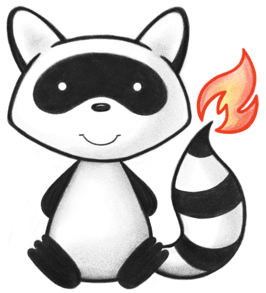
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 052 */ 053@ResourceDef(name="CoverageEligibilityRequest", profile="http://hl7.org/fhir/StructureDefinition/CoverageEligibilityRequest") 054public class CoverageEligibilityRequest extends DomainResource { 055 056 public enum EligibilityRequestPurpose { 057 /** 058 * The prior authorization requirements for the listed, or discovered if specified, converages for the categories of service and/or specifed biling codes are requested. 059 */ 060 AUTHREQUIREMENTS, 061 /** 062 * The plan benefits and optionally benefits consumed for the listed, or discovered if specified, converages are requested. 063 */ 064 BENEFITS, 065 /** 066 * The insurer is requested to report on any coverages which they are aware of in addition to any specifed. 067 */ 068 DISCOVERY, 069 /** 070 * A check that the specified coverages are in-force is requested. 071 */ 072 VALIDATION, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static EligibilityRequestPurpose fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("auth-requirements".equals(codeString)) 081 return AUTHREQUIREMENTS; 082 if ("benefits".equals(codeString)) 083 return BENEFITS; 084 if ("discovery".equals(codeString)) 085 return DISCOVERY; 086 if ("validation".equals(codeString)) 087 return VALIDATION; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown EligibilityRequestPurpose code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case AUTHREQUIREMENTS: return "auth-requirements"; 096 case BENEFITS: return "benefits"; 097 case DISCOVERY: return "discovery"; 098 case VALIDATION: return "validation"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case AUTHREQUIREMENTS: return "http://hl7.org/fhir/eligibilityrequest-purpose"; 106 case BENEFITS: return "http://hl7.org/fhir/eligibilityrequest-purpose"; 107 case DISCOVERY: return "http://hl7.org/fhir/eligibilityrequest-purpose"; 108 case VALIDATION: return "http://hl7.org/fhir/eligibilityrequest-purpose"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case AUTHREQUIREMENTS: return "The prior authorization requirements for the listed, or discovered if specified, converages for the categories of service and/or specifed biling codes are requested."; 116 case BENEFITS: return "The plan benefits and optionally benefits consumed for the listed, or discovered if specified, converages are requested."; 117 case DISCOVERY: return "The insurer is requested to report on any coverages which they are aware of in addition to any specifed."; 118 case VALIDATION: return "A check that the specified coverages are in-force is requested."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case AUTHREQUIREMENTS: return "Coverage auth-requirements"; 126 case BENEFITS: return "Coverage benefits"; 127 case DISCOVERY: return "Coverage Discovery"; 128 case VALIDATION: return "Coverage Validation"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class EligibilityRequestPurposeEnumFactory implements EnumFactory<EligibilityRequestPurpose> { 136 public EligibilityRequestPurpose fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("auth-requirements".equals(codeString)) 141 return EligibilityRequestPurpose.AUTHREQUIREMENTS; 142 if ("benefits".equals(codeString)) 143 return EligibilityRequestPurpose.BENEFITS; 144 if ("discovery".equals(codeString)) 145 return EligibilityRequestPurpose.DISCOVERY; 146 if ("validation".equals(codeString)) 147 return EligibilityRequestPurpose.VALIDATION; 148 throw new IllegalArgumentException("Unknown EligibilityRequestPurpose code '"+codeString+"'"); 149 } 150 public Enumeration<EligibilityRequestPurpose> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.NULL, code); 158 if ("auth-requirements".equals(codeString)) 159 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.AUTHREQUIREMENTS, code); 160 if ("benefits".equals(codeString)) 161 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.BENEFITS, code); 162 if ("discovery".equals(codeString)) 163 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.DISCOVERY, code); 164 if ("validation".equals(codeString)) 165 return new Enumeration<EligibilityRequestPurpose>(this, EligibilityRequestPurpose.VALIDATION, code); 166 throw new FHIRException("Unknown EligibilityRequestPurpose code '"+codeString+"'"); 167 } 168 public String toCode(EligibilityRequestPurpose code) { 169 if (code == EligibilityRequestPurpose.NULL) 170 return null; 171 if (code == EligibilityRequestPurpose.AUTHREQUIREMENTS) 172 return "auth-requirements"; 173 if (code == EligibilityRequestPurpose.BENEFITS) 174 return "benefits"; 175 if (code == EligibilityRequestPurpose.DISCOVERY) 176 return "discovery"; 177 if (code == EligibilityRequestPurpose.VALIDATION) 178 return "validation"; 179 return "?"; 180 } 181 public String toSystem(EligibilityRequestPurpose code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class CoverageEligibilityRequestEventComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * A coded event such as when a service is expected or a card printed. 190 */ 191 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 192 @Description(shortDefinition="Specific event", formalDefinition="A coded event such as when a service is expected or a card printed." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/datestype") 194 protected CodeableConcept type; 195 196 /** 197 * A date or period in the past or future indicating when the event occurred or is expectd to occur. 198 */ 199 @Child(name = "when", type = {DateTimeType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="Occurance date or period", formalDefinition="A date or period in the past or future indicating when the event occurred or is expectd to occur." ) 201 protected DataType when; 202 203 private static final long serialVersionUID = -634897375L; 204 205 /** 206 * Constructor 207 */ 208 public CoverageEligibilityRequestEventComponent() { 209 super(); 210 } 211 212 /** 213 * Constructor 214 */ 215 public CoverageEligibilityRequestEventComponent(CodeableConcept type, DataType when) { 216 super(); 217 this.setType(type); 218 this.setWhen(when); 219 } 220 221 /** 222 * @return {@link #type} (A coded event such as when a service is expected or a card printed.) 223 */ 224 public CodeableConcept getType() { 225 if (this.type == null) 226 if (Configuration.errorOnAutoCreate()) 227 throw new Error("Attempt to auto-create CoverageEligibilityRequestEventComponent.type"); 228 else if (Configuration.doAutoCreate()) 229 this.type = new CodeableConcept(); // cc 230 return this.type; 231 } 232 233 public boolean hasType() { 234 return this.type != null && !this.type.isEmpty(); 235 } 236 237 /** 238 * @param value {@link #type} (A coded event such as when a service is expected or a card printed.) 239 */ 240 public CoverageEligibilityRequestEventComponent setType(CodeableConcept value) { 241 this.type = value; 242 return this; 243 } 244 245 /** 246 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 247 */ 248 public DataType getWhen() { 249 return this.when; 250 } 251 252 /** 253 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 254 */ 255 public DateTimeType getWhenDateTimeType() throws FHIRException { 256 if (this.when == null) 257 this.when = new DateTimeType(); 258 if (!(this.when instanceof DateTimeType)) 259 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.when.getClass().getName()+" was encountered"); 260 return (DateTimeType) this.when; 261 } 262 263 public boolean hasWhenDateTimeType() { 264 return this.when instanceof DateTimeType; 265 } 266 267 /** 268 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 269 */ 270 public Period getWhenPeriod() throws FHIRException { 271 if (this.when == null) 272 this.when = new Period(); 273 if (!(this.when instanceof Period)) 274 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 275 return (Period) this.when; 276 } 277 278 public boolean hasWhenPeriod() { 279 return this.when instanceof Period; 280 } 281 282 public boolean hasWhen() { 283 return this.when != null && !this.when.isEmpty(); 284 } 285 286 /** 287 * @param value {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 288 */ 289 public CoverageEligibilityRequestEventComponent setWhen(DataType value) { 290 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 291 throw new FHIRException("Not the right type for CoverageEligibilityRequest.event.when[x]: "+value.fhirType()); 292 this.when = value; 293 return this; 294 } 295 296 protected void listChildren(List<Property> children) { 297 super.listChildren(children); 298 children.add(new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type)); 299 children.add(new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when)); 300 } 301 302 @Override 303 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 304 switch (_hash) { 305 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type); 306 case 1312831238: /*when[x]*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 307 case 3648314: /*when*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 308 case -1785502475: /*whenDateTime*/ return new Property("when[x]", "dateTime", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 309 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 310 default: return super.getNamedProperty(_hash, _name, _checkValid); 311 } 312 313 } 314 315 @Override 316 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 317 switch (hash) { 318 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 319 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 320 default: return super.getProperty(hash, name, checkValid); 321 } 322 323 } 324 325 @Override 326 public Base setProperty(int hash, String name, Base value) throws FHIRException { 327 switch (hash) { 328 case 3575610: // type 329 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 330 return value; 331 case 3648314: // when 332 this.when = TypeConvertor.castToType(value); // DataType 333 return value; 334 default: return super.setProperty(hash, name, value); 335 } 336 337 } 338 339 @Override 340 public Base setProperty(String name, Base value) throws FHIRException { 341 if (name.equals("type")) { 342 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 343 } else if (name.equals("when[x]")) { 344 this.when = TypeConvertor.castToType(value); // DataType 345 } else 346 return super.setProperty(name, value); 347 return value; 348 } 349 350 @Override 351 public void removeChild(String name, Base value) throws FHIRException { 352 if (name.equals("type")) { 353 this.type = null; 354 } else if (name.equals("when[x]")) { 355 this.when = null; 356 } else 357 super.removeChild(name, value); 358 359 } 360 361 @Override 362 public Base makeProperty(int hash, String name) throws FHIRException { 363 switch (hash) { 364 case 3575610: return getType(); 365 case 1312831238: return getWhen(); 366 case 3648314: return getWhen(); 367 default: return super.makeProperty(hash, name); 368 } 369 370 } 371 372 @Override 373 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 374 switch (hash) { 375 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 376 case 3648314: /*when*/ return new String[] {"dateTime", "Period"}; 377 default: return super.getTypesForProperty(hash, name); 378 } 379 380 } 381 382 @Override 383 public Base addChild(String name) throws FHIRException { 384 if (name.equals("type")) { 385 this.type = new CodeableConcept(); 386 return this.type; 387 } 388 else if (name.equals("whenDateTime")) { 389 this.when = new DateTimeType(); 390 return this.when; 391 } 392 else if (name.equals("whenPeriod")) { 393 this.when = new Period(); 394 return this.when; 395 } 396 else 397 return super.addChild(name); 398 } 399 400 public CoverageEligibilityRequestEventComponent copy() { 401 CoverageEligibilityRequestEventComponent dst = new CoverageEligibilityRequestEventComponent(); 402 copyValues(dst); 403 return dst; 404 } 405 406 public void copyValues(CoverageEligibilityRequestEventComponent dst) { 407 super.copyValues(dst); 408 dst.type = type == null ? null : type.copy(); 409 dst.when = when == null ? null : when.copy(); 410 } 411 412 @Override 413 public boolean equalsDeep(Base other_) { 414 if (!super.equalsDeep(other_)) 415 return false; 416 if (!(other_ instanceof CoverageEligibilityRequestEventComponent)) 417 return false; 418 CoverageEligibilityRequestEventComponent o = (CoverageEligibilityRequestEventComponent) other_; 419 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true); 420 } 421 422 @Override 423 public boolean equalsShallow(Base other_) { 424 if (!super.equalsShallow(other_)) 425 return false; 426 if (!(other_ instanceof CoverageEligibilityRequestEventComponent)) 427 return false; 428 CoverageEligibilityRequestEventComponent o = (CoverageEligibilityRequestEventComponent) other_; 429 return true; 430 } 431 432 public boolean isEmpty() { 433 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when); 434 } 435 436 public String fhirType() { 437 return "CoverageEligibilityRequest.event"; 438 439 } 440 441 } 442 443 @Block() 444 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 445 /** 446 * A number to uniquely identify supporting information entries. 447 */ 448 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 449 @Description(shortDefinition="Information instance identifier", formalDefinition="A number to uniquely identify supporting information entries." ) 450 protected PositiveIntType sequence; 451 452 /** 453 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 454 */ 455 @Child(name = "information", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=false) 456 @Description(shortDefinition="Data to be provided", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 457 protected Reference information; 458 459 /** 460 * The supporting materials are applicable for all detail items, product/servce categories and specific billing codes. 461 */ 462 @Child(name = "appliesToAll", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 463 @Description(shortDefinition="Applies to all items", formalDefinition="The supporting materials are applicable for all detail items, product/servce categories and specific billing codes." ) 464 protected BooleanType appliesToAll; 465 466 private static final long serialVersionUID = -1430960090L; 467 468 /** 469 * Constructor 470 */ 471 public SupportingInformationComponent() { 472 super(); 473 } 474 475 /** 476 * Constructor 477 */ 478 public SupportingInformationComponent(int sequence, Reference information) { 479 super(); 480 this.setSequence(sequence); 481 this.setInformation(information); 482 } 483 484 /** 485 * @return {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 486 */ 487 public PositiveIntType getSequenceElement() { 488 if (this.sequence == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 491 else if (Configuration.doAutoCreate()) 492 this.sequence = new PositiveIntType(); // bb 493 return this.sequence; 494 } 495 496 public boolean hasSequenceElement() { 497 return this.sequence != null && !this.sequence.isEmpty(); 498 } 499 500 public boolean hasSequence() { 501 return this.sequence != null && !this.sequence.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 506 */ 507 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 508 this.sequence = value; 509 return this; 510 } 511 512 /** 513 * @return A number to uniquely identify supporting information entries. 514 */ 515 public int getSequence() { 516 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 517 } 518 519 /** 520 * @param value A number to uniquely identify supporting information entries. 521 */ 522 public SupportingInformationComponent setSequence(int value) { 523 if (this.sequence == null) 524 this.sequence = new PositiveIntType(); 525 this.sequence.setValue(value); 526 return this; 527 } 528 529 /** 530 * @return {@link #information} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 531 */ 532 public Reference getInformation() { 533 if (this.information == null) 534 if (Configuration.errorOnAutoCreate()) 535 throw new Error("Attempt to auto-create SupportingInformationComponent.information"); 536 else if (Configuration.doAutoCreate()) 537 this.information = new Reference(); // cc 538 return this.information; 539 } 540 541 public boolean hasInformation() { 542 return this.information != null && !this.information.isEmpty(); 543 } 544 545 /** 546 * @param value {@link #information} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 547 */ 548 public SupportingInformationComponent setInformation(Reference value) { 549 this.information = value; 550 return this; 551 } 552 553 /** 554 * @return {@link #appliesToAll} (The supporting materials are applicable for all detail items, product/servce categories and specific billing codes.). This is the underlying object with id, value and extensions. The accessor "getAppliesToAll" gives direct access to the value 555 */ 556 public BooleanType getAppliesToAllElement() { 557 if (this.appliesToAll == null) 558 if (Configuration.errorOnAutoCreate()) 559 throw new Error("Attempt to auto-create SupportingInformationComponent.appliesToAll"); 560 else if (Configuration.doAutoCreate()) 561 this.appliesToAll = new BooleanType(); // bb 562 return this.appliesToAll; 563 } 564 565 public boolean hasAppliesToAllElement() { 566 return this.appliesToAll != null && !this.appliesToAll.isEmpty(); 567 } 568 569 public boolean hasAppliesToAll() { 570 return this.appliesToAll != null && !this.appliesToAll.isEmpty(); 571 } 572 573 /** 574 * @param value {@link #appliesToAll} (The supporting materials are applicable for all detail items, product/servce categories and specific billing codes.). This is the underlying object with id, value and extensions. The accessor "getAppliesToAll" gives direct access to the value 575 */ 576 public SupportingInformationComponent setAppliesToAllElement(BooleanType value) { 577 this.appliesToAll = value; 578 return this; 579 } 580 581 /** 582 * @return The supporting materials are applicable for all detail items, product/servce categories and specific billing codes. 