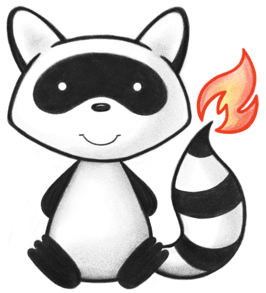
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, gaps in care, etc. 052 */ 053@ResourceDef(name="DetectedIssue", profile="http://hl7.org/fhir/StructureDefinition/DetectedIssue") 054public class DetectedIssue extends DomainResource { 055 056 public enum DetectedIssueSeverity { 057 /** 058 * Indicates the issue may be life-threatening or has the potential to cause permanent injury. 059 */ 060 HIGH, 061 /** 062 * Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury. 063 */ 064 MODERATE, 065 /** 066 * Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject. 067 */ 068 LOW, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static DetectedIssueSeverity fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("high".equals(codeString)) 077 return HIGH; 078 if ("moderate".equals(codeString)) 079 return MODERATE; 080 if ("low".equals(codeString)) 081 return LOW; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case HIGH: return "high"; 090 case MODERATE: return "moderate"; 091 case LOW: return "low"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case HIGH: return "http://hl7.org/fhir/detectedissue-severity"; 099 case MODERATE: return "http://hl7.org/fhir/detectedissue-severity"; 100 case LOW: return "http://hl7.org/fhir/detectedissue-severity"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case HIGH: return "Indicates the issue may be life-threatening or has the potential to cause permanent injury."; 108 case MODERATE: return "Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 109 case LOW: return "Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case HIGH: return "High"; 117 case MODERATE: return "Moderate"; 118 case LOW: return "Low"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class DetectedIssueSeverityEnumFactory implements EnumFactory<DetectedIssueSeverity> { 126 public DetectedIssueSeverity fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("high".equals(codeString)) 131 return DetectedIssueSeverity.HIGH; 132 if ("moderate".equals(codeString)) 133 return DetectedIssueSeverity.MODERATE; 134 if ("low".equals(codeString)) 135 return DetectedIssueSeverity.LOW; 136 throw new IllegalArgumentException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 137 } 138 public Enumeration<DetectedIssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 146 if ("high".equals(codeString)) 147 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.HIGH, code); 148 if ("moderate".equals(codeString)) 149 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.MODERATE, code); 150 if ("low".equals(codeString)) 151 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.LOW, code); 152 throw new FHIRException("Unknown DetectedIssueSeverity code '"+codeString+"'"); 153 } 154 public String toCode(DetectedIssueSeverity code) { 155 if (code == DetectedIssueSeverity.NULL) 156 return null; 157 if (code == DetectedIssueSeverity.HIGH) 158 return "high"; 159 if (code == DetectedIssueSeverity.MODERATE) 160 return "moderate"; 161 if (code == DetectedIssueSeverity.LOW) 162 return "low"; 163 return "?"; 164 } 165 public String toSystem(DetectedIssueSeverity code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum DetectedIssueStatus { 171 /** 172 * This is an initial or interim observation: data may be incomplete or unverified. 173 */ 174 PRELIMINARY, 175 /** 176 * The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc. would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied. 177 */ 178 FINAL, 179 /** 180 * The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 181 */ 182 ENTEREDINERROR, 183 /** 184 * Indicates the detected issue has been mitigated 185 */ 186 MITIGATED, 187 /** 188 * added to help the parsers with the generic types 189 */ 190 NULL; 191 public static DetectedIssueStatus fromCode(String codeString) throws FHIRException { 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("preliminary".equals(codeString)) 195 return PRELIMINARY; 196 if ("final".equals(codeString)) 197 return FINAL; 198 if ("entered-in-error".equals(codeString)) 199 return ENTEREDINERROR; 200 if ("mitigated".equals(codeString)) 201 return MITIGATED; 202 if (Configuration.isAcceptInvalidEnums()) 203 return null; 204 else 205 throw new FHIRException("Unknown DetectedIssueStatus code '"+codeString+"'"); 206 } 207 public String toCode() { 208 switch (this) { 209 case PRELIMINARY: return "preliminary"; 210 case FINAL: return "final"; 211 case ENTEREDINERROR: return "entered-in-error"; 212 case MITIGATED: return "mitigated"; 213 case NULL: return null; 214 default: return "?"; 215 } 216 } 217 public String getSystem() { 218 switch (this) { 219 case PRELIMINARY: return "http://hl7.org/fhir/observation-status"; 220 case FINAL: return "http://hl7.org/fhir/observation-status"; 221 case ENTEREDINERROR: return "http://hl7.org/fhir/observation-status"; 222 case MITIGATED: return "http://hl7.org/fhir/detectedissue-status"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 public String getDefinition() { 228 switch (this) { 229 case PRELIMINARY: return "This is an initial or interim observation: data may be incomplete or unverified."; 230 case FINAL: return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc. would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 231 case ENTEREDINERROR: return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 232 case MITIGATED: return "Indicates the detected issue has been mitigated"; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 public String getDisplay() { 238 switch (this) { 239 case PRELIMINARY: return "Preliminary"; 240 case FINAL: return "Final"; 241 case ENTEREDINERROR: return "Entered in Error"; 242 case MITIGATED: return "Mitigated"; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 } 248 249 public static class DetectedIssueStatusEnumFactory implements EnumFactory<DetectedIssueStatus> { 250 public DetectedIssueStatus fromCode(String codeString) throws IllegalArgumentException { 251 if (codeString == null || "".equals(codeString)) 252 if (codeString == null || "".equals(codeString)) 253 return null; 254 if ("preliminary".equals(codeString)) 255 return DetectedIssueStatus.PRELIMINARY; 256 if ("final".equals(codeString)) 257 return DetectedIssueStatus.FINAL; 258 if ("entered-in-error".equals(codeString)) 259 return DetectedIssueStatus.ENTEREDINERROR; 260 if ("mitigated".equals(codeString)) 261 return DetectedIssueStatus.MITIGATED; 262 throw new IllegalArgumentException("Unknown DetectedIssueStatus code '"+codeString+"'"); 263 } 264 public Enumeration<DetectedIssueStatus> fromType(PrimitiveType<?> code) throws FHIRException { 265 if (code == null) 266 return null; 267 if (code.isEmpty()) 268 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 269 String codeString = ((PrimitiveType) code).asStringValue(); 270 if (codeString == null || "".equals(codeString)) 271 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 272 if ("preliminary".equals(codeString)) 273 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.PRELIMINARY, code); 274 if ("final".equals(codeString)) 275 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.FINAL, code); 276 if ("entered-in-error".equals(codeString)) 277 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.ENTEREDINERROR, code); 278 if ("mitigated".equals(codeString)) 279 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.MITIGATED, code); 280 throw new FHIRException("Unknown DetectedIssueStatus code '"+codeString+"'"); 281 } 282 public String toCode(DetectedIssueStatus code) { 283 if (code == DetectedIssueStatus.NULL) 284 return null; 285 if (code == DetectedIssueStatus.PRELIMINARY) 286 return "preliminary"; 287 if (code == DetectedIssueStatus.FINAL) 288 return "final"; 289 if (code == DetectedIssueStatus.ENTEREDINERROR) 290 return "entered-in-error"; 291 if (code == DetectedIssueStatus.MITIGATED) 292 return "mitigated"; 293 return "?"; 294 } 295 public String toSystem(DetectedIssueStatus code) { 296 return code.getSystem(); 297 } 298 } 299 300 @Block() 301 public static class DetectedIssueEvidenceComponent extends BackboneElement implements IBaseBackboneElement { 302 /** 303 * A manifestation that led to the recording of this detected issue. 304 */ 305 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 306 @Description(shortDefinition="Manifestation", formalDefinition="A manifestation that led to the recording of this detected issue." ) 307 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/manifestation-or-symptom") 308 protected List<CodeableConcept> code; 309 310 /** 311 * Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport. 312 */ 313 @Child(name = "detail", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 314 @Description(shortDefinition="Supporting information", formalDefinition="Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport." ) 315 protected List<Reference> detail; 316 317 private static final long serialVersionUID = -672691342L; 318 319 /** 320 * Constructor 321 */ 322 public DetectedIssueEvidenceComponent() { 323 super(); 324 } 325 326 /** 327 * @return {@link #code} (A manifestation that led to the recording of this detected issue.) 328 */ 329 public List<CodeableConcept> getCode() { 330 if (this.code == null) 331 this.code = new ArrayList<CodeableConcept>(); 332 return this.code; 333 } 334 335 /** 336 * @return Returns a reference to <code>this</code> for easy method chaining 337 */ 338 public DetectedIssueEvidenceComponent setCode(List<CodeableConcept> theCode) { 339 this.code = theCode; 340 return this; 341 } 342 343 public boolean hasCode() { 344 if (this.code == null) 345 return false; 346 for (CodeableConcept item : this.code) 347 if (!item.isEmpty()) 348 return true; 349 return false; 350 } 351 352 public CodeableConcept addCode() { //3 353 CodeableConcept t = new CodeableConcept(); 354 if (this.code == null) 355 this.code = new ArrayList<CodeableConcept>(); 356 this.code.add(t); 357 return t; 358 } 359 360 public DetectedIssueEvidenceComponent addCode(CodeableConcept t) { //3 361 if (t == null) 362 return this; 363 if (this.code == null) 364 this.code = new ArrayList<CodeableConcept>(); 365 this.code.add(t); 366 return this; 367 } 368 369 /** 370 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 371 */ 372 public CodeableConcept getCodeFirstRep() { 373 if (getCode().isEmpty()) { 374 addCode(); 375 } 376 return getCode().get(0); 377 } 378 379 /** 380 * @return {@link #detail} (Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.) 381 */ 382 public List<Reference> getDetail() { 383 if (this.detail == null) 384 this.detail = new ArrayList<Reference>(); 385 return this.detail; 386 } 387 388 /** 389 * @return Returns a reference to <code>this</code> for easy method chaining 390 */ 391 public DetectedIssueEvidenceComponent setDetail(List<Reference> theDetail) { 392 this.detail = theDetail; 393 return this; 394 } 395 396 public boolean hasDetail() { 397 if (this.detail == null) 398 return false; 399 for (Reference item : this.detail) 400 if (!item.isEmpty()) 401 return true; 402 return false; 403 } 404 405 public Reference addDetail() { //3 406 Reference t = new Reference(); 407 if (this.detail == null) 408 this.detail = new ArrayList<Reference>(); 409 this.detail.add(t); 410 return t; 411 } 412 413 public DetectedIssueEvidenceComponent addDetail(Reference t) { //3 414 if (t == null) 415 return this; 416 if (this.detail == null) 417 this.detail = new ArrayList<Reference>(); 418 this.detail.add(t); 419 return this; 420 } 421 422 /** 423 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 424 */ 425 public Reference getDetailFirstRep() { 426 if (getDetail().isEmpty()) { 427 addDetail(); 428 } 429 return getDetail().get(0); 430 } 431 432 protected void listChildren(List<Property> children) { 433 super.listChildren(children); 434 children.add(new Property("code", "CodeableConcept", "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code)); 435 children.add(new Property("detail", "Reference(Any)", "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 0, java.lang.Integer.MAX_VALUE, detail)); 436 } 437 438 @Override 439 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 440 switch (_hash) { 441 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code); 442 case -1335224239: /*detail*/ return new Property("detail", "Reference(Any)", "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 0, java.lang.Integer.MAX_VALUE, detail); 443 default: return super.getNamedProperty(_hash, _name, _checkValid); 444 } 445 446 } 447 448 @Override 449 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 450 switch (hash) { 451 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 452 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 453 default: return super.getProperty(hash, name, checkValid); 454 } 455 456 } 457 458 @Override 459 public Base setProperty(int hash, String name, Base value) throws FHIRException { 460 switch (hash) { 461 case 3059181: // code 462 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 463 return value; 464 case -1335224239: // detail 465 this.getDetail().add(TypeConvertor.castToReference(value)); // Reference 466 return value; 467 default: return super.setProperty(hash, name, value); 468 } 469 470 } 471 472 @Override 473 public Base setProperty(String name, Base value) throws FHIRException { 474 if (name.equals("code")) { 475 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 476 } else if (name.equals("detail")) { 477 this.getDetail().add(TypeConvertor.castToReference(value)); 478 } else 479 return super.setProperty(name, value); 480 return value; 481 } 482 483 @Override 484 public void removeChild(String name, Base value) throws FHIRException { 485 if (name.equals("code")) { 486 this.getCode().remove(value); 487 } else if (name.equals("detail")) { 488 this.getDetail().remove(value); 489 } else 490 super.removeChild(name, value); 491 492 } 493 494 @Override 495 public Base makeProperty(int hash, String name) throws FHIRException { 496 switch (hash) { 497 case 3059181: return addCode(); 498 case -1335224239: return addDetail(); 499 default: return super.makeProperty(hash, name); 500 } 501 502 } 503 504 @Override 505 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 506 switch (hash) { 507 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 508 case -1335224239: /*detail*/ return new String[] {"Reference"}; 509 default: return super.getTypesForProperty(hash, name); 510 } 511 512 } 513 514 @Override 515 public Base addChild(String name) throws FHIRException { 516 if (name.equals("code")) { 517 return addCode(); 518 } 519 else if (name.equals("detail")) { 520 return addDetail(); 521 } 522 else 523 return super.addChild(name); 524 } 525 526 public DetectedIssueEvidenceComponent copy() { 527 DetectedIssueEvidenceComponent dst = new DetectedIssueEvidenceComponent(); 528 copyValues(dst); 529 return dst; 530 } 531 532 public void copyValues(DetectedIssueEvidenceComponent dst) { 533 super.copyValues(dst); 534 if (code != null) { 535 dst.code = new ArrayList<CodeableConcept>(); 536 for (CodeableConcept i : code) 537 dst.code.add(i.copy()); 538 }; 539 if (detail != null) { 540 dst.detail = new ArrayList<Reference>(); 541 for (Reference i : detail) 542 dst.detail.add(i.copy()); 543 }; 544 } 545 546 @Override 547 public boolean equalsDeep(Base other_) { 548 if (!super.equalsDeep(other_)) 549 return false; 550 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 551 return false; 552 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 553 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 554 } 555 556 @Override 557 public boolean equalsShallow(Base other_) { 558 if (!super.equalsShallow(other_)) 559 return false; 560 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 561 return false; 562 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 563 return true; 564 } 565 566 public boolean isEmpty() { 567 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, detail); 568 } 569 570 public String fhirType() { 571 return "DetectedIssue.evidence"; 572 573 } 574 575 } 576 577 @Block() 578 public static class DetectedIssueMitigationComponent extends BackboneElement implements IBaseBackboneElement { 579 /** 580 * Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue. 581 */ 582 @Child(name = "action", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 583 @Description(shortDefinition="What mitigation?", formalDefinition="Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue." ) 584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-mitigation-action") 585 protected CodeableConcept action; 586 587 /** 588 * Indicates when the mitigating action was documented. 589 */ 590 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 591 @Description(shortDefinition="Date committed", formalDefinition="Indicates when the mitigating action was documented." ) 592 protected DateTimeType date; 593 594 /** 595 * Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring. 596 */ 597 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class}, order=3, min=0, max=1, modifier=false, summary=false) 598 @Description(shortDefinition="Who is committing?", formalDefinition="Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring." ) 599 protected Reference author; 600 601 /** 602 * Clinicians may add additional notes or justifications about the mitigation action. For example, patient can have this drug because they have had it before without any issues. Multiple justifications may be provided. 603 */ 604 @Child(name = "note", type = {Annotation.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 605 @Description(shortDefinition="Additional notes about the mitigation", formalDefinition="Clinicians may add additional notes or justifications about the mitigation action. For example, patient can have this drug because they have had it before without any issues. Multiple justifications may be provided." ) 606 protected List<Annotation> note; 607 608 private static final long serialVersionUID = 1504361133L; 609 610 /** 611 * Constructor 612 */ 613 public DetectedIssueMitigationComponent() { 614 super(); 615 } 616 617 /** 618 * Constructor 619 */ 620 public DetectedIssueMitigationComponent(CodeableConcept action) { 621 super(); 622 this.setAction(action); 623 } 624 625 /** 626 * @return {@link #action} (Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.) 627 */ 628 public CodeableConcept getAction() { 629 if (this.action == null) 630 if (Configuration.errorOnAutoCreate()) 631 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.action"); 632 else if (Configuration.doAutoCreate()) 633 this.action = new CodeableConcept(); // cc 634 return this.action; 635 } 636 637 public boolean hasAction() { 638 return this.action != null && !this.action.isEmpty(); 639 } 640 641 /** 642 * @param value {@link #action} (Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.) 643 */ 644 public DetectedIssueMitigationComponent setAction(CodeableConcept value) { 645 this.action = value; 646 return this; 647 } 648 649 /** 650 * @return {@link #date} (Indicates when the mitigating action was documented.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 651 */ 652 public DateTimeType getDateElement() { 653 if (this.date == null) 654 if (Configuration.errorOnAutoCreate()) 655 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.date"); 656 else if (Configuration.doAutoCreate()) 657 this.date = new DateTimeType(); // bb 658 return this.date; 659 } 660 661 public boolean hasDateElement() { 662 return this.date != null && !this.date.isEmpty(); 663 } 664 665 public boolean hasDate() { 666 return this.date != null && !this.date.isEmpty(); 667 } 668 669 /** 670 * @param value {@link #date} (Indicates when the mitigating action was documented.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 671 */ 672 public DetectedIssueMitigationComponent setDateElement(DateTimeType value) { 673 this.date = value; 674 return this; 675 } 676 677 /** 678 * @return Indicates when the mitigating action was documented. 679 */ 680 public Date getDate() { 681 return this.date == null ? null : this.date.getValue(); 682 } 683 684 /** 685 * @param value Indicates when the mitigating action was documented. 686 */ 687 public DetectedIssueMitigationComponent setDate(Date value) { 688 if (value == null) 689 this.date = null; 690 else { 691 if (this.date == null) 692 this.date = new DateTimeType(); 693 this.date.setValue(value); 694 } 695 return this; 696 } 697 698 /** 699 * @return {@link #author} (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 700 */ 701 public Reference getAuthor() { 702 if (this.author == null) 703 if (Configuration.errorOnAutoCreate()) 704 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 705 else if (Configuration.doAutoCreate()) 706 this.author = new Reference(); // cc 707 return this.author; 708 } 709 710 public boolean hasAuthor() { 711 return this.author != null && !this.author.isEmpty(); 712 } 713 714 /** 715 * @param value {@link #author} (Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.) 716 */ 717 public DetectedIssueMitigationComponent setAuthor(Reference value) { 718 this.author = value; 719 return this; 720 } 721 722 /** 723 * @return {@link #note} (Clinicians may add additional notes or justifications about the mitigation action. For example, patient can have this drug because they have had it before without any issues. Multiple justifications may be provided.) 724 */ 725 public List<Annotation> getNote() { 726 if (this.note == null) 727 this.note = new ArrayList<Annotation>(); 728 return this.note; 729 } 730 731 /** 732 * @return Returns a reference to <code>this</code> for easy method chaining 733 */ 734 public DetectedIssueMitigationComponent setNote(List<Annotation> theNote) { 735 this.note = theNote; 736 return this; 737 } 738 739 public boolean hasNote() { 740 if (this.note == null) 741 return false; 742 for (Annotation item : this.note) 743 if (!item.isEmpty()) 744 return true; 745 return false; 746 } 747 748 public Annotation addNote() { //3 749 Annotation t = new Annotation(); 750 if (this.note == null) 751 this.note = new ArrayList<Annotation>(); 752 this.note.add(t); 753 return t; 754 } 755 756 public DetectedIssueMitigationComponent addNote(Annotation t) { //3 757 if (t == null) 758 return this; 759 if (this.note == null) 760 this.note = new ArrayList<Annotation>(); 761 this.note.add(t); 762 return this; 763 } 764 765 /** 766 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 767 */ 768 public Annotation getNoteFirstRep() { 769 if (getNote().isEmpty()) { 770 addNote(); 771 } 772 return getNote().get(0); 773 } 774 775 protected void listChildren(List<Property> children) { 776 super.listChildren(children); 777 children.add(new Property("action", "CodeableConcept", "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 0, 1, action)); 778 children.add(new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date)); 779 children.add(new Property("author", "Reference(Practitioner|PractitionerRole)", "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 0, 1, author)); 780 children.add(new Property("note", "Annotation", "Clinicians may add additional notes or justifications about the mitigation action. For example, patient can have this drug because they have had it before without any issues. Multiple justifications may be provided.", 0, java.lang.Integer.MAX_VALUE, note)); 781 } 782 783 @Override 784 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 785 switch (_hash) { 786 case -1422950858: /*action*/ return new Property("action", "CodeableConcept", "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 0, 1, action); 787 case 3076014: /*date*/ return new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date); 788 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole)", "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 0, 1, author); 789 case 3387378: /*note*/ return new Property("note", "Annotation", "Clinicians may add additional notes or justifications about the mitigation action. For example, patient can have this drug because they have had it before without any issues. Multiple justifications may be provided.", 0, java.lang.Integer.MAX_VALUE, note); 790 default: return super.getNamedProperty(_hash, _name, _checkValid); 791 } 792 793 } 794 795 @Override 796 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 797 switch (hash) { 798 case -1422950858: /*action*/ return this.action == null ? new Base[0] : new Base[] {this.action}; // CodeableConcept 799 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 800 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 801 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 802 default: return super.getProperty(hash, name, checkValid); 803 } 804 805 } 806 807 @Override 808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 809 switch (hash) { 810 case -1422950858: // action 