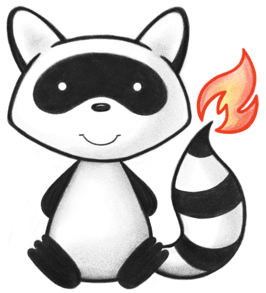
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * A record of association of a device. 051 */ 052@ResourceDef(name="DeviceAssociation", profile="http://hl7.org/fhir/StructureDefinition/DeviceAssociation") 053public class DeviceAssociation extends DomainResource { 054 055 @Block() 056 public static class DeviceAssociationOperationComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Device operational condition corresponding to the association. 059 */ 060 @Child(name = "status", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="Device operational condition", formalDefinition="Device operational condition corresponding to the association." ) 062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceassociation-operationstatus") 063 protected CodeableConcept status; 064 065 /** 066 * The individual performing the action enabled by the device. 067 */ 068 @Child(name = "operator", type = {Patient.class, Practitioner.class, RelatedPerson.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 069 @Description(shortDefinition="The individual performing the action enabled by the device", formalDefinition="The individual performing the action enabled by the device." ) 070 protected List<Reference> operator; 071 072 /** 073 * Begin and end dates and times for the device's operation. 074 */ 075 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 076 @Description(shortDefinition="Begin and end dates and times for the device's operation", formalDefinition="Begin and end dates and times for the device's operation." ) 077 protected Period period; 078 079 private static final long serialVersionUID = -1587836408L; 080 081 /** 082 * Constructor 083 */ 084 public DeviceAssociationOperationComponent() { 085 super(); 086 } 087 088 /** 089 * Constructor 090 */ 091 public DeviceAssociationOperationComponent(CodeableConcept status) { 092 super(); 093 this.setStatus(status); 094 } 095 096 /** 097 * @return {@link #status} (Device operational condition corresponding to the association.) 098 */ 099 public CodeableConcept getStatus() { 100 if (this.status == null) 101 if (Configuration.errorOnAutoCreate()) 102 throw new Error("Attempt to auto-create DeviceAssociationOperationComponent.status"); 103 else if (Configuration.doAutoCreate()) 104 this.status = new CodeableConcept(); // cc 105 return this.status; 106 } 107 108 public boolean hasStatus() { 109 return this.status != null && !this.status.isEmpty(); 110 } 111 112 /** 113 * @param value {@link #status} (Device operational condition corresponding to the association.) 114 */ 115 public DeviceAssociationOperationComponent setStatus(CodeableConcept value) { 116 this.status = value; 117 return this; 118 } 119 120 /** 121 * @return {@link #operator} (The individual performing the action enabled by the device.) 122 */ 123 public List<Reference> getOperator() { 124 if (this.operator == null) 125 this.operator = new ArrayList<Reference>(); 126 return this.operator; 127 } 128 129 /** 130 * @return Returns a reference to <code>this</code> for easy method chaining 131 */ 132 public DeviceAssociationOperationComponent setOperator(List<Reference> theOperator) { 133 this.operator = theOperator; 134 return this; 135 } 136 137 public boolean hasOperator() { 138 if (this.operator == null) 139 return false; 140 for (Reference item : this.operator) 141 if (!item.isEmpty()) 142 return true; 143 return false; 144 } 145 146 public Reference addOperator() { //3 147 Reference t = new Reference(); 148 if (this.operator == null) 149 this.operator = new ArrayList<Reference>(); 150 this.operator.add(t); 151 return t; 152 } 153 154 public DeviceAssociationOperationComponent addOperator(Reference t) { //3 155 if (t == null) 156 return this; 157 if (this.operator == null) 158 this.operator = new ArrayList<Reference>(); 159 this.operator.add(t); 160 return this; 161 } 162 163 /** 164 * @return The first repetition of repeating field {@link #operator}, creating it if it does not already exist {3} 165 */ 166 public Reference getOperatorFirstRep() { 167 if (getOperator().isEmpty()) { 168 addOperator(); 169 } 170 return getOperator().get(0); 171 } 172 173 /** 174 * @return {@link #period} (Begin and end dates and times for the device's operation.) 175 */ 176 public Period getPeriod() { 177 if (this.period == null) 178 if (Configuration.errorOnAutoCreate()) 179 throw new Error("Attempt to auto-create DeviceAssociationOperationComponent.period"); 180 else if (Configuration.doAutoCreate()) 181 this.period = new Period(); // cc 182 return this.period; 183 } 184 185 public boolean hasPeriod() { 186 return this.period != null && !this.period.isEmpty(); 187 } 188 189 /** 190 * @param value {@link #period} (Begin and end dates and times for the device's operation.) 