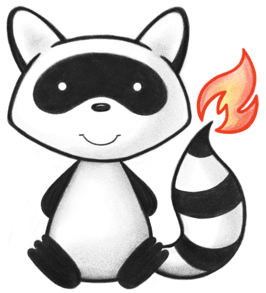
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * DataRequirement Type: Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 050 */ 051@DatatypeDef(name="DataRequirement") 052public class DataRequirement extends DataType implements ICompositeType { 053 054 public enum SortDirection { 055 /** 056 * Sort by the value ascending, so that lower values appear first. 057 */ 058 ASCENDING, 059 /** 060 * Sort by the value descending, so that lower values appear last. 061 */ 062 DESCENDING, 063 /** 064 * added to help the parsers with the generic types 065 */ 066 NULL; 067 public static SortDirection fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("ascending".equals(codeString)) 071 return ASCENDING; 072 if ("descending".equals(codeString)) 073 return DESCENDING; 074 if (Configuration.isAcceptInvalidEnums()) 075 return null; 076 else 077 throw new FHIRException("Unknown SortDirection code '"+codeString+"'"); 078 } 079 public String toCode() { 080 switch (this) { 081 case ASCENDING: return "ascending"; 082 case DESCENDING: return "descending"; 083 case NULL: return null; 084 default: return "?"; 085 } 086 } 087 public String getSystem() { 088 switch (this) { 089 case ASCENDING: return "http://hl7.org/fhir/sort-direction"; 090 case DESCENDING: return "http://hl7.org/fhir/sort-direction"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getDefinition() { 096 switch (this) { 097 case ASCENDING: return "Sort by the value ascending, so that lower values appear first."; 098 case DESCENDING: return "Sort by the value descending, so that lower values appear last."; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDisplay() { 104 switch (this) { 105 case ASCENDING: return "Ascending"; 106 case DESCENDING: return "Descending"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 } 112 113 public static class SortDirectionEnumFactory implements EnumFactory<SortDirection> { 114 public SortDirection fromCode(String codeString) throws IllegalArgumentException { 115 if (codeString == null || "".equals(codeString)) 116 if (codeString == null || "".equals(codeString)) 117 return null; 118 if ("ascending".equals(codeString)) 119 return SortDirection.ASCENDING; 120 if ("descending".equals(codeString)) 121 return SortDirection.DESCENDING; 122 throw new IllegalArgumentException("Unknown SortDirection code '"+codeString+"'"); 123 } 124 public Enumeration<SortDirection> fromType(PrimitiveType<?> code) throws FHIRException { 125 if (code == null) 126 return null; 127 if (code.isEmpty()) 128 return new Enumeration<SortDirection>(this, SortDirection.NULL, code); 129 String codeString = ((PrimitiveType) code).asStringValue(); 130 if (codeString == null || "".equals(codeString)) 131 return new Enumeration<SortDirection>(this, SortDirection.NULL, code); 132 if ("ascending".equals(codeString)) 133 return new Enumeration<SortDirection>(this, SortDirection.ASCENDING, code); 134 if ("descending".equals(codeString)) 135 return new Enumeration<SortDirection>(this, SortDirection.DESCENDING, code); 136 throw new FHIRException("Unknown SortDirection code '"+codeString+"'"); 137 } 138 public String toCode(SortDirection code) { 139 if (code == SortDirection.NULL) 140 return null; 141 if (code == SortDirection.ASCENDING) 142 return "ascending"; 143 if (code == SortDirection.DESCENDING) 144 return "descending"; 145 return "?"; 146 } 147 public String toSystem(SortDirection code) { 148 return code.getSystem(); 149 } 150 } 151 152 public enum ValueFilterComparator { 153 /** 154 * the value for the parameter in the resource is equal to the provided value. 155 */ 156 EQ, 157 /** 158 * the value for the parameter in the resource is greater than the provided value. 159 */ 160 GT, 161 /** 162 * the value for the parameter in the resource is less than the provided value. 163 */ 164 LT, 165 /** 166 * the value for the parameter in the resource is greater or equal to the provided value. 167 */ 168 GE, 169 /** 170 * the value for the parameter in the resource is less or equal to the provided value. 171 */ 172 LE, 173 /** 174 * the value for the parameter in the resource starts after the provided value. 175 */ 176 SA, 177 /** 178 * the value for the parameter in the resource ends before the provided value. 179 */ 180 EB, 181 /** 182 * added to help the parsers with the generic types 183 */ 184 NULL; 185 public static ValueFilterComparator fromCode(String codeString) throws FHIRException { 186 if (codeString == null || "".equals(codeString)) 187 return null; 188 if ("eq".equals(codeString)) 189 return EQ; 190 if ("gt".equals(codeString)) 191 return GT; 192 if ("lt".equals(codeString)) 193 return LT; 194 if ("ge".equals(codeString)) 195 return GE; 196 if ("le".equals(codeString)) 197 return LE; 198 if ("sa".equals(codeString)) 199 return SA; 200 if ("eb".equals(codeString)) 201 return EB; 202 if (Configuration.isAcceptInvalidEnums()) 203 return null; 204 else 205 throw new FHIRException("Unknown ValueFilterComparator code '"+codeString+"'"); 206 } 207 public String toCode() { 208 switch (this) { 209 case EQ: return "eq"; 210 case GT: return "gt"; 211 case LT: return "lt"; 212 case GE: return "ge"; 213 case LE: return "le"; 214 case SA: return "sa"; 215 case EB: return "eb"; 216 case NULL: return null; 217 default: return "?"; 218 } 219 } 220 public String getSystem() { 221 switch (this) { 222 case EQ: return "http://hl7.org/fhir/search-comparator"; 223 case GT: return "http://hl7.org/fhir/search-comparator"; 224 case LT: return "http://hl7.org/fhir/search-comparator"; 225 case GE: return "http://hl7.org/fhir/search-comparator"; 226 case LE: return "http://hl7.org/fhir/search-comparator"; 227 case SA: return "http://hl7.org/fhir/search-comparator"; 228 case EB: return "http://hl7.org/fhir/search-comparator"; 229 case NULL: return null; 230 default: return "?"; 231 } 232 } 233 public String getDefinition() { 234 switch (this) { 235 case EQ: return "the value for the parameter in the resource is equal to the provided value."; 236 case GT: return "the value for the parameter in the resource is greater than the provided value."; 237 case LT: return "the value for the parameter in the resource is less than the provided value."; 238 case GE: return "the value for the parameter in the resource is greater or equal to the provided value."; 239 case LE: return "the value for the parameter in the resource is less or equal to the provided value."; 240 case SA: return "the value for the parameter in the resource starts after the provided value."; 241 case EB: return "the value for the parameter in the resource ends before the provided value."; 242 case NULL: return null; 243 default: return "?"; 244 } 245 } 246 public String getDisplay() { 247 switch (this) { 248 case EQ: return "Equals"; 249 case GT: return "Greater Than"; 250 case LT: return "Less Than"; 251 case GE: return "Greater or Equals"; 252 case LE: return "Less of Equal"; 253 case SA: return "Starts After"; 254 case EB: return "Ends Before"; 255 case NULL: return null; 256 default: return "?"; 257 } 258 } 259 } 260 261 public static class ValueFilterComparatorEnumFactory implements EnumFactory<ValueFilterComparator> { 262 public ValueFilterComparator fromCode(String codeString) throws IllegalArgumentException { 263 if (codeString == null || "".equals(codeString)) 264 if (codeString == null || "".equals(codeString)) 265 return null; 266 if ("eq".equals(codeString)) 267 return ValueFilterComparator.EQ; 268 if ("gt".equals(codeString)) 269 return ValueFilterComparator.GT; 270 if ("lt".equals(codeString)) 271 return ValueFilterComparator.LT; 272 if ("ge".equals(codeString)) 273 return ValueFilterComparator.GE; 274 if ("le".equals(codeString)) 275 return ValueFilterComparator.LE; 276 if ("sa".equals(codeString)) 277 return ValueFilterComparator.SA; 278 if ("eb".equals(codeString)) 279 return ValueFilterComparator.EB; 280 throw new IllegalArgumentException("Unknown ValueFilterComparator code '"+codeString+"'"); 281 } 282 public Enumeration<ValueFilterComparator> fromType(PrimitiveType<?> code) throws FHIRException { 283 if (code == null) 284 return null; 285 if (code.isEmpty()) 286 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.NULL, code); 287 String codeString = ((PrimitiveType) code).asStringValue(); 288 if (codeString == null || "".equals(codeString)) 289 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.NULL, code); 290 if ("eq".equals(codeString)) 291 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.EQ, code); 292 if ("gt".equals(codeString)) 293 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.GT, code); 294 if ("lt".equals(codeString)) 295 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.LT, code); 296 if ("ge".equals(codeString)) 297 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.GE, code); 298 if ("le".equals(codeString)) 299 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.LE, code); 300 if ("sa".equals(codeString)) 301 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.SA, code); 302 if ("eb".equals(codeString)) 303 return new Enumeration<ValueFilterComparator>(this, ValueFilterComparator.EB, code); 304 throw new FHIRException("Unknown ValueFilterComparator code '"+codeString+"'"); 305 } 306 public String toCode(ValueFilterComparator code) { 307 if (code == ValueFilterComparator.NULL) 308 return null; 309 if (code == ValueFilterComparator.EQ) 310 return "eq"; 311 if (code == ValueFilterComparator.GT) 312 return "gt"; 313 if (code == ValueFilterComparator.LT) 314 return "lt"; 315 if (code == ValueFilterComparator.GE) 316 return "ge"; 317 if (code == ValueFilterComparator.LE) 318 return "le"; 319 if (code == ValueFilterComparator.SA) 320 return "sa"; 321 if (code == ValueFilterComparator.EB) 322 return "eb"; 323 return "?"; 324 } 325 public String toSystem(ValueFilterComparator code) { 326 return code.getSystem(); 327 } 328 } 329 330 @Block() 331 public static class DataRequirementCodeFilterComponent extends Element implements IBaseDatatypeElement { 332 /** 333 * The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept. 334 */ 335 @Child(name = "path", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 336 @Description(shortDefinition="A code-valued attribute to filter on", formalDefinition="The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept." ) 337 protected StringType path; 338 339 /** 340 * A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept. 341 */ 342 @Child(name = "searchParam", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 343 @Description(shortDefinition="A coded (token) parameter to search on", formalDefinition="A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept." ) 344 protected StringType searchParam; 345 346 /** 347 * The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset. 348 */ 349 @Child(name = "valueSet", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 350 @Description(shortDefinition="ValueSet for the filter", formalDefinition="The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset." ) 351 protected CanonicalType valueSet; 352 353 /** 354 * The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes. 355 */ 356 @Child(name = "code", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 357 @Description(shortDefinition="What code is expected", formalDefinition="The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes." ) 358 protected List<Coding> code; 359 360 private static final long serialVersionUID = -1286212752L; 361 362 /** 363 * Constructor 364 */ 365 public DataRequirementCodeFilterComponent() { 366 super(); 367 } 368 369 /** 370 * @return {@link #path} (The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 371 */ 372 public StringType getPathElement() { 373 if (this.path == null) 374 if (Configuration.errorOnAutoCreate()) 375 throw new Error("Attempt to auto-create DataRequirementCodeFilterComponent.path"); 376 else if (Configuration.doAutoCreate()) 377 this.path = new StringType(); // bb 378 return this.path; 379 } 380 381 public boolean hasPathElement() { 382 return this.path != null && !this.path.isEmpty(); 383 } 384 385 public boolean hasPath() { 386 return this.path != null && !this.path.isEmpty(); 387 } 388 389 /** 390 * @param value {@link #path} (The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 391 */ 392 public DataRequirementCodeFilterComponent setPathElement(StringType value) { 393 this.path = value; 394 return this; 395 } 396 397 /** 398 * @return The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept. 399 */ 400 public String getPath() { 401 return this.path == null ? null : this.path.getValue(); 402 } 403 404 /** 405 * @param value The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept. 406 */ 407 public DataRequirementCodeFilterComponent setPath(String value) { 408 if (Utilities.noString(value)) 409 this.path = null; 410 else { 411 if (this.path == null) 412 this.path = new StringType(); 413 this.path.setValue(value); 414 } 415 return this; 416 } 417 418 /** 419 * @return {@link #searchParam} (A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept.). This is the underlying object with id, value and extensions. The accessor "getSearchParam" gives direct access to the value 420 */ 421 public StringType getSearchParamElement() { 422 if (this.searchParam == null) 423 if (Configuration.errorOnAutoCreate()) 424 throw new Error("Attempt to auto-create DataRequirementCodeFilterComponent.searchParam"); 425 else if (Configuration.doAutoCreate()) 426 this.searchParam = new StringType(); // bb 427 return this.searchParam; 428 } 429 430 public boolean hasSearchParamElement() { 431 return this.searchParam != null && !this.searchParam.isEmpty(); 432 } 433 434 public boolean hasSearchParam() { 435 return this.searchParam != null && !this.searchParam.isEmpty(); 436 } 437 438 /** 439 * @param value {@link #searchParam} (A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept.). This is the underlying object with id, value and extensions. The accessor "getSearchParam" gives direct access to the value 440 */ 441 public DataRequirementCodeFilterComponent setSearchParamElement(StringType value) { 442 this.searchParam = value; 443 return this; 444 } 445 446 /** 447 * @return A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept. 448 */ 449 public String getSearchParam() { 450 return this.searchParam == null ? null : this.searchParam.getValue(); 451 } 452 453 /** 454 * @param value A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept. 455 */ 456 public DataRequirementCodeFilterComponent setSearchParam(String value) { 457 if (Utilities.noString(value)) 458 this.searchParam = null; 459 else { 460 if (this.searchParam == null) 461 this.searchParam = new StringType(); 462 this.searchParam.setValue(value); 463 } 464 return this; 465 } 466 467 /** 468 * @return {@link #valueSet} (The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 469 */ 470 public CanonicalType getValueSetElement() { 471 if (this.valueSet == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create DataRequirementCodeFilterComponent.valueSet"); 474 else if (Configuration.doAutoCreate()) 475 this.valueSet = new CanonicalType(); // bb 476 return this.valueSet; 477 } 478 479 public boolean hasValueSetElement() { 480 return this.valueSet != null && !this.valueSet.isEmpty(); 481 } 482 483 public boolean hasValueSet() { 484 return this.valueSet != null && !this.valueSet.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #valueSet} (The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 489 */ 490 public DataRequirementCodeFilterComponent setValueSetElement(CanonicalType value) { 491 this.valueSet = value; 492 return this; 493 } 494 495 /** 496 * @return The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset. 497 */ 498 public String getValueSet() { 499 return this.valueSet == null ? null : this.valueSet.getValue(); 500 } 501 502 /** 503 * @param value The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset. 504 */ 505 public DataRequirementCodeFilterComponent setValueSet(String value) { 506 if (Utilities.noString(value)) 507 this.valueSet = null; 508 else { 509 if (this.valueSet == null) 510 this.valueSet = new CanonicalType(); 511 this.valueSet.setValue(value); 512 } 513 return this; 514 } 515 516 /** 517 * @return {@link #code} (The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes.) 518 */ 519 public List<Coding> getCode() { 520 if (this.code == null) 521 this.code = new ArrayList<Coding>(); 522 return this.code; 523 } 524 525 /** 526 * @return Returns a reference to <code>this</code> for easy method chaining 527 */ 528 public DataRequirementCodeFilterComponent setCode(List<Coding> theCode) { 529 this.code = theCode; 530 return this; 531 } 532 533 public boolean hasCode() { 534 if (this.code == null) 535 return false; 536 for (Coding item : this.code) 537 if (!item.isEmpty()) 538 return true; 539 return false; 540 } 541 542 public Coding addCode() { //3 543 Coding t = new Coding(); 544 if (this.code == null) 545 this.code = new ArrayList<Coding>(); 546 this.code.add(t); 547 return t; 548 } 549 550 public DataRequirementCodeFilterComponent addCode(Coding t) { //3 551 if (t == null) 552 return this; 553 if (this.code == null) 554 this.code = new ArrayList<Coding>(); 555 this.code.add(t); 556 return this; 557 } 558 559 /** 560 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 561 */ 562 public Coding getCodeFirstRep() { 563 if (getCode().isEmpty()) { 564 addCode(); 565 } 566 return getCode().get(0); 567 } 568 569 protected void listChildren(List<Property> children) { 570 super.listChildren(children); 571 children.add(new Property("path", "string", "The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.", 0, 1, path)); 572 children.add(new Property("searchParam", "string", "A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept.", 0, 1, searchParam)); 573 children.add(new Property("valueSet", "canonical(ValueSet)", "The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 0, 1, valueSet)); 574 children.add(new Property("code", "Coding", "The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes.", 0, java.lang.Integer.MAX_VALUE, code)); 575 } 576 577 @Override 578 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 579 switch (_hash) { 580 case 3433509: /*path*/ return new Property("path", "string", "The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.", 0, 1, path); 581 case -553645115: /*searchParam*/ return new Property("searchParam", "string", "A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept.", 0, 1, searchParam); 582 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 0, 1, valueSet); 583 case 3059181: /*code*/ return new Property("code", "Coding", "The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes.", 0, java.lang.Integer.MAX_VALUE, code); 584 default: return super.getNamedProperty(_hash, _name, _checkValid); 585 } 586 587 } 588 589 @Override 590 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 591 switch (hash) { 592 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 593 case -553645115: /*searchParam*/ return this.searchParam == null ? new Base[0] : new Base[] {this.searchParam}; // StringType 594 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 595 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 596 default: return super.getProperty(hash, name, checkValid); 597 } 598 599 } 600 601 @Override 602 public Base setProperty(int hash, String name, Base value) throws FHIRException { 603 switch (hash) { 604 case 3433509: // path 605 this.path = TypeConvertor.castToString(value); // StringType 606 return value; 607 case -553645115: // searchParam 608 this.searchParam = TypeConvertor.castToString(value); // StringType 609 return value; 610 case -1410174671: // valueSet 611 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 612 return value; 613 case 3059181: // code 614 this.getCode().add(TypeConvertor.castToCoding(value)); // Coding 615 return value; 616 default: return super.setProperty(hash, name, value); 617 } 618 619 } 620 621 @Override 622 public Base setProperty(String name, Base value) throws FHIRException { 623 if (name.equals("path")) { 624 this.path = TypeConvertor.castToString(value); // StringType 625 } else if (name.equals("searchParam")) { 626 this.searchParam = TypeConvertor.castToString(value); // StringType 627 } else if (name.equals("valueSet")) { 628 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 629 } else if (name.equals("code")) { 630 this.getCode().add(TypeConvertor.castToCoding(value)); 631 } else 632 return super.setProperty(name, value); 633 return value; 634 } 635 636 @Override 637 public void removeChild(String name, Base value) throws FHIRException { 638 if (name.equals("path")) { 639 this.path = null; 640 } else if (name.equals("searchParam")) { 641 this.searchParam = null; 642 } else if (name.equals("valueSet")) { 643 this.valueSet = null; 644 } else if (name.equals("code")) { 645 this.getCode().remove(value); 646 } else 647 super.removeChild(name, value); 648 649 } 650 651 @Override 652 public Base makeProperty(int hash, String name) throws FHIRException { 653 switch (hash) { 654 case 3433509: return getPathElement(); 655 case -553645115: return getSearchParamElement(); 656 case -1410174671: return getValueSetElement(); 657 case 3059181: return addCode(); 658 default: return super.makeProperty(hash, name); 659 } 660 661 } 662 663 @Override 664 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 665 switch (hash) { 666 case 3433509: /*path*/ return new String[] {"string"}; 667 case -553645115: /*searchParam*/ return new String[] {"string"}; 668 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 669 case 3059181: /*code*/ return new String[] {"Coding"}; 670 default: return super.getTypesForProperty(hash, name); 671 } 672 673 } 674 675 @Override 676 public Base addChild(String name) throws FHIRException { 677 if (name.equals("path")) { 678 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.codeFilter.path"); 679 } 680 else if (name.equals("searchParam")) { 681 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.codeFilter.searchParam"); 682 } 683 else if (name.equals("valueSet")) { 684 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.codeFilter.valueSet"); 685 } 686 else if (name.equals("code")) { 687 return addCode(); 688 } 689 else 690 return super.addChild(name); 691 } 692 693 public DataRequirementCodeFilterComponent copy() { 694 DataRequirementCodeFilterComponent dst = new DataRequirementCodeFilterComponent(); 695 copyValues(dst); 696 return dst; 697 } 698 699 public void copyValues(DataRequirementCodeFilterComponent dst) { 700 super.copyValues(dst); 701 dst.path = path == null ? null : path.copy(); 702 dst.searchParam = searchParam == null ? null : searchParam.copy(); 703 dst.valueSet = valueSet == null ? null : valueSet.copy(); 704 if (code != null) { 705 dst.code = new ArrayList<Coding>(); 706 for (Coding i : code) 707 dst.code.add(i.copy()); 708 }; 709 } 710 711 @Override 712 public boolean equalsDeep(Base other_) { 713 if (!super.equalsDeep(other_)) 714 return false; 715 if (!(other_ instanceof DataRequirementCodeFilterComponent)) 716 return false; 717 DataRequirementCodeFilterComponent o = (DataRequirementCodeFilterComponent) other_; 718 return compareDeep(path, o.path, true) && compareDeep(searchParam, o.searchParam, true) && compareDeep(valueSet, o.valueSet, true) 719 && compareDeep(code, o.code, true); 720 } 721 722 @Override 723 public boolean equalsShallow(Base other_) { 724 if (!super.equalsShallow(other_)) 725 return false; 726 if (!