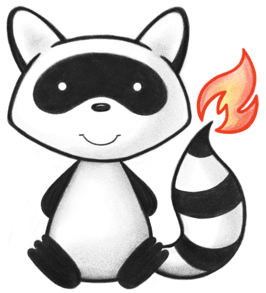
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This is a specialized resource that defines the characteristics and capabilities of a device. 052 */ 053@ResourceDef(name="DeviceDefinition", profile="http://hl7.org/fhir/StructureDefinition/DeviceDefinition") 054public class DeviceDefinition extends DomainResource { 055 056 public enum DeviceCorrectiveActionScope { 057 /** 058 * The corrective action was intended for all units of the same model. 059 */ 060 MODEL, 061 /** 062 * The corrective action was intended for a specific batch of units identified by a lot number. 063 */ 064 LOTNUMBERS, 065 /** 066 * The corrective action was intended for an individual unit (or a set of units) individually identified by serial number. 067 */ 068 SERIALNUMBERS, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static DeviceCorrectiveActionScope fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("model".equals(codeString)) 077 return MODEL; 078 if ("lot-numbers".equals(codeString)) 079 return LOTNUMBERS; 080 if ("serial-numbers".equals(codeString)) 081 return SERIALNUMBERS; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown DeviceCorrectiveActionScope code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case MODEL: return "model"; 090 case LOTNUMBERS: return "lot-numbers"; 091 case SERIALNUMBERS: return "serial-numbers"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case MODEL: return "http://hl7.org/fhir/device-correctiveactionscope"; 099 case LOTNUMBERS: return "http://hl7.org/fhir/device-correctiveactionscope"; 100 case SERIALNUMBERS: return "http://hl7.org/fhir/device-correctiveactionscope"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case MODEL: return "The corrective action was intended for all units of the same model."; 108 case LOTNUMBERS: return "The corrective action was intended for a specific batch of units identified by a lot number."; 109 case SERIALNUMBERS: return "The corrective action was intended for an individual unit (or a set of units) individually identified by serial number."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case MODEL: return "Model"; 117 case LOTNUMBERS: return "Lot Numbers"; 118 case SERIALNUMBERS: return "Serial Numbers"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class DeviceCorrectiveActionScopeEnumFactory implements EnumFactory<DeviceCorrectiveActionScope> { 126 public DeviceCorrectiveActionScope fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("model".equals(codeString)) 131 return DeviceCorrectiveActionScope.MODEL; 132 if ("lot-numbers".equals(codeString)) 133 return DeviceCorrectiveActionScope.LOTNUMBERS; 134 if ("serial-numbers".equals(codeString)) 135 return DeviceCorrectiveActionScope.SERIALNUMBERS; 136 throw new IllegalArgumentException("Unknown DeviceCorrectiveActionScope code '"+codeString+"'"); 137 } 138 public Enumeration<DeviceCorrectiveActionScope> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<DeviceCorrectiveActionScope>(this, DeviceCorrectiveActionScope.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<DeviceCorrectiveActionScope>(this, DeviceCorrectiveActionScope.NULL, code); 146 if ("model".equals(codeString)) 147 return new Enumeration<DeviceCorrectiveActionScope>(this, DeviceCorrectiveActionScope.MODEL, code); 148 if ("lot-numbers".equals(codeString)) 149 return new Enumeration<DeviceCorrectiveActionScope>(this, DeviceCorrectiveActionScope.LOTNUMBERS, code); 150 if ("serial-numbers".equals(codeString)) 151 return new Enumeration<DeviceCorrectiveActionScope>(this, DeviceCorrectiveActionScope.SERIALNUMBERS, code); 152 throw new FHIRException("Unknown DeviceCorrectiveActionScope code '"+codeString+"'"); 153 } 154 public String toCode(DeviceCorrectiveActionScope code) { 155 if (code == DeviceCorrectiveActionScope.NULL) 156 return null; 157 if (code == DeviceCorrectiveActionScope.MODEL) 158 return "model"; 159 if (code == DeviceCorrectiveActionScope.LOTNUMBERS) 160 return "lot-numbers"; 161 if (code == DeviceCorrectiveActionScope.SERIALNUMBERS) 162 return "serial-numbers"; 163 return "?"; 164 } 165 public String toSystem(DeviceCorrectiveActionScope code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum DeviceDefinitionRegulatoryIdentifierType { 171 /** 172 * EUDAMED's basic UDI-DI identifier. 173 */ 174 BASIC, 175 /** 176 * EUDAMED's master UDI-DI identifier. 177 */ 178 MASTER, 179 /** 180 * The identifier is a license number. 181 */ 182 LICENSE, 183 /** 184 * added to help the parsers with the generic types 185 */ 186 NULL; 187 public static DeviceDefinitionRegulatoryIdentifierType fromCode(String codeString) throws FHIRException { 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("basic".equals(codeString)) 191 return BASIC; 192 if ("master".equals(codeString)) 193 return MASTER; 194 if ("license".equals(codeString)) 195 return LICENSE; 196 if (Configuration.isAcceptInvalidEnums()) 197 return null; 198 else 199 throw new FHIRException("Unknown DeviceDefinitionRegulatoryIdentifierType code '"+codeString+"'"); 200 } 201 public String toCode() { 202 switch (this) { 203 case BASIC: return "basic"; 204 case MASTER: return "master"; 205 case LICENSE: return "license"; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 public String getSystem() { 211 switch (this) { 212 case BASIC: return "http://hl7.org/fhir/devicedefinition-regulatory-identifier-type"; 213 case MASTER: return "http://hl7.org/fhir/devicedefinition-regulatory-identifier-type"; 214 case LICENSE: return "http://hl7.org/fhir/devicedefinition-regulatory-identifier-type"; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getDefinition() { 220 switch (this) { 221 case BASIC: return "EUDAMED's basic UDI-DI identifier."; 222 case MASTER: return "EUDAMED's master UDI-DI identifier."; 223 case LICENSE: return "The identifier is a license number."; 224 case NULL: return null; 225 default: return "?"; 226 } 227 } 228 public String getDisplay() { 229 switch (this) { 230 case BASIC: return "Basic"; 231 case MASTER: return "Master"; 232 case LICENSE: return "License"; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 } 238 239 public static class DeviceDefinitionRegulatoryIdentifierTypeEnumFactory implements EnumFactory<DeviceDefinitionRegulatoryIdentifierType> { 240 public DeviceDefinitionRegulatoryIdentifierType fromCode(String codeString) throws IllegalArgumentException { 241 if (codeString == null || "".equals(codeString)) 242 if (codeString == null || "".equals(codeString)) 243 return null; 244 if ("basic".equals(codeString)) 245 return DeviceDefinitionRegulatoryIdentifierType.BASIC; 246 if ("master".equals(codeString)) 247 return DeviceDefinitionRegulatoryIdentifierType.MASTER; 248 if ("license".equals(codeString)) 249 return DeviceDefinitionRegulatoryIdentifierType.LICENSE; 250 throw new IllegalArgumentException("Unknown DeviceDefinitionRegulatoryIdentifierType code '"+codeString+"'"); 251 } 252 public Enumeration<DeviceDefinitionRegulatoryIdentifierType> fromType(PrimitiveType<?> code) throws FHIRException { 253 if (code == null) 254 return null; 255 if (code.isEmpty()) 256 return new Enumeration<DeviceDefinitionRegulatoryIdentifierType>(this, DeviceDefinitionRegulatoryIdentifierType.NULL, code); 257 String codeString = ((PrimitiveType) code).asStringValue(); 258 if (codeString == null || "".equals(codeString)) 259 return new Enumeration<DeviceDefinitionRegulatoryIdentifierType>(this, DeviceDefinitionRegulatoryIdentifierType.NULL, code); 260 if ("basic".equals(codeString)) 261 return new Enumeration<DeviceDefinitionRegulatoryIdentifierType>(this, DeviceDefinitionRegulatoryIdentifierType.BASIC, code); 262 if ("master".equals(codeString)) 263 return new Enumeration<DeviceDefinitionRegulatoryIdentifierType>(this, DeviceDefinitionRegulatoryIdentifierType.MASTER, code); 264 if ("license".equals(codeString)) 265 return new Enumeration<DeviceDefinitionRegulatoryIdentifierType>(this, DeviceDefinitionRegulatoryIdentifierType.LICENSE, code); 266 throw new FHIRException("Unknown DeviceDefinitionRegulatoryIdentifierType code '"+codeString+"'"); 267 } 268 public String toCode(DeviceDefinitionRegulatoryIdentifierType code) { 269 if (code == DeviceDefinitionRegulatoryIdentifierType.NULL) 270 return null; 271 if (code == DeviceDefinitionRegulatoryIdentifierType.BASIC) 272 return "basic"; 273 if (code == DeviceDefinitionRegulatoryIdentifierType.MASTER) 274 return "master"; 275 if (code == DeviceDefinitionRegulatoryIdentifierType.LICENSE) 276 return "license"; 277 return "?"; 278 } 279 public String toSystem(DeviceDefinitionRegulatoryIdentifierType code) { 280 return code.getSystem(); 281 } 282 } 283 284 public enum DeviceProductionIdentifierInUDI { 285 /** 286 * The label includes the lot number. 287 */ 288 LOTNUMBER, 289 /** 290 * The label includes the manufacture date. 291 */ 292 MANUFACTUREDDATE, 293 /** 294 * The label includes the serial number. 