191 */ 192 public DeviceAssociationOperationComponent setPeriod(Period value) { 193 this.period = value; 194 return this; 195 } 196 197 protected void listChildren(List<Property> children) { 198 super.listChildren(children); 199 children.add(new Property("status", "CodeableConcept", "Device operational condition corresponding to the association.", 0, 1, status)); 200 children.add(new Property("operator", "Reference(Patient|Practitioner|RelatedPerson)", "The individual performing the action enabled by the device.", 0, java.lang.Integer.MAX_VALUE, operator)); 201 children.add(new Property("period", "Period", "Begin and end dates and times for the device's operation.", 0, 1, period)); 202 } 203 204 @Override 205 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 206 switch (_hash) { 207 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Device operational condition corresponding to the association.", 0, 1, status); 208 case -500553564: /*operator*/ return new Property("operator", "Reference(Patient|Practitioner|RelatedPerson)", "The individual performing the action enabled by the device.", 0, java.lang.Integer.MAX_VALUE, operator); 209 case -991726143: /*period*/ return new Property("period", "Period", "Begin and end dates and times for the device's operation.", 0, 1, period); 210 default: return super.getNamedProperty(_hash, _name, _checkValid); 211 } 212 213 } 214 215 @Override 216 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 217 switch (hash) { 218 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 219 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : this.operator.toArray(new Base[this.operator.size()]); // Reference 220 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 221 default: return super.getProperty(hash, name, checkValid); 222 } 223 224 } 225 226 @Override 227 public Base setProperty(int hash, String name, Base value) throws FHIRException { 228 switch (hash) { 229 case -892481550: // status 230 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 231 return value; 232 case -500553564: // operator 233 this.getOperator().add(TypeConvertor.castToReference(value)); // Reference 234 return value; 235 case -991726143: // period 236 this.period = TypeConvertor.castToPeriod(value); // Period 237 return value; 238 default: return super.setProperty(hash, name, value); 239 } 240 241 } 242 243 @Override 244 public Base setProperty(String name, Base value) throws FHIRException { 245 if (name.equals("status")) { 246 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 247 } else if (name.equals("operator")) { 248 this.getOperator().add(TypeConvertor.castToReference(value)); 249 } else if (name.equals("period")) { 250 this.period = TypeConvertor.castToPeriod(value); // Period 251 } else 252 return super.setProperty(name, value); 253 return value; 254 } 255 256 @Override 257 public Base makeProperty(int hash, String name) throws FHIRException { 258 switch (hash) { 259 case -892481550: return getStatus(); 260 case -500553564: return addOperator(); 261 case -991726143: return getPeriod(); 262 default: return super.makeProperty(hash, name); 263 } 264 265 } 266 267 @Override 268 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 269 switch (hash) { 270 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 271 case -500553564: /*operator*/ return new String[] {"Reference"}; 272 case -991726143: /*period*/ return new String[] {"Period"}; 273 default: return super.getTypesForProperty(hash, name); 274 } 275 276 } 277 278 @Override 279 public Base addChild(String name) throws FHIRException { 280 if (name.equals("status")) { 281 this.status = new CodeableConcept(); 282 return this.status; 283 } 284 else if (name.equals("operator")) { 285 return addOperator(); 286 } 287 else if (name.equals("period")) { 288 this.period = new Period(); 289 return this.period; 290 } 291 else 292 return super.addChild(name); 293 } 294 295 public DeviceAssociationOperationComponent copy() { 296 DeviceAssociationOperationComponent dst = new DeviceAssociationOperationComponent(); 297 copyValues(dst); 298 return dst; 299 } 300 301 public void copyValues(DeviceAssociationOperationComponent dst) { 302 super.copyValues(dst); 303 dst.status = status == null ? null : status.copy(); 304 if (operator != null) { 305 dst.operator = new ArrayList<Reference>(); 306 for (Reference i : operator) 307 dst.operator.add(i.copy()); 308 }; 309 dst.period = period == null ? null : period.copy(); 310 } 311 312 @Override 313 public boolean equalsDeep(Base other_) { 314 if (!super.equalsDeep(other_)) 315 return false; 316 if (!(other_ instanceof DeviceAssociationOperationComponent)) 317 return false; 318 DeviceAssociationOperationComponent o = (DeviceAssociationOperationComponent) other_; 319 return compareDeep(status, o.status, true) && compareDeep(operator, o.operator, true) && compareDeep(period, o.period, true) 320 ; 321 } 322 323 @Override 324 public boolean equalsShallow(Base other_) { 325 if (!super.equalsShallow(other_)) 326 return false; 327 if (!(other_ instanceof DeviceAssociationOperationComponent)) 328 return false; 329 DeviceAssociationOperationComponent o = (DeviceAssociationOperationComponent) other_; 330 return true; 331 } 332 333 public boolean isEmpty() { 334 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, operator, period 335 ); 336 } 337 338 public String fhirType() { 339 return "DeviceAssociation.operation"; 340 341 } 342 343 } 344 345 /** 346 * Instance identifier. 