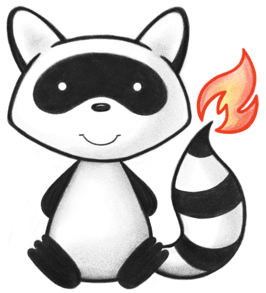
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource describes the properties (regulated, has real time clock, etc.), adminstrative (manufacturer name, model number, serial number, firmware, etc.), and type (knee replacement, blood pressure cuff, MRI, etc.) of a physical unit (these values do not change much within a given module, for example the serail number, manufacturer name, and model number). An actual unit may consist of several modules in a distinct hierarchy and these are represented by multiple Device resources and bound through the 'parent' element. 052 */ 053@ResourceDef(name="Device", profile="http://hl7.org/fhir/StructureDefinition/Device") 054public class Device extends DomainResource { 055 056 public enum FHIRDeviceStatus { 057 /** 058 * The device record is current and is appropriate for reference in new instances. 059 */ 060 ACTIVE, 061 /** 062 * The device record is not current and is not appropriate for reference in new instances. 063 */ 064 INACTIVE, 065 /** 066 * The device record is not current and is not appropriate for reference in new instances. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static FHIRDeviceStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/device-status"; 099 case INACTIVE: return "http://hl7.org/fhir/device-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/device-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The device record is current and is appropriate for reference in new instances."; 108 case INACTIVE: return "The device record is not current and is not appropriate for reference in new instances."; 109 case ENTEREDINERROR: return "The device record is not current and is not appropriate for reference in new instances."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class FHIRDeviceStatusEnumFactory implements EnumFactory<FHIRDeviceStatus> { 126 public FHIRDeviceStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return FHIRDeviceStatus.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return FHIRDeviceStatus.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return FHIRDeviceStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 137 } 138 public Enumeration<FHIRDeviceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ACTIVE, code); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.INACTIVE, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 153 } 154 public String toCode(FHIRDeviceStatus code) { 155 if (code == FHIRDeviceStatus.NULL) 156 return null; 157 if (code == FHIRDeviceStatus.ACTIVE) 158 return "active"; 159 if (code == FHIRDeviceStatus.INACTIVE) 160 return "inactive"; 161 if (code == FHIRDeviceStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(FHIRDeviceStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum UDIEntryType { 171 /** 172 * a barcodescanner captured the data from the device label. 173 */ 174 BARCODE, 175 /** 176 * An RFID chip reader captured the data from the device label. 177 */ 178 RFID, 179 /** 180 * The data was read from the label by a person and manually entered. (e.g. via a keyboard). 181 */ 182 MANUAL, 183 /** 184 * The data originated from a patient's implant card and was read by an operator. 185 */ 186 CARD, 187 /** 188 * The data originated from a patient source and was not directly scanned or read from a label or card. 189 */ 190 SELFREPORTED, 191 /** 192 * The UDI information was received electronically from the device through a communication protocol, such as the IEEE 11073 20601 version 4 exchange protocol over Bluetooth or USB. 193 */ 194 ELECTRONICTRANSMISSION, 195 /** 196 * The method of data capture has not been determined. 197 */ 198 UNKNOWN, 199 /** 200 * added to help the parsers with the generic types 201 */ 202 NULL; 203 public static UDIEntryType fromCode(String codeString) throws FHIRException { 204 if (codeString == null || "".equals(codeString)) 205 return null; 206 if ("barcode".equals(codeString)) 207 return BARCODE; 208 if ("rfid".equals(codeString)) 209 return RFID; 210 if ("manual".equals(codeString)) 211 return MANUAL; 212 if ("card".equals(codeString)) 213 return CARD; 214 if ("self-reported".equals(codeString)) 215 return SELFREPORTED; 216 if ("electronic-transmission".equals(codeString)) 217 return ELECTRONICTRANSMISSION; 218 if ("unknown".equals(codeString)) 219 return UNKNOWN; 220 if (Configuration.isAcceptInvalidEnums()) 221 return null; 222 else 223 throw new FHIRException("Unknown UDIEntryType code '"+codeString+"'"); 224 } 225 public String toCode() { 226 switch (this) { 227 case BARCODE: return "barcode"; 228 case RFID: return "rfid"; 229 case MANUAL: return "manual"; 230 case CARD: return "card"; 231 case SELFREPORTED: return "self-reported"; 232 case ELECTRONICTRANSMISSION: return "electronic-transmission"; 233 case UNKNOWN: return "unknown"; 234 case NULL: return null; 235 default: return "?"; 236 } 237 } 238 public String getSystem() { 239 switch (this) { 240 case BARCODE: return "http://hl7.org/fhir/udi-entry-type"; 241 case RFID: return "http://hl7.org/fhir/udi-entry-type"; 242 case MANUAL: return "http://hl7.org/fhir/udi-entry-type"; 243 case CARD: return "http://hl7.org/fhir/udi-entry-type"; 244 case SELFREPORTED: return "http://hl7.org/fhir/udi-entry-type"; 245 case ELECTRONICTRANSMISSION: return "http://hl7.org/fhir/udi-entry-type"; 246 case UNKNOWN: return "http://hl7.org/fhir/udi-entry-type"; 247 case NULL: return null; 248 default: return "?"; 249 } 250 } 251 public String getDefinition() { 252 switch (this) { 253 case BARCODE: return "a barcodescanner captured the data from the device label."; 254 case RFID: return "An RFID chip reader captured the data from the device label."; 255 case MANUAL: return "The data was read from the label by a person and manually entered. (e.g. via a keyboard)."; 256 case CARD: return "The data originated from a patient's implant card and was read by an operator."; 257 case SELFREPORTED: return "The data originated from a patient source and was not directly scanned or read from a label or card."; 258 case ELECTRONICTRANSMISSION: return "The UDI information was received electronically from the device through a communication protocol, such as the IEEE 11073 20601 version 4 exchange protocol over Bluetooth or USB."; 259 case UNKNOWN: return "The method of data capture has not been determined."; 260 case NULL: return null; 261 default: return "?"; 262 } 263 } 264 public String getDisplay() { 265 switch (this) { 266 case BARCODE: return "Barcode"; 267 case RFID: return "RFID"; 268 case MANUAL: return "Manual"; 269 case CARD: return "Card"; 270 case SELFREPORTED: return "Self Reported"; 271 case ELECTRONICTRANSMISSION: return "Electronic Transmission"; 272 case UNKNOWN: return "Unknown"; 273 case NULL: return null; 274 default: return "?"; 275 } 276 } 277 } 278 279 public static class UDIEntryTypeEnumFactory implements EnumFactory<UDIEntryType> { 280 public UDIEntryType fromCode(String codeString) throws IllegalArgumentException { 281 if (codeString == null || "".equals(codeString)) 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("barcode".equals(codeString)) 285 return UDIEntryType.BARCODE; 286 if ("rfid".equals(codeString)) 287 return UDIEntryType.RFID; 288 if ("manual".equals(codeString)) 289 return UDIEntryType.MANUAL; 290 if ("card".equals(codeString)) 291 return UDIEntryType.CARD; 292 if ("self-reported".equals(codeString)) 293 return UDIEntryType.SELFREPORTED; 294 if ("electronic-transmission".equals(codeString)) 295 return UDIEntryType.ELECTRONICTRANSMISSION; 296 if ("unknown".equals(codeString)) 297 return UDIEntryType.UNKNOWN; 298 throw new IllegalArgumentException("Unknown UDIEntryType code '"+codeString+"'"); 299 } 300 public Enumeration<UDIEntryType> fromType(PrimitiveType<?> code) throws FHIRException { 301 if (code == null) 302 return null; 303 if (code.isEmpty()) 304 return new Enumeration<UDIEntryType>(this, UDIEntryType.NULL, code); 305 String codeString = ((PrimitiveType) code).asStringValue(); 306 if (codeString == null || "".equals(codeString)) 307 return new Enumeration<UDIEntryType>(this, UDIEntryType.NULL, code); 308 if ("barcode".equals(codeString)) 309 return new Enumeration<UDIEntryType>(this, UDIEntryType.BARCODE, code); 310 if ("rfid".equals(codeString)) 311 return new Enumeration<UDIEntryType>(this, UDIEntryType.RFID, code); 312 if ("manual".equals(codeString)) 313 return new Enumeration<UDIEntryType>(this, UDIEntryType.MANUAL, code); 314 if ("card".equals(codeString)) 315 return new Enumeration<UDIEntryType>(this, UDIEntryType.CARD, code); 316 if ("self-reported".equals(codeString)) 317 return new Enumeration<UDIEntryType>(this, UDIEntryType.SELFREPORTED, code); 318 if ("electronic-transmission".equals(codeString)) 319 return new Enumeration<UDIEntryType>(this, UDIEntryType.ELECTRONICTRANSMISSION, code); 320 if ("unknown".equals(codeString)) 321 return new Enumeration<UDIEntryType>(this, UDIEntryType.UNKNOWN, code); 322 throw new FHIRException("Unknown UDIEntryType code '"+codeString+"'"); 323 } 324 public String toCode(UDIEntryType code) { 325 if (code == UDIEntryType.NULL) 326 return null; 327 if (code == UDIEntryType.BARCODE) 328 return "barcode"; 329 if (code == UDIEntryType.RFID) 330 return "rfid"; 331 if (code == UDIEntryType.MANUAL) 332 return "manual"; 333 if (code == UDIEntryType.CARD) 334 return "card"; 335 if (code == UDIEntryType.SELFREPORTED) 336 return "self-reported"; 337 if (code == UDIEntryType.ELECTRONICTRANSMISSION) 338 return "electronic-transmission"; 339 if (code == UDIEntryType.UNKNOWN) 340 return "unknown"; 341 return "?"; 342 } 343 public String toSystem(UDIEntryType code) { 344 return code.getSystem(); 345 } 346 } 347 348 @Block() 349 public static class DeviceUdiCarrierComponent extends BackboneElement implements IBaseBackboneElement { 350 /** 351 * The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 352 */ 353 @Child(name = "deviceIdentifier", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 354 @Description(shortDefinition="Mandatory fixed portion of UDI", formalDefinition="The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device." ) 355 protected StringType deviceIdentifier; 356 357 /** 358 * Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 3591) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 3602) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 3613) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 3624) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di. 363 */ 364 @Child(name = "issuer", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 365 @Description(shortDefinition="UDI Issuing Organization", formalDefinition="Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: \n1) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, \n3) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di." ) 366 protected UriType issuer; 367 368 /** 369 * The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi. 370 */ 371 @Child(name = "jurisdiction", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=false) 372 @Description(shortDefinition="Regional UDI authority", formalDefinition="The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi." ) 373 protected UriType jurisdiction; 374 375 /** 376 * The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 377 */ 378 @Child(name = "carrierAIDC", type = {Base64BinaryType.class}, order=4, min=0, max=1, modifier=false, summary=true) 379 @Description(shortDefinition="UDI Machine Readable Barcode String", formalDefinition="The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded." ) 380 protected Base64BinaryType carrierAIDC; 381 382 /** 383 * The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 384 */ 385 @Child(name = "carrierHRF", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 386 @Description(shortDefinition="UDI Human Readable Barcode String", formalDefinition="The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device." ) 387 protected StringType carrierHRF; 388 389 /** 390 * A coded entry to indicate how the data was entered. 391 */ 392 @Child(name = "entryType", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 393 @Description(shortDefinition="barcode | rfid | manual | card | self-reported | electronic-transmission | unknown", formalDefinition="A coded entry to indicate how the data was entered." ) 394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/udi-entry-type") 395 protected Enumeration<UDIEntryType> entryType; 396 397 private static final long serialVersionUID = -191630425L; 398 399 /** 400 * Constructor 401 */ 402 public DeviceUdiCarrierComponent() { 403 super(); 404 } 405 406 /** 407 * Constructor 408 */ 409 public DeviceUdiCarrierComponent(String deviceIdentifier, String issuer) { 410 super(); 411 this.setDeviceIdentifier(deviceIdentifier); 412 this.setIssuer(issuer); 413 } 414 415 /** 416 * @return {@link #deviceIdentifier} (The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 417 */ 418 public StringType getDeviceIdentifierElement() { 419 if (this.deviceIdentifier == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.deviceIdentifier"); 422 else if (Configuration.doAutoCreate()) 423 this.deviceIdentifier = new StringType(); // bb 424 return this.deviceIdentifier; 425 } 426 427 public boolean hasDeviceIdentifierElement() { 428 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 429 } 430 431 public boolean hasDeviceIdentifier() { 432 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 433 } 434 435 /** 436 * @param value {@link #deviceIdentifier} (The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 437 */ 438 public DeviceUdiCarrierComponent setDeviceIdentifierElement(StringType value) { 439 this.deviceIdentifier = value; 440 return this; 441 } 442 443 /** 444 * @return The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 445 */ 446 public String getDeviceIdentifier() { 447 return this.deviceIdentifier == null ? null : this.deviceIdentifier.getValue(); 448 } 449 450 /** 451 * @param value The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 452 */ 453 public DeviceUdiCarrierComponent setDeviceIdentifier(String value) { 454 if (this.deviceIdentifier == null) 455 this.deviceIdentifier = new StringType(); 456 this.deviceIdentifier.setValue(value); 457 return this; 458 } 459 460 /** 461 * @return {@link #issuer} (Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 4621) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 4632) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 4643) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4654) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 466 */ 467 public UriType getIssuerElement() { 468 if (this.issuer == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.issuer"); 471 else if (Configuration.doAutoCreate()) 472 this.issuer = new UriType(); // bb 473 return this.issuer; 474 } 475 476 public boolean hasIssuerElement() { 477 return this.issuer != null && !this.issuer.isEmpty(); 478 } 479 480 public boolean hasIssuer() { 481 return this.issuer != null && !this.issuer.isEmpty(); 482 } 483 484 /** 485 * @param value {@link #issuer} (Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 4861) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 4872) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 4883) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4894) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 490 */ 491 public DeviceUdiCarrierComponent setIssuerElement(UriType value) { 492 this.issuer = value; 493 return this; 494 } 495 496 /** 497 * @return Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 4981) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 4992) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 5003) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 5014) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di. 502 */ 503 public String getIssuer() { 504 return this.issuer == null ? null : this.issuer.getValue(); 505 } 506 507 /** 508 * @param value Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 5091) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 5102) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 5113) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 5124) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di. 513 */ 514 public DeviceUdiCarrierComponent setIssuer(String value) { 515 if (this.issuer == null) 516 this.issuer = new UriType(); 517 this.issuer.setValue(value); 518 return this; 519 } 520 521 /** 522 * @return {@link #jurisdiction} (The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 523 */ 524 public UriType getJurisdictionElement() { 525 if (this.jurisdiction == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.jurisdiction"); 528 else if (Configuration.doAutoCreate()) 529 this.jurisdiction = new UriType(); // bb 530 return this.jurisdiction; 531 } 532 533 public boolean hasJurisdictionElement() { 534 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 535 } 536 537 public boolean hasJurisdiction() { 538 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #jurisdiction} (The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 543 */ 544 public DeviceUdiCarrierComponent setJurisdictionElement(UriType value) { 545 this.jurisdiction = value; 546 return this; 547 } 548 549 /** 550 * @return The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi. 551 */ 552 public String getJurisdiction() { 553 return this.jurisdiction == null ? null : this.jurisdiction.getValue(); 554 } 555 556 /** 557 * @param value The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi. 558 */ 559 public DeviceUdiCarrierComponent setJurisdiction(String value) { 560 if (Utilities.noString(value)) 561 this.jurisdiction = null; 562 else { 563 if (this.jurisdiction == null) 564 this.jurisdiction = new UriType(); 565 this.jurisdiction.setValue(value); 566 } 567 return this; 568 } 569 570 /** 571 * @return {@link #carrierAIDC} (The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getCarrierAIDC" gives direct access to the value 572 */ 573 public Base64BinaryType getCarrierAIDCElement() { 574 if (this.carrierAIDC == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.carrierAIDC"); 577 else if (Configuration.doAutoCreate()) 578 this.carrierAIDC = new Base64BinaryType(); // bb 579 return this.carrierAIDC; 580 } 581 582 public boolean hasCarrierAIDCElement() { 583 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 584 } 585 586 public boolean hasCarrierAIDC() { 587 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 588 } 589 590 /** 591 * @param value {@link #carrierAIDC} (The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getCarrierAIDC" gives direct access to the value 592 */ 593 public DeviceUdiCarrierComponent setCarrierAIDCElement(Base64BinaryType value) { 594 this.carrierAIDC = value; 595 return this; 596 } 597 598 /** 599 * @return The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 600 */ 601 public byte[] getCarrierAIDC() { 602 return this.carrierAIDC == null ? null : this.carrierAIDC.getValue(); 603 } 604 605 /** 606 * @param value The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 607 */ 608 public DeviceUdiCarrierComponent setCarrierAIDC(byte[] value) { 609 if (value == null) 610 this.carrierAIDC = null; 611 else { 612 if (this.carrierAIDC == null) 613 this.carrierAIDC = new Base64BinaryType(); 614 this.carrierAIDC.setValue(value); 615 } 616 return this; 617 } 618 619 /** 620 * @return {@link #carrierHRF} (The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.). This is the underlying object with id, value and extensions. The accessor "getCarrierHRF" gives direct access to the value 621 */ 622 public StringType getCarrierHRFElement() { 623 if (this.carrierHRF == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.carrierHRF"); 626 else if (Configuration.doAutoCreate()) 627 this.carrierHRF = new StringType(); // bb 628 return this.carrierHRF; 629 } 630 631 public boolean hasCarrierHRFElement() { 632 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 633 } 634 635 public boolean hasCarrierHRF() { 636 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 637 } 638 639 /** 640 * @param value {@link #carrierHRF} (The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.). This is the underlying object with id, value and extensions. The accessor "getCarrierHRF" gives direct access to the value 641 */ 642 public DeviceUdiCarrierComponent setCarrierHRFElement(StringType value) { 643 this.carrierHRF = value; 644 return this; 645 } 646 647 /** 648 * @return The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 649 */ 650 public String getCarrierHRF() { 651 return this.carrierHRF == null ? null : this.carrierHRF.getValue(); 652 } 653 654 /** 655 * @param value The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 656 */ 657 public DeviceUdiCarrierComponent setCarrierHRF(String value) { 658 if (Utilities.noString(value)) 659 this.carrierHRF = null; 660 else { 661 if (this.carrierHRF == null) 662 this.carrierHRF = new StringType(); 663 this.carrierHRF.setValue(value); 664 } 665 return this; 666 } 667 668 /** 669 * @return {@link #entryType} (A coded entry to indicate how the data was entered.). This is the underlying object with id, value and extensions. The accessor "getEntryType" gives direct access to the value 670 */ 671 public Enumeration<UDIEntryType> getEntryTypeElement() { 672 if (this.entryType == null) 673 if (Configuration.errorOnAutoCreate()) 674 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.entryType"); 675 else if (Configuration.doAutoCreate()) 676 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); // bb 677 return this.entryType; 678 } 679 680 public boolean hasEntryTypeElement() { 681 return this.entryType != null && !this.entryType.isEmpty(); 682 } 683 684 public boolean hasEntryType() { 685 return this.entryType != null && !this.entryType.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #entryType} (A coded entry to indicate how the data was entered.). This is the underlying object with id, value and extensions. The accessor "getEntryType" gives direct access to the value 690 */ 691 public DeviceUdiCarrierComponent setEntryTypeElement(Enumeration<UDIEntryType> value) { 692 this.entryType = value; 693 return this; 694 } 695 696 /** 697 * @return A coded entry to indicate how the data was entered. 698 */ 699 public UDIEntryType getEntryType() { 700 return this.entryType == null ? null : this.entryType.getValue(); 701 } 702 703 /** 704 * @param value A coded entry to indicate how the data was entered. 