295 */ 296 SERIALNUMBER, 297 /** 298 * The label includes the expiration date. 299 */ 300 EXPIRATIONDATE, 301 /** 302 * The label includes the biological source identifier. 303 */ 304 BIOLOGICALSOURCE, 305 /** 306 * The label includes the software version. 307 */ 308 SOFTWAREVERSION, 309 /** 310 * added to help the parsers with the generic types 311 */ 312 NULL; 313 public static DeviceProductionIdentifierInUDI fromCode(String codeString) throws FHIRException { 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("lot-number".equals(codeString)) 317 return LOTNUMBER; 318 if ("manufactured-date".equals(codeString)) 319 return MANUFACTUREDDATE; 320 if ("serial-number".equals(codeString)) 321 return SERIALNUMBER; 322 if ("expiration-date".equals(codeString)) 323 return EXPIRATIONDATE; 324 if ("biological-source".equals(codeString)) 325 return BIOLOGICALSOURCE; 326 if ("software-version".equals(codeString)) 327 return SOFTWAREVERSION; 328 if (Configuration.isAcceptInvalidEnums()) 329 return null; 330 else 331 throw new FHIRException("Unknown DeviceProductionIdentifierInUDI code '"+codeString+"'"); 332 } 333 public String toCode() { 334 switch (this) { 335 case LOTNUMBER: return "lot-number"; 336 case MANUFACTUREDDATE: return "manufactured-date"; 337 case SERIALNUMBER: return "serial-number"; 338 case EXPIRATIONDATE: return "expiration-date"; 339 case BIOLOGICALSOURCE: return "biological-source"; 340 case SOFTWAREVERSION: return "software-version"; 341 case NULL: return null; 342 default: return "?"; 343 } 344 } 345 public String getSystem() { 346 switch (this) { 347 case LOTNUMBER: return "http://hl7.org/fhir/device-productidentifierinudi"; 348 case MANUFACTUREDDATE: return "http://hl7.org/fhir/device-productidentifierinudi"; 349 case SERIALNUMBER: return "http://hl7.org/fhir/device-productidentifierinudi"; 350 case EXPIRATIONDATE: return "http://hl7.org/fhir/device-productidentifierinudi"; 351 case BIOLOGICALSOURCE: return "http://hl7.org/fhir/device-productidentifierinudi"; 352 case SOFTWAREVERSION: return "http://hl7.org/fhir/device-productidentifierinudi"; 353 case NULL: return null; 354 default: return "?"; 355 } 356 } 357 public String getDefinition() { 358 switch (this) { 359 case LOTNUMBER: return "The label includes the lot number."; 360 case MANUFACTUREDDATE: return "The label includes the manufacture date."; 361 case SERIALNUMBER: return "The label includes the serial number."; 362 case EXPIRATIONDATE: return "The label includes the expiration date."; 363 case BIOLOGICALSOURCE: return "The label includes the biological source identifier."; 364 case SOFTWAREVERSION: return "The label includes the software version."; 365 case NULL: return null; 366 default: return "?"; 367 } 368 } 369 public String getDisplay() { 370 switch (this) { 371 case LOTNUMBER: return "Lot Number"; 372 case MANUFACTUREDDATE: return "Manufactured date"; 373 case SERIALNUMBER: return "Serial Number"; 374 case EXPIRATIONDATE: return "Expiration date"; 375 case BIOLOGICALSOURCE: return "Biological source"; 376 case SOFTWAREVERSION: return "Software Version"; 377 case NULL: return null; 378 default: return "?"; 379 } 380 } 381 } 382 383 public static class DeviceProductionIdentifierInUDIEnumFactory implements EnumFactory<DeviceProductionIdentifierInUDI> { 384 public DeviceProductionIdentifierInUDI fromCode(String codeString) throws IllegalArgumentException { 385 if (codeString == null || "".equals(codeString)) 386 if (codeString == null || "".equals(codeString)) 387 return null; 388 if ("lot-number".equals(codeString)) 389 return DeviceProductionIdentifierInUDI.LOTNUMBER; 390 if ("manufactured-date".equals(codeString)) 391 return DeviceProductionIdentifierInUDI.MANUFACTUREDDATE; 392 if ("serial-number".equals(codeString)) 393 return DeviceProductionIdentifierInUDI.SERIALNUMBER; 394 if ("expiration-date".equals(codeString)) 395 return DeviceProductionIdentifierInUDI.EXPIRATIONDATE; 396 if ("biological-source".equals(codeString)) 397 return DeviceProductionIdentifierInUDI.BIOLOGICALSOURCE; 398 if ("software-version".equals(codeString)) 399 return DeviceProductionIdentifierInUDI.SOFTWAREVERSION; 400 throw new IllegalArgumentException("Unknown DeviceProductionIdentifierInUDI code '"+codeString+"'"); 401 } 402 public Enumeration<DeviceProductionIdentifierInUDI> fromType(PrimitiveType<?> code) throws FHIRException { 403 if (code == null) 404 return null; 405 if (code.isEmpty()) 406 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.NULL, code); 407 String codeString = ((PrimitiveType) code).asStringValue(); 408 if (codeString == null || "".equals(codeString)) 409 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.NULL, code); 410 if ("lot-number".equals(codeString)) 411 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.LOTNUMBER, code); 412 if ("manufactured-date".equals(codeString)) 413 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.MANUFACTUREDDATE, code); 414 if ("serial-number".equals(codeString)) 415 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.SERIALNUMBER, code); 416 if ("expiration-date".equals(codeString)) 417 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.EXPIRATIONDATE, code); 418 if ("biological-source".equals(codeString)) 419 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.BIOLOGICALSOURCE, code); 420 if ("software-version".equals(codeString)) 421 return new Enumeration<DeviceProductionIdentifierInUDI>(this, DeviceProductionIdentifierInUDI.SOFTWAREVERSION, code); 422 throw new FHIRException("Unknown DeviceProductionIdentifierInUDI code '"+codeString+"'"); 423 } 424 public String toCode(DeviceProductionIdentifierInUDI code) { 425 if (code == DeviceProductionIdentifierInUDI.NULL) 426 return null; 427 if (code == DeviceProductionIdentifierInUDI.LOTNUMBER) 428 return "lot-number"; 429 if (code == DeviceProductionIdentifierInUDI.MANUFACTUREDDATE) 430 return "manufactured-date"; 431 if (code == DeviceProductionIdentifierInUDI.SERIALNUMBER) 432 return "serial-number"; 433 if (code == DeviceProductionIdentifierInUDI.EXPIRATIONDATE) 434 return "expiration-date"; 435 if (code == DeviceProductionIdentifierInUDI.BIOLOGICALSOURCE) 436 return "biological-source"; 437 if (code == DeviceProductionIdentifierInUDI.SOFTWAREVERSION) 438 return "software-version"; 439 return "?"; 440 } 441 public String toSystem(DeviceProductionIdentifierInUDI code) { 442 return code.getSystem(); 443 } 444 } 445 446 @Block() 447 public static class DeviceDefinitionUdiDeviceIdentifierComponent extends BackboneElement implements IBaseBackboneElement { 448 /** 449 * The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier. 450 */ 451 @Child(name = "deviceIdentifier", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 452 @Description(shortDefinition="The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier", formalDefinition="The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier." ) 453 protected StringType deviceIdentifier; 454 455 /** 456 * The organization that assigns the identifier algorithm. 457 */ 458 @Child(name = "issuer", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=false) 459 @Description(shortDefinition="The organization that assigns the identifier algorithm", formalDefinition="The organization that assigns the identifier algorithm." ) 460 protected UriType issuer; 461 462 /** 463 * The jurisdiction to which the deviceIdentifier applies. 464 */ 465 @Child(name = "jurisdiction", type = {UriType.class}, order=3, min=1, max=1, modifier=false, summary=false) 466 @Description(shortDefinition="The jurisdiction to which the deviceIdentifier applies", formalDefinition="The jurisdiction to which the deviceIdentifier applies." ) 467 protected UriType jurisdiction; 468 469 /** 470 * Indicates where and when the device is available on the market. 