purpose for the action stipulated by this Contract Provision." ) 6681 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 6682 protected CodeableConcept intent; 6683 6684 /** 6685 * Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse. 6686 */ 6687 @Child(name = "linkId", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6688 @Description(shortDefinition="Pointer to specific item", formalDefinition="Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse." ) 6689 protected List<StringType> linkId; 6690 6691 /** 6692 * Current state of the term action. 6693 */ 6694 @Child(name = "status", type = {CodeableConcept.class}, order=6, min=1, max=1, modifier=false, summary=false) 6695 @Description(shortDefinition="State of the action", formalDefinition="Current state of the term action." ) 6696 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-actionstatus") 6697 protected CodeableConcept status; 6698 6699 /** 6700 * Encounter or Episode with primary association to the specified term activity. 6701 */ 6702 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=7, min=0, max=1, modifier=false, summary=false) 6703 @Description(shortDefinition="Episode associated with action", formalDefinition="Encounter or Episode with primary association to the specified term activity." ) 6704 protected Reference context; 6705 6706 /** 6707 * Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse. 6708 */ 6709 @Child(name = "contextLinkId", type = {StringType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6710 @Description(shortDefinition="Pointer to specific item", formalDefinition="Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse." ) 6711 protected List<StringType> contextLinkId; 6712 6713 /** 6714 * When action happens. 6715 */ 6716 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=9, min=0, max=1, modifier=false, summary=false) 6717 @Description(shortDefinition="When action happens", formalDefinition="When action happens." ) 6718 protected DataType occurrence; 6719 6720 /** 6721 * Who or what initiated the action and has responsibility for its activation. 6722 */ 6723 @Child(name = "requester", type = {Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Device.class, Group.class, Organization.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6724 @Description(shortDefinition="Who asked for action", formalDefinition="Who or what initiated the action and has responsibility for its activation." ) 6725 protected List<Reference> requester; 6726 6727 /** 6728 * Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse. 6729 */ 6730 @Child(name = "requesterLinkId", type = {StringType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6731 @Description(shortDefinition="Pointer to specific item", formalDefinition="Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse." ) 6732 protected List<StringType> requesterLinkId; 6733 6734 /** 6735 * The type of individual that is desired or required to perform or not perform the action. 6736 */ 6737 @Child(name = "performerType", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6738 @Description(shortDefinition="Kind of service performer", formalDefinition="The type of individual that is desired or required to perform or not perform the action." ) 6739 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 6740 protected List<CodeableConcept> performerType; 6741 6742 /** 6743 * The type of role or competency of an individual desired or required to perform or not perform the action. 6744 */ 6745 @Child(name = "performerRole", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 6746 @Description(shortDefinition="Competency of the performer", formalDefinition="The type of role or competency of an individual desired or required to perform or not perform the action." ) 6747 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-role-type") 6748 protected CodeableConcept performerRole; 6749 6750 /** 6751 * Indicates who or what is being asked to perform (or not perform) the ction. 6752 */ 6753 @Child(name = "performer", type = {RelatedPerson.class, Patient.class, Practitioner.class, PractitionerRole.class, CareTeam.class, Device.class, Substance.class, Organization.class, Location.class}, order=14, min=0, max=1, modifier=false, summary=false) 6754 @Description(shortDefinition="Actor that wil execute (or not) the action", formalDefinition="Indicates who or what is being asked to perform (or not perform) the ction." ) 6755 protected Reference performer; 6756 6757 /** 6758 * Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse. 6759 */ 6760 @Child(name = "performerLinkId", type = {StringType.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6761 @Description(shortDefinition="Pointer to specific item", formalDefinition="Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse." ) 6762 protected List<StringType> performerLinkId; 6763 6764 /** 6765 * Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited. Either a coded concept, or another resource whose existence justifies permitting or not permitting this action. 6766 */ 6767 @Child(name = "reason", type = {CodeableReference.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6768 @Description(shortDefinition="Why is action (not) needed?", formalDefinition="Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited. Either a coded concept, or another resource whose existence justifies permitting or not permitting this action." ) 6769 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 6770 protected List<CodeableReference> reason; 6771 6772 /** 6773 * Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse. 6774 */ 6775 @Child(name = "reasonLinkId", type = {StringType.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6776 @Description(shortDefinition="Pointer to specific item", formalDefinition="Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse." ) 6777 protected List<StringType> reasonLinkId; 6778 6779 /** 6780 * Comments made about the term action made by the requester, performer, subject or other participants. 6781 */ 6782 @Child(name = "note", type = {Annotation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6783 @Description(shortDefinition="Comments about the action", formalDefinition="Comments made about the term action made by the requester, performer, subject or other participants." ) 6784 protected List<Annotation> note; 6785 6786 /** 6787 * Security labels that protects the action. 6788 */ 6789 @Child(name = "securityLabelNumber", type = {UnsignedIntType.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6790 @Description(shortDefinition="Action restriction numbers", formalDefinition="Security labels that protects the action." ) 6791 protected List<UnsignedIntType> securityLabelNumber; 6792 6793 private static final long serialVersionUID = 337159017L; 6794 6795 /** 6796 * Constructor 6797 */ 6798 public ActionComponent() { 6799 super(); 6800 } 6801 6802 /** 6803 * Constructor 6804 */ 6805 public ActionComponent(CodeableConcept type, CodeableConcept intent, CodeableConcept status) { 6806 super(); 6807 this.setType(type); 6808 this.setIntent(intent); 6809 this.setStatus(status); 6810 } 6811 6812 /** 6813 * @return {@link #doNotPerform} (True if the term prohibits the action.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 6814 */ 6815 public BooleanType getDoNotPerformElement() { 6816 if (this.doNotPerform == null) 6817 if (Configuration.errorOnAutoCreate()) 6818 throw new Error("Attempt to auto-create ActionComponent.doNotPerform"); 6819 else if (Configuration.doAutoCreate()) 6820 this.doNotPerform = new BooleanType(); // bb 6821 return this.doNotPerform; 6822 } 6823 6824 public boolean hasDoNotPerformElement() { 6825 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 6826 } 6827 6828 public boolean hasDoNotPerform() { 6829 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 6830 } 6831 6832 /** 6833 * @param value {@link #doNotPerform} (True if the term prohibits the action.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 6834 */ 6835 public ActionComponent setDoNotPerformElement(BooleanType value) { 6836 this.doNotPerform = value; 6837 return this; 6838 } 6839 6840 /** 6841 * @return True if the term prohibits the action. 6842 */ 6843 public boolean getDoNotPerform() { 6844 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 6845 } 6846 6847 /** 6848 * @param value True if the term prohibits the action. 6849 */ 6850 public ActionComponent setDoNotPerform(boolean value) { 6851 if (this.doNotPerform == null) 6852 this.doNotPerform = new BooleanType(); 6853 this.doNotPerform.setValue(value); 6854 return this; 6855 } 6856 6857 /** 6858 * @return {@link #type} (Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.) 6859 */ 6860 public CodeableConcept getType() { 6861 if (this.type == null) 6862 if (Configuration.errorOnAutoCreate()) 6863 throw new Error("Attempt to auto-create ActionComponent.type"); 6864 else if (Configuration.doAutoCreate()) 6865 this.type = new CodeableConcept(); // cc 6866 return this.type; 6867 } 6868 6869 public boolean hasType() { 6870 return this.type != null && !this.type.isEmpty(); 6871 } 6872 6873 /** 6874 * @param value {@link #type} (Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.) 6875 */ 6876 public ActionComponent setType(CodeableConcept value) { 6877 this.type = value; 6878 return this; 6879 } 6880 6881 /** 6882 * @return {@link #subject} (Entity of the action.) 6883 */ 6884 public List<ActionSubjectComponent> getSubject() { 6885 if (this.subject == null) 6886 this.subject = new ArrayList<ActionSubjectComponent>(); 6887 return this.subject; 6888 } 6889 6890 /** 6891 * @return Returns a reference to <code>this</code> for easy method chaining 6892 */ 6893 public ActionComponent setSubject(List<ActionSubjectComponent> theSubject) { 6894 this.subject = theSubject; 6895 return this; 6896 } 6897 6898 public boolean hasSubject() { 6899 if (this.subject == null) 6900 return false; 6901 for (ActionSubjectComponent item : this.subject) 6902 if (!item.isEmpty()) 6903 return true; 6904 return false; 6905 } 6906 6907 public ActionSubjectComponent addSubject() { //3 6908 ActionSubjectComponent t = new ActionSubjectComponent(); 6909 if (this.subject == null) 6910 this.subject = new ArrayList<ActionSubjectComponent>(); 6911 this.subject.add(t); 6912 return t; 6913 } 6914 6915 public ActionComponent addSubject(ActionSubjectComponent t) { //3 6916 if (t == null) 6917 return this; 6918 if (this.subject == null) 6919 this.subject = new ArrayList<ActionSubjectComponent>(); 6920 this.subject.add(t); 6921 return this; 6922 } 6923 6924 /** 6925 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 6926 */ 6927 public ActionSubjectComponent getSubjectFirstRep() { 6928 if (getSubject().isEmpty()) { 6929 addSubject(); 6930 } 6931 return getSubject().get(0); 6932 } 6933 6934 /** 6935 * @return {@link #intent} (Reason or purpose for the action stipulated by this Contract Provision.) 6936 */ 6937 public CodeableConcept getIntent() { 6938 if (this.intent == null) 6939 if (Configuration.errorOnAutoCreate()) 6940 throw new Error("Attempt to auto-create ActionComponent.intent"); 6941 else if (Configuration.doAutoCreate()) 6942 this.intent = new CodeableConcept(); // cc 6943 return this.intent; 6944 } 6945 6946 public boolean hasIntent() { 6947 return this.intent != null && !this.intent.isEmpty(); 6948 } 6949 6950 /** 6951 * @param value {@link #intent} (Reason or purpose for the action stipulated by this Contract Provision.) 6952 */ 6953 public ActionComponent setIntent(CodeableConcept value) { 6954 this.intent = value; 6955 return this; 6956 } 6957 6958 /** 6959 * @return {@link #linkId} (Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.) 6960 */ 6961 public List<StringType> getLinkId() { 6962 if (this.linkId == null) 6963 this.linkId = new ArrayList<StringType>(); 6964 return this.linkId; 6965 } 6966 6967 /** 6968 * @return Returns a reference to <code>this</code> for easy method chaining 6969 */ 6970 public ActionComponent setLinkId(List<StringType> theLinkId) { 6971 this.linkId = theLinkId; 6972 return this; 6973 } 6974 6975 public boolean hasLinkId() { 6976 if (this.linkId == null) 6977 return false; 6978 for (StringType item : this.linkId) 6979 if (!item.isEmpty()) 6980 return true; 6981 return false; 6982 } 6983 6984 /** 6985 * @return {@link #linkId} (Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.) 6986 */ 6987 public StringType addLinkIdElement() {//2 6988 StringType t = new StringType(); 6989 if (this.linkId == null) 6990 this.linkId = new ArrayList<StringType>(); 6991 this.linkId.add(t); 6992 return t; 6993 } 6994 6995 /** 6996 * @param value {@link #linkId} (Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.) 6997 */ 6998 public ActionComponent addLinkId(String value) { //1 6999 StringType t = new StringType(); 7000 t.setValue(value); 7001 if (this.linkId == null) 7002 this.linkId = new ArrayList<StringType>(); 7003 this.linkId.add(t); 7004 return this; 7005 } 7006 7007 /** 7008 * @param value {@link #linkId} (Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.) 7009 */ 7010 public boolean hasLinkId(String value) { 7011 if (this.linkId == null) 7012 return false; 7013 for (StringType v : this.linkId) 7014 if (v.getValue().equals(value)) // string 7015 return true; 7016 return false; 7017 } 7018 7019 /** 7020 * @return {@link #status} (Current state of the term action.) 7021 */ 7022 public CodeableConcept getStatus() { 7023 if (this.status == null) 7024 if (Configuration.errorOnAutoCreate()) 7025 throw new Error("Attempt to auto-create ActionComponent.status"); 7026 else if (Configuration.doAutoCreate()) 7027 this.status = new CodeableConcept(); // cc 7028 return this.status; 7029 } 7030 7031 public boolean hasStatus() { 7032 return this.status != null && !this.status.isEmpty(); 7033 } 7034 7035 /** 7036 * @param value {@link #status} (Current state of the term action.) 7037 */ 7038 public ActionComponent setStatus(CodeableConcept value) { 7039 this.status = value; 7040 return this; 7041 } 7042 7043 /** 7044 * @return {@link #context} (Encounter or Episode with primary association to the specified term activity.) 7045 */ 7046 public Reference getContext() { 7047 if (this.context == null) 7048 if (Configuration.errorOnAutoCreate()) 7049 throw new Error("Attempt to auto-create ActionComponent.context"); 7050 else if (Configuration.doAutoCreate()) 7051 this.context = new Reference(); // cc 7052 return this.context; 7053 } 7054 7055 public boolean hasContext() { 7056 return this.context != null && !this.context.isEmpty(); 7057 } 7058 7059 /** 7060 * @param value {@link #context} (Encounter or Episode with primary association to the specified term activity.) 7061 */ 7062 public ActionComponent setContext(Reference value) { 7063 this.context = value; 7064 return this; 7065 } 7066 7067 /** 7068 * @return {@link #contextLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7069 */ 7070 public List<StringType> getContextLinkId() { 7071 if (this.contextLinkId == null) 7072 this.contextLinkId = new ArrayList<StringType>(); 7073 return this.contextLinkId; 7074 } 7075 7076 /** 7077 * @return Returns a reference to <code>this</code> for easy method chaining 7078 */ 7079 public ActionComponent setContextLinkId(List<StringType> theContextLinkId) { 7080 this.contextLinkId = theContextLinkId; 7081 return this; 7082 } 7083 7084 public boolean hasContextLinkId() { 7085 if (this.contextLinkId == null) 7086 return false; 7087 for (StringType item : this.contextLinkId) 7088 if (!item.isEmpty()) 7089 return true; 7090 return false; 7091 } 7092 7093 /** 7094 * @return {@link #contextLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7095 */ 7096 public StringType addContextLinkIdElement() {//2 7097 StringType t = new StringType(); 7098 if (this.contextLinkId == null) 7099 this.contextLinkId = new ArrayList<StringType>(); 7100 this.contextLinkId.add(t); 7101 return t; 7102 } 7103 7104 /** 7105 * @param value {@link #contextLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7106 */ 7107 public ActionComponent addContextLinkId(String value) { //1 7108 StringType t = new StringType(); 7109 t.setValue(value); 7110 if (this.contextLinkId == null) 7111 this.contextLinkId = new ArrayList<StringType>(); 7112 this.contextLinkId.add(t); 7113 return this; 7114 } 7115 7116 /** 7117 * @param value {@link #contextLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7118 */ 7119 public boolean hasContextLinkId(String value) { 7120 if (this.contextLinkId == null) 7121 return false; 7122 for (StringType v : this.contextLinkId) 7123 if (v.getValue().equals(value)) // string 7124 return true; 7125 return false; 7126 } 7127 7128 /** 7129 * @return {@link #occurrence} (When action happens.) 7130 */ 7131 public DataType getOccurrence() { 7132 return this.occurrence; 7133 } 7134 7135 /** 7136 * @return {@link #occurrence} (When action happens.) 7137 */ 7138 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 7139 if (this.occurrence == null) 7140 this.occurrence = new DateTimeType(); 7141 if (!(this.occurrence instanceof DateTimeType)) 7142 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 7143 return (DateTimeType) this.occurrence; 7144 } 7145 7146 public boolean hasOccurrenceDateTimeType() { 7147 return this != null && this.occurrence instanceof DateTimeType; 7148 } 7149 7150 /** 7151 * @return {@link #occurrence} (When action happens.) 7152 */ 7153 public Period getOccurrencePeriod() throws FHIRException { 7154 if (this.occurrence == null) 7155 this.occurrence = new Period(); 7156 if (!(this.occurrence instanceof Period)) 7157 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 7158 return (Period) this.occurrence; 7159 } 7160 7161 public boolean hasOccurrencePeriod() { 7162 return this != null && this.occurrence instanceof Period; 7163 } 7164 7165 /** 7166 * @return {@link #occurrence} (When action happens.) 7167 */ 7168 public Timing getOccurrenceTiming() throws FHIRException { 7169 if (this.occurrence == null) 7170 this.occurrence = new Timing(); 7171 if (!(this.occurrence instanceof Timing)) 7172 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 7173 return (Timing) this.occurrence; 7174 } 7175 7176 public boolean hasOccurrenceTiming() { 7177 return this != null && this.occurrence instanceof Timing; 7178 } 7179 7180 public boolean hasOccurrence() { 7181 return this.occurrence != null && !this.occurrence.isEmpty(); 7182 } 7183 7184 /** 7185 * @param value {@link #occurrence} (When action happens.) 7186 */ 7187 public ActionComponent setOccurrence(DataType value) { 7188 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 7189 throw new FHIRException("Not the right type for Contract.term.action.occurrence[x]: "+value.fhirType()); 7190 this.occurrence = value; 7191 return this; 7192 } 7193 7194 /** 7195 * @return {@link #requester} (Who or what initiated the action and has responsibility for its activation.) 7196 */ 7197 public List<Reference> getRequester() { 7198 if (this.requester == null) 7199 this.requester = new ArrayList<Reference>(); 7200 return this.requester; 7201 } 7202 7203 /** 7204 * @return Returns a reference to <code>this</code> for easy method chaining 7205 */ 7206 public ActionComponent setRequester(List<Reference> theRequester) { 7207 this.requester = theRequester; 7208 return this; 7209 } 7210 7211 public boolean hasRequester() { 7212 if (this.requester == null) 7213 return false; 7214 for (Reference item : this.requester) 7215 if (!item.isEmpty()) 7216 return true; 7217 return false; 7218 } 7219 7220 public Reference addRequester() { //3 7221 Reference t = new Reference(); 7222 if (this.requester == null) 7223 this.requester = new ArrayList<Reference>(); 7224 this.requester.add(t); 7225 return t; 7226 } 7227 7228 public ActionComponent addRequester(Reference t) { //3 7229 if (t == null) 7230 return this; 7231 if (this.requester == null) 7232 this.requester = new ArrayList<Reference>(); 7233 this.requester.add(t); 7234 return this; 7235 } 7236 7237 /** 7238 * @return The first repetition of repeating field {@link #requester}, creating it if it does not already exist {3} 7239 */ 7240 public Reference getRequesterFirstRep() { 7241 if (getRequester().isEmpty()) { 7242 addRequester(); 7243 } 7244 return getRequester().get(0); 7245 } 7246 7247 /** 7248 * @return {@link #requesterLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7249 */ 7250 public List<StringType> getRequesterLinkId() { 7251 if (this.requesterLinkId == null) 7252 this.requesterLinkId = new ArrayList<StringType>(); 7253 return this.requesterLinkId; 7254 } 7255 7256 /** 7257 * @return Returns a reference to <code>this</code> for easy method chaining 7258 */ 7259 public ActionComponent setRequesterLinkId(List<StringType> theRequesterLinkId) { 7260 this.requesterLinkId = theRequesterLinkId; 7261 return this; 7262 } 7263 7264 public boolean hasRequesterLinkId() { 7265 if (this.requesterLinkId == null) 7266 return false; 7267 for (StringType item : this.requesterLinkId) 7268 if (!item.isEmpty()) 7269 return true; 7270 return false; 7271 } 7272 7273 /** 7274 * @return {@link #requesterLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7275 */ 7276 public StringType addRequesterLinkIdElement() {//2 7277 StringType t = new StringType(); 7278 if (this.requesterLinkId == null) 7279 this.requesterLinkId = new ArrayList<StringType>(); 7280 this.requesterLinkId.add(t); 7281 return t; 7282 } 7283 7284 /** 7285 * @param value {@link #requesterLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7286 */ 7287 public ActionComponent addRequesterLinkId(String value) { //1 7288 StringType t = new StringType(); 7289 t.setValue(value); 7290 if (this.requesterLinkId == null) 7291 this.requesterLinkId = new ArrayList<StringType>(); 7292 this.requesterLinkId.add(t); 7293 return this; 7294 } 7295 7296 /** 7297 * @param value {@link #requesterLinkId} (Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.) 7298 */ 7299 public boolean hasRequesterLinkId(String value) { 7300 if (this.requesterLinkId == null) 7301 return false; 7302 for (StringType v : this.requesterLinkId) 7303 if (v.getValue().equals(value)) // string 7304 return true; 7305 return false; 7306 } 7307 7308 /** 7309 * @return {@link #performerType} (The type of individual that is desired or required to perform or not perform the action.) 7310 */ 7311 public List<CodeableConcept> getPerformerType() { 7312 if (this.performerType == null) 7313 this.performerType = new ArrayList<CodeableConcept>(); 7314 return this.performerType; 7315 } 7316 7317 /** 7318 * @return Returns a reference to <code>this</code> for easy method chaining 7319 */ 7320 public ActionComponent setPerformerType(List<CodeableConcept> thePerformerType) { 7321 this.performerType = thePerformerType; 7322 return this; 7323 } 7324 7325 public boolean hasPerformerType() { 7326 if (this.performerType == null) 7327 return false; 7328 for (CodeableConcept item : this.performerType) 7329 if (!item.isEmpty()) 7330 return true; 7331 return false; 7332 } 7333 7334 public CodeableConcept addPerformerType() { //3 7335 CodeableConcept t = new CodeableConcept(); 7336 if (this.performerType == null) 7337 this.performerType = new ArrayList<CodeableConcept>(); 7338 this.performerType.add(t); 7339 return t; 7340 } 7341 7342 public ActionComponent addPerformerType(CodeableConcept t) { //3 7343 if (t == null) 7344 return this; 7345 if (this.performerType == null) 7346 this.performerType = new ArrayList<CodeableConcept>(); 7347 this.performerType.add(t); 7348 return this; 7349 } 7350 7351 /** 7352 * @return The first repetition of repeating field {@link #performerType}, creating it if it does not already exist {3} 7353 */ 7354 public CodeableConcept getPerformerTypeFirstRep() { 7355 if (getPerformerType().isEmpty()) { 7356 addPerformerType(); 7357 } 7358 return getPerformerType().get(0); 7359 } 7360 7361 /** 7362 * @return {@link #performerRole} (The type of role or competency of an individual desired or required to perform or not perform the action.) 7363 */ 7364 public CodeableConcept getPerformerRole() { 7365 if (this.performerRole == null) 7366 if (Configuration.errorOnAutoCreate()) 7367 throw new Error("Attempt to auto-create ActionComponent.performerRole"); 7368 else if (Configuration.doAutoCreate()) 7369 this.performerRole = new CodeableConcept(); // cc 7370 return this.performerRole; 7371 } 7372 7373 public boolean hasPerformerRole() { 7374 return this.performerRole != null && !this.performerRole.isEmpty(); 7375 } 7376 7377 /** 7378 * @param value {@link #performerRole} (The type of role or competency of an individual desired or required to perform or not perform the action.) 7379 */ 7380 public ActionComponent setPerformerRole(CodeableConcept value) { 7381 this.performerRole = value; 7382 return this; 7383 } 7384 7385 /** 7386 * @return {@link #performer} (Indicates who or what is being asked to perform (or not perform) the ction.) 7387 */ 7388 public Reference getPerformer() { 7389 if (this.performer == null) 7390 if (Configuration.errorOnAutoCreate()) 7391 throw new Error("Attempt to auto-create ActionComponent.performer"); 7392 else if (Configuration.doAutoCreate()) 7393 this.performer = new Reference(); // cc 7394 return this.performer; 7395 } 7396 7397 public boolean hasPerformer() { 7398 return this.performer != null && !this.performer.isEmpty(); 7399 } 7400 7401 /** 7402 * @param value {@link #performer} (Indicates who or what is being asked to perform (or not perform) the ction.) 7403 */ 7404 public ActionComponent setPerformer(Reference value) { 7405 this.performer = value; 7406 return this; 7407 } 7408 7409 /** 7410 * @return {@link #performerLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7411 */ 7412 public List<StringType> getPerformerLinkId() { 7413 if (this.performerLinkId == null) 7414 this.performerLinkId = new ArrayList<StringType>(); 7415 return this.performerLinkId; 7416 } 7417 7418 /** 7419 * @return Returns a reference to <code>this</code> for easy method chaining 7420 */ 7421 public ActionComponent setPerformerLinkId(List<StringType> thePerformerLinkId) { 7422 this.performerLinkId = thePerformerLinkId; 7423 return this; 7424 } 7425 7426 public boolean hasPerformerLinkId() { 7427 if (this.performerLinkId == null) 7428 return false; 7429 for (StringType item : this.performerLinkId) 7430 if (!item.isEmpty()) 7431 return true; 7432 return false; 7433 } 7434 7435 /** 7436 * @return {@link #performerLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7437 */ 7438 public StringType addPerformerLinkIdElement() {//2 7439 StringType t = new StringType(); 7440 if (this.performerLinkId == null) 7441 this.performerLinkId = new ArrayList<StringType>(); 7442 this.performerLinkId.add(t); 7443 return t; 7444 } 7445 7446 /** 7447 * @param value {@link #performerLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7448 */ 7449 public ActionComponent addPerformerLinkId(String value) { //1 7450 StringType t = new StringType(); 7451 t.setValue(value); 7452 if (this.performerLinkId == null) 7453 this.performerLinkId = new ArrayList<StringType>(); 7454 this.performerLinkId.add(t); 7455 return this; 7456 } 7457 7458 /** 7459 * @param value {@link #performerLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7460 */ 7461 public boolean hasPerformerLinkId(String value) { 7462 if (this.performerLinkId == null) 7463 return false; 7464 for (StringType v : this.performerLinkId) 7465 if (v.getValue().equals(value)) // string 7466 return true; 7467 return false; 7468 } 7469 7470 /** 7471 * @return {@link #reason} (Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited. Either a coded concept, or another resource whose existence justifies permitting or not permitting this action.) 