705 */ 706 public DeviceUdiCarrierComponent setEntryType(UDIEntryType value) { 707 if (value == null) 708 this.entryType = null; 709 else { 710 if (this.entryType == null) 711 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); 712 this.entryType.setValue(value); 713 } 714 return this; 715 } 716 717 protected void listChildren(List<Property> children) { 718 super.listChildren(children); 719 children.add(new Property("deviceIdentifier", "string", "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 0, 1, deviceIdentifier)); 720 children.add(new Property("issuer", "uri", "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: \n1) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, \n3) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.", 0, 1, issuer)); 721 children.add(new Property("jurisdiction", "uri", "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.", 0, 1, jurisdiction)); 722 children.add(new Property("carrierAIDC", "base64Binary", "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 0, 1, carrierAIDC)); 723 children.add(new Property("carrierHRF", "string", "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 0, 1, carrierHRF)); 724 children.add(new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 0, 1, entryType)); 725 } 726 727 @Override 728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 729 switch (_hash) { 730 case 1322005407: /*deviceIdentifier*/ return new Property("deviceIdentifier", "string", "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 0, 1, deviceIdentifier); 731 case -1179159879: /*issuer*/ return new Property("issuer", "uri", "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: \n1) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, \n3) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.", 0, 1, issuer); 732 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "uri", "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.", 0, 1, jurisdiction); 733 case -768521825: /*carrierAIDC*/ return new Property("carrierAIDC", "base64Binary", "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 0, 1, carrierAIDC); 734 case 806499972: /*carrierHRF*/ return new Property("carrierHRF", "string", "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 0, 1, carrierHRF); 735 case -479362356: /*entryType*/ return new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 0, 1, entryType); 736 default: return super.getNamedProperty(_hash, _name, _checkValid); 737 } 738 739 } 740 741 @Override 742 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 743 switch (hash) { 744 case 1322005407: /*deviceIdentifier*/ return this.deviceIdentifier == null ? new Base[0] : new Base[] {this.deviceIdentifier}; // StringType 745 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // UriType 746 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // UriType 747 case -768521825: /*carrierAIDC*/ return this.carrierAIDC == null ? new Base[0] : new Base[] {this.carrierAIDC}; // Base64BinaryType 748 case 806499972: /*carrierHRF*/ return this.carrierHRF == null ? new Base[0] : new Base[] {this.carrierHRF}; // StringType 749 case -479362356: /*entryType*/ return this.entryType == null ? new Base[0] : new Base[] {this.entryType}; // Enumeration<UDIEntryType> 750 default: return super.getProperty(hash, name, checkValid); 751 } 752 753 } 754 755 @Override 756 public Base setProperty(int hash, String name, Base value) throws FHIRException { 757 switch (hash) { 758 case 1322005407: // deviceIdentifier 759 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 760 return value; 761 case -1179159879: // issuer 762 this.issuer = TypeConvertor.castToUri(value); // UriType 763 return value; 764 case -507075711: // jurisdiction 765 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 766 return value; 767 case -768521825: // carrierAIDC 768 this.carrierAIDC = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 769 return value; 770 case 806499972: // carrierHRF 771 this.carrierHRF = TypeConvertor.castToString(value); // StringType 772 return value; 773 case -479362356: // entryType 774 value = new UDIEntryTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 775 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 776 return value; 777 default: return super.setProperty(hash, name, value); 778 } 779 780 } 781 782 @Override 783 public Base setProperty(String name, Base value) throws FHIRException { 784 if (name.equals("deviceIdentifier")) { 785 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 786 } else if (name.equals("issuer")) { 787 this.issuer = TypeConvertor.castToUri(value); // UriType 788 } else if (name.equals("jurisdiction")) { 789 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 790 } else if (name.equals("carrierAIDC")) { 791 this.carrierAIDC = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 792 } else if (name.equals("carrierHRF")) { 793 this.carrierHRF = TypeConvertor.castToString(value); // StringType 794 } else if (name.equals("entryType")) { 795 value = new UDIEntryTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 796 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 797 } else 798 return super.setProperty(name, value); 799 return value; 800 } 801 802 @Override 803 public void removeChild(String name, Base value) throws FHIRException { 804 if (name.equals("deviceIdentifier")) { 805 this.deviceIdentifier = null; 806 } else if (name.equals("issuer")) { 807 this.issuer = null; 808 } else if (name.equals("jurisdiction")) { 809 this.jurisdiction = null; 810 } else if (name.equals("carrierAIDC")) { 811 this.carrierAIDC = null; 812 } else if (name.equals("carrierHRF")) { 813 this.carrierHRF = null; 814 } else if (name.equals("entryType")) { 815 value = new UDIEntryTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 816 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 817 } else 818 super.removeChild(name, value); 819 820 } 821 822 @Override 823 public Base makeProperty(int hash, String name) throws FHIRException { 824 switch (hash) { 825 case 1322005407: return getDeviceIdentifierElement(); 826 case -1179159879: return getIssuerElement(); 827 case -507075711: return getJurisdictionElement(); 828 case -768521825: return getCarrierAIDCElement(); 829 case 806499972: return getCarrierHRFElement(); 830 case -479362356: return getEntryTypeElement(); 831 default: return super.makeProperty(hash, name); 832 } 833 834 } 835 836 @Override 837 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 838 switch (hash) { 839 case 1322005407: /*deviceIdentifier*/ return new String[] {"string"}; 840 case -1179159879: /*issuer*/ return new String[] {"uri"}; 841 case -507075711: /*jurisdiction*/ return new String[] {"uri"}; 842 case -768521825: /*carrierAIDC*/ return new String[] {"base64Binary"}; 843 case 806499972: /*carrierHRF*/ return new String[] {"string"}; 844 case -479362356: /*entryType*/ return new String[] {"code"}; 845 default: return super.getTypesForProperty(hash, name); 846 } 847 848 } 849 850 @Override 851 public Base addChild(String name) throws FHIRException { 852 if (name.equals("deviceIdentifier")) { 853 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.deviceIdentifier"); 854 } 855 else if (name.equals("issuer")) { 856 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.issuer"); 857 } 858 else if (name.equals("jurisdiction")) { 859 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.jurisdiction"); 860 } 861 else if (name.equals("carrierAIDC")) { 862 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.carrierAIDC"); 863 } 864 else if (name.equals("carrierHRF")) { 865 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.carrierHRF"); 866 } 867 else if (name.equals("entryType")) { 868 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.entryType"); 869 } 870 else 871 return super.addChild(name); 872 } 873 874 public DeviceUdiCarrierComponent copy() { 875 DeviceUdiCarrierComponent dst = new DeviceUdiCarrierComponent(); 876 copyValues(dst); 877 return dst; 878 } 879 880 public void copyValues(DeviceUdiCarrierComponent dst) { 881 super.copyValues(dst); 882 dst.deviceIdentifier = deviceIdentifier == null ? null : deviceIdentifier.copy(); 883 dst.issuer = issuer == null ? null : issuer.copy(); 884 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 885 dst.carrierAIDC = carrierAIDC == null ? null : carrierAIDC.copy(); 886 dst.carrierHRF = carrierHRF == null ? null : carrierHRF.copy(); 887 dst.entryType = entryType == null ? null : entryType.copy(); 888 } 889 890 @Override 891 public boolean equalsDeep(Base other_) { 892 if (!super.equalsDeep(other_)) 893 return false; 894 if (!(other_ instanceof DeviceUdiCarrierComponent)) 895 return false; 896 DeviceUdiCarrierComponent o = (DeviceUdiCarrierComponent) other_; 897 return compareDeep(deviceIdentifier, o.deviceIdentifier, true) && compareDeep(issuer, o.issuer, true) 898 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(carrierAIDC, o.carrierAIDC, true) 899 && compareDeep(carrierHRF, o.carrierHRF, true) && compareDeep(entryType, o.entryType, true); 900 } 901 902 @Override 903 public boolean equalsShallow(Base other_) { 904 if (!super.equalsShallow(other_)) 905 return false; 906 if (!(other_ instanceof DeviceUdiCarrierComponent)) 907 return false; 908 DeviceUdiCarrierComponent o = (DeviceUdiCarrierComponent) other_; 909 return compareValues(deviceIdentifier, o.deviceIdentifier, true) && compareValues(issuer, o.issuer, true) 910 && compareValues(jurisdiction, o.jurisdiction, true) && compareValues(carrierAIDC, o.carrierAIDC, true) 911 && compareValues(carrierHRF, o.carrierHRF, true) && compareValues(entryType, o.entryType, true); 912 } 913 914 public boolean isEmpty() { 915 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(deviceIdentifier, issuer, jurisdiction 916 , carrierAIDC, carrierHRF, entryType); 917 } 918 919 public String fhirType() { 920 return "Device.udiCarrier"; 921 922 } 923 924 } 925 926 @Block() 927 public static class DeviceNameComponent extends BackboneElement implements IBaseBackboneElement { 928 /** 929 * The actual name that identifies the device. 930 */ 931 @Child(name = "value", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 932 @Description(shortDefinition="The term that names the device", formalDefinition="The actual name that identifies the device." ) 933 protected StringType value; 934 935 /** 936 * Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName. 937 */ 938 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 939 @Description(shortDefinition="registered-name | user-friendly-name | patient-reported-name", formalDefinition="Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName." ) 940 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-nametype") 941 protected Enumeration<DeviceNameType> type; 942 943 /** 944 * Indicates the default or preferred name to be displayed. 945 */ 946 @Child(name = "display", type = {BooleanType.class}, order=3, min=0, max=1, modifier=true, summary=true) 947 @Description(shortDefinition="The preferred device name", formalDefinition="Indicates the default or preferred name to be displayed." ) 948 protected BooleanType display; 949 950 private static final long serialVersionUID = 1911928470L; 951 952 /** 953 * Constructor 954 */ 955 public DeviceNameComponent() { 956 super(); 957 } 958 959 /** 960 * Constructor 961 */ 962 public DeviceNameComponent(String value, DeviceNameType type) { 963 super(); 964 this.setValue(value); 965 this.setType(type); 966 } 967 968 /** 969 * @return {@link #value} (The actual name that identifies the device.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 970 */ 971 public StringType getValueElement() { 972 if (this.value == null) 973 if (Configuration.errorOnAutoCreate()) 974 throw new Error("Attempt to auto-create DeviceNameComponent.value"); 975 else if (Configuration.doAutoCreate()) 976 this.value = new StringType(); // bb 977 return this.value; 978 } 979 980 public boolean hasValueElement() { 981 return this.value != null && !this.value.isEmpty(); 982 } 983 984 public boolean hasValue() { 985 return this.value != null && !this.value.isEmpty(); 986 } 987 988 /** 989 * @param value {@link #value} (The actual name that identifies the device.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 990 */ 991 public DeviceNameComponent setValueElement(StringType value) { 992 this.value = value; 993 return this; 994 } 995 996 /** 997 * @return The actual name that identifies the device. 998 */ 999 public String getValue() { 1000 return this.value == null ? null : this.value.getValue(); 1001 } 1002 1003 /** 1004 * @param value The actual name that identifies the device. 1005 */ 1006 public DeviceNameComponent setValue(String value) { 1007 if (this.value == null) 1008 this.value = new StringType(); 1009 this.value.setValue(value); 1010 return this; 1011 } 1012 1013 /** 1014 * @return {@link #type} (Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1015 */ 1016 public Enumeration<DeviceNameType> getTypeElement() { 1017 if (this.type == null) 1018 if (Configuration.errorOnAutoCreate()) 1019 throw new Error("Attempt to auto-create DeviceNameComponent.type"); 1020 else if (Configuration.doAutoCreate()) 1021 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); // bb 1022 return this.type; 1023 } 1024 1025 public boolean hasTypeElement() { 1026 return this.type != null && !this.type.isEmpty(); 1027 } 1028 1029 public boolean hasType() { 1030 return this.type != null && !this.type.isEmpty(); 1031 } 1032 1033 /** 1034 * @param value {@link #type} (Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1035 */ 1036 public DeviceNameComponent setTypeElement(Enumeration<DeviceNameType> value) { 1037 this.type = value; 1038 return this; 1039 } 1040 1041 /** 1042 * @return Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName. 1043 */ 1044 public DeviceNameType getType() { 1045 return this.type == null ? null : this.type.getValue(); 1046 } 1047 1048 /** 1049 * @param value Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName. 1050 */ 1051 public DeviceNameComponent setType(DeviceNameType value) { 1052 if (this.type == null) 1053 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); 1054 this.type.setValue(value); 1055 return this; 1056 } 1057 1058 /** 1059 * @return {@link #display} (Indicates the default or preferred name to be displayed.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1060 */ 1061 public BooleanType getDisplayElement() { 1062 if (this.display == null) 1063 if (Configuration.errorOnAutoCreate()) 1064 throw new Error("Attempt to auto-create DeviceNameComponent.display"); 1065 else if (Configuration.doAutoCreate()) 1066 this.display = new BooleanType(); // bb 1067 return this.display; 1068 } 1069 1070 public boolean hasDisplayElement() { 1071 return this.display != null && !this.display.isEmpty(); 1072 } 1073 1074 public boolean hasDisplay() { 1075 return this.display != null && !this.display.isEmpty(); 1076 } 1077 1078 /** 1079 * @param value {@link #display} (Indicates the default or preferred name to be displayed.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1080 */ 1081 public DeviceNameComponent setDisplayElement(BooleanType value) { 1082 this.display = value; 1083 return this; 1084 } 1085 1086 /** 1087 * @return Indicates the default or preferred name to be displayed. 1088 */ 1089 public boolean getDisplay() { 1090 return this.display == null || this.display.isEmpty() ? false : this.display.getValue(); 1091 } 1092 1093 /** 1094 * @param value Indicates the default or preferred name to be displayed. 1095 */ 1096 public DeviceNameComponent setDisplay(boolean value) { 1097 if (this.display == null) 1098 this.display = new BooleanType(); 1099 this.display.setValue(value); 1100 return this; 1101 } 1102 1103 protected void listChildren(List<Property> children) { 1104 super.listChildren(children); 1105 children.add(new Property("value", "string", "The actual name that identifies the device.", 0, 1, value)); 1106 children.add(new Property("type", "code", "Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.", 0, 1, type)); 1107 children.add(new Property("display", "boolean", "Indicates the default or preferred name to be displayed.", 0, 1, display)); 1108 } 1109 1110 @Override 1111 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1112 switch (_hash) { 1113 case 111972721: /*value*/ return new Property("value", "string", "The actual name that identifies the device.", 0, 1, value); 1114 case 3575610: /*type*/ return new Property("type", "code", "Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.", 0, 1, type); 1115 case 1671764162: /*display*/ return new Property("display", "boolean", "Indicates the default or preferred name to be displayed.", 0, 1, display); 1116 default: return super.getNamedProperty(_hash, _name, _checkValid); 1117 } 1118 1119 } 1120 1121 @Override 1122 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1123 switch (hash) { 1124 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1125 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DeviceNameType> 1126 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // BooleanType 1127 default: return super.getProperty(hash, name, checkValid); 1128 } 1129 1130 } 1131 1132 @Override 1133 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1134 switch (hash) { 1135 case 111972721: // value 1136 this.value = TypeConvertor.castToString(value); // StringType 1137 return value; 1138 case 3575610: // type 1139 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1140 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1141 return value; 1142 case 1671764162: // display 1143 this.display = TypeConvertor.castToBoolean(value); // BooleanType 1144 return value; 1145 default: return super.setProperty(hash, name, value); 1146 } 1147 1148 } 1149 1150 @Override 1151 public Base setProperty(String name, Base value) throws FHIRException { 1152 if (name.equals("value")) { 1153 this.value = TypeConvertor.castToString(value); // StringType 1154 } else if (name.equals("type")) { 1155 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1156 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1157 } else if (name.equals("display")) { 1158 this.display = TypeConvertor.castToBoolean(value); // BooleanType 1159 } else 1160 return super.setProperty(name, value); 1161 return value; 1162 } 1163 1164 @Override 1165 public void removeChild(String name, Base value) throws FHIRException { 1166 if (name.equals("value")) { 1167 this.value = null; 1168 } else if (name.equals("type")) { 1169 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1170 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1171 } else if (name.equals("display")) { 1172 this.display = null; 1173 } else 1174 super.removeChild(name, value); 1175 1176 } 1177 1178 @Override 1179 public Base makeProperty(int hash, String name) throws FHIRException { 1180 switch (hash) { 1181 case 111972721: return getValueElement(); 1182 case 3575610: return getTypeElement(); 1183 case 1671764162: return getDisplayElement(); 1184 default: return super.makeProperty(hash, name); 1185 } 1186 1187 } 1188 1189 @Override 1190 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1191 switch (hash) { 1192 case 111972721: /*value*/ return new String[] {"string"}; 1193 case 3575610: /*type*/ return new String[] {"code"}; 1194 case 1671764162: /*display*/ return new String[] {"boolean"}; 1195 default: return super.getTypesForProperty(hash, name); 1196 } 1197 1198 } 1199 1200 @Override 1201 public Base addChild(String name) throws FHIRException { 1202 if (name.equals("value")) { 1203 throw new FHIRException("Cannot call addChild on a singleton property Device.name.value"); 1204 } 1205 else if (name.equals("type")) { 1206 throw new FHIRException("Cannot call addChild on a singleton property Device.name.type"); 1207 } 1208 else if (name.equals("display")) { 1209 throw new FHIRException("Cannot call addChild on a singleton property Device.name.display"); 1210 } 1211 else 1212 return super.addChild(name); 1213 } 1214 1215 public DeviceNameComponent copy() { 1216 DeviceNameComponent dst = new DeviceNameComponent(); 1217 copyValues(dst); 1218 return dst; 1219 } 1220 1221 public void copyValues(DeviceNameComponent dst) { 1222 super.copyValues(dst); 1223 dst.value = value == null ? null : value.copy(); 1224 dst.type = type == null ? null : type.copy(); 1225 dst.display = display == null ? null : display.copy(); 1226 } 1227 1228 @Override 1229 public boolean equalsDeep(Base other_) { 1230 if (!super.equalsDeep(other_)) 1231 return false; 1232 if (!(other_ instanceof DeviceNameComponent)) 1233 return false; 1234 DeviceNameComponent o = (DeviceNameComponent) other_; 1235 return compareDeep(value, o.value, true) && compareDeep(type, o.type, true) && compareDeep(display, o.display, true) 1236 ; 1237 } 1238 1239 @Override 1240 public boolean equalsShallow(Base other_) { 1241 if (!super.equalsShallow(other_)) 1242 return false; 1243 if (!(other_ instanceof DeviceNameComponent)) 1244 return false; 1245 DeviceNameComponent o = (DeviceNameComponent) other_; 1246 return compareValues(value, o.value, true) && compareValues(type, o.type, true) && compareValues(display, o.display, true) 1247 ; 1248 } 1249 1250 public boolean isEmpty() { 1251 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, type, display); 1252 } 1253 1254 public String fhirType() { 1255 return "Device.name"; 1256 1257 } 1258 1259 } 1260 1261 @Block() 1262 public static class DeviceVersionComponent extends BackboneElement implements IBaseBackboneElement { 1263 /** 1264 * The type of the device version, e.g. manufacturer, approved, internal. 1265 */ 1266 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1267 @Description(shortDefinition="The type of the device version, e.g. manufacturer, approved, internal", formalDefinition="The type of the device version, e.g. manufacturer, approved, internal." ) 1268 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-versiontype") 1269 protected CodeableConcept type; 1270 1271 /** 1272 * The hardware or software module of the device to which the version applies. 1273 */ 1274 @Child(name = "component", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 1275 @Description(shortDefinition="The hardware or software module of the device to which the version applies", formalDefinition="The hardware or software module of the device to which the version applies." ) 1276 protected Identifier component; 1277 1278 /** 1279 * The date the version was installed on the device. 1280 */ 1281 @Child(name = "installDate", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1282 @Description(shortDefinition="The date the version was installed on the device", formalDefinition="The date the version was installed on the device." ) 1283 protected DateTimeType installDate; 1284 1285 /** 1286 * The version text. 1287 */ 1288 @Child(name = "value", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 1289 @Description(shortDefinition="The version text", formalDefinition="The version text." ) 1290 protected StringType value; 1291 1292 private static final long serialVersionUID = 1358422741L; 1293 1294 /** 1295 * Constructor 1296 */ 1297 public DeviceVersionComponent() { 1298 super(); 1299 } 1300 1301 /** 1302 * Constructor 1303 */ 1304 public DeviceVersionComponent(String value) { 1305 super(); 1306 this.setValue(value); 1307 } 1308 1309 /** 1310 * @return {@link #type} (The type of the device version, e.g. manufacturer, approved, internal.) 1311 */ 1312 public CodeableConcept getType() { 1313 if (this.type == null) 1314 if (Configuration.errorOnAutoCreate()) 1315 throw new Error("Attempt to auto-create DeviceVersionComponent.type"); 1316 else if (Configuration.doAutoCreate()) 1317 this.type = new CodeableConcept(); // cc 1318 return this.type; 1319 } 1320 1321 public boolean hasType() { 1322 return this.type != null && !this.type.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #type} (The type of the device version, e.g. manufacturer, approved, internal.) 1327 */ 1328 public DeviceVersionComponent setType(CodeableConcept value) { 1329 this.type = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #component} (The hardware or software module of the device to which the version applies.) 1335 */ 1336 public Identifier getComponent() { 1337 if (this.component == null) 1338 if (Configuration.errorOnAutoCreate()) 1339 throw new Error("Attempt to auto-create DeviceVersionComponent.component"); 1340 else if (Configuration.doAutoCreate()) 1341 this.component = new Identifier(); // cc 1342 return this.component; 1343 } 1344 1345 public boolean hasComponent() { 1346 return this.component != null && !this.component.isEmpty(); 1347 } 1348 1349 /** 1350 * @param value {@link #component} (The hardware or software module of the device to which the version applies.) 1351 */ 1352 public DeviceVersionComponent setComponent(Identifier value) { 1353 this.component = value; 1354 return this; 1355 } 1356 1357 /** 1358 * @return {@link #installDate} (The date the version was installed on the device.). This is the underlying object with id, value and extensions. The accessor "getInstallDate" gives direct access to the value 1359 */ 1360 public DateTimeType getInstallDateElement() { 1361 if (this.installDate == null) 1362 if (Configuration.errorOnAutoCreate()) 1363 throw new Error("Attempt to auto-create DeviceVersionComponent.installDate"); 1364 else if (Configuration.doAutoCreate()) 1365 this.installDate = new DateTimeType(); // bb 1366 return this.installDate; 1367 } 1368 1369 public boolean hasInstallDateElement() { 1370 return this.installDate != null && !this.installDate.isEmpty(); 1371 } 1372 1373 public boolean hasInstallDate() { 1374 return this.installDate != null && !this.installDate.isEmpty(); 1375 } 1376 1377 /** 1378 * @param value {@link #installDate} (The date the version was installed on the device.). This is the underlying object with id, value and extensions. The accessor "getInstallDate" gives direct access to the value 1379 */ 1380 public DeviceVersionComponent setInstallDateElement(DateTimeType value) { 1381 this.installDate = value; 1382 return this; 1383 } 1384 1385 /** 1386 * @return The date the version was installed on the device. 