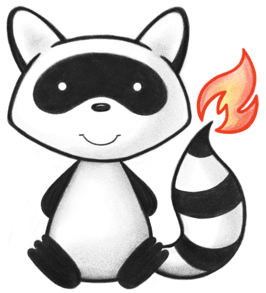
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.math.BigDecimal; 035import java.math.MathContext; 036import java.math.RoundingMode; 037 038import org.hl7.fhir.instance.model.api.IBaseDecimalDatatype; 039 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041 042/** 043 * Primitive type "decimal" in FHIR: A rational number 044 */ 045@DatatypeDef(name = "decimal") 046public class DecimalType extends PrimitiveType<BigDecimal> implements Comparable<DecimalType>, IBaseDecimalDatatype { 047 048 private static final long serialVersionUID = 3L; 049 050 /** 051 * Constructor 052 */ 053 public DecimalType() { 054 super(); 055 } 056 057 /** 058 * Constructor 059 */ 060 public DecimalType(BigDecimal theValue) { 061 setValue(theValue); 062 } 063 064 /** 065 * Constructor 066 */ 067 public DecimalType(double theValue) { 068 // Use the valueOf here because the constructor gives wacky precision 069 // changes due to the floating point conversion 070 setValue(BigDecimal.valueOf(theValue)); 071 } 072 073 /** 074 * Constructor 075 */ 076 public DecimalType(long theValue) { 077 setValue(theValue); 078 } 079 080 /** 081 * Constructor 082 */ 083 public DecimalType(String theValue) { 084 setValueAsString(theValue); 085 } 086 087 @Override 088 public int compareTo(DecimalType theObj) { 089 if (getValue() == null && theObj.getValue() == null) { 090 return 0; 091 } 092 if (getValue() != null && theObj.getValue() == null) { 093 return 1; 094 } 095 if (getValue() == null && theObj.getValue() != null) { 096 return -1; 097 } 098 return getValue().compareTo(theObj.getValue()); 099 } 100 101 @Override 102 protected String encode(BigDecimal theValue) { 103 return getValue().toString(); 104 } 105 106 /** 107 * Gets the value as an integer, using {@link BigDecimal#intValue()} 108 */ 109 public int getValueAsInteger() { 110 return getValue().intValue(); 111 } 112 113 public Number getValueAsNumber() { 114 return getValue(); 115 } 116 117 @Override 118 protected BigDecimal parse(String theValue) { 119 return new BigDecimal(theValue); 120 } 121 122 /** 123 * Rounds the value to the given prevision 124 * 125 * @see MathContext#getPrecision() 126 */ 127 public void round(int thePrecision) { 128 if (getValue() != null) { 129 BigDecimal newValue = getValue().round(new MathContext(thePrecision)); 130 setValue(newValue); 131 } 132 } 133 134 /** 135 * Rounds the value to the given prevision 136 * 137 * @see MathContext#getPrecision() 138 * @see MathContext#getRoundingMode() 139 */ 140 public void round(int thePrecision, RoundingMode theRoundingMode) { 141 if (getValue() != null) { 142 BigDecimal newValue = getValue().round(new MathContext(thePrecision, theRoundingMode)); 143 setValue(newValue); 144 } 145 } 146 147 /** 148 * Sets a new value using an integer 149 */ 150 public void setValueAsInteger(int theValue) { 151 setValue(BigDecimal.valueOf(theValue)); 152 } 153 154 /** 155 * Sets a new value using a long 156 */ 157 public void setValue(long theValue) { 158 setValue(BigDecimal.valueOf(theValue)); 159 } 160 161 /** 162 * Sets a new value using a double 163 */ 164 public void setValue(double theValue) { 165 setValue(BigDecimal.valueOf(theValue)); 166 } 167 168 public void setValueAsString(String theString) { 169 setValue(new BigDecimal(theString)); 170 setRepresentation(theString); 171 } 172 173 @Override 174 public DecimalType copy() { 175 DecimalType ret = new DecimalType(getValue()); 176 copyValues(ret); 177 return ret; 178 } 179 180 public String fhirType() { 181 return "decimal"; 182 } 183 184 /** 185 * A parser can provide a literal representation for the decimal value that preserves 186 * the presented form. 187 * 188 * All sorts of bad things can happen if this method is used to set the string representation 189 * to anything other than what was parsed into the actual value. Don't do that 190 * 191 * @param value 192 * @return 193 */ 194 public DecimalType setRepresentation(String value) { 195 forceStringValue(value); 196 return this; 197 } 198}