7472 */ 7473 public List<CodeableReference> getReason() { 7474 if (this.reason == null) 7475 this.reason = new ArrayList<CodeableReference>(); 7476 return this.reason; 7477 } 7478 7479 /** 7480 * @return Returns a reference to <code>this</code> for easy method chaining 7481 */ 7482 public ActionComponent setReason(List<CodeableReference> theReason) { 7483 this.reason = theReason; 7484 return this; 7485 } 7486 7487 public boolean hasReason() { 7488 if (this.reason == null) 7489 return false; 7490 for (CodeableReference item : this.reason) 7491 if (!item.isEmpty()) 7492 return true; 7493 return false; 7494 } 7495 7496 public CodeableReference addReason() { //3 7497 CodeableReference t = new CodeableReference(); 7498 if (this.reason == null) 7499 this.reason = new ArrayList<CodeableReference>(); 7500 this.reason.add(t); 7501 return t; 7502 } 7503 7504 public ActionComponent addReason(CodeableReference t) { //3 7505 if (t == null) 7506 return this; 7507 if (this.reason == null) 7508 this.reason = new ArrayList<CodeableReference>(); 7509 this.reason.add(t); 7510 return this; 7511 } 7512 7513 /** 7514 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 7515 */ 7516 public CodeableReference getReasonFirstRep() { 7517 if (getReason().isEmpty()) { 7518 addReason(); 7519 } 7520 return getReason().get(0); 7521 } 7522 7523 /** 7524 * @return {@link #reasonLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7525 */ 7526 public List<StringType> getReasonLinkId() { 7527 if (this.reasonLinkId == null) 7528 this.reasonLinkId = new ArrayList<StringType>(); 7529 return this.reasonLinkId; 7530 } 7531 7532 /** 7533 * @return Returns a reference to <code>this</code> for easy method chaining 7534 */ 7535 public ActionComponent setReasonLinkId(List<StringType> theReasonLinkId) { 7536 this.reasonLinkId = theReasonLinkId; 7537 return this; 7538 } 7539 7540 public boolean hasReasonLinkId() { 7541 if (this.reasonLinkId == null) 7542 return false; 7543 for (StringType item : this.reasonLinkId) 7544 if (!item.isEmpty()) 7545 return true; 7546 return false; 7547 } 7548 7549 /** 7550 * @return {@link #reasonLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7551 */ 7552 public StringType addReasonLinkIdElement() {//2 7553 StringType t = new StringType(); 7554 if (this.reasonLinkId == null) 7555 this.reasonLinkId = new ArrayList<StringType>(); 7556 this.reasonLinkId.add(t); 7557 return t; 7558 } 7559 7560 /** 7561 * @param value {@link #reasonLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7562 */ 7563 public ActionComponent addReasonLinkId(String value) { //1 7564 StringType t = new StringType(); 7565 t.setValue(value); 7566 if (this.reasonLinkId == null) 7567 this.reasonLinkId = new ArrayList<StringType>(); 7568 this.reasonLinkId.add(t); 7569 return this; 7570 } 7571 7572 /** 7573 * @param value {@link #reasonLinkId} (Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.) 7574 */ 7575 public boolean hasReasonLinkId(String value) { 7576 if (this.reasonLinkId == null) 7577 return false; 7578 for (StringType v : this.reasonLinkId) 7579 if (v.getValue().equals(value)) // string 7580 return true; 7581 return false; 7582 } 7583 7584 /** 7585 * @return {@link #note} (Comments made about the term action made by the requester, performer, subject or other participants.) 7586 */ 7587 public List<Annotation> getNote() { 7588 if (this.note == null) 7589 this.note = new ArrayList<Annotation>(); 7590 return this.note; 7591 } 7592 7593 /** 7594 * @return Returns a reference to <code>this</code> for easy method chaining 7595 */ 7596 public ActionComponent setNote(List<Annotation> theNote) { 7597 this.note = theNote; 7598 return this; 7599 } 7600 7601 public boolean hasNote() { 7602 if (this.note == null) 7603 return false; 7604 for (Annotation item : this.note) 7605 if (!item.isEmpty()) 7606 return true; 7607 return false; 7608 } 7609 7610 public Annotation addNote() { //3 7611 Annotation t = new Annotation(); 7612 if (this.note == null) 7613 this.note = new ArrayList<Annotation>(); 7614 this.note.add(t); 7615 return t; 7616 } 7617 7618 public ActionComponent addNote(Annotation t) { //3 7619 if (t == null) 7620 return this; 7621 if (this.note == null) 7622 this.note = new ArrayList<Annotation>(); 7623 this.note.add(t); 7624 return this; 7625 } 7626 7627 /** 7628 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 7629 */ 7630 public Annotation getNoteFirstRep() { 7631 if (getNote().isEmpty()) { 7632 addNote(); 7633 } 7634 return getNote().get(0); 7635 } 7636 7637 /** 7638 * @return {@link #securityLabelNumber} (Security labels that protects the action.) 7639 */ 7640 public List<UnsignedIntType> getSecurityLabelNumber() { 7641 if (this.securityLabelNumber == null) 7642 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7643 return this.securityLabelNumber; 7644 } 7645 7646 /** 7647 * @return Returns a reference to <code>this</code> for easy method chaining 7648 */ 7649 public ActionComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 7650 this.securityLabelNumber = theSecurityLabelNumber; 7651 return this; 7652 } 7653 7654 public boolean hasSecurityLabelNumber() { 7655 if (this.securityLabelNumber == null) 7656 return false; 7657 for (UnsignedIntType item : this.securityLabelNumber) 7658 if (!item.isEmpty()) 7659 return true; 7660 return false; 7661 } 7662 7663 /** 7664 * @return {@link #securityLabelNumber} (Security labels that protects the action.) 7665 */ 7666 public UnsignedIntType addSecurityLabelNumberElement() {//2 7667 UnsignedIntType t = new UnsignedIntType(); 7668 if (this.securityLabelNumber == null) 7669 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7670 this.securityLabelNumber.add(t); 7671 return t; 7672 } 7673 7674 /** 7675 * @param value {@link #securityLabelNumber} (Security labels that protects the action.) 7676 */ 7677 public ActionComponent addSecurityLabelNumber(int value) { //1 7678 UnsignedIntType t = new UnsignedIntType(); 7679 t.setValue(value); 7680 if (this.securityLabelNumber == null) 7681 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7682 this.securityLabelNumber.add(t); 7683 return this; 7684 } 7685 7686 /** 7687 * @param value {@link #securityLabelNumber} (Security labels that protects the action.) 7688 */ 7689 public boolean hasSecurityLabelNumber(int value) { 7690 if (this.securityLabelNumber == null) 7691 return false; 7692 for (UnsignedIntType v : this.securityLabelNumber) 7693 if (v.getValue().equals(value)) // unsignedInt 7694 return true; 7695 return false; 7696 } 7697 7698 protected void listChildren(List<Property> children) { 7699 super.listChildren(children); 7700 children.add(new Property("doNotPerform", "boolean", "True if the term prohibits the action.", 0, 1, doNotPerform)); 7701 children.add(new Property("type", "CodeableConcept", "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.", 0, 1, type)); 7702 children.add(new Property("subject", "", "Entity of the action.", 0, java.lang.Integer.MAX_VALUE, subject)); 7703 children.add(new Property("intent", "CodeableConcept", "Reason or purpose for the action stipulated by this Contract Provision.", 0, 1, intent)); 7704 children.add(new Property("linkId", "string", "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, linkId)); 7705 children.add(new Property("status", "CodeableConcept", "Current state of the term action.", 0, 1, status)); 7706 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "Encounter or Episode with primary association to the specified term activity.", 0, 1, context)); 7707 children.add(new Property("contextLinkId", "string", "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, contextLinkId)); 7708 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, occurrence)); 7709 children.add(new Property("requester", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", "Who or what initiated the action and has responsibility for its activation.", 0, java.lang.Integer.MAX_VALUE, requester)); 7710 children.add(new Property("requesterLinkId", "string", "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, requesterLinkId)); 7711 children.add(new Property("performerType", "CodeableConcept", "The type of individual that is desired or required to perform or not perform the action.", 0, java.lang.Integer.MAX_VALUE, performerType)); 7712 children.add(new Property("performerRole", "CodeableConcept", "The type of role or competency of an individual desired or required to perform or not perform the action.", 0, 1, performerRole)); 7713 children.add(new Property("performer", "Reference(RelatedPerson|Patient|Practitioner|PractitionerRole|CareTeam|Device|Substance|Organization|Location)", "Indicates who or what is being asked to perform (or not perform) the ction.", 0, 1, performer)); 7714 children.add(new Property("performerLinkId", "string", "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, performerLinkId)); 7715 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference|Questionnaire|QuestionnaireResponse)", "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited. Either a coded concept, or another resource whose existence justifies permitting or not permitting this action.", 0, java.lang.Integer.MAX_VALUE, reason)); 7716 children.add(new Property("reasonLinkId", "string", "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, reasonLinkId)); 7717 children.add(new Property("note", "Annotation", "Comments made about the term action made by the requester, performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 7718 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the action.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber)); 7719 } 7720 7721 @Override 7722 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7723 switch (_hash) { 7724 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "True if the term prohibits the action.", 0, 1, doNotPerform); 7725 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.", 0, 1, type); 7726 case -1867885268: /*subject*/ return new Property("subject", "", "Entity of the action.", 0, java.lang.Integer.MAX_VALUE, subject); 7727 case -1183762788: /*intent*/ return new Property("intent", "CodeableConcept", "Reason or purpose for the action stipulated by this Contract Provision.", 0, 1, intent); 7728 case -1102667083: /*linkId*/ return new Property("linkId", "string", "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, linkId); 7729 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Current state of the term action.", 0, 1, status); 7730 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "Encounter or Episode with primary association to the specified term activity.", 0, 1, context); 7731 case -288783036: /*contextLinkId*/ return new Property("contextLinkId", "string", "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, contextLinkId); 7732 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, occurrence); 7733 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, occurrence); 7734 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "When action happens.", 0, 1, occurrence); 7735 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "When action happens.", 0, 1, occurrence); 7736 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "When action happens.", 0, 1, occurrence); 7737 case 693933948: /*requester*/ return new Property("requester", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", "Who or what initiated the action and has responsibility for its activation.", 0, java.lang.Integer.MAX_VALUE, requester); 7738 case -1468032687: /*requesterLinkId*/ return new Property("requesterLinkId", "string", "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, requesterLinkId); 7739 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "The type of individual that is desired or required to perform or not perform the action.", 0, java.lang.Integer.MAX_VALUE, performerType); 7740 case -901513884: /*performerRole*/ return new Property("performerRole", "CodeableConcept", "The type of role or competency of an individual desired or required to perform or not perform the action.", 0, 1, performerRole); 7741 case 481140686: /*performer*/ return new Property("performer", "Reference(RelatedPerson|Patient|Practitioner|PractitionerRole|CareTeam|Device|Substance|Organization|Location)", "Indicates who or what is being asked to perform (or not perform) the ction.", 0, 1, performer); 7742 case 1051302947: /*performerLinkId*/ return new Property("performerLinkId", "string", "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, performerLinkId); 7743 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference|Questionnaire|QuestionnaireResponse)", "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited. Either a coded concept, or another resource whose existence justifies permitting or not permitting this action.", 0, java.lang.Integer.MAX_VALUE, reason); 7744 case -1557963239: /*reasonLinkId*/ return new Property("reasonLinkId", "string", "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 0, java.lang.Integer.MAX_VALUE, reasonLinkId); 7745 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the term action made by the requester, performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 7746 case -149460995: /*securityLabelNumber*/ return new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the action.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 7747 default: return super.getNamedProperty(_hash, _name, _checkValid); 7748 } 7749 7750 } 7751 7752 @Override 7753 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7754 switch (hash) { 7755 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 7756 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 7757 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // ActionSubjectComponent 7758 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // CodeableConcept 7759 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 7760 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 7761 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 7762 case -288783036: /*contextLinkId*/ return this.contextLinkId == null ? new Base[0] : this.contextLinkId.toArray(new Base[this.contextLinkId.size()]); // StringType 7763 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 7764 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : this.requester.toArray(new Base[this.requester.size()]); // Reference 7765 case -1468032687: /*requesterLinkId*/ return this.requesterLinkId == null ? new Base[0] : this.requesterLinkId.toArray(new Base[this.requesterLinkId.size()]); // StringType 7766 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : this.performerType.toArray(new Base[this.performerType.size()]); // CodeableConcept 7767 case -901513884: /*performerRole*/ return this.performerRole == null ? new Base[0] : new Base[] {this.performerRole}; // CodeableConcept 7768 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 7769 case 1051302947: /*performerLinkId*/ return this.performerLinkId == null ? new Base[0] : this.performerLinkId.toArray(new Base[this.performerLinkId.size()]); // StringType 7770 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 7771 case -1557963239: /*reasonLinkId*/ return this.reasonLinkId == null ? new Base[0] : this.reasonLinkId.toArray(new Base[this.reasonLinkId.size()]); // StringType 7772 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 7773 case -149460995: /*securityLabelNumber*/ return this.securityLabelNumber == null ? new Base[0] : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 7774 default: return super.getProperty(hash, name, checkValid); 7775 } 7776 7777 } 7778 7779 @Override 7780 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7781 switch (hash) { 7782 case -1788508167: // doNotPerform 7783 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 7784 return value; 7785 case 3575610: // type 7786 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7787 return value; 7788 case -1867885268: // subject 7789 this.getSubject().add((ActionSubjectComponent) value); // ActionSubjectComponent 7790 return value; 7791 case -1183762788: // intent 7792 this.intent = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7793 return value; 7794 case -1102667083: // linkId 7795 this.getLinkId().add(TypeConvertor.castToString(value)); // StringType 7796 return value; 7797 case -892481550: // status 7798 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7799 return value; 7800 case 951530927: // context 7801 this.context = TypeConvertor.castToReference(value); // Reference 7802 return value; 7803 case -288783036: // contextLinkId 7804 this.getContextLinkId().add(TypeConvertor.castToString(value)); // StringType 7805 return value; 7806 case 1687874001: // occurrence 7807 this.occurrence = TypeConvertor.castToType(value); // DataType 7808 return value; 7809 case 693933948: // requester 7810 this.getRequester().add(TypeConvertor.castToReference(value)); // Reference 7811 return value; 7812 case -1468032687: // requesterLinkId 7813 this.getRequesterLinkId().add(TypeConvertor.castToString(value)); // StringType 7814 return value; 7815 case -901444568: // performerType 7816 this.getPerformerType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7817 return value; 7818 case -901513884: // performerRole 7819 this.performerRole = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7820 return value; 7821 case 481140686: // performer 7822 this.performer = TypeConvertor.castToReference(value); // Reference 7823 return value; 7824 case 1051302947: // performerLinkId 7825 this.getPerformerLinkId().add(TypeConvertor.castToString(value)); // StringType 7826 return value; 7827 case -934964668: // reason 7828 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 7829 return value; 7830 case -1557963239: // reasonLinkId 7831 this.getReasonLinkId().add(TypeConvertor.castToString(value)); // StringType 7832 return value; 7833 case 3387378: // note 7834 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 7835 return value; 7836 case -149460995: // securityLabelNumber 7837 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); // UnsignedIntType 7838 return value; 7839 default: return super.setProperty(hash, name, value); 7840 } 7841 7842 } 7843 7844 @Override 7845 public Base setProperty(String name, Base value) throws FHIRException { 7846 if (name.equals("doNotPerform")) { 7847 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 7848 } else if (name.equals("type")) { 7849 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7850 } else if (name.equals("subject")) { 7851 this.getSubject().add((ActionSubjectComponent) value); 7852 } else if (name.equals("intent")) { 7853 this.intent = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7854 } else if (name.equals("linkId")) { 7855 this.getLinkId().add(TypeConvertor.castToString(value)); 7856 } else if (name.equals("status")) { 7857 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7858 } else if (name.equals("context")) { 7859 this.context = TypeConvertor.castToReference(value); // Reference 7860 } else if (name.equals("contextLinkId")) { 7861 this.getContextLinkId().add(TypeConvertor.castToString(value)); 7862 } else if (name.equals("occurrence[x]")) { 7863 this.occurrence = TypeConvertor.castToType(value); // DataType 7864 } else if (name.equals("requester")) { 7865 this.getRequester().add(TypeConvertor.castToReference(value)); 7866 } else if (name.equals("requesterLinkId")) { 7867 this.getRequesterLinkId().add(TypeConvertor.castToString(value)); 7868 } else if (name.equals("performerType")) { 7869 this.getPerformerType().add(TypeConvertor.castToCodeableConcept(value)); 7870 } else if (name.equals("performerRole")) { 7871 this.performerRole = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7872 } else if (name.equals("performer")) { 7873 this.performer = TypeConvertor.castToReference(value); // Reference 7874 } else if (name.equals("performerLinkId")) { 7875 this.getPerformerLinkId().add(TypeConvertor.castToString(value)); 7876 } else if (name.equals("reason")) { 7877 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 7878 } else if (name.equals("reasonLinkId")) { 7879 this.getReasonLinkId().add(TypeConvertor.castToString(value)); 7880 } else if (name.equals("note")) { 7881 this.getNote().add(TypeConvertor.castToAnnotation(value)); 7882 } else if (name.equals("securityLabelNumber")) { 7883 this.getSecurityLabelNumber().add(TypeConvertor.castToUnsignedInt(value)); 7884 } else 7885 return super.setProperty(name, value); 7886 return value; 7887 } 7888 7889 @Override 7890 public void removeChild(String name, Base value) throws FHIRException { 7891 if (name.equals("doNotPerform")) { 7892 this.doNotPerform = null; 7893 } else if (name.equals("type")) { 7894 this.type = null; 7895 } else if (name.equals("subject")) { 7896 this.getSubject().remove((ActionSubjectComponent) value); 7897 } else if (name.equals("intent")) { 7898 this.intent = null; 7899 } else if (name.equals("linkId")) { 7900 this.getLinkId().remove(value); 7901 } else if (name.equals("status")) { 7902 this.status = null; 7903 } else if (name.equals("context")) { 7904 this.context = null; 7905 } else if (name.equals("contextLinkId")) { 7906 this.getContextLinkId().remove(value); 7907 } else if (name.equals("occurrence[x]")) { 7908 this.occurrence = null; 7909 } else if (name.equals("requester")) { 7910 this.getRequester().remove(value); 7911 } else if (name.equals("requesterLinkId")) { 7912 this.getRequesterLinkId().remove(value); 7913 } else if (name.equals("performerType")) { 7914 this.getPerformerType().remove(value); 7915 } else if (name.equals("performerRole")) { 7916 this.performerRole = null; 7917 } else if (name.equals("performer")) { 7918 this.performer = null; 7919 } else if (name.equals("performerLinkId")) { 7920 this.getPerformerLinkId().remove(value); 7921 } else if (name.equals("reason")) { 7922 this.getReason().remove(value); 7923 } else if (name.equals("reasonLinkId")) { 7924 this.getReasonLinkId().remove(value); 7925 } else if (name.equals("note")) { 7926 this.getNote().remove(value); 7927 } else if (name.equals("securityLabelNumber")) { 7928 this.getSecurityLabelNumber().remove(value); 7929 } else 7930 super.removeChild(name, value); 7931 7932 } 7933 7934 @Override 7935 public Base makeProperty(int hash, String name) throws FHIRException { 7936 switch (hash) { 7937 case -1788508167: return getDoNotPerformElement(); 7938 case 3575610: return getType(); 7939 case -1867885268: return addSubject(); 7940 case -1183762788: return getIntent(); 7941 case -1102667083: return addLinkIdElement(); 7942 case -892481550: return getStatus(); 7943 case 951530927: return getContext(); 7944 case -288783036: return addContextLinkIdElement(); 7945 case -2022646513: return getOccurrence(); 7946 case 1687874001: return getOccurrence(); 7947 case 693933948: return addRequester(); 7948 case -1468032687: return addRequesterLinkIdElement(); 7949 case -901444568: return addPerformerType(); 7950 case -901513884: return getPerformerRole(); 7951 case 481140686: return getPerformer(); 7952 case 1051302947: return addPerformerLinkIdElement(); 7953 case -934964668: return addReason(); 7954 case -1557963239: return addReasonLinkIdElement(); 7955 case 3387378: return addNote(); 7956 case -149460995: return addSecurityLabelNumberElement(); 7957 default: return super.makeProperty(hash, name); 7958 } 7959 7960 } 7961 7962 @Override 7963 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7964 switch (hash) { 7965 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 7966 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 7967 case -1867885268: /*subject*/ return new String[] {}; 7968 case -1183762788: /*intent*/ return new String[] {"CodeableConcept"}; 7969 case -1102667083: /*linkId*/ return new String[] {"string"}; 7970 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 7971 case 951530927: /*context*/ return new String[] {"Reference"}; 7972 case -288783036: /*contextLinkId*/ return new String[] {"string"}; 7973 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 7974 case 693933948: /*requester*/ return new String[] {"Reference"}; 7975 case -1468032687: /*requesterLinkId*/ return new String[] {"string"}; 7976 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 7977 case -901513884: /*performerRole*/ return new String[] {"CodeableConcept"}; 7978 case 481140686: /*performer*/ return new String[] {"Reference"}; 7979 case 1051302947: /*performerLinkId*/ return new String[] {"string"}; 7980 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 7981 case -1557963239: /*reasonLinkId*/ return new String[] {"string"}; 7982 case 3387378: /*note*/ return new String[] {"Annotation"}; 7983 case -149460995: /*securityLabelNumber*/ return new String[] {"unsignedInt"}; 7984 default: return super.getTypesForProperty(hash, name); 7985 } 7986 7987 } 7988 7989 @Override 7990 public Base addChild(String name) throws FHIRException { 7991 if (name.equals("doNotPerform")) { 7992 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.action.doNotPerform"); 7993 } 7994 else if (name.equals("type")) { 7995 this.type = new CodeableConcept(); 7996 return this.type; 7997 } 7998 else if (name.equals("subject")) { 7999 return addSubject(); 8000 } 8001 else if (name.equals("intent")) { 8002 this.intent = new CodeableConcept(); 8003 return this.intent; 8004 } 8005 else if (name.equals("linkId")) { 8006 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.action.linkId"); 8007 } 8008 else if (name.equals("status")) { 8009 this.status = new CodeableConcept(); 8010 return this.status; 8011 } 8012 else if (name.equals("context")) { 8013 this.context = new Reference(); 8014 return this.context; 8015 } 8016 else if (name.equals("contextLinkId")) { 8017 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.action.contextLinkId"); 8018 } 8019 else if (name.equals("occurrenceDateTime")) { 8020 this.occurrence = new DateTimeType(); 8021 return this.occurrence; 8022 } 8023 else if (name.equals("occurrencePeriod")) { 8024 this.occurrence = new Period(); 8025 return this.occurrence; 8026 } 8027 else if (name.equals("occurrenceTiming")) { 8028 this.occurrence = new Timing(); 8029 return this.occurrence; 8030 } 8031 else if (name.equals("requester")) { 8032 return addRequester(); 8033 } 8034 else if (name.equals("requesterLinkId")) { 8035 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.action.requesterLinkId"); 8036 } 8037 else if (name.equals("performerType")) { 8038 return addPerformerType(); 8039 } 8040 else if (name.equals("performerRole")) { 8041 this.performerRole = new CodeableConcept(); 8042 return this.performerRole; 8043 } 8044 else if (name.equals("performer")) { 8045 this.performer = new Reference(); 8046 return this.performer; 8047 } 8048 else if (name.equals("performerLinkId")) { 8049 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.action.performerLinkId"); 8050 } 8051 else if (name.equals("reason")) { 8052 return addReason(); 8053 } 8054 else if (name.equals("reasonLinkId")) { 8055 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.action.reasonLinkId"); 8056 } 8057 else if (name.equals("note")) { 8058 return addNote(); 8059 } 8060 else if (name.equals("securityLabelNumber")) { 8061 throw new FHIRException("Cannot call addChild on a singleton property Contract.term.action.securityLabelNumber"); 8062 } 8063 else 8064 return super.addChild(name); 8065 } 8066 8067 public ActionComponent copy() { 8068 ActionComponent dst = new ActionComponent(); 8069 copyValues(dst); 8070 return dst; 8071 } 8072 8073 public void copyValues(ActionComponent dst) { 8074 super.copyValues(dst); 8075 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 8076 dst.type = type == null ? null : type.copy(); 8077 if (subject != null) { 8078 dst.subject = new ArrayList<ActionSubjectComponent>(); 8079 for (ActionSubjectComponent i : subject) 8080 dst.subject.add(i.copy()); 8081 }; 8082 dst.intent = intent == null ? null : intent.copy(); 8083 if (linkId != null) { 8084 dst.linkId = new ArrayList<StringType>(); 8085 for (StringType i : linkId) 8086 dst.linkId.add(i.copy()); 8087 }; 8088 dst.status = status == null ? null : status.copy(); 8089 dst.context = context == null ? null : context.copy(); 8090 if (contextLinkId != null) { 8091 dst.contextLinkId = new ArrayList<StringType>(); 8092 for (StringType i : contextLinkId) 8093 dst.contextLinkId.add(i.copy()); 8094 }; 8095 dst.occurrence = occurrence == null ? null : occurrence.copy(); 8096 if (requester != null) { 8097 dst.requester = new ArrayList<Reference>(); 8098 for (Reference i : requester) 8099 dst.requester.add(i.copy()); 8100 }; 8101 if (requesterLinkId != null) { 8102 dst.requesterLinkId = new ArrayList<StringType>(); 8103 for (StringType i : requesterLinkId) 8104 dst.requesterLinkId.add(i.copy()); 8105 }; 8106 if (performerType != null) { 8107 dst.performerType = new ArrayList<CodeableConcept>(); 8108 for (CodeableConcept i : performerType) 8109 dst.performerType.add(i.copy()); 8110 }; 8111 dst.performerRole = performerRole == null ? null : performerRole.copy(); 8112 dst.performer = performer == null ? null : performer.copy(); 8113 if (performerLinkId != null) { 8114 dst.performerLinkId = new ArrayList<StringType>(); 8115 for (StringType i : performerLinkId) 8116 dst.performerLinkId.add(i.copy()); 8117 }; 8118 if (reason != null) { 8119 dst.reason = new ArrayList<CodeableReference>(); 8120 for (CodeableReference i : reason) 8121 dst.reason.add(i.copy()); 8122 }; 8123 if (reasonLinkId != null) { 8124 dst.reasonLinkId = new ArrayList<StringType>(); 8125 for (StringType i : reasonLinkId) 8126 dst.reasonLinkId.add(i.copy()); 8127 }; 8128 if (note != null) { 8129 dst.note = new ArrayList<Annotation>(); 8130 for (Annotation i : note) 8131 dst.note.add(i.copy()); 8132 }; 8133 if (securityLabelNumber != null) { 8134 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 8135 for (UnsignedIntType i : securityLabelNumber) 8136 dst.securityLabelNumber.add(i.copy()); 8137 }; 8138 } 8139 8140 @Override 8141 public boolean equalsDeep(Base other_) { 8142 if (!super.equalsDeep(other_)) 8143 return false; 8144 if (!