(other_ instanceof DataRequirementCodeFilterComponent)) 727 return false; 728 DataRequirementCodeFilterComponent o = (DataRequirementCodeFilterComponent) other_; 729 return compareValues(path, o.path, true) && compareValues(searchParam, o.searchParam, true) && compareValues(valueSet, o.valueSet, true) 730 ; 731 } 732 733 public boolean isEmpty() { 734 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, searchParam, valueSet 735 , code); 736 } 737 738 public String fhirType() { 739 return "DataRequirement.codeFilter"; 740 741 } 742 743 } 744 745 @Block() 746 public static class DataRequirementDateFilterComponent extends Element implements IBaseDatatypeElement { 747 /** 748 * The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing. 749 */ 750 @Child(name = "path", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 751 @Description(shortDefinition="A date-valued attribute to filter on", formalDefinition="The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing." ) 752 protected StringType path; 753 754 /** 755 * A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing. 756 */ 757 @Child(name = "searchParam", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 758 @Description(shortDefinition="A date valued parameter to search on", formalDefinition="A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing." ) 759 protected StringType searchParam; 760 761 /** 762 * The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now. 763 */ 764 @Child(name = "value", type = {DateTimeType.class, Period.class, Duration.class}, order=3, min=0, max=1, modifier=false, summary=true) 765 @Description(shortDefinition="The value of the filter, as a Period, DateTime, or Duration value", formalDefinition="The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now." ) 766 protected DataType value; 767 768 private static final long serialVersionUID = 1649787979L; 769 770 /** 771 * Constructor 772 */ 773 public DataRequirementDateFilterComponent() { 774 super(); 775 } 776 777 /** 778 * @return {@link #path} (The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 779 */ 780 public StringType getPathElement() { 781 if (this.path == null) 782 if (Configuration.errorOnAutoCreate()) 783 throw new Error("Attempt to auto-create DataRequirementDateFilterComponent.path"); 784 else if (Configuration.doAutoCreate()) 785 this.path = new StringType(); // bb 786 return this.path; 787 } 788 789 public boolean hasPathElement() { 790 return this.path != null && !this.path.isEmpty(); 791 } 792 793 public boolean hasPath() { 794 return this.path != null && !this.path.isEmpty(); 795 } 796 797 /** 798 * @param value {@link #path} (The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 799 */ 800 public DataRequirementDateFilterComponent setPathElement(StringType value) { 801 this.path = value; 802 return this; 803 } 804 805 /** 806 * @return The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing. 807 */ 808 public String getPath() { 809 return this.path == null ? null : this.path.getValue(); 810 } 811 812 /** 813 * @param value The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing. 814 */ 815 public DataRequirementDateFilterComponent setPath(String value) { 816 if (Utilities.noString(value)) 817 this.path = null; 818 else { 819 if (this.path == null) 820 this.path = new StringType(); 821 this.path.setValue(value); 822 } 823 return this; 824 } 825 826 /** 827 * @return {@link #searchParam} (A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing.). This is the underlying object with id, value and extensions. The accessor "getSearchParam" gives direct access to the value 828 */ 829 public StringType getSearchParamElement() { 830 if (this.searchParam == null) 831 if (Configuration.errorOnAutoCreate()) 832 throw new Error("Attempt to auto-create DataRequirementDateFilterComponent.searchParam"); 833 else if (Configuration.doAutoCreate()) 834 this.searchParam = new StringType(); // bb 835 return this.searchParam; 836 } 837 838 public boolean hasSearchParamElement() { 839 return this.searchParam != null && !this.searchParam.isEmpty(); 840 } 841 842 public boolean hasSearchParam() { 843 return this.searchParam != null && !this.searchParam.isEmpty(); 844 } 845 846 /** 847 * @param value {@link #searchParam} (A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing.). This is the underlying object with id, value and extensions. The accessor "getSearchParam" gives direct access to the value 848 */ 849 public DataRequirementDateFilterComponent setSearchParamElement(StringType value) { 850 this.searchParam = value; 851 return this; 852 } 853 854 /** 855 * @return A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing. 856 */ 857 public String getSearchParam() { 858 return this.searchParam == null ? null : this.searchParam.getValue(); 859 } 860 861 /** 862 * @param value A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing. 863 */ 864 public DataRequirementDateFilterComponent setSearchParam(String value) { 865 if (Utilities.noString(value)) 866 this.searchParam = null; 867 else { 868 if (this.searchParam == null) 869 this.searchParam = new StringType(); 870 this.searchParam.setValue(value); 871 } 872 return this; 873 } 874 875 /** 876 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.) 877 */ 878 public DataType getValue() { 879 return this.value; 880 } 881 882 /** 883 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.) 884 */ 885 public DateTimeType getValueDateTimeType() throws FHIRException { 886 if (this.value == null) 887 this.value = new DateTimeType(); 888 if (!(this.value instanceof DateTimeType)) 889 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 890 return (DateTimeType) this.value; 891 } 892 893 public boolean hasValueDateTimeType() { 894 return this != null && this.value instanceof DateTimeType; 895 } 896 897 /** 898 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.) 899 */ 900 public Period getValuePeriod() throws FHIRException { 901 if (this.value == null) 902 this.value = new Period(); 903 if (!(this.value instanceof Period)) 904 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 905 return (Period) this.value; 906 } 907 908 public boolean hasValuePeriod() { 909 return this != null && this.value instanceof Period; 910 } 911 912 /** 913 * @return {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.) 914 */ 915 public Duration getValueDuration() throws FHIRException { 916 if (this.value == null) 917 this.value = new Duration(); 918 if (!(this.value instanceof Duration)) 919 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 920 return (Duration) this.value; 921 } 922 923 public boolean hasValueDuration() { 924 return this != null && this.value instanceof Duration; 925 } 926 927 public boolean hasValue() { 928 return this.value != null && !this.value.isEmpty(); 929 } 930 931 /** 932 * @param value {@link #value} (The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.) 933 */ 934 public DataRequirementDateFilterComponent setValue(DataType value) { 935 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration)) 936 throw new FHIRException("Not the right type for DataRequirement.dateFilter.value[x]: "+value.fhirType()); 937 this.value = value; 938 return this; 939 } 940 941 protected void listChildren(List<Property> children) { 942 super.listChildren(children); 943 children.add(new Property("path", "string", "The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing.", 0, 1, path)); 944 children.add(new Property("searchParam", "string", "A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing.", 0, 1, searchParam)); 945 children.add(new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 0, 1, value)); 946 } 947 948 @Override 949 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 950 switch (_hash) { 951 case 3433509: /*path*/ return new Property("path", "string", "The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing.", 0, 1, path); 952 case -553645115: /*searchParam*/ return new Property("searchParam", "string", "A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing.", 0, 1, searchParam); 953 case -1410166417: /*value[x]*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 0, 1, value); 954 case 111972721: /*value*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 0, 1, value); 955 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 0, 1, value); 956 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 0, 1, value); 957 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 0, 1, value); 958 default: return super.getNamedProperty(_hash, _name, _checkValid); 959 } 960 961 } 962 963 @Override 964 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 965 switch (hash) { 966 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 967 case -553645115: /*searchParam*/ return this.searchParam == null ? new Base[0] : new Base[] {this.searchParam}; // StringType 968 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 969 default: return super.getProperty(hash, name, checkValid); 970 } 971 972 } 973 974 @Override 975 public Base setProperty(int hash, String name, Base value) throws FHIRException { 976 switch (hash) { 977 case 3433509: // path 978 this.path = TypeConvertor.castToString(value); // StringType 979 return value; 980 case -553645115: // searchParam 981 this.searchParam = TypeConvertor.castToString(value); // StringType 982 return value; 983 case 111972721: // value 984 this.value = TypeConvertor.castToType(value); // DataType 985 return value; 986 default: return super.setProperty(hash, name, value); 987 } 988 989 } 990 991 @Override 992 public Base setProperty(String name, Base value) throws FHIRException { 993 if (name.equals("path")) { 994 this.path = TypeConvertor.castToString(value); // StringType 995 } else if (name.equals("searchParam")) { 996 this.searchParam = TypeConvertor.castToString(value); // StringType 997 } else if (name.equals("value[x]")) { 998 this.value = TypeConvertor.castToType(value); // DataType 999 } else 1000 return super.setProperty(name, value); 1001 return value; 1002 } 1003 1004 @Override 1005 public void removeChild(String name, Base value) throws FHIRException { 1006 if (name.equals("path")) { 1007 this.path = null; 1008 } else if (name.equals("searchParam")) { 1009 this.searchParam = null; 1010 } else if (name.equals("value[x]")) { 1011 this.value = null; 1012 } else 1013 super.removeChild(name, value); 1014 1015 } 1016 1017 @Override 1018 public Base makeProperty(int hash, String name) throws FHIRException { 1019 switch (hash) { 1020 case 3433509: return getPathElement(); 1021 case -553645115: return getSearchParamElement(); 1022 case -1410166417: return getValue(); 1023 case 111972721: return getValue(); 1024 default: return super.makeProperty(hash, name); 1025 } 1026 1027 } 1028 1029 @Override 1030 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1031 switch (hash) { 1032 case 3433509: /*path*/ return new String[] {"string"}; 1033 case -553645115: /*searchParam*/ return new String[] {"string"}; 1034 case 111972721: /*value*/ return new String[] {"dateTime", "Period", "Duration"}; 1035 default: return super.getTypesForProperty(hash, name); 1036 } 1037 1038 } 1039 1040 @Override 1041 public Base addChild(String name) throws FHIRException { 1042 if (name.equals("path")) { 1043 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.dateFilter.path"); 1044 } 1045 else if (name.equals("searchParam")) { 1046 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.dateFilter.searchParam"); 1047 } 1048 else if (name.equals("valueDateTime")) { 1049 this.value = new DateTimeType(); 1050 return this.value; 1051 } 1052 else if (name.equals("valuePeriod")) { 1053 this.value = new Period(); 1054 return this.value; 1055 } 1056 else if (name.equals("valueDuration")) { 1057 this.value = new Duration(); 1058 return this.value; 1059 } 1060 else 1061 return super.addChild(name); 1062 } 1063 1064 public DataRequirementDateFilterComponent copy() { 1065 DataRequirementDateFilterComponent dst = new DataRequirementDateFilterComponent(); 1066 copyValues(dst); 1067 return dst; 1068 } 1069 1070 public void copyValues(DataRequirementDateFilterComponent dst) { 1071 super.copyValues(dst); 1072 dst.path = path == null ? null : path.copy(); 1073 dst.searchParam = searchParam == null ? null : searchParam.copy(); 1074 dst.value = value == null ? null : value.copy(); 1075 } 1076 1077 @Override 1078 public boolean equalsDeep(Base other_) { 1079 if (!super.equalsDeep(other_)) 1080 return false; 1081 if (!(other_ instanceof DataRequirementDateFilterComponent)) 1082 return false; 1083 DataRequirementDateFilterComponent o = (DataRequirementDateFilterComponent) other_; 1084 return compareDeep(path, o.path, true) && compareDeep(searchParam, o.searchParam, true) && compareDeep(value, o.value, true) 1085 ; 1086 } 1087 1088 @Override 1089 public boolean equalsShallow(Base other_) { 1090 if (!super.equalsShallow(other_)) 1091 return false; 1092 if (!