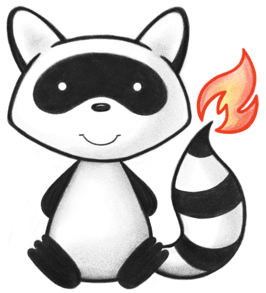
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Contributor Type: A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 050 */ 051@DatatypeDef(name="Contributor") 052public class Contributor extends DataType implements ICompositeType { 053 054 public enum ContributorType { 055 /** 056 * An author of the content of the module. 057 */ 058 AUTHOR, 059 /** 060 * An editor of the content of the module. 061 */ 062 EDITOR, 063 /** 064 * A reviewer of the content of the module. 065 */ 066 REVIEWER, 067 /** 068 * An endorser of the content of the module. 069 */ 070 ENDORSER, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static ContributorType fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("author".equals(codeString)) 079 return AUTHOR; 080 if ("editor".equals(codeString)) 081 return EDITOR; 082 if ("reviewer".equals(codeString)) 083 return REVIEWER; 084 if ("endorser".equals(codeString)) 085 return ENDORSER; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown ContributorType code '"+codeString+"'"); 090 } 091 public String toCode() { 092 switch (this) { 093 case AUTHOR: return "author"; 094 case EDITOR: return "editor"; 095 case REVIEWER: return "reviewer"; 096 case ENDORSER: return "endorser"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getSystem() { 102 switch (this) { 103 case AUTHOR: return "http://hl7.org/fhir/contributor-type"; 104 case EDITOR: return "http://hl7.org/fhir/contributor-type"; 105 case REVIEWER: return "http://hl7.org/fhir/contributor-type"; 106 case ENDORSER: return "http://hl7.org/fhir/contributor-type"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case AUTHOR: return "An author of the content of the module."; 114 case EDITOR: return "An editor of the content of the module."; 115 case REVIEWER: return "A reviewer of the content of the module."; 116 case ENDORSER: return "An endorser of the content of the module."; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDisplay() { 122 switch (this) { 123 case AUTHOR: return "Author"; 124 case EDITOR: return "Editor"; 125 case REVIEWER: return "Reviewer"; 126 case ENDORSER: return "Endorser"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 } 132 133 public static class ContributorTypeEnumFactory implements EnumFactory<ContributorType> { 134 public ContributorType fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("author".equals(codeString)) 139 return ContributorType.AUTHOR; 140 if ("editor".equals(codeString)) 141 return ContributorType.EDITOR; 142 if ("reviewer".equals(codeString)) 143 return ContributorType.REVIEWER; 144 if ("endorser".equals(codeString)) 145 return ContributorType.ENDORSER; 146 throw new IllegalArgumentException("Unknown ContributorType code '"+codeString+"'"); 147 } 148 public Enumeration<ContributorType> fromType(PrimitiveType<?> code) throws FHIRException { 149 if (code == null) 150 return null; 151 if (code.isEmpty()) 152 return new Enumeration<ContributorType>(this, ContributorType.NULL, code); 153 String codeString = ((PrimitiveType) code).asStringValue(); 154 if (codeString == null || "".equals(codeString)) 155 return new Enumeration<ContributorType>(this, ContributorType.NULL, code); 156 if ("author".equals(codeString)) 157 return new Enumeration<ContributorType>(this, ContributorType.AUTHOR, code); 158 if ("editor".equals(codeString)) 159 return new Enumeration<ContributorType>(this, ContributorType.EDITOR, code); 160 if ("reviewer".equals(codeString)) 161 return new Enumeration<ContributorType>(this, ContributorType.REVIEWER, code); 162 if ("endorser".equals(codeString)) 163 return new Enumeration<ContributorType>(this, ContributorType.ENDORSER, code); 164 throw new FHIRException("Unknown ContributorType code '"+codeString+"'"); 165 } 166 public String toCode(ContributorType code) { 167 if (code == ContributorType.NULL) 168 return null; 169 if (code == ContributorType.AUTHOR) 170 return "author"; 171 if (code == ContributorType.EDITOR) 172 return "editor"; 173 if (code == ContributorType.REVIEWER) 174 return "reviewer"; 175 if (code == ContributorType.ENDORSER) 176 return "endorser"; 177 return "?"; 178 } 179 public String toSystem(ContributorType code) { 180 return code.getSystem(); 181 } 182 } 183 184 /** 185 * The type of contributor. 186 */ 187 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 188 @Description(shortDefinition="author | editor | reviewer | endorser", formalDefinition="The type of contributor." ) 189 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/contributor-type") 190 protected Enumeration<ContributorType> type; 191 192 /** 193 * The name of the individual or organization responsible for the contribution. 194 */ 195 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 196 @Description(shortDefinition="Who contributed the content", formalDefinition="The name of the individual or organization responsible for the contribution." ) 197 protected StringType name; 198 199 /** 200 * Contact details to assist a user in finding and communicating with the contributor. 201 */ 202 @Child(name = "contact", type = {ContactDetail.