583 */ 584 public boolean getAppliesToAll() { 585 return this.appliesToAll == null || this.appliesToAll.isEmpty() ? false : this.appliesToAll.getValue(); 586 } 587 588 /** 589 * @param value The supporting materials are applicable for all detail items, product/servce categories and specific billing codes. 590 */ 591 public SupportingInformationComponent setAppliesToAll(boolean value) { 592 if (this.appliesToAll == null) 593 this.appliesToAll = new BooleanType(); 594 this.appliesToAll.setValue(value); 595 return this; 596 } 597 598 protected void listChildren(List<Property> children) { 599 super.listChildren(children); 600 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 601 children.add(new Property("information", "Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, information)); 602 children.add(new Property("appliesToAll", "boolean", "The supporting materials are applicable for all detail items, product/servce categories and specific billing codes.", 0, 1, appliesToAll)); 603 } 604 605 @Override 606 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 607 switch (_hash) { 608 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence); 609 case 1968600364: /*information*/ return new Property("information", "Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, information); 610 case -1096846342: /*appliesToAll*/ return new Property("appliesToAll", "boolean", "The supporting materials are applicable for all detail items, product/servce categories and specific billing codes.", 0, 1, appliesToAll); 611 default: return super.getNamedProperty(_hash, _name, _checkValid); 612 } 613 614 } 615 616 @Override 617 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 618 switch (hash) { 619 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 620 case 1968600364: /*information*/ return this.information == null ? new Base[0] : new Base[] {this.information}; // Reference 621 case -1096846342: /*appliesToAll*/ return this.appliesToAll == null ? new Base[0] : new Base[] {this.appliesToAll}; // BooleanType 622 default: return super.getProperty(hash, name, checkValid); 623 } 624 625 } 626 627 @Override 628 public Base setProperty(int hash, String name, Base value) throws FHIRException { 629 switch (hash) { 630 case 1349547969: // sequence 631 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 632 return value; 633 case 1968600364: // information 634 this.information = TypeConvertor.castToReference(value); // Reference 635 return value; 636 case -1096846342: // appliesToAll 637 this.appliesToAll = TypeConvertor.castToBoolean(value); // BooleanType 638 return value; 639 default: return super.setProperty(hash, name, value); 640 } 641 642 } 643 644 @Override 645 public Base setProperty(String name, Base value) throws FHIRException { 646 if (name.equals("sequence")) { 647 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 648 } else if (name.equals("information")) { 649 this.information = TypeConvertor.castToReference(value); // Reference 650 } else if (name.equals("appliesToAll")) { 651 this.appliesToAll = TypeConvertor.castToBoolean(value); // BooleanType 652 } else 653 return super.setProperty(name, value); 654 return value; 655 } 656 657 @Override 658 public void removeChild(String name, Base value) throws FHIRException { 659 if (name.equals("sequence")) { 660 this.sequence = null; 661 } else if (name.equals("information")) { 662 this.information = null; 663 } else if (name.equals("appliesToAll")) { 664 this.appliesToAll = null; 665 } else 666 super.removeChild(name, value); 667 668 } 669 670 @Override 671 public Base makeProperty(int hash, String name) throws FHIRException { 672 switch (hash) { 673 case 1349547969: return getSequenceElement(); 674 case 1968600364: return getInformation(); 675 case -1096846342: return getAppliesToAllElement(); 676 default: return super.makeProperty(hash, name); 677 } 678 679 } 680 681 @Override 682 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 683 switch (hash) { 684 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 685 case 1968600364: /*information*/ return new String[] {"Reference"}; 686 case -1096846342: /*appliesToAll*/ return new String[] {"boolean"}; 687 default: return super.getTypesForProperty(hash, name); 688 } 689 690 } 691 692 @Override 693 public Base addChild(String name) throws FHIRException { 694 if (name.equals("sequence")) { 695 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.supportingInfo.sequence"); 696 } 697 else if (name.equals("information")) { 698 this.information = new Reference(); 699 return this.information; 700 } 701 else if (name.equals("appliesToAll")) { 702 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.supportingInfo.appliesToAll"); 703 } 704 else 705 return super.addChild(name); 706 } 707 708 public SupportingInformationComponent copy() { 709 SupportingInformationComponent dst = new SupportingInformationComponent(); 710 copyValues(dst); 711 return dst; 712 } 713 714 public void copyValues(SupportingInformationComponent dst) { 715 super.copyValues(dst); 716 dst.sequence = sequence == null ? null : sequence.copy(); 717 dst.information = information == null ? null : information.copy(); 718 dst.appliesToAll = appliesToAll == null ? null : appliesToAll.copy(); 719 } 720 721 @Override 722 public boolean equalsDeep(Base other_) { 723 if (!super.equalsDeep(other_)) 724 return false; 725 if (!(other_ instanceof SupportingInformationComponent)) 726 return false; 727 SupportingInformationComponent o = (SupportingInformationComponent) other_; 728 return compareDeep(sequence, o.sequence, true) && compareDeep(information, o.information, true) 729 && compareDeep(appliesToAll, o.appliesToAll, true); 730 } 731 732 @Override 733 public boolean equalsShallow(Base other_) { 734 if (!super.equalsShallow(other_)) 735 return false; 736 if (!(other_ instanceof SupportingInformationComponent)) 737 return false; 738 SupportingInformationComponent o = (SupportingInformationComponent) other_; 739 return compareValues(sequence, o.sequence, true) && compareValues(appliesToAll, o.appliesToAll, true) 740 ; 741 } 742 743 public boolean isEmpty() { 744 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, information, appliesToAll 745 ); 746 } 747 748 public String fhirType() { 749 return "CoverageEligibilityRequest.supportingInfo"; 750 751 } 752 753 } 754 755 @Block() 756 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 757 /** 758 * A flag to indicate that this Coverage is to be used for evaluation of this request when set to true. 759 */ 760 @Child(name = "focal", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 761 @Description(shortDefinition="Applicable coverage", formalDefinition="A flag to indicate that this Coverage is to be used for evaluation of this request when set to true." ) 762 protected BooleanType focal; 763 764 /** 765 * Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system. 766 */ 767 @Child(name = "coverage", type = {Coverage.class}, order=2, min=1, max=1, modifier=false, summary=false) 768 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system." ) 769 protected Reference coverage; 770 771 /** 772 * A business agreement number established between the provider and the insurer for special business processing purposes. 773 */ 774 @Child(name = "businessArrangement", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 775 @Description(shortDefinition="Additional provider contract number", formalDefinition="A business agreement number established between the provider and the insurer for special business processing purposes." ) 776 protected StringType businessArrangement; 777 778 private static final long serialVersionUID = -1656150261L; 779 780 /** 781 * Constructor 782 */ 783 public InsuranceComponent() { 784 super(); 785 } 786 787 /** 788 * Constructor 789 */ 790 public InsuranceComponent(Reference coverage) { 791 super(); 792 this.setCoverage(coverage); 793 } 794 795 /** 796 * @return {@link #focal} (A flag to indicate that this Coverage is to be used for evaluation of this request when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 797 */ 798 public BooleanType getFocalElement() { 799 if (this.focal == null) 800 if (Configuration.errorOnAutoCreate()) 801 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 802 else if (Configuration.doAutoCreate()) 803 this.focal = new BooleanType(); // bb 804 return this.focal; 805 } 806 807 public boolean hasFocalElement() { 808 return this.focal != null && !this.focal.isEmpty(); 809 } 810 811 public boolean hasFocal() { 812 return this.focal != null && !this.focal.isEmpty(); 813 } 814 815 /** 816 * @param value {@link #focal} (A flag to indicate that this Coverage is to be used for evaluation of this request when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 817 */ 818 public InsuranceComponent setFocalElement(BooleanType value) { 819 this.focal = value; 820 return this; 821 } 822 823 /** 824 * @return A flag to indicate that this Coverage is to be used for evaluation of this request when set to true. 825 */ 826 public boolean getFocal() { 827 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 828 } 829 830 /** 831 * @param value A flag to indicate that this Coverage is to be used for evaluation of this request when set to true. 832 */ 833 public InsuranceComponent setFocal(boolean value) { 834 if (this.focal == null) 835 this.focal = new BooleanType(); 836 this.focal.setValue(value); 837 return this; 838 } 839 840 /** 841 * @return {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 842 */ 843 public Reference getCoverage() { 844 if (this.coverage == null) 845 if (Configuration.errorOnAutoCreate()) 846 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 847 else if (Configuration.doAutoCreate()) 848 this.coverage = new Reference(); // cc 849 return this.coverage; 850 } 851 852 public boolean hasCoverage() { 853 return this.coverage != null && !this.coverage.isEmpty(); 854 } 855 856 /** 857 * @param value {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 858 */ 859 public InsuranceComponent setCoverage(Reference value) { 860 this.coverage = value; 861 return this; 862 } 863 864 /** 865 * @return {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 866 */ 867 public StringType getBusinessArrangementElement() { 868 if (this.businessArrangement == null) 869 if (Configuration.errorOnAutoCreate()) 870 throw new Error("Attempt to auto-create InsuranceComponent.businessArrangement"); 871 else if (Configuration.doAutoCreate()) 872 this.businessArrangement = new StringType(); // bb 873 return this.businessArrangement; 874 } 875 876 public boolean hasBusinessArrangementElement() { 877 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 878 } 879 880 public boolean hasBusinessArrangement() { 881 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 882 } 883 884 /** 885 * @param value {@link #businessArrangement} (A business agreement number established between the provider and the insurer for special business processing purposes.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 886 */ 887 public InsuranceComponent setBusinessArrangementElement(StringType value) { 888 this.businessArrangement = value; 889 return this; 890 } 891 892 /** 893 * @return A business agreement number established between the provider and the insurer for special business processing purposes. 894 */ 895 public String getBusinessArrangement() { 896 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 897 } 898 899 /** 900 * @param value A business agreement number established between the provider and the insurer for special business processing purposes. 901 */ 902 public InsuranceComponent setBusinessArrangement(String value) { 903 if (Utilities.noString(value)) 904 this.businessArrangement = null; 905 else { 906 if (this.businessArrangement == null) 907 this.businessArrangement = new StringType(); 908 this.businessArrangement.setValue(value); 909 } 910 return this; 911 } 912 913 protected void listChildren(List<Property> children) { 914 super.listChildren(children); 915 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for evaluation of this request when set to true.", 0, 1, focal)); 916 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage)); 917 children.add(new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement)); 918 } 919 920 @Override 921 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 922 switch (_hash) { 923 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for evaluation of this request when set to true.", 0, 1, focal); 924 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage); 925 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "A business agreement number established between the provider and the insurer for special business processing purposes.", 0, 1, businessArrangement); 926 default: return super.getNamedProperty(_hash, _name, _checkValid); 927 } 928 929 } 930 931 @Override 932 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 933 switch (hash) { 934 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 935 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 936 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 937 default: return super.getProperty(hash, name, checkValid); 938 } 939 940 } 941 942 @Override 943 public Base setProperty(int hash, String name, Base value) throws FHIRException { 944 switch (hash) { 945 case 97604197: // focal 946 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 947 return value; 948 case -351767064: // coverage 949 this.coverage = TypeConvertor.castToReference(value); // Reference 950 return value; 951 case 259920682: // businessArrangement 952 this.businessArrangement = TypeConvertor.castToString(value); // StringType 953 return value; 954 default: return super.setProperty(hash, name, value); 955 } 956 957 } 958 959 @Override 960 public Base setProperty(String name, Base value) throws FHIRException { 961 if (name.equals("focal")) { 962 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 963 } else if (name.equals("coverage")) { 964 this.coverage = TypeConvertor.castToReference(value); // Reference 965 } else if (name.equals("businessArrangement")) { 966 this.businessArrangement = TypeConvertor.castToString(value); // StringType 967 } else 968 return super.setProperty(name, value); 969 return value; 970 } 971 972 @Override 973 public void removeChild(String name, Base value) throws FHIRException { 974 if (name.equals("focal")) { 975 this.focal = null; 976 } else if (name.equals("coverage")) { 977 this.coverage = null; 978 } else if (name.equals("businessArrangement")) { 979 this.businessArrangement = null; 980 } else 981 super.removeChild(name, value); 982 983 } 984 985 @Override 986 public Base makeProperty(int hash, String name) throws FHIRException { 987 switch (hash) { 988 case 97604197: return getFocalElement(); 989 case -351767064: return getCoverage(); 990 case 259920682: return getBusinessArrangementElement(); 991 default: return super.makeProperty(hash, name); 992 } 993 994 } 995 996 @Override 997 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 998 switch (hash) { 999 case 97604197: /*focal*/ return new String[] {"boolean"}; 1000 case -351767064: /*coverage*/ return new String[] {"Reference"}; 1001 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 1002 default: return super.getTypesForProperty(hash, name); 1003 } 1004 1005 } 1006 1007 @Override 1008 public Base addChild(String name) throws FHIRException { 1009 if (name.equals("focal")) { 1010 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.insurance.focal"); 1011 } 1012 else if (name.equals("coverage")) { 1013 this.coverage = new Reference(); 1014 return this.coverage; 1015 } 1016 else if (name.equals("businessArrangement")) { 1017 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.insurance.businessArrangement"); 1018 } 1019 else 1020 return super.addChild(name); 1021 } 1022 1023 public InsuranceComponent copy() { 1024 InsuranceComponent dst = new InsuranceComponent(); 1025 copyValues(dst); 1026 return dst; 1027 } 1028 1029 public void copyValues(InsuranceComponent dst) { 1030 super.copyValues(dst); 1031 dst.focal = focal == null ? null : focal.copy(); 1032 dst.coverage = coverage == null ? null : coverage.copy(); 1033 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 1034 } 1035 1036 @Override 1037 public boolean equalsDeep(Base other_) { 1038 if (!super.equalsDeep(other_)) 1039 return false; 1040 if (!