1387 */ 1388 public Date getInstallDate() { 1389 return this.installDate == null ? null : this.installDate.getValue(); 1390 } 1391 1392 /** 1393 * @param value The date the version was installed on the device. 1394 */ 1395 public DeviceVersionComponent setInstallDate(Date value) { 1396 if (value == null) 1397 this.installDate = null; 1398 else { 1399 if (this.installDate == null) 1400 this.installDate = new DateTimeType(); 1401 this.installDate.setValue(value); 1402 } 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #value} (The version text.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1408 */ 1409 public StringType getValueElement() { 1410 if (this.value == null) 1411 if (Configuration.errorOnAutoCreate()) 1412 throw new Error("Attempt to auto-create DeviceVersionComponent.value"); 1413 else if (Configuration.doAutoCreate()) 1414 this.value = new StringType(); // bb 1415 return this.value; 1416 } 1417 1418 public boolean hasValueElement() { 1419 return this.value != null && !this.value.isEmpty(); 1420 } 1421 1422 public boolean hasValue() { 1423 return this.value != null && !this.value.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #value} (The version text.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1428 */ 1429 public DeviceVersionComponent setValueElement(StringType value) { 1430 this.value = value; 1431 return this; 1432 } 1433 1434 /** 1435 * @return The version text. 1436 */ 1437 public String getValue() { 1438 return this.value == null ? null : this.value.getValue(); 1439 } 1440 1441 /** 1442 * @param value The version text. 1443 */ 1444 public DeviceVersionComponent setValue(String value) { 1445 if (this.value == null) 1446 this.value = new StringType(); 1447 this.value.setValue(value); 1448 return this; 1449 } 1450 1451 protected void listChildren(List<Property> children) { 1452 super.listChildren(children); 1453 children.add(new Property("type", "CodeableConcept", "The type of the device version, e.g. manufacturer, approved, internal.", 0, 1, type)); 1454 children.add(new Property("component", "Identifier", "The hardware or software module of the device to which the version applies.", 0, 1, component)); 1455 children.add(new Property("installDate", "dateTime", "The date the version was installed on the device.", 0, 1, installDate)); 1456 children.add(new Property("value", "string", "The version text.", 0, 1, value)); 1457 } 1458 1459 @Override 1460 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1461 switch (_hash) { 1462 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the device version, e.g. manufacturer, approved, internal.", 0, 1, type); 1463 case -1399907075: /*component*/ return new Property("component", "Identifier", "The hardware or software module of the device to which the version applies.", 0, 1, component); 1464 case 2143044585: /*installDate*/ return new Property("installDate", "dateTime", "The date the version was installed on the device.", 0, 1, installDate); 1465 case 111972721: /*value*/ return new Property("value", "string", "The version text.", 0, 1, value); 1466 default: return super.getNamedProperty(_hash, _name, _checkValid); 1467 } 1468 1469 } 1470 1471 @Override 1472 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1473 switch (hash) { 1474 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1475 case -1399907075: /*component*/ return this.component == null ? new Base[0] : new Base[] {this.component}; // Identifier 1476 case 2143044585: /*installDate*/ return this.installDate == null ? new Base[0] : new Base[] {this.installDate}; // DateTimeType 1477 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1478 default: return super.getProperty(hash, name, checkValid); 1479 } 1480 1481 } 1482 1483 @Override 1484 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1485 switch (hash) { 1486 case 3575610: // type 1487 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1488 return value; 1489 case -1399907075: // component 1490 this.component = TypeConvertor.castToIdentifier(value); // Identifier 1491 return value; 1492 case 2143044585: // installDate 1493 this.installDate = TypeConvertor.castToDateTime(value); // DateTimeType 1494 return value; 1495 case 111972721: // value 1496 this.value = TypeConvertor.castToString(value); // StringType 1497 return value; 1498 default: return super.setProperty(hash, name, value); 1499 } 1500 1501 } 1502 1503 @Override 1504 public Base setProperty(String name, Base value) throws FHIRException { 1505 if (name.equals("type")) { 1506 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1507 } else if (name.equals("component")) { 1508 this.component = TypeConvertor.castToIdentifier(value); // Identifier 1509 } else if (name.equals("installDate")) { 1510 this.installDate = TypeConvertor.castToDateTime(value); // DateTimeType 1511 } else if (name.equals("value")) { 1512 this.value = TypeConvertor.castToString(value); // StringType 1513 } else 1514 return super.setProperty(name, value); 1515 return value; 1516 } 1517 1518 @Override 1519 public void removeChild(String name, Base value) throws FHIRException { 1520 if (name.equals("type")) { 1521 this.type = null; 1522 } else if (name.equals("component")) { 1523 this.component = null; 1524 } else if (name.equals("installDate")) { 1525 this.installDate = null; 1526 } else if (name.equals("value")) { 1527 this.value = null; 1528 } else 1529 super.removeChild(name, value); 1530 1531 } 1532 1533 @Override 1534 public Base makeProperty(int hash, String name) throws FHIRException { 1535 switch (hash) { 1536 case 3575610: return getType(); 1537 case -1399907075: return getComponent(); 1538 case 2143044585: return getInstallDateElement(); 1539 case 111972721: return getValueElement(); 1540 default: return super.makeProperty(hash, name); 1541 } 1542 1543 } 1544 1545 @Override 1546 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1547 switch (hash) { 1548 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1549 case -1399907075: /*component*/ return new String[] {"Identifier"}; 1550 case 2143044585: /*installDate*/ return new String[] {"dateTime"}; 1551 case 111972721: /*value*/ return new String[] {"string"}; 1552 default: return super.getTypesForProperty(hash, name); 1553 } 1554 1555 } 1556 1557 @Override 1558 public Base addChild(String name) throws FHIRException { 1559 if (name.equals("type")) { 1560 this.type = new CodeableConcept(); 1561 return this.type; 1562 } 1563 else if (name.equals("component")) { 1564 this.component = new Identifier(); 1565 return this.component; 1566 } 1567 else if (name.equals("installDate")) { 1568 throw new FHIRException("Cannot call addChild on a singleton property Device.version.installDate"); 1569 } 1570 else if (name.equals("value")) { 1571 throw new FHIRException("Cannot call addChild on a singleton property Device.version.value"); 1572 } 1573 else 1574 return super.addChild(name); 1575 } 1576 1577 public DeviceVersionComponent copy() { 1578 DeviceVersionComponent dst = new DeviceVersionComponent(); 1579 copyValues(dst); 1580 return dst; 1581 } 1582 1583 public void copyValues(DeviceVersionComponent dst) { 1584 super.copyValues(dst); 1585 dst.type = type == null ? null : type.copy(); 1586 dst.component = component == null ? null : component.copy(); 1587 dst.installDate = installDate == null ? null : installDate.copy(); 1588 dst.value = value == null ? null : value.copy(); 1589 } 1590 1591 @Override 1592 public boolean equalsDeep(Base other_) { 1593 if (!super.equalsDeep(other_)) 1594 return false; 1595 if (!(other_ instanceof DeviceVersionComponent)) 1596 return false; 1597 DeviceVersionComponent o = (DeviceVersionComponent) other_; 1598 return compareDeep(type, o.type, true) && compareDeep(component, o.component, true) && compareDeep(installDate, o.installDate, true) 1599 && compareDeep(value, o.value, true); 1600 } 1601 1602 @Override 1603 public boolean equalsShallow(Base other_) { 1604 if (!super.equalsShallow(other_)) 1605 return false; 1606 if (!(other_ instanceof DeviceVersionComponent)) 1607 return false; 1608 DeviceVersionComponent o = (DeviceVersionComponent) other_; 1609 return compareValues(installDate, o.installDate, true) && compareValues(value, o.value, true); 1610 } 1611 1612 public boolean isEmpty() { 1613 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, component, installDate 1614 , value); 1615 } 1616 1617 public String fhirType() { 1618 return "Device.version"; 1619 1620 } 1621 1622 } 1623 1624 @Block() 1625 public static class DeviceConformsToComponent extends BackboneElement implements IBaseBackboneElement { 1626 /** 1627 * Describes the type of the standard, specification, or formal guidance. 1628 */ 1629 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1630 @Description(shortDefinition="Describes the common type of the standard, specification, or formal guidance. communication | performance | measurement", formalDefinition="Describes the type of the standard, specification, or formal guidance." ) 1631 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-specification-category") 1632 protected CodeableConcept category; 1633 1634 /** 1635 * Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres. 1636 */ 1637 @Child(name = "specification", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1638 @Description(shortDefinition="Identifies the standard, specification, or formal guidance that the device adheres to", formalDefinition="Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres." ) 1639 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-specification-type") 1640 protected CodeableConcept specification; 1641 1642 /** 1643 * Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label. 1644 */ 1645 @Child(name = "version", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1646 @Description(shortDefinition="Specific form or variant of the standard", formalDefinition="Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label." ) 1647 protected StringType version; 1648 1649 private static final long serialVersionUID = 1592712180L; 1650 1651 /** 1652 * Constructor 1653 */ 1654 public DeviceConformsToComponent() { 1655 super(); 1656 } 1657 1658 /** 1659 * Constructor 1660 */ 1661 public DeviceConformsToComponent(CodeableConcept specification) { 1662 super(); 1663 this.setSpecification(specification); 1664 } 1665 1666 /** 1667 * @return {@link #category} (Describes the type of the standard, specification, or formal guidance.) 1668 */ 1669 public CodeableConcept getCategory() { 1670 if (this.category == null) 1671 if (Configuration.errorOnAutoCreate()) 1672 throw new Error("Attempt to auto-create DeviceConformsToComponent.category"); 1673 else if (Configuration.doAutoCreate()) 1674 this.category = new CodeableConcept(); // cc 1675 return this.category; 1676 } 1677 1678 public boolean hasCategory() { 1679 return this.category != null && !this.category.isEmpty(); 1680 } 1681 1682 /** 1683 * @param value {@link #category} (Describes the type of the standard, specification, or formal guidance.) 1684 */ 1685 public DeviceConformsToComponent setCategory(CodeableConcept value) { 1686 this.category = value; 1687 return this; 1688 } 1689 1690 /** 1691 * @return {@link #specification} (Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.) 1692 */ 1693 public CodeableConcept getSpecification() { 1694 if (this.specification == null) 1695 if (Configuration.errorOnAutoCreate()) 1696 throw new Error("Attempt to auto-create DeviceConformsToComponent.specification"); 1697 else if (Configuration.doAutoCreate()) 1698 this.specification = new CodeableConcept(); // cc 1699 return this.specification; 1700 } 1701 1702 public boolean hasSpecification() { 1703 return this.specification != null && !this.specification.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #specification} (Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.) 1708 */ 1709 public DeviceConformsToComponent setSpecification(CodeableConcept value) { 1710 this.specification = value; 1711 return this; 1712 } 1713 1714 /** 1715 * @return {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1716 */ 1717 public StringType getVersionElement() { 1718 if (this.version == null) 1719 if (Configuration.errorOnAutoCreate()) 1720 throw new Error("Attempt to auto-create DeviceConformsToComponent.version"); 1721 else if (Configuration.doAutoCreate()) 1722 this.version = new StringType(); // bb 1723 return this.version; 1724 } 1725 1726 public boolean hasVersionElement() { 1727 return this.version != null && !this.version.isEmpty(); 1728 } 1729 1730 public boolean hasVersion() { 1731 return this.version != null && !this.version.isEmpty(); 1732 } 1733 1734 /** 1735 * @param value {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1736 */ 1737 public DeviceConformsToComponent setVersionElement(StringType value) { 1738 this.version = value; 1739 return this; 1740 } 1741 1742 /** 1743 * @return Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label. 1744 */ 1745 public String getVersion() { 1746 return this.version == null ? null : this.version.getValue(); 1747 } 1748 1749 /** 1750 * @param value Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label. 1751 */ 1752 public DeviceConformsToComponent setVersion(String value) { 1753 if (Utilities.noString(value)) 1754 this.version = null; 1755 else { 1756 if (this.version == null) 1757 this.version = new StringType(); 1758 this.version.setValue(value); 1759 } 1760 return this; 1761 } 1762 1763 protected void listChildren(List<Property> children) { 1764 super.listChildren(children); 1765 children.add(new Property("category", "CodeableConcept", "Describes the type of the standard, specification, or formal guidance.", 0, 1, category)); 1766 children.add(new Property("specification", "CodeableConcept", "Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.", 0, 1, specification)); 1767 children.add(new Property("version", "string", "Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.", 0, 1, version)); 1768 } 1769 1770 @Override 1771 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1772 switch (_hash) { 1773 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Describes the type of the standard, specification, or formal guidance.", 0, 1, category); 1774 case 1307197699: /*specification*/ return new Property("specification", "CodeableConcept", "Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.", 0, 1, specification); 1775 case 351608024: /*version*/ return new Property("version", "string", "Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.", 0, 1, version); 1776 default: return super.getNamedProperty(_hash, _name, _checkValid); 1777 } 1778 1779 } 1780 1781 @Override 1782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1783 switch (hash) { 1784 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1785 case 1307197699: /*specification*/ return this.specification == null ? new Base[0] : new Base[] {this.specification}; // CodeableConcept 1786 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1787 default: return super.getProperty(hash, name, checkValid); 1788 } 1789 1790 } 1791 1792 @Override 1793 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1794 switch (hash) { 1795 case 50511102: // category 1796 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1797 return value; 1798 case 1307197699: // specification 1799 this.specification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1800 return value; 1801 case 351608024: // version 1802 this.version = TypeConvertor.castToString(value); // StringType 1803 return value; 1804 default: return super.setProperty(hash, name, value); 1805 } 1806 1807 } 1808 1809 @Override 1810 public Base setProperty(String name, Base value) throws FHIRException { 1811 if (name.equals("category")) { 1812 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1813 } else if (name.equals("specification")) { 1814 this.specification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1815 } else if (name.equals("version")) { 1816 this.version = TypeConvertor.castToString(value); // StringType 1817 } else 1818 return super.setProperty(name, value); 1819 return value; 1820 } 1821 1822 @Override 1823 public void removeChild(String name, Base value) throws FHIRException { 1824 if (name.equals("category")) { 1825 this.category = null; 1826 } else if (name.equals("specification")) { 1827 this.specification = null; 1828 } else if (name.equals("version")) { 1829 this.version = null; 1830 } else 1831 super.removeChild(name, value); 1832 1833 } 1834 1835 @Override 1836 public Base makeProperty(int hash, String name) throws FHIRException { 1837 switch (hash) { 1838 case 50511102: return getCategory(); 1839 case 1307197699: return getSpecification(); 1840 case 351608024: return getVersionElement(); 1841 default: return super.makeProperty(hash, name); 1842 } 1843 1844 } 1845 1846 @Override 1847 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1848 switch (hash) { 1849 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1850 case 1307197699: /*specification*/ return new String[] {"CodeableConcept"}; 1851 case 351608024: /*version*/ return new String[] {"string"}; 1852 default: return super.getTypesForProperty(hash, name); 1853 } 1854 1855 } 1856 1857 @Override 1858 public Base addChild(String name) throws FHIRException { 1859 if (name.equals("category")) { 1860 this.category = new CodeableConcept(); 1861 return this.category; 1862 } 1863 else if (name.equals("specification")) { 1864 this.specification = new CodeableConcept(); 1865 return this.specification; 1866 } 1867 else if (name.equals("version")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property Device.conformsTo.version"); 1869 } 1870 else 1871 return super.addChild(name); 1872 } 1873 1874 public DeviceConformsToComponent copy() { 1875 DeviceConformsToComponent dst = new DeviceConformsToComponent(); 1876 copyValues(dst); 1877 return dst; 1878 } 1879 1880 public void copyValues(DeviceConformsToComponent dst) { 1881 super.copyValues(dst); 1882 dst.category = category == null ? null : category.copy(); 1883 dst.specification = specification == null ? null : specification.copy(); 1884 dst.version = version == null ? null : version.copy(); 1885 } 1886 1887 @Override 1888 public boolean equalsDeep(Base other_) { 1889 if (!super.equalsDeep(other_)) 1890 return false; 1891 if (!(other_ instanceof DeviceConformsToComponent)) 1892 return false; 1893 DeviceConformsToComponent o = (DeviceConformsToComponent) other_; 1894 return compareDeep(category, o.category, true) && compareDeep(specification, o.specification, true) 1895 && compareDeep(version, o.version, true); 1896 } 1897 1898 @Override 1899 public boolean equalsShallow(Base other_) { 1900 if (!super.equalsShallow(other_)) 1901 return false; 1902 if (!(other_ instanceof DeviceConformsToComponent)) 1903 return false; 1904 DeviceConformsToComponent o = (DeviceConformsToComponent) other_; 1905 return compareValues(version, o.version, true); 1906 } 1907 1908 public boolean isEmpty() { 1909 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, specification, version 1910 ); 1911 } 1912 1913 public String fhirType() { 1914 return "Device.conformsTo"; 1915 1916 } 1917 1918 } 1919 1920 @Block() 1921 public static class DevicePropertyComponent extends BackboneElement implements IBaseBackboneElement { 1922 /** 1923 * Code that specifies the property, such as resolution, color, size, being represented. 1924 */ 1925 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1926 @Description(shortDefinition="Code that specifies the property being represented", formalDefinition="Code that specifies the property, such as resolution, color, size, being represented." ) 1927 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-property-type") 1928 protected CodeableConcept type; 1929 1930 /** 1931 * The value of the property specified by the associated property.type code. 1932 */ 1933 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Attachment.class}, order=2, min=1, max=1, modifier=false, summary=false) 1934 @Description(shortDefinition="Value of the property", formalDefinition="The value of the property specified by the associated property.type code." ) 1935 protected DataType value; 1936 1937 private static final long serialVersionUID = -1659186716L; 1938 1939 /** 1940 * Constructor 1941 */ 1942 public DevicePropertyComponent() { 1943 super(); 1944 } 1945 1946 /** 1947 * Constructor 1948 */ 1949 public DevicePropertyComponent(CodeableConcept type, DataType value) { 1950 super(); 1951 this.setType(type); 1952 this.setValue(value); 1953 } 1954 1955 /** 1956 * @return {@link #type} (Code that specifies the property, such as resolution, color, size, being represented.) 1957 */ 1958 public CodeableConcept getType() { 1959 if (this.type == null) 1960 if (Configuration.errorOnAutoCreate()) 1961 throw new Error("Attempt to auto-create DevicePropertyComponent.type"); 1962 else if (Configuration.doAutoCreate()) 1963 this.type = new CodeableConcept(); // cc 1964 return this.type; 1965 } 1966 1967 public boolean hasType() { 1968 return this.type != null && !this.type.isEmpty(); 1969 } 1970 1971 /** 1972 * @param value {@link #type} (Code that specifies the property, such as resolution, color, size, being represented.) 1973 */ 1974 public DevicePropertyComponent setType(CodeableConcept value) { 1975 this.type = value; 1976 return this; 1977 } 1978 1979 /** 1980 * @return {@link #value} (The value of the property specified by the associated property.type code.) 1981 */ 1982 public DataType getValue() { 1983 return this.value; 1984 } 1985 1986 /** 1987 * @return {@link #value} (The value of the property specified by the associated property.type code.) 1988 */ 1989 public Quantity getValueQuantity() throws FHIRException { 1990 if (this.value == null) 1991 this.value = new Quantity(); 1992 if (!(this.value instanceof Quantity)) 1993 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1994 return (Quantity) this.value; 1995 } 1996 1997 public boolean hasValueQuantity() { 1998 return this.value instanceof Quantity; 1999 } 2000 2001 /** 2002 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2003 */ 2004 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2005 if (this.value == null) 2006 this.value = new CodeableConcept(); 2007 if (!(this.value instanceof CodeableConcept)) 2008 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2009 return (CodeableConcept) this.value; 2010 } 2011 2012 public boolean hasValueCodeableConcept() { 2013 return this.value instanceof CodeableConcept; 2014 } 2015 2016 /** 2017 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2018 */ 2019 public StringType getValueStringType() throws FHIRException { 2020 if (this.value == null) 2021 this.value = new StringType(); 2022 if (!(this.value instanceof StringType)) 2023 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2024 return (StringType) this.value; 2025 } 2026 2027 public boolean hasValueStringType() { 2028 return this.value instanceof StringType; 2029 } 2030 2031 /** 2032 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2033 */ 2034 public BooleanType getValueBooleanType() throws FHIRException { 2035 if (this.value == null) 2036 this.value = new BooleanType(); 2037 if (!(this.value instanceof BooleanType)) 2038 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2039 return (BooleanType) this.value; 2040 } 2041 2042 public boolean hasValueBooleanType() { 2043 return this.value instanceof BooleanType; 2044 } 2045 2046 /** 2047 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2048 */ 2049 public IntegerType getValueIntegerType() throws FHIRException { 2050 if (this.value == null) 2051 this.value = new IntegerType(); 2052 if (!