(other_ instanceof DataRequirementDateFilterComponent)) 1093 return false; 1094 DataRequirementDateFilterComponent o = (DataRequirementDateFilterComponent) other_; 1095 return compareValues(path, o.path, true) && compareValues(searchParam, o.searchParam, true); 1096 } 1097 1098 public boolean isEmpty() { 1099 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, searchParam, value 1100 ); 1101 } 1102 1103 public String fhirType() { 1104 return "DataRequirement.dateFilter"; 1105 1106 } 1107 1108 } 1109 1110 @Block() 1111 public static class DataRequirementValueFilterComponent extends Element implements IBaseDatatypeElement { 1112 /** 1113 * The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter. 1114 */ 1115 @Child(name = "path", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1116 @Description(shortDefinition="An attribute to filter on", formalDefinition="The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter." ) 1117 protected StringType path; 1118 1119 /** 1120 * A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter. 1121 */ 1122 @Child(name = "searchParam", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1123 @Description(shortDefinition="A parameter to search on", formalDefinition="A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter." ) 1124 protected StringType searchParam; 1125 1126 /** 1127 * The comparator to be used to determine whether the value is matching. 1128 */ 1129 @Child(name = "comparator", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1130 @Description(shortDefinition="eq | gt | lt | ge | le | sa | eb", formalDefinition="The comparator to be used to determine whether the value is matching." ) 1131 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/value-filter-comparator") 1132 protected Enumeration<ValueFilterComparator> comparator; 1133 1134 /** 1135 * The value of the filter. 1136 */ 1137 @Child(name = "value", type = {DateTimeType.class, Period.class, Duration.class}, order=4, min=0, max=1, modifier=false, summary=true) 1138 @Description(shortDefinition="The value of the filter, as a Period, DateTime, or Duration value", formalDefinition="The value of the filter." ) 1139 protected DataType value; 1140 1141 private static final long serialVersionUID = 2106988483L; 1142 1143 /** 1144 * Constructor 1145 */ 1146 public DataRequirementValueFilterComponent() { 1147 super(); 1148 } 1149 1150 /** 1151 * @return {@link #path} (The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1152 */ 1153 public StringType getPathElement() { 1154 if (this.path == null) 1155 if (Configuration.errorOnAutoCreate()) 1156 throw new Error("Attempt to auto-create DataRequirementValueFilterComponent.path"); 1157 else if (Configuration.doAutoCreate()) 1158 this.path = new StringType(); // bb 1159 return this.path; 1160 } 1161 1162 public boolean hasPathElement() { 1163 return this.path != null && !this.path.isEmpty(); 1164 } 1165 1166 public boolean hasPath() { 1167 return this.path != null && !this.path.isEmpty(); 1168 } 1169 1170 /** 1171 * @param value {@link #path} (The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1172 */ 1173 public DataRequirementValueFilterComponent setPathElement(StringType value) { 1174 this.path = value; 1175 return this; 1176 } 1177 1178 /** 1179 * @return The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter. 1180 */ 1181 public String getPath() { 1182 return this.path == null ? null : this.path.getValue(); 1183 } 1184 1185 /** 1186 * @param value The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter. 1187 */ 1188 public DataRequirementValueFilterComponent setPath(String value) { 1189 if (Utilities.noString(value)) 1190 this.path = null; 1191 else { 1192 if (this.path == null) 1193 this.path = new StringType(); 1194 this.path.setValue(value); 1195 } 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #searchParam} (A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter.). This is the underlying object with id, value and extensions. The accessor "getSearchParam" gives direct access to the value 1201 */ 1202 public StringType getSearchParamElement() { 1203 if (this.searchParam == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create DataRequirementValueFilterComponent.searchParam"); 1206 else if (Configuration.doAutoCreate()) 1207 this.searchParam = new StringType(); // bb 1208 return this.searchParam; 1209 } 1210 1211 public boolean hasSearchParamElement() { 1212 return this.searchParam != null && !this.searchParam.isEmpty(); 1213 } 1214 1215 public boolean hasSearchParam() { 1216 return this.searchParam != null && !this.searchParam.isEmpty(); 1217 } 1218 1219 /** 1220 * @param value {@link #searchParam} (A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter.). This is the underlying object with id, value and extensions. The accessor "getSearchParam" gives direct access to the value 1221 */ 1222 public DataRequirementValueFilterComponent setSearchParamElement(StringType value) { 1223 this.searchParam = value; 1224 return this; 1225 } 1226 1227 /** 1228 * @return A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter. 1229 */ 1230 public String getSearchParam() { 1231 return this.searchParam == null ? null : this.searchParam.getValue(); 1232 } 1233 1234 /** 1235 * @param value A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter. 1236 */ 1237 public DataRequirementValueFilterComponent setSearchParam(String value) { 1238 if (Utilities.noString(value)) 1239 this.searchParam = null; 1240 else { 1241 if (this.searchParam == null) 1242 this.searchParam = new StringType(); 1243 this.searchParam.setValue(value); 1244 } 1245 return this; 1246 } 1247 1248 /** 1249 * @return {@link #comparator} (The comparator to be used to determine whether the value is matching.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 1250 */ 1251 public Enumeration<ValueFilterComparator> getComparatorElement() { 1252 if (this.comparator == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create DataRequirementValueFilterComponent.comparator"); 1255 else if (Configuration.doAutoCreate()) 1256 this.comparator = new Enumeration<ValueFilterComparator>(new ValueFilterComparatorEnumFactory()); // bb 1257 return this.comparator; 1258 } 1259 1260 public boolean hasComparatorElement() { 1261 return this.comparator != null && !this.comparator.isEmpty(); 1262 } 1263 1264 public boolean hasComparator() { 1265 return this.comparator != null && !this.comparator.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #comparator} (The comparator to be used to determine whether the value is matching.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 1270 */ 1271 public DataRequirementValueFilterComponent setComparatorElement(Enumeration<ValueFilterComparator> value) { 1272 this.comparator = value; 1273 return this; 1274 } 1275 1276 /** 1277 * @return The comparator to be used to determine whether the value is matching. 1278 */ 1279 public ValueFilterComparator getComparator() { 1280 return this.comparator == null ? null : this.comparator.getValue(); 1281 } 1282 1283 /** 1284 * @param value The comparator to be used to determine whether the value is matching. 1285 */ 1286 public DataRequirementValueFilterComponent setComparator(ValueFilterComparator value) { 1287 if (value == null) 1288 this.comparator = null; 1289 else { 1290 if (this.comparator == null) 1291 this.comparator = new Enumeration<ValueFilterComparator>(new ValueFilterComparatorEnumFactory()); 1292 this.comparator.setValue(value); 1293 } 1294 return this; 1295 } 1296 1297 /** 1298 * @return {@link #value} (The value of the filter.) 1299 */ 1300 public DataType getValue() { 1301 return this.value; 1302 } 1303 1304 /** 1305 * @return {@link #value} (The value of the filter.) 1306 */ 1307 public DateTimeType getValueDateTimeType() throws FHIRException { 1308 if (this.value == null) 1309 this.value = new DateTimeType(); 1310 if (!(this.value instanceof DateTimeType)) 1311 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1312 return (DateTimeType) this.value; 1313 } 1314 1315 public boolean hasValueDateTimeType() { 1316 return this != null && this.value instanceof DateTimeType; 1317 } 1318 1319 /** 1320 * @return {@link #value} (The value of the filter.) 1321 */ 1322 public Period getValuePeriod() throws FHIRException { 1323 if (this.value == null) 1324 this.value = new Period(); 1325 if (!(this.value instanceof Period)) 1326 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 1327 return (Period) this.value; 1328 } 1329 1330 public boolean hasValuePeriod() { 1331 return this != null && this.value instanceof Period; 1332 } 1333 1334 /** 1335 * @return {@link #value} (The value of the filter.) 1336 */ 1337 public Duration getValueDuration() throws FHIRException { 1338 if (this.value == null) 1339 this.value = new Duration(); 1340 if (!(this.value instanceof Duration)) 1341 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 1342 return (Duration) this.value; 1343 } 1344 1345 public boolean hasValueDuration() { 1346 return this != null && this.value instanceof Duration; 1347 } 1348 1349 public boolean hasValue() { 1350 return this.value != null && !this.value.isEmpty(); 1351 } 1352 1353 /** 1354 * @param value {@link #value} (The value of the filter.) 1355 */ 1356 public DataRequirementValueFilterComponent setValue(DataType value) { 1357 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration)) 1358 throw new FHIRException("Not the right type for DataRequirement.valueFilter.value[x]: "+value.fhirType()); 1359 this.value = value; 1360 return this; 1361 } 1362 1363 protected void listChildren(List<Property> children) { 1364 super.listChildren(children); 1365 children.add(new Property("path", "string", "The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter.", 0, 1, path)); 1366 children.add(new Property("searchParam", "string", "A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter.", 0, 1, searchParam)); 1367 children.add(new Property("comparator", "code", "The comparator to be used to determine whether the value is matching.", 0, 1, comparator)); 1368 children.add(new Property("value[x]", "dateTime|Period|Duration", "The value of the filter.", 0, 1, value)); 1369 } 1370 1371 @Override 1372 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1373 switch (_hash) { 1374 case 3433509: /*path*/ return new Property("path", "string", "The attribute of the filter. The specified path SHALL be a FHIRPath resolvable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of a type that is comparable to the valueFilter.value[x] element for the filter.", 0, 1, path); 1375 case -553645115: /*searchParam*/ return new Property("searchParam", "string", "A search parameter defined on the specified type of the DataRequirement, and which searches on elements of a type compatible with the type of the valueFilter.value[x] for the filter.", 0, 1, searchParam); 1376 case -844673834: /*comparator*/ return new Property("comparator", "code", "The comparator to be used to determine whether the value is matching.", 0, 1, comparator); 1377 case -1410166417: /*value[x]*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter.", 0, 1, value); 1378 case 111972721: /*value*/ return new Property("value[x]", "dateTime|Period|Duration", "The value of the filter.", 0, 1, value); 1379 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the filter.", 0, 1, value); 1380 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The value of the filter.", 0, 1, value); 1381 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the filter.", 0, 1, value); 1382 default: return super.getNamedProperty(_hash, _name, _checkValid); 1383 } 1384 1385 } 1386 1387 @Override 1388 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1389 switch (hash) { 1390 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1391 case -553645115: /*searchParam*/ return this.searchParam == null ? new Base[0] : new Base[] {this.searchParam}; // StringType 1392 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : new Base[] {this.comparator}; // Enumeration<ValueFilterComparator> 1393 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1394 default: return super.getProperty(hash, name, checkValid); 1395 } 1396 1397 } 1398 1399 @Override 1400 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1401 switch (hash) { 1402 case 3433509: // path 1403 this.path = TypeConvertor.castToString(value); // StringType 1404 return value; 1405 case -553645115: // searchParam 1406 this.searchParam = TypeConvertor.castToString(value); // StringType 1407 return value; 