(other_ instanceof ActionComponent)) 8145 return false; 8146 ActionComponent o = (ActionComponent) other_; 8147 return compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 8148 && compareDeep(intent, o.intent, true) && compareDeep(linkId, o.linkId, true) && compareDeep(status, o.status, true) 8149 && compareDeep(context, o.context, true) && compareDeep(contextLinkId, o.contextLinkId, true) && compareDeep(occurrence, o.occurrence, true) 8150 && compareDeep(requester, o.requester, true) && compareDeep(requesterLinkId, o.requesterLinkId, true) 8151 && compareDeep(performerType, o.performerType, true) && compareDeep(performerRole, o.performerRole, true) 8152 && compareDeep(performer, o.performer, true) && compareDeep(performerLinkId, o.performerLinkId, true) 8153 && compareDeep(reason, o.reason, true) && compareDeep(reasonLinkId, o.reasonLinkId, true) && compareDeep(note, o.note, true) 8154 && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 8155 } 8156 8157 @Override 8158 public boolean equalsShallow(Base other_) { 8159 if (!super.equalsShallow(other_)) 8160 return false; 8161 if (!(other_ instanceof ActionComponent)) 8162 return false; 8163 ActionComponent o = (ActionComponent) other_; 8164 return compareValues(doNotPerform, o.doNotPerform, true) && compareValues(linkId, o.linkId, true) && compareValues(contextLinkId, o.contextLinkId, true) 8165 && compareValues(requesterLinkId, o.requesterLinkId, true) && compareValues(performerLinkId, o.performerLinkId, true) 8166 && compareValues(reasonLinkId, o.reasonLinkId, true) && compareValues(securityLabelNumber, o.securityLabelNumber, true) 8167 ; 8168 } 8169 8170 public boolean isEmpty() { 8171 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(doNotPerform, type, subject 8172 , intent, linkId, status, context, contextLinkId, occurrence, requester, requesterLinkId 8173 , performerType, performerRole, performer, performerLinkId, reason, reasonLinkId 8174 , note, securityLabelNumber); 8175 } 8176 8177 public String fhirType() { 8178 return "Contract.term.action"; 8179 8180 } 8181 8182 } 8183 8184 @Block() 8185 public static class ActionSubjectComponent extends BackboneElement implements IBaseBackboneElement { 8186 /** 8187 * The entity the action is performed or not performed on or for. 8188 */ 8189 @Child(name = "reference", type = {Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Device.class, Group.class, Organization.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8190 @Description(shortDefinition="Entity of the action", formalDefinition="The entity the action is performed or not performed on or for." ) 8191 protected List<Reference> reference; 8192 8193 /** 8194 * Role type of agent assigned roles in this Contract. 8195 */ 8196 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 8197 @Description(shortDefinition="Role type of the agent", formalDefinition="Role type of agent assigned roles in this Contract." ) 8198 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-actorrole") 8199 protected CodeableConcept role; 8200 8201 private static final long serialVersionUID = -1599592477L; 8202 8203 /** 8204 * Constructor 8205 */ 8206 public ActionSubjectComponent() { 8207 super(); 8208 } 8209 8210 /** 8211 * Constructor 8212 */ 8213 public ActionSubjectComponent(Reference reference) { 8214 super(); 8215 this.addReference(reference); 8216 } 8217 8218 /** 8219 * @return {@link #reference} (The entity the action is performed or not performed on or for.) 8220 */ 8221 public List<Reference> getReference() { 8222 if (this.reference == null) 8223 this.reference = new ArrayList<Reference>(); 8224 return this.reference; 8225 } 8226 8227 /** 8228 * @return Returns a reference to <code>this</code> for easy method chaining 8229 */ 8230 public ActionSubjectComponent setReference(List<Reference> theReference) { 8231 this.reference = theReference; 8232 return this; 8233 } 8234 8235 public boolean hasReference() { 8236 if (this.reference == null) 8237 return false; 8238 for (Reference item : this.reference) 8239 if (!item.isEmpty()) 8240 return true; 8241 return false; 8242 } 8243 8244 public Reference addReference() { //3 8245 Reference t = new Reference(); 8246 if (this.reference == null) 8247 this.reference = new ArrayList<Reference>(); 8248 this.reference.add(t); 8249 return t; 8250 } 8251 8252 public ActionSubjectComponent addReference(Reference t) { //3 8253 if (t == null) 8254 return this; 8255 if (this.reference == null) 8256 this.reference = new ArrayList<Reference>(); 8257 this.reference.add(t); 8258 return this; 8259 } 8260 8261 /** 8262 * @return The first repetition of repeating field {@link #reference}, creating it if it does not already exist {3} 8263 */ 8264 public Reference getReferenceFirstRep() { 8265 if (getReference().isEmpty()) { 8266 addReference(); 8267 } 8268 return getReference().get(0); 8269 } 8270 8271 /** 8272 * @return {@link #role} (Role type of agent assigned roles in this Contract.) 8273 */ 8274 public CodeableConcept getRole() { 8275 if (this.role == null) 8276 if (Configuration.errorOnAutoCreate()) 8277 throw new Error("Attempt to auto-create ActionSubjectComponent.role"); 8278 else if (Configuration.doAutoCreate()) 8279 this.role = new CodeableConcept(); // cc 8280 return this.role; 8281 } 8282 8283 public boolean hasRole() { 8284 return this.role != null && !this.role.isEmpty(); 8285 } 8286 8287 /** 8288 * @param value {@link #role} (Role type of agent assigned roles in this Contract.) 8289 */ 8290 public ActionSubjectComponent setRole(CodeableConcept value) { 8291 this.role = value; 8292 return this; 8293 } 8294 8295 protected void listChildren(List<Property> children) { 8296 super.listChildren(children); 8297 children.add(new Property("reference", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", "The entity the action is performed or not performed on or for.", 0, java.lang.Integer.MAX_VALUE, reference)); 8298 children.add(new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 0, 1, role)); 8299 } 8300 8301 @Override 8302 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8303 switch (_hash) { 8304 case -925155509: /*reference*/ return new Property("reference", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", "The entity the action is performed or not performed on or for.", 0, java.lang.Integer.MAX_VALUE, reference); 8305 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 0, 1, role); 8306 default: return super.getNamedProperty(_hash, _name, _checkValid); 8307 } 8308 8309 } 8310 8311 @Override 8312 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8313 switch (hash) { 8314 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // Reference 8315 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 8316 default: return super.getProperty(hash, name, checkValid); 8317 } 8318 8319 } 8320 8321 @Override 8322 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8323 switch (hash) { 8324 case -925155509: // reference 8325 this.getReference().add(TypeConvertor.castToReference(value)); // Reference 8326 return value; 8327 case 3506294: // role 8328 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8329 return value; 8330 default: return super.setProperty(hash, name, value); 8331 } 8332 8333 } 8334 8335 @Override 8336 public Base setProperty(String name, Base value) throws FHIRException { 8337 if (name.equals("reference")) { 8338 this.getReference().add(TypeConvertor.castToReference(value)); 8339 } else if (name.equals("role")) { 8340 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8341 } else 8342 return super.setProperty(name, value); 8343 return value; 8344 } 8345 8346 @Override 8347 public void removeChild(String name, Base value) throws FHIRException { 8348 if (name.equals("reference")) { 8349 this.getReference().remove(value); 8350 } else if (name.equals("role")) { 8351 this.role = null; 8352 } else 8353 super.removeChild(name, value); 8354 8355 } 8356 8357 @Override 8358 public Base makeProperty(int hash, String name) throws FHIRException { 8359 switch (hash) { 8360 case -925155509: return addReference(); 8361 case 3506294: return getRole(); 8362 default: return super.makeProperty(hash, name); 8363 } 8364 8365 } 8366 8367 @Override 8368 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8369 switch (hash) { 8370 case -925155509: /*reference*/ return new String[] {"Reference"}; 8371 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 8372 default: return super.getTypesForProperty(hash, name); 8373 } 8374 8375 } 8376 8377 @Override 8378 public Base addChild(String name) throws FHIRException { 8379 if (name.equals("reference")) { 8380 return addReference(); 8381 } 8382 else if (name.equals("role")) { 8383 this.role = new CodeableConcept(); 8384 return this.role; 8385 } 8386 else 8387 return super.addChild(name); 8388 } 8389 8390 public ActionSubjectComponent copy() { 8391 ActionSubjectComponent dst = new ActionSubjectComponent(); 8392 copyValues(dst); 8393 return dst; 8394 } 8395 8396 public void copyValues(ActionSubjectComponent dst) { 8397 super.copyValues(dst); 8398 if (reference != null) { 8399 dst.reference = new ArrayList<Reference>(); 8400 for (Reference i : reference) 8401 dst.reference.add(i.copy()); 8402 }; 8403 dst.role = role == null ? null : role.copy(); 8404 } 8405 8406 @Override 8407 public boolean equalsDeep(Base other_) { 8408 if (!super.equalsDeep(other_)) 8409 return false; 8410 if (!(other_ instanceof ActionSubjectComponent)) 8411 return false; 8412 ActionSubjectComponent o = (ActionSubjectComponent) other_; 8413 return compareDeep(reference, o.reference, true) && compareDeep(role, o.role, true); 8414 } 8415 8416 @Override 8417 public boolean equalsShallow(Base other_) { 8418 if (!super.equalsShallow(other_)) 8419 return false; 8420 if (!(other_ instanceof ActionSubjectComponent)) 8421 return false; 8422 ActionSubjectComponent o = (ActionSubjectComponent) other_; 8423 return true; 8424 } 8425 8426 public boolean isEmpty() { 8427 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, role); 8428 } 8429 8430 public String fhirType() { 8431 return "Contract.term.action.subject"; 8432 8433 } 8434 8435 } 8436 8437 @Block() 8438 public static class SignatoryComponent extends BackboneElement implements IBaseBackboneElement { 8439 /** 8440 * Role of this Contract signer, e.g. notary, grantee. 8441 */ 8442 @Child(name = "type", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 8443 @Description(shortDefinition="Contract Signatory Role", formalDefinition="Role of this Contract signer, e.g. notary, grantee." ) 8444 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-signer-type") 8445 protected Coding type; 8446 8447 /** 8448 * Party which is a signator to this Contract. 8449 */ 8450 @Child(name = "party", type = {Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 8451 @Description(shortDefinition="Contract Signatory Party", formalDefinition="Party which is a signator to this Contract." ) 8452 protected Reference party; 8453 8454 /** 8455 * Legally binding Contract DSIG signature contents in Base64. 8456 */ 8457 @Child(name = "signature", type = {Signature.class}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8458 @Description(shortDefinition="Contract Documentation Signature", formalDefinition="Legally binding Contract DSIG signature contents in Base64." ) 8459 protected List<Signature> signature; 8460 8461 private static final long serialVersionUID = 1384929729L; 8462 8463 /** 8464 * Constructor 8465 */ 8466 public SignatoryComponent() { 8467 super(); 8468 } 8469 8470 /** 8471 * Constructor 8472 */ 8473 public SignatoryComponent(Coding type, Reference party, Signature signature) { 8474 super(); 8475 this.setType(type); 8476 this.setParty(party); 8477 this.addSignature(signature); 8478 } 8479 8480 /** 8481 * @return {@link #type} (Role of this Contract signer, e.g. notary, grantee.) 8482 */ 8483 public Coding getType() { 8484 if (this.type == null) 8485 if (Configuration.errorOnAutoCreate()) 8486 throw new Error("Attempt to auto-create SignatoryComponent.type"); 8487 else if (Configuration.doAutoCreate()) 8488 this.type = new Coding(); // cc 8489 return this.type; 8490 } 8491 8492 public boolean hasType() { 8493 return this.type != null && !this.type.isEmpty(); 8494 } 8495 8496 /** 8497 * @param value {@link #type} (Role of this Contract signer, e.g. notary, grantee.) 8498 */ 8499 public SignatoryComponent setType(Coding value) { 8500 this.type = value; 8501 return this; 8502 } 8503 8504 /** 8505 * @return {@link #party} (Party which is a signator to this Contract.) 8506 */ 8507 public Reference getParty() { 8508 if (this.party == null) 8509 if (Configuration.errorOnAutoCreate()) 8510 throw new Error("Attempt to auto-create SignatoryComponent.party"); 8511 else if (Configuration.doAutoCreate()) 8512 this.party = new Reference(); // cc 8513 return this.party; 8514 } 8515 8516 public boolean hasParty() { 8517 return this.party != null && !this.party.isEmpty(); 8518 } 8519 8520 /** 8521 * @param value {@link #party} (Party which is a signator to this Contract.) 8522 */ 8523 public SignatoryComponent setParty(Reference value) { 8524 this.party = value; 8525 return this; 8526 } 8527 8528 /** 8529 * @return {@link #signature} (Legally binding Contract DSIG signature contents in Base64.) 8530 */ 8531 public List<Signature> getSignature() { 8532 if (this.signature == null) 8533 this.signature = new ArrayList<Signature>(); 8534 return this.signature; 8535 } 8536 8537 /** 8538 * @return Returns a reference to <code>this</code> for easy method chaining 8539 */ 8540 public SignatoryComponent setSignature(List<Signature> theSignature) { 8541 this.signature = theSignature; 8542 return this; 8543 } 8544 8545 public boolean hasSignature() { 8546 if (this.signature == null) 8547 return false; 8548 for (Signature item : this.signature) 8549 if (!item.isEmpty()) 8550 return true; 8551 return false; 8552 } 8553 8554 public Signature addSignature() { //3 8555 Signature t = new Signature(); 8556 if (this.signature == null) 8557 this.signature = new ArrayList<Signature>(); 8558 this.signature.add(t); 8559 return t; 8560 } 8561 8562 public SignatoryComponent addSignature(Signature t) { //3 8563 if (t == null) 8564 return this; 8565 if (this.signature == null) 8566 this.signature = new ArrayList<Signature>(); 8567 this.signature.add(t); 8568 return this; 8569 } 8570 8571 /** 8572 * @return The first repetition of repeating field {@link #signature}, creating it if it does not already exist {3} 8573 */ 8574 public Signature getSignatureFirstRep() { 8575 if (getSignature().isEmpty()) { 8576 addSignature(); 8577 } 8578 return getSignature().get(0); 8579 } 8580 8581 protected void listChildren(List<Property> children) { 8582 super.listChildren(children); 8583 children.add(new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, type)); 8584 children.add(new Property("party", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Party which is a signator to this Contract.", 0, 1, party)); 8585 children.add(new Property("signature", "Signature", "Legally binding Contract DSIG signature contents in Base64.", 0, java.lang.Integer.MAX_VALUE, signature)); 8586 } 8587 8588 @Override 8589 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8590 switch (_hash) { 8591 case 3575610: /*type*/ return new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, type); 8592 case 106437350: /*party*/ return new Property("party", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Party which is a signator to this Contract.", 0, 1, party); 8593 case 1073584312: /*signature*/ return new Property("signature", "Signature", "Legally binding Contract DSIG signature contents in Base64.", 0, java.lang.Integer.MAX_VALUE, signature); 8594 default: return super.getNamedProperty(_hash, _name, _checkValid); 8595 } 8596 8597 } 8598 8599 @Override 8600 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8601 switch (hash) { 8602 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 8603 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 8604 case 1073584312: /*signature*/ return this.signature == null ? new Base[0] : this.signature.toArray(new Base[this.signature.size()]); // Signature 8605 default: return super.getProperty(hash, name, checkValid); 8606 } 8607 8608 } 8609 8610 @Override 8611 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8612 switch (hash) { 8613 case 3575610: // type 8614 this.type = TypeConvertor.castToCoding(value); // Coding 8615 return value; 8616 case 106437350: // party 8617 this.party = TypeConvertor.castToReference(value); // Reference 8618 return value; 8619 case 1073584312: // signature 8620 this.getSignature().add(TypeConvertor.castToSignature(value)); // Signature 8621 return value; 8622 default: return super.setProperty(hash, name, value); 8623 } 8624 8625 } 8626 8627 @Override 8628 public Base setProperty(String name, Base value) throws FHIRException { 8629 if (name.equals("type")) { 8630 this.type = TypeConvertor.castToCoding(value); // Coding 8631 } else if (name.equals("party")) { 8632 this.party = TypeConvertor.castToReference(value); // Reference 8633 } else if (name.equals("signature")) { 8634 this.getSignature().add(TypeConvertor.castToSignature(value)); 8635 } else 8636 return super.setProperty(name, value); 8637 return value; 8638 } 8639 8640 @Override 8641 public void removeChild(String name, Base value) throws FHIRException { 8642 if (name.equals("type")) { 8643 this.type = null; 8644 } else if (name.equals("party")) { 8645 this.party = null; 8646 } else if (name.equals("signature")) { 8647 this.getSignature().remove(value); 8648 } else 8649 super.removeChild(name, value); 8650 8651 } 8652 8653 @Override 8654 public Base makeProperty(int hash, String name) throws FHIRException { 8655 switch (hash) { 8656 case 3575610: return getType(); 8657 case 106437350: return getParty(); 8658 case 1073584312: return addSignature(); 8659 default: return super.makeProperty(hash, name); 8660 } 8661 8662 } 8663 8664 @Override 8665 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8666 switch (hash) { 8667 case 3575610: /*type*/ return new String[] {"Coding"}; 8668 case 106437350: /*party*/ return new String[] {"Reference"}; 8669 case 1073584312: /*signature*/ return new String[] {"Signature"}; 8670 default: return super.getTypesForProperty(hash, name); 8671 } 8672 8673 } 8674 8675 @Override 8676 public Base addChild(String name) throws FHIRException { 8677 if (name.equals("type")) { 8678 this.type = new Coding(); 8679 return this.type; 8680 } 8681 else if (name.equals("party")) { 8682 this.party = new Reference(); 8683 return this.party; 8684 } 8685 else if (name.equals("signature")) { 8686 return addSignature(); 8687 } 8688 else 8689 return super.addChild(name); 8690 } 8691 8692 public SignatoryComponent copy() { 8693 SignatoryComponent dst = new SignatoryComponent(); 8694 copyValues(dst); 8695 return dst; 8696 } 8697 8698 public void copyValues(SignatoryComponent dst) { 8699 super.copyValues(dst); 8700 dst.type = type == null ? null : type.copy(); 8701 dst.party = party == null ? null : party.copy(); 8702 if (signature != null) { 8703 dst.signature = new ArrayList<Signature>(); 8704 for (Signature i : signature) 8705 dst.signature.add(i.copy()); 8706 }; 8707 } 8708 8709 @Override 8710 public boolean equalsDeep(Base other_) { 8711 if (!super.equalsDeep(other_)) 8712 return false; 8713 if (!(other_ instanceof SignatoryComponent)) 8714 return false; 8715 SignatoryComponent o = (SignatoryComponent) other_; 8716 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true) && compareDeep(signature, o.signature, true) 8717 ; 8718 } 8719 8720 @Override 8721 public boolean equalsShallow(Base other_) { 8722 if (!super.equalsShallow(other_)) 8723 return false; 8724 if (!(other_ instanceof SignatoryComponent)) 8725 return false; 8726 SignatoryComponent o = (SignatoryComponent) other_; 8727 return true; 8728 } 8729 8730 public boolean isEmpty() { 8731 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party, signature); 8732 } 8733 8734 public String fhirType() { 8735 return "Contract.signer"; 8736 8737 } 8738 8739 } 8740 8741 @Block() 8742 public static class FriendlyLanguageComponent extends BackboneElement implements IBaseBackboneElement { 8743 /** 8744 * Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability. 8745 */ 8746 @Child(name = "content", type = {Attachment.class, Composition.class, DocumentReference.class, QuestionnaireResponse.class}, order=1, min=1, max=1, modifier=false, summary=false) 8747 @Description(shortDefinition="Easily comprehended representation of this Contract", formalDefinition="Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability." ) 8748 protected DataType content; 8749 8750 private static final long serialVersionUID = -1954179063L; 8751 8752 /** 8753 * Constructor 8754 */ 8755 public FriendlyLanguageComponent() { 8756 super(); 8757 } 8758 8759 /** 8760 * Constructor 8761 */ 8762 public FriendlyLanguageComponent(DataType content) { 8763 super(); 8764 this.setContent(content); 8765 } 8766 8767 /** 8768 * @return {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 8769 */ 8770 public DataType getContent() { 8771 return this.content; 8772 } 8773 8774 /** 8775 * @return {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 8776 */ 8777 public Attachment getContentAttachment() throws FHIRException { 8778 if (this.content == null) 8779 this.content = new Attachment(); 8780 if (!(this.content instanceof Attachment)) 8781 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 8782 return (Attachment) this.content; 8783 } 8784 8785 public boolean hasContentAttachment() { 8786 return this != null && this.content instanceof Attachment; 8787 } 8788 8789 /** 8790 * @return {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 8791 */ 8792 public Reference getContentReference() throws FHIRException { 8793 if (this.content == null) 8794 this.content = new Reference(); 8795 if (!(this.content instanceof Reference)) 8796 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 8797 return (Reference) this.content; 8798 } 8799 8800 public boolean hasContentReference() { 8801 return this != null && this.content instanceof Reference; 8802 } 8803 8804 public boolean hasContent() { 8805 return this.content != null && !this.content.isEmpty(); 8806 } 8807 8808 /** 8809 * @param value {@link #content} (Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.) 8810 */ 8811 public FriendlyLanguageComponent setContent(DataType value) { 8812 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 8813 throw new FHIRException("Not the right type for Contract.friendly.content[x]: "+value.fhirType()); 8814 this.content = value; 8815 return this; 8816 } 8817 8818 protected void listChildren(List<Property> children) { 8819 super.listChildren(children); 8820 children.add(new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content)); 8821 } 8822 8823 @Override 8824 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8825 switch (_hash) { 8826 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 8827 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 8828 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 8829 case 1193747154: /*contentReference*/ return new Property("content[x]", "Reference(Composition|DocumentReference|QuestionnaireResponse)", "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 0, 1, content); 8830 default: return super.getNamedProperty(_hash, _name, _checkValid); 8831 } 8832 8833 } 8834 8835 @Override 8836 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8837 switch (hash) { 8838 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // DataType 8839 default: return super.getProperty(hash, name, checkValid); 8840 } 8841 8842 } 8843 8844 @Override 8845 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8846 switch (hash) { 8847 case 951530617: // content 8848 this.content = TypeConvertor.castToType(value); // DataType 8849 return value; 8850 default: return super.setProperty(hash, name, value); 8851 } 8852 8853 } 8854 8855 @Override 8856 public Base setProperty(String name, Base value) throws FHIRException { 8857 if (name.equals("content[x]")) { 8858 this.content = TypeConvertor.castToType(value); // DataType 8859 } else 8860 return super.setProperty(name, value); 8861 return value; 8862 } 8863 8864 @Override 8865 public void removeChild(String name, Base value) throws FHIRException { 8866 if (name.equals("content[x]")) { 8867 this.content = null; 8868 } else 8869 super.removeChild(name, value); 8870 8871 } 8872 8873 @Override 8874 public Base makeProperty(int hash, String name) throws FHIRException { 8875 switch (hash) { 8876 case 264548711: return getContent(); 8877 case 951530617: return getContent(); 8878 default: return super.makeProperty(hash, name); 8879 } 8880 8881 } 8882 8883 @Override 8884 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8885 switch (hash) { 8886 case 951530617: /*content*/ return new String[] {"Attachment", "Reference"}; 8887 default: return super.getTypesForProperty(hash, name); 8888 } 8889 8890 } 8891 8892 @Override 8893 public Base addChild(String name) throws FHIRException { 8894 if (name.equals("contentAttachment")) { 8895 this.content = new Attachment(); 8896 return this.content; 8897 } 8898 else if (name.equals("contentReference")) { 8899 this.content = new Reference(); 8900 return this.content; 8901 } 8902 else 8903 return super.addChild(name); 8904 } 8905 8906 public FriendlyLanguageComponent copy() { 8907 FriendlyLanguageComponent dst = new FriendlyLanguageComponent(); 8908 copyValues(dst); 8909 return dst; 8910 } 8911 8912 public void copyValues(FriendlyLanguageComponent dst) { 8913 super.copyValues(dst); 8914 dst.content = content == null ? null : content.copy(); 8915 } 8916 8917 @Override 8918 public boolean equalsDeep(Base other_) { 8919 if (!super.equalsDeep(other_)) 8920 return false; 8921 if (!