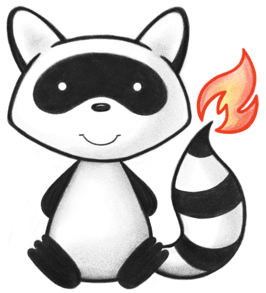
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource. 052 */ 053@ResourceDef(name="CoverageEligibilityResponse", profile="http://hl7.org/fhir/StructureDefinition/CoverageEligibilityResponse") 054public class CoverageEligibilityResponse extends DomainResource { 055 056 public enum EligibilityOutcome { 057 /** 058 * The Claim/Pre-authorization/Pre-determination has been received but processing has not begun. 059 */ 060 QUEUED, 061 /** 062 * The processing has completed without errors 063 */ 064 COMPLETE, 065 /** 066 * One or more errors have been detected in the Claim 067 */ 068 ERROR, 069 /** 070 * No errors have been detected in the Claim and some of the adjudication has been performed. 071 */ 072 PARTIAL, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static EligibilityOutcome fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("queued".equals(codeString)) 081 return QUEUED; 082 if ("complete".equals(codeString)) 083 return COMPLETE; 084 if ("error".equals(codeString)) 085 return ERROR; 086 if ("partial".equals(codeString)) 087 return PARTIAL; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown EligibilityOutcome code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case QUEUED: return "queued"; 096 case COMPLETE: return "complete"; 097 case ERROR: return "error"; 098 case PARTIAL: return "partial"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case QUEUED: return "http://hl7.org/fhir/eligibility-outcome"; 106 case COMPLETE: return "http://hl7.org/fhir/eligibility-outcome"; 107 case ERROR: return "http://hl7.org/fhir/eligibility-outcome"; 108 case PARTIAL: return "http://hl7.org/fhir/eligibility-outcome"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case QUEUED: return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 116 case COMPLETE: return "The processing has completed without errors"; 117 case ERROR: return "One or more errors have been detected in the Claim"; 118 case PARTIAL: return "No errors have been detected in the Claim and some of the adjudication has been performed."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case QUEUED: return "Queued"; 126 case COMPLETE: return "Processing Complete"; 127 case ERROR: return "Error"; 128 case PARTIAL: return "Partial Processing"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class EligibilityOutcomeEnumFactory implements EnumFactory<EligibilityOutcome> { 136 public EligibilityOutcome fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("queued".equals(codeString)) 141 return EligibilityOutcome.QUEUED; 142 if ("complete".equals(codeString)) 143 return EligibilityOutcome.COMPLETE; 144 if ("error".equals(codeString)) 145 return EligibilityOutcome.ERROR; 146 if ("partial".equals(codeString)) 147 return EligibilityOutcome.PARTIAL; 148 throw new IllegalArgumentException("Unknown EligibilityOutcome code '"+codeString+"'"); 149 } 150 public Enumeration<EligibilityOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<EligibilityOutcome>(this, EligibilityOutcome.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<EligibilityOutcome>(this, EligibilityOutcome.NULL, code); 158 if ("queued".equals(codeString)) 159 return new Enumeration<EligibilityOutcome>(this, EligibilityOutcome.QUEUED, code); 160 if ("complete".equals(codeString)) 161 return new Enumeration<EligibilityOutcome>(this, EligibilityOutcome.COMPLETE, code); 162 if ("error".equals(codeString)) 163 return new Enumeration<EligibilityOutcome>(this, EligibilityOutcome.ERROR, code); 164 if ("partial".equals(codeString)) 165 return new Enumeration<EligibilityOutcome>(this, EligibilityOutcome.PARTIAL, code); 166 throw new FHIRException("Unknown EligibilityOutcome code '"+codeString+"'"); 167 } 168 public String toCode(EligibilityOutcome code) { 169 if (code == EligibilityOutcome.NULL) 170 return null; 171 if (code == EligibilityOutcome.QUEUED) 172 return "queued"; 173 if (code == EligibilityOutcome.COMPLETE) 174 return "complete"; 175 if (code == EligibilityOutcome.ERROR) 176 return "error"; 177 if (code == EligibilityOutcome.PARTIAL) 178 return "partial"; 179 return "?"; 180 } 181 public String toSystem(EligibilityOutcome code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum EligibilityResponsePurpose { 187 /** 188 * The prior authorization requirements for the listed, or discovered if specified, converages for the categories of service and/or specifed biling codes are requested. 189 */ 190 AUTHREQUIREMENTS, 191 /** 192 * The plan benefits and optionally benefits consumed for the listed, or discovered if specified, converages are requested. 193 */ 194 BENEFITS, 195 /** 196 * The insurer is requested to report on any coverages which they are aware of in addition to any specifed. 197 */ 198 DISCOVERY, 199 /** 200 * A check that the specified coverages are in-force is requested. 201 */ 202 VALIDATION, 203 /** 204 * added to help the parsers with the generic types 205 */ 206 NULL; 207 public static EligibilityResponsePurpose fromCode(String codeString) throws FHIRException { 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("auth-requirements".equals(codeString)) 211 return AUTHREQUIREMENTS; 212 if ("benefits".equals(codeString)) 213 return BENEFITS; 214 if ("discovery".equals(codeString)) 215 return DISCOVERY; 216 if ("validation".equals(codeString)) 217 return VALIDATION; 218 if (Configuration.isAcceptInvalidEnums()) 219 return null; 220 else 221 throw new FHIRException("Unknown EligibilityResponsePurpose code '"+codeString+"'"); 222 } 223 public String toCode() { 224 switch (this) { 225 case AUTHREQUIREMENTS: return "auth-requirements"; 226 case BENEFITS: return "benefits"; 227 case DISCOVERY: return "discovery"; 228 case VALIDATION: return "validation"; 229 case NULL: return null; 230 default: return "?"; 231 } 232 } 233 public String getSystem() { 234 switch (this) { 235 case AUTHREQUIREMENTS: return "http://hl7.org/fhir/eligibilityresponse-purpose"; 236 case BENEFITS: return "http://hl7.org/fhir/eligibilityresponse-purpose"; 237 case DISCOVERY: return "http://hl7.org/fhir/eligibilityresponse-purpose"; 238 case VALIDATION: return "http://hl7.org/fhir/eligibilityresponse-purpose"; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 public String getDefinition() { 244 switch (this) { 245 case AUTHREQUIREMENTS: return "The prior authorization requirements for the listed, or discovered if specified, converages for the categories of service and/or specifed biling codes are requested."; 246 case BENEFITS: return "The plan benefits and optionally benefits consumed for the listed, or discovered if specified, converages are requested."; 247 case DISCOVERY: return "The insurer is requested to report on any coverages which they are aware of in addition to any specifed."; 248 case VALIDATION: return "A check that the specified coverages are in-force is requested."; 249 case NULL: return null; 250 default: return "?"; 251 } 252 } 253 public String getDisplay() { 254 switch (this) { 255 case AUTHREQUIREMENTS: return "Coverage auth-requirements"; 256 case BENEFITS: return "Coverage benefits"; 257 case DISCOVERY: return "Coverage Discovery"; 258 case VALIDATION: return "Coverage Validation"; 259 case NULL: return null; 260 default: return "?"; 261 } 262 } 263 } 264 265 public static class EligibilityResponsePurposeEnumFactory implements EnumFactory<EligibilityResponsePurpose> { 266 public EligibilityResponsePurpose fromCode(String codeString) throws IllegalArgumentException { 267 if (codeString == null || "".equals(codeString)) 268 if (codeString == null || "".equals(codeString)) 269 return null; 270 if ("auth-requirements".equals(codeString)) 271 return EligibilityResponsePurpose.AUTHREQUIREMENTS; 272 if ("benefits".equals(codeString)) 273 return EligibilityResponsePurpose.BENEFITS; 274 if ("discovery".equals(codeString)) 275 return EligibilityResponsePurpose.DISCOVERY; 276 if ("validation".equals(codeString)) 277 return EligibilityResponsePurpose.VALIDATION; 278 throw new IllegalArgumentException("Unknown EligibilityResponsePurpose code '"+codeString+"'"); 279 } 280 public Enumeration<EligibilityResponsePurpose> fromType(PrimitiveType<?> code) throws FHIRException { 281 if (code == null) 282 return null; 283 if (code.isEmpty()) 284 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.NULL, code); 285 String codeString = ((PrimitiveType) code).asStringValue(); 286 if (codeString == null || "".equals(codeString)) 287 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.NULL, code); 288 if ("auth-requirements".equals(codeString)) 289 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.AUTHREQUIREMENTS, code); 290 if ("benefits".equals(codeString)) 291 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.BENEFITS, code); 292 if ("discovery".equals(codeString)) 293 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.DISCOVERY, code); 294 if ("validation".equals(codeString)) 295 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.VALIDATION, code); 296 throw new FHIRException("Unknown EligibilityResponsePurpose code '"+codeString+"'"); 297 } 298 public String toCode(EligibilityResponsePurpose code) { 299 if (code == EligibilityResponsePurpose.NULL) 300 return null; 301 if (code == EligibilityResponsePurpose.AUTHREQUIREMENTS) 302 return "auth-requirements"; 303 if (code == EligibilityResponsePurpose.BENEFITS) 304 return "benefits"; 305 if (code == EligibilityResponsePurpose.DISCOVERY) 306 return "discovery"; 307 if (code == EligibilityResponsePurpose.VALIDATION) 308 return "validation"; 309 return "?"; 310 } 311 public String toSystem(EligibilityResponsePurpose code) { 312 return code.getSystem(); 313 } 314 } 315 316 @Block() 317 public static class CoverageEligibilityResponseEventComponent extends BackboneElement implements IBaseBackboneElement { 318 /** 319 * A coded event such as when a service is expected or a card printed. 320 */ 321 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 322 @Description(shortDefinition="Specific event", formalDefinition="A coded event such as when a service is expected or a card printed." ) 323 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/datestype") 324 protected CodeableConcept type; 325 326 /** 327 * A date or period in the past or future indicating when the event occurred or is expectd to occur. 328 */ 329 @Child(name = "when", type = {DateTimeType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 330 @Description(shortDefinition="Occurance date or period", formalDefinition="A date or period in the past or future indicating when the event occurred or is expectd to occur." ) 331 protected DataType when; 332 333 private static final long serialVersionUID = -634897375L; 334 335 /** 336 * Constructor 337 */ 338 public CoverageEligibilityResponseEventComponent() { 339 super(); 340 } 341 342 /** 343 * Constructor 344 */ 345 public CoverageEligibilityResponseEventComponent(CodeableConcept type, DataType when) { 346 super(); 347 this.setType(type); 348 this.setWhen(when); 349 } 350 351 /** 352 * @return {@link #type} (A coded event such as when a service is expected or a card printed.) 353 */ 354 public CodeableConcept getType() { 355 if (this.type == null) 356 if (Configuration.errorOnAutoCreate()) 357 throw new Error("Attempt to auto-create CoverageEligibilityResponseEventComponent.type"); 358 else if (Configuration.doAutoCreate()) 359 this.type = new CodeableConcept(); // cc 360 return this.type; 361 } 362 363 public boolean hasType() { 364 return this.type != null && !this.type.isEmpty(); 365 } 366 367 /** 368 * @param value {@link #type} (A coded event such as when a service is expected or a card printed.) 369 */ 370 public CoverageEligibilityResponseEventComponent setType(CodeableConcept value) { 371 this.type = value; 372 return this; 373 } 374 375 /** 376 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 377 */ 378 public DataType getWhen() { 379 return this.when; 380 } 381 382 /** 383 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 384 */ 385 public DateTimeType getWhenDateTimeType() throws FHIRException { 386 if (this.when == null) 387 this.when = new DateTimeType(); 388 if (!(this.when instanceof DateTimeType)) 389 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.when.getClass().getName()+" was encountered"); 390 return (DateTimeType) this.when; 391 } 392 393 public boolean hasWhenDateTimeType() { 394 return this.when instanceof DateTimeType; 395 } 396 397 /** 398 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 399 */ 400 public Period getWhenPeriod() throws FHIRException { 401 if (this.when == null) 402 this.when = new Period(); 403 if (!(this.when instanceof Period)) 404 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 405 return (Period) this.when; 406 } 407 408 public boolean hasWhenPeriod() { 409 return this.when instanceof Period; 410 } 411 412 public boolean hasWhen() { 413 return this.when != null && !this.when.isEmpty(); 414 } 415 416 /** 417 * @param value {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 418 */ 419 public CoverageEligibilityResponseEventComponent setWhen(DataType value) { 420 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 421 throw new FHIRException("Not the right type for CoverageEligibilityResponse.event.when[x]: "+value.fhirType()); 422 this.when = value; 423 return this; 424 } 425 426 protected void listChildren(List<Property> children) { 427 super.listChildren(children); 428 children.add(new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type)); 429 children.add(new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when)); 430 } 431 432 @Override 433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 434 switch (_hash) { 435 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type); 436 case 1312831238: /*when[x]*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 437 case 3648314: /*when*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 438 case -1785502475: /*whenDateTime*/ return new Property("when[x]", "dateTime", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 439 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 440 default: return super.getNamedProperty(_hash, _name, _checkValid); 441 } 442 443 } 444 445 @Override 446 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 447 switch (hash) { 448 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 449 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 450 default: return super.getProperty(hash, name, checkValid); 451 } 452 453 } 454 455 @Override 456 public Base setProperty(int hash, String name, Base value) throws FHIRException { 457 switch (hash) { 458 case 3575610: // type 459 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 460 return value; 461 case 3648314: // when 462 this.when = TypeConvertor.castToType(value); // DataType 463 return value; 464 default: return super.setProperty(hash, name, value); 465 } 466 467 } 468 469 @Override 470 public Base setProperty(String name, Base value) throws FHIRException { 471 if (name.equals("type")) { 472 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 473 } else if (name.equals("when[x]")) { 474 this.when = TypeConvertor.castToType(value); // DataType 475 } else 476 return super.setProperty(name, value); 477 return value; 478 } 479 480 @Override 481 public void removeChild(String name, Base value) throws FHIRException { 482 if (name.equals("type")) { 483 this.type = null; 484 } else if (name.equals("when[x]")) { 485 this.when = null; 486 } else 487 super.removeChild(name, value); 488 489 } 490 491 @Override 492 public Base makeProperty(int hash, String name) throws FHIRException { 493 switch (hash) { 494 case 3575610: return getType(); 495 case 1312831238: return getWhen(); 496 case 3648314: return getWhen(); 497 default: return super.makeProperty(hash, name); 498 } 499 500 } 501 502 @Override 503 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 504 switch (hash) { 505 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 506 case 3648314: /*when*/ return new String[] {"dateTime", "Period"}; 507 default: return super.getTypesForProperty(hash, name); 508 } 509 510 } 511 512 @Override 513 public Base addChild(String name) throws FHIRException { 514 if (name.equals("type")) { 515 this.type = new CodeableConcept(); 516 return this.type; 517 } 518 else if (name.equals("whenDateTime")) { 519 this.when = new DateTimeType(); 520 return this.when; 521 } 522 else if (name.equals("whenPeriod")) { 523 this.when = new Period(); 524 return this.when; 525 } 526 else 527 return super.addChild(name); 528 } 529 530 public CoverageEligibilityResponseEventComponent copy() { 531 CoverageEligibilityResponseEventComponent dst = new CoverageEligibilityResponseEventComponent(); 532 copyValues(dst); 533 return dst; 534 } 535 536 public void copyValues(CoverageEligibilityResponseEventComponent dst) { 537 super.copyValues(dst); 538 dst.type = type == null ? null : type.copy(); 539 dst.when = when == null ? null : when.copy(); 540 } 541 542 @Override 543 public boolean equalsDeep(Base other_) { 544 if (!super.equalsDeep(other_)) 545 return false; 546 if (!(other_ instanceof CoverageEligibilityResponseEventComponent)) 547 return false; 548 CoverageEligibilityResponseEventComponent o = (CoverageEligibilityResponseEventComponent) other_; 549 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true); 550 } 551 552 @Override 553 public boolean equalsShallow(Base other_) { 554 if (!super.equalsShallow(other_)) 555 return false; 556 if (!(other_ instanceof CoverageEligibilityResponseEventComponent)) 557 return false; 558 CoverageEligibilityResponseEventComponent o = (CoverageEligibilityResponseEventComponent) other_; 559 return true; 560 } 561 562 public boolean isEmpty() { 563 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when); 564 } 565 566 public String fhirType() { 567 return "CoverageEligibilityResponse.event"; 568 569 } 570 571 } 572 573 @Block() 574 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 575 /** 576 * Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system. 577 */ 578 @Child(name = "coverage", type = {Coverage.class}, order=1, min=1, max=1, modifier=false, summary=true) 579 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system." ) 580 protected Reference coverage; 581 582 /** 583 * Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates. 584 */ 585 @Child(name = "inforce", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 586 @Description(shortDefinition="Coverage inforce indicator", formalDefinition="Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates." ) 587 protected BooleanType inforce; 588 589 /** 590 * The term of the benefits documented in this response. 591 */ 592 @Child(name = "benefitPeriod", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 593 @Description(shortDefinition="When the benefits are applicable", formalDefinition="The term of the benefits documented in this response." ) 594 protected Period benefitPeriod; 595 596 /** 597 * Benefits and optionally current balances, and authorization details by category or service. 598 */ 599 @Child(name = "item", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 600 @Description(shortDefinition="Benefits and authorization details", formalDefinition="Benefits and optionally current balances, and authorization details by category or service." ) 601 protected List<ItemsComponent> item; 602 603 private static final long serialVersionUID = 1473928476L; 604 605 /** 606 * Constructor 607 */ 608 public InsuranceComponent() { 609 super(); 610 } 611 612 /** 613 * Constructor 614 */ 615 public InsuranceComponent(Reference coverage) { 616 super(); 617 this.setCoverage(coverage); 618 } 619 620 /** 621 * @return {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 622 */ 623 public Reference getCoverage() { 624 if (this.coverage == null) 625 if (Configuration.errorOnAutoCreate()) 626 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 627 else if (Configuration.doAutoCreate()) 628 this.coverage = new Reference(); // cc 629 return this.coverage; 630 } 631 632 public boolean hasCoverage() { 633 return this.coverage != null && !this.coverage.