1408 case -844673834: // comparator 1409 value = new ValueFilterComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1410 this.comparator = (Enumeration) value; // Enumeration<ValueFilterComparator> 1411 return value; 1412 case 111972721: // value 1413 this.value = TypeConvertor.castToType(value); // DataType 1414 return value; 1415 default: return super.setProperty(hash, name, value); 1416 } 1417 1418 } 1419 1420 @Override 1421 public Base setProperty(String name, Base value) throws FHIRException { 1422 if (name.equals("path")) { 1423 this.path = TypeConvertor.castToString(value); // StringType 1424 } else if (name.equals("searchParam")) { 1425 this.searchParam = TypeConvertor.castToString(value); // StringType 1426 } else if (name.equals("comparator")) { 1427 value = new ValueFilterComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1428 this.comparator = (Enumeration) value; // Enumeration<ValueFilterComparator> 1429 } else if (name.equals("value[x]")) { 1430 this.value = TypeConvertor.castToType(value); // DataType 1431 } else 1432 return super.setProperty(name, value); 1433 return value; 1434 } 1435 1436 @Override 1437 public void removeChild(String name, Base value) throws FHIRException { 1438 if (name.equals("path")) { 1439 this.path = null; 1440 } else if (name.equals("searchParam")) { 1441 this.searchParam = null; 1442 } else if (name.equals("comparator")) { 1443 value = new ValueFilterComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1444 this.comparator = (Enumeration) value; // Enumeration<ValueFilterComparator> 1445 } else if (name.equals("value[x]")) { 1446 this.value = null; 1447 } else 1448 super.removeChild(name, value); 1449 1450 } 1451 1452 @Override 1453 public Base makeProperty(int hash, String name) throws FHIRException { 1454 switch (hash) { 1455 case 3433509: return getPathElement(); 1456 case -553645115: return getSearchParamElement(); 1457 case -844673834: return getComparatorElement(); 1458 case -1410166417: return getValue(); 1459 case 111972721: return getValue(); 1460 default: return super.makeProperty(hash, name); 1461 } 1462 1463 } 1464 1465 @Override 1466 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1467 switch (hash) { 1468 case 3433509: /*path*/ return new String[] {"string"}; 1469 case -553645115: /*searchParam*/ return new String[] {"string"}; 1470 case -844673834: /*comparator*/ return new String[] {"code"}; 1471 case 111972721: /*value*/ return new String[] {"dateTime", "Period", "Duration"}; 1472 default: return super.getTypesForProperty(hash, name); 1473 } 1474 1475 } 1476 1477 @Override 1478 public Base addChild(String name) throws FHIRException { 1479 if (name.equals("path")) { 1480 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.valueFilter.path"); 1481 } 1482 else if (name.equals("searchParam")) { 1483 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.valueFilter.searchParam"); 1484 } 1485 else if (name.equals("comparator")) { 1486 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.valueFilter.comparator"); 1487 } 1488 else if (name.equals("valueDateTime")) { 1489 this.value = new DateTimeType(); 1490 return this.value; 1491 } 1492 else if (name.equals("valuePeriod")) { 1493 this.value = new Period(); 1494 return this.value; 1495 } 1496 else if (name.equals("valueDuration")) { 1497 this.value = new Duration(); 1498 return this.value; 1499 } 1500 else 1501 return super.addChild(name); 1502 } 1503 1504 public DataRequirementValueFilterComponent copy() { 1505 DataRequirementValueFilterComponent dst = new DataRequirementValueFilterComponent(); 1506 copyValues(dst); 1507 return dst; 1508 } 1509 1510 public void copyValues(DataRequirementValueFilterComponent dst) { 1511 super.copyValues(dst); 1512 dst.path = path == null ? null : path.copy(); 1513 dst.searchParam = searchParam == null ? null : searchParam.copy(); 1514 dst.comparator = comparator == null ? null : comparator.copy(); 1515 dst.value = value == null ? null : value.copy(); 1516 } 1517 1518 @Override 1519 public boolean equalsDeep(Base other_) { 1520 if (!super.equalsDeep(other_)) 1521 return false; 1522 if (!(other_ instanceof DataRequirementValueFilterComponent)) 1523 return false; 1524 DataRequirementValueFilterComponent o = (DataRequirementValueFilterComponent) other_; 1525 return compareDeep(path, o.path, true) && compareDeep(searchParam, o.searchParam, true) && compareDeep(comparator, o.comparator, true) 1526 && compareDeep(value, o.value, true); 1527 } 1528 1529 @Override 1530 public boolean equalsShallow(Base other_) { 1531 if (!super.equalsShallow(other_)) 1532 return false; 1533 if (!(other_ instanceof DataRequirementValueFilterComponent)) 1534 return false; 1535 DataRequirementValueFilterComponent o = (DataRequirementValueFilterComponent) other_; 1536 return compareValues(path, o.path, true) && compareValues(searchParam, o.searchParam, true) && compareValues(comparator, o.comparator, true) 1537 ; 1538 } 1539 1540 public boolean isEmpty() { 1541 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, searchParam, comparator 1542 , value); 1543 } 1544 1545 public String fhirType() { 1546 return "DataRequirement.valueFilter"; 1547 1548 } 1549 1550 } 1551 1552 @Block() 1553 public static class DataRequirementSortComponent extends Element implements IBaseDatatypeElement { 1554 /** 1555 * The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. 1556 */ 1557 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1558 @Description(shortDefinition="The name of the attribute to perform the sort", formalDefinition="The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant." ) 1559 protected StringType path; 1560 1561 /** 1562 * The direction of the sort, ascending or descending. 1563 */ 1564 @Child(name = "direction", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1565 @Description(shortDefinition="ascending | descending", formalDefinition="The direction of the sort, ascending or descending." ) 1566 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/sort-direction") 1567 protected Enumeration<SortDirection> direction; 1568 1569 private static final long serialVersionUID = -694498683L; 1570 1571 /** 1572 * Constructor 1573 */ 1574 public DataRequirementSortComponent() { 1575 super(); 1576 } 1577 1578 /** 1579 * Constructor 1580 */ 1581 public DataRequirementSortComponent(String path, SortDirection direction) { 1582 super(); 1583 this.setPath(path); 1584 this.setDirection(direction); 1585 } 1586 1587 /** 1588 * @return {@link #path} (The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1589 */ 1590 public StringType getPathElement() { 1591 if (this.path == null) 1592 if (Configuration.errorOnAutoCreate()) 1593 throw new Error("Attempt to auto-create DataRequirementSortComponent.path"); 1594 else if (Configuration.doAutoCreate()) 1595 this.path = new StringType(); // bb 1596 return this.path; 1597 } 1598 1599 public boolean hasPathElement() { 1600 return this.path != null && !this.path.isEmpty(); 1601 } 1602 1603 public boolean hasPath() { 1604 return this.path != null && !this.path.isEmpty(); 1605 } 1606 1607 /** 1608 * @param value {@link #path} (The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1609 */ 1610 public DataRequirementSortComponent setPathElement(StringType value) { 1611 this.path = value; 1612 return this; 1613 } 1614 1615 /** 1616 * @return The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. 1617 */ 1618 public String getPath() { 1619 return this.path == null ? null : this.path.getValue(); 1620 } 1621 1622 /** 1623 * @param value The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant. 1624 */ 1625 public DataRequirementSortComponent setPath(String value) { 1626 if (this.path == null) 1627 this.path = new StringType(); 1628 this.path.setValue(value); 1629 return this; 1630 } 1631 1632 /** 1633 * @return {@link #direction} (The direction of the sort, ascending or descending.). This is the underlying object with id, value and extensions. The accessor "getDirection" gives direct access to the value 1634 */ 1635 public Enumeration<SortDirection> getDirectionElement() { 1636 if (this.direction == null) 1637 if (Configuration.errorOnAutoCreate()) 1638 throw new Error("Attempt to auto-create DataRequirementSortComponent.direction"); 1639 else if (Configuration.doAutoCreate()) 1640 this.direction = new Enumeration<SortDirection>(new SortDirectionEnumFactory()); // bb 1641 return this.direction; 1642 } 1643 1644 public boolean hasDirectionElement() { 1645 return this.direction != null && !this.direction.isEmpty(); 1646 } 1647 1648 public boolean hasDirection() { 1649 return this.direction != null && !this.direction.isEmpty(); 1650 } 1651 1652 /** 1653 * @param value {@link #direction} (The direction of the sort, ascending or descending.). This is the underlying object with id, value and extensions. The accessor "getDirection" gives direct access to the value 1654 */ 1655 public DataRequirementSortComponent setDirectionElement(Enumeration<SortDirection> value) { 1656 this.direction = value; 1657 return this; 1658 } 1659 1660 /** 1661 * @return The direction of the sort, ascending or descending. 1662 */ 1663 public SortDirection getDirection() { 1664 return this.direction == null ? null : this.direction.getValue(); 1665 } 1666 1667 /** 1668 * @param value The direction of the sort, ascending or descending. 1669 */ 1670 public DataRequirementSortComponent setDirection(SortDirection value) { 1671 if (this.direction == null) 1672 this.direction = new Enumeration<SortDirection>(new SortDirectionEnumFactory()); 1673 this.direction.setValue(value); 1674 return this; 1675 } 1676 1677 protected void listChildren(List<Property> children) { 1678 super.listChildren(children); 1679 children.add(new Property("path", "string", "The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant.", 0, 1, path)); 1680 children.add(new Property("direction", "code", "The direction of the sort, ascending or descending.", 0, 1, direction)); 1681 } 1682 1683 @Override 1684 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1685 switch (_hash) { 1686 case 3433509: /*path*/ return new Property("path", "string", "The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant.", 0, 1, path); 1687 case -962590849: /*direction*/ return new Property("direction", "code", "The direction of the sort, ascending or descending.", 0, 1, direction); 1688 default: return super.getNamedProperty(_hash, _name, _checkValid); 1689 } 1690 1691 } 1692 1693 @Override 1694 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1695 switch (hash) { 1696 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1697 case -962590849: /*direction*/ return this.direction == null ? new Base[0] : new Base[] {this.direction}; // Enumeration<SortDirection> 1698 default: return super.getProperty(hash, name, checkValid); 1699 } 1700 1701 } 1702 1703 @Override 1704 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1705 switch (hash) { 1706 case 3433509: // path 1707 this.path = TypeConvertor.castToString(value); // StringType 1708 return value; 1709 case -962590849: // direction 1710 value = new SortDirectionEnumFactory().fromType(TypeConvertor.castToCode(value)); 1711 this.direction = (Enumeration) value; // Enumeration<SortDirection> 1712 return value; 1713 default: return super.setProperty(hash, name, value); 1714 } 1715 1716 } 1717 1718 @Override 1719 public Base setProperty(String name, Base value) throws FHIRException { 1720 if (name.equals("path")) { 1721 this.path = TypeConvertor.castToString(value); // StringType 1722 } else if (name.equals("direction")) { 1723 value = new SortDirectionEnumFactory().fromType(TypeConvertor.castToCode(value)); 1724 this.direction = (Enumeration) value; // Enumeration<SortDirection> 1725 } else 1726 return super.setProperty(name, value); 1727 return value; 1728 } 1729 1730 @Override 1731 public void removeChild(String name, Base value) throws FHIRException { 1732 if (name.equals("path")) { 1733 this.path = null; 1734 } else if (name.equals("direction")) { 1735 value = new SortDirectionEnumFactory().fromType(TypeConvertor.castToCode(value)); 1736 this.direction = (Enumeration) value; // Enumeration<SortDirection> 1737 } else 1738 super.removeChild(name, value); 1739 1740 } 1741 1742 @Override 1743 public Base makeProperty(int hash, String name) throws FHIRException { 1744 switch (hash) { 1745 case 3433509: return getPathElement(); 1746 case -962590849: return getDirectionElement(); 1747 default: return super.makeProperty(hash, name); 1748 } 1749 1750 } 1751 1752 @Override 1753 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1754 switch (hash) { 1755 case 3433509: /*path*/ return new String[] {"string"}; 1756 case -962590849: /*direction*/ return new String[] {"code"}; 1757 default: return super.getTypesForProperty(hash, name); 1758 } 1759 1760 } 1761 1762 @Override 1763 public Base addChild(String name) throws FHIRException { 1764 if (name.equals("path")) { 1765 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.sort.path"); 1766 } 1767 else if (name.equals("direction")) { 1768 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.sort.direction"); 1769 } 1770 else 1771 return super.addChild(name); 1772 } 1773 1774 public DataRequirementSortComponent copy() { 1775 DataRequirementSortComponent dst = new DataRequirementSortComponent(); 1776 copyValues(dst); 1777 return dst; 1778 } 1779 1780 public void copyValues(DataRequirementSortComponent dst) { 1781 super.copyValues(dst); 1782 dst.path = path == null ? null : path.copy(); 1783 dst.direction = direction == null ? null : direction.copy(); 1784 } 1785 1786 @Override 1787 public boolean equalsDeep(Base other_) { 1788 if (!super.equalsDeep(other_)) 1789 return false; 1790 if (!