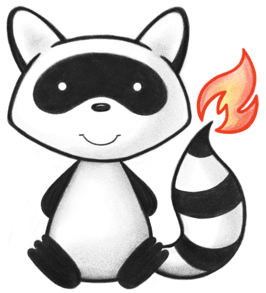
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment. 052 */ 053@ResourceDef(name="Coverage", profile="http://hl7.org/fhir/StructureDefinition/Coverage") 054public class Coverage extends DomainResource { 055 056 public enum Kind { 057 /** 058 * The Coverage provides the identifiers and card-level details of an insurance policy. 059 */ 060 INSURANCE, 061 /** 062 * One or more persons and/or organizations are paying for the services rendered. 063 */ 064 SELFPAY, 065 /** 066 * Some other organization is paying for the service. 067 */ 068 OTHER, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static Kind fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("insurance".equals(codeString)) 077 return INSURANCE; 078 if ("self-pay".equals(codeString)) 079 return SELFPAY; 080 if ("other".equals(codeString)) 081 return OTHER; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown Kind code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case INSURANCE: return "insurance"; 090 case SELFPAY: return "self-pay"; 091 case OTHER: return "other"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case INSURANCE: return "http://hl7.org/fhir/coverage-kind"; 099 case SELFPAY: return "http://hl7.org/fhir/coverage-kind"; 100 case OTHER: return "http://hl7.org/fhir/coverage-kind"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case INSURANCE: return "The Coverage provides the identifiers and card-level details of an insurance policy."; 108 case SELFPAY: return "One or more persons and/or organizations are paying for the services rendered."; 109 case OTHER: return "Some other organization is paying for the service."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case INSURANCE: return "Insurance"; 117 case SELFPAY: return "Self-pay"; 118 case OTHER: return "Other"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class KindEnumFactory implements EnumFactory<Kind> { 126 public Kind fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("insurance".equals(codeString)) 131 return Kind.INSURANCE; 132 if ("self-pay".equals(codeString)) 133 return Kind.SELFPAY; 134 if ("other".equals(codeString)) 135 return Kind.OTHER; 136 throw new IllegalArgumentException("Unknown Kind code '"+codeString+"'"); 137 } 138 public Enumeration<Kind> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<Kind>(this, Kind.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<Kind>(this, Kind.NULL, code); 146 if ("insurance".equals(codeString)) 147 return new Enumeration<Kind>(this, Kind.INSURANCE, code); 148 if ("self-pay".equals(codeString)) 149 return new Enumeration<Kind>(this, Kind.SELFPAY, code); 150 if ("other".equals(codeString)) 151 return new Enumeration<Kind>(this, Kind.OTHER, code); 152 throw new FHIRException("Unknown Kind code '"+codeString+"'"); 153 } 154 public String toCode(Kind code) { 155 if (code == Kind.NULL) 156 return null; 157 if (code == Kind.INSURANCE) 158 return "insurance"; 159 if (code == Kind.SELFPAY) 160 return "self-pay"; 161 if (code == Kind.OTHER) 162 return "other"; 163 return "?"; 164 } 165 public String toSystem(Kind code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class CoveragePaymentByComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The list of parties providing non-insurance payment for the treatment costs. 174 */ 175 @Child(name = "party", type = {Patient.class, RelatedPerson.class, Organization.class}, order=1, min=1, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="Parties performing self-payment", formalDefinition="The list of parties providing non-insurance payment for the treatment costs." ) 177 protected Reference party; 178 179 /** 180 * Description of the financial responsibility. 181 */ 182 @Child(name = "responsibility", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 183 @Description(shortDefinition="Party's responsibility", formalDefinition=" Description of the financial responsibility." ) 184 protected StringType responsibility; 185 186 private static final long serialVersionUID = -1279858336L; 187 188 /** 189 * Constructor 190 */ 191 public CoveragePaymentByComponent() { 192 super(); 193 } 194 195 /** 196 * Constructor 197 */ 198 public CoveragePaymentByComponent(Reference party) { 199 super(); 200 this.setParty(party); 201 } 202 203 /** 204 * @return {@link #party} (The list of parties providing non-insurance payment for the treatment costs.) 205 */ 206 public Reference getParty() { 207 if (this.party == null) 208 if (Configuration.errorOnAutoCreate()) 209 throw new Error("Attempt to auto-create CoveragePaymentByComponent.party"); 210 else if (Configuration.doAutoCreate()) 211 this.party = new Reference(); // cc 212 return this.party; 213 } 214 215 public boolean hasParty() { 216 return this.party != null && !this.party.isEmpty(); 217 } 218 219 /** 220 * @param value {@link #party} (The list of parties providing non-insurance payment for the treatment costs.) 221 */ 222 public CoveragePaymentByComponent setParty(Reference value) { 223 this.party = value; 224 return this; 225 } 226 227 /** 228 * @return {@link #responsibility} ( Description of the financial responsibility.). This is the underlying object with id, value and extensions. The accessor "getResponsibility" gives direct access to the value 229 */ 230 public StringType getResponsibilityElement() { 231 if (this.responsibility == null) 232 if (Configuration.errorOnAutoCreate()) 233 throw new Error("Attempt to auto-create CoveragePaymentByComponent.responsibility"); 234 else if (Configuration.doAutoCreate()) 235 this.responsibility = new StringType(); // bb 236 return this.responsibility; 237 } 238 239 public boolean hasResponsibilityElement() { 240 return this.responsibility != null && !this.responsibility.isEmpty(); 241 } 242 243 public boolean hasResponsibility() { 244 return this.responsibility != null && !this.responsibility.isEmpty(); 245 } 246 247 /** 248 * @param value {@link #responsibility} ( Description of the financial responsibility.). This is the underlying object with id, value and extensions. The accessor "getResponsibility" gives direct access to the value 249 */ 250 public CoveragePaymentByComponent setResponsibilityElement(StringType value) { 251 this.responsibility = value; 252 return this; 253 } 254 255 /** 256 * @return Description of the financial responsibility. 257 */ 258 public String getResponsibility() { 259 return this.responsibility == null ? null : this.responsibility.getValue(); 260 } 261 262 /** 263 * @param value Description of the financial responsibility. 264 */ 265 public CoveragePaymentByComponent setResponsibility(String value) { 266 if (Utilities.noString(value)) 267 this.responsibility = null; 268 else { 269 if (this.responsibility == null) 270 this.responsibility = new StringType(); 271 this.responsibility.setValue(value); 272 } 273 return this; 274 } 275 276 protected void listChildren(List<Property> children) { 277 super.listChildren(children); 278 children.add(new Property("party", "Reference(Patient|RelatedPerson|Organization)", "The list of parties providing non-insurance payment for the treatment costs.", 0, 1, party)); 279 children.add(new Property("responsibility", "string", " Description of the financial responsibility.", 0, 1, responsibility)); 280 } 281 282 @Override 283 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 284 switch (_hash) { 285 case 106437350: /*party*/ return new Property("party", "Reference(Patient|RelatedPerson|Organization)", "The list of parties providing non-insurance payment for the treatment costs.", 0, 1, party); 286 case -228897266: /*responsibility*/ return new Property("responsibility", "string", " Description of the financial responsibility.", 0, 1, responsibility); 287 default: return super.getNamedProperty(_hash, _name, _checkValid); 288 } 289 290 } 291 292 @Override 293 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 294 switch (hash) { 295 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 296 case -228897266: /*responsibility*/ return this.responsibility == null ? new Base[0] : new Base[] {this.responsibility}; // StringType 297 default: return super.getProperty(hash, name, checkValid); 298 } 299 300 } 301 302 @Override 303 public Base setProperty(int hash, String name, Base value) throws FHIRException { 304 switch (hash) { 305 case 106437350: // party 306 this.party = TypeConvertor.castToReference(value); // Reference 307 return value; 308 case -228897266: // responsibility 309 this.responsibility = TypeConvertor.castToString(value); // StringType 310 return value; 311 default: return super.setProperty(hash, name, value); 312 } 313 314 } 315 316 @Override 317 public Base setProperty(String name, Base value) throws FHIRException { 318 if (name.equals("party")) { 319 this.party = TypeConvertor.castToReference(value); // Reference 320 } else if (name.equals("responsibility")) { 321 this.responsibility = TypeConvertor.castToString(value); // StringType 322 } else 323 return super.setProperty(name, value); 324 return value; 325 } 326 327 @Override 328 public void removeChild(String name, Base value) throws FHIRException { 329 if (name.equals("party")) { 330 this.party = null; 331 } else if (name.equals("responsibility")) { 332 this.responsibility = null; 333 } else 334 super.removeChild(name, value); 335 336 } 337 338 @Override 339 public Base makeProperty(int hash, String name) throws FHIRException { 340 switch (hash) { 341 case 106437350: return getParty(); 342 case -228897266: return getResponsibilityElement(); 343 default: return super.makeProperty(hash, name); 344 } 345 346 } 347 348 @Override 349 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 350 switch (hash) { 351 case 106437350: /*party*/ return new String[] {"Reference"}; 352 case -228897266: /*responsibility*/ return new String[] {"string"}; 353 default: return super.getTypesForProperty(hash, name); 354 } 355 356 } 357 358 @Override 359 public Base addChild(String name) throws FHIRException { 360 if (name.equals("party")) { 361 this.party = new Reference(); 362 return this.party; 363 } 364 else if (name.equals("responsibility")) { 365 throw new FHIRException("Cannot call addChild on a singleton property Coverage.paymentBy.responsibility"); 366 } 367 else 368 return super.addChild(name); 369 } 370 371 public CoveragePaymentByComponent copy() { 372 CoveragePaymentByComponent dst = new CoveragePaymentByComponent(); 373 copyValues(dst); 374 return dst; 375 } 376 377 public void copyValues(CoveragePaymentByComponent dst) { 378 super.copyValues(dst); 379 dst.party = party == null ? null : party.copy(); 380 dst.responsibility = responsibility == null ? null : responsibility.copy(); 381 } 382 383 @Override 384 public boolean equalsDeep(Base other_) { 385 if (!super.equalsDeep(other_)) 386 return false; 387 if (!(other_ instanceof CoveragePaymentByComponent)) 388 return false; 389 CoveragePaymentByComponent o = (CoveragePaymentByComponent) other_; 390 return compareDeep(party, o.party, true) && compareDeep(responsibility, o.responsibility, true) 391 ; 392 } 393 394 @Override 395 public boolean equalsShallow(Base other_) { 396 if (!super.equalsShallow(other_)) 397 return false; 398 if (!(other_ instanceof CoveragePaymentByComponent)) 399 return false; 400 CoveragePaymentByComponent o = (CoveragePaymentByComponent) other_; 401 return compareValues(responsibility, o.responsibility, true); 402 } 403 404 public boolean isEmpty() { 405 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(party, responsibility); 406 } 407 408 public String fhirType() { 409 return "Coverage.paymentBy"; 410 411 } 412 413 } 414 415 @Block() 416 public static class ClassComponent extends BackboneElement implements IBaseBackboneElement { 417 /** 418 * The type of classification for which an insurer-specific class label or number and optional name is provided. For example, type may be used to identify a class of coverage or employer group, policy, or plan. 419 */ 420 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 421 @Description(shortDefinition="Type of class such as 'group' or 'plan'", formalDefinition="The type of classification for which an insurer-specific class label or number and optional name is provided. For example, type may be used to identify a class of coverage or employer group, policy, or plan." ) 422 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverage-class") 423 protected CodeableConcept type; 424 425 /** 426 * The alphanumeric identifier associated with the insurer issued label. 427 */ 428 @Child(name = "value", type = {Identifier.class}, order=2, min=1, max=1, modifier=false, summary=true) 429 @Description(shortDefinition="Value associated with the type", formalDefinition="The alphanumeric identifier associated with the insurer issued label." ) 430 protected Identifier value; 431 432 /** 433 * A short description for the class. 434 */ 435 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 436 @Description(shortDefinition="Human readable description of the type and value", formalDefinition="A short description for the class." ) 437 protected StringType name; 438 439 private static final long serialVersionUID = 1395172201L; 440 441 /** 442 * Constructor 443 */ 444 public ClassComponent() { 445 super(); 446 } 447 448 /** 449 * Constructor 450 */ 451 public ClassComponent(CodeableConcept type, Identifier value) { 452 super(); 453 this.setType(type); 454 this.setValue(value); 455 } 456 457 /** 458 * @return {@link #type} (The type of classification for which an insurer-specific class label or number and optional name is provided. For example, type may be used to identify a class of coverage or employer group, policy, or plan.) 459 */ 460 public CodeableConcept getType() { 461 if (this.type == null) 462 if (Configuration.errorOnAutoCreate()) 463 throw new Error("Attempt to auto-create ClassComponent.type"); 464 else if (Configuration.doAutoCreate()) 465 this.type = new CodeableConcept(); // cc 466 return this.type; 467 } 468 469 public boolean hasType() { 470 return this.type != null && !this.type.isEmpty(); 471 } 472 473 /** 474 * @param value {@link #type} (The type of classification for which an insurer-specific class label or number and optional name is provided. For example, type may be used to identify a class of coverage or employer group, policy, or plan.) 475 */ 476 public ClassComponent setType(CodeableConcept value) { 477 this.type = value; 478 return this; 479 } 480 481 /** 482 * @return {@link #value} (The alphanumeric identifier associated with the insurer issued label.) 483 */ 484 public Identifier getValue() { 485 if (this.value == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create ClassComponent.value"); 488 else if (Configuration.doAutoCreate()) 489 this.value = new Identifier(); // cc 490 return this.value; 491 } 492 493 public boolean hasValue() { 494 return this.value != null && !this.value.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #value} (The alphanumeric identifier associated with the insurer issued label.) 499 */ 500 public ClassComponent setValue(Identifier value) { 501 this.value = value; 502 return this; 503 } 504 505 /** 506 * @return {@link #name} (A short description for the class.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 507 */ 508 public StringType getNameElement() { 509 if (this.name == null) 510 if (Configuration.errorOnAutoCreate()) 511 throw new Error("Attempt to auto-create ClassComponent.name"); 512 else if (Configuration.doAutoCreate()) 513 this.name = new StringType(); // bb 514 return this.name; 515 } 516 517 public boolean hasNameElement() { 518 return this.name != null && !this.name.isEmpty(); 519 } 520 521 public boolean hasName() { 522 return this.name != null && !this.name.isEmpty(); 523 } 524 525 /** 526 * @param value {@link #name} (A short description for the class.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 527 */ 528 public ClassComponent setNameElement(StringType value) { 529 this.name = value; 530 return this; 531 } 532 533 /** 534 * @return A short description for the class. 535 */ 536 public String getName() { 537 return this.name == null ? null : this.name.getValue(); 538 } 539 540 /** 541 * @param value A short description for the class. 