(other_ instanceof InsuranceComponent)) 1041 return false; 1042 InsuranceComponent o = (InsuranceComponent) other_; 1043 return compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) && compareDeep(businessArrangement, o.businessArrangement, true) 1044 ; 1045 } 1046 1047 @Override 1048 public boolean equalsShallow(Base other_) { 1049 if (!super.equalsShallow(other_)) 1050 return false; 1051 if (!(other_ instanceof InsuranceComponent)) 1052 return false; 1053 InsuranceComponent o = (InsuranceComponent) other_; 1054 return compareValues(focal, o.focal, true) && compareValues(businessArrangement, o.businessArrangement, true) 1055 ; 1056 } 1057 1058 public boolean isEmpty() { 1059 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(focal, coverage, businessArrangement 1060 ); 1061 } 1062 1063 public String fhirType() { 1064 return "CoverageEligibilityRequest.insurance"; 1065 1066 } 1067 1068 } 1069 1070 @Block() 1071 public static class DetailsComponent extends BackboneElement implements IBaseBackboneElement { 1072 /** 1073 * Exceptions, special conditions and supporting information applicable for this service or product line. 1074 */ 1075 @Child(name = "supportingInfoSequence", type = {PositiveIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1076 @Description(shortDefinition="Applicable exception or supporting information", formalDefinition="Exceptions, special conditions and supporting information applicable for this service or product line." ) 1077 protected List<PositiveIntType> supportingInfoSequence; 1078 1079 /** 1080 * Code to identify the general type of benefits under which products and services are provided. 1081 */ 1082 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1083 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 1084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 1085 protected CodeableConcept category; 1086 1087 /** 1088 * This contains the product, service, drug or other billing code for the item. 1089 */ 1090 @Child(name = "productOrService", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1091 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="This contains the product, service, drug or other billing code for the item." ) 1092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 1093 protected CodeableConcept productOrService; 1094 1095 /** 1096 * Item typification or modifiers codes to convey additional context for the product or service. 1097 */ 1098 @Child(name = "modifier", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1099 @Description(shortDefinition="Product or service billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 1100 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 1101 protected List<CodeableConcept> modifier; 1102 1103 /** 1104 * The practitioner who is responsible for the product or service to be rendered to the patient. 1105 */ 1106 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class}, order=5, min=0, max=1, modifier=false, summary=false) 1107 @Description(shortDefinition="Perfoming practitioner", formalDefinition="The practitioner who is responsible for the product or service to be rendered to the patient." ) 1108 protected Reference provider; 1109 1110 /** 1111 * The number of repetitions of a service or product. 1112 */ 1113 @Child(name = "quantity", type = {Quantity.class}, order=6, min=0, max=1, modifier=false, summary=false) 1114 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 1115 protected Quantity quantity; 1116 1117 /** 1118 * The amount charged to the patient by the provider for a single unit. 1119 */ 1120 @Child(name = "unitPrice", type = {Money.class}, order=7, min=0, max=1, modifier=false, summary=false) 1121 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="The amount charged to the patient by the provider for a single unit." ) 1122 protected Money unitPrice; 1123 1124 /** 1125 * Facility where the services will be provided. 1126 */ 1127 @Child(name = "facility", type = {Location.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 1128 @Description(shortDefinition="Servicing facility", formalDefinition="Facility where the services will be provided." ) 1129 protected Reference facility; 1130 1131 /** 1132 * Patient diagnosis for which care is sought. 1133 */ 1134 @Child(name = "diagnosis", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1135 @Description(shortDefinition="Applicable diagnosis", formalDefinition="Patient diagnosis for which care is sought." ) 1136 protected List<DiagnosisComponent> diagnosis; 1137 1138 /** 1139 * The plan/proposal/order describing the proposed service in detail. 1140 */ 1141 @Child(name = "detail", type = {Reference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1142 @Description(shortDefinition="Product or service details", formalDefinition="The plan/proposal/order describing the proposed service in detail." ) 1143 protected List<Reference> detail; 1144 1145 private static final long serialVersionUID = 1615052611L; 1146 1147 /** 1148 * Constructor 1149 */ 1150 public DetailsComponent() { 1151 super(); 1152 } 1153 1154 /** 1155 * @return {@link #supportingInfoSequence} (Exceptions, special conditions and supporting information applicable for this service or product line.) 1156 */ 1157 public List<PositiveIntType> getSupportingInfoSequence() { 1158 if (this.supportingInfoSequence == null) 1159 this.supportingInfoSequence = new ArrayList<PositiveIntType>(); 1160 return this.supportingInfoSequence; 1161 } 1162 1163 /** 1164 * @return Returns a reference to <code>this</code> for easy method chaining 1165 */ 1166 public DetailsComponent setSupportingInfoSequence(List<PositiveIntType> theSupportingInfoSequence) { 1167 this.supportingInfoSequence = theSupportingInfoSequence; 1168 return this; 1169 } 1170 1171 public boolean hasSupportingInfoSequence() { 1172 if (this.supportingInfoSequence == null) 1173 return false; 1174 for (PositiveIntType item : this.supportingInfoSequence) 1175 if (!item.isEmpty()) 1176 return true; 1177 return false; 1178 } 1179 1180 /** 1181 * @return {@link #supportingInfoSequence} (Exceptions, special conditions and supporting information applicable for this service or product line.) 1182 */ 1183 public PositiveIntType addSupportingInfoSequenceElement() {//2 1184 PositiveIntType t = new PositiveIntType(); 1185 if (this.supportingInfoSequence == null) 1186 this.supportingInfoSequence = new ArrayList<PositiveIntType>(); 1187 this.supportingInfoSequence.add(t); 1188 return t; 1189 } 1190 1191 /** 1192 * @param value {@link #supportingInfoSequence} (Exceptions, special conditions and supporting information applicable for this service or product line.) 1193 */ 1194 public DetailsComponent addSupportingInfoSequence(int value) { //1 1195 PositiveIntType t = new PositiveIntType(); 1196 t.setValue(value); 1197 if (this.supportingInfoSequence == null) 1198 this.supportingInfoSequence = new ArrayList<PositiveIntType>(); 1199 this.supportingInfoSequence.add(t); 1200 return this; 1201 } 1202 1203 /** 1204 * @param value {@link #supportingInfoSequence} (Exceptions, special conditions and supporting information applicable for this service or product line.) 1205 */ 1206 public boolean hasSupportingInfoSequence(int value) { 1207 if (this.supportingInfoSequence == null) 1208 return false; 1209 for (PositiveIntType v : this.supportingInfoSequence) 1210 if (v.getValue().equals(value)) // positiveInt 1211 return true; 1212 return false; 1213 } 1214 1215 /** 1216 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 1217 */ 1218 public CodeableConcept getCategory() { 1219 if (this.category == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create DetailsComponent.category"); 1222 else if (Configuration.doAutoCreate()) 1223 this.category = new CodeableConcept(); // cc 1224 return this.category; 1225 } 1226 1227 public boolean hasCategory() { 1228 return this.category != null && !this.category.isEmpty(); 1229 } 1230 1231 /** 1232 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 1233 */ 1234 public DetailsComponent setCategory(CodeableConcept value) { 1235 this.category = value; 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #productOrService} (This contains the product, service, drug or other billing code for the item.) 1241 */ 1242 public CodeableConcept getProductOrService() { 1243 if (this.productOrService == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create DetailsComponent.productOrService"); 1246 else if (Configuration.doAutoCreate()) 1247 this.productOrService = new CodeableConcept(); // cc 1248 return this.productOrService; 1249 } 1250 1251 public boolean hasProductOrService() { 1252 return this.productOrService != null && !this.productOrService.isEmpty(); 1253 } 1254 1255 /** 1256 * @param value {@link #productOrService} (This contains the product, service, drug or other billing code for the item.) 1257 */ 1258 public DetailsComponent setProductOrService(CodeableConcept value) { 1259 this.productOrService = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 1265 */ 1266 public List<CodeableConcept> getModifier() { 1267 if (this.modifier == null) 1268 this.modifier = new ArrayList<CodeableConcept>(); 1269 return this.modifier; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public DetailsComponent setModifier(List<CodeableConcept> theModifier) { 1276 this.modifier = theModifier; 1277 return this; 1278 } 1279 1280 public boolean hasModifier() { 1281 if (this.modifier == null) 1282 return false; 1283 for (CodeableConcept item : this.modifier) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public CodeableConcept addModifier() { //3 1290 CodeableConcept t = new CodeableConcept(); 1291 if (this.modifier == null) 1292 this.modifier = new ArrayList<CodeableConcept>(); 1293 this.modifier.add(t); 1294 return t; 1295 } 1296 1297 public DetailsComponent addModifier(CodeableConcept t) { //3 1298 if (t == null) 1299 return this; 1300 if (this.modifier == null) 1301 this.modifier = new ArrayList<CodeableConcept>(); 1302 this.modifier.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 1308 */ 1309 public CodeableConcept getModifierFirstRep() { 1310 if (getModifier().isEmpty()) { 1311 addModifier(); 1312 } 1313 return getModifier().get(0); 1314 } 1315 1316 /** 1317 * @return {@link #provider} (The practitioner who is responsible for the product or service to be rendered to the patient.) 1318 */ 1319 public Reference getProvider() { 1320 if (this.provider == null) 1321 if (Configuration.errorOnAutoCreate()) 1322 throw new Error("Attempt to auto-create DetailsComponent.provider"); 1323 else if (Configuration.doAutoCreate()) 1324 this.provider = new Reference(); // cc 1325 return this.provider; 1326 } 1327 1328 public boolean hasProvider() { 1329 return this.provider != null && !this.provider.isEmpty(); 1330 } 1331 1332 /** 1333 * @param value {@link #provider} (The practitioner who is responsible for the product or service to be rendered to the patient.) 1334 */ 1335 public DetailsComponent setProvider(Reference value) { 1336 this.provider = value; 1337 return this; 1338 } 1339 1340 /** 1341 * @return {@link #quantity} (The number of repetitions of a service or product.) 1342 */ 1343 public Quantity getQuantity() { 1344 if (this.quantity == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error("Attempt to auto-create DetailsComponent.quantity"); 1347 else if (Configuration.doAutoCreate()) 1348 this.quantity = new Quantity(); // cc 1349 return this.quantity; 1350 } 1351 1352 public boolean hasQuantity() { 1353 return this.quantity != null && !this.quantity.isEmpty(); 1354 } 1355 1356 /** 1357 * @param value {@link #quantity} (The number of repetitions of a service or product.) 1358 */ 1359 public DetailsComponent setQuantity(Quantity value) { 1360 this.quantity = value; 1361 return this; 1362 } 1363 1364 /** 1365 * @return {@link #unitPrice} (The amount charged to the patient by the provider for a single unit.) 1366 */ 1367 public Money getUnitPrice() { 1368 if (this.unitPrice == null) 1369 if (Configuration.errorOnAutoCreate()) 1370 throw new Error("Attempt to auto-create DetailsComponent.unitPrice"); 1371 else if (Configuration.doAutoCreate()) 1372 this.unitPrice = new Money(); // cc 1373 return this.unitPrice; 1374 } 1375 1376 public boolean hasUnitPrice() { 1377 return this.unitPrice != null && !this.unitPrice.isEmpty(); 1378 } 1379 1380 /** 1381 * @param value {@link #unitPrice} (The amount charged to the patient by the provider for a single unit.) 1382 */ 1383 public DetailsComponent setUnitPrice(Money value) { 1384 this.unitPrice = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #facility} (Facility where the services will be provided.) 1390 */ 1391 public Reference getFacility() { 1392 if (this.facility == null) 1393 if (Configuration.errorOnAutoCreate()) 1394 throw new Error("Attempt to auto-create DetailsComponent.facility"); 1395 else if (Configuration.doAutoCreate()) 1396 this.facility = new Reference(); // cc 1397 return this.facility; 1398 } 1399 1400 public boolean hasFacility() { 1401 return this.facility != null && !this.facility.isEmpty(); 1402 } 1403 1404 /** 1405 * @param value {@link #facility} (Facility where the services will be provided.) 1406 */ 1407 public DetailsComponent setFacility(Reference value) { 1408 this.facility = value; 1409 return this; 1410 } 1411 1412 /** 1413 * @return {@link #diagnosis} (Patient diagnosis for which care is sought.) 1414 */ 1415 public List<DiagnosisComponent> getDiagnosis() { 1416 if (this.diagnosis == null) 1417 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1418 return this.diagnosis; 1419 } 1420 1421 /** 1422 * @return Returns a reference to <code>this</code> for easy method chaining 1423 */ 1424 public DetailsComponent setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 1425 this.diagnosis = theDiagnosis; 1426 return this; 1427 } 1428 1429 public boolean hasDiagnosis() { 1430 if (this.diagnosis == null) 1431 return false; 1432 for (DiagnosisComponent item : this.diagnosis) 1433 if (!item.isEmpty()) 1434 return true; 1435 return false; 1436 } 1437 1438 public DiagnosisComponent addDiagnosis() { //3 1439 DiagnosisComponent t = new DiagnosisComponent(); 1440 if (this.diagnosis == null) 1441 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1442 this.diagnosis.add(t); 1443 return t; 1444 } 1445 1446 public DetailsComponent addDiagnosis(DiagnosisComponent t) { //3 1447 if (t == null) 1448 return this; 1449 if (this.diagnosis == null) 1450 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1451 this.diagnosis.add(t); 1452 return this; 1453 } 1454 1455 /** 1456 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 1457 */ 1458 public DiagnosisComponent getDiagnosisFirstRep() { 1459 if (getDiagnosis().isEmpty()) { 1460 addDiagnosis(); 1461 } 1462 return getDiagnosis().get(0); 1463 } 1464 1465 /** 1466 * @return {@link #detail} (The plan/proposal/order describing the proposed service in detail.) 