(this.value instanceof IntegerType)) 2053 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2054 return (IntegerType) this.value; 2055 } 2056 2057 public boolean hasValueIntegerType() { 2058 return this.value instanceof IntegerType; 2059 } 2060 2061 /** 2062 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2063 */ 2064 public Range getValueRange() throws FHIRException { 2065 if (this.value == null) 2066 this.value = new Range(); 2067 if (!(this.value instanceof Range)) 2068 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2069 return (Range) this.value; 2070 } 2071 2072 public boolean hasValueRange() { 2073 return this.value instanceof Range; 2074 } 2075 2076 /** 2077 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2078 */ 2079 public Attachment getValueAttachment() throws FHIRException { 2080 if (this.value == null) 2081 this.value = new Attachment(); 2082 if (!(this.value instanceof Attachment)) 2083 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 2084 return (Attachment) this.value; 2085 } 2086 2087 public boolean hasValueAttachment() { 2088 return this.value instanceof Attachment; 2089 } 2090 2091 public boolean hasValue() { 2092 return this.value != null && !this.value.isEmpty(); 2093 } 2094 2095 /** 2096 * @param value {@link #value} (The value of the property specified by the associated property.type code.) 2097 */ 2098 public DevicePropertyComponent setValue(DataType value) { 2099 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Attachment)) 2100 throw new FHIRException("Not the right type for Device.property.value[x]: "+value.fhirType()); 2101 this.value = value; 2102 return this; 2103 } 2104 2105 protected void listChildren(List<Property> children) { 2106 super.listChildren(children); 2107 children.add(new Property("type", "CodeableConcept", "Code that specifies the property, such as resolution, color, size, being represented.", 0, 1, type)); 2108 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value)); 2109 } 2110 2111 @Override 2112 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2113 switch (_hash) { 2114 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code that specifies the property, such as resolution, color, size, being represented.", 0, 1, type); 2115 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 2116 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 2117 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the property specified by the associated property.type code.", 0, 1, value); 2118 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the property specified by the associated property.type code.", 0, 1, value); 2119 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the property specified by the associated property.type code.", 0, 1, value); 2120 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the property specified by the associated property.type code.", 0, 1, value); 2121 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the property specified by the associated property.type code.", 0, 1, value); 2122 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the property specified by the associated property.type code.", 0, 1, value); 2123 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 2124 default: return super.getNamedProperty(_hash, _name, _checkValid); 2125 } 2126 2127 } 2128 2129 @Override 2130 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2131 switch (hash) { 2132 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2133 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2134 default: return super.getProperty(hash, name, checkValid); 2135 } 2136 2137 } 2138 2139 @Override 2140 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2141 switch (hash) { 2142 case 3575610: // type 2143 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2144 return value; 2145 case 111972721: // value 2146 this.value = TypeConvertor.castToType(value); // DataType 2147 return value; 2148 default: return super.setProperty(hash, name, value); 2149 } 2150 2151 } 2152 2153 @Override 2154 public Base setProperty(String name, Base value) throws FHIRException { 2155 if (name.equals("type")) { 2156 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2157 } else if (name.equals("value[x]")) { 2158 this.value = TypeConvertor.castToType(value); // DataType 2159 } else 2160 return super.setProperty(name, value); 2161 return value; 2162 } 2163 2164 @Override 2165 public void removeChild(String name, Base value) throws FHIRException { 2166 if (name.equals("type")) { 2167 this.type = null; 2168 } else if (name.equals("value[x]")) { 2169 this.value = null; 2170 } else 2171 super.removeChild(name, value); 2172 2173 } 2174 2175 @Override 2176 public Base makeProperty(int hash, String name) throws FHIRException { 2177 switch (hash) { 2178 case 3575610: return getType(); 2179 case -1410166417: return getValue(); 2180 case 111972721: return getValue(); 2181 default: return super.makeProperty(hash, name); 2182 } 2183 2184 } 2185 2186 @Override 2187 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2188 switch (hash) { 2189 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2190 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Attachment"}; 2191 default: return super.getTypesForProperty(hash, name); 2192 } 2193 2194 } 2195 2196 @Override 2197 public Base addChild(String name) throws FHIRException { 2198 if (name.equals("type")) { 2199 this.type = new CodeableConcept(); 2200 return this.type; 2201 } 2202 else if (name.equals("valueQuantity")) { 2203 this.value = new Quantity(); 2204 return this.value; 2205 } 2206 else if (name.equals("valueCodeableConcept")) { 2207 this.value = new CodeableConcept(); 2208 return this.value; 2209 } 2210 else if (name.equals("valueString")) { 2211 this.value = new StringType(); 2212 return this.value; 2213 } 2214 else if (name.equals("valueBoolean")) { 2215 this.value = new BooleanType(); 2216 return this.value; 2217 } 2218 else if (name.equals("valueInteger")) { 2219 this.value = new IntegerType(); 2220 return this.value; 2221 } 2222 else if (name.equals("valueRange")) { 2223 this.value = new Range(); 2224 return this.value; 2225 } 2226 else if (name.equals("valueAttachment")) { 2227 this.value = new Attachment(); 2228 return this.value; 2229 } 2230 else 2231 return super.addChild(name); 2232 } 2233 2234 public DevicePropertyComponent copy() { 2235 DevicePropertyComponent dst = new DevicePropertyComponent(); 2236 copyValues(dst); 2237 return dst; 2238 } 2239 2240 public void copyValues(DevicePropertyComponent dst) { 2241 super.copyValues(dst); 2242 dst.type = type == null ? null : type.copy(); 2243 dst.value = value == null ? null : value.copy(); 2244 } 2245 2246 @Override 2247 public boolean equalsDeep(Base other_) { 2248 if (!super.equalsDeep(other_)) 2249 return false; 2250 if (!(other_ instanceof DevicePropertyComponent)) 2251 return false; 2252 DevicePropertyComponent o = (DevicePropertyComponent) other_; 2253 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2254 } 2255 2256 @Override 2257 public boolean equalsShallow(Base other_) { 2258 if (!super.equalsShallow(other_)) 2259 return false; 2260 if (!(other_ instanceof DevicePropertyComponent)) 2261 return false; 2262 DevicePropertyComponent o = (DevicePropertyComponent) other_; 2263 return true; 2264 } 2265 2266 public boolean isEmpty() { 2267 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2268 } 2269 2270 public String fhirType() { 2271 return "Device.property"; 2272 2273 } 2274 2275 } 2276 2277 /** 2278 * Unique instance identifiers assigned to a device by manufacturers other organizations or owners. 2279 */ 2280 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2281 @Description(shortDefinition="Instance identifier", formalDefinition="Unique instance identifiers assigned to a device by manufacturers other organizations or owners." ) 2282 protected List<Identifier> identifier; 2283 2284 /** 2285 * The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name. 2286 */ 2287 @Child(name = "displayName", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2288 @Description(shortDefinition="The name used to display by default when the device is referenced", formalDefinition="The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name." ) 2289 protected StringType displayName; 2290 2291 /** 2292 * The reference to the definition for the device. 2293 */ 2294 @Child(name = "definition", type = {CodeableReference.class}, order=2, min=0, max=1, modifier=false, summary=false) 2295 @Description(shortDefinition="The reference to the definition for the device", formalDefinition="The reference to the definition for the device." ) 2296 protected CodeableReference definition; 2297 2298 /** 2299 * Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold. 2300 */ 2301 @Child(name = "udiCarrier", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2302 @Description(shortDefinition="Unique Device Identifier (UDI) Barcode string", formalDefinition="Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold." ) 2303 protected List<DeviceUdiCarrierComponent> udiCarrier; 2304 2305 /** 2306 * The Device record status. This is not the status of the device like availability. 2307 */ 2308 @Child(name = "status", type = {CodeType.class}, order=4, min=0, max=1, modifier=true, summary=true) 2309 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="The Device record status. This is not the status of the device like availability." ) 2310 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-status") 2311 protected Enumeration<FHIRDeviceStatus> status; 2312 2313 /** 2314 * The availability of the device. 2315 */ 2316 @Child(name = "availabilityStatus", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 2317 @Description(shortDefinition="lost | damaged | destroyed | available", formalDefinition="The availability of the device." ) 2318 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-availability-status") 2319 protected CodeableConcept availabilityStatus; 2320 2321 /** 2322 * An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled. 2323 */ 2324 @Child(name = "biologicalSourceEvent", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 2325 @Description(shortDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled", formalDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled." ) 2326 protected Identifier biologicalSourceEvent; 2327 2328 /** 2329 * A name of the manufacturer or entity legally responsible for the device. 2330 */ 2331 @Child(name = "manufacturer", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 2332 @Description(shortDefinition="Name of device manufacturer", formalDefinition="A name of the manufacturer or entity legally responsible for the device." ) 2333 protected StringType manufacturer; 2334 2335 /** 2336 * The date and time when the device was manufactured. 2337 */ 2338 @Child(name = "manufactureDate", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2339 @Description(shortDefinition="Date when the device was made", formalDefinition="The date and time when the device was manufactured." ) 2340 protected DateTimeType manufactureDate; 2341 2342 /** 2343 * The date and time beyond which this device is no longer valid or should not be used (if applicable). 2344 */ 2345 @Child(name = "expirationDate", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 2346 @Description(shortDefinition="Date and time of expiry of this device (if applicable)", formalDefinition="The date and time beyond which this device is no longer valid or should not be used (if applicable)." ) 2347 protected DateTimeType expirationDate; 2348 2349 /** 2350 * Lot number assigned by the manufacturer. 2351 */ 2352 @Child(name = "lotNumber", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 2353 @Description(shortDefinition="Lot number of manufacture", formalDefinition="Lot number assigned by the manufacturer." ) 2354 protected StringType lotNumber; 2355 2356 /** 2357 * The serial number assigned by the organization when the device was manufactured. 2358 */ 2359 @Child(name = "serialNumber", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2360 @Description(shortDefinition="Serial number assigned by the manufacturer", formalDefinition="The serial number assigned by the organization when the device was manufactured." ) 2361 protected StringType serialNumber; 2362 2363 /** 2364 * This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition. 2365 */ 2366 @Child(name = "name", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2367 @Description(shortDefinition="The name or names of the device as known to the manufacturer and/or patient", formalDefinition="This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition." ) 2368 protected List<DeviceNameComponent> name; 2369 2370 /** 2371 * The manufacturer's model number for the device. 2372 */ 2373 @Child(name = "modelNumber", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 2374 @Description(shortDefinition="The manufacturer's model number for the device", formalDefinition="The manufacturer's model number for the device." ) 2375 protected StringType modelNumber; 2376 2377 /** 2378 * The part number or catalog number of the device. 2379 */ 2380 @Child(name = "partNumber", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 2381 @Description(shortDefinition="The part number or catalog number of the device", formalDefinition="The part number or catalog number of the device." ) 2382 protected StringType partNumber; 2383 2384 /** 2385 * Devices may be associated with one or more categories. 2386 */ 2387 @Child(name = "category", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2388 @Description(shortDefinition="Indicates a high-level grouping of the device", formalDefinition="Devices may be associated with one or more categories." ) 2389 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-category") 2390 protected List<CodeableConcept> category; 2391 2392 /** 2393 * The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device. 2394 */ 2395 @Child(name = "type", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2396 @Description(shortDefinition="The kind or type of device", formalDefinition="The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device." ) 2397 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-type") 2398 protected List<CodeableConcept> type; 2399 2400 /** 2401 * The actual design of the device or software version running on the device. 2402 */ 2403 @Child(name = "version", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2404 @Description(shortDefinition="The actual design of the device or software version running on the device", formalDefinition="The actual design of the device or software version running on the device." ) 2405 protected List<DeviceVersionComponent> version; 2406 2407 /** 2408 * Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards. 2409 */ 2410 @Child(name = "conformsTo", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2411 @Description(shortDefinition="Identifies the standards, specifications, or formal guidances for the capabilities supported by the device", formalDefinition="Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards." ) 2412 protected List<DeviceConformsToComponent> conformsTo; 2413 2414 /** 2415 * Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes. 2416 */ 2417 @Child(name = "property", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2418 @Description(shortDefinition="Inherent, essentially fixed, characteristics of the device. e.g., time properties, size, material, etc.", formalDefinition="Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes." ) 2419 protected List<DevicePropertyComponent> property; 2420 2421 /** 2422 * The designated condition for performing a task with the device. 2423 */ 2424 @Child(name = "mode", type = {CodeableConcept.class}, order=20, min=0, max=1, modifier=false, summary=false) 2425 @Description(shortDefinition="The designated condition for performing a task", formalDefinition="The designated condition for performing a task with the device." ) 2426 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-operation-mode") 2427 protected CodeableConcept mode; 2428 2429 /** 2430 * The series of occurrences that repeats during the operation of the device. 2431 */ 2432 @Child(name = "cycle", type = {Count.class}, order=21, min=0, max=1, modifier=false, summary=false) 2433 @Description(shortDefinition="The series of occurrences that repeats during the operation of the device", formalDefinition="The series of occurrences that repeats during the operation of the device." ) 2434 protected Count cycle; 2435 2436 /** 2437 * A measurement of time during the device's operation (e.g., days, hours, mins, etc.). 2438 */ 2439 @Child(name = "duration", type = {Duration.class}, order=22, min=0, max=1, modifier=false, summary=false) 2440 @Description(shortDefinition="A measurement of time during the device's operation (e.g., days, hours, mins, etc.)", formalDefinition="A measurement of time during the device's operation (e.g., days, hours, mins, etc.)." ) 2441 protected Duration duration; 2442 2443 /** 2444 * An organization that is responsible for the provision and ongoing maintenance of the device. 2445 */ 2446 @Child(name = "owner", type = {Organization.class}, order=23, min=0, max=1, modifier=false, summary=false) 2447 @Description(shortDefinition="Organization responsible for device", formalDefinition="An organization that is responsible for the provision and ongoing maintenance of the device." ) 2448 protected Reference owner; 2449 2450 /** 2451 * Contact details for an organization or a particular human that is responsible for the device. 2452 */ 2453 @Child(name = "contact", type = {ContactPoint.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2454 @Description(shortDefinition="Details for human/organization for support", formalDefinition="Contact details for an organization or a particular human that is responsible for the device." ) 2455 protected List<ContactPoint> contact; 2456 2457 /** 2458 * The place where the device can be found. 2459 */ 2460 @Child(name = "location", type = {Location.class}, order=25, min=0, max=1, modifier=false, summary=false) 2461 @Description(shortDefinition="Where the device is found", formalDefinition="The place where the device can be found." ) 2462 protected Reference location; 2463 2464 /** 2465 * A network address on which the device may be contacted directly. 2466 */ 2467 @Child(name = "url", type = {UriType.class}, order=26, min=0, max=1, modifier=false, summary=false) 2468 @Description(shortDefinition="Network address to contact device", formalDefinition="A network address on which the device may be contacted directly." ) 2469 protected UriType url; 2470 2471 /** 2472 * Technical endpoints providing access to services provided by the device defined at this resource. 2473 */ 2474 @Child(name = "endpoint", type = {Endpoint.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2475 @Description(shortDefinition="Technical endpoints providing access to electronic services provided by the device", formalDefinition="Technical endpoints providing access to services provided by the device defined at this resource." ) 2476 protected List<Reference> endpoint; 2477 2478 /** 2479 * The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data). 2480 */ 2481 @Child(name = "gateway", type = {CodeableReference.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2482 @Description(shortDefinition="Linked device acting as a communication/data collector, translator or controller", formalDefinition="The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data)." ) 2483 protected List<CodeableReference> gateway; 2484 2485 /** 2486 * Descriptive information, usage information or implantation information that is not captured in an existing element. 2487 */ 2488 @Child(name = "note", type = {Annotation.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2489 @Description(shortDefinition="Device notes and comments", formalDefinition="Descriptive information, usage information or implantation information that is not captured in an existing element." ) 2490 protected List<Annotation> note; 2491 2492 /** 2493 * Provides additional safety characteristics about a medical device. For example devices containing latex. 2494 */ 2495 @Child(name = "safety", type = {CodeableConcept.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2496 @Description(shortDefinition="Safety Characteristics of Device", formalDefinition="Provides additional safety characteristics about a medical device. For example devices containing latex." ) 2497 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-safety") 2498 protected List<CodeableConcept> safety; 2499 2500 /** 2501 * The higher level or encompassing device that this device is a logical part of. 2502 */ 2503 @Child(name = "parent", type = {Device.class}, order=31, min=0, max=1, modifier=false, summary=false) 2504 @Description(shortDefinition="The higher level or encompassing device that this device is a logical part of", formalDefinition="The higher level or encompassing device that this device is a logical part of." ) 2505 protected Reference parent; 2506 2507 private static final long serialVersionUID = 2120085847L; 2508 2509 /** 2510 * Constructor 2511 */ 2512 public Device() { 2513 super(); 2514 } 2515 2516 /** 2517 * @return {@link #identifier} (Unique instance identifiers assigned to a device by manufacturers other organizations or owners.) 2518 */ 2519 public List<Identifier> getIdentifier() { 2520 if (this.identifier == null) 2521 this.identifier = new ArrayList<Identifier>(); 2522 return this.identifier; 2523 } 2524 2525 /** 2526 * @return Returns a reference to <code>this</code> for easy method chaining 2527 */ 2528 public Device setIdentifier(List<Identifier> theIdentifier) { 2529 this.identifier = theIdentifier; 2530 return this; 2531 } 2532 2533 public boolean hasIdentifier() { 2534 if (this.identifier == null) 2535 return false; 2536 for (Identifier item : this.identifier) 2537 if (!item.isEmpty()) 2538 return true; 2539 return false; 2540 } 2541 2542 public Identifier addIdentifier() { //3 2543 Identifier t = new Identifier(); 2544 if (this.identifier == null) 2545 this.identifier = new ArrayList<Identifier>(); 2546 this.identifier.add(t); 2547 return t; 2548 } 2549 2550 public Device addIdentifier(Identifier t) { //3 2551 if (t == null) 2552 return this; 2553 if (this.identifier == null) 2554 this.identifier = new ArrayList<Identifier>(); 2555 this.identifier.add(t); 2556 return this; 2557 } 2558 2559 /** 2560 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2561 */ 2562 public Identifier getIdentifierFirstRep() { 2563 if (getIdentifier().isEmpty()) { 2564 addIdentifier(); 2565 } 2566 return getIdentifier().get(0); 2567 } 2568 2569 /** 2570 * @return {@link #displayName} (The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.). This is the underlying object with id, value and extensions. The accessor "getDisplayName" gives direct access to the value 2571 */ 2572 public StringType getDisplayNameElement() { 2573 if (this.displayName == null) 2574 if (Configuration.errorOnAutoCreate()) 2575 throw new Error("Attempt to auto-create Device.displayName"); 2576 else if (Configuration.doAutoCreate()) 2577 this.displayName = new StringType(); // bb 2578 return this.displayName; 2579 } 2580 2581 public boolean hasDisplayNameElement() { 2582 return this.displayName != null && !this.displayName.isEmpty(); 2583 } 2584 2585 public boolean hasDisplayName() { 2586 return this.displayName != null && !this.displayName.isEmpty(); 2587 } 2588 2589 /** 2590 * @param value {@link #displayName} (The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.). This is the underlying object with id, value and extensions. The accessor "getDisplayName" gives direct access to the value 2591 */ 2592 public Device setDisplayNameElement(StringType value) { 2593 this.displayName = value; 2594 return this; 2595 } 2596 2597 /** 2598 * @return The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name. 2599 */ 2600 public String getDisplayName() { 2601 return this.displayName == null ? null : this.displayName.getValue(); 2602 } 2603 2604 /** 2605 * @param value The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name. 2606 */ 2607 public Device setDisplayName(String value) { 2608 if (Utilities.noString(value)) 2609 this.displayName = null; 2610 else { 2611 if (this.displayName == null) 2612 this.displayName = new StringType(); 2613 this.displayName.setValue(value); 2614 } 2615 return this; 2616 } 2617 2618 /** 2619 * @return {@link #definition} (The reference to the definition for the device.) 