542 */ 543 public ClassComponent setName(String value) { 544 if (Utilities.noString(value)) 545 this.name = null; 546 else { 547 if (this.name == null) 548 this.name = new StringType(); 549 this.name.setValue(value); 550 } 551 return this; 552 } 553 554 protected void listChildren(List<Property> children) { 555 super.listChildren(children); 556 children.add(new Property("type", "CodeableConcept", "The type of classification for which an insurer-specific class label or number and optional name is provided. For example, type may be used to identify a class of coverage or employer group, policy, or plan.", 0, 1, type)); 557 children.add(new Property("value", "Identifier", "The alphanumeric identifier associated with the insurer issued label.", 0, 1, value)); 558 children.add(new Property("name", "string", "A short description for the class.", 0, 1, name)); 559 } 560 561 @Override 562 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 563 switch (_hash) { 564 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of classification for which an insurer-specific class label or number and optional name is provided. For example, type may be used to identify a class of coverage or employer group, policy, or plan.", 0, 1, type); 565 case 111972721: /*value*/ return new Property("value", "Identifier", "The alphanumeric identifier associated with the insurer issued label.", 0, 1, value); 566 case 3373707: /*name*/ return new Property("name", "string", "A short description for the class.", 0, 1, name); 567 default: return super.getNamedProperty(_hash, _name, _checkValid); 568 } 569 570 } 571 572 @Override 573 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 574 switch (hash) { 575 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 576 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Identifier 577 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 578 default: return super.getProperty(hash, name, checkValid); 579 } 580 581 } 582 583 @Override 584 public Base setProperty(int hash, String name, Base value) throws FHIRException { 585 switch (hash) { 586 case 3575610: // type 587 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 588 return value; 589 case 111972721: // value 590 this.value = TypeConvertor.castToIdentifier(value); // Identifier 591 return value; 592 case 3373707: // name 593 this.name = TypeConvertor.castToString(value); // StringType 594 return value; 595 default: return super.setProperty(hash, name, value); 596 } 597 598 } 599 600 @Override 601 public Base setProperty(String name, Base value) throws FHIRException { 602 if (name.equals("type")) { 603 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 604 } else if (name.equals("value")) { 605 this.value = TypeConvertor.castToIdentifier(value); // Identifier 606 } else if (name.equals("name")) { 607 this.name = TypeConvertor.castToString(value); // StringType 608 } else 609 return super.setProperty(name, value); 610 return value; 611 } 612 613 @Override 614 public void removeChild(String name, Base value) throws FHIRException { 615 if (name.equals("type")) { 616 this.type = null; 617 } else if (name.equals("value")) { 618 this.value = null; 619 } else if (name.equals("name")) { 620 this.name = null; 621 } else 622 super.removeChild(name, value); 623 624 } 625 626 @Override 627 public Base makeProperty(int hash, String name) throws FHIRException { 628 switch (hash) { 629 case 3575610: return getType(); 630 case 111972721: return getValue(); 631 case 3373707: return getNameElement(); 632 default: return super.makeProperty(hash, name); 633 } 634 635 } 636 637 @Override 638 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 639 switch (hash) { 640 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 641 case 111972721: /*value*/ return new String[] {"Identifier"}; 642 case 3373707: /*name*/ return new String[] {"string"}; 643 default: return super.getTypesForProperty(hash, name); 644 } 645 646 } 647 648 @Override 649 public Base addChild(String name) throws FHIRException { 650 if (name.equals("type")) { 651 this.type = new CodeableConcept(); 652 return this.type; 653 } 654 else if (name.equals("value")) { 655 this.value = new Identifier(); 656 return this.value; 657 } 658 else if (name.equals("name")) { 659 throw new FHIRException("Cannot call addChild on a singleton property Coverage.class.name"); 660 } 661 else 662 return super.addChild(name); 663 } 664 665 public ClassComponent copy() { 666 ClassComponent dst = new ClassComponent(); 667 copyValues(dst); 668 return dst; 669 } 670 671 public void copyValues(ClassComponent dst) { 672 super.copyValues(dst); 673 dst.type = type == null ? null : type.copy(); 674 dst.value = value == null ? null : value.copy(); 675 dst.name = name == null ? null : name.copy(); 676 } 677 678 @Override 679 public boolean equalsDeep(Base other_) { 680 if (!super.equalsDeep(other_)) 681 return false; 682 if (!(other_ instanceof ClassComponent)) 683 return false; 684 ClassComponent o = (ClassComponent) other_; 685 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(name, o.name, true) 686 ; 687 } 688 689 @Override 690 public boolean equalsShallow(Base other_) { 691 if (!super.equalsShallow(other_)) 692 return false; 693 if (!(other_ instanceof ClassComponent)) 694 return false; 695 ClassComponent o = (ClassComponent) other_; 696 return compareValues(name, o.name, true); 697 } 698 699 public boolean isEmpty() { 700 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, name); 701 } 702 703 public String fhirType() { 704 return "Coverage.class"; 705 706 } 707 708 } 709 710 @Block() 711 public static class CostToBeneficiaryComponent extends BackboneElement implements IBaseBackboneElement { 712 /** 713 * The category of patient centric costs associated with treatment. 714 */ 715 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 716 @Description(shortDefinition="Cost category", formalDefinition="The category of patient centric costs associated with treatment." ) 717 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverage-copay-type") 718 protected CodeableConcept type; 719 720 /** 721 * Code to identify the general type of benefits under which products and services are provided. 722 */ 723 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 724 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 725 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 726 protected CodeableConcept category; 727 728 /** 729 * Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers. 730 */ 731 @Child(name = "network", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 732 @Description(shortDefinition="In or out of network", formalDefinition="Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers." ) 733 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-network") 734 protected CodeableConcept network; 735 736 /** 737 * Indicates if the benefits apply to an individual or to the family. 738 */ 739 @Child(name = "unit", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 740 @Description(shortDefinition="Individual or family", formalDefinition="Indicates if the benefits apply to an individual or to the family." ) 741 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-unit") 742 protected CodeableConcept unit; 743 744 /** 745 * The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'. 746 */ 747 @Child(name = "term", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 748 @Description(shortDefinition="Annual or lifetime", formalDefinition="The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'." ) 749 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-term") 750 protected CodeableConcept term; 751 752 /** 753 * The amount due from the patient for the cost category. 754 */ 755 @Child(name = "value", type = {Quantity.class, Money.class}, order=6, min=0, max=1, modifier=false, summary=true) 756 @Description(shortDefinition="The amount or percentage due from the beneficiary", formalDefinition="The amount due from the patient for the cost category." ) 757 protected DataType value; 758 759 /** 760 * A suite of codes indicating exceptions or reductions to patient costs and their effective periods. 761 */ 762 @Child(name = "exception", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 763 @Description(shortDefinition="Exceptions for patient payments", formalDefinition="A suite of codes indicating exceptions or reductions to patient costs and their effective periods." ) 764 protected List<ExemptionComponent> exception; 765 766 private static final long serialVersionUID = 472499753L; 767 768 /** 769 * Constructor 770 */ 771 public CostToBeneficiaryComponent() { 772 super(); 773 } 774 775 /** 776 * @return {@link #type} (The category of patient centric costs associated with treatment.) 777 */ 778 public CodeableConcept getType() { 779 if (this.type == null) 780 if (Configuration.errorOnAutoCreate()) 781 throw new Error("Attempt to auto-create CostToBeneficiaryComponent.type"); 782 else if (Configuration.doAutoCreate()) 783 this.type = new CodeableConcept(); // cc 784 return this.type; 785 } 786 787 public boolean hasType() { 788 return this.type != null && !this.type.isEmpty(); 789 } 790 791 /** 792 * @param value {@link #type} (The category of patient centric costs associated with treatment.) 793 */ 794 public CostToBeneficiaryComponent setType(CodeableConcept value) { 795 this.type = value; 796 return this; 797 } 798 799 /** 800 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 801 */ 802 public CodeableConcept getCategory() { 803 if (this.category == null) 804 if (Configuration.errorOnAutoCreate()) 805 throw new Error("Attempt to auto-create CostToBeneficiaryComponent.category"); 806 else if (Configuration.doAutoCreate()) 807 this.category = new CodeableConcept(); // cc 808 return this.category; 809 } 810 811 public boolean hasCategory() { 812 return this.category != null && !this.category.isEmpty(); 813 } 814 815 /** 816 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 817 */ 818 public CostToBeneficiaryComponent setCategory(CodeableConcept value) { 819 this.category = value; 820 return this; 821 } 822 823 /** 824 * @return {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 825 */ 826 public CodeableConcept getNetwork() { 827 if (this.network == null) 828 if (Configuration.errorOnAutoCreate()) 829 throw new Error("Attempt to auto-create CostToBeneficiaryComponent.network"); 830 else if (Configuration.doAutoCreate()) 831 this.network = new CodeableConcept(); // cc 832 return this.network; 833 } 834 835 public boolean hasNetwork() { 836 return this.network != null && !this.network.isEmpty(); 837 } 838 839 /** 840 * @param value {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 841 */ 842 public CostToBeneficiaryComponent setNetwork(CodeableConcept value) { 843 this.network = value; 844 return this; 845 } 846 847 /** 848 * @return {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 849 */ 850 public CodeableConcept getUnit() { 851 if (this.unit == null) 852 if (Configuration.errorOnAutoCreate()) 853 throw new Error("Attempt to auto-create CostToBeneficiaryComponent.unit"); 854 else if (Configuration.doAutoCreate()) 855 this.unit = new CodeableConcept(); // cc 856 return this.unit; 857 } 858 859 public boolean hasUnit() { 860 return this.unit != null && !this.unit.isEmpty(); 861 } 862 863 /** 864 * @param value {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 865 */ 866 public CostToBeneficiaryComponent setUnit(CodeableConcept value) { 867 this.unit = value; 868 return this; 869 } 870 871 /** 872 * @return {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 873 */ 874 public CodeableConcept getTerm() { 875 if (this.term == null) 876 if (Configuration.errorOnAutoCreate()) 877 throw new Error("Attempt to auto-create CostToBeneficiaryComponent.term"); 878 else if (Configuration.doAutoCreate()) 879 this.term = new CodeableConcept(); // cc 880 return this.term; 881 } 882 883 public boolean hasTerm() { 884 return this.term != null && !this.term.isEmpty(); 885 } 886 887 /** 888 * @param value {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 889 */ 890 public CostToBeneficiaryComponent setTerm(CodeableConcept value) { 891 this.term = value; 892 return this; 893 } 894 895 /** 896 * @return {@link #value} (The amount due from the patient for the cost category.) 897 */ 898 public DataType getValue() { 899 return this.value; 900 } 901 902 /** 903 * @return {@link #value} (The amount due from the patient for the cost category.) 904 */ 905 public Quantity getValueQuantity() throws FHIRException { 906 if (this.value == null) 907 this.value = new Quantity(); 908 if (!(this.value instanceof Quantity)) 909 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 910 return (Quantity) this.value; 911 } 912 913 public boolean hasValueQuantity() { 914 return this != null && this.value instanceof Quantity; 915 } 916 917 /** 918 * @return {@link #value} (The amount due from the patient for the cost category.) 919 */ 920 public Money getValueMoney() throws FHIRException { 921 if (this.value == null) 922 this.value = new Money(); 923 if (!(this.value instanceof Money)) 924 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 925 return (Money) this.value; 926 } 927 928 public boolean hasValueMoney() { 929 return this != null && this.value instanceof Money; 930 } 931 932 public boolean hasValue() { 933 return this.value != null && !this.value.isEmpty(); 934 } 935 936 /** 937 * @param value {@link #value} (The amount due from the patient for the cost category.) 938 */ 939 public CostToBeneficiaryComponent setValue(DataType value) { 940 if (value != null && !(value instanceof Quantity || value instanceof Money)) 941 throw new FHIRException("Not the right type for Coverage.costToBeneficiary.value[x]: "+value.fhirType()); 942 this.value = value; 943 return this; 944 } 945 946 /** 947 * @return {@link #exception} (A suite of codes indicating exceptions or reductions to patient costs and their effective periods.) 948 */ 949 public List<ExemptionComponent> getException() { 950 if (this.exception == null) 951 this.exception = new ArrayList<ExemptionComponent>(); 952 return this.exception; 953 } 954 955 /** 956 * @return Returns a reference to <code>this</code> for easy method chaining 957 */ 958 public CostToBeneficiaryComponent setException(List<ExemptionComponent> theException) { 959 this.exception = theException; 960 return this; 961 } 962 963 public boolean hasException() { 964 if (this.exception == null) 965 return false; 966 for (ExemptionComponent item : this.exception) 967 if (!item.isEmpty()) 968 return true; 969 return false; 970 } 971 972 public ExemptionComponent addException() { //3 973 ExemptionComponent t = new ExemptionComponent(); 974 if (this.exception == null) 975 this.exception = new ArrayList<ExemptionComponent>(); 976 this.exception.add(t); 977 return t; 978 } 979 980 public CostToBeneficiaryComponent addException(ExemptionComponent t) { //3 981 if (t == null) 982 return this; 983 if (this.exception == null) 984 this.exception = new ArrayList<ExemptionComponent>(); 985 this.exception.add(t); 986 return this; 987 } 988 989 /** 990 * @return The first repetition of repeating field {@link #exception}, creating it if it does not already exist {3} 991 */ 992 public ExemptionComponent getExceptionFirstRep() { 993 if (getException().isEmpty()) { 994 addException(); 995 } 996 return getException().get(0); 997 } 998 999 protected void listChildren(List<Property> children) { 1000 super.listChildren(children); 1001 children.add(new Property("type", "CodeableConcept", "The category of patient centric costs associated with treatment.", 0, 1, type)); 1002 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 1003 children.add(new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network)); 1004 children.add(new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit)); 1005 children.add(new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term)); 1006 children.add(new Property("value[x]", "Quantity|Money", "The amount due from the patient for the cost category.", 0, 1, value)); 1007 children.add(new Property("exception", "", "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.", 0, java.lang.Integer.MAX_VALUE, exception)); 1008 } 1009 1010 @Override 1011 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1012 switch (_hash) { 1013 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of patient centric costs associated with treatment.", 0, 1, type); 1014 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 1015 case 1843485230: /*network*/ return new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network); 1016 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit); 1017 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term); 1018 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|Money", "The amount due from the patient for the cost category.", 0, 1, value); 1019 case 111972721: /*value*/ return new Property("value[x]", "Quantity|Money", "The amount due from the patient for the cost category.", 0, 1, value); 1020 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The amount due from the patient for the cost category.", 0, 1, value); 1021 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "The amount due from the patient for the cost category.", 0, 1, value); 1022 case 1481625679: /*exception*/ return new Property("exception", "", "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.", 0, java.lang.Integer.MAX_VALUE, exception); 1023 default: return super.getNamedProperty(_hash, _name, _checkValid); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1030 switch (hash) { 1031 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1032 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1033 