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 638 */ 639 public InsuranceComponent setCoverage(Reference value) { 640 this.coverage = value; 641 return this; 642 } 643 644 /** 645 * @return {@link #inforce} (Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.). This is the underlying object with id, value and extensions. The accessor "getInforce" gives direct access to the value 646 */ 647 public BooleanType getInforceElement() { 648 if (this.inforce == null) 649 if (Configuration.errorOnAutoCreate()) 650 throw new Error("Attempt to auto-create InsuranceComponent.inforce"); 651 else if (Configuration.doAutoCreate()) 652 this.inforce = new BooleanType(); // bb 653 return this.inforce; 654 } 655 656 public boolean hasInforceElement() { 657 return this.inforce != null && !this.inforce.isEmpty(); 658 } 659 660 public boolean hasInforce() { 661 return this.inforce != null && !this.inforce.isEmpty(); 662 } 663 664 /** 665 * @param value {@link #inforce} (Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.). This is the underlying object with id, value and extensions. The accessor "getInforce" gives direct access to the value 666 */ 667 public InsuranceComponent setInforceElement(BooleanType value) { 668 this.inforce = value; 669 return this; 670 } 671 672 /** 673 * @return Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates. 674 */ 675 public boolean getInforce() { 676 return this.inforce == null || this.inforce.isEmpty() ? false : this.inforce.getValue(); 677 } 678 679 /** 680 * @param value Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates. 681 */ 682 public InsuranceComponent setInforce(boolean value) { 683 if (this.inforce == null) 684 this.inforce = new BooleanType(); 685 this.inforce.setValue(value); 686 return this; 687 } 688 689 /** 690 * @return {@link #benefitPeriod} (The term of the benefits documented in this response.) 691 */ 692 public Period getBenefitPeriod() { 693 if (this.benefitPeriod == null) 694 if (Configuration.errorOnAutoCreate()) 695 throw new Error("Attempt to auto-create InsuranceComponent.benefitPeriod"); 696 else if (Configuration.doAutoCreate()) 697 this.benefitPeriod = new Period(); // cc 698 return this.benefitPeriod; 699 } 700 701 public boolean hasBenefitPeriod() { 702 return this.benefitPeriod != null && !this.benefitPeriod.isEmpty(); 703 } 704 705 /** 706 * @param value {@link #benefitPeriod} (The term of the benefits documented in this response.) 707 */ 708 public InsuranceComponent setBenefitPeriod(Period value) { 709 this.benefitPeriod = value; 710 return this; 711 } 712 713 /** 714 * @return {@link #item} (Benefits and optionally current balances, and authorization details by category or service.) 715 */ 716 public List<ItemsComponent> getItem() { 717 if (this.item == null) 718 this.item = new ArrayList<ItemsComponent>(); 719 return this.item; 720 } 721 722 /** 723 * @return Returns a reference to <code>this</code> for easy method chaining 724 */ 725 public InsuranceComponent setItem(List<ItemsComponent> theItem) { 726 this.item = theItem; 727 return this; 728 } 729 730 public boolean hasItem() { 731 if (this.item == null) 732 return false; 733 for (ItemsComponent item : this.item) 734 if (!item.isEmpty()) 735 return true; 736 return false; 737 } 738 739 public ItemsComponent addItem() { //3 740 ItemsComponent t = new ItemsComponent(); 741 if (this.item == null) 742 this.item = new ArrayList<ItemsComponent>(); 743 this.item.add(t); 744 return t; 745 } 746 747 public InsuranceComponent addItem(ItemsComponent t) { //3 748 if (t == null) 749 return this; 750 if (this.item == null) 751 this.item = new ArrayList<ItemsComponent>(); 752 this.item.add(t); 753 return this; 754 } 755 756 /** 757 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 758 */ 759 public ItemsComponent getItemFirstRep() { 760 if (getItem().isEmpty()) { 761 addItem(); 762 } 763 return getItem().get(0); 764 } 765 766 protected void listChildren(List<Property> children) { 767 super.listChildren(children); 768 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage)); 769 children.add(new Property("inforce", "boolean", "Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.", 0, 1, inforce)); 770 children.add(new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 1, benefitPeriod)); 771 children.add(new Property("item", "", "Benefits and optionally current balances, and authorization details by category or service.", 0, java.lang.Integer.MAX_VALUE, item)); 772 } 773 774 @Override 775 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 776 switch (_hash) { 777 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage); 778 case 1945431270: /*inforce*/ return new Property("inforce", "boolean", "Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.", 0, 1, inforce); 779 case -407369416: /*benefitPeriod*/ return new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 1, benefitPeriod); 780 case 3242771: /*item*/ return new Property("item", "", "Benefits and optionally current balances, and authorization details by category or service.", 0, java.lang.Integer.MAX_VALUE, item); 781 default: return super.getNamedProperty(_hash, _name, _checkValid); 782 } 783 784 } 785 786 @Override 787 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 788 switch (hash) { 789 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 790 case 1945431270: /*inforce*/ return this.inforce == null ? new Base[0] : new Base[] {this.inforce}; // BooleanType 791 case -407369416: /*benefitPeriod*/ return this.benefitPeriod == null ? new Base[0] : new Base[] {this.benefitPeriod}; // Period 792 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemsComponent 793 default: return super.getProperty(hash, name, checkValid); 794 } 795 796 } 797 798 @Override 799 public Base setProperty(int hash, String name, Base value) throws FHIRException { 800 switch (hash) { 801 case -351767064: // coverage 802 this.coverage = TypeConvertor.castToReference(value); // Reference 803 return value; 804 case 1945431270: // inforce 805 this.inforce = TypeConvertor.castToBoolean(value); // BooleanType 806 return value; 807 case -407369416: // benefitPeriod 808 this.benefitPeriod = TypeConvertor.castToPeriod(value); // Period 809 return value; 810 case 3242771: // item 811 this.getItem().add((ItemsComponent) value); // ItemsComponent 812 return value; 813 default: return super.setProperty(hash, name, value); 814 } 815 816 } 817 818 @Override 819 public Base setProperty(String name, Base value) throws FHIRException { 820 if (name.equals("coverage")) { 821 this.coverage = TypeConvertor.castToReference(value); // Reference 822 } else if (name.equals("inforce")) { 823 this.inforce = TypeConvertor.castToBoolean(value); // BooleanType 824 } else if (name.equals("benefitPeriod")) { 825 this.benefitPeriod = TypeConvertor.castToPeriod(value); // Period 826 } else if (name.equals("item")) { 827 this.getItem().add((ItemsComponent) value); 828 } else 829 return super.setProperty(name, value); 830 return value; 831 } 832 833 @Override 834 public void removeChild(String name, Base value) throws FHIRException { 835 if (name.equals("coverage")) { 836 this.coverage = null; 837 } else if (name.equals("inforce")) { 838 this.inforce = null; 839 } else if (name.equals("benefitPeriod")) { 840 this.benefitPeriod = null; 841 } else if (name.equals("item")) { 842 this.getItem().remove((ItemsComponent) value); 843 } else 844 super.removeChild(name, value); 845 846 } 847 848 @Override 849 public Base makeProperty(int hash, String name) throws FHIRException { 850 switch (hash) { 851 case -351767064: return getCoverage(); 852 case 1945431270: return getInforceElement(); 853 case -407369416: return getBenefitPeriod(); 854 case 3242771: return addItem(); 855 default: return super.makeProperty(hash, name); 856 } 857 858 } 859 860 @Override 861 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 862 switch (hash) { 863 case -351767064: /*coverage*/ return new String[] {"Reference"}; 864 case 1945431270: /*inforce*/ return new String[] {"boolean"}; 865 case -407369416: /*benefitPeriod*/ return new String[] {"Period"}; 866 case 3242771: /*item*/ return new String[] {}; 867 default: return super.getTypesForProperty(hash, name); 868 } 869 870 } 871 872 @Override 873 public Base addChild(String name) throws FHIRException { 874 if (name.equals("coverage")) { 875 this.coverage = new Reference(); 876 return this.coverage; 877 } 878 else if (name.equals("inforce")) { 879 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.insurance.inforce"); 880 } 881 else if (name.equals("benefitPeriod")) { 882 this.benefitPeriod = new Period(); 883 return this.benefitPeriod; 884 } 885 else if (name.equals("item")) { 886 return addItem(); 887 } 888 else 889 return super.addChild(name); 890 } 891 892 public InsuranceComponent copy() { 893 InsuranceComponent dst = new InsuranceComponent(); 894 copyValues(dst); 895 return dst; 896 } 897 898 public void copyValues(InsuranceComponent dst) { 899 super.copyValues(dst); 900 dst.coverage = coverage == null ? null : coverage.copy(); 901 dst.inforce = inforce == null ? null : inforce.copy(); 902 dst.benefitPeriod = benefitPeriod == null ? null : benefitPeriod.copy(); 903 if (item != null) { 904 dst.item = new ArrayList<ItemsComponent>(); 905 for (ItemsComponent i : item) 906 dst.item.add(i.copy()); 907 }; 908 } 909 910 @Override 911 public boolean equalsDeep(Base other_) { 912 if (!super.equalsDeep(other_)) 913 return false; 914 if (!(other_ instanceof InsuranceComponent)) 915 return false; 916 InsuranceComponent o = (InsuranceComponent) other_; 917 return compareDeep(coverage, o.coverage, true) && compareDeep(inforce, o.inforce, true) && compareDeep(benefitPeriod, o.benefitPeriod, true) 918 && compareDeep(item, o.item, true); 919 } 920 921 @Override 922 public boolean equalsShallow(Base other_) { 923 if (!super.equalsShallow(other_)) 924 return false; 925 if (!(other_ instanceof InsuranceComponent)) 926 return false; 927 InsuranceComponent o = (InsuranceComponent) other_; 928 return compareValues(inforce, o.inforce, true); 929 } 930 931 public boolean isEmpty() { 932 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, inforce, benefitPeriod 933 , item); 934 } 935 936 public String fhirType() { 937 return "CoverageEligibilityResponse.insurance"; 938 939 } 940 941 } 942 943 @Block() 944 public static class ItemsComponent extends BackboneElement implements IBaseBackboneElement { 945 /** 946 * Code to identify the general type of benefits under which products and services are provided. 947 */ 948 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 949 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 950 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 951 protected CodeableConcept category; 952 953 /** 954 * This contains the product, service, drug or other billing code for the item. 955 */ 956 @Child(name = "productOrService", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 957 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="This contains the product, service, drug or other billing code for the item." ) 958 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 959 protected CodeableConcept productOrService; 960 961 /** 962 * Item typification or modifiers codes to convey additional context for the product or service. 963 */ 964 @Child(name = "modifier", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 965 @Description(shortDefinition="Product or service billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 967 protected List<CodeableConcept> modifier; 968 969 /** 970 * The practitioner who is eligible for the provision of the product or service. 971 */ 972 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class}, order=4, min=0, max=1, modifier=false, summary=false) 973 @Description(shortDefinition="Performing practitioner", formalDefinition="The practitioner who is eligible for the provision of the product or service." ) 974 protected Reference provider; 975 976 /** 977 * True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 978 */ 979 @Child(name = "excluded", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=false) 980 @Description(shortDefinition="Excluded from the plan", formalDefinition="True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage." ) 981 protected BooleanType excluded; 982 983 /** 984 * A short name or tag for the benefit. 985 */ 986 @Child(name = "name", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 987 @Description(shortDefinition="Short name for the benefit", formalDefinition="A short name or tag for the benefit." ) 988 protected StringType name; 989 990 /** 991 * A richer description of the benefit or services covered. 992 */ 993 @Child(name = "description", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 994 @Description(shortDefinition="Description of the benefit or services covered", formalDefinition="A richer description of the benefit or services covered." ) 995 protected StringType description; 996 997 /** 998 * Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers. 999 */ 1000 @Child(name = "network", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 1001 @Description(shortDefinition="In or out of network", formalDefinition="Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers." ) 1002 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-network") 1003 protected CodeableConcept network; 1004 1005 /** 1006 * Indicates if the benefits apply to an individual or to the family. 1007 */ 1008 @Child(name = "unit", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 1009 @Description(shortDefinition="Individual or family", formalDefinition="Indicates if the benefits apply to an individual or to the family." ) 1010 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-unit") 1011 protected CodeableConcept unit; 1012 1013 /** 1014 * The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'. 1015 */ 1016 @Child(name = "term", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 1017 @Description(shortDefinition="Annual or lifetime", formalDefinition="The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'." ) 1018 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-term") 1019 protected CodeableConcept term; 1020 1021 /** 1022 * Benefits used to date. 1023 */ 1024 @Child(name = "benefit", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1025 @Description(shortDefinition="Benefit Summary", formalDefinition="Benefits used to date." ) 1026 protected List<BenefitComponent> benefit; 1027 1028 /** 1029 * A boolean flag indicating whether a preauthorization is required prior to actual service delivery. 1030 */ 1031 @Child(name = "authorizationRequired", type = {BooleanType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1032 @Description(shortDefinition="Authorization required flag", formalDefinition="A boolean flag indicating whether a preauthorization is required prior to actual service delivery." ) 1033 protected BooleanType authorizationRequired; 1034 1035 /** 1036 * Codes or comments regarding information or actions associated with the preauthorization. 1037 */ 1038 @Child(name = "authorizationSupporting", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1039 @Description(shortDefinition="Type of required supporting materials", formalDefinition="Codes or comments regarding information or actions associated with the preauthorization." ) 1040 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/coverageeligibilityresponse-ex-auth-support") 1041 protected List<CodeableConcept> authorizationSupporting; 1042 1043 /** 1044 * A web location for obtaining requirements or descriptive information regarding the preauthorization. 1045 */ 1046 @Child(name = "authorizationUrl", type = {UriType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1047 @Description(shortDefinition="Preauthorization requirements endpoint", formalDefinition="A web location for obtaining requirements or descriptive information regarding the preauthorization." ) 1048 protected UriType authorizationUrl; 1049 1050 private static final long serialVersionUID = 1706159945L; 1051 1052 /** 1053 * Constructor 1054 */ 1055 public ItemsComponent() { 1056 super(); 1057 } 1058 1059 /** 1060 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 1061 */ 1062 public CodeableConcept getCategory() { 1063 if (this.category == null) 1064 if (Configuration.errorOnAutoCreate()) 1065 throw new Error("Attempt to auto-create ItemsComponent.category"); 1066 else if (Configuration.doAutoCreate()) 1067 this.category = new CodeableConcept(); // cc 1068 return this.category; 1069 } 1070 1071 public boolean hasCategory() { 1072 return this.category != null && !this.category.isEmpty(); 1073 } 1074 1075 /** 1076 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 1077 */ 1078 public ItemsComponent setCategory(CodeableConcept value) { 1079 this.category = value; 1080 return this; 1081 } 1082 1083 /** 1084 * @return {@link #productOrService} (This contains the product, service, drug or other billing code for the item.) 1085 */ 1086 public CodeableConcept getProductOrService() { 1087 if (this.productOrService == null) 1088 if (Configuration.errorOnAutoCreate()) 1089 throw new Error("Attempt to auto-create ItemsComponent.productOrService"); 1090 else if (Configuration.doAutoCreate()) 1091 this.productOrService = new CodeableConcept(); // cc 1092 return this.productOrService; 1093 } 1094 1095 public boolean hasProductOrService() { 1096 return this.productOrService != null && !this.productOrService.isEmpty(); 1097 } 1098 1099 /** 1100 * @param value {@link #productOrService} (This contains the product, service, drug or other billing code for the item.) 1101 */ 1102 public ItemsComponent setProductOrService(CodeableConcept value) { 1103 this.productOrService = value; 1104 return this; 1105 } 1106 1107 /** 1108 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 1109 */ 1110 public List<CodeableConcept> getModifier() { 1111 if (this.modifier == null) 1112 this.modifier = new ArrayList<CodeableConcept>(); 1113 return this.modifier; 1114 } 1115 1116 /** 1117 * @return Returns a reference to <code>this</code> for easy method chaining 1118 */ 1119 public ItemsComponent setModifier(List<CodeableConcept> theModifier) { 1120 this.modifier = theModifier; 1121 return this; 1122 } 1123 1124 public boolean hasModifier() { 1125 if (this.modifier == null) 1126 return false; 1127 for (CodeableConcept item : this.modifier) 1128 if (!item.isEmpty()) 1129 return true; 1130 return false; 1131 } 1132 1133 public CodeableConcept addModifier() { //3 1134 CodeableConcept t = new CodeableConcept(); 1135 if (this.modifier == null) 1136 this.modifier = new ArrayList<CodeableConcept>(); 1137 this.modifier.add(t); 1138 return t; 1139 } 1140 1141 public ItemsComponent addModifier(CodeableConcept t) { //3 1142 if (t == null) 1143 return this; 1144 if (this.modifier == null) 1145 this.modifier = new ArrayList<CodeableConcept>(); 1146 this.modifier.add(t); 1147 return this; 1148 } 1149 1150 /** 1151 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 1152 */ 1153 public CodeableConcept getModifierFirstRep() { 1154 if (getModifier().isEmpty()) { 1155 addModifier(); 1156 } 1157 return getModifier().get(0); 1158 } 1159 1160 /** 1161 * @return {@link #provider} (The practitioner who is eligible for the provision of the product or service.) 1162 */ 1163 public Reference getProvider() { 1164 if (this.provider == null) 1165 if (Configuration.errorOnAutoCreate()) 1166 throw new Error("Attempt to auto-create ItemsComponent.provider"); 1167 else if (Configuration.doAutoCreate()) 1168 this.provider = new Reference(); // cc 1169 return this.provider; 1170 } 1171 1172 public boolean hasProvider() { 1173 return this.provider != null && !this.provider.isEmpty(); 1174 } 1175 1176 /** 1177 * @param value {@link #provider} (The practitioner who is eligible for the provision of the product or service.) 1178 */ 1179 public ItemsComponent setProvider(Reference value) { 1180 this.provider = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 1186 */ 1187 public BooleanType getExcludedElement() { 1188 if (this.excluded == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create ItemsComponent.excluded"); 1191 else if (Configuration.doAutoCreate()) 1192 this.excluded = new BooleanType(); // bb 1193 return this.excluded; 1194 } 1195 1196 public boolean hasExcludedElement() { 1197 return this.excluded != null && !this.excluded.isEmpty(); 1198 } 1199 1200 public boolean hasExcluded() { 1201 return this.excluded != null && !this.excluded.isEmpty(); 1202 } 1203 1204 /** 1205 * @param value {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 1206 */ 1207 public ItemsComponent setExcludedElement(BooleanType value) { 1208 this.excluded = value; 1209 return this; 1210 } 1211 1212 /** 1213 * @return True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 1214 */ 1215 public boolean getExcluded() { 1216 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 1217 } 1218 1219 /** 1220 * @param value True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 1221 */ 1222 public ItemsComponent setExcluded(boolean value) { 1223 if (this.excluded == null) 1224 this.excluded = new BooleanType(); 1225 this.excluded.setValue(value); 1226 return this; 1227 } 1228 1229 /** 1230 * @return {@link #name} (A short name or tag for the benefit.