1467 */ 1468 public List<Reference> getDetail() { 1469 if (this.detail == null) 1470 this.detail = new ArrayList<Reference>(); 1471 return this.detail; 1472 } 1473 1474 /** 1475 * @return Returns a reference to <code>this</code> for easy method chaining 1476 */ 1477 public DetailsComponent setDetail(List<Reference> theDetail) { 1478 this.detail = theDetail; 1479 return this; 1480 } 1481 1482 public boolean hasDetail() { 1483 if (this.detail == null) 1484 return false; 1485 for (Reference item : this.detail) 1486 if (!item.isEmpty()) 1487 return true; 1488 return false; 1489 } 1490 1491 public Reference addDetail() { //3 1492 Reference t = new Reference(); 1493 if (this.detail == null) 1494 this.detail = new ArrayList<Reference>(); 1495 this.detail.add(t); 1496 return t; 1497 } 1498 1499 public DetailsComponent addDetail(Reference t) { //3 1500 if (t == null) 1501 return this; 1502 if (this.detail == null) 1503 this.detail = new ArrayList<Reference>(); 1504 this.detail.add(t); 1505 return this; 1506 } 1507 1508 /** 1509 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 1510 */ 1511 public Reference getDetailFirstRep() { 1512 if (getDetail().isEmpty()) { 1513 addDetail(); 1514 } 1515 return getDetail().get(0); 1516 } 1517 1518 protected void listChildren(List<Property> children) { 1519 super.listChildren(children); 1520 children.add(new Property("supportingInfoSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, supportingInfoSequence)); 1521 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 1522 children.add(new Property("productOrService", "CodeableConcept", "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService)); 1523 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 1524 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole)", "The practitioner who is responsible for the product or service to be rendered to the patient.", 0, 1, provider)); 1525 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 1526 children.add(new Property("unitPrice", "Money", "The amount charged to the patient by the provider for a single unit.", 0, 1, unitPrice)); 1527 children.add(new Property("facility", "Reference(Location|Organization)", "Facility where the services will be provided.", 0, 1, facility)); 1528 children.add(new Property("diagnosis", "", "Patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 1529 children.add(new Property("detail", "Reference(Any)", "The plan/proposal/order describing the proposed service in detail.", 0, java.lang.Integer.MAX_VALUE, detail)); 1530 } 1531 1532 @Override 1533 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1534 switch (_hash) { 1535 case -595860510: /*supportingInfoSequence*/ return new Property("supportingInfoSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, supportingInfoSequence); 1536 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 1537 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService); 1538 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 1539 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole)", "The practitioner who is responsible for the product or service to be rendered to the patient.", 0, 1, provider); 1540 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 1541 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "The amount charged to the patient by the provider for a single unit.", 0, 1, unitPrice); 1542 case 501116579: /*facility*/ return new Property("facility", "Reference(Location|Organization)", "Facility where the services will be provided.", 0, 1, facility); 1543 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "Patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 1544 case -1335224239: /*detail*/ return new Property("detail", "Reference(Any)", "The plan/proposal/order describing the proposed service in detail.", 0, java.lang.Integer.MAX_VALUE, detail); 1545 default: return super.getNamedProperty(_hash, _name, _checkValid); 1546 } 1547 1548 } 1549 1550 @Override 1551 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1552 switch (hash) { 1553 case -595860510: /*supportingInfoSequence*/ return this.supportingInfoSequence == null ? new Base[0] : this.supportingInfoSequence.toArray(new Base[this.supportingInfoSequence.size()]); // PositiveIntType 1554 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1555 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 1556 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 1557 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1558 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1559 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 1560 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 1561 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 1562 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 1563 default: return super.getProperty(hash, name, checkValid); 1564 } 1565 1566 } 1567 1568 @Override 1569 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1570 switch (hash) { 1571 case -595860510: // supportingInfoSequence 1572 this.getSupportingInfoSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 1573 return value; 1574 case 50511102: // category 1575 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1576 return value; 1577 case 1957227299: // productOrService 1578 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1579 return value; 1580 case -615513385: // modifier 1581 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1582 return value; 1583 case -987494927: // provider 1584 this.provider = TypeConvertor.castToReference(value); // Reference 1585 return value; 1586 case -1285004149: // quantity 1587 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1588 return value; 1589 case -486196699: // unitPrice 1590 this.unitPrice = TypeConvertor.castToMoney(value); // Money 1591 return value; 1592 case 501116579: // facility 1593 this.facility = TypeConvertor.castToReference(value); // Reference 1594 return value; 1595 case 1196993265: // diagnosis 1596 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 1597 return value; 1598 case -1335224239: // detail 1599 this.getDetail().add(TypeConvertor.castToReference(value)); // Reference 1600 return value; 1601 default: return super.setProperty(hash, name, value); 1602 } 1603 1604 } 1605 1606 @Override 1607 public Base setProperty(String name, Base value) throws FHIRException { 1608 if (name.equals("supportingInfoSequence")) { 1609 this.getSupportingInfoSequence().add(TypeConvertor.castToPositiveInt(value)); 1610 } else if (name.equals("category")) { 1611 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1612 } else if (name.equals("productOrService")) { 1613 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1614 } else if (name.equals("modifier")) { 1615 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 1616 } else if (name.equals("provider")) { 1617 this.provider = TypeConvertor.castToReference(value); // Reference 1618 } else if (name.equals("quantity")) { 1619 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1620 } else if (name.equals("unitPrice")) { 1621 this.unitPrice = TypeConvertor.castToMoney(value); // Money 1622 } else if (name.equals("facility")) { 1623 this.facility = TypeConvertor.castToReference(value); // Reference 1624 } else if (name.equals("diagnosis")) { 1625 this.getDiagnosis().add((DiagnosisComponent) value); 1626 } else if (name.equals("detail")) { 1627 this.getDetail().add(TypeConvertor.castToReference(value)); 1628 } else 1629 return super.setProperty(name, value); 1630 return value; 1631 } 1632 1633 @Override 1634 public void removeChild(String name, Base value) throws FHIRException { 1635 if (name.equals("supportingInfoSequence")) { 1636 this.getSupportingInfoSequence().remove(value); 1637 } else if (name.equals("category")) { 1638 this.category = null; 1639 } else if (name.equals("productOrService")) { 1640 this.productOrService = null; 1641 } else if (name.equals("modifier")) { 1642 this.getModifier().remove(value); 1643 } else if (name.equals("provider")) { 1644 this.provider = null; 1645 } else if (name.equals("quantity")) { 1646 this.quantity = null; 1647 } else if (name.equals("unitPrice")) { 1648 this.unitPrice = null; 1649 } else if (name.equals("facility")) { 1650 this.facility = null; 1651 } else if (name.equals("diagnosis")) { 1652 this.getDiagnosis().remove((DiagnosisComponent) value); 1653 } else if (name.equals("detail")) { 1654 this.getDetail().remove(value); 1655 } else 1656 super.removeChild(name, value); 1657 1658 } 1659 1660 @Override 1661 public Base makeProperty(int hash, String name) throws FHIRException { 1662 switch (hash) { 1663 case -595860510: return addSupportingInfoSequenceElement(); 1664 case 50511102: return getCategory(); 1665 case 1957227299: return getProductOrService(); 1666 case -615513385: return addModifier(); 1667 case -987494927: return getProvider(); 1668 case -1285004149: return getQuantity(); 1669 case -486196699: return getUnitPrice(); 1670 case 501116579: return getFacility(); 1671 case 1196993265: return addDiagnosis(); 1672 case -1335224239: return addDetail(); 1673 default: return super.makeProperty(hash, name); 1674 } 1675 1676 } 1677 1678 @Override 1679 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1680 switch (hash) { 1681 case -595860510: /*supportingInfoSequence*/ return new String[] {"positiveInt"}; 1682 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1683 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 1684 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 1685 case -987494927: /*provider*/ return new String[] {"Reference"}; 1686 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1687 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 1688 case 501116579: /*facility*/ return new String[] {"Reference"}; 1689 case 1196993265: /*diagnosis*/ return new String[] {}; 1690 case -1335224239: /*detail*/ return new String[] {"Reference"}; 1691 default: return super.getTypesForProperty(hash, name); 1692 } 1693 1694 } 1695 1696 @Override 1697 public Base addChild(String name) throws FHIRException { 1698 if (name.equals("supportingInfoSequence")) { 1699 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.item.supportingInfoSequence"); 1700 } 1701 else if (name.equals("category")) { 1702 this.category = new CodeableConcept(); 1703 return this.category; 1704 } 1705 else if (name.equals("productOrService")) { 1706 this.productOrService = new CodeableConcept(); 1707 return this.productOrService; 1708 } 1709 else if (name.equals("modifier")) { 1710 return addModifier(); 1711 } 1712 else if (name.equals("provider")) { 1713 this.provider = new Reference(); 1714 return this.provider; 1715 } 1716 else if (name.equals("quantity")) { 1717 this.quantity = new Quantity(); 1718 return this.quantity; 1719 } 1720 else if (name.equals("unitPrice")) { 1721 this.unitPrice = new Money(); 1722 return this.unitPrice; 1723 } 1724 else if (name.equals("facility")) { 1725 this.facility = new Reference(); 1726 return this.facility; 1727 } 1728 else if (name.equals("diagnosis")) { 1729 return addDiagnosis(); 1730 } 1731 else if (name.equals("detail")) { 1732 return addDetail(); 1733 } 1734 else 1735 return super.addChild(name); 1736 } 1737 1738 public DetailsComponent copy() { 1739 DetailsComponent dst = new DetailsComponent(); 1740 copyValues(dst); 1741 return dst; 1742 } 1743 1744 public void copyValues(DetailsComponent dst) { 1745 super.copyValues(dst); 1746 if (supportingInfoSequence != null) { 1747 dst.supportingInfoSequence = new ArrayList<PositiveIntType>(); 1748 for (PositiveIntType i : supportingInfoSequence) 1749 dst.supportingInfoSequence.add(i.copy()); 1750 }; 1751 dst.category = category == null ? null : category.copy(); 1752 dst.productOrService = productOrService == null ? null : productOrService.copy(); 1753 if (modifier != null) { 1754 dst.modifier = new ArrayList<CodeableConcept>(); 1755 for (CodeableConcept i : modifier) 1756 dst.modifier.add(i.copy()); 1757 }; 1758 dst.provider = provider == null ? null : provider.copy(); 1759 dst.quantity = quantity == null ? null : quantity.copy(); 1760 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 1761 dst.facility = facility == null ? null : facility.copy(); 1762 if (diagnosis != null) { 1763 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 1764 for (DiagnosisComponent i : diagnosis) 1765 dst.diagnosis.add(i.copy()); 1766 }; 1767 if (detail != null) { 1768 dst.detail = new ArrayList<Reference>(); 1769 for (Reference i : detail) 1770 dst.detail.add(i.copy()); 1771 }; 1772 } 1773 1774 @Override 1775 public boolean equalsDeep(Base other_) { 1776 if (!super.equalsDeep(other_)) 1777 return false; 1778 if (!(other_ instanceof DetailsComponent)) 1779 return false; 1780 DetailsComponent o = (DetailsComponent) other_; 1781 return compareDeep(supportingInfoSequence, o.supportingInfoSequence, true) && compareDeep(category, o.category, true) 1782 && compareDeep(productOrService, o.productOrService, true) && compareDeep(modifier, o.modifier, true) 1783 && compareDeep(provider, o.provider, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 1784 && compareDeep(facility, o.facility, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(detail, o.detail, true) 1785 ; 1786 } 1787 1788 @Override 1789 public boolean equalsShallow(Base other_) { 1790 if (!super.equalsShallow(other_)) 1791 return false; 1792 if (!(other_ instanceof DetailsComponent)) 1793 return false; 1794 DetailsComponent o = (DetailsComponent) other_; 1795 return compareValues(supportingInfoSequence, o.supportingInfoSequence, true); 1796 } 1797 1798 public boolean isEmpty() { 1799 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(supportingInfoSequence, category 1800 , productOrService, modifier, provider, quantity, unitPrice, facility, diagnosis 1801 , detail); 1802 } 1803 1804 public String fhirType() { 1805 return "CoverageEligibilityRequest.item"; 1806 1807 } 1808 1809 } 1810 1811 @Block() 1812 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1813 /** 1814 * The nature of illness or problem in a coded form or as a reference to an external defined Condition. 1815 */ 1816 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=1, min=0, max=1, modifier=false, summary=false) 1817 @Description(shortDefinition="Nature of illness or problem", formalDefinition="The nature of illness or problem in a coded form or as a reference to an external defined Condition." ) 1818 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1819 protected DataType diagnosis; 1820 1821 private static final long serialVersionUID = 947131409L; 1822 1823 /** 1824 * Constructor 1825 */ 1826 public DiagnosisComponent() { 1827 super(); 1828 } 1829 1830 /** 1831 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1832 */ 1833 public DataType getDiagnosis() { 1834 return this.diagnosis; 1835 } 1836 1837 /** 1838 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1839 */ 1840 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1841 if (this.diagnosis == null) 1842 this.diagnosis = new CodeableConcept(); 1843 if (!(this.diagnosis instanceof CodeableConcept)) 1844 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1845 return (CodeableConcept) this.diagnosis; 1846 } 1847 1848 public boolean hasDiagnosisCodeableConcept() { 1849 return this.diagnosis instanceof CodeableConcept; 1850 } 1851 1852 /** 1853 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1854 */ 1855 public Reference getDiagnosisReference() throws FHIRException { 1856 if (this.diagnosis == null) 1857 this.diagnosis = new Reference(); 1858 if (!(this.diagnosis instanceof Reference)) 1859 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1860 return (Reference) this.diagnosis; 1861 } 1862 1863 public boolean hasDiagnosisReference() { 1864 return this.diagnosis instanceof Reference; 1865 } 1866 1867 public boolean hasDiagnosis() { 1868 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1869 } 1870 1871 /** 1872 * @param value {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1873 */ 1874 public DiagnosisComponent setDiagnosis(DataType value) { 1875 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1876 throw new FHIRException("Not the right type for CoverageEligibilityRequest.item.diagnosis.diagnosis[x]: "+value.fhirType()); 1877 this.diagnosis = value; 1878 return this; 1879 } 1880 1881 protected void listChildren(List<Property> children) { 1882 super.listChildren(children); 1883 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis)); 1884 } 1885 1886 @Override 1887 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1888 switch (_hash) { 1889 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1890 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1891 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1892 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 1893 default: return super.getNamedProperty(_hash, _name, _checkValid); 1894 } 1895 1896 } 1897 1898 @Override 1899 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1900 switch (hash) { 1901 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // DataType 1902 default: return super.getProperty(hash, name, checkValid); 1903 } 1904 1905 } 1906 1907 @Override 1908 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1909 switch (hash) { 1910 case 1196993265: // diagnosis 1911 this.diagnosis = TypeConvertor.castToType(value); // DataType 1912 return value; 1913 default: return super.setProperty(hash, name, value); 1914 } 1915 1916 } 1917 1918 @Override 1919 public Base setProperty(String name, Base value) throws FHIRException { 1920 if (name.equals("diagnosis[x]")) { 1921 this.diagnosis = TypeConvertor.castToType(value); // DataType 1922 } else 1923 return super.setProperty(name, value); 1924 return value; 1925 } 1926 1927 @Override 1928 public void removeChild(String name, Base value) throws FHIRException { 1929 if (name.equals("diagnosis[x]")) { 1930 this.diagnosis = null; 1931 } else 1932 super.removeChild(name, value); 1933 1934 } 1935 1936 @Override 1937 public Base makeProperty(int hash, String name) throws FHIRException { 1938 switch (hash) { 1939 case -1487009809: return getDiagnosis(); 1940 case 1196993265: return getDiagnosis(); 1941 default: return super.makeProperty(hash, name); 1942 } 1943 1944 } 1945 1946 @Override 1947 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1948 switch (hash) { 1949 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 1950 default: return super.getTypesForProperty(hash, name); 1951 } 1952 1953 } 1954 1955 @Override 1956 public Base addChild(String name) throws FHIRException { 1957 if (name.equals("diagnosisCodeableConcept")) { 1958 this.diagnosis = new CodeableConcept(); 1959 return this.diagnosis; 1960 } 1961 else if (name.equals("diagnosisReference")) { 1962 this.diagnosis = new Reference(); 1963 return this.diagnosis; 1964 } 1965 else 1966 return super.addChild(name); 1967 } 1968 1969 public DiagnosisComponent copy() { 1970 DiagnosisComponent dst = new DiagnosisComponent(); 1971 copyValues(dst); 1972 return dst; 1973 } 1974 1975 public void copyValues(DiagnosisComponent dst) { 1976 super.copyValues(dst); 1977 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 1978 } 1979 1980 @Override 1981 public boolean equalsDeep(Base other_) { 1982 if (!super.equalsDeep(other_)) 1983 return false; 1984 if (!