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // CodeableConcept 1034 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 1035 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 1036 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1037 case 1481625679: /*exception*/ return this.exception == null ? new Base[0] : this.exception.toArray(new Base[this.exception.size()]); // ExemptionComponent 1038 default: return super.getProperty(hash, name, checkValid); 1039 } 1040 1041 } 1042 1043 @Override 1044 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1045 switch (hash) { 1046 case 3575610: // type 1047 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1048 return value; 1049 case 50511102: // category 1050 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1051 return value; 1052 case 1843485230: // network 1053 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1054 return value; 1055 case 3594628: // unit 1056 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1057 return value; 1058 case 3556460: // term 1059 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1060 return value; 1061 case 111972721: // value 1062 this.value = TypeConvertor.castToType(value); // DataType 1063 return value; 1064 case 1481625679: // exception 1065 this.getException().add((ExemptionComponent) value); // ExemptionComponent 1066 return value; 1067 default: return super.setProperty(hash, name, value); 1068 } 1069 1070 } 1071 1072 @Override 1073 public Base setProperty(String name, Base value) throws FHIRException { 1074 if (name.equals("type")) { 1075 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1076 } else if (name.equals("category")) { 1077 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1078 } else if (name.equals("network")) { 1079 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1080 } else if (name.equals("unit")) { 1081 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1082 } else if (name.equals("term")) { 1083 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1084 } else if (name.equals("value[x]")) { 1085 this.value = TypeConvertor.castToType(value); // DataType 1086 } else if (name.equals("exception")) { 1087 this.getException().add((ExemptionComponent) value); 1088 } else 1089 return super.setProperty(name, value); 1090 return value; 1091 } 1092 1093 @Override 1094 public void removeChild(String name, Base value) throws FHIRException { 1095 if (name.equals("type")) { 1096 this.type = null; 1097 } else if (name.equals("category")) { 1098 this.category = null; 1099 } else if (name.equals("network")) { 1100 this.network = null; 1101 } else if (name.equals("unit")) { 1102 this.unit = null; 1103 } else if (name.equals("term")) { 1104 this.term = null; 1105 } else if (name.equals("value[x]")) { 1106 this.value = null; 1107 } else if (name.equals("exception")) { 1108 this.getException().remove((ExemptionComponent) value); 1109 } else 1110 super.removeChild(name, value); 1111 1112 } 1113 1114 @Override 1115 public Base makeProperty(int hash, String name) throws FHIRException { 1116 switch (hash) { 1117 case 3575610: return getType(); 1118 case 50511102: return getCategory(); 1119 case 1843485230: return getNetwork(); 1120 case 3594628: return getUnit(); 1121 case 3556460: return getTerm(); 1122 case -1410166417: return getValue(); 1123 case 111972721: return getValue(); 1124 case 1481625679: return addException(); 1125 default: return super.makeProperty(hash, name); 1126 } 1127 1128 } 1129 1130 @Override 1131 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1132 switch (hash) { 1133 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1134 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1135 case 1843485230: /*network*/ return new String[] {"CodeableConcept"}; 1136 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 1137 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 1138 case 111972721: /*value*/ return new String[] {"Quantity", "Money"}; 1139 case 1481625679: /*exception*/ return new String[] {}; 1140 default: return super.getTypesForProperty(hash, name); 1141 } 1142 1143 } 1144 1145 @Override 1146 public Base addChild(String name) throws FHIRException { 1147 if (name.equals("type")) { 1148 this.type = new CodeableConcept(); 1149 return this.type; 1150 } 1151 else if (name.equals("category")) { 1152 this.category = new CodeableConcept(); 1153 return this.category; 1154 } 1155 else if (name.equals("network")) { 1156 this.network = new CodeableConcept(); 1157 return this.network; 1158 } 1159 else if (name.equals("unit")) { 1160 this.unit = new CodeableConcept(); 1161 return this.unit; 1162 } 1163 else if (name.equals("term")) { 1164 this.term = new CodeableConcept(); 1165 return this.term; 1166 } 1167 else if (name.equals("valueQuantity")) { 1168 this.value = new Quantity(); 1169 return this.value; 1170 } 1171 else if (name.equals("valueMoney")) { 1172 this.value = new Money(); 1173 return this.value; 1174 } 1175 else if (name.equals("exception")) { 1176 return addException(); 1177 } 1178 else 1179 return super.addChild(name); 1180 } 1181 1182 public CostToBeneficiaryComponent copy() { 1183 CostToBeneficiaryComponent dst = new CostToBeneficiaryComponent(); 1184 copyValues(dst); 1185 return dst; 1186 } 1187 1188 public void copyValues(CostToBeneficiaryComponent dst) { 1189 super.copyValues(dst); 1190 dst.type = type == null ? null : type.copy(); 1191 dst.category = category == null ? null : category.copy(); 1192 dst.network = network == null ? null : network.copy(); 1193 dst.unit = unit == null ? null : unit.copy(); 1194 dst.term = term == null ? null : term.copy(); 1195 dst.value = value == null ? null : value.copy(); 1196 if (exception != null) { 1197 dst.exception = new ArrayList<ExemptionComponent>(); 1198 for (ExemptionComponent i : exception) 1199 dst.exception.add(i.copy()); 1200 }; 1201 } 1202 1203 @Override 1204 public boolean equalsDeep(Base other_) { 1205 if (!super.equalsDeep(other_)) 1206 return false; 1207 if (!(other_ instanceof CostToBeneficiaryComponent)) 1208 return false; 1209 CostToBeneficiaryComponent o = (CostToBeneficiaryComponent) other_; 1210 return compareDeep(type, o.type, true) && compareDeep(category, o.category, true) && compareDeep(network, o.network, true) 1211 && compareDeep(unit, o.unit, true) && compareDeep(term, o.term, true) && compareDeep(value, o.value, true) 1212 && compareDeep(exception, o.exception, true); 1213 } 1214 1215 @Override 1216 public boolean equalsShallow(Base other_) { 1217 if (!super.equalsShallow(other_)) 1218 return false; 1219 if (!(other_ instanceof CostToBeneficiaryComponent)) 1220 return false; 1221 CostToBeneficiaryComponent o = (CostToBeneficiaryComponent) other_; 1222 return true; 1223 } 1224 1225 public boolean isEmpty() { 1226 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, category, network 1227 , unit, term, value, exception); 1228 } 1229 1230 public String fhirType() { 1231 return "Coverage.costToBeneficiary"; 1232 1233 } 1234 1235 } 1236 1237 @Block() 1238 public static class ExemptionComponent extends BackboneElement implements IBaseBackboneElement { 1239 /** 1240 * The code for the specific exception. 1241 */ 1242 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1243 @Description(shortDefinition="Exception category", formalDefinition="The code for the specific exception." ) 1244 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverage-financial-exception") 1245 protected CodeableConcept type; 1246 1247 /** 1248 * The timeframe the exception is in force. 1249 */ 1250 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 1251 @Description(shortDefinition="The effective period of the exception", formalDefinition="The timeframe the exception is in force." ) 1252 protected Period period; 1253 1254 private static final long serialVersionUID = 523191991L; 1255 1256 /** 1257 * Constructor 1258 */ 1259 public ExemptionComponent() { 1260 super(); 1261 } 1262 1263 /** 1264 * Constructor 1265 */ 1266 public ExemptionComponent(CodeableConcept type) { 1267 super(); 1268 this.setType(type); 1269 } 1270 1271 /** 1272 * @return {@link #type} (The code for the specific exception.) 1273 */ 1274 public CodeableConcept getType() { 1275 if (this.type == null) 1276 if (Configuration.errorOnAutoCreate()) 1277 throw new Error("Attempt to auto-create ExemptionComponent.type"); 1278 else if (Configuration.doAutoCreate()) 1279 this.type = new CodeableConcept(); // cc 1280 return this.type; 1281 } 1282 1283 public boolean hasType() { 1284 return this.type != null && !this.type.isEmpty(); 1285 } 1286 1287 /** 1288 * @param value {@link #type} (The code for the specific exception.) 1289 */ 1290 public ExemptionComponent setType(CodeableConcept value) { 1291 this.type = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return {@link #period} (The timeframe the exception is in force.) 1297 */ 1298 public Period getPeriod() { 1299 if (this.period == null) 1300 if (Configuration.errorOnAutoCreate()) 1301 throw new Error("Attempt to auto-create ExemptionComponent.period"); 1302 else if (Configuration.doAutoCreate()) 1303 this.period = new Period(); // cc 1304 return this.period; 1305 } 1306 1307 public boolean hasPeriod() { 1308 return this.period != null && !this.period.isEmpty(); 1309 } 1310 1311 /** 1312 * @param value {@link #period} (The timeframe the exception is in force.) 1313 */ 1314 public ExemptionComponent setPeriod(Period value) { 1315 this.period = value; 1316 return this; 1317 } 1318 1319 protected void listChildren(List<Property> children) { 1320 super.listChildren(children); 1321 children.add(new Property("type", "CodeableConcept", "The code for the specific exception.", 0, 1, type)); 1322 children.add(new Property("period", "Period", "The timeframe the exception is in force.", 0, 1, period)); 1323 } 1324 1325 @Override 1326 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1327 switch (_hash) { 1328 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The code for the specific exception.", 0, 1, type); 1329 case -991726143: /*period*/ return new Property("period", "Period", "The timeframe the exception is in force.", 0, 1, period); 1330 default: return super.getNamedProperty(_hash, _name, _checkValid); 1331 } 1332 1333 } 1334 1335 @Override 1336 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1337 switch (hash) { 1338 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1339 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1340 default: return super.getProperty(hash, name, checkValid); 1341 } 1342 1343 } 1344 1345 @Override 1346 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1347 switch (hash) { 1348 case 3575610: // type 1349 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1350 return value; 1351 case -991726143: // period 1352 this.period = TypeConvertor.castToPeriod(value); // Period 1353 return value; 1354 default: return super.setProperty(hash, name, value); 1355 } 1356 1357 } 1358 1359 @Override 1360 public Base setProperty(String name, Base value) throws FHIRException { 1361 if (name.equals("type")) { 1362 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1363 } else if (name.equals("period")) { 1364 this.period = TypeConvertor.castToPeriod(value); // Period 1365 } else 1366 return super.setProperty(name, value); 1367 return value; 1368 } 1369 1370 @Override 1371 public void removeChild(String name, Base value) throws FHIRException { 1372 if (name.equals("type")) { 1373 this.type = null; 1374 } else if (name.equals("period")) { 1375 this.period = null; 1376 } else 1377 super.removeChild(name, value); 1378 1379 } 1380 1381 @Override 1382 public Base makeProperty(int hash, String name) throws FHIRException { 1383 switch (hash) { 1384 case 3575610: return getType(); 1385 case -991726143: return getPeriod(); 1386 default: return super.makeProperty(hash, name); 1387 } 1388 1389 } 1390 1391 @Override 1392 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1393 switch (hash) { 1394 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1395 case -991726143: /*period*/ return new String[] {"Period"}; 1396 default: return super.getTypesForProperty(hash, name); 1397 } 1398 1399 } 1400 1401 @Override 1402 public Base addChild(String name) throws FHIRException { 1403 if (name.equals("type")) { 1404 this.type = new CodeableConcept(); 1405 return this.type; 1406 } 1407 else if (name.equals("period")) { 1408 this.period = new Period(); 1409 return this.period; 1410 } 1411 else 1412 return super.addChild(name); 1413 } 1414 1415 public ExemptionComponent copy() { 1416 ExemptionComponent dst = new ExemptionComponent(); 1417 copyValues(dst); 1418 return dst; 1419 } 1420 1421 public void copyValues(ExemptionComponent dst) { 1422 super.copyValues(dst); 1423 dst.type = type == null ? null : type.copy(); 1424 dst.period = period == null ? null : period.copy(); 1425 } 1426 1427 @Override 1428 public boolean equalsDeep(Base other_) { 1429 if (!super.equalsDeep(other_)) 1430 return false; 1431 if (!(other_ instanceof ExemptionComponent)) 1432 return false; 1433 ExemptionComponent o = (ExemptionComponent) other_; 1434 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true); 1435 } 1436 1437 @Override 1438 public boolean equalsShallow(Base other_) { 1439 if (!super.equalsShallow(other_)) 1440 return false; 1441 if (!(other_ instanceof ExemptionComponent)) 1442 return false; 1443 ExemptionComponent o = (ExemptionComponent) other_; 1444 return true; 1445 } 1446 1447 public boolean isEmpty() { 1448 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period); 1449 } 1450 1451 public String fhirType() { 1452 return "Coverage.costToBeneficiary.exception"; 1453 1454 } 1455 1456 } 1457 1458 /** 1459 * The identifier of the coverage as issued by the insurer. 1460 */ 1461 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1462 @Description(shortDefinition="Business identifier(s) for this coverage", formalDefinition="The identifier of the coverage as issued by the insurer." ) 1463 protected List<Identifier> identifier; 1464 1465 /** 1466 * The status of the resource instance. 1467 */ 1468 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1469 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 1470 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 1471 protected Enumeration<FinancialResourceStatusCodes> status; 1472 1473 /** 1474 * The nature of the coverage be it insurance, or cash payment such as self-pay. 1475 */ 1476 @Child(name = "kind", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1477 @Description(shortDefinition="insurance | self-pay | other", formalDefinition="The nature of the coverage be it insurance, or cash payment such as self-pay." ) 1478 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverage-kind") 1479 protected Enumeration<Kind> kind; 1480 1481 /** 1482 * Link to the paying party and optionally what specifically they will be responsible to pay. 1483 */ 1484 @Child(name = "paymentBy", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1485 @Description(shortDefinition="Self-pay parties and responsibility", formalDefinition="Link to the paying party and optionally what specifically they will be responsible to pay." ) 1486 protected List<CoveragePaymentByComponent> paymentBy; 1487 1488 /** 1489 * The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization. 1490 */ 1491 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1492 @Description(shortDefinition="Coverage category such as medical or accident", formalDefinition="The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization." ) 1493 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverage-type") 1494 protected CodeableConcept type; 1495 1496 /** 1497 * The party who 'owns' the insurance policy. 1498 */ 1499 @Child(name = "policyHolder", type = {Patient.class, RelatedPerson.class, Organization.class}, order=5, min=0, max=1, modifier=false, summary=true) 1500 @Description(shortDefinition="Owner of the policy", formalDefinition="The party who 'owns' the insurance policy." ) 1501 protected Reference policyHolder; 1502 1503 /** 1504 * The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due. 1505 */ 1506 @Child(name = "subscriber", type = {Patient.class, RelatedPerson.class}, order=6, min=0, max=1, modifier=false, summary=true) 1507 @Description(shortDefinition="Subscriber to the policy", formalDefinition="The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due." ) 1508 protected Reference subscriber; 1509 1510 /** 1511 * The insurer assigned ID for the Subscriber. 1512 */ 1513 @Child(name = "subscriberId", type = {Identifier.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1514 @Description(shortDefinition="ID assigned to the subscriber", formalDefinition="The insurer assigned ID for the Subscriber." ) 1515 protected List<Identifier> subscriberId; 1516 1517 /** 1518 * The party who benefits from the insurance coverage; the patient when products and/or services are provided. 