(other_ instanceof FriendlyLanguageComponent)) 8922 return false; 8923 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 8924 return compareDeep(content, o.content, true); 8925 } 8926 8927 @Override 8928 public boolean equalsShallow(Base other_) { 8929 if (!super.equalsShallow(other_)) 8930 return false; 8931 if (!(other_ instanceof FriendlyLanguageComponent)) 8932 return false; 8933 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 8934 return true; 8935 } 8936 8937 public boolean isEmpty() { 8938 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 8939 } 8940 8941 public String fhirType() { 8942 return "Contract.friendly"; 8943 8944 } 8945 8946 } 8947 8948 @Block() 8949 public static class LegalLanguageComponent extends BackboneElement implements IBaseBackboneElement { 8950 /** 8951 * Contract legal text in human renderable form. 8952 */ 8953 @Child(name = "content", type = {Attachment.class, Composition.class, DocumentReference.class, QuestionnaireResponse.class}, order=1, min=1, max=1, modifier=false, summary=false) 8954 @Description(shortDefinition="Contract Legal Text", formalDefinition="Contract legal text in human renderable form." ) 8955 protected DataType content; 8956 8957 private static final long serialVersionUID = -1954179063L; 8958 8959 /** 8960 * Constructor 8961 */ 8962 public LegalLanguageComponent() { 8963 super(); 8964 } 8965 8966 /** 8967 * Constructor 8968 */ 8969 public LegalLanguageComponent(DataType content) { 8970 super(); 8971 this.setContent(content); 8972 } 8973 8974 /** 8975 * @return {@link #content} (Contract legal text in human renderable form.) 8976 */ 8977 public DataType getContent() { 8978 return this.content; 8979 } 8980 8981 /** 8982 * @return {@link #content} (Contract legal text in human renderable form.) 8983 */ 8984 public Attachment getContentAttachment() throws FHIRException { 8985 if (this.content == null) 8986 this.content = new Attachment(); 8987 if (!(this.content instanceof Attachment)) 8988 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 8989 return (Attachment) this.content; 8990 } 8991 8992 public boolean hasContentAttachment() { 8993 return this != null && this.content instanceof Attachment; 8994 } 8995 8996 /** 8997 * @return {@link #content} (Contract legal text in human renderable form.) 8998 */ 8999 public Reference getContentReference() throws FHIRException { 9000 if (this.content == null) 9001 this.content = new Reference(); 9002 if (!(this.content instanceof Reference)) 9003 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 9004 return (Reference) this.content; 9005 } 9006 9007 public boolean hasContentReference() { 9008 return this != null && this.content instanceof Reference; 9009 } 9010 9011 public boolean hasContent() { 9012 return this.content != null && !this.content.isEmpty(); 9013 } 9014 9015 /** 9016 * @param value {@link #content} (Contract legal text in human renderable form.) 9017 */ 9018 public LegalLanguageComponent setContent(DataType value) { 9019 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 9020 throw new FHIRException("Not the right type for Contract.legal.content[x]: "+value.fhirType()); 9021 this.content = value; 9022 return this; 9023 } 9024 9025 protected void listChildren(List<Property> children) { 9026 super.listChildren(children); 9027 children.add(new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content)); 9028 } 9029 9030 @Override 9031 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9032 switch (_hash) { 9033 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content); 9034 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content); 9035 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment", "Contract legal text in human renderable form.", 0, 1, content); 9036 case 1193747154: /*contentReference*/ return new Property("content[x]", "Reference(Composition|DocumentReference|QuestionnaireResponse)", "Contract legal text in human renderable form.", 0, 1, content); 9037 default: return super.getNamedProperty(_hash, _name, _checkValid); 9038 } 9039 9040 } 9041 9042 @Override 9043 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9044 switch (hash) { 9045 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // DataType 9046 default: return super.getProperty(hash, name, checkValid); 9047 } 9048 9049 } 9050 9051 @Override 9052 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9053 switch (hash) { 9054 case 951530617: // content 9055 this.content = TypeConvertor.castToType(value); // DataType 9056 return value; 9057 default: return super.setProperty(hash, name, value); 9058 } 9059 9060 } 9061 9062 @Override 9063 public Base setProperty(String name, Base value) throws FHIRException { 9064 if (name.equals("content[x]")) { 9065 this.content = TypeConvertor.castToType(value); // DataType 9066 } else 9067 return super.setProperty(name, value); 9068 return value; 9069 } 9070 9071 @Override 9072 public void removeChild(String name, Base value) throws FHIRException { 9073 if (name.equals("content[x]")) { 9074 this.content = null; 9075 } else 9076 super.removeChild(name, value); 9077 9078 } 9079 9080 @Override 9081 public Base makeProperty(int hash, String name) throws FHIRException { 9082 switch (hash) { 9083 case 264548711: return getContent(); 9084 case 951530617: return getContent(); 9085 default: return super.makeProperty(hash, name); 9086 } 9087 9088 } 9089 9090 @Override 9091 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9092 switch (hash) { 9093 case 951530617: /*content*/ return new String[] {"Attachment", "Reference"}; 9094 default: return super.getTypesForProperty(hash, name); 9095 } 9096 9097 } 9098 9099 @Override 9100 public Base addChild(String name) throws FHIRException { 9101 if (name.equals("contentAttachment")) { 9102 this.content = new Attachment(); 9103 return this.content; 9104 } 9105 else if (name.equals("contentReference")) { 9106 this.content = new Reference(); 9107 return this.content; 9108 } 9109 else 9110 return super.addChild(name); 9111 } 9112 9113 public LegalLanguageComponent copy() { 9114 LegalLanguageComponent dst = new LegalLanguageComponent(); 9115 copyValues(dst); 9116 return dst; 9117 } 9118 9119 public void copyValues(LegalLanguageComponent dst) { 9120 super.copyValues(dst); 9121 dst.content = content == null ? null : content.copy(); 9122 } 9123 9124 @Override 9125 public boolean equalsDeep(Base other_) { 9126 if (!super.equalsDeep(other_)) 9127 return false; 9128 if (!(other_ instanceof LegalLanguageComponent)) 9129 return false; 9130 LegalLanguageComponent o = (LegalLanguageComponent) other_; 9131 return compareDeep(content, o.content, true); 9132 } 9133 9134 @Override 9135 public boolean equalsShallow(Base other_) { 9136 if (!super.equalsShallow(other_)) 9137 return false; 9138 if (!(other_ instanceof LegalLanguageComponent)) 9139 return false; 9140 LegalLanguageComponent o = (LegalLanguageComponent) other_; 9141 return true; 9142 } 9143 9144 public boolean isEmpty() { 9145 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 9146 } 9147 9148 public String fhirType() { 9149 return "Contract.legal"; 9150 9151 } 9152 9153 } 9154 9155 @Block() 9156 public static class ComputableLanguageComponent extends BackboneElement implements IBaseBackboneElement { 9157 /** 9158 * Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal). 9159 */ 9160 @Child(name = "content", type = {Attachment.class, DocumentReference.class}, order=1, min=1, max=1, modifier=false, summary=false) 9161 @Description(shortDefinition="Computable Contract Rules", formalDefinition="Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal)." ) 9162 protected DataType content; 9163 9164 private static final long serialVersionUID = -1954179063L; 9165 9166 /** 9167 * Constructor 9168 */ 9169 public ComputableLanguageComponent() { 9170 super(); 9171 } 9172 9173 /** 9174 * Constructor 9175 */ 9176 public ComputableLanguageComponent(DataType content) { 9177 super(); 9178 this.setContent(content); 9179 } 9180 9181 /** 9182 * @return {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 9183 */ 9184 public DataType getContent() { 9185 return this.content; 9186 } 9187 9188 /** 9189 * @return {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 9190 */ 9191 public Attachment getContentAttachment() throws FHIRException { 9192 if (this.content == null) 9193 this.content = new Attachment(); 9194 if (!(this.content instanceof Attachment)) 9195 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.content.getClass().getName()+" was encountered"); 9196 return (Attachment) this.content; 9197 } 9198 9199 public boolean hasContentAttachment() { 9200 return this != null && this.content instanceof Attachment; 9201 } 9202 9203 /** 9204 * @return {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 9205 */ 9206 public Reference getContentReference() throws FHIRException { 9207 if (this.content == null) 9208 this.content = new Reference(); 9209 if (!(this.content instanceof Reference)) 9210 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.content.getClass().getName()+" was encountered"); 9211 return (Reference) this.content; 9212 } 9213 9214 public boolean hasContentReference() { 9215 return this != null && this.content instanceof Reference; 9216 } 9217 9218 public boolean hasContent() { 9219 return this.content != null && !this.content.isEmpty(); 9220 } 9221 9222 /** 9223 * @param value {@link #content} (Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).) 9224 */ 9225 public ComputableLanguageComponent setContent(DataType value) { 9226 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 9227 throw new FHIRException("Not the right type for Contract.rule.content[x]: "+value.fhirType()); 9228 this.content = value; 9229 return this; 9230 } 9231 9232 protected void listChildren(List<Property> children) { 9233 super.listChildren(children); 9234 children.add(new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content)); 9235 } 9236 9237 @Override 9238 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9239 switch (_hash) { 9240 case 264548711: /*content[x]*/ return new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 9241 case 951530617: /*content*/ return new Property("content[x]", "Attachment|Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 9242 case -702028164: /*contentAttachment*/ return new Property("content[x]", "Attachment", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 9243 case 1193747154: /*contentReference*/ return new Property("content[x]", "Reference(DocumentReference)", "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 9244 default: return super.getNamedProperty(_hash, _name, _checkValid); 9245 } 9246 9247 } 9248 9249 @Override 9250 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9251 switch (hash) { 9252 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // DataType 9253 default: return super.getProperty(hash, name, checkValid); 9254 } 9255 9256 } 9257 9258 @Override 9259 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9260 switch (hash) { 9261 case 951530617: // content 9262 this.content = TypeConvertor.castToType(value); // DataType 9263 return value; 9264 default: return super.setProperty(hash, name, value); 9265 } 9266 9267 } 9268 9269 @Override 9270 public Base setProperty(String name, Base value) throws FHIRException { 9271 if (name.equals("content[x]")) { 9272 this.content = TypeConvertor.castToType(value); // DataType 9273 } else 9274 return super.setProperty(name, value); 9275 return value; 9276 } 9277 9278 @Override 9279 public void removeChild(String name, Base value) throws FHIRException { 9280 if (name.equals("content[x]")) { 9281 this.content = null; 9282 } else 9283 super.removeChild(name, value); 9284 9285 } 9286 9287 @Override 9288 public Base makeProperty(int hash, String name) throws FHIRException { 9289 switch (hash) { 9290 case 264548711: return getContent(); 9291 case 951530617: return getContent(); 9292 default: return super.makeProperty(hash, name); 9293 } 9294 9295 } 9296 9297 @Override 9298 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9299 switch (hash) { 9300 case 951530617: /*content*/ return new String[] {"Attachment", "Reference"}; 9301 default: return super.getTypesForProperty(hash, name); 9302 } 9303 9304 } 9305 9306 @Override 9307 public Base addChild(String name) throws FHIRException { 9308 if (name.equals("contentAttachment")) { 9309 this.content = new Attachment(); 9310 return this.content; 9311 } 9312 else if (name.equals("contentReference")) { 9313 this.content = new Reference(); 9314 return this.content; 9315 } 9316 else 9317 return super.addChild(name); 9318 } 9319 9320 public ComputableLanguageComponent copy() { 9321 ComputableLanguageComponent dst = new ComputableLanguageComponent(); 9322 copyValues(dst); 9323 return dst; 9324 } 9325 9326 public void copyValues(ComputableLanguageComponent dst) { 9327 super.copyValues(dst); 9328 dst.content = content == null ? null : content.copy(); 9329 } 9330 9331 @Override 9332 public boolean equalsDeep(Base other_) { 9333 if (!super.equalsDeep(other_)) 9334 return false; 9335 if (!(other_ instanceof ComputableLanguageComponent)) 9336 return false; 9337 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 9338 return compareDeep(content, o.content, true); 9339 } 9340 9341 @Override 9342 public boolean equalsShallow(Base other_) { 9343 if (!super.equalsShallow(other_)) 9344 return false; 9345 if (!(other_ instanceof ComputableLanguageComponent)) 9346 return false; 9347 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 9348 return true; 9349 } 9350 9351 public boolean isEmpty() { 9352 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 9353 } 9354 9355 public String fhirType() { 9356 return "Contract.rule"; 9357 9358 } 9359 9360 } 9361 9362 /** 9363 * Unique identifier for this Contract or a derivative that references a Source Contract. 9364 */ 9365 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 9366 @Description(shortDefinition="Contract number", formalDefinition="Unique identifier for this Contract or a derivative that references a Source Contract." ) 9367 protected List<Identifier> identifier; 9368 9369 /** 9370 * Canonical identifier for this contract, represented as a URI (globally unique). 9371 */ 9372 @Child(name = "url", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 9373 @Description(shortDefinition="Basal definition", formalDefinition="Canonical identifier for this contract, represented as a URI (globally unique)." ) 9374 protected UriType url; 9375 9376 /** 9377 * An edition identifier used for business purposes to label business significant variants. 9378 */ 9379 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 9380 @Description(shortDefinition="Business edition", formalDefinition="An edition identifier used for business purposes to label business significant variants." ) 9381 protected StringType version; 9382 9383 /** 9384 * The status of the resource instance. 9385 */ 9386 @Child(name = "status", type = {CodeType.class}, order=3, min=0, max=1, modifier=true, summary=true) 9387 @Description(shortDefinition="amended | appended | cancelled | disputed | entered-in-error | executable +", formalDefinition="The status of the resource instance." ) 9388 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-status") 9389 protected Enumeration<ContractResourceStatusCodes> status; 9390 9391 /** 9392 * Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement. 9393 */ 9394 @Child(name = "legalState", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 9395 @Description(shortDefinition="Negotiation status", formalDefinition="Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement." ) 9396 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-legalstate") 9397 protected CodeableConcept legalState; 9398 9399 /** 9400 * The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract. 9401 */ 9402 @Child(name = "instantiatesCanonical", type = {Contract.class}, order=5, min=0, max=1, modifier=false, summary=false) 9403 @Description(shortDefinition="Source Contract Definition", formalDefinition="The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract." ) 9404 protected Reference instantiatesCanonical; 9405 9406 /** 9407 * The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract. 9408 */ 9409 @Child(name = "instantiatesUri", type = {UriType.class}, order=6, min=0, max=1, modifier=false, summary=false) 9410 @Description(shortDefinition="External Contract Definition", formalDefinition="The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract." ) 9411 protected UriType instantiatesUri; 9412 9413 /** 9414 * The minimal content derived from the basal information source at a specific stage in its lifecycle. 9415 */ 9416 @Child(name = "contentDerivative", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 9417 @Description(shortDefinition="Content derived from the basal information", formalDefinition="The minimal content derived from the basal information source at a specific stage in its lifecycle." ) 9418 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-content-derivative") 9419 protected CodeableConcept contentDerivative; 9420 9421 /** 9422 * When this Contract was issued. 9423 */ 9424 @Child(name = "issued", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 9425 @Description(shortDefinition="When this Contract was issued", formalDefinition="When this Contract was issued." ) 9426 protected DateTimeType issued; 9427 9428 /** 9429 * Relevant time or time-period when this Contract is applicable. 9430 */ 9431 @Child(name = "applies", type = {Period.class}, order=9, min=0, max=1, modifier=false, summary=true) 9432 @Description(shortDefinition="Effective time", formalDefinition="Relevant time or time-period when this Contract is applicable." ) 9433 protected Period applies; 9434 9435 /** 9436 * Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract. 9437 */ 9438 @Child(name = "expirationType", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 9439 @Description(shortDefinition="Contract cessation cause", formalDefinition="Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract." ) 9440 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-expiration-type") 9441 protected CodeableConcept expirationType; 9442 9443 /** 9444 * The target entity impacted by or of interest to parties to the agreement. 9445 */ 9446 @Child(name = "subject", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 9447 @Description(shortDefinition="Contract Target Entity", formalDefinition="The target entity impacted by or of interest to parties to the agreement." ) 9448 protected List<Reference> subject; 9449 9450 /** 9451 * A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies. 9452 */ 9453 @Child(name = "authority", type = {Organization.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9454 @Description(shortDefinition="Authority under which this Contract has standing", formalDefinition="A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies." ) 9455 protected List<Reference> authority; 9456 9457 /** 9458 * Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources. 9459 */ 9460 @Child(name = "domain", type = {Location.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9461 @Description(shortDefinition="A sphere of control governed by an authoritative jurisdiction, organization, or person", formalDefinition="Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources." ) 9462 protected List<Reference> domain; 9463 9464 /** 9465 * Sites in which the contract is complied with, exercised, or in force. 9466 */ 9467 @Child(name = "site", type = {Location.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9468 @Description(shortDefinition="Specific Location", formalDefinition="Sites in which the contract is complied with, exercised, or in force." ) 9469 protected List<Reference> site; 9470 9471 /** 9472 * A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation. 9473 */ 9474 @Child(name = "name", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=true) 9475 @Description(shortDefinition="Computer friendly designation", formalDefinition="A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 9476 protected StringType name; 9477 9478 /** 9479 * A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state. 9480 */ 9481 @Child(name = "title", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=true) 9482 @Description(shortDefinition="Human Friendly name", formalDefinition="A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state." ) 9483 protected StringType title; 9484 9485 /** 9486 * A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state. 9487 */ 9488 @Child(name = "subtitle", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 9489 @Description(shortDefinition="Subordinate Friendly name", formalDefinition="A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state." ) 9490 protected StringType subtitle; 9491 9492 /** 9493 * Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation. 9494 */ 9495 @Child(name = "alias", type = {StringType.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9496 @Description(shortDefinition="Acronym or short name", formalDefinition="Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation." ) 9497 protected List<StringType> alias; 9498 9499 /** 9500 * The individual or organization that authored the Contract definition, derivative, or instance in any legal state. 9501 */ 9502 @Child(name = "author", type = {Patient.class, Practitioner.class, PractitionerRole.class, Organization.class}, order=19, min=0, max=1, modifier=false, summary=false) 9503 @Description(shortDefinition="Source of Contract", formalDefinition="The individual or organization that authored the Contract definition, derivative, or instance in any legal state." ) 9504 protected Reference author; 9505 9506 /** 9507 * A selector of legal concerns for this Contract definition, derivative, or instance in any legal state. 9508 */ 9509 @Child(name = "scope", type = {CodeableConcept.class}, order=20, min=0, max=1, modifier=false, summary=false) 9510 @Description(shortDefinition="Range of Legal Concerns", formalDefinition="A selector of legal concerns for this Contract definition, derivative, or instance in any legal state." ) 9511 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-scope") 9512 protected CodeableConcept scope; 9513 9514 /** 9515 * Narrows the range of legal concerns to focus on the achievement of specific contractual objectives. 9516 */ 9517 @Child(name = "topic", type = {CodeableConcept.class, Reference.class}, order=21, min=0, max=1, modifier=false, summary=false) 9518 @Description(shortDefinition="Focus of contract interest", formalDefinition="Narrows the range of legal concerns to focus on the achievement of specific contractual objectives." ) 9519 protected DataType topic; 9520 9521 /** 9522 * A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract. 9523 */ 9524 @Child(name = "type", type = {CodeableConcept.class}, order=22, min=0, max=1, modifier=false, summary=true) 9525 @Description(shortDefinition="Legal instrument category", formalDefinition="A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract." ) 9526 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-type") 9527 protected CodeableConcept type; 9528 9529 /** 9530 * Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope. 9531 */ 9532 @Child(name = "subType", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 9533 @Description(shortDefinition="Subtype within the context of type", formalDefinition="Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope." ) 9534 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contract-subtype") 9535 protected List<CodeableConcept> subType; 9536 9537 /** 9538 * Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract. 9539 */ 9540 @Child(name = "contentDefinition", type = {}, order=24, min=0, max=1, modifier=false, summary=false) 9541 @Description(shortDefinition="Contract precursor content", formalDefinition="Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract." ) 9542 protected ContentDefinitionComponent contentDefinition; 9543 9544 /** 9545 * One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups. 9546 */ 9547 @Child(name = "term", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9548 @Description(shortDefinition="Contract Term List", formalDefinition="One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups." ) 9549 protected List<TermComponent> term; 9550 9551 /** 9552 * Information that may be needed by/relevant to the performer in their execution of this term action. 9553 */ 9554 @Child(name = "supportingInfo", type = {Reference.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9555 @Description(shortDefinition="Extra Information", formalDefinition="Information that may be needed by/relevant to the performer in their execution of this term action." ) 9556 protected List<Reference> supportingInfo; 9557 9558 /** 9559 * Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provenance.entity indicates the target that was changed in the update (see [Provenance.entity](provenance-definitions.html#Provenance.entity)). 9560 */ 9561 @Child(name = "relevantHistory", type = {Provenance.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9562 @Description(shortDefinition="Key event in Contract History", formalDefinition="Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provenance.entity indicates the target that was changed in the update (see [Provenance.entity](provenance-definitions.html#Provenance.entity))." ) 9563 protected List<Reference> relevantHistory; 9564 9565 /** 9566 * Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness. 9567 */ 9568 @Child(name = "signer", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9569 @Description(shortDefinition="Contract Signatory", formalDefinition="Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness." ) 9570 protected List<SignatoryComponent> signer; 9571 9572 /** 9573 * The "patient friendly language" versionof the Contract in whole or in parts. "Patient friendly language" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement. 9574 */ 9575 @Child(name = "friendly", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9576 @Description(shortDefinition="Contract Friendly Language", formalDefinition="The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement." ) 9577 protected List<FriendlyLanguageComponent> friendly; 9578 9579 /** 9580 * List of Legal expressions or representations of this Contract. 9581 */ 9582 @Child(name = "legal", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9583 @Description(shortDefinition="Contract Legal Language", formalDefinition="List of Legal expressions or representations of this Contract." ) 9584 protected List<LegalLanguageComponent> legal; 9585 9586 /** 9587 * List of Computable Policy Rule Language Representations of this Contract. 9588 */ 9589 @Child(name = "rule", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9590 @Description(shortDefinition="Computable Contract Language", formalDefinition="List of Computable Policy Rule Language Representations of this Contract." ) 9591 protected List<ComputableLanguageComponent> rule; 9592 9593 /** 9594 * Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract. 9595 */ 9596 @Child(name = "legallyBinding", type = {Attachment.class, Composition.class, DocumentReference.class, QuestionnaireResponse.class, Contract.class}, order=32, min=0, max=1, modifier=false, summary=false) 9597 @Description(shortDefinition="Binding Contract", formalDefinition="Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract." ) 9598 protected DataType legallyBinding; 9599 9600 private static final long serialVersionUID = -792431459L; 9601 9602 /** 9603 * Constructor 9604 */ 9605 public Contract() { 9606 super(); 9607 } 9608 9609 /** 9610 * @return {@link #identifier} (Unique identifier for this Contract or a derivative that references a Source Contract.) 