347 */ 348 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 349 @Description(shortDefinition="Instance identifier", formalDefinition="Instance identifier." ) 350 protected List<Identifier> identifier; 351 352 /** 353 * Reference to the devices associated with the patient or group. 354 */ 355 @Child(name = "device", type = {Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 356 @Description(shortDefinition="Reference to the devices associated with the patient or group", formalDefinition="Reference to the devices associated with the patient or group." ) 357 protected Reference device; 358 359 /** 360 * Describes the relationship between the device and subject. 361 */ 362 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 363 @Description(shortDefinition="Describes the relationship between the device and subject", formalDefinition="Describes the relationship between the device and subject." ) 364 protected List<CodeableConcept> category; 365 366 /** 367 * Indicates the state of the Device association. 368 */ 369 @Child(name = "status", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 370 @Description(shortDefinition="implanted | explanted | attached | entered-in-error | unknown", formalDefinition="Indicates the state of the Device association." ) 371 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceassociation-status") 372 protected CodeableConcept status; 373 374 /** 375 * The reasons given for the current association status. 376 */ 377 @Child(name = "statusReason", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 378 @Description(shortDefinition="The reasons given for the current association status", formalDefinition="The reasons given for the current association status." ) 379 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceassociation-status-reason") 380 protected List<CodeableConcept> statusReason; 381 382 /** 383 * The individual, group of individuals or device that the device is on or associated with. 384 */ 385 @Child(name = "subject", type = {Patient.class, Group.class, Practitioner.class, RelatedPerson.class, Device.class}, order=5, min=0, max=1, modifier=false, summary=true) 386 @Description(shortDefinition="The individual, group of individuals or device that the device is on or associated with", formalDefinition="The individual, group of individuals or device that the device is on or associated with." ) 387 protected Reference subject; 388 389 /** 390 * Current anatomical location of the device in/on subject. 391 */ 392 @Child(name = "bodyStructure", type = {BodyStructure.class}, order=6, min=0, max=1, modifier=false, summary=true) 393 @Description(shortDefinition="Current anatomical location of the device in/on subject", formalDefinition="Current anatomical location of the device in/on subject." ) 394 protected Reference bodyStructure; 395 396 /** 397 * Begin and end dates and times for the device association. 398 */ 399 @Child(name = "period", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 400 @Description(shortDefinition="Begin and end dates and times for the device association", formalDefinition="Begin and end dates and times for the device association." ) 401 protected Period period; 402 403 /** 404 * The details about the device when it is in use to describe its operation. 405 */ 406 @Child(name = "operation", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 407 @Description(shortDefinition="The details about the device when it is in use to describe its operation", formalDefinition="The details about the device when it is in use to describe its operation." ) 408 protected List<DeviceAssociationOperationComponent> operation; 409 410 private static final long serialVersionUID = 1892071017L; 411 412 /** 413 * Constructor 414 */ 415 public DeviceAssociation() { 416 super(); 417 } 418 419 /** 420 * Constructor 421 */ 422 public DeviceAssociation(Reference device, CodeableConcept status) { 423 super(); 424 this.setDevice(device); 425 this.setStatus(status); 426 } 427 428 /** 429 * @return {@link #identifier} (Instance identifier.) 430 */ 431 public List<Identifier> getIdentifier() { 432 if (this.identifier == null) 433 this.identifier = new ArrayList<Identifier>(); 434 return this.identifier; 435 } 436 437 /** 438 * @return Returns a reference to <code>this</code> for easy method chaining 439 */ 440 public DeviceAssociation setIdentifier(List<Identifier> theIdentifier) { 441 this.identifier = theIdentifier; 442 return this; 443 } 444 445 public boolean hasIdentifier() { 446 if (this.identifier == null) 447 return false; 448 for (Identifier item : this.identifier) 449 if (!item.isEmpty()) 450 return true; 451 return false; 452 } 453 454 public Identifier addIdentifier() { //3 455 Identifier t = new Identifier(); 456 if (this.identifier == null) 457 this.identifier = new ArrayList<Identifier>(); 458 this.identifier.add(t); 459 return t; 460 } 461 462 public DeviceAssociation addIdentifier(Identifier t) { //3 463 if (t == null) 464 return this; 465 if (this.identifier == null) 466 this.identifier = new ArrayList<Identifier>(); 467 this.identifier.add(t); 468 return this; 469 } 470 471 /** 472 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 473 */ 474 public Identifier getIdentifierFirstRep() { 475 if (getIdentifier().isEmpty()) { 476 addIdentifier(); 477 } 478 return getIdentifier().get(0); 479 } 480 481 /** 482 * @return {@link #device} (Reference to the devices associated with the patient or group.) 