471 */ 472 @Child(name = "marketDistribution", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 473 @Description(shortDefinition="Indicates whether and when the device is available on the market", formalDefinition="Indicates where and when the device is available on the market." ) 474 protected List<UdiDeviceIdentifierMarketDistributionComponent> marketDistribution; 475 476 private static final long serialVersionUID = -1819796108L; 477 478 /** 479 * Constructor 480 */ 481 public DeviceDefinitionUdiDeviceIdentifierComponent() { 482 super(); 483 } 484 485 /** 486 * Constructor 487 */ 488 public DeviceDefinitionUdiDeviceIdentifierComponent(String deviceIdentifier, String issuer, String jurisdiction) { 489 super(); 490 this.setDeviceIdentifier(deviceIdentifier); 491 this.setIssuer(issuer); 492 this.setJurisdiction(jurisdiction); 493 } 494 495 /** 496 * @return {@link #deviceIdentifier} (The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 497 */ 498 public StringType getDeviceIdentifierElement() { 499 if (this.deviceIdentifier == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create DeviceDefinitionUdiDeviceIdentifierComponent.deviceIdentifier"); 502 else if (Configuration.doAutoCreate()) 503 this.deviceIdentifier = new StringType(); // bb 504 return this.deviceIdentifier; 505 } 506 507 public boolean hasDeviceIdentifierElement() { 508 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 509 } 510 511 public boolean hasDeviceIdentifier() { 512 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #deviceIdentifier} (The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 517 */ 518 public DeviceDefinitionUdiDeviceIdentifierComponent setDeviceIdentifierElement(StringType value) { 519 this.deviceIdentifier = value; 520 return this; 521 } 522 523 /** 524 * @return The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier. 525 */ 526 public String getDeviceIdentifier() { 527 return this.deviceIdentifier == null ? null : this.deviceIdentifier.getValue(); 528 } 529 530 /** 531 * @param value The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier. 532 */ 533 public DeviceDefinitionUdiDeviceIdentifierComponent setDeviceIdentifier(String value) { 534 if (this.deviceIdentifier == null) 535 this.deviceIdentifier = new StringType(); 536 this.deviceIdentifier.setValue(value); 537 return this; 538 } 539 540 /** 541 * @return {@link #issuer} (The organization that assigns the identifier algorithm.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 542 */ 543 public UriType getIssuerElement() { 544 if (this.issuer == null) 545 if (Configuration.errorOnAutoCreate()) 546 throw new Error("Attempt to auto-create DeviceDefinitionUdiDeviceIdentifierComponent.issuer"); 547 else if (Configuration.doAutoCreate()) 548 this.issuer = new UriType(); // bb 549 return this.issuer; 550 } 551 552 public boolean hasIssuerElement() { 553 return this.issuer != null && !this.issuer.isEmpty(); 554 } 555 556 public boolean hasIssuer() { 557 return this.issuer != null && !this.issuer.isEmpty(); 558 } 559 560 /** 561 * @param value {@link #issuer} (The organization that assigns the identifier algorithm.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 562 */ 563 public DeviceDefinitionUdiDeviceIdentifierComponent setIssuerElement(UriType value) { 564 this.issuer = value; 565 return this; 566 } 567 568 /** 569 * @return The organization that assigns the identifier algorithm. 570 */ 571 public String getIssuer() { 572 return this.issuer == null ? null : this.issuer.getValue(); 573 } 574 575 /** 576 * @param value The organization that assigns the identifier algorithm. 577 */ 578 public DeviceDefinitionUdiDeviceIdentifierComponent setIssuer(String value) { 579 if (this.issuer == null) 580 this.issuer = new UriType(); 581 this.issuer.setValue(value); 582 return this; 583 } 584 585 /** 586 * @return {@link #jurisdiction} (The jurisdiction to which the deviceIdentifier applies.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 587 */ 588 public UriType getJurisdictionElement() { 589 if (this.jurisdiction == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create DeviceDefinitionUdiDeviceIdentifierComponent.jurisdiction"); 592 else if (Configuration.doAutoCreate()) 593 this.jurisdiction = new UriType(); // bb 594 return this.jurisdiction; 595 } 596 597 public boolean hasJurisdictionElement() { 598 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 599 } 600 601 public boolean hasJurisdiction() { 602 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 603 } 604 605 /** 606 * @param value {@link #jurisdiction} (The jurisdiction to which the deviceIdentifier applies.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 607 */ 608 public DeviceDefinitionUdiDeviceIdentifierComponent setJurisdictionElement(UriType value) { 609 this.jurisdiction = value; 610 return this; 611 } 612 613 /** 614 * @return The jurisdiction to which the deviceIdentifier applies. 615 */ 616 public String getJurisdiction() { 617 return this.jurisdiction == null ? null : this.jurisdiction.getValue(); 618 } 619 620 /** 621 * @param value The jurisdiction to which the deviceIdentifier applies. 622 */ 623 public DeviceDefinitionUdiDeviceIdentifierComponent setJurisdiction(String value) { 624 if (this.jurisdiction == null) 625 this.jurisdiction = new UriType(); 626 this.jurisdiction.setValue(value); 627 return this; 628 } 629 630 /** 631 * @return {@link #marketDistribution} (Indicates where and when the device is available on the market.) 632 */ 633 public List<UdiDeviceIdentifierMarketDistributionComponent> getMarketDistribution() { 634 if (this.marketDistribution == null) 635 this.marketDistribution = new ArrayList<UdiDeviceIdentifierMarketDistributionComponent>(); 636 return this.marketDistribution; 637 } 638 639 /** 640 * @return Returns a reference to <code>this</code> for easy method chaining 641 */ 642 public DeviceDefinitionUdiDeviceIdentifierComponent setMarketDistribution(List<UdiDeviceIdentifierMarketDistributionComponent> theMarketDistribution) { 643 this.marketDistribution = theMarketDistribution; 644 return this; 645 } 646 647 public boolean hasMarketDistribution() { 648 if (this.marketDistribution == null) 649 return false; 650 for (UdiDeviceIdentifierMarketDistributionComponent item : this.marketDistribution) 651 if (!item.isEmpty()) 652 return true; 653 return false; 654 } 655 656 public UdiDeviceIdentifierMarketDistributionComponent addMarketDistribution() { //3 657 UdiDeviceIdentifierMarketDistributionComponent t = new UdiDeviceIdentifierMarketDistributionComponent(); 658 if (this.marketDistribution == null) 659 this.marketDistribution = new ArrayList<UdiDeviceIdentifierMarketDistributionComponent>(); 660 this.marketDistribution.add(t); 661 return t; 662 } 663 664 public DeviceDefinitionUdiDeviceIdentifierComponent addMarketDistribution(UdiDeviceIdentifierMarketDistributionComponent t) { //3 665 if (t == null) 666 return this; 667 if (this.marketDistribution == null) 668 this.marketDistribution = new ArrayList<UdiDeviceIdentifierMarketDistributionComponent>(); 669 this.marketDistribution.add(t); 670 return this; 671 } 672 673 /** 674 * @return The first repetition of repeating field {@link #marketDistribution}, creating it if it does not already exist {3} 675 */ 676 public UdiDeviceIdentifierMarketDistributionComponent getMarketDistributionFirstRep() { 677 if (getMarketDistribution().isEmpty()) { 678 addMarketDistribution(); 679 } 680 return getMarketDistribution().get(0); 681 } 682 683 protected void listChildren(List<Property> children) { 684 super.listChildren(children); 685 children.add(new Property("deviceIdentifier", "string", "The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier.", 0, 1, deviceIdentifier)); 686 children.add(new Property("issuer", "uri", "The organization that assigns the identifier algorithm.", 0, 1, issuer)); 687 children.add(new Property("jurisdiction", "uri", "The jurisdiction to which the deviceIdentifier applies.", 0, 1, jurisdiction)); 688 children.add(new Property("marketDistribution", "", "Indicates where and when the device is available on the market.", 0, java.lang.Integer.MAX_VALUE, marketDistribution)); 689 } 690 691 @Override 692 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 693 switch (_hash) { 694 case 1322005407: /*deviceIdentifier*/ return new Property("deviceIdentifier", "string", "The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdiction provided in the DeviceDefinition.udiDeviceIdentifier.", 0, 1, deviceIdentifier); 695 case -1179159879: /*issuer*/ return new Property("issuer", "uri", "The organization that assigns the identifier algorithm.", 0, 1, issuer); 696 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "uri", "The jurisdiction to which the deviceIdentifier applies.", 0, 1, jurisdiction); 697 case 530037984: /*marketDistribution*/ return new Property("marketDistribution", "", "Indicates where and when the device is available on the market.", 0, java.lang.Integer.MAX_VALUE, marketDistribution); 698 default: return super.getNamedProperty(_hash, _name, _checkValid); 699 } 700 701 } 702 703 @Override 704 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 705 switch (hash) { 706 case 1322005407: /*deviceIdentifier*/ return this.deviceIdentifier == null ? new Base[0] : new Base[] {this.deviceIdentifier}; // StringType 707 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // UriType 708 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // UriType 709 case 530037984: /*marketDistribution*/ return this.marketDistribution == null ? new Base[0] : this.marketDistribution.toArray(new Base[this.marketDistribution.size()]); // UdiDeviceIdentifierMarketDistributionComponent 710 default: return super.getProperty(hash, name, checkValid); 711 } 712 713 } 714 715 @Override 716 public Base setProperty(int hash, String name, Base value) throws FHIRException { 717 switch (hash) { 718 case 1322005407: // deviceIdentifier 719 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 720 return value; 721 case -1179159879: // issuer 722 this.issuer = TypeConvertor.castToUri(value); // UriType 723 return value; 724 case -507075711: // jurisdiction 725 