9611 */ 9612 public List<Identifier> getIdentifier() { 9613 if (this.identifier == null) 9614 this.identifier = new ArrayList<Identifier>(); 9615 return this.identifier; 9616 } 9617 9618 /** 9619 * @return Returns a reference to <code>this</code> for easy method chaining 9620 */ 9621 public Contract setIdentifier(List<Identifier> theIdentifier) { 9622 this.identifier = theIdentifier; 9623 return this; 9624 } 9625 9626 public boolean hasIdentifier() { 9627 if (this.identifier == null) 9628 return false; 9629 for (Identifier item : this.identifier) 9630 if (!item.isEmpty()) 9631 return true; 9632 return false; 9633 } 9634 9635 public Identifier addIdentifier() { //3 9636 Identifier t = new Identifier(); 9637 if (this.identifier == null) 9638 this.identifier = new ArrayList<Identifier>(); 9639 this.identifier.add(t); 9640 return t; 9641 } 9642 9643 public Contract addIdentifier(Identifier t) { //3 9644 if (t == null) 9645 return this; 9646 if (this.identifier == null) 9647 this.identifier = new ArrayList<Identifier>(); 9648 this.identifier.add(t); 9649 return this; 9650 } 9651 9652 /** 9653 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 9654 */ 9655 public Identifier getIdentifierFirstRep() { 9656 if (getIdentifier().isEmpty()) { 9657 addIdentifier(); 9658 } 9659 return getIdentifier().get(0); 9660 } 9661 9662 /** 9663 * @return {@link #url} (Canonical identifier for this contract, represented as a URI (globally unique).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 9664 */ 9665 public UriType getUrlElement() { 9666 if (this.url == null) 9667 if (Configuration.errorOnAutoCreate()) 9668 throw new Error("Attempt to auto-create Contract.url"); 9669 else if (Configuration.doAutoCreate()) 9670 this.url = new UriType(); // bb 9671 return this.url; 9672 } 9673 9674 public boolean hasUrlElement() { 9675 return this.url != null && !this.url.isEmpty(); 9676 } 9677 9678 public boolean hasUrl() { 9679 return this.url != null && !this.url.isEmpty(); 9680 } 9681 9682 /** 9683 * @param value {@link #url} (Canonical identifier for this contract, represented as a URI (globally unique).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 9684 */ 9685 public Contract setUrlElement(UriType value) { 9686 this.url = value; 9687 return this; 9688 } 9689 9690 /** 9691 * @return Canonical identifier for this contract, represented as a URI (globally unique). 9692 */ 9693 public String getUrl() { 9694 return this.url == null ? null : this.url.getValue(); 9695 } 9696 9697 /** 9698 * @param value Canonical identifier for this contract, represented as a URI (globally unique). 9699 */ 9700 public Contract setUrl(String value) { 9701 if (Utilities.noString(value)) 9702 this.url = null; 9703 else { 9704 if (this.url == null) 9705 this.url = new UriType(); 9706 this.url.setValue(value); 9707 } 9708 return this; 9709 } 9710 9711 /** 9712 * @return {@link #version} (An edition identifier used for business purposes to label business significant variants.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 9713 */ 9714 public StringType getVersionElement() { 9715 if (this.version == null) 9716 if (Configuration.errorOnAutoCreate()) 9717 throw new Error("Attempt to auto-create Contract.version"); 9718 else if (Configuration.doAutoCreate()) 9719 this.version = new StringType(); // bb 9720 return this.version; 9721 } 9722 9723 public boolean hasVersionElement() { 9724 return this.version != null && !this.version.isEmpty(); 9725 } 9726 9727 public boolean hasVersion() { 9728 return this.version != null && !this.version.isEmpty(); 9729 } 9730 9731 /** 9732 * @param value {@link #version} (An edition identifier used for business purposes to label business significant variants.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 9733 */ 9734 public Contract setVersionElement(StringType value) { 9735 this.version = value; 9736 return this; 9737 } 9738 9739 /** 9740 * @return An edition identifier used for business purposes to label business significant variants. 9741 */ 9742 public String getVersion() { 9743 return this.version == null ? null : this.version.getValue(); 9744 } 9745 9746 /** 9747 * @param value An edition identifier used for business purposes to label business significant variants. 9748 */ 9749 public Contract setVersion(String value) { 9750 if (Utilities.noString(value)) 9751 this.version = null; 9752 else { 9753 if (this.version == null) 9754 this.version = new StringType(); 9755 this.version.setValue(value); 9756 } 9757 return this; 9758 } 9759 9760 /** 9761 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 9762 */ 9763 public Enumeration<ContractResourceStatusCodes> getStatusElement() { 9764 if (this.status == null) 9765 if (Configuration.errorOnAutoCreate()) 9766 throw new Error("Attempt to auto-create Contract.status"); 9767 else if (Configuration.doAutoCreate()) 9768 this.status = new Enumeration<ContractResourceStatusCodes>(new ContractResourceStatusCodesEnumFactory()); // bb 9769 return this.status; 9770 } 9771 9772 public boolean hasStatusElement() { 9773 return this.status != null && !this.status.isEmpty(); 9774 } 9775 9776 public boolean hasStatus() { 9777 return this.status != null && !this.status.isEmpty(); 9778 } 9779 9780 /** 9781 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 9782 */ 9783 public Contract setStatusElement(Enumeration<ContractResourceStatusCodes> value) { 9784 this.status = value; 9785 return this; 9786 } 9787 9788 /** 9789 * @return The status of the resource instance. 9790 */ 9791 public ContractResourceStatusCodes getStatus() { 9792 return this.status == null ? null : this.status.getValue(); 9793 } 9794 9795 /** 9796 * @param value The status of the resource instance. 9797 */ 9798 public Contract setStatus(ContractResourceStatusCodes value) { 9799 if (value == null) 9800 this.status = null; 9801 else { 9802 if (this.status == null) 9803 this.status = new Enumeration<ContractResourceStatusCodes>(new ContractResourceStatusCodesEnumFactory()); 9804 this.status.setValue(value); 9805 } 9806 return this; 9807 } 9808 9809 /** 9810 * @return {@link #legalState} (Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.) 9811 */ 9812 public CodeableConcept getLegalState() { 9813 if (this.legalState == null) 9814 if (Configuration.errorOnAutoCreate()) 9815 throw new Error("Attempt to auto-create Contract.legalState"); 9816 else if (Configuration.doAutoCreate()) 9817 this.legalState = new CodeableConcept(); // cc 9818 return this.legalState; 9819 } 9820 9821 public boolean hasLegalState() { 9822 return this.legalState != null && !this.legalState.isEmpty(); 9823 } 9824 9825 /** 9826 * @param value {@link #legalState} (Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.) 9827 */ 9828 public Contract setLegalState(CodeableConcept value) { 9829 this.legalState = value; 9830 return this; 9831 } 9832 9833 /** 9834 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.) 9835 */ 9836 public Reference getInstantiatesCanonical() { 9837 if (this.instantiatesCanonical == null) 9838 if (Configuration.errorOnAutoCreate()) 9839 throw new Error("Attempt to auto-create Contract.instantiatesCanonical"); 9840 else if (Configuration.doAutoCreate()) 9841 this.instantiatesCanonical = new Reference(); // cc 9842 return this.instantiatesCanonical; 9843 } 9844 9845 public boolean hasInstantiatesCanonical() { 9846 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 9847 } 9848 9849 /** 9850 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.) 9851 */ 9852 public Contract setInstantiatesCanonical(Reference value) { 9853 this.instantiatesCanonical = value; 9854 return this; 9855 } 9856 9857 /** 9858 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 9859 */ 9860 public UriType getInstantiatesUriElement() { 9861 if (this.instantiatesUri == null) 9862 if (Configuration.errorOnAutoCreate()) 9863 throw new Error("Attempt to auto-create Contract.instantiatesUri"); 9864 else if (Configuration.doAutoCreate()) 9865 this.instantiatesUri = new UriType(); // bb 9866 return this.instantiatesUri; 9867 } 9868 9869 public boolean hasInstantiatesUriElement() { 9870 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 9871 } 9872 9873 public boolean hasInstantiatesUri() { 9874 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 9875 } 9876 9877 /** 9878 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 9879 */ 9880 public Contract setInstantiatesUriElement(UriType value) { 9881 this.instantiatesUri = value; 9882 return this; 9883 } 9884 9885 /** 9886 * @return The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract. 9887 */ 9888 public String getInstantiatesUri() { 9889 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 9890 } 9891 9892 /** 9893 * @param value The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract. 9894 */ 9895 public Contract setInstantiatesUri(String value) { 9896 if (Utilities.noString(value)) 9897 this.instantiatesUri = null; 9898 else { 9899 if (this.instantiatesUri == null) 9900 this.instantiatesUri = new UriType(); 9901 this.instantiatesUri.setValue(value); 9902 } 9903 return this; 9904 } 9905 9906 /** 9907 * @return {@link #contentDerivative} (The minimal content derived from the basal information source at a specific stage in its lifecycle.) 9908 */ 9909 public CodeableConcept getContentDerivative() { 9910 if (this.contentDerivative == null) 9911 if (Configuration.errorOnAutoCreate()) 9912 throw new Error("Attempt to auto-create Contract.contentDerivative"); 9913 else if (Configuration.doAutoCreate()) 9914 this.contentDerivative = new CodeableConcept(); // cc 9915 return this.contentDerivative; 9916 } 9917 9918 public boolean hasContentDerivative() { 9919 return this.contentDerivative != null && !this.contentDerivative.isEmpty(); 9920 } 9921 9922 /** 9923 * @param value {@link #contentDerivative} (The minimal content derived from the basal information source at a specific stage in its lifecycle.) 9924 */ 9925 public Contract setContentDerivative(CodeableConcept value) { 9926 this.contentDerivative = value; 9927 return this; 9928 } 9929 9930 /** 9931 * @return {@link #issued} (When this Contract was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 9932 */ 9933 public DateTimeType getIssuedElement() { 9934 if (this.issued == null) 9935 if (Configuration.errorOnAutoCreate()) 9936 throw new Error("Attempt to auto-create Contract.issued"); 9937 else if (Configuration.doAutoCreate()) 9938 this.issued = new DateTimeType(); // bb 9939 return this.issued; 9940 } 9941 9942 public boolean hasIssuedElement() { 9943 return this.issued != null && !this.issued.isEmpty(); 9944 } 9945 9946 public boolean hasIssued() { 9947 return this.issued != null && !this.issued.isEmpty(); 9948 } 9949 9950 /** 9951 * @param value {@link #issued} (When this Contract was issued.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 9952 */ 9953 public Contract setIssuedElement(DateTimeType value) { 9954 this.issued = value; 9955 return this; 9956 } 9957 9958 /** 9959 * @return When this Contract was issued. 9960 */ 9961 public Date getIssued() { 9962 return this.issued == null ? null : this.issued.getValue(); 9963 } 9964 9965 /** 9966 * @param value When this Contract was issued. 9967 */ 9968 public Contract setIssued(Date value) { 9969 if (value == null) 9970 this.issued = null; 9971 else { 9972 if (this.issued == null) 9973 this.issued = new DateTimeType(); 9974 this.issued.setValue(value); 9975 } 9976 return this; 9977 } 9978 9979 /** 9980 * @return {@link #applies} (Relevant time or time-period when this Contract is applicable.) 9981 */ 9982 public Period getApplies() { 9983 if (this.applies == null) 9984 if (Configuration.errorOnAutoCreate()) 9985 throw new Error("Attempt to auto-create Contract.applies"); 9986 else if (Configuration.doAutoCreate()) 9987 this.applies = new Period(); // cc 9988 return this.applies; 9989 } 9990 9991 public boolean hasApplies() { 9992 return this.applies != null && !this.applies.isEmpty(); 9993 } 9994 9995 /** 9996 * @param value {@link #applies} (Relevant time or time-period when this Contract is applicable.) 9997 */ 9998 public Contract setApplies(Period value) { 9999 this.applies = value; 10000 return this; 10001 } 10002 10003 /** 10004 * @return {@link #expirationType} (Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.) 10005 */ 10006 public CodeableConcept getExpirationType() { 10007 if (this.expirationType == null) 10008 if (Configuration.errorOnAutoCreate()) 10009 throw new Error("Attempt to auto-create Contract.expirationType"); 10010 else if (Configuration.doAutoCreate()) 10011 this.expirationType = new CodeableConcept(); // cc 10012 return this.expirationType; 10013 } 10014 10015 public boolean hasExpirationType() { 10016 return this.expirationType != null && !this.expirationType.isEmpty(); 10017 } 10018 10019 /** 10020 * @param value {@link #expirationType} (Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.) 10021 */ 10022 public Contract setExpirationType(CodeableConcept value) { 10023 this.expirationType = value; 10024 return this; 10025 } 10026 10027 /** 10028 * @return {@link #subject} (The target entity impacted by or of interest to parties to the agreement.) 10029 */ 10030 public List<Reference> getSubject() { 10031 if (this.subject == null) 10032 this.subject = new ArrayList<Reference>(); 10033 return this.subject; 10034 } 10035 10036 /** 10037 * @return Returns a reference to <code>this</code> for easy method chaining 10038 */ 10039 public Contract setSubject(List<Reference> theSubject) { 10040 this.subject = theSubject; 10041 return this; 10042 } 10043 10044 public boolean hasSubject() { 10045 if (this.subject == null) 10046 return false; 10047 for (Reference item : this.subject) 10048 if (!item.isEmpty()) 10049 return true; 10050 return false; 10051 } 10052 10053 public Reference addSubject() { //3 10054 Reference t = new Reference(); 10055 if (this.subject == null) 10056 this.subject = new ArrayList<Reference>(); 10057 this.subject.add(t); 10058 return t; 10059 } 10060 10061 public Contract addSubject(Reference t) { //3 10062 if (t == null) 10063 return this; 10064 if (this.subject == null) 10065 this.subject = new ArrayList<Reference>(); 10066 this.subject.add(t); 10067 return this; 10068 } 10069 10070 /** 10071 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 10072 */ 10073 public Reference getSubjectFirstRep() { 10074 if (getSubject().isEmpty()) { 10075 addSubject(); 10076 } 10077 return getSubject().get(0); 10078 } 10079 10080 /** 10081 * @return {@link #authority} (A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.) 10082 */ 10083 public List<Reference> getAuthority() { 10084 if (this.authority == null) 10085 this.authority = new ArrayList<Reference>(); 10086 return this.authority; 10087 } 10088 10089 /** 10090 * @return Returns a reference to <code>this</code> for easy method chaining 10091 */ 10092 public Contract setAuthority(List<Reference> theAuthority) { 10093 this.authority = theAuthority; 10094 return this; 10095 } 10096 10097 public boolean hasAuthority() { 10098 if (this.authority == null) 10099 return false; 10100 for (Reference item : this.authority) 10101 if (!item.isEmpty()) 10102 return true; 10103 return false; 10104 } 10105 10106 public Reference addAuthority() { //3 10107 Reference t = new Reference(); 10108 if (this.authority == null) 10109 this.authority = new ArrayList<Reference>(); 10110 this.authority.add(t); 10111 return t; 10112 } 10113 10114 public Contract addAuthority(Reference t) { //3 10115 if (t == null) 10116 return this; 10117 if (this.authority == null) 10118 this.authority = new ArrayList<Reference>(); 10119 this.authority.add(t); 10120 return this; 10121 } 10122 10123 /** 10124 * @return The first repetition of repeating field {@link #authority}, creating it if it does not already exist {3} 10125 */ 10126 public Reference getAuthorityFirstRep() { 10127 if (getAuthority().isEmpty()) { 10128 addAuthority(); 10129 } 10130 return getAuthority().get(0); 10131 } 10132 10133 /** 10134 * @return {@link #domain} (Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.) 10135 */ 10136 public List<Reference> getDomain() { 10137 if (this.domain == null) 10138 this.domain = new ArrayList<Reference>(); 10139 return this.domain; 10140 } 10141 10142 /** 10143 * @return Returns a reference to <code>this</code> for easy method chaining 10144 */ 10145 public Contract setDomain(List<Reference> theDomain) { 10146 this.domain = theDomain; 10147 return this; 10148 } 10149 10150 public boolean hasDomain() { 10151 if (this.domain == null) 10152 return false; 10153 for (Reference item : this.domain) 10154 if (!item.isEmpty()) 10155 return true; 10156 return false; 10157 } 10158 10159 public Reference addDomain() { //3 10160 Reference t = new Reference(); 10161 if (this.domain == null) 10162 this.domain = new ArrayList<Reference>(); 10163 this.domain.add(t); 10164 return t; 10165 } 10166 10167 public Contract addDomain(Reference t) { //3 10168 if (t == null) 10169 return this; 10170 if (this.domain == null) 10171 this.domain = new ArrayList<Reference>(); 10172 this.domain.add(t); 10173 return this; 10174 } 10175 10176 /** 10177 * @return The first repetition of repeating field {@link #domain}, creating it if it does not already exist {3} 10178 */ 10179 public Reference getDomainFirstRep() { 10180 if (getDomain().isEmpty()) { 10181 addDomain(); 10182 } 10183 return getDomain().get(0); 10184 } 10185 10186 /** 10187 * @return {@link #site} (Sites in which the contract is complied with, exercised, or in force.) 10188 */ 10189 public List<Reference> getSite() { 10190 if (this.site == null) 10191 this.site = new ArrayList<Reference>(); 10192 return this.site; 10193 } 10194 10195 /** 10196 * @return Returns a reference to <code>this</code> for easy method chaining 10197 */ 10198 public Contract setSite(List<Reference> theSite) { 10199 this.site = theSite; 10200 return this; 10201 } 10202 10203 public boolean hasSite() { 10204 if (this.site == null) 10205 return false; 10206 for (Reference item : this.site) 10207 if (!item.isEmpty()) 10208 return true; 10209 return false; 10210 } 10211 10212 public Reference addSite() { //3 10213 Reference t = new Reference(); 10214 if (this.site == null) 10215 this.site = new ArrayList<Reference>(); 10216 this.site.add(t); 10217 return t; 10218 } 10219 10220 public Contract addSite(Reference t) { //3 10221 if (t == null) 10222 return this; 10223 if (this.site == null) 10224 this.site = new ArrayList<Reference>(); 10225 this.site.add(t); 10226 return this; 10227 } 10228 10229 /** 10230 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 10231 */ 10232 public Reference getSiteFirstRep() { 10233 if (getSite().isEmpty()) { 10234 addSite(); 10235 } 10236 return getSite().get(0); 10237 } 10238 10239 /** 10240 * @return {@link #name} (A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10241 */ 10242 public StringType getNameElement() { 10243 if (this.name == null) 10244 if (Configuration.errorOnAutoCreate()) 10245 throw new Error("Attempt to auto-create Contract.name"); 10246 else if (Configuration.doAutoCreate()) 10247 this.name = new StringType(); // bb 10248 return this.name; 10249 } 10250 10251 public boolean hasNameElement() { 10252 return this.name != null && !this.name.isEmpty(); 10253 } 10254 10255 public boolean hasName() { 10256 return this.name != null && !this.name.isEmpty(); 10257 } 10258 10259 /** 10260 * @param value {@link #name} (A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10261 */ 10262 public Contract setNameElement(StringType value) { 10263 this.name = value; 10264 return this; 10265 } 10266 10267 /** 10268 * @return A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation. 10269 */ 10270 public String getName() { 10271 return this.name == null ? null : this.name.getValue(); 10272 } 10273 10274 /** 10275 * @param value A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation. 10276 */ 10277 public Contract setName(String value) { 10278 if (Utilities.noString(value)) 10279 this.name = null; 10280 else { 10281 if (this.name == null) 10282 this.name = new StringType(); 10283 this.name.setValue(value); 10284 } 10285 return this; 10286 } 10287 10288 /** 10289 * @return {@link #title} (A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 10290 */ 10291 public StringType getTitleElement() { 10292 if (this.title == null) 10293 if (Configuration.errorOnAutoCreate()) 10294 throw new Error("Attempt to auto-create Contract.title"); 10295 else if (Configuration.doAutoCreate()) 10296 this.title = new StringType(); // bb 10297 return this.title; 10298 } 10299 10300 public boolean hasTitleElement() { 10301 return this.title != null && !this.title.isEmpty(); 10302 } 10303 10304 public boolean hasTitle() { 10305 return this.title != null && !this.title.isEmpty(); 10306 } 10307 10308 /** 10309 * @param value {@link #title} (A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 10310 */ 10311 public Contract setTitleElement(StringType value) { 10312 this.title = value; 10313 return this; 10314 } 10315 10316 /** 10317 * @return A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state. 10318 */ 10319 public String getTitle() { 10320 return this.title == null ? null : this.title.getValue(); 10321 } 10322 10323 /** 10324 * @param value A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state. 10325 */ 10326 public Contract setTitle(String value) { 10327 if (Utilities.noString(value)) 10328 this.title = null; 10329 else { 10330 if (this.title == null) 10331 this.title = new StringType(); 10332 this.title.setValue(value); 10333 } 10334 return this; 10335 } 10336 10337 /** 10338 * @return {@link #subtitle} (A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 10339 */ 10340 public StringType getSubtitleElement() { 10341 if (this.subtitle == null) 10342 if (Configuration.errorOnAutoCreate()) 10343 throw new Error("Attempt to auto-create Contract.subtitle"); 10344 else if (Configuration.doAutoCreate()) 10345 this.subtitle = new StringType(); // bb 10346 return this.subtitle; 10347 } 10348 10349 public boolean hasSubtitleElement() { 10350 return this.subtitle != null && !this.subtitle.isEmpty(); 10351 } 10352 10353 public boolean hasSubtitle() { 10354 return this.subtitle != null && !this.subtitle.isEmpty(); 10355 } 10356 10357 /** 10358 * @param value {@link #subtitle} (A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 10359 */ 10360 public Contract setSubtitleElement(StringType value) { 10361 this.subtitle = value; 10362 return this; 10363 } 10364 10365 /** 10366 * @return A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state. 10367 */ 10368 public String getSubtitle() { 10369 return this.subtitle == null ? null : this.subtitle.getValue(); 10370 } 10371 10372 /** 10373 * @param value A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state. 10374 */ 10375 public Contract setSubtitle(String value) { 10376 if (Utilities.noString(value)) 10377 this.subtitle = null; 10378 else { 10379 if (this.subtitle == null) 10380 this.subtitle = new StringType(); 10381 this.subtitle.setValue(value); 10382 } 10383 return this; 10384 } 10385 10386 /** 10387 * @return {@link #alias} (Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.) 10388 */ 10389 public List<StringType> getAlias() { 10390 if (this.alias == null) 10391 this.alias = new ArrayList<StringType>(); 10392 return this.alias; 10393 } 10394 10395 /** 10396 * @return Returns a reference to <code>this</code> for easy method chaining 10397 */ 10398 public Contract setAlias(List<StringType> theAlias) { 10399 this.alias = theAlias; 10400 return this; 10401 } 10402 10403 public boolean hasAlias() { 10404 if (this.alias == null) 10405 return false; 10406 for (StringType item : this.alias) 10407 if (!item.isEmpty()) 10408 return true; 10409 return false; 10410 } 10411 10412 /** 10413 * @return {@link #alias} (Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.) 10414 */ 10415 public StringType addAliasElement() {//2 10416 StringType t = new StringType(); 10417 if (this.alias == null) 10418 this.alias = new ArrayList<StringType>(); 10419 this.alias.add(t); 10420 return t; 10421 } 10422 10423 /** 10424 * @param value {@link #alias} (Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.) 10425 */ 10426 public Contract addAlias(String value) { //1 10427 StringType t = new StringType(); 10428 t.setValue(value); 10429 if (this.alias == null) 10430 this.alias = new ArrayList<StringType>(); 10431 this.alias.add(t); 10432 return this; 10433 } 10434 10435 /** 10436 * @param value {@link #alias} (Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.) 10437 */ 10438 public boolean hasAlias(String value) { 10439 if (this.alias == null) 10440 return false; 10441 for (StringType v : this.alias) 10442 if (v.getValue().equals(value)) // string 10443 return true; 10444 return false; 10445 } 10446 10447 /** 10448 * @return {@link #author} (The individual or organization that authored the Contract definition, derivative, or instance in any legal state.) 10449 */ 10450 public Reference getAuthor() { 10451 if (this.author == null) 10452 if (Configuration.errorOnAutoCreate()) 10453 throw new Error("Attempt to auto-create Contract.author"); 10454 else if (Configuration.doAutoCreate()) 10455 this.author = new Reference(); // cc 10456 return this.author; 10457 } 10458 10459 public boolean hasAuthor() { 10460 return this.author != null && !this.author.isEmpty(); 10461 } 10462 10463 /** 10464 * @param value {@link #author} (The individual or organization that authored the Contract definition, derivative, or instance in any legal state.) 10465 */ 10466 public Contract setAuthor(Reference value) { 10467 this.author = value; 10468 return this; 10469 } 10470 10471 /** 10472 * @return {@link #scope} (A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.) 10473 */ 10474 public CodeableConcept getScope() { 10475 if (this.scope == null) 10476 if (Configuration.errorOnAutoCreate()) 10477 throw new Error("Attempt to auto-create Contract.scope"); 10478 else if (Configuration.doAutoCreate()) 10479 this.scope = new CodeableConcept(); // cc 10480 return this.scope; 10481 } 10482 10483 public boolean hasScope() { 10484 return this.scope != null && !this.scope.isEmpty(); 10485 } 10486 10487 /** 10488 * @param value {@link #scope} (A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.) 10489 */ 10490 public Contract setScope(CodeableConcept value) { 10491 this.scope = value; 10492 return this; 10493 } 10494 10495 /** 10496 * @return {@link #topic} (Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.) 10497 */ 10498 public DataType getTopic() { 10499 return this.topic; 10500 } 10501 10502 /** 10503 * @return {@link #topic} (Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.) 10504 */ 10505 public CodeableConcept getTopicCodeableConcept() throws FHIRException { 10506 if (this.topic == null) 10507 this.topic = new CodeableConcept(); 10508 if (!(this.topic instanceof CodeableConcept)) 10509 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.topic.getClass().getName()+" was encountered"); 10510 return (CodeableConcept) this.topic; 10511 } 10512 10513 public boolean hasTopicCodeableConcept() { 10514 return this != null && this.topic instanceof CodeableConcept; 10515 } 10516 10517 /** 10518 * @return {@link #topic} (Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.) 10519 */ 10520 public Reference getTopicReference() throws FHIRException { 10521 if (this.topic == null) 10522 this.topic = new Reference(); 10523 if (!(this.topic instanceof Reference)) 10524 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.topic.getClass().getName()+" was encountered"); 10525 return (Reference) this.topic; 10526 } 10527 10528 public boolean hasTopicReference() { 10529 return this != null && this.topic instanceof Reference; 10530 } 10531 10532 public boolean hasTopic() { 10533 return this.topic != null && !this.topic.isEmpty(); 10534 } 10535 10536 /** 10537 * @param value {@link #topic} (Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.) 