811 this.action = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 812 return value; 813 case 3076014: // date 814 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 815 return value; 816 case -1406328437: // author 817 this.author = TypeConvertor.castToReference(value); // Reference 818 return value; 819 case 3387378: // note 820 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 821 return value; 822 default: return super.setProperty(hash, name, value); 823 } 824 825 } 826 827 @Override 828 public Base setProperty(String name, Base value) throws FHIRException { 829 if (name.equals("action")) { 830 this.action = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 831 } else if (name.equals("date")) { 832 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 833 } else if (name.equals("author")) { 834 this.author = TypeConvertor.castToReference(value); // Reference 835 } else if (name.equals("note")) { 836 this.getNote().add(TypeConvertor.castToAnnotation(value)); 837 } else 838 return super.setProperty(name, value); 839 return value; 840 } 841 842 @Override 843 public void removeChild(String name, Base value) throws FHIRException { 844 if (name.equals("action")) { 845 this.action = null; 846 } else if (name.equals("date")) { 847 this.date = null; 848 } else if (name.equals("author")) { 849 this.author = null; 850 } else if (name.equals("note")) { 851 this.getNote().remove(value); 852 } else 853 super.removeChild(name, value); 854 855 } 856 857 @Override 858 public Base makeProperty(int hash, String name) throws FHIRException { 859 switch (hash) { 860 case -1422950858: return getAction(); 861 case 3076014: return getDateElement(); 862 case -1406328437: return getAuthor(); 863 case 3387378: return addNote(); 864 default: return super.makeProperty(hash, name); 865 } 866 867 } 868 869 @Override 870 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 871 switch (hash) { 872 case -1422950858: /*action*/ return new String[] {"CodeableConcept"}; 873 case 3076014: /*date*/ return new String[] {"dateTime"}; 874 case -1406328437: /*author*/ return new String[] {"Reference"}; 875 case 3387378: /*note*/ return new String[] {"Annotation"}; 876 default: return super.getTypesForProperty(hash, name); 877 } 878 879 } 880 881 @Override 882 public Base addChild(String name) throws FHIRException { 883 if (name.equals("action")) { 884 this.action = new CodeableConcept(); 885 return this.action; 886 } 887 else if (name.equals("date")) { 888 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.mitigation.date"); 889 } 890 else if (name.equals("author")) { 891 this.author = new Reference(); 892 return this.author; 893 } 894 else if (name.equals("note")) { 895 return addNote(); 896 } 897 else 898 return super.addChild(name); 899 } 900 901 public DetectedIssueMitigationComponent copy() { 902 DetectedIssueMitigationComponent dst = new DetectedIssueMitigationComponent(); 903 copyValues(dst); 904 return dst; 905 } 906 907 public void copyValues(DetectedIssueMitigationComponent dst) { 908 super.copyValues(dst); 909 dst.action = action == null ? null : action.copy(); 910 dst.date = date == null ? null : date.copy(); 911 dst.author = author == null ? null : author.copy(); 912 if (note != null) { 913 dst.note = new ArrayList<Annotation>(); 914 for (Annotation i : note) 915 dst.note.add(i.copy()); 916 }; 917 } 918 919 @Override 920 public boolean equalsDeep(Base other_) { 921 if (!super.equalsDeep(other_)) 922 return false; 923 if (!(other_ instanceof DetectedIssueMitigationComponent)) 924 return false; 925 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 926 return compareDeep(action, o.action, true) && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) 927 && compareDeep(note, o.note, true); 928 } 929 930 @Override 931 public boolean equalsShallow(Base other_) { 932 if (!super.equalsShallow(other_)) 933 return false; 934 if (!(other_ instanceof DetectedIssueMitigationComponent)) 935 return false; 936 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 937 return compareValues(date, o.date, true); 938 } 939 940 public boolean isEmpty() { 941 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, date, author, note 942 ); 943 } 944 945 public String fhirType() { 946 return "DetectedIssue.mitigation"; 947 948 } 949 950 } 951 952 /** 953 * Business identifier associated with the detected issue record. 954 */ 955 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 956 @Description(shortDefinition="Unique id for the detected issue", formalDefinition="Business identifier associated with the detected issue record." ) 957 protected List<Identifier> identifier; 958 959 /** 960 * Indicates the status of the detected issue. 961 */ 962 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 963 @Description(shortDefinition="preliminary | final | entered-in-error | mitigated", formalDefinition="Indicates the status of the detected issue." ) 964 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-status") 965 protected Enumeration<DetectedIssueStatus> status; 966 967 /** 968 * A code that classifies the general type of detected issue. 969 */ 970 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 971 @Description(shortDefinition="Type of detected issue, e.g. drug-drug, duplicate therapy, etc", formalDefinition="A code that classifies the general type of detected issue." ) 972 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-category") 973 protected List<CodeableConcept> category; 974 975 /** 976 * Identifies the specific type of issue identified. 977 */ 978 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 979 @Description(shortDefinition="Specific type of detected issue, e.g. drug-drug, duplicate therapy, etc", formalDefinition="Identifies the specific type of issue identified." ) 980 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-category") 981 protected CodeableConcept code; 982 983 /** 984 * Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 985 */ 986 @Child(name = "severity", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 987 @Description(shortDefinition="high | moderate | low", formalDefinition="Indicates the degree of importance associated with the identified issue based on the potential impact on the patient." ) 988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/detectedissue-severity") 989 protected Enumeration<DetectedIssueSeverity> severity; 990 991 /** 992 * Indicates the subject whose record the detected issue is associated with. 993 */ 994 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class, Organization.class, Procedure.class, Practitioner.class, Medication.class, Substance.class, BiologicallyDerivedProduct.class, NutritionProduct.class}, order=5, min=0, max=1, modifier=false, summary=true) 995 @Description(shortDefinition="Associated subject", formalDefinition="Indicates the subject whose record the detected issue is associated with." ) 996 protected Reference subject; 997 998 /** 999 * The encounter during which this issue was detected. 1000 */ 1001 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 1002 @Description(shortDefinition="Encounter detected issue is part of", formalDefinition="The encounter during which this issue was detected." ) 1003 protected Reference encounter; 1004 1005 /** 1006 * The date or period when the detected issue was initially identified. 1007 */ 1008 @Child(name = "identified", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 1009 @Description(shortDefinition="When identified", formalDefinition="The date or period when the detected issue was initially identified." ) 1010 protected DataType identified; 1011 1012 /** 1013 * Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review. 1014 */ 1015 @Child(name = "author", type = {Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Device.class}, order=8, min=0, max=1, modifier=false, summary=true) 1016 @Description(shortDefinition="The provider or device that identified the issue", formalDefinition="Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review." ) 1017 protected Reference author; 1018 1019 /** 1020 * Indicates the resource representing the current activity or proposed activity that is potentially problematic. 1021 */ 1022 @Child(name = "implicated", type = {Reference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1023 @Description(shortDefinition="Problem resource", formalDefinition="Indicates the resource representing the current activity or proposed activity that is potentially problematic." ) 1024 protected List<Reference> implicated; 1025 1026 /** 1027 * Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport. 1028 */ 1029 @Child(name = "evidence", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1030 @Description(shortDefinition="Supporting evidence", formalDefinition="Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport." ) 1031 protected List<DetectedIssueEvidenceComponent> evidence; 1032 1033 /** 1034 * A textual explanation of the detected issue. 1035 */ 1036 @Child(name = "detail", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1037 @Description(shortDefinition="Description and context", formalDefinition="A textual explanation of the detected issue." ) 1038 protected MarkdownType detail; 1039 1040 /** 1041 * The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 1042 */ 1043 @Child(name = "reference", type = {UriType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1044 @Description(shortDefinition="Authority for issue", formalDefinition="The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified." ) 1045 protected UriType reference; 1046 1047 /** 1048 * Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action. 1049 */ 1050 @Child(name = "mitigation", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1051 @Description(shortDefinition="Step taken to address", formalDefinition="Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action." ) 1052 protected List<DetectedIssueMitigationComponent> mitigation; 1053 1054 private static final long serialVersionUID = 1031242519L; 1055 1056 /** 1057 * Constructor 1058 */ 1059 public DetectedIssue() { 1060 super(); 1061 } 1062 1063 /** 1064 * Constructor 1065 */ 1066 public DetectedIssue(DetectedIssueStatus status) { 1067 super(); 1068 this.setStatus(status); 1069 } 1070 1071 /** 1072 * @return {@link #identifier} (Business identifier associated with the detected issue record.) 1073 */ 1074 public List<Identifier> getIdentifier() { 1075 if (this.identifier == null) 1076 this.identifier = new ArrayList<Identifier>(); 1077 return this.identifier; 1078 } 1079 1080 /** 1081 * @return Returns a reference to <code>this</code> for easy method chaining 1082 */ 1083 public DetectedIssue setIdentifier(List<Identifier> theIdentifier) { 1084 this.identifier = theIdentifier; 1085 return this; 1086 } 1087 1088 public boolean hasIdentifier() { 1089 if (this.identifier == null) 1090 return false; 1091 for (Identifier item : this.identifier) 1092 if (!item.isEmpty()) 1093 return true; 1094 return false; 1095 } 1096 1097 public Identifier addIdentifier() { //3 1098 Identifier t = new Identifier(); 1099 if (this.identifier == null) 1100 this.identifier = new ArrayList<Identifier>(); 1101 this.identifier.add(t); 1102 return t; 1103 } 1104 1105 public DetectedIssue addIdentifier(Identifier t) { //3 1106 if (t == null) 1107 return this; 1108 if (this.identifier == null) 1109 this.identifier = new ArrayList<Identifier>(); 1110 this.identifier.add(t); 1111 return this; 1112 } 1113 1114 /** 1115 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1116 */ 1117 public Identifier getIdentifierFirstRep() { 1118 if (getIdentifier().isEmpty()) { 1119 addIdentifier(); 1120 } 1121 return getIdentifier().get(0); 1122 } 1123 1124 /** 1125 * @return {@link #status} (Indicates the status of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1126 */ 1127 public Enumeration<DetectedIssueStatus> getStatusElement() { 1128 if (this.status == null) 1129 if (Configuration.errorOnAutoCreate()) 1130 throw new Error("Attempt to auto-create DetectedIssue.status"); 1131 else if (Configuration.doAutoCreate()) 1132 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); // bb 1133 return this.status; 1134 } 1135 1136 public boolean hasStatusElement() { 1137 return this.status != null && !this.status.isEmpty(); 1138 } 1139 1140 public boolean hasStatus() { 1141 return this.status != null && !this.status.isEmpty(); 1142 } 1143 1144 /** 1145 * @param value {@link #status} (Indicates the status of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1146 */ 1147 public DetectedIssue setStatusElement(Enumeration<DetectedIssueStatus> value) { 1148 this.status = value; 1149 return this; 1150 } 1151 1152 /** 1153 * @return Indicates the status of the detected issue. 