483 */ 484 public Reference getDevice() { 485 if (this.device == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create DeviceAssociation.device"); 488 else if (Configuration.doAutoCreate()) 489 this.device = new Reference(); // cc 490 return this.device; 491 } 492 493 public boolean hasDevice() { 494 return this.device != null && !this.device.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #device} (Reference to the devices associated with the patient or group.) 499 */ 500 public DeviceAssociation setDevice(Reference value) { 501 this.device = value; 502 return this; 503 } 504 505 /** 506 * @return {@link #category} (Describes the relationship between the device and subject.) 507 */ 508 public List<CodeableConcept> getCategory() { 509 if (this.category == null) 510 this.category = new ArrayList<CodeableConcept>(); 511 return this.category; 512 } 513 514 /** 515 * @return Returns a reference to <code>this</code> for easy method chaining 516 */ 517 public DeviceAssociation setCategory(List<CodeableConcept> theCategory) { 518 this.category = theCategory; 519 return this; 520 } 521 522 public boolean hasCategory() { 523 if (this.category == null) 524 return false; 525 for (CodeableConcept item : this.category) 526 if (!item.isEmpty()) 527 return true; 528 return false; 529 } 530 531 public CodeableConcept addCategory() { //3 532 CodeableConcept t = new CodeableConcept(); 533 if (this.category == null) 534 this.category = new ArrayList<CodeableConcept>(); 535 this.category.add(t); 536 return t; 537 } 538 539 public DeviceAssociation addCategory(CodeableConcept t) { //3 540 if (t == null) 541 return this; 542 if (this.category == null) 543 this.category = new ArrayList<CodeableConcept>(); 544 this.category.add(t); 545 return this; 546 } 547 548 /** 549 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 550 */ 551 public CodeableConcept getCategoryFirstRep() { 552 if (getCategory().isEmpty()) { 553 addCategory(); 554 } 555 return getCategory().get(0); 556 } 557 558 /** 559 * @return {@link #status} (Indicates the state of the Device association.) 560 */ 561 public CodeableConcept getStatus() { 562 if (this.status == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create DeviceAssociation.status"); 565 else if (Configuration.doAutoCreate()) 566 this.status = new CodeableConcept(); // cc 567 return this.status; 568 } 569 570 public boolean hasStatus() { 571 return this.status != null && !this.status.isEmpty(); 572 } 573 574 /** 575 * @param value {@link #status} (Indicates the state of the Device association.) 576 */ 577 public DeviceAssociation setStatus(CodeableConcept value) { 578 this.status = value; 579 return this; 580 } 581 582 /** 583 * @return {@link #statusReason} (The reasons given for the current association status.) 584 */ 585 public List<CodeableConcept> getStatusReason() { 586 if (this.statusReason == null) 587 this.statusReason = new ArrayList<CodeableConcept>(); 588 return this.statusReason; 589 } 590 591 /** 592 * @return Returns a reference to <code>this</code> for easy method chaining 593 */ 594 public DeviceAssociation setStatusReason(List<CodeableConcept> theStatusReason) { 595 this.statusReason = theStatusReason; 596 return this; 597 } 598 599 public boolean hasStatusReason() { 600 if (this.statusReason == null) 601 return false; 602 for (CodeableConcept item : this.statusReason) 603 if (!item.isEmpty()) 604 return true; 605 return false; 606 } 607 608 public CodeableConcept addStatusReason() { //3 609 CodeableConcept t = new CodeableConcept(); 610 if (this.statusReason == null) 611 this.statusReason = new ArrayList<CodeableConcept>(); 612 this.statusReason.add(t); 613 return t; 614 } 615 616 public DeviceAssociation addStatusReason(CodeableConcept t) { //3 617 if (t == null) 618 return this; 619 if (this.statusReason == null) 620 this.statusReason = new ArrayList<CodeableConcept>(); 621 this.statusReason.add(t); 622 return this; 623 } 624 625 /** 626 * @return The first repetition of repeating field {@link #statusReason}, creating it if it does not already exist {3} 627 */ 628 public CodeableConcept getStatusReasonFirstRep() { 629 if (getStatusReason().isEmpty()) { 630 addStatusReason(); 631 } 632 return getStatusReason().get(0); 633 } 634 635 /** 636 * @return {@link #subject} (The individual, group of individuals or device that the device is on or associated with.) 637 */ 638 public Reference getSubject() { 639 if (this.subject == null) 640 if (Configuration.errorOnAutoCreate()) 641 throw new Error("Attempt to auto-create DeviceAssociation.subject"); 642 else if (Configuration.doAutoCreate()) 643 this.subject = new Reference(); // cc 644 return this.subject; 645 } 646 647 public boolean hasSubject() { 648 return this.subject != null && !this.subject.isEmpty(); 649 } 650 651 /** 652 * @param value {@link #subject} (The individual, group of individuals or device that the device is on or associated with.) 653 */ 654 public DeviceAssociation setSubject(Reference value) { 655 this.subject = value; 656 return this; 657 } 658 659 /** 660 * @return {@link #bodyStructure} (Current anatomical location of the device in/on subject.) 