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1231 */ 1232 public StringType getNameElement() { 1233 if (this.name == null) 1234 if (Configuration.errorOnAutoCreate()) 1235 throw new Error("Attempt to auto-create ItemsComponent.name"); 1236 else if (Configuration.doAutoCreate()) 1237 this.name = new StringType(); // bb 1238 return this.name; 1239 } 1240 1241 public boolean hasNameElement() { 1242 return this.name != null && !this.name.isEmpty(); 1243 } 1244 1245 public boolean hasName() { 1246 return this.name != null && !this.name.isEmpty(); 1247 } 1248 1249 /** 1250 * @param value {@link #name} (A short name or tag for the benefit.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1251 */ 1252 public ItemsComponent setNameElement(StringType value) { 1253 this.name = value; 1254 return this; 1255 } 1256 1257 /** 1258 * @return A short name or tag for the benefit. 1259 */ 1260 public String getName() { 1261 return this.name == null ? null : this.name.getValue(); 1262 } 1263 1264 /** 1265 * @param value A short name or tag for the benefit. 1266 */ 1267 public ItemsComponent setName(String value) { 1268 if (Utilities.noString(value)) 1269 this.name = null; 1270 else { 1271 if (this.name == null) 1272 this.name = new StringType(); 1273 this.name.setValue(value); 1274 } 1275 return this; 1276 } 1277 1278 /** 1279 * @return {@link #description} (A richer description of the benefit or services covered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1280 */ 1281 public StringType getDescriptionElement() { 1282 if (this.description == null) 1283 if (Configuration.errorOnAutoCreate()) 1284 throw new Error("Attempt to auto-create ItemsComponent.description"); 1285 else if (Configuration.doAutoCreate()) 1286 this.description = new StringType(); // bb 1287 return this.description; 1288 } 1289 1290 public boolean hasDescriptionElement() { 1291 return this.description != null && !this.description.isEmpty(); 1292 } 1293 1294 public boolean hasDescription() { 1295 return this.description != null && !this.description.isEmpty(); 1296 } 1297 1298 /** 1299 * @param value {@link #description} (A richer description of the benefit or services covered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1300 */ 1301 public ItemsComponent setDescriptionElement(StringType value) { 1302 this.description = value; 1303 return this; 1304 } 1305 1306 /** 1307 * @return A richer description of the benefit or services covered. 1308 */ 1309 public String getDescription() { 1310 return this.description == null ? null : this.description.getValue(); 1311 } 1312 1313 /** 1314 * @param value A richer description of the benefit or services covered. 1315 */ 1316 public ItemsComponent setDescription(String value) { 1317 if (Utilities.noString(value)) 1318 this.description = null; 1319 else { 1320 if (this.description == null) 1321 this.description = new StringType(); 1322 this.description.setValue(value); 1323 } 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 1329 */ 1330 public CodeableConcept getNetwork() { 1331 if (this.network == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create ItemsComponent.network"); 1334 else if (Configuration.doAutoCreate()) 1335 this.network = new CodeableConcept(); // cc 1336 return this.network; 1337 } 1338 1339 public boolean hasNetwork() { 1340 return this.network != null && !this.network.isEmpty(); 1341 } 1342 1343 /** 1344 * @param value {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 1345 */ 1346 public ItemsComponent setNetwork(CodeableConcept value) { 1347 this.network = value; 1348 return this; 1349 } 1350 1351 /** 1352 * @return {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 1353 */ 1354 public CodeableConcept getUnit() { 1355 if (this.unit == null) 1356 if (Configuration.errorOnAutoCreate()) 1357 throw new Error("Attempt to auto-create ItemsComponent.unit"); 1358 else if (Configuration.doAutoCreate()) 1359 this.unit = new CodeableConcept(); // cc 1360 return this.unit; 1361 } 1362 1363 public boolean hasUnit() { 1364 return this.unit != null && !this.unit.isEmpty(); 1365 } 1366 1367 /** 1368 * @param value {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 1369 */ 1370 public ItemsComponent setUnit(CodeableConcept value) { 1371 this.unit = value; 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 1377 */ 1378 public CodeableConcept getTerm() { 1379 if (this.term == null) 1380 if (Configuration.errorOnAutoCreate()) 1381 throw new Error("Attempt to auto-create ItemsComponent.term"); 1382 else if (Configuration.doAutoCreate()) 1383 this.term = new CodeableConcept(); // cc 1384 return this.term; 1385 } 1386 1387 public boolean hasTerm() { 1388 return this.term != null && !this.term.isEmpty(); 1389 } 1390 1391 /** 1392 * @param value {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 1393 */ 1394 public ItemsComponent setTerm(CodeableConcept value) { 1395 this.term = value; 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #benefit} (Benefits used to date.) 1401 */ 1402 public List<BenefitComponent> getBenefit() { 1403 if (this.benefit == null) 1404 this.benefit = new ArrayList<BenefitComponent>(); 1405 return this.benefit; 1406 } 1407 1408 /** 1409 * @return Returns a reference to <code>this</code> for easy method chaining 1410 */ 1411 public ItemsComponent setBenefit(List<BenefitComponent> theBenefit) { 1412 this.benefit = theBenefit; 1413 return this; 1414 } 1415 1416 public boolean hasBenefit() { 1417 if (this.benefit == null) 1418 return false; 1419 for (BenefitComponent item : this.benefit) 1420 if (!item.isEmpty()) 1421 return true; 1422 return false; 1423 } 1424 1425 public BenefitComponent addBenefit() { //3 1426 BenefitComponent t = new BenefitComponent(); 1427 if (this.benefit == null) 1428 this.benefit = new ArrayList<BenefitComponent>(); 1429 this.benefit.add(t); 1430 return t; 1431 } 1432 1433 public ItemsComponent addBenefit(BenefitComponent t) { //3 1434 if (t == null) 1435 return this; 1436 if (this.benefit == null) 1437 this.benefit = new ArrayList<BenefitComponent>(); 1438 this.benefit.add(t); 1439 return this; 1440 } 1441 1442 /** 1443 * @return The first repetition of repeating field {@link #benefit}, creating it if it does not already exist {3} 1444 */ 1445 public BenefitComponent getBenefitFirstRep() { 1446 if (getBenefit().isEmpty()) { 1447 addBenefit(); 1448 } 1449 return getBenefit().get(0); 1450 } 1451 1452 /** 1453 * @return {@link #authorizationRequired} (A boolean flag indicating whether a preauthorization is required prior to actual service delivery.). This is the underlying object with id, value and extensions. The accessor "getAuthorizationRequired" gives direct access to the value 1454 */ 1455 public BooleanType getAuthorizationRequiredElement() { 1456 if (this.authorizationRequired == null) 1457 if (Configuration.errorOnAutoCreate()) 1458 throw new Error("Attempt to auto-create ItemsComponent.authorizationRequired"); 1459 else if (Configuration.doAutoCreate()) 1460 this.authorizationRequired = new BooleanType(); // bb 1461 return this.authorizationRequired; 1462 } 1463 1464 public boolean hasAuthorizationRequiredElement() { 1465 return this.authorizationRequired != null && !this.authorizationRequired.isEmpty(); 1466 } 1467 1468 public boolean hasAuthorizationRequired() { 1469 return this.authorizationRequired != null && !this.authorizationRequired.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #authorizationRequired} (A boolean flag indicating whether a preauthorization is required prior to actual service delivery.). This is the underlying object with id, value and extensions. The accessor "getAuthorizationRequired" gives direct access to the value 1474 */ 1475 public ItemsComponent setAuthorizationRequiredElement(BooleanType value) { 1476 this.authorizationRequired = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return A boolean flag indicating whether a preauthorization is required prior to actual service delivery. 1482 */ 1483 public boolean getAuthorizationRequired() { 1484 return this.authorizationRequired == null || this.authorizationRequired.isEmpty() ? false : this.authorizationRequired.getValue(); 1485 } 1486 1487 /** 1488 * @param value A boolean flag indicating whether a preauthorization is required prior to actual service delivery. 1489 */ 1490 public ItemsComponent setAuthorizationRequired(boolean value) { 1491 if (this.authorizationRequired == null) 1492 this.authorizationRequired = new BooleanType(); 1493 this.authorizationRequired.setValue(value); 1494 return this; 1495 } 1496 1497 /** 1498 * @return {@link #authorizationSupporting} (Codes or comments regarding information or actions associated with the preauthorization.) 1499 */ 1500 public List<CodeableConcept> getAuthorizationSupporting() { 1501 if (this.authorizationSupporting == null) 1502 this.authorizationSupporting = new ArrayList<CodeableConcept>(); 1503 return this.authorizationSupporting; 1504 } 1505 1506 /** 1507 * @return Returns a reference to <code>this</code> for easy method chaining 1508 */ 1509 public ItemsComponent setAuthorizationSupporting(List<CodeableConcept> theAuthorizationSupporting) { 1510 this.authorizationSupporting = theAuthorizationSupporting; 1511 return this; 1512 } 1513 1514 public boolean hasAuthorizationSupporting() { 1515 if (this.authorizationSupporting == null) 1516 return false; 1517 for (CodeableConcept item : this.authorizationSupporting) 1518 if (!item.isEmpty()) 1519 return true; 1520 return false; 1521 } 1522 1523 public CodeableConcept addAuthorizationSupporting() { //3 1524 CodeableConcept t = new CodeableConcept(); 1525 if (this.authorizationSupporting == null) 1526 this.authorizationSupporting = new ArrayList<CodeableConcept>(); 1527 this.authorizationSupporting.add(t); 1528 return t; 1529 } 1530 1531 public ItemsComponent addAuthorizationSupporting(CodeableConcept t) { //3 1532 if (t == null) 1533 return this; 1534 if (this.authorizationSupporting == null) 1535 this.authorizationSupporting = new ArrayList<CodeableConcept>(); 1536 this.authorizationSupporting.add(t); 1537 return this; 1538 } 1539 1540 /** 1541 * @return The first repetition of repeating field {@link #authorizationSupporting}, creating it if it does not already exist {3} 1542 */ 1543 public CodeableConcept getAuthorizationSupportingFirstRep() { 1544 if (getAuthorizationSupporting().isEmpty()) { 1545 addAuthorizationSupporting(); 1546 } 1547 return getAuthorizationSupporting().get(0); 1548 } 1549 1550 /** 1551 * @return {@link #authorizationUrl} (A web location for obtaining requirements or descriptive information regarding the preauthorization.). This is the underlying object with id, value and extensions. The accessor "getAuthorizationUrl" gives direct access to the value 1552 */ 1553 public UriType getAuthorizationUrlElement() { 1554 if (this.authorizationUrl == null) 1555 if (Configuration.errorOnAutoCreate()) 1556 throw new Error("Attempt to auto-create ItemsComponent.authorizationUrl"); 1557 else if (Configuration.doAutoCreate()) 1558 this.authorizationUrl = new UriType(); // bb 1559 return this.authorizationUrl; 1560 } 1561 1562 public boolean hasAuthorizationUrlElement() { 1563 return this.authorizationUrl != null && !this.authorizationUrl.isEmpty(); 1564 } 1565 1566 public boolean hasAuthorizationUrl() { 1567 return this.authorizationUrl != null && !this.authorizationUrl.isEmpty(); 1568 } 1569 1570 /** 1571 * @param value {@link #authorizationUrl} (A web location for obtaining requirements or descriptive information regarding the preauthorization.). This is the underlying object with id, value and extensions. The accessor "getAuthorizationUrl" gives direct access to the value 1572 */ 1573 public ItemsComponent setAuthorizationUrlElement(UriType value) { 1574 this.authorizationUrl = value; 1575 return this; 1576 } 1577 1578 /** 1579 * @return A web location for obtaining requirements or descriptive information regarding the preauthorization. 1580 */ 1581 public String getAuthorizationUrl() { 1582 return this.authorizationUrl == null ? null : this.authorizationUrl.getValue(); 1583 } 1584 1585 /** 1586 * @param value A web location for obtaining requirements or descriptive information regarding the preauthorization. 1587 */ 1588 public ItemsComponent setAuthorizationUrl(String value) { 1589 if (Utilities.noString(value)) 1590 this.authorizationUrl = null; 1591 else { 1592 if (this.authorizationUrl == null) 1593 this.authorizationUrl = new UriType(); 1594 this.authorizationUrl.setValue(value); 1595 } 1596 return this; 1597 } 1598 1599 protected void listChildren(List<Property> children) { 1600 super.listChildren(children); 1601 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 1602 children.add(new Property("productOrService", "CodeableConcept", "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService)); 1603 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 1604 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole)", "The practitioner who is eligible for the provision of the product or service.", 0, 1, provider)); 1605 children.add(new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 0, 1, excluded)); 1606 children.add(new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name)); 1607 children.add(new Property("description", "string", "A richer description of the benefit or services covered.", 0, 1, description)); 1608 children.add(new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network)); 1609 children.add(new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit)); 1610 children.add(new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term)); 1611 children.add(new Property("benefit", "", "Benefits used to date.", 0, java.lang.Integer.MAX_VALUE, benefit)); 1612 children.add(new Property("authorizationRequired", "boolean", "A boolean flag indicating whether a preauthorization is required prior to actual service delivery.", 0, 1, authorizationRequired)); 1613 children.add(new Property("authorizationSupporting", "CodeableConcept", "Codes or comments regarding information or actions associated with the preauthorization.", 0, java.lang.Integer.MAX_VALUE, authorizationSupporting)); 1614 children.add(new Property("authorizationUrl", "uri", "A web location for obtaining requirements or descriptive information regarding the preauthorization.", 0, 1, authorizationUrl)); 1615 } 1616 1617 @Override 1618 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1619 switch (_hash) { 1620 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 1621 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService); 1622 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 1623 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole)", "The practitioner who is eligible for the provision of the product or service.", 0, 1, provider); 1624 case 1994055114: /*excluded*/ return new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 0, 1, excluded); 1625 case 3373707: /*name*/ return new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name); 1626 case -1724546052: /*description*/ return new Property("description", "string", "A richer description of the benefit or services covered.", 0, 1, description); 1627 case 1843485230: /*network*/ return new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network); 1628 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit); 1629 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term); 1630 case -222710633: /*benefit*/ return new Property("benefit", "", "Benefits used to date.", 0, java.lang.Integer.MAX_VALUE, benefit); 1631 case 374204216: /*authorizationRequired*/ return new Property("authorizationRequired", "boolean", "A boolean flag indicating whether a preauthorization is required prior to actual service delivery.", 0, 1, authorizationRequired); 1632 case -1931146484: /*authorizationSupporting*/ return new Property("authorizationSupporting", "CodeableConcept", "Codes or comments regarding information or actions associated with the preauthorization.", 0, java.lang.Integer.MAX_VALUE, authorizationSupporting); 1633 case 1409445430: /*authorizationUrl*/ return new Property("authorizationUrl", "uri", "A web location for obtaining requirements or descriptive information regarding the preauthorization.", 0, 1, authorizationUrl); 1634 default: return super.getNamedProperty(_hash, _name, _checkValid); 1635 } 1636 1637 } 1638 1639 @Override 1640 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1641 switch (hash) { 1642 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1643 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 1644 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 1645 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1646 case 1994055114: /*excluded*/ return this.excluded == null ? new Base[0] : new Base[] {this.excluded}; // BooleanType 1647 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1648 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1649 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // CodeableConcept 1650 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 1651 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 1652 case -222710633: /*benefit*/ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // BenefitComponent 1653 case 374204216: /*authorizationRequired*/ return this.authorizationRequired == null ? new Base[0] : new Base[] {this.authorizationRequired}; // BooleanType 1654 case -1931146484: /*authorizationSupporting*/ return this.authorizationSupporting == null ? new Base[0] : this.authorizationSupporting.toArray(new Base[this.authorizationSupporting.size()]); // CodeableConcept 1655 case 1409445430: /*authorizationUrl*/ return this.authorizationUrl == null ? new Base[0] : new Base[] {this.authorizationUrl}; // UriType 1656 default: return super.getProperty(hash, name, checkValid); 1657 } 1658 1659 } 1660 1661 @Override 1662 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1663 switch (hash) { 1664 case 50511102: // category 1665 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1666 return value; 1667 case 1957227299: // productOrService 1668 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1669 return value; 1670 case -615513385: // modifier 1671 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1672 return value; 1673 case -987494927: // provider 1674 this.provider = TypeConvertor.castToReference(value); // Reference 1675 return value; 1676 case 1994055114: // excluded 1677 this.excluded = TypeConvertor.castToBoolean(value); // BooleanType 1678 return value; 1679 case 3373707: // name 1680 this.name = TypeConvertor.castToString(value); // StringType 1681 return value; 1682 case -1724546052: // description 1683 this.description = TypeConvertor.castToString(value); // StringType 1684 return value; 1685 case 1843485230: // network 1686 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1687 return value; 1688 case 3594628: // unit 1689 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1690 return value; 1691 case 3556460: // term 1692 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1693 return value; 1694 case -222710633: // benefit 1695 this.getBenefit().add((BenefitComponent) value); // BenefitComponent 1696 return value; 1697 case 374204216: // authorizationRequired 1698 this.authorizationRequired = TypeConvertor.castToBoolean(value); // BooleanType 1699 return value; 1700 case -1931146484: // authorizationSupporting 1701 this.getAuthorizationSupporting().