1519 */ 1520 @Child(name = "beneficiary", type = {Patient.class}, order=8, min=1, max=1, modifier=false, summary=true) 1521 @Description(shortDefinition="Plan beneficiary", formalDefinition="The party who benefits from the insurance coverage; the patient when products and/or services are provided." ) 1522 protected Reference beneficiary; 1523 1524 /** 1525 * A designator for a dependent under the coverage. 1526 */ 1527 @Child(name = "dependent", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1528 @Description(shortDefinition="Dependent number", formalDefinition="A designator for a dependent under the coverage." ) 1529 protected StringType dependent; 1530 1531 /** 1532 * The relationship of beneficiary (patient) to the subscriber. 1533 */ 1534 @Child(name = "relationship", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 1535 @Description(shortDefinition="Beneficiary relationship to the subscriber", formalDefinition="The relationship of beneficiary (patient) to the subscriber." ) 1536 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscriber-relationship") 1537 protected CodeableConcept relationship; 1538 1539 /** 1540 * Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force. 1541 */ 1542 @Child(name = "period", type = {Period.class}, order=11, min=0, max=1, modifier=false, summary=true) 1543 @Description(shortDefinition="Coverage start and end dates", formalDefinition="Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force." ) 1544 protected Period period; 1545 1546 /** 1547 * The program or plan underwriter, payor, insurance company. 1548 */ 1549 @Child(name = "insurer", type = {Organization.class}, order=12, min=0, max=1, modifier=false, summary=true) 1550 @Description(shortDefinition="Issuer of the policy", formalDefinition="The program or plan underwriter, payor, insurance company." ) 1551 protected Reference insurer; 1552 1553 /** 1554 * A suite of underwriter specific classifiers. 1555 */ 1556 @Child(name = "class", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1557 @Description(shortDefinition="Additional coverage classifications", formalDefinition="A suite of underwriter specific classifiers." ) 1558 protected List<ClassComponent> class_; 1559 1560 /** 1561 * The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis. 1562 */ 1563 @Child(name = "order", type = {PositiveIntType.class}, order=14, min=0, max=1, modifier=false, summary=true) 1564 @Description(shortDefinition="Relative order of the coverage", formalDefinition="The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis." ) 1565 protected PositiveIntType order; 1566 1567 /** 1568 * The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1569 */ 1570 @Child(name = "network", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=true) 1571 @Description(shortDefinition="Insurer network", formalDefinition="The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply." ) 1572 protected StringType network; 1573 1574 /** 1575 * A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card. 1576 */ 1577 @Child(name = "costToBeneficiary", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1578 @Description(shortDefinition="Patient payments for services/products", formalDefinition="A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card." ) 1579 protected List<CostToBeneficiaryComponent> costToBeneficiary; 1580 1581 /** 1582 * When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs. 1583 */ 1584 @Child(name = "subrogation", type = {BooleanType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1585 @Description(shortDefinition="Reimbursement to insurer", formalDefinition="When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs." ) 1586 protected BooleanType subrogation; 1587 1588 /** 1589 * The policy(s) which constitute this insurance coverage. 1590 */ 1591 @Child(name = "contract", type = {Contract.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1592 @Description(shortDefinition="Contract details", formalDefinition="The policy(s) which constitute this insurance coverage." ) 1593 protected List<Reference> contract; 1594 1595 /** 1596 * The insurance plan details, benefits and costs, which constitute this insurance coverage. 1597 */ 1598 @Child(name = "insurancePlan", type = {InsurancePlan.class}, order=19, min=0, max=1, modifier=false, summary=false) 1599 @Description(shortDefinition="Insurance plan details", formalDefinition="The insurance plan details, benefits and costs, which constitute this insurance coverage." ) 1600 protected Reference insurancePlan; 1601 1602 private static final long serialVersionUID = -1129388911L; 1603 1604 /** 1605 * Constructor 1606 */ 1607 public Coverage() { 1608 super(); 1609 } 1610 1611 /** 1612 * Constructor 1613 */ 1614 public Coverage(FinancialResourceStatusCodes status, Kind kind, Reference beneficiary) { 1615 super(); 1616 this.setStatus(status); 1617 this.setKind(kind); 1618 this.setBeneficiary(beneficiary); 1619 } 1620 1621 /** 1622 * @return {@link #identifier} (The identifier of the coverage as issued by the insurer.) 1623 */ 1624 public List<Identifier> getIdentifier() { 1625 if (this.identifier == null) 1626 this.identifier = new ArrayList<Identifier>(); 1627 return this.identifier; 1628 } 1629 1630 /** 1631 * @return Returns a reference to <code>this</code> for easy method chaining 1632 */ 1633 public Coverage setIdentifier(List<Identifier> theIdentifier) { 1634 this.identifier = theIdentifier; 1635 return this; 1636 } 1637 1638 public boolean hasIdentifier() { 1639 if (this.identifier == null) 1640 return false; 1641 for (Identifier item : this.identifier) 1642 if (!item.isEmpty()) 1643 return true; 1644 return false; 1645 } 1646 1647 public Identifier addIdentifier() { //3 1648 Identifier t = new Identifier(); 1649 if (this.identifier == null) 1650 this.identifier = new ArrayList<Identifier>(); 1651 this.identifier.add(t); 1652 return t; 1653 } 1654 1655 public Coverage addIdentifier(Identifier t) { //3 1656 if (t == null) 1657 return this; 1658 if (this.identifier == null) 1659 this.identifier = new ArrayList<Identifier>(); 1660 this.identifier.add(t); 1661 return this; 1662 } 1663 1664 /** 1665 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1666 */ 1667 public Identifier getIdentifierFirstRep() { 1668 if (getIdentifier().isEmpty()) { 1669 addIdentifier(); 1670 } 1671 return getIdentifier().get(0); 1672 } 1673 1674 /** 1675 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1676 */ 1677 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 1678 if (this.status == null) 1679 if (Configuration.errorOnAutoCreate()) 1680 throw new Error("Attempt to auto-create Coverage.status"); 1681 else if (Configuration.doAutoCreate()) 1682 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 1683 return this.status; 1684 } 1685 1686 public boolean hasStatusElement() { 1687 return this.status != null && !this.status.isEmpty(); 1688 } 1689 1690 public boolean hasStatus() { 1691 return this.status != null && !this.status.isEmpty(); 1692 } 1693 1694 /** 1695 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1696 */ 1697 public Coverage setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 1698 this.status = value; 1699 return this; 1700 } 1701 1702 /** 1703 * @return The status of the resource instance. 1704 */ 1705 public FinancialResourceStatusCodes getStatus() { 1706 return this.status == null ? null : this.status.getValue(); 1707 } 1708 1709 /** 1710 * @param value The status of the resource instance. 1711 */ 1712 public Coverage setStatus(FinancialResourceStatusCodes value) { 1713 if (this.status == null) 1714 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 1715 this.status.setValue(value); 1716 return this; 1717 } 1718 1719 /** 1720 * @return {@link #kind} (The nature of the coverage be it insurance, or cash payment such as self-pay.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1721 */ 1722 public Enumeration<Kind> getKindElement() { 1723 if (this.kind == null) 1724 if (Configuration.errorOnAutoCreate()) 1725 throw new Error("Attempt to auto-create Coverage.kind"); 1726 else if (Configuration.doAutoCreate()) 1727 this.kind = new Enumeration<Kind>(new KindEnumFactory()); // bb 1728 return this.kind; 1729 } 1730 1731 public boolean hasKindElement() { 1732 return this.kind != null && !this.kind.isEmpty(); 1733 } 1734 1735 public boolean hasKind() { 1736 return this.kind != null && !this.kind.isEmpty(); 1737 } 1738 1739 /** 1740 * @param value {@link #kind} (The nature of the coverage be it insurance, or cash payment such as self-pay.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1741 */ 1742 public Coverage setKindElement(Enumeration<Kind> value) { 1743 this.kind = value; 1744 return this; 1745 } 1746 1747 /** 1748 * @return The nature of the coverage be it insurance, or cash payment such as self-pay. 1749 */ 1750 public Kind getKind() { 1751 return this.kind == null ? null : this.kind.getValue(); 1752 } 1753 1754 /** 1755 * @param value The nature of the coverage be it insurance, or cash payment such as self-pay. 1756 */ 1757 public Coverage setKind(Kind value) { 1758 if (this.kind == null) 1759 this.kind = new Enumeration<Kind>(new KindEnumFactory()); 1760 this.kind.setValue(value); 1761 return this; 1762 } 1763 1764 /** 1765 * @return {@link #paymentBy} (Link to the paying party and optionally what specifically they will be responsible to pay.) 1766 */ 1767 public List<CoveragePaymentByComponent> getPaymentBy() { 1768 if (this.paymentBy == null) 1769 this.paymentBy = new ArrayList<CoveragePaymentByComponent>(); 1770 return this.paymentBy; 1771 } 1772 1773 /** 1774 * @return Returns a reference to <code>this</code> for easy method chaining 1775 */ 1776 public Coverage setPaymentBy(List<CoveragePaymentByComponent> thePaymentBy) { 1777 this.paymentBy = thePaymentBy; 1778 return this; 1779 } 1780 1781 public boolean hasPaymentBy() { 1782 if (this.paymentBy == null) 1783 return false; 1784 for (CoveragePaymentByComponent item : this.paymentBy) 1785 if (!item.isEmpty()) 1786 return true; 1787 return false; 1788 } 1789 1790 public CoveragePaymentByComponent addPaymentBy() { //3 1791 CoveragePaymentByComponent t = new CoveragePaymentByComponent(); 1792 if (this.paymentBy == null) 1793 this.paymentBy = new ArrayList<CoveragePaymentByComponent>(); 1794 this.paymentBy.add(t); 1795 return t; 1796 } 1797 1798 public Coverage addPaymentBy(CoveragePaymentByComponent t) { //3 1799 if (t == null) 1800 return this; 1801 if (this.paymentBy == null) 1802 this.paymentBy = new ArrayList<CoveragePaymentByComponent>(); 1803 this.paymentBy.add(t); 1804 return this; 1805 } 1806 1807 /** 1808 * @return The first repetition of repeating field {@link #paymentBy}, creating it if it does not already exist {3} 1809 */ 1810 public CoveragePaymentByComponent getPaymentByFirstRep() { 1811 if (getPaymentBy().isEmpty()) { 1812 addPaymentBy(); 1813 } 1814 return getPaymentBy().get(0); 1815 } 1816 1817 /** 1818 * @return {@link #type} (The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.) 1819 */ 1820 public CodeableConcept getType() { 1821 if (this.type == null) 1822 if (Configuration.errorOnAutoCreate()) 1823 throw new Error("Attempt to auto-create Coverage.type"); 1824 else if (Configuration.doAutoCreate()) 1825 this.type = new CodeableConcept(); // cc 1826 return this.type; 1827 } 1828 1829 public boolean hasType() { 1830 return this.type != null && !this.type.isEmpty(); 1831 } 1832 1833 /** 1834 * @param value {@link #type} (The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.) 1835 */ 1836 public Coverage setType(CodeableConcept value) { 1837 this.type = value; 1838 return this; 1839 } 1840 1841 /** 1842 * @return {@link #policyHolder} (The party who 'owns' the insurance policy.) 1843 */ 1844 public Reference getPolicyHolder() { 1845 if (this.policyHolder == null) 1846 if (Configuration.errorOnAutoCreate()) 1847 throw new Error("Attempt to auto-create Coverage.policyHolder"); 1848 else if (Configuration.doAutoCreate()) 1849 this.policyHolder = new Reference(); // cc 1850 return this.policyHolder; 1851 } 1852 1853 public boolean hasPolicyHolder() { 1854 return this.policyHolder != null && !this.policyHolder.isEmpty(); 1855 } 1856 1857 /** 1858 * @param value {@link #policyHolder} (The party who 'owns' the insurance policy.) 1859 */ 1860 public Coverage setPolicyHolder(Reference value) { 1861 this.policyHolder = value; 1862 return this; 1863 } 1864 1865 /** 1866 * @return {@link #subscriber} (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1867 */ 1868 public Reference getSubscriber() { 1869 if (this.subscriber == null) 1870 if (Configuration.errorOnAutoCreate()) 1871 throw new Error("Attempt to auto-create Coverage.subscriber"); 1872 else if (Configuration.doAutoCreate()) 1873 this.subscriber = new Reference(); // cc 1874 return this.subscriber; 1875 } 1876 1877 public boolean hasSubscriber() { 1878 return this.subscriber != null && !this.subscriber.isEmpty(); 1879 } 1880 1881 /** 1882 * @param value {@link #subscriber} (The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.) 1883 */ 1884 public Coverage setSubscriber(Reference value) { 1885 this.subscriber = value; 1886 return this; 1887 } 1888 1889 /** 1890 * @return {@link #subscriberId} (The insurer assigned ID for the Subscriber.) 1891 */ 1892 public List<Identifier> getSubscriberId() { 1893 if (this.subscriberId == null) 1894 this.subscriberId = new ArrayList<Identifier>(); 1895 return this.subscriberId; 1896 } 1897 1898 /** 1899 * @return Returns a reference to <code>this</code> for easy method chaining 1900 */ 1901 public Coverage setSubscriberId(List<Identifier> theSubscriberId) { 1902 this.subscriberId = theSubscriberId; 1903 return this; 1904 } 1905 1906 public boolean hasSubscriberId() { 1907 if (this.subscriberId == null) 1908 return false; 1909 for (Identifier item : this.subscriberId) 1910 if (!item.isEmpty()) 1911 return true; 1912 return false; 1913 } 1914 1915 public Identifier addSubscriberId() { //3 1916 Identifier t = new Identifier(); 1917 if (this.subscriberId == null) 1918 this.subscriberId = new ArrayList<Identifier>(); 1919 this.subscriberId.add(t); 1920 return t; 1921 } 1922 1923 public Coverage addSubscriberId(Identifier t) { //3 1924 if (t == null) 1925 return this; 1926 if (this.subscriberId == null) 1927 this.subscriberId = new ArrayList<Identifier>(); 1928 this.subscriberId.add(t); 1929 return this; 1930 } 1931 1932 /** 1933 * @return The first repetition of repeating field {@link #subscriberId}, creating it if it does not already exist {3} 1934 */ 1935 public Identifier getSubscriberIdFirstRep() { 1936 if (getSubscriberId().isEmpty()) { 1937 addSubscriberId(); 1938 } 1939 return getSubscriberId().get(0); 1940 } 1941 1942 /** 1943 * @return {@link #beneficiary} (The party who benefits from the insurance coverage; the patient when products and/or services are provided.) 1944 */ 1945 public Reference getBeneficiary() { 1946 if (this.beneficiary == null) 1947 if (Configuration.errorOnAutoCreate()) 1948 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1949 else if (Configuration.doAutoCreate()) 1950 this.beneficiary = new Reference(); // cc 1951 return this.beneficiary; 1952 } 1953 1954 public boolean hasBeneficiary() { 1955 return this.beneficiary != null && !this.beneficiary.isEmpty(); 1956 } 1957 1958 /** 1959 * @param value {@link #beneficiary} (The party who benefits from the insurance coverage; the patient when products and/or services are provided.) 1960 */ 1961 public Coverage setBeneficiary(Reference value) { 1962 this.beneficiary = value; 1963 return this; 1964 } 1965 1966 /** 1967 * @return {@link #dependent} (A designator for a dependent under the coverage.). This is the underlying object with id, value and extensions. The accessor "getDependent" gives direct access to the value 1968 */ 1969 public StringType getDependentElement() { 1970 if (this.dependent == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create Coverage.dependent"); 1973 else if (Configuration.doAutoCreate()) 1974 this.dependent = new StringType(); // bb 1975 return this.dependent; 1976 } 1977 1978 public boolean hasDependentElement() { 1979 return this.dependent != null && !this.dependent.isEmpty(); 1980 } 1981 1982 public boolean hasDependent() { 1983 return this.dependent != null && !this.dependent.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #dependent} (A designator for a dependent under the coverage.). This is the underlying object with id, value and extensions. The accessor "getDependent" gives direct access to the value 1988 */ 1989 public Coverage setDependentElement(StringType value) { 1990 this.dependent = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return A designator for a dependent under the coverage. 1996 */ 1997 public String getDependent() { 1998 return this.dependent == null ? null : this.dependent.getValue(); 1999 } 2000 2001 /** 2002 * @param value A designator for a dependent under the coverage. 