(other_ instanceof DiagnosisComponent)) 1985 return false; 1986 DiagnosisComponent o = (DiagnosisComponent) other_; 1987 return compareDeep(diagnosis, o.diagnosis, true); 1988 } 1989 1990 @Override 1991 public boolean equalsShallow(Base other_) { 1992 if (!super.equalsShallow(other_)) 1993 return false; 1994 if (!(other_ instanceof DiagnosisComponent)) 1995 return false; 1996 DiagnosisComponent o = (DiagnosisComponent) other_; 1997 return true; 1998 } 1999 2000 public boolean isEmpty() { 2001 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(diagnosis); 2002 } 2003 2004 public String fhirType() { 2005 return "CoverageEligibilityRequest.item.diagnosis"; 2006 2007 } 2008 2009 } 2010 2011 /** 2012 * A unique identifier assigned to this coverage eligiblity request. 2013 */ 2014 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2015 @Description(shortDefinition="Business Identifier for coverage eligiblity request", formalDefinition="A unique identifier assigned to this coverage eligiblity request." ) 2016 protected List<Identifier> identifier; 2017 2018 /** 2019 * The status of the resource instance. 2020 */ 2021 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2022 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 2023 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 2024 protected Enumeration<FinancialResourceStatusCodes> status; 2025 2026 /** 2027 * When the requestor expects the processor to complete processing. 2028 */ 2029 @Child(name = "priority", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2030 @Description(shortDefinition="Desired processing priority", formalDefinition="When the requestor expects the processor to complete processing." ) 2031 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 2032 protected CodeableConcept priority; 2033 2034 /** 2035 * Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified. 2036 */ 2037 @Child(name = "purpose", type = {CodeType.class}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2038 @Description(shortDefinition="auth-requirements | benefits | discovery | validation", formalDefinition="Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified." ) 2039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/eligibilityrequest-purpose") 2040 protected List<Enumeration<EligibilityRequestPurpose>> purpose; 2041 2042 /** 2043 * The party who is the beneficiary of the supplied coverage and for whom eligibility is sought. 2044 */ 2045 @Child(name = "patient", type = {Patient.class}, order=4, min=1, max=1, modifier=false, summary=true) 2046 @Description(shortDefinition="Intended recipient of products and services", formalDefinition="The party who is the beneficiary of the supplied coverage and for whom eligibility is sought." ) 2047 protected Reference patient; 2048 2049 /** 2050 * Information code for an event with a corresponding date or period. 2051 */ 2052 @Child(name = "event", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2053 @Description(shortDefinition="Event information", formalDefinition="Information code for an event with a corresponding date or period." ) 2054 protected List<CoverageEligibilityRequestEventComponent> event; 2055 2056 /** 2057 * The date or dates when the enclosed suite of services were performed or completed. 2058 */ 2059 @Child(name = "serviced", type = {DateType.class, Period.class}, order=6, min=0, max=1, modifier=false, summary=false) 2060 @Description(shortDefinition="Estimated date or dates of service", formalDefinition="The date or dates when the enclosed suite of services were performed or completed." ) 2061 protected DataType serviced; 2062 2063 /** 2064 * The date when this resource was created. 2065 */ 2066 @Child(name = "created", type = {DateTimeType.class}, order=7, min=1, max=1, modifier=false, summary=true) 2067 @Description(shortDefinition="Creation date", formalDefinition="The date when this resource was created." ) 2068 protected DateTimeType created; 2069 2070 /** 2071 * Person who created the request. 2072 */ 2073 @Child(name = "enterer", type = {Practitioner.class, PractitionerRole.class}, order=8, min=0, max=1, modifier=false, summary=false) 2074 @Description(shortDefinition="Author", formalDefinition="Person who created the request." ) 2075 protected Reference enterer; 2076 2077 /** 2078 * The provider which is responsible for the request. 2079 */ 2080 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 2081 @Description(shortDefinition="Party responsible for the request", formalDefinition="The provider which is responsible for the request." ) 2082 protected Reference provider; 2083 2084 /** 2085 * The Insurer who issued the coverage in question and is the recipient of the request. 2086 */ 2087 @Child(name = "insurer", type = {Organization.class}, order=10, min=1, max=1, modifier=false, summary=true) 2088 @Description(shortDefinition="Coverage issuer", formalDefinition="The Insurer who issued the coverage in question and is the recipient of the request." ) 2089 protected Reference insurer; 2090 2091 /** 2092 * Facility where the services are intended to be provided. 2093 */ 2094 @Child(name = "facility", type = {Location.class}, order=11, min=0, max=1, modifier=false, summary=false) 2095 @Description(shortDefinition="Servicing facility", formalDefinition="Facility where the services are intended to be provided." ) 2096 protected Reference facility; 2097 2098 /** 2099 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. 2100 */ 2101 @Child(name = "supportingInfo", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2102 @Description(shortDefinition="Supporting information", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues." ) 2103 protected List<SupportingInformationComponent> supportingInfo; 2104 2105 /** 2106 * Financial instruments for reimbursement for the health care products and services. 2107 */ 2108 @Child(name = "insurance", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2109 @Description(shortDefinition="Patient insurance information", formalDefinition="Financial instruments for reimbursement for the health care products and services." ) 2110 protected List<InsuranceComponent> insurance; 2111 2112 /** 2113 * Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor. 2114 */ 2115 @Child(name = "item", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2116 @Description(shortDefinition="Item to be evaluated for eligibiity", formalDefinition="Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor." ) 2117 protected List<DetailsComponent> item; 2118 2119 private static final long serialVersionUID = 2008965302L; 2120 2121 /** 2122 * Constructor 2123 */ 2124 public CoverageEligibilityRequest() { 2125 super(); 2126 } 2127 2128 /** 2129 * Constructor 2130 */ 2131 public CoverageEligibilityRequest(FinancialResourceStatusCodes status, EligibilityRequestPurpose purpose, Reference patient, Date created, Reference insurer) { 2132 super(); 2133 this.setStatus(status); 2134 this.addPurpose(purpose); 2135 this.setPatient(patient); 2136 this.setCreated(created); 2137 this.setInsurer(insurer); 2138 } 2139 2140 /** 2141 * @return {@link #identifier} (A unique identifier assigned to this coverage eligiblity request.) 2142 */ 2143 public List<Identifier> getIdentifier() { 2144 if (this.identifier == null) 2145 this.identifier = new ArrayList<Identifier>(); 2146 return this.identifier; 2147 } 2148 2149 /** 2150 * @return Returns a reference to <code>this</code> for easy method chaining 2151 */ 2152 public CoverageEligibilityRequest setIdentifier(List<Identifier> theIdentifier) { 2153 this.identifier = theIdentifier; 2154 return this; 2155 } 2156 2157 public boolean hasIdentifier() { 2158 if (this.identifier == null) 2159 return false; 2160 for (Identifier item : this.identifier) 2161 if (!item.isEmpty()) 2162 return true; 2163 return false; 2164 } 2165 2166 public Identifier addIdentifier() { //3 2167 Identifier t = new Identifier(); 2168 if (this.identifier == null) 2169 this.identifier = new ArrayList<Identifier>(); 2170 this.identifier.add(t); 2171 return t; 2172 } 2173 2174 public CoverageEligibilityRequest addIdentifier(Identifier t) { //3 2175 if (t == null) 2176 return this; 2177 if (this.identifier == null) 2178 this.identifier = new ArrayList<Identifier>(); 2179 this.identifier.add(t); 2180 return this; 2181 } 2182 2183 /** 2184 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2185 */ 2186 public Identifier getIdentifierFirstRep() { 2187 if (getIdentifier().isEmpty()) { 2188 addIdentifier(); 2189 } 2190 return getIdentifier().get(0); 2191 } 2192 2193 /** 2194 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2195 */ 2196 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 2197 if (this.status == null) 2198 if (Configuration.errorOnAutoCreate()) 2199 throw new Error("Attempt to auto-create CoverageEligibilityRequest.status"); 2200 else if (Configuration.doAutoCreate()) 2201 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 2202 return this.status; 2203 } 2204 2205 public boolean hasStatusElement() { 2206 return this.status != null && !this.status.isEmpty(); 2207 } 2208 2209 public boolean hasStatus() { 2210 return this.status != null && !this.status.isEmpty(); 2211 } 2212 2213 /** 2214 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2215 */ 2216 public CoverageEligibilityRequest setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 2217 this.status = value; 2218 return this; 2219 } 2220 2221 /** 2222 * @return The status of the resource instance. 2223 */ 2224 public FinancialResourceStatusCodes getStatus() { 2225 return this.status == null ? null : this.status.getValue(); 2226 } 2227 2228 /** 2229 * @param value The status of the resource instance. 2230 */ 2231 public CoverageEligibilityRequest setStatus(FinancialResourceStatusCodes value) { 2232 if (this.status == null) 2233 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 2234 this.status.setValue(value); 2235 return this; 2236 } 2237 2238 /** 2239 * @return {@link #priority} (When the requestor expects the processor to complete processing.) 2240 */ 2241 public CodeableConcept getPriority() { 2242 if (this.priority == null) 2243 if (Configuration.errorOnAutoCreate()) 2244 throw new Error("Attempt to auto-create CoverageEligibilityRequest.priority"); 2245 else if (Configuration.doAutoCreate()) 2246 this.priority = new CodeableConcept(); // cc 2247 return this.priority; 2248 } 2249 2250 public boolean hasPriority() { 2251 return this.priority != null && !this.priority.isEmpty(); 2252 } 2253 2254 /** 2255 * @param value {@link #priority} (When the requestor expects the processor to complete processing.) 2256 */ 2257 public CoverageEligibilityRequest setPriority(CodeableConcept value) { 2258 this.priority = value; 2259 return this; 2260 } 2261 2262 /** 2263 * @return {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2264 */ 2265 public List<Enumeration<EligibilityRequestPurpose>> getPurpose() { 2266 if (this.purpose == null) 2267 this.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 2268 return this.purpose; 2269 } 2270 2271 /** 2272 * @return Returns a reference to <code>this</code> for easy method chaining 2273 */ 2274 public CoverageEligibilityRequest setPurpose(List<Enumeration<EligibilityRequestPurpose>> thePurpose) { 2275 this.purpose = thePurpose; 2276 return this; 2277 } 2278 2279 public boolean hasPurpose() { 2280 if (this.purpose == null) 2281 return false; 2282 for (Enumeration<EligibilityRequestPurpose> item : this.purpose) 2283 if (!item.isEmpty()) 2284 return true; 2285 return false; 2286 } 2287 2288 /** 2289 * @return {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2290 */ 2291 public Enumeration<EligibilityRequestPurpose> addPurposeElement() {//2 2292 Enumeration<EligibilityRequestPurpose> t = new Enumeration<EligibilityRequestPurpose>(new EligibilityRequestPurposeEnumFactory()); 2293 if (this.purpose == null) 2294 this.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 2295 this.purpose.add(t); 2296 return t; 2297 } 2298 2299 /** 2300 * @param value {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2301 */ 2302 public CoverageEligibilityRequest addPurpose(EligibilityRequestPurpose value) { //1 2303 Enumeration<EligibilityRequestPurpose> t = new Enumeration<EligibilityRequestPurpose>(new EligibilityRequestPurposeEnumFactory()); 2304 t.setValue(value); 2305 if (this.purpose == null) 2306 this.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 2307 this.purpose.add(t); 2308 return this; 2309 } 2310 2311 /** 2312 * @param value {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2313 */ 2314 public boolean hasPurpose(EligibilityRequestPurpose value) { 2315 if (this.purpose == null) 2316 return false; 2317 for (Enumeration<EligibilityRequestPurpose> v : this.purpose) 2318 if (v.getValue().equals(value)) // code 2319 return true; 2320 return false; 2321 } 2322 2323 /** 2324 * @return {@link #patient} (The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.) 2325 */ 2326 public Reference getPatient() { 2327 if (this.patient == null) 2328 if (Configuration.errorOnAutoCreate()) 2329 throw new Error("Attempt to auto-create CoverageEligibilityRequest.patient"); 2330 else if (Configuration.doAutoCreate()) 2331 this.patient = new Reference(); // cc 2332 return this.patient; 2333 } 2334 2335 public boolean hasPatient() { 2336 return this.patient != null && !this.patient.isEmpty(); 2337 } 2338 2339 /** 2340 * @param value {@link #patient} (The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.) 2341 */ 2342 public CoverageEligibilityRequest setPatient(Reference value) { 2343 this.patient = value; 2344 return this; 2345 } 2346 2347 /** 2348 * @return {@link #event} (Information code for an event with a corresponding date or period.) 2349 */ 2350 public List<CoverageEligibilityRequestEventComponent> getEvent() { 2351 if (this.event == null) 2352 this.event = new ArrayList<CoverageEligibilityRequestEventComponent>(); 2353 return this.event; 2354 } 2355 2356 /** 2357 * @return Returns a reference to <code>this</code> for easy method chaining 2358 */ 2359 public CoverageEligibilityRequest setEvent(List<CoverageEligibilityRequestEventComponent> theEvent) { 2360 this.event = theEvent; 2361 return this; 2362 } 2363 2364 public boolean hasEvent() { 2365 if (this.event == null) 2366 return false; 2367 for (CoverageEligibilityRequestEventComponent item : this.event) 2368 if (!item.isEmpty()) 2369 return true; 2370 return false; 2371 } 2372 2373 public CoverageEligibilityRequestEventComponent addEvent() { //3 2374 CoverageEligibilityRequestEventComponent t = new CoverageEligibilityRequestEventComponent(); 2375 if (this.event == null) 2376 this.event = new ArrayList<CoverageEligibilityRequestEventComponent>(); 2377 this.event.add(t); 2378 return t; 2379 } 2380 2381 public CoverageEligibilityRequest addEvent(CoverageEligibilityRequestEventComponent t) { //3 2382 if (t == null) 2383 return this; 2384 if (this.event == null) 2385 this.event = new ArrayList<CoverageEligibilityRequestEventComponent>(); 2386 this.event.add(t); 2387 return this; 2388 } 2389 2390 /** 2391 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 2392 */ 2393 public CoverageEligibilityRequestEventComponent getEventFirstRep() { 2394 if (getEvent().isEmpty()) { 2395 addEvent(); 2396 } 2397 return getEvent().get(0); 2398 } 2399 2400 /** 2401 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 2402 */ 2403 public DataType getServiced() { 2404 return this.serviced; 2405 } 2406 2407 /** 2408 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 2409 */ 2410 public DateType getServicedDateType() throws FHIRException { 2411 if (this.serviced == null) 2412 this.serviced = new DateType(); 2413 if (!(this.serviced instanceof DateType)) 2414 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 2415 return (DateType) this.serviced; 2416 } 2417 2418 public boolean hasServicedDateType() { 2419 return this.serviced instanceof DateType; 2420 } 2421 2422 /** 2423 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 2424 */ 2425 public Period getServicedPeriod() throws FHIRException { 2426 if (this.serviced == null) 2427 this.serviced = new Period(); 2428 if (!