10538 */ 10539 public Contract setTopic(DataType value) { 10540 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 10541 throw new FHIRException("Not the right type for Contract.topic[x]: "+value.fhirType()); 10542 this.topic = value; 10543 return this; 10544 } 10545 10546 /** 10547 * @return {@link #type} (A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.) 10548 */ 10549 public CodeableConcept getType() { 10550 if (this.type == null) 10551 if (Configuration.errorOnAutoCreate()) 10552 throw new Error("Attempt to auto-create Contract.type"); 10553 else if (Configuration.doAutoCreate()) 10554 this.type = new CodeableConcept(); // cc 10555 return this.type; 10556 } 10557 10558 public boolean hasType() { 10559 return this.type != null && !this.type.isEmpty(); 10560 } 10561 10562 /** 10563 * @param value {@link #type} (A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.) 10564 */ 10565 public Contract setType(CodeableConcept value) { 10566 this.type = value; 10567 return this; 10568 } 10569 10570 /** 10571 * @return {@link #subType} (Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.) 10572 */ 10573 public List<CodeableConcept> getSubType() { 10574 if (this.subType == null) 10575 this.subType = new ArrayList<CodeableConcept>(); 10576 return this.subType; 10577 } 10578 10579 /** 10580 * @return Returns a reference to <code>this</code> for easy method chaining 10581 */ 10582 public Contract setSubType(List<CodeableConcept> theSubType) { 10583 this.subType = theSubType; 10584 return this; 10585 } 10586 10587 public boolean hasSubType() { 10588 if (this.subType == null) 10589 return false; 10590 for (CodeableConcept item : this.subType) 10591 if (!item.isEmpty()) 10592 return true; 10593 return false; 10594 } 10595 10596 public CodeableConcept addSubType() { //3 10597 CodeableConcept t = new CodeableConcept(); 10598 if (this.subType == null) 10599 this.subType = new ArrayList<CodeableConcept>(); 10600 this.subType.add(t); 10601 return t; 10602 } 10603 10604 public Contract addSubType(CodeableConcept t) { //3 10605 if (t == null) 10606 return this; 10607 if (this.subType == null) 10608 this.subType = new ArrayList<CodeableConcept>(); 10609 this.subType.add(t); 10610 return this; 10611 } 10612 10613 /** 10614 * @return The first repetition of repeating field {@link #subType}, creating it if it does not already exist {3} 10615 */ 10616 public CodeableConcept getSubTypeFirstRep() { 10617 if (getSubType().isEmpty()) { 10618 addSubType(); 10619 } 10620 return getSubType().get(0); 10621 } 10622 10623 /** 10624 * @return {@link #contentDefinition} (Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.) 10625 */ 10626 public ContentDefinitionComponent getContentDefinition() { 10627 if (this.contentDefinition == null) 10628 if (Configuration.errorOnAutoCreate()) 10629 throw new Error("Attempt to auto-create Contract.contentDefinition"); 10630 else if (Configuration.doAutoCreate()) 10631 this.contentDefinition = new ContentDefinitionComponent(); // cc 10632 return this.contentDefinition; 10633 } 10634 10635 public boolean hasContentDefinition() { 10636 return this.contentDefinition != null && !this.contentDefinition.isEmpty(); 10637 } 10638 10639 /** 10640 * @param value {@link #contentDefinition} (Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.) 10641 */ 10642 public Contract setContentDefinition(ContentDefinitionComponent value) { 10643 this.contentDefinition = value; 10644 return this; 10645 } 10646 10647 /** 10648 * @return {@link #term} (One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.) 10649 */ 10650 public List<TermComponent> getTerm() { 10651 if (this.term == null) 10652 this.term = new ArrayList<TermComponent>(); 10653 return this.term; 10654 } 10655 10656 /** 10657 * @return Returns a reference to <code>this</code> for easy method chaining 10658 */ 10659 public Contract setTerm(List<TermComponent> theTerm) { 10660 this.term = theTerm; 10661 return this; 10662 } 10663 10664 public boolean hasTerm() { 10665 if (this.term == null) 10666 return false; 10667 for (TermComponent item : this.term) 10668 if (!item.isEmpty()) 10669 return true; 10670 return false; 10671 } 10672 10673 public TermComponent addTerm() { //3 10674 TermComponent t = new TermComponent(); 10675 if (this.term == null) 10676 this.term = new ArrayList<TermComponent>(); 10677 this.term.add(t); 10678 return t; 10679 } 10680 10681 public Contract addTerm(TermComponent t) { //3 10682 if (t == null) 10683 return this; 10684 if (this.term == null) 10685 this.term = new ArrayList<TermComponent>(); 10686 this.term.add(t); 10687 return this; 10688 } 10689 10690 /** 10691 * @return The first repetition of repeating field {@link #term}, creating it if it does not already exist {3} 10692 */ 10693 public TermComponent getTermFirstRep() { 10694 if (getTerm().isEmpty()) { 10695 addTerm(); 10696 } 10697 return getTerm().get(0); 10698 } 10699 10700 /** 10701 * @return {@link #supportingInfo} (Information that may be needed by/relevant to the performer in their execution of this term action.) 10702 */ 10703 public List<Reference> getSupportingInfo() { 10704 if (this.supportingInfo == null) 10705 this.supportingInfo = new ArrayList<Reference>(); 10706 return this.supportingInfo; 10707 } 10708 10709 /** 10710 * @return Returns a reference to <code>this</code> for easy method chaining 10711 */ 10712 public Contract setSupportingInfo(List<Reference> theSupportingInfo) { 10713 this.supportingInfo = theSupportingInfo; 10714 return this; 10715 } 10716 10717 public boolean hasSupportingInfo() { 10718 if (this.supportingInfo == null) 10719 return false; 10720 for (Reference item : this.supportingInfo) 10721 if (!item.isEmpty()) 10722 return true; 10723 return false; 10724 } 10725 10726 public Reference addSupportingInfo() { //3 10727 Reference t = new Reference(); 10728 if (this.supportingInfo == null) 10729 this.supportingInfo = new ArrayList<Reference>(); 10730 this.supportingInfo.add(t); 10731 return t; 10732 } 10733 10734 public Contract addSupportingInfo(Reference t) { //3 10735 if (t == null) 10736 return this; 10737 if (this.supportingInfo == null) 10738 this.supportingInfo = new ArrayList<Reference>(); 10739 this.supportingInfo.add(t); 10740 return this; 10741 } 10742 10743 /** 10744 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 10745 */ 10746 public Reference getSupportingInfoFirstRep() { 10747 if (getSupportingInfo().isEmpty()) { 10748 addSupportingInfo(); 10749 } 10750 return getSupportingInfo().get(0); 10751 } 10752 10753 /** 10754 * @return {@link #relevantHistory} (Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provenance.entity indicates the target that was changed in the update (see [Provenance.entity](provenance-definitions.html#Provenance.entity)).) 10755 */ 10756 public List<Reference> getRelevantHistory() { 10757 if (this.relevantHistory == null) 10758 this.relevantHistory = new ArrayList<Reference>(); 10759 return this.relevantHistory; 10760 } 10761 10762 /** 10763 * @return Returns a reference to <code>this</code> for easy method chaining 10764 */ 10765 public Contract setRelevantHistory(List<Reference> theRelevantHistory) { 10766 this.relevantHistory = theRelevantHistory; 10767 return this; 10768 } 10769 10770 public boolean hasRelevantHistory() { 10771 if (this.relevantHistory == null) 10772 return false; 10773 for (Reference item : this.relevantHistory) 10774 if (!item.isEmpty()) 10775 return true; 10776 return false; 10777 } 10778 10779 public Reference addRelevantHistory() { //3 10780 Reference t = new Reference(); 10781 if (this.relevantHistory == null) 10782 this.relevantHistory = new ArrayList<Reference>(); 10783 this.relevantHistory.add(t); 10784 return t; 10785 } 10786 10787 public Contract addRelevantHistory(Reference t) { //3 10788 if (t == null) 10789 return this; 10790 if (this.relevantHistory == null) 10791 this.relevantHistory = new ArrayList<Reference>(); 10792 this.relevantHistory.add(t); 10793 return this; 10794 } 10795 10796 /** 10797 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist {3} 10798 */ 10799 public Reference getRelevantHistoryFirstRep() { 10800 if (getRelevantHistory().isEmpty()) { 10801 addRelevantHistory(); 10802 } 10803 return getRelevantHistory().get(0); 10804 } 10805 10806 /** 10807 * @return {@link #signer} (Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.) 10808 */ 10809 public List<SignatoryComponent> getSigner() { 10810 if (this.signer == null) 10811 this.signer = new ArrayList<SignatoryComponent>(); 10812 return this.signer; 10813 } 10814 10815 /** 10816 * @return Returns a reference to <code>this</code> for easy method chaining 10817 */ 10818 public Contract setSigner(List<SignatoryComponent> theSigner) { 10819 this.signer = theSigner; 10820 return this; 10821 } 10822 10823 public boolean hasSigner() { 10824 if (this.signer == null) 10825 return false; 10826 for (SignatoryComponent item : this.signer) 10827 if (!item.isEmpty()) 10828 return true; 10829 return false; 10830 } 10831 10832 public SignatoryComponent addSigner() { //3 10833 SignatoryComponent t = new SignatoryComponent(); 10834 if (this.signer == null) 10835 this.signer = new ArrayList<SignatoryComponent>(); 10836 this.signer.add(t); 10837 return t; 10838 } 10839 10840 public Contract addSigner(SignatoryComponent t) { //3 10841 if (t == null) 10842 return this; 10843 if (this.signer == null) 10844 this.signer = new ArrayList<SignatoryComponent>(); 10845 this.signer.add(t); 10846 return this; 10847 } 10848 10849 /** 10850 * @return The first repetition of repeating field {@link #signer}, creating it if it does not already exist {3} 10851 */ 10852 public SignatoryComponent getSignerFirstRep() { 10853 if (getSigner().isEmpty()) { 10854 addSigner(); 10855 } 10856 return getSigner().get(0); 10857 } 10858 10859 /** 10860 * @return {@link #friendly} (The "patient friendly language" versionof the Contract in whole or in parts. "Patient friendly language" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.) 10861 */ 10862 public List<FriendlyLanguageComponent> getFriendly() { 10863 if (this.friendly == null) 10864 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 10865 return this.friendly; 10866 } 10867 10868 /** 10869 * @return Returns a reference to <code>this</code> for easy method chaining 10870 */ 10871 public Contract setFriendly(List<FriendlyLanguageComponent> theFriendly) { 10872 this.friendly = theFriendly; 10873 return this; 10874 } 10875 10876 public boolean hasFriendly() { 10877 if (this.friendly == null) 10878 return false; 10879 for (FriendlyLanguageComponent item : this.friendly) 10880 if (!item.isEmpty()) 10881 return true; 10882 return false; 10883 } 10884 10885 public FriendlyLanguageComponent addFriendly() { //3 10886 FriendlyLanguageComponent t = new FriendlyLanguageComponent(); 10887 if (this.friendly == null) 10888 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 10889 this.friendly.add(t); 10890 return t; 10891 } 10892 10893 public Contract addFriendly(FriendlyLanguageComponent t) { //3 10894 if (t == null) 10895 return this; 10896 if (this.friendly == null) 10897 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 10898 this.friendly.add(t); 10899 return this; 10900 } 10901 10902 /** 10903 * @return The first repetition of repeating field {@link #friendly}, creating it if it does not already exist {3} 10904 */ 10905 public FriendlyLanguageComponent getFriendlyFirstRep() { 10906 if (getFriendly().isEmpty()) { 10907 addFriendly(); 10908 } 10909 return getFriendly().get(0); 10910 } 10911 10912 /** 10913 * @return {@link #legal} (List of Legal expressions or representations of this Contract.) 10914 */ 10915 public List<LegalLanguageComponent> getLegal() { 10916 if (this.legal == null) 10917 this.legal = new ArrayList<LegalLanguageComponent>(); 10918 return this.legal; 10919 } 10920 10921 /** 10922 * @return Returns a reference to <code>this</code> for easy method chaining 10923 */ 10924 public Contract setLegal(List<LegalLanguageComponent> theLegal) { 10925 this.legal = theLegal; 10926 return this; 10927 } 10928 10929 public boolean hasLegal() { 10930 if (this.legal == null) 10931 return false; 10932 for (LegalLanguageComponent item : this.legal) 10933 if (!item.isEmpty()) 10934 return true; 10935 return false; 10936 } 10937 10938 public LegalLanguageComponent addLegal() { //3 10939 LegalLanguageComponent t = new LegalLanguageComponent(); 10940 if (this.legal == null) 10941 this.legal = new ArrayList<LegalLanguageComponent>(); 10942 this.legal.add(t); 10943 return t; 10944 } 10945 10946 public Contract addLegal(LegalLanguageComponent t) { //3 10947 if (t == null) 10948 return this; 10949 if (this.legal == null) 10950 this.legal = new ArrayList<LegalLanguageComponent>(); 10951 this.legal.add(t); 10952 return this; 10953 } 10954 10955 /** 10956 * @return The first repetition of repeating field {@link #legal}, creating it if it does not already exist {3} 10957 */ 10958 public LegalLanguageComponent getLegalFirstRep() { 10959 if (getLegal().isEmpty()) { 10960 addLegal(); 10961 } 10962 return getLegal().get(0); 10963 } 10964 10965 /** 10966 * @return {@link #rule} (List of Computable Policy Rule Language Representations of this Contract.) 10967 */ 10968 public List<ComputableLanguageComponent> getRule() { 10969 if (this.rule == null) 10970 this.rule = new ArrayList<ComputableLanguageComponent>(); 10971 return this.rule; 10972 } 10973 10974 /** 10975 * @return Returns a reference to <code>this</code> for easy method chaining 10976 */ 10977 public Contract setRule(List<ComputableLanguageComponent> theRule) { 10978 this.rule = theRule; 10979 return this; 10980 } 10981 10982 public boolean hasRule() { 10983 if (this.rule == null) 10984 return false; 10985 for (ComputableLanguageComponent item : this.rule) 10986 if (!item.isEmpty()) 10987 return true; 10988 return false; 10989 } 10990 10991 public ComputableLanguageComponent addRule() { //3 10992 ComputableLanguageComponent t = new ComputableLanguageComponent(); 10993 if (this.rule == null) 10994 this.rule = new ArrayList<ComputableLanguageComponent>(); 10995 this.rule.add(t); 10996 return t; 10997 } 10998 10999 public Contract addRule(ComputableLanguageComponent t) { //3 11000 if (t == null) 11001 return this; 11002 if (this.rule == null) 11003 this.rule = new ArrayList<ComputableLanguageComponent>(); 11004 this.rule.add(t); 11005 return this; 11006 } 11007 11008 /** 11009 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist {3} 11010 */ 11011 public ComputableLanguageComponent getRuleFirstRep() { 11012 if (getRule().isEmpty()) { 11013 addRule(); 11014 } 11015 return getRule().get(0); 11016 } 11017 11018 /** 11019 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 11020 */ 11021 public DataType getLegallyBinding() { 11022 return this.legallyBinding; 11023 } 11024 11025 /** 11026 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 11027 */ 11028 public Attachment getLegallyBindingAttachment() throws FHIRException { 11029 if (this.legallyBinding == null) 11030 this.legallyBinding = new Attachment(); 11031 if (!(this.legallyBinding instanceof Attachment)) 11032 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.legallyBinding.getClass().getName()+" was encountered"); 11033 return (Attachment) this.legallyBinding; 11034 } 11035 11036 public boolean hasLegallyBindingAttachment() { 11037 return this != null && this.legallyBinding instanceof Attachment; 11038 } 11039 11040 /** 11041 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 11042 */ 11043 public Reference getLegallyBindingReference() throws FHIRException { 11044 if (this.legallyBinding == null) 11045 this.legallyBinding = new Reference(); 11046 if (!(this.legallyBinding instanceof Reference)) 11047 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.legallyBinding.getClass().getName()+" was encountered"); 11048 return (Reference) this.legallyBinding; 11049 } 11050 11051 public boolean hasLegallyBindingReference() { 11052 return this != null && this.legallyBinding instanceof Reference; 11053 } 11054 11055 public boolean hasLegallyBinding() { 11056 return this.legallyBinding != null && !this.legallyBinding.isEmpty(); 11057 } 11058 11059 /** 11060 * @param value {@link #legallyBinding} (Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the "source of truth" and which would be the basis for legal action related to enforcement of this Contract.) 11061 */ 11062 public Contract setLegallyBinding(DataType value) { 11063 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 11064 throw new FHIRException("Not the right type for Contract.legallyBinding[x]: "+value.fhirType()); 11065 this.legallyBinding = value; 11066 return this; 11067 } 11068 11069 protected void listChildren(List<Property> children) { 11070 super.listChildren(children); 11071 children.add(new Property("identifier", "Identifier", "Unique identifier for this Contract or a derivative that references a Source Contract.", 0, java.lang.Integer.MAX_VALUE, identifier)); 11072 children.add(new Property("url", "uri", "Canonical identifier for this contract, represented as a URI (globally unique).", 0, 1, url)); 11073 children.add(new Property("version", "string", "An edition identifier used for business purposes to label business significant variants.", 0, 1, version)); 11074 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 11075 children.add(new Property("legalState", "CodeableConcept", "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.", 0, 1, legalState)); 11076 children.add(new Property("instantiatesCanonical", "Reference(Contract)", "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.", 0, 1, instantiatesCanonical)); 11077 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.", 0, 1, instantiatesUri)); 11078 children.add(new Property("contentDerivative", "CodeableConcept", "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, contentDerivative)); 11079 children.add(new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued)); 11080 children.add(new Property("applies", "Period", "Relevant time or time-period when this Contract is applicable.", 0, 1, applies)); 11081 children.add(new Property("expirationType", "CodeableConcept", "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.", 0, 1, expirationType)); 11082 children.add(new Property("subject", "Reference(Any)", "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, subject)); 11083 children.add(new Property("authority", "Reference(Organization)", "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 0, java.lang.Integer.MAX_VALUE, authority)); 11084 children.add(new Property("domain", "Reference(Location)", "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 0, java.lang.Integer.MAX_VALUE, domain)); 11085 children.add(new Property("site", "Reference(Location)", "Sites in which the contract is complied with, exercised, or in force.", 0, java.lang.Integer.MAX_VALUE, site)); 11086 children.add(new Property("name", "string", "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 11087 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.", 0, 1, title)); 11088 children.add(new Property("subtitle", "string", "A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.", 0, 1, subtitle)); 11089 children.add(new Property("alias", "string", "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.", 0, java.lang.Integer.MAX_VALUE, alias)); 11090 children.add(new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization)", "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.", 0, 1, author)); 11091 children.add(new Property("scope", "CodeableConcept", "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.", 0, 1, scope)); 11092 children.add(new Property("topic[x]", "CodeableConcept|Reference(Any)", "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, topic)); 11093 children.add(new Property("type", "CodeableConcept", "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.", 0, 1, type)); 11094 children.add(new Property("subType", "CodeableConcept", "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.", 0, java.lang.Integer.MAX_VALUE, subType)); 11095 children.add(new Property("contentDefinition", "", "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.", 0, 1, contentDefinition)); 11096 children.add(new Property("term", "", "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 0, java.lang.Integer.MAX_VALUE, term)); 11097 children.add(new Property("supportingInfo", "Reference(Any)", "Information that may be needed by/relevant to the performer in their execution of this term action.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 11098 children.add(new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provenance.entity indicates the target that was changed in the update (see [Provenance.entity](provenance-definitions.html#Provenance.entity)).", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 11099 children.add(new Property("signer", "", "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 0, java.lang.Integer.MAX_VALUE, signer)); 11100 children.add(new Property("friendly", "", "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 0, java.lang.Integer.MAX_VALUE, friendly)); 11101 children.add(new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, legal)); 11102 children.add(new Property("rule", "", "List of Computable Policy Rule Language Representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, rule)); 11103 children.add(new Property("legallyBinding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, legallyBinding)); 11104 } 11105 11106 @Override 11107 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11108 switch (_hash) { 11109 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this Contract or a derivative that references a Source Contract.", 0, java.lang.Integer.MAX_VALUE, identifier); 11110 case 116079: /*url*/ return new Property("url", "uri", "Canonical identifier for this contract, represented as a URI (globally unique).", 0, 1, url); 11111 case 351608024: /*version*/ return new Property("version", "string", "An edition identifier used for business purposes to label business significant variants.", 0, 1, version); 11112 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 11113 case 568606040: /*legalState*/ return new Property("legalState", "CodeableConcept", "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.", 0, 1, legalState); 11114 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "Reference(Contract)", "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.", 0, 1, instantiatesCanonical); 11115 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.", 0, 1, instantiatesUri); 11116 case -92412192: /*contentDerivative*/ return new Property("contentDerivative", "CodeableConcept", "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, contentDerivative); 11117 case -1179159893: /*issued*/ return new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued); 11118 case -793235316: /*applies*/ return new Property("applies", "Period", "Relevant time or time-period when this Contract is applicable.", 0, 1, applies); 11119 case -668311927: /*expirationType*/ return new Property("expirationType", "CodeableConcept", "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.", 0, 1, expirationType); 11120 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, subject); 11121 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 0, java.lang.Integer.MAX_VALUE, authority); 11122 case -1326197564: /*domain*/ return new Property("domain", "Reference(Location)", "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 0, java.lang.Integer.MAX_VALUE, domain); 11123 case 3530567: /*site*/ return new Property("site", "Reference(Location)", "Sites in which the contract is complied with, exercised, or in force.", 0, java.lang.Integer.MAX_VALUE, site); 11124 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 11125 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.", 0, 1, title); 11126 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "A more detailed or qualifying explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.", 0, 1, subtitle); 11127 case 92902992: /*alias*/ return new Property("alias", "string", "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.", 0, java.lang.Integer.MAX_VALUE, alias); 11128 case -1406328437: /*author*/ return new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization)", "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.", 0, 1, author); 11129 case 109264468: /*scope*/ return new Property("scope", "CodeableConcept", "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.", 0, 1, scope); 11130 case -957295375: /*topic[x]*/ return new Property("topic[x]", "CodeableConcept|Reference(Any)", "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, topic); 11131 case 110546223: /*topic*/ return new Property("topic[x]", "CodeableConcept|Reference(Any)", "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, topic); 11132 case 777778802: /*topicCodeableConcept*/ return new Property("topic[x]", "CodeableConcept", "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, topic); 11133 case -343345444: /*topicReference*/ return new Property("topic[x]", "Reference(Any)", "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, topic); 11134 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.", 0, 1, type); 11135 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.", 0, java.lang.Integer.MAX_VALUE, subType); 11136 case 247055020: /*contentDefinition*/ return new Property("contentDefinition", "", "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.", 0, 1, contentDefinition); 11137 case 3556460: /*term*/ return new Property("term", "", "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 0, java.lang.Integer.MAX_VALUE, term); 11138 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Information that may be needed by/relevant to the performer in their execution of this term action.