1154 */ 1155 public DetectedIssueStatus getStatus() { 1156 return this.status == null ? null : this.status.getValue(); 1157 } 1158 1159 /** 1160 * @param value Indicates the status of the detected issue. 1161 */ 1162 public DetectedIssue setStatus(DetectedIssueStatus value) { 1163 if (this.status == null) 1164 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); 1165 this.status.setValue(value); 1166 return this; 1167 } 1168 1169 /** 1170 * @return {@link #category} (A code that classifies the general type of detected issue.) 1171 */ 1172 public List<CodeableConcept> getCategory() { 1173 if (this.category == null) 1174 this.category = new ArrayList<CodeableConcept>(); 1175 return this.category; 1176 } 1177 1178 /** 1179 * @return Returns a reference to <code>this</code> for easy method chaining 1180 */ 1181 public DetectedIssue setCategory(List<CodeableConcept> theCategory) { 1182 this.category = theCategory; 1183 return this; 1184 } 1185 1186 public boolean hasCategory() { 1187 if (this.category == null) 1188 return false; 1189 for (CodeableConcept item : this.category) 1190 if (!item.isEmpty()) 1191 return true; 1192 return false; 1193 } 1194 1195 public CodeableConcept addCategory() { //3 1196 CodeableConcept t = new CodeableConcept(); 1197 if (this.category == null) 1198 this.category = new ArrayList<CodeableConcept>(); 1199 this.category.add(t); 1200 return t; 1201 } 1202 1203 public DetectedIssue addCategory(CodeableConcept t) { //3 1204 if (t == null) 1205 return this; 1206 if (this.category == null) 1207 this.category = new ArrayList<CodeableConcept>(); 1208 this.category.add(t); 1209 return this; 1210 } 1211 1212 /** 1213 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1214 */ 1215 public CodeableConcept getCategoryFirstRep() { 1216 if (getCategory().isEmpty()) { 1217 addCategory(); 1218 } 1219 return getCategory().get(0); 1220 } 1221 1222 /** 1223 * @return {@link #code} (Identifies the specific type of issue identified.) 1224 */ 1225 public CodeableConcept getCode() { 1226 if (this.code == null) 1227 if (Configuration.errorOnAutoCreate()) 1228 throw new Error("Attempt to auto-create DetectedIssue.code"); 1229 else if (Configuration.doAutoCreate()) 1230 this.code = new CodeableConcept(); // cc 1231 return this.code; 1232 } 1233 1234 public boolean hasCode() { 1235 return this.code != null && !this.code.isEmpty(); 1236 } 1237 1238 /** 1239 * @param value {@link #code} (Identifies the specific type of issue identified.) 1240 */ 1241 public DetectedIssue setCode(CodeableConcept value) { 1242 this.code = value; 1243 return this; 1244 } 1245 1246 /** 1247 * @return {@link #severity} (Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 1248 */ 1249 public Enumeration<DetectedIssueSeverity> getSeverityElement() { 1250 if (this.severity == null) 1251 if (Configuration.errorOnAutoCreate()) 1252 throw new Error("Attempt to auto-create DetectedIssue.severity"); 1253 else if (Configuration.doAutoCreate()) 1254 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); // bb 1255 return this.severity; 1256 } 1257 1258 public boolean hasSeverityElement() { 1259 return this.severity != null && !this.severity.isEmpty(); 1260 } 1261 1262 public boolean hasSeverity() { 1263 return this.severity != null && !this.severity.isEmpty(); 1264 } 1265 1266 /** 1267 * @param value {@link #severity} (Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 1268 */ 1269 public DetectedIssue setSeverityElement(Enumeration<DetectedIssueSeverity> value) { 1270 this.severity = value; 1271 return this; 1272 } 1273 1274 /** 1275 * @return Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 1276 */ 1277 public DetectedIssueSeverity getSeverity() { 1278 return this.severity == null ? null : this.severity.getValue(); 1279 } 1280 1281 /** 1282 * @param value Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. 1283 */ 1284 public DetectedIssue setSeverity(DetectedIssueSeverity value) { 1285 if (value == null) 1286 this.severity = null; 1287 else { 1288 if (this.severity == null) 1289 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); 1290 this.severity.setValue(value); 1291 } 1292 return this; 1293 } 1294 1295 /** 1296 * @return {@link #subject} (Indicates the subject whose record the detected issue is associated with.) 1297 */ 1298 public Reference getSubject() { 1299 if (this.subject == null) 1300 if (Configuration.errorOnAutoCreate()) 1301 throw new Error("Attempt to auto-create DetectedIssue.subject"); 1302 else if (Configuration.doAutoCreate()) 1303 this.subject = new Reference(); // cc 1304 return this.subject; 1305 } 1306 1307 public boolean hasSubject() { 1308 return this.subject != null && !this.subject.isEmpty(); 1309 } 1310 1311 /** 1312 * @param value {@link #subject} (Indicates the subject whose record the detected issue is associated with.) 1313 */ 1314 public DetectedIssue setSubject(Reference value) { 1315 this.subject = value; 1316 return this; 1317 } 1318 1319 /** 1320 * @return {@link #encounter} (The encounter during which this issue was detected.) 1321 */ 1322 public Reference getEncounter() { 1323 if (this.encounter == null) 1324 if (Configuration.errorOnAutoCreate()) 1325 throw new Error("Attempt to auto-create DetectedIssue.encounter"); 1326 else if (Configuration.doAutoCreate()) 1327 this.encounter = new Reference(); // cc 1328 return this.encounter; 1329 } 1330 1331 public boolean hasEncounter() { 1332 return this.encounter != null && !this.encounter.isEmpty(); 1333 } 1334 1335 /** 1336 * @param value {@link #encounter} (The encounter during which this issue was detected.) 1337 */ 1338 public DetectedIssue setEncounter(Reference value) { 1339 this.encounter = value; 1340 return this; 1341 } 1342 1343 /** 1344 * @return {@link #identified} (The date or period when the detected issue was initially identified.) 1345 */ 1346 public DataType getIdentified() { 1347 return this.identified; 1348 } 1349 1350 /** 1351 * @return {@link #identified} (The date or period when the detected issue was initially identified.) 1352 */ 1353 public DateTimeType getIdentifiedDateTimeType() throws FHIRException { 1354 if (this.identified == null) 1355 this.identified = new DateTimeType(); 1356 if (!(this.identified instanceof DateTimeType)) 1357 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.identified.getClass().getName()+" was encountered"); 1358 return (DateTimeType) this.identified; 1359 } 1360 1361 public boolean hasIdentifiedDateTimeType() { 1362 return this.identified instanceof DateTimeType; 1363 } 1364 1365 /** 1366 * @return {@link #identified} (The date or period when the detected issue was initially identified.) 1367 */ 1368 public Period getIdentifiedPeriod() throws FHIRException { 1369 if (this.identified == null) 1370 this.identified = new Period(); 1371 if (!(this.identified instanceof Period)) 1372 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.identified.getClass().getName()+" was encountered"); 1373 return (Period) this.identified; 1374 } 1375 1376 public boolean hasIdentifiedPeriod() { 1377 return this.identified instanceof Period; 1378 } 1379 1380 public boolean hasIdentified() { 1381 return this.identified != null && !this.identified.isEmpty(); 1382 } 1383 1384 /** 1385 * @param value {@link #identified} (The date or period when the detected issue was initially identified.) 1386 */ 1387 public DetectedIssue setIdentified(DataType value) { 1388 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1389 throw new FHIRException("Not the right type for DetectedIssue.identified[x]: "+value.fhirType()); 1390 this.identified = value; 1391 return this; 1392 } 1393 1394 /** 1395 * @return {@link #author} (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1396 */ 1397 public Reference getAuthor() { 1398 if (this.author == null) 1399 if (Configuration.errorOnAutoCreate()) 1400 throw new Error("Attempt to auto-create DetectedIssue.author"); 1401 else if (Configuration.doAutoCreate()) 1402 this.author = new Reference(); // cc 1403 return this.author; 1404 } 1405 1406 public boolean hasAuthor() { 1407 return this.author != null && !this.author.isEmpty(); 1408 } 1409 1410 /** 1411 * @param value {@link #author} (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) 1412 */ 1413 public DetectedIssue setAuthor(Reference value) { 1414 this.author = value; 1415 return this; 1416 } 1417 1418 /** 1419 * @return {@link #implicated} (Indicates the resource representing the current activity or proposed activity that is potentially problematic.) 1420 */ 1421 public List<Reference> getImplicated() { 1422 if (this.implicated == null) 1423 this.implicated = new ArrayList<Reference>(); 1424 return this.implicated; 1425 } 1426 1427 /** 1428 * @return Returns a reference to <code>this</code> for easy method chaining 1429 */ 1430 public DetectedIssue setImplicated(List<Reference> theImplicated) { 1431 this.implicated = theImplicated; 1432 return this; 1433 } 1434 1435 public boolean hasImplicated() { 1436 if (this.implicated == null) 1437 return false; 1438 for (Reference item : this.implicated) 1439 if (!item.isEmpty()) 1440 return true; 1441 return false; 1442 } 1443 1444 public Reference addImplicated() { //3 1445 Reference t = new Reference(); 1446 if (this.implicated == null) 1447 this.implicated = new ArrayList<Reference>(); 1448 this.implicated.add(t); 1449 return t; 1450 } 1451 1452 public DetectedIssue addImplicated(Reference t) { //3 1453 if (t == null) 1454 return this; 1455 if (this.implicated == null) 1456 this.implicated = new ArrayList<Reference>(); 1457 this.implicated.add(t); 1458 return this; 1459 } 1460 1461 /** 1462 * @return The first repetition of repeating field {@link #implicated}, creating it if it does not already exist {3} 1463 */ 1464 public Reference getImplicatedFirstRep() { 1465 if (getImplicated().isEmpty()) { 1466 addImplicated(); 1467 } 1468 return getImplicated().get(0); 1469 } 1470 1471 /** 1472 * @return {@link #evidence} (Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.) 1473 */ 1474 public List<DetectedIssueEvidenceComponent> getEvidence() { 1475 if (this.evidence == null) 1476 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1477 return this.evidence; 1478 } 1479 1480 /** 1481 * @return Returns a reference to <code>this</code> for easy method chaining 1482 */ 1483 public DetectedIssue setEvidence(List<DetectedIssueEvidenceComponent> theEvidence) { 1484 this.evidence = theEvidence; 1485 return this; 1486 } 1487 1488 public boolean hasEvidence() { 1489 if (this.evidence == null) 1490 return false; 1491 for (DetectedIssueEvidenceComponent item : this.evidence) 1492 if (!item.isEmpty()) 1493 return true; 1494 return false; 1495 } 1496 1497 public DetectedIssueEvidenceComponent addEvidence() { //3 1498 DetectedIssueEvidenceComponent t = new DetectedIssueEvidenceComponent(); 1499 if (this.evidence == null) 1500 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1501 this.evidence.add(t); 1502 return t; 1503 } 1504 1505 public DetectedIssue addEvidence(DetectedIssueEvidenceComponent t) { //3 1506 if (t == null) 1507 return this; 1508 if (this.evidence == null) 1509 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1510 this.evidence.add(t); 1511 return this; 1512 } 1513 1514 /** 1515 * @return The first repetition of repeating field {@link #evidence}, creating it if it does not already exist {3} 1516 */ 1517 public DetectedIssueEvidenceComponent getEvidenceFirstRep() { 1518 if (getEvidence().isEmpty()) { 1519 addEvidence(); 1520 } 1521 return getEvidence().get(0); 1522 } 1523 1524 /** 1525 * @return {@link #detail} (A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1526 */ 1527 public MarkdownType getDetailElement() { 1528 if (this.detail == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create DetectedIssue.detail"); 1531 else if (Configuration.doAutoCreate()) 1532 this.detail = new MarkdownType(); // bb 1533 return this.detail; 1534 } 1535 1536 public boolean hasDetailElement() { 1537 return this.detail != null && !this.detail.isEmpty(); 1538 } 1539 1540 public boolean hasDetail() { 1541 return this.detail != null && !this.detail.