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1702 return value; 1703 case 1409445430: // authorizationUrl 1704 this.authorizationUrl = TypeConvertor.castToUri(value); // UriType 1705 return value; 1706 default: return super.setProperty(hash, name, value); 1707 } 1708 1709 } 1710 1711 @Override 1712 public Base setProperty(String name, Base value) throws FHIRException { 1713 if (name.equals("category")) { 1714 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1715 } else if (name.equals("productOrService")) { 1716 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1717 } else if (name.equals("modifier")) { 1718 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 1719 } else if (name.equals("provider")) { 1720 this.provider = TypeConvertor.castToReference(value); // Reference 1721 } else if (name.equals("excluded")) { 1722 this.excluded = TypeConvertor.castToBoolean(value); // BooleanType 1723 } else if (name.equals("name")) { 1724 this.name = TypeConvertor.castToString(value); // StringType 1725 } else if (name.equals("description")) { 1726 this.description = TypeConvertor.castToString(value); // StringType 1727 } else if (name.equals("network")) { 1728 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1729 } else if (name.equals("unit")) { 1730 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1731 } else if (name.equals("term")) { 1732 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1733 } else if (name.equals("benefit")) { 1734 this.getBenefit().add((BenefitComponent) value); 1735 } else if (name.equals("authorizationRequired")) { 1736 this.authorizationRequired = TypeConvertor.castToBoolean(value); // BooleanType 1737 } else if (name.equals("authorizationSupporting")) { 1738 this.getAuthorizationSupporting().add(TypeConvertor.castToCodeableConcept(value)); 1739 } else if (name.equals("authorizationUrl")) { 1740 this.authorizationUrl = TypeConvertor.castToUri(value); // UriType 1741 } else 1742 return super.setProperty(name, value); 1743 return value; 1744 } 1745 1746 @Override 1747 public void removeChild(String name, Base value) throws FHIRException { 1748 if (name.equals("category")) { 1749 this.category = null; 1750 } else if (name.equals("productOrService")) { 1751 this.productOrService = null; 1752 } else if (name.equals("modifier")) { 1753 this.getModifier().remove(value); 1754 } else if (name.equals("provider")) { 1755 this.provider = null; 1756 } else if (name.equals("excluded")) { 1757 this.excluded = null; 1758 } else if (name.equals("name")) { 1759 this.name = null; 1760 } else if (name.equals("description")) { 1761 this.description = null; 1762 } else if (name.equals("network")) { 1763 this.network = null; 1764 } else if (name.equals("unit")) { 1765 this.unit = null; 1766 } else if (name.equals("term")) { 1767 this.term = null; 1768 } else if (name.equals("benefit")) { 1769 this.getBenefit().remove((BenefitComponent) value); 1770 } else if (name.equals("authorizationRequired")) { 1771 this.authorizationRequired = null; 1772 } else if (name.equals("authorizationSupporting")) { 1773 this.getAuthorizationSupporting().remove(value); 1774 } else if (name.equals("authorizationUrl")) { 1775 this.authorizationUrl = null; 1776 } else 1777 super.removeChild(name, value); 1778 1779 } 1780 1781 @Override 1782 public Base makeProperty(int hash, String name) throws FHIRException { 1783 switch (hash) { 1784 case 50511102: return getCategory(); 1785 case 1957227299: return getProductOrService(); 1786 case -615513385: return addModifier(); 1787 case -987494927: return getProvider(); 1788 case 1994055114: return getExcludedElement(); 1789 case 3373707: return getNameElement(); 1790 case -1724546052: return getDescriptionElement(); 1791 case 1843485230: return getNetwork(); 1792 case 3594628: return getUnit(); 1793 case 3556460: return getTerm(); 1794 case -222710633: return addBenefit(); 1795 case 374204216: return getAuthorizationRequiredElement(); 1796 case -1931146484: return addAuthorizationSupporting(); 1797 case 1409445430: return getAuthorizationUrlElement(); 1798 default: return super.makeProperty(hash, name); 1799 } 1800 1801 } 1802 1803 @Override 1804 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1805 switch (hash) { 1806 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1807 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 1808 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 1809 case -987494927: /*provider*/ return new String[] {"Reference"}; 1810 case 1994055114: /*excluded*/ return new String[] {"boolean"}; 1811 case 3373707: /*name*/ return new String[] {"string"}; 1812 case -1724546052: /*description*/ return new String[] {"string"}; 1813 case 1843485230: /*network*/ return new String[] {"CodeableConcept"}; 1814 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 1815 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 1816 case -222710633: /*benefit*/ return new String[] {}; 1817 case 374204216: /*authorizationRequired*/ return new String[] {"boolean"}; 1818 case -1931146484: /*authorizationSupporting*/ return new String[] {"CodeableConcept"}; 1819 case 1409445430: /*authorizationUrl*/ return new String[] {"uri"}; 1820 default: return super.getTypesForProperty(hash, name); 1821 } 1822 1823 } 1824 1825 @Override 1826 public Base addChild(String name) throws FHIRException { 1827 if (name.equals("category")) { 1828 this.category = new CodeableConcept(); 1829 return this.category; 1830 } 1831 else if (name.equals("productOrService")) { 1832 this.productOrService = new CodeableConcept(); 1833 return this.productOrService; 1834 } 1835 else if (name.equals("modifier")) { 1836 return addModifier(); 1837 } 1838 else if (name.equals("provider")) { 1839 this.provider = new Reference(); 1840 return this.provider; 1841 } 1842 else if (name.equals("excluded")) { 1843 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.insurance.item.excluded"); 1844 } 1845 else if (name.equals("name")) { 1846 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.insurance.item.name"); 1847 } 1848 else if (name.equals("description")) { 1849 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.insurance.item.description"); 1850 } 1851 else if (name.equals("network")) { 1852 this.network = new CodeableConcept(); 1853 return this.network; 1854 } 1855 else if (name.equals("unit")) { 1856 this.unit = new CodeableConcept(); 1857 return this.unit; 1858 } 1859 else if (name.equals("term")) { 1860 this.term = new CodeableConcept(); 1861 return this.term; 1862 } 1863 else if (name.equals("benefit")) { 1864 return addBenefit(); 1865 } 1866 else if (name.equals("authorizationRequired")) { 1867 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.insurance.item.authorizationRequired"); 1868 } 1869 else if (name.equals("authorizationSupporting")) { 1870 return addAuthorizationSupporting(); 1871 } 1872 else if (name.equals("authorizationUrl")) { 1873 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.insurance.item.authorizationUrl"); 1874 } 1875 else 1876 return super.addChild(name); 1877 } 1878 1879 public ItemsComponent copy() { 1880 ItemsComponent dst = new ItemsComponent(); 1881 copyValues(dst); 1882 return dst; 1883 } 1884 1885 public void copyValues(ItemsComponent dst) { 1886 super.copyValues(dst); 1887 dst.category = category == null ? null : category.copy(); 1888 dst.productOrService = productOrService == null ? null : productOrService.copy(); 1889 if (modifier != null) { 1890 dst.modifier = new ArrayList<CodeableConcept>(); 1891 for (CodeableConcept i : modifier) 1892 dst.modifier.add(i.copy()); 1893 }; 1894 dst.provider = provider == null ? null : provider.copy(); 1895 dst.excluded = excluded == null ? null : excluded.copy(); 1896 dst.name = name == null ? null : name.copy(); 1897 dst.description = description == null ? null : description.copy(); 1898 dst.network = network == null ? null : network.copy(); 1899 dst.unit = unit == null ? null : unit.copy(); 1900 dst.term = term == null ? null : term.copy(); 1901 if (benefit != null) { 1902 dst.benefit = new ArrayList<BenefitComponent>(); 1903 for (BenefitComponent i : benefit) 1904 dst.benefit.add(i.copy()); 1905 }; 1906 dst.authorizationRequired = authorizationRequired == null ? null : authorizationRequired.copy(); 1907 if (authorizationSupporting != null) { 1908 dst.authorizationSupporting = new ArrayList<CodeableConcept>(); 1909 for (CodeableConcept i : authorizationSupporting) 1910 dst.authorizationSupporting.add(i.copy()); 1911 }; 1912 dst.authorizationUrl = authorizationUrl == null ? null : authorizationUrl.copy(); 1913 } 1914 1915 @Override 1916 public boolean equalsDeep(Base other_) { 1917 if (!super.equalsDeep(other_)) 1918 return false; 1919 if (!(other_ instanceof ItemsComponent)) 1920 return false; 1921 ItemsComponent o = (ItemsComponent) other_; 1922 return compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 1923 && compareDeep(modifier, o.modifier, true) && compareDeep(provider, o.provider, true) && compareDeep(excluded, o.excluded, true) 1924 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(network, o.network, true) 1925 && compareDeep(unit, o.unit, true) && compareDeep(term, o.term, true) && compareDeep(benefit, o.benefit, true) 1926 && compareDeep(authorizationRequired, o.authorizationRequired, true) && compareDeep(authorizationSupporting, o.authorizationSupporting, true) 1927 && compareDeep(authorizationUrl, o.authorizationUrl, true); 1928 } 1929 1930 @Override 1931 public boolean equalsShallow(Base other_) { 1932 if (!super.equalsShallow(other_)) 1933 return false; 1934 if (!(other_ instanceof ItemsComponent)) 1935 return false; 1936 ItemsComponent o = (ItemsComponent) other_; 1937 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 1938 && compareValues(authorizationRequired, o.authorizationRequired, true) && compareValues(authorizationUrl, o.authorizationUrl, true) 1939 ; 1940 } 1941 1942 public boolean isEmpty() { 1943 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, productOrService 1944 , modifier, provider, excluded, name, description, network, unit, term, benefit 1945 , authorizationRequired, authorizationSupporting, authorizationUrl); 1946 } 1947 1948 public String fhirType() { 1949 return "CoverageEligibilityResponse.insurance.item"; 1950 1951 } 1952 1953 } 1954 1955 @Block() 1956 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 1957 /** 1958 * Classification of benefit being provided. 1959 */ 1960 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1961 @Description(shortDefinition="Benefit classification", formalDefinition="Classification of benefit being provided." ) 1962 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-type") 1963 protected CodeableConcept type; 1964 1965 /** 1966 * The quantity of the benefit which is permitted under the coverage. 1967 */ 1968 @Child(name = "allowed", type = {UnsignedIntType.class, StringType.class, Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 1969 @Description(shortDefinition="Benefits allowed", formalDefinition="The quantity of the benefit which is permitted under the coverage." ) 1970 protected DataType allowed; 1971 1972 /** 1973 * The quantity of the benefit which have been consumed to date. 1974 */ 1975 @Child(name = "used", type = {UnsignedIntType.class, StringType.class, Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 1976 @Description(shortDefinition="Benefits used", formalDefinition="The quantity of the benefit which have been consumed to date." ) 1977 protected DataType used; 1978 1979 private static final long serialVersionUID = 1900247614L; 1980 1981 /** 1982 * Constructor 1983 */ 1984 public BenefitComponent() { 1985 super(); 1986 } 1987 1988 /** 1989 * Constructor 1990 */ 1991 public BenefitComponent(CodeableConcept type) { 1992 super(); 1993 this.setType(type); 1994 } 1995 1996 /** 1997 * @return {@link #type} (Classification of benefit being provided.) 1998 */ 1999 public CodeableConcept getType() { 2000 if (this.type == null) 2001 if (Configuration.errorOnAutoCreate()) 2002 throw new Error("Attempt to auto-create BenefitComponent.type"); 2003 else if (Configuration.doAutoCreate()) 2004 this.type = new CodeableConcept(); // cc 2005 return this.type; 2006 } 2007 2008 public boolean hasType() { 2009 return this.type != null && !this.type.isEmpty(); 2010 } 2011 2012 /** 2013 * @param value {@link #type} (Classification of benefit being provided.) 2014 */ 2015 public BenefitComponent setType(CodeableConcept value) { 2016 this.type = value; 2017 return this; 2018 } 2019 2020 /** 2021 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 2022 */ 2023 public DataType getAllowed() { 2024 return this.allowed; 2025 } 2026 2027 /** 2028 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 2029 */ 2030 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 2031 if (this.allowed == null) 2032 this.allowed = new UnsignedIntType(); 2033 if (!(this.allowed instanceof UnsignedIntType)) 2034 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 2035 return (UnsignedIntType) this.allowed; 2036 } 2037 2038 public boolean hasAllowedUnsignedIntType() { 2039 return this.allowed instanceof UnsignedIntType; 2040 } 2041 2042 /** 2043 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 2044 */ 2045 public StringType getAllowedStringType() throws FHIRException { 2046 if (this.allowed == null) 2047 this.allowed = new StringType(); 2048 if (!(this.allowed instanceof StringType)) 2049 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 2050 return (StringType) this.allowed; 2051 } 2052 2053 public boolean hasAllowedStringType() { 2054 return this.allowed instanceof StringType; 2055 } 2056 2057 /** 2058 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 2059 */ 2060 public Money getAllowedMoney() throws FHIRException { 2061 if (this.allowed == null) 2062 this.allowed = new Money(); 2063 if (!(this.allowed instanceof Money)) 2064 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.allowed.getClass().getName()+" was encountered"); 2065 return (Money) this.allowed; 2066 } 2067 2068 public boolean hasAllowedMoney() { 2069 return this.allowed instanceof Money; 2070 } 2071 2072 public boolean hasAllowed() { 2073 return this.allowed != null && !this.allowed.isEmpty(); 2074 } 2075 2076 /** 2077 * @param value {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 2078 */ 2079 public BenefitComponent setAllowed(DataType value) { 2080 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 2081 throw new FHIRException("Not the right type for CoverageEligibilityResponse.insurance.item.benefit.allowed[x]: "+value.fhirType()); 2082 this.allowed = value; 2083 return this; 2084 } 2085 2086 /** 2087 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 2088 */ 2089 public DataType getUsed() { 2090 return this.used; 2091 } 2092 2093 /** 2094 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 2095 */ 2096 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 2097 if (this.used == null) 2098 this.used = new UnsignedIntType(); 2099 if (!(this.used instanceof UnsignedIntType)) 2100 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.used.getClass().getName()+" was encountered"); 2101 return (UnsignedIntType) this.used; 2102 } 2103 2104 public boolean hasUsedUnsignedIntType() { 2105 return this.used instanceof UnsignedIntType; 2106 } 2107 2108 /** 2109 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 2110 */ 2111 public StringType getUsedStringType() throws FHIRException { 2112 if (this.used == null) 2113 this.used = new StringType(); 2114 if (!(this.used instanceof StringType)) 2115 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.used.getClass().getName()+" was encountered"); 2116 return (StringType) this.used; 2117 } 2118 2119 public boolean hasUsedStringType() { 2120 return this.used instanceof StringType; 2121 } 2122 2123 /** 2124 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 2125 */ 2126 public Money getUsedMoney() throws FHIRException { 2127 if (this.used == null) 2128 this.used = new Money(); 2129 if (!(this.used instanceof Money)) 2130 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.used.getClass().getName()+" was encountered"); 2131 return (Money) this.used; 2132 } 2133 2134 public boolean hasUsedMoney() { 2135 return this.used instanceof Money; 2136 } 2137 2138 public boolean hasUsed() { 2139 return this.used != null && !this.used.isEmpty(); 2140 } 2141 2142 /** 2143 * @param value {@link #used} (The quantity of the benefit which have been consumed to date.) 2144 */ 2145 public BenefitComponent setUsed(DataType value) { 2146 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 2147 throw new FHIRException("Not the right type for CoverageEligibilityResponse.insurance.item.benefit.used[x]: "+value.fhirType()); 2148 this.used = value; 2149 return this; 2150 } 2151 2152 protected void listChildren(List<Property> children) { 2153 super.listChildren(children); 2154 children.add(new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type)); 2155 children.add(new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed)); 2156 children.add(new Property("used[x]", "unsignedInt|string|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used)); 2157 } 2158 2159 @Override 2160 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2161 switch (_hash) { 2162 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type); 2163 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2164 case -911343192: /*allowed*/ return new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2165 case 1668802034: /*allowedUnsignedInt*/ return new Property("allowed[x]", "unsignedInt", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2166 case -2135265319: /*allowedString*/ return new Property("allowed[x]", "string", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2167 case -351668232: /*allowedMoney*/ return new Property("allowed[x]", "Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2168 case -147553373: /*used[x]*/ return new Property("used[x]", "unsignedInt|string|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2169 case 3599293: /*used*/ return new Property("used[x]", "unsignedInt|string|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2170 case 1252740285: /*usedUnsignedInt*/ return new Property("used[x]", "unsignedInt", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2171 case 2051978798: /*usedString*/ return new Property("used[x]", "string", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2172 case -78048509: /*usedMoney*/ return new Property("used[x]", "Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2173 default: return super.getNamedProperty(_hash, _name, _checkValid); 2174 } 2175 2176 } 2177 2178 @Override 2179 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2180 switch (hash) { 2181 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2182 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // DataType 2183 case 3599293: /*used*/ return this.used == null ? new Base[0] : new Base[] {this.used}; // DataType 2184 default: return super.getProperty(hash, name, checkValid); 2185 } 2186 2187 } 2188 2189 @Override 2190 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2191 switch (hash) { 2192 case 3575610: // type 2193 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2194 return value; 2195 case -911343192: // allowed 2196 this.allowed = TypeConvertor.castToType(value); // DataType 2197 return value; 2198 case 3599293: // used 2199 this.used = TypeConvertor.castToType(value); // DataType 2200 return value; 2201 default: return super.setProperty(hash, name, value); 2202 } 2203 2204 } 2205 2206 @Override 2207 public Base setProperty(String name, Base value) throws FHIRException { 2208 if (name.equals("type")) { 2209 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2210 } else if (name.equals("allowed[x]")) { 2211 this.allowed = TypeConvertor.castToType(value); // DataType 2212 } else if (name.equals("used[x]")) { 2213 this.used = TypeConvertor.castToType(value); // DataType 2214 } else 2215 return super.setProperty(name, value); 2216 return value; 2217 } 2218 2219 @Override 2220 public void removeChild(String name, Base value) throws FHIRException { 2221 if (name.equals("type")) { 2222 this.type = null; 2223 } else if (name.equals("allowed[x]")) { 2224 this.allowed = null; 2225 } else if (name.equals("used[x]")) { 2226 this.used = null; 2227 } else 2228 super.removeChild(name, value); 2229 2230 } 2231 2232 @Override 2233 public Base makeProperty(int hash, String name) throws FHIRException { 2234 switch (hash) { 2235 case 3575610: return getType(); 2236 case -1336663592: return getAllowed(); 2237 case -911343192: return getAllowed(); 2238 case -147553373: return getUsed(); 2239 case 3599293: return getUsed(); 2240 default: return super.makeProperty(hash, name); 2241 } 2242 2243 } 2244 2245 @Override 2246 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2247 switch (hash) { 2248 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2249 case -911343192: /*allowed*/ return new String[] {"unsignedInt", "string", "Money"}; 2250 case 3599293: /*used*/ return new String[] {"unsignedInt", "string", "Money"}; 2251 default: return super.getTypesForProperty(hash, name); 2252 } 2253 2254 } 2255 2256 @Override 2257 public Base addChild(String name) throws FHIRException { 2258 if (name.equals("type")) { 2259 this.type = new CodeableConcept(); 2260 return this.type; 2261 } 2262 else if (name.equals("allowedUnsignedInt")) { 2263 this.allowed = new UnsignedIntType(); 2264 return this.allowed; 2265 } 2266 else if (name.equals("allowedString")) { 2267 this.allowed = new StringType(); 2268 return this.allowed; 2269 } 2270 else if (name.equals("allowedMoney")) { 2271 this.allowed = new Money(); 2272 return this.allowed; 2273 } 2274 else if (name.equals("usedUnsignedInt")) { 2275 this.used = new UnsignedIntType(); 2276 return this.used; 2277 } 2278 else if (name.equals("usedString")) { 2279 this.used = new StringType(); 2280 return this.used; 2281 } 2282 else if (name.equals("usedMoney")) { 2283 this.used = new Money(); 2284 return this.used; 2285 } 2286 else 2287 return super.addChild(name); 2288 } 2289 2290 public BenefitComponent copy() { 2291 BenefitComponent dst = new BenefitComponent(); 2292 copyValues(dst); 2293 return dst; 2294 } 2295 2296 public void copyValues(BenefitComponent dst) { 2297 super.copyValues(dst); 2298 dst.type = type == null ? null : type.copy(); 2299 dst.allowed = allowed == null ? null : allowed.copy(); 2300 dst.used = used == null ? null : used.copy(); 2301 } 2302 2303 @Override 2304 public boolean equalsDeep(Base other_) { 2305 if (!super.equalsDeep(other_)) 2306 return false; 2307 if (!