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 11139 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provenance.entity indicates the target that was changed in the update (see [Provenance.entity](provenance-definitions.html#Provenance.entity)).", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 11140 case -902467798: /*signer*/ return new Property("signer", "", "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 0, java.lang.Integer.MAX_VALUE, signer); 11141 case -1423054677: /*friendly*/ return new Property("friendly", "", "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 0, java.lang.Integer.MAX_VALUE, friendly); 11142 case 102851257: /*legal*/ return new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, legal); 11143 case 3512060: /*rule*/ return new Property("rule", "", "List of Computable Policy Rule Language Representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, rule); 11144 case -772497791: /*legallyBinding[x]*/ return new Property("legallyBinding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, legallyBinding); 11145 case -126751329: /*legallyBinding*/ return new Property("legallyBinding[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, legallyBinding); 11146 case 344057890: /*legallyBindingAttachment*/ return new Property("legallyBinding[x]", "Attachment", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, legallyBinding); 11147 case -296528788: /*legallyBindingReference*/ return new Property("legallyBinding[x]", "Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 0, 1, legallyBinding); 11148 default: return super.getNamedProperty(_hash, _name, _checkValid); 11149 } 11150 11151 } 11152 11153 @Override 11154 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11155 switch (hash) { 11156 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 11157 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 11158 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 11159 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ContractResourceStatusCodes> 11160 case 568606040: /*legalState*/ return this.legalState == null ? new Base[0] : new Base[] {this.legalState}; // CodeableConcept 11161 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : new Base[] {this.instantiatesCanonical}; // Reference 11162 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : new Base[] {this.instantiatesUri}; // UriType 11163 case -92412192: /*contentDerivative*/ return this.contentDerivative == null ? new Base[0] : new Base[] {this.contentDerivative}; // CodeableConcept 11164 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // DateTimeType 11165 case -793235316: /*applies*/ return this.applies == null ? new Base[0] : new Base[] {this.applies}; // Period 11166 case -668311927: /*expirationType*/ return this.expirationType == null ? new Base[0] : new Base[] {this.expirationType}; // CodeableConcept 11167 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 11168 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : this.authority.toArray(new Base[this.authority.size()]); // Reference 11169 case -1326197564: /*domain*/ return this.domain == null ? new Base[0] : this.domain.toArray(new Base[this.domain.size()]); // Reference 11170 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // Reference 11171 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 11172 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 11173 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 11174 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 11175 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 11176 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : new Base[] {this.scope}; // CodeableConcept 11177 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : new Base[] {this.topic}; // DataType 11178 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 11179 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 11180 case 247055020: /*contentDefinition*/ return this.contentDefinition == null ? new Base[0] : new Base[] {this.contentDefinition}; // ContentDefinitionComponent 11181 case 3556460: /*term*/ return this.term == null ? new Base[0] : this.term.toArray(new Base[this.term.size()]); // TermComponent 11182 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 11183 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 11184 case -902467798: /*signer*/ return this.signer == null ? new Base[0] : this.signer.toArray(new Base[this.signer.size()]); // SignatoryComponent 11185 case -1423054677: /*friendly*/ return this.friendly == null ? new Base[0] : this.friendly.toArray(new Base[this.friendly.size()]); // FriendlyLanguageComponent 11186 case 102851257: /*legal*/ return this.legal == null ? new Base[0] : this.legal.toArray(new Base[this.legal.size()]); // LegalLanguageComponent 11187 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // ComputableLanguageComponent 11188 case -126751329: /*legallyBinding*/ return this.legallyBinding == null ? new Base[0] : new Base[] {this.legallyBinding}; // DataType 11189 default: return super.getProperty(hash, name, checkValid); 11190 } 11191 11192 } 11193 11194 @Override 11195 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11196 switch (hash) { 11197 case -1618432855: // identifier 11198 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 11199 return value; 11200 case 116079: // url 11201 this.url = TypeConvertor.castToUri(value); // UriType 11202 return value; 11203 case 351608024: // version 11204 this.version = TypeConvertor.castToString(value); // StringType 11205 return value; 11206 case -892481550: // status 11207 value = new ContractResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 11208 this.status = (Enumeration) value; // Enumeration<ContractResourceStatusCodes> 11209 return value; 11210 case 568606040: // legalState 11211 this.legalState = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11212 return value; 11213 case 8911915: // instantiatesCanonical 11214 this.instantiatesCanonical = TypeConvertor.castToReference(value); // Reference 11215 return value; 11216 case -1926393373: // instantiatesUri 11217 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 11218 return value; 11219 case -92412192: // contentDerivative 11220 this.contentDerivative = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11221 return value; 11222 case -1179159893: // issued 11223 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 11224 return value; 11225 case -793235316: // applies 11226 this.applies = TypeConvertor.castToPeriod(value); // Period 11227 return value; 11228 case -668311927: // expirationType 11229 this.expirationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11230 return value; 11231 case -1867885268: // subject 11232 this.getSubject().add(TypeConvertor.castToReference(value)); // Reference 11233 return value; 11234 case 1475610435: // authority 11235 this.getAuthority().add(TypeConvertor.castToReference(value)); // Reference 11236 return value; 11237 case -1326197564: // domain 11238 this.getDomain().add(TypeConvertor.castToReference(value)); // Reference 11239 return value; 11240 case 3530567: // site 11241 this.getSite().add(TypeConvertor.castToReference(value)); // Reference 11242 return value; 11243 case 3373707: // name 11244 this.name = TypeConvertor.castToString(value); // StringType 11245 return value; 11246 case 110371416: // title 11247 this.title = TypeConvertor.castToString(value); // StringType 11248 return value; 11249 case -2060497896: // subtitle 11250 this.subtitle = TypeConvertor.castToString(value); // StringType 11251 return value; 11252 case 92902992: // alias 11253 this.getAlias().add(TypeConvertor.castToString(value)); // StringType 11254 return value; 11255 case -1406328437: // author 11256 this.author = TypeConvertor.castToReference(value); // Reference 11257 return value; 11258 case 109264468: // scope 11259 this.scope = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11260 return value; 11261 case 110546223: // topic 11262 this.topic = TypeConvertor.castToType(value); // DataType 11263 return value; 11264 case 3575610: // type 11265 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11266 return value; 11267 case -1868521062: // subType 11268 this.getSubType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 11269 return value; 11270 case 247055020: // contentDefinition 11271 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 11272 return value; 11273 case 3556460: // term 11274 this.getTerm().add((TermComponent) value); // TermComponent 11275 return value; 11276 case 1922406657: // supportingInfo 11277 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); // Reference 11278 return value; 11279 case 1538891575: // relevantHistory 11280 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); // Reference 11281 return value; 11282 case -902467798: // signer 11283 this.getSigner().add((SignatoryComponent) value); // SignatoryComponent 11284 return value; 11285 case -1423054677: // friendly 11286 this.getFriendly().add((FriendlyLanguageComponent) value); // FriendlyLanguageComponent 11287 return value; 11288 case 102851257: // legal 11289 this.getLegal().add((LegalLanguageComponent) value); // LegalLanguageComponent 11290 return value; 11291 case 3512060: // rule 11292 this.getRule().add((ComputableLanguageComponent) value); // ComputableLanguageComponent 11293 return value; 11294 case -126751329: // legallyBinding 11295 this.legallyBinding = TypeConvertor.castToType(value); // DataType 11296 return value; 11297 default: return super.setProperty(hash, name, value); 11298 } 11299 11300 } 11301 11302 @Override 11303 public Base setProperty(String name, Base value) throws FHIRException { 11304 if (name.equals("identifier")) { 11305 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 11306 } else if (name.equals("url")) { 11307 this.url = TypeConvertor.castToUri(value); // UriType 11308 } else if (name.equals("version")) { 11309 this.version = TypeConvertor.castToString(value); // StringType 11310 } else if (name.equals("status")) { 11311 value = new ContractResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 11312 this.status = (Enumeration) value; // Enumeration<ContractResourceStatusCodes> 11313 } else if (name.equals("legalState")) { 11314 this.legalState = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11315 } else if (name.equals("instantiatesCanonical")) { 11316 this.instantiatesCanonical = TypeConvertor.castToReference(value); // Reference 11317 } else if (name.equals("instantiatesUri")) { 11318 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 11319 } else if (name.equals("contentDerivative")) { 11320 this.contentDerivative = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11321 } else if (name.equals("issued")) { 11322 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 11323 } else if (name.equals("applies")) { 11324 this.applies = TypeConvertor.castToPeriod(value); // Period 11325 } else if (name.equals("expirationType")) { 11326 this.expirationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11327 } else if (name.equals("subject")) { 11328 this.getSubject().add(TypeConvertor.castToReference(value)); 11329 } else if (name.equals("authority")) { 11330 this.getAuthority().add(TypeConvertor.castToReference(value)); 11331 } else if (name.equals("domain")) { 11332 this.getDomain().add(TypeConvertor.castToReference(value)); 11333 } else if (name.equals("site")) { 11334 this.getSite().add(TypeConvertor.castToReference(value)); 11335 } else if (name.equals("name")) { 11336 this.name = TypeConvertor.castToString(value); // StringType 11337 } else if (name.equals("title")) { 11338 this.title = TypeConvertor.castToString(value); // StringType 11339 } else if (name.equals("subtitle")) { 11340 this.subtitle = TypeConvertor.castToString(value); // StringType 11341 } else if (name.equals("alias")) { 11342 this.getAlias().add(TypeConvertor.castToString(value)); 11343 } else if (name.equals("author")) { 11344 this.author = TypeConvertor.castToReference(value); // Reference 11345 } else if (name.equals("scope")) { 11346 this.scope = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11347 } else if (name.equals("topic[x]")) { 11348 this.topic = TypeConvertor.castToType(value); // DataType 11349 } else if (name.equals("type")) { 11350 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11351 } else if (name.equals("subType")) { 11352 this.getSubType().add(TypeConvertor.castToCodeableConcept(value)); 11353 } else if (name.equals("contentDefinition")) { 11354 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 11355 } else if (name.equals("term")) { 11356 this.getTerm().add((TermComponent) value); 11357 } else if (name.equals("supportingInfo")) { 11358 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); 11359 } else if (name.equals("relevantHistory")) { 11360 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); 11361 } else if (name.equals("signer")) { 11362 this.getSigner().add((SignatoryComponent) value); 11363 } else if (name.equals("friendly")) { 11364 this.getFriendly().add((FriendlyLanguageComponent) value); 11365 } else if (name.equals("legal")) { 11366 this.getLegal().add((LegalLanguageComponent) value); 11367 } else if (name.equals("rule")) { 11368 this.getRule().add((ComputableLanguageComponent) value); 11369 } else if (name.equals("legallyBinding[x]")) { 11370 this.legallyBinding = TypeConvertor.castToType(value); // DataType 11371 } else 11372 return super.setProperty(name, value); 11373 return value; 11374 } 11375 11376 @Override 11377 public void removeChild(String name, Base value) throws FHIRException { 11378 if (name.equals("identifier")) { 11379 this.getIdentifier().remove(value); 11380 } else if (name.equals("url")) { 11381 this.url = null; 11382 } else if (name.equals("version")) { 11383 this.version = null; 11384 } else if (name.equals("status")) { 11385 value = new ContractResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 11386 this.status = (Enumeration) value; // Enumeration<ContractResourceStatusCodes> 11387 } else if (name.equals("legalState")) { 11388 this.legalState = null; 11389 } else if (name.equals("instantiatesCanonical")) { 11390 this.instantiatesCanonical = null; 11391 } else if (name.equals("instantiatesUri")) { 11392 this.instantiatesUri = null; 11393 } else if (name.equals("contentDerivative")) { 11394 this.contentDerivative = null; 11395 } else if (name.equals("issued")) { 11396 this.issued = null; 11397 } else if (name.equals("applies")) { 11398 this.applies = null; 11399 } else if (name.equals("expirationType")) { 11400 this.expirationType = null; 11401 } else if (name.equals("subject")) { 11402 this.getSubject().remove(value); 11403 } else if (name.equals("authority")) { 11404 this.getAuthority().remove(value); 11405 } else if (name.equals("domain")) { 11406 this.getDomain().remove(value); 11407 } else if (name.equals("site")) { 11408 this.getSite().remove(value); 11409 } else if (name.equals("name")) { 11410 this.name = null; 11411 } else if (name.equals("title")) { 11412 this.title = null; 11413 } else if (name.equals("subtitle")) { 11414 this.subtitle = null; 11415 } else if (name.equals("alias")) { 11416 this.getAlias().remove(value); 11417 } else if (name.equals("author")) { 11418 this.author = null; 11419 } else if (name.equals("scope")) { 11420 this.scope = null; 11421 } else if (name.equals("topic[x]")) { 11422 this.topic = null; 11423 } else if (name.equals("type")) { 11424 this.type = null; 11425 } else if (name.equals("subType")) { 11426 this.getSubType().remove(value); 11427 } else if (name.equals("contentDefinition")) { 11428 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 11429 } else if (name.equals("term")) { 11430 this.getTerm().remove((TermComponent) value); 11431 } else if (name.equals("supportingInfo")) { 11432 this.getSupportingInfo().remove(value); 11433 } else if (name.equals("relevantHistory")) { 11434 this.getRelevantHistory().remove(value); 11435 } else if (name.equals("signer")) { 11436 this.getSigner().remove((SignatoryComponent) value); 11437 } else if (name.equals("friendly")) { 11438 this.getFriendly().remove((FriendlyLanguageComponent) value); 11439 } else if (name.equals("legal")) { 11440 this.getLegal().remove((LegalLanguageComponent) value); 11441 } else if (name.equals("rule")) { 11442 this.getRule().remove((ComputableLanguageComponent) value); 11443 } else if (name.equals("legallyBinding[x]")) { 11444 this.legallyBinding = null; 11445 } else 11446 super.removeChild(name, value); 11447 11448 } 11449 11450 @Override 11451 public Base makeProperty(int hash, String name) throws FHIRException { 11452 switch (hash) { 11453 case -1618432855: return addIdentifier(); 11454 case 116079: return getUrlElement(); 11455 case 351608024: return getVersionElement(); 11456 case -892481550: return getStatusElement(); 11457 case 568606040: return getLegalState(); 11458 case 8911915: return getInstantiatesCanonical(); 11459 case -1926393373: return getInstantiatesUriElement(); 11460 case -92412192: return getContentDerivative(); 11461 case -1179159893: return getIssuedElement(); 11462 case -793235316: return getApplies(); 11463 case -668311927: return getExpirationType(); 11464 case -1867885268: return addSubject(); 11465 case 1475610435: return addAuthority(); 11466 case -1326197564: return addDomain(); 11467 case 3530567: return addSite(); 11468 case 3373707: return getNameElement(); 11469 case 110371416: return getTitleElement(); 11470 case -2060497896: return getSubtitleElement(); 11471 case 92902992: return addAliasElement(); 11472 case -1406328437: return getAuthor(); 11473 case 109264468: return getScope(); 11474 case -957295375: return getTopic(); 11475 case 110546223: return getTopic(); 11476 case 3575610: return getType(); 11477 case -1868521062: return addSubType(); 11478 case 247055020: return getContentDefinition(); 11479 case 3556460: return addTerm(); 11480 case 1922406657: return addSupportingInfo(); 11481 case 1538891575: return addRelevantHistory(); 11482 case -902467798: return addSigner(); 11483 case -1423054677: return addFriendly(); 11484 case 102851257: return addLegal(); 11485 case 3512060: return addRule(); 11486 case -772497791: return getLegallyBinding(); 11487 case -126751329: return getLegallyBinding(); 11488 default: return super.makeProperty(hash, name); 11489 } 11490 11491 } 11492 11493 @Override 11494 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11495 switch (hash) { 11496 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 11497 case 116079: /*url*/ return new String[] {"uri"}; 11498 case 351608024: /*version*/ return new String[] {"string"}; 11499 case -892481550: /*status*/ return new String[] {"code"}; 11500 case 568606040: /*legalState*/ return new String[] {"CodeableConcept"}; 11501 case 8911915: /*instantiatesCanonical*/ return new String[] {"Reference"}; 11502 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 11503 case -92412192: /*contentDerivative*/ return new String[] {"CodeableConcept"}; 11504 case -1179159893: /*issued*/ return new String[] {"dateTime"}; 11505 case -793235316: /*applies*/ return new String[] {"Period"}; 11506 case -668311927: /*expirationType*/ return new String[] {"CodeableConcept"}; 11507 case -1867885268: /*subject*/ return new String[] {"Reference"}; 11508 case 1475610435: /*authority*/ return new String[] {"Reference"}; 11509 case -1326197564: /*domain*/ return new String[] {"Reference"}; 11510 case 3530567: /*site*/ return new String[] {"Reference"}; 11511 case 3373707: /*name*/ return new String[] {"string"}; 11512 case 110371416: /*title*/ return new String[] {"string"}; 11513 case -2060497896: /*subtitle*/ return new String[] {"string"}; 11514 case 92902992: /*alias*/ return new String[] {"string"}; 11515 case -1406328437: /*author*/ return new String[] {"Reference"}; 11516 case 109264468: /*scope*/ return new String[] {"CodeableConcept"}; 11517 case 110546223: /*topic*/ return new String[] {"CodeableConcept", "Reference"}; 11518 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 11519 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 11520 case 247055020: /*contentDefinition*/ return new String[] {}; 11521 case 3556460: /*term*/ return new String[] {}; 11522 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 11523 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 11524 case -902467798: /*signer*/ return new String[] {}; 11525 case -1423054677: /*friendly*/ return new String[] {}; 11526 case 102851257: /*legal*/ return new String[] {}; 11527 case 3512060: /*rule*/ return new String[] {}; 11528 case -126751329: /*legallyBinding*/ return new String[] {"Attachment", "Reference"}; 11529 default: return super.getTypesForProperty(hash, name); 11530 } 11531 11532 } 11533 11534 @Override 11535 public Base addChild(String name) throws FHIRException { 11536 if (name.equals("identifier")) { 11537 return addIdentifier(); 11538 } 11539 else if (name.equals("url")) { 11540 throw new FHIRException("Cannot call addChild on a singleton property Contract.url"); 11541 } 11542 else if (name.equals("version")) { 11543 throw new FHIRException("Cannot call addChild on a singleton property Contract.version"); 11544 } 11545 else if (name.equals("status")) { 11546 throw new FHIRException("Cannot call addChild on a singleton property Contract.status"); 11547 } 11548 else if (name.equals("legalState")) { 11549 this.legalState = new CodeableConcept(); 11550 return this.legalState; 11551 } 11552 else if (name.equals("instantiatesCanonical")) { 11553 this.instantiatesCanonical = new Reference(); 11554 return this.instantiatesCanonical; 11555 } 11556 else if (name.equals("instantiatesUri")) { 11557 throw new FHIRException("Cannot call addChild on a singleton property Contract.instantiatesUri"); 11558 } 11559 else if (name.equals("contentDerivative")) { 11560 this.contentDerivative = new CodeableConcept(); 11561 return this.contentDerivative; 11562 } 11563 else if (name.equals("issued")) { 11564 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 11565 } 11566 else if (name.equals("applies")) { 11567 this.applies = new Period(); 11568 return this.applies; 11569 } 11570 else if (name.equals("expirationType")) { 11571 this.expirationType = new CodeableConcept(); 11572 return this.expirationType; 11573 } 11574 else if (name.equals("subject")) { 11575 return addSubject(); 11576 } 11577 else if (name.equals("authority")) { 11578 return addAuthority(); 11579 } 11580 else if (name.equals("domain")) { 11581 return addDomain(); 11582 } 11583 else if (name.equals("site")) { 11584 return addSite(); 11585 } 11586 else if (name.equals("name")) { 11587 throw new FHIRException("Cannot call addChild on a singleton property Contract.name"); 11588 } 11589 else if (name.equals("title")) { 11590 throw new FHIRException("Cannot call addChild on a singleton property Contract.title"); 11591 } 11592 else if (name.equals("subtitle")) { 11593 throw new FHIRException("Cannot call addChild on a singleton property Contract.subtitle"); 11594 } 11595 else if (name.equals("alias")) { 11596 throw new FHIRException("Cannot call addChild on a singleton property Contract.alias"); 11597 } 11598 else if (name.equals("author")) { 11599 this.author = new Reference(); 11600 return this.author; 11601 } 11602 else if (name.equals("scope")) { 11603 this.scope = new CodeableConcept(); 11604 return this.scope; 11605 } 11606 else if (name.equals("topicCodeableConcept")) { 11607 this.topic = new CodeableConcept(); 11608 return this.topic; 11609 } 11610 else if (name.equals("topicReference")) { 11611 this.topic = new Reference(); 11612 return this.topic; 11613 } 11614 else if (name.equals("type")) { 11615 this.type = new CodeableConcept(); 11616 return this.type; 11617 } 11618 else if (name.equals("subType")) { 11619 return addSubType(); 11620 } 11621 else if (name.equals("contentDefinition")) { 11622 this.contentDefinition = new ContentDefinitionComponent(); 11623 return this.contentDefinition; 11624 } 11625 else if (name.equals("term")) { 11626 return addTerm(); 11627 } 11628 else if (name.equals("supportingInfo")) { 11629 return addSupportingInfo(); 11630 } 11631 else if (name.equals("relevantHistory")) { 11632 return addRelevantHistory(); 11633 } 11634 else if (name.equals("signer")) { 11635 return addSigner(); 11636 } 11637 else if (name.equals("friendly")) { 11638 return addFriendly(); 11639 } 11640 else if (name.equals("legal")) { 11641 return addLegal(); 11642 } 11643 else if (name.equals("rule")) { 11644 return addRule(); 11645 } 11646 else if (name.equals("legallyBindingAttachment")) { 11647 this.legallyBinding = new Attachment(); 11648 return this.legallyBinding; 11649 } 11650 else if (name.equals("legallyBindingReference")) { 11651 this.legallyBinding = new Reference(); 11652 return this.legallyBinding; 11653 } 11654 else 11655 return super.addChild(name); 11656 } 11657 11658 public String fhirType() { 11659 return "Contract"; 11660 11661 } 11662 11663 public Contract copy() { 11664 Contract dst = new Contract(); 11665 copyValues(dst); 11666 return dst; 11667 } 11668 11669 public void copyValues(Contract dst) { 11670 super.copyValues(dst); 11671 if (identifier != null) { 11672 dst.identifier = new ArrayList<Identifier>(); 11673 for (Identifier i : identifier) 11674 dst.identifier.add(i.copy()); 11675 }; 11676 dst.url = url == null ? null : url.copy(); 11677 dst.version = version == null ? null : version.copy(); 11678 dst.status = status == null ? null : status.copy(); 11679 dst.legalState = legalState == null ? null : legalState.copy(); 11680 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 11681 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 11682 dst.contentDerivative = contentDerivative == null ? null : contentDerivative.copy(); 11683 dst.issued = issued == null ? null : issued.copy(); 11684 dst.applies = applies == null ? null : applies.copy(); 11685 dst.expirationType = expirationType == null ? null : expirationType.copy(); 11686 if (subject != null) { 11687 dst.subject = new ArrayList<Reference>(); 11688 for (Reference i : subject) 11689 dst.subject.add(i.copy()); 11690 }; 11691 if (authority != null) { 11692 dst.authority = new ArrayList<Reference>(); 11693 for (Reference i : authority) 11694 dst.authority.add(i.copy()); 11695 }; 11696 if (domain != null) { 11697 dst.domain = new ArrayList<Reference>(); 11698 for (Reference i : domain) 11699 dst.domain.add(i.copy()); 11700 }; 11701 if (site != null) { 11702 dst.site = new ArrayList<Reference>(); 11703 for (Reference i : site) 11704 dst.site.add(i.copy()); 11705 }; 11706 dst.name = name == null ? null : name.copy(); 11707 dst.title = title == null ? null : title.copy(); 11708 dst.subtitle = subtitle == null ? null : subtitle.copy(); 11709 if (alias != null) { 11710 dst.alias = new ArrayList<StringType>(); 11711 for (StringType i : alias) 11712 dst.alias.add(i.copy()); 11713 }; 11714 dst.author = author == null ? null : author.copy(); 11715 dst.scope = scope == null ? null : scope.copy(); 11716 dst.topic = topic == null ? null : topic.copy(); 11717 dst.type = type == null ? null : type.copy(); 11718 if (subType != null) { 11719 dst.subType = new ArrayList<CodeableConcept>(); 11720 for (CodeableConcept i : subType) 11721 dst.subType.add(i.copy()); 11722 }; 11723 dst.contentDefinition = contentDefinition == null ? null : contentDefinition.copy(); 11724 if (term != null) { 11725 dst.term = new ArrayList<TermComponent>(); 11726 for (TermComponent i : term) 11727 dst.term.add(i.copy()); 11728 }; 11729 if (supportingInfo != null) { 11730 dst.supportingInfo = new ArrayList<Reference>(); 11731 for (Reference i : supportingInfo) 11732 dst.supportingInfo.add(i.copy()); 11733 }; 11734 if (relevantHistory != null) { 11735 dst.relevantHistory = new ArrayList<Reference>(); 11736 for (Reference i : relevantHistory) 11737 dst.relevantHistory.add(i.copy()); 11738 }; 11739 if (signer != null) { 11740 dst.signer = new ArrayList<SignatoryComponent>(); 11741 for (SignatoryComponent i : signer) 11742 dst.signer.add(i.copy()); 11743 }; 11744 if (friendly != null) { 11745 dst.friendly = new ArrayList<FriendlyLanguageComponent>(); 11746 for (FriendlyLanguageComponent i : friendly) 11747 dst.friendly.add(i.copy()); 11748 }; 11749 if (legal != null) { 11750 dst.legal = new ArrayList<LegalLanguageComponent>(); 11751 for (LegalLanguageComponent i : legal) 11752 dst.legal.add(i.copy()); 11753 }; 11754 if (rule != null) { 11755 dst.rule = new ArrayList<ComputableLanguageComponent>(); 11756 for (ComputableLanguageComponent i : rule) 11757 dst.rule.add(i.copy()); 11758 }; 11759 dst.legallyBinding = legallyBinding == null ? null : legallyBinding.copy(); 11760 } 11761 11762 protected Contract typedCopy() { 11763 return copy(); 11764 } 11765 11766 @Override 11767 public boolean equalsDeep(Base other_) { 11768 if (!super.equalsDeep(other_)) 11769 return false; 11770 if (!(other_ instanceof Contract)) 11771 return false; 11772 Contract o = (Contract) other_; 11773 return compareDeep(identifier, o.identifier, true) && compareDeep(url, o.url, true) && compareDeep(version, o.version, true) 11774 && compareDeep(status, o.status, true) && compareDeep(legalState, o.legalState, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 11775 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(contentDerivative, o.contentDerivative, true) 11776 && compareDeep(issued, o.issued, true) && compareDeep(applies, o.applies, true) && compareDeep(expirationType, o.expirationType, true) 11777 && compareDeep(subject, o.subject, true) && compareDeep(authority, o.authority, true) && compareDeep(domain, o.domain, true) 11778 && compareDeep(site, o.site, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 11779 && compareDeep(subtitle, o.subtitle, true) && compareDeep(alias, o.alias, true) && compareDeep(author, o.author, true) 11780 && compareDeep(scope, o.scope, true) && compareDeep(topic, o.topic, true) && compareDeep(type, o.type, true) 11781 && compareDeep(subType, o.subType, true) && compareDeep(contentDefinition, o.contentDefinition, true) 11782 && compareDeep(term, o.term, true) && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(relevantHistory, o.relevantHistory, true) 11783 && compareDeep(signer, o.signer, true) && compareDeep(friendly, o.friendly, true) && compareDeep(legal, o.legal, true) 11784 && compareDeep(rule, o.rule, true) && compareDeep(legallyBinding, o.legallyBinding, true); 11785 } 11786 11787 @Override 11788 public boolean equalsShallow(Base other_) { 11789 if (!super.equalsShallow(other_)) 11790 return false; 11791 if (!