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #detail} (A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1546 */ 1547 public DetectedIssue setDetailElement(MarkdownType value) { 1548 this.detail = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return A textual explanation of the detected issue. 1554 */ 1555 public String getDetail() { 1556 return this.detail == null ? null : this.detail.getValue(); 1557 } 1558 1559 /** 1560 * @param value A textual explanation of the detected issue. 1561 */ 1562 public DetectedIssue setDetail(String value) { 1563 if (Utilities.noString(value)) 1564 this.detail = null; 1565 else { 1566 if (this.detail == null) 1567 this.detail = new MarkdownType(); 1568 this.detail.setValue(value); 1569 } 1570 return this; 1571 } 1572 1573 /** 1574 * @return {@link #reference} (The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1575 */ 1576 public UriType getReferenceElement() { 1577 if (this.reference == null) 1578 if (Configuration.errorOnAutoCreate()) 1579 throw new Error("Attempt to auto-create DetectedIssue.reference"); 1580 else if (Configuration.doAutoCreate()) 1581 this.reference = new UriType(); // bb 1582 return this.reference; 1583 } 1584 1585 public boolean hasReferenceElement() { 1586 return this.reference != null && !this.reference.isEmpty(); 1587 } 1588 1589 public boolean hasReference() { 1590 return this.reference != null && !this.reference.isEmpty(); 1591 } 1592 1593 /** 1594 * @param value {@link #reference} (The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 1595 */ 1596 public DetectedIssue setReferenceElement(UriType value) { 1597 this.reference = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 1603 */ 1604 public String getReference() { 1605 return this.reference == null ? null : this.reference.getValue(); 1606 } 1607 1608 /** 1609 * @param value The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. 1610 */ 1611 public DetectedIssue setReference(String value) { 1612 if (Utilities.noString(value)) 1613 this.reference = null; 1614 else { 1615 if (this.reference == null) 1616 this.reference = new UriType(); 1617 this.reference.setValue(value); 1618 } 1619 return this; 1620 } 1621 1622 /** 1623 * @return {@link #mitigation} (Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.) 1624 */ 1625 public List<DetectedIssueMitigationComponent> getMitigation() { 1626 if (this.mitigation == null) 1627 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1628 return this.mitigation; 1629 } 1630 1631 /** 1632 * @return Returns a reference to <code>this</code> for easy method chaining 1633 */ 1634 public DetectedIssue setMitigation(List<DetectedIssueMitigationComponent> theMitigation) { 1635 this.mitigation = theMitigation; 1636 return this; 1637 } 1638 1639 public boolean hasMitigation() { 1640 if (this.mitigation == null) 1641 return false; 1642 for (DetectedIssueMitigationComponent item : this.mitigation) 1643 if (!item.isEmpty()) 1644 return true; 1645 return false; 1646 } 1647 1648 public DetectedIssueMitigationComponent addMitigation() { //3 1649 DetectedIssueMitigationComponent t = new DetectedIssueMitigationComponent(); 1650 if (this.mitigation == null) 1651 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1652 this.mitigation.add(t); 1653 return t; 1654 } 1655 1656 public DetectedIssue addMitigation(DetectedIssueMitigationComponent t) { //3 1657 if (t == null) 1658 return this; 1659 if (this.mitigation == null) 1660 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1661 this.mitigation.add(t); 1662 return this; 1663 } 1664 1665 /** 1666 * @return The first repetition of repeating field {@link #mitigation}, creating it if it does not already exist {3} 1667 */ 1668 public DetectedIssueMitigationComponent getMitigationFirstRep() { 1669 if (getMitigation().isEmpty()) { 1670 addMitigation(); 1671 } 1672 return getMitigation().get(0); 1673 } 1674 1675 protected void listChildren(List<Property> children) { 1676 super.listChildren(children); 1677 children.add(new Property("identifier", "Identifier", "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1678 children.add(new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status)); 1679 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of detected issue.", 0, java.lang.Integer.MAX_VALUE, category)); 1680 children.add(new Property("code", "CodeableConcept", "Identifies the specific type of issue identified.", 0, 1, code)); 1681 children.add(new Property("severity", "code", "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 0, 1, severity)); 1682 children.add(new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|BiologicallyDerivedProduct|NutritionProduct)", "Indicates the subject whose record the detected issue is associated with.", 0, 1, subject)); 1683 children.add(new Property("encounter", "Reference(Encounter)", "The encounter during which this issue was detected.", 0, 1, encounter)); 1684 children.add(new Property("identified[x]", "dateTime|Period", "The date or period when the detected issue was initially identified.", 0, 1, identified)); 1685 children.add(new Property("author", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device)", "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 0, 1, author)); 1686 children.add(new Property("implicated", "Reference(Any)", "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 0, java.lang.Integer.MAX_VALUE, implicated)); 1687 children.add(new Property("evidence", "", "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 0, java.lang.Integer.MAX_VALUE, evidence)); 1688 children.add(new Property("detail", "markdown", "A textual explanation of the detected issue.", 0, 1, detail)); 1689 children.add(new Property("reference", "uri", "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 0, 1, reference)); 1690 children.add(new Property("mitigation", "", "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 0, java.lang.Integer.MAX_VALUE, mitigation)); 1691 } 1692 1693 @Override 1694 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1695 switch (_hash) { 1696 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier); 1697 case -892481550: /*status*/ return new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status); 1698 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of detected issue.", 0, java.lang.Integer.MAX_VALUE, category); 1699 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identifies the specific type of issue identified.", 0, 1, code); 1700 case 1478300413: /*severity*/ return new Property("severity", "code", "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 0, 1, severity); 1701 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|BiologicallyDerivedProduct|NutritionProduct)", "Indicates the subject whose record the detected issue is associated with.", 0, 1, subject); 1702 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter during which this issue was detected.", 0, 1, encounter); 1703 case 569355781: /*identified[x]*/ return new Property("identified[x]", "dateTime|Period", "The date or period when the detected issue was initially identified.", 0, 1, identified); 1704 case -1618432869: /*identified*/ return new Property("identified[x]", "dateTime|Period", "The date or period when the detected issue was initially identified.", 0, 1, identified); 1705 case -968788650: /*identifiedDateTime*/ return new Property("identified[x]", "dateTime", "The date or period when the detected issue was initially identified.", 0, 1, identified); 1706 case 520482364: /*identifiedPeriod*/ return new Property("identified[x]", "Period", "The date or period when the detected issue was initially identified.", 0, 1, identified); 1707 case -1406328437: /*author*/ return new Property("author", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device)", "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 0, 1, author); 1708 case -810216884: /*implicated*/ return new Property("implicated", "Reference(Any)", "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 0, java.lang.Integer.MAX_VALUE, implicated); 1709 case 382967383: /*evidence*/ return new Property("evidence", "", "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 0, java.lang.Integer.MAX_VALUE, evidence); 1710 case -1335224239: /*detail*/ return new Property("detail", "markdown", "A textual explanation of the detected issue.", 0, 1, detail); 1711 case -925155509: /*reference*/ return new Property("reference", "uri", "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 0, 1, reference); 1712 case 1293793087: /*mitigation*/ return new Property("mitigation", "", "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 0, java.lang.Integer.MAX_VALUE, mitigation); 1713 default: return super.getNamedProperty(_hash, _name, _checkValid); 1714 } 1715 1716 } 1717 1718 @Override 1719 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1720 switch (hash) { 1721 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1722 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DetectedIssueStatus> 1723 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1724 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1725 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<DetectedIssueSeverity> 1726 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1727 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1728 case -1618432869: /*identified*/ return this.identified == null ? new Base[0] : new Base[] {this.identified}; // DataType 1729 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1730 case -810216884: /*implicated*/ return this.implicated == null ? new Base[0] : this.implicated.toArray(new Base[this.implicated.size()]); // Reference 1731 case 382967383: /*evidence*/ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // DetectedIssueEvidenceComponent 1732 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // MarkdownType 1733 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // UriType 1734 case 1293793087: /*mitigation*/ return this.mitigation == null ? new Base[0] : this.mitigation.toArray(new Base[this.mitigation.size()]); // DetectedIssueMitigationComponent 1735 default: return super.getProperty(hash, name, checkValid); 1736 } 1737 1738 } 1739 1740 @Override 1741 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1742 switch (hash) { 1743 case -1618432855: // identifier 1744 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1745 return value; 1746 case -892481550: // status 1747 value = new DetectedIssueStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1748 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 1749 return value; 1750 case 50511102: // category 1751 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1752 return value; 1753 case 3059181: // code 1754 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1755 return value; 1756 case 1478300413: // severity 1757 value = new DetectedIssueSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1758 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 1759 return value; 1760 case -1867885268: // subject 1761 this.subject = TypeConvertor.castToReference(value); // Reference 1762 return value; 1763 case 1524132147: // encounter 1764 this.encounter = TypeConvertor.castToReference(value); // Reference 1765 return value; 1766 case -1618432869: // identified 1767 this.identified = TypeConvertor.castToType(value); // DataType 1768 return value; 1769 case -1406328437: // author 1770 this.author = TypeConvertor.castToReference(value); // Reference 1771 return value; 1772 case -810216884: // implicated 1773 this.getImplicated().add(TypeConvertor.castToReference(value)); // Reference 1774 return value; 1775 case 382967383: // evidence 1776 this.getEvidence().add((DetectedIssueEvidenceComponent) value); // DetectedIssueEvidenceComponent 1777 return value; 1778 case -1335224239: // detail 1779 this.detail = TypeConvertor.castToMarkdown(value); // MarkdownType 1780 return value; 1781 case -925155509: // reference 1782 this.reference = TypeConvertor.castToUri(value); // UriType 1783 return value; 1784 case 1293793087: // mitigation 1785 this.getMitigation().add((DetectedIssueMitigationComponent) value); // DetectedIssueMitigationComponent 1786 return value; 1787 default: return super.setProperty(hash, name, value); 1788 } 1789 1790 } 1791 1792 @Override 1793 public Base setProperty(String name, Base value) throws FHIRException { 1794 if (name.equals("identifier")) { 1795 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1796 } else if (name.equals("status")) { 1797 value = new DetectedIssueStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1798 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 1799 } else if (name.equals("category")) { 1800 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1801 } else if (name.equals("code")) { 1802 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1803 } else if (name.equals("severity")) { 1804 value = new DetectedIssueSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1805 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 1806 } else if (name.equals("subject")) { 1807 this.subject = TypeConvertor.castToReference(value); // Reference 1808 } else if (name.equals("encounter")) { 1809 this.encounter = TypeConvertor.castToReference(value); // Reference 1810 } else if (name.equals("identified[x]")) { 1811 this.identified = TypeConvertor.castToType(value); // DataType 1812 } else if (name.equals("author")) { 1813 this.author = TypeConvertor.castToReference(value); // Reference 1814 } else if (name.equals("implicated")) { 1815 this.getImplicated().add(TypeConvertor.castToReference(value)); 1816 } else if (name.equals("evidence")) { 1817 this.getEvidence().add((DetectedIssueEvidenceComponent) value); 1818 } else if (name.equals("detail")) { 1819 this.detail = TypeConvertor.castToMarkdown(value); // MarkdownType 1820 } else if (name.equals("reference")) { 1821 this.reference = TypeConvertor.castToUri(value); // UriType 1822 } else if (name.equals("mitigation")) { 1823 this.getMitigation().add((DetectedIssueMitigationComponent) value); 1824 } else 1825 return super.setProperty(name, value); 1826 return value; 1827 } 1828 1829 @Override 1830 public void removeChild(String name, Base value) throws FHIRException { 1831 if (name.equals("identifier")) { 1832 this.getIdentifier().remove(value); 1833 } else if (name.equals("status")) { 1834 value = new DetectedIssueStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1835 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 1836 } else if (name.equals("category")) { 1837 this.getCategory().remove(value); 1838 } else if (name.equals("code")) { 1839 this.code = null; 1840 } else if (name.equals("severity")) { 1841 value = new DetectedIssueSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1842 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 1843 } else if (name.equals("subject")) { 1844 this.subject = null; 1845 } else if (name.equals("encounter")) { 1846 this.encounter = null; 1847 } else if (name.equals("identified[x]")) { 1848 this.identified = null; 1849 } else if (name.equals("author")) { 1850 this.author = null; 1851 } else if (name.equals("implicated")) { 1852 this.getImplicated().remove(value); 1853 } else if (name.equals("evidence")) { 1854 this.getEvidence().remove((DetectedIssueEvidenceComponent) value); 1855 } else if (name.equals("detail")) { 1856 this.detail = null; 1857 } else if (name.equals("reference")) { 1858 this.reference = null; 1859 } else if (name.equals("mitigation")) { 1860 this.getMitigation().remove((DetectedIssueMitigationComponent) value); 1861 } else 1862 super.removeChild(name, value); 1863 1864 } 1865 1866 @Override 1867 public Base makeProperty(int hash, String name) throws FHIRException { 1868 switch (hash) { 1869 case -1618432855: return addIdentifier(); 1870 case -892481550: return getStatusElement(); 1871 case 50511102: return addCategory(); 1872 case 3059181: return getCode(); 1873 case 1478300413: return getSeverityElement(); 1874 case -1867885268: return getSubject(); 1875 case 1524132147: return getEncounter(); 1876 case 569355781: return getIdentified(); 1877 case -1618432869: return getIdentified(); 1878 case -1406328437: return getAuthor(); 1879 case -810216884: return addImplicated(); 1880 case 382967383: return addEvidence(); 1881 case -1335224239: return getDetailElement(); 1882 case -925155509: return getReferenceElement(); 1883 case 1293793087: return addMitigation(); 1884 default: return super.makeProperty(hash, name); 1885 } 1886 1887 } 1888 1889 @Override 1890 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1891 switch (hash) { 1892 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1893 case -892481550: /*status*/ return new String[] {"code"}; 1894 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1895 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1896 case 1478300413: /*severity*/ return new String[] {"code"}; 1897 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1898 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1899 case -1618432869: /*identified*/ return new String[] {"dateTime", "Period"}; 1900 case -1406328437: /*author*/ return new String[] {"Reference"}; 1901 case -810216884: /*implicated*/ return new String[] {"Reference"}; 1902 case 382967383: /*evidence*/ return new String[] {}; 1903 case -1335224239: /*detail*/ return new String[] {"markdown"}; 1904 case -925155509: /*reference*/ return new String[] {"uri"}; 1905 case 1293793087: /*mitigation*/ return new String[] {}; 1906 default: return super.getTypesForProperty(hash, name); 1907 } 1908 1909 } 1910 1911 @Override 1912 public Base addChild(String name) throws FHIRException { 1913 if (name.equals("identifier")) { 1914 return addIdentifier(); 1915 } 1916 else if (name.equals("status")) { 1917 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.status"); 1918 } 1919 else if (name.equals("category")) { 1920 return addCategory(); 1921 } 1922 else if (name.equals("code")) { 1923 this.code = new CodeableConcept(); 1924 return this.code; 1925 } 1926 else if (name.equals("severity")) { 1927 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.severity"); 1928 } 1929 else if (name.equals("subject")) { 1930 this.subject = new Reference(); 1931 return this.subject; 1932 } 1933 else if (name.equals("encounter")) { 1934 this.encounter = new Reference(); 1935 return this.encounter; 1936 } 1937 else if (name.equals("identifiedDateTime")) { 1938 this.identified = new DateTimeType(); 1939 return this.identified; 1940 } 1941 else if (name.equals("identifiedPeriod")) { 1942 this.identified = new Period(); 1943 return this.identified; 1944 } 1945 else if (name.equals("author")) { 1946 this.author = new Reference(); 1947 return this.author; 1948 } 1949 else if (name.equals("implicated")) { 1950 return addImplicated(); 1951 } 1952 else if (name.equals("evidence")) { 1953 return addEvidence(); 1954 } 1955 else if (name.equals("detail")) { 1956 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.detail"); 1957 } 1958 else if (name.equals("reference")) { 1959 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.reference"); 1960 } 1961 else if (name.equals("mitigation")) { 1962 return addMitigation(); 1963 } 1964 else 1965 return super.addChild(name); 1966 } 1967 1968 public String fhirType() { 1969 return "DetectedIssue"; 1970 1971 } 1972 1973 public DetectedIssue copy() { 1974 DetectedIssue dst = new DetectedIssue(); 1975 copyValues(dst); 1976 return dst; 1977 } 1978 1979 public void copyValues(DetectedIssue dst) { 1980 super.copyValues(dst); 1981 if (identifier != null) { 1982 dst.identifier = new ArrayList<Identifier>(); 1983 for (Identifier i : identifier) 1984 dst.identifier.add(i.copy()); 1985 }; 1986 dst.status = status == null ? null : status.copy(); 1987 if (category != null) { 1988 dst.category = new ArrayList<CodeableConcept>(); 1989 for (CodeableConcept i : category) 1990 dst.category.add(i.copy()); 1991 }; 1992 dst.code = code == null ? null : code.copy(); 1993 dst.severity = severity == null ? null : severity.copy(); 1994 dst.subject = subject == null ? null : subject.copy(); 1995 dst.encounter = encounter == null ? null : encounter.copy(); 1996 dst.identified = identified == null ? null : identified.copy(); 1997 dst.author = author == null ? null : author.copy(); 1998 if (implicated != null) { 1999 dst.implicated = new ArrayList<Reference>(); 2000 for (Reference i : implicated) 2001 dst.implicated.add(i.copy()); 2002 }; 2003 if (evidence != null) { 2004 dst.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 2005 for (DetectedIssueEvidenceComponent i : evidence) 2006 dst.evidence.add(i.copy()); 2007 }; 2008 dst.detail = detail == null ? null : detail.copy(); 2009 dst.reference = reference == null ? null : reference.copy(); 2010 if (mitigation != null) { 2011 dst.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 2012 for (DetectedIssueMitigationComponent i : mitigation) 2013 dst.mitigation.add(i.copy()); 2014 }; 2015 } 2016 2017 protected DetectedIssue typedCopy() { 2018 return copy(); 2019 } 2020 2021 @Override 2022 public boolean equalsDeep(Base other_) { 2023 if (!super.equalsDeep(other_)) 2024 return false; 2025 if (!(other_ instanceof DetectedIssue)) 2026 return false; 2027 DetectedIssue o = (DetectedIssue) other_; 2028 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 2029 && compareDeep(code, o.code, true) && compareDeep(severity, o.severity, true) && compareDeep(subject, o.subject, true) 2030 && compareDeep(encounter, o.encounter, true) && compareDeep(identified, o.identified, true) && compareDeep(author, o.author, true) 2031 && compareDeep(implicated, o.implicated, true) && compareDeep(evidence, o.evidence, true) && compareDeep(detail, o.detail, true) 2032 && compareDeep(reference, o.reference, true) && compareDeep(mitigation, o.mitigation, true); 2033 } 2034 2035 @Override 2036 public boolean equalsShallow(Base other_) { 2037 if (!super.equalsShallow(other_)) 2038 return false; 2039 if (!(other_ instanceof DetectedIssue)) 2040 return false; 2041 DetectedIssue o = (DetectedIssue) other_; 2042 return compareValues(status, o.status, true) && compareValues(severity, o.severity, true) && compareValues(detail, o.detail, true) 2043 && compareValues(reference, o.reference, true); 2044 } 2045 2046 public boolean isEmpty() { 2047 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 2048 , code, severity, subject, encounter, identified, author, implicated, evidence 2049 , detail, reference, mitigation); 2050 } 2051 2052 @Override 2053 public ResourceType getResourceType() { 2054 return ResourceType.DetectedIssue; 2055 } 2056 2057 /** 2058 * Search parameter: <b>author</b> 2059 * <p> 2060 * Description: <b>The provider or device that identified the issue</b><br> 2061 * Type: <b>reference</b><br> 2062 * Path: <b>DetectedIssue.author</b><br> 2063 * </p> 2064 */ 2065 @SearchParamDefinition(name="author", path="DetectedIssue.author", description="The provider or device that identified the issue", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2066 public static final String SP_AUTHOR = "author"; 2067 /** 2068 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2069 * <p> 2070 * Description: <b>The provider or device that identified the issue</b><br> 2071 * Type: <b>reference</b><br> 2072 * Path: <b>DetectedIssue.author</b><br> 2073 * </p> 2074 */ 2075 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2076 2077/** 2078 * Constant for fluent queries to be used to add include statements. Specifies 2079 * the path value of "<b>DetectedIssue:author</b>". 2080 */ 2081 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("DetectedIssue:author").toLocked(); 2082 2083 /** 2084 * Search parameter: <b>category</b> 2085 * <p> 2086 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, etc.</b><br> 2087 * Type: <b>token</b><br> 2088 * Path: <b>DetectedIssue.category</b><br> 2089 * </p> 2090 */ 2091 @SearchParamDefinition(name="category", path="DetectedIssue.category", description="Issue Category, e.g. drug-drug, duplicate therapy, etc.", type="token" ) 2092 public static final String SP_CATEGORY = "category"; 2093 /** 2094 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2095 * <p> 2096 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, etc.