(other_ instanceof DataRequirementSortComponent)) 1791 return false; 1792 DataRequirementSortComponent o = (DataRequirementSortComponent) other_; 1793 return compareDeep(path, o.path, true) && compareDeep(direction, o.direction, true); 1794 } 1795 1796 @Override 1797 public boolean equalsShallow(Base other_) { 1798 if (!super.equalsShallow(other_)) 1799 return false; 1800 if (!(other_ instanceof DataRequirementSortComponent)) 1801 return false; 1802 DataRequirementSortComponent o = (DataRequirementSortComponent) other_; 1803 return compareValues(path, o.path, true) && compareValues(direction, o.direction, true); 1804 } 1805 1806 public boolean isEmpty() { 1807 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, direction); 1808 } 1809 1810 public String fhirType() { 1811 return "DataRequirement.sort"; 1812 1813 } 1814 1815 } 1816 1817 /** 1818 * The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile. 1819 */ 1820 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 1821 @Description(shortDefinition="The type of the required data", formalDefinition="The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile." ) 1822 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 1823 protected Enumeration<FHIRTypes> type; 1824 1825 /** 1826 * The profile of the required data, specified as the uri of the profile definition. 1827 */ 1828 @Child(name = "profile", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1829 @Description(shortDefinition="The profile of the required data", formalDefinition="The profile of the required data, specified as the uri of the profile definition." ) 1830 protected List<CanonicalType> profile; 1831 1832 /** 1833 * The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed. 1834 */ 1835 @Child(name = "subject", type = {CodeableConcept.class, Group.class}, order=2, min=0, max=1, modifier=false, summary=true) 1836 @Description(shortDefinition="E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition="The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed." ) 1837 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 1838 protected DataType subject; 1839 1840 /** 1841 * Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. 1842 1843The value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 1844 */ 1845 @Child(name = "mustSupport", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1846 @Description(shortDefinition="Indicates specific structure elements that are referenced by the knowledge module", formalDefinition="Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. \n\nThe value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details)." ) 1847 protected List<StringType> mustSupport; 1848 1849 /** 1850 * Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed. 1851 */ 1852 @Child(name = "codeFilter", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1853 @Description(shortDefinition="What codes are expected", formalDefinition="Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed." ) 1854 protected List<DataRequirementCodeFilterComponent> codeFilter; 1855 1856 /** 1857 * Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed. 1858 */ 1859 @Child(name = "dateFilter", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1860 @Description(shortDefinition="What dates/date ranges are expected", formalDefinition="Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed." ) 1861 protected List<DataRequirementDateFilterComponent> dateFilter; 1862 1863 /** 1864 * Value filters specify additional constraints on the data for elements other than code-valued or date-valued. Each value filter specifies an additional constraint on the data (i.e. valueFilters are AND'ed, not OR'ed). 1865 */ 1866 @Child(name = "valueFilter", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1867 @Description(shortDefinition="What values are expected", formalDefinition="Value filters specify additional constraints on the data for elements other than code-valued or date-valued. Each value filter specifies an additional constraint on the data (i.e. valueFilters are AND'ed, not OR'ed)." ) 1868 protected List<DataRequirementValueFilterComponent> valueFilter; 1869 1870 /** 1871 * Specifies a maximum number of results that are required (uses the _count search parameter). 1872 */ 1873 @Child(name = "limit", type = {PositiveIntType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1874 @Description(shortDefinition="Number of results", formalDefinition="Specifies a maximum number of results that are required (uses the _count search parameter)." ) 1875 protected PositiveIntType limit; 1876 1877 /** 1878 * Specifies the order of the results to be returned. 1879 */ 1880 @Child(name = "sort", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1881 @Description(shortDefinition="Order of the results", formalDefinition="Specifies the order of the results to be returned." ) 1882 protected List<DataRequirementSortComponent> sort; 1883 1884 private static final long serialVersionUID = -2078097376L; 1885 1886 /** 1887 * Constructor 1888 */ 1889 public DataRequirement() { 1890 super(); 1891 } 1892 1893 /** 1894 * Constructor 1895 */ 1896 public DataRequirement(FHIRTypes type) { 1897 super(); 1898 this.setType(type); 1899 } 1900 1901 /** 1902 * @return {@link #type} (The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1903 */ 1904 public Enumeration<FHIRTypes> getTypeElement() { 1905 if (this.type == null) 1906 if (Configuration.errorOnAutoCreate()) 1907 throw new Error("Attempt to auto-create DataRequirement.type"); 1908 else if (Configuration.doAutoCreate()) 1909 this.type = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); // bb 1910 return this.type; 1911 } 1912 1913 public boolean hasTypeElement() { 1914 return this.type != null && !this.type.isEmpty(); 1915 } 1916 1917 public boolean hasType() { 1918 return this.type != null && !this.type.isEmpty(); 1919 } 1920 1921 /** 1922 * @param value {@link #type} (The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1923 */ 1924 public DataRequirement setTypeElement(Enumeration<FHIRTypes> value) { 1925 this.type = value; 1926 return this; 1927 } 1928 1929 /** 1930 * @return The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile. 1931 */ 1932 public FHIRTypes getType() { 1933 return this.type == null ? null : this.type.getValue(); 1934 } 1935 1936 /** 1937 * @param value The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile. 1938 */ 1939 public DataRequirement setType(FHIRTypes value) { 1940 if (this.type == null) 1941 this.type = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); 1942 this.type.setValue(value); 1943 return this; 1944 } 1945 1946 /** 1947 * @return {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 1948 */ 1949 public List<CanonicalType> getProfile() { 1950 if (this.profile == null) 1951 this.profile = new ArrayList<CanonicalType>(); 1952 return this.profile; 1953 } 1954 1955 /** 1956 * @return Returns a reference to <code>this</code> for easy method chaining 1957 */ 1958 public DataRequirement setProfile(List<CanonicalType> theProfile) { 1959 this.profile = theProfile; 1960 return this; 1961 } 1962 1963 public boolean hasProfile() { 1964 if (this.profile == null) 1965 return false; 1966 for (CanonicalType item : this.profile) 1967 if (!item.isEmpty()) 1968 return true; 1969 return false; 1970 } 1971 1972 /** 1973 * @return {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 1974 */ 1975 public CanonicalType addProfileElement() {//2 1976 CanonicalType t = new CanonicalType(); 1977 if (this.profile == null) 1978 this.profile = new ArrayList<CanonicalType>(); 1979 this.profile.add(t); 1980 return t; 1981 } 1982 1983 /** 1984 * @param value {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 1985 */ 1986 public DataRequirement addProfile(String value) { //1 1987 CanonicalType t = new CanonicalType(); 1988 t.setValue(value); 1989 if (this.profile == null) 1990 this.profile = new ArrayList<CanonicalType>(); 1991 this.profile.add(t); 1992 return this; 1993 } 1994 1995 /** 1996 * @param value {@link #profile} (The profile of the required data, specified as the uri of the profile definition.) 1997 */ 1998 public boolean hasProfile(String value) { 1999 if (this.profile == null) 2000 return false; 2001 for (CanonicalType v : this.profile) 2002 if (v.getValue().equals(value)) // canonical 2003 return true; 2004 return false; 2005 } 2006 2007 /** 2008 * @return {@link #subject} (The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.) 2009 */ 2010 public DataType getSubject() { 2011 return this.subject; 2012 } 2013 2014 /** 2015 * @return {@link #subject} (The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.) 2016 */ 2017 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2018 if (this.subject == null) 2019 this.subject = new CodeableConcept(); 2020 if (!(this.subject instanceof CodeableConcept)) 2021 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 2022 return (CodeableConcept) this.subject; 2023 } 2024 2025 public boolean hasSubjectCodeableConcept() { 2026 return this != null && this.subject instanceof CodeableConcept; 2027 } 2028 2029 /** 2030 * @return {@link #subject} (The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.) 2031 */ 2032 public Reference getSubjectReference() throws FHIRException { 2033 if (this.subject == null) 2034 this.subject = new Reference(); 2035 if (!(this.subject instanceof Reference)) 2036 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 2037 return (Reference) this.subject; 2038 } 2039 2040 public boolean hasSubjectReference() { 2041 return this != null && this.subject instanceof Reference; 2042 } 2043 2044 public boolean hasSubject() { 2045 return this.subject != null && !this.subject.isEmpty(); 2046 } 2047 2048 /** 2049 * @param value {@link #subject} (The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.) 2050 */ 2051 public DataRequirement setSubject(DataType value) { 2052 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2053 throw new FHIRException("Not the right type for DataRequirement.subject[x]: "+value.fhirType()); 2054 this.subject = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. 2060 2061The value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).) 2062 */ 2063 public List<StringType> getMustSupport() { 2064 if (this.mustSupport == null) 2065 this.mustSupport = new ArrayList<StringType>(); 2066 return this.mustSupport; 2067 } 2068 2069 /** 2070 * @return Returns a reference to <code>this</code> for easy method chaining 2071 */ 2072 public DataRequirement setMustSupport(List<StringType> theMustSupport) { 2073 this.mustSupport = theMustSupport; 2074 return this; 2075 } 2076 2077 public boolean hasMustSupport() { 2078 if (this.mustSupport == null) 2079 return false; 2080 for (StringType item : this.mustSupport) 2081 if (!item.isEmpty()) 2082 return true; 2083 return false; 2084 } 2085 2086 /** 2087 * @return {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. 