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 726 return value; 727 case 530037984: // marketDistribution 728 this.getMarketDistribution().add((UdiDeviceIdentifierMarketDistributionComponent) value); // UdiDeviceIdentifierMarketDistributionComponent 729 return value; 730 default: return super.setProperty(hash, name, value); 731 } 732 733 } 734 735 @Override 736 public Base setProperty(String name, Base value) throws FHIRException { 737 if (name.equals("deviceIdentifier")) { 738 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 739 } else if (name.equals("issuer")) { 740 this.issuer = TypeConvertor.castToUri(value); // UriType 741 } else if (name.equals("jurisdiction")) { 742 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 743 } else if (name.equals("marketDistribution")) { 744 this.getMarketDistribution().add((UdiDeviceIdentifierMarketDistributionComponent) value); 745 } else 746 return super.setProperty(name, value); 747 return value; 748 } 749 750 @Override 751 public void removeChild(String name, Base value) throws FHIRException { 752 if (name.equals("deviceIdentifier")) { 753 this.deviceIdentifier = null; 754 } else if (name.equals("issuer")) { 755 this.issuer = null; 756 } else if (name.equals("jurisdiction")) { 757 this.jurisdiction = null; 758 } else if (name.equals("marketDistribution")) { 759 this.getMarketDistribution().remove((UdiDeviceIdentifierMarketDistributionComponent) value); 760 } else 761 super.removeChild(name, value); 762 763 } 764 765 @Override 766 public Base makeProperty(int hash, String name) throws FHIRException { 767 switch (hash) { 768 case 1322005407: return getDeviceIdentifierElement(); 769 case -1179159879: return getIssuerElement(); 770 case -507075711: return getJurisdictionElement(); 771 case 530037984: return addMarketDistribution(); 772 default: return super.makeProperty(hash, name); 773 } 774 775 } 776 777 @Override 778 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 779 switch (hash) { 780 case 1322005407: /*deviceIdentifier*/ return new String[] {"string"}; 781 case -1179159879: /*issuer*/ return new String[] {"uri"}; 782 case -507075711: /*jurisdiction*/ return new String[] {"uri"}; 783 case 530037984: /*marketDistribution*/ return new String[] {}; 784 default: return super.getTypesForProperty(hash, name); 785 } 786 787 } 788 789 @Override 790 public Base addChild(String name) throws FHIRException { 791 if (name.equals("deviceIdentifier")) { 792 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.udiDeviceIdentifier.deviceIdentifier"); 793 } 794 else if (name.equals("issuer")) { 795 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.udiDeviceIdentifier.issuer"); 796 } 797 else if (name.equals("jurisdiction")) { 798 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.udiDeviceIdentifier.jurisdiction"); 799 } 800 else if (name.equals("marketDistribution")) { 801 return addMarketDistribution(); 802 } 803 else 804 return super.addChild(name); 805 } 806 807 public DeviceDefinitionUdiDeviceIdentifierComponent copy() { 808 DeviceDefinitionUdiDeviceIdentifierComponent dst = new DeviceDefinitionUdiDeviceIdentifierComponent(); 809 copyValues(dst); 810 return dst; 811 } 812 813 public void copyValues(DeviceDefinitionUdiDeviceIdentifierComponent dst) { 814 super.copyValues(dst); 815 dst.deviceIdentifier = deviceIdentifier == null ? null : deviceIdentifier.copy(); 816 dst.issuer = issuer == null ? null : issuer.copy(); 817 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 818 if (marketDistribution != null) { 819 dst.marketDistribution = new ArrayList<UdiDeviceIdentifierMarketDistributionComponent>(); 820 for (UdiDeviceIdentifierMarketDistributionComponent i : marketDistribution) 821 dst.marketDistribution.add(i.copy()); 822 }; 823 } 824 825 @Override 826 public boolean equalsDeep(Base other_) { 827 if (!super.equalsDeep(other_)) 828 return false; 829 if (!(other_ instanceof DeviceDefinitionUdiDeviceIdentifierComponent)) 830 return false; 831 DeviceDefinitionUdiDeviceIdentifierComponent o = (DeviceDefinitionUdiDeviceIdentifierComponent) other_; 832 return compareDeep(deviceIdentifier, o.deviceIdentifier, true) && compareDeep(issuer, o.issuer, true) 833 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(marketDistribution, o.marketDistribution, true) 834 ; 835 } 836 837 @Override 838 public boolean equalsShallow(Base other_) { 839 if (!super.equalsShallow(other_)) 840 return false; 841 if (!(other_ instanceof DeviceDefinitionUdiDeviceIdentifierComponent)) 842 return false; 843 DeviceDefinitionUdiDeviceIdentifierComponent o = (DeviceDefinitionUdiDeviceIdentifierComponent) other_; 844 return compareValues(deviceIdentifier, o.deviceIdentifier, true) && compareValues(issuer, o.issuer, true) 845 && compareValues(jurisdiction, o.jurisdiction, true); 846 } 847 848 public boolean isEmpty() { 849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(deviceIdentifier, issuer, jurisdiction 850 , marketDistribution); 851 } 852 853 public String fhirType() { 854 return "DeviceDefinition.udiDeviceIdentifier"; 855 856 } 857 858 } 859 860 @Block() 861 public static class UdiDeviceIdentifierMarketDistributionComponent extends BackboneElement implements IBaseBackboneElement { 862 /** 863 * Begin and end dates for the commercial distribution of the device. 864 */ 865 @Child(name = "marketPeriod", type = {Period.class}, order=1, min=1, max=1, modifier=false, summary=false) 866 @Description(shortDefinition="Begin and end dates for the commercial distribution of the device", formalDefinition="Begin and end dates for the commercial distribution of the device." ) 867 protected Period marketPeriod; 868 869 /** 870 * National state or territory to which the marketDistribution recers, typically where the device is commercialized. 871 */ 872 @Child(name = "subJurisdiction", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=false) 873 @Description(shortDefinition="National state or territory where the device is commercialized", formalDefinition="National state or territory to which the marketDistribution recers, typically where the device is commercialized." ) 874 protected UriType subJurisdiction; 875 876 private static final long serialVersionUID = -1459036847L; 877 878 /** 879 * Constructor 880 */ 881 public UdiDeviceIdentifierMarketDistributionComponent() { 882 super(); 883 } 884 885 /** 886 * Constructor 887 */ 888 public UdiDeviceIdentifierMarketDistributionComponent(Period marketPeriod, String subJurisdiction) { 889 super(); 890 this.setMarketPeriod(marketPeriod); 891 this.setSubJurisdiction(subJurisdiction); 892 } 893 894 /** 895 * @return {@link #marketPeriod} (Begin and end dates for the commercial distribution of the device.) 896 */ 897 public Period getMarketPeriod() { 898 if (this.marketPeriod == null) 899 if (Configuration.errorOnAutoCreate()) 900 throw new Error("Attempt to auto-create UdiDeviceIdentifierMarketDistributionComponent.marketPeriod"); 901 else if (Configuration.doAutoCreate()) 902 this.marketPeriod = new Period(); // cc 903 return this.marketPeriod; 904 } 905 906 public boolean hasMarketPeriod() { 907 return this.marketPeriod != null && !this.marketPeriod.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #marketPeriod} (Begin and end dates for the commercial distribution of the device.) 912 */ 913 public UdiDeviceIdentifierMarketDistributionComponent setMarketPeriod(Period value) { 914 this.marketPeriod = value; 915 return this; 916 } 917 918 /** 919 * @return {@link #subJurisdiction} (National state or territory to which the marketDistribution recers, typically where the device is commercialized.). This is the underlying object with id, value and extensions. The accessor "getSubJurisdiction" gives direct access to the value 920 */ 921 public UriType getSubJurisdictionElement() { 922 if (this.subJurisdiction == null) 923 if (Configuration.errorOnAutoCreate()) 924 throw new Error("Attempt to auto-create UdiDeviceIdentifierMarketDistributionComponent.subJurisdiction"); 925 else if (Configuration.doAutoCreate()) 926 this.subJurisdiction = new UriType(); // bb 927 return this.subJurisdiction; 928 } 929 930 public boolean hasSubJurisdictionElement() { 931 return this.subJurisdiction != null && !this.subJurisdiction.isEmpty(); 932 } 933 934 public boolean hasSubJurisdiction() { 935 return this.subJurisdiction != null && !this.subJurisdiction.isEmpty(); 936 } 937 938 /** 939 * @param value {@link #subJurisdiction} (National state or territory to which the marketDistribution recers, typically where the device is commercialized.). This is the underlying object with id, value and extensions. The accessor "getSubJurisdiction" gives direct access to the value 940 */ 941 public UdiDeviceIdentifierMarketDistributionComponent setSubJurisdictionElement(UriType value) { 942 this.subJurisdiction = value; 943 return this; 944 } 945 946 /** 947 * @return National state or territory to which the marketDistribution recers, typically where the device is commercialized. 