2003 */ 2004 public Coverage setDependent(String value) { 2005 if (Utilities.noString(value)) 2006 this.dependent = null; 2007 else { 2008 if (this.dependent == null) 2009 this.dependent = new StringType(); 2010 this.dependent.setValue(value); 2011 } 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #relationship} (The relationship of beneficiary (patient) to the subscriber.) 2017 */ 2018 public CodeableConcept getRelationship() { 2019 if (this.relationship == null) 2020 if (Configuration.errorOnAutoCreate()) 2021 throw new Error("Attempt to auto-create Coverage.relationship"); 2022 else if (Configuration.doAutoCreate()) 2023 this.relationship = new CodeableConcept(); // cc 2024 return this.relationship; 2025 } 2026 2027 public boolean hasRelationship() { 2028 return this.relationship != null && !this.relationship.isEmpty(); 2029 } 2030 2031 /** 2032 * @param value {@link #relationship} (The relationship of beneficiary (patient) to the subscriber.) 2033 */ 2034 public Coverage setRelationship(CodeableConcept value) { 2035 this.relationship = value; 2036 return this; 2037 } 2038 2039 /** 2040 * @return {@link #period} (Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.) 2041 */ 2042 public Period getPeriod() { 2043 if (this.period == null) 2044 if (Configuration.errorOnAutoCreate()) 2045 throw new Error("Attempt to auto-create Coverage.period"); 2046 else if (Configuration.doAutoCreate()) 2047 this.period = new Period(); // cc 2048 return this.period; 2049 } 2050 2051 public boolean hasPeriod() { 2052 return this.period != null && !this.period.isEmpty(); 2053 } 2054 2055 /** 2056 * @param value {@link #period} (Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.) 2057 */ 2058 public Coverage setPeriod(Period value) { 2059 this.period = value; 2060 return this; 2061 } 2062 2063 /** 2064 * @return {@link #insurer} (The program or plan underwriter, payor, insurance company.) 2065 */ 2066 public Reference getInsurer() { 2067 if (this.insurer == null) 2068 if (Configuration.errorOnAutoCreate()) 2069 throw new Error("Attempt to auto-create Coverage.insurer"); 2070 else if (Configuration.doAutoCreate()) 2071 this.insurer = new Reference(); // cc 2072 return this.insurer; 2073 } 2074 2075 public boolean hasInsurer() { 2076 return this.insurer != null && !this.insurer.isEmpty(); 2077 } 2078 2079 /** 2080 * @param value {@link #insurer} (The program or plan underwriter, payor, insurance company.) 2081 */ 2082 public Coverage setInsurer(Reference value) { 2083 this.insurer = value; 2084 return this; 2085 } 2086 2087 /** 2088 * @return {@link #class_} (A suite of underwriter specific classifiers.) 2089 */ 2090 public List<ClassComponent> getClass_() { 2091 if (this.class_ == null) 2092 this.class_ = new ArrayList<ClassComponent>(); 2093 return this.class_; 2094 } 2095 2096 /** 2097 * @return Returns a reference to <code>this</code> for easy method chaining 2098 */ 2099 public Coverage setClass_(List<ClassComponent> theClass_) { 2100 this.class_ = theClass_; 2101 return this; 2102 } 2103 2104 public boolean hasClass_() { 2105 if (this.class_ == null) 2106 return false; 2107 for (ClassComponent item : this.class_) 2108 if (!item.isEmpty()) 2109 return true; 2110 return false; 2111 } 2112 2113 public ClassComponent addClass_() { //3 2114 ClassComponent t = new ClassComponent(); 2115 if (this.class_ == null) 2116 this.class_ = new ArrayList<ClassComponent>(); 2117 this.class_.add(t); 2118 return t; 2119 } 2120 2121 public Coverage addClass_(ClassComponent t) { //3 2122 if (t == null) 2123 return this; 2124 if (this.class_ == null) 2125 this.class_ = new ArrayList<ClassComponent>(); 2126 this.class_.add(t); 2127 return this; 2128 } 2129 2130 /** 2131 * @return The first repetition of repeating field {@link #class_}, creating it if it does not already exist {3} 2132 */ 2133 public ClassComponent getClass_FirstRep() { 2134 if (getClass_().isEmpty()) { 2135 addClass_(); 2136 } 2137 return getClass_().get(0); 2138 } 2139 2140 /** 2141 * @return {@link #order} (The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis.). This is the underlying object with id, value and extensions. The accessor "getOrder" gives direct access to the value 2142 */ 2143 public PositiveIntType getOrderElement() { 2144 if (this.order == null) 2145 if (Configuration.errorOnAutoCreate()) 2146 throw new Error("Attempt to auto-create Coverage.order"); 2147 else if (Configuration.doAutoCreate()) 2148 this.order = new PositiveIntType(); // bb 2149 return this.order; 2150 } 2151 2152 public boolean hasOrderElement() { 2153 return this.order != null && !this.order.isEmpty(); 2154 } 2155 2156 public boolean hasOrder() { 2157 return this.order != null && !this.order.isEmpty(); 2158 } 2159 2160 /** 2161 * @param value {@link #order} (The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis.). This is the underlying object with id, value and extensions. The accessor "getOrder" gives direct access to the value 2162 */ 2163 public Coverage setOrderElement(PositiveIntType value) { 2164 this.order = value; 2165 return this; 2166 } 2167 2168 /** 2169 * @return The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis. 2170 */ 2171 public int getOrder() { 2172 return this.order == null || this.order.isEmpty() ? 0 : this.order.getValue(); 2173 } 2174 2175 /** 2176 * @param value The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis. 2177 */ 2178 public Coverage setOrder(int value) { 2179 if (this.order == null) 2180 this.order = new PositiveIntType(); 2181 this.order.setValue(value); 2182 return this; 2183 } 2184 2185 /** 2186 * @return {@link #network} (The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.). This is the underlying object with id, value and extensions. The accessor "getNetwork" gives direct access to the value 2187 */ 2188 public StringType getNetworkElement() { 2189 if (this.network == null) 2190 if (Configuration.errorOnAutoCreate()) 2191 throw new Error("Attempt to auto-create Coverage.network"); 2192 else if (Configuration.doAutoCreate()) 2193 this.network = new StringType(); // bb 2194 return this.network; 2195 } 2196 2197 public boolean hasNetworkElement() { 2198 return this.network != null && !this.network.isEmpty(); 2199 } 2200 2201 public boolean hasNetwork() { 2202 return this.network != null && !this.network.isEmpty(); 2203 } 2204 2205 /** 2206 * @param value {@link #network} (The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.). This is the underlying object with id, value and extensions. The accessor "getNetwork" gives direct access to the value 2207 */ 2208 public Coverage setNetworkElement(StringType value) { 2209 this.network = value; 2210 return this; 2211 } 2212 2213 /** 2214 * @return The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 2215 */ 2216 public String getNetwork() { 2217 return this.network == null ? null : this.network.getValue(); 2218 } 2219 2220 /** 2221 * @param value The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply. 2222 */ 2223 public Coverage setNetwork(String value) { 2224 if (Utilities.noString(value)) 2225 this.network = null; 2226 else { 2227 if (this.network == null) 2228 this.network = new StringType(); 2229 this.network.setValue(value); 2230 } 2231 return this; 2232 } 2233 2234 /** 2235 * @return {@link #costToBeneficiary} (A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.) 2236 */ 2237 public List<CostToBeneficiaryComponent> getCostToBeneficiary() { 2238 if (this.costToBeneficiary == null) 2239 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2240 return this.costToBeneficiary; 2241 } 2242 2243 /** 2244 * @return Returns a reference to <code>this</code> for easy method chaining 2245 */ 2246 public Coverage setCostToBeneficiary(List<CostToBeneficiaryComponent> theCostToBeneficiary) { 2247 this.costToBeneficiary = theCostToBeneficiary; 2248 return this; 2249 } 2250 2251 public boolean hasCostToBeneficiary() { 2252 if (this.costToBeneficiary == null) 2253 return false; 2254 for (CostToBeneficiaryComponent item : this.costToBeneficiary) 2255 if (!item.isEmpty()) 2256 return true; 2257 return false; 2258 } 2259 2260 public CostToBeneficiaryComponent addCostToBeneficiary() { //3 2261 CostToBeneficiaryComponent t = new CostToBeneficiaryComponent(); 2262 if (this.costToBeneficiary == null) 2263 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2264 this.costToBeneficiary.add(t); 2265 return t; 2266 } 2267 2268 public Coverage addCostToBeneficiary(CostToBeneficiaryComponent t) { //3 2269 if (t == null) 2270 return this; 2271 if (this.costToBeneficiary == null) 2272 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2273 this.costToBeneficiary.add(t); 2274 return this; 2275 } 2276 2277 /** 2278 * @return The first repetition of repeating field {@link #costToBeneficiary}, creating it if it does not already exist {3} 2279 */ 2280 public CostToBeneficiaryComponent getCostToBeneficiaryFirstRep() { 2281 if (getCostToBeneficiary().isEmpty()) { 2282 addCostToBeneficiary(); 2283 } 2284 return getCostToBeneficiary().get(0); 2285 } 2286 2287 /** 2288 * @return {@link #subrogation} (When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.). This is the underlying object with id, value and extensions. The accessor "getSubrogation" gives direct access to the value 2289 */ 2290 public BooleanType getSubrogationElement() { 2291 if (this.subrogation == null) 2292 if (Configuration.errorOnAutoCreate()) 2293 throw new Error("Attempt to auto-create Coverage.subrogation"); 2294 else if (Configuration.doAutoCreate()) 2295 this.subrogation = new BooleanType(); // bb 2296 return this.subrogation; 2297 } 2298 2299 public boolean hasSubrogationElement() { 2300 return this.subrogation != null && !this.subrogation.isEmpty(); 2301 } 2302 2303 public boolean hasSubrogation() { 2304 return this.subrogation != null && !this.subrogation.isEmpty(); 2305 } 2306 2307 /** 2308 * @param value {@link #subrogation} (When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.). This is the underlying object with id, value and extensions. The accessor "getSubrogation" gives direct access to the value 2309 */ 2310 public Coverage setSubrogationElement(BooleanType value) { 2311 this.subrogation = value; 2312 return this; 2313 } 2314 2315 /** 2316 * @return When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs. 2317 */ 2318 public boolean getSubrogation() { 2319 return this.subrogation == null || this.subrogation.isEmpty() ? false : this.subrogation.getValue(); 2320 } 2321 2322 /** 2323 * @param value When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs. 2324 */ 2325 public Coverage setSubrogation(boolean value) { 2326 if (this.subrogation == null) 2327 this.subrogation = new BooleanType(); 2328 this.subrogation.setValue(value); 2329 return this; 2330 } 2331 2332 /** 2333 * @return {@link #contract} (The policy(s) which constitute this insurance coverage.) 2334 */ 2335 public List<Reference> getContract() { 2336 if (this.contract == null) 2337 this.contract = new ArrayList<Reference>(); 2338 return this.contract; 2339 } 2340 2341 /** 2342 * @return Returns a reference to <code>this</code> for easy method chaining 2343 */ 2344 public Coverage setContract(List<Reference> theContract) { 2345 this.contract = theContract; 2346 return this; 2347 } 2348 2349 public boolean hasContract() { 2350 if (this.contract == null) 2351 return false; 2352 for (Reference item : this.contract) 2353 if (!item.isEmpty()) 2354 return true; 2355 return false; 2356 } 2357 2358 public Reference addContract() { //3 2359 Reference t = new Reference(); 2360 if (this.contract == null) 2361 this.contract = new ArrayList<Reference>(); 2362 this.contract.add(t); 2363 return t; 2364 } 2365 2366 public Coverage addContract(Reference t) { //3 2367 if (t == null) 2368 return this; 2369 if (this.contract == null) 2370 this.contract = new ArrayList<Reference>(); 2371 this.contract.add(t); 2372 return this; 2373 } 2374 2375 /** 2376 * @return The first repetition of repeating field {@link #contract}, creating it if it does not already exist {3} 2377 */ 2378 public Reference getContractFirstRep() { 2379 if (getContract().isEmpty()) { 2380 addContract(); 2381 } 2382 return getContract().get(0); 2383 } 2384 2385 /** 2386 * @return {@link #insurancePlan} (The insurance plan details, benefits and costs, which constitute this insurance coverage.) 2387 */ 2388 public Reference getInsurancePlan() { 2389 if (this.insurancePlan == null) 2390 if (Configuration.errorOnAutoCreate()) 2391 throw new Error("Attempt to auto-create Coverage.insurancePlan"); 2392 else if (Configuration.doAutoCreate()) 2393 this.insurancePlan = new Reference(); // cc 2394 return this.insurancePlan; 2395 } 2396 2397 public boolean hasInsurancePlan() { 2398 return this.insurancePlan != null && !this.insurancePlan.isEmpty(); 2399 } 2400 2401 /** 2402 * @param value {@link #insurancePlan} (The insurance plan details, benefits and costs, which constitute this insurance coverage.) 2403 */ 2404 public Coverage setInsurancePlan(Reference value) { 2405 this.insurancePlan = value; 2406 return this; 2407 } 2408 2409 protected void listChildren(List<Property> children) { 2410 super.listChildren(children); 2411 children.add(new Property("identifier", "Identifier", "The identifier of the coverage as issued by the insurer.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2412 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2413 children.add(new Property("kind", "code", "The nature of the coverage be it insurance, or cash payment such as self-pay.", 0, 1, kind)); 2414 children.add(new Property("paymentBy", "", "Link to the paying party and optionally what specifically they will be responsible to pay.", 0, java.lang.Integer.MAX_VALUE, paymentBy)); 2415 children.add(new Property("type", "CodeableConcept", "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 0, 1, type)); 2416 children.add(new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", "The party who 'owns' the insurance policy.", 0, 1, policyHolder)); 2417 children.add(new Property("subscriber", "Reference(Patient|RelatedPerson)", "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 0, 1, subscriber)); 2418 children.add(new Property("subscriberId", "Identifier", "The insurer assigned ID for the Subscriber.", 0, java.lang.Integer.MAX_VALUE, subscriberId)); 2419 children.add(new Property("beneficiary", "Reference(Patient)", "The party who benefits from the insurance coverage; the patient when products and/or services are provided.", 0, 1, beneficiary)); 2420 children.add(new Property("dependent", "string", "A designator for a dependent under the coverage.", 0, 1, dependent)); 2421 children.add(new Property("relationship", "CodeableConcept", "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship)); 2422 children.add(new Property("period", "Period", "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 0, 1, period)); 2423 children.add(new Property("insurer", "Reference(Organization)", "The program or plan underwriter, payor, insurance company.", 0, 1, insurer)); 2424 children.add(new Property("class", "", "A suite of underwriter specific classifiers.", 0, java.lang.Integer.MAX_VALUE, class_)); 2425 children.add(new Property("order", "positiveInt", "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis.", 0, 1, order)); 2426 children.add(new Property("network", "string", "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 0, 1, network)); 2427 children.add(new Property("costToBeneficiary", "", "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.", 0, java.lang.Integer.MAX_VALUE, costToBeneficiary)); 2428 children.add(new Property("subrogation", "boolean", "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.", 0, 1, subrogation)); 2429 children.add(new Property("contract", "Reference(Contract)", "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract)); 2430 children.add(new Property("insurancePlan", "Reference(InsurancePlan)", "The insurance plan details, benefits and costs, which constitute this insurance coverage.", 0, 1, insurancePlan)); 2431 } 2432 2433 @Override 2434 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2435 switch (_hash) { 2436 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier of the coverage as issued by the insurer.", 0, java.lang.Integer.MAX_VALUE, identifier); 2437 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2438 case 3292052: /*kind*/ return new Property("kind", "code", "The nature of the coverage be it insurance, or cash payment such as self-pay.", 0, 1, kind); 2439 case -86519555: /*paymentBy*/ return new Property("paymentBy", "", "Link to the paying party and optionally what specifically they will be responsible to pay.", 0, java.lang.Integer.MAX_VALUE, paymentBy); 2440 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 0, 1, type); 2441 case 2046898558: /*policyHolder*/ return new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", "The party who 'owns' the insurance policy.", 0, 1, policyHolder); 2442 case -1219769240: /*subscriber*/ return new Property("subscriber", "Reference(Patient|RelatedPerson)", "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 