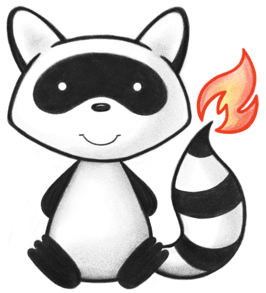
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * A record of association of a device. 051 */ 052@ResourceDef(name="DeviceAssociation", profile="http://hl7.org/fhir/StructureDefinition/DeviceAssociation") 053public class DeviceAssociation extends DomainResource { 054 055 @Block() 056 public static class DeviceAssociationOperationComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Device operational condition corresponding to the association. 059 */ 060 @Child(name = "status", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="Device operational condition", formalDefinition="Device operational condition corresponding to the association." ) 062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceassociation-operationstatus") 063 protected CodeableConcept status; 064 065 /** 066 * The individual performing the action enabled by the device. 067 */ 068 @Child(name = "operator", type = {Patient.class, Practitioner.class, RelatedPerson.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 069 @Description(shortDefinition="The individual performing the action enabled by the device", formalDefinition="The individual performing the action enabled by the device." ) 070 protected List<Reference> operator; 071 072 /** 073 * Begin and end dates and times for the device's operation. 074 */ 075 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 076 @Description(shortDefinition="Begin and end dates and times for the device's operation", formalDefinition="Begin and end dates and times for the device's operation." ) 077 protected Period period; 078 079 private static final long serialVersionUID = -1587836408L; 080 081 /** 082 * Constructor 083 */ 084 public DeviceAssociationOperationComponent() { 085 super(); 086 } 087 088 /** 089 * Constructor 090 */ 091 public DeviceAssociationOperationComponent(CodeableConcept status) { 092 super(); 093 this.setStatus(status); 094 } 095 096 /** 097 * @return {@link #status} (Device operational condition corresponding to the association.) 098 */ 099 public CodeableConcept getStatus() { 100 if (this.status == null) 101 if (Configuration.errorOnAutoCreate()) 102 throw new Error("Attempt to auto-create DeviceAssociationOperationComponent.status"); 103 else if (Configuration.doAutoCreate()) 104 this.status = new CodeableConcept(); // cc 105 return this.status; 106 } 107 108 public boolean hasStatus() { 109 return this.status != null && !this.status.isEmpty(); 110 } 111 112 /** 113 * @param value {@link #status} (Device operational condition corresponding to the association.) 114 */ 115 public DeviceAssociationOperationComponent setStatus(CodeableConcept value) { 116 this.status = value; 117 return this; 118 } 119 120 /** 121 * @return {@link #operator} (The individual performing the action enabled by the device.) 122 */ 123 public List<Reference> getOperator() { 124 if (this.operator == null) 125 this.operator = new ArrayList<Reference>(); 126 return this.operator; 127 } 128 129 /** 130 * @return Returns a reference to <code>this</code> for easy method chaining 131 */ 132 public DeviceAssociationOperationComponent setOperator(List<Reference> theOperator) { 133 this.operator = theOperator; 134 return this; 135 } 136 137 public boolean hasOperator() { 138 if (this.operator == null) 139 return false; 140 for (Reference item : this.operator) 141 if (!item.isEmpty()) 142 return true; 143 return false; 144 } 145 146 public Reference addOperator() { //3 147 Reference t = new Reference(); 148 if (this.operator == null) 149 this.operator = new ArrayList<Reference>(); 150 this.operator.add(t); 151 return t; 152 } 153 154 public DeviceAssociationOperationComponent addOperator(Reference t) { //3 155 if (t == null) 156 return this; 157 if (this.operator == null) 158 this.operator = new ArrayList<Reference>(); 159 this.operator.add(t); 160 return this; 161 } 162 163 /** 164 * @return The first repetition of repeating field {@link #operator}, creating it if it does not already exist {3} 165 */ 166 public Reference getOperatorFirstRep() { 167 if (getOperator().isEmpty()) { 168 addOperator(); 169 } 170 return getOperator().get(0); 171 } 172 173 /** 174 * @return {@link #period} (Begin and end dates and times for the device's operation.) 175 */ 176 public Period getPeriod() { 177 if (this.period == null) 178 if (Configuration.errorOnAutoCreate()) 179 throw new Error("Attempt to auto-create DeviceAssociationOperationComponent.period"); 180 else if (Configuration.doAutoCreate()) 181 this.period = new Period(); // cc 182 return this.period; 183 } 184 185 public boolean hasPeriod() { 186 return this.period != null && !this.period.isEmpty(); 187 } 188 189 /** 190 * @param value {@link #period} (Begin and end dates and times for the device's operation.) 