661 */ 662 public Reference getBodyStructure() { 663 if (this.bodyStructure == null) 664 if (Configuration.errorOnAutoCreate()) 665 throw new Error("Attempt to auto-create DeviceAssociation.bodyStructure"); 666 else if (Configuration.doAutoCreate()) 667 this.bodyStructure = new Reference(); // cc 668 return this.bodyStructure; 669 } 670 671 public boolean hasBodyStructure() { 672 return this.bodyStructure != null && !this.bodyStructure.isEmpty(); 673 } 674 675 /** 676 * @param value {@link #bodyStructure} (Current anatomical location of the device in/on subject.) 677 */ 678 public DeviceAssociation setBodyStructure(Reference value) { 679 this.bodyStructure = value; 680 return this; 681 } 682 683 /** 684 * @return {@link #period} (Begin and end dates and times for the device association.) 685 */ 686 public Period getPeriod() { 687 if (this.period == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create DeviceAssociation.period"); 690 else if (Configuration.doAutoCreate()) 691 this.period = new Period(); // cc 692 return this.period; 693 } 694 695 public boolean hasPeriod() { 696 return this.period != null && !this.period.isEmpty(); 697 } 698 699 /** 700 * @param value {@link #period} (Begin and end dates and times for the device association.) 701 */ 702 public DeviceAssociation setPeriod(Period value) { 703 this.period = value; 704 return this; 705 } 706 707 /** 708 * @return {@link #operation} (The details about the device when it is in use to describe its operation.) 709 */ 710 public List<DeviceAssociationOperationComponent> getOperation() { 711 if (this.operation == null) 712 this.operation = new ArrayList<DeviceAssociationOperationComponent>(); 713 return this.operation; 714 } 715 716 /** 717 * @return Returns a reference to <code>this</code> for easy method chaining 718 */ 719 public DeviceAssociation setOperation(List<DeviceAssociationOperationComponent> theOperation) { 720 this.operation = theOperation; 721 return this; 722 } 723 724 public boolean hasOperation() { 725 if (this.operation == null) 726 return false; 727 for (DeviceAssociationOperationComponent item : this.operation) 728 if (!item.isEmpty()) 729 return true; 730 return false; 731 } 732 733 public DeviceAssociationOperationComponent addOperation() { //3 734 DeviceAssociationOperationComponent t = new DeviceAssociationOperationComponent(); 735 if (this.operation == null) 736 this.operation = new ArrayList<DeviceAssociationOperationComponent>(); 737 this.operation.add(t); 738 return t; 739 } 740 741 public DeviceAssociation addOperation(DeviceAssociationOperationComponent t) { //3 742 if (t == null) 743 return this; 744 if (this.operation == null) 745 this.operation = new ArrayList<DeviceAssociationOperationComponent>(); 746 this.operation.add(t); 747 return this; 748 } 749 750 /** 751 * @return The first repetition of repeating field {@link #operation}, creating it if it does not already exist {3} 752 */ 753 public DeviceAssociationOperationComponent getOperationFirstRep() { 754 if (getOperation().isEmpty()) { 755 addOperation(); 756 } 757 return getOperation().get(0); 758 } 759 760 protected void listChildren(List<Property> children) { 761 super.listChildren(children); 762 children.add(new Property("identifier", "Identifier", "Instance identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 763 children.add(new Property("device", "Reference(Device)", "Reference to the devices associated with the patient or group.", 0, 1, device)); 764 children.add(new Property("category", "CodeableConcept", "Describes the relationship between the device and subject.", 0, java.lang.Integer.MAX_VALUE, category)); 765 children.add(new Property("status", "CodeableConcept", "Indicates the state of the Device association.", 0, 1, status)); 766 children.add(new Property("statusReason", "CodeableConcept", "The reasons given for the current association status.", 0, java.lang.Integer.MAX_VALUE, statusReason)); 767 children.add(new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|Device)", "The individual, group of individuals or device that the device is on or associated with.", 0, 1, subject)); 768 children.add(new Property("bodyStructure", "Reference(BodyStructure)", "Current anatomical location of the device in/on subject.", 0, 1, bodyStructure)); 769 children.add(new Property("period", "Period", "Begin and end dates and times for the device association.", 0, 1, period)); 770 children.add(new Property("operation", "", "The details about the device when it is in use to describe its operation.", 0, java.lang.Integer.MAX_VALUE, operation)); 771 } 772 773 @Override 774 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 775 switch (_hash) { 776 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Instance identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 777 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "Reference to the devices associated with the patient or group.", 0, 1, device); 778 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Describes the relationship between the device and subject.", 0, java.lang.Integer.MAX_VALUE, category); 779 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Indicates the state of the Device association.", 0, 1, status); 780 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "The reasons given for the current association status.", 0, java.lang.Integer.MAX_VALUE, statusReason); 781 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|Device)", "The individual, group of individuals or device that the device is on or associated with.", 0, 1, subject); 782 case -1001731599: /*bodyStructure*/ return new Property("bodyStructure", "Reference(BodyStructure)", "Current anatomical location of the device in/on subject.", 0, 1, bodyStructure); 783 case -991726143: /*period*/ return new Property("period", "Period", "Begin and end dates and times for the device association.", 0, 1, period); 784 case 1662702951: /*operation*/ return new Property("operation", "", "The details about the device when it is in use to describe its operation.", 0, java.lang.Integer.MAX_VALUE, operation); 785 default: return super.getNamedProperty(_hash, _name, _checkValid); 786 } 787 788 } 789 790 @Override 791 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 792 switch (hash) { 793 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 794 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 795 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 796 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 797 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 798 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 799 case -1001731599: /*bodyStructure*/ return this.bodyStructure == null ? new Base[0] : new Base[] {this.bodyStructure}; // Reference 800 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 801 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : this.operation.toArray(new Base[this.operation.size()]); // DeviceAssociationOperationComponent 802 default: return super.getProperty(hash, name, checkValid); 803 } 804 805 } 806 807 @Override 808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 809 switch (hash) { 810 case -1618432855: // identifier 811 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 812 return value; 813 case -1335157162: // device 814 this.device = TypeConvertor.castToReference(value); // Reference 815 return value; 816 case 50511102: // category 817 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 818 return value; 819 case -892481550: // status 820 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 821 return value; 822 case 2051346646: // statusReason 823 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 824 return value; 825 case -1867885268: // subject 826 this.subject = TypeConvertor.castToReference(value); // Reference 827 return value; 828 case -1001731599: // bodyStructure 829 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 830 return value; 831 case -991726143: // period 832 this.period = TypeConvertor.castToPeriod(value); // Period 833 return value; 834 case 1662702951: // operation 835 this.getOperation().add((DeviceAssociationOperationComponent) value); // DeviceAssociationOperationComponent 836 return value; 837 default: return super.setProperty(hash, name, value); 838 } 839 840 } 841 842 @Override 843 public Base setProperty(String name, Base value) throws FHIRException { 844 if (name.equals("identifier")) { 845 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 846 } else if (name.equals("device")) { 847 this.device = TypeConvertor.castToReference(value); // Reference 848 } else if (name.equals("category")) { 849 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 850 } else if (name.equals("status")) { 851 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 852 } else if (name.equals("statusReason")) { 853 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); 854 } else if (name.equals("subject")) { 855 this.subject = TypeConvertor.castToReference(value); // Reference 856 } else if (name.equals("bodyStructure")) { 857 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 858 } else if (name.equals("period")) { 859 this.period = TypeConvertor.castToPeriod(value); // Period 860 } else if (name.equals("operation")) { 861 this.getOperation().add((DeviceAssociationOperationComponent) value); 862 } else 863 return super.setProperty(name, value); 864 return value; 865 } 866 867 @Override 868 public Base makeProperty(int hash, String name) throws FHIRException { 869 switch (hash) { 870 case -1618432855: return addIdentifier(); 871 case -1335157162: return getDevice(); 872 case 50511102: return addCategory(); 873 case -892481550: return getStatus(); 874 case 2051346646: return addStatusReason(); 875 case -1867885268: return getSubject(); 876 case -1001731599: return getBodyStructure(); 877 case -991726143: return getPeriod(); 878 case 1662702951: return addOperation(); 879 default: return super.makeProperty(hash, name); 880 } 881 882 } 883 884 @Override 885 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 886 switch (hash) { 887 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 888 case -1335157162: /*device*/ return new String[] {"Reference"}; 889 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 890 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 891 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 892 case -1867885268: /*subject*/ return new String[] {"Reference"}; 893 case -1001731599: /*bodyStructure*/ return new String[] {"Reference"}; 894 case -991726143: /*period*/ return new String[] {"Period"}; 895 case 1662702951: /*operation*/ return new String[] {}; 896 default: return super.getTypesForProperty(hash, name); 897 } 898 899 } 900 901 @Override 902 public Base addChild(String name) throws FHIRException { 903 if (name.equals("identifier")) { 904 return addIdentifier(); 905 } 906 else if (name.equals("device")) { 907 this.device = new Reference(); 908 return this.device; 909 } 910 else if (name.equals("category")) { 911 return addCategory(); 912 } 913 else if (name.equals("status")) { 914 this.status = new CodeableConcept(); 915 return this.status; 916 } 917 else if (name.equals("statusReason")) { 918 return addStatusReason(); 919 } 920 else if (name.equals("subject")) { 921 this.subject = new Reference(); 922 return this.subject; 923 } 924 else if (name.equals("bodyStructure")) { 925 this.bodyStructure = new Reference(); 926 return this.bodyStructure; 927 } 928 else if (name.equals("period")) { 929 this.period = new Period(); 930 return this.period; 931 } 932 else if (name.equals("operation")) { 933 return addOperation(); 934 } 935 else 936 return super.addChild(name); 937 } 938 939 public String fhirType() { 940 return "DeviceAssociation"; 941 942 } 943 944 public DeviceAssociation copy() { 945 DeviceAssociation dst = new DeviceAssociation(); 946 copyValues(dst); 947 return dst; 948 } 949 950 public void copyValues(DeviceAssociation dst) { 951 super.copyValues(dst); 952 if (identifier != null) { 953 dst.identifier = new ArrayList<Identifier>(); 954 for (Identifier i : identifier) 955 dst.identifier.add(i.copy()); 956 }; 957 dst.device = device == null ? null : device.copy(); 958 if (category != null) { 959 dst.category = new ArrayList<CodeableConcept>(); 960 for (CodeableConcept i : category) 961 dst.category.add(i.copy()); 962 }; 963 dst.status = status == null ? null : status.copy(); 964 if (statusReason != null) { 965 dst.statusReason = new ArrayList<CodeableConcept>(); 966 for (CodeableConcept i : statusReason) 967 dst.statusReason.add(i.copy()); 968 }; 969 dst.subject = subject == null ? null : subject.copy(); 970 dst.bodyStructure = bodyStructure == null ? null : bodyStructure.copy(); 971 dst.period = period == null ? null : period.copy(); 972 if (operation != null) { 973 dst.operation = new ArrayList<DeviceAssociationOperationComponent>(); 974 for (DeviceAssociationOperationComponent i : operation) 975 dst.operation.add(i.copy()); 976 }; 977 } 978 979 protected DeviceAssociation typedCopy() { 980 return copy(); 981 } 982 983 @Override 984 public boolean equalsDeep(Base other_) { 985 if (!super.equalsDeep(other_)) 986 return false; 987 if (!(other_ instanceof DeviceAssociation)) 988 return false; 989 DeviceAssociation o = (DeviceAssociation) other_; 990 return compareDeep(identifier, o.identifier, true) && compareDeep(device, o.device, true) && compareDeep(category, o.category, true) 991 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) && compareDeep(subject, o.subject, true) 992 && compareDeep(bodyStructure, o.bodyStructure, true) && compareDeep(period, o.period, true) && compareDeep(operation, o.operation, true) 993 ; 994 } 995 996 @Override 997 public boolean equalsShallow(Base other_) { 998 if (!super.equalsShallow(other_)) 999 return false; 1000 if (!(other_ instanceof DeviceAssociation)) 1001 return false; 1002 DeviceAssociation o = (DeviceAssociation) other_; 1003 return true; 1004 } 1005 1006 public boolean isEmpty() { 1007 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, device, category 1008 , status, statusReason, subject, bodyStructure, period, operation); 1009 } 1010 1011 @Override 1012 public ResourceType getResourceType() { 1013 return ResourceType.DeviceAssociation; 1014 } 1015 1016 /** 1017 * Search parameter: <b>device</b> 1018 * <p> 1019 * Description: <b>Search for products that match this code</b><br> 1020 * Type: <b>reference</b><br> 1021 * Path: <b>DeviceAssociation.device</b><br> 1022 * </p> 1023 */ 1024 @SearchParamDefinition(name="device", path="DeviceAssociation.device", description="Search for products that match this code", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 1025 public static final String SP_DEVICE = "device"; 1026 /** 1027 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1028 * <p> 1029 * Description: <b>Search for products that match this code</b><br> 1030 * Type: <b>reference</b><br> 1031 * Path: <b>DeviceAssociation.device</b><br> 1032 * </p> 1033 */ 1034 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 1035 1036/** 1037 * Constant for fluent queries to be used to add include statements. Specifies 1038 * the path value of "<b>DeviceAssociation:device</b>". 