2620 */ 2621 public CodeableReference getDefinition() { 2622 if (this.definition == null) 2623 if (Configuration.errorOnAutoCreate()) 2624 throw new Error("Attempt to auto-create Device.definition"); 2625 else if (Configuration.doAutoCreate()) 2626 this.definition = new CodeableReference(); // cc 2627 return this.definition; 2628 } 2629 2630 public boolean hasDefinition() { 2631 return this.definition != null && !this.definition.isEmpty(); 2632 } 2633 2634 /** 2635 * @param value {@link #definition} (The reference to the definition for the device.) 2636 */ 2637 public Device setDefinition(CodeableReference value) { 2638 this.definition = value; 2639 return this; 2640 } 2641 2642 /** 2643 * @return {@link #udiCarrier} (Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.) 2644 */ 2645 public List<DeviceUdiCarrierComponent> getUdiCarrier() { 2646 if (this.udiCarrier == null) 2647 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2648 return this.udiCarrier; 2649 } 2650 2651 /** 2652 * @return Returns a reference to <code>this</code> for easy method chaining 2653 */ 2654 public Device setUdiCarrier(List<DeviceUdiCarrierComponent> theUdiCarrier) { 2655 this.udiCarrier = theUdiCarrier; 2656 return this; 2657 } 2658 2659 public boolean hasUdiCarrier() { 2660 if (this.udiCarrier == null) 2661 return false; 2662 for (DeviceUdiCarrierComponent item : this.udiCarrier) 2663 if (!item.isEmpty()) 2664 return true; 2665 return false; 2666 } 2667 2668 public DeviceUdiCarrierComponent addUdiCarrier() { //3 2669 DeviceUdiCarrierComponent t = new DeviceUdiCarrierComponent(); 2670 if (this.udiCarrier == null) 2671 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2672 this.udiCarrier.add(t); 2673 return t; 2674 } 2675 2676 public Device addUdiCarrier(DeviceUdiCarrierComponent t) { //3 2677 if (t == null) 2678 return this; 2679 if (this.udiCarrier == null) 2680 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2681 this.udiCarrier.add(t); 2682 return this; 2683 } 2684 2685 /** 2686 * @return The first repetition of repeating field {@link #udiCarrier}, creating it if it does not already exist {3} 2687 */ 2688 public DeviceUdiCarrierComponent getUdiCarrierFirstRep() { 2689 if (getUdiCarrier().isEmpty()) { 2690 addUdiCarrier(); 2691 } 2692 return getUdiCarrier().get(0); 2693 } 2694 2695 /** 2696 * @return {@link #status} (The Device record status. This is not the status of the device like availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2697 */ 2698 public Enumeration<FHIRDeviceStatus> getStatusElement() { 2699 if (this.status == null) 2700 if (Configuration.errorOnAutoCreate()) 2701 throw new Error("Attempt to auto-create Device.status"); 2702 else if (Configuration.doAutoCreate()) 2703 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); // bb 2704 return this.status; 2705 } 2706 2707 public boolean hasStatusElement() { 2708 return this.status != null && !this.status.isEmpty(); 2709 } 2710 2711 public boolean hasStatus() { 2712 return this.status != null && !this.status.isEmpty(); 2713 } 2714 2715 /** 2716 * @param value {@link #status} (The Device record status. This is not the status of the device like availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2717 */ 2718 public Device setStatusElement(Enumeration<FHIRDeviceStatus> value) { 2719 this.status = value; 2720 return this; 2721 } 2722 2723 /** 2724 * @return The Device record status. This is not the status of the device like availability. 2725 */ 2726 public FHIRDeviceStatus getStatus() { 2727 return this.status == null ? null : this.status.getValue(); 2728 } 2729 2730 /** 2731 * @param value The Device record status. This is not the status of the device like availability. 2732 */ 2733 public Device setStatus(FHIRDeviceStatus value) { 2734 if (value == null) 2735 this.status = null; 2736 else { 2737 if (this.status == null) 2738 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); 2739 this.status.setValue(value); 2740 } 2741 return this; 2742 } 2743 2744 /** 2745 * @return {@link #availabilityStatus} (The availability of the device.) 2746 */ 2747 public CodeableConcept getAvailabilityStatus() { 2748 if (this.availabilityStatus == null) 2749 if (Configuration.errorOnAutoCreate()) 2750 throw new Error("Attempt to auto-create Device.availabilityStatus"); 2751 else if (Configuration.doAutoCreate()) 2752 this.availabilityStatus = new CodeableConcept(); // cc 2753 return this.availabilityStatus; 2754 } 2755 2756 public boolean hasAvailabilityStatus() { 2757 return this.availabilityStatus != null && !this.availabilityStatus.isEmpty(); 2758 } 2759 2760 /** 2761 * @param value {@link #availabilityStatus} (The availability of the device.) 2762 */ 2763 public Device setAvailabilityStatus(CodeableConcept value) { 2764 this.availabilityStatus = value; 2765 return this; 2766 } 2767 2768 /** 2769 * @return {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 2770 */ 2771 public Identifier getBiologicalSourceEvent() { 2772 if (this.biologicalSourceEvent == null) 2773 if (Configuration.errorOnAutoCreate()) 2774 throw new Error("Attempt to auto-create Device.biologicalSourceEvent"); 2775 else if (Configuration.doAutoCreate()) 2776 this.biologicalSourceEvent = new Identifier(); // cc 2777 return this.biologicalSourceEvent; 2778 } 2779 2780 public boolean hasBiologicalSourceEvent() { 2781 return this.biologicalSourceEvent != null && !this.biologicalSourceEvent.isEmpty(); 2782 } 2783 2784 /** 2785 * @param value {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 2786 */ 2787 public Device setBiologicalSourceEvent(Identifier value) { 2788 this.biologicalSourceEvent = value; 2789 return this; 2790 } 2791 2792 /** 2793 * @return {@link #manufacturer} (A name of the manufacturer or entity legally responsible for the device.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value 2794 */ 2795 public StringType getManufacturerElement() { 2796 if (this.manufacturer == null) 2797 if (Configuration.errorOnAutoCreate()) 2798 throw new Error("Attempt to auto-create Device.manufacturer"); 2799 else if (Configuration.doAutoCreate()) 2800 this.manufacturer = new StringType(); // bb 2801 return this.manufacturer; 2802 } 2803 2804 public boolean hasManufacturerElement() { 2805 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2806 } 2807 2808 public boolean hasManufacturer() { 2809 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2810 } 2811 2812 /** 2813 * @param value {@link #manufacturer} (A name of the manufacturer or entity legally responsible for the device.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value 2814 */ 2815 public Device setManufacturerElement(StringType value) { 2816 this.manufacturer = value; 2817 return this; 2818 } 2819 2820 /** 2821 * @return A name of the manufacturer or entity legally responsible for the device. 2822 */ 2823 public String getManufacturer() { 2824 return this.manufacturer == null ? null : this.manufacturer.getValue(); 2825 } 2826 2827 /** 2828 * @param value A name of the manufacturer or entity legally responsible for the device. 2829 */ 2830 public Device setManufacturer(String value) { 2831 if (Utilities.noString(value)) 2832 this.manufacturer = null; 2833 else { 2834 if (this.manufacturer == null) 2835 this.manufacturer = new StringType(); 2836 this.manufacturer.setValue(value); 2837 } 2838 return this; 2839 } 2840 2841 /** 2842 * @return {@link #manufactureDate} (The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value 2843 */ 2844 public DateTimeType getManufactureDateElement() { 2845 if (this.manufactureDate == null) 2846 if (Configuration.errorOnAutoCreate()) 2847 throw new Error("Attempt to auto-create Device.manufactureDate"); 2848 else if (Configuration.doAutoCreate()) 2849 this.manufactureDate = new DateTimeType(); // bb 2850 return this.manufactureDate; 2851 } 2852 2853 public boolean hasManufactureDateElement() { 2854 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 2855 } 2856 2857 public boolean hasManufactureDate() { 2858 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 2859 } 2860 2861 /** 2862 * @param value {@link #manufactureDate} (The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value 2863 */ 2864 public Device setManufactureDateElement(DateTimeType value) { 2865 this.manufactureDate = value; 2866 return this; 2867 } 2868 2869 /** 2870 * @return The date and time when the device was manufactured. 2871 */ 2872 public Date getManufactureDate() { 2873 return this.manufactureDate == null ? null : this.manufactureDate.getValue(); 2874 } 2875 2876 /** 2877 * @param value The date and time when the device was manufactured. 2878 */ 2879 public Device setManufactureDate(Date value) { 2880 if (value == null) 2881 this.manufactureDate = null; 2882 else { 2883 if (this.manufactureDate == null) 2884 this.manufactureDate = new DateTimeType(); 2885 this.manufactureDate.setValue(value); 2886 } 2887 return this; 2888 } 2889 2890 /** 2891 * @return {@link #expirationDate} (The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2892 */ 2893 public DateTimeType getExpirationDateElement() { 2894 if (this.expirationDate == null) 2895 if (Configuration.errorOnAutoCreate()) 2896 throw new Error("Attempt to auto-create Device.expirationDate"); 2897 else if (Configuration.doAutoCreate()) 2898 this.expirationDate = new DateTimeType(); // bb 2899 return this.expirationDate; 2900 } 2901 2902 public boolean hasExpirationDateElement() { 2903 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2904 } 2905 2906 public boolean hasExpirationDate() { 2907 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2908 } 2909 2910 /** 2911 * @param value {@link #expirationDate} (The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2912 */ 2913 public Device setExpirationDateElement(DateTimeType value) { 2914 this.expirationDate = value; 2915 return this; 2916 } 2917 2918 /** 2919 * @return The date and time beyond which this device is no longer valid or should not be used (if applicable). 2920 */ 2921 public Date getExpirationDate() { 2922 return this.expirationDate == null ? null : this.expirationDate.getValue(); 2923 } 2924 2925 /** 2926 * @param value The date and time beyond which this device is no longer valid or should not be used (if applicable). 2927 */ 2928 public Device setExpirationDate(Date value) { 2929 if (value == null) 2930 this.expirationDate = null; 2931 else { 2932 if (this.expirationDate == null) 2933 this.expirationDate = new DateTimeType(); 2934 this.expirationDate.setValue(value); 2935 } 2936 return this; 2937 } 2938 2939 /** 2940 * @return {@link #lotNumber} (Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2941 */ 2942 public StringType getLotNumberElement() { 2943 if (this.lotNumber == null) 2944 if (Configuration.errorOnAutoCreate()) 2945 throw new Error("Attempt to auto-create Device.lotNumber"); 2946 else if (Configuration.doAutoCreate()) 2947 this.lotNumber = new StringType(); // bb 2948 return this.lotNumber; 2949 } 2950 2951 public boolean hasLotNumberElement() { 2952 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2953 } 2954 2955 public boolean hasLotNumber() { 2956 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2957 } 2958 2959 /** 2960 * @param value {@link #lotNumber} (Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2961 */ 2962 public Device setLotNumberElement(StringType value) { 2963 this.lotNumber = value; 2964 return this; 2965 } 2966 2967 /** 2968 * @return Lot number assigned by the manufacturer. 2969 */ 2970 public String getLotNumber() { 2971 return this.lotNumber == null ? null : this.lotNumber.getValue(); 2972 } 2973 2974 /** 2975 * @param value Lot number assigned by the manufacturer. 2976 */ 2977 public Device setLotNumber(String value) { 2978 if (Utilities.noString(value)) 2979 this.lotNumber = null; 2980 else { 2981 if (this.lotNumber == null) 2982 this.lotNumber = new StringType(); 2983 this.lotNumber.setValue(value); 2984 } 2985 return this; 2986 } 2987 2988 /** 2989 * @return {@link #serialNumber} (The serial number assigned by the organization when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getSerialNumber" gives direct access to the value 2990 */ 2991 public StringType getSerialNumberElement() { 2992 if (this.serialNumber == null) 2993 if (Configuration.errorOnAutoCreate()) 2994 throw new Error("Attempt to auto-create Device.serialNumber"); 2995 else if (Configuration.doAutoCreate()) 2996 this.serialNumber = new StringType(); // bb 2997 return this.serialNumber; 2998 } 2999 3000 public boolean hasSerialNumberElement() { 3001 return this.serialNumber != null && !this.serialNumber.isEmpty(); 3002 } 3003 3004 public boolean hasSerialNumber() { 3005 return this.serialNumber != null && !this.serialNumber.isEmpty(); 3006 } 3007 3008 /** 3009 * @param value {@link #serialNumber} (The serial number assigned by the organization when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getSerialNumber" gives direct access to the value 3010 */ 3011 public Device setSerialNumberElement(StringType value) { 3012 this.serialNumber = value; 3013 return this; 3014 } 3015 3016 /** 3017 * @return The serial number assigned by the organization when the device was manufactured. 3018 */ 3019 public String getSerialNumber() { 3020 return this.serialNumber == null ? null : this.serialNumber.getValue(); 3021 } 3022 3023 /** 3024 * @param value The serial number assigned by the organization when the device was manufactured. 3025 */ 3026 public Device setSerialNumber(String value) { 3027 if (Utilities.noString(value)) 3028 this.serialNumber = null; 3029 else { 3030 if (this.serialNumber == null) 3031 this.serialNumber = new StringType(); 3032 this.serialNumber.setValue(value); 3033 } 3034 return this; 3035 } 3036 3037 /** 3038 * @return {@link #name} (This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.) 3039 */ 3040 public List<DeviceNameComponent> getName() { 3041 if (this.name == null) 3042 this.name = new ArrayList<DeviceNameComponent>(); 3043 return this.name; 3044 } 3045 3046 /** 3047 * @return Returns a reference to <code>this</code> for easy method chaining 3048 */ 3049 public Device setName(List<DeviceNameComponent> theName) { 3050 this.name = theName; 3051 return this; 3052 } 3053 3054 public boolean hasName() { 3055 if (this.name == null) 3056 return false; 3057 for (DeviceNameComponent item : this.name) 3058 if (!item.isEmpty()) 3059 return true; 3060 return false; 3061 } 3062 3063 public DeviceNameComponent addName() { //3 3064 DeviceNameComponent t = new DeviceNameComponent(); 3065 if (this.name == null) 3066 this.name = new ArrayList<DeviceNameComponent>(); 3067 this.name.add(t); 3068 return t; 3069 } 3070 3071 public Device addName(DeviceNameComponent t) { //3 3072 if (t == null) 3073 return this; 3074 if (this.name == null) 3075 this.name = new ArrayList<DeviceNameComponent>(); 3076 this.name.add(t); 3077 return this; 3078 } 3079 3080 /** 3081 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 3082 */ 3083 public DeviceNameComponent getNameFirstRep() { 3084 if (getName().isEmpty()) { 3085 addName(); 3086 } 3087 return getName().get(0); 3088 } 3089 3090 /** 3091 * @return {@link #modelNumber} (The manufacturer's model number for the device.). This is the underlying object with id, value and extensions. The accessor "getModelNumber" gives direct access to the value 3092 */ 3093 public StringType getModelNumberElement() { 3094 if (this.modelNumber == null) 3095 if (Configuration.errorOnAutoCreate()) 3096 throw new Error("Attempt to auto-create Device.modelNumber"); 3097 else if (Configuration.doAutoCreate()) 3098 this.modelNumber = new StringType(); // bb 3099 return this.modelNumber; 3100 } 3101 3102 public boolean hasModelNumberElement() { 3103 return this.modelNumber != null && !this.modelNumber.isEmpty(); 3104 } 3105 3106 public boolean hasModelNumber() { 3107 return this.modelNumber != null && !this.modelNumber.isEmpty(); 3108 } 3109 3110 /** 3111 * @param value {@link #modelNumber} (The manufacturer's model number for the device.). This is the underlying object with id, value and extensions. The accessor "getModelNumber" gives direct access to the value 3112 */ 3113 public Device setModelNumberElement(StringType value) { 3114 this.modelNumber = value; 3115 return this; 3116 } 3117 3118 /** 3119 * @return The manufacturer's model number for the device. 3120 */ 3121 public String getModelNumber() { 3122 return this.modelNumber == null ? null : this.modelNumber.getValue(); 3123 } 3124 3125 /** 3126 * @param value The manufacturer's model number for the device. 3127 */ 3128 public Device setModelNumber(String value) { 3129 if (Utilities.noString(value)) 3130 this.modelNumber = null; 3131 else { 3132 if (this.modelNumber == null) 3133 this.modelNumber = new StringType(); 3134 this.modelNumber.setValue(value); 3135 } 3136 return this; 3137 } 3138 3139 /** 3140 * @return {@link #partNumber} (The part number or catalog number of the device.). This is the underlying object with id, value and extensions. The accessor "getPartNumber" gives direct access to the value 3141 */ 3142 public StringType getPartNumberElement() { 3143 if (this.partNumber == null) 3144 if (Configuration.errorOnAutoCreate()) 3145 throw new Error("Attempt to auto-create Device.partNumber"); 3146 else if (Configuration.doAutoCreate()) 3147 this.partNumber = new StringType(); // bb 3148 return this.partNumber; 3149 } 3150 3151 public boolean hasPartNumberElement() { 3152 return this.partNumber != null && !this.partNumber.isEmpty(); 3153 } 3154 3155 public boolean hasPartNumber() { 3156 return this.partNumber != null && !this.partNumber.isEmpty(); 3157 } 3158 3159 /** 3160 * @param value {@link #partNumber} (The part number or catalog number of the device.). This is the underlying object with id, value and extensions. The accessor "getPartNumber" gives direct access to the value 3161 */ 3162 public Device setPartNumberElement(StringType value) { 3163 this.partNumber = value; 3164 return this; 3165 } 3166 3167 /** 3168 * @return The part number or catalog number of the device. 3169 */ 3170 public String getPartNumber() { 3171 return this.partNumber == null ? null : this.partNumber.getValue(); 3172 } 3173 3174 /** 3175 * @param value The part number or catalog number of the device. 3176 */ 3177 public Device setPartNumber(String value) { 3178 if (Utilities.noString(value)) 3179 this.partNumber = null; 3180 else { 3181 if (this.partNumber == null) 3182 this.partNumber = new StringType(); 3183 this.partNumber.setValue(value); 3184 } 3185 return this; 3186 } 3187 3188 /** 3189 * @return {@link #category} (Devices may be associated with one or more categories.) 3190 */ 3191 public List<CodeableConcept> getCategory() { 3192 if (this.category == null) 3193 this.category = new ArrayList<CodeableConcept>(); 3194 return this.category; 3195 } 3196 3197 /** 3198 * @return Returns a reference to <code>this</code> for easy method chaining 3199 */ 3200 public Device setCategory(List<CodeableConcept> theCategory) { 3201 this.category = theCategory; 3202 return this; 3203 } 3204 3205 public boolean hasCategory() { 3206 if (this.category == null) 3207 return false; 3208 for (CodeableConcept item : this.category) 3209 if (!item.isEmpty()) 3210 return true; 3211 return false; 3212 } 3213 3214 public CodeableConcept addCategory() { //3 3215 CodeableConcept t = new CodeableConcept(); 3216 if (this.category == null) 3217 this.category = new ArrayList<CodeableConcept>(); 3218 this.category.add(t); 3219 return t; 3220 } 3221 3222 public Device addCategory(CodeableConcept t) { //3 3223 if (t == null) 3224 return this; 3225 if (this.category == null) 3226 this.category = new ArrayList<CodeableConcept>(); 3227 this.category.add(t); 3228 return this; 3229 } 3230 3231 /** 3232 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 3233 */ 3234 public CodeableConcept getCategoryFirstRep() { 3235 if (getCategory().isEmpty()) { 3236 addCategory(); 3237 } 3238 return getCategory().get(0); 3239 } 3240 3241 /** 3242 * @return {@link #type} (The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device.) 3243 */ 3244 public List<CodeableConcept> getType() { 3245 if (this.type == null) 3246 this.type = new ArrayList<CodeableConcept>(); 3247 return this.type; 3248 } 3249 3250 /** 3251 * @return Returns a reference to <code>this</code> for easy method chaining 3252 */ 3253 public Device setType(List<CodeableConcept> theType) { 3254 this.type = theType; 3255 return this; 3256 } 3257 3258 public boolean hasType() { 3259 if (this.type == null) 3260 return false; 3261 for (CodeableConcept item : this.type) 3262 if (!item.isEmpty()) 3263 return true; 3264 return false; 3265 } 3266 3267 public CodeableConcept addType() { //3 3268 CodeableConcept t = new CodeableConcept(); 3269 if (this.type == null) 3270 this.type = new ArrayList<CodeableConcept>(); 3271 this.type.add(t); 3272 return t; 3273 } 3274 3275 public Device addType(CodeableConcept t) { //3 3276 if (t == null) 3277 return this; 3278 if (this.type == null) 3279 this.type = new ArrayList<CodeableConcept>(); 3280 this.type.add(t); 3281 return this; 3282 } 3283 3284 /** 3285 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 3286 */ 3287 public CodeableConcept getTypeFirstRep() { 3288 if (getType().isEmpty()) { 3289 addType(); 3290 } 3291 return getType().get(0); 3292 } 3293 3294 /** 3295 * @return {@link #version} (The actual design of the device or software version running on the device.) 3296 */ 3297 public List<DeviceVersionComponent> getVersion() { 3298 if (this.version == null) 3299 this.version = new ArrayList<DeviceVersionComponent>(); 3300 return this.version; 3301 } 3302 3303 /** 3304 * @return Returns a reference to <code>this</code> for easy method chaining 3305 */ 3306 public Device setVersion(List<DeviceVersionComponent> theVersion) { 3307 this.version = theVersion; 3308 return this; 3309 } 3310 3311 public boolean hasVersion() { 3312 if (this.version == null) 3313 return false; 3314 for (DeviceVersionComponent item : this.version) 3315 if (!item.isEmpty()) 3316 return true; 3317 return false; 3318 } 3319 3320 public DeviceVersionComponent addVersion() { //3 3321 DeviceVersionComponent t = new DeviceVersionComponent(); 3322 if (this.version == null) 3323 this.version = new ArrayList<DeviceVersionComponent>(); 3324 this.version.add(t); 3325 return t; 3326 } 3327 3328 public Device addVersion(DeviceVersionComponent t) { //3 3329 if (t == null) 3330 return this; 3331 if (this.version == null) 3332 this.version = new ArrayList<DeviceVersionComponent>(); 3333 this.version.add(t); 3334 return this; 3335 } 3336 3337 /** 3338 * @return The first repetition of repeating field {@link #version}, creating it if it does not already exist {3} 3339 */ 3340 public DeviceVersionComponent getVersionFirstRep() { 3341 if (getVersion().isEmpty()) { 3342 addVersion(); 3343 } 3344 return getVersion().get(0); 3345 } 3346 3347 /** 3348 * @return {@link #conformsTo} (Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.) 