0, 1, subscriber); 2443 case 327834531: /*subscriberId*/ return new Property("subscriberId", "Identifier", "The insurer assigned ID for the Subscriber.", 0, java.lang.Integer.MAX_VALUE, subscriberId); 2444 case -565102875: /*beneficiary*/ return new Property("beneficiary", "Reference(Patient)", "The party who benefits from the insurance coverage; the patient when products and/or services are provided.", 0, 1, beneficiary); 2445 case -1109226753: /*dependent*/ return new Property("dependent", "string", "A designator for a dependent under the coverage.", 0, 1, dependent); 2446 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship); 2447 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 0, 1, period); 2448 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The program or plan underwriter, payor, insurance company.", 0, 1, insurer); 2449 case 94742904: /*class*/ return new Property("class", "", "A suite of underwriter specific classifiers.", 0, java.lang.Integer.MAX_VALUE, class_); 2450 case 106006350: /*order*/ return new Property("order", "positiveInt", "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care. For example; a patient might have (0) auto insurance (1) their own health insurance and (2) spouse's health insurance. When claiming for treatments which were not the result of an auto accident then only coverages (1) and (2) above would be applicatble and would apply in the order specified in parenthesis.", 0, 1, order); 2451 case 1843485230: /*network*/ return new Property("network", "string", "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 0, 1, network); 2452 case -1866474851: /*costToBeneficiary*/ return new Property("costToBeneficiary", "", "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.", 0, java.lang.Integer.MAX_VALUE, costToBeneficiary); 2453 case 837389739: /*subrogation*/ return new Property("subrogation", "boolean", "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.", 0, 1, subrogation); 2454 case -566947566: /*contract*/ return new Property("contract", "Reference(Contract)", "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract); 2455 case 1992141091: /*insurancePlan*/ return new Property("insurancePlan", "Reference(InsurancePlan)", "The insurance plan details, benefits and costs, which constitute this insurance coverage.", 0, 1, insurancePlan); 2456 default: return super.getNamedProperty(_hash, _name, _checkValid); 2457 } 2458 2459 } 2460 2461 @Override 2462 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2463 switch (hash) { 2464 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2465 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 2466 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<Kind> 2467 case -86519555: /*paymentBy*/ return this.paymentBy == null ? new Base[0] : this.paymentBy.toArray(new Base[this.paymentBy.size()]); // CoveragePaymentByComponent 2468 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2469 case 2046898558: /*policyHolder*/ return this.policyHolder == null ? new Base[0] : new Base[] {this.policyHolder}; // Reference 2470 case -1219769240: /*subscriber*/ return this.subscriber == null ? new Base[0] : new Base[] {this.subscriber}; // Reference 2471 case 327834531: /*subscriberId*/ return this.subscriberId == null ? new Base[0] : this.subscriberId.toArray(new Base[this.subscriberId.size()]); // Identifier 2472 case -565102875: /*beneficiary*/ return this.beneficiary == null ? new Base[0] : new Base[] {this.beneficiary}; // Reference 2473 case -1109226753: /*dependent*/ return this.dependent == null ? new Base[0] : new Base[] {this.dependent}; // StringType 2474 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 2475 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 2476 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 2477 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // ClassComponent 2478 case 106006350: /*order*/ return this.order == null ? new Base[0] : new Base[] {this.order}; // PositiveIntType 2479 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // StringType 2480 case -1866474851: /*costToBeneficiary*/ return this.costToBeneficiary == null ? new Base[0] : this.costToBeneficiary.toArray(new Base[this.costToBeneficiary.size()]); // CostToBeneficiaryComponent 2481 case 837389739: /*subrogation*/ return this.subrogation == null ? new Base[0] : new Base[] {this.subrogation}; // BooleanType 2482 case -566947566: /*contract*/ return this.contract == null ? new Base[0] : this.contract.toArray(new Base[this.contract.size()]); // Reference 2483 case 1992141091: /*insurancePlan*/ return this.insurancePlan == null ? new Base[0] : new Base[] {this.insurancePlan}; // Reference 2484 default: return super.getProperty(hash, name, checkValid); 2485 } 2486 2487 } 2488 2489 @Override 2490 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2491 switch (hash) { 2492 case -1618432855: // identifier 2493 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2494 return value; 2495 case -892481550: // status 2496 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2497 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2498 return value; 2499 case 3292052: // kind 2500 value = new KindEnumFactory().fromType(TypeConvertor.castToCode(value)); 2501 this.kind = (Enumeration) value; // Enumeration<Kind> 2502 return value; 2503 case -86519555: // paymentBy 2504 this.getPaymentBy().add((CoveragePaymentByComponent) value); // CoveragePaymentByComponent 2505 return value; 2506 case 3575610: // type 2507 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2508 return value; 2509 case 2046898558: // policyHolder 2510 this.policyHolder = TypeConvertor.castToReference(value); // Reference 2511 return value; 2512 case -1219769240: // subscriber 2513 this.subscriber = TypeConvertor.castToReference(value); // Reference 2514 return value; 2515 case 327834531: // subscriberId 2516 this.getSubscriberId().add(TypeConvertor.castToIdentifier(value)); // Identifier 2517 return value; 2518 case -565102875: // beneficiary 2519 this.beneficiary = TypeConvertor.castToReference(value); // Reference 2520 return value; 2521 case -1109226753: // dependent 2522 this.dependent = TypeConvertor.castToString(value); // StringType 2523 return value; 2524 case -261851592: // relationship 2525 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2526 return value; 2527 case -991726143: // period 2528 this.period = TypeConvertor.castToPeriod(value); // Period 2529 return value; 2530 case 1957615864: // insurer 2531 this.insurer = TypeConvertor.castToReference(value); // Reference 2532 return value; 2533 case 94742904: // class 2534 this.getClass_().add((ClassComponent) value); // ClassComponent 2535 return value; 2536 case 106006350: // order 2537 this.order = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2538 return value; 2539 case 1843485230: // network 2540 this.network = TypeConvertor.castToString(value); // StringType 2541 return value; 2542 case -1866474851: // costToBeneficiary 2543 this.getCostToBeneficiary().add((CostToBeneficiaryComponent) value); // CostToBeneficiaryComponent 2544 return value; 2545 case 837389739: // subrogation 2546 this.subrogation = TypeConvertor.castToBoolean(value); // BooleanType 2547 return value; 2548 case -566947566: // contract 2549 this.getContract().add(TypeConvertor.castToReference(value)); // Reference 2550 return value; 2551 case 1992141091: // insurancePlan 2552 this.insurancePlan = TypeConvertor.castToReference(value); // Reference 2553 return value; 2554 default: return super.setProperty(hash, name, value); 2555 } 2556 2557 } 2558 2559 @Override 2560 public Base setProperty(String name, Base value) throws FHIRException { 2561 if (name.equals("identifier")) { 2562 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2563 } else if (name.equals("status")) { 2564 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2565 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2566 } else if (name.equals("kind")) { 2567 value = new KindEnumFactory().fromType(TypeConvertor.castToCode(value)); 2568 this.kind = (Enumeration) value; // Enumeration<Kind> 2569 } else if (name.equals("paymentBy")) { 2570 this.getPaymentBy().add((CoveragePaymentByComponent) value); 2571 } else if (name.equals("type")) { 2572 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2573 } else if (name.equals("policyHolder")) { 2574 this.policyHolder = TypeConvertor.castToReference(value); // Reference 2575 } else if (name.equals("subscriber")) { 2576 this.subscriber = TypeConvertor.castToReference(value); // Reference 2577 } else if (name.equals("subscriberId")) { 2578 this.getSubscriberId().add(TypeConvertor.castToIdentifier(value)); 2579 } else if (name.equals("beneficiary")) { 2580 this.beneficiary = TypeConvertor.castToReference(value); // Reference 2581 } else if (name.equals("dependent")) { 2582 this.dependent = TypeConvertor.castToString(value); // StringType 2583 } else if (name.equals("relationship")) { 2584 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2585 } else if (name.equals("period")) { 2586 this.period = TypeConvertor.castToPeriod(value); // Period 2587 } else if (name.equals("insurer")) { 2588 this.insurer = TypeConvertor.castToReference(value); // Reference 2589 } else if (name.equals("class")) { 2590 this.getClass_().add((ClassComponent) value); 2591 } else if (name.equals("order")) { 2592 this.order = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2593 } else if (name.equals("network")) { 2594 this.network = TypeConvertor.castToString(value); // StringType 2595 } else if (name.equals("costToBeneficiary")) { 2596 this.getCostToBeneficiary().add((CostToBeneficiaryComponent) value); 2597 } else if (name.equals("subrogation")) { 2598 this.subrogation = TypeConvertor.castToBoolean(value); // BooleanType 2599 } else if (name.equals("contract")) { 2600 this.getContract().add(TypeConvertor.castToReference(value)); 2601 } else if (name.equals("insurancePlan")) { 2602 this.insurancePlan = TypeConvertor.castToReference(value); // Reference 2603 } else 2604 return super.setProperty(name, value); 2605 return value; 2606 } 2607 2608 @Override 2609 public void removeChild(String name, Base value) throws FHIRException { 2610 if (name.equals("identifier")) { 2611 this.getIdentifier().remove(value); 2612 } else if (name.equals("status")) { 2613 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2614 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2615 } else if (name.equals("kind")) { 2616 value = new KindEnumFactory().fromType(TypeConvertor.castToCode(value)); 2617 this.kind = (Enumeration) value; // Enumeration<Kind> 2618 } else if (name.equals("paymentBy")) { 2619 this.getPaymentBy().remove((CoveragePaymentByComponent) value); 2620 } else if (name.equals("type")) { 2621 this.type = null; 2622 } else if (name.equals("policyHolder")) { 2623 this.policyHolder = null; 2624 } else if (name.equals("subscriber")) { 2625 this.subscriber = null; 2626 } else if (name.equals("subscriberId")) { 2627 this.getSubscriberId().remove(value); 2628 } else if (name.equals("beneficiary")) { 2629 this.beneficiary = null; 2630 } else if (name.equals("dependent")) { 2631 this.dependent = null; 2632 } else if (name.equals("relationship")) { 2633 this.relationship = null; 2634 } else if (name.equals("period")) { 2635 this.period = null; 2636 } else if (name.equals("insurer")) { 2637 this.insurer = null; 2638 } else if (name.equals("class")) { 2639 this.getClass_().remove((ClassComponent) value); 2640 } else if (name.equals("order")) { 2641 this.order = null; 2642 } else if (name.equals("network")) { 2643 this.network = null; 2644 } else if (name.equals("costToBeneficiary")) { 2645 this.getCostToBeneficiary().remove((CostToBeneficiaryComponent) value); 2646 } else if (name.equals("subrogation")) { 2647 this.subrogation = null; 2648 } else if (name.equals("contract")) { 2649 this.getContract().remove(value); 2650 } else if (name.equals("insurancePlan")) { 2651 this.insurancePlan = null; 2652 } else 2653 super.removeChild(name, value); 2654 2655 } 2656 2657 @Override 2658 public Base makeProperty(int hash, String name) throws FHIRException { 2659 switch (hash) { 2660 case -1618432855: return addIdentifier(); 2661 case -892481550: return getStatusElement(); 2662 case 3292052: return getKindElement(); 2663 case -86519555: return addPaymentBy(); 2664 case 3575610: return getType(); 2665 case 2046898558: return getPolicyHolder(); 2666 case -1219769240: return getSubscriber(); 2667 case 327834531: return addSubscriberId(); 2668 case -565102875: return getBeneficiary(); 2669 case -1109226753: return getDependentElement(); 2670 case -261851592: return getRelationship(); 2671 case -991726143: return getPeriod(); 2672 case 1957615864: return getInsurer(); 2673 case 94742904: return addClass_(); 2674 case 106006350: return getOrderElement(); 2675 case 1843485230: return getNetworkElement(); 2676 case -1866474851: return addCostToBeneficiary(); 2677 case 837389739: return getSubrogationElement(); 2678 case -566947566: return addContract(); 2679 case 1992141091: return getInsurancePlan(); 2680 default: return super.makeProperty(hash, name); 2681 } 2682 2683 } 2684 2685 @Override 2686 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2687 switch (hash) { 2688 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2689 case -892481550: /*status*/ return new String[] {"code"}; 2690 case 3292052: /*kind*/ return new String[] {"code"}; 2691 case -86519555: /*paymentBy*/ return new String[] {}; 2692 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2693 case 2046898558: /*policyHolder*/ return new String[] {"Reference"}; 2694 case -1219769240: /*subscriber*/ return new String[] {"Reference"}; 2695 case 327834531: /*subscriberId*/ return new String[] {"Identifier"}; 2696 case -565102875: /*beneficiary*/ return new String[] {"Reference"}; 2697 case -1109226753: /*dependent*/ return new String[] {"string"}; 2698 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 2699 case -991726143: /*period*/ return new String[] {"Period"}; 2700 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 2701 case 94742904: /*class*/ return new String[] {}; 2702 case 106006350: /*order*/ return new String[] {"positiveInt"}; 2703 case 1843485230: /*network*/ return new String[] {"string"}; 2704 case -1866474851: /*costToBeneficiary*/ return new String[] {}; 2705 case 837389739: /*subrogation*/ return new String[] {"boolean"}; 2706 case -566947566: /*contract*/ return new String[] {"Reference"}; 2707 case 1992141091: /*insurancePlan*/ return new String[] {"Reference"}; 2708 default: return super.getTypesForProperty(hash, name); 2709 } 2710 2711 } 2712 2713 @Override 2714 public Base addChild(String name) throws FHIRException { 2715 if (name.equals("identifier")) { 2716 return addIdentifier(); 2717 } 2718 else if (name.equals("status")) { 2719 throw new FHIRException("Cannot call addChild on a singleton property Coverage.status"); 2720 } 2721 else if (name.equals("kind")) { 2722 throw new FHIRException("Cannot call addChild on a singleton property Coverage.kind"); 2723 } 2724 else if (name.equals("paymentBy")) { 2725 return addPaymentBy(); 2726 } 2727 else if (name.equals("type")) { 2728 this.type = new CodeableConcept(); 2729 return this.type; 2730 } 2731 else if (name.equals("policyHolder")) { 2732 this.policyHolder = new Reference(); 2733 return this.policyHolder; 2734 } 2735 else if (name.equals("subscriber")) { 2736 this.subscriber = new Reference(); 2737 return this.subscriber; 2738 } 2739 else if (name.equals("subscriberId")) { 2740 return addSubscriberId(); 2741 } 2742 else if (name.equals("beneficiary")) { 2743 this.beneficiary = new Reference(); 2744 return this.beneficiary; 2745 } 2746 else if (name.equals("dependent")) { 2747 throw new FHIRException("Cannot call addChild on a singleton property Coverage.dependent"); 2748 } 2749 else if (name.equals("relationship")) { 2750 this.relationship = new CodeableConcept(); 2751 return this.relationship; 2752 } 2753 else if (name.equals("period")) { 2754 this.period = new Period(); 2755 return this.period; 2756 } 2757 else if (name.equals("insurer")) { 2758 this.insurer = new Reference(); 2759 return this.insurer; 2760 } 2761 else if (name.equals("class")) { 2762 return addClass_(); 2763 } 2764 else if (name.equals("order")) { 2765 throw new FHIRException("Cannot call addChild on a singleton property Coverage.order"); 2766 } 2767 else if (name.equals("network")) { 2768 throw new FHIRException("Cannot call addChild on a singleton property Coverage.network"); 2769 } 2770 else if (name.equals("costToBeneficiary")) { 2771 return addCostToBeneficiary(); 2772 } 2773 else if (name.equals("subrogation")) { 2774 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subrogation"); 2775 } 2776 else if (name.equals("contract")) { 2777 return addContract(); 2778 } 2779 else if (name.equals("insurancePlan")) { 2780 this.insurancePlan = new Reference(); 2781 return this.insurancePlan; 2782 } 2783 else 2784 return super.addChild(name); 2785 } 2786 2787 public String fhirType() { 2788 return "Coverage"; 2789 2790 } 2791 2792 public Coverage copy() { 2793 Coverage dst = new Coverage(); 2794 copyValues(dst); 2795 return dst; 2796 } 2797 2798 public void copyValues(Coverage dst) { 2799 super.copyValues(dst); 2800 if (identifier != null) { 2801 dst.identifier = new ArrayList<Identifier>(); 2802 for (Identifier i : identifier) 2803 dst.identifier.add(i.copy()); 2804 }; 2805 dst.status = status == null ? null : status.copy(); 2806 dst.kind = kind == null ? null : kind.copy(); 2807 if (paymentBy != null) { 2808 dst.paymentBy = new ArrayList<CoveragePaymentByComponent>(); 2809 for (CoveragePaymentByComponent i : paymentBy) 2810 dst.paymentBy.add(i.copy()); 2811 }; 2812 dst.type = type == null ? null : type.copy(); 2813 dst.policyHolder = policyHolder == null ? null : policyHolder.copy(); 2814 dst.subscriber = subscriber == null ? null : subscriber.copy(); 2815 if (subscriberId != null) { 2816 dst.subscriberId = new ArrayList<Identifier>(); 2817 for (Identifier i : subscriberId) 2818 dst.subscriberId.add(i.copy()); 2819 }; 2820 dst.beneficiary = beneficiary == null ? null : beneficiary.copy(); 2821 dst.dependent = dependent == null ? null : dependent.copy(); 2822 dst.relationship = relationship == null ? null : relationship.copy(); 2823 dst.period = period == null ? null : period.copy(); 2824 dst.insurer = insurer == null ? null : insurer.copy(); 2825 if (class_ != null) { 2826 dst.class_ = new ArrayList<ClassComponent>(); 2827 for (ClassComponent i : class_) 2828 dst.class_.add(i.copy()); 2829 }; 2830 dst.order = order == null ? null : order.copy(); 2831 dst.network = network == null ? null : network.copy(); 2832 if (costToBeneficiary != null) { 2833 dst.