948 */ 949 public String getSubJurisdiction() { 950 return this.subJurisdiction == null ? null : this.subJurisdiction.getValue(); 951 } 952 953 /** 954 * @param value National state or territory to which the marketDistribution recers, typically where the device is commercialized. 955 */ 956 public UdiDeviceIdentifierMarketDistributionComponent setSubJurisdiction(String value) { 957 if (this.subJurisdiction == null) 958 this.subJurisdiction = new UriType(); 959 this.subJurisdiction.setValue(value); 960 return this; 961 } 962 963 protected void listChildren(List<Property> children) { 964 super.listChildren(children); 965 children.add(new Property("marketPeriod", "Period", "Begin and end dates for the commercial distribution of the device.", 0, 1, marketPeriod)); 966 children.add(new Property("subJurisdiction", "uri", "National state or territory to which the marketDistribution recers, typically where the device is commercialized.", 0, 1, subJurisdiction)); 967 } 968 969 @Override 970 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 971 switch (_hash) { 972 case -183772899: /*marketPeriod*/ return new Property("marketPeriod", "Period", "Begin and end dates for the commercial distribution of the device.", 0, 1, marketPeriod); 973 case -777497119: /*subJurisdiction*/ return new Property("subJurisdiction", "uri", "National state or territory to which the marketDistribution recers, typically where the device is commercialized.", 0, 1, subJurisdiction); 974 default: return super.getNamedProperty(_hash, _name, _checkValid); 975 } 976 977 } 978 979 @Override 980 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 981 switch (hash) { 982 case -183772899: /*marketPeriod*/ return this.marketPeriod == null ? new Base[0] : new Base[] {this.marketPeriod}; // Period 983 case -777497119: /*subJurisdiction*/ return this.subJurisdiction == null ? new Base[0] : new Base[] {this.subJurisdiction}; // UriType 984 default: return super.getProperty(hash, name, checkValid); 985 } 986 987 } 988 989 @Override 990 public Base setProperty(int hash, String name, Base value) throws FHIRException { 991 switch (hash) { 992 case -183772899: // marketPeriod 993 this.marketPeriod = TypeConvertor.castToPeriod(value); // Period 994 return value; 995 case -777497119: // subJurisdiction 996 this.subJurisdiction = TypeConvertor.castToUri(value); // UriType 997 return value; 998 default: return super.setProperty(hash, name, value); 999 } 1000 1001 } 1002 1003 @Override 1004 public Base setProperty(String name, Base value) throws FHIRException { 1005 if (name.equals("marketPeriod")) { 1006 this.marketPeriod = TypeConvertor.castToPeriod(value); // Period 1007 } else if (name.equals("subJurisdiction")) { 1008 this.subJurisdiction = TypeConvertor.castToUri(value); // UriType 1009 } else 1010 return super.setProperty(name, value); 1011 return value; 1012 } 1013 1014 @Override 1015 public void removeChild(String name, Base value) throws FHIRException { 1016 if (name.equals("marketPeriod")) { 1017 this.marketPeriod = null; 1018 } else if (name.equals("subJurisdiction")) { 1019 this.subJurisdiction = null; 1020 } else 1021 super.removeChild(name, value); 1022 1023 } 1024 1025 @Override 1026 public Base makeProperty(int hash, String name) throws FHIRException { 1027 switch (hash) { 1028 case -183772899: return getMarketPeriod(); 1029 case -777497119: return getSubJurisdictionElement(); 1030 default: return super.makeProperty(hash, name); 1031 } 1032 1033 } 1034 1035 @Override 1036 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1037 switch (hash) { 1038 case -183772899: /*marketPeriod*/ return new String[] {"Period"}; 1039 case -777497119: /*subJurisdiction*/ return new String[] {"uri"}; 1040 default: return super.getTypesForProperty(hash, name); 1041 } 1042 1043 } 1044 1045 @Override 1046 public Base addChild(String name) throws FHIRException { 1047 if (name.equals("marketPeriod")) { 1048 this.marketPeriod = new Period(); 1049 return this.marketPeriod; 1050 } 1051 else if (name.equals("subJurisdiction")) { 1052 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.udiDeviceIdentifier.marketDistribution.subJurisdiction"); 1053 } 1054 else 1055 return super.addChild(name); 1056 } 1057 1058 public UdiDeviceIdentifierMarketDistributionComponent copy() { 1059 UdiDeviceIdentifierMarketDistributionComponent dst = new UdiDeviceIdentifierMarketDistributionComponent(); 1060 copyValues(dst); 1061 return dst; 1062 } 1063 1064 public void copyValues(UdiDeviceIdentifierMarketDistributionComponent dst) { 1065 super.copyValues(dst); 1066 dst.marketPeriod = marketPeriod == null ? null : marketPeriod.copy(); 1067 dst.subJurisdiction = subJurisdiction == null ? null : subJurisdiction.copy(); 1068 } 1069 1070 @Override 1071 public boolean equalsDeep(Base other_) { 1072 if (!super.equalsDeep(other_)) 1073 return false; 1074 if (!(other_ instanceof UdiDeviceIdentifierMarketDistributionComponent)) 1075 return false; 1076 UdiDeviceIdentifierMarketDistributionComponent o = (UdiDeviceIdentifierMarketDistributionComponent) other_; 1077 return compareDeep(marketPeriod, o.marketPeriod, true) && compareDeep(subJurisdiction, o.subJurisdiction, true) 1078 ; 1079 } 1080 1081 @Override 1082 public boolean equalsShallow(Base other_) { 1083 if (!super.equalsShallow(other_)) 1084 return false; 1085 if (!(other_ instanceof UdiDeviceIdentifierMarketDistributionComponent)) 1086 return false; 1087 UdiDeviceIdentifierMarketDistributionComponent o = (UdiDeviceIdentifierMarketDistributionComponent) other_; 1088 return compareValues(subJurisdiction, o.subJurisdiction, true); 1089 } 1090 1091 public boolean isEmpty() { 1092 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(marketPeriod, subJurisdiction 1093 ); 1094 } 1095 1096 public String fhirType() { 1097 return "DeviceDefinition.udiDeviceIdentifier.marketDistribution"; 1098 1099 } 1100 1101 } 1102 1103 @Block() 1104 public static class DeviceDefinitionRegulatoryIdentifierComponent extends BackboneElement implements IBaseBackboneElement { 1105 /** 1106 * The type of identifier itself. 1107 */ 1108 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1109 @Description(shortDefinition="basic | master | license", formalDefinition="The type of identifier itself." ) 1110 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicedefinition-regulatory-identifier-type") 1111 protected Enumeration<DeviceDefinitionRegulatoryIdentifierType> type; 1112 1113 /** 1114 * The identifier itself. 1115 */ 1116 @Child(name = "deviceIdentifier", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1117 @Description(shortDefinition="The identifier itself", formalDefinition="The identifier itself." ) 1118 protected StringType deviceIdentifier; 1119 1120 /** 1121 * The organization that issued this identifier. 1122 */ 1123 @Child(name = "issuer", type = {UriType.class}, order=3, min=1, max=1, modifier=false, summary=false) 1124 @Description(shortDefinition="The organization that issued this identifier", formalDefinition="The organization that issued this identifier." ) 1125 protected UriType issuer; 1126 1127 /** 1128 * The jurisdiction to which the deviceIdentifier applies. 1129 */ 1130 @Child(name = "jurisdiction", type = {UriType.class}, order=4, min=1, max=1, modifier=false, summary=false) 1131 @Description(shortDefinition="The jurisdiction to which the deviceIdentifier applies", formalDefinition="The jurisdiction to which the deviceIdentifier applies." ) 1132 protected UriType jurisdiction; 1133 1134 private static final long serialVersionUID = 1438058623L; 1135 1136 /** 1137 * Constructor 1138 */ 1139 public DeviceDefinitionRegulatoryIdentifierComponent() { 1140 super(); 1141 } 1142 1143 /** 1144 * Constructor 1145 */ 1146 public DeviceDefinitionRegulatoryIdentifierComponent(DeviceDefinitionRegulatoryIdentifierType type, String deviceIdentifier, String issuer, String jurisdiction) { 1147 super(); 1148 this.setType(type); 1149 this.setDeviceIdentifier(deviceIdentifier); 1150 this.setIssuer(issuer); 1151 this.setJurisdiction(jurisdiction); 1152 } 1153 1154 /** 1155 * @return {@link #type} (The type of identifier itself.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1156 */ 1157 public Enumeration<DeviceDefinitionRegulatoryIdentifierType> getTypeElement() { 1158 if (this.type == null) 1159 if (Configuration.errorOnAutoCreate()) 1160 throw new Error("Attempt to auto-create DeviceDefinitionRegulatoryIdentifierComponent.type"); 1161 else if (Configuration.doAutoCreate()) 1162 this.type = new Enumeration<DeviceDefinitionRegulatoryIdentifierType>(new DeviceDefinitionRegulatoryIdentifierTypeEnumFactory()); // bb 1163 return this.type; 1164 } 1165 1166 public boolean hasTypeElement() { 1167 return this.type != null && !this.type.isEmpty(); 1168 } 1169 1170 public boolean hasType() { 1171 return this.type != null && !this.type.isEmpty(); 1172 } 1173 1174 /** 1175 * @param value {@link #type} (The type of identifier itself.