(other_ instanceof BenefitComponent)) 2308 return false; 2309 BenefitComponent o = (BenefitComponent) other_; 2310 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) && compareDeep(used, o.used, true) 2311 ; 2312 } 2313 2314 @Override 2315 public boolean equalsShallow(Base other_) { 2316 if (!super.equalsShallow(other_)) 2317 return false; 2318 if (!(other_ instanceof BenefitComponent)) 2319 return false; 2320 BenefitComponent o = (BenefitComponent) other_; 2321 return true; 2322 } 2323 2324 public boolean isEmpty() { 2325 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 2326 } 2327 2328 public String fhirType() { 2329 return "CoverageEligibilityResponse.insurance.item.benefit"; 2330 2331 } 2332 2333 } 2334 2335 @Block() 2336 public static class ErrorsComponent extends BackboneElement implements IBaseBackboneElement { 2337 /** 2338 * An error code,from a specified code system, which details why the eligibility check could not be performed. 2339 */ 2340 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 2341 @Description(shortDefinition="Error code detailing processing issues", formalDefinition="An error code,from a specified code system, which details why the eligibility check could not be performed." ) 2342 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-error") 2343 protected CodeableConcept code; 2344 2345 /** 2346 * A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised. 2347 */ 2348 @Child(name = "expression", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2349 @Description(shortDefinition="FHIRPath of element(s) related to issue", formalDefinition="A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised." ) 2350 protected List<StringType> expression; 2351 2352 private static final long serialVersionUID = -344349215L; 2353 2354 /** 2355 * Constructor 2356 */ 2357 public ErrorsComponent() { 2358 super(); 2359 } 2360 2361 /** 2362 * Constructor 2363 */ 2364 public ErrorsComponent(CodeableConcept code) { 2365 super(); 2366 this.setCode(code); 2367 } 2368 2369 /** 2370 * @return {@link #code} (An error code,from a specified code system, which details why the eligibility check could not be performed.) 2371 */ 2372 public CodeableConcept getCode() { 2373 if (this.code == null) 2374 if (Configuration.errorOnAutoCreate()) 2375 throw new Error("Attempt to auto-create ErrorsComponent.code"); 2376 else if (Configuration.doAutoCreate()) 2377 this.code = new CodeableConcept(); // cc 2378 return this.code; 2379 } 2380 2381 public boolean hasCode() { 2382 return this.code != null && !this.code.isEmpty(); 2383 } 2384 2385 /** 2386 * @param value {@link #code} (An error code,from a specified code system, which details why the eligibility check could not be performed.) 2387 */ 2388 public ErrorsComponent setCode(CodeableConcept value) { 2389 this.code = value; 2390 return this; 2391 } 2392 2393 /** 2394 * @return {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 2395 */ 2396 public List<StringType> getExpression() { 2397 if (this.expression == null) 2398 this.expression = new ArrayList<StringType>(); 2399 return this.expression; 2400 } 2401 2402 /** 2403 * @return Returns a reference to <code>this</code> for easy method chaining 2404 */ 2405 public ErrorsComponent setExpression(List<StringType> theExpression) { 2406 this.expression = theExpression; 2407 return this; 2408 } 2409 2410 public boolean hasExpression() { 2411 if (this.expression == null) 2412 return false; 2413 for (StringType item : this.expression) 2414 if (!item.isEmpty()) 2415 return true; 2416 return false; 2417 } 2418 2419 /** 2420 * @return {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 2421 */ 2422 public StringType addExpressionElement() {//2 2423 StringType t = new StringType(); 2424 if (this.expression == null) 2425 this.expression = new ArrayList<StringType>(); 2426 this.expression.add(t); 2427 return t; 2428 } 2429 2430 /** 2431 * @param value {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 2432 */ 2433 public ErrorsComponent addExpression(String value) { //1 2434 StringType t = new StringType(); 2435 t.setValue(value); 2436 if (this.expression == null) 2437 this.expression = new ArrayList<StringType>(); 2438 this.expression.add(t); 2439 return this; 2440 } 2441 2442 /** 2443 * @param value {@link #expression} (A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.) 2444 */ 2445 public boolean hasExpression(String value) { 2446 if (this.expression == null) 2447 return false; 2448 for (StringType v : this.expression) 2449 if (v.getValue().equals(value)) // string 2450 return true; 2451 return false; 2452 } 2453 2454 protected void listChildren(List<Property> children) { 2455 super.listChildren(children); 2456 children.add(new Property("code", "CodeableConcept", "An error code,from a specified code system, which details why the eligibility check could not be performed.", 0, 1, code)); 2457 children.add(new Property("expression", "string", "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression)); 2458 } 2459 2460 @Override 2461 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2462 switch (_hash) { 2463 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "An error code,from a specified code system, which details why the eligibility check could not be performed.", 0, 1, code); 2464 case -1795452264: /*expression*/ return new Property("expression", "string", "A [simple subset of FHIRPath](fhirpath.html#simple) limited to element names, repetition indicators and the default child accessor that identifies one of the elements in the resource that caused this issue to be raised.", 0, java.lang.Integer.MAX_VALUE, expression); 2465 default: return super.getNamedProperty(_hash, _name, _checkValid); 2466 } 2467 2468 } 2469 2470 @Override 2471 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2472 switch (hash) { 2473 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2474 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : this.expression.toArray(new Base[this.expression.size()]); // StringType 2475 default: return super.getProperty(hash, name, checkValid); 2476 } 2477 2478 } 2479 2480 @Override 2481 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2482 switch (hash) { 2483 case 3059181: // code 2484 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2485 return value; 2486 case -1795452264: // expression 2487 this.getExpression().add(TypeConvertor.castToString(value)); // StringType 2488 return value; 2489 default: return super.setProperty(hash, name, value); 2490 } 2491 2492 } 2493 2494 @Override 2495 public Base setProperty(String name, Base value) throws FHIRException { 2496 if (name.equals("code")) { 2497 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2498 } else if (name.equals("expression")) { 2499 this.getExpression().add(TypeConvertor.castToString(value)); 2500 } else 2501 return super.setProperty(name, value); 2502 return value; 2503 } 2504 2505 @Override 2506 public void removeChild(String name, Base value) throws FHIRException { 2507 if (name.equals("code")) { 2508 this.code = null; 2509 } else if (name.equals("expression")) { 2510 this.getExpression().remove(value); 2511 } else 2512 super.removeChild(name, value); 2513 2514 } 2515 2516 @Override 2517 public Base makeProperty(int hash, String name) throws FHIRException { 2518 switch (hash) { 2519 case 3059181: return getCode(); 2520 case -1795452264: return addExpressionElement(); 2521 default: return super.makeProperty(hash, name); 2522 } 2523 2524 } 2525 2526 @Override 2527 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2528 switch (hash) { 2529 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2530 case -1795452264: /*expression*/ return new String[] {"string"}; 2531 default: return super.getTypesForProperty(hash, name); 2532 } 2533 2534 } 2535 2536 @Override 2537 public Base addChild(String name) throws FHIRException { 2538 if (name.equals("code")) { 2539 this.code = new CodeableConcept(); 2540 return this.code; 2541 } 2542 else if (name.equals("expression")) { 2543 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.error.expression"); 2544 } 2545 else 2546 return super.addChild(name); 2547 } 2548 2549 public ErrorsComponent copy() { 2550 ErrorsComponent dst = new ErrorsComponent(); 2551 copyValues(dst); 2552 return dst; 2553 } 2554 2555 public void copyValues(ErrorsComponent dst) { 2556 super.copyValues(dst); 2557 dst.code = code == null ? null : code.copy(); 2558 if (expression != null) { 2559 dst.expression = new ArrayList<StringType>(); 2560 for (StringType i : expression) 2561 dst.expression.add(i.copy()); 2562 }; 2563 } 2564 2565 @Override 2566 public boolean equalsDeep(Base other_) { 2567 if (!super.equalsDeep(other_)) 2568 return false; 2569 if (!(other_ instanceof ErrorsComponent)) 2570 return false; 2571 ErrorsComponent o = (ErrorsComponent) other_; 2572 return compareDeep(code, o.code, true) && compareDeep(expression, o.expression, true); 2573 } 2574 2575 @Override 2576 public boolean equalsShallow(Base other_) { 2577 if (!super.equalsShallow(other_)) 2578 return false; 2579 if (!(other_ instanceof ErrorsComponent)) 2580 return false; 2581 ErrorsComponent o = (ErrorsComponent) other_; 2582 return compareValues(expression, o.expression, true); 2583 } 2584 2585 public boolean isEmpty() { 2586 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, expression); 2587 } 2588 2589 public String fhirType() { 2590 return "CoverageEligibilityResponse.error"; 2591 2592 } 2593 2594 } 2595 2596 /** 2597 * A unique identifier assigned to this coverage eligiblity request. 2598 */ 2599 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2600 @Description(shortDefinition="Business Identifier for coverage eligiblity request", formalDefinition="A unique identifier assigned to this coverage eligiblity request." ) 2601 protected List<Identifier> identifier; 2602 2603 /** 2604 * The status of the resource instance. 2605 */ 2606 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2607 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 2608 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 2609 protected Enumeration<FinancialResourceStatusCodes> status; 2610 2611 /** 2612 * Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified. 2613 */ 2614 @Child(name = "purpose", type = {CodeType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2615 @Description(shortDefinition="auth-requirements | benefits | discovery | validation", formalDefinition="Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified." ) 2616 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/eligibilityresponse-purpose") 2617 protected List<Enumeration<EligibilityResponsePurpose>> purpose; 2618 2619 /** 2620 * The party who is the beneficiary of the supplied coverage and for whom eligibility is sought. 2621 */ 2622 @Child(name = "patient", type = {Patient.class}, order=3, min=1, max=1, modifier=false, summary=true) 2623 @Description(shortDefinition="Intended recipient of products and services", formalDefinition="The party who is the beneficiary of the supplied coverage and for whom eligibility is sought." ) 2624 protected Reference patient; 2625 2626 /** 2627 * Information code for an event with a corresponding date or period. 2628 */ 2629 @Child(name = "event", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2630 @Description(shortDefinition="Event information", formalDefinition="Information code for an event with a corresponding date or period." ) 2631 protected List<CoverageEligibilityResponseEventComponent> event; 2632 2633 /** 2634 * The date or dates when the enclosed suite of services were performed or completed. 2635 */ 2636 @Child(name = "serviced", type = {DateType.class, Period.class}, order=5, min=0, max=1, modifier=false, summary=false) 2637 @Description(shortDefinition="Estimated date or dates of service", formalDefinition="The date or dates when the enclosed suite of services were performed or completed." ) 2638 protected DataType serviced; 2639 2640 /** 2641 * The date this resource was created. 2642 */ 2643 @Child(name = "created", type = {DateTimeType.class}, order=6, min=1, max=1, modifier=false, summary=true) 2644 @Description(shortDefinition="Response creation date", formalDefinition="The date this resource was created." ) 2645 protected DateTimeType created; 2646 2647 /** 2648 * The provider which is responsible for the request. 2649 */ 2650 @Child(name = "requestor", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=7, min=0, max=1, modifier=false, summary=false) 2651 @Description(shortDefinition="Party responsible for the request", formalDefinition="The provider which is responsible for the request." ) 2652 protected Reference requestor; 2653 2654 /** 2655 * Reference to the original request resource. 2656 */ 2657 @Child(name = "request", type = {CoverageEligibilityRequest.class}, order=8, min=1, max=1, modifier=false, summary=true) 2658 @Description(shortDefinition="Eligibility request reference", formalDefinition="Reference to the original request resource." ) 2659 protected Reference request; 2660 2661 /** 2662 * The outcome of the request processing. 2663 */ 2664 @Child(name = "outcome", type = {CodeType.class}, order=9, min=1, max=1, modifier=false, summary=true) 2665 @Description(shortDefinition="queued | complete | error | partial", formalDefinition="The outcome of the request processing." ) 2666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/eligibility-outcome") 2667 protected Enumeration<EligibilityOutcome> outcome; 2668 2669 /** 2670 * A human readable description of the status of the adjudication. 2671 */ 2672 @Child(name = "disposition", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 2673 @Description(shortDefinition="Disposition Message", formalDefinition="A human readable description of the status of the adjudication." ) 2674 protected StringType disposition; 2675 2676 /** 2677 * The Insurer who issued the coverage in question and is the author of the response. 2678 */ 2679 @Child(name = "insurer", type = {Organization.class}, order=11, min=1, max=1, modifier=false, summary=true) 2680 @Description(shortDefinition="Coverage issuer", formalDefinition="The Insurer who issued the coverage in question and is the author of the response." ) 2681 protected Reference insurer; 2682 2683 /** 2684 * Financial instruments for reimbursement for the health care products and services. 2685 */ 2686 @Child(name = "insurance", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2687 @Description(shortDefinition="Patient insurance information", formalDefinition="Financial instruments for reimbursement for the health care products and services." ) 2688 protected List<InsuranceComponent> insurance; 2689 2690 /** 2691 * A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred. 2692 */ 2693 @Child(name = "preAuthRef", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 2694 @Description(shortDefinition="Preauthorization reference", formalDefinition="A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred." ) 2695 protected StringType preAuthRef; 2696 2697 /** 2698 * A code for the form to be used for printing the content. 2699 */ 2700 @Child(name = "form", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 2701 @Description(shortDefinition="Printed form identifier", formalDefinition="A code for the form to be used for printing the content." ) 2702 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 2703 protected CodeableConcept form; 2704 2705 /** 2706 * Errors encountered during the processing of the request. 2707 */ 2708 @Child(name = "error", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2709 @Description(shortDefinition="Processing errors", formalDefinition="Errors encountered during the processing of the request." ) 2710 protected List<ErrorsComponent> error; 2711 2712 private static final long serialVersionUID = 1099912055L; 2713 2714 /** 2715 * Constructor 2716 */ 2717 public CoverageEligibilityResponse() { 2718 super(); 2719 } 2720 2721 /** 2722 * Constructor 2723 */ 2724 public CoverageEligibilityResponse(FinancialResourceStatusCodes status, EligibilityResponsePurpose purpose, Reference patient, Date created, Reference request, EligibilityOutcome outcome, Reference insurer) { 2725 super(); 2726 this.setStatus(status); 2727 this.addPurpose(purpose); 2728 this.setPatient(patient); 2729 this.setCreated(created); 2730 this.setRequest(request); 2731 this.setOutcome(outcome); 2732 this.setInsurer(insurer); 2733 } 2734 2735 /** 2736 * @return {@link #identifier} (A unique identifier assigned to this coverage eligiblity request.) 2737 */ 2738 public List<Identifier> getIdentifier() { 2739 if (this.identifier == null) 2740 this.identifier = new ArrayList<Identifier>(); 2741 return this.identifier; 2742 } 2743 2744 /** 2745 * @return Returns a reference to <code>this</code> for easy method chaining 2746 */ 2747 public CoverageEligibilityResponse setIdentifier(List<Identifier> theIdentifier) { 2748 this.identifier = theIdentifier; 2749 return this; 2750 } 2751 2752 public boolean hasIdentifier() { 2753 if (this.identifier == null) 2754 return false; 2755 for (Identifier item : this.identifier) 2756 if (!item.isEmpty()) 2757 return true; 2758 return false; 2759 } 2760 2761 public Identifier addIdentifier() { //3 2762 Identifier t = new Identifier(); 2763 if (this.identifier == null) 2764 this.identifier = new ArrayList<Identifier>(); 2765 this.identifier.add(t); 2766 return t; 2767 } 2768 2769 public CoverageEligibilityResponse addIdentifier(Identifier t) { //3 2770 if (t == null) 2771 return this; 2772 if (this.identifier == null) 2773 this.identifier = new ArrayList<Identifier>(); 2774 this.identifier.add(t); 2775 return this; 2776 } 2777 2778 /** 2779 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2780 */ 2781 public Identifier getIdentifierFirstRep() { 2782 if (getIdentifier().isEmpty()) { 2783 addIdentifier(); 2784 } 2785 return getIdentifier().get(0); 2786 } 2787 2788 /** 2789 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2790 */ 2791 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 2792 if (this.status == null) 2793 if (Configuration.errorOnAutoCreate()) 2794 throw new Error("Attempt to auto-create CoverageEligibilityResponse.status"); 2795 else if (Configuration.doAutoCreate()) 2796 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 2797 return this.status; 2798 } 2799 2800 public boolean hasStatusElement() { 2801 return this.status != null && !this.status.isEmpty(); 2802 } 2803 2804 public boolean hasStatus() { 2805 return this.status != null && !this.status.isEmpty(); 2806 } 2807 2808 /** 2809 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2810 */ 2811 public CoverageEligibilityResponse setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 2812 this.status = value; 2813 return this; 2814 } 2815 2816 /** 2817 * @return The status of the resource instance. 2818 */ 2819 public FinancialResourceStatusCodes getStatus() { 2820 return this.status == null ? null : this.status.getValue(); 2821 } 2822 2823 /** 2824 * @param value The status of the resource instance. 2825 */ 2826 public CoverageEligibilityResponse setStatus(FinancialResourceStatusCodes value) { 2827 if (this.status == null) 2828 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 2829 this.status.setValue(value); 2830 return this; 2831 } 2832 2833 /** 2834 * @return {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2835 */ 2836 public List<Enumeration<EligibilityResponsePurpose>> getPurpose() { 2837 if (this.purpose == null) 2838 this.