191 */ 192 public DeviceAssociationOperationComponent setPeriod(Period value) { 193 this.period = value; 194 return this; 195 } 196 197 protected void listChildren(List<Property> children) { 198 super.listChildren(children); 199 children.add(new Property("status", "CodeableConcept", "Device operational condition corresponding to the association.", 0, 1, status)); 200 children.add(new Property("operator", "Reference(Patient|Practitioner|RelatedPerson)", "The individual performing the action enabled by the device.", 0, java.lang.Integer.MAX_VALUE, operator)); 201 children.add(new Property("period", "Period", "Begin and end dates and times for the device's operation.", 0, 1, period)); 202 } 203 204 @Override 205 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 206 switch (_hash) { 207 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Device operational condition corresponding to the association.", 0, 1, status); 208 case -500553564: /*operator*/ return new Property("operator", "Reference(Patient|Practitioner|RelatedPerson)", "The individual performing the action enabled by the device.", 0, java.lang.Integer.MAX_VALUE, operator); 209 case -991726143: /*period*/ return new Property("period", "Period", "Begin and end dates and times for the device's operation.", 0, 1, period); 210 default: return super.getNamedProperty(_hash, _name, _checkValid); 211 } 212 213 } 214 215 @Override 216 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 217 switch (hash) { 218 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 219 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : this.operator.toArray(new Base[this.operator.size()]); // Reference 220 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 221 default: return super.getProperty(hash, name, checkValid); 222 } 223 224 } 225 226 @Override 227 public Base setProperty(int hash, String name, Base value) throws FHIRException { 228 switch (hash) { 229 case -892481550: // status 230 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 231 return value; 232 case -500553564: // operator 233 this.getOperator().add(TypeConvertor.castToReference(value)); // Reference 234 return value; 235 case -991726143: // period 236 this.period = TypeConvertor.castToPeriod(value); // Period 237 return value; 238 default: return super.setProperty(hash, name, value); 239 } 240 241 } 242 243 @Override 244 public Base setProperty(String name, Base value) throws FHIRException { 245 if (name.equals("status")) { 246 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 247 } else if (name.equals("operator")) { 248 this.getOperator().add(TypeConvertor.castToReference(value)); 249 } else if (name.equals("period")) { 250 this.period = TypeConvertor.castToPeriod(value); // Period 251 } else 252 return super.setProperty(name, value); 253 return value; 254 } 255 256 @Override 257 public void removeChild(String name, Base value) throws FHIRException { 258 if (name.equals("status")) { 259 this.status = null; 260 } else if (name.equals("operator")) { 261 this.getOperator().remove(value); 262 } else if (name.equals("period")) { 263 this.period = null; 264 } else 265 super.removeChild(name, value); 266 267 } 268 269 @Override 270 public Base makeProperty(int hash, String name) throws FHIRException { 271 switch (hash) { 272 case -892481550: return getStatus(); 273 case -500553564: return addOperator(); 274 case -991726143: return getPeriod(); 275 default: return super.makeProperty(hash, name); 276 } 277 278 } 279 280 @Override 281 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 282 switch (hash) { 283 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 284 case -500553564: /*operator*/ return new String[] {"Reference"}; 285 case -991726143: /*period*/ return new String[] {"Period"}; 286 default: return super.getTypesForProperty(hash, name); 287 } 288 289 } 290 291 @Override 292 public Base addChild(String name) throws FHIRException { 293 if (name.equals("status")) { 294 this.status = new CodeableConcept(); 295 return this.status; 296 } 297 else if (name.equals("operator")) { 298 return addOperator(); 299 } 300 else if (name.equals("period")) { 301 this.period = new Period(); 302 return this.period; 303 } 304 else 305 return super.addChild(name); 306 } 307 308 public DeviceAssociationOperationComponent copy() { 309 DeviceAssociationOperationComponent dst = new DeviceAssociationOperationComponent(); 310 copyValues(dst); 311 return dst; 312 } 313 314 public void copyValues(DeviceAssociationOperationComponent dst) { 315 super.copyValues(dst); 316 dst.status = status == null ? null : status.copy(); 317 if (operator != null) { 318 dst.operator = new ArrayList<Reference>(); 319 for (Reference i : operator) 320 dst.operator.add(i.copy()); 321 }; 322 dst.period = period == null ? null : period.copy(); 323 } 324 325 @Override 326 public boolean equalsDeep(Base other_) { 327 if (!super.equalsDeep(other_)) 328 return false; 329 if (!(other_ instanceof DeviceAssociationOperationComponent)) 330 return false; 331 DeviceAssociationOperationComponent o = (DeviceAssociationOperationComponent) other_; 332 return compareDeep(status, o.status, true) && compareDeep(operator, o.operator, true) && compareDeep(period, o.period, true) 333 ; 334 } 335 336 @Override 337 public boolean equalsShallow(Base other_) { 338 if (!super.equalsShallow(other_)) 339 return false; 340 if (!(other_ instanceof DeviceAssociationOperationComponent)) 341 return false; 342 DeviceAssociationOperationComponent o = (DeviceAssociationOperationComponent) other_; 343 return true; 344 } 345 346 public boolean isEmpty() { 347 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, operator, period 348 ); 349 } 350 351 public String fhirType() { 352 return "DeviceAssociation.operation"; 353 354 } 355 356 } 357 358 /** 359 * Instance identifier. 