</b><br> 2097 * Type: <b>token</b><br> 2098 * Path: <b>DetectedIssue.category</b><br> 2099 * </p> 2100 */ 2101 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2102 2103 /** 2104 * Search parameter: <b>identified</b> 2105 * <p> 2106 * Description: <b>When identified</b><br> 2107 * Type: <b>date</b><br> 2108 * Path: <b>DetectedIssue.identified.ofType(dateTime) | DetectedIssue.identified.ofType(Period)</b><br> 2109 * </p> 2110 */ 2111 @SearchParamDefinition(name="identified", path="DetectedIssue.identified.ofType(dateTime) | DetectedIssue.identified.ofType(Period)", description="When identified", type="date" ) 2112 public static final String SP_IDENTIFIED = "identified"; 2113 /** 2114 * <b>Fluent Client</b> search parameter constant for <b>identified</b> 2115 * <p> 2116 * Description: <b>When identified</b><br> 2117 * Type: <b>date</b><br> 2118 * Path: <b>DetectedIssue.identified.ofType(dateTime) | DetectedIssue.identified.ofType(Period)</b><br> 2119 * </p> 2120 */ 2121 public static final ca.uhn.fhir.rest.gclient.DateClientParam IDENTIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_IDENTIFIED); 2122 2123 /** 2124 * Search parameter: <b>implicated</b> 2125 * <p> 2126 * Description: <b>Problem resource</b><br> 2127 * Type: <b>reference</b><br> 2128 * Path: <b>DetectedIssue.implicated</b><br> 2129 * </p> 2130 */ 2131 @SearchParamDefinition(name="implicated", path="DetectedIssue.implicated", description="Problem resource", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2132 public static final String SP_IMPLICATED = "implicated"; 2133 /** 2134 * <b>Fluent Client</b> search parameter constant for <b>implicated</b> 2135 * <p> 2136 * Description: <b>Problem resource</b><br> 2137 * Type: <b>reference</b><br> 2138 * Path: <b>DetectedIssue.implicated</b><br> 2139 * </p> 2140 */ 2141 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMPLICATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_IMPLICATED); 2142 2143/** 2144 * Constant for fluent queries to be used to add include statements. Specifies 2145 * the path value of "<b>DetectedIssue:implicated</b>". 2146 */ 2147 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMPLICATED = new ca.uhn.fhir.model.api.Include("DetectedIssue:implicated").toLocked(); 2148 2149 /** 2150 * Search parameter: <b>status</b> 2151 * <p> 2152 * Description: <b>The status of the issue</b><br> 2153 * Type: <b>token</b><br> 2154 * Path: <b>DetectedIssue.status</b><br> 2155 * </p> 2156 */ 2157 @SearchParamDefinition(name="status", path="DetectedIssue.status", description="The status of the issue", type="token" ) 2158 public static final String SP_STATUS = "status"; 2159 /** 2160 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2161 * <p> 2162 * Description: <b>The status of the issue</b><br> 2163 * Type: <b>token</b><br> 2164 * Path: <b>DetectedIssue.status</b><br> 2165 * </p> 2166 */ 2167 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2168 2169 /** 2170 * Search parameter: <b>subject</b> 2171 * <p> 2172 * Description: <b>Associated subject</b><br> 2173 * Type: <b>reference</b><br> 2174 * Path: <b>DetectedIssue.subject</b><br> 2175 * </p> 2176 */ 2177 @SearchParamDefinition(name="subject", path="DetectedIssue.subject", description="Associated subject", type="reference", target={BiologicallyDerivedProduct.class, Device.class, Group.class, Location.class, Medication.class, NutritionProduct.class, Organization.class, Patient.class, Practitioner.class, Procedure.class, Substance.class } ) 2178 public static final String SP_SUBJECT = "subject"; 2179 /** 2180 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2181 * <p> 2182 * Description: <b>Associated subject</b><br> 2183 * Type: <b>reference</b><br> 2184 * Path: <b>DetectedIssue.subject</b><br> 2185 * </p> 2186 */ 2187 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2188 2189/** 2190 * Constant for fluent queries to be used to add include statements. Specifies 2191 * the path value of "<b>DetectedIssue:subject</b>". 2192 */ 2193 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DetectedIssue:subject").toLocked(); 2194 2195 /** 2196 * Search parameter: <b>code</b> 2197 * <p> 2198 * Description: <b>Multiple Resources: 2199 2200* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2201* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2202* [AuditEvent](auditevent.html): More specific code for the event 2203* [Basic](basic.html): Kind of Resource 2204* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2205* [Condition](condition.html): Code for the condition 2206* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2207* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2208* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2209* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2210* [ImagingSelection](imagingselection.html): The imaging selection status 2211* [List](list.html): What the purpose of this list is 2212* [Medication](medication.html): Returns medications for a specific code 2213* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2214* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2215* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2216* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2217* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2218* [Observation](observation.html): The code of the observation type 2219* [Procedure](procedure.html): A code to identify a procedure 2220* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2221* [Task](task.html): Search by task code 2222</b><br> 2223 * Type: <b>token</b><br> 2224 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2225 * </p> 2226 */ 2227 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2228 public static final String SP_CODE = "code"; 2229 /** 2230 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2231 * <p> 2232 * Description: <b>Multiple Resources: 2233 2234* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2235* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2236* [AuditEvent](auditevent.html): More specific code for the event 2237* [Basic](basic.html): Kind of Resource 2238* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2239* [Condition](condition.html): Code for the condition 2240* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2241* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2242* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2243* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2244* [ImagingSelection](imagingselection.html): The imaging selection status 2245* [List](list.html): What the purpose of this list is 2246* [Medication](medication.html): Returns medications for a specific code 2247* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2248* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2249* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2250* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2251* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2252* [Observation](observation.html): The code of the observation type 2253* [Procedure](procedure.html): A code to identify a procedure 2254* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2255* [Task](task.html): Search by task code 2256</b><br> 2257 * Type: <b>token</b><br> 2258 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2259 * </p> 2260 */ 2261 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2262 2263 /** 2264 * Search parameter: <b>identifier</b> 2265 * <p> 2266 * Description: <b>Multiple Resources: 2267 2268* [Account](account.html): Account number 2269* [AdverseEvent](adverseevent.html): Business identifier for the event 2270* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2271* [Appointment](appointment.html): An Identifier of the Appointment 2272* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2273* [Basic](basic.html): Business identifier 2274* [BodyStructure](bodystructure.html): Bodystructure identifier 2275* [CarePlan](careplan.html): External Ids for this plan 2276* [CareTeam](careteam.html): External Ids for this team 2277* [ChargeItem](chargeitem.html): Business Identifier for item 2278* [Claim](claim.html): The primary identifier of the financial resource 2279* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2280* [ClinicalImpression](clinicalimpression.html): Business identifier 2281* [Communication](communication.html): Unique identifier 2282* [CommunicationRequest](communicationrequest.html): Unique identifier 2283* [Composition](composition.html): Version-independent identifier for the Composition 2284* [Condition](condition.html): A unique identifier of the condition record 2285* [Consent](consent.html): Identifier for this record (external references) 2286* [Contract](contract.html): The identity of the contract 2287* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2288* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2289* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2290* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2291* [DeviceRequest](devicerequest.html): Business identifier for request/order 2292* [DeviceUsage](deviceusage.html): Search by identifier 2293* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2294* [DocumentReference](documentreference.html): Identifier of the attachment binary 2295* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2296* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2297* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2298* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2299* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2300* [Flag](flag.html): Business identifier 2301* [Goal](goal.html): External Ids for this goal 2302* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2303* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2304* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2305* [Immunization](immunization.html): Business identifier 2306* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2307* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2308* [Invoice](invoice.html): Business Identifier for item 2309* [List](list.html): Business identifier 2310* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2311* [Medication](medication.html): Returns medications with this external identifier 2312* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2313* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2314* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2315* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2316* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2317* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2318* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2319* [Observation](observation.html): The unique id for a particular observation 2320* [Person](person.html): A person Identifier 2321* [Procedure](procedure.html): A unique identifier for a procedure 2322* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2323* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2324* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2325* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2326* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2327* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2328* [Specimen](specimen.html): The unique identifier associated with the specimen 2329* [SupplyDelivery](supplydelivery.html): External identifier 2330* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2331* [Task](task.html): Search for a task instance by its business identifier 2332* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2333</b><br> 2334 * Type: <b>token</b><br> 2335 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2336 * </p> 2337 */ 2338 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2339 public static final String SP_IDENTIFIER = "identifier"; 2340 /** 2341 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2342 * <p> 2343 * Description: <b>Multiple Resources: 2344 2345* [Account](account.html): Account number 2346* [AdverseEvent](adverseevent.html): Business identifier for the event 2347* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2348* [Appointment](appointment.html): An Identifier of the Appointment 2349* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2350* [Basic](basic.html): Business identifier 2351* [BodyStructure](bodystructure.html): Bodystructure identifier 2352* [CarePlan](careplan.html): External Ids for this plan 2353* [CareTeam](careteam.html): External Ids for this team 2354* [ChargeItem](chargeitem.html): Business Identifier for item 2355* [Claim](claim.html): The primary identifier of the financial resource 2356* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2357* [ClinicalImpression](clinicalimpression.html): Business identifier 2358* [Communication](communication.html): Unique identifier 2359* [CommunicationRequest](communicationrequest.html): Unique identifier 2360* [Composition](composition.html): Version-independent identifier for the Composition 2361* [Condition](condition.html): A unique identifier of the condition record 2362* [Consent](consent.html): Identifier