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 203 @Description(shortDefinition="Contact details of the contributor", formalDefinition="Contact details to assist a user in finding and communicating with the contributor." ) 204 protected List<ContactDetail> contact; 205 206 private static final long serialVersionUID = -609887113L; 207 208 /** 209 * Constructor 210 */ 211 public Contributor() { 212 super(); 213 } 214 215 /** 216 * Constructor 217 */ 218 public Contributor(ContributorType type, String name) { 219 super(); 220 this.setType(type); 221 this.setName(name); 222 } 223 224 /** 225 * @return {@link #type} (The type of contributor.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 226 */ 227 public Enumeration<ContributorType> getTypeElement() { 228 if (this.type == null) 229 if (Configuration.errorOnAutoCreate()) 230 throw new Error("Attempt to auto-create Contributor.type"); 231 else if (Configuration.doAutoCreate()) 232 this.type = new Enumeration<ContributorType>(new ContributorTypeEnumFactory()); // bb 233 return this.type; 234 } 235 236 public boolean hasTypeElement() { 237 return this.type != null && !this.type.isEmpty(); 238 } 239 240 public boolean hasType() { 241 return this.type != null && !this.type.isEmpty(); 242 } 243 244 /** 245 * @param value {@link #type} (The type of contributor.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 246 */ 247 public Contributor setTypeElement(Enumeration<ContributorType> value) { 248 this.type = value; 249 return this; 250 } 251 252 /** 253 * @return The type of contributor. 254 */ 255 public ContributorType getType() { 256 return this.type == null ? null : this.type.getValue(); 257 } 258 259 /** 260 * @param value The type of contributor. 261 */ 262 public Contributor setType(ContributorType value) { 263 if (this.type == null) 264 this.type = new Enumeration<ContributorType>(new ContributorTypeEnumFactory()); 265 this.type.setValue(value); 266 return this; 267 } 268 269 /** 270 * @return {@link #name} (The name of the individual or organization responsible for the contribution.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 271 */ 272 public StringType getNameElement() { 273 if (this.name == null) 274 if (Configuration.errorOnAutoCreate()) 275 throw new Error("Attempt to auto-create Contributor.name"); 276 else if (Configuration.doAutoCreate()) 277 this.name = new StringType(); // bb 278 return this.name; 279 } 280 281 public boolean hasNameElement() { 282 return this.name != null && !this.name.isEmpty(); 283 } 284 285 public boolean hasName() { 286 return this.name != null && !this.name.isEmpty(); 287 } 288 289 /** 290 * @param value {@link #name} (The name of the individual or organization responsible for the contribution.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 291 */ 292 public Contributor setNameElement(StringType value) { 293 this.name = value; 294 return this; 295 } 296 297 /** 298 * @return The name of the individual or organization responsible for the contribution. 299 */ 300 public String getName() { 301 return this.name == null ? null : this.name.getValue(); 302 } 303 304 /** 305 * @param value The name of the individual or organization responsible for the contribution. 306 */ 307 public Contributor setName(String value) { 308 if (this.name == null) 309 this.name = new StringType(); 310 this.name.setValue(value); 311 return this; 312 } 313 314 /** 315 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the contributor.) 316 */ 317 public List<ContactDetail> getContact() { 318 if (this.contact == null) 319 this.contact = new ArrayList<ContactDetail>(); 320 return this.contact; 321 } 322 323 /** 324 * @return Returns a reference to <code>this</code> for easy method chaining 325 */ 326 public Contributor setContact(List<ContactDetail> theContact) { 327 this.contact = theContact; 328 return this; 329 } 330 331 public boolean hasContact() { 332 if (this.contact == null) 333 return false; 334 for (ContactDetail item : this.contact) 335 if (!item.isEmpty()) 336 return true; 337 return false; 338 } 339 340 public ContactDetail addContact() { //3 341 ContactDetail t = new ContactDetail(); 342 if (this.contact == null) 343 this.contact = new ArrayList<ContactDetail>(); 344 this.contact.add(t); 345 return t; 346 } 347 348 public Contributor addContact(ContactDetail t) { //3 349 if (t == null) 350 return this; 351 if (this.contact == null) 352 this.contact = new ArrayList<ContactDetail>(); 353 this.contact.add(t); 354 return this; 355 } 356 357 /** 358 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 359 */ 360 public ContactDetail getContactFirstRep() { 361 if (getContact().isEmpty()) { 362 addContact(); 363 } 364 return getContact().get(0); 365 } 366 367 protected void listChildren(List<Property> children) { 368 super.listChildren(children); 369 children.add(new Property("type", "code", "The type of contributor.", 0, 1, type)); 370 children.add(new Property("name", "string", "The name of the individual or organization responsible for the contribution.", 0, 1, name)); 371 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the