360 */ 361 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 362 @Description(shortDefinition="Instance identifier", formalDefinition="Instance identifier." ) 363 protected List<Identifier> identifier; 364 365 /** 366 * Reference to the devices associated with the patient or group. 367 */ 368 @Child(name = "device", type = {Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 369 @Description(shortDefinition="Reference to the devices associated with the patient or group", formalDefinition="Reference to the devices associated with the patient or group." ) 370 protected Reference device; 371 372 /** 373 * Describes the relationship between the device and subject. 374 */ 375 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 376 @Description(shortDefinition="Describes the relationship between the device and subject", formalDefinition="Describes the relationship between the device and subject." ) 377 protected List<CodeableConcept> category; 378 379 /** 380 * Indicates the state of the Device association. 381 */ 382 @Child(name = "status", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 383 @Description(shortDefinition="implanted | explanted | attached | entered-in-error | unknown", formalDefinition="Indicates the state of the Device association." ) 384 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceassociation-status") 385 protected CodeableConcept status; 386 387 /** 388 * The reasons given for the current association status. 389 */ 390 @Child(name = "statusReason", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 391 @Description(shortDefinition="The reasons given for the current association status", formalDefinition="The reasons given for the current association status." ) 392 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceassociation-status-reason") 393 protected List<CodeableConcept> statusReason; 394 395 /** 396 * The individual, group of individuals or device that the device is on or associated with. 397 */ 398 @Child(name = "subject", type = {Patient.class, Group.class, Practitioner.class, RelatedPerson.class, Device.class}, order=5, min=0, max=1, modifier=false, summary=true) 399 @Description(shortDefinition="The individual, group of individuals or device that the device is on or associated with", formalDefinition="The individual, group of individuals or device that the device is on or associated with." ) 400 protected Reference subject; 401 402 /** 403 * Current anatomical location of the device in/on subject. 404 */ 405 @Child(name = "bodyStructure", type = {BodyStructure.class}, order=6, min=0, max=1, modifier=false, summary=true) 406 @Description(shortDefinition="Current anatomical location of the device in/on subject", formalDefinition="Current anatomical location of the device in/on subject." ) 407 protected Reference bodyStructure; 408 409 /** 410 * Begin and end dates and times for the device association. 411 */ 412 @Child(name = "period", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 413 @Description(shortDefinition="Begin and end dates and times for the device association", formalDefinition="Begin and end dates and times for the device association." ) 414 protected Period period; 415 416 /** 417 * The details about the device when it is in use to describe its operation. 418 */ 419 @Child(name = "operation", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 420 @Description(shortDefinition="The details about the device when it is in use to describe its operation", formalDefinition="The details about the device when it is in use to describe its operation." ) 421 protected List<DeviceAssociationOperationComponent> operation; 422 423 private static final long serialVersionUID = 1892071017L; 424 425 /** 426 * Constructor 427 */ 428 public DeviceAssociation() { 429 super(); 430 } 431 432 /** 433 * Constructor 434 */ 435 public DeviceAssociation(Reference device, CodeableConcept status) { 436 super(); 437 this.setDevice(device); 438 this.setStatus(status); 439 } 440 441 /** 442 * @return {@link #identifier} (Instance identifier.) 443 */ 444 public List<Identifier> getIdentifier() { 445 if (this.identifier == null) 446 this.identifier = new ArrayList<Identifier>(); 447 return this.identifier; 448 } 449 450 /** 451 * @return Returns a reference to <code>this</code> for easy method chaining 452 */ 453 public DeviceAssociation setIdentifier(List<Identifier> theIdentifier) { 454 this.identifier = theIdentifier; 455 return this; 456 } 457 458 public boolean hasIdentifier() { 459 if (this.identifier == null) 460 return false; 461 for (Identifier item : this.identifier) 462 if (!item.isEmpty()) 463 return true; 464 return false; 465 } 466 467 public Identifier addIdentifier() { //3 468 Identifier t = new Identifier(); 469 if (this.identifier == null) 470 this.identifier = new ArrayList<Identifier>(); 471 this.identifier.add(t); 472 return t; 473 } 474 475 public DeviceAssociation addIdentifier(Identifier t) { //3 476 if (t == null) 477 return this; 478 if (this.identifier == null) 479 this.identifier = new ArrayList<Identifier>(); 480 this.identifier.add(t); 481 return this; 482 } 483 484 /** 485 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 486 */ 487 public Identifier getIdentifierFirstRep() { 488 if (getIdentifier().isEmpty()) { 489 addIdentifier(); 490 } 491 return getIdentifier().get(0); 492 } 493 494 /** 495 * @return {@link #device} (Reference to the devices associated with the patient or group.) 