2088 2089The value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).) 2090 */ 2091 public StringType addMustSupportElement() {//2 2092 StringType t = new StringType(); 2093 if (this.mustSupport == null) 2094 this.mustSupport = new ArrayList<StringType>(); 2095 this.mustSupport.add(t); 2096 return t; 2097 } 2098 2099 /** 2100 * @param value {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. 2101 2102The value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).) 2103 */ 2104 public DataRequirement addMustSupport(String value) { //1 2105 StringType t = new StringType(); 2106 t.setValue(value); 2107 if (this.mustSupport == null) 2108 this.mustSupport = new ArrayList<StringType>(); 2109 this.mustSupport.add(t); 2110 return this; 2111 } 2112 2113 /** 2114 * @param value {@link #mustSupport} (Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. 2115 2116The value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).) 2117 */ 2118 public boolean hasMustSupport(String value) { 2119 if (this.mustSupport == null) 2120 return false; 2121 for (StringType v : this.mustSupport) 2122 if (v.getValue().equals(value)) // string 2123 return true; 2124 return false; 2125 } 2126 2127 /** 2128 * @return {@link #codeFilter} (Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed.) 2129 */ 2130 public List<DataRequirementCodeFilterComponent> getCodeFilter() { 2131 if (this.codeFilter == null) 2132 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 2133 return this.codeFilter; 2134 } 2135 2136 /** 2137 * @return Returns a reference to <code>this</code> for easy method chaining 2138 */ 2139 public DataRequirement setCodeFilter(List<DataRequirementCodeFilterComponent> theCodeFilter) { 2140 this.codeFilter = theCodeFilter; 2141 return this; 2142 } 2143 2144 public boolean hasCodeFilter() { 2145 if (this.codeFilter == null) 2146 return false; 2147 for (DataRequirementCodeFilterComponent item : this.codeFilter) 2148 if (!item.isEmpty()) 2149 return true; 2150 return false; 2151 } 2152 2153 public DataRequirementCodeFilterComponent addCodeFilter() { //3 2154 DataRequirementCodeFilterComponent t = new DataRequirementCodeFilterComponent(); 2155 if (this.codeFilter == null) 2156 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 2157 this.codeFilter.add(t); 2158 return t; 2159 } 2160 2161 public DataRequirement addCodeFilter(DataRequirementCodeFilterComponent t) { //3 2162 if (t == null) 2163 return this; 2164 if (this.codeFilter == null) 2165 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 2166 this.codeFilter.add(t); 2167 return this; 2168 } 2169 2170 /** 2171 * @return The first repetition of repeating field {@link #codeFilter}, creating it if it does not already exist {3} 2172 */ 2173 public DataRequirementCodeFilterComponent getCodeFilterFirstRep() { 2174 if (getCodeFilter().isEmpty()) { 2175 addCodeFilter(); 2176 } 2177 return getCodeFilter().get(0); 2178 } 2179 2180 /** 2181 * @return {@link #dateFilter} (Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed.) 2182 */ 2183 public List<DataRequirementDateFilterComponent> getDateFilter() { 2184 if (this.dateFilter == null) 2185 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 2186 return this.dateFilter; 2187 } 2188 2189 /** 2190 * @return Returns a reference to <code>this</code> for easy method chaining 2191 */ 2192 public DataRequirement setDateFilter(List<DataRequirementDateFilterComponent> theDateFilter) { 2193 this.dateFilter = theDateFilter; 2194 return this; 2195 } 2196 2197 public boolean hasDateFilter() { 2198 if (this.dateFilter == null) 2199 return false; 2200 for (DataRequirementDateFilterComponent item : this.dateFilter) 2201 if (!item.isEmpty()) 2202 return true; 2203 return false; 2204 } 2205 2206 public DataRequirementDateFilterComponent addDateFilter() { //3 2207 DataRequirementDateFilterComponent t = new DataRequirementDateFilterComponent(); 2208 if (this.dateFilter == null) 2209 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 2210 this.dateFilter.add(t); 2211 return t; 2212 } 2213 2214 public DataRequirement addDateFilter(DataRequirementDateFilterComponent t) { //3 2215 if (t == null) 2216 return this; 2217 if (this.dateFilter == null) 2218 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 2219 this.dateFilter.add(t); 2220 return this; 2221 } 2222 2223 /** 2224 * @return The first repetition of repeating field {@link #dateFilter}, creating it if it does not already exist {3} 2225 */ 2226 public DataRequirementDateFilterComponent getDateFilterFirstRep() { 2227 if (getDateFilter().isEmpty()) { 2228 addDateFilter(); 2229 } 2230 return getDateFilter().get(0); 2231 } 2232 2233 /** 2234 * @return {@link #valueFilter} (Value filters specify additional constraints on the data for elements other than code-valued or date-valued. Each value filter specifies an additional constraint on the data (i.e. valueFilters are AND'ed, not OR'ed).) 2235 */ 2236 public List<DataRequirementValueFilterComponent> getValueFilter() { 2237 if (this.valueFilter == null) 2238 this.valueFilter = new ArrayList<DataRequirementValueFilterComponent>(); 2239 return this.valueFilter; 2240 } 2241 2242 /** 2243 * @return Returns a reference to <code>this</code> for easy method chaining 2244 */ 2245 public DataRequirement setValueFilter(List<DataRequirementValueFilterComponent> theValueFilter) { 2246 this.valueFilter = theValueFilter; 2247 return this; 2248 } 2249 2250 public boolean hasValueFilter() { 2251 if (this.valueFilter == null) 2252 return false; 2253 for (DataRequirementValueFilterComponent item : this.valueFilter) 2254 if (!item.isEmpty()) 2255 return true; 2256 return false; 2257 } 2258 2259 public DataRequirementValueFilterComponent addValueFilter() { //3 2260 DataRequirementValueFilterComponent t = new DataRequirementValueFilterComponent(); 2261 if (this.valueFilter == null) 2262 this.valueFilter = new ArrayList<DataRequirementValueFilterComponent>(); 2263 this.valueFilter.add(t); 2264 return t; 2265 } 2266 2267 public DataRequirement addValueFilter(DataRequirementValueFilterComponent t) { //3 2268 if (t == null) 2269 return this; 2270 if (this.valueFilter == null) 2271 this.valueFilter = new ArrayList<DataRequirementValueFilterComponent>(); 2272 this.valueFilter.add(t); 2273 return this; 2274 } 2275 2276 /** 2277 * @return The first repetition of repeating field {@link #valueFilter}, creating it if it does not already exist {3} 2278 */ 2279 public DataRequirementValueFilterComponent getValueFilterFirstRep() { 2280 if (getValueFilter().isEmpty()) { 2281 addValueFilter(); 2282 } 2283 return getValueFilter().get(0); 2284 } 2285 2286 /** 2287 * @return {@link #limit} (Specifies a maximum number of results that are required (uses the _count search parameter).). This is the underlying object with id, value and extensions. The accessor "getLimit" gives direct access to the value 2288 */ 2289 public PositiveIntType getLimitElement() { 2290 if (this.limit == null) 2291 if (Configuration.errorOnAutoCreate()) 2292 throw new Error("Attempt to auto-create DataRequirement.limit"); 2293 else if (Configuration.doAutoCreate()) 2294 this.limit = new PositiveIntType(); // bb 2295 return this.limit; 2296 } 2297 2298 public boolean hasLimitElement() { 2299 return this.limit != null && !this.limit.isEmpty(); 2300 } 2301 2302 public boolean hasLimit() { 2303 return this.limit != null && !this.limit.isEmpty(); 2304 } 2305 2306 /** 2307 * @param value {@link #limit} (Specifies a maximum number of results that are required (uses the _count search parameter).). This is the underlying object with id, value and extensions. The accessor "getLimit" gives direct access to the value 2308 */ 2309 public DataRequirement setLimitElement(PositiveIntType value) { 2310 this.limit = value; 2311 return this; 2312 } 2313 2314 /** 2315 * @return Specifies a maximum number of results that are required (uses the _count search parameter). 2316 */ 2317 public int getLimit() { 2318 return this.limit == null || this.limit.isEmpty() ? 0 : this.limit.getValue(); 2319 } 2320 2321 /** 2322 * @param value Specifies a maximum number of results that are required (uses the _count search parameter). 2323 */ 2324 public DataRequirement setLimit(int value) { 2325 if (this.limit == null) 2326 this.limit = new PositiveIntType(); 2327 this.limit.setValue(value); 2328 return this; 2329 } 2330 2331 /** 2332 * @return {@link #sort} (Specifies the order of the results to be returned.) 2333 */ 2334 public List<DataRequirementSortComponent> getSort() { 2335 if (this.sort == null) 2336 this.sort = new ArrayList<DataRequirementSortComponent>(); 2337 return this.sort; 2338 } 2339 2340 /** 2341 * @return Returns a reference to <code>this</code> for easy method chaining 2342 */ 2343 public DataRequirement setSort(List<DataRequirementSortComponent> theSort) { 2344 this.sort = theSort; 2345 return this; 2346 } 2347 2348 public boolean hasSort() { 2349 if (this.sort == null) 2350 return false; 2351 for (DataRequirementSortComponent item : this.sort) 2352 if (!item.isEmpty()) 2353 return true; 2354 return false; 2355 } 2356 2357 public DataRequirementSortComponent addSort() { //3 2358 DataRequirementSortComponent t = new DataRequirementSortComponent(); 2359 if (this.sort == null) 2360 this.sort = new ArrayList<DataRequirementSortComponent>(); 2361 this.sort.add(t); 2362 return t; 2363 } 2364 2365 public DataRequirement addSort(DataRequirementSortComponent t) { //3 2366 if (t == null) 2367 return this; 2368 if (this.sort == null) 2369 this.sort = new ArrayList<DataRequirementSortComponent>(); 2370 this.sort.add(t); 2371 return this; 2372 } 2373 2374 /** 2375 * @return The first repetition of repeating field {@link #sort}, creating it if it does not already exist {3} 2376 */ 2377 public DataRequirementSortComponent getSortFirstRep() { 2378 if (getSort().isEmpty()) { 2379 addSort(); 2380 } 2381 return getSort().get(0); 2382 } 2383 2384 protected void listChildren(List<Property> children) { 2385 super.listChildren(children); 2386 children.add(new Property("type", "code", "The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.", 0, 1, type)); 2387 children.add(new Property("profile", "canonical(StructureDefinition)", "The profile of the required data, specified as the uri of the profile definition.", 0, java.lang.Integer.MAX_VALUE, profile)); 2388 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 0, 1, subject)); 2389 children.add(new Property("mustSupport", "string", "Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. \n\nThe value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, java.lang.Integer.MAX_VALUE, mustSupport)); 2390 children.add(new Property("codeFilter", "", "Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed.", 0, java.lang.Integer.MAX_VALUE, codeFilter)); 2391 children.add(new Property("dateFilter", "", "Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed.", 0, java.lang.Integer.MAX_VALUE, dateFilter)); 2392 children.add(new Property("valueFilter", "", "Value filters specify additional constraints on the data for elements other than code-valued or date-valued. Each value filter specifies an additional constraint on the data (i.e. valueFilters are AND'ed, not OR'ed).", 0, java.lang.Integer.MAX_VALUE, valueFilter)); 2393 children.add(new Property("limit", "positiveInt", "Specifies a maximum number of results that are required (uses the _count search parameter).", 0, 1, limit)); 2394 children.add(new Property("sort", "", "Specifies the order of the results to be returned.", 0, java.lang.Integer.MAX_VALUE, sort)); 2395 } 2396 2397 @Override 2398 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2399 switch (_hash) { 2400 case 3575610: /*type*/ return new Property("type", "code", "The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.", 0, 1, type); 2401 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "The profile of the required data, specified as the uri of the profile definition.", 0, java.lang.Integer.MAX_VALUE, profile); 2402 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 0, 1, subject); 2403 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 0, 1, subject); 2404 