contributor.", 0, java.lang.Integer.MAX_VALUE, contact)); 372 } 373 374 @Override 375 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 376 switch (_hash) { 377 case 3575610: /*type*/ return new Property("type", "code", "The type of contributor.", 0, 1, type); 378 case 3373707: /*name*/ return new Property("name", "string", "The name of the individual or organization responsible for the contribution.", 0, 1, name); 379 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the contributor.", 0, java.lang.Integer.MAX_VALUE, contact); 380 default: return super.getNamedProperty(_hash, _name, _checkValid); 381 } 382 383 } 384 385 @Override 386 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 387 switch (hash) { 388 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ContributorType> 389 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 390 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 391 default: return super.getProperty(hash, name, checkValid); 392 } 393 394 } 395 396 @Override 397 public Base setProperty(int hash, String name, Base value) throws FHIRException { 398 switch (hash) { 399 case 3575610: // type 400 value = new ContributorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 401 this.type = (Enumeration) value; // Enumeration<ContributorType> 402 return value; 403 case 3373707: // name 404 this.name = TypeConvertor.castToString(value); // StringType 405 return value; 406 case 951526432: // contact 407 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 408 return value; 409 default: return super.setProperty(hash, name, value); 410 } 411 412 } 413 414 @Override 415 public Base setProperty(String name, Base value) throws FHIRException { 416 if (name.equals("type")) { 417 value = new ContributorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 418 this.type = (Enumeration) value; // Enumeration<ContributorType> 419 } else if (name.equals("name")) { 420 this.name = TypeConvertor.castToString(value); // StringType 421 } else if (name.equals("contact")) { 422 this.getContact().add(TypeConvertor.castToContactDetail(value)); 423 } else 424 return super.setProperty(name, value); 425 return value; 426 } 427 428 @Override 429 public void removeChild(String name, Base value) throws FHIRException { 430 if (name.equals("type")) { 431 value = new ContributorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 432 this.type = (Enumeration) value; // Enumeration<ContributorType> 433 } else if (name.equals("name")) { 434 this.name = null; 435 } else if (name.equals("contact")) { 436 this.getContact().remove(value); 437 } else 438 super.removeChild(name, value); 439 440 } 441 442 @Override 443 public Base makeProperty(int hash, String name) throws FHIRException { 444 switch (hash) { 445 case 3575610: return getTypeElement(); 446 case 3373707: return getNameElement(); 447 case 951526432: return addContact(); 448 default: return super.makeProperty(hash, name); 449 } 450 451 } 452 453 @Override 454 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 455 switch (hash) { 456 case 3575610: /*type*/ return new String[] {"code"}; 457 case 3373707: /*name*/ return new String[] {"string"}; 458 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 459 default: return super.getTypesForProperty(hash, name); 460 } 461 462 } 463 464 @Override 465 public Base addChild(String name) throws FHIRException { 466 if (name.equals("type")) { 467 throw new FHIRException("Cannot call addChild on a singleton property Contributor.type"); 468 } 469 else if (name.equals("name")) { 470 throw new FHIRException("Cannot call addChild on a singleton property Contributor.name"); 471 } 472 else if (name.equals("contact")) { 473 return addContact(); 474 } 475 else 476 return super.addChild(name); 477 } 478 479 public String fhirType() { 480 return "Contributor"; 481 482 } 483 484 public Contributor copy() { 485 Contributor dst = new Contributor(); 486 copyValues(dst); 487 return dst; 488 } 489 490 public void copyValues(Contributor dst) { 491 super.copyValues(dst); 492 dst.type = type == null ? null : type.copy(); 493 dst.name = name == null ? null : name.copy(); 494 if (contact != null) { 495 dst.contact = new ArrayList<ContactDetail>(); 496 for (ContactDetail i : contact) 497 dst.contact.add(i.copy()); 498 }; 499 } 500 501 protected Contributor typedCopy() { 502 return copy(); 503 } 504 505 @Override 506 public boolean equalsDeep(Base other_) { 507 if (!super.equalsDeep(other_)) 508 return false; 509 if (!(other_ instanceof Contributor)) 510 return false; 511 Contributor o = (Contributor) other_; 512 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(contact, o.contact, true) 513 ; 514 } 515 516 @Override 517 public boolean equalsShallow(Base other_) { 518 if (!super.equalsShallow(other_)) 519 return false; 520 if (!(other_ instanceof Contributor)) 521 return false; 522 Contributor o = (Contributor) other_; 523 return compareValues(type, o.type, true) && compareValues(name, o.name, true); 524 } 525 526 public boolean isEmpty() { 527 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name, contact); 528 } 529 530 531} 532