496 */ 497 public Reference getDevice() { 498 if (this.device == null) 499 if (Configuration.errorOnAutoCreate()) 500 throw new Error("Attempt to auto-create DeviceAssociation.device"); 501 else if (Configuration.doAutoCreate()) 502 this.device = new Reference(); // cc 503 return this.device; 504 } 505 506 public boolean hasDevice() { 507 return this.device != null && !this.device.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #device} (Reference to the devices associated with the patient or group.) 512 */ 513 public DeviceAssociation setDevice(Reference value) { 514 this.device = value; 515 return this; 516 } 517 518 /** 519 * @return {@link #category} (Describes the relationship between the device and subject.) 520 */ 521 public List<CodeableConcept> getCategory() { 522 if (this.category == null) 523 this.category = new ArrayList<CodeableConcept>(); 524 return this.category; 525 } 526 527 /** 528 * @return Returns a reference to <code>this</code> for easy method chaining 529 */ 530 public DeviceAssociation setCategory(List<CodeableConcept> theCategory) { 531 this.category = theCategory; 532 return this; 533 } 534 535 public boolean hasCategory() { 536 if (this.category == null) 537 return false; 538 for (CodeableConcept item : this.category) 539 if (!item.isEmpty()) 540 return true; 541 return false; 542 } 543 544 public CodeableConcept addCategory() { //3 545 CodeableConcept t = new CodeableConcept(); 546 if (this.category == null) 547 this.category = new ArrayList<CodeableConcept>(); 548 this.category.add(t); 549 return t; 550 } 551 552 public DeviceAssociation addCategory(CodeableConcept t) { //3 553 if (t == null) 554 return this; 555 if (this.category == null) 556 this.category = new ArrayList<CodeableConcept>(); 557 this.category.add(t); 558 return this; 559 } 560 561 /** 562 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 563 */ 564 public CodeableConcept getCategoryFirstRep() { 565 if (getCategory().isEmpty()) { 566 addCategory(); 567 } 568 return getCategory().get(0); 569 } 570 571 /** 572 * @return {@link #status} (Indicates the state of the Device association.) 573 */ 574 public CodeableConcept getStatus() { 575 if (this.status == null) 576 if (Configuration.errorOnAutoCreate()) 577 throw new Error("Attempt to auto-create DeviceAssociation.status"); 578 else if (Configuration.doAutoCreate()) 579 this.status = new CodeableConcept(); // cc 580 return this.status; 581 } 582 583 public boolean hasStatus() { 584 return this.status != null && !this.status.isEmpty(); 585 } 586 587 /** 588 * @param value {@link #status} (Indicates the state of the Device association.) 589 */ 590 public DeviceAssociation setStatus(CodeableConcept value) { 591 this.status = value; 592 return this; 593 } 594 595 /** 596 * @return {@link #statusReason} (The reasons given for the current association status.) 597 */ 598 public List<CodeableConcept> getStatusReason() { 599 if (this.statusReason == null) 600 this.statusReason = new ArrayList<CodeableConcept>(); 601 return this.statusReason; 602 } 603 604 /** 605 * @return Returns a reference to <code>this</code> for easy method chaining 606 */ 607 public DeviceAssociation setStatusReason(List<CodeableConcept> theStatusReason) { 608 this.statusReason = theStatusReason; 609 return this; 610 } 611 612 public boolean hasStatusReason() { 613 if (this.statusReason == null) 614 return false; 615 for (CodeableConcept item : this.statusReason) 616 if (!item.isEmpty()) 617 return true; 618 return false; 619 } 620 621 public CodeableConcept addStatusReason() { //3 622 CodeableConcept t = new CodeableConcept(); 623 if (this.statusReason == null) 624 this.statusReason = new ArrayList<CodeableConcept>(); 625 this.statusReason.add(t); 626 return t; 627 } 628 629 public DeviceAssociation addStatusReason(CodeableConcept t) { //3 630 if (t == null) 631 return this; 632 if (this.statusReason == null) 633 this.statusReason = new ArrayList<CodeableConcept>(); 634 this.statusReason.add(t); 635 return this; 636 } 637 638 /** 639 * @return The first repetition of repeating field {@link #statusReason}, creating it if it does not already exist {3} 640 */ 641 public CodeableConcept getStatusReasonFirstRep() { 642 if (getStatusReason().isEmpty()) { 643 addStatusReason(); 644 } 645 return getStatusReason().get(0); 646 } 647 648 /** 649 * @return {@link #subject} (The individual, group of individuals or device that the device is on or associated with.) 650 */ 651 public Reference getSubject() { 652 if (this.subject == null) 653 if (Configuration.errorOnAutoCreate()) 654 throw new Error("Attempt to auto-create DeviceAssociation.subject"); 655 else if (Configuration.doAutoCreate()) 656 this.subject = new Reference(); // cc 657 return this.subject; 658 } 659 660 public boolean hasSubject() { 661 return this.subject != null && !this.subject.isEmpty(); 662 } 663 664 /** 665 * @param value {@link #subject} (The individual, group of individuals or device that the device is on or associated with.) 666 */ 667 public DeviceAssociation setSubject(Reference value) { 668 this.subject = value; 669 return this; 670 } 671 672 /** 673 * @return {@link #bodyStructure} (Current anatomical location of the device in/on subject.) 