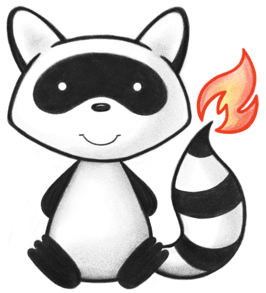
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 035import ca.uhn.fhir.model.api.annotation.DatatypeDef; 036import org.apache.commons.lang3.time.DateUtils; 037 038import java.util.Calendar; 039import java.util.Date; 040import java.util.TimeZone; 041import java.util.zip.DataFormatException; 042 043/** 044 * Represents a FHIR dateTime datatype. Valid precisions values for this type are: 045 * <ul> 046 * <li>{@link TemporalPrecisionEnum#YEAR} 047 * <li>{@link TemporalPrecisionEnum#MONTH} 048 * <li>{@link TemporalPrecisionEnum#DAY} 049 * <li>{@link TemporalPrecisionEnum#SECOND} 050 * <li>{@link TemporalPrecisionEnum#MILLI} 051 * </ul> 052 */ 053@DatatypeDef(name = "dateTime") 054public class DateTimeType extends BaseDateTimeType { 055 056 private static final long serialVersionUID = 3L; 057 058 /** 059 * The default precision for this type 060 */ 061 public static final TemporalPrecisionEnum DEFAULT_PRECISION = TemporalPrecisionEnum.SECOND; 062 063 /** 064 * Constructor 065 */ 066 public DateTimeType() { 067 super(); 068 } 069 070 /** 071 * Create a new DateTimeDt with seconds precision and the local time zone 072 */ 073 public DateTimeType(Date theDate) { 074 super(theDate, DEFAULT_PRECISION, TimeZone.getDefault()); 075 } 076 077 /** 078 * Constructor which accepts a date value and a precision value. Valid precisions values for this type are: 079 * <ul> 080 * <li>{@link TemporalPrecisionEnum#YEAR} 081 * <li>{@link TemporalPrecisionEnum#MONTH} 082 * <li>{@link TemporalPrecisionEnum#DAY} 083 * <li>{@link TemporalPrecisionEnum#SECOND} 084 * <li>{@link TemporalPrecisionEnum#MILLI} 085 * </ul> 086 * 087 * @throws DataFormatException 088 * If the specified precision is not allowed for this type 089 */ 090 public DateTimeType(Date theDate, TemporalPrecisionEnum thePrecision) { 091 super(theDate, thePrecision, TimeZone.getDefault()); 092 } 093 094 /** 095 * Create a new instance using a string date/time 096 * 097 * @throws DataFormatException 098 * If the specified precision is not allowed for this type 099 */ 100 public DateTimeType(String theValue) { 101 super(theValue); 102 } 103 104 /** 105 * Constructor which accepts a date value, precision value, and time zone. Valid precisions values for this type 106 * are: 107 * <ul> 108 * <li>{@link TemporalPrecisionEnum#YEAR} 109 * <li>{@link TemporalPrecisionEnum#MONTH} 110 * <li>{@link TemporalPrecisionEnum#DAY} 111 * <li>{@link TemporalPrecisionEnum#SECOND} 112 * <li>{@link TemporalPrecisionEnum#MILLI} 113 * </ul> 114 */ 115 public DateTimeType(Date theDate, TemporalPrecisionEnum thePrecision, TimeZone theTimezone) { 116 super(theDate, thePrecision, theTimezone); 117 } 118 119 /** 120 * Constructor 121 */ 122 public DateTimeType(Calendar theCalendar) { 123 if (theCalendar != null) { 124 setValue(theCalendar.getTime()); 125 setPrecision(DEFAULT_PRECISION); 126 setTimeZone(theCalendar.getTimeZone()); 127 } 128 } 129 130 @Override 131 boolean isPrecisionAllowed(TemporalPrecisionEnum thePrecision) { 132 switch (thePrecision) { 133 case YEAR: 134 case MONTH: 135 case DAY: 136 case SECOND: 137 case MILLI: 138 return true; 139 default: 140 return false; 141 } 142 } 143 144 /** 145 * Returns a new instance of DateTimeType with the current system time and SECOND precision and the system local time 146 * zone 147 */ 148 public static DateTimeType now() { 149 return new DateTimeType(new Date(), TemporalPrecisionEnum.SECOND, TimeZone.getDefault()); 150 } 151 152 /** 153 * Returns the default precision for this datatype 154 * 155 * @see #DEFAULT_PRECISION 156 */ 157 @Override 158 protected TemporalPrecisionEnum getDefaultPrecisionForDatatype() { 159 return DEFAULT_PRECISION; 160 } 161 162 @Override 163 public DateTimeType copy() { 164 DateTimeType ret = new DateTimeType(getValueAsString()); 165 copyValues(ret); 166 return ret; 167 } 168 169 /** 170 * Creates a new instance by parsing an HL7 v3 format date time string 171 */ 172 public static DateTimeType parseV3(String theV3String) { 173 DateTimeType retVal = new DateTimeType(); 174 retVal.setValueAsV3String(theV3String); 175 return retVal; 176 } 177 178 public static DateTimeType today() { 179 DateTimeType retVal = now(); 180 retVal.setPrecision(TemporalPrecisionEnum.DAY); 181 return retVal; 182 } 183 184 public boolean getTzSign() { 185 return getTimeZone().getRawOffset() >= 0; 186 } 187 188 public int getTzHour() { 189 return (int) (getTimeZone().getRawOffset() / DateUtils.MILLIS_PER_MINUTE) / 60; 190 } 191 192 public int getTzMin() { 193 return (int) (getTimeZone().getRawOffset() / DateUtils.MILLIS_PER_MINUTE) % 60; 194 } 195 196 197 public String fhirType() { 198 return "dateTime"; 199 } 200 201 public String getAsV3() { 202 String r = getValueAsString(); 203 r = stripChar(r, 16, ':'); 204 r = stripChar(r, 13, ':'); 205 r = stripChar(r, 10, 'T'); 206 r = stripChar(r, 7, '-'); 207 r = stripChar(r, 4, '-'); 208 r = r.replaceAll(":", ""); // might be in the timezone 209 return r; 210 } 211 212 private String stripChar(String r, int i, char c) { 213 if (r.length() <= i || r.charAt(i) != c) 214 return r; 215 return r.substring(0, i)+r.substring(i+1); 216 } 217 218 @Override 219 public boolean isDateTime() { 220 return true; 221 } 222 223}