(this.serviced instanceof Period)) 2429 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 2430 return (Period) this.serviced; 2431 } 2432 2433 public boolean hasServicedPeriod() { 2434 return this.serviced instanceof Period; 2435 } 2436 2437 public boolean hasServiced() { 2438 return this.serviced != null && !this.serviced.isEmpty(); 2439 } 2440 2441 /** 2442 * @param value {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 2443 */ 2444 public CoverageEligibilityRequest setServiced(DataType value) { 2445 if (value != null && !(value instanceof DateType || value instanceof Period)) 2446 throw new FHIRException("Not the right type for CoverageEligibilityRequest.serviced[x]: "+value.fhirType()); 2447 this.serviced = value; 2448 return this; 2449 } 2450 2451 /** 2452 * @return {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 2453 */ 2454 public DateTimeType getCreatedElement() { 2455 if (this.created == null) 2456 if (Configuration.errorOnAutoCreate()) 2457 throw new Error("Attempt to auto-create CoverageEligibilityRequest.created"); 2458 else if (Configuration.doAutoCreate()) 2459 this.created = new DateTimeType(); // bb 2460 return this.created; 2461 } 2462 2463 public boolean hasCreatedElement() { 2464 return this.created != null && !this.created.isEmpty(); 2465 } 2466 2467 public boolean hasCreated() { 2468 return this.created != null && !this.created.isEmpty(); 2469 } 2470 2471 /** 2472 * @param value {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 2473 */ 2474 public CoverageEligibilityRequest setCreatedElement(DateTimeType value) { 2475 this.created = value; 2476 return this; 2477 } 2478 2479 /** 2480 * @return The date when this resource was created. 2481 */ 2482 public Date getCreated() { 2483 return this.created == null ? null : this.created.getValue(); 2484 } 2485 2486 /** 2487 * @param value The date when this resource was created. 2488 */ 2489 public CoverageEligibilityRequest setCreated(Date value) { 2490 if (this.created == null) 2491 this.created = new DateTimeType(); 2492 this.created.setValue(value); 2493 return this; 2494 } 2495 2496 /** 2497 * @return {@link #enterer} (Person who created the request.) 2498 */ 2499 public Reference getEnterer() { 2500 if (this.enterer == null) 2501 if (Configuration.errorOnAutoCreate()) 2502 throw new Error("Attempt to auto-create CoverageEligibilityRequest.enterer"); 2503 else if (Configuration.doAutoCreate()) 2504 this.enterer = new Reference(); // cc 2505 return this.enterer; 2506 } 2507 2508 public boolean hasEnterer() { 2509 return this.enterer != null && !this.enterer.isEmpty(); 2510 } 2511 2512 /** 2513 * @param value {@link #enterer} (Person who created the request.) 2514 */ 2515 public CoverageEligibilityRequest setEnterer(Reference value) { 2516 this.enterer = value; 2517 return this; 2518 } 2519 2520 /** 2521 * @return {@link #provider} (The provider which is responsible for the request.) 2522 */ 2523 public Reference getProvider() { 2524 if (this.provider == null) 2525 if (Configuration.errorOnAutoCreate()) 2526 throw new Error("Attempt to auto-create CoverageEligibilityRequest.provider"); 2527 else if (Configuration.doAutoCreate()) 2528 this.provider = new Reference(); // cc 2529 return this.provider; 2530 } 2531 2532 public boolean hasProvider() { 2533 return this.provider != null && !this.provider.isEmpty(); 2534 } 2535 2536 /** 2537 * @param value {@link #provider} (The provider which is responsible for the request.) 2538 */ 2539 public CoverageEligibilityRequest setProvider(Reference value) { 2540 this.provider = value; 2541 return this; 2542 } 2543 2544 /** 2545 * @return {@link #insurer} (The Insurer who issued the coverage in question and is the recipient of the request.) 2546 */ 2547 public Reference getInsurer() { 2548 if (this.insurer == null) 2549 if (Configuration.errorOnAutoCreate()) 2550 throw new Error("Attempt to auto-create CoverageEligibilityRequest.insurer"); 2551 else if (Configuration.doAutoCreate()) 2552 this.insurer = new Reference(); // cc 2553 return this.insurer; 2554 } 2555 2556 public boolean hasInsurer() { 2557 return this.insurer != null && !this.insurer.isEmpty(); 2558 } 2559 2560 /** 2561 * @param value {@link #insurer} (The Insurer who issued the coverage in question and is the recipient of the request.) 2562 */ 2563 public CoverageEligibilityRequest setInsurer(Reference value) { 2564 this.insurer = value; 2565 return this; 2566 } 2567 2568 /** 2569 * @return {@link #facility} (Facility where the services are intended to be provided.) 2570 */ 2571 public Reference getFacility() { 2572 if (this.facility == null) 2573 if (Configuration.errorOnAutoCreate()) 2574 throw new Error("Attempt to auto-create CoverageEligibilityRequest.facility"); 2575 else if (Configuration.doAutoCreate()) 2576 this.facility = new Reference(); // cc 2577 return this.facility; 2578 } 2579 2580 public boolean hasFacility() { 2581 return this.facility != null && !this.facility.isEmpty(); 2582 } 2583 2584 /** 2585 * @param value {@link #facility} (Facility where the services are intended to be provided.) 2586 */ 2587 public CoverageEligibilityRequest setFacility(Reference value) { 2588 this.facility = value; 2589 return this; 2590 } 2591 2592 /** 2593 * @return {@link #supportingInfo} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.) 2594 */ 2595 public List<SupportingInformationComponent> getSupportingInfo() { 2596 if (this.supportingInfo == null) 2597 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 2598 return this.supportingInfo; 2599 } 2600 2601 /** 2602 * @return Returns a reference to <code>this</code> for easy method chaining 2603 */ 2604 public CoverageEligibilityRequest setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 2605 this.supportingInfo = theSupportingInfo; 2606 return this; 2607 } 2608 2609 public boolean hasSupportingInfo() { 2610 if (this.supportingInfo == null) 2611 return false; 2612 for (SupportingInformationComponent item : this.supportingInfo) 2613 if (!item.isEmpty()) 2614 return true; 2615 return false; 2616 } 2617 2618 public SupportingInformationComponent addSupportingInfo() { //3 2619 SupportingInformationComponent t = new SupportingInformationComponent(); 2620 if (this.supportingInfo == null) 2621 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 2622 this.supportingInfo.add(t); 2623 return t; 2624 } 2625 2626 public CoverageEligibilityRequest addSupportingInfo(SupportingInformationComponent t) { //3 2627 if (t == null) 2628 return this; 2629 if (this.supportingInfo == null) 2630 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 2631 this.supportingInfo.add(t); 2632 return this; 2633 } 2634 2635 /** 2636 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 2637 */ 2638 public SupportingInformationComponent getSupportingInfoFirstRep() { 2639 if (getSupportingInfo().isEmpty()) { 2640 addSupportingInfo(); 2641 } 2642 return getSupportingInfo().get(0); 2643 } 2644 2645 /** 2646 * @return {@link #insurance} (Financial instruments for reimbursement for the health care products and services.) 2647 */ 2648 public List<InsuranceComponent> getInsurance() { 2649 if (this.insurance == null) 2650 this.insurance = new ArrayList<InsuranceComponent>(); 2651 return this.insurance; 2652 } 2653 2654 /** 2655 * @return Returns a reference to <code>this</code> for easy method chaining 2656 */ 2657 public CoverageEligibilityRequest setInsurance(List<InsuranceComponent> theInsurance) { 2658 this.insurance = theInsurance; 2659 return this; 2660 } 2661 2662 public boolean hasInsurance() { 2663 if (this.insurance == null) 2664 return false; 2665 for (InsuranceComponent item : this.insurance) 2666 if (!item.isEmpty()) 2667 return true; 2668 return false; 2669 } 2670 2671 public InsuranceComponent addInsurance() { //3 2672 InsuranceComponent t = new InsuranceComponent(); 2673 if (this.insurance == null) 2674 this.insurance = new ArrayList<InsuranceComponent>(); 2675 this.insurance.add(t); 2676 return t; 2677 } 2678 2679 public CoverageEligibilityRequest addInsurance(InsuranceComponent t) { //3 2680 if (t == null) 2681 return this; 2682 if (this.insurance == null) 2683 this.insurance = new ArrayList<InsuranceComponent>(); 2684 this.insurance.add(t); 2685 return this; 2686 } 2687 2688 /** 2689 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 2690 */ 2691 public InsuranceComponent getInsuranceFirstRep() { 2692 if (getInsurance().isEmpty()) { 2693 addInsurance(); 2694 } 2695 return getInsurance().get(0); 2696 } 2697 2698 /** 2699 * @return {@link #item} (Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor.) 2700 */ 2701 public List<DetailsComponent> getItem() { 2702 if (this.item == null) 2703 this.item = new ArrayList<DetailsComponent>(); 2704 return this.item; 2705 } 2706 2707 /** 2708 * @return Returns a reference to <code>this</code> for easy method chaining 2709 */ 2710 public CoverageEligibilityRequest setItem(List<DetailsComponent> theItem) { 2711 this.item = theItem; 2712 return this; 2713 } 2714 2715 public boolean hasItem() { 2716 if (this.item == null) 2717 return false; 2718 for (DetailsComponent item : this.item) 2719 if (!item.isEmpty()) 2720 return true; 2721 return false; 2722 } 2723 2724 public DetailsComponent addItem() { //3 2725 DetailsComponent t = new DetailsComponent(); 2726 if (this.item == null) 2727 this.item = new ArrayList<DetailsComponent>(); 2728 this.item.add(t); 2729 return t; 2730 } 2731 2732 public CoverageEligibilityRequest addItem(DetailsComponent t) { //3 2733 if (t == null) 2734 return this; 2735 if (this.item == null) 2736 this.item = new ArrayList<DetailsComponent>(); 2737 this.item.add(t); 2738 return this; 2739 } 2740 2741 /** 2742 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 2743 */ 2744 public DetailsComponent getItemFirstRep() { 2745 if (getItem().isEmpty()) { 2746 addItem(); 2747 } 2748 return getItem().get(0); 2749 } 2750 2751 protected void listChildren(List<Property> children) { 2752 super.listChildren(children); 2753 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this coverage eligiblity request.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2754 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2755 children.add(new Property("priority", "CodeableConcept", "When the requestor expects the processor to complete processing.", 0, 1, priority)); 2756 children.add(new Property("purpose", "code", "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 0, java.lang.Integer.MAX_VALUE, purpose)); 2757 children.add(new Property("patient", "Reference(Patient)", "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, patient)); 2758 children.add(new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event)); 2759 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 2760 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 2761 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole)", "Person who created the request.", 0, 1, enterer)); 2762 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the request.", 0, 1, provider)); 2763 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who issued the coverage in question and is the recipient of the request.", 0, 1, insurer)); 2764 children.add(new Property("facility", "Reference(Location)", "Facility where the services are intended to be provided.", 0, 1, facility)); 2765 children.add(new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 2766 children.add(new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services.", 0, java.lang.Integer.MAX_VALUE, insurance)); 2767 children.add(new Property("item", "", "Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor.", 0, java.lang.Integer.MAX_VALUE, item)); 2768 } 2769 2770 @Override 2771 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2772 switch (_hash) { 2773 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this coverage eligiblity request.", 0, java.lang.Integer.MAX_VALUE, identifier); 2774 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2775 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "When the requestor expects the processor to complete processing.", 0, 1, priority); 2776 case -220463842: /*purpose*/ return new Property("purpose", "code", "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 0, java.lang.Integer.MAX_VALUE, purpose); 2777 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, patient); 2778 case 96891546: /*event*/ return new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event); 2779 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 2780 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 2781 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 2782 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 2783 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created); 2784 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|PractitionerRole)", "Person who created the request.", 0, 1, enterer); 2785 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the request.", 0, 1, provider); 2786 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who issued the coverage in question and is the recipient of the request.", 0, 1, insurer); 2787 case 501116579: /*facility*/ return new Property("facility", "Reference(Location)", "Facility where the services are intended to be provided.", 0, 1, facility); 2788 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2789 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services.", 0, java.lang.Integer.MAX_VALUE, insurance); 2790 case 3242771: /*item*/ return new Property("item", "", "Service categories or billable services for which benefit details and/or an authorization prior to service delivery may be required by the payor.", 0, java.lang.Integer.MAX_VALUE, item); 2791 default: return super.getNamedProperty(_hash, _name, _checkValid); 2792 } 2793 2794 } 2795 2796 @Override 2797 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2798 switch (hash) { 2799 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2800 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 2801 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 2802 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Enumeration<EligibilityRequestPurpose> 2803 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2804 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CoverageEligibilityRequestEventComponent 2805 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 2806 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 2807 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 2808 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 2809 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 2810 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 2811 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 2812 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 2813 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // DetailsComponent 2814 default: return super.getProperty(hash, name, checkValid); 2815 } 2816 2817 } 2818 2819 @Override 2820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2821 switch (hash) { 2822 case -1618432855: // identifier 2823 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2824 return value; 2825 case -892481550: // status 2826 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2827 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2828 return value; 2829 case -1165461084: // priority 2830 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2831 return value; 2832 case -220463842: // purpose 2833 value = new EligibilityRequestPurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2834 this.getPurpose().add((Enumeration) value); // Enumeration<EligibilityRequestPurpose> 2835 return value; 2836 case -791418107: // patient 2837 this.patient = TypeConvertor.castToReference(value); // Reference 2838 return value; 2839 case 96891546: // event 2840 this.getEvent().add((CoverageEligibilityRequestEventComponent) value); // CoverageEligibilityRequestEventComponent 2841 return value; 2842 case 1379209295: // serviced 2843 this.serviced = TypeConvertor.castToType(value); // DataType 2844 return value; 2845 case 1028554472: // created 2846 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 2847 return value; 2848 case -1591951995: // enterer 2849 this.enterer = TypeConvertor.castToReference(value); // Reference 2850 return value; 2851 case -987494927: // provider 2852 this.provider = TypeConvertor.castToReference(value); // Reference 2853 return value; 2854 case 1957615864: // insurer 2855 this.insurer = TypeConvertor.castToReference(value); // Reference 2856 return value; 2857 case 501116579: // facility 2858 this.facility = TypeConvertor.castToReference(value); // Reference 2859 return value; 2860 case 1922406657: // supportingInfo 2861 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 2862 return value; 2863 case 73049818: // insurance 2864 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 2865 return value; 2866 case 3242771: // item 2867 this.getItem().add((DetailsComponent) value); // DetailsComponent 2868 return value; 2869 default: return super.setProperty(hash, name, value); 2870 } 2871 2872 } 2873 2874 @Override 