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 2839 return this.purpose; 2840 } 2841 2842 /** 2843 * @return Returns a reference to <code>this</code> for easy method chaining 2844 */ 2845 public CoverageEligibilityResponse setPurpose(List<Enumeration<EligibilityResponsePurpose>> thePurpose) { 2846 this.purpose = thePurpose; 2847 return this; 2848 } 2849 2850 public boolean hasPurpose() { 2851 if (this.purpose == null) 2852 return false; 2853 for (Enumeration<EligibilityResponsePurpose> item : this.purpose) 2854 if (!item.isEmpty()) 2855 return true; 2856 return false; 2857 } 2858 2859 /** 2860 * @return {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2861 */ 2862 public Enumeration<EligibilityResponsePurpose> addPurposeElement() {//2 2863 Enumeration<EligibilityResponsePurpose> t = new Enumeration<EligibilityResponsePurpose>(new EligibilityResponsePurposeEnumFactory()); 2864 if (this.purpose == null) 2865 this.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 2866 this.purpose.add(t); 2867 return t; 2868 } 2869 2870 /** 2871 * @param value {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2872 */ 2873 public CoverageEligibilityResponse addPurpose(EligibilityResponsePurpose value) { //1 2874 Enumeration<EligibilityResponsePurpose> t = new Enumeration<EligibilityResponsePurpose>(new EligibilityResponsePurposeEnumFactory()); 2875 t.setValue(value); 2876 if (this.purpose == null) 2877 this.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 2878 this.purpose.add(t); 2879 return this; 2880 } 2881 2882 /** 2883 * @param value {@link #purpose} (Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.) 2884 */ 2885 public boolean hasPurpose(EligibilityResponsePurpose value) { 2886 if (this.purpose == null) 2887 return false; 2888 for (Enumeration<EligibilityResponsePurpose> v : this.purpose) 2889 if (v.getValue().equals(value)) // code 2890 return true; 2891 return false; 2892 } 2893 2894 /** 2895 * @return {@link #patient} (The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.) 2896 */ 2897 public Reference getPatient() { 2898 if (this.patient == null) 2899 if (Configuration.errorOnAutoCreate()) 2900 throw new Error("Attempt to auto-create CoverageEligibilityResponse.patient"); 2901 else if (Configuration.doAutoCreate()) 2902 this.patient = new Reference(); // cc 2903 return this.patient; 2904 } 2905 2906 public boolean hasPatient() { 2907 return this.patient != null && !this.patient.isEmpty(); 2908 } 2909 2910 /** 2911 * @param value {@link #patient} (The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.) 2912 */ 2913 public CoverageEligibilityResponse setPatient(Reference value) { 2914 this.patient = value; 2915 return this; 2916 } 2917 2918 /** 2919 * @return {@link #event} (Information code for an event with a corresponding date or period.) 2920 */ 2921 public List<CoverageEligibilityResponseEventComponent> getEvent() { 2922 if (this.event == null) 2923 this.event = new ArrayList<CoverageEligibilityResponseEventComponent>(); 2924 return this.event; 2925 } 2926 2927 /** 2928 * @return Returns a reference to <code>this</code> for easy method chaining 2929 */ 2930 public CoverageEligibilityResponse setEvent(List<CoverageEligibilityResponseEventComponent> theEvent) { 2931 this.event = theEvent; 2932 return this; 2933 } 2934 2935 public boolean hasEvent() { 2936 if (this.event == null) 2937 return false; 2938 for (CoverageEligibilityResponseEventComponent item : this.event) 2939 if (!item.isEmpty()) 2940 return true; 2941 return false; 2942 } 2943 2944 public CoverageEligibilityResponseEventComponent addEvent() { //3 2945 CoverageEligibilityResponseEventComponent t = new CoverageEligibilityResponseEventComponent(); 2946 if (this.event == null) 2947 this.event = new ArrayList<CoverageEligibilityResponseEventComponent>(); 2948 this.event.add(t); 2949 return t; 2950 } 2951 2952 public CoverageEligibilityResponse addEvent(CoverageEligibilityResponseEventComponent t) { //3 2953 if (t == null) 2954 return this; 2955 if (this.event == null) 2956 this.event = new ArrayList<CoverageEligibilityResponseEventComponent>(); 2957 this.event.add(t); 2958 return this; 2959 } 2960 2961 /** 2962 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 2963 */ 2964 public CoverageEligibilityResponseEventComponent getEventFirstRep() { 2965 if (getEvent().isEmpty()) { 2966 addEvent(); 2967 } 2968 return getEvent().get(0); 2969 } 2970 2971 /** 2972 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 2973 */ 2974 public DataType getServiced() { 2975 return this.serviced; 2976 } 2977 2978 /** 2979 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 2980 */ 2981 public DateType getServicedDateType() throws FHIRException { 2982 if (this.serviced == null) 2983 this.serviced = new DateType(); 2984 if (!(this.serviced instanceof DateType)) 2985 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 2986 return (DateType) this.serviced; 2987 } 2988 2989 public boolean hasServicedDateType() { 2990 return this.serviced instanceof DateType; 2991 } 2992 2993 /** 2994 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 2995 */ 2996 public Period getServicedPeriod() throws FHIRException { 2997 if (this.serviced == null) 2998 this.serviced = new Period(); 2999 if (!(this.serviced instanceof Period)) 3000 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 3001 return (Period) this.serviced; 3002 } 3003 3004 public boolean hasServicedPeriod() { 3005 return this.serviced instanceof Period; 3006 } 3007 3008 public boolean hasServiced() { 3009 return this.serviced != null && !this.serviced.isEmpty(); 3010 } 3011 3012 /** 3013 * @param value {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3014 */ 3015 public CoverageEligibilityResponse setServiced(DataType value) { 3016 if (value != null && !(value instanceof DateType || value instanceof Period)) 3017 throw new FHIRException("Not the right type for CoverageEligibilityResponse.serviced[x]: "+value.fhirType()); 3018 this.serviced = value; 3019 return this; 3020 } 3021 3022 /** 3023 * @return {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 3024 */ 3025 public DateTimeType getCreatedElement() { 3026 if (this.created == null) 3027 if (Configuration.errorOnAutoCreate()) 3028 throw new Error("Attempt to auto-create CoverageEligibilityResponse.created"); 3029 else if (Configuration.doAutoCreate()) 3030 this.created = new DateTimeType(); // bb 3031 return this.created; 3032 } 3033 3034 public boolean hasCreatedElement() { 3035 return this.created != null && !this.created.isEmpty(); 3036 } 3037 3038 public boolean hasCreated() { 3039 return this.created != null && !this.created.isEmpty(); 3040 } 3041 3042 /** 3043 * @param value {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 3044 */ 3045 public CoverageEligibilityResponse setCreatedElement(DateTimeType value) { 3046 this.created = value; 3047 return this; 3048 } 3049 3050 /** 3051 * @return The date this resource was created. 3052 */ 3053 public Date getCreated() { 3054 return this.created == null ? null : this.created.getValue(); 3055 } 3056 3057 /** 3058 * @param value The date this resource was created. 3059 */ 3060 public CoverageEligibilityResponse setCreated(Date value) { 3061 if (this.created == null) 3062 this.created = new DateTimeType(); 3063 this.created.setValue(value); 3064 return this; 3065 } 3066 3067 /** 3068 * @return {@link #requestor} (The provider which is responsible for the request.) 3069 */ 3070 public Reference getRequestor() { 3071 if (this.requestor == null) 3072 if (Configuration.errorOnAutoCreate()) 3073 throw new Error("Attempt to auto-create CoverageEligibilityResponse.requestor"); 3074 else if (Configuration.doAutoCreate()) 3075 this.requestor = new Reference(); // cc 3076 return this.requestor; 3077 } 3078 3079 public boolean hasRequestor() { 3080 return this.requestor != null && !this.requestor.isEmpty(); 3081 } 3082 3083 /** 3084 * @param value {@link #requestor} (The provider which is responsible for the request.) 3085 */ 3086 public CoverageEligibilityResponse setRequestor(Reference value) { 3087 this.requestor = value; 3088 return this; 3089 } 3090 3091 /** 3092 * @return {@link #request} (Reference to the original request resource.) 3093 */ 3094 public Reference getRequest() { 3095 if (this.request == null) 3096 if (Configuration.errorOnAutoCreate()) 3097 throw new Error("Attempt to auto-create CoverageEligibilityResponse.request"); 3098 else if (Configuration.doAutoCreate()) 3099 this.request = new Reference(); // cc 3100 return this.request; 3101 } 3102 3103 public boolean hasRequest() { 3104 return this.request != null && !this.request.isEmpty(); 3105 } 3106 3107 /** 3108 * @param value {@link #request} (Reference to the original request resource.) 3109 */ 3110 public CoverageEligibilityResponse setRequest(Reference value) { 3111 this.request = value; 3112 return this; 3113 } 3114 3115 /** 3116 * @return {@link #outcome} (The outcome of the request processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 3117 */ 3118 public Enumeration<EligibilityOutcome> getOutcomeElement() { 3119 if (this.outcome == null) 3120 if (Configuration.errorOnAutoCreate()) 3121 throw new Error("Attempt to auto-create CoverageEligibilityResponse.outcome"); 3122 else if (Configuration.doAutoCreate()) 3123 this.outcome = new Enumeration<EligibilityOutcome>(new EligibilityOutcomeEnumFactory()); // bb 3124 return this.outcome; 3125 } 3126 3127 public boolean hasOutcomeElement() { 3128 return this.outcome != null && !this.outcome.isEmpty(); 3129 } 3130 3131 public boolean hasOutcome() { 3132 return this.outcome != null && !this.outcome.isEmpty(); 3133 } 3134 3135 /** 3136 * @param value {@link #outcome} (The outcome of the request processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 3137 */ 3138 public CoverageEligibilityResponse setOutcomeElement(Enumeration<EligibilityOutcome> value) { 3139 this.outcome = value; 3140 return this; 3141 } 3142 3143 /** 3144 * @return The outcome of the request processing. 3145 */ 3146 public EligibilityOutcome getOutcome() { 3147 return this.outcome == null ? null : this.outcome.getValue(); 3148 } 3149 3150 /** 3151 * @param value The outcome of the request processing. 3152 */ 3153 public CoverageEligibilityResponse setOutcome(EligibilityOutcome value) { 3154 if (this.outcome == null) 3155 this.outcome = new Enumeration<EligibilityOutcome>(new EligibilityOutcomeEnumFactory()); 3156 this.outcome.setValue(value); 3157 return this; 3158 } 3159 3160 /** 3161 * @return {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 3162 */ 3163 public StringType getDispositionElement() { 3164 if (this.disposition == null) 3165 if (Configuration.errorOnAutoCreate()) 3166 throw new Error("Attempt to auto-create CoverageEligibilityResponse.disposition"); 3167 else if (Configuration.doAutoCreate()) 3168 this.disposition = new StringType(); // bb 3169 return this.disposition; 3170 } 3171 3172 public boolean hasDispositionElement() { 3173 return this.disposition != null && !this.disposition.isEmpty(); 3174 } 3175 3176 public boolean hasDisposition() { 3177 return this.disposition != null && !this.disposition.isEmpty(); 3178 } 3179 3180 /** 3181 * @param value {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 3182 */ 3183 public CoverageEligibilityResponse setDispositionElement(StringType value) { 3184 this.disposition = value; 3185 return this; 3186 } 3187 3188 /** 3189 * @return A human readable description of the status of the adjudication. 3190 */ 3191 public String getDisposition() { 3192 return this.disposition == null ? null : this.disposition.getValue(); 3193 } 3194 3195 /** 3196 * @param value A human readable description of the status of the adjudication. 3197 */ 3198 public CoverageEligibilityResponse setDisposition(String value) { 3199 if (Utilities.noString(value)) 3200 this.disposition = null; 3201 else { 3202 if (this.disposition == null) 3203 this.disposition = new StringType(); 3204 this.disposition.setValue(value); 3205 } 3206 return this; 3207 } 3208 3209 /** 3210 * @return {@link #insurer} (The Insurer who issued the coverage in question and is the author of the response.) 3211 */ 3212 public Reference getInsurer() { 3213 if (this.insurer == null) 3214 if (Configuration.errorOnAutoCreate()) 3215 throw new Error("Attempt to auto-create CoverageEligibilityResponse.insurer"); 3216 else if (Configuration.doAutoCreate()) 3217 this.insurer = new Reference(); // cc 3218 return this.insurer; 3219 } 3220 3221 public boolean hasInsurer() { 3222 return this.insurer != null && !this.insurer.isEmpty(); 3223 } 3224 3225 /** 3226 * @param value {@link #insurer} (The Insurer who issued the coverage in question and is the author of the response.) 3227 */ 3228 public CoverageEligibilityResponse setInsurer(Reference value) { 3229 this.insurer = value; 3230 return this; 3231 } 3232 3233 /** 3234 * @return {@link #insurance} (Financial instruments for reimbursement for the health care products and services.) 3235 */ 3236 public List<InsuranceComponent> getInsurance() { 3237 if (this.insurance == null) 3238 this.insurance = new ArrayList<InsuranceComponent>(); 3239 return this.insurance; 3240 } 3241 3242 /** 3243 * @return Returns a reference to <code>this</code> for easy method chaining 3244 */ 3245 public CoverageEligibilityResponse setInsurance(List<InsuranceComponent> theInsurance) { 3246 this.insurance = theInsurance; 3247 return this; 3248 } 3249 3250 public boolean hasInsurance() { 3251 if (this.insurance == null) 3252 return false; 3253 for (InsuranceComponent item : this.insurance) 3254 if (!item.isEmpty()) 3255 return true; 3256 return false; 3257 } 3258 3259 public InsuranceComponent addInsurance() { //3 3260 InsuranceComponent t = new InsuranceComponent(); 3261 if (this.insurance == null) 3262 this.insurance = new ArrayList<InsuranceComponent>(); 3263 this.insurance.add(t); 3264 return t; 3265 } 3266 3267 public CoverageEligibilityResponse addInsurance(InsuranceComponent t) { //3 3268 if (t == null) 3269 return this; 3270 if (this.insurance == null) 3271 this.insurance = new ArrayList<InsuranceComponent>(); 3272 this.insurance.add(t); 3273 return this; 3274 } 3275 3276 /** 3277 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 3278 */ 3279 public InsuranceComponent getInsuranceFirstRep() { 3280 if (getInsurance().isEmpty()) { 3281 addInsurance(); 3282 } 3283 return getInsurance().get(0); 3284 } 3285 3286 /** 3287 * @return {@link #preAuthRef} (A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 3288 */ 3289 public StringType getPreAuthRefElement() { 3290 if (this.preAuthRef == null) 3291 if (Configuration.errorOnAutoCreate()) 3292 throw new Error("Attempt to auto-create CoverageEligibilityResponse.preAuthRef"); 3293 else if (Configuration.doAutoCreate()) 3294 this.preAuthRef = new StringType(); // bb 3295 return this.preAuthRef; 3296 } 3297 3298 public boolean hasPreAuthRefElement() { 3299 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 3300 } 3301 3302 public boolean hasPreAuthRef() { 3303 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 3304 } 3305 3306 /** 3307 * @param value {@link #preAuthRef} (A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 3308 */ 3309 public CoverageEligibilityResponse setPreAuthRefElement(StringType value) { 3310 this.preAuthRef = value; 3311 return this; 3312 } 3313 3314 /** 3315 * @return A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred. 3316 */ 3317 public String getPreAuthRef() { 3318 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 3319 } 3320 3321 /** 3322 * @param value A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred. 3323 */ 3324 public CoverageEligibilityResponse setPreAuthRef(String value) { 3325 if (Utilities.noString(value)) 3326 this.preAuthRef = null; 3327 else { 3328 if (this.preAuthRef == null) 3329 this.preAuthRef = new StringType(); 3330 this.preAuthRef.setValue(value); 3331 } 3332 return this; 3333 } 3334 3335 /** 3336 * @return {@link #form} (A code for the form to be used for printing the content.) 3337 */ 3338 public CodeableConcept getForm() { 3339 if (this.form == null) 3340 if (Configuration.errorOnAutoCreate()) 3341 throw new Error("Attempt to auto-create CoverageEligibilityResponse.form"); 3342 else if (Configuration.doAutoCreate()) 3343 this.form = new CodeableConcept(); // cc 3344 return this.form; 3345 } 3346 3347 public boolean hasForm() { 3348 return this.form != null && !this.form.isEmpty(); 3349 } 3350 3351 /** 3352 * @param value {@link #form} (A code for the form to be used for printing the content.) 3353 */ 3354 public CoverageEligibilityResponse setForm(CodeableConcept value) { 3355 this.form = value; 3356 return this; 3357 } 3358 3359 /** 3360 * @return {@link #error} (Errors encountered during the processing of the request.) 3361 */ 3362 public List<ErrorsComponent> getError() { 3363 if (this.error == null) 3364 this.error = new ArrayList<ErrorsComponent>(); 3365 return this.error; 3366 } 3367 3368 /** 3369 * @return Returns a reference to <code>this</code> for easy method chaining 3370 */ 3371 public CoverageEligibilityResponse setError(List<ErrorsComponent> theError) { 3372 this.error = theError; 3373 return this; 3374 } 3375 3376 public boolean hasError() { 3377 if (this.error == null) 3378 return false; 3379 for (ErrorsComponent item : this.error) 3380 if (!item.isEmpty()) 3381 return true; 3382 return false; 3383 } 3384 3385 public ErrorsComponent addError() { //3 3386 ErrorsComponent t = new ErrorsComponent(); 3387 if (this.error == null) 3388 this.error = new ArrayList<ErrorsComponent>(); 3389 this.error.add(t); 3390 return t; 3391 } 3392 3393 public CoverageEligibilityResponse addError(ErrorsComponent t) { //3 3394 if (t == null) 3395 return this; 3396 if (this.error == null) 3397 this.error = new ArrayList<ErrorsComponent>(); 3398 this.error.add(t); 3399 return this; 3400 } 3401 3402 /** 3403 * @return The first repetition of repeating field {@link #error}, creating it if it does not already exist {3} 3404 */ 3405 public ErrorsComponent getErrorFirstRep() { 3406 if (getError().isEmpty()) { 3407 addError(); 3408 } 3409 return getError().get(0); 3410 } 3411 3412 protected void listChildren(List<Property> children) { 3413 super.listChildren(children); 3414 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this coverage eligiblity request.