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2834 for (CostToBeneficiaryComponent i : costToBeneficiary) 2835 dst.costToBeneficiary.add(i.copy()); 2836 }; 2837 dst.subrogation = subrogation == null ? null : subrogation.copy(); 2838 if (contract != null) { 2839 dst.contract = new ArrayList<Reference>(); 2840 for (Reference i : contract) 2841 dst.contract.add(i.copy()); 2842 }; 2843 dst.insurancePlan = insurancePlan == null ? null : insurancePlan.copy(); 2844 } 2845 2846 protected Coverage typedCopy() { 2847 return copy(); 2848 } 2849 2850 @Override 2851 public boolean equalsDeep(Base other_) { 2852 if (!super.equalsDeep(other_)) 2853 return false; 2854 if (!(other_ instanceof Coverage)) 2855 return false; 2856 Coverage o = (Coverage) other_; 2857 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(kind, o.kind, true) 2858 && compareDeep(paymentBy, o.paymentBy, true) && compareDeep(type, o.type, true) && compareDeep(policyHolder, o.policyHolder, true) 2859 && compareDeep(subscriber, o.subscriber, true) && compareDeep(subscriberId, o.subscriberId, true) 2860 && compareDeep(beneficiary, o.beneficiary, true) && compareDeep(dependent, o.dependent, true) && compareDeep(relationship, o.relationship, true) 2861 && compareDeep(period, o.period, true) && compareDeep(insurer, o.insurer, true) && compareDeep(class_, o.class_, true) 2862 && compareDeep(order, o.order, true) && compareDeep(network, o.network, true) && compareDeep(costToBeneficiary, o.costToBeneficiary, true) 2863 && compareDeep(subrogation, o.subrogation, true) && compareDeep(contract, o.contract, true) && compareDeep(insurancePlan, o.insurancePlan, true) 2864 ; 2865 } 2866 2867 @Override 2868 public boolean equalsShallow(Base other_) { 2869 if (!super.equalsShallow(other_)) 2870 return false; 2871 if (!(other_ instanceof Coverage)) 2872 return false; 2873 Coverage o = (Coverage) other_; 2874 return compareValues(status, o.status, true) && compareValues(kind, o.kind, true) && compareValues(dependent, o.dependent, true) 2875 && compareValues(order, o.order, true) && compareValues(network, o.network, true) && compareValues(subrogation, o.subrogation, true) 2876 ; 2877 } 2878 2879 public boolean isEmpty() { 2880 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, kind 2881 , paymentBy, type, policyHolder, subscriber, subscriberId, beneficiary, dependent 2882 , relationship, period, insurer, class_, order, network, costToBeneficiary, subrogation 2883 , contract, insurancePlan); 2884 } 2885 2886 @Override 2887 public ResourceType getResourceType() { 2888 return ResourceType.Coverage; 2889 } 2890 2891 /** 2892 * Search parameter: <b>beneficiary</b> 2893 * <p> 2894 * Description: <b>Covered party</b><br> 2895 * Type: <b>reference</b><br> 2896 * Path: <b>Coverage.beneficiary</b><br> 2897 * </p> 2898 */ 2899 @SearchParamDefinition(name="beneficiary", path="Coverage.beneficiary", description="Covered party", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2900 public static final String SP_BENEFICIARY = "beneficiary"; 2901 /** 2902 * <b>Fluent Client</b> search parameter constant for <b>beneficiary</b> 2903 * <p> 2904 * Description: <b>Covered party</b><br> 2905 * Type: <b>reference</b><br> 2906 * Path: <b>Coverage.beneficiary</b><br> 2907 * </p> 2908 */ 2909 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BENEFICIARY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BENEFICIARY); 2910 2911/** 2912 * Constant for fluent queries to be used to add include statements. Specifies 2913 * the path value of "<b>Coverage:beneficiary</b>". 2914 */ 2915 public static final ca.uhn.fhir.model.api.Include INCLUDE_BENEFICIARY = new ca.uhn.fhir.model.api.Include("Coverage:beneficiary").toLocked(); 2916 2917 /** 2918 * Search parameter: <b>class-type</b> 2919 * <p> 2920 * Description: <b>Coverage class (e.g. plan, group)</b><br> 2921 * Type: <b>token</b><br> 2922 * Path: <b>Coverage.class.type</b><br> 2923 * </p> 2924 */ 2925 @SearchParamDefinition(name="class-type", path="Coverage.class.type", description="Coverage class (e.g. plan, group)", type="token" ) 2926 public static final String SP_CLASS_TYPE = "class-type"; 2927 /** 2928 * <b>Fluent Client</b> search parameter constant for <b>class-type</b> 2929 * <p> 2930 * Description: <b>Coverage class (e.g. plan, group)</b><br> 2931 * Type: <b>token</b><br> 2932 * Path: <b>Coverage.class.type</b><br> 2933 * </p> 2934 */ 2935 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASS_TYPE); 2936 2937 /** 2938 * Search parameter: <b>class-value</b> 2939 * <p> 2940 * Description: <b>Value of the class (e.g. Plan number, group number)</b><br> 2941 * Type: <b>token</b><br> 2942 * Path: <b>Coverage.class.value</b><br> 2943 * </p> 2944 */ 2945 @SearchParamDefinition(name="class-value", path="Coverage.class.value", description="Value of the class (e.g. Plan number, group number)", type="token" ) 2946 public static final String SP_CLASS_VALUE = "class-value"; 2947 /** 2948 * <b>Fluent Client</b> search parameter constant for <b>class-value</b> 2949 * <p> 2950 * Description: <b>Value of the class (e.g. Plan number, group number)</b><br> 2951 * Type: <b>token</b><br> 2952 * Path: <b>Coverage.class.value</b><br> 2953 * </p> 2954 */ 2955 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS_VALUE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASS_VALUE); 2956 2957 /** 2958 * Search parameter: <b>dependent</b> 2959 * <p> 2960 * Description: <b>Dependent number</b><br> 2961 * Type: <b>string</b><br> 2962 * Path: <b>Coverage.dependent</b><br> 2963 * </p> 2964 */ 2965 @SearchParamDefinition(name="dependent", path="Coverage.dependent", description="Dependent number", type="string" ) 2966 public static final String SP_DEPENDENT = "dependent"; 2967 /** 2968 * <b>Fluent Client</b> search parameter constant for <b>dependent</b> 2969 * <p> 2970 * Description: <b>Dependent number</b><br> 2971 * Type: <b>string</b><br> 2972 * Path: <b>Coverage.dependent</b><br> 2973 * </p> 2974 */ 2975 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEPENDENT = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DEPENDENT); 2976 2977 /** 2978 * Search parameter: <b>insurer</b> 2979 * <p> 2980 * Description: <b>The identity of the insurer</b><br> 2981 * Type: <b>reference</b><br> 2982 * Path: <b>Coverage.insurer</b><br> 2983 * </p> 2984 */ 2985 @SearchParamDefinition(name="insurer", path="Coverage.insurer", description="The identity of the insurer", type="reference", target={Organization.class } ) 2986 public static final String SP_INSURER = "insurer"; 2987 /** 2988 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 2989 * <p> 2990 * Description: <b>The identity of the insurer</b><br> 2991 * Type: <b>reference</b><br> 2992 * Path: <b>Coverage.insurer</b><br> 2993 * </p> 2994 */ 2995 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 2996 2997/** 2998 * Constant for fluent queries to be used to add include statements. Specifies 2999 * the path value of "<b>Coverage:insurer</b>". 3000 */ 3001 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("Coverage:insurer").toLocked(); 3002 3003 /** 3004 * Search parameter: <b>paymentby-party</b> 3005 * <p> 3006 * Description: <b>Parties who will pay for services</b><br> 3007 * Type: <b>reference</b><br> 3008 * Path: <b>Coverage.paymentBy.party</b><br> 3009 * </p> 3010 */ 3011 @SearchParamDefinition(name="paymentby-party", path="Coverage.paymentBy.party", description="Parties who will pay for services", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 3012 public static final String SP_PAYMENTBY_PARTY = "paymentby-party"; 3013 /** 3014 * <b>Fluent Client</b> search parameter constant for <b>paymentby-party</b> 3015 * <p> 3016 * Description: <b>Parties who will pay for services</b><br> 3017 * Type: <b>reference</b><br> 3018 * Path: <b>Coverage.paymentBy.party</b><br> 3019 * </p> 3020 */ 3021 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYMENTBY_PARTY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYMENTBY_PARTY); 3022 3023/** 3024 * Constant for fluent queries to be used to add include statements. Specifies 3025 * the path value of "<b>Coverage:paymentby-party</b>". 3026 */ 3027 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYMENTBY_PARTY = new ca.uhn.fhir.model.api.Include("Coverage:paymentby-party").toLocked(); 3028 3029 /** 3030 * Search parameter: <b>policy-holder</b> 3031 * <p> 3032 * Description: <b>Reference to the policyholder</b><br> 3033 * Type: <b>reference</b><br> 3034 * Path: <b>Coverage.policyHolder</b><br> 3035 * </p> 3036 */ 3037 @SearchParamDefinition(name="policy-holder", path="Coverage.policyHolder", description="Reference to the policyholder", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 3038 public static final String SP_POLICY_HOLDER = "policy-holder"; 3039 /** 3040 * <b>Fluent Client</b> search parameter constant for <b>policy-holder</b> 3041 * <p> 3042 * Description: <b>Reference to the policyholder</b><br> 3043 * Type: <b>reference</b><br> 3044 * Path: <b>Coverage.policyHolder</b><br> 3045 * </p> 3046 */ 3047 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam POLICY_HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_POLICY_HOLDER); 3048 3049/** 3050 * Constant for fluent queries to be used to add include statements. Specifies 3051 * the path value of "<b>Coverage:policy-holder</b>". 3052 */ 3053 public static final ca.uhn.fhir.model.api.Include INCLUDE_POLICY_HOLDER = new ca.uhn.fhir.model.api.Include("Coverage:policy-holder").toLocked(); 3054 3055 /** 3056 * Search parameter: <b>status</b> 3057 * <p> 3058 * Description: <b>The status of the Coverage</b><br> 3059 * Type: <b>token</b><br> 3060 * Path: <b>Coverage.status</b><br> 3061 * </p> 3062 */ 3063 @SearchParamDefinition(name="status", path="Coverage.status", description="The status of the Coverage", type="token" ) 3064 public static final String SP_STATUS = "status"; 3065 /** 3066 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3067 * <p> 3068 * Description: <b>The status of the Coverage</b><br> 3069 * Type: <b>token</b><br> 3070 * Path: <b>Coverage.status</b><br> 3071 * </p> 3072 */ 3073 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3074 3075 /** 3076 * Search parameter: <b>subscriber</b> 3077 * <p> 3078 * Description: <b>Reference to the subscriber</b><br> 3079 * Type: <b>reference</b><br> 3080 * Path: <b>Coverage.subscriber</b><br> 3081 * </p> 3082 */ 3083 @SearchParamDefinition(name="subscriber", path="Coverage.subscriber", description="Reference to the subscriber", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Patient.class, RelatedPerson.class } ) 3084 public static final String SP_SUBSCRIBER = "subscriber"; 3085 /** 3086 * <b>Fluent Client</b> search parameter constant for <b>subscriber</b> 3087 * <p> 3088 * Description: <b>Reference to the subscriber</b><br> 3089 * Type: <b>reference</b><br> 3090 * Path: <b>Coverage.subscriber</b><br> 3091 * </p> 3092 */ 3093 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSCRIBER); 3094 3095/** 3096 * Constant for fluent queries to be used to add include statements. Specifies 3097 * the path value of "<b>Coverage:subscriber</b>". 3098 */ 3099 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSCRIBER = new ca.uhn.fhir.model.api.Include("Coverage:subscriber").toLocked(); 3100 3101 /** 3102 * Search parameter: <b>subscriberid</b> 3103 * <p> 3104 * Description: <b>Identifier of the subscriber</b><br> 3105 * Type: <b>token</b><br> 3106 * Path: <b>Coverage.subscriberId</b><br> 3107 * </p> 3108 */ 3109 @SearchParamDefinition(name="subscriberid", path="Coverage.subscriberId", description="Identifier of the subscriber", type="token" ) 3110 public static final String SP_SUBSCRIBERID = "subscriberid"; 3111 /** 3112 * <b>Fluent Client</b> search parameter constant for <b>subscriberid</b> 3113 * <p> 3114 * Description: <b>Identifier of the subscriber</b><br> 3115 * Type: <b>token</b><br> 3116 * Path: <b>Coverage.subscriberId</b><br> 3117 * </p> 3118 */ 3119 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBSCRIBERID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUBSCRIBERID); 3120 3121 /** 3122 * Search parameter: <b>identifier</b> 3123 * <p> 3124 * Description: <b>Multiple Resources: 3125 3126* [Account](account.html): Account number 3127* [AdverseEvent](adverseevent.html): Business identifier for the event 3128* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3129* [Appointment](appointment.html): An Identifier of the Appointment 3130* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3131* [Basic](basic.html): Business identifier 3132* [BodyStructure](bodystructure.html): Bodystructure identifier 3133* [CarePlan](careplan.html): External Ids for this plan 3134* [CareTeam](careteam.html): External Ids for this team 3135* [ChargeItem](chargeitem.html): Business Identifier for item 3136* [Claim](claim.html): The primary identifier of the financial resource 3137* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3138* [ClinicalImpression](clinicalimpression.html): Business identifier 3139* [Communication](communication.html): Unique identifier 3140* [CommunicationRequest](communicationrequest.html): Unique identifier 3141* [Composition](composition.html): Version-independent identifier for the Composition 3142* [Condition](condition.html): A unique identifier of the condition record 3143* [Consent](consent.html): Identifier for this record (external references) 3144* [Contract](contract.html): The identity of the contract 3145* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3146* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3147* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3148* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3149* [DeviceRequest](devicerequest.html): Business identifier for request/order 3150* [DeviceUsage](deviceusage.html): Search by identifier 3151* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3152* [DocumentReference](documentreference.html): Identifier of the attachment binary 3153* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3154* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3155* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3156* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3157* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3158* [Flag](flag.html): Business identifier 3159* [Goal](goal.html): External Ids for this goal 3160* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3161* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3162* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3163* [Immunization](immunization.html): Business identifier 3164* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3165* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3166* [Invoice](invoice.html): Business Identifier for item 3167* [List](list.html): Business identifier 3168* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3169* [Medication](medication.html): Returns medications with this external identifier 