2875 public Base setProperty(String name, Base value) throws FHIRException { 2876 if (name.equals("identifier")) { 2877 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2878 } else if (name.equals("status")) { 2879 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2880 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2881 } else if (name.equals("priority")) { 2882 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2883 } else if (name.equals("purpose")) { 2884 value = new EligibilityRequestPurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2885 this.getPurpose().add((Enumeration) value); 2886 } else if (name.equals("patient")) { 2887 this.patient = TypeConvertor.castToReference(value); // Reference 2888 } else if (name.equals("event")) { 2889 this.getEvent().add((CoverageEligibilityRequestEventComponent) value); 2890 } else if (name.equals("serviced[x]")) { 2891 this.serviced = TypeConvertor.castToType(value); // DataType 2892 } else if (name.equals("created")) { 2893 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 2894 } else if (name.equals("enterer")) { 2895 this.enterer = TypeConvertor.castToReference(value); // Reference 2896 } else if (name.equals("provider")) { 2897 this.provider = TypeConvertor.castToReference(value); // Reference 2898 } else if (name.equals("insurer")) { 2899 this.insurer = TypeConvertor.castToReference(value); // Reference 2900 } else if (name.equals("facility")) { 2901 this.facility = TypeConvertor.castToReference(value); // Reference 2902 } else if (name.equals("supportingInfo")) { 2903 this.getSupportingInfo().add((SupportingInformationComponent) value); 2904 } else if (name.equals("insurance")) { 2905 this.getInsurance().add((InsuranceComponent) value); 2906 } else if (name.equals("item")) { 2907 this.getItem().add((DetailsComponent) value); 2908 } else 2909 return super.setProperty(name, value); 2910 return value; 2911 } 2912 2913 @Override 2914 public void removeChild(String name, Base value) throws FHIRException { 2915 if (name.equals("identifier")) { 2916 this.getIdentifier().remove(value); 2917 } else if (name.equals("status")) { 2918 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2919 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 2920 } else if (name.equals("priority")) { 2921 this.priority = null; 2922 } else if (name.equals("purpose")) { 2923 value = new EligibilityRequestPurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2924 this.getPurpose().remove((Enumeration) value); 2925 } else if (name.equals("patient")) { 2926 this.patient = null; 2927 } else if (name.equals("event")) { 2928 this.getEvent().remove((CoverageEligibilityRequestEventComponent) value); 2929 } else if (name.equals("serviced[x]")) { 2930 this.serviced = null; 2931 } else if (name.equals("created")) { 2932 this.created = null; 2933 } else if (name.equals("enterer")) { 2934 this.enterer = null; 2935 } else if (name.equals("provider")) { 2936 this.provider = null; 2937 } else if (name.equals("insurer")) { 2938 this.insurer = null; 2939 } else if (name.equals("facility")) { 2940 this.facility = null; 2941 } else if (name.equals("supportingInfo")) { 2942 this.getSupportingInfo().remove((SupportingInformationComponent) value); 2943 } else if (name.equals("insurance")) { 2944 this.getInsurance().remove((InsuranceComponent) value); 2945 } else if (name.equals("item")) { 2946 this.getItem().remove((DetailsComponent) value); 2947 } else 2948 super.removeChild(name, value); 2949 2950 } 2951 2952 @Override 2953 public Base makeProperty(int hash, String name) throws FHIRException { 2954 switch (hash) { 2955 case -1618432855: return addIdentifier(); 2956 case -892481550: return getStatusElement(); 2957 case -1165461084: return getPriority(); 2958 case -220463842: return addPurposeElement(); 2959 case -791418107: return getPatient(); 2960 case 96891546: return addEvent(); 2961 case -1927922223: return getServiced(); 2962 case 1379209295: return getServiced(); 2963 case 1028554472: return getCreatedElement(); 2964 case -1591951995: return getEnterer(); 2965 case -987494927: return getProvider(); 2966 case 1957615864: return getInsurer(); 2967 case 501116579: return getFacility(); 2968 case 1922406657: return addSupportingInfo(); 2969 case 73049818: return addInsurance(); 2970 case 3242771: return addItem(); 2971 default: return super.makeProperty(hash, name); 2972 } 2973 2974 } 2975 2976 @Override 2977 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2978 switch (hash) { 2979 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2980 case -892481550: /*status*/ return new String[] {"code"}; 2981 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 2982 case -220463842: /*purpose*/ return new String[] {"code"}; 2983 case -791418107: /*patient*/ return new String[] {"Reference"}; 2984 case 96891546: /*event*/ return new String[] {}; 2985 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 2986 case 1028554472: /*created*/ return new String[] {"dateTime"}; 2987 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 2988 case -987494927: /*provider*/ return new String[] {"Reference"}; 2989 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 2990 case 501116579: /*facility*/ return new String[] {"Reference"}; 2991 case 1922406657: /*supportingInfo*/ return new String[] {}; 2992 case 73049818: /*insurance*/ return new String[] {}; 2993 case 3242771: /*item*/ return new String[] {}; 2994 default: return super.getTypesForProperty(hash, name); 2995 } 2996 2997 } 2998 2999 @Override 3000 public Base addChild(String name) throws FHIRException { 3001 if (name.equals("identifier")) { 3002 return addIdentifier(); 3003 } 3004 else if (name.equals("status")) { 3005 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.status"); 3006 } 3007 else if (name.equals("priority")) { 3008 this.priority = new CodeableConcept(); 3009 return this.priority; 3010 } 3011 else if (name.equals("purpose")) { 3012 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.purpose"); 3013 } 3014 else if (name.equals("patient")) { 3015 this.patient = new Reference(); 3016 return this.patient; 3017 } 3018 else if (name.equals("event")) { 3019 return addEvent(); 3020 } 3021 else if (name.equals("servicedDate")) { 3022 this.serviced = new DateType(); 3023 return this.serviced; 3024 } 3025 else if (name.equals("servicedPeriod")) { 3026 this.serviced = new Period(); 3027 return this.serviced; 3028 } 3029 else if (name.equals("created")) { 3030 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityRequest.created"); 3031 } 3032 else if (name.equals("enterer")) { 3033 this.enterer = new Reference(); 3034 return this.enterer; 3035 } 3036 else if (name.equals("provider")) { 3037 this.provider = new Reference(); 3038 return this.provider; 3039 } 3040 else if (name.equals("insurer")) { 3041 this.insurer = new Reference(); 3042 return this.insurer; 3043 } 3044 else if (name.equals("facility")) { 3045 this.facility = new Reference(); 3046 return this.facility; 3047 } 3048 else if (name.equals("supportingInfo")) { 3049 return addSupportingInfo(); 3050 } 3051 else if (name.equals("insurance")) { 3052 return addInsurance(); 3053 } 3054 else if (name.equals("item")) { 3055 return addItem(); 3056 } 3057 else 3058 return super.addChild(name); 3059 } 3060 3061 public String fhirType() { 3062 return "CoverageEligibilityRequest"; 3063 3064 } 3065 3066 public CoverageEligibilityRequest copy() { 3067 CoverageEligibilityRequest dst = new CoverageEligibilityRequest(); 3068 copyValues(dst); 3069 return dst; 3070 } 3071 3072 public void copyValues(CoverageEligibilityRequest dst) { 3073 super.copyValues(dst); 3074 if (identifier != null) { 3075 dst.identifier = new ArrayList<Identifier>(); 3076 for (Identifier i : identifier) 3077 dst.identifier.add(i.copy()); 3078 }; 3079 dst.status = status == null ? null : status.copy(); 3080 dst.priority = priority == null ? null : priority.copy(); 3081 if (purpose != null) { 3082 dst.purpose = new ArrayList<Enumeration<EligibilityRequestPurpose>>(); 3083 for (Enumeration<EligibilityRequestPurpose> i : purpose) 3084 dst.purpose.add(i.copy()); 3085 }; 3086 dst.patient = patient == null ? null : patient.copy(); 3087 if (event != null) { 3088 dst.event = new ArrayList<CoverageEligibilityRequestEventComponent>(); 3089 for (CoverageEligibilityRequestEventComponent i : event) 3090 dst.event.add(i.copy()); 3091 }; 3092 dst.serviced = serviced == null ? null : serviced.copy(); 3093 dst.created = created == null ? null : created.copy(); 3094 dst.enterer = enterer == null ? null : enterer.copy(); 3095 dst.provider = provider == null ? null : provider.copy(); 3096 dst.insurer = insurer == null ? null : insurer.copy(); 3097 dst.facility = facility == null ? null : facility.copy(); 3098 if (supportingInfo != null) { 3099 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 3100 for (SupportingInformationComponent i : supportingInfo) 3101 dst.supportingInfo.add(i.copy()); 3102 }; 3103 if (insurance != null) { 3104 dst.insurance = new ArrayList<InsuranceComponent>(); 3105 for (InsuranceComponent i : insurance) 3106 dst.insurance.add(i.copy()); 3107 }; 3108 if (item != null) { 3109 dst.item = new ArrayList<DetailsComponent>(); 3110 for (DetailsComponent i : item) 3111 dst.item.add(i.copy()); 3112 }; 3113 } 3114 3115 protected CoverageEligibilityRequest typedCopy() { 3116 return copy(); 3117 } 3118 3119 @Override 3120 public boolean equalsDeep(Base other_) { 3121 if (!super.equalsDeep(other_)) 3122 return false; 3123 if (!(other_ instanceof CoverageEligibilityRequest)) 3124 return false; 3125 CoverageEligibilityRequest o = (CoverageEligibilityRequest) other_; 3126 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(priority, o.priority, true) 3127 && compareDeep(purpose, o.purpose, true) && compareDeep(patient, o.patient, true) && compareDeep(event, o.event, true) 3128 && compareDeep(serviced, o.serviced, true) && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) 3129 && compareDeep(provider, o.provider, true) && compareDeep(insurer, o.insurer, true) && compareDeep(facility, o.facility, true) 3130 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(insurance, o.insurance, true) 3131 && compareDeep(item, o.item, true); 3132 } 3133 3134 @Override 3135 public boolean equalsShallow(Base other_) { 3136 if (!super.equalsShallow(other_)) 3137 return false; 3138 if (!(other_ instanceof CoverageEligibilityRequest)) 3139 return false; 3140 CoverageEligibilityRequest o = (CoverageEligibilityRequest) other_; 3141 return compareValues(status, o.status, true) && compareValues(purpose, o.purpose, true) && compareValues(created, o.created, true) 3142 ; 3143 } 3144 3145 public boolean isEmpty() { 3146 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, priority 3147 , purpose, patient, event, serviced, created, enterer, provider, insurer, facility 3148 , supportingInfo, insurance, item); 3149 } 3150 3151 @Override 3152 public ResourceType getResourceType() { 3153 return ResourceType.CoverageEligibilityRequest; 3154 } 3155 3156 /** 3157 * Search parameter: <b>created</b> 3158 * <p> 3159 * Description: <b>The creation date for the EOB</b><br> 3160 * Type: <b>date</b><br> 3161 * Path: <b>CoverageEligibilityRequest.created</b><br> 3162 * </p> 3163 */ 3164 @SearchParamDefinition(name="created", path="CoverageEligibilityRequest.created", description="The creation date for the EOB", type="date" ) 3165 public static final String SP_CREATED = "created"; 3166 /** 3167 * <b>Fluent Client</b> search parameter constant for <b>created</b> 3168 * <p> 3169 * Description: <b>The creation date for the EOB</b><br> 3170 * Type: <b>date</b><br> 3171 * Path: <b>CoverageEligibilityRequest.created</b><br> 3172 * </p> 3173 */ 3174 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 3175 3176 /** 3177 * Search parameter: <b>enterer</b> 3178 * <p> 3179 * Description: <b>The party who is responsible for the request</b><br> 3180 * Type: <b>reference</b><br> 3181 * Path: <b>CoverageEligibilityRequest.enterer</b><br> 3182 * </p> 3183 */ 3184 @SearchParamDefinition(name="enterer", path="CoverageEligibilityRequest.enterer", description="The party who is responsible for the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class, PractitionerRole.class } ) 3185 public static final String SP_ENTERER = "enterer"; 3186 /** 3187 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 3188 * <p> 3189 * Description: <b>The party who is responsible for the request</b><br> 3190 * Type: <b>reference</b><br> 3191 * Path: <b>CoverageEligibilityRequest.enterer</b><br> 3192 * </p> 3193 */ 3194 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 3195 3196/** 3197 * Constant for fluent queries to be used to add include statements. Specifies 3198 * the path value of "<b>CoverageEligibilityRequest:enterer</b>". 3199 */ 3200 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("CoverageEligibilityRequest:enterer").toLocked(); 3201 3202 /** 3203 * Search parameter: <b>facility</b> 3204 * <p> 3205 * Description: <b>Facility responsible for the goods and services</b><br> 3206 * Type: <b>reference</b><br> 3207 * Path: <b>CoverageEligibilityRequest.facility</b><br> 3208 * </p> 3209 */ 3210 @SearchParamDefinition(name="facility", path="CoverageEligibilityRequest.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class } ) 3211 public static final String SP_FACILITY = "facility"; 3212 /** 3213 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 3214 * <p> 3215 * Description: <b>Facility responsible for the goods and services</b><br> 3216 * Type: <b>reference</b><br> 3217 * Path: <b>CoverageEligibilityRequest.facility</b><br> 3218 * </p> 3219 */ 3220 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 3221 3222/** 3223 * Constant for fluent queries to be used to add include statements. Specifies 3224 * the path value of "<b>CoverageEligibilityRequest:facility</b>". 3225 */ 3226 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("CoverageEligibilityRequest:facility").toLocked(); 3227 3228 /** 3229 * Search parameter: <b>provider</b> 3230 * <p> 3231 * Description: <b>The reference to the provider</b><br> 3232 * Type: <b>reference</b><br> 3233 * Path: <b>CoverageEligibilityRequest.provider</b><br> 3234 * </p> 3235 */ 3236 @SearchParamDefinition(name="provider", path="CoverageEligibilityRequest.provider", description="The reference to the provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 3237 public static final String SP_PROVIDER = "provider"; 3238 /** 3239 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 3240 * <p> 3241 * Description: <b>The reference to the provider</b><br> 3242 * Type: <b>reference</b><br> 3243 * Path: <b>CoverageEligibilityRequest.provider</b><br> 3244 * </p> 3245 */ 3246 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 3247 3248/** 3249 * Constant for fluent queries to be used to add include statements. Specifies 3250 * the path value of "<b>CoverageEligibilityRequest:provider</b>". 