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3415 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 3416 children.add(new Property("purpose", "code", "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 0, java.lang.Integer.MAX_VALUE, purpose)); 3417 children.add(new Property("patient", "Reference(Patient)", "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, patient)); 3418 children.add(new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event)); 3419 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 3420 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 3421 children.add(new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the request.", 0, 1, requestor)); 3422 children.add(new Property("request", "Reference(CoverageEligibilityRequest)", "Reference to the original request resource.", 0, 1, request)); 3423 children.add(new Property("outcome", "code", "The outcome of the request processing.", 0, 1, outcome)); 3424 children.add(new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition)); 3425 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who issued the coverage in question and is the author of the response.", 0, 1, insurer)); 3426 children.add(new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services.", 0, java.lang.Integer.MAX_VALUE, insurance)); 3427 children.add(new Property("preAuthRef", "string", "A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred.", 0, 1, preAuthRef)); 3428 children.add(new Property("form", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, form)); 3429 children.add(new Property("error", "", "Errors encountered during the processing of the request.", 0, java.lang.Integer.MAX_VALUE, error)); 3430 } 3431 3432 @Override 3433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3434 switch (_hash) { 3435 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this coverage eligiblity request.", 0, java.lang.Integer.MAX_VALUE, identifier); 3436 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 3437 case -220463842: /*purpose*/ return new Property("purpose", "code", "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 0, java.lang.Integer.MAX_VALUE, purpose); 3438 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, patient); 3439 case 96891546: /*event*/ return new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event); 3440 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3441 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3442 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3443 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3444 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 3445 case 693934258: /*requestor*/ return new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the request.", 0, 1, requestor); 3446 case 1095692943: /*request*/ return new Property("request", "Reference(CoverageEligibilityRequest)", "Reference to the original request resource.", 0, 1, request); 3447 case -1106507950: /*outcome*/ return new Property("outcome", "code", "The outcome of the request processing.", 0, 1, outcome); 3448 case 583380919: /*disposition*/ return new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition); 3449 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who issued the coverage in question and is the author of the response.", 0, 1, insurer); 3450 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services.", 0, java.lang.Integer.MAX_VALUE, insurance); 3451 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred.", 0, 1, preAuthRef); 3452 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, form); 3453 case 96784904: /*error*/ return new Property("error", "", "Errors encountered during the processing of the request.", 0, java.lang.Integer.MAX_VALUE, error); 3454 default: return super.getNamedProperty(_hash, _name, _checkValid); 3455 } 3456 3457 } 3458 3459 @Override 3460 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3461 switch (hash) { 3462 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3463 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 3464 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Enumeration<EligibilityResponsePurpose> 3465 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 3466 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CoverageEligibilityResponseEventComponent 3467 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 3468 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 3469 case 693934258: /*requestor*/ return this.requestor == null ? new Base[0] : new Base[] {this.requestor}; // Reference 3470 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 3471 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Enumeration<EligibilityOutcome> 3472 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 3473 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 3474 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 3475 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : new Base[] {this.preAuthRef}; // StringType 3476 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 3477 case 96784904: /*error*/ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // ErrorsComponent 3478 default: return super.getProperty(hash, name, checkValid); 3479 } 3480 3481 } 3482 3483 @Override 3484 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3485 switch (hash) { 3486 case -1618432855: // identifier 3487 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3488 return value; 3489 case -892481550: // status 3490 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 3491 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 3492 return value; 3493 case -220463842: // purpose 3494 value = new EligibilityResponsePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3495 this.getPurpose().add((Enumeration) value); // Enumeration<EligibilityResponsePurpose> 3496 return value; 3497 case -791418107: // patient 3498 this.patient = TypeConvertor.castToReference(value); // Reference 3499 return value; 3500 case 96891546: // event 3501 this.getEvent().add((CoverageEligibilityResponseEventComponent) value); // CoverageEligibilityResponseEventComponent 3502 return value; 3503 case 1379209295: // serviced 3504 this.serviced = TypeConvertor.castToType(value); // DataType 3505 return value; 3506 case 1028554472: // created 3507 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 3508 return value; 3509 case 693934258: // requestor 3510 this.requestor = TypeConvertor.castToReference(value); // Reference 3511 return value; 3512 case 1095692943: // request 3513 this.request = TypeConvertor.castToReference(value); // Reference 3514 return value; 3515 case -1106507950: // outcome 3516 value = new EligibilityOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3517 this.outcome = (Enumeration) value; // Enumeration<EligibilityOutcome> 3518 return value; 3519 case 583380919: // disposition 3520 this.disposition = TypeConvertor.castToString(value); // StringType 3521 return value; 3522 case 1957615864: // insurer 3523 this.insurer = TypeConvertor.castToReference(value); // Reference 3524 return value; 3525 case 73049818: // insurance 3526 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 3527 return value; 3528 case 522246568: // preAuthRef 3529 this.preAuthRef = TypeConvertor.castToString(value); // StringType 3530 return value; 3531 case 3148996: // form 3532 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3533 return value; 3534 case 96784904: // error 3535 this.getError().add((ErrorsComponent) value); // ErrorsComponent 3536 return value; 3537 default: return super.setProperty(hash, name, value); 3538 } 3539 3540 } 3541 3542 @Override 3543 public Base setProperty(String name, Base value) throws FHIRException { 3544 if (name.equals("identifier")) { 3545 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3546 } else if (name.equals("status")) { 3547 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 3548 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 3549 } else if (name.equals("purpose")) { 3550 value = new EligibilityResponsePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3551 this.getPurpose().add((Enumeration) value); 3552 } else if (name.equals("patient")) { 3553 this.patient = TypeConvertor.castToReference(value); // Reference 3554 } else if (name.equals("event")) { 3555 this.getEvent().add((CoverageEligibilityResponseEventComponent) value); 3556 } else if (name.equals("serviced[x]")) { 3557 this.serviced = TypeConvertor.castToType(value); // DataType 3558 } else if (name.equals("created")) { 3559 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 3560 } else if (name.equals("requestor")) { 3561 this.requestor = TypeConvertor.castToReference(value); // Reference 3562 } else if (name.equals("request")) { 3563 this.request = TypeConvertor.castToReference(value); // Reference 3564 } else if (name.equals("outcome")) { 3565 value = new EligibilityOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3566 this.outcome = (Enumeration) value; // Enumeration<EligibilityOutcome> 3567 } else if (name.equals("disposition")) { 3568 this.disposition = TypeConvertor.castToString(value); // StringType 3569 } else if (name.equals("insurer")) { 3570 this.insurer = TypeConvertor.castToReference(value); // Reference 3571 } else if (name.equals("insurance")) { 3572 this.getInsurance().add((InsuranceComponent) value); 3573 } else if (name.equals("preAuthRef")) { 3574 this.preAuthRef = TypeConvertor.castToString(value); // StringType 3575 } else if (name.equals("form")) { 3576 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3577 } else if (name.equals("error")) { 3578 this.getError().add((ErrorsComponent) value); 3579 } else 3580 return super.setProperty(name, value); 3581 return value; 3582 } 3583 3584 @Override 3585 public void removeChild(String name, Base value) throws FHIRException { 3586 if (name.equals("identifier")) { 3587 this.getIdentifier().remove(value); 3588 } else if (name.equals("status")) { 3589 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 3590 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 3591 } else if (name.equals("purpose")) { 3592 value = new EligibilityResponsePurposeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3593 this.getPurpose().remove((Enumeration) value); 3594 } else if (name.equals("patient")) { 3595 this.patient = null; 3596 } else if (name.equals("event")) { 3597 this.getEvent().remove((CoverageEligibilityResponseEventComponent) value); 3598 } else if (name.equals("serviced[x]")) { 3599 this.serviced = null; 3600 } else if (name.equals("created")) { 3601 this.created = null; 3602 } else if (name.equals("requestor")) { 3603 this.requestor = null; 3604 } else if (name.equals("request")) { 3605 this.request = null; 3606 } else if (name.equals("outcome")) { 3607 value = new EligibilityOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3608 this.outcome = (Enumeration) value; // Enumeration<EligibilityOutcome> 3609 } else if (name.equals("disposition")) { 3610 this.disposition = null; 3611 } else if (name.equals("insurer")) { 3612 this.insurer = null; 3613 } else if (name.equals("insurance")) { 3614 this.getInsurance().remove((InsuranceComponent) value); 3615 } else if (name.equals("preAuthRef")) { 3616 this.preAuthRef = null; 3617 } else if (name.equals("form")) { 3618 this.form = null; 3619 } else if (name.equals("error")) { 3620 this.getError().remove((ErrorsComponent) value); 3621 } else 3622 super.removeChild(name, value); 3623 3624 } 3625 3626 @Override 3627 public Base makeProperty(int hash, String name) throws FHIRException { 3628 switch (hash) { 3629 case -1618432855: return addIdentifier(); 3630 case -892481550: return getStatusElement(); 3631 case -220463842: return addPurposeElement(); 3632 case -791418107: return getPatient(); 3633 case 96891546: return addEvent(); 3634 case -1927922223: return getServiced(); 3635 case 1379209295: return getServiced(); 3636 case 1028554472: return getCreatedElement(); 3637 case 693934258: return getRequestor(); 3638 case 1095692943: return getRequest(); 3639 case -1106507950: return getOutcomeElement(); 3640 case 583380919: return getDispositionElement(); 3641 case 1957615864: return getInsurer(); 3642 case 73049818: return addInsurance(); 3643 case 522246568: return getPreAuthRefElement(); 3644 case 3148996: return getForm(); 3645 case 96784904: return addError(); 3646 default: return super.makeProperty(hash, name); 3647 } 3648 3649 } 3650 3651 @Override 3652 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3653 switch (hash) { 3654 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3655 case -892481550: /*status*/ return new String[] {"code"}; 3656 case -220463842: /*purpose*/ return new String[] {"code"}; 3657 case -791418107: /*patient*/ return new String[] {"Reference"}; 3658 case 96891546: /*event*/ return new String[] {}; 3659 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 3660 case 1028554472: /*created*/ return new String[] {"dateTime"}; 3661 case 693934258: /*requestor*/ return new String[] {"Reference"}; 3662 case 1095692943: /*request*/ return new String[] {"Reference"}; 3663 case -1106507950: /*outcome*/ return new String[] {"code"}; 3664 case 583380919: /*disposition*/ return new String[] {"string"}; 3665 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 3666 case 73049818: /*insurance*/ return new String[] {}; 3667 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 3668 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 3669 case 96784904: /*error*/ return new String[] {}; 3670 default: return super.getTypesForProperty(hash, name); 3671 } 3672 3673 } 3674 3675 @Override 3676 public Base addChild(String name) throws FHIRException { 3677 if (name.equals("identifier")) { 3678 return addIdentifier(); 3679 } 3680 else if (name.equals("status")) { 3681 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.status"); 3682 } 3683 else if (name.equals("purpose")) { 3684 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.purpose"); 3685 } 3686 else if (name.equals("patient")) { 3687 this.patient = new Reference(); 3688 return this.patient; 3689 } 3690 else if (name.equals("event")) { 3691 return addEvent(); 3692 } 3693 else if (name.equals("servicedDate")) { 3694 this.serviced = new DateType(); 3695 return this.serviced; 3696 } 3697 else if (name.equals("servicedPeriod")) { 3698 this.serviced = new Period(); 3699 return this.serviced; 3700 } 3701 else if (name.equals("created")) { 3702 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.created"); 3703 } 3704 else if (name.equals("requestor")) { 3705 this.requestor = new Reference(); 3706 return this.requestor; 3707 } 3708 else if (name.equals("request")) { 3709 this.request = new Reference(); 3710 return this.request; 3711 } 3712 else if (name.equals("outcome")) { 3713 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.outcome"); 3714 } 3715 else if (name.equals("disposition")) { 3716 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.disposition"); 3717 } 3718 else if (name.equals("insurer")) { 3719 this.insurer = new Reference(); 3720 return this.insurer; 3721 } 3722 else if (name.equals("insurance")) { 3723 return addInsurance(); 3724 } 3725 else if (name.equals("preAuthRef")) { 3726 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.preAuthRef"); 3727 } 3728 else if (name.equals("form")) { 3729 this.form = new CodeableConcept(); 3730 return this.form; 3731 } 3732 else if (name.equals("error")) { 3733 return addError(); 3734 } 3735 else 3736 return super.addChild(name); 3737 } 3738 3739 public String fhirType() { 3740 return "CoverageEligibilityResponse"; 3741 3742 } 3743 3744 public CoverageEligibilityResponse copy() { 3745 CoverageEligibilityResponse dst = new CoverageEligibilityResponse(); 3746 copyValues(dst); 3747 return dst; 3748 } 3749 3750 public void copyValues(CoverageEligibilityResponse dst) { 3751 super.copyValues(dst); 3752 if (identifier != null) { 3753 dst.identifier = new ArrayList<Identifier>(); 3754 for (Identifier i : identifier) 3755 dst.identifier.add(i.copy()); 3756 }; 3757 dst.status = status == null ? null : status.copy(); 3758 if (purpose != null) { 3759 dst.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 3760 for (Enumeration<EligibilityResponsePurpose> i : purpose) 3761 dst.purpose.add(i.copy()); 3762 }; 3763 dst.patient = patient == null ? null : patient.copy(); 3764 if (event != null) { 3765 dst.event = new ArrayList<CoverageEligibilityResponseEventComponent>(); 3766 for (CoverageEligibilityResponseEventComponent i : event) 3767 dst.event.add(i.copy()); 3768 }; 3769 dst.serviced = serviced == null ? null : serviced.copy(); 3770 dst.created = created == null ? null : created.copy(); 3771 dst.requestor = requestor == null ? null : requestor.copy(); 3772 dst.request = request == null ? null : request.copy(); 3773 dst.outcome = outcome == null ? null : outcome.copy(); 3774 dst.disposition = disposition == null ? null : disposition.copy(); 3775 dst.insurer = insurer == null ? null : insurer.copy(); 3776 if (insurance != null) { 3777 dst.insurance = new ArrayList<InsuranceComponent>(); 3778 for (InsuranceComponent i : insurance) 3779 dst.insurance.add(i.copy()); 3780 }; 3781 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 3782 dst.form = form == null ? null : form.copy(); 3783 if (error != null) { 3784 dst.error = new ArrayList<ErrorsComponent>(); 3785 for (ErrorsComponent i : error) 3786 dst.error.add(i.copy()); 3787 }; 3788 } 3789 3790 protected CoverageEligibilityResponse typedCopy() { 3791 return copy(); 3792 } 3793 3794 @Override 3795 public boolean equalsDeep(Base other_) { 3796 if (!super.equalsDeep(other_)) 3797 return false; 3798 if (!(other_ instanceof CoverageEligibilityResponse)) 3799 return false; 3800 CoverageEligibilityResponse o = (CoverageEligibilityResponse) other_; 3801 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(purpose, o.purpose, true) 3802 && compareDeep(patient, o.patient, true) && compareDeep(event, o.event, true) && compareDeep(serviced, o.serviced, true) 3803 && compareDeep(created, o.created, true) && compareDeep(requestor, o.requestor, true) && compareDeep(request, o.request, true) 3804 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) && compareDeep(insurer, o.insurer, true) 3805 && compareDeep(insurance, o.insurance, true) && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(form, o.form, true) 3806 && compareDeep(error, o.error, true); 3807 } 3808 3809 @Override 3810 public boolean equalsShallow(Base other_) { 3811 if (!super.equalsShallow(other_)) 3812 return false; 3813 if (!(other_ instanceof CoverageEligibilityResponse)) 3814 return false; 3815 CoverageEligibilityResponse o = (CoverageEligibilityResponse) other_; 3816 return compareValues(status, o.status, true) && compareValues(purpose, o.purpose, true) && compareValues(created, o.created, true) 3817 && compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true) 3818 ; 3819 } 3820 3821 public boolean isEmpty() { 3822 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, purpose 3823 , patient, event, serviced, created, requestor, request, outcome, disposition 3824 , insurer, insurance, preAuthRef, form, error); 3825 } 3826 3827 @Override 3828 public ResourceType getResourceType() { 3829 return ResourceType.CoverageEligibilityResponse; 3830 } 3831 3832 /** 3833 * Search parameter: <b>created</b> 3834 * <p> 3835 * Description: <b>The creation date</b><br> 3836 * Type: <b>date</b><br> 3837 * Path: <b>CoverageEligibilityResponse.created</b><br> 3838 * </p> 3839 */ 3840 @SearchParamDefinition(name="created", path="CoverageEligibilityResponse.created", description="The creation date", type="date" ) 3841 public static final String SP_CREATED = "created"; 3842 /** 3843 * <b>Fluent Client</b> search parameter constant for <b>created</b> 3844 * <p> 3845 * Description: <b>The creation date</b><br> 3846 * Type: <b>date</b><br> 3847 * Path: <b>CoverageEligibilityResponse.created</b><br> 3848 * </p> 3849 */ 3850 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 3851 3852 /** 3853 * Search parameter: <b>disposition</b> 3854 * <p> 3855 * Description: <b>The contents of the disposition message</b><br> 3856 * Type: <b>string</b><br> 3857 * Path: <b>CoverageEligibilityResponse.disposition</b><br> 3858 * </p> 3859 */ 3860 @SearchParamDefinition(name="disposition", path="CoverageEligibilityResponse.disposition", description="The contents of the disposition message", type="string" ) 3861 public static final String SP_DISPOSITION = "disposition"; 3862 /** 3863 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 3864 * <p> 3865 * Description: <b>The contents of the disposition message</b><br> 3866 * Type: <b>string</b><br> 3867 * Path: <b>CoverageEligibilityResponse.disposition</b><br> 3868 * </p> 3869 */ 3870 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 3871 3872 /** 3873 * Search parameter: <b>insurer</b> 3874 * <p> 3875 * Description: <b>The organization which generated this resource</b><br> 3876 * Type: <b>reference</b><br> 3877 * Path: <b>CoverageEligibilityResponse.insurer</b><br> 3878 * </p> 3879 */ 3880 @SearchParamDefinition(name="insurer", path="CoverageEligibilityResponse.insurer", description="The organization which generated this resource", type="reference", target={Organization.class } ) 3881 public static final String SP_INSURER = "insurer"; 3882 /** 3883 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 3884 * <p> 3885 * Description: <b>The organization which generated this resource</b><br> 3886 * Type: <b>reference</b><br> 3887 * Path: <b>CoverageEligibilityResponse.insurer</b><br> 3888 * </p> 3889 */ 3890 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 3891 3892/** 3893 * Constant for fluent queries to be used to add include statements. Specifies 3894 * the path value of "<b>CoverageEligibilityResponse:insurer</b>". 3895 */ 3896 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("CoverageEligibilityResponse:insurer").toLocked(); 3897 3898 /** 3899 * Search parameter: <b>outcome</b> 3900 * <p> 3901 * Description: <b>The processing outcome</b><br> 3902 * Type: <b>token</b><br> 3903 * Path: <b>CoverageEligibilityResponse.outcome</b><br> 3904 * </p> 3905 */ 3906 @SearchParamDefinition(name="outcome", path="CoverageEligibilityResponse.outcome", description="The processing outcome", type="token" ) 3907 public static final String SP_OUTCOME = "outcome"; 3908 /** 3909 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 3910 * <p> 3911 * Description: <b>The processing outcome</b><br> 3912 * Type: <b>token</b><br> 3913 * Path: <b>CoverageEligibilityResponse.outcome</b><br> 3914 * </p> 3915 */ 3916 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 3917 3918 /** 3919 * Search parameter: <b>request</b> 3920 * <p> 3921 * Description: <b>The EligibilityRequest reference</b><br> 3922 * Type: <b>reference</b><br> 3923 * Path: <b>CoverageEligibilityResponse.request</b><br> 3924 * </p> 3925 */ 3926 @SearchParamDefinition(name="request", path="CoverageEligibilityResponse.request", description="The EligibilityRequest reference", type="reference", target={CoverageEligibilityRequest.class } ) 3927 public static final String SP_REQUEST = "request"; 3928 /** 3929 * <b>Fluent Client</b> search parameter constant for <b>request</b> 3930 * <p> 3931 * Description: <b>The EligibilityRequest reference</b><br> 3932 * Type: <b>reference</b><br> 3933 * Path: <b>CoverageEligibilityResponse.request</b><br> 3934 * </p> 3935 */ 3936 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 3937 3938/** 3939 * Constant for fluent queries to be used to add include statements. Specifies 3940 * the path value of "<b>CoverageEligibilityResponse:request</b>". 