1039 */ 1040 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceAssociation:device").toLocked(); 1041 1042 /** 1043 * Search parameter: <b>identifier</b> 1044 * <p> 1045 * Description: <b>The identifier of the device association</b><br> 1046 * Type: <b>token</b><br> 1047 * Path: <b>DeviceAssociation.identifier</b><br> 1048 * </p> 1049 */ 1050 @SearchParamDefinition(name="identifier", path="DeviceAssociation.identifier", description="The identifier of the device association", type="token" ) 1051 public static final String SP_IDENTIFIER = "identifier"; 1052 /** 1053 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1054 * <p> 1055 * Description: <b>The identifier of the device association</b><br> 1056 * Type: <b>token</b><br> 1057 * Path: <b>DeviceAssociation.identifier</b><br> 1058 * </p> 1059 */ 1060 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1061 1062 /** 1063 * Search parameter: <b>operator</b> 1064 * <p> 1065 * Description: <b>The identity of a operator for whom to list associations</b><br> 1066 * Type: <b>reference</b><br> 1067 * Path: <b>DeviceAssociation.operation.operator</b><br> 1068 * </p> 1069 */ 1070 @SearchParamDefinition(name="operator", path="DeviceAssociation.operation.operator", description="The identity of a operator for whom to list associations", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Patient.class, Practitioner.class, RelatedPerson.class } ) 1071 public static final String SP_OPERATOR = "operator"; 1072 /** 1073 * <b>Fluent Client</b> search parameter constant for <b>operator</b> 1074 * <p> 1075 * Description: <b>The identity of a operator for whom to list associations</b><br> 1076 * Type: <b>reference</b><br> 1077 * Path: <b>DeviceAssociation.operation.operator</b><br> 1078 * </p> 1079 */ 1080 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OPERATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OPERATOR); 1081 1082/** 1083 * Constant for fluent queries to be used to add include statements. Specifies 1084 * the path value of "<b>DeviceAssociation:operator</b>". 1085 */ 1086 public static final ca.uhn.fhir.model.api.Include INCLUDE_OPERATOR = new ca.uhn.fhir.model.api.Include("DeviceAssociation:operator").toLocked(); 1087 1088 /** 1089 * Search parameter: <b>patient</b> 1090 * <p> 1091 * Description: <b>The identity of a patient for whom to list associations</b><br> 1092 * Type: <b>reference</b><br> 1093 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1094 * </p> 1095 */ 1096 @SearchParamDefinition(name="patient", path="DeviceAssociation.subject.where(resolve() is Patient)", description="The identity of a patient for whom to list associations", type="reference", target={Patient.class } ) 1097 public static final String SP_PATIENT = "patient"; 1098 /** 1099 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1100 * <p> 1101 * Description: <b>The identity of a patient for whom to list associations</b><br> 1102 * Type: <b>reference</b><br> 1103 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1104 * </p> 1105 */ 1106 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1107 1108/** 1109 * Constant for fluent queries to be used to add include statements. Specifies 1110 * the path value of "<b>DeviceAssociation:patient</b>". 1111 */ 1112 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceAssociation:patient").toLocked(); 1113 1114 /** 1115 * Search parameter: <b>status</b> 1116 * <p> 1117 * Description: <b>The status of the device associations</b><br> 1118 * Type: <b>token</b><br> 1119 * Path: <b>DeviceAssociation.status</b><br> 1120 * </p> 1121 */ 1122 @SearchParamDefinition(name="status", path="DeviceAssociation.status", description="The status of the device associations", type="token" ) 1123 public static final String SP_STATUS = "status"; 1124 /** 1125 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1126 * <p> 1127 * Description: <b>The status of the device associations</b><br> 1128 * Type: <b>token</b><br> 1129 * Path: <b>DeviceAssociation.status</b><br> 1130 * </p> 1131 */ 1132 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1133 1134 /** 1135 * Search parameter: <b>subject</b> 1136 * <p> 1137 * Description: <b>The identity of a patient for whom to list associations</b><br> 1138 * Type: <b>reference</b><br> 1139 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1140 * </p> 1141 */ 1142 @SearchParamDefinition(name="subject", path="DeviceAssociation.subject.where(resolve() is Patient)", description="The identity of a patient for whom to list associations", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1143 public static final String SP_SUBJECT = "subject"; 1144 /** 1145 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1146 * <p> 1147 * Description: <b>The identity of a patient for whom to list associations</b><br> 1148 * Type: <b>reference</b><br> 1149 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1150 * </p> 1151 */ 1152 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1153 1154/** 1155 * Constant for fluent queries to be used to add include statements. Specifies 1156 * the path value of "<b>DeviceAssociation:subject</b>". 1157 */ 1158 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DeviceAssociation:subject").toLocked(); 1159 1160 1161} 1162