for this record (external references) 2363* [Contract](contract.html): The identity of the contract 2364* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2365* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2366* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2367* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2368* [DeviceRequest](devicerequest.html): Business identifier for request/order 2369* [DeviceUsage](deviceusage.html): Search by identifier 2370* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2371* [DocumentReference](documentreference.html): Identifier of the attachment binary 2372* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2373* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2374* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2375* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2376* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2377* [Flag](flag.html): Business identifier 2378* [Goal](goal.html): External Ids for this goal 2379* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2380* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2381* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2382* [Immunization](immunization.html): Business identifier 2383* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2384* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2385* [Invoice](invoice.html): Business Identifier for item 2386* [List](list.html): Business identifier 2387* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2388* [Medication](medication.html): Returns medications with this external identifier 2389* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2390* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2391* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2392* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2393* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2394* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2395* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2396* [Observation](observation.html): The unique id for a particular observation 2397* [Person](person.html): A person Identifier 2398* [Procedure](procedure.html): A unique identifier for a procedure 2399* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2400* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2401* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2402* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2403* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2404* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2405* [Specimen](specimen.html): The unique identifier associated with the specimen 2406* [SupplyDelivery](supplydelivery.html): External identifier 2407* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2408* [Task](task.html): Search for a task instance by its business identifier 2409* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2410</b><br> 2411 * Type: <b>token</b><br> 2412 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2413 * </p> 2414 */ 2415 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2416 2417 /** 2418 * Search parameter: <b>patient</b> 2419 * <p> 2420 * Description: <b>Multiple Resources: 2421 2422* [Account](account.html): The entity that caused the expenses 2423* [AdverseEvent](adverseevent.html): Subject impacted by event 2424* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2425* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2426* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2427* [AuditEvent](auditevent.html): Where the activity involved patient data 2428* [Basic](basic.html): Identifies the focus of this resource 2429* [BodyStructure](bodystructure.html): Who this is about 2430* [CarePlan](careplan.html): Who the care plan is for 2431* [CareTeam](careteam.html): Who care team is for 2432* [ChargeItem](chargeitem.html): Individual service was done for/to 2433* [Claim](claim.html): Patient receiving the products or services 2434* [ClaimResponse](claimresponse.html): The subject of care 2435* [ClinicalImpression](clinicalimpression.html): Patient assessed 2436* [Communication](communication.html): Focus of message 2437* [CommunicationRequest](communicationrequest.html): Focus of message 2438* [Composition](composition.html): Who and/or what the composition is about 2439* [Condition](condition.html): Who has the condition? 2440* [Consent](consent.html): Who the consent applies to 2441* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2442* [Coverage](coverage.html): Retrieve coverages for a patient 2443* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2444* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2445* [DetectedIssue](detectedissue.html): Associated patient 2446* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2447* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2448* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2449* [DocumentReference](documentreference.html): Who/what is the subject of the document 2450* [Encounter](encounter.html): The patient present at the encounter 2451* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2452* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2453* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2454* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2455* [Flag](flag.html): The identity of a subject to list flags for 2456* [Goal](goal.html): Who this goal is intended for 2457* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2458* [ImagingSelection](imagingselection.html): Who the study is about 2459* [ImagingStudy](imagingstudy.html): Who the study is about 2460* [Immunization](immunization.html): The patient for the vaccination record 2461* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2462* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2463* [Invoice](invoice.html): Recipient(s) of goods and services 2464* [List](list.html): If all resources have the same subject 2465* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2466* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2467* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2468* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2469* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2470* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2471* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2472* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2473* [Observation](observation.html): The subject that the observation is about (if patient) 2474* [Person](person.html): The Person links to this Patient 2475* [Procedure](procedure.html): Search by subject - a patient 2476* [Provenance](provenance.html): Where the activity involved patient data 2477* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2478* [RelatedPerson](relatedperson.html): The patient this related person is related to 2479* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2480* [ResearchSubject](researchsubject.html): Who or what is part of study 2481* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2482* [ServiceRequest](servicerequest.html): Search by subject - a patient 2483* [Specimen](specimen.html): The patient the specimen comes from 2484* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2485* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2486* [Task](task.html): Search by patient 2487* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2488</b><br> 2489 * Type: <b>reference</b><br> 2490 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2491 * </p> 2492 */ 2493 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2494 public static final String SP_PATIENT = "patient"; 2495 /** 2496 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2497 * <p> 2498 * Description: <b>Multiple Resources: 2499 2500* [Account](account.html): The entity that caused the expenses 2501* [AdverseEvent](adverseevent.html): Subject impacted by event 2502* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2503* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2504* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2505* [AuditEvent](auditevent.html): Where the activity involved patient data 2506* [Basic](basic.html): Identifies the focus of this resource 2507* [BodyStructure](bodystructure.html): Who this is about 2508* [CarePlan](careplan.html): Who the care plan is for 2509* [CareTeam](careteam.html): Who care team is for 2510* [ChargeItem](chargeitem.html): Individual service was done for/to 2511* [Claim](claim.html): Patient receiving the products or services 2512* [ClaimResponse](claimresponse.html): The subject of care 2513* [ClinicalImpression](clinicalimpression.html): Patient assessed 2514* [Communication](communication.html): Focus of message 2515* [CommunicationRequest](communicationrequest.html): Focus of message 2516* [Composition](composition.html): Who and/or what the composition is about 2517* [Condition](condition.html): Who has the condition? 2518* [Consent](consent.html): Who the consent applies to 2519* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2520* [Coverage](coverage.html): Retrieve coverages for a patient 2521* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2522* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2523* [DetectedIssue](detectedissue.html): Associated patient 2524* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2525* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2526* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2527* [DocumentReference](documentreference.html): Who/what is the subject of the document 2528* [Encounter](encounter.html): The patient present at the encounter 2529* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2530* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2531* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2532* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2533* [Flag](flag.html): The identity of a subject to list flags for 2534* [Goal](goal.html): Who this goal is intended for 2535* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2536* [ImagingSelection](imagingselection.html): Who the study is about 2537* [ImagingStudy](imagingstudy.html): Who the study is about 2538* [Immunization](immunization.html): The patient for the vaccination record 2539* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2540* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2541* [Invoice](invoice.html): Recipient(s) of goods and services 2542* [List](list.html): If all resources have the same subject 2543* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2544* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2545* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2546* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2547* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2548* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2549* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2550* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2551* [Observation](observation.html): The subject that the observation is about (if patient) 2552* [Person](person.html): The Person links to this Patient 2553* [Procedure](procedure.html): Search by subject - a patient 2554* [Provenance](provenance.html): Where the activity involved patient data 2555* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2556* [RelatedPerson](relatedperson.html): The patient this related person is related to 2557* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2558* [ResearchSubject](researchsubject.html): Who or what is part of study 2559* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2560* [ServiceRequest](servicerequest.html): Search by subject - a patient 2561* [Specimen](specimen.html): The patient the specimen comes from 2562* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2563* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2564* [Task](task.html): Search by patient 2565* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2566</b><br> 2567 * Type: <b>reference</b><br> 2568 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2569 * </p> 2570 */ 2571 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2572 2573/** 2574 * Constant for fluent queries to be used to add include statements. Specifies 2575 * the path value of "<b>DetectedIssue:patient</b>". 2576 */ 2577 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DetectedIssue:patient").toLocked(); 2578 2579 2580} 2581