3349 */ 3350 public List<DeviceConformsToComponent> getConformsTo() { 3351 if (this.conformsTo == null) 3352 this.conformsTo = new ArrayList<DeviceConformsToComponent>(); 3353 return this.conformsTo; 3354 } 3355 3356 /** 3357 * @return Returns a reference to <code>this</code> for easy method chaining 3358 */ 3359 public Device setConformsTo(List<DeviceConformsToComponent> theConformsTo) { 3360 this.conformsTo = theConformsTo; 3361 return this; 3362 } 3363 3364 public boolean hasConformsTo() { 3365 if (this.conformsTo == null) 3366 return false; 3367 for (DeviceConformsToComponent item : this.conformsTo) 3368 if (!item.isEmpty()) 3369 return true; 3370 return false; 3371 } 3372 3373 public DeviceConformsToComponent addConformsTo() { //3 3374 DeviceConformsToComponent t = new DeviceConformsToComponent(); 3375 if (this.conformsTo == null) 3376 this.conformsTo = new ArrayList<DeviceConformsToComponent>(); 3377 this.conformsTo.add(t); 3378 return t; 3379 } 3380 3381 public Device addConformsTo(DeviceConformsToComponent t) { //3 3382 if (t == null) 3383 return this; 3384 if (this.conformsTo == null) 3385 this.conformsTo = new ArrayList<DeviceConformsToComponent>(); 3386 this.conformsTo.add(t); 3387 return this; 3388 } 3389 3390 /** 3391 * @return The first repetition of repeating field {@link #conformsTo}, creating it if it does not already exist {3} 3392 */ 3393 public DeviceConformsToComponent getConformsToFirstRep() { 3394 if (getConformsTo().isEmpty()) { 3395 addConformsTo(); 3396 } 3397 return getConformsTo().get(0); 3398 } 3399 3400 /** 3401 * @return {@link #property} (Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes.) 3402 */ 3403 public List<DevicePropertyComponent> getProperty() { 3404 if (this.property == null) 3405 this.property = new ArrayList<DevicePropertyComponent>(); 3406 return this.property; 3407 } 3408 3409 /** 3410 * @return Returns a reference to <code>this</code> for easy method chaining 3411 */ 3412 public Device setProperty(List<DevicePropertyComponent> theProperty) { 3413 this.property = theProperty; 3414 return this; 3415 } 3416 3417 public boolean hasProperty() { 3418 if (this.property == null) 3419 return false; 3420 for (DevicePropertyComponent item : this.property) 3421 if (!item.isEmpty()) 3422 return true; 3423 return false; 3424 } 3425 3426 public DevicePropertyComponent addProperty() { //3 3427 DevicePropertyComponent t = new DevicePropertyComponent(); 3428 if (this.property == null) 3429 this.property = new ArrayList<DevicePropertyComponent>(); 3430 this.property.add(t); 3431 return t; 3432 } 3433 3434 public Device addProperty(DevicePropertyComponent t) { //3 3435 if (t == null) 3436 return this; 3437 if (this.property == null) 3438 this.property = new ArrayList<DevicePropertyComponent>(); 3439 this.property.add(t); 3440 return this; 3441 } 3442 3443 /** 3444 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 3445 */ 3446 public DevicePropertyComponent getPropertyFirstRep() { 3447 if (getProperty().isEmpty()) { 3448 addProperty(); 3449 } 3450 return getProperty().get(0); 3451 } 3452 3453 /** 3454 * @return {@link #mode} (The designated condition for performing a task with the device.) 3455 */ 3456 public CodeableConcept getMode() { 3457 if (this.mode == null) 3458 if (Configuration.errorOnAutoCreate()) 3459 throw new Error("Attempt to auto-create Device.mode"); 3460 else if (Configuration.doAutoCreate()) 3461 this.mode = new CodeableConcept(); // cc 3462 return this.mode; 3463 } 3464 3465 public boolean hasMode() { 3466 return this.mode != null && !this.mode.isEmpty(); 3467 } 3468 3469 /** 3470 * @param value {@link #mode} (The designated condition for performing a task with the device.) 3471 */ 3472 public Device setMode(CodeableConcept value) { 3473 this.mode = value; 3474 return this; 3475 } 3476 3477 /** 3478 * @return {@link #cycle} (The series of occurrences that repeats during the operation of the device.) 3479 */ 3480 public Count getCycle() { 3481 if (this.cycle == null) 3482 if (Configuration.errorOnAutoCreate()) 3483 throw new Error("Attempt to auto-create Device.cycle"); 3484 else if (Configuration.doAutoCreate()) 3485 this.cycle = new Count(); // cc 3486 return this.cycle; 3487 } 3488 3489 public boolean hasCycle() { 3490 return this.cycle != null && !this.cycle.isEmpty(); 3491 } 3492 3493 /** 3494 * @param value {@link #cycle} (The series of occurrences that repeats during the operation of the device.) 3495 */ 3496 public Device setCycle(Count value) { 3497 this.cycle = value; 3498 return this; 3499 } 3500 3501 /** 3502 * @return {@link #duration} (A measurement of time during the device's operation (e.g., days, hours, mins, etc.).) 3503 */ 3504 public Duration getDuration() { 3505 if (this.duration == null) 3506 if (Configuration.errorOnAutoCreate()) 3507 throw new Error("Attempt to auto-create Device.duration"); 3508 else if (Configuration.doAutoCreate()) 3509 this.duration = new Duration(); // cc 3510 return this.duration; 3511 } 3512 3513 public boolean hasDuration() { 3514 return this.duration != null && !this.duration.isEmpty(); 3515 } 3516 3517 /** 3518 * @param value {@link #duration} (A measurement of time during the device's operation (e.g., days, hours, mins, etc.).) 3519 */ 3520 public Device setDuration(Duration value) { 3521 this.duration = value; 3522 return this; 3523 } 3524 3525 /** 3526 * @return {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 3527 */ 3528 public Reference getOwner() { 3529 if (this.owner == null) 3530 if (Configuration.errorOnAutoCreate()) 3531 throw new Error("Attempt to auto-create Device.owner"); 3532 else if (Configuration.doAutoCreate()) 3533 this.owner = new Reference(); // cc 3534 return this.owner; 3535 } 3536 3537 public boolean hasOwner() { 3538 return this.owner != null && !this.owner.isEmpty(); 3539 } 3540 3541 /** 3542 * @param value {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 3543 */ 3544 public Device setOwner(Reference value) { 3545 this.owner = value; 3546 return this; 3547 } 3548 3549 /** 3550 * @return {@link #contact} (Contact details for an organization or a particular human that is responsible for the device.) 3551 */ 3552 public List<ContactPoint> getContact() { 3553 if (this.contact == null) 3554 this.contact = new ArrayList<ContactPoint>(); 3555 return this.contact; 3556 } 3557 3558 /** 3559 * @return Returns a reference to <code>this</code> for easy method chaining 3560 */ 3561 public Device setContact(List<ContactPoint> theContact) { 3562 this.contact = theContact; 3563 return this; 3564 } 3565 3566 public boolean hasContact() { 3567 if (this.contact == null) 3568 return false; 3569 for (ContactPoint item : this.contact) 3570 if (!item.isEmpty()) 3571 return true; 3572 return false; 3573 } 3574 3575 public ContactPoint addContact() { //3 3576 ContactPoint t = new ContactPoint(); 3577 if (this.contact == null) 3578 this.contact = new ArrayList<ContactPoint>(); 3579 this.contact.add(t); 3580 return t; 3581 } 3582 3583 public Device addContact(ContactPoint t) { //3 3584 if (t == null) 3585 return this; 3586 if (this.contact == null) 3587 this.contact = new ArrayList<ContactPoint>(); 3588 this.contact.add(t); 3589 return this; 3590 } 3591 3592 /** 3593 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3594 */ 3595 public ContactPoint getContactFirstRep() { 3596 if (getContact().isEmpty()) { 3597 addContact(); 3598 } 3599 return getContact().get(0); 3600 } 3601 3602 /** 3603 * @return {@link #location} (The place where the device can be found.) 3604 */ 3605 public Reference getLocation() { 3606 if (this.location == null) 3607 if (Configuration.errorOnAutoCreate()) 3608 throw new Error("Attempt to auto-create Device.location"); 3609 else if (Configuration.doAutoCreate()) 3610 this.location = new Reference(); // cc 3611 return this.location; 3612 } 3613 3614 public boolean hasLocation() { 3615 return this.location != null && !this.location.isEmpty(); 3616 } 3617 3618 /** 3619 * @param value {@link #location} (The place where the device can be found.) 3620 */ 3621 public Device setLocation(Reference value) { 3622 this.location = value; 3623 return this; 3624 } 3625 3626 /** 3627 * @return {@link #url} (A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3628 */ 3629 public UriType getUrlElement() { 3630 if (this.url == null) 3631 if (Configuration.errorOnAutoCreate()) 3632 throw new Error("Attempt to auto-create Device.url"); 3633 else if (Configuration.doAutoCreate()) 3634 this.url = new UriType(); // bb 3635 return this.url; 3636 } 3637 3638 public boolean hasUrlElement() { 3639 return this.url != null && !this.url.isEmpty(); 3640 } 3641 3642 public boolean hasUrl() { 3643 return this.url != null && !this.url.isEmpty(); 3644 } 3645 3646 /** 3647 * @param value {@link #url} (A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3648 */ 3649 public Device setUrlElement(UriType value) { 3650 this.url = value; 3651 return this; 3652 } 3653 3654 /** 3655 * @return A network address on which the device may be contacted directly. 3656 */ 3657 public String getUrl() { 3658 return this.url == null ? null : this.url.getValue(); 3659 } 3660 3661 /** 3662 * @param value A network address on which the device may be contacted directly. 3663 */ 3664 public Device setUrl(String value) { 3665 if (Utilities.noString(value)) 3666 this.url = null; 3667 else { 3668 if (this.url == null) 3669 this.url = new UriType(); 3670 this.url.setValue(value); 3671 } 3672 return this; 3673 } 3674 3675 /** 3676 * @return {@link #endpoint} (Technical endpoints providing access to services provided by the device defined at this resource.) 3677 */ 3678 public List<Reference> getEndpoint() { 3679 if (this.endpoint == null) 3680 this.endpoint = new ArrayList<Reference>(); 3681 return this.endpoint; 3682 } 3683 3684 /** 3685 * @return Returns a reference to <code>this</code> for easy method chaining 3686 */ 3687 public Device setEndpoint(List<Reference> theEndpoint) { 3688 this.endpoint = theEndpoint; 3689 return this; 3690 } 3691 3692 public boolean hasEndpoint() { 3693 if (this.endpoint == null) 3694 return false; 3695 for (Reference item : this.endpoint) 3696 if (!item.isEmpty()) 3697 return true; 3698 return false; 3699 } 3700 3701 public Reference addEndpoint() { //3 3702 Reference t = new Reference(); 3703 if (this.endpoint == null) 3704 this.endpoint = new ArrayList<Reference>(); 3705 this.endpoint.add(t); 3706 return t; 3707 } 3708 3709 public Device addEndpoint(Reference t) { //3 3710 if (t == null) 3711 return this; 3712 if (this.endpoint == null) 3713 this.endpoint = new ArrayList<Reference>(); 3714 this.endpoint.add(t); 3715 return this; 3716 } 3717 3718 /** 3719 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 3720 */ 3721 public Reference getEndpointFirstRep() { 3722 if (getEndpoint().isEmpty()) { 3723 addEndpoint(); 3724 } 3725 return getEndpoint().get(0); 3726 } 3727 3728 /** 3729 * @return {@link #gateway} (The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data).) 3730 */ 3731 public List<CodeableReference> getGateway() { 3732 if (this.gateway == null) 3733 this.gateway = new ArrayList<CodeableReference>(); 3734 return this.gateway; 3735 } 3736 3737 /** 3738 * @return Returns a reference to <code>this</code> for easy method chaining 3739 */ 3740 public Device setGateway(List<CodeableReference> theGateway) { 3741 this.gateway = theGateway; 3742 return this; 3743 } 3744 3745 public boolean hasGateway() { 3746 if (this.gateway == null) 3747 return false; 3748 for (CodeableReference item : this.gateway) 3749 if (!item.isEmpty()) 3750 return true; 3751 return false; 3752 } 3753 3754 public CodeableReference addGateway() { //3 3755 CodeableReference t = new CodeableReference(); 3756 if (this.gateway == null) 3757 this.gateway = new ArrayList<CodeableReference>(); 3758 this.gateway.add(t); 3759 return t; 3760 } 3761 3762 public Device addGateway(CodeableReference t) { //3 3763 if (t == null) 3764 return this; 3765 if (this.gateway == null) 3766 this.gateway = new ArrayList<CodeableReference>(); 3767 this.gateway.add(t); 3768 return this; 3769 } 3770 3771 /** 3772 * @return The first repetition of repeating field {@link #gateway}, creating it if it does not already exist {3} 3773 */ 3774 public CodeableReference getGatewayFirstRep() { 3775 if (getGateway().isEmpty()) { 3776 addGateway(); 3777 } 3778 return getGateway().get(0); 3779 } 3780 3781 /** 3782 * @return {@link #note} (Descriptive information, usage information or implantation information that is not captured in an existing element.) 3783 */ 3784 public List<Annotation> getNote() { 3785 if (this.note == null) 3786 this.note = new ArrayList<Annotation>(); 3787 return this.note; 3788 } 3789 3790 /** 3791 * @return Returns a reference to <code>this</code> for easy method chaining 3792 */ 3793 public Device setNote(List<Annotation> theNote) { 3794 this.note = theNote; 3795 return this; 3796 } 3797 3798 public boolean hasNote() { 3799 if (this.note == null) 3800 return false; 3801 for (Annotation item : this.note) 3802 if (!item.isEmpty()) 3803 return true; 3804 return false; 3805 } 3806 3807 public Annotation addNote() { //3 3808 Annotation t = new Annotation(); 3809 if (this.note == null) 3810 this.note = new ArrayList<Annotation>(); 3811 this.note.add(t); 3812 return t; 3813 } 3814 3815 public Device addNote(Annotation t) { //3 3816 if (t == null) 3817 return this; 3818 if (this.note == null) 3819 this.note = new ArrayList<Annotation>(); 3820 this.note.add(t); 3821 return this; 3822 } 3823 3824 /** 3825 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3826 */ 3827 public Annotation getNoteFirstRep() { 3828 if (getNote().isEmpty()) { 3829 addNote(); 3830 } 3831 return getNote().get(0); 3832 } 3833 3834 /** 3835 * @return {@link #safety} (Provides additional safety characteristics about a medical device. For example devices containing latex.) 3836 */ 3837 public List<CodeableConcept> getSafety() { 3838 if (this.safety == null) 3839 this.safety = new ArrayList<CodeableConcept>(); 3840 return this.safety; 3841 } 3842 3843 /** 3844 * @return Returns a reference to <code>this</code> for easy method chaining 3845 */ 3846 public Device setSafety(List<CodeableConcept> theSafety) { 3847 this.safety = theSafety; 3848 return this; 3849 } 3850 3851 public boolean hasSafety() { 3852 if (this.safety == null) 3853 return false; 3854 for (CodeableConcept item : this.safety) 3855 if (!item.isEmpty()) 3856 return true; 3857 return false; 3858 } 3859 3860 public CodeableConcept addSafety() { //3 3861 CodeableConcept t = new CodeableConcept(); 3862 if (this.safety == null) 3863 this.safety = new ArrayList<CodeableConcept>(); 3864 this.safety.add(t); 3865 return t; 3866 } 3867 3868 public Device addSafety(CodeableConcept t) { //3 3869 if (t == null) 3870 return this; 3871 if (this.safety == null) 3872 this.safety = new ArrayList<CodeableConcept>(); 3873 this.safety.add(t); 3874 return this; 3875 } 3876 3877 /** 3878 * @return The first repetition of repeating field {@link #safety}, creating it if it does not already exist {3} 3879 */ 3880 public CodeableConcept getSafetyFirstRep() { 3881 if (getSafety().isEmpty()) { 3882 addSafety(); 3883 } 3884 return getSafety().get(0); 3885 } 3886 3887 /** 3888 * @return {@link #parent} (The higher level or encompassing device that this device is a logical part of.) 3889 */ 3890 public Reference getParent() { 3891 if (this.parent == null) 3892 if (Configuration.errorOnAutoCreate()) 3893 throw new Error("Attempt to auto-create Device.parent"); 3894 else if (Configuration.doAutoCreate()) 3895 this.parent = new Reference(); // cc 3896 return this.parent; 3897 } 3898 3899 public boolean hasParent() { 3900 return this.parent != null && !this.parent.isEmpty(); 3901 } 3902 3903 /** 3904 * @param value {@link #parent} (The higher level or encompassing device that this device is a logical part of.) 3905 */ 3906 public Device setParent(Reference value) { 3907 this.parent = value; 3908 return this; 3909 } 3910 3911 protected void listChildren(List<Property> children) { 3912 super.listChildren(children); 3913 children.add(new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3914 children.add(new Property("displayName", "string", "The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.", 0, 1, displayName)); 3915 children.add(new Property("definition", "CodeableReference(DeviceDefinition)", "The reference to the definition for the device.", 0, 1, definition)); 3916 children.add(new Property("udiCarrier", "", "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 0, java.lang.Integer.MAX_VALUE, udiCarrier)); 3917 children.add(new Property("status", "code", "The Device record status. This is not the status of the device like availability.", 0, 1, status)); 3918 children.add(new Property("availabilityStatus", "CodeableConcept", "The availability of the device.", 0, 1, availabilityStatus)); 3919 children.add(new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent)); 3920 children.add(new Property("manufacturer", "string", "A name of the manufacturer or entity legally responsible for the device.", 0, 1, manufacturer)); 3921 children.add(new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 0, 1, manufactureDate)); 3922 children.add(new Property("expirationDate", "dateTime", "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, expirationDate)); 3923 children.add(new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, lotNumber)); 3924 children.add(new Property("serialNumber", "string", "The serial number assigned by the organization when the device was manufactured.", 0, 1, serialNumber)); 3925 children.add(new Property("name", "", "This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.", 0, java.lang.Integer.MAX_VALUE, name)); 3926 children.add(new Property("modelNumber", "string", "The manufacturer's model number for the device.", 0, 1, modelNumber)); 3927 children.add(new Property("partNumber", "string", "The part number or catalog number of the device.", 0, 1, partNumber)); 3928 children.add(new Property("category", "CodeableConcept", "Devices may be associated with one or more categories.", 0, java.lang.Integer.MAX_VALUE, category)); 3929 children.add(new Property("type", "CodeableConcept", "The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device.", 0, java.lang.Integer.MAX_VALUE, type)); 3930 children.add(new Property("version", "", "The actual design of the device or software version running on the device.", 0, java.lang.Integer.MAX_VALUE, version)); 3931 children.add(new Property("conformsTo", "", "Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.", 0, java.lang.Integer.MAX_VALUE, conformsTo)); 3932 children.add(new Property("property", "", "Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes.", 0, java.lang.Integer.MAX_VALUE, property)); 3933 children.add(new Property("mode", "CodeableConcept", "The designated condition for performing a task with the device.", 0, 1, mode)); 3934 children.add(new Property("cycle", "Count", "The series of occurrences that repeats during the operation of the device.", 0, 1, cycle)); 3935 children.add(new Property("duration", "Duration", "A measurement of time during the device's operation (e.g., days, hours, mins, etc.).", 0, 1, duration)); 3936 children.add(new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner)); 3937 children.add(new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact)); 3938 children.add(new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1, location)); 3939 children.add(new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url)); 3940 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services provided by the device defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 3941 children.add(new Property("gateway", "CodeableReference(Device)", "The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data).", 0, java.lang.Integer.MAX_VALUE, gateway)); 3942 children.add(new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note)); 3943 children.add(new Property("safety", "CodeableConcept", "Provides additional safety characteristics about a medical device. For example devices containing latex.", 0, java.lang.Integer.MAX_VALUE, safety)); 3944 children.add(new Property("parent", "Reference(Device)", "The higher level or encompassing device that this device is a logical part of.", 0, 1, parent)); 3945 } 3946 3947 @Override 3948 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3949 switch (_hash) { 3950 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, java.lang.Integer.MAX_VALUE, identifier); 3951 case 1714148973: /*displayName*/ return new Property("displayName", "string", "The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.", 0, 1, displayName); 3952 case -1014418093: /*definition*/ return new Property("definition", "CodeableReference(DeviceDefinition)", "The reference to the definition for the device.", 0, 1, definition); 3953 case -1343558178: /*udiCarrier*/ return new Property("udiCarrier", "", "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 0, java.lang.Integer.MAX_VALUE, udiCarrier); 3954 case -892481550: /*status*/ return new Property("status", "code", "The Device record status. This is not the status of the device like availability.", 0, 1, status); 3955 case 804659501: /*availabilityStatus*/ return new Property("availabilityStatus", "CodeableConcept", "The availability of the device.", 0, 1, availabilityStatus); 3956 case -654468482: /*biologicalSourceEvent*/ return new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent); 3957 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "string", "A name of the manufacturer or entity legally responsible for the device.", 0, 1, manufacturer); 3958 case 416714767: /*manufactureDate*/ return new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 0, 1, manufactureDate); 3959 case -668811523: /*expirationDate*/ return new Property("expirationDate", "dateTime", "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, expirationDate); 3960 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, lotNumber); 3961 case 83787357: /*serialNumber*/ return new Property("serialNumber", "string", "The serial number assigned by the organization when the device was manufactured.", 0, 1, serialNumber); 3962 case 3373707: /*name*/ return new Property("name", "", "This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.", 0, java.lang.Integer.MAX_VALUE, name); 3963 case 346619858: /*modelNumber*/ return new Property("modelNumber", "string", "The manufacturer's model number for the device.", 0, 1, modelNumber); 3964 case -731502308: /*partNumber*/ return new Property("partNumber", "string", "The part number or catalog number of the device.", 0, 1, partNumber); 3965 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Devices may be associated with one or more categories.", 0, java.lang.Integer.MAX_VALUE, category); 3966 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device.", 0, java.lang.Integer.MAX_VALUE, type); 3967 case 351608024: /*version*/ return new Property("version", "", "The actual design of the device or software version running on the device.", 0, java.lang.Integer.MAX_VALUE, version); 3968 case 1014198088: /*conformsTo*/ return new Property("conformsTo", "", "Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.", 0, java.lang.Integer.MAX_VALUE, conformsTo); 3969 case -993141291: /*property*/ return new Property("property", "", "Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes.", 0, java.lang.Integer.MAX_VALUE, property); 3970 case 3357091: /*mode*/ return new Property("mode", "CodeableConcept", "The designated condition for performing a task with the device.", 0, 1, mode); 3971 case 95131878: /*cycle*/ return new Property("cycle", "Count", "The series of occurrences that repeats during the operation of the device.", 0, 1, cycle); 3972 case -1992012396: /*duration*/ return new Property("duration", "Duration", "A measurement of time during the device's operation (e.g., days, hours, mins, etc.).", 0, 1, duration); 3973 case 106164915: /*owner*/ return new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner); 3974 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact); 3975 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1, location); 3976 case 116079: /*url*/ return new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url); 3977 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services provided by the device defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint); 3978 case -189118908: /*gateway*/ return new Property("gateway", "CodeableReference(Device)", "The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data).", 0, java.lang.Integer.MAX_VALUE, gateway); 3979 case 3387378: /*note*/ return new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note); 3980 case -909893934: /*safety*/ return new Property("safety", "CodeableConcept", "Provides additional safety characteristics about a medical device. For example devices containing latex.", 0, java.lang.Integer.MAX_VALUE, safety); 3981 case -995424086: /*parent*/ return new Property("parent", "Reference(Device)", "The higher level or encompassing device that this device is a logical