3170* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3171* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3172* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3173* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3174* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3175* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3176* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3177* [Observation](observation.html): The unique id for a particular observation 3178* [Person](person.html): A person Identifier 3179* [Procedure](procedure.html): A unique identifier for a procedure 3180* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3181* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3182* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3183* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3184* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3185* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3186* [Specimen](specimen.html): The unique identifier associated with the specimen 3187* [SupplyDelivery](supplydelivery.html): External identifier 3188* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3189* [Task](task.html): Search for a task instance by its business identifier 3190* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3191</b><br> 3192 * Type: <b>token</b><br> 3193 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3194 * </p> 3195 */ 3196 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3197 public static final String SP_IDENTIFIER = "identifier"; 3198 /** 3199 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3200 * <p> 3201 * Description: <b>Multiple Resources: 3202 3203* [Account](account.html): Account number 3204* [AdverseEvent](adverseevent.html): Business identifier for the event 3205* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3206* [Appointment](appointment.html): An Identifier of the Appointment 3207* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3208* [Basic](basic.html): Business identifier 3209* [BodyStructure](bodystructure.html): Bodystructure identifier 3210* [CarePlan](careplan.html): External Ids for this plan 3211* [CareTeam](careteam.html): External Ids for this team 3212* [ChargeItem](chargeitem.html): Business Identifier for item 3213* [Claim](claim.html): The primary identifier of the financial resource 3214* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3215* [ClinicalImpression](clinicalimpression.html): Business identifier 3216* [Communication](communication.html): Unique identifier 3217* [CommunicationRequest](communicationrequest.html): Unique identifier 3218* [Composition](composition.html): Version-independent identifier for the Composition 3219* [Condition](condition.html): A unique identifier of the condition record 3220* [Consent](consent.html): Identifier for this record (external references) 3221* [Contract](contract.html): The identity of the contract 3222* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3223* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3224* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3225* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3226* [DeviceRequest](devicerequest.html): Business identifier for request/order 3227* [DeviceUsage](deviceusage.html): Search by identifier 3228* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3229* [DocumentReference](documentreference.html): Identifier of the attachment binary 3230* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3231* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3232* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3233* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3234* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3235* [Flag](flag.html): Business identifier 3236* [Goal](goal.html): External Ids for this goal 3237* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3238* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3239* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3240* [Immunization](immunization.html): Business identifier 3241* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3242* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3243* [Invoice](invoice.html): Business Identifier for item 3244* [List](list.html): Business identifier 3245* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3246* [Medication](medication.html): Returns medications with this external identifier 3247* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3248* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3249* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3250* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3251* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3252* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3253* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3254* [Observation](observation.html): The unique id for a particular observation 3255* [Person](person.html): A person Identifier 3256* [Procedure](procedure.html): A unique identifier for a procedure 3257* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3258* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3259* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3260* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3261* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3262* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3263* [Specimen](specimen.html): The unique identifier associated with the specimen 3264* [SupplyDelivery](supplydelivery.html): External identifier 3265* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3266* [Task](task.html): Search for a task instance by its business identifier 3267* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3268</b><br> 3269 * Type: <b>token</b><br> 3270 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3271 * </p> 3272 */ 3273 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3274 3275 /** 3276 * Search parameter: <b>patient</b> 3277 * <p> 3278 * Description: <b>Multiple Resources: 3279 3280* [Account](account.html): The entity that caused the expenses 3281* [AdverseEvent](adverseevent.html): Subject impacted by event 3282* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3283* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3284* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3285* [AuditEvent](auditevent.html): Where the activity involved patient data 3286* [Basic](basic.html): Identifies the focus of this resource 3287* [BodyStructure](bodystructure.html): Who this is about 3288* [CarePlan](careplan.html): Who the care plan is for 3289* [CareTeam](careteam.html): Who care team is for 3290* [ChargeItem](chargeitem.html): Individual service was done for/to 3291* [Claim](claim.html): Patient receiving the products or services 3292* [ClaimResponse](claimresponse.html): The subject of care 3293* [ClinicalImpression](clinicalimpression.html): Patient assessed 3294* [Communication](communication.html): Focus of message 3295* [CommunicationRequest](communicationrequest.html): Focus of message 3296* [Composition](composition.html): Who and/or what the composition is about 3297* [Condition](condition.html): Who has the condition? 3298* [Consent](consent.html): Who the consent applies to 3299* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3300* [Coverage](coverage.html): Retrieve coverages for a patient 3301* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3302* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3303* [DetectedIssue](detectedissue.html): Associated patient 3304* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3305* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3306* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3307* [DocumentReference](documentreference.html): Who/what is the subject of the document 3308* [Encounter](encounter.html): The patient present at the encounter 3309* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3310* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3311* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3312* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3313* [Flag](flag.html): The identity of a subject to list flags for 3314* [Goal](goal.html): Who this goal is intended for 3315* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3316* [ImagingSelection](imagingselection.html): Who the study is about 3317* [ImagingStudy](imagingstudy.html): Who the study is about 3318* [Immunization](immunization.html): The patient for the vaccination record 3319* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3320* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3321* [Invoice](invoice.html): Recipient(s) of goods and services 3322* [List](list.html): If all resources have the same subject 3323* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3324* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3325* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3326* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3327* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3328* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3329* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3330* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3331* [Observation](observation.html): The subject that the observation is about (if patient) 3332* [Person](person.html): The Person links to this Patient 3333* [Procedure](procedure.html): Search by subject - a patient 3334* [Provenance](provenance.html): Where the activity involved patient data 3335* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3336* [RelatedPerson](relatedperson.html): The patient this related person is related to 3337* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3338* [ResearchSubject](researchsubject.html): Who or what is part of study 3339* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3340* [ServiceRequest](servicerequest.html): Search by subject - a patient 3341* [Specimen](specimen.html): The patient the specimen comes from 3342* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3343* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3344* [Task](task.html): Search by patient 3345* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3346</b><br> 3347 * Type: <b>reference</b><br> 3348 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3349 * </p> 3350 */ 3351 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3352 public static final String SP_PATIENT = "patient"; 3353 /** 3354 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3355 * <p> 3356 * Description: <b>Multiple Resources: 3357 3358* [Account](account.html): The entity that caused the expenses 3359* [AdverseEvent](adverseevent.html): Subject impacted by event 3360* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3361* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3362* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3363* [AuditEvent](auditevent.html): Where the activity involved patient data 3364* [Basic](basic.html): Identifies the focus of this resource 3365* [BodyStructure](bodystructure.html): Who this is about 3366* [CarePlan](careplan.html): Who the care plan is for 3367* [CareTeam](careteam.html): Who care team is for 3368* [ChargeItem](chargeitem.html): Individual service was done for/to 3369* [Claim](claim.html): Patient receiving the products or services 3370* [ClaimResponse](claimresponse.html): The subject of care 3371* [ClinicalImpression](clinicalimpression.html): Patient assessed 3372* [Communication](communication.html): Focus of message 3373* [CommunicationRequest](communicationrequest.html): Focus of message 3374* [Composition](composition.html): Who and/or what the composition is about 3375* [Condition](condition.html): Who has the condition? 3376* [Consent](consent.html): Who the consent applies to 3377* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3378* [Coverage](coverage.html): Retrieve coverages for a patient 3379* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3380* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3381* [DetectedIssue](detectedissue.html): Associated patient 3382* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3383* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3384* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3385* [DocumentReference](documentreference.html): Who/what is the subject of the document 3386* [Encounter](encounter.html): The patient present at the encounter 3387* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3388* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3389* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3390* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3391* [Flag](flag.html): The identity of a subject to list flags for 3392* [Goal](goal.html): Who this goal is intended for 3393* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3394* [ImagingSelection](imagingselection.html): Who the study is about 3395* [ImagingStudy](imagingstudy.html): Who the study is about 3396* [Immunization](immunization.html): The patient for the vaccination record 3397* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3398* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3399* [Invoice](invoice.html): Recipient(s) of goods and services 3400* [List](list.html): If all resources have the same subject 3401* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3402* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3403* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3404* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3405* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3406* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3407* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3408* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3409* [Observation](observation.html): The subject that the observation is about (if patient) 3410* [Person](person.html): The Person links to this Patient 3411* [Procedure](procedure.html): Search by subject - a patient 3412* [Provenance](provenance.html): Where the activity involved patient data 3413* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3414* [RelatedPerson](relatedperson.html): The patient this related person is related to 3415* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3416* [ResearchSubject](researchsubject.html): Who or what is part of study 3417* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3418* [ServiceRequest](servicerequest.html): Search by subject - a patient 3419* [Specimen](specimen.html): The patient the specimen comes from 3420* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3421* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3422* [Task](task.html): Search by patient 3423* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3424</b><br> 3425 * Type: <b>reference</b><br> 3426 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3427 * </p> 3428 */ 3429 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3430 3431/** 3432 * Constant for fluent queries to be used to add include statements. Specifies 3433 * the path value of "<b>Coverage:patient</b>". 3434 */ 3435 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Coverage:patient").toLocked(); 3436 3437 /** 3438 * Search parameter: <b>type</b> 3439 * <p> 3440 * Description: <b>Multiple Resources: 3441 3442* [Account](account.html): E.g. patient, expense, depreciation 3443* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3444* [Composition](composition.html): Kind of composition (LOINC if possible) 3445* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3446* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3447* [Encounter](encounter.html): Specific type of encounter 3448* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3449* [Invoice](invoice.html): Type of Invoice 3450* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3451* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3452* [Specimen](specimen.html): The specimen type 3453</b><br> 3454 * Type: <b>token</b><br> 3455 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3456 * </p> 3457 */ 3458 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 3459 public static final String SP_TYPE = "type"; 3460 /** 3461 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3462 * <p> 3463 * Description: <b>Multiple Resources: 3464 3465* [Account](account.html): E.g. patient, expense, depreciation 3466* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3467* [Composition](composition.html): Kind of composition (LOINC if possible) 3468* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3469* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3470* [Encounter](encounter.html): Specific type of encounter 3471* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3472* [Invoice](invoice.html): Type of Invoice 3473* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3474* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3475* [Specimen](specimen.html): The specimen type 3476</b><br> 3477 * Type: <b>token</b><br> 3478 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3479 * </p> 3480 */ 3481 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3482 3483 3484} 3485