(other_ instanceof Contract)) 11792 return false; 11793 Contract o = (Contract) other_; 11794 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(status, o.status, true) 11795 && compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(issued, o.issued, true) 11796 && compareValues(name, o.name, true) && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) 11797 && compareValues(alias, o.alias, true); 11798 } 11799 11800 public boolean isEmpty() { 11801 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, url, version 11802 , status, legalState, instantiatesCanonical, instantiatesUri, contentDerivative, issued 11803 , applies, expirationType, subject, authority, domain, site, name, title, subtitle 11804 , alias, author, scope, topic, type, subType, contentDefinition, term, supportingInfo 11805 , relevantHistory, signer, friendly, legal, rule, legallyBinding); 11806 } 11807 11808 @Override 11809 public ResourceType getResourceType() { 11810 return ResourceType.Contract; 11811 } 11812 11813 /** 11814 * Search parameter: <b>authority</b> 11815 * <p> 11816 * Description: <b>The authority of the contract</b><br> 11817 * Type: <b>reference</b><br> 11818 * Path: <b>Contract.authority</b><br> 11819 * </p> 11820 */ 11821 @SearchParamDefinition(name="authority", path="Contract.authority", description="The authority of the contract", type="reference", target={Organization.class } ) 11822 public static final String SP_AUTHORITY = "authority"; 11823 /** 11824 * <b>Fluent Client</b> search parameter constant for <b>authority</b> 11825 * <p> 11826 * Description: <b>The authority of the contract</b><br> 11827 * Type: <b>reference</b><br> 11828 * Path: <b>Contract.authority</b><br> 11829 * </p> 11830 */ 11831 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHORITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHORITY); 11832 11833/** 11834 * Constant for fluent queries to be used to add include statements. Specifies 11835 * the path value of "<b>Contract:authority</b>". 11836 */ 11837 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHORITY = new ca.uhn.fhir.model.api.Include("Contract:authority").toLocked(); 11838 11839 /** 11840 * Search parameter: <b>domain</b> 11841 * <p> 11842 * Description: <b>The domain of the contract</b><br> 11843 * Type: <b>reference</b><br> 11844 * Path: <b>Contract.domain</b><br> 11845 * </p> 11846 */ 11847 @SearchParamDefinition(name="domain", path="Contract.domain", description="The domain of the contract", type="reference", target={Location.class } ) 11848 public static final String SP_DOMAIN = "domain"; 11849 /** 11850 * <b>Fluent Client</b> search parameter constant for <b>domain</b> 11851 * <p> 11852 * Description: <b>The domain of the contract</b><br> 11853 * Type: <b>reference</b><br> 11854 * Path: <b>Contract.domain</b><br> 11855 * </p> 11856 */ 11857 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DOMAIN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DOMAIN); 11858 11859/** 11860 * Constant for fluent queries to be used to add include statements. Specifies 11861 * the path value of "<b>Contract:domain</b>". 11862 */ 11863 public static final ca.uhn.fhir.model.api.Include INCLUDE_DOMAIN = new ca.uhn.fhir.model.api.Include("Contract:domain").toLocked(); 11864 11865 /** 11866 * Search parameter: <b>instantiates</b> 11867 * <p> 11868 * Description: <b>A source definition of the contract</b><br> 11869 * Type: <b>uri</b><br> 11870 * Path: <b>Contract.instantiatesUri</b><br> 11871 * </p> 11872 */ 11873 @SearchParamDefinition(name="instantiates", path="Contract.instantiatesUri", description="A source definition of the contract", type="uri" ) 11874 public static final String SP_INSTANTIATES = "instantiates"; 11875 /** 11876 * <b>Fluent Client</b> search parameter constant for <b>instantiates</b> 11877 * <p> 11878 * Description: <b>A source definition of the contract</b><br> 11879 * Type: <b>uri</b><br> 11880 * Path: <b>Contract.instantiatesUri</b><br> 11881 * </p> 11882 */ 11883 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES); 11884 11885 /** 11886 * Search parameter: <b>issued</b> 11887 * <p> 11888 * Description: <b>The date/time the contract was issued</b><br> 11889 * Type: <b>date</b><br> 11890 * Path: <b>Contract.issued</b><br> 11891 * </p> 11892 */ 11893 @SearchParamDefinition(name="issued", path="Contract.issued", description="The date/time the contract was issued", type="date" ) 11894 public static final String SP_ISSUED = "issued"; 11895 /** 11896 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 11897 * <p> 11898 * Description: <b>The date/time the contract was issued</b><br> 11899 * Type: <b>date</b><br> 11900 * Path: <b>Contract.issued</b><br> 11901 * </p> 11902 */ 11903 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 11904 11905 /** 11906 * Search parameter: <b>signer</b> 11907 * <p> 11908 * Description: <b>Contract Signatory Party</b><br> 11909 * Type: <b>reference</b><br> 11910 * Path: <b>Contract.signer.party</b><br> 11911 * </p> 11912 */ 11913 @SearchParamDefinition(name="signer", path="Contract.signer.party", description="Contract Signatory Party", type="reference", target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 11914 public static final String SP_SIGNER = "signer"; 11915 /** 11916 * <b>Fluent Client</b> search parameter constant for <b>signer</b> 11917 * <p> 11918 * Description: <b>Contract Signatory Party</b><br> 11919 * Type: <b>reference</b><br> 11920 * Path: <b>Contract.signer.party</b><br> 11921 * </p> 11922 */ 11923 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SIGNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SIGNER); 11924 11925/** 11926 * Constant for fluent queries to be used to add include statements. Specifies 11927 * the path value of "<b>Contract:signer</b>". 11928 */ 11929 public static final ca.uhn.fhir.model.api.Include INCLUDE_SIGNER = new ca.uhn.fhir.model.api.Include("Contract:signer").toLocked(); 11930 11931 /** 11932 * Search parameter: <b>status</b> 11933 * <p> 11934 * Description: <b>The status of the contract</b><br> 11935 * Type: <b>token</b><br> 11936 * Path: <b>Contract.status</b><br> 11937 * </p> 11938 */ 11939 @SearchParamDefinition(name="status", path="Contract.status", description="The status of the contract", type="token" ) 11940 public static final String SP_STATUS = "status"; 11941 /** 11942 * <b>Fluent Client</b> search parameter constant for <b>status</b> 11943 * <p> 11944 * Description: <b>The status of the contract</b><br> 11945 * Type: <b>token</b><br> 11946 * Path: <b>Contract.status</b><br> 11947 * </p> 11948 */ 11949 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 11950 11951 /** 11952 * Search parameter: <b>subject</b> 11953 * <p> 11954 * Description: <b>The identity of the subject of the contract</b><br> 11955 * Type: <b>reference</b><br> 11956 * Path: <b>Contract.subject</b><br> 11957 * </p> 11958 */ 11959 @SearchParamDefinition(name="subject", path="Contract.subject", description="The identity of the subject of the contract", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 11960 public static final String SP_SUBJECT = "subject"; 11961 /** 11962 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 11963 * <p> 11964 * Description: <b>The identity of the subject of the contract</b><br> 11965 * Type: <b>reference</b><br> 11966 * Path: <b>Contract.subject</b><br> 11967 * </p> 11968 */ 11969 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 11970 11971/** 11972 * Constant for fluent queries to be used to add include statements. Specifies 11973 * the path value of "<b>Contract:subject</b>". 11974 */ 11975 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Contract:subject").toLocked(); 11976 11977 /** 11978 * Search parameter: <b>url</b> 11979 * <p> 11980 * Description: <b>The basal contract definition</b><br> 11981 * Type: <b>uri</b><br> 11982 * Path: <b>Contract.url</b><br> 11983 * </p> 11984 */ 11985 @SearchParamDefinition(name="url", path="Contract.url", description="The basal contract definition", type="uri" ) 11986 public static final String SP_URL = "url"; 11987 /** 11988 * <b>Fluent Client</b> search parameter constant for <b>url</b> 11989 * <p> 11990 * Description: <b>The basal contract definition</b><br> 11991 * Type: <b>uri</b><br> 11992 * Path: <b>Contract.url</b><br> 11993 * </p> 11994 */ 11995 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 11996 11997 /** 11998 * Search parameter: <b>identifier</b> 11999 * <p> 12000 * Description: <b>Multiple Resources: 12001 12002* [Account](account.html): Account number 12003* [AdverseEvent](adverseevent.html): Business identifier for the event 12004* [AllergyIntolerance](allergyintolerance.html): External ids for this item 12005* [Appointment](appointment.html): An Identifier of the Appointment 12006* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 12007* [Basic](basic.html): Business identifier 12008* [BodyStructure](bodystructure.html): Bodystructure identifier 12009* [CarePlan](careplan.html): External Ids for this plan 12010* [CareTeam](careteam.html): External Ids for this team 12011* [ChargeItem](chargeitem.html): Business Identifier for item 12012* [Claim](claim.html): The primary identifier of the financial resource 12013* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 12014* [ClinicalImpression](clinicalimpression.html): Business identifier 12015* [Communication](communication.html): Unique identifier 12016* [CommunicationRequest](communicationrequest.html): Unique identifier 12017* [Composition](composition.html): Version-independent identifier for the Composition 12018* [Condition](condition.html): A unique identifier of the condition record 12019* [Consent](consent.html): Identifier for this record (external references) 12020* [Contract](contract.html): The identity of the contract 12021* [Coverage](coverage.html): The primary identifier of the insured and the coverage 12022* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 12023* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 12024* [DetectedIssue](detectedissue.html): Unique id for the detected issue 12025* [DeviceRequest](devicerequest.html): Business identifier for request/order 12026* [DeviceUsage](deviceusage.html): Search by identifier 12027* [DiagnosticReport](diagnosticreport.html): An identifier for the report 12028* [DocumentReference](documentreference.html): Identifier of the attachment binary 12029* [Encounter](encounter.html): Identifier(s) by which this encounter is known 12030* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 12031* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 12032* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 12033* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 12034* [Flag](flag.html): Business identifier 12035* [Goal](goal.html): External Ids for this goal 12036* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 12037* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 12038* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 12039* [Immunization](immunization.html): Business identifier 12040* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 12041* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 12042* [Invoice](invoice.html): Business Identifier for item 12043* [List](list.html): Business identifier 12044* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 12045* [Medication](medication.html): Returns medications with this external identifier 12046* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 12047* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 12048* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 12049* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 12050* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 12051* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 12052* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 12053* [Observation](observation.html): The unique id for a particular observation 12054* [Person](person.html): A person Identifier 12055* [Procedure](procedure.html): A unique identifier for a procedure 12056* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 12057* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 12058* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 12059* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 12060* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 12061* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 12062* [Specimen](specimen.html): The unique identifier associated with the specimen 12063* [SupplyDelivery](supplydelivery.html): External identifier 12064* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 12065* [Task](task.html): Search for a task instance by its business identifier 12066* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 12067</b><br> 12068 * Type: <b>token</b><br> 12069 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 12070 * </p> 12071 */ 12072 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 12073 public static final String SP_IDENTIFIER = "identifier"; 12074 /** 12075 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 12076 * <p> 12077 * Description: <b>Multiple Resources: 12078 12079* [Account](account.html): Account number 12080* [AdverseEvent](adverseevent.html): Business identifier for the event 12081* [AllergyIntolerance](allergyintolerance.html): External ids for this item 12082* [Appointment](appointment.html): An Identifier of the Appointment 12083* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 12084* [Basic](basic.html): Business identifier 12085* [BodyStructure](bodystructure.html): Bodystructure identifier 12086* [CarePlan](careplan.html): External Ids for this plan 12087* [CareTeam](careteam.html): External Ids for this team 12088* [ChargeItem](chargeitem.html): Business Identifier for item 12089* [Claim](claim.html): The primary identifier of the financial resource 12090* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 12091* [ClinicalImpression](clinicalimpression.html): Business identifier 12092* [Communication](communication.html): Unique identifier 12093* [CommunicationRequest](communicationrequest.html): Unique identifier 12094* [Composition](composition.html): Version-independent identifier for the Composition 12095* [Condition](condition.html): A unique identifier of the condition record 12096* [Consent](consent.html): Identifier for this record (external references) 12097* [Contract](contract.html): The identity of the contract 12098* [Coverage](coverage.html): The primary identifier of the insured and the coverage 12099* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 12100* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 12101* [DetectedIssue](detectedissue.html): Unique id for the detected issue 12102* [DeviceRequest](devicerequest.html): Business identifier for request/order 12103* [DeviceUsage](deviceusage.html): Search by identifier 12104* [DiagnosticReport](diagnosticreport.html): An identifier for the report 12105* [DocumentReference](documentreference.html): Identifier of the attachment binary 12106* [Encounter](encounter.html): Identifier(s) by which this encounter is known 12107* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 12108* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 12109* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 12110* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 12111* [Flag](flag.html): Business identifier 12112* [Goal](goal.html): External Ids for this goal 12113* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 12114* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 12115* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 12116* [Immunization](immunization.html): Business identifier 12117* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 12118* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 12119* [Invoice](invoice.html): Business Identifier for item 12120* [List](list.html): Business identifier 12121* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 12122* [Medication](medication.html): Returns medications with this external identifier 12123* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 12124* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 12125* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 12126* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 12127* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 12128* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 12129* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 12130* [Observation](observation.html): The unique id for a particular observation 12131* [Person](person.html): A person Identifier 12132* [Procedure](procedure.html): A unique identifier for a procedure 12133* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 12134* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 12135* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 12136* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 12137* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 12138* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 12139* [Specimen](specimen.html): The unique identifier associated with the specimen 12140* [SupplyDelivery](supplydelivery.html): External identifier 12141* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 12142* [Task](task.html): Search for a task instance by its business identifier 12143* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 12144</b><br> 12145 * Type: <b>token</b><br> 12146 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 12147 * </p> 12148 */ 12149 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 12150 12151 /** 12152 * Search parameter: <b>patient</b> 12153 * <p> 12154 * Description: <b>Multiple Resources: 12155 12156* [Account](account.html): The entity that caused the expenses 12157* [AdverseEvent](adverseevent.html): Subject impacted by event 12158* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 12159* [Appointment](appointment.html): One of the individuals of the appointment is this patient 12160* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 12161* [AuditEvent](auditevent.html): Where the activity involved patient data 12162* [Basic](basic.html): Identifies the focus of this resource 12163* [BodyStructure](bodystructure.html): Who this is about 12164* [CarePlan](careplan.html): Who the care plan is for 12165* [CareTeam](careteam.html): Who care team is for 12166* [ChargeItem](chargeitem.html): Individual service was done for/to 12167* [Claim](claim.html): Patient receiving the products or services 12168* [ClaimResponse](claimresponse.html): The subject of care 12169* [ClinicalImpression](clinicalimpression.html): Patient assessed 12170* [Communication](communication.html): Focus of message 12171* [CommunicationRequest](communicationrequest.html): Focus of message 12172* [Composition](composition.html): Who and/or what the composition is about 12173* [Condition](condition.html): Who has the condition? 12174* [Consent](consent.html): Who the consent applies to 12175* [Contract](contract.html): The identity of the subject of the contract (if a patient) 12176* [Coverage](coverage.html): Retrieve coverages for a patient 12177* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 12178* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 12179* [DetectedIssue](detectedissue.html): Associated patient 12180* [DeviceRequest](devicerequest.html): Individual the service is ordered for 12181* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 12182* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 12183* [DocumentReference](documentreference.html): Who/what is the subject of the document 12184* [Encounter](encounter.html): The patient present at the encounter 12185* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 12186* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 12187* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 12188* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 12189* [Flag](flag.html): The identity of a subject to list flags for 12190* [Goal](goal.html): Who this goal is intended for 12191* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 12192* [ImagingSelection](imagingselection.html): Who the study is about 12193* [ImagingStudy](imagingstudy.html): Who the study is about 12194* [Immunization](immunization.html): The patient for the vaccination record 12195* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 12196* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 12197* [Invoice](invoice.html): Recipient(s) of goods and services 12198* [List](list.html): If all resources have the same subject 12199* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 12200* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 12201* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 12202* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 12203* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 12204* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 12205* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 12206* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 12207* [Observation](observation.html): The subject that the observation is about (if patient) 12208* [Person](person.html): The Person links to this Patient 12209* [Procedure](procedure.html): Search by subject - a patient 12210* [Provenance](provenance.html): Where the activity involved patient data 12211* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 12212* [RelatedPerson](relatedperson.html): The patient this related person is related to 12213* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 12214* [ResearchSubject](researchsubject.html): Who or what is part of study 12215* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 12216* [ServiceRequest](servicerequest.html): Search by subject - a patient 12217* [Specimen](specimen.html): The patient the specimen comes from 12218* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 12219* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 12220* [Task](task.html): Search by patient 12221* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 12222</b><br> 12223 * Type: <b>reference</b><br> 12224 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 12225 * </p> 12226 */ 12227 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 12228 public static final String SP_PATIENT = "patient"; 12229 /** 12230 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 12231 * <p> 12232 * Description: <b>Multiple Resources: 12233 12234* [Account](account.html): The entity that caused the expenses 12235* [AdverseEvent](adverseevent.html): Subject impacted by event 12236* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 12237* [Appointment](appointment.html): One of the individuals of the appointment is this patient 12238* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 12239* [AuditEvent](auditevent.html): Where the activity involved patient data 12240* [Basic](basic.html): Identifies the focus of this resource 12241* [BodyStructure](bodystructure.html): Who this is about 12242* [CarePlan](careplan.html): Who the care plan is for 12243* [CareTeam](careteam.html): Who care team is for 12244* [ChargeItem](chargeitem.html): Individual service was done for/to 12245* [Claim](claim.html): Patient receiving the products or services 12246* [ClaimResponse](claimresponse.html): The subject of care 12247* [ClinicalImpression](clinicalimpression.html): Patient assessed 12248* [Communication](communication.html): Focus of message 12249* [CommunicationRequest](communicationrequest.html): Focus of message 12250* [Composition](composition.html): Who and/or what the composition is about 12251* [Condition](condition.html): Who has the condition? 12252* [Consent](consent.html): Who the consent applies to 12253* [Contract](contract.html): The identity of the subject of the contract (if a patient) 12254* [Coverage](coverage.html): Retrieve coverages for a patient 12255* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 12256* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 12257* [DetectedIssue](detectedissue.html): Associated patient 12258* [DeviceRequest](devicerequest.html): Individual the service is ordered for 12259* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 12260* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 12261* [DocumentReference](documentreference.html): Who/what is the subject of the document 12262* [Encounter](encounter.html): The patient present at the encounter 12263* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 12264* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 12265* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 12266* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 12267* [Flag](flag.html): The identity of a subject to list flags for 12268* [Goal](goal.html): Who this goal is intended for 12269* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 12270* [ImagingSelection](imagingselection.html): Who the study is about 12271* [ImagingStudy](imagingstudy.html): Who the study is about 12272* [Immunization](immunization.html): The patient for the vaccination record 12273* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 12274* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 12275* [Invoice](invoice.html): Recipient(s) of goods and services 12276* [List](list.html): If all resources have the same subject 12277* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 12278* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 12279* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 12280* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 12281* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 12282* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 12283* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 12284* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 12285* [Observation](observation.html): The subject that the observation is about (if patient) 12286* [Person](person.html): The Person links to this Patient 12287* [Procedure](procedure.html): Search by subject - a patient 12288* [Provenance](provenance.html): Where the activity involved patient data 12289* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 12290* [RelatedPerson](relatedperson.html): The patient this related person is related to 12291* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 12292* [ResearchSubject](researchsubject.html): Who or what is part of study 12293* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 12294* [ServiceRequest](servicerequest.html): Search by subject - a patient 12295* [Specimen](specimen.html): The patient the specimen comes from 12296* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 12297* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 12298* [Task](task.html): Search by patient 12299* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 12300</b><br> 12301 * Type: <b>reference</b><br> 12302 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 12303 * </p> 12304 */ 12305 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 12306 12307/** 12308 * Constant for fluent queries to be used to add include statements. Specifies 12309 * the path value of "<b>Contract:patient</b>". 12310 */ 12311 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Contract:patient").toLocked(); 12312 12313 12314} 12315