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1176 */ 1177 public DeviceDefinitionRegulatoryIdentifierComponent setTypeElement(Enumeration<DeviceDefinitionRegulatoryIdentifierType> value) { 1178 this.type = value; 1179 return this; 1180 } 1181 1182 /** 1183 * @return The type of identifier itself. 1184 */ 1185 public DeviceDefinitionRegulatoryIdentifierType getType() { 1186 return this.type == null ? null : this.type.getValue(); 1187 } 1188 1189 /** 1190 * @param value The type of identifier itself. 1191 */ 1192 public DeviceDefinitionRegulatoryIdentifierComponent setType(DeviceDefinitionRegulatoryIdentifierType value) { 1193 if (this.type == null) 1194 this.type = new Enumeration<DeviceDefinitionRegulatoryIdentifierType>(new DeviceDefinitionRegulatoryIdentifierTypeEnumFactory()); 1195 this.type.setValue(value); 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #deviceIdentifier} (The identifier itself.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 1201 */ 1202 public StringType getDeviceIdentifierElement() { 1203 if (this.deviceIdentifier == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create DeviceDefinitionRegulatoryIdentifierComponent.deviceIdentifier"); 1206 else if (Configuration.doAutoCreate()) 1207 this.deviceIdentifier = new StringType(); // bb 1208 return this.deviceIdentifier; 1209 } 1210 1211 public boolean hasDeviceIdentifierElement() { 1212 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 1213 } 1214 1215 public boolean hasDeviceIdentifier() { 1216 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 1217 } 1218 1219 /** 1220 * @param value {@link #deviceIdentifier} (The identifier itself.). This is the underlying object with id, value and extensions. The accessor "getDeviceIdentifier" gives direct access to the value 1221 */ 1222 public DeviceDefinitionRegulatoryIdentifierComponent setDeviceIdentifierElement(StringType value) { 1223 this.deviceIdentifier = value; 1224 return this; 1225 } 1226 1227 /** 1228 * @return The identifier itself. 1229 */ 1230 public String getDeviceIdentifier() { 1231 return this.deviceIdentifier == null ? null : this.deviceIdentifier.getValue(); 1232 } 1233 1234 /** 1235 * @param value The identifier itself. 1236 */ 1237 public DeviceDefinitionRegulatoryIdentifierComponent setDeviceIdentifier(String value) { 1238 if (this.deviceIdentifier == null) 1239 this.deviceIdentifier = new StringType(); 1240 this.deviceIdentifier.setValue(value); 1241 return this; 1242 } 1243 1244 /** 1245 * @return {@link #issuer} (The organization that issued this identifier.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 1246 */ 1247 public UriType getIssuerElement() { 1248 if (this.issuer == null) 1249 if (Configuration.errorOnAutoCreate()) 1250 throw new Error("Attempt to auto-create DeviceDefinitionRegulatoryIdentifierComponent.issuer"); 1251 else if (Configuration.doAutoCreate()) 1252 this.issuer = new UriType(); // bb 1253 return this.issuer; 1254 } 1255 1256 public boolean hasIssuerElement() { 1257 return this.issuer != null && !this.issuer.isEmpty(); 1258 } 1259 1260 public boolean hasIssuer() { 1261 return this.issuer != null && !this.issuer.isEmpty(); 1262 } 1263 1264 /** 1265 * @param value {@link #issuer} (The organization that issued this identifier.). This is the underlying object with id, value and extensions. The accessor "getIssuer" gives direct access to the value 1266 */ 1267 public DeviceDefinitionRegulatoryIdentifierComponent setIssuerElement(UriType value) { 1268 this.issuer = value; 1269 return this; 1270 } 1271 1272 /** 1273 * @return The organization that issued this identifier. 1274 */ 1275 public String getIssuer() { 1276 return this.issuer == null ? null : this.issuer.getValue(); 1277 } 1278 1279 /** 1280 * @param value The organization that issued this identifier. 1281 */ 1282 public DeviceDefinitionRegulatoryIdentifierComponent setIssuer(String value) { 1283 if (this.issuer == null) 1284 this.issuer = new UriType(); 1285 this.issuer.setValue(value); 1286 return this; 1287 } 1288 1289 /** 1290 * @return {@link #jurisdiction} (The jurisdiction to which the deviceIdentifier applies.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 1291 */ 1292 public UriType getJurisdictionElement() { 1293 if (this.jurisdiction == null) 1294 if (Configuration.errorOnAutoCreate()) 1295 throw new Error("Attempt to auto-create DeviceDefinitionRegulatoryIdentifierComponent.jurisdiction"); 1296 else if (Configuration.doAutoCreate()) 1297 this.jurisdiction = new UriType(); // bb 1298 return this.jurisdiction; 1299 } 1300 1301 public boolean hasJurisdictionElement() { 1302 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 1303 } 1304 1305 public boolean hasJurisdiction() { 1306 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 1307 } 1308 1309 /** 1310 * @param value {@link #jurisdiction} (The jurisdiction to which the deviceIdentifier applies.). This is the underlying object with id, value and extensions. The accessor "getJurisdiction" gives direct access to the value 1311 */ 1312 public DeviceDefinitionRegulatoryIdentifierComponent setJurisdictionElement(UriType value) { 1313 this.jurisdiction = value; 1314 return this; 1315 } 1316 1317 /** 1318 * @return The jurisdiction to which the deviceIdentifier applies. 1319 */ 1320 public String getJurisdiction() { 1321 return this.jurisdiction == null ? null : this.jurisdiction.getValue(); 1322 } 1323 1324 /** 1325 * @param value The jurisdiction to which the deviceIdentifier applies. 1326 */ 1327 public DeviceDefinitionRegulatoryIdentifierComponent setJurisdiction(String value) { 1328 if (this.jurisdiction == null) 1329 this.jurisdiction = new UriType(); 1330 this.jurisdiction.setValue(value); 1331 return this; 1332 } 1333 1334 protected void listChildren(List<Property> children) { 1335 super.listChildren(children); 1336 children.add(new Property("type", "code", "The type of identifier itself.", 0, 1, type)); 1337 children.add(new Property("deviceIdentifier", "string", "The identifier itself.", 0, 1, deviceIdentifier)); 1338 children.add(new Property("issuer", "uri", "The organization that issued this identifier.", 0, 1, issuer)); 1339 children.add(new Property("jurisdiction", "uri", "The jurisdiction to which the deviceIdentifier applies.", 0, 1, jurisdiction)); 1340 } 1341 1342 @Override 1343 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1344 switch (_hash) { 1345 case 3575610: /*type*/ return new Property("type", "code", "The type of identifier itself.", 0, 1, type); 1346 case 1322005407: /*deviceIdentifier*/ return new Property("deviceIdentifier", "string", "The identifier itself.", 0, 1, deviceIdentifier); 1347 case -1179159879: /*issuer*/ return new Property("issuer", "uri", "The organization that issued this identifier.", 0, 1, issuer); 1348 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "uri", "The jurisdiction to which the deviceIdentifier applies.", 0, 1, jurisdiction); 1349 default: return super.getNamedProperty(_hash, _name, _checkValid); 1350 } 1351 1352 } 1353 1354 @Override 1355 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1356 switch (hash) { 1357 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DeviceDefinitionRegulatoryIdentifierType> 1358 case 1322005407: /*deviceIdentifier*/ return this.deviceIdentifier == null ? new Base[0] : new Base[] {this.deviceIdentifier}; // StringType 1359 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // UriType 1360 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // UriType 1361 default: return super.getProperty(hash, name, checkValid); 1362 } 1363 1364 } 1365 1366 @Override 1367 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1368 switch (hash) { 1369 case 3575610: // type 1370 value = new DeviceDefinitionRegulatoryIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1371 this.type = (Enumeration) value; // Enumeration<DeviceDefinitionRegulatoryIdentifierType> 1372 return value; 1373 case 1322005407: // deviceIdentifier 1374 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 1375 return value; 1376 case -1179159879: // issuer 1377 this.issuer = TypeConvertor.castToUri(value); // UriType 1378 return value; 1379 case -507075711: // jurisdiction 1380 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 1381 return value; 1382 default: return super.setProperty(hash, name, value); 1383 } 1384 1385 } 1386 1387 @Override 1388 public Base setProperty(String name, Base value) throws FHIRException { 1389 if (name.equals("type")) { 1390 value = new DeviceDefinitionRegulatoryIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1391 this.type = (Enumeration) value; // Enumeration<DeviceDefinitionRegulatoryIdentifierType> 1392 } else if (name.equals("deviceIdentifier")) { 1393 this.deviceIdentifier = TypeConvertor.castToString(value); // StringType 1394 } else if (name.equals("issuer")) { 1395 this.issuer = TypeConvertor.castToUri(value); // UriType 1396 } else if (name.equals("jurisdiction")) { 1397 this.jurisdiction = TypeConvertor.castToUri(value); // UriType 1398 } else 1399 return super.setProperty(name, value); 1400 return value; 1401 } 1402 1403 @Override 1404 public void removeChild(String name, Base value) throws FHIRException { 1405 if (name.equals("type")) { 1406 value = new DeviceDefinitionRegulatoryIdentifierTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1407 this.type = (Enumeration) value; // Enumeration<DeviceDefinitionRegulatoryIdentifierType> 1408 } else if (name.equals("deviceIdentifier")) { 1409 this.deviceIdentifier = null; 1410 } else if (name.equals("issuer")) { 1411 this.issuer = null; 1412 } else if (name.equals("jurisdiction")) { 1413 this.jurisdiction = null; 1414 } else 1415 super.removeChild(name, value); 1416 1417 } 1418 1419 @Override 1420 public Base makeProperty(int hash, String name) throws FHIRException { 1421 switch (hash) { 1422 case 3575610: return getTypeElement(); 1423 case 1322005407: return getDeviceIdentifierElement(); 1424 case -1179159879: return getIssuerElement(); 1425 case -507075711: return getJurisdictionElement(); 1426 default: return super.makeProperty(hash, name); 1427 } 1428 1429 } 1430 1431 @Override 1432 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1433 switch (hash) { 1434 case 3575610: /*type*/ return new String[] {"code"}; 1435 case 1322005407: /*deviceIdentifier*/ return new String[] {"string"}; 1436 case -1179159879: /*issuer*/ return new String[] {"uri"}; 1437 case -507075711: /*jurisdiction*/ return new String[] {"uri"}; 1438 default: return super.getTypesForProperty(hash, name); 1439 } 1440 1441 } 1442 1443 @Override 1444 public Base addChild(String name) throws FHIRException { 1445 if (name.equals("type")) { 1446 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.regulatoryIdentifier.type"); 1447 } 1448 else if (name.equals("deviceIdentifier")) { 1449 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.regulatoryIdentifier.deviceIdentifier"); 1450 } 1451 else if (name.equals("issuer")) { 1452 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.regulatoryIdentifier.issuer"); 1453 } 1454 else if (name.equals("jurisdiction")) { 1455 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.regulatoryIdentifier.jurisdiction"); 1456 } 1457 else 1458 return super.addChild(name); 1459 } 1460 1461 public DeviceDefinitionRegulatoryIdentifierComponent copy() { 1462 DeviceDefinitionRegulatoryIdentifierComponent dst = new DeviceDefinitionRegulatoryIdentifierComponent(); 1463 copyValues(dst); 1464 return dst; 1465 } 1466 1467 public void copyValues(DeviceDefinitionRegulatoryIdentifierComponent dst) { 1468 super.copyValues(dst); 1469 dst.type = type == null ? null : type.copy(); 1470 dst.deviceIdentifier = deviceIdentifier == null ? null : deviceIdentifier.copy(); 1471 dst.issuer = issuer == null ? null : issuer.copy(); 1472 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 1473 } 1474 1475 @Override 1476 public boolean equalsDeep(Base other_) { 1477 if (!super.equalsDeep(other_)) 1478 return false; 1479 if (!(other_ instanceof DeviceDefinitionRegulatoryIdentifierComponent)) 1480 return false; 1481 DeviceDefinitionRegulatoryIdentifierComponent o = (DeviceDefinitionRegulatoryIdentifierComponent) other_; 1482 return compareDeep(type, o.type, true) && compareDeep(deviceIdentifier, o.deviceIdentifier, true) 1483 && compareDeep(issuer, o.issuer, true) && compareDeep(jurisdiction, o.jurisdiction, true); 1484 } 1485 1486 @Override 1487 public boolean equalsShallow(Base other_) { 1488 if (!super.equalsShallow(other_)) 1489 return false; 1490 if (!(other_ instanceof DeviceDefinitionRegulatoryIdentifierComponent)) 1491 return false; 1492 DeviceDefinitionRegulatoryIdentifierComponent o = (DeviceDefinitionRegulatoryIdentifierComponent) other_; 1493 return compareValues(type, o.type, true) && compareValues(deviceIdentifier, o.deviceIdentifier, true) 1494 && compareValues(issuer, o.issuer, true) && compareValues(jurisdiction, o.jurisdiction, true); 1495 } 1496 1497 public boolean isEmpty() { 1498 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, deviceIdentifier, issuer 1499 , jurisdiction); 1500 } 1501 1502 public String fhirType() { 1503 return "DeviceDefinition.regulatoryIdentifier"; 1504 1505 } 1506 1507 } 1508 1509 @Block() 1510 public static class DeviceDefinitionDeviceNameComponent extends BackboneElement implements IBaseBackboneElement { 1511 /** 1512 * A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other. 1513 */ 1514 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1515 @Description(shortDefinition="A name that is used to refer to the device", formalDefinition="A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other." ) 1516 protected StringType name; 1517 1518 /** 1519 * The type of deviceName. 1520RegisteredName | UserFriendlyName | PatientReportedName. 1521 */ 1522 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1523 @Description(shortDefinition="registered-name | user-friendly-name | patient-reported-name", formalDefinition="The type of deviceName.\nRegisteredName | UserFriendlyName | PatientReportedName." ) 1524 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-nametype") 1525 protected Enumeration<DeviceNameType> type; 1526 1527 private static final long serialVersionUID = 918983440L; 1528 1529 /** 1530 * Constructor 1531 */ 1532 public DeviceDefinitionDeviceNameComponent() { 1533 super(); 1534 } 1535 1536 /** 1537 * Constructor 1538 */ 1539 public DeviceDefinitionDeviceNameComponent(String name, DeviceNameType type) { 1540 super(); 1541 this.setName(name); 1542 this.setType(type); 1543 } 1544 1545 /** 1546 * @return {@link #name} (A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1547 */ 1548 public StringType getNameElement() { 1549 if (this.name == null) 1550 if (Configuration.errorOnAutoCreate()) 1551 throw new Error("Attempt to auto-create DeviceDefinitionDeviceNameComponent.name"); 1552 else if (Configuration.doAutoCreate()) 1553 this.name = new StringType(); // bb 1554 return this.name; 1555 } 1556 1557 public boolean hasNameElement() { 1558 return this.name != null && !this.name.isEmpty(); 1559 } 1560 1561 public boolean hasName() { 1562 return this.name != null && !this.name.isEmpty(); 1563 } 1564 1565 /** 1566 * @param value {@link #name} (A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1567 */ 1568 public DeviceDefinitionDeviceNameComponent setNameElement(StringType value) { 1569 this.name = value; 1570 return this; 1571 } 1572 1573 /** 1574 * @return A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other. 1575 */ 1576 public String getName() { 1577 return this.name == null ? null : this.name.getValue(); 1578 } 1579 1580 /** 1581 * @param value A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other. 1582 */ 1583 public DeviceDefinitionDeviceNameComponent setName(String value) { 1584 if (this.name == null) 1585 this.name = new StringType(); 1586 this.name.setValue(value); 1587 return this; 1588 } 1589 1590 /** 1591 * @return {@link #type} (The type of deviceName. 1592RegisteredName | UserFriendlyName | PatientReportedName.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1593 */ 1594 public Enumeration<DeviceNameType> getTypeElement() { 1595 if (this.type == null) 1596 if (Configuration.errorOnAutoCreate()) 1597 throw new Error("Attempt to auto-create DeviceDefinitionDeviceNameComponent.type"); 1598 else if (Configuration.doAutoCreate()) 1599 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); // bb 1600 return this.type; 1601 } 1602 1603 public boolean hasTypeElement() { 1604 return this.type != null && !this.type.isEmpty(); 1605 } 1606 1607 public boolean hasType() { 1608 return this.type != null && !this.type.isEmpty(); 1609 } 1610 1611 /** 1612 * @param value {@link #type} (The type of deviceName. 1613RegisteredName | UserFriendlyName | PatientReportedName.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1614 */ 1615 public DeviceDefinitionDeviceNameComponent setTypeElement(Enumeration<DeviceNameType> value) { 1616 this.type = value; 1617 return this; 1618 } 1619 1620 /** 1621 * @return The type of deviceName. 1622RegisteredName | UserFriendlyName | PatientReportedName. 1623 */ 1624 public DeviceNameType getType() { 1625 return this.type == null ? null : this.type.getValue(); 1626 } 1627 1628 /** 1629 * @param value The type of deviceName. 1630RegisteredName | UserFriendlyName | PatientReportedName. 1631 */ 1632 public DeviceDefinitionDeviceNameComponent setType(DeviceNameType value) { 1633 if (this.type == null) 1634 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); 1635 this.type.setValue(value); 1636 return this; 1637 } 1638 1639 protected void listChildren(List<Property> children) { 1640 super.listChildren(children); 1641 children.add(new Property("name", "string", "A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other.", 0, 1, name)); 1642 children.add(new Property("type", "code", "The type of deviceName.