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 0, 1, subject); 2405 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group)", "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 0, 1, subject); 2406 case -1402857082: /*mustSupport*/ return new Property("mustSupport", "string", "Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. \n\nThe value of mustSupport SHALL be a FHIRPath resolvable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, java.lang.Integer.MAX_VALUE, mustSupport); 2407 case -1303674939: /*codeFilter*/ return new Property("codeFilter", "", "Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed.", 0, java.lang.Integer.MAX_VALUE, codeFilter); 2408 case 149531846: /*dateFilter*/ return new Property("dateFilter", "", "Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed.", 0, java.lang.Integer.MAX_VALUE, dateFilter); 2409 case -1807110071: /*valueFilter*/ return new Property("valueFilter", "", "Value filters specify additional constraints on the data for elements other than code-valued or date-valued. Each value filter specifies an additional constraint on the data (i.e. valueFilters are AND'ed, not OR'ed).", 0, java.lang.Integer.MAX_VALUE, valueFilter); 2410 case 102976443: /*limit*/ return new Property("limit", "positiveInt", "Specifies a maximum number of results that are required (uses the _count search parameter).", 0, 1, limit); 2411 case 3536286: /*sort*/ return new Property("sort", "", "Specifies the order of the results to be returned.", 0, java.lang.Integer.MAX_VALUE, sort); 2412 default: return super.getNamedProperty(_hash, _name, _checkValid); 2413 } 2414 2415 } 2416 2417 @Override 2418 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2419 switch (hash) { 2420 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<FHIRTypes> 2421 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 2422 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 2423 case -1402857082: /*mustSupport*/ return this.mustSupport == null ? new Base[0] : this.mustSupport.toArray(new Base[this.mustSupport.size()]); // StringType 2424 case -1303674939: /*codeFilter*/ return this.codeFilter == null ? new Base[0] : this.codeFilter.toArray(new Base[this.codeFilter.size()]); // DataRequirementCodeFilterComponent 2425 case 149531846: /*dateFilter*/ return this.dateFilter == null ? new Base[0] : this.dateFilter.toArray(new Base[this.dateFilter.size()]); // DataRequirementDateFilterComponent 2426 case -1807110071: /*valueFilter*/ return this.valueFilter == null ? new Base[0] : this.valueFilter.toArray(new Base[this.valueFilter.size()]); // DataRequirementValueFilterComponent 2427 case 102976443: /*limit*/ return this.limit == null ? new Base[0] : new Base[] {this.limit}; // PositiveIntType 2428 case 3536286: /*sort*/ return this.sort == null ? new Base[0] : this.sort.toArray(new Base[this.sort.size()]); // DataRequirementSortComponent 2429 default: return super.getProperty(hash, name, checkValid); 2430 } 2431 2432 } 2433 2434 @Override 2435 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2436 switch (hash) { 2437 case 3575610: // type 2438 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2439 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 2440 return value; 2441 case -309425751: // profile 2442 this.getProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2443 return value; 2444 case -1867885268: // subject 2445 this.subject = TypeConvertor.castToType(value); // DataType 2446 return value; 2447 case -1402857082: // mustSupport 2448 this.getMustSupport().add(TypeConvertor.castToString(value)); // StringType 2449 return value; 2450 case -1303674939: // codeFilter 2451 this.getCodeFilter().add((DataRequirementCodeFilterComponent) value); // DataRequirementCodeFilterComponent 2452 return value; 2453 case 149531846: // dateFilter 2454 this.getDateFilter().add((DataRequirementDateFilterComponent) value); // DataRequirementDateFilterComponent 2455 return value; 2456 case -1807110071: // valueFilter 2457 this.getValueFilter().add((DataRequirementValueFilterComponent) value); // DataRequirementValueFilterComponent 2458 return value; 2459 case 102976443: // limit 2460 this.limit = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2461 return value; 2462 case 3536286: // sort 2463 this.getSort().add((DataRequirementSortComponent) value); // DataRequirementSortComponent 2464 return value; 2465 default: return super.setProperty(hash, name, value); 2466 } 2467 2468 } 2469 2470 @Override 2471 public Base setProperty(String name, Base value) throws FHIRException { 2472 if (name.equals("type")) { 2473 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2474 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 2475 } else if (name.equals("profile")) { 2476 this.getProfile().add(TypeConvertor.castToCanonical(value)); 2477 } else if (name.equals("subject[x]")) { 2478 this.subject = TypeConvertor.castToType(value); // DataType 2479 } else if (name.equals("mustSupport")) { 2480 this.getMustSupport().add(TypeConvertor.castToString(value)); 2481 } else if (name.equals("codeFilter")) { 2482 this.getCodeFilter().add((DataRequirementCodeFilterComponent) value); 2483 } else if (name.equals("dateFilter")) { 2484 this.getDateFilter().add((DataRequirementDateFilterComponent) value); 2485 } else if (name.equals("valueFilter")) { 2486 this.getValueFilter().add((DataRequirementValueFilterComponent) value); 2487 } else if (name.equals("limit")) { 2488 this.limit = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2489 } else if (name.equals("sort")) { 2490 this.getSort().add((DataRequirementSortComponent) value); 2491 } else 2492 return super.setProperty(name, value); 2493 return value; 2494 } 2495 2496 @Override 2497 public void removeChild(String name, Base value) throws FHIRException { 2498 if (name.equals("type")) { 2499 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2500 this.type = (Enumeration) value; // Enumeration<FHIRTypes> 2501 } else if (name.equals("profile")) { 2502 this.getProfile().remove(value); 2503 } else if (name.equals("subject[x]")) { 2504 this.subject = null; 2505 } else if (name.equals("mustSupport")) { 2506 this.getMustSupport().remove(value); 2507 } else if (name.equals("codeFilter")) { 2508 this.getCodeFilter().remove((DataRequirementCodeFilterComponent) value); 2509 } else if (name.equals("dateFilter")) { 2510 this.getDateFilter().remove((DataRequirementDateFilterComponent) value); 2511 } else if (name.equals("valueFilter")) { 2512 this.getValueFilter().remove((DataRequirementValueFilterComponent) value); 2513 } else if (name.equals("limit")) { 2514 this.limit = null; 2515 } else if (name.equals("sort")) { 2516 this.getSort().remove((DataRequirementSortComponent) value); 2517 } else 2518 super.removeChild(name, value); 2519 2520 } 2521 2522 @Override 2523 public Base makeProperty(int hash, String name) throws FHIRException { 2524 switch (hash) { 2525 case 3575610: return getTypeElement(); 2526 case -309425751: return addProfileElement(); 2527 case -573640748: return getSubject(); 2528 case -1867885268: return getSubject(); 2529 case -1402857082: return addMustSupportElement(); 2530 case -1303674939: return addCodeFilter(); 2531 case 149531846: return addDateFilter(); 2532 case -1807110071: return addValueFilter(); 2533 case 102976443: return getLimitElement(); 2534 case 3536286: return addSort(); 2535 default: return super.makeProperty(hash, name); 2536 } 2537 2538 } 2539 2540 @Override 2541 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2542 switch (hash) { 2543 case 3575610: /*type*/ return new String[] {"code"}; 2544 case -309425751: /*profile*/ return new String[] {"canonical"}; 2545 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference"}; 2546 case -1402857082: /*mustSupport*/ return new String[] {"string"}; 2547 case -1303674939: /*codeFilter*/ return new String[] {}; 2548 case 149531846: /*dateFilter*/ return new String[] {}; 2549 case -1807110071: /*valueFilter*/ return new String[] {}; 2550 case 102976443: /*limit*/ return new String[] {"positiveInt"}; 2551 case 3536286: /*sort*/ return new String[] {}; 2552 default: return super.getTypesForProperty(hash, name); 2553 } 2554 2555 } 2556 2557 @Override 2558 public Base addChild(String name) throws FHIRException { 2559 if (name.equals("type")) { 2560 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.type"); 2561 } 2562 else if (name.equals("profile")) { 2563 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.profile"); 2564 } 2565 else if (name.equals("subjectCodeableConcept")) { 2566 this.subject = new CodeableConcept(); 2567 return this.subject; 2568 } 2569 else if (name.equals("subjectReference")) { 2570 this.subject = new Reference(); 2571 return this.subject; 2572 } 2573 else if (name.equals("mustSupport")) { 2574 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.mustSupport"); 2575 } 2576 else if (name.equals("codeFilter")) { 2577 return addCodeFilter(); 2578 } 2579 else if (name.equals("dateFilter")) { 2580 return addDateFilter(); 2581 } 2582 else if (name.equals("valueFilter")) { 2583 return addValueFilter(); 2584 } 2585 else if (name.equals("limit")) { 2586 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.limit"); 2587 } 2588 else if (name.equals("sort")) { 2589 return addSort(); 2590 } 2591 else 2592 return super.addChild(name); 2593 } 2594 2595 public String fhirType() { 2596 return "DataRequirement"; 2597 2598 } 2599 2600 public DataRequirement copy() { 2601 DataRequirement dst = new DataRequirement(); 2602 copyValues(dst); 2603 return dst; 2604 } 2605 2606 public void copyValues(DataRequirement dst) { 2607 super.copyValues(dst); 2608 dst.type = type == null ? null : type.copy(); 2609 if (profile != null) { 2610 dst.profile = new ArrayList<CanonicalType>(); 2611 for (CanonicalType i : profile) 2612 dst.profile.add(i.copy()); 2613 }; 2614 dst.subject = subject == null ? null : subject.copy(); 2615 if (mustSupport != null) { 2616 dst.mustSupport = new ArrayList<StringType>(); 2617 for (StringType i : mustSupport) 2618 dst.mustSupport.add(i.copy()); 2619 }; 2620 if (codeFilter != null) { 2621 dst.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 2622 for (DataRequirementCodeFilterComponent i : codeFilter) 2623 dst.codeFilter.add(i.copy()); 2624 }; 2625 if (dateFilter != null) { 2626 dst.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 2627 for (DataRequirementDateFilterComponent i : dateFilter) 2628 dst.dateFilter.add(i.copy()); 2629 }; 2630 if (valueFilter != null) { 2631 dst.valueFilter = new ArrayList<DataRequirementValueFilterComponent>(); 2632 for (DataRequirementValueFilterComponent i : valueFilter) 2633 dst.valueFilter.add(i.copy()); 2634 }; 2635 dst.limit = limit == null ? null : limit.copy(); 2636 if (sort != null) { 2637 dst.sort = new ArrayList<DataRequirementSortComponent>(); 2638 for (DataRequirementSortComponent i : sort) 2639 dst.sort.add(i.copy()); 2640 }; 2641 } 2642 2643 protected DataRequirement typedCopy() { 2644 return copy(); 2645 } 2646 2647 @Override 2648 public boolean equalsDeep(Base other_) { 2649 if (!super.equalsDeep(other_)) 2650 return false; 2651 if (!(other_ instanceof DataRequirement)) 2652 return false; 2653 DataRequirement o = (DataRequirement) other_; 2654 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) && compareDeep(subject, o.subject, true) 2655 && compareDeep(mustSupport, o.mustSupport, true) && compareDeep(codeFilter, o.codeFilter, true) 2656 && compareDeep(dateFilter, o.dateFilter, true) && compareDeep(valueFilter, o.valueFilter, true) 2657 && compareDeep(limit, o.limit, true) && compareDeep(sort, o.sort, true); 2658 } 2659 2660 @Override 2661 public boolean equalsShallow(Base other_) { 2662 if (!super.equalsShallow(other_)) 2663 return false; 2664 if (!(other_ instanceof DataRequirement)) 2665 return false; 2666 DataRequirement o = (DataRequirement) other_; 2667 return compareValues(type, o.type, true) && compareValues(profile, o.profile, true) && compareValues(mustSupport, o.mustSupport, true) 2668 && compareValues(limit, o.limit, true); 2669 } 2670 2671 public boolean isEmpty() { 2672 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, subject, mustSupport 2673 , codeFilter, dateFilter, valueFilter, limit, sort); 2674 } 2675 2676 2677} 2678