part of.", 0, 1, parent); 3982 default: return super.getNamedProperty(_hash, _name, _checkValid); 3983 } 3984 3985 } 3986 3987 @Override 3988 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3989 switch (hash) { 3990 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3991 case 1714148973: /*displayName*/ return this.displayName == null ? new Base[0] : new Base[] {this.displayName}; // StringType 3992 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CodeableReference 3993 case -1343558178: /*udiCarrier*/ return this.udiCarrier == null ? new Base[0] : this.udiCarrier.toArray(new Base[this.udiCarrier.size()]); // DeviceUdiCarrierComponent 3994 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FHIRDeviceStatus> 3995 case 804659501: /*availabilityStatus*/ return this.availabilityStatus == null ? new Base[0] : new Base[] {this.availabilityStatus}; // CodeableConcept 3996 case -654468482: /*biologicalSourceEvent*/ return this.biologicalSourceEvent == null ? new Base[0] : new Base[] {this.biologicalSourceEvent}; // Identifier 3997 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // StringType 3998 case 416714767: /*manufactureDate*/ return this.manufactureDate == null ? new Base[0] : new Base[] {this.manufactureDate}; // DateTimeType 3999 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateTimeType 4000 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 4001 case 83787357: /*serialNumber*/ return this.serialNumber == null ? new Base[0] : new Base[] {this.serialNumber}; // StringType 4002 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // DeviceNameComponent 4003 case 346619858: /*modelNumber*/ return this.modelNumber == null ? new Base[0] : new Base[] {this.modelNumber}; // StringType 4004 case -731502308: /*partNumber*/ return this.partNumber == null ? new Base[0] : new Base[] {this.partNumber}; // StringType 4005 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 4006 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 4007 case 351608024: /*version*/ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // DeviceVersionComponent 4008 case 1014198088: /*conformsTo*/ return this.conformsTo == null ? new Base[0] : this.conformsTo.toArray(new Base[this.conformsTo.size()]); // DeviceConformsToComponent 4009 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // DevicePropertyComponent 4010 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // CodeableConcept 4011 case 95131878: /*cycle*/ return this.cycle == null ? new Base[0] : new Base[] {this.cycle}; // Count 4012 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // Duration 4013 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 4014 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 4015 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 4016 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4017 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 4018 case -189118908: /*gateway*/ return this.gateway == null ? new Base[0] : this.gateway.toArray(new Base[this.gateway.size()]); // CodeableReference 4019 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4020 case -909893934: /*safety*/ return this.safety == null ? new Base[0] : this.safety.toArray(new Base[this.safety.size()]); // CodeableConcept 4021 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // Reference 4022 default: return super.getProperty(hash, name, checkValid); 4023 } 4024 4025 } 4026 4027 @Override 4028 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4029 switch (hash) { 4030 case -1618432855: // identifier 4031 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4032 return value; 4033 case 1714148973: // displayName 4034 this.displayName = TypeConvertor.castToString(value); // StringType 4035 return value; 4036 case -1014418093: // definition 4037 this.definition = TypeConvertor.castToCodeableReference(value); // CodeableReference 4038 return value; 4039 case -1343558178: // udiCarrier 4040 this.getUdiCarrier().add((DeviceUdiCarrierComponent) value); // DeviceUdiCarrierComponent 4041 return value; 4042 case -892481550: // status 4043 value = new FHIRDeviceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4044 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4045 return value; 4046 case 804659501: // availabilityStatus 4047 this.availabilityStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4048 return value; 4049 case -654468482: // biologicalSourceEvent 4050 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 4051 return value; 4052 case -1969347631: // manufacturer 4053 this.manufacturer = TypeConvertor.castToString(value); // StringType 4054 return value; 4055 case 416714767: // manufactureDate 4056 this.manufactureDate = TypeConvertor.castToDateTime(value); // DateTimeType 4057 return value; 4058 case -668811523: // expirationDate 4059 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 4060 return value; 4061 case 462547450: // lotNumber 4062 this.lotNumber = TypeConvertor.castToString(value); // StringType 4063 return value; 4064 case 83787357: // serialNumber 4065 this.serialNumber = TypeConvertor.castToString(value); // StringType 4066 return value; 4067 case 3373707: // name 4068 this.getName().add((DeviceNameComponent) value); // DeviceNameComponent 4069 return value; 4070 case 346619858: // modelNumber 4071 this.modelNumber = TypeConvertor.castToString(value); // StringType 4072 return value; 4073 case -731502308: // partNumber 4074 this.partNumber = TypeConvertor.castToString(value); // StringType 4075 return value; 4076 case 50511102: // category 4077 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4078 return value; 4079 case 3575610: // type 4080 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4081 return value; 4082 case 351608024: // version 4083 this.getVersion().add((DeviceVersionComponent) value); // DeviceVersionComponent 4084 return value; 4085 case 1014198088: // conformsTo 4086 this.getConformsTo().add((DeviceConformsToComponent) value); // DeviceConformsToComponent 4087 return value; 4088 case -993141291: // property 4089 this.getProperty().add((DevicePropertyComponent) value); // DevicePropertyComponent 4090 return value; 4091 case 3357091: // mode 4092 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4093 return value; 4094 case 95131878: // cycle 4095 this.cycle = TypeConvertor.castToCount(value); // Count 4096 return value; 4097 case -1992012396: // duration 4098 this.duration = TypeConvertor.castToDuration(value); // Duration 4099 return value; 4100 case 106164915: // owner 4101 this.owner = TypeConvertor.castToReference(value); // Reference 4102 return value; 4103 case 951526432: // contact 4104 this.getContact().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 4105 return value; 4106 case 1901043637: // location 4107 this.location = TypeConvertor.castToReference(value); // Reference 4108 return value; 4109 case 116079: // url 4110 this.url = TypeConvertor.castToUri(value); // UriType 4111 return value; 4112 case 1741102485: // endpoint 4113 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 4114 return value; 4115 case -189118908: // gateway 4116 this.getGateway().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4117 return value; 4118 case 3387378: // note 4119 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4120 return value; 4121 case -909893934: // safety 4122 this.getSafety().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4123 return value; 4124 case -995424086: // parent 4125 this.parent = TypeConvertor.castToReference(value); // Reference 4126 return value; 4127 default: return super.setProperty(hash, name, value); 4128 } 4129 4130 } 4131 4132 @Override 4133 public Base setProperty(String name, Base value) throws FHIRException { 4134 if (name.equals("identifier")) { 4135 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4136 } else if (name.equals("displayName")) { 4137 this.displayName = TypeConvertor.castToString(value); // StringType 4138 } else if (name.equals("definition")) { 4139 this.definition = TypeConvertor.castToCodeableReference(value); // CodeableReference 4140 } else if (name.equals("udiCarrier")) { 4141 this.getUdiCarrier().add((DeviceUdiCarrierComponent) value); 4142 } else if (name.equals("status")) { 4143 value = new FHIRDeviceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4144 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4145 } else if (name.equals("availabilityStatus")) { 4146 this.availabilityStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4147 } else if (name.equals("biologicalSourceEvent")) { 4148 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 4149 } else if (name.equals("manufacturer")) { 4150 this.manufacturer = TypeConvertor.castToString(value); // StringType 4151 } else if (name.equals("manufactureDate")) { 4152 this.manufactureDate = TypeConvertor.castToDateTime(value); // DateTimeType 4153 } else if (name.equals("expirationDate")) { 4154 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 4155 } else if (name.equals("lotNumber")) { 4156 this.lotNumber = TypeConvertor.castToString(value); // StringType 4157 } else if (name.equals("serialNumber")) { 4158 this.serialNumber = TypeConvertor.castToString(value); // StringType 4159 } else if (name.equals("name")) { 4160 this.getName().add((DeviceNameComponent) value); 4161 } else if (name.equals("modelNumber")) { 4162 this.modelNumber = TypeConvertor.castToString(value); // StringType 4163 } else if (name.equals("partNumber")) { 4164 this.partNumber = TypeConvertor.castToString(value); // StringType 4165 } else if (name.equals("category")) { 4166 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 4167 } else if (name.equals("type")) { 4168 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 4169 } else if (name.equals("version")) { 4170 this.getVersion().add((DeviceVersionComponent) value); 4171 } else if (name.equals("conformsTo")) { 4172 this.getConformsTo().add((DeviceConformsToComponent) value); 4173 } else if (name.equals("property")) { 4174 this.getProperty().add((DevicePropertyComponent) value); 4175 } else if (name.equals("mode")) { 4176 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4177 } else if (name.equals("cycle")) { 4178 this.cycle = TypeConvertor.castToCount(value); // Count 4179 } else if (name.equals("duration")) { 4180 this.duration = TypeConvertor.castToDuration(value); // Duration 4181 } else if (name.equals("owner")) { 4182 this.owner = TypeConvertor.castToReference(value); // Reference 4183 } else if (name.equals("contact")) { 4184 this.getContact().add(TypeConvertor.castToContactPoint(value)); 4185 } else if (name.equals("location")) { 4186 this.location = TypeConvertor.castToReference(value); // Reference 4187 } else if (name.equals("url")) { 4188 this.url = TypeConvertor.castToUri(value); // UriType 4189 } else if (name.equals("endpoint")) { 4190 this.getEndpoint().add(TypeConvertor.castToReference(value)); 4191 } else if (name.equals("gateway")) { 4192 this.getGateway().add(TypeConvertor.castToCodeableReference(value)); 4193 } else if (name.equals("note")) { 4194 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4195 } else if (name.equals("safety")) { 4196 this.getSafety().add(TypeConvertor.castToCodeableConcept(value)); 4197 } else if (name.equals("parent")) { 4198 this.parent = TypeConvertor.castToReference(value); // Reference 4199 } else 4200 return super.setProperty(name, value); 4201 return value; 4202 } 4203 4204 @Override 4205 public void removeChild(String name, Base value) throws FHIRException { 4206 if (name.equals("identifier")) { 4207 this.getIdentifier().remove(value); 4208 } else if (name.equals("displayName")) { 4209 this.displayName = null; 4210 } else if (name.equals("definition")) { 4211 this.definition = null; 4212 } else if (name.equals("udiCarrier")) { 4213 this.getUdiCarrier().remove((DeviceUdiCarrierComponent) value); 4214 } else if (name.equals("status")) { 4215 value = new FHIRDeviceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4216 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4217 } else if (name.equals("availabilityStatus")) { 4218 this.availabilityStatus = null; 4219 } else if (name.equals("biologicalSourceEvent")) { 4220 this.biologicalSourceEvent = null; 4221 } else if (name.equals("manufacturer")) { 4222 this.manufacturer = null; 4223 } else if (name.equals("manufactureDate")) { 4224 this.manufactureDate = null; 4225 } else if (name.equals("expirationDate")) { 4226 this.expirationDate = null; 4227 } else if (name.equals("lotNumber")) { 4228 this.lotNumber = null; 4229 } else if (name.equals("serialNumber")) { 4230 this.serialNumber = null; 4231 } else if (name.equals("name")) { 4232 this.getName().remove((DeviceNameComponent) value); 4233 } else if (name.equals("modelNumber")) { 4234 this.modelNumber = null; 4235 } else if (name.equals("partNumber")) { 4236 this.partNumber = null; 4237 } else if (name.equals("category")) { 4238 this.getCategory().remove(value); 4239 } else if (name.equals("type")) { 4240 this.getType().remove(value); 4241 } else if (name.equals("version")) { 4242 this.getVersion().remove((DeviceVersionComponent) value); 4243 } else if (name.equals("conformsTo")) { 4244 this.getConformsTo().remove((DeviceConformsToComponent) value); 4245 } else if (name.equals("property")) { 4246 this.getProperty().remove((DevicePropertyComponent) value); 4247 } else if (name.equals("mode")) { 4248 this.mode = null; 4249 } else if (name.equals("cycle")) { 4250 this.cycle = null; 4251 } else if (name.equals("duration")) { 4252 this.duration = null; 4253 } else if (name.equals("owner")) { 4254 this.owner = null; 4255 } else if (name.equals("contact")) { 4256 this.getContact().remove(value); 4257 } else if (name.equals("location")) { 4258 this.location = null; 4259 } else if (name.equals("url")) { 4260 this.url = null; 4261 } else if (name.equals("endpoint")) { 4262 this.getEndpoint().remove(value); 4263 } else if (name.equals("gateway")) { 4264 this.getGateway().remove(value); 4265 } else if (name.equals("note")) { 4266 this.getNote().remove(value); 4267 } else if (name.equals("safety")) { 4268 this.getSafety().remove(value); 4269 } else if (name.equals("parent")) { 4270 this.parent = null; 4271 } else 4272 super.removeChild(name, value); 4273 4274 } 4275 4276 @Override 4277 public Base makeProperty(int hash, String name) throws FHIRException { 4278 switch (hash) { 4279 case -1618432855: return addIdentifier(); 4280 case 1714148973: return getDisplayNameElement(); 4281 case -1014418093: return getDefinition(); 4282 case -1343558178: return addUdiCarrier(); 4283 case -892481550: return getStatusElement(); 4284 case 804659501: return getAvailabilityStatus(); 4285 case -654468482: return getBiologicalSourceEvent(); 4286 case -1969347631: return getManufacturerElement(); 4287 case 416714767: return getManufactureDateElement(); 4288 case -668811523: return getExpirationDateElement(); 4289 case 462547450: return getLotNumberElement(); 4290 case 83787357: return getSerialNumberElement(); 4291 case 3373707: return addName(); 4292 case 346619858: return getModelNumberElement(); 4293 case -731502308: return getPartNumberElement(); 4294 case 50511102: return addCategory(); 4295 case 3575610: return addType(); 4296 case 351608024: return addVersion(); 4297 case 1014198088: return addConformsTo(); 4298 case -993141291: return addProperty(); 4299 case 3357091: return getMode(); 4300 case 95131878: return getCycle(); 4301 case -1992012396: return getDuration(); 4302 case 106164915: return getOwner(); 4303 case 951526432: return addContact(); 4304 case 1901043637: return getLocation(); 4305 case 116079: return getUrlElement(); 4306 case 1741102485: return addEndpoint(); 4307 case -189118908: return addGateway(); 4308 case 3387378: return addNote(); 4309 case -909893934: return addSafety(); 4310 case -995424086: return getParent(); 4311 default: return super.makeProperty(hash, name); 4312 } 4313 4314 } 4315 4316 @Override 4317 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4318 switch (hash) { 4319 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4320 case 1714148973: /*displayName*/ return new String[] {"string"}; 4321 case -1014418093: /*definition*/ return new String[] {"CodeableReference"}; 4322 case -1343558178: /*udiCarrier*/ return new String[] {}; 4323 case -892481550: /*status*/ return new String[] {"code"}; 4324 case 804659501: /*availabilityStatus*/ return new String[] {"CodeableConcept"}; 4325 case -654468482: /*biologicalSourceEvent*/ return new String[] {"Identifier"}; 4326 case -1969347631: /*manufacturer*/ return new String[] {"string"}; 4327 case 416714767: /*manufactureDate*/ return new String[] {"dateTime"}; 4328 case -668811523: /*expirationDate*/ return new String[] {"dateTime"}; 4329 case 462547450: /*lotNumber*/ return new String[] {"string"}; 4330 case 83787357: /*serialNumber*/ return new String[] {"string"}; 4331 case 3373707: /*name*/ return new String[] {}; 4332 case 346619858: /*modelNumber*/ return new String[] {"string"}; 4333 case -731502308: /*partNumber*/ return new String[] {"string"}; 4334 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4335 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4336 case 351608024: /*version*/ return new String[] {}; 4337 case 1014198088: /*conformsTo*/ return new String[] {}; 4338 case -993141291: /*property*/ return new String[] {}; 4339 case 3357091: /*mode*/ return new String[] {"CodeableConcept"}; 4340 case 95131878: /*cycle*/ return new String[] {"Count"}; 4341 case -1992012396: /*duration*/ return new String[] {"Duration"}; 4342 case 106164915: /*owner*/ return new String[] {"Reference"}; 4343 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 4344 case 1901043637: /*location*/ return new String[] {"Reference"}; 4345 case 116079: /*url*/ return new String[] {"uri"}; 4346 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 4347 case -189118908: /*gateway*/ return new String[] {"CodeableReference"}; 4348 case 3387378: /*note*/ return new String[] {"Annotation"}; 4349 case -909893934: /*safety*/ return new String[] {"CodeableConcept"}; 4350 case -995424086: /*parent*/ return new String[] {"Reference"}; 4351 default: return super.getTypesForProperty(hash, name); 4352 } 4353 4354 } 4355 4356 @Override 4357 public Base addChild(String name) throws FHIRException { 4358 if (name.equals("identifier")) { 4359 return addIdentifier(); 4360 } 4361 else if (name.equals("displayName")) { 4362 throw new FHIRException("Cannot call addChild on a singleton property Device.displayName"); 4363 } 4364 else if (name.equals("definition")) { 4365 this.definition = new CodeableReference(); 4366 return this.definition; 4367 } 4368 else if (name.equals("udiCarrier")) { 4369 return addUdiCarrier(); 4370 } 4371 else if (name.equals("status")) { 4372 throw new FHIRException("Cannot call addChild on a singleton property Device.status"); 4373 } 4374 else if (name.equals("availabilityStatus")) { 4375 this.availabilityStatus = new CodeableConcept(); 4376 return this.availabilityStatus; 4377 } 4378 else if (name.equals("biologicalSourceEvent")) { 4379 this.biologicalSourceEvent = new Identifier(); 4380 return this.biologicalSourceEvent; 4381 } 4382 else if (name.equals("manufacturer")) { 4383 throw new FHIRException("Cannot call addChild on a singleton property Device.manufacturer"); 4384 } 4385 else if (name.equals("manufactureDate")) { 4386 throw new FHIRException("Cannot call addChild on a singleton property Device.manufactureDate"); 4387 } 4388 else if (name.equals("expirationDate")) { 4389 throw new FHIRException("Cannot call addChild on a singleton property Device.expirationDate"); 4390 } 4391 else if (name.equals("lotNumber")) { 4392 throw new FHIRException("Cannot call addChild on a singleton property Device.lotNumber"); 4393 } 4394 else if (name.equals("serialNumber")) { 4395 throw new FHIRException("Cannot call addChild on a singleton property Device.serialNumber"); 4396 } 4397 else if (name.equals("name")) { 4398 return addName(); 4399 } 4400 else if (name.equals("modelNumber")) { 4401 throw new FHIRException("Cannot call addChild on a singleton property Device.modelNumber"); 4402 } 4403 else if (name.equals("partNumber")) { 4404 throw new FHIRException("Cannot call addChild on a singleton property Device.partNumber"); 4405 } 4406 else if (name.equals("category")) { 4407 return addCategory(); 4408 } 4409 else if (name.equals("type")) { 4410 return addType(); 4411 } 4412 else if (name.equals("version")) { 4413 return addVersion(); 4414 } 4415 else if (name.equals("conformsTo")) { 4416 return addConformsTo(); 4417 } 4418 else if (name.equals("property")) { 4419 return addProperty(); 4420 } 4421 else if (name.equals("mode")) { 4422 this.mode = new CodeableConcept(); 4423 return this.mode; 4424 } 4425 else if (name.equals("cycle")) { 4426 this.cycle = new Count(); 4427 return this.cycle; 4428 } 4429 else if (name.equals("duration")) { 4430 this.duration = new Duration(); 4431 return this.duration; 4432 } 4433 else if (name.equals("owner")) { 4434 this.owner = new Reference(); 4435 return this.owner; 4436 } 4437 else if (name.equals("contact")) { 4438 return addContact(); 4439 } 4440 else if (name.equals("location")) { 4441 this.location = new Reference(); 4442 return this.location; 4443 } 4444 else if (name.equals("url")) { 4445 throw new FHIRException("Cannot call addChild on a singleton property Device.url"); 4446 } 4447 else if (name.equals("endpoint")) { 4448 return addEndpoint(); 4449 } 4450 else if (name.equals("gateway")) { 4451 return addGateway(); 4452 } 4453 else if (name.equals("note")) { 4454 return addNote(); 4455 } 4456 else if (name.equals("safety")) { 4457 return addSafety(); 4458 } 4459 else if (name.equals("parent")) { 4460 this.parent = new Reference(); 4461 return this.parent; 4462 } 4463 else 4464 return super.addChild(name); 4465 } 4466 4467 public String fhirType() { 4468 return "Device"; 4469 4470 } 4471 4472 public Device copy() { 4473 Device dst = new Device(); 4474 copyValues(dst); 4475 return dst; 4476 } 4477 4478 public void copyValues(Device dst) { 4479 super.copyValues(dst); 4480 if (identifier != null) { 4481 dst.identifier = new ArrayList<Identifier>(); 4482 for (Identifier i : identifier) 4483 dst.identifier.add(i.copy()); 4484 }; 4485 dst.displayName = displayName == null ? null : displayName.copy(); 4486 dst.definition = definition == null ? null : definition.copy(); 4487 if (udiCarrier != null) { 4488 dst.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 4489 for (DeviceUdiCarrierComponent i : udiCarrier) 4490 dst.udiCarrier.add(i.copy()); 4491 }; 4492 dst.status = status == null ? null : status.copy(); 4493 dst.availabilityStatus = availabilityStatus == null ? null : availabilityStatus.copy(); 4494 dst.biologicalSourceEvent = biologicalSourceEvent == null ? null : biologicalSourceEvent.copy(); 4495 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 4496 dst.manufactureDate = manufactureDate == null ? null : manufactureDate.copy(); 4497 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 4498 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 4499 dst.serialNumber = serialNumber == null ? null : serialNumber.copy(); 4500 if (name != null) { 4501 dst.name = new ArrayList<DeviceNameComponent>(); 4502 for (DeviceNameComponent i : name) 4503 dst.name.add(i.copy()); 4504 }; 4505 dst.modelNumber = modelNumber == null ? null : modelNumber.copy(); 4506 dst.partNumber = partNumber == null ? null : partNumber.copy(); 4507 if (category != null) { 4508 dst.category = new ArrayList<CodeableConcept>(); 4509 for (CodeableConcept i : category) 4510 dst.category.add(i.copy()); 4511 }; 4512 if (type != null) { 4513 dst.type = new ArrayList<CodeableConcept>(); 4514 for (CodeableConcept i : type) 4515 dst.type.add(i.copy()); 4516 }; 4517 if (version != null) { 4518 dst.version = new ArrayList<DeviceVersionComponent>(); 4519 for (DeviceVersionComponent i : version) 4520 dst.version.add(i.copy()); 4521 }; 4522 if (conformsTo != null) { 4523 dst.conformsTo = new ArrayList<DeviceConformsToComponent>(); 4524 for (DeviceConformsToComponent i : conformsTo) 4525 dst.conformsTo.add(i.copy()); 4526 }; 4527 if (property != null) { 4528 dst.property = new ArrayList<DevicePropertyComponent>(); 4529 for (DevicePropertyComponent i : property) 4530 dst.property.add(i.copy()); 4531 }; 4532 dst.mode = mode == null ? null : mode.copy(); 4533 dst.cycle = cycle == null ? null : cycle.copy(); 4534 dst.duration = duration == null ? null : duration.copy(); 4535 dst.owner = owner == null ? null : owner.copy(); 4536 if (contact != null) { 4537 dst.contact = new ArrayList<ContactPoint>(); 4538 for (ContactPoint i : contact) 4539 dst.contact.add(i.copy()); 4540 }; 4541 dst.location = location == null ? null : location.copy(); 4542 dst.url = url == null ? null : url.copy(); 4543 if (endpoint != null) { 4544 dst.endpoint = new ArrayList<Reference>(); 4545 for (Reference i : endpoint) 4546 dst.endpoint.add(i.copy()); 4547 }; 4548 if (gateway != null) { 4549 dst.gateway = new ArrayList<CodeableReference>(); 4550 for (CodeableReference i : gateway) 4551 dst.gateway.add(i.copy()); 4552 }; 4553 if (note != null) { 4554 dst.note = new ArrayList<Annotation>(); 4555 for (Annotation i : note) 4556 dst.note.add(i.copy()); 4557 }; 4558 if (safety != null) { 4559 dst.safety = new ArrayList<CodeableConcept>(); 4560 for (CodeableConcept i : safety) 4561 dst.safety.add(i.copy()); 4562 }; 4563 dst.parent = parent == null ? null : parent.copy(); 4564 } 4565 4566 protected Device typedCopy() { 4567 return copy(); 4568 } 4569 4570 @Override 4571 public boolean equalsDeep(Base other_) { 4572 if (!super.equalsDeep(other_)) 4573 return false; 4574 if (!