\nRegisteredName | UserFriendlyName | PatientReportedName.", 0, 1, type)); 1643 } 1644 1645 @Override 1646 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1647 switch (_hash) { 1648 case 3373707: /*name*/ return new Property("name", "string", "A human-friendly name that is used to refer to the device - depending on the type, it can be the brand name, the common name or alias, or other.", 0, 1, name); 1649 case 3575610: /*type*/ return new Property("type", "code", "The type of deviceName.\nRegisteredName | UserFriendlyName | PatientReportedName.", 0, 1, type); 1650 default: return super.getNamedProperty(_hash, _name, _checkValid); 1651 } 1652 1653 } 1654 1655 @Override 1656 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1657 switch (hash) { 1658 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1659 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DeviceNameType> 1660 default: return super.getProperty(hash, name, checkValid); 1661 } 1662 1663 } 1664 1665 @Override 1666 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1667 switch (hash) { 1668 case 3373707: // name 1669 this.name = TypeConvertor.castToString(value); // StringType 1670 return value; 1671 case 3575610: // type 1672 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1673 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1674 return value; 1675 default: return super.setProperty(hash, name, value); 1676 } 1677 1678 } 1679 1680 @Override 1681 public Base setProperty(String name, Base value) throws FHIRException { 1682 if (name.equals("name")) { 1683 this.name = TypeConvertor.castToString(value); // StringType 1684 } else if (name.equals("type")) { 1685 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1686 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1687 } else 1688 return super.setProperty(name, value); 1689 return value; 1690 } 1691 1692 @Override 1693 public void removeChild(String name, Base value) throws FHIRException { 1694 if (name.equals("name")) { 1695 this.name = null; 1696 } else if (name.equals("type")) { 1697 value = new DeviceNameTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1698 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 1699 } else 1700 super.removeChild(name, value); 1701 1702 } 1703 1704 @Override 1705 public Base makeProperty(int hash, String name) throws FHIRException { 1706 switch (hash) { 1707 case 3373707: return getNameElement(); 1708 case 3575610: return getTypeElement(); 1709 default: return super.makeProperty(hash, name); 1710 } 1711 1712 } 1713 1714 @Override 1715 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1716 switch (hash) { 1717 case 3373707: /*name*/ return new String[] {"string"}; 1718 case 3575610: /*type*/ return new String[] {"code"}; 1719 default: return super.getTypesForProperty(hash, name); 1720 } 1721 1722 } 1723 1724 @Override 1725 public Base addChild(String name) throws FHIRException { 1726 if (name.equals("name")) { 1727 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.deviceName.name"); 1728 } 1729 else if (name.equals("type")) { 1730 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.deviceName.type"); 1731 } 1732 else 1733 return super.addChild(name); 1734 } 1735 1736 public DeviceDefinitionDeviceNameComponent copy() { 1737 DeviceDefinitionDeviceNameComponent dst = new DeviceDefinitionDeviceNameComponent(); 1738 copyValues(dst); 1739 return dst; 1740 } 1741 1742 public void copyValues(DeviceDefinitionDeviceNameComponent dst) { 1743 super.copyValues(dst); 1744 dst.name = name == null ? null : name.copy(); 1745 dst.type = type == null ? null : type.copy(); 1746 } 1747 1748 @Override 1749 public boolean equalsDeep(Base other_) { 1750 if (!super.equalsDeep(other_)) 1751 return false; 1752 if (!(other_ instanceof DeviceDefinitionDeviceNameComponent)) 1753 return false; 1754 DeviceDefinitionDeviceNameComponent o = (DeviceDefinitionDeviceNameComponent) other_; 1755 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true); 1756 } 1757 1758 @Override 1759 public boolean equalsShallow(Base other_) { 1760 if (!super.equalsShallow(other_)) 1761 return false; 1762 if (!(other_ instanceof DeviceDefinitionDeviceNameComponent)) 1763 return false; 1764 DeviceDefinitionDeviceNameComponent o = (DeviceDefinitionDeviceNameComponent) other_; 1765 return compareValues(name, o.name, true) && compareValues(type, o.type, true); 1766 } 1767 1768 public boolean isEmpty() { 1769 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type); 1770 } 1771 1772 public String fhirType() { 1773 return "DeviceDefinition.deviceName"; 1774 1775 } 1776 1777 } 1778 1779 @Block() 1780 public static class DeviceDefinitionClassificationComponent extends BackboneElement implements IBaseBackboneElement { 1781 /** 1782 * A classification or risk class of the device model. 1783 */ 1784 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1785 @Description(shortDefinition="A classification or risk class of the device model", formalDefinition="A classification or risk class of the device model." ) 1786 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-type") 1787 protected CodeableConcept type; 1788 1789 /** 1790 * Further information qualifying this classification of the device model. 1791 */ 1792 @Child(name = "justification", type = {RelatedArtifact.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1793 @Description(shortDefinition="Further information qualifying this classification of the device model", formalDefinition="Further information qualifying this classification of the device model." ) 1794 protected List<RelatedArtifact> justification; 1795 1796 private static final long serialVersionUID = -1343788026L; 1797 1798 /** 1799 * Constructor 1800 */ 1801 public DeviceDefinitionClassificationComponent() { 1802 super(); 1803 } 1804 1805 /** 1806 * Constructor 1807 */ 1808 public DeviceDefinitionClassificationComponent(CodeableConcept type) { 1809 super(); 1810 this.setType(type); 1811 } 1812 1813 /** 1814 * @return {@link #type} (A classification or risk class of the device model.) 1815 */ 1816 public CodeableConcept getType() { 1817 if (this.type == null) 1818 if (Configuration.errorOnAutoCreate()) 1819 throw new Error("Attempt to auto-create DeviceDefinitionClassificationComponent.type"); 1820 else if (Configuration.doAutoCreate()) 1821 this.type = new CodeableConcept(); // cc 1822 return this.type; 1823 } 1824 1825 public boolean hasType() { 1826 return this.type != null && !this.type.isEmpty(); 1827 } 1828 1829 /** 1830 * @param value {@link #type} (A classification or risk class of the device model.) 1831 */ 1832 public DeviceDefinitionClassificationComponent setType(CodeableConcept value) { 1833 this.type = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return {@link #justification} (Further information qualifying this classification of the device model.) 1839 */ 1840 public List<RelatedArtifact> getJustification() { 1841 if (this.justification == null) 1842 this.justification = new ArrayList<RelatedArtifact>(); 1843 return this.justification; 1844 } 1845 1846 /** 1847 * @return Returns a reference to <code>this</code> for easy method chaining 1848 */ 1849 public DeviceDefinitionClassificationComponent setJustification(List<RelatedArtifact> theJustification) { 1850 this.justification = theJustification; 1851 return this; 1852 } 1853 1854 public boolean hasJustification() { 1855 if (this.justification == null) 1856 return false; 1857 for (RelatedArtifact item : this.justification) 1858 if (!item.isEmpty()) 1859 return true; 1860 return false; 1861 } 1862 1863 public RelatedArtifact addJustification() { //3 1864 RelatedArtifact t = new RelatedArtifact(); 1865 if (this.justification == null) 1866 this.justification = new ArrayList<RelatedArtifact>(); 1867 this.justification.add(t); 1868 return t; 1869 } 1870 1871 public DeviceDefinitionClassificationComponent addJustification(RelatedArtifact t) { //3 1872 if (t == null) 1873 return this; 1874 if (this.justification == null) 1875 this.justification = new ArrayList<RelatedArtifact>(); 1876 this.justification.add(t); 1877 return this; 1878 } 1879 1880 /** 1881 * @return The first repetition of repeating field {@link #justification}, creating it if it does not already exist {3} 1882 */ 1883 public RelatedArtifact getJustificationFirstRep() { 1884 if (getJustification().isEmpty()) { 1885 addJustification(); 1886 } 1887 return getJustification().get(0); 1888 } 1889 1890 protected void listChildren(List<Property> children) { 1891 super.listChildren(children); 1892 children.add(new Property("type", "CodeableConcept", "A classification or risk class of the device model.", 0, 1, type)); 1893 children.add(new Property("justification", "RelatedArtifact", "Further information qualifying this classification of the device model.", 0, java.lang.Integer.MAX_VALUE, justification)); 1894 } 1895 1896 @Override 1897 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1898 switch (_hash) { 1899 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A classification or risