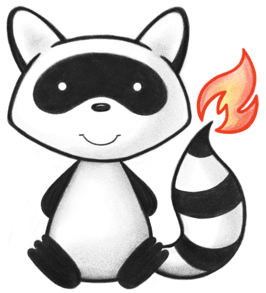
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource describes the properties (regulated, has real time clock, etc.), adminstrative (manufacturer name, model number, serial number, firmware, etc.), and type (knee replacement, blood pressure cuff, MRI, etc.) of a physical unit (these values do not change much within a given module, for example the serail number, manufacturer name, and model number). An actual unit may consist of several modules in a distinct hierarchy and these are represented by multiple Device resources and bound through the 'parent' element. 052 */ 053@ResourceDef(name="Device", profile="http://hl7.org/fhir/StructureDefinition/Device") 054public class Device extends DomainResource { 055 056 public enum FHIRDeviceStatus { 057 /** 058 * The device record is current and is appropriate for reference in new instances. 059 */ 060 ACTIVE, 061 /** 062 * The device record is not current and is not appropriate for reference in new instances. 063 */ 064 INACTIVE, 065 /** 066 * The device record is not current and is not appropriate for reference in new instances. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static FHIRDeviceStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/device-status"; 099 case INACTIVE: return "http://hl7.org/fhir/device-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/device-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The device record is current and is appropriate for reference in new instances."; 108 case INACTIVE: return "The device record is not current and is not appropriate for reference in new instances."; 109 case ENTEREDINERROR: return "The device record is not current and is not appropriate for reference in new instances."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class FHIRDeviceStatusEnumFactory implements EnumFactory<FHIRDeviceStatus> { 126 public FHIRDeviceStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return FHIRDeviceStatus.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return FHIRDeviceStatus.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return FHIRDeviceStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 137 } 138 public Enumeration<FHIRDeviceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ACTIVE, code); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.INACTIVE, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<FHIRDeviceStatus>(this, FHIRDeviceStatus.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown FHIRDeviceStatus code '"+codeString+"'"); 153 } 154 public String toCode(FHIRDeviceStatus code) { 155 if (code == FHIRDeviceStatus.ACTIVE) 156 return "active"; 157 if (code == FHIRDeviceStatus.INACTIVE) 158 return "inactive"; 159 if (code == FHIRDeviceStatus.ENTEREDINERROR) 160 return "entered-in-error"; 161 return "?"; 162 } 163 public String toSystem(FHIRDeviceStatus code) { 164 return code.getSystem(); 165 } 166 } 167 168 public enum UDIEntryType { 169 /** 170 * a barcodescanner captured the data from the device label. 171 */ 172 BARCODE, 173 /** 174 * An RFID chip reader captured the data from the device label. 175 */ 176 RFID, 177 /** 178 * The data was read from the label by a person and manually entered. (e.g. via a keyboard). 179 */ 180 MANUAL, 181 /** 182 * The data originated from a patient's implant card and was read by an operator. 183 */ 184 CARD, 185 /** 186 * The data originated from a patient source and was not directly scanned or read from a label or card. 187 */ 188 SELFREPORTED, 189 /** 190 * The UDI information was received electronically from the device through a communication protocol, such as the IEEE 11073 20601 version 4 exchange protocol over Bluetooth or USB. 191 */ 192 ELECTRONICTRANSMISSION, 193 /** 194 * The method of data capture has not been determined. 195 */ 196 UNKNOWN, 197 /** 198 * added to help the parsers with the generic types 199 */ 200 NULL; 201 public static UDIEntryType fromCode(String codeString) throws FHIRException { 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("barcode".equals(codeString)) 205 return BARCODE; 206 if ("rfid".equals(codeString)) 207 return RFID; 208 if ("manual".equals(codeString)) 209 return MANUAL; 210 if ("card".equals(codeString)) 211 return CARD; 212 if ("self-reported".equals(codeString)) 213 return SELFREPORTED; 214 if ("electronic-transmission".equals(codeString)) 215 return ELECTRONICTRANSMISSION; 216 if ("unknown".equals(codeString)) 217 return UNKNOWN; 218 if (Configuration.isAcceptInvalidEnums()) 219 return null; 220 else 221 throw new FHIRException("Unknown UDIEntryType code '"+codeString+"'"); 222 } 223 public String toCode() { 224 switch (this) { 225 case BARCODE: return "barcode"; 226 case RFID: return "rfid"; 227 case MANUAL: return "manual"; 228 case CARD: return "card"; 229 case SELFREPORTED: return "self-reported"; 230 case ELECTRONICTRANSMISSION: return "electronic-transmission"; 231 case UNKNOWN: return "unknown"; 232 case NULL: return null; 233 default: return "?"; 234 } 235 } 236 public String getSystem() { 237 switch (this) { 238 case BARCODE: return "http://hl7.org/fhir/udi-entry-type"; 239 case RFID: return "http://hl7.org/fhir/udi-entry-type"; 240 case MANUAL: return "http://hl7.org/fhir/udi-entry-type"; 241 case CARD: return "http://hl7.org/fhir/udi-entry-type"; 242 case SELFREPORTED: return "http://hl7.org/fhir/udi-entry-type"; 243 case ELECTRONICTRANSMISSION: return "http://hl7.org/fhir/udi-entry-type"; 244 case UNKNOWN: return "http://hl7.org/fhir/udi-entry-type"; 245 case NULL: return null; 246 default: return "?"; 247 } 248 } 249 public String getDefinition() { 250 switch (this) { 251 case BARCODE: return "a barcodescanner captured the data from the device label."; 252 case RFID: return "An RFID chip reader captured the data from the device label."; 253 case MANUAL: return "The data was read from the label by a person and manually entered. (e.g. via a keyboard)."; 254 case CARD: return "The data originated from a patient's implant card and was read by an operator."; 255 case SELFREPORTED: return "The data originated from a patient source and was not directly scanned or read from a label or card."; 256 case ELECTRONICTRANSMISSION: return "The UDI information was received electronically from the device through a communication protocol, such as the IEEE 11073 20601 version 4 exchange protocol over Bluetooth or USB."; 257 case UNKNOWN: return "The method of data capture has not been determined."; 258 case NULL: return null; 259 default: return "?"; 260 } 261 } 262 public String getDisplay() { 263 switch (this) { 264 case BARCODE: return "Barcode"; 265 case RFID: return "RFID"; 266 case MANUAL: return "Manual"; 267 case CARD: return "Card"; 268 case SELFREPORTED: return "Self Reported"; 269 case ELECTRONICTRANSMISSION: return "Electronic Transmission"; 270 case UNKNOWN: return "Unknown"; 271 case NULL: return null; 272 default: return "?"; 273 } 274 } 275 } 276 277 public static class UDIEntryTypeEnumFactory implements EnumFactory<UDIEntryType> { 278 public UDIEntryType fromCode(String codeString) throws IllegalArgumentException { 279 if (codeString == null || "".equals(codeString)) 280 if (codeString == null || "".equals(codeString)) 281 return null; 282 if ("barcode".equals(codeString)) 283 return UDIEntryType.BARCODE; 284 if ("rfid".equals(codeString)) 285 return UDIEntryType.RFID; 286 if ("manual".equals(codeString)) 287 return UDIEntryType.MANUAL; 288 if ("card".equals(codeString)) 289 return UDIEntryType.CARD; 290 if ("self-reported".equals(codeString)) 291 return UDIEntryType.SELFREPORTED; 292 if ("electronic-transmission".equals(codeString)) 293 return UDIEntryType.ELECTRONICTRANSMISSION; 294 if ("unknown".equals(codeString)) 295 return UDIEntryType.UNKNOWN; 296 throw new IllegalArgumentException("Unknown UDIEntryType code '"+codeString+"'"); 297 } 298 public Enumeration<UDIEntryType> fromType(PrimitiveType<?> code) throws FHIRException { 299 if (code == null) 300 return null; 301 if (code.isEmpty()) 302 return new Enumeration<UDIEntryType>(this, UDIEntryType.NULL, code); 303 String codeString = ((PrimitiveType) code).asStringValue(); 304 if (codeString == null || "".equals(codeString)) 305 return new Enumeration<UDIEntryType>(this, UDIEntryType.NULL, code); 306 if ("barcode".equals(codeString)) 307 return new Enumeration<UDIEntryType>(this, UDIEntryType.BARCODE, code); 308 if ("rfid".equals(codeString)) 309 return new Enumeration<UDIEntryType>(this, UDIEntryType.RFID, code); 310 if ("manual".equals(codeString)) 311 return new Enumeration<UDIEntryType>(this, UDIEntryType.MANUAL, code); 312 if ("card".equals(codeString)) 313 return new Enumeration<UDIEntryType>(this, UDIEntryType.CARD, code); 314 if ("self-reported".equals(codeString)) 315 return new Enumeration<UDIEntryType>(this, UDIEntryType.SELFREPORTED, code); 316 if ("electronic-transmission".equals(codeString)) 317 return new Enumeration<UDIEntryType>(this, UDIEntryType.ELECTRONICTRANSMISSION, code); 318 if ("unknown".equals(codeString)) 319 return new Enumeration<UDIEntryType>(this, UDIEntryType.UNKNOWN, code); 320 throw new FHIRException("Unknown UDIEntryType code '"+codeString+"'"); 321 } 322 public String toCode(UDIEntryType code) { 323 if (code == UDIEntryType.BARCODE) 324 return "barcode"; 325 if (code == UDIEntryType.RFID) 326 return "rfid"; 327 if (code == UDIEntryType.MANUAL) 328 return "manual"; 329 if (code == UDIEntryType.CARD) 330 return "card"; 331 if (code == UDIEntryType.SELFREPORTED) 332 return "self-reported"; 333 if (code == UDIEntryType.ELECTRONICTRANSMISSION) 334 return "electronic-transmission"; 335 if (code == UDIEntryType.UNKNOWN) 336 return "unknown"; 337 return "?"; 338 } 339 public String toSystem(UDIEntryType code) { 340 return code.getSystem(); 341 } 342 } 343 344 @Block() 345 public static class DeviceUdiCarrierComponent extends BackboneElement implements IBaseBackboneElement { 346 /** 347 * The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 348 */ 349 @Child(name = "deviceIdentifier", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 350 @Description(shortDefinition="Mandatory fixed portion of UDI", formalDefinition="The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device." ) 351 protected StringType deviceIdentifier; 352 353 /** 354 * Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 3551) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 3562) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 3573) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 3584) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di. 359 */ 360 @Child(name = "issuer", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 361 @Description(shortDefinition="UDI Issuing Organization", formalDefinition="Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: \n1) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, \n3) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di." ) 362 protected UriType issuer; 363 364 /** 365 * The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi. 366 */ 367 @Child(name = "jurisdiction", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=false) 368 @Description(shortDefinition="Regional UDI authority", formalDefinition="The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi." ) 369 protected UriType jurisdiction; 370 371 /** 372 * The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 373 */ 374 @Child(name = "carrierAIDC", type = {Base64BinaryType.class}, order=4, min=0, max=1, modifier=false, summary=true) 375 @Description(shortDefinition="UDI Machine Readable Barcode String", formalDefinition="The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded." ) 376 protected Base64BinaryType carrierAIDC; 377 378 /** 379 * The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 380 */ 381 @Child(name = "carrierHRF", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 382 @Description(shortDefinition="UDI Human Readable Barcode String", formalDefinition="The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device." ) 383 protected StringType carrierHRF; 384 385 /** 386 * A coded entry to indicate how the data was entered. 387 */ 388 @Child(name = "entryType", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 389 @Description(shortDefinition="barcode | rfid | manual | card | self-reported | electronic-transmission | unknown", formalDefinition="A coded entry to indicate how the data was entered." ) 390 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/udi-entry-type") 391 protected Enumeration<UDIEntryType> entryType; 392 393 private static final long serialVersionUID = -191630425L; 394 395 /** 396 * Constructor 397 */ 398 public DeviceUdiCarrierComponent() { 399 super(); 400 } 401 402 /** 403 * Constructor 404 */ 405 public DeviceUdiCarrierComponent(String deviceIdentifier, String issuer) { 406 super(); 407 this.setDeviceIdentifier(deviceIdentifier); 408 this.setIssuer(issuer); 409 } 410 411 /** 412 * @return {@link #deviceIdentifier} (The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 413 */ 414 public StringType getDeviceIdentifierElement() { 415 if (this.deviceIdentifier == null) 416 if (Configuration.errorOnAutoCreate()) 417 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.deviceIdentifier"); 418 else if (Configuration.doAutoCreate()) 419 this.deviceIdentifier = new StringType(); // bb 420 return this.deviceIdentifier; 421 } 422 423 public boolean hasDeviceIdentifierElement() { 424 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 425 } 426 427 public boolean hasDeviceIdentifier() { 428 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 429 } 430 431 /** 432 * @param value {@link #deviceIdentifier} (The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 433 */ 434 public DeviceUdiCarrierComponent setDeviceIdentifierElement(StringType value) { 435 this.deviceIdentifier = value; 436 return this; 437 } 438 439 /** 440 * @return The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 441 */ 442 public String getDeviceIdentifier() { 443 return this.deviceIdentifier == null ? null : this.deviceIdentifier.getValue(); 444 } 445 446 /** 447 * @param value The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device. 448 */ 449 public DeviceUdiCarrierComponent setDeviceIdentifier(String value) { 450 if (this.deviceIdentifier == null) 451 this.deviceIdentifier = new StringType(); 452 this.deviceIdentifier.setValue(value); 453 return this; 454 } 455 456 /** 457 * @return {@link #issuer} (Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 4581) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 4592) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 4603) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4614) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 462 */ 463 public UriType getIssuerElement() { 464 if (this.issuer == null) 465 if (Configuration.errorOnAutoCreate()) 466 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.issuer"); 467 else if (Configuration.doAutoCreate()) 468 this.issuer = new UriType(); // bb 469 return this.issuer; 470 } 471 472 public boolean hasIssuerElement() { 473 return this.issuer != null && !this.issuer.isEmpty(); 474 } 475 476 public boolean hasIssuer() { 477 return this.issuer != null && !this.issuer.isEmpty(); 478 } 479 480 /** 481 * @param value {@link #issuer} (Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 4821) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 4832) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 4843) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4854) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 486 */ 487 public DeviceUdiCarrierComponent setIssuerElement(UriType value) { 488 this.issuer = value; 489 return this; 490 } 491 492 /** 493 * @return Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 4941) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 4952) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 4963) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 4974) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di. 498 */ 499 public String getIssuer() { 500 return this.issuer == null ? null : this.issuer.getValue(); 501 } 502 503 /** 504 * @param value Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: 5051) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, 5062) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, 5073) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, 5084) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di. 509 */ 510 public DeviceUdiCarrierComponent setIssuer(String value) { 511 if (this.issuer == null) 512 this.issuer = new UriType(); 513 this.issuer.setValue(value); 514 return this; 515 } 516 517 /** 518 * @return {@link #jurisdiction} (The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 519 */ 520 public UriType getJurisdictionElement() { 521 if (this.jurisdiction == null) 522 if (Configuration.errorOnAutoCreate()) 523 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.jurisdiction"); 524 else if (Configuration.doAutoCreate()) 525 this.jurisdiction = new UriType(); // bb 526 return this.jurisdiction; 527 } 528 529 public boolean hasJurisdictionElement() { 530 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 531 } 532 533 public boolean hasJurisdiction() { 534 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #jurisdiction} (The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 539 */ 540 public DeviceUdiCarrierComponent setJurisdictionElement(UriType value) { 541 this.jurisdiction = value; 542 return this; 543 } 544 545 /** 546 * @return The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi. 547 */ 548 public String getJurisdiction() { 549 return this.jurisdiction == null ? null : this.jurisdiction.getValue(); 550 } 551 552 /** 553 * @param value The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi. 554 */ 555 public DeviceUdiCarrierComponent setJurisdiction(String value) { 556 if (Utilities.noString(value)) 557 this.jurisdiction = null; 558 else { 559 if (this.jurisdiction == null) 560 this.jurisdiction = new UriType(); 561 this.jurisdiction.setValue(value); 562 } 563 return this; 564 } 565 566 /** 567 * @return {@link #carrierAIDC} (The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getCarrierAIDC" gives direct access to the value 568 */ 569 public Base64BinaryType getCarrierAIDCElement() { 570 if (this.carrierAIDC == null) 571 if (Configuration.errorOnAutoCreate()) 572 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.carrierAIDC"); 573 else if (Configuration.doAutoCreate()) 574 this.carrierAIDC = new Base64BinaryType(); // bb 575 return this.carrierAIDC; 576 } 577 578 public boolean hasCarrierAIDCElement() { 579 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 580 } 581 582 public boolean hasCarrierAIDC() { 583 return this.carrierAIDC != null && !this.carrierAIDC.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #carrierAIDC} (The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.). This is the underlying object with id, value and extensions. The accessor "getCarrierAIDC" gives direct access to the value 588 */ 589 public DeviceUdiCarrierComponent setCarrierAIDCElement(Base64BinaryType value) { 590 this.carrierAIDC = value; 591 return this; 592 } 593 594 /** 595 * @return The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 596 */ 597 public byte[] getCarrierAIDC() { 598 return this.carrierAIDC == null ? null : this.carrierAIDC.getValue(); 599 } 600 601 /** 602 * @param value The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded. 603 */ 604 public DeviceUdiCarrierComponent setCarrierAIDC(byte[] value) { 605 if (value == null) 606 this.carrierAIDC = null; 607 else { 608 if (this.carrierAIDC == null) 609 this.carrierAIDC = new Base64BinaryType(); 610 this.carrierAIDC.setValue(value); 611 } 612 return this; 613 } 614 615 /** 616 * @return {@link #carrierHRF} (The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.). This is the underlying object with id, value and extensions. The accessor "getCarrierHRF" gives direct access to the value 617 */ 618 public StringType getCarrierHRFElement() { 619 if (this.carrierHRF == null) 620 if (Configuration.errorOnAutoCreate()) 621 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.carrierHRF"); 622 else if (Configuration.doAutoCreate()) 623 this.carrierHRF = new StringType(); // bb 624 return this.carrierHRF; 625 } 626 627 public boolean hasCarrierHRFElement() { 628 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 629 } 630 631 public boolean hasCarrierHRF() { 632 return this.carrierHRF != null && !this.carrierHRF.isEmpty(); 633 } 634 635 /** 636 * @param value {@link #carrierHRF} (The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.). This is the underlying object with id, value and extensions. The accessor "getCarrierHRF" gives direct access to the value 637 */ 638 public DeviceUdiCarrierComponent setCarrierHRFElement(StringType value) { 639 this.carrierHRF = value; 640 return this; 641 } 642 643 /** 644 * @return The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 645 */ 646 public String getCarrierHRF() { 647 return this.carrierHRF == null ? null : this.carrierHRF.getValue(); 648 } 649 650 /** 651 * @param value The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device. 652 */ 653 public DeviceUdiCarrierComponent setCarrierHRF(String value) { 654 if (Utilities.noString(value)) 655 this.carrierHRF = null; 656 else { 657 if (this.carrierHRF == null) 658 this.carrierHRF = new StringType(); 659 this.carrierHRF.setValue(value); 660 } 661 return this; 662 } 663 664 /** 665 * @return {@link #entryType} (A coded entry to indicate how the data was entered.). This is the underlying object with id, value and extensions. The accessor "getEntryType" gives direct access to the value 666 */ 667 public Enumeration<UDIEntryType> getEntryTypeElement() { 668 if (this.entryType == null) 669 if (Configuration.errorOnAutoCreate()) 670 throw new Error("Attempt to auto-create DeviceUdiCarrierComponent.entryType"); 671 else if (Configuration.doAutoCreate()) 672 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); // bb 673 return this.entryType; 674 } 675 676 public boolean hasEntryTypeElement() { 677 return this.entryType != null && !this.entryType.isEmpty(); 678 } 679 680 public boolean hasEntryType() { 681 return this.entryType != null && !this.entryType.isEmpty(); 682 } 683 684 /** 685 * @param value {@link #entryType} (A coded entry to indicate how the data was entered.). This is the underlying object with id, value and extensions. The accessor "getEntryType" gives direct access to the value 686 */ 687 public DeviceUdiCarrierComponent setEntryTypeElement(Enumeration<UDIEntryType> value) { 688 this.entryType = value; 689 return this; 690 } 691 692 /** 693 * @return A coded entry to indicate how the data was entered. 694 */ 695 public UDIEntryType getEntryType() { 696 return this.entryType == null ? null : this.entryType.getValue(); 697 } 698 699 /** 700 * @param value A coded entry to indicate how the data was entered. 701 */ 702 public DeviceUdiCarrierComponent setEntryType(UDIEntryType value) { 703 if (value == null) 704 this.entryType = null; 705 else { 706 if (this.entryType == null) 707 this.entryType = new Enumeration<UDIEntryType>(new UDIEntryTypeEnumFactory()); 708 this.entryType.setValue(value); 709 } 710 return this; 711 } 712 713 protected void listChildren(List<Property> children) { 714 super.listChildren(children); 715 children.add(new Property("deviceIdentifier", "string", "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 0, 1, deviceIdentifier)); 716 children.add(new Property("issuer", "uri", "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: \n1) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, \n3) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.", 0, 1, issuer)); 717 children.add(new Property("jurisdiction", "uri", "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.", 0, 1, jurisdiction)); 718 children.add(new Property("carrierAIDC", "base64Binary", "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 0, 1, carrierAIDC)); 719 children.add(new Property("carrierHRF", "string", "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 0, 1, carrierHRF)); 720 children.add(new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 0, 1, entryType)); 721 } 722 723 @Override 724 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 725 switch (_hash) { 726 case 1322005407: /*deviceIdentifier*/ return new Property("deviceIdentifier", "string", "The device identifier (DI) is a mandatory, fixed portion of a UDI that identifies the labeler and the specific version or model of a device.", 0, 1, deviceIdentifier); 727 case -1179159879: /*issuer*/ return new Property("issuer", "uri", "Organization that is charged with issuing UDIs for devices. For example, the US FDA issuers include: \n1) GS1: http://hl7.org/fhir/NamingSystem/gs1-di, \n2) HIBCC: http://hl7.org/fhir/NamingSystem/hibcc-diI, \n3) ICCBBA for blood containers: http://hl7.org/fhir/NamingSystem/iccbba-blood-di, \n4) ICCBA for other devices: http://hl7.org/fhir/NamingSystem/iccbba-other-di # Informationsstelle für Arzneispezialitäten (IFA GmbH) (EU only): http://hl7.org/fhir/NamingSystem/ifa-gmbh-di.", 0, 1, issuer); 728 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "uri", "The identity of the authoritative source for UDI generation within a jurisdiction. All UDIs are globally unique within a single namespace with the appropriate repository uri as the system. For example, UDIs of devices managed in the U.S. by the FDA, the value is http://hl7.org/fhir/NamingSystem/us-fda-udi or in the European Union by the European Commission http://hl7.org/fhir/NamingSystem/eu-ec-udi.", 0, 1, jurisdiction); 729 case -768521825: /*carrierAIDC*/ return new Property("carrierAIDC", "base64Binary", "The full UDI carrier of the Automatic Identification and Data Capture (AIDC) technology representation of the barcode string as printed on the packaging of the device - e.g., a barcode or RFID. Because of limitations on character sets in XML and the need to round-trip JSON data through XML, AIDC Formats *SHALL* be base64 encoded.", 0, 1, carrierAIDC); 730 case 806499972: /*carrierHRF*/ return new Property("carrierHRF", "string", "The full UDI carrier as the human readable form (HRF) representation of the barcode string as printed on the packaging of the device.", 0, 1, carrierHRF); 731 case -479362356: /*entryType*/ return new Property("entryType", "code", "A coded entry to indicate how the data was entered.", 0, 1, entryType); 732 default: return super.getNamedProperty(_hash, _name, _checkValid); 733 } 734 735 } 736 737 @Override 738 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 739 switch (hash) { 740 case 1322005407: /*deviceIdentifier*/ return this.deviceIdentifier == null ? new Base[0] : new Base[] {this.deviceIdentifier}; // StringType 741 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // UriType 742 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // UriType 743 case -768521825: /*carrierAIDC*/ return this.carrierAIDC == null ? new Base[0] : new Base[] {this.carrierAIDC}; // Base64BinaryType 744 case 806499972: /*carrierHRF*/ return this.carrierHRF == null ? new Base[0] : new Base[] {this.carrierHRF}; // StringType 745 case -479362356: /*entryType*/ return this.entryType == null ? new Base[0] : new Base[] {this.entryType}; // Enumeration<UDIEntryType> 746 default: return super.getProperty(hash, name, checkValid); 747 } 748 749 } 750 751 @Override 752 public Base setProperty(int hash, String name, Base value) throws FHIRException { 753 switch (hash) { 754 case 1322005407: // deviceIdentifier 755 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 756 return value; 757 case -1179159879: // issuer 758 this.issuer = TypeConvertor.castToUri(value); // UriType 759 return value; 760 case -507075711: // jurisdiction 761 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 762 return value; 763 case -768521825: // carrierAIDC 764 this.carrierAIDC = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 765 return value; 766 case 806499972: // carrierHRF 767 this.carrierHRF = TypeConvertor.castToString(value); // StringType 768 return value; 769 case -479362356: // entryType 770 value = new UDIEntryTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 771 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 772 return value; 773 default: return super.setProperty(hash, name, value); 774 } 775 776 } 777 778 @Override 779 public Base setProperty(String name, Base value) throws FHIRException { 780 if (name.equals("deviceIdentifier")) { 781 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 782 } else if (name.equals("issuer")) { 783 this.issuer = TypeConvertor.castToUri(value); // UriType 784 } else if (name.equals("jurisdiction")) { 785 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 786 } else if (name.equals("carrierAIDC")) { 787 this.carrierAIDC = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 788 } else if (name.equals("carrierHRF")) { 789 this.carrierHRF = TypeConvertor.castToString(value); // StringType 790 } else if (name.equals("entryType")) { 791 value = new UDIEntryTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 792 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 793 } else 794 return super.setProperty(name, value); 795 return value; 796 } 797 798 @Override 799 public void removeChild(String name, Base value) throws FHIRException { 800 if (name.equals("deviceIdentifier")) { 801 this.deviceIdentifier = null; 802 } else if (name.equals("issuer")) { 803 this.issuer = null; 804 } else if (name.equals("jurisdiction")) { 805 this.jurisdiction = null; 806 } else if (name.equals("carrierAIDC")) { 807 this.carrierAIDC = null; 808 } else if (name.equals("carrierHRF")) { 809 this.carrierHRF = null; 810 } else if (name.equals("entryType")) { 811 value = new UDIEntryTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 812 this.entryType = (Enumeration) value; // Enumeration<UDIEntryType> 813 } else 814 super.removeChild(name, value); 815 816 } 817 818 @Override 819 public Base makeProperty(int hash, String name) throws FHIRException { 820 switch (hash) { 821 case 1322005407: return getDeviceIdentifierElement(); 822 case -1179159879: return getIssuerElement(); 823 case -507075711: return getJurisdictionElement(); 824 case -768521825: return getCarrierAIDCElement(); 825 case 806499972: return getCarrierHRFElement(); 826 case -479362356: return getEntryTypeElement(); 827 default: return super.makeProperty(hash, name); 828 } 829 830 } 831 832 @Override 833 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 834 switch (hash) { 835 case 1322005407: /*deviceIdentifier*/ return new String[] {"string"}; 836 case -1179159879: /*issuer*/ return new String[] {"uri"}; 837 case -507075711: /*jurisdiction*/ return new String[] {"uri"}; 838 case -768521825: /*carrierAIDC*/ return new String[] {"base64Binary"}; 839 case 806499972: /*carrierHRF*/ return new String[] {"string"}; 840 case -479362356: /*entryType*/ return new String[] {"code"}; 841 default: return super.getTypesForProperty(hash, name); 842 } 843 844 } 845 846 @Override 847 public Base addChild(String name) throws FHIRException { 848 if (name.equals("deviceIdentifier")) { 849 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.deviceIdentifier"); 850 } 851 else if (name.equals("issuer")) { 852 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.issuer"); 853 } 854 else if (name.equals("jurisdiction")) { 855 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.jurisdiction"); 856 } 857 else if (name.equals("carrierAIDC")) { 858 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.carrierAIDC"); 859 } 860 else if (name.equals("carrierHRF")) { 861 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.carrierHRF"); 862 } 863 else if (name.equals("entryType")) { 864 throw new FHIRException("Cannot call addChild on a singleton property Device.udiCarrier.entryType"); 865 } 866 else 867 return super.addChild(name); 868 } 869 870 public DeviceUdiCarrierComponent copy() { 871 DeviceUdiCarrierComponent dst = new DeviceUdiCarrierComponent(); 872 copyValues(dst); 873 return dst; 874 } 875 876 public void copyValues(DeviceUdiCarrierComponent dst) { 877 super.copyValues(dst); 878 dst.deviceIdentifier = deviceIdentifier == null ? null : deviceIdentifier.copy(); 879 dst.issuer = issuer == null ? null : issuer.copy(); 880 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 881 dst.carrierAIDC = carrierAIDC == null ? null : carrierAIDC.copy(); 882 dst.carrierHRF = carrierHRF == null ? null : carrierHRF.copy(); 883 dst.entryType = entryType == null ? null : entryType.copy(); 884 } 885 886 @Override 887 public boolean equalsDeep(Base other_) { 888 if (!super.equalsDeep(other_)) 889 return false; 890 if (!(other_ instanceof DeviceUdiCarrierComponent)) 891 return false; 892 DeviceUdiCarrierComponent o = (DeviceUdiCarrierComponent) other_; 893 return compareDeep(deviceIdentifier, o.deviceIdentifier, true) && compareDeep(issuer, o.issuer, true) 894 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(carrierAIDC, o.carrierAIDC, true) 895 && compareDeep(carrierHRF, o.carrierHRF, true) && compareDeep(entryType, o.entryType, true); 896 } 897 898 @Override 899 public boolean equalsShallow(Base other_) { 900 if (!super.equalsShallow(other_)) 901 return false; 902 if (!(other_ instanceof DeviceUdiCarrierComponent)) 903 return false; 904 DeviceUdiCarrierComponent o = (DeviceUdiCarrierComponent) other_; 905 return compareValues(deviceIdentifier, o.deviceIdentifier, true) && compareValues(issuer, o.issuer, true) 906 && compareValues(jurisdiction, o.jurisdiction, true) && compareValues(carrierAIDC, o.carrierAIDC, true) 907 && compareValues(carrierHRF, o.carrierHRF, true) && compareValues(entryType, o.entryType, true); 908 } 909 910 public boolean isEmpty() { 911 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(deviceIdentifier, issuer, jurisdiction 912 , carrierAIDC, carrierHRF, entryType); 913 } 914 915 public String fhirType() { 916 return "Device.udiCarrier"; 917 918 } 919 920 } 921 922 @Block() 923 public static class DeviceNameComponent extends BackboneElement implements IBaseBackboneElement { 924 /** 925 * The actual name that identifies the device. 926 */ 927 @Child(name = "value", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 928 @Description(shortDefinition="The term that names the device", formalDefinition="The actual name that identifies the device." ) 929 protected StringType value; 930 931 /** 932 * Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName. 933 */ 934 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 935 @Description(shortDefinition="registered-name | user-friendly-name | patient-reported-name", formalDefinition="Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName." ) 936 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-nametype") 937 protected Enumeration<DeviceNameType> type; 938 939 /** 940 * Indicates the default or preferred name to be displayed. 941 */ 942 @Child(name = "display", type = {BooleanType.class}, order=3, min=0, max=1, modifier=true, summary=true) 943 @Description(shortDefinition="The preferred device name", formalDefinition="Indicates the default or preferred name to be displayed." ) 944 protected BooleanType display; 945 946 private static final long serialVersionUID = 1911928470L; 947 948 /** 949 * Constructor 950 */ 951 public DeviceNameComponent() { 952 super(); 953 } 954 955 /** 956 * Constructor 957 */ 958 public DeviceNameComponent(String value, DeviceNameType type) { 959 super(); 960 this.setValue(value); 961 this.setType(type); 962 } 963 964 /** 965 * @return {@link #value} (The actual name that identifies the device.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 966 */ 967 public StringType getValueElement() { 968 if (this.value == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create DeviceNameComponent.value"); 971 else if (Configuration.doAutoCreate()) 972 this.value = new StringType(); // bb 973 return this.value; 974 } 975 976 public boolean hasValueElement() { 977 return this.value != null && !this.value.isEmpty(); 978 } 979 980 public boolean hasValue() { 981 return this.value != null && !this.value.isEmpty(); 982 } 983 984 /** 985 * @param value {@link #value} (The actual name that identifies the device.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 986 */ 987 public DeviceNameComponent setValueElement(StringType value) { 988 this.value = value; 989 return this; 990 } 991 992 /** 993 * @return The actual name that identifies the device. 994 */ 995 public String getValue() { 996 return this.value == null ? null : this.value.getValue(); 997 } 998 999 /** 1000 * @param value The actual name that identifies the device. 1001 */ 1002 public DeviceNameComponent setValue(String value) { 1003 if (this.value == null) 1004 this.value = new StringType(); 1005 this.value.setValue(value); 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #type} (Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1011 */ 1012 public Enumeration<DeviceNameType> getTypeElement() { 1013 if (this.type == null) 1014 if (Configuration.errorOnAutoCreate()) 1015 throw new Error("Attempt to auto-create DeviceNameComponent.type"); 1016 else if (Configuration.doAutoCreate()) 1017 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); // bb 1018 return this.type; 1019 } 1020 1021 public boolean hasTypeElement() { 1022 return this.type != null && !this.type.isEmpty(); 1023 } 1024 1025 public boolean hasType() { 1026 return this.type != null && !this.type.isEmpty(); 1027 } 1028 1029 /** 1030 * @param value {@link #type} (Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1031 */ 1032 public DeviceNameComponent setTypeElement(Enumeration<DeviceNameType> value) { 1033 this.type = value; 1034 return this; 1035 } 1036 1037 /** 1038 * @return Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName. 1039 */ 1040 public DeviceNameType getType() { 1041 return this.type == null ? null : this.type.getValue(); 1042 } 1043 1044 /** 1045 * @param value Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName. 1046 */ 1047 public DeviceNameComponent setType(DeviceNameType value) { 1048 if (this.type == null) 1049 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); 1050 this.type.setValue(value); 1051 return this; 1052 } 1053 1054 /** 1055 * @return {@link #display} (Indicates the default or preferred name to be displayed.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1056 */ 1057 public BooleanType getDisplayElement() { 1058 if (this.display == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create DeviceNameComponent.display"); 1061 else if (Configuration.doAutoCreate()) 1062 this.display = new BooleanType(); // bb 1063 return this.display; 1064 } 1065 1066 public boolean hasDisplayElement() { 1067 return this.display != null && !this.display.isEmpty(); 1068 } 1069 1070 public boolean hasDisplay() { 1071 return this.display != null && !this.display.isEmpty(); 1072 } 1073 1074 /** 1075 * @param value {@link #display} (Indicates the default or preferred name to be displayed.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1076 */ 1077 public DeviceNameComponent setDisplayElement(BooleanType value) { 1078 this.display = value; 1079 return this; 1080 } 1081 1082 /** 1083 * @return Indicates the default or preferred name to be displayed. 1084 */ 1085 public boolean getDisplay() { 1086 return this.display == null || this.display.isEmpty() ? false : this.display.getValue(); 1087 } 1088 1089 /** 1090 * @param value Indicates the default or preferred name to be displayed. 1091 */ 1092 public DeviceNameComponent setDisplay(boolean value) { 1093 if (this.display == null) 1094 this.display = new BooleanType(); 1095 this.display.setValue(value); 1096 return this; 1097 } 1098 1099 protected void listChildren(List<Property> children) { 1100 super.listChildren(children); 1101 children.add(new Property("value", "string", "The actual name that identifies the device.", 0, 1, value)); 1102 children.add(new Property("type", "code", "Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.", 0, 1, type)); 1103 children.add(new Property("display", "boolean", "Indicates the default or preferred name to be displayed.", 0, 1, display)); 1104 } 1105 1106 @Override 1107 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1108 switch (_hash) { 1109 case 111972721: /*value*/ return new Property("value", "string", "The actual name that identifies the device.", 0, 1, value); 1110 case 3575610: /*type*/ return new Property("type", "code", "Indicates the kind of name. RegisteredName | UserFriendlyName | PatientReportedName.", 0, 1, type); 1111 case 1671764162: /*display*/ return new Property("display", "boolean", "Indicates the default or preferred name to be displayed.", 0, 1, display); 1112 default: return super.getNamedProperty(_hash, _name, _checkValid); 1113 } 1114 1115 } 1116 1117 @Override 1118 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1119 switch (hash) { 1120 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1121 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DeviceNameType> 1122 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // BooleanType 1123 default: return super.getProperty(hash, name, checkValid); 1124 } 1125 1126 } 1127 1128 @Override 1129 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1130 switch (hash) { 1131 case 111972721: // value 1132 this.value = TypeConvertor.castToString(value); // StringType 1133 return value; 1134 case 3575610: // type 1135 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1136 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1137 return value; 1138 case 1671764162: // display 1139 this.display = TypeConvertor.castToBoolean(value); // BooleanType 1140 return value; 1141 default: return super.setProperty(hash, name, value); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base setProperty(String name, Base value) throws FHIRException { 1148 if (name.equals("value")) { 1149 this.value = TypeConvertor.castToString(value); // StringType 1150 } else if (name.equals("type")) { 1151 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1152 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1153 } else if (name.equals("display")) { 1154 this.display = TypeConvertor.castToBoolean(value); // BooleanType 1155 } else 1156 return super.setProperty(name, value); 1157 return value; 1158 } 1159 1160 @Override 1161 public void removeChild(String name, Base value) throws FHIRException { 1162 if (name.equals("value")) { 1163 this.value = null; 1164 } else if (name.equals("type")) { 1165 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1166 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1167 } else if (name.equals("display")) { 1168 this.display = null; 1169 } else 1170 super.removeChild(name, value); 1171 1172 } 1173 1174 @Override 1175 public Base makeProperty(int hash, String name) throws FHIRException { 1176 switch (hash) { 1177 case 111972721: return getValueElement(); 1178 case 3575610: return getTypeElement(); 1179 case 1671764162: return getDisplayElement(); 1180 default: return super.makeProperty(hash, name); 1181 } 1182 1183 } 1184 1185 @Override 1186 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1187 switch (hash) { 1188 case 111972721: /*value*/ return new String[] {"string"}; 1189 case 3575610: /*type*/ return new String[] {"code"}; 1190 case 1671764162: /*display*/ return new String[] {"boolean"}; 1191 default: return super.getTypesForProperty(hash, name); 1192 } 1193 1194 } 1195 1196 @Override 1197 public Base addChild(String name) throws FHIRException { 1198 if (name.equals("value")) { 1199 throw new FHIRException("Cannot call addChild on a singleton property Device.name.value"); 1200 } 1201 else if (name.equals("type")) { 1202 throw new FHIRException("Cannot call addChild on a singleton property Device.name.type"); 1203 } 1204 else if (name.equals("display")) { 1205 throw new FHIRException("Cannot call addChild on a singleton property Device.name.display"); 1206 } 1207 else 1208 return super.addChild(name); 1209 } 1210 1211 public DeviceNameComponent copy() { 1212 DeviceNameComponent dst = new DeviceNameComponent(); 1213 copyValues(dst); 1214 return dst; 1215 } 1216 1217 public void copyValues(DeviceNameComponent dst) { 1218 super.copyValues(dst); 1219 dst.value = value == null ? null : value.copy(); 1220 dst.type = type == null ? null : type.copy(); 1221 dst.display = display == null ? null : display.copy(); 1222 } 1223 1224 @Override 1225 public boolean equalsDeep(Base other_) { 1226 if (!super.equalsDeep(other_)) 1227 return false; 1228 if (!(other_ instanceof DeviceNameComponent)) 1229 return false; 1230 DeviceNameComponent o = (DeviceNameComponent) other_; 1231 return compareDeep(value, o.value, true) && compareDeep(type, o.type, true) && compareDeep(display, o.display, true) 1232 ; 1233 } 1234 1235 @Override 1236 public boolean equalsShallow(Base other_) { 1237 if (!super.equalsShallow(other_)) 1238 return false; 1239 if (!(other_ instanceof DeviceNameComponent)) 1240 return false; 1241 DeviceNameComponent o = (DeviceNameComponent) other_; 1242 return compareValues(value, o.value, true) && compareValues(type, o.type, true) && compareValues(display, o.display, true) 1243 ; 1244 } 1245 1246 public boolean isEmpty() { 1247 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, type, display); 1248 } 1249 1250 public String fhirType() { 1251 return "Device.name"; 1252 1253 } 1254 1255 } 1256 1257 @Block() 1258 public static class DeviceVersionComponent extends BackboneElement implements IBaseBackboneElement { 1259 /** 1260 * The type of the device version, e.g. manufacturer, approved, internal. 1261 */ 1262 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1263 @Description(shortDefinition="The type of the device version, e.g. manufacturer, approved, internal", formalDefinition="The type of the device version, e.g. manufacturer, approved, internal." ) 1264 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-versiontype") 1265 protected CodeableConcept type; 1266 1267 /** 1268 * The hardware or software module of the device to which the version applies. 1269 */ 1270 @Child(name = "component", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 1271 @Description(shortDefinition="The hardware or software module of the device to which the version applies", formalDefinition="The hardware or software module of the device to which the version applies." ) 1272 protected Identifier component; 1273 1274 /** 1275 * The date the version was installed on the device. 1276 */ 1277 @Child(name = "installDate", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1278 @Description(shortDefinition="The date the version was installed on the device", formalDefinition="The date the version was installed on the device." ) 1279 protected DateTimeType installDate; 1280 1281 /** 1282 * The version text. 1283 */ 1284 @Child(name = "value", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 1285 @Description(shortDefinition="The version text", formalDefinition="The version text." ) 1286 protected StringType value; 1287 1288 private static final long serialVersionUID = 1358422741L; 1289 1290 /** 1291 * Constructor 1292 */ 1293 public DeviceVersionComponent() { 1294 super(); 1295 } 1296 1297 /** 1298 * Constructor 1299 */ 1300 public DeviceVersionComponent(String value) { 1301 super(); 1302 this.setValue(value); 1303 } 1304 1305 /** 1306 * @return {@link #type} (The type of the device version, e.g. manufacturer, approved, internal.) 1307 */ 1308 public CodeableConcept getType() { 1309 if (this.type == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create DeviceVersionComponent.type"); 1312 else if (Configuration.doAutoCreate()) 1313 this.type = new CodeableConcept(); // cc 1314 return this.type; 1315 } 1316 1317 public boolean hasType() { 1318 return this.type != null && !this.type.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #type} (The type of the device version, e.g. manufacturer, approved, internal.) 1323 */ 1324 public DeviceVersionComponent setType(CodeableConcept value) { 1325 this.type = value; 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #component} (The hardware or software module of the device to which the version applies.) 1331 */ 1332 public Identifier getComponent() { 1333 if (this.component == null) 1334 if (Configuration.errorOnAutoCreate()) 1335 throw new Error("Attempt to auto-create DeviceVersionComponent.component"); 1336 else if (Configuration.doAutoCreate()) 1337 this.component = new Identifier(); // cc 1338 return this.component; 1339 } 1340 1341 public boolean hasComponent() { 1342 return this.component != null && !this.component.isEmpty(); 1343 } 1344 1345 /** 1346 * @param value {@link #component} (The hardware or software module of the device to which the version applies.) 1347 */ 1348 public DeviceVersionComponent setComponent(Identifier value) { 1349 this.component = value; 1350 return this; 1351 } 1352 1353 /** 1354 * @return {@link #installDate} (The date the version was installed on the device.). This is the underlying object with id, value and extensions. The accessor "getInstallDate" gives direct access to the value 1355 */ 1356 public DateTimeType getInstallDateElement() { 1357 if (this.installDate == null) 1358 if (Configuration.errorOnAutoCreate()) 1359 throw new Error("Attempt to auto-create DeviceVersionComponent.installDate"); 1360 else if (Configuration.doAutoCreate()) 1361 this.installDate = new DateTimeType(); // bb 1362 return this.installDate; 1363 } 1364 1365 public boolean hasInstallDateElement() { 1366 return this.installDate != null && !this.installDate.isEmpty(); 1367 } 1368 1369 public boolean hasInstallDate() { 1370 return this.installDate != null && !this.installDate.isEmpty(); 1371 } 1372 1373 /** 1374 * @param value {@link #installDate} (The date the version was installed on the device.). This is the underlying object with id, value and extensions. The accessor "getInstallDate" gives direct access to the value 1375 */ 1376 public DeviceVersionComponent setInstallDateElement(DateTimeType value) { 1377 this.installDate = value; 1378 return this; 1379 } 1380 1381 /** 1382 * @return The date the version was installed on the device. 1383 */ 1384 public Date getInstallDate() { 1385 return this.installDate == null ? null : this.installDate.getValue(); 1386 } 1387 1388 /** 1389 * @param value The date the version was installed on the device. 1390 */ 1391 public DeviceVersionComponent setInstallDate(Date value) { 1392 if (value == null) 1393 this.installDate = null; 1394 else { 1395 if (this.installDate == null) 1396 this.installDate = new DateTimeType(); 1397 this.installDate.setValue(value); 1398 } 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #value} (The version text.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1404 */ 1405 public StringType getValueElement() { 1406 if (this.value == null) 1407 if (Configuration.errorOnAutoCreate()) 1408 throw new Error("Attempt to auto-create DeviceVersionComponent.value"); 1409 else if (Configuration.doAutoCreate()) 1410 this.value = new StringType(); // bb 1411 return this.value; 1412 } 1413 1414 public boolean hasValueElement() { 1415 return this.value != null && !this.value.isEmpty(); 1416 } 1417 1418 public boolean hasValue() { 1419 return this.value != null && !this.value.isEmpty(); 1420 } 1421 1422 /** 1423 * @param value {@link #value} (The version text.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1424 */ 1425 public DeviceVersionComponent setValueElement(StringType value) { 1426 this.value = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return The version text. 1432 */ 1433 public String getValue() { 1434 return this.value == null ? null : this.value.getValue(); 1435 } 1436 1437 /** 1438 * @param value The version text. 1439 */ 1440 public DeviceVersionComponent setValue(String value) { 1441 if (this.value == null) 1442 this.value = new StringType(); 1443 this.value.setValue(value); 1444 return this; 1445 } 1446 1447 protected void listChildren(List<Property> children) { 1448 super.listChildren(children); 1449 children.add(new Property("type", "CodeableConcept", "The type of the device version, e.g. manufacturer, approved, internal.", 0, 1, type)); 1450 children.add(new Property("component", "Identifier", "The hardware or software module of the device to which the version applies.", 0, 1, component)); 1451 children.add(new Property("installDate", "dateTime", "The date the version was installed on the device.", 0, 1, installDate)); 1452 children.add(new Property("value", "string", "The version text.", 0, 1, value)); 1453 } 1454 1455 @Override 1456 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1457 switch (_hash) { 1458 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the device version, e.g. manufacturer, approved, internal.", 0, 1, type); 1459 case -1399907075: /*component*/ return new Property("component", "Identifier", "The hardware or software module of the device to which the version applies.", 0, 1, component); 1460 case 2143044585: /*installDate*/ return new Property("installDate", "dateTime", "The date the version was installed on the device.", 0, 1, installDate); 1461 case 111972721: /*value*/ return new Property("value", "string", "The version text.", 0, 1, value); 1462 default: return super.getNamedProperty(_hash, _name, _checkValid); 1463 } 1464 1465 } 1466 1467 @Override 1468 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1469 switch (hash) { 1470 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1471 case -1399907075: /*component*/ return this.component == null ? new Base[0] : new Base[] {this.component}; // Identifier 1472 case 2143044585: /*installDate*/ return this.installDate == null ? new Base[0] : new Base[] {this.installDate}; // DateTimeType 1473 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1474 default: return super.getProperty(hash, name, checkValid); 1475 } 1476 1477 } 1478 1479 @Override 1480 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1481 switch (hash) { 1482 case 3575610: // type 1483 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1484 return value; 1485 case -1399907075: // component 1486 this.component = TypeConvertor.castToIdentifier(value); // Identifier 1487 return value; 1488 case 2143044585: // installDate 1489 this.installDate = TypeConvertor.castToDateTime(value); // DateTimeType 1490 return value; 1491 case 111972721: // value 1492 this.value = TypeConvertor.castToString(value); // StringType 1493 return value; 1494 default: return super.setProperty(hash, name, value); 1495 } 1496 1497 } 1498 1499 @Override 1500 public Base setProperty(String name, Base value) throws FHIRException { 1501 if (name.equals("type")) { 1502 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1503 } else if (name.equals("component")) { 1504 this.component = TypeConvertor.castToIdentifier(value); // Identifier 1505 } else if (name.equals("installDate")) { 1506 this.installDate = TypeConvertor.castToDateTime(value); // DateTimeType 1507 } else if (name.equals("value")) { 1508 this.value = TypeConvertor.castToString(value); // StringType 1509 } else 1510 return super.setProperty(name, value); 1511 return value; 1512 } 1513 1514 @Override 1515 public void removeChild(String name, Base value) throws FHIRException { 1516 if (name.equals("type")) { 1517 this.type = null; 1518 } else if (name.equals("component")) { 1519 this.component = null; 1520 } else if (name.equals("installDate")) { 1521 this.installDate = null; 1522 } else if (name.equals("value")) { 1523 this.value = null; 1524 } else 1525 super.removeChild(name, value); 1526 1527 } 1528 1529 @Override 1530 public Base makeProperty(int hash, String name) throws FHIRException { 1531 switch (hash) { 1532 case 3575610: return getType(); 1533 case -1399907075: return getComponent(); 1534 case 2143044585: return getInstallDateElement(); 1535 case 111972721: return getValueElement(); 1536 default: return super.makeProperty(hash, name); 1537 } 1538 1539 } 1540 1541 @Override 1542 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1543 switch (hash) { 1544 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1545 case -1399907075: /*component*/ return new String[] {"Identifier"}; 1546 case 2143044585: /*installDate*/ return new String[] {"dateTime"}; 1547 case 111972721: /*value*/ return new String[] {"string"}; 1548 default: return super.getTypesForProperty(hash, name); 1549 } 1550 1551 } 1552 1553 @Override 1554 public Base addChild(String name) throws FHIRException { 1555 if (name.equals("type")) { 1556 this.type = new CodeableConcept(); 1557 return this.type; 1558 } 1559 else if (name.equals("component")) { 1560 this.component = new Identifier(); 1561 return this.component; 1562 } 1563 else if (name.equals("installDate")) { 1564 throw new FHIRException("Cannot call addChild on a singleton property Device.version.installDate"); 1565 } 1566 else if (name.equals("value")) { 1567 throw new FHIRException("Cannot call addChild on a singleton property Device.version.value"); 1568 } 1569 else 1570 return super.addChild(name); 1571 } 1572 1573 public DeviceVersionComponent copy() { 1574 DeviceVersionComponent dst = new DeviceVersionComponent(); 1575 copyValues(dst); 1576 return dst; 1577 } 1578 1579 public void copyValues(DeviceVersionComponent dst) { 1580 super.copyValues(dst); 1581 dst.type = type == null ? null : type.copy(); 1582 dst.component = component == null ? null : component.copy(); 1583 dst.installDate = installDate == null ? null : installDate.copy(); 1584 dst.value = value == null ? null : value.copy(); 1585 } 1586 1587 @Override 1588 public boolean equalsDeep(Base other_) { 1589 if (!super.equalsDeep(other_)) 1590 return false; 1591 if (!(other_ instanceof DeviceVersionComponent)) 1592 return false; 1593 DeviceVersionComponent o = (DeviceVersionComponent) other_; 1594 return compareDeep(type, o.type, true) && compareDeep(component, o.component, true) && compareDeep(installDate, o.installDate, true) 1595 && compareDeep(value, o.value, true); 1596 } 1597 1598 @Override 1599 public boolean equalsShallow(Base other_) { 1600 if (!super.equalsShallow(other_)) 1601 return false; 1602 if (!(other_ instanceof DeviceVersionComponent)) 1603 return false; 1604 DeviceVersionComponent o = (DeviceVersionComponent) other_; 1605 return compareValues(installDate, o.installDate, true) && compareValues(value, o.value, true); 1606 } 1607 1608 public boolean isEmpty() { 1609 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, component, installDate 1610 , value); 1611 } 1612 1613 public String fhirType() { 1614 return "Device.version"; 1615 1616 } 1617 1618 } 1619 1620 @Block() 1621 public static class DeviceConformsToComponent extends BackboneElement implements IBaseBackboneElement { 1622 /** 1623 * Describes the type of the standard, specification, or formal guidance. 1624 */ 1625 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1626 @Description(shortDefinition="Describes the common type of the standard, specification, or formal guidance. communication | performance | measurement", formalDefinition="Describes the type of the standard, specification, or formal guidance." ) 1627 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-specification-category") 1628 protected CodeableConcept category; 1629 1630 /** 1631 * Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres. 1632 */ 1633 @Child(name = "specification", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1634 @Description(shortDefinition="Identifies the standard, specification, or formal guidance that the device adheres to", formalDefinition="Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres." ) 1635 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-specification-type") 1636 protected CodeableConcept specification; 1637 1638 /** 1639 * Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label. 1640 */ 1641 @Child(name = "version", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1642 @Description(shortDefinition="Specific form or variant of the standard", formalDefinition="Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label." ) 1643 protected StringType version; 1644 1645 private static final long serialVersionUID = 1592712180L; 1646 1647 /** 1648 * Constructor 1649 */ 1650 public DeviceConformsToComponent() { 1651 super(); 1652 } 1653 1654 /** 1655 * Constructor 1656 */ 1657 public DeviceConformsToComponent(CodeableConcept specification) { 1658 super(); 1659 this.setSpecification(specification); 1660 } 1661 1662 /** 1663 * @return {@link #category} (Describes the type of the standard, specification, or formal guidance.) 1664 */ 1665 public CodeableConcept getCategory() { 1666 if (this.category == null) 1667 if (Configuration.errorOnAutoCreate()) 1668 throw new Error("Attempt to auto-create DeviceConformsToComponent.category"); 1669 else if (Configuration.doAutoCreate()) 1670 this.category = new CodeableConcept(); // cc 1671 return this.category; 1672 } 1673 1674 public boolean hasCategory() { 1675 return this.category != null && !this.category.isEmpty(); 1676 } 1677 1678 /** 1679 * @param value {@link #category} (Describes the type of the standard, specification, or formal guidance.) 1680 */ 1681 public DeviceConformsToComponent setCategory(CodeableConcept value) { 1682 this.category = value; 1683 return this; 1684 } 1685 1686 /** 1687 * @return {@link #specification} (Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.) 1688 */ 1689 public CodeableConcept getSpecification() { 1690 if (this.specification == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create DeviceConformsToComponent.specification"); 1693 else if (Configuration.doAutoCreate()) 1694 this.specification = new CodeableConcept(); // cc 1695 return this.specification; 1696 } 1697 1698 public boolean hasSpecification() { 1699 return this.specification != null && !this.specification.isEmpty(); 1700 } 1701 1702 /** 1703 * @param value {@link #specification} (Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.) 1704 */ 1705 public DeviceConformsToComponent setSpecification(CodeableConcept value) { 1706 this.specification = value; 1707 return this; 1708 } 1709 1710 /** 1711 * @return {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1712 */ 1713 public StringType getVersionElement() { 1714 if (this.version == null) 1715 if (Configuration.errorOnAutoCreate()) 1716 throw new Error("Attempt to auto-create DeviceConformsToComponent.version"); 1717 else if (Configuration.doAutoCreate()) 1718 this.version = new StringType(); // bb 1719 return this.version; 1720 } 1721 1722 public boolean hasVersionElement() { 1723 return this.version != null && !this.version.isEmpty(); 1724 } 1725 1726 public boolean hasVersion() { 1727 return this.version != null && !this.version.isEmpty(); 1728 } 1729 1730 /** 1731 * @param value {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1732 */ 1733 public DeviceConformsToComponent setVersionElement(StringType value) { 1734 this.version = value; 1735 return this; 1736 } 1737 1738 /** 1739 * @return Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label. 1740 */ 1741 public String getVersion() { 1742 return this.version == null ? null : this.version.getValue(); 1743 } 1744 1745 /** 1746 * @param value Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label. 1747 */ 1748 public DeviceConformsToComponent setVersion(String value) { 1749 if (Utilities.noString(value)) 1750 this.version = null; 1751 else { 1752 if (this.version == null) 1753 this.version = new StringType(); 1754 this.version.setValue(value); 1755 } 1756 return this; 1757 } 1758 1759 protected void listChildren(List<Property> children) { 1760 super.listChildren(children); 1761 children.add(new Property("category", "CodeableConcept", "Describes the type of the standard, specification, or formal guidance.", 0, 1, category)); 1762 children.add(new Property("specification", "CodeableConcept", "Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.", 0, 1, specification)); 1763 children.add(new Property("version", "string", "Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.", 0, 1, version)); 1764 } 1765 1766 @Override 1767 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1768 switch (_hash) { 1769 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Describes the type of the standard, specification, or formal guidance.", 0, 1, category); 1770 case 1307197699: /*specification*/ return new Property("specification", "CodeableConcept", "Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.", 0, 1, specification); 1771 case 351608024: /*version*/ return new Property("version", "string", "Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.", 0, 1, version); 1772 default: return super.getNamedProperty(_hash, _name, _checkValid); 1773 } 1774 1775 } 1776 1777 @Override 1778 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1779 switch (hash) { 1780 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1781 case 1307197699: /*specification*/ return this.specification == null ? new Base[0] : new Base[] {this.specification}; // CodeableConcept 1782 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1783 default: return super.getProperty(hash, name, checkValid); 1784 } 1785 1786 } 1787 1788 @Override 1789 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1790 switch (hash) { 1791 case 50511102: // category 1792 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1793 return value; 1794 case 1307197699: // specification 1795 this.specification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1796 return value; 1797 case 351608024: // version 1798 this.version = TypeConvertor.castToString(value); // StringType 1799 return value; 1800 default: return super.setProperty(hash, name, value); 1801 } 1802 1803 } 1804 1805 @Override 1806 public Base setProperty(String name, Base value) throws FHIRException { 1807 if (name.equals("category")) { 1808 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1809 } else if (name.equals("specification")) { 1810 this.specification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1811 } else if (name.equals("version")) { 1812 this.version = TypeConvertor.castToString(value); // StringType 1813 } else 1814 return super.setProperty(name, value); 1815 return value; 1816 } 1817 1818 @Override 1819 public void removeChild(String name, Base value) throws FHIRException { 1820 if (name.equals("category")) { 1821 this.category = null; 1822 } else if (name.equals("specification")) { 1823 this.specification = null; 1824 } else if (name.equals("version")) { 1825 this.version = null; 1826 } else 1827 super.removeChild(name, value); 1828 1829 } 1830 1831 @Override 1832 public Base makeProperty(int hash, String name) throws FHIRException { 1833 switch (hash) { 1834 case 50511102: return getCategory(); 1835 case 1307197699: return getSpecification(); 1836 case 351608024: return getVersionElement(); 1837 default: return super.makeProperty(hash, name); 1838 } 1839 1840 } 1841 1842 @Override 1843 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1844 switch (hash) { 1845 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1846 case 1307197699: /*specification*/ return new String[] {"CodeableConcept"}; 1847 case 351608024: /*version*/ return new String[] {"string"}; 1848 default: return super.getTypesForProperty(hash, name); 1849 } 1850 1851 } 1852 1853 @Override 1854 public Base addChild(String name) throws FHIRException { 1855 if (name.equals("category")) { 1856 this.category = new CodeableConcept(); 1857 return this.category; 1858 } 1859 else if (name.equals("specification")) { 1860 this.specification = new CodeableConcept(); 1861 return this.specification; 1862 } 1863 else if (name.equals("version")) { 1864 throw new FHIRException("Cannot call addChild on a singleton property Device.conformsTo.version"); 1865 } 1866 else 1867 return super.addChild(name); 1868 } 1869 1870 public DeviceConformsToComponent copy() { 1871 DeviceConformsToComponent dst = new DeviceConformsToComponent(); 1872 copyValues(dst); 1873 return dst; 1874 } 1875 1876 public void copyValues(DeviceConformsToComponent dst) { 1877 super.copyValues(dst); 1878 dst.category = category == null ? null : category.copy(); 1879 dst.specification = specification == null ? null : specification.copy(); 1880 dst.version = version == null ? null : version.copy(); 1881 } 1882 1883 @Override 1884 public boolean equalsDeep(Base other_) { 1885 if (!super.equalsDeep(other_)) 1886 return false; 1887 if (!(other_ instanceof DeviceConformsToComponent)) 1888 return false; 1889 DeviceConformsToComponent o = (DeviceConformsToComponent) other_; 1890 return compareDeep(category, o.category, true) && compareDeep(specification, o.specification, true) 1891 && compareDeep(version, o.version, true); 1892 } 1893 1894 @Override 1895 public boolean equalsShallow(Base other_) { 1896 if (!super.equalsShallow(other_)) 1897 return false; 1898 if (!(other_ instanceof DeviceConformsToComponent)) 1899 return false; 1900 DeviceConformsToComponent o = (DeviceConformsToComponent) other_; 1901 return compareValues(version, o.version, true); 1902 } 1903 1904 public boolean isEmpty() { 1905 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, specification, version 1906 ); 1907 } 1908 1909 public String fhirType() { 1910 return "Device.conformsTo"; 1911 1912 } 1913 1914 } 1915 1916 @Block() 1917 public static class DevicePropertyComponent extends BackboneElement implements IBaseBackboneElement { 1918 /** 1919 * Code that specifies the property, such as resolution, color, size, being represented. 1920 */ 1921 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1922 @Description(shortDefinition="Code that specifies the property being represented", formalDefinition="Code that specifies the property, such as resolution, color, size, being represented." ) 1923 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-property-type") 1924 protected CodeableConcept type; 1925 1926 /** 1927 * The value of the property specified by the associated property.type code. 1928 */ 1929 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Attachment.class}, order=2, min=1, max=1, modifier=false, summary=false) 1930 @Description(shortDefinition="Value of the property", formalDefinition="The value of the property specified by the associated property.type code." ) 1931 protected DataType value; 1932 1933 private static final long serialVersionUID = -1659186716L; 1934 1935 /** 1936 * Constructor 1937 */ 1938 public DevicePropertyComponent() { 1939 super(); 1940 } 1941 1942 /** 1943 * Constructor 1944 */ 1945 public DevicePropertyComponent(CodeableConcept type, DataType value) { 1946 super(); 1947 this.setType(type); 1948 this.setValue(value); 1949 } 1950 1951 /** 1952 * @return {@link #type} (Code that specifies the property, such as resolution, color, size, being represented.) 1953 */ 1954 public CodeableConcept getType() { 1955 if (this.type == null) 1956 if (Configuration.errorOnAutoCreate()) 1957 throw new Error("Attempt to auto-create DevicePropertyComponent.type"); 1958 else if (Configuration.doAutoCreate()) 1959 this.type = new CodeableConcept(); // cc 1960 return this.type; 1961 } 1962 1963 public boolean hasType() { 1964 return this.type != null && !this.type.isEmpty(); 1965 } 1966 1967 /** 1968 * @param value {@link #type} (Code that specifies the property, such as resolution, color, size, being represented.) 1969 */ 1970 public DevicePropertyComponent setType(CodeableConcept value) { 1971 this.type = value; 1972 return this; 1973 } 1974 1975 /** 1976 * @return {@link #value} (The value of the property specified by the associated property.type code.) 1977 */ 1978 public DataType getValue() { 1979 return this.value; 1980 } 1981 1982 /** 1983 * @return {@link #value} (The value of the property specified by the associated property.type code.) 1984 */ 1985 public Quantity getValueQuantity() throws FHIRException { 1986 if (this.value == null) 1987 this.value = new Quantity(); 1988 if (!(this.value instanceof Quantity)) 1989 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1990 return (Quantity) this.value; 1991 } 1992 1993 public boolean hasValueQuantity() { 1994 return this != null && this.value instanceof Quantity; 1995 } 1996 1997 /** 1998 * @return {@link #value} (The value of the property specified by the associated property.type code.) 1999 */ 2000 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2001 if (this.value == null) 2002 this.value = new CodeableConcept(); 2003 if (!(this.value instanceof CodeableConcept)) 2004 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2005 return (CodeableConcept) this.value; 2006 } 2007 2008 public boolean hasValueCodeableConcept() { 2009 return this != null && this.value instanceof CodeableConcept; 2010 } 2011 2012 /** 2013 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2014 */ 2015 public StringType getValueStringType() throws FHIRException { 2016 if (this.value == null) 2017 this.value = new StringType(); 2018 if (!(this.value instanceof StringType)) 2019 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2020 return (StringType) this.value; 2021 } 2022 2023 public boolean hasValueStringType() { 2024 return this != null && this.value instanceof StringType; 2025 } 2026 2027 /** 2028 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2029 */ 2030 public BooleanType getValueBooleanType() throws FHIRException { 2031 if (this.value == null) 2032 this.value = new BooleanType(); 2033 if (!(this.value instanceof BooleanType)) 2034 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2035 return (BooleanType) this.value; 2036 } 2037 2038 public boolean hasValueBooleanType() { 2039 return this != null && this.value instanceof BooleanType; 2040 } 2041 2042 /** 2043 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2044 */ 2045 public IntegerType getValueIntegerType() throws FHIRException { 2046 if (this.value == null) 2047 this.value = new IntegerType(); 2048 if (!(this.value instanceof IntegerType)) 2049 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2050 return (IntegerType) this.value; 2051 } 2052 2053 public boolean hasValueIntegerType() { 2054 return this != null && this.value instanceof IntegerType; 2055 } 2056 2057 /** 2058 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2059 */ 2060 public Range getValueRange() throws FHIRException { 2061 if (this.value == null) 2062 this.value = new Range(); 2063 if (!(this.value instanceof Range)) 2064 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2065 return (Range) this.value; 2066 } 2067 2068 public boolean hasValueRange() { 2069 return this != null && this.value instanceof Range; 2070 } 2071 2072 /** 2073 * @return {@link #value} (The value of the property specified by the associated property.type code.) 2074 */ 2075 public Attachment getValueAttachment() throws FHIRException { 2076 if (this.value == null) 2077 this.value = new Attachment(); 2078 if (!(this.value instanceof Attachment)) 2079 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 2080 return (Attachment) this.value; 2081 } 2082 2083 public boolean hasValueAttachment() { 2084 return this != null && this.value instanceof Attachment; 2085 } 2086 2087 public boolean hasValue() { 2088 return this.value != null && !this.value.isEmpty(); 2089 } 2090 2091 /** 2092 * @param value {@link #value} (The value of the property specified by the associated property.type code.) 2093 */ 2094 public DevicePropertyComponent setValue(DataType value) { 2095 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Attachment)) 2096 throw new FHIRException("Not the right type for Device.property.value[x]: "+value.fhirType()); 2097 this.value = value; 2098 return this; 2099 } 2100 2101 protected void listChildren(List<Property> children) { 2102 super.listChildren(children); 2103 children.add(new Property("type", "CodeableConcept", "Code that specifies the property, such as resolution, color, size, being represented.", 0, 1, type)); 2104 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value)); 2105 } 2106 2107 @Override 2108 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2109 switch (_hash) { 2110 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code that specifies the property, such as resolution, color, size, being represented.", 0, 1, type); 2111 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 2112 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 2113 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the property specified by the associated property.type code.", 0, 1, value); 2114 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the property specified by the associated property.type code.", 0, 1, value); 2115 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the property specified by the associated property.type code.", 0, 1, value); 2116 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the property specified by the associated property.type code.", 0, 1, value); 2117 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the property specified by the associated property.type code.", 0, 1, value); 2118 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the property specified by the associated property.type code.", 0, 1, value); 2119 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 2120 default: return super.getNamedProperty(_hash, _name, _checkValid); 2121 } 2122 2123 } 2124 2125 @Override 2126 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2127 switch (hash) { 2128 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2129 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2130 default: return super.getProperty(hash, name, checkValid); 2131 } 2132 2133 } 2134 2135 @Override 2136 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2137 switch (hash) { 2138 case 3575610: // type 2139 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2140 return value; 2141 case 111972721: // value 2142 this.value = TypeConvertor.castToType(value); // DataType 2143 return value; 2144 default: return super.setProperty(hash, name, value); 2145 } 2146 2147 } 2148 2149 @Override 2150 public Base setProperty(String name, Base value) throws FHIRException { 2151 if (name.equals("type")) { 2152 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2153 } else if (name.equals("value[x]")) { 2154 this.value = TypeConvertor.castToType(value); // DataType 2155 } else 2156 return super.setProperty(name, value); 2157 return value; 2158 } 2159 2160 @Override 2161 public void removeChild(String name, Base value) throws FHIRException { 2162 if (name.equals("type")) { 2163 this.type = null; 2164 } else if (name.equals("value[x]")) { 2165 this.value = null; 2166 } else 2167 super.removeChild(name, value); 2168 2169 } 2170 2171 @Override 2172 public Base makeProperty(int hash, String name) throws FHIRException { 2173 switch (hash) { 2174 case 3575610: return getType(); 2175 case -1410166417: return getValue(); 2176 case 111972721: return getValue(); 2177 default: return super.makeProperty(hash, name); 2178 } 2179 2180 } 2181 2182 @Override 2183 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2184 switch (hash) { 2185 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2186 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Attachment"}; 2187 default: return super.getTypesForProperty(hash, name); 2188 } 2189 2190 } 2191 2192 @Override 2193 public Base addChild(String name) throws FHIRException { 2194 if (name.equals("type")) { 2195 this.type = new CodeableConcept(); 2196 return this.type; 2197 } 2198 else if (name.equals("valueQuantity")) { 2199 this.value = new Quantity(); 2200 return this.value; 2201 } 2202 else if (name.equals("valueCodeableConcept")) { 2203 this.value = new CodeableConcept(); 2204 return this.value; 2205 } 2206 else if (name.equals("valueString")) { 2207 this.value = new StringType(); 2208 return this.value; 2209 } 2210 else if (name.equals("valueBoolean")) { 2211 this.value = new BooleanType(); 2212 return this.value; 2213 } 2214 else if (name.equals("valueInteger")) { 2215 this.value = new IntegerType(); 2216 return this.value; 2217 } 2218 else if (name.equals("valueRange")) { 2219 this.value = new Range(); 2220 return this.value; 2221 } 2222 else if (name.equals("valueAttachment")) { 2223 this.value = new Attachment(); 2224 return this.value; 2225 } 2226 else 2227 return super.addChild(name); 2228 } 2229 2230 public DevicePropertyComponent copy() { 2231 DevicePropertyComponent dst = new DevicePropertyComponent(); 2232 copyValues(dst); 2233 return dst; 2234 } 2235 2236 public void copyValues(DevicePropertyComponent dst) { 2237 super.copyValues(dst); 2238 dst.type = type == null ? null : type.copy(); 2239 dst.value = value == null ? null : value.copy(); 2240 } 2241 2242 @Override 2243 public boolean equalsDeep(Base other_) { 2244 if (!super.equalsDeep(other_)) 2245 return false; 2246 if (!(other_ instanceof DevicePropertyComponent)) 2247 return false; 2248 DevicePropertyComponent o = (DevicePropertyComponent) other_; 2249 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2250 } 2251 2252 @Override 2253 public boolean equalsShallow(Base other_) { 2254 if (!super.equalsShallow(other_)) 2255 return false; 2256 if (!(other_ instanceof DevicePropertyComponent)) 2257 return false; 2258 DevicePropertyComponent o = (DevicePropertyComponent) other_; 2259 return true; 2260 } 2261 2262 public boolean isEmpty() { 2263 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2264 } 2265 2266 public String fhirType() { 2267 return "Device.property"; 2268 2269 } 2270 2271 } 2272 2273 /** 2274 * Unique instance identifiers assigned to a device by manufacturers other organizations or owners. 2275 */ 2276 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2277 @Description(shortDefinition="Instance identifier", formalDefinition="Unique instance identifiers assigned to a device by manufacturers other organizations or owners." ) 2278 protected List<Identifier> identifier; 2279 2280 /** 2281 * The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name. 2282 */ 2283 @Child(name = "displayName", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2284 @Description(shortDefinition="The name used to display by default when the device is referenced", formalDefinition="The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name." ) 2285 protected StringType displayName; 2286 2287 /** 2288 * The reference to the definition for the device. 2289 */ 2290 @Child(name = "definition", type = {CodeableReference.class}, order=2, min=0, max=1, modifier=false, summary=false) 2291 @Description(shortDefinition="The reference to the definition for the device", formalDefinition="The reference to the definition for the device." ) 2292 protected CodeableReference definition; 2293 2294 /** 2295 * Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold. 2296 */ 2297 @Child(name = "udiCarrier", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2298 @Description(shortDefinition="Unique Device Identifier (UDI) Barcode string", formalDefinition="Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold." ) 2299 protected List<DeviceUdiCarrierComponent> udiCarrier; 2300 2301 /** 2302 * The Device record status. This is not the status of the device like availability. 2303 */ 2304 @Child(name = "status", type = {CodeType.class}, order=4, min=0, max=1, modifier=true, summary=true) 2305 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="The Device record status. This is not the status of the device like availability." ) 2306 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-status") 2307 protected Enumeration<FHIRDeviceStatus> status; 2308 2309 /** 2310 * The availability of the device. 2311 */ 2312 @Child(name = "availabilityStatus", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 2313 @Description(shortDefinition="lost | damaged | destroyed | available", formalDefinition="The availability of the device." ) 2314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-availability-status") 2315 protected CodeableConcept availabilityStatus; 2316 2317 /** 2318 * An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled. 2319 */ 2320 @Child(name = "biologicalSourceEvent", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 2321 @Description(shortDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled", formalDefinition="An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled." ) 2322 protected Identifier biologicalSourceEvent; 2323 2324 /** 2325 * A name of the manufacturer or entity legally responsible for the device. 2326 */ 2327 @Child(name = "manufacturer", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 2328 @Description(shortDefinition="Name of device manufacturer", formalDefinition="A name of the manufacturer or entity legally responsible for the device." ) 2329 protected StringType manufacturer; 2330 2331 /** 2332 * The date and time when the device was manufactured. 2333 */ 2334 @Child(name = "manufactureDate", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2335 @Description(shortDefinition="Date when the device was made", formalDefinition="The date and time when the device was manufactured." ) 2336 protected DateTimeType manufactureDate; 2337 2338 /** 2339 * The date and time beyond which this device is no longer valid or should not be used (if applicable). 2340 */ 2341 @Child(name = "expirationDate", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 2342 @Description(shortDefinition="Date and time of expiry of this device (if applicable)", formalDefinition="The date and time beyond which this device is no longer valid or should not be used (if applicable)." ) 2343 protected DateTimeType expirationDate; 2344 2345 /** 2346 * Lot number assigned by the manufacturer. 2347 */ 2348 @Child(name = "lotNumber", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 2349 @Description(shortDefinition="Lot number of manufacture", formalDefinition="Lot number assigned by the manufacturer." ) 2350 protected StringType lotNumber; 2351 2352 /** 2353 * The serial number assigned by the organization when the device was manufactured. 2354 */ 2355 @Child(name = "serialNumber", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2356 @Description(shortDefinition="Serial number assigned by the manufacturer", formalDefinition="The serial number assigned by the organization when the device was manufactured." ) 2357 protected StringType serialNumber; 2358 2359 /** 2360 * This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition. 2361 */ 2362 @Child(name = "name", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2363 @Description(shortDefinition="The name or names of the device as known to the manufacturer and/or patient", formalDefinition="This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition." ) 2364 protected List<DeviceNameComponent> name; 2365 2366 /** 2367 * The manufacturer's model number for the device. 2368 */ 2369 @Child(name = "modelNumber", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 2370 @Description(shortDefinition="The manufacturer's model number for the device", formalDefinition="The manufacturer's model number for the device." ) 2371 protected StringType modelNumber; 2372 2373 /** 2374 * The part number or catalog number of the device. 2375 */ 2376 @Child(name = "partNumber", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 2377 @Description(shortDefinition="The part number or catalog number of the device", formalDefinition="The part number or catalog number of the device." ) 2378 protected StringType partNumber; 2379 2380 /** 2381 * Devices may be associated with one or more categories. 2382 */ 2383 @Child(name = "category", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2384 @Description(shortDefinition="Indicates a high-level grouping of the device", formalDefinition="Devices may be associated with one or more categories." ) 2385 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-category") 2386 protected List<CodeableConcept> category; 2387 2388 /** 2389 * The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device. 2390 */ 2391 @Child(name = "type", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2392 @Description(shortDefinition="The kind or type of device", formalDefinition="The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device." ) 2393 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-type") 2394 protected List<CodeableConcept> type; 2395 2396 /** 2397 * The actual design of the device or software version running on the device. 2398 */ 2399 @Child(name = "version", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2400 @Description(shortDefinition="The actual design of the device or software version running on the device", formalDefinition="The actual design of the device or software version running on the device." ) 2401 protected List<DeviceVersionComponent> version; 2402 2403 /** 2404 * Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards. 2405 */ 2406 @Child(name = "conformsTo", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2407 @Description(shortDefinition="Identifies the standards, specifications, or formal guidances for the capabilities supported by the device", formalDefinition="Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards." ) 2408 protected List<DeviceConformsToComponent> conformsTo; 2409 2410 /** 2411 * Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes. 2412 */ 2413 @Child(name = "property", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2414 @Description(shortDefinition="Inherent, essentially fixed, characteristics of the device. e.g., time properties, size, material, etc.", formalDefinition="Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes." ) 2415 protected List<DevicePropertyComponent> property; 2416 2417 /** 2418 * The designated condition for performing a task with the device. 2419 */ 2420 @Child(name = "mode", type = {CodeableConcept.class}, order=20, min=0, max=1, modifier=false, summary=false) 2421 @Description(shortDefinition="The designated condition for performing a task", formalDefinition="The designated condition for performing a task with the device." ) 2422 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-operation-mode") 2423 protected CodeableConcept mode; 2424 2425 /** 2426 * The series of occurrences that repeats during the operation of the device. 2427 */ 2428 @Child(name = "cycle", type = {Count.class}, order=21, min=0, max=1, modifier=false, summary=false) 2429 @Description(shortDefinition="The series of occurrences that repeats during the operation of the device", formalDefinition="The series of occurrences that repeats during the operation of the device." ) 2430 protected Count cycle; 2431 2432 /** 2433 * A measurement of time during the device's operation (e.g., days, hours, mins, etc.). 2434 */ 2435 @Child(name = "duration", type = {Duration.class}, order=22, min=0, max=1, modifier=false, summary=false) 2436 @Description(shortDefinition="A measurement of time during the device's operation (e.g., days, hours, mins, etc.)", formalDefinition="A measurement of time during the device's operation (e.g., days, hours, mins, etc.)." ) 2437 protected Duration duration; 2438 2439 /** 2440 * An organization that is responsible for the provision and ongoing maintenance of the device. 2441 */ 2442 @Child(name = "owner", type = {Organization.class}, order=23, min=0, max=1, modifier=false, summary=false) 2443 @Description(shortDefinition="Organization responsible for device", formalDefinition="An organization that is responsible for the provision and ongoing maintenance of the device." ) 2444 protected Reference owner; 2445 2446 /** 2447 * Contact details for an organization or a particular human that is responsible for the device. 2448 */ 2449 @Child(name = "contact", type = {ContactPoint.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2450 @Description(shortDefinition="Details for human/organization for support", formalDefinition="Contact details for an organization or a particular human that is responsible for the device." ) 2451 protected List<ContactPoint> contact; 2452 2453 /** 2454 * The place where the device can be found. 2455 */ 2456 @Child(name = "location", type = {Location.class}, order=25, min=0, max=1, modifier=false, summary=false) 2457 @Description(shortDefinition="Where the device is found", formalDefinition="The place where the device can be found." ) 2458 protected Reference location; 2459 2460 /** 2461 * A network address on which the device may be contacted directly. 2462 */ 2463 @Child(name = "url", type = {UriType.class}, order=26, min=0, max=1, modifier=false, summary=false) 2464 @Description(shortDefinition="Network address to contact device", formalDefinition="A network address on which the device may be contacted directly." ) 2465 protected UriType url; 2466 2467 /** 2468 * Technical endpoints providing access to services provided by the device defined at this resource. 2469 */ 2470 @Child(name = "endpoint", type = {Endpoint.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2471 @Description(shortDefinition="Technical endpoints providing access to electronic services provided by the device", formalDefinition="Technical endpoints providing access to services provided by the device defined at this resource." ) 2472 protected List<Reference> endpoint; 2473 2474 /** 2475 * The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data). 2476 */ 2477 @Child(name = "gateway", type = {CodeableReference.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2478 @Description(shortDefinition="Linked device acting as a communication/data collector, translator or controller", formalDefinition="The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data)." ) 2479 protected List<CodeableReference> gateway; 2480 2481 /** 2482 * Descriptive information, usage information or implantation information that is not captured in an existing element. 2483 */ 2484 @Child(name = "note", type = {Annotation.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2485 @Description(shortDefinition="Device notes and comments", formalDefinition="Descriptive information, usage information or implantation information that is not captured in an existing element." ) 2486 protected List<Annotation> note; 2487 2488 /** 2489 * Provides additional safety characteristics about a medical device. For example devices containing latex. 2490 */ 2491 @Child(name = "safety", type = {CodeableConcept.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2492 @Description(shortDefinition="Safety Characteristics of Device", formalDefinition="Provides additional safety characteristics about a medical device. For example devices containing latex." ) 2493 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-safety") 2494 protected List<CodeableConcept> safety; 2495 2496 /** 2497 * The higher level or encompassing device that this device is a logical part of. 2498 */ 2499 @Child(name = "parent", type = {Device.class}, order=31, min=0, max=1, modifier=false, summary=false) 2500 @Description(shortDefinition="The higher level or encompassing device that this device is a logical part of", formalDefinition="The higher level or encompassing device that this device is a logical part of." ) 2501 protected Reference parent; 2502 2503 private static final long serialVersionUID = 2120085847L; 2504 2505 /** 2506 * Constructor 2507 */ 2508 public Device() { 2509 super(); 2510 } 2511 2512 /** 2513 * @return {@link #identifier} (Unique instance identifiers assigned to a device by manufacturers other organizations or owners.) 2514 */ 2515 public List<Identifier> getIdentifier() { 2516 if (this.identifier == null) 2517 this.identifier = new ArrayList<Identifier>(); 2518 return this.identifier; 2519 } 2520 2521 /** 2522 * @return Returns a reference to <code>this</code> for easy method chaining 2523 */ 2524 public Device setIdentifier(List<Identifier> theIdentifier) { 2525 this.identifier = theIdentifier; 2526 return this; 2527 } 2528 2529 public boolean hasIdentifier() { 2530 if (this.identifier == null) 2531 return false; 2532 for (Identifier item : this.identifier) 2533 if (!item.isEmpty()) 2534 return true; 2535 return false; 2536 } 2537 2538 public Identifier addIdentifier() { //3 2539 Identifier t = new Identifier(); 2540 if (this.identifier == null) 2541 this.identifier = new ArrayList<Identifier>(); 2542 this.identifier.add(t); 2543 return t; 2544 } 2545 2546 public Device addIdentifier(Identifier t) { //3 2547 if (t == null) 2548 return this; 2549 if (this.identifier == null) 2550 this.identifier = new ArrayList<Identifier>(); 2551 this.identifier.add(t); 2552 return this; 2553 } 2554 2555 /** 2556 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2557 */ 2558 public Identifier getIdentifierFirstRep() { 2559 if (getIdentifier().isEmpty()) { 2560 addIdentifier(); 2561 } 2562 return getIdentifier().get(0); 2563 } 2564 2565 /** 2566 * @return {@link #displayName} (The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.). This is the underlying object with id, value and extensions. The accessor "getDisplayName" gives direct access to the value 2567 */ 2568 public StringType getDisplayNameElement() { 2569 if (this.displayName == null) 2570 if (Configuration.errorOnAutoCreate()) 2571 throw new Error("Attempt to auto-create Device.displayName"); 2572 else if (Configuration.doAutoCreate()) 2573 this.displayName = new StringType(); // bb 2574 return this.displayName; 2575 } 2576 2577 public boolean hasDisplayNameElement() { 2578 return this.displayName != null && !this.displayName.isEmpty(); 2579 } 2580 2581 public boolean hasDisplayName() { 2582 return this.displayName != null && !this.displayName.isEmpty(); 2583 } 2584 2585 /** 2586 * @param value {@link #displayName} (The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.). This is the underlying object with id, value and extensions. The accessor "getDisplayName" gives direct access to the value 2587 */ 2588 public Device setDisplayNameElement(StringType value) { 2589 this.displayName = value; 2590 return this; 2591 } 2592 2593 /** 2594 * @return The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name. 2595 */ 2596 public String getDisplayName() { 2597 return this.displayName == null ? null : this.displayName.getValue(); 2598 } 2599 2600 /** 2601 * @param value The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name. 2602 */ 2603 public Device setDisplayName(String value) { 2604 if (Utilities.noString(value)) 2605 this.displayName = null; 2606 else { 2607 if (this.displayName == null) 2608 this.displayName = new StringType(); 2609 this.displayName.setValue(value); 2610 } 2611 return this; 2612 } 2613 2614 /** 2615 * @return {@link #definition} (The reference to the definition for the device.) 2616 */ 2617 public CodeableReference getDefinition() { 2618 if (this.definition == null) 2619 if (Configuration.errorOnAutoCreate()) 2620 throw new Error("Attempt to auto-create Device.definition"); 2621 else if (Configuration.doAutoCreate()) 2622 this.definition = new CodeableReference(); // cc 2623 return this.definition; 2624 } 2625 2626 public boolean hasDefinition() { 2627 return this.definition != null && !this.definition.isEmpty(); 2628 } 2629 2630 /** 2631 * @param value {@link #definition} (The reference to the definition for the device.) 2632 */ 2633 public Device setDefinition(CodeableReference value) { 2634 this.definition = value; 2635 return this; 2636 } 2637 2638 /** 2639 * @return {@link #udiCarrier} (Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.) 2640 */ 2641 public List<DeviceUdiCarrierComponent> getUdiCarrier() { 2642 if (this.udiCarrier == null) 2643 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2644 return this.udiCarrier; 2645 } 2646 2647 /** 2648 * @return Returns a reference to <code>this</code> for easy method chaining 2649 */ 2650 public Device setUdiCarrier(List<DeviceUdiCarrierComponent> theUdiCarrier) { 2651 this.udiCarrier = theUdiCarrier; 2652 return this; 2653 } 2654 2655 public boolean hasUdiCarrier() { 2656 if (this.udiCarrier == null) 2657 return false; 2658 for (DeviceUdiCarrierComponent item : this.udiCarrier) 2659 if (!item.isEmpty()) 2660 return true; 2661 return false; 2662 } 2663 2664 public DeviceUdiCarrierComponent addUdiCarrier() { //3 2665 DeviceUdiCarrierComponent t = new DeviceUdiCarrierComponent(); 2666 if (this.udiCarrier == null) 2667 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2668 this.udiCarrier.add(t); 2669 return t; 2670 } 2671 2672 public Device addUdiCarrier(DeviceUdiCarrierComponent t) { //3 2673 if (t == null) 2674 return this; 2675 if (this.udiCarrier == null) 2676 this.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 2677 this.udiCarrier.add(t); 2678 return this; 2679 } 2680 2681 /** 2682 * @return The first repetition of repeating field {@link #udiCarrier}, creating it if it does not already exist {3} 2683 */ 2684 public DeviceUdiCarrierComponent getUdiCarrierFirstRep() { 2685 if (getUdiCarrier().isEmpty()) { 2686 addUdiCarrier(); 2687 } 2688 return getUdiCarrier().get(0); 2689 } 2690 2691 /** 2692 * @return {@link #status} (The Device record status. This is not the status of the device like availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2693 */ 2694 public Enumeration<FHIRDeviceStatus> getStatusElement() { 2695 if (this.status == null) 2696 if (Configuration.errorOnAutoCreate()) 2697 throw new Error("Attempt to auto-create Device.status"); 2698 else if (Configuration.doAutoCreate()) 2699 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); // bb 2700 return this.status; 2701 } 2702 2703 public boolean hasStatusElement() { 2704 return this.status != null && !this.status.isEmpty(); 2705 } 2706 2707 public boolean hasStatus() { 2708 return this.status != null && !this.status.isEmpty(); 2709 } 2710 2711 /** 2712 * @param value {@link #status} (The Device record status. This is not the status of the device like availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2713 */ 2714 public Device setStatusElement(Enumeration<FHIRDeviceStatus> value) { 2715 this.status = value; 2716 return this; 2717 } 2718 2719 /** 2720 * @return The Device record status. This is not the status of the device like availability. 2721 */ 2722 public FHIRDeviceStatus getStatus() { 2723 return this.status == null ? null : this.status.getValue(); 2724 } 2725 2726 /** 2727 * @param value The Device record status. This is not the status of the device like availability. 2728 */ 2729 public Device setStatus(FHIRDeviceStatus value) { 2730 if (value == null) 2731 this.status = null; 2732 else { 2733 if (this.status == null) 2734 this.status = new Enumeration<FHIRDeviceStatus>(new FHIRDeviceStatusEnumFactory()); 2735 this.status.setValue(value); 2736 } 2737 return this; 2738 } 2739 2740 /** 2741 * @return {@link #availabilityStatus} (The availability of the device.) 2742 */ 2743 public CodeableConcept getAvailabilityStatus() { 2744 if (this.availabilityStatus == null) 2745 if (Configuration.errorOnAutoCreate()) 2746 throw new Error("Attempt to auto-create Device.availabilityStatus"); 2747 else if (Configuration.doAutoCreate()) 2748 this.availabilityStatus = new CodeableConcept(); // cc 2749 return this.availabilityStatus; 2750 } 2751 2752 public boolean hasAvailabilityStatus() { 2753 return this.availabilityStatus != null && !this.availabilityStatus.isEmpty(); 2754 } 2755 2756 /** 2757 * @param value {@link #availabilityStatus} (The availability of the device.) 2758 */ 2759 public Device setAvailabilityStatus(CodeableConcept value) { 2760 this.availabilityStatus = value; 2761 return this; 2762 } 2763 2764 /** 2765 * @return {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 2766 */ 2767 public Identifier getBiologicalSourceEvent() { 2768 if (this.biologicalSourceEvent == null) 2769 if (Configuration.errorOnAutoCreate()) 2770 throw new Error("Attempt to auto-create Device.biologicalSourceEvent"); 2771 else if (Configuration.doAutoCreate()) 2772 this.biologicalSourceEvent = new Identifier(); // cc 2773 return this.biologicalSourceEvent; 2774 } 2775 2776 public boolean hasBiologicalSourceEvent() { 2777 return this.biologicalSourceEvent != null && !this.biologicalSourceEvent.isEmpty(); 2778 } 2779 2780 /** 2781 * @param value {@link #biologicalSourceEvent} (An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.) 2782 */ 2783 public Device setBiologicalSourceEvent(Identifier value) { 2784 this.biologicalSourceEvent = value; 2785 return this; 2786 } 2787 2788 /** 2789 * @return {@link #manufacturer} (A name of the manufacturer or entity legally responsible for the device.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value 2790 */ 2791 public StringType getManufacturerElement() { 2792 if (this.manufacturer == null) 2793 if (Configuration.errorOnAutoCreate()) 2794 throw new Error("Attempt to auto-create Device.manufacturer"); 2795 else if (Configuration.doAutoCreate()) 2796 this.manufacturer = new StringType(); // bb 2797 return this.manufacturer; 2798 } 2799 2800 public boolean hasManufacturerElement() { 2801 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2802 } 2803 2804 public boolean hasManufacturer() { 2805 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2806 } 2807 2808 /** 2809 * @param value {@link #manufacturer} (A name of the manufacturer or entity legally responsible for the device.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value 2810 */ 2811 public Device setManufacturerElement(StringType value) { 2812 this.manufacturer = value; 2813 return this; 2814 } 2815 2816 /** 2817 * @return A name of the manufacturer or entity legally responsible for the device. 2818 */ 2819 public String getManufacturer() { 2820 return this.manufacturer == null ? null : this.manufacturer.getValue(); 2821 } 2822 2823 /** 2824 * @param value A name of the manufacturer or entity legally responsible for the device. 2825 */ 2826 public Device setManufacturer(String value) { 2827 if (Utilities.noString(value)) 2828 this.manufacturer = null; 2829 else { 2830 if (this.manufacturer == null) 2831 this.manufacturer = new StringType(); 2832 this.manufacturer.setValue(value); 2833 } 2834 return this; 2835 } 2836 2837 /** 2838 * @return {@link #manufactureDate} (The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value 2839 */ 2840 public DateTimeType getManufactureDateElement() { 2841 if (this.manufactureDate == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create Device.manufactureDate"); 2844 else if (Configuration.doAutoCreate()) 2845 this.manufactureDate = new DateTimeType(); // bb 2846 return this.manufactureDate; 2847 } 2848 2849 public boolean hasManufactureDateElement() { 2850 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 2851 } 2852 2853 public boolean hasManufactureDate() { 2854 return this.manufactureDate != null && !this.manufactureDate.isEmpty(); 2855 } 2856 2857 /** 2858 * @param value {@link #manufactureDate} (The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value 2859 */ 2860 public Device setManufactureDateElement(DateTimeType value) { 2861 this.manufactureDate = value; 2862 return this; 2863 } 2864 2865 /** 2866 * @return The date and time when the device was manufactured. 2867 */ 2868 public Date getManufactureDate() { 2869 return this.manufactureDate == null ? null : this.manufactureDate.getValue(); 2870 } 2871 2872 /** 2873 * @param value The date and time when the device was manufactured. 2874 */ 2875 public Device setManufactureDate(Date value) { 2876 if (value == null) 2877 this.manufactureDate = null; 2878 else { 2879 if (this.manufactureDate == null) 2880 this.manufactureDate = new DateTimeType(); 2881 this.manufactureDate.setValue(value); 2882 } 2883 return this; 2884 } 2885 2886 /** 2887 * @return {@link #expirationDate} (The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2888 */ 2889 public DateTimeType getExpirationDateElement() { 2890 if (this.expirationDate == null) 2891 if (Configuration.errorOnAutoCreate()) 2892 throw new Error("Attempt to auto-create Device.expirationDate"); 2893 else if (Configuration.doAutoCreate()) 2894 this.expirationDate = new DateTimeType(); // bb 2895 return this.expirationDate; 2896 } 2897 2898 public boolean hasExpirationDateElement() { 2899 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2900 } 2901 2902 public boolean hasExpirationDate() { 2903 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2904 } 2905 2906 /** 2907 * @param value {@link #expirationDate} (The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2908 */ 2909 public Device setExpirationDateElement(DateTimeType value) { 2910 this.expirationDate = value; 2911 return this; 2912 } 2913 2914 /** 2915 * @return The date and time beyond which this device is no longer valid or should not be used (if applicable). 2916 */ 2917 public Date getExpirationDate() { 2918 return this.expirationDate == null ? null : this.expirationDate.getValue(); 2919 } 2920 2921 /** 2922 * @param value The date and time beyond which this device is no longer valid or should not be used (if applicable). 2923 */ 2924 public Device setExpirationDate(Date value) { 2925 if (value == null) 2926 this.expirationDate = null; 2927 else { 2928 if (this.expirationDate == null) 2929 this.expirationDate = new DateTimeType(); 2930 this.expirationDate.setValue(value); 2931 } 2932 return this; 2933 } 2934 2935 /** 2936 * @return {@link #lotNumber} (Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2937 */ 2938 public StringType getLotNumberElement() { 2939 if (this.lotNumber == null) 2940 if (Configuration.errorOnAutoCreate()) 2941 throw new Error("Attempt to auto-create Device.lotNumber"); 2942 else if (Configuration.doAutoCreate()) 2943 this.lotNumber = new StringType(); // bb 2944 return this.lotNumber; 2945 } 2946 2947 public boolean hasLotNumberElement() { 2948 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2949 } 2950 2951 public boolean hasLotNumber() { 2952 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2953 } 2954 2955 /** 2956 * @param value {@link #lotNumber} (Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2957 */ 2958 public Device setLotNumberElement(StringType value) { 2959 this.lotNumber = value; 2960 return this; 2961 } 2962 2963 /** 2964 * @return Lot number assigned by the manufacturer. 2965 */ 2966 public String getLotNumber() { 2967 return this.lotNumber == null ? null : this.lotNumber.getValue(); 2968 } 2969 2970 /** 2971 * @param value Lot number assigned by the manufacturer. 2972 */ 2973 public Device setLotNumber(String value) { 2974 if (Utilities.noString(value)) 2975 this.lotNumber = null; 2976 else { 2977 if (this.lotNumber == null) 2978 this.lotNumber = new StringType(); 2979 this.lotNumber.setValue(value); 2980 } 2981 return this; 2982 } 2983 2984 /** 2985 * @return {@link #serialNumber} (The serial number assigned by the organization when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getSerialNumber" gives direct access to the value 2986 */ 2987 public StringType getSerialNumberElement() { 2988 if (this.serialNumber == null) 2989 if (Configuration.errorOnAutoCreate()) 2990 throw new Error("Attempt to auto-create Device.serialNumber"); 2991 else if (Configuration.doAutoCreate()) 2992 this.serialNumber = new StringType(); // bb 2993 return this.serialNumber; 2994 } 2995 2996 public boolean hasSerialNumberElement() { 2997 return this.serialNumber != null && !this.serialNumber.isEmpty(); 2998 } 2999 3000 public boolean hasSerialNumber() { 3001 return this.serialNumber != null && !this.serialNumber.isEmpty(); 3002 } 3003 3004 /** 3005 * @param value {@link #serialNumber} (The serial number assigned by the organization when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getSerialNumber" gives direct access to the value 3006 */ 3007 public Device setSerialNumberElement(StringType value) { 3008 this.serialNumber = value; 3009 return this; 3010 } 3011 3012 /** 3013 * @return The serial number assigned by the organization when the device was manufactured. 3014 */ 3015 public String getSerialNumber() { 3016 return this.serialNumber == null ? null : this.serialNumber.getValue(); 3017 } 3018 3019 /** 3020 * @param value The serial number assigned by the organization when the device was manufactured. 3021 */ 3022 public Device setSerialNumber(String value) { 3023 if (Utilities.noString(value)) 3024 this.serialNumber = null; 3025 else { 3026 if (this.serialNumber == null) 3027 this.serialNumber = new StringType(); 3028 this.serialNumber.setValue(value); 3029 } 3030 return this; 3031 } 3032 3033 /** 3034 * @return {@link #name} (This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.) 3035 */ 3036 public List<DeviceNameComponent> getName() { 3037 if (this.name == null) 3038 this.name = new ArrayList<DeviceNameComponent>(); 3039 return this.name; 3040 } 3041 3042 /** 3043 * @return Returns a reference to <code>this</code> for easy method chaining 3044 */ 3045 public Device setName(List<DeviceNameComponent> theName) { 3046 this.name = theName; 3047 return this; 3048 } 3049 3050 public boolean hasName() { 3051 if (this.name == null) 3052 return false; 3053 for (DeviceNameComponent item : this.name) 3054 if (!item.isEmpty()) 3055 return true; 3056 return false; 3057 } 3058 3059 public DeviceNameComponent addName() { //3 3060 DeviceNameComponent t = new DeviceNameComponent(); 3061 if (this.name == null) 3062 this.name = new ArrayList<DeviceNameComponent>(); 3063 this.name.add(t); 3064 return t; 3065 } 3066 3067 public Device addName(DeviceNameComponent t) { //3 3068 if (t == null) 3069 return this; 3070 if (this.name == null) 3071 this.name = new ArrayList<DeviceNameComponent>(); 3072 this.name.add(t); 3073 return this; 3074 } 3075 3076 /** 3077 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 3078 */ 3079 public DeviceNameComponent getNameFirstRep() { 3080 if (getName().isEmpty()) { 3081 addName(); 3082 } 3083 return getName().get(0); 3084 } 3085 3086 /** 3087 * @return {@link #modelNumber} (The manufacturer's model number for the device.). This is the underlying object with id, value and extensions. The accessor "getModelNumber" gives direct access to the value 3088 */ 3089 public StringType getModelNumberElement() { 3090 if (this.modelNumber == null) 3091 if (Configuration.errorOnAutoCreate()) 3092 throw new Error("Attempt to auto-create Device.modelNumber"); 3093 else if (Configuration.doAutoCreate()) 3094 this.modelNumber = new StringType(); // bb 3095 return this.modelNumber; 3096 } 3097 3098 public boolean hasModelNumberElement() { 3099 return this.modelNumber != null && !this.modelNumber.isEmpty(); 3100 } 3101 3102 public boolean hasModelNumber() { 3103 return this.modelNumber != null && !this.modelNumber.isEmpty(); 3104 } 3105 3106 /** 3107 * @param value {@link #modelNumber} (The manufacturer's model number for the device.). This is the underlying object with id, value and extensions. The accessor "getModelNumber" gives direct access to the value 3108 */ 3109 public Device setModelNumberElement(StringType value) { 3110 this.modelNumber = value; 3111 return this; 3112 } 3113 3114 /** 3115 * @return The manufacturer's model number for the device. 3116 */ 3117 public String getModelNumber() { 3118 return this.modelNumber == null ? null : this.modelNumber.getValue(); 3119 } 3120 3121 /** 3122 * @param value The manufacturer's model number for the device. 3123 */ 3124 public Device setModelNumber(String value) { 3125 if (Utilities.noString(value)) 3126 this.modelNumber = null; 3127 else { 3128 if (this.modelNumber == null) 3129 this.modelNumber = new StringType(); 3130 this.modelNumber.setValue(value); 3131 } 3132 return this; 3133 } 3134 3135 /** 3136 * @return {@link #partNumber} (The part number or catalog number of the device.). This is the underlying object with id, value and extensions. The accessor "getPartNumber" gives direct access to the value 3137 */ 3138 public StringType getPartNumberElement() { 3139 if (this.partNumber == null) 3140 if (Configuration.errorOnAutoCreate()) 3141 throw new Error("Attempt to auto-create Device.partNumber"); 3142 else if (Configuration.doAutoCreate()) 3143 this.partNumber = new StringType(); // bb 3144 return this.partNumber; 3145 } 3146 3147 public boolean hasPartNumberElement() { 3148 return this.partNumber != null && !this.partNumber.isEmpty(); 3149 } 3150 3151 public boolean hasPartNumber() { 3152 return this.partNumber != null && !this.partNumber.isEmpty(); 3153 } 3154 3155 /** 3156 * @param value {@link #partNumber} (The part number or catalog number of the device.). This is the underlying object with id, value and extensions. The accessor "getPartNumber" gives direct access to the value 3157 */ 3158 public Device setPartNumberElement(StringType value) { 3159 this.partNumber = value; 3160 return this; 3161 } 3162 3163 /** 3164 * @return The part number or catalog number of the device. 3165 */ 3166 public String getPartNumber() { 3167 return this.partNumber == null ? null : this.partNumber.getValue(); 3168 } 3169 3170 /** 3171 * @param value The part number or catalog number of the device. 3172 */ 3173 public Device setPartNumber(String value) { 3174 if (Utilities.noString(value)) 3175 this.partNumber = null; 3176 else { 3177 if (this.partNumber == null) 3178 this.partNumber = new StringType(); 3179 this.partNumber.setValue(value); 3180 } 3181 return this; 3182 } 3183 3184 /** 3185 * @return {@link #category} (Devices may be associated with one or more categories.) 3186 */ 3187 public List<CodeableConcept> getCategory() { 3188 if (this.category == null) 3189 this.category = new ArrayList<CodeableConcept>(); 3190 return this.category; 3191 } 3192 3193 /** 3194 * @return Returns a reference to <code>this</code> for easy method chaining 3195 */ 3196 public Device setCategory(List<CodeableConcept> theCategory) { 3197 this.category = theCategory; 3198 return this; 3199 } 3200 3201 public boolean hasCategory() { 3202 if (this.category == null) 3203 return false; 3204 for (CodeableConcept item : this.category) 3205 if (!item.isEmpty()) 3206 return true; 3207 return false; 3208 } 3209 3210 public CodeableConcept addCategory() { //3 3211 CodeableConcept t = new CodeableConcept(); 3212 if (this.category == null) 3213 this.category = new ArrayList<CodeableConcept>(); 3214 this.category.add(t); 3215 return t; 3216 } 3217 3218 public Device addCategory(CodeableConcept t) { //3 3219 if (t == null) 3220 return this; 3221 if (this.category == null) 3222 this.category = new ArrayList<CodeableConcept>(); 3223 this.category.add(t); 3224 return this; 3225 } 3226 3227 /** 3228 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 3229 */ 3230 public CodeableConcept getCategoryFirstRep() { 3231 if (getCategory().isEmpty()) { 3232 addCategory(); 3233 } 3234 return getCategory().get(0); 3235 } 3236 3237 /** 3238 * @return {@link #type} (The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device.) 3239 */ 3240 public List<CodeableConcept> getType() { 3241 if (this.type == null) 3242 this.type = new ArrayList<CodeableConcept>(); 3243 return this.type; 3244 } 3245 3246 /** 3247 * @return Returns a reference to <code>this</code> for easy method chaining 3248 */ 3249 public Device setType(List<CodeableConcept> theType) { 3250 this.type = theType; 3251 return this; 3252 } 3253 3254 public boolean hasType() { 3255 if (this.type == null) 3256 return false; 3257 for (CodeableConcept item : this.type) 3258 if (!item.isEmpty()) 3259 return true; 3260 return false; 3261 } 3262 3263 public CodeableConcept addType() { //3 3264 CodeableConcept t = new CodeableConcept(); 3265 if (this.type == null) 3266 this.type = new ArrayList<CodeableConcept>(); 3267 this.type.add(t); 3268 return t; 3269 } 3270 3271 public Device addType(CodeableConcept t) { //3 3272 if (t == null) 3273 return this; 3274 if (this.type == null) 3275 this.type = new ArrayList<CodeableConcept>(); 3276 this.type.add(t); 3277 return this; 3278 } 3279 3280 /** 3281 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 3282 */ 3283 public CodeableConcept getTypeFirstRep() { 3284 if (getType().isEmpty()) { 3285 addType(); 3286 } 3287 return getType().get(0); 3288 } 3289 3290 /** 3291 * @return {@link #version} (The actual design of the device or software version running on the device.) 3292 */ 3293 public List<DeviceVersionComponent> getVersion() { 3294 if (this.version == null) 3295 this.version = new ArrayList<DeviceVersionComponent>(); 3296 return this.version; 3297 } 3298 3299 /** 3300 * @return Returns a reference to <code>this</code> for easy method chaining 3301 */ 3302 public Device setVersion(List<DeviceVersionComponent> theVersion) { 3303 this.version = theVersion; 3304 return this; 3305 } 3306 3307 public boolean hasVersion() { 3308 if (this.version == null) 3309 return false; 3310 for (DeviceVersionComponent item : this.version) 3311 if (!item.isEmpty()) 3312 return true; 3313 return false; 3314 } 3315 3316 public DeviceVersionComponent addVersion() { //3 3317 DeviceVersionComponent t = new DeviceVersionComponent(); 3318 if (this.version == null) 3319 this.version = new ArrayList<DeviceVersionComponent>(); 3320 this.version.add(t); 3321 return t; 3322 } 3323 3324 public Device addVersion(DeviceVersionComponent t) { //3 3325 if (t == null) 3326 return this; 3327 if (this.version == null) 3328 this.version = new ArrayList<DeviceVersionComponent>(); 3329 this.version.add(t); 3330 return this; 3331 } 3332 3333 /** 3334 * @return The first repetition of repeating field {@link #version}, creating it if it does not already exist {3} 3335 */ 3336 public DeviceVersionComponent getVersionFirstRep() { 3337 if (getVersion().isEmpty()) { 3338 addVersion(); 3339 } 3340 return getVersion().get(0); 3341 } 3342 3343 /** 3344 * @return {@link #conformsTo} (Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.) 3345 */ 3346 public List<DeviceConformsToComponent> getConformsTo() { 3347 if (this.conformsTo == null) 3348 this.conformsTo = new ArrayList<DeviceConformsToComponent>(); 3349 return this.conformsTo; 3350 } 3351 3352 /** 3353 * @return Returns a reference to <code>this</code> for easy method chaining 3354 */ 3355 public Device setConformsTo(List<DeviceConformsToComponent> theConformsTo) { 3356 this.conformsTo = theConformsTo; 3357 return this; 3358 } 3359 3360 public boolean hasConformsTo() { 3361 if (this.conformsTo == null) 3362 return false; 3363 for (DeviceConformsToComponent item : this.conformsTo) 3364 if (!item.isEmpty()) 3365 return true; 3366 return false; 3367 } 3368 3369 public DeviceConformsToComponent addConformsTo() { //3 3370 DeviceConformsToComponent t = new DeviceConformsToComponent(); 3371 if (this.conformsTo == null) 3372 this.conformsTo = new ArrayList<DeviceConformsToComponent>(); 3373 this.conformsTo.add(t); 3374 return t; 3375 } 3376 3377 public Device addConformsTo(DeviceConformsToComponent t) { //3 3378 if (t == null) 3379 return this; 3380 if (this.conformsTo == null) 3381 this.conformsTo = new ArrayList<DeviceConformsToComponent>(); 3382 this.conformsTo.add(t); 3383 return this; 3384 } 3385 3386 /** 3387 * @return The first repetition of repeating field {@link #conformsTo}, creating it if it does not already exist {3} 3388 */ 3389 public DeviceConformsToComponent getConformsToFirstRep() { 3390 if (getConformsTo().isEmpty()) { 3391 addConformsTo(); 3392 } 3393 return getConformsTo().get(0); 3394 } 3395 3396 /** 3397 * @return {@link #property} (Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes.) 3398 */ 3399 public List<DevicePropertyComponent> getProperty() { 3400 if (this.property == null) 3401 this.property = new ArrayList<DevicePropertyComponent>(); 3402 return this.property; 3403 } 3404 3405 /** 3406 * @return Returns a reference to <code>this</code> for easy method chaining 3407 */ 3408 public Device setProperty(List<DevicePropertyComponent> theProperty) { 3409 this.property = theProperty; 3410 return this; 3411 } 3412 3413 public boolean hasProperty() { 3414 if (this.property == null) 3415 return false; 3416 for (DevicePropertyComponent item : this.property) 3417 if (!item.isEmpty()) 3418 return true; 3419 return false; 3420 } 3421 3422 public DevicePropertyComponent addProperty() { //3 3423 DevicePropertyComponent t = new DevicePropertyComponent(); 3424 if (this.property == null) 3425 this.property = new ArrayList<DevicePropertyComponent>(); 3426 this.property.add(t); 3427 return t; 3428 } 3429 3430 public Device addProperty(DevicePropertyComponent t) { //3 3431 if (t == null) 3432 return this; 3433 if (this.property == null) 3434 this.property = new ArrayList<DevicePropertyComponent>(); 3435 this.property.add(t); 3436 return this; 3437 } 3438 3439 /** 3440 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 3441 */ 3442 public DevicePropertyComponent getPropertyFirstRep() { 3443 if (getProperty().isEmpty()) { 3444 addProperty(); 3445 } 3446 return getProperty().get(0); 3447 } 3448 3449 /** 3450 * @return {@link #mode} (The designated condition for performing a task with the device.) 3451 */ 3452 public CodeableConcept getMode() { 3453 if (this.mode == null) 3454 if (Configuration.errorOnAutoCreate()) 3455 throw new Error("Attempt to auto-create Device.mode"); 3456 else if (Configuration.doAutoCreate()) 3457 this.mode = new CodeableConcept(); // cc 3458 return this.mode; 3459 } 3460 3461 public boolean hasMode() { 3462 return this.mode != null && !this.mode.isEmpty(); 3463 } 3464 3465 /** 3466 * @param value {@link #mode} (The designated condition for performing a task with the device.) 3467 */ 3468 public Device setMode(CodeableConcept value) { 3469 this.mode = value; 3470 return this; 3471 } 3472 3473 /** 3474 * @return {@link #cycle} (The series of occurrences that repeats during the operation of the device.) 3475 */ 3476 public Count getCycle() { 3477 if (this.cycle == null) 3478 if (Configuration.errorOnAutoCreate()) 3479 throw new Error("Attempt to auto-create Device.cycle"); 3480 else if (Configuration.doAutoCreate()) 3481 this.cycle = new Count(); // cc 3482 return this.cycle; 3483 } 3484 3485 public boolean hasCycle() { 3486 return this.cycle != null && !this.cycle.isEmpty(); 3487 } 3488 3489 /** 3490 * @param value {@link #cycle} (The series of occurrences that repeats during the operation of the device.) 3491 */ 3492 public Device setCycle(Count value) { 3493 this.cycle = value; 3494 return this; 3495 } 3496 3497 /** 3498 * @return {@link #duration} (A measurement of time during the device's operation (e.g., days, hours, mins, etc.).) 3499 */ 3500 public Duration getDuration() { 3501 if (this.duration == null) 3502 if (Configuration.errorOnAutoCreate()) 3503 throw new Error("Attempt to auto-create Device.duration"); 3504 else if (Configuration.doAutoCreate()) 3505 this.duration = new Duration(); // cc 3506 return this.duration; 3507 } 3508 3509 public boolean hasDuration() { 3510 return this.duration != null && !this.duration.isEmpty(); 3511 } 3512 3513 /** 3514 * @param value {@link #duration} (A measurement of time during the device's operation (e.g., days, hours, mins, etc.).) 3515 */ 3516 public Device setDuration(Duration value) { 3517 this.duration = value; 3518 return this; 3519 } 3520 3521 /** 3522 * @return {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 3523 */ 3524 public Reference getOwner() { 3525 if (this.owner == null) 3526 if (Configuration.errorOnAutoCreate()) 3527 throw new Error("Attempt to auto-create Device.owner"); 3528 else if (Configuration.doAutoCreate()) 3529 this.owner = new Reference(); // cc 3530 return this.owner; 3531 } 3532 3533 public boolean hasOwner() { 3534 return this.owner != null && !this.owner.isEmpty(); 3535 } 3536 3537 /** 3538 * @param value {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 3539 */ 3540 public Device setOwner(Reference value) { 3541 this.owner = value; 3542 return this; 3543 } 3544 3545 /** 3546 * @return {@link #contact} (Contact details for an organization or a particular human that is responsible for the device.) 3547 */ 3548 public List<ContactPoint> getContact() { 3549 if (this.contact == null) 3550 this.contact = new ArrayList<ContactPoint>(); 3551 return this.contact; 3552 } 3553 3554 /** 3555 * @return Returns a reference to <code>this</code> for easy method chaining 3556 */ 3557 public Device setContact(List<ContactPoint> theContact) { 3558 this.contact = theContact; 3559 return this; 3560 } 3561 3562 public boolean hasContact() { 3563 if (this.contact == null) 3564 return false; 3565 for (ContactPoint item : this.contact) 3566 if (!item.isEmpty()) 3567 return true; 3568 return false; 3569 } 3570 3571 public ContactPoint addContact() { //3 3572 ContactPoint t = new ContactPoint(); 3573 if (this.contact == null) 3574 this.contact = new ArrayList<ContactPoint>(); 3575 this.contact.add(t); 3576 return t; 3577 } 3578 3579 public Device addContact(ContactPoint t) { //3 3580 if (t == null) 3581 return this; 3582 if (this.contact == null) 3583 this.contact = new ArrayList<ContactPoint>(); 3584 this.contact.add(t); 3585 return this; 3586 } 3587 3588 /** 3589 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3590 */ 3591 public ContactPoint getContactFirstRep() { 3592 if (getContact().isEmpty()) { 3593 addContact(); 3594 } 3595 return getContact().get(0); 3596 } 3597 3598 /** 3599 * @return {@link #location} (The place where the device can be found.) 3600 */ 3601 public Reference getLocation() { 3602 if (this.location == null) 3603 if (Configuration.errorOnAutoCreate()) 3604 throw new Error("Attempt to auto-create Device.location"); 3605 else if (Configuration.doAutoCreate()) 3606 this.location = new Reference(); // cc 3607 return this.location; 3608 } 3609 3610 public boolean hasLocation() { 3611 return this.location != null && !this.location.isEmpty(); 3612 } 3613 3614 /** 3615 * @param value {@link #location} (The place where the device can be found.) 3616 */ 3617 public Device setLocation(Reference value) { 3618 this.location = value; 3619 return this; 3620 } 3621 3622 /** 3623 * @return {@link #url} (A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3624 */ 3625 public UriType getUrlElement() { 3626 if (this.url == null) 3627 if (Configuration.errorOnAutoCreate()) 3628 throw new Error("Attempt to auto-create Device.url"); 3629 else if (Configuration.doAutoCreate()) 3630 this.url = new UriType(); // bb 3631 return this.url; 3632 } 3633 3634 public boolean hasUrlElement() { 3635 return this.url != null && !this.url.isEmpty(); 3636 } 3637 3638 public boolean hasUrl() { 3639 return this.url != null && !this.url.isEmpty(); 3640 } 3641 3642 /** 3643 * @param value {@link #url} (A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3644 */ 3645 public Device setUrlElement(UriType value) { 3646 this.url = value; 3647 return this; 3648 } 3649 3650 /** 3651 * @return A network address on which the device may be contacted directly. 3652 */ 3653 public String getUrl() { 3654 return this.url == null ? null : this.url.getValue(); 3655 } 3656 3657 /** 3658 * @param value A network address on which the device may be contacted directly. 3659 */ 3660 public Device setUrl(String value) { 3661 if (Utilities.noString(value)) 3662 this.url = null; 3663 else { 3664 if (this.url == null) 3665 this.url = new UriType(); 3666 this.url.setValue(value); 3667 } 3668 return this; 3669 } 3670 3671 /** 3672 * @return {@link #endpoint} (Technical endpoints providing access to services provided by the device defined at this resource.) 3673 */ 3674 public List<Reference> getEndpoint() { 3675 if (this.endpoint == null) 3676 this.endpoint = new ArrayList<Reference>(); 3677 return this.endpoint; 3678 } 3679 3680 /** 3681 * @return Returns a reference to <code>this</code> for easy method chaining 3682 */ 3683 public Device setEndpoint(List<Reference> theEndpoint) { 3684 this.endpoint = theEndpoint; 3685 return this; 3686 } 3687 3688 public boolean hasEndpoint() { 3689 if (this.endpoint == null) 3690 return false; 3691 for (Reference item : this.endpoint) 3692 if (!item.isEmpty()) 3693 return true; 3694 return false; 3695 } 3696 3697 public Reference addEndpoint() { //3 3698 Reference t = new Reference(); 3699 if (this.endpoint == null) 3700 this.endpoint = new ArrayList<Reference>(); 3701 this.endpoint.add(t); 3702 return t; 3703 } 3704 3705 public Device addEndpoint(Reference t) { //3 3706 if (t == null) 3707 return this; 3708 if (this.endpoint == null) 3709 this.endpoint = new ArrayList<Reference>(); 3710 this.endpoint.add(t); 3711 return this; 3712 } 3713 3714 /** 3715 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 3716 */ 3717 public Reference getEndpointFirstRep() { 3718 if (getEndpoint().isEmpty()) { 3719 addEndpoint(); 3720 } 3721 return getEndpoint().get(0); 3722 } 3723 3724 /** 3725 * @return {@link #gateway} (The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data).) 3726 */ 3727 public List<CodeableReference> getGateway() { 3728 if (this.gateway == null) 3729 this.gateway = new ArrayList<CodeableReference>(); 3730 return this.gateway; 3731 } 3732 3733 /** 3734 * @return Returns a reference to <code>this</code> for easy method chaining 3735 */ 3736 public Device setGateway(List<CodeableReference> theGateway) { 3737 this.gateway = theGateway; 3738 return this; 3739 } 3740 3741 public boolean hasGateway() { 3742 if (this.gateway == null) 3743 return false; 3744 for (CodeableReference item : this.gateway) 3745 if (!item.isEmpty()) 3746 return true; 3747 return false; 3748 } 3749 3750 public CodeableReference addGateway() { //3 3751 CodeableReference t = new CodeableReference(); 3752 if (this.gateway == null) 3753 this.gateway = new ArrayList<CodeableReference>(); 3754 this.gateway.add(t); 3755 return t; 3756 } 3757 3758 public Device addGateway(CodeableReference t) { //3 3759 if (t == null) 3760 return this; 3761 if (this.gateway == null) 3762 this.gateway = new ArrayList<CodeableReference>(); 3763 this.gateway.add(t); 3764 return this; 3765 } 3766 3767 /** 3768 * @return The first repetition of repeating field {@link #gateway}, creating it if it does not already exist {3} 3769 */ 3770 public CodeableReference getGatewayFirstRep() { 3771 if (getGateway().isEmpty()) { 3772 addGateway(); 3773 } 3774 return getGateway().get(0); 3775 } 3776 3777 /** 3778 * @return {@link #note} (Descriptive information, usage information or implantation information that is not captured in an existing element.) 3779 */ 3780 public List<Annotation> getNote() { 3781 if (this.note == null) 3782 this.note = new ArrayList<Annotation>(); 3783 return this.note; 3784 } 3785 3786 /** 3787 * @return Returns a reference to <code>this</code> for easy method chaining 3788 */ 3789 public Device setNote(List<Annotation> theNote) { 3790 this.note = theNote; 3791 return this; 3792 } 3793 3794 public boolean hasNote() { 3795 if (this.note == null) 3796 return false; 3797 for (Annotation item : this.note) 3798 if (!item.isEmpty()) 3799 return true; 3800 return false; 3801 } 3802 3803 public Annotation addNote() { //3 3804 Annotation t = new Annotation(); 3805 if (this.note == null) 3806 this.note = new ArrayList<Annotation>(); 3807 this.note.add(t); 3808 return t; 3809 } 3810 3811 public Device addNote(Annotation t) { //3 3812 if (t == null) 3813 return this; 3814 if (this.note == null) 3815 this.note = new ArrayList<Annotation>(); 3816 this.note.add(t); 3817 return this; 3818 } 3819 3820 /** 3821 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3822 */ 3823 public Annotation getNoteFirstRep() { 3824 if (getNote().isEmpty()) { 3825 addNote(); 3826 } 3827 return getNote().get(0); 3828 } 3829 3830 /** 3831 * @return {@link #safety} (Provides additional safety characteristics about a medical device. For example devices containing latex.) 3832 */ 3833 public List<CodeableConcept> getSafety() { 3834 if (this.safety == null) 3835 this.safety = new ArrayList<CodeableConcept>(); 3836 return this.safety; 3837 } 3838 3839 /** 3840 * @return Returns a reference to <code>this</code> for easy method chaining 3841 */ 3842 public Device setSafety(List<CodeableConcept> theSafety) { 3843 this.safety = theSafety; 3844 return this; 3845 } 3846 3847 public boolean hasSafety() { 3848 if (this.safety == null) 3849 return false; 3850 for (CodeableConcept item : this.safety) 3851 if (!item.isEmpty()) 3852 return true; 3853 return false; 3854 } 3855 3856 public CodeableConcept addSafety() { //3 3857 CodeableConcept t = new CodeableConcept(); 3858 if (this.safety == null) 3859 this.safety = new ArrayList<CodeableConcept>(); 3860 this.safety.add(t); 3861 return t; 3862 } 3863 3864 public Device addSafety(CodeableConcept t) { //3 3865 if (t == null) 3866 return this; 3867 if (this.safety == null) 3868 this.safety = new ArrayList<CodeableConcept>(); 3869 this.safety.add(t); 3870 return this; 3871 } 3872 3873 /** 3874 * @return The first repetition of repeating field {@link #safety}, creating it if it does not already exist {3} 3875 */ 3876 public CodeableConcept getSafetyFirstRep() { 3877 if (getSafety().isEmpty()) { 3878 addSafety(); 3879 } 3880 return getSafety().get(0); 3881 } 3882 3883 /** 3884 * @return {@link #parent} (The higher level or encompassing device that this device is a logical part of.) 3885 */ 3886 public Reference getParent() { 3887 if (this.parent == null) 3888 if (Configuration.errorOnAutoCreate()) 3889 throw new Error("Attempt to auto-create Device.parent"); 3890 else if (Configuration.doAutoCreate()) 3891 this.parent = new Reference(); // cc 3892 return this.parent; 3893 } 3894 3895 public boolean hasParent() { 3896 return this.parent != null && !this.parent.isEmpty(); 3897 } 3898 3899 /** 3900 * @param value {@link #parent} (The higher level or encompassing device that this device is a logical part of.) 3901 */ 3902 public Device setParent(Reference value) { 3903 this.parent = value; 3904 return this; 3905 } 3906 3907 protected void listChildren(List<Property> children) { 3908 super.listChildren(children); 3909 children.add(new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3910 children.add(new Property("displayName", "string", "The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.", 0, 1, displayName)); 3911 children.add(new Property("definition", "CodeableReference(DeviceDefinition)", "The reference to the definition for the device.", 0, 1, definition)); 3912 children.add(new Property("udiCarrier", "", "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 0, java.lang.Integer.MAX_VALUE, udiCarrier)); 3913 children.add(new Property("status", "code", "The Device record status. This is not the status of the device like availability.", 0, 1, status)); 3914 children.add(new Property("availabilityStatus", "CodeableConcept", "The availability of the device.", 0, 1, availabilityStatus)); 3915 children.add(new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent)); 3916 children.add(new Property("manufacturer", "string", "A name of the manufacturer or entity legally responsible for the device.", 0, 1, manufacturer)); 3917 children.add(new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 0, 1, manufactureDate)); 3918 children.add(new Property("expirationDate", "dateTime", "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, expirationDate)); 3919 children.add(new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, lotNumber)); 3920 children.add(new Property("serialNumber", "string", "The serial number assigned by the organization when the device was manufactured.", 0, 1, serialNumber)); 3921 children.add(new Property("name", "", "This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.", 0, java.lang.Integer.MAX_VALUE, name)); 3922 children.add(new Property("modelNumber", "string", "The manufacturer's model number for the device.", 0, 1, modelNumber)); 3923 children.add(new Property("partNumber", "string", "The part number or catalog number of the device.", 0, 1, partNumber)); 3924 children.add(new Property("category", "CodeableConcept", "Devices may be associated with one or more categories.", 0, java.lang.Integer.MAX_VALUE, category)); 3925 children.add(new Property("type", "CodeableConcept", "The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device.", 0, java.lang.Integer.MAX_VALUE, type)); 3926 children.add(new Property("version", "", "The actual design of the device or software version running on the device.", 0, java.lang.Integer.MAX_VALUE, version)); 3927 children.add(new Property("conformsTo", "", "Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.", 0, java.lang.Integer.MAX_VALUE, conformsTo)); 3928 children.add(new Property("property", "", "Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes.", 0, java.lang.Integer.MAX_VALUE, property)); 3929 children.add(new Property("mode", "CodeableConcept", "The designated condition for performing a task with the device.", 0, 1, mode)); 3930 children.add(new Property("cycle", "Count", "The series of occurrences that repeats during the operation of the device.", 0, 1, cycle)); 3931 children.add(new Property("duration", "Duration", "A measurement of time during the device's operation (e.g., days, hours, mins, etc.).", 0, 1, duration)); 3932 children.add(new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner)); 3933 children.add(new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact)); 3934 children.add(new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1, location)); 3935 children.add(new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url)); 3936 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services provided by the device defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 3937 children.add(new Property("gateway", "CodeableReference(Device)", "The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data).", 0, java.lang.Integer.MAX_VALUE, gateway)); 3938 children.add(new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note)); 3939 children.add(new Property("safety", "CodeableConcept", "Provides additional safety characteristics about a medical device. For example devices containing latex.", 0, java.lang.Integer.MAX_VALUE, safety)); 3940 children.add(new Property("parent", "Reference(Device)", "The higher level or encompassing device that this device is a logical part of.", 0, 1, parent)); 3941 } 3942 3943 @Override 3944 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3945 switch (_hash) { 3946 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by manufacturers other organizations or owners.", 0, java.lang.Integer.MAX_VALUE, identifier); 3947 case 1714148973: /*displayName*/ return new Property("displayName", "string", "The name used to display by default when the device is referenced. Based on intent of use by the resource creator, this may reflect one of the names in Device.name, or may be another simple name.", 0, 1, displayName); 3948 case -1014418093: /*definition*/ return new Property("definition", "CodeableReference(DeviceDefinition)", "The reference to the definition for the device.", 0, 1, definition); 3949 case -1343558178: /*udiCarrier*/ return new Property("udiCarrier", "", "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 0, java.lang.Integer.MAX_VALUE, udiCarrier); 3950 case -892481550: /*status*/ return new Property("status", "code", "The Device record status. This is not the status of the device like availability.", 0, 1, status); 3951 case 804659501: /*availabilityStatus*/ return new Property("availabilityStatus", "CodeableConcept", "The availability of the device.", 0, 1, availabilityStatus); 3952 case -654468482: /*biologicalSourceEvent*/ return new Property("biologicalSourceEvent", "Identifier", "An identifier that supports traceability to the event during which material in this product from one or more biological entities was obtained or pooled.", 0, 1, biologicalSourceEvent); 3953 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "string", "A name of the manufacturer or entity legally responsible for the device.", 0, 1, manufacturer); 3954 case 416714767: /*manufactureDate*/ return new Property("manufactureDate", "dateTime", "The date and time when the device was manufactured.", 0, 1, manufactureDate); 3955 case -668811523: /*expirationDate*/ return new Property("expirationDate", "dateTime", "The date and time beyond which this device is no longer valid or should not be used (if applicable).", 0, 1, expirationDate); 3956 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "Lot number assigned by the manufacturer.", 0, 1, lotNumber); 3957 case 83787357: /*serialNumber*/ return new Property("serialNumber", "string", "The serial number assigned by the organization when the device was manufactured.", 0, 1, serialNumber); 3958 case 3373707: /*name*/ return new Property("name", "", "This represents the manufacturer's name of the device as provided by the device, from a UDI label, or by a person describing the Device. This typically would be used when a person provides the name(s) or when the device represents one of the names available from DeviceDefinition.", 0, java.lang.Integer.MAX_VALUE, name); 3959 case 346619858: /*modelNumber*/ return new Property("modelNumber", "string", "The manufacturer's model number for the device.", 0, 1, modelNumber); 3960 case -731502308: /*partNumber*/ return new Property("partNumber", "string", "The part number or catalog number of the device.", 0, 1, partNumber); 3961 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Devices may be associated with one or more categories.", 0, java.lang.Integer.MAX_VALUE, category); 3962 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind or type of device. A device instance may have more than one type - in which case those are the types that apply to the specific instance of the device.", 0, java.lang.Integer.MAX_VALUE, type); 3963 case 351608024: /*version*/ return new Property("version", "", "The actual design of the device or software version running on the device.", 0, java.lang.Integer.MAX_VALUE, version); 3964 case 1014198088: /*conformsTo*/ return new Property("conformsTo", "", "Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.", 0, java.lang.Integer.MAX_VALUE, conformsTo); 3965 case -993141291: /*property*/ return new Property("property", "", "Static or essentially fixed characteristics or features of the device (e.g., time or timing attributes, resolution, accuracy, intended use or instructions for use, and physical attributes) that are not otherwise captured in more specific attributes.", 0, java.lang.Integer.MAX_VALUE, property); 3966 case 3357091: /*mode*/ return new Property("mode", "CodeableConcept", "The designated condition for performing a task with the device.", 0, 1, mode); 3967 case 95131878: /*cycle*/ return new Property("cycle", "Count", "The series of occurrences that repeats during the operation of the device.", 0, 1, cycle); 3968 case -1992012396: /*duration*/ return new Property("duration", "Duration", "A measurement of time during the device's operation (e.g., days, hours, mins, etc.).", 0, 1, duration); 3969 case 106164915: /*owner*/ return new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner); 3970 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact); 3971 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The place where the device can be found.", 0, 1, location); 3972 case 116079: /*url*/ return new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url); 3973 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services provided by the device defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint); 3974 case -189118908: /*gateway*/ return new Property("gateway", "CodeableReference(Device)", "The linked device acting as a communication controller, data collector, translator, or concentrator for the current device (e.g., mobile phone application that relays a blood pressure device's data).", 0, java.lang.Integer.MAX_VALUE, gateway); 3975 case 3387378: /*note*/ return new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note); 3976 case -909893934: /*safety*/ return new Property("safety", "CodeableConcept", "Provides additional safety characteristics about a medical device. For example devices containing latex.", 0, java.lang.Integer.MAX_VALUE, safety); 3977 case -995424086: /*parent*/ return new Property("parent", "Reference(Device)", "The higher level or encompassing device that this device is a logical part of.", 0, 1, parent); 3978 default: return super.getNamedProperty(_hash, _name, _checkValid); 3979 } 3980 3981 } 3982 3983 @Override 3984 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3985 switch (hash) { 3986 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3987 case 1714148973: /*displayName*/ return this.displayName == null ? new Base[0] : new Base[] {this.displayName}; // StringType 3988 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CodeableReference 3989 case -1343558178: /*udiCarrier*/ return this.udiCarrier == null ? new Base[0] : this.udiCarrier.toArray(new Base[this.udiCarrier.size()]); // DeviceUdiCarrierComponent 3990 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FHIRDeviceStatus> 3991 case 804659501: /*availabilityStatus*/ return this.availabilityStatus == null ? new Base[0] : new Base[] {this.availabilityStatus}; // CodeableConcept 3992 case -654468482: /*biologicalSourceEvent*/ return this.biologicalSourceEvent == null ? new Base[0] : new Base[] {this.biologicalSourceEvent}; // Identifier 3993 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // StringType 3994 case 416714767: /*manufactureDate*/ return this.manufactureDate == null ? new Base[0] : new Base[] {this.manufactureDate}; // DateTimeType 3995 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateTimeType 3996 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 3997 case 83787357: /*serialNumber*/ return this.serialNumber == null ? new Base[0] : new Base[] {this.serialNumber}; // StringType 3998 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // DeviceNameComponent 3999 case 346619858: /*modelNumber*/ return this.modelNumber == null ? new Base[0] : new Base[] {this.modelNumber}; // StringType 4000 case -731502308: /*partNumber*/ return this.partNumber == null ? new Base[0] : new Base[] {this.partNumber}; // StringType 4001 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 4002 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 4003 case 351608024: /*version*/ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // DeviceVersionComponent 4004 case 1014198088: /*conformsTo*/ return this.conformsTo == null ? new Base[0] : this.conformsTo.toArray(new Base[this.conformsTo.size()]); // DeviceConformsToComponent 4005 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // DevicePropertyComponent 4006 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // CodeableConcept 4007 case 95131878: /*cycle*/ return this.cycle == null ? new Base[0] : new Base[] {this.cycle}; // Count 4008 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // Duration 4009 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 4010 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 4011 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 4012 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4013 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 4014 case -189118908: /*gateway*/ return this.gateway == null ? new Base[0] : this.gateway.toArray(new Base[this.gateway.size()]); // CodeableReference 4015 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4016 case -909893934: /*safety*/ return this.safety == null ? new Base[0] : this.safety.toArray(new Base[this.safety.size()]); // CodeableConcept 4017 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // Reference 4018 default: return super.getProperty(hash, name, checkValid); 4019 } 4020 4021 } 4022 4023 @Override 4024 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4025 switch (hash) { 4026 case -1618432855: // identifier 4027 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4028 return value; 4029 case 1714148973: // displayName 4030 this.displayName = TypeConvertor.castToString(value); // StringType 4031 return value; 4032 case -1014418093: // definition 4033 this.definition = TypeConvertor.castToCodeableReference(value); // CodeableReference 4034 return value; 4035 case -1343558178: // udiCarrier 4036 this.getUdiCarrier().add((DeviceUdiCarrierComponent) value); // DeviceUdiCarrierComponent 4037 return value; 4038 case -892481550: // status 4039 value = new FHIRDeviceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4040 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4041 return value; 4042 case 804659501: // availabilityStatus 4043 this.availabilityStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4044 return value; 4045 case -654468482: // biologicalSourceEvent 4046 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 4047 return value; 4048 case -1969347631: // manufacturer 4049 this.manufacturer = TypeConvertor.castToString(value); // StringType 4050 return value; 4051 case 416714767: // manufactureDate 4052 this.manufactureDate = TypeConvertor.castToDateTime(value); // DateTimeType 4053 return value; 4054 case -668811523: // expirationDate 4055 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 4056 return value; 4057 case 462547450: // lotNumber 4058 this.lotNumber = TypeConvertor.castToString(value); // StringType 4059 return value; 4060 case 83787357: // serialNumber 4061 this.serialNumber = TypeConvertor.castToString(value); // StringType 4062 return value; 4063 case 3373707: // name 4064 this.getName().add((DeviceNameComponent) value); // DeviceNameComponent 4065 return value; 4066 case 346619858: // modelNumber 4067 this.modelNumber = TypeConvertor.castToString(value); // StringType 4068 return value; 4069 case -731502308: // partNumber 4070 this.partNumber = TypeConvertor.castToString(value); // StringType 4071 return value; 4072 case 50511102: // category 4073 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4074 return value; 4075 case 3575610: // type 4076 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4077 return value; 4078 case 351608024: // version 4079 this.getVersion().add((DeviceVersionComponent) value); // DeviceVersionComponent 4080 return value; 4081 case 1014198088: // conformsTo 4082 this.getConformsTo().add((DeviceConformsToComponent) value); // DeviceConformsToComponent 4083 return value; 4084 case -993141291: // property 4085 this.getProperty().add((DevicePropertyComponent) value); // DevicePropertyComponent 4086 return value; 4087 case 3357091: // mode 4088 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4089 return value; 4090 case 95131878: // cycle 4091 this.cycle = TypeConvertor.castToCount(value); // Count 4092 return value; 4093 case -1992012396: // duration 4094 this.duration = TypeConvertor.castToDuration(value); // Duration 4095 return value; 4096 case 106164915: // owner 4097 this.owner = TypeConvertor.castToReference(value); // Reference 4098 return value; 4099 case 951526432: // contact 4100 this.getContact().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 4101 return value; 4102 case 1901043637: // location 4103 this.location = TypeConvertor.castToReference(value); // Reference 4104 return value; 4105 case 116079: // url 4106 this.url = TypeConvertor.castToUri(value); // UriType 4107 return value; 4108 case 1741102485: // endpoint 4109 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 4110 return value; 4111 case -189118908: // gateway 4112 this.getGateway().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4113 return value; 4114 case 3387378: // note 4115 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4116 return value; 4117 case -909893934: // safety 4118 this.getSafety().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4119 return value; 4120 case -995424086: // parent 4121 this.parent = TypeConvertor.castToReference(value); // Reference 4122 return value; 4123 default: return super.setProperty(hash, name, value); 4124 } 4125 4126 } 4127 4128 @Override 4129 public Base setProperty(String name, Base value) throws FHIRException { 4130 if (name.equals("identifier")) { 4131 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4132 } else if (name.equals("displayName")) { 4133 this.displayName = TypeConvertor.castToString(value); // StringType 4134 } else if (name.equals("definition")) { 4135 this.definition = TypeConvertor.castToCodeableReference(value); // CodeableReference 4136 } else if (name.equals("udiCarrier")) { 4137 this.getUdiCarrier().add((DeviceUdiCarrierComponent) value); 4138 } else if (name.equals("status")) { 4139 value = new FHIRDeviceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4140 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4141 } else if (name.equals("availabilityStatus")) { 4142 this.availabilityStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4143 } else if (name.equals("biologicalSourceEvent")) { 4144 this.biologicalSourceEvent = TypeConvertor.castToIdentifier(value); // Identifier 4145 } else if (name.equals("manufacturer")) { 4146 this.manufacturer = TypeConvertor.castToString(value); // StringType 4147 } else if (name.equals("manufactureDate")) { 4148 this.manufactureDate = TypeConvertor.castToDateTime(value); // DateTimeType 4149 } else if (name.equals("expirationDate")) { 4150 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 4151 } else if (name.equals("lotNumber")) { 4152 this.lotNumber = TypeConvertor.castToString(value); // StringType 4153 } else if (name.equals("serialNumber")) { 4154 this.serialNumber = TypeConvertor.castToString(value); // StringType 4155 } else if (name.equals("name")) { 4156 this.getName().add((DeviceNameComponent) value); 4157 } else if (name.equals("modelNumber")) { 4158 this.modelNumber = TypeConvertor.castToString(value); // StringType 4159 } else if (name.equals("partNumber")) { 4160 this.partNumber = TypeConvertor.castToString(value); // StringType 4161 } else if (name.equals("category")) { 4162 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 4163 } else if (name.equals("type")) { 4164 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 4165 } else if (name.equals("version")) { 4166 this.getVersion().add((DeviceVersionComponent) value); 4167 } else if (name.equals("conformsTo")) { 4168 this.getConformsTo().add((DeviceConformsToComponent) value); 4169 } else if (name.equals("property")) { 4170 this.getProperty().add((DevicePropertyComponent) value); 4171 } else if (name.equals("mode")) { 4172 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4173 } else if (name.equals("cycle")) { 4174 this.cycle = TypeConvertor.castToCount(value); // Count 4175 } else if (name.equals("duration")) { 4176 this.duration = TypeConvertor.castToDuration(value); // Duration 4177 } else if (name.equals("owner")) { 4178 this.owner = TypeConvertor.castToReference(value); // Reference 4179 } else if (name.equals("contact")) { 4180 this.getContact().add(TypeConvertor.castToContactPoint(value)); 4181 } else if (name.equals("location")) { 4182 this.location = TypeConvertor.castToReference(value); // Reference 4183 } else if (name.equals("url")) { 4184 this.url = TypeConvertor.castToUri(value); // UriType 4185 } else if (name.equals("endpoint")) { 4186 this.getEndpoint().add(TypeConvertor.castToReference(value)); 4187 } else if (name.equals("gateway")) { 4188 this.getGateway().add(TypeConvertor.castToCodeableReference(value)); 4189 } else if (name.equals("note")) { 4190 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4191 } else if (name.equals("safety")) { 4192 this.getSafety().add(TypeConvertor.castToCodeableConcept(value)); 4193 } else if (name.equals("parent")) { 4194 this.parent = TypeConvertor.castToReference(value); // Reference 4195 } else 4196 return super.setProperty(name, value); 4197 return value; 4198 } 4199 4200 @Override 4201 public void removeChild(String name, Base value) throws FHIRException { 4202 if (name.equals("identifier")) { 4203 this.getIdentifier().remove(value); 4204 } else if (name.equals("displayName")) { 4205 this.displayName = null; 4206 } else if (name.equals("definition")) { 4207 this.definition = null; 4208 } else if (name.equals("udiCarrier")) { 4209 this.getUdiCarrier().remove((DeviceUdiCarrierComponent) value); 4210 } else if (name.equals("status")) { 4211 value = new FHIRDeviceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4212 this.status = (Enumeration) value; // Enumeration<FHIRDeviceStatus> 4213 } else if (name.equals("availabilityStatus")) { 4214 this.availabilityStatus = null; 4215 } else if (name.equals("biologicalSourceEvent")) { 4216 this.biologicalSourceEvent = null; 4217 } else if (name.equals("manufacturer")) { 4218 this.manufacturer = null; 4219 } else if (name.equals("manufactureDate")) { 4220 this.manufactureDate = null; 4221 } else if (name.equals("expirationDate")) { 4222 this.expirationDate = null; 4223 } else if (name.equals("lotNumber")) { 4224 this.lotNumber = null; 4225 } else if (name.equals("serialNumber")) { 4226 this.serialNumber = null; 4227 } else if (name.equals("name")) { 4228 this.getName().remove((DeviceNameComponent) value); 4229 } else if (name.equals("modelNumber")) { 4230 this.modelNumber = null; 4231 } else if (name.equals("partNumber")) { 4232 this.partNumber = null; 4233 } else if (name.equals("category")) { 4234 this.getCategory().remove(value); 4235 } else if (name.equals("type")) { 4236 this.getType().remove(value); 4237 } else if (name.equals("version")) { 4238 this.getVersion().remove((DeviceVersionComponent) value); 4239 } else if (name.equals("conformsTo")) { 4240 this.getConformsTo().remove((DeviceConformsToComponent) value); 4241 } else if (name.equals("property")) { 4242 this.getProperty().remove((DevicePropertyComponent) value); 4243 } else if (name.equals("mode")) { 4244 this.mode = null; 4245 } else if (name.equals("cycle")) { 4246 this.cycle = null; 4247 } else if (name.equals("duration")) { 4248 this.duration = null; 4249 } else if (name.equals("owner")) { 4250 this.owner = null; 4251 } else if (name.equals("contact")) { 4252 this.getContact().remove(value); 4253 } else if (name.equals("location")) { 4254 this.location = null; 4255 } else if (name.equals("url")) { 4256 this.url = null; 4257 } else if (name.equals("endpoint")) { 4258 this.getEndpoint().remove(value); 4259 } else if (name.equals("gateway")) { 4260 this.getGateway().remove(value); 4261 } else if (name.equals("note")) { 4262 this.getNote().remove(value); 4263 } else if (name.equals("safety")) { 4264 this.getSafety().remove(value); 4265 } else if (name.equals("parent")) { 4266 this.parent = null; 4267 } else 4268 super.removeChild(name, value); 4269 4270 } 4271 4272 @Override 4273 public Base makeProperty(int hash, String name) throws FHIRException { 4274 switch (hash) { 4275 case -1618432855: return addIdentifier(); 4276 case 1714148973: return getDisplayNameElement(); 4277 case -1014418093: return getDefinition(); 4278 case -1343558178: return addUdiCarrier(); 4279 case -892481550: return getStatusElement(); 4280 case 804659501: return getAvailabilityStatus(); 4281 case -654468482: return getBiologicalSourceEvent(); 4282 case -1969347631: return getManufacturerElement(); 4283 case 416714767: return getManufactureDateElement(); 4284 case -668811523: return getExpirationDateElement(); 4285 case 462547450: return getLotNumberElement(); 4286 case 83787357: return getSerialNumberElement(); 4287 case 3373707: return addName(); 4288 case 346619858: return getModelNumberElement(); 4289 case -731502308: return getPartNumberElement(); 4290 case 50511102: return addCategory(); 4291 case 3575610: return addType(); 4292 case 351608024: return addVersion(); 4293 case 1014198088: return addConformsTo(); 4294 case -993141291: return addProperty(); 4295 case 3357091: return getMode(); 4296 case 95131878: return getCycle(); 4297 case -1992012396: return getDuration(); 4298 case 106164915: return getOwner(); 4299 case 951526432: return addContact(); 4300 case 1901043637: return getLocation(); 4301 case 116079: return getUrlElement(); 4302 case 1741102485: return addEndpoint(); 4303 case -189118908: return addGateway(); 4304 case 3387378: return addNote(); 4305 case -909893934: return addSafety(); 4306 case -995424086: return getParent(); 4307 default: return super.makeProperty(hash, name); 4308 } 4309 4310 } 4311 4312 @Override 4313 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4314 switch (hash) { 4315 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4316 case 1714148973: /*displayName*/ return new String[] {"string"}; 4317 case -1014418093: /*definition*/ return new String[] {"CodeableReference"}; 4318 case -1343558178: /*udiCarrier*/ return new String[] {}; 4319 case -892481550: /*status*/ return new String[] {"code"}; 4320 case 804659501: /*availabilityStatus*/ return new String[] {"CodeableConcept"}; 4321 case -654468482: /*biologicalSourceEvent*/ return new String[] {"Identifier"}; 4322 case -1969347631: /*manufacturer*/ return new String[] {"string"}; 4323 case 416714767: /*manufactureDate*/ return new String[] {"dateTime"}; 4324 case -668811523: /*expirationDate*/ return new String[] {"dateTime"}; 4325 case 462547450: /*lotNumber*/ return new String[] {"string"}; 4326 case 83787357: /*serialNumber*/ return new String[] {"string"}; 4327 case 3373707: /*name*/ return new String[] {}; 4328 case 346619858: /*modelNumber*/ return new String[] {"string"}; 4329 case -731502308: /*partNumber*/ return new String[] {"string"}; 4330 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4331 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4332 case 351608024: /*version*/ return new String[] {}; 4333 case 1014198088: /*conformsTo*/ return new String[] {}; 4334 case -993141291: /*property*/ return new String[] {}; 4335 case 3357091: /*mode*/ return new String[] {"CodeableConcept"}; 4336 case 95131878: /*cycle*/ return new String[] {"Count"}; 4337 case -1992012396: /*duration*/ return new String[] {"Duration"}; 4338 case 106164915: /*owner*/ return new String[] {"Reference"}; 4339 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 4340 case 1901043637: /*location*/ return new String[] {"Reference"}; 4341 case 116079: /*url*/ return new String[] {"uri"}; 4342 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 4343 case -189118908: /*gateway*/ return new String[] {"CodeableReference"}; 4344 case 3387378: /*note*/ return new String[] {"Annotation"}; 4345 case -909893934: /*safety*/ return new String[] {"CodeableConcept"}; 4346 case -995424086: /*parent*/ return new String[] {"Reference"}; 4347 default: return super.getTypesForProperty(hash, name); 4348 } 4349 4350 } 4351 4352 @Override 4353 public Base addChild(String name) throws FHIRException { 4354 if (name.equals("identifier")) { 4355 return addIdentifier(); 4356 } 4357 else if (name.equals("displayName")) { 4358 throw new FHIRException("Cannot call addChild on a singleton property Device.displayName"); 4359 } 4360 else if (name.equals("definition")) { 4361 this.definition = new CodeableReference(); 4362 return this.definition; 4363 } 4364 else if (name.equals("udiCarrier")) { 4365 return addUdiCarrier(); 4366 } 4367 else if (name.equals("status")) { 4368 throw new FHIRException("Cannot call addChild on a singleton property Device.status"); 4369 } 4370 else if (name.equals("availabilityStatus")) { 4371 this.availabilityStatus = new CodeableConcept(); 4372 return this.availabilityStatus; 4373 } 4374 else if (name.equals("biologicalSourceEvent")) { 4375 this.biologicalSourceEvent = new Identifier(); 4376 return this.biologicalSourceEvent; 4377 } 4378 else if (name.equals("manufacturer")) { 4379 throw new FHIRException("Cannot call addChild on a singleton property Device.manufacturer"); 4380 } 4381 else if (name.equals("manufactureDate")) { 4382 throw new FHIRException("Cannot call addChild on a singleton property Device.manufactureDate"); 4383 } 4384 else if (name.equals("expirationDate")) { 4385 throw new FHIRException("Cannot call addChild on a singleton property Device.expirationDate"); 4386 } 4387 else if (name.equals("lotNumber")) { 4388 throw new FHIRException("Cannot call addChild on a singleton property Device.lotNumber"); 4389 } 4390 else if (name.equals("serialNumber")) { 4391 throw new FHIRException("Cannot call addChild on a singleton property Device.serialNumber"); 4392 } 4393 else if (name.equals("name")) { 4394 return addName(); 4395 } 4396 else if (name.equals("modelNumber")) { 4397 throw new FHIRException("Cannot call addChild on a singleton property Device.modelNumber"); 4398 } 4399 else if (name.equals("partNumber")) { 4400 throw new FHIRException("Cannot call addChild on a singleton property Device.partNumber"); 4401 } 4402 else if (name.equals("category")) { 4403 return addCategory(); 4404 } 4405 else if (name.equals("type")) { 4406 return addType(); 4407 } 4408 else if (name.equals("version")) { 4409 return addVersion(); 4410 } 4411 else if (name.equals("conformsTo")) { 4412 return addConformsTo(); 4413 } 4414 else if (name.equals("property")) { 4415 return addProperty(); 4416 } 4417 else if (name.equals("mode")) { 4418 this.mode = new CodeableConcept(); 4419 return this.mode; 4420 } 4421 else if (name.equals("cycle")) { 4422 this.cycle = new Count(); 4423 return this.cycle; 4424 } 4425 else if (name.equals("duration")) { 4426 this.duration = new Duration(); 4427 return this.duration; 4428 } 4429 else if (name.equals("owner")) { 4430 this.owner = new Reference(); 4431 return this.owner; 4432 } 4433 else if (name.equals("contact")) { 4434 return addContact(); 4435 } 4436 else if (name.equals("location")) { 4437 this.location = new Reference(); 4438 return this.location; 4439 } 4440 else if (name.equals("url")) { 4441 throw new FHIRException("Cannot call addChild on a singleton property Device.url"); 4442 } 4443 else if (name.equals("endpoint")) { 4444 return addEndpoint(); 4445 } 4446 else if (name.equals("gateway")) { 4447 return addGateway(); 4448 } 4449 else if (name.equals("note")) { 4450 return addNote(); 4451 } 4452 else if (name.equals("safety")) { 4453 return addSafety(); 4454 } 4455 else if (name.equals("parent")) { 4456 this.parent = new Reference(); 4457 return this.parent; 4458 } 4459 else 4460 return super.addChild(name); 4461 } 4462 4463 public String fhirType() { 4464 return "Device"; 4465 4466 } 4467 4468 public Device copy() { 4469 Device dst = new Device(); 4470 copyValues(dst); 4471 return dst; 4472 } 4473 4474 public void copyValues(Device dst) { 4475 super.copyValues(dst); 4476 if (identifier != null) { 4477 dst.identifier = new ArrayList<Identifier>(); 4478 for (Identifier i : identifier) 4479 dst.identifier.add(i.copy()); 4480 }; 4481 dst.displayName = displayName == null ? null : displayName.copy(); 4482 dst.definition = definition == null ? null : definition.copy(); 4483 if (udiCarrier != null) { 4484 dst.udiCarrier = new ArrayList<DeviceUdiCarrierComponent>(); 4485 for (DeviceUdiCarrierComponent i : udiCarrier) 4486 dst.udiCarrier.add(i.copy()); 4487 }; 4488 dst.status = status == null ? null : status.copy(); 4489 dst.availabilityStatus = availabilityStatus == null ? null : availabilityStatus.copy(); 4490 dst.biologicalSourceEvent = biologicalSourceEvent == null ? null : biologicalSourceEvent.copy(); 4491 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 4492 dst.manufactureDate = manufactureDate == null ? null : manufactureDate.copy(); 4493 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 4494 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 4495 dst.serialNumber = serialNumber == null ? null : serialNumber.copy(); 4496 if (name != null) { 4497 dst.name = new ArrayList<DeviceNameComponent>(); 4498 for (DeviceNameComponent i : name) 4499 dst.name.add(i.copy()); 4500 }; 4501 dst.modelNumber = modelNumber == null ? null : modelNumber.copy(); 4502 dst.partNumber = partNumber == null ? null : partNumber.copy(); 4503 if (category != null) { 4504 dst.category = new ArrayList<CodeableConcept>(); 4505 for (CodeableConcept i : category) 4506 dst.category.add(i.copy()); 4507 }; 4508 if (type != null) { 4509 dst.type = new ArrayList<CodeableConcept>(); 4510 for (CodeableConcept i : type) 4511 dst.type.add(i.copy()); 4512 }; 4513 if (version != null) { 4514 dst.version = new ArrayList<DeviceVersionComponent>(); 4515 for (DeviceVersionComponent i : version) 4516 dst.version.add(i.copy()); 4517 }; 4518 if (conformsTo != null) { 4519 dst.conformsTo = new ArrayList<DeviceConformsToComponent>(); 4520 for (DeviceConformsToComponent i : conformsTo) 4521 dst.conformsTo.add(i.copy()); 4522 }; 4523 if (property != null) { 4524 dst.property = new ArrayList<DevicePropertyComponent>(); 4525 for (DevicePropertyComponent i : property) 4526 dst.property.add(i.copy()); 4527 }; 4528 dst.mode = mode == null ? null : mode.copy(); 4529 dst.cycle = cycle == null ? null : cycle.copy(); 4530 dst.duration = duration == null ? null : duration.copy(); 4531 dst.owner = owner == null ? null : owner.copy(); 4532 if (contact != null) { 4533 dst.contact = new ArrayList<ContactPoint>(); 4534 for (ContactPoint i : contact) 4535 dst.contact.add(i.copy()); 4536 }; 4537 dst.location = location == null ? null : location.copy(); 4538 dst.url = url == null ? null : url.copy(); 4539 if (endpoint != null) { 4540 dst.endpoint = new ArrayList<Reference>(); 4541 for (Reference i : endpoint) 4542 dst.endpoint.add(i.copy()); 4543 }; 4544 if (gateway != null) { 4545 dst.gateway = new ArrayList<CodeableReference>(); 4546 for (CodeableReference i : gateway) 4547 dst.gateway.add(i.copy()); 4548 }; 4549 if (note != null) { 4550 dst.note = new ArrayList<Annotation>(); 4551 for (Annotation i : note) 4552 dst.note.add(i.copy()); 4553 }; 4554 if (safety != null) { 4555 dst.safety = new ArrayList<CodeableConcept>(); 4556 for (CodeableConcept i : safety) 4557 dst.safety.add(i.copy()); 4558 }; 4559 dst.parent = parent == null ? null : parent.copy(); 4560 } 4561 4562 protected Device typedCopy() { 4563 return copy(); 4564 } 4565 4566 @Override 4567 public boolean equalsDeep(Base other_) { 4568 if (!super.equalsDeep(other_)) 4569 return false; 4570 if (!(other_ instanceof Device)) 4571 return false; 4572 Device o = (Device) other_; 4573 return compareDeep(identifier, o.identifier, true) && compareDeep(displayName, o.displayName, true) 4574 && compareDeep(definition, o.definition, true) && compareDeep(udiCarrier, o.udiCarrier, true) && compareDeep(status, o.status, true) 4575 && compareDeep(availabilityStatus, o.availabilityStatus, true) && compareDeep(biologicalSourceEvent, o.biologicalSourceEvent, true) 4576 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(manufactureDate, o.manufactureDate, true) 4577 && compareDeep(expirationDate, o.expirationDate, true) && compareDeep(lotNumber, o.lotNumber, true) 4578 && compareDeep(serialNumber, o.serialNumber, true) && compareDeep(name, o.name, true) && compareDeep(modelNumber, o.modelNumber, true) 4579 && compareDeep(partNumber, o.partNumber, true) && compareDeep(category, o.category, true) && compareDeep(type, o.type, true) 4580 && compareDeep(version, o.version, true) && compareDeep(conformsTo, o.conformsTo, true) && compareDeep(property, o.property, true) 4581 && compareDeep(mode, o.mode, true) && compareDeep(cycle, o.cycle, true) && compareDeep(duration, o.duration, true) 4582 && compareDeep(owner, o.owner, true) && compareDeep(contact, o.contact, true) && compareDeep(location, o.location, true) 4583 && compareDeep(url, o.url, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(gateway, o.gateway, true) 4584 && compareDeep(note, o.note, true) && compareDeep(safety, o.safety, true) && compareDeep(parent, o.parent, true) 4585 ; 4586 } 4587 4588 @Override 4589 public boolean equalsShallow(Base other_) { 4590 if (!super.equalsShallow(other_)) 4591 return false; 4592 if (!(other_ instanceof Device)) 4593 return false; 4594 Device o = (Device) other_; 4595 return compareValues(displayName, o.displayName, true) && compareValues(status, o.status, true) && compareValues(manufacturer, o.manufacturer, true) 4596 && compareValues(manufactureDate, o.manufactureDate, true) && compareValues(expirationDate, o.expirationDate, true) 4597 && compareValues(lotNumber, o.lotNumber, true) && compareValues(serialNumber, o.serialNumber, true) 4598 && compareValues(modelNumber, o.modelNumber, true) && compareValues(partNumber, o.partNumber, true) 4599 && compareValues(url, o.url, true); 4600 } 4601 4602 public boolean isEmpty() { 4603 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, displayName, definition 4604 , udiCarrier, status, availabilityStatus, biologicalSourceEvent, manufacturer, manufactureDate 4605 , expirationDate, lotNumber, serialNumber, name, modelNumber, partNumber, category 4606 , type, version, conformsTo, property, mode, cycle, duration, owner, contact 4607 , location, url, endpoint, gateway, note, safety, parent); 4608 } 4609 4610 @Override 4611 public ResourceType getResourceType() { 4612 return ResourceType.Device; 4613 } 4614 4615 /** 4616 * Search parameter: <b>biological-source-event</b> 4617 * <p> 4618 * Description: <b>The biological source for the device</b><br> 4619 * Type: <b>token</b><br> 4620 * Path: <b>Device.biologicalSourceEvent</b><br> 4621 * </p> 4622 */ 4623 @SearchParamDefinition(name="biological-source-event", path="Device.biologicalSourceEvent", description="The biological source for the device", type="token" ) 4624 public static final String SP_BIOLOGICAL_SOURCE_EVENT = "biological-source-event"; 4625 /** 4626 * <b>Fluent Client</b> search parameter constant for <b>biological-source-event</b> 4627 * <p> 4628 * Description: <b>The biological source for the device</b><br> 4629 * Type: <b>token</b><br> 4630 * Path: <b>Device.biologicalSourceEvent</b><br> 4631 * </p> 4632 */ 4633 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BIOLOGICAL_SOURCE_EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BIOLOGICAL_SOURCE_EVENT); 4634 4635 /** 4636 * Search parameter: <b>code-value-concept</b> 4637 * <p> 4638 * Description: <b>Code and value parameter pair</b><br> 4639 * Type: <b>composite</b><br> 4640 * Path: <b>Device</b><br> 4641 * </p> 4642 */ 4643 @SearchParamDefinition(name="code-value-concept", path="Device", description="Code and value parameter pair", type="composite", compositeOf={"specification", "version"} ) 4644 public static final String SP_CODE_VALUE_CONCEPT = "code-value-concept"; 4645 /** 4646 * <b>Fluent Client</b> search parameter constant for <b>code-value-concept</b> 4647 * <p> 4648 * Description: <b>Code and value parameter pair</b><br> 4649 * Type: <b>composite</b><br> 4650 * Path: <b>Device</b><br> 4651 * </p> 4652 */ 4653 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam> CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam>(SP_CODE_VALUE_CONCEPT); 4654 4655 /** 4656 * Search parameter: <b>code</b> 4657 * <p> 4658 * Description: <b>The definition / type of the device (code)</b><br> 4659 * Type: <b>token</b><br> 4660 * Path: <b>Device.definition.concept</b><br> 4661 * </p> 4662 */ 4663 @SearchParamDefinition(name="code", path="Device.definition.concept", description="The definition / type of the device (code)", type="token" ) 4664 public static final String SP_CODE = "code"; 4665 /** 4666 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4667 * <p> 4668 * Description: <b>The definition / type of the device (code)</b><br> 4669 * Type: <b>token</b><br> 4670 * Path: <b>Device.definition.concept</b><br> 4671 * </p> 4672 */ 4673 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4674 4675 /** 4676 * Search parameter: <b>definition</b> 4677 * <p> 4678 * Description: <b>The definition / type of the device</b><br> 4679 * Type: <b>reference</b><br> 4680 * Path: <b>Device.definition.reference</b><br> 4681 * </p> 4682 */ 4683 @SearchParamDefinition(name="definition", path="Device.definition.reference", description="The definition / type of the device", type="reference", target={DeviceDefinition.class } ) 4684 public static final String SP_DEFINITION = "definition"; 4685 /** 4686 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 4687 * <p> 4688 * Description: <b>The definition / type of the device</b><br> 4689 * Type: <b>reference</b><br> 4690 * Path: <b>Device.definition.reference</b><br> 4691 * </p> 4692 */ 4693 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 4694 4695/** 4696 * Constant for fluent queries to be used to add include statements. Specifies 4697 * the path value of "<b>Device:definition</b>". 4698 */ 4699 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("Device:definition").toLocked(); 4700 4701 /** 4702 * Search parameter: <b>device-name</b> 4703 * <p> 4704 * Description: <b>A server defined search that may match any of the string fields in Device.name or Device.type.</b><br> 4705 * Type: <b>string</b><br> 4706 * Path: <b>Device.name.value | Device.type.coding.display | Device.type.text</b><br> 4707 * </p> 4708 */ 4709 @SearchParamDefinition(name="device-name", path="Device.name.value | Device.type.coding.display | Device.type.text", description="A server defined search that may match any of the string fields in Device.name or Device.type.", type="string" ) 4710 public static final String SP_DEVICE_NAME = "device-name"; 4711 /** 4712 * <b>Fluent Client</b> search parameter constant for <b>device-name</b> 4713 * <p> 4714 * Description: <b>A server defined search that may match any of the string fields in Device.name or Device.type.</b><br> 4715 * Type: <b>string</b><br> 4716 * Path: <b>Device.name.value | Device.type.coding.display | Device.type.text</b><br> 4717 * </p> 4718 */ 4719 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEVICE_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DEVICE_NAME); 4720 4721 /** 4722 * Search parameter: <b>expiration-date</b> 4723 * <p> 4724 * Description: <b>The expiration date of the device</b><br> 4725 * Type: <b>date</b><br> 4726 * Path: <b>Device.expirationDate</b><br> 4727 * </p> 4728 */ 4729 @SearchParamDefinition(name="expiration-date", path="Device.expirationDate", description="The expiration date of the device", type="date" ) 4730 public static final String SP_EXPIRATION_DATE = "expiration-date"; 4731 /** 4732 * <b>Fluent Client</b> search parameter constant for <b>expiration-date</b> 4733 * <p> 4734 * Description: <b>The expiration date of the device</b><br> 4735 * Type: <b>date</b><br> 4736 * Path: <b>Device.expirationDate</b><br> 4737 * </p> 4738 */ 4739 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRATION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EXPIRATION_DATE); 4740 4741 /** 4742 * Search parameter: <b>identifier</b> 4743 * <p> 4744 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 4745 * Type: <b>token</b><br> 4746 * Path: <b>Device.identifier</b><br> 4747 * </p> 4748 */ 4749 @SearchParamDefinition(name="identifier", path="Device.identifier", description="Instance id from manufacturer, owner, and others", type="token" ) 4750 public static final String SP_IDENTIFIER = "identifier"; 4751 /** 4752 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4753 * <p> 4754 * Description: <b>Instance id from manufacturer, owner, and others</b><br> 4755 * Type: <b>token</b><br> 4756 * Path: <b>Device.identifier</b><br> 4757 * </p> 4758 */ 4759 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4760 4761 /** 4762 * Search parameter: <b>location</b> 4763 * <p> 4764 * Description: <b>A location, where the resource is found</b><br> 4765 * Type: <b>reference</b><br> 4766 * Path: <b>Device.location</b><br> 4767 * </p> 4768 */ 4769 @SearchParamDefinition(name="location", path="Device.location", description="A location, where the resource is found", type="reference", target={Location.class } ) 4770 public static final String SP_LOCATION = "location"; 4771 /** 4772 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4773 * <p> 4774 * Description: <b>A location, where the resource is found</b><br> 4775 * Type: <b>reference</b><br> 4776 * Path: <b>Device.location</b><br> 4777 * </p> 4778 */ 4779 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 4780 4781/** 4782 * Constant for fluent queries to be used to add include statements. Specifies 4783 * the path value of "<b>Device:location</b>". 4784 */ 4785 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Device:location").toLocked(); 4786 4787 /** 4788 * Search parameter: <b>lot-number</b> 4789 * <p> 4790 * Description: <b>The lot number of the device</b><br> 4791 * Type: <b>string</b><br> 4792 * Path: <b>Device.lotNumber</b><br> 4793 * </p> 4794 */ 4795 @SearchParamDefinition(name="lot-number", path="Device.lotNumber", description="The lot number of the device", type="string" ) 4796 public static final String SP_LOT_NUMBER = "lot-number"; 4797 /** 4798 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 4799 * <p> 4800 * Description: <b>The lot number of the device</b><br> 4801 * Type: <b>string</b><br> 4802 * Path: <b>Device.lotNumber</b><br> 4803 * </p> 4804 */ 4805 public static final ca.uhn.fhir.rest.gclient.StringClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_LOT_NUMBER); 4806 4807 /** 4808 * Search parameter: <b>manufacture-date</b> 4809 * <p> 4810 * Description: <b>The manufacture date of the device</b><br> 4811 * Type: <b>date</b><br> 4812 * Path: <b>Device.manufactureDate</b><br> 4813 * </p> 4814 */ 4815 @SearchParamDefinition(name="manufacture-date", path="Device.manufactureDate", description="The manufacture date of the device", type="date" ) 4816 public static final String SP_MANUFACTURE_DATE = "manufacture-date"; 4817 /** 4818 * <b>Fluent Client</b> search parameter constant for <b>manufacture-date</b> 4819 * <p> 4820 * Description: <b>The manufacture date of the device</b><br> 4821 * Type: <b>date</b><br> 4822 * Path: <b>Device.manufactureDate</b><br> 4823 * </p> 4824 */ 4825 public static final ca.uhn.fhir.rest.gclient.DateClientParam MANUFACTURE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_MANUFACTURE_DATE); 4826 4827 /** 4828 * Search parameter: <b>manufacturer</b> 4829 * <p> 4830 * Description: <b>The manufacturer of the device</b><br> 4831 * Type: <b>string</b><br> 4832 * Path: <b>Device.manufacturer</b><br> 4833 * </p> 4834 */ 4835 @SearchParamDefinition(name="manufacturer", path="Device.manufacturer", description="The manufacturer of the device", type="string" ) 4836 public static final String SP_MANUFACTURER = "manufacturer"; 4837 /** 4838 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 4839 * <p> 4840 * Description: <b>The manufacturer of the device</b><br> 4841 * Type: <b>string</b><br> 4842 * Path: <b>Device.manufacturer</b><br> 4843 * </p> 4844 */ 4845 public static final ca.uhn.fhir.rest.gclient.StringClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_MANUFACTURER); 4846 4847 /** 4848 * Search parameter: <b>model</b> 4849 * <p> 4850 * Description: <b>The model of the device</b><br> 4851 * Type: <b>string</b><br> 4852 * Path: <b>Device.modelNumber</b><br> 4853 * </p> 4854 */ 4855 @SearchParamDefinition(name="model", path="Device.modelNumber", description="The model of the device", type="string" ) 4856 public static final String SP_MODEL = "model"; 4857 /** 4858 * <b>Fluent Client</b> search parameter constant for <b>model</b> 4859 * <p> 4860 * Description: <b>The model of the device</b><br> 4861 * Type: <b>string</b><br> 4862 * Path: <b>Device.modelNumber</b><br> 4863 * </p> 4864 */ 4865 public static final ca.uhn.fhir.rest.gclient.StringClientParam MODEL = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_MODEL); 4866 4867 /** 4868 * Search parameter: <b>organization</b> 4869 * <p> 4870 * Description: <b>The organization responsible for the device</b><br> 4871 * Type: <b>reference</b><br> 4872 * Path: <b>Device.owner</b><br> 4873 * </p> 4874 */ 4875 @SearchParamDefinition(name="organization", path="Device.owner", description="The organization responsible for the device", type="reference", target={Organization.class } ) 4876 public static final String SP_ORGANIZATION = "organization"; 4877 /** 4878 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 4879 * <p> 4880 * Description: <b>The organization responsible for the device</b><br> 4881 * Type: <b>reference</b><br> 4882 * Path: <b>Device.owner</b><br> 4883 * </p> 4884 */ 4885 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 4886 4887/** 4888 * Constant for fluent queries to be used to add include statements. Specifies 4889 * the path value of "<b>Device:organization</b>". 4890 */ 4891 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Device:organization").toLocked(); 4892 4893 /** 4894 * Search parameter: <b>parent</b> 4895 * <p> 4896 * Description: <b>The parent device</b><br> 4897 * Type: <b>reference</b><br> 4898 * Path: <b>Device.parent</b><br> 4899 * </p> 4900 */ 4901 @SearchParamDefinition(name="parent", path="Device.parent", description="The parent device", type="reference", target={Device.class } ) 4902 public static final String SP_PARENT = "parent"; 4903 /** 4904 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 4905 * <p> 4906 * Description: <b>The parent device</b><br> 4907 * Type: <b>reference</b><br> 4908 * Path: <b>Device.parent</b><br> 4909 * </p> 4910 */ 4911 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 4912 4913/** 4914 * Constant for fluent queries to be used to add include statements. Specifies 4915 * the path value of "<b>Device:parent</b>". 4916 */ 4917 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("Device:parent").toLocked(); 4918 4919 /** 4920 * Search parameter: <b>serial-number</b> 4921 * <p> 4922 * Description: <b>The serial number of the device</b><br> 4923 * Type: <b>string</b><br> 4924 * Path: <b>Device.serialNumber | Device.identifier.where(type='SNO')</b><br> 4925 * </p> 4926 */ 4927 @SearchParamDefinition(name="serial-number", path="Device.serialNumber | Device.identifier.where(type='SNO')", description="The serial number of the device", type="string" ) 4928 public static final String SP_SERIAL_NUMBER = "serial-number"; 4929 /** 4930 * <b>Fluent Client</b> search parameter constant for <b>serial-number</b> 4931 * <p> 4932 * Description: <b>The serial number of the device</b><br> 4933 * Type: <b>string</b><br> 4934 * Path: <b>Device.serialNumber | Device.identifier.where(type='SNO')</b><br> 4935 * </p> 4936 */ 4937 public static final ca.uhn.fhir.rest.gclient.StringClientParam SERIAL_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SERIAL_NUMBER); 4938 4939 /** 4940 * Search parameter: <b>specification-version</b> 4941 * <p> 4942 * Description: <b>A composite of both specification and version</b><br> 4943 * Type: <b>composite</b><br> 4944 * Path: <b>Device.conformsTo</b><br> 4945 * </p> 4946 */ 4947 @SearchParamDefinition(name="specification-version", path="Device.conformsTo", description="A composite of both specification and version", type="composite", compositeOf={"specification", "version"} ) 4948 public static final String SP_SPECIFICATION_VERSION = "specification-version"; 4949 /** 4950 * <b>Fluent Client</b> search parameter constant for <b>specification-version</b> 4951 * <p> 4952 * Description: <b>A composite of both specification and version</b><br> 4953 * Type: <b>composite</b><br> 4954 * Path: <b>Device.conformsTo</b><br> 4955 * </p> 4956 */ 4957 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam> SPECIFICATION_VERSION = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam>(SP_SPECIFICATION_VERSION); 4958 4959 /** 4960 * Search parameter: <b>specification</b> 4961 * <p> 4962 * Description: <b>The standards, specifications, or formal guidances.</b><br> 4963 * Type: <b>token</b><br> 4964 * Path: <b>Device.conformsTo.specification</b><br> 4965 * </p> 4966 */ 4967 @SearchParamDefinition(name="specification", path="Device.conformsTo.specification", description="The standards, specifications, or formal guidances.", type="token" ) 4968 public static final String SP_SPECIFICATION = "specification"; 4969 /** 4970 * <b>Fluent Client</b> search parameter constant for <b>specification</b> 4971 * <p> 4972 * Description: <b>The standards, specifications, or formal guidances.</b><br> 4973 * Type: <b>token</b><br> 4974 * Path: <b>Device.conformsTo.specification</b><br> 4975 * </p> 4976 */ 4977 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIFICATION); 4978 4979 /** 4980 * Search parameter: <b>status</b> 4981 * <p> 4982 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 4983 * Type: <b>token</b><br> 4984 * Path: <b>Device.status</b><br> 4985 * </p> 4986 */ 4987 @SearchParamDefinition(name="status", path="Device.status", description="active | inactive | entered-in-error | unknown", type="token" ) 4988 public static final String SP_STATUS = "status"; 4989 /** 4990 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4991 * <p> 4992 * Description: <b>active | inactive | entered-in-error | unknown</b><br> 4993 * Type: <b>token</b><br> 4994 * Path: <b>Device.status</b><br> 4995 * </p> 4996 */ 4997 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4998 4999 /** 5000 * Search parameter: <b>type</b> 5001 * <p> 5002 * Description: <b>The type of the device</b><br> 5003 * Type: <b>token</b><br> 5004 * Path: <b>Device.type</b><br> 5005 * </p> 5006 */ 5007 @SearchParamDefinition(name="type", path="Device.type", description="The type of the device", type="token" ) 5008 public static final String SP_TYPE = "type"; 5009 /** 5010 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5011 * <p> 5012 * Description: <b>The type of the device</b><br> 5013 * Type: <b>token</b><br> 5014 * Path: <b>Device.type</b><br> 5015 * </p> 5016 */ 5017 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 5018 5019 /** 5020 * Search parameter: <b>udi-carrier</b> 5021 * <p> 5022 * Description: <b>UDI Barcode (RFID or other technology) string in *HRF* format.</b><br> 5023 * Type: <b>string</b><br> 5024 * Path: <b>Device.udiCarrier.carrierHRF</b><br> 5025 * </p> 5026 */ 5027 @SearchParamDefinition(name="udi-carrier", path="Device.udiCarrier.carrierHRF", description="UDI Barcode (RFID or other technology) string in *HRF* format.", type="string" ) 5028 public static final String SP_UDI_CARRIER = "udi-carrier"; 5029 /** 5030 * <b>Fluent Client</b> search parameter constant for <b>udi-carrier</b> 5031 * <p> 5032 * Description: <b>UDI Barcode (RFID or other technology) string in *HRF* format.</b><br> 5033 * Type: <b>string</b><br> 5034 * Path: <b>Device.udiCarrier.carrierHRF</b><br> 5035 * </p> 5036 */ 5037 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_CARRIER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_UDI_CARRIER); 5038 5039 /** 5040 * Search parameter: <b>udi-di</b> 5041 * <p> 5042 * Description: <b>The udi Device Identifier (DI)</b><br> 5043 * Type: <b>string</b><br> 5044 * Path: <b>Device.udiCarrier.deviceIdentifier</b><br> 5045 * </p> 5046 */ 5047 @SearchParamDefinition(name="udi-di", path="Device.udiCarrier.deviceIdentifier", description="The udi Device Identifier (DI)", type="string" ) 5048 public static final String SP_UDI_DI = "udi-di"; 5049 /** 5050 * <b>Fluent Client</b> search parameter constant for <b>udi-di</b> 5051 * <p> 5052 * Description: <b>The udi Device Identifier (DI)</b><br> 5053 * Type: <b>string</b><br> 5054 * Path: <b>Device.udiCarrier.deviceIdentifier</b><br> 5055 * </p> 5056 */ 5057 public static final ca.uhn.fhir.rest.gclient.StringClientParam UDI_DI = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_UDI_DI); 5058 5059 /** 5060 * Search parameter: <b>url</b> 5061 * <p> 5062 * Description: <b>Network address to contact device</b><br> 5063 * Type: <b>uri</b><br> 5064 * Path: <b>Device.url</b><br> 5065 * </p> 5066 */ 5067 @SearchParamDefinition(name="url", path="Device.url", description="Network address to contact device", type="uri" ) 5068 public static final String SP_URL = "url"; 5069 /** 5070 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5071 * <p> 5072 * Description: <b>Network address to contact device</b><br> 5073 * Type: <b>uri</b><br> 5074 * Path: <b>Device.url</b><br> 5075 * </p> 5076 */ 5077 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5078 5079 /** 5080 * Search parameter: <b>version</b> 5081 * <p> 5082 * Description: <b>The specific version of the device</b><br> 5083 * Type: <b>string</b><br> 5084 * Path: <b>Device.version.value</b><br> 5085 * </p> 5086 */ 5087 @SearchParamDefinition(name="version", path="Device.version.value", description="The specific version of the device", type="string" ) 5088 public static final String SP_VERSION = "version"; 5089 /** 5090 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5091 * <p> 5092 * Description: <b>The specific version of the device</b><br> 5093 * Type: <b>string</b><br> 5094 * Path: <b>Device.version.value</b><br> 5095 * </p> 5096 */ 5097 public static final ca.uhn.fhir.rest.gclient.StringClientParam VERSION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VERSION); 5098 5099 5100} 5101