3251 */ 3252 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("CoverageEligibilityRequest:provider").toLocked(); 3253 3254 /** 3255 * Search parameter: <b>status</b> 3256 * <p> 3257 * Description: <b>The status of the EligibilityRequest</b><br> 3258 * Type: <b>token</b><br> 3259 * Path: <b>CoverageEligibilityRequest.status</b><br> 3260 * </p> 3261 */ 3262 @SearchParamDefinition(name="status", path="CoverageEligibilityRequest.status", description="The status of the EligibilityRequest", type="token" ) 3263 public static final String SP_STATUS = "status"; 3264 /** 3265 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3266 * <p> 3267 * Description: <b>The status of the EligibilityRequest</b><br> 3268 * Type: <b>token</b><br> 3269 * Path: <b>CoverageEligibilityRequest.status</b><br> 3270 * </p> 3271 */ 3272 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3273 3274 /** 3275 * Search parameter: <b>identifier</b> 3276 * <p> 3277 * Description: <b>Multiple Resources: 3278 3279* [Account](account.html): Account number 3280* [AdverseEvent](adverseevent.html): Business identifier for the event 3281* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3282* [Appointment](appointment.html): An Identifier of the Appointment 3283* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3284* [Basic](basic.html): Business identifier 3285* [BodyStructure](bodystructure.html): Bodystructure identifier 3286* [CarePlan](careplan.html): External Ids for this plan 3287* [CareTeam](careteam.html): External Ids for this team 3288* [ChargeItem](chargeitem.html): Business Identifier for item 3289* [Claim](claim.html): The primary identifier of the financial resource 3290* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3291* [ClinicalImpression](clinicalimpression.html): Business identifier 3292* [Communication](communication.html): Unique identifier 3293* [CommunicationRequest](communicationrequest.html): Unique identifier 3294* [Composition](composition.html): Version-independent identifier for the Composition 3295* [Condition](condition.html): A unique identifier of the condition record 3296* [Consent](consent.html): Identifier for this record (external references) 3297* [Contract](contract.html): The identity of the contract 3298* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3299* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3300* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3301* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3302* [DeviceRequest](devicerequest.html): Business identifier for request/order 3303* [DeviceUsage](deviceusage.html): Search by identifier 3304* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3305* [DocumentReference](documentreference.html): Identifier of the attachment binary 3306* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3307* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3308* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3309* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3310* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3311* [Flag](flag.html): Business identifier 3312* [Goal](goal.html): External Ids for this goal 3313* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3314* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3315* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3316* [Immunization](immunization.html): Business identifier 3317* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3318* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3319* [Invoice](invoice.html): Business Identifier for item 3320* [List](list.html): Business identifier 3321* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3322* [Medication](medication.html): Returns medications with this external identifier 3323* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3324* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3325* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3326* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3327* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3328* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3329* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3330* [Observation](observation.html): The unique id for a particular observation 3331* [Person](person.html): A person Identifier 3332* [Procedure](procedure.html): A unique identifier for a procedure 3333* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3334* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3335* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3336* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3337* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3338* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3339* [Specimen](specimen.html): The unique identifier associated with the specimen 3340* [SupplyDelivery](supplydelivery.html): External identifier 3341* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3342* [Task](task.html): Search for a task instance by its business identifier 3343* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3344</b><br> 3345 * Type: <b>token</b><br> 3346 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3347 * </p> 3348 */ 3349 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3350 public static final String SP_IDENTIFIER = "identifier"; 3351 /** 3352 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3353 * <p> 3354 * Description: <b>Multiple Resources: 3355 3356* [Account](account.html): Account number 3357* [AdverseEvent](adverseevent.html): Business identifier for the event 3358* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3359* [Appointment](appointment.html): An Identifier of the Appointment 3360* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3361* [Basic](basic.html): Business identifier 3362* [BodyStructure](bodystructure.html): Bodystructure identifier 3363* [CarePlan](careplan.html): External Ids for this plan 3364* [CareTeam](careteam.html): External Ids for this team 3365* [ChargeItem](chargeitem.html): Business Identifier for item 3366* [Claim](claim.html): The primary identifier of the financial resource 3367* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3368* [ClinicalImpression](clinicalimpression.html): Business identifier 3369* [Communication](communication.html): Unique identifier 3370* [CommunicationRequest](communicationrequest.html): Unique identifier 3371* [Composition](composition.html): Version-independent identifier for the Composition 3372* [Condition](condition.html): A unique identifier of the condition record 3373* [Consent](consent.html): Identifier for this record (external references) 3374* [Contract](contract.html): The identity of the contract 3375* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3376* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3377* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3378* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3379* [DeviceRequest](devicerequest.html): Business identifier for request/order 3380* [DeviceUsage](deviceusage.html): Search by identifier 3381* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3382* [DocumentReference](documentreference.html): Identifier of the attachment binary 3383* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3384* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3385* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3386* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3387* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3388* [Flag](flag.html): Business identifier 3389* [Goal](goal.html): External Ids for this goal 3390* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3391* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3392* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3393* [Immunization](immunization.html): Business identifier 3394* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3395* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3396* [Invoice](invoice.html): Business Identifier for item 3397* [List](list.html): Business identifier 3398* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3399* [Medication](medication.html): Returns medications with this external identifier 3400* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3401* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3402* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3403* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3404* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3405* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3406* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3407* [Observation](observation.html): The unique id for a particular observation 3408* [Person](person.html): A person Identifier 3409* [Procedure](procedure.html): A unique identifier for a procedure 3410* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3411* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3412* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3413* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3414* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3415* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3416* [Specimen](specimen.html): The unique identifier associated with the specimen 3417* [SupplyDelivery](supplydelivery.html): External identifier 3418* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3419* [Task](task.html): Search for a task instance by its business identifier 3420* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3421</b><br> 3422 * Type: <b>token</b><br> 3423 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3424 * </p> 3425 */ 3426 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3427 3428 /** 3429 * Search parameter: <b>patient</b> 3430 * <p> 3431 * Description: <b>Multiple Resources: 3432 3433* [Account](account.html): The entity that caused the expenses 3434* [AdverseEvent](adverseevent.html): Subject impacted by event 3435* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3436* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3437* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3438* [AuditEvent](auditevent.html): Where the activity involved patient data 3439* [Basic](basic.html): Identifies the focus of this resource 3440* [BodyStructure](bodystructure.html): Who this is about 3441* [CarePlan](careplan.html): Who the care plan is for 3442* [CareTeam](careteam.html): Who care team is for 3443* [ChargeItem](chargeitem.html): Individual service was done for/to 3444* [Claim](claim.html): Patient receiving the products or services 3445* [ClaimResponse](claimresponse.html): The subject of care 3446* [ClinicalImpression](clinicalimpression.html): Patient assessed 3447* [Communication](communication.html): Focus of message 3448* [CommunicationRequest](communicationrequest.html): Focus of message 3449* [Composition](composition.html): Who and/or what the composition is about 3450* [Condition](condition.html): Who has the condition? 3451* [Consent](consent.html): Who the consent applies to 3452* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3453* [Coverage](coverage.html): Retrieve coverages for a patient 3454* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3455* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3456* [DetectedIssue](detectedissue.html): Associated patient 3457* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3458* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3459* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3460* [DocumentReference](documentreference.html): Who/what is the subject of the document 3461* [Encounter](encounter.html): The patient present at the encounter 3462* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3463* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3464* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3465* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3466* [Flag](flag.html): The identity of a subject to list flags for 3467* [Goal](goal.html): Who this goal is intended for 3468* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3469* [ImagingSelection](imagingselection.html): Who the study is about 3470* [ImagingStudy](imagingstudy.html): Who the study is about 3471* [Immunization](immunization.html): The patient for the vaccination record 3472* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3473* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3474* [Invoice](invoice.html): Recipient(s) of goods and services 3475* [List](list.html): If all resources have the same subject 3476* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3477* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3478* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3479* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3480* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3481* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3482* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3483* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3484* [Observation](observation.html): The subject that the observation is about (if patient) 3485* [Person](person.html): The Person links to this Patient 3486* [Procedure](procedure.html): Search by subject - a patient 3487* [Provenance](provenance.html): Where the activity involved patient data 3488* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3489* [RelatedPerson](relatedperson.html): The patient this related person is related to 3490* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3491* [ResearchSubject](researchsubject.html): Who or what is part of study 3492* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3493* [ServiceRequest](servicerequest.html): Search by subject - a patient 3494* [Specimen](specimen.html): The patient the specimen comes from 3495* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3496* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3497* [Task](task.html): Search by patient 3498* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3499</b><br> 3500 * Type: <b>reference</b><br> 3501 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3502 * </p> 3503 */ 3504 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3505 public static final String SP_PATIENT = "patient"; 3506 /** 3507 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3508 * <p> 3509 * Description: <b>Multiple Resources: 3510 3511* [Account](account.html): The entity that caused the expenses 3512* [AdverseEvent](adverseevent.html): Subject impacted by event 3513* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3514* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3515* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3516* [AuditEvent](auditevent.html): Where the activity involved patient data 3517* [Basic](basic.html): Identifies the focus of this resource 3518* [BodyStructure](bodystructure.html): Who this is about 3519* [CarePlan](careplan.html): Who the care plan is for 3520* [CareTeam](careteam.html): Who care team is for 3521* [ChargeItem](chargeitem.html): Individual service was done for/to 3522* [Claim](claim.html): Patient receiving the products or services 3523* [ClaimResponse](claimresponse.html): The subject of care 3524* [ClinicalImpression](clinicalimpression.html): Patient assessed 3525* [Communication](communication.html): Focus of message 3526* [CommunicationRequest](communicationrequest.html): Focus of message 3527* [Composition](composition.html): Who and/or what the composition is about 3528* [Condition](condition.html): Who has the condition? 3529* [Consent](consent.html): Who the consent applies to 3530* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3531* [Coverage](coverage.html): Retrieve coverages for a patient 3532* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3533* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3534* [DetectedIssue](detectedissue.html): Associated patient 3535* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3536* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3537* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3538* [DocumentReference](documentreference.html): Who/what is the subject of the document 3539* [Encounter](encounter.html): The patient present at the encounter 3540* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3541* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3542* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3543* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3544* [Flag](flag.html): The identity of a subject to list flags for 3545* [Goal](goal.html): Who this goal is intended for 3546* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3547* [ImagingSelection](imagingselection.html): Who the study is about 3548* [ImagingStudy](imagingstudy.html): Who the study is about 3549* [Immunization](immunization.html): The patient for the vaccination record 3550* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3551* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3552* [Invoice](invoice.html): Recipient(s) of goods and services 3553* [List](list.html): If all resources have the same subject 3554* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3555* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3556* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3557* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3558* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3559* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3560* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3561* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3562* [Observation](observation.html): The subject that the observation is about (if patient) 3563* [Person](person.html): The Person links to this Patient 3564* [Procedure](procedure.html): Search by subject - a patient 3565* [Provenance](provenance.html): Where the activity involved patient data 3566* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3567* [RelatedPerson](relatedperson.html): The patient this related person is related to 3568* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3569* [ResearchSubject](researchsubject.html): Who or what is part of study 3570* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3571* [ServiceRequest](servicerequest.html): Search by subject - a patient 3572* [Specimen](specimen.html): The patient the specimen comes from 3573* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3574* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3575* [Task](task.html): Search by patient 3576* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3577</b><br> 3578 * Type: <b>reference</b><br> 3579 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3580 * </p> 3581 */ 3582 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3583 3584/** 3585 * Constant for fluent queries to be used to add include statements. Specifies 3586 * the path value of "<b>CoverageEligibilityRequest:patient</b>". 3587 */ 3588 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CoverageEligibilityRequest:patient").toLocked(); 3589 3590 3591} 3592