674 */ 675 public Reference getBodyStructure() { 676 if (this.bodyStructure == null) 677 if (Configuration.errorOnAutoCreate()) 678 throw new Error("Attempt to auto-create DeviceAssociation.bodyStructure"); 679 else if (Configuration.doAutoCreate()) 680 this.bodyStructure = new Reference(); // cc 681 return this.bodyStructure; 682 } 683 684 public boolean hasBodyStructure() { 685 return this.bodyStructure != null && !this.bodyStructure.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #bodyStructure} (Current anatomical location of the device in/on subject.) 690 */ 691 public DeviceAssociation setBodyStructure(Reference value) { 692 this.bodyStructure = value; 693 return this; 694 } 695 696 /** 697 * @return {@link #period} (Begin and end dates and times for the device association.) 698 */ 699 public Period getPeriod() { 700 if (this.period == null) 701 if (Configuration.errorOnAutoCreate()) 702 throw new Error("Attempt to auto-create DeviceAssociation.period"); 703 else if (Configuration.doAutoCreate()) 704 this.period = new Period(); // cc 705 return this.period; 706 } 707 708 public boolean hasPeriod() { 709 return this.period != null && !this.period.isEmpty(); 710 } 711 712 /** 713 * @param value {@link #period} (Begin and end dates and times for the device association.) 714 */ 715 public DeviceAssociation setPeriod(Period value) { 716 this.period = value; 717 return this; 718 } 719 720 /** 721 * @return {@link #operation} (The details about the device when it is in use to describe its operation.) 722 */ 723 public List<DeviceAssociationOperationComponent> getOperation() { 724 if (this.operation == null) 725 this.operation = new ArrayList<DeviceAssociationOperationComponent>(); 726 return this.operation; 727 } 728 729 /** 730 * @return Returns a reference to <code>this</code> for easy method chaining 731 */ 732 public DeviceAssociation setOperation(List<DeviceAssociationOperationComponent> theOperation) { 733 this.operation = theOperation; 734 return this; 735 } 736 737 public boolean hasOperation() { 738 if (this.operation == null) 739 return false; 740 for (DeviceAssociationOperationComponent item : this.operation) 741 if (!item.isEmpty()) 742 return true; 743 return false; 744 } 745 746 public DeviceAssociationOperationComponent addOperation() { //3 747 DeviceAssociationOperationComponent t = new DeviceAssociationOperationComponent(); 748 if (this.operation == null) 749 this.operation = new ArrayList<DeviceAssociationOperationComponent>(); 750 this.operation.add(t); 751 return t; 752 } 753 754 public DeviceAssociation addOperation(DeviceAssociationOperationComponent t) { //3 755 if (t == null) 756 return this; 757 if (this.operation == null) 758 this.operation = new ArrayList<DeviceAssociationOperationComponent>(); 759 this.operation.add(t); 760 return this; 761 } 762 763 /** 764 * @return The first repetition of repeating field {@link #operation}, creating it if it does not already exist {3} 765 */ 766 public DeviceAssociationOperationComponent getOperationFirstRep() { 767 if (getOperation().isEmpty()) { 768 addOperation(); 769 } 770 return getOperation().get(0); 771 } 772 773 protected void listChildren(List<Property> children) { 774 super.listChildren(children); 775 children.add(new Property("identifier", "Identifier", "Instance identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 776 children.add(new Property("device", "Reference(Device)", "Reference to the devices associated with the patient or group.", 0, 1, device)); 777 children.add(new Property("category", "CodeableConcept", "Describes the relationship between the device and subject.", 0, java.lang.Integer.MAX_VALUE, category)); 778 children.add(new Property("status", "CodeableConcept", "Indicates the state of the Device association.", 0, 1, status)); 779 children.add(new Property("statusReason", "CodeableConcept", "The reasons given for the current association status.", 0, java.lang.Integer.MAX_VALUE, statusReason)); 780 children.add(new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|Device)", "The individual, group of individuals or device that the device is on or associated with.", 0, 1, subject)); 781 children.add(new Property("bodyStructure", "Reference(BodyStructure)", "Current anatomical location of the device in/on subject.", 0, 1, bodyStructure)); 782 children.add(new Property("period", "Period", "Begin and end dates and times for the device association.", 0, 1, period)); 783 children.add(new Property("operation", "", "The details about the device when it is in use to describe its operation.", 0, java.lang.Integer.MAX_VALUE, operation)); 784 } 785 786 @Override 787 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 788 switch (_hash) { 789 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Instance identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 790 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "Reference to the devices associated with the patient or group.", 0, 1, device); 791 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Describes the relationship between the device and subject.", 0, java.lang.Integer.MAX_VALUE, category); 792 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Indicates the state of the Device association.", 0, 1, status); 793 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "The reasons given for the current association status.", 0, java.lang.Integer.MAX_VALUE, statusReason); 794 