3941 */ 3942 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("CoverageEligibilityResponse:request").toLocked(); 3943 3944 /** 3945 * Search parameter: <b>requestor</b> 3946 * <p> 3947 * Description: <b>The EligibilityRequest provider</b><br> 3948 * Type: <b>reference</b><br> 3949 * Path: <b>CoverageEligibilityResponse.requestor</b><br> 3950 * </p> 3951 */ 3952 @SearchParamDefinition(name="requestor", path="CoverageEligibilityResponse.requestor", description="The EligibilityRequest provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 3953 public static final String SP_REQUESTOR = "requestor"; 3954 /** 3955 * <b>Fluent Client</b> search parameter constant for <b>requestor</b> 3956 * <p> 3957 * Description: <b>The EligibilityRequest provider</b><br> 3958 * Type: <b>reference</b><br> 3959 * Path: <b>CoverageEligibilityResponse.requestor</b><br> 3960 * </p> 3961 */ 3962 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTOR); 3963 3964/** 3965 * Constant for fluent queries to be used to add include statements. Specifies 3966 * the path value of "<b>CoverageEligibilityResponse:requestor</b>". 3967 */ 3968 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTOR = new ca.uhn.fhir.model.api.Include("CoverageEligibilityResponse:requestor").toLocked(); 3969 3970 /** 3971 * Search parameter: <b>status</b> 3972 * <p> 3973 * Description: <b>The EligibilityRequest status</b><br> 3974 * Type: <b>token</b><br> 3975 * Path: <b>CoverageEligibilityResponse.status</b><br> 3976 * </p> 3977 */ 3978 @SearchParamDefinition(name="status", path="CoverageEligibilityResponse.status", description="The EligibilityRequest status", type="token" ) 3979 public static final String SP_STATUS = "status"; 3980 /** 3981 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3982 * <p> 3983 * Description: <b>The EligibilityRequest status</b><br> 3984 * Type: <b>token</b><br> 3985 * Path: <b>CoverageEligibilityResponse.status</b><br> 3986 * </p> 3987 */ 3988 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3989 3990 /** 3991 * Search parameter: <b>identifier</b> 3992 * <p> 3993 * Description: <b>Multiple Resources: 3994 3995* [Account](account.html): Account number 3996* [AdverseEvent](adverseevent.html): Business identifier for the event 3997* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3998* [Appointment](appointment.html): An Identifier of the Appointment 3999* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4000* [Basic](basic.html): Business identifier 4001* [BodyStructure](bodystructure.html): Bodystructure identifier 4002* [CarePlan](careplan.html): External Ids for this plan 4003* [CareTeam](careteam.html): External Ids for this team 4004* [ChargeItem](chargeitem.html): Business Identifier for item 4005* [Claim](claim.html): The primary identifier of the financial resource 4006* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4007* [ClinicalImpression](clinicalimpression.html): Business identifier 4008* [Communication](communication.html): Unique identifier 4009* [CommunicationRequest](communicationrequest.html): Unique identifier 4010* [Composition](composition.html): Version-independent identifier for the Composition 4011* [Condition](condition.html): A unique identifier of the condition record 4012* [Consent](consent.html): Identifier for this record (external references) 4013* [Contract](contract.html): The identity of the contract 4014* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4015* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4016* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4017* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4018* [DeviceRequest](devicerequest.html): Business identifier for request/order 4019* [DeviceUsage](deviceusage.html): Search by identifier 4020* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4021* [DocumentReference](documentreference.html): Identifier of the attachment binary 4022* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4023* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4024* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4025* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4026* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4027* [Flag](flag.html): Business identifier 4028* [Goal](goal.html): External Ids for this goal 4029* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4030* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4031* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4032* [Immunization](immunization.html): Business identifier 4033* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4034* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4035* [Invoice](invoice.html): Business Identifier for item 4036* [List](list.html): Business identifier 4037* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4038* [Medication](medication.html): Returns medications with this external identifier 4039* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4040* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4041* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4042* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4043* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4044* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4045* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4046* [Observation](observation.html): The unique id for a particular observation 4047* [Person](person.html): A person Identifier 4048* [Procedure](procedure.html): A unique identifier for a procedure 4049* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4050* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4051* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4052* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4053* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4054* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4055* [Specimen](specimen.html): The unique identifier associated with the specimen 4056* [SupplyDelivery](supplydelivery.html): External identifier 4057* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4058* [Task](task.html): Search for a task instance by its business identifier 4059* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4060</b><br> 4061 * Type: <b>token</b><br> 4062 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4063 * </p> 4064 */ 4065 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4066 public static final String SP_IDENTIFIER = "identifier"; 4067 /** 4068 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4069 * <p> 4070 * Description: <b>Multiple Resources: 4071 4072* [Account](account.html): Account number 4073* [AdverseEvent](adverseevent.html): Business identifier for the event 4074* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4075* [Appointment](appointment.html): An Identifier of the Appointment 4076* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4077* [Basic](basic.html): Business identifier 4078* [BodyStructure](bodystructure.html): Bodystructure identifier 4079* [CarePlan](careplan.html): External Ids for this plan 4080* [CareTeam](careteam.html): External Ids for this team 4081* [ChargeItem](chargeitem.html): Business Identifier for item 4082* [Claim](claim.html): The primary identifier of the financial resource 4083* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4084* [ClinicalImpression](clinicalimpression.html): Business identifier 4085* [Communication](communication.html): Unique identifier 4086* [CommunicationRequest](communicationrequest.html): Unique identifier 4087* [Composition](composition.html): Version-independent identifier for the Composition 4088* [Condition](condition.html): A unique identifier of the condition record 4089* [Consent](consent.html): Identifier for this record (external references) 4090* [Contract](contract.html): The identity of the contract 4091* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4092* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4093* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4094* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4095* [DeviceRequest](devicerequest.html): Business identifier for request/order 4096* [DeviceUsage](deviceusage.html): Search by identifier 4097* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4098* [DocumentReference](documentreference.html): Identifier of the attachment binary 4099* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4100* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4101* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4102* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4103* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4104* [Flag](flag.html): Business identifier 4105* [Goal](goal.html): External Ids for this goal 4106* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4107* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4108* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4109* [Immunization](immunization.html): Business identifier 4110* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4111* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4112* [Invoice](invoice.html): Business Identifier for item 4113* [List](list.html): Business identifier 4114* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4115* [Medication](medication.html): Returns medications with this external identifier 4116* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4117* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4118* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4119* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4120* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4121* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4122* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4123* [Observation](observation.html): The unique id for a particular observation 4124* [Person](person.html): A person Identifier 4125* [Procedure](procedure.html): A unique identifier for a procedure 4126* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4127* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4128* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4129* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4130* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4131* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4132* [Specimen](specimen.html): The unique identifier associated with the specimen 4133* [SupplyDelivery](supplydelivery.html): External identifier 4134* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4135* [Task](task.html): Search for a task instance by its business identifier 4136* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4137</b><br> 4138 * Type: <b>token</b><br> 4139 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4140 * </p> 4141 */ 4142 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4143 4144 /** 4145 * Search parameter: <b>patient</b> 4146 * <p> 4147 * Description: <b>Multiple Resources: 4148 4149* [Account](account.html): The entity that caused the expenses 4150* [AdverseEvent](adverseevent.html): Subject impacted by event 4151* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4152* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4153* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4154* [AuditEvent](auditevent.html): Where the activity involved patient data 4155* [Basic](basic.html): Identifies the focus of this resource 4156* [BodyStructure](bodystructure.html): Who this is about 4157* [CarePlan](careplan.html): Who the care plan is for 4158* [CareTeam](careteam.html): Who care team is for 4159* [ChargeItem](chargeitem.html): Individual service was done for/to 4160* [Claim](claim.html): Patient receiving the products or services 4161* [ClaimResponse](claimresponse.html): The subject of care 4162* [ClinicalImpression](clinicalimpression.html): Patient assessed 4163* [Communication](communication.html): Focus of message 4164* [CommunicationRequest](communicationrequest.html): Focus of message 4165* [Composition](composition.html): Who and/or what the composition is about 4166* [Condition](condition.html): Who has the condition? 4167* [Consent](consent.html): Who the consent applies to 4168* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4169* [Coverage](coverage.html): Retrieve coverages for a patient 4170* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4171* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4172* [DetectedIssue](detectedissue.html): Associated patient 4173* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4174* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4175* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4176* [DocumentReference](documentreference.html): Who/what is the subject of the document 4177* [Encounter](encounter.html): The patient present at the encounter 4178* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4179* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4180* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4181* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4182* [Flag](flag.html): The identity of a subject to list flags for 4183* [Goal](goal.html): Who this goal is intended for 4184* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4185* [ImagingSelection](imagingselection.html): Who the study is about 4186* [ImagingStudy](imagingstudy.html): Who the study is about 4187* [Immunization](immunization.html): The patient for the vaccination record 4188* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4189* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4190* [Invoice](invoice.html): Recipient(s) of goods and services 4191* [List](list.html): If all resources have the same subject 4192* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4193* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4194* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4195* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4196* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4197* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4198* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4199* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4200* [Observation](observation.html): The subject that the observation is about (if patient) 4201* [Person](person.html): The Person links to this Patient 4202* [Procedure](procedure.html): Search by subject - a patient 4203* [Provenance](provenance.html): Where the activity involved patient data 4204* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4205* [RelatedPerson](relatedperson.html): The patient this related person is related to 4206* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4207* [ResearchSubject](researchsubject.html): Who or what is part of study 4208* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4209* [ServiceRequest](servicerequest.html): Search by subject - a patient 4210* [Specimen](specimen.html): The patient the specimen comes from 4211* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4212* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4213* [Task](task.html): Search by patient 4214* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4215</b><br> 4216 * Type: <b>reference</b><br> 4217 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4218 * </p> 4219 */ 4220 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 4221 public static final String SP_PATIENT = "patient"; 4222 /** 4223 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4224 * <p> 4225 * Description: <b>Multiple Resources: 4226 4227* [Account](account.html): The entity that caused the expenses 4228* [AdverseEvent](adverseevent.html): Subject impacted by event 4229* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4230* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4231* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4232* [AuditEvent](auditevent.html): Where the activity involved patient data 4233* [Basic](basic.html): Identifies the focus of this resource 4234* [BodyStructure](bodystructure.html): Who this is about 4235* [CarePlan](careplan.html): Who the care plan is for 4236* [CareTeam](careteam.html): Who care team is for 4237* [ChargeItem](chargeitem.html): Individual service was done for/to 4238* [Claim](claim.html): Patient receiving the products or services 4239* [ClaimResponse](claimresponse.html): The subject of care 4240* [ClinicalImpression](clinicalimpression.html): Patient assessed 4241* [Communication](communication.html): Focus of message 4242* [CommunicationRequest](communicationrequest.html): Focus of message 4243* [Composition](composition.html): Who and/or what the composition is about 4244* [Condition](condition.html): Who has the condition? 4245* [Consent](consent.html): Who the consent applies to 4246* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4247* [Coverage](coverage.html): Retrieve coverages for a patient 4248* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4249* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4250* [DetectedIssue](detectedissue.html): Associated patient 4251* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4252* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4253* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4254* [DocumentReference](documentreference.html): Who/what is the subject of the document 4255* [Encounter](encounter.html): The patient present at the encounter 4256* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4257* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4258* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4259* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4260* [Flag](flag.html): The identity of a subject to list flags for 4261* [Goal](goal.html): Who this goal is intended for 4262* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4263* [ImagingSelection](imagingselection.html): Who the study is about 4264* [ImagingStudy](imagingstudy.html): Who the study is about 4265* [Immunization](immunization.html): The patient for the vaccination record 4266* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4267* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4268* [Invoice](invoice.html): Recipient(s) of goods and services 4269* [List](list.html): If all resources have the same subject 4270* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4271* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4272* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4273* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4274* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4275* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4276* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4277* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4278* [Observation](observation.html): The subject that the observation is about (if patient) 4279* [Person](person.html): The Person links to this Patient 4280* [Procedure](procedure.html): Search by subject - a patient 4281* [Provenance](provenance.html): Where the activity involved patient data 4282* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4283* [RelatedPerson](relatedperson.html): The patient this related person is related to 4284* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4285* [ResearchSubject](researchsubject.html): Who or what is part of study 4286* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4287* [ServiceRequest](servicerequest.html): Search by subject - a patient 4288* [Specimen](specimen.html): The patient the specimen comes from 4289* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4290* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4291* [Task](task.html): Search by patient 4292* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4293</b><br> 4294 * Type: <b>reference</b><br> 4295 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4296 * </p> 4297 */ 4298 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4299 4300/** 4301 * Constant for fluent queries to be used to add include statements. Specifies 4302 * the path value of "<b>CoverageEligibilityResponse:patient</b>". 4303 */ 4304 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("CoverageEligibilityResponse:patient").toLocked(); 4305 4306 4307} 4308