(other_ instanceof Device)) 4575 return false; 4576 Device o = (Device) other_; 4577 return compareDeep(identifier, o.identifier, true) && compareDeep(displayName, o.displayName, true) 4578 && compareDeep(definition, o.definition, true) && compareDeep(udiCarrier, o.udiCarrier, true) && compareDeep(status, o.status, true) 4579 && compareDeep(availabilityStatus, o.availabilityStatus, true) && compareDeep(biologicalSourceEvent, o.biologicalSourceEvent, true) 4580 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(manufactureDate, o.manufactureDate, true) 4581 && compareDeep(expirationDate, o.expirationDate, true) && compareDeep(lotNumber, o.lotNumber, true) 4582 && compareDeep(serialNumber, o.serialNumber, true) && compareDeep(name, o.name, true) && compareDeep(modelNumber, o.modelNumber, true) 4583 && compareDeep(partNumber, o.partNumber, true) && compareDeep(category, o.category, true) && compareDeep(type, o.type, true) 4584 && compareDeep(version, o.version, true) && compareDeep(conformsTo, o.conformsTo, true) && compareDeep(property, o.property, true) 4585 && compareDeep(mode, o.mode, true) && compareDeep(cycle, o.cycle, true) && compareDeep(duration, o.duration, true) 4586 && compareDeep(owner, o.owner, true) && compareDeep(contact, o.contact, true) && compareDeep(location, o.location, true) 4587 && compareDeep(url, o.url, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(gateway, o.gateway, true) 4588 && compareDeep(note, o.note, true) && compareDeep(safety, o.safety, true) && compareDeep(parent, o.parent, true) 4589 ; 4590 } 4591 4592 @Override 4593 public boolean equalsShallow(Base other_) { 4594 if (!super.equalsShallow(other_)) 4595 return false; 4596 if (!(other_ instanceof Device)) 4597 return false; 4598 Device o = (Device) other_; 4599 return compareValues(displayName, o.displayName, true) && compareValues(status, o.status, true) && compareValues(manufacturer, o.manufacturer, true) 4600 && compareValues(manufactureDate, o.manufactureDate, true) && compareValues(expirationDate, o.expirationDate, true) 4601 && compareValues(lotNumber, o.lotNumber, true) && compareValues(serialNumber, o.serialNumber, true) 4602 && compareValues(modelNumber, o.modelNumber, true) && compareValues(partNumber, o.partNumber, true) 4603 && compareValues(url, o.url, true); 4604 } 4605 4606 public boolean isEmpty() { 4607 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, displayName, definition 4608 , udiCarrier, status, availabilityStatus, biologicalSourceEvent, manufacturer, manufactureDate 4609 , expirationDate, lotNumber, serialNumber, name, modelNumber, partNumber, category 4610 , type, version, conformsTo, property, mode, cycle, duration, owner, contact 4611 , location, url, endpoint, gateway, note, safety, parent); 4612 } 4613 4614 @Override 4615 public ResourceType getResourceType() { 4616 return ResourceType.Device; 4617 } 4618 4619 /** 4620 * Search parameter: <b>biological-source-event</b> 4621 * <p> 4622 * Description: <b>The biological source for the device</b><br> 4623 * Type: <b>token</b><br> 4624 * Path: <b>Device.biologicalSourceEvent</b><br> 4625 * </p> 4626 */ 4627 @SearchParamDefinition(name="biological-source-event", path="Device.biologicalSourceEvent", description="The biological source for the device", type="token" ) 4628 public static final String SP_BIOLOGICAL_SOURCE_EVENT = "biological-source-event"; 4629 /** 4630 * <b>Fluent Client</b> search parameter constant for <b>biological-source-event</b> 4631 * <p> 4632 * Description: <b>The biological source for the device</b><br> 4633 * Type: <b>token</b><br> 4634 * Path: <b>Device.biologicalSourceEvent</b><br> 4635 * </p> 4636 */ 4637 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BIOLOGICAL_SOURCE_EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BIOLOGICAL_SOURCE_EVENT); 4638 4639 /** 4640 * Search parameter: <b>code-value-concept</b> 4641 * <p> 4642 * Description: <b>Code and value parameter pair</b><br> 4643 * Type: <b>composite</b><br> 4644 * Path: <b>Device</b><br> 4645 * </p> 4646 */ 4647 @SearchParamDefinition(name="code-value-concept", path="Device", description="Code and value parameter pair", type="composite", compositeOf={"specification", "version"} ) 4648 public static final String SP_CODE_VALUE_CONCEPT = "code-value-concept"; 4649 /** 4650 * <b>Fluent Client</b> search parameter constant for <b>code-value-concept</b> 4651 * <p> 4652 * Description: <b>Code and value parameter pair</b><br> 4653 * Type: <b>composite</b><br> 4654 * Path: <b>Device</b><br> 4655 * </p> 4656 */ 4657 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam> CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam>(SP_CODE_VALUE_CONCEPT); 4658 4659 /** 4660 * Search parameter: <b>code</b> 4661 * <p> 4662 * Description: <b>The definition / type of the device (code)</b><br> 4663 * Type: <b>token</b><br> 4664 * Path: <b>Device.definition.concept</b><br> 4665 * </p> 4666 */ 4667 @SearchParamDefinition(name="code", path="Device.definition.concept", description="The definition / type of the device (code)", type="token" ) 4668 public static final String SP_CODE = "code"; 4669 /** 4670 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4671 * <p> 4672 * Description: <b>The definition / type of the device (code)</b><br> 4673 * Type: <b>token</b><br> 4674 * Path: <b>Device.definition.concept</b><br> 4675 * </p> 4676 */ 4677 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4678 4679 /** 4680 * Search parameter: <b>definition</b> 4681 * <p> 4682 * Description: <b>The definition / type of the device</b><br> 4683 * Type: <b>reference</b><br> 4684 * Path: <b>Device.definition.reference</b><br> 4685 * </p> 4686 */ 4687 @SearchParamDefinition(name="definition", path="Device.definition.reference", description="The definition / type of the device", type="reference", target={DeviceDefinition.class } ) 4688 public static final String SP_DEFINITION = "definition"; 4689 /** 4690 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 4691 * <p> 4692 * Description: <b>The definition / type of the device</b><br> 4693 * Type: <b>reference</b><br> 4694 * Path: <b>Device.definition.reference</b><br> 4695 * </p> 4696 */ 4697 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 4698 4699/** 4700 * Constant for fluent queries to be used to add include statements. Specifies 4701 * the path value of "<b>Device:definition</b>". 4702 */ 4703 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("Device:definition").toLocked(); 4704 4705 /** 4706 * Search parameter: <b>device-name</b> 4707 * <p> 4708 * Description: <b>A server defined search that may match any of the string fields in Device.name or Device.type.</b><br> 4709 * Type: <b>string</b><br> 4710 * Path: <b>Device.name.value | Device.type.coding.display | Device.type.text</b><br> 4711 * </p> 4712 */ 4713 @SearchParamDefinition(name="device-name", path="Device.name.value | Device.type.coding.display | Device.type.text", description="A server defined search that may match any of the string fields in Device.name or Device.type.", type="string" ) 4714 public static final String SP_DEVICE_NAME = "device-name"; 4715 /** 4716 * <b>Fluent Client</b> search parameter constant for <b>device-name</b> 4717 * <p> 4718 * Description: <b>A server defined search that may match any of the string fields in Device.name or Device.type.</b><br> 4719 * Type: <b>string</b><br> 4720 * Path: <b>Device.name.value | Device.type.coding.display | Device.type.text</b><br> 4721 * </p> 4722 */ 4723 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEVICE_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DEVICE_NAME); 4724 4725 /** 4726 * Search parameter: <b>expiration-date</b> 4727 * <p> 4728 * Description: <b>The expiration date of the device</b><br> 4729 * Type: <b>date</b><br> 4730 * Path: <b>Device.expirationDate</b><br> 4731 * </p> 4732 */ 4733 @SearchParamDefinition(name="expiration-date", path="Device.expirationDate", description="The expiration date of the device", type="date" ) 4734 public static final String SP_EXPIRATION_DATE = "expiration-date"; 4735 /** 4736 * <b>Fluent Client</b> search parameter constant for <b>expiration-date</b> 4737 * <p> 4738 * Description: <b>The expiration date of the device</b><br> 4739 * Type: <b>date</b><br> 4740 * Path: <b>Device.expirationDate</b><br> 4741 * </p> 4742 */ 4743 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRATION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EXPIRATION_DATE); 4744 4745 /** 4746 * Search parameter: <b>identifier</b> 4747 * <p> 4748 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 4749 * Type: <b>token</b><br> 4750 * Path: <b>Device.identifier</b><br> 4751 * </p> 4752 */ 4753 @SearchParamDefinition(name="identifier", path="Device.identifier", description="Instance id from manufacturer, owner, and others", type="token" ) 4754 public static final String SP_IDENTIFIER = "identifier"; 4755 /** 4756 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4757 * <p> 4758 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 4759 * Type: <b>token</b><br> 4760 * Path: <b>Device.identifier</b><br> 4761 * </p> 4762 */ 4763 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4764 4765 /** 4766 * Search parameter: <b>location</b> 4767 * <p> 4768 * Description: <b>A location, where the resource is found</b><br> 4769 * Type: <b>reference</b><br> 4770 * Path: <b>Device.location</b><br> 4771 * </p> 4772 */ 4773 @SearchParamDefinition(name="location", path="Device.location", description="A location, where the resource is found", type="reference", target={Location.class } ) 4774 public static final String SP_LOCATION = "location"; 4775 /** 4776 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4777 * <p> 4778 * Description: <b>A location, where the resource is found</b><br> 4779 * Type: <b>reference</b><br> 4780 * Path: <b>Device.location</b><br> 4781 * </p> 4782 */ 4783 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 4784 4785/** 4786 * Constant for fluent queries to be used to add include statements. Specifies 4787 * the path value of "<b>Device:location</b>". 4788 */ 4789 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Device:location").toLocked(); 4790 4791 /** 4792 * Search parameter: <b>lot-number</b> 4793 * <p> 4794 * Description: <b>The lot number of the device</b><br> 4795 * Type: <b>string</b><br> 4796 * Path: <b>Device.lotNumber</b><br> 4797 * </p> 4798 */ 4799 @SearchParamDefinition(name="lot-number", path="Device.lotNumber", description="The lot number of the device", type="string" ) 4800 public static final String SP_LOT_NUMBER = "lot-number"; 4801 /** 4802 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 4803 * <p> 4804 * Description: <b>The lot number of the device</b><br> 4805 * Type: <b>string</b><br> 4806 * Path: <b>Device.lotNumber</b><br> 4807 * </p> 4808 */ 4809 public static final ca.uhn.fhir.rest.gclient.StringClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_LOT_NUMBER); 4810 4811 /** 4812 * Search parameter: <b>manufacture-date</b> 4813 * <p> 4814 * Description: <b>The manufacture date of the device</b><br> 4815 * Type: <b>date</b><br> 4816 * Path: <b>Device.manufactureDate</b><br> 4817 * </p> 4818 */ 4819 @SearchParamDefinition(name="manufacture-date", path="Device.manufactureDate", description="The manufacture date of the device", type="date" ) 4820 public static final String SP_MANUFACTURE_DATE = "manufacture-date"; 4821 /** 4822 * <b>Fluent Client</b> search parameter constant for <b>manufacture-date</b> 4823 * <p> 4824 * Description: <b>The manufacture date of the device</b><br> 4825 * Type: <b>date</b><br> 4826 * Path: <b>Device.manufactureDate</b><br> 4827 * </p> 4828 */ 4829 public static final ca.uhn.fhir.rest.gclient.DateClientParam MANUFACTURE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_MANUFACTURE_DATE); 4830 4831 /** 4832 * Search parameter: <b>manufacturer</b> 4833 * <p> 4834 * Description: <b>The manufacturer of the device</b><br> 4835 * Type: <b>string</b><br> 4836 * Path: <b>Device.manufacturer</b><br> 4837 * </p> 4838 */ 4839 @SearchParamDefinition(name="manufacturer", path="Device.manufacturer", description="The manufacturer of the device", type="string" ) 4840 public static final String SP_MANUFACTURER = "manufacturer"; 4841 /** 4842 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 4843 * <p> 4844 * Description: <b>The manufacturer of the device</b><br> 4845 * Type: <b>string</b><br> 4846 * Path: <b>Device.manufacturer</b><br> 4847 * </p> 4848 */ 4849 public static final ca.uhn.fhir.rest.gclient.StringClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_MANUFACTURER); 4850 4851 /** 4852 * Search parameter: <b>model</b> 4853 * <p> 4854 * Description: <b>The model of the device</b><br> 4855 * Type: <b>string</b><br> 4856 * Path: <b>Device.modelNumber</b><br> 4857 * </p> 4858 */ 4859 @SearchParamDefinition(name="model", path="Device.modelNumber", description="The model of the device", type="string" ) 4860 public static final String SP_MODEL = "model"; 4861 /** 4862 * <b>Fluent Client</b> search parameter constant for <b>model</b> 4863 * <p> 4864 * Description: <b>The model of the device</b><br> 4865 * Type: <b>string</b><br> 4866 * Path: <b>Device.modelNumber</b><br> 4867 * </p> 4868 */ 4869 public static final ca.uhn.fhir.rest.gclient.StringClientParam MODEL = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_MODEL); 4870 4871 /** 4872 * Search parameter: <b>organization</b> 4873 * <p> 4874 * Description: <b>The organization responsible for the device</b><br> 4875 * Type: <b>reference</b><br> 4876 * Path: <b>Device.owner</b><br> 4877 * </p> 4878 */ 4879 @SearchParamDefinition(name="organization", path="Device.owner", description="The organization responsible for the device", type="reference", target={Organization.class } ) 4880 public static final String SP_ORGANIZATION = "organization"; 4881 /** 4882 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 4883 * <p> 4884 * Description: <b>The organization responsible for the device</b><br> 4885 * Type: <b>reference</b><br> 4886 * Path: <b>Device.owner</b><br> 4887 * </p> 4888 */ 4889 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 4890 4891/** 4892 * Constant for fluent queries to be used to add include statements. Specifies 4893 * the path value of "<b>Device:organization</b>". 4894 */ 4895 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Device:organization").toLocked(); 4896 4897 /** 4898 * Search parameter: <b>parent</b> 4899 * <p> 4900 * Description: <b>The parent device</b><br> 4901 * Type: <b>reference</b><br> 4902 * Path: <b>Device.parent</b><br> 4903 * </p> 4904 */ 4905 @SearchParamDefinition(name="parent", path="Device.parent", description="The parent device", type="reference", target={Device.class } ) 4906 public static final String SP_PARENT = "parent"; 4907 /** 4908 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 4909 * <p> 4910 * Description: <b>The parent device</b><br> 4911 * Type: <b>reference</b><br> 4912 * Path: <b>Device.parent</b><br> 4913 * </p> 4914 */ 4915 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 4916 4917/** 4918 * Constant for fluent queries to be used to add include statements. Specifies 4919 * the path value of "<b>Device:parent</b>". 4920 */ 4921 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("Device:parent").toLocked(); 4922 4923 /** 4924 * Search parameter: <b>serial-number</b> 4925 * <p> 4926 * Description: <b>The serial number of the device</b><br> 4927 * Type: <b>string</b><br> 4928 * Path: <b>Device.serialNumber | Device.identifier.where(type='SNO')</b><br> 4929 * </p> 4930 */ 4931 @SearchParamDefinition(name="serial-number", path="Device.serialNumber | Device.identifier.where(type='SNO')", description="The serial number of the device", type="string" ) 4932 public static final String SP_SERIAL_NUMBER = "serial-number"; 4933 /** 4934 * <b>Fluent Client</b> search parameter constant for <b>serial-number</b> 4935 * <p> 4936 * Description: <b>The serial number of the device</b><br> 4937 * Type: <b>string</b><br> 4938 * Path: <b>Device.serialNumber | Device.identifier.where(type='SNO')</b><br> 4939 * </p> 4940 */ 4941 public static final ca.uhn.fhir.rest.gclient.StringClientParam SERIAL_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SERIAL_NUMBER); 4942 4943 /** 4944 * Search parameter: <b>specification-version</b> 4945 * <p> 4946 * Description: <b>A composite of both specification and version</b><br> 4947 * Type: <b>composite</b><br> 4948 * Path: <b>Device.conformsTo</b><br> 4949 * </p> 4950 */ 4951 @SearchParamDefinition(name="specification-version", path="Device.conformsTo", description="A composite of both specification and version", type="composite", compositeOf={"specification", "version"} ) 4952 public static final String SP_SPECIFICATION_VERSION = "specification-version"; 4953 /** 4954 * <b>Fluent Client</b> search parameter constant for <b>specification-version</b> 4955 * <p> 4956 * Description: <b>A composite of both specification and version</b><br> 4957 * Type: <b>composite</b><br> 4958 * Path: <b>Device.conformsTo</b><br> 4959 * </p> 4960 */ 4961 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam> SPECIFICATION_VERSION = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam>(SP_SPECIFICATION_VERSION); 4962 4963 /** 4964 * Search parameter: <b>specification</b> 4965 * <p> 4966 * Description: <b>The standards, specifications, or formal guidances.</b><br> 4967 * Type: <b>token</b><br> 4968 * Path: <b>Device.conformsTo.specification</b><br> 4969 * </p> 4970 */ 4971 @SearchParamDefinition(name="specification", path="Device.conformsTo.specification", description="The standards, specifications, or formal guidances.", type="token" ) 4972 public static final String SP_SPECIFICATION = "specification"; 4973 /** 4974 * <b>Fluent Client</b> search parameter constant for <b>specification</b> 4975 * <p> 4976 * Description: <b>The standards, specifications, or formal guidances.</b><br> 4977 * Type: <b>token</b><br> 4978 * Path: <b>Device.conformsTo.specification</b><br> 4979 * </p> 4980 */ 4981 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIFICATION); 4982 4983 /** 4984 * Search parameter: <b>status</b> 4985 * <p> 4986 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 4987 * Type: <b>token</b><br> 4988 * Path: <b>Device.status</b><br> 4989 * </p> 4990 */ 4991 @SearchParamDefinition(name="status", path="Device.status", description="active | inactive | entered-in-error | unknown", type="token" ) 4992 public static final String SP_STATUS = "status"; 4993 /** 4994 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4995 * <p> 4996 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 4997 * Type: <b>token</b><br> 4998 * Path: <b>Device.status</b><br> 4999 * </p> 5000 */ 5001 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5002 5003 /** 5004 * Search parameter: <b>type</b> 5005 * <p> 5006 * Description: <b>The type of the device</b><br> 5007 * Type: <b>token</b><br> 5008 * Path: <b>Device.type</b><br> 5009 * </p> 5010 */ 5011 @SearchParamDefinition(name="type", path="Device.type", description="The type of the device", type="token" ) 5012 public static final String SP_TYPE = "type"; 5013 /** 5014 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5015 * <p> 5016 * Description: <b>The type of the device</b><br> 5017 * Type: <b>token</b><br> 5018 * Path: <b>Device.type</b><br> 5019 * </p> 5020 */ 5021 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 5022 5023 /** 5024 * Search parameter: <b>udi-carrier</b> 5025 * <p> 5026 * Description: <b>UDI Barcode (RFID or other technology) string in *HRF* format.</b><br> 5027 * Type: <b>string</b><br> 5028 * Path: <b>Device.udiCarrier.carrierHRF</b><br> 5029 * </p> 5030 */ 5031 @SearchParamDefinition(name="udi-carrier", path="Device.udiCarrier.carrierHRF", description="UDI Barcode (RFID or other technology) string in *HRF* format.", type="string" ) 5032 public static final String SP_UDI_CARRIER = "udi-carrier"; 5033 /** 5034 * <b>Fluent Client</b> search parameter constant for <b>udi-carrier</b> 5035 * <p> 5036 * Description: <b>UDI Barcode (RFID or other technology) string in *HRF* format.</b><br> 5037 * Type: <b>string</b><br> 5038 * Path: <b>Device.udiCarrier.carrierHRF</b><br> 5039 * </p> 5040 */ 5041 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_CARRIER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_UDI_CARRIER); 5042 5043 /** 5044 * Search parameter: <b>udi-di</b> 5045 * <p> 5046 * Description: <b>The udi Device Identifier (DI)</b><br> 5047 * Type: <b>string</b><br> 5048 * Path: <b>Device.udiCarrier.deviceIdentifier</b><br> 5049 * </p> 5050 */ 5051 @SearchParamDefinition(name="udi-di", path="Device.udiCarrier.deviceIdentifier", description="The udi Device Identifier (DI)", type="string" ) 5052 public static final String SP_UDI_DI = "udi-di"; 5053 /** 5054 * <b>Fluent Client</b> search parameter constant for <b>udi-di</b> 5055 * <p> 5056 * Description: <b>The udi Device Identifier (DI)</b><br> 5057 * Type: <b>string</b><br> 5058 * Path: <b>Device.udiCarrier.deviceIdentifier</b><br> 5059 * </p> 5060 */ 5061 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_DI = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_UDI_DI); 5062 5063 /** 5064 * Search parameter: <b>url</b> 5065 * <p> 5066 * Description: <b>Network address to contact device</b><br> 5067 * Type: <b>uri</b><br> 5068 * Path: <b>Device.url</b><br> 5069 * </p> 5070 */ 5071 @SearchParamDefinition(name="url", path="Device.url", description="Network address to contact device", type="uri" ) 5072 public static final String SP_URL = "url"; 5073 /** 5074 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5075 * <p> 5076 * Description: <b>Network address to contact device</b><br> 5077 * Type: <b>uri</b><br> 5078 * Path: <b>Device.url</b><br> 5079 * </p> 5080 */ 5081 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5082 5083 /** 5084 * Search parameter: <b>version</b> 5085 * <p> 5086 * Description: <b>The specific version of the device</b><br> 5087 * Type: <b>string</b><br> 5088 * Path: <b>Device.version.value</b><br> 5089 * </p> 5090 */ 5091 @SearchParamDefinition(name="version", path="Device.version.value", description="The specific version of the device", type="string" ) 5092 public static final String SP_VERSION = "version"; 5093 /** 5094 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5095 * <p> 5096 * Description: <b>The specific version of the device</b><br> 5097 * Type: <b>string</b><br> 5098 * Path: <b>Device.version.value</b><br> 5099 * </p> 5100 */ 5101 public static final ca.uhn.fhir.rest.gclient.StringClientParam VERSION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VERSION); 5102 5103 5104} 5105