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Practitioner|RelatedPerson|Device)", "The individual, group of individuals or device that the device is on or associated with.", 0, 1, subject); 795 case -1001731599: /*bodyStructure*/ return new Property("bodyStructure", "Reference(BodyStructure)", "Current anatomical location of the device in/on subject.", 0, 1, bodyStructure); 796 case -991726143: /*period*/ return new Property("period", "Period", "Begin and end dates and times for the device association.", 0, 1, period); 797 case 1662702951: /*operation*/ return new Property("operation", "", "The details about the device when it is in use to describe its operation.", 0, java.lang.Integer.MAX_VALUE, operation); 798 default: return super.getNamedProperty(_hash, _name, _checkValid); 799 } 800 801 } 802 803 @Override 804 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 805 switch (hash) { 806 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 807 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 808 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 809 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 810 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 811 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 812 case -1001731599: /*bodyStructure*/ return this.bodyStructure == null ? new Base[0] : new Base[] {this.bodyStructure}; // Reference 813 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 814 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : this.operation.toArray(new Base[this.operation.size()]); // DeviceAssociationOperationComponent 815 default: return super.getProperty(hash, name, checkValid); 816 } 817 818 } 819 820 @Override 821 public Base setProperty(int hash, String name, Base value) throws FHIRException { 822 switch (hash) { 823 case -1618432855: // identifier 824 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 825 return value; 826 case -1335157162: // device 827 this.device = TypeConvertor.castToReference(value); // Reference 828 return value; 829 case 50511102: // category 830 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 831 return value; 832 case -892481550: // status 833 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 834 return value; 835 case 2051346646: // statusReason 836 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 837 return value; 838 case -1867885268: // subject 839 this.subject = TypeConvertor.castToReference(value); // Reference 840 return value; 841 case -1001731599: // bodyStructure 842 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 843 return value; 844 case -991726143: // period 845 this.period = TypeConvertor.castToPeriod(value); // Period 846 return value; 847 case 1662702951: // operation 848 this.getOperation().add((DeviceAssociationOperationComponent) value); // DeviceAssociationOperationComponent 849 return value; 850 default: return super.setProperty(hash, name, value); 851 } 852 853 } 854 855 @Override 856 public Base setProperty(String name, Base value) throws FHIRException { 857 if (name.equals("identifier")) { 858 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 859 } else if (name.equals("device")) { 860 this.device = TypeConvertor.castToReference(value); // Reference 861 } else if (name.equals("category")) { 862 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 863 } else if (name.equals("status")) { 864 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 865 } else if (name.equals("statusReason")) { 866 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); 867 } else if (name.equals("subject")) { 868 this.subject = TypeConvertor.castToReference(value); // Reference 869 } else if (name.equals("bodyStructure")) { 870 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 871 } else if (name.equals("period")) { 872 this.period = TypeConvertor.castToPeriod(value); // Period 873 } else if (name.equals("operation")) { 874 this.getOperation().add((DeviceAssociationOperationComponent) value); 875 } else 876 return super.setProperty(name, value); 877 return value; 878 } 879 880 @Override 881 public void removeChild(String name, Base value) throws FHIRException { 882 if (name.equals("identifier")) { 883 this.getIdentifier().remove(value); 884 } else if (name.equals("device")) { 885 this.device = null; 886 } else if (name.equals("category")) { 887 this.getCategory().remove(value); 888 } else if (name.equals("status")) { 889 this.status = null; 890 } else if (name.equals("statusReason")) { 891 this.getStatusReason().remove(value); 892 } else if (name.equals("subject")) { 893 this.subject = null; 894 } else if (name.equals("bodyStructure")) { 895 this.bodyStructure = null; 896 } else if (name.equals("period")) { 897 this.period = null; 898 } else if (name.equals("operation")) { 899 this.getOperation().remove((DeviceAssociationOperationComponent) value); 900 } else 901 super.removeChild(name, value); 902 903 } 904 905 @Override 906 public Base makeProperty(int hash, String name) throws FHIRException { 907 switch (hash) { 908 case -1618432855: return addIdentifier(); 909 case -1335157162: return getDevice(); 910 case 50511102: return addCategory(); 911 case -892481550: return getStatus(); 912 case 2051346646: return addStatusReason(); 913 case -1867885268: return getSubject(); 914 case -1001731599: return getBodyStructure(); 915 case -991726143: return getPeriod(); 916 case 1662702951: return addOperation(); 917 default: return super.makeProperty(hash, name); 918 } 919 920 } 921 922 @Override 923 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 924 switch (hash) { 925 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 926 case -1335157162: /*device*/ return new String[] {"Reference"}; 927 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 928 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 929 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 930 case -1867885268: /*subject*/ return new String[] {"Reference"}; 931 case -1001731599: /*bodyStructure*/ return new String[] {"Reference"}; 932 case -991726143: /*period*/ return new String[] {"Period"}; 933 case 1662702951: /*operation*/ return new String[] {}; 934 default: return super.getTypesForProperty(hash, name); 935 } 936 937 } 938 939 @Override 940 public Base addChild(String name) throws FHIRException { 941 if (name.equals("identifier")) { 942 return addIdentifier(); 943 } 944 else if (name.equals("device")) { 945 this.device = new Reference(); 946 return this.device; 947 } 948 else if (name.equals("category")) { 949 return addCategory(); 950 } 951 else if (name.equals("status")) { 952 this.status = new CodeableConcept(); 953 return this.status; 954 } 955 else if (name.equals("statusReason")) { 956 return addStatusReason(); 957 } 958 else if (name.equals("subject")) { 959 this.subject = new Reference(); 960 return this.subject; 961 } 962 else if (name.equals("bodyStructure")) { 963 this.bodyStructure = new Reference(); 964 return this.bodyStructure; 965 } 966 else if (name.equals("period")) { 967 this.period = new Period(); 968 return this.period; 969 } 970 else if (name.equals("operation")) { 971 return addOperation(); 972 } 973 else 974 return super.addChild(name); 975 } 976 977 public String fhirType() { 978 return "DeviceAssociation"; 979 980 } 981 982 public DeviceAssociation copy() { 983 DeviceAssociation dst = new DeviceAssociation(); 984 copyValues(dst); 985 return dst; 986 } 987 988 public void copyValues(DeviceAssociation dst) { 989 super.copyValues(dst); 990 if (identifier != null) { 991 dst.identifier = new ArrayList<Identifier>(); 992 for (Identifier i : identifier) 993 dst.identifier.add(i.copy()); 994 }; 995 dst.device = device == null ? null : device.copy(); 996 if (category != null) { 997 dst.category = new ArrayList<CodeableConcept>(); 998 for (CodeableConcept i : category) 999 dst.category.add(i.copy()); 1000 }; 1001 dst.status = status == null ? null : status.copy(); 1002 if (statusReason != null) { 1003 dst.statusReason = new ArrayList<CodeableConcept>(); 1004 for (CodeableConcept i : statusReason) 1005 dst.statusReason.add(i.copy()); 1006 }; 1007 dst.subject = subject == null ? null : subject.copy(); 1008 dst.bodyStructure = bodyStructure == null ? null : bodyStructure.copy(); 1009 dst.period = period == null ? null : period.copy(); 1010 if (operation != null) { 1011 dst.operation = new ArrayList<DeviceAssociationOperationComponent>(); 1012 for (DeviceAssociationOperationComponent i : operation) 1013 dst.operation.add(i.copy()); 1014 }; 1015 } 1016 1017 protected DeviceAssociation typedCopy() { 1018 return copy(); 1019 } 1020 1021 @Override 1022 public boolean equalsDeep(Base other_) { 1023 if (!super.equalsDeep(other_)) 1024 return false; 1025 if (!(other_ instanceof DeviceAssociation)) 1026 return false; 1027 DeviceAssociation o = (DeviceAssociation) other_; 1028 return compareDeep(identifier, o.identifier, true) && compareDeep(device, o.device, true) && compareDeep(category, o.category, true) 1029 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) && compareDeep(subject, o.subject, true) 1030 && compareDeep(bodyStructure, o.bodyStructure, true) && compareDeep(period, o.period, true) && compareDeep(operation, o.operation, true) 1031 ; 1032 } 1033 1034 @Override 1035 public boolean equalsShallow(Base other_) { 1036 if (!super.equalsShallow(other_)) 1037 return false; 1038 if (!(other_ instanceof DeviceAssociation)) 1039 return false; 1040 DeviceAssociation o = (DeviceAssociation) other_; 1041 return true; 1042 } 1043 1044 public boolean isEmpty() { 1045 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, device, category 1046 , status, statusReason, subject, bodyStructure, period, operation); 1047 } 1048 1049 @Override 1050 public ResourceType getResourceType() { 1051 return ResourceType.DeviceAssociation; 1052 } 1053 1054 /** 1055 * Search parameter: <b>device</b> 1056 * <p> 1057 * Description: <b>Search for products that match this code</b><br> 1058 * Type: <b>reference</b><br> 1059 * Path: <b>DeviceAssociation.device</b><br> 1060 * </p> 1061 */ 1062 @SearchParamDefinition(name="device", path="DeviceAssociation.device", description="Search for products that match this code", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 1063 public static final String SP_DEVICE = "device"; 1064 /** 1065 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1066 * <p> 1067 * Description: <b>Search for products that match this code</b><br> 1068 * Type: <b>reference</b><br> 1069 * Path: <b>DeviceAssociation.device</b><br> 1070 * </p> 1071 */ 1072 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 1073 1074/** 1075 * Constant for fluent queries to be used to add include statements. Specifies 1076 * the path value of "<b>DeviceAssociation:device</b>". 1077 */ 1078 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceAssociation:device").toLocked(); 1079 1080 /** 1081 * Search parameter: <b>identifier</b> 1082 * <p> 1083 * Description: <b>The identifier of the device association</b><br> 1084 * Type: <b>token</b><br> 1085 * Path: <b>DeviceAssociation.identifier</b><br> 1086 * </p> 1087 */ 1088 @SearchParamDefinition(name="identifier", path="DeviceAssociation.identifier", description="The identifier of the device association", type="token" ) 1089 public static final String SP_IDENTIFIER = "identifier"; 1090 /** 1091 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1092 * <p> 1093 * Description: <b>The identifier of the device association</b><br> 1094 * Type: <b>token</b><br> 1095 * Path: <b>DeviceAssociation.identifier</b><br> 1096 * </p> 1097 */ 1098 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1099 1100 /** 1101 * Search parameter: <b>operator</b> 1102 * <p> 1103 * Description: <b>The identity of a operator for whom to list associations</b><br> 1104 * Type: <b>reference</b><br> 1105 * Path: <b>DeviceAssociation.operation.operator</b><br> 1106 * </p> 1107 */ 1108 @SearchParamDefinition(name="operator", path="DeviceAssociation.operation.operator", description="The identity of a operator for whom to list associations", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Patient.class, Practitioner.class, RelatedPerson.class } ) 1109 public static final String SP_OPERATOR = "operator"; 1110 /** 1111 * <b>Fluent Client</b> search parameter constant for <b>operator</b> 1112 * <p> 1113 * Description: <b>The identity of a operator for whom to list associations</b><br> 1114 * Type: <b>reference</b><br> 1115 * Path: <b>DeviceAssociation.operation.operator</b><br> 1116 * </p> 1117 */ 1118 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OPERATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OPERATOR); 1119 1120/** 1121 * Constant for fluent queries to be used to add include statements. Specifies 1122 * the path value of "<b>DeviceAssociation:operator</b>". 1123 */ 1124 public static final ca.uhn.fhir.model.api.Include INCLUDE_OPERATOR = new ca.uhn.fhir.model.api.Include("DeviceAssociation:operator").toLocked(); 1125 1126 /** 1127 * Search parameter: <b>patient</b> 1128 * <p> 1129 * Description: <b>The identity of a patient for whom to list associations</b><br> 1130 * Type: <b>reference</b><br> 1131 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1132 * </p> 1133 */ 1134 @SearchParamDefinition(name="patient", path="DeviceAssociation.subject.where(resolve() is Patient)", description="The identity of a patient for whom to list associations", type="reference", target={Patient.class } ) 1135 public static final String SP_PATIENT = "patient"; 1136 /** 1137 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1138 * <p> 1139 * Description: <b>The identity of a patient for whom to list associations</b><br> 1140 * Type: <b>reference</b><br> 1141 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1142 * </p> 1143 */ 1144 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1145 1146/** 1147 * Constant for fluent queries to be used to add include statements. Specifies 1148 * the path value of "<b>DeviceAssociation:patient</b>". 1149 */ 1150 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceAssociation:patient").toLocked(); 1151 1152 /** 1153 * Search parameter: <b>status</b> 1154 * <p> 1155 * Description: <b>The status of the device associations</b><br> 1156 * Type: <b>token</b><br> 1157 * Path: <b>DeviceAssociation.status</b><br> 1158 * </p> 1159 */ 1160 @SearchParamDefinition(name="status", path="DeviceAssociation.status", description="The status of the device associations", type="token" ) 1161 public static final String SP_STATUS = "status"; 1162 /** 1163 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1164 * <p> 1165 * Description: <b>The status of the device associations</b><br> 1166 * Type: <b>token</b><br> 1167 * Path: <b>DeviceAssociation.status</b><br> 1168 * </p> 1169 */ 1170 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1171 1172 /** 1173 * Search parameter: <b>subject</b> 1174 * <p> 1175 * Description: <b>The identity of a patient for whom to list associations</b><br> 1176 * Type: <b>reference</b><br> 1177 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1178 * </p> 1179 */ 1180 @SearchParamDefinition(name="subject", path="DeviceAssociation.subject.where(resolve() is Patient)", description="The identity of a patient for whom to list associations", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1181 public static final String SP_SUBJECT = "subject"; 1182 /** 1183 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1184 * <p> 1185 * Description: <b>The identity of a patient for whom to list associations</b><br> 1186 * Type: <b>reference</b><br> 1187 * Path: <b>DeviceAssociation.subject.where(resolve() is Patient)</b><br> 1188 * </p> 1189 */ 1190 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1191 1192/** 1193 * Constant for fluent queries to be used to add include statements. Specifies 1194 * the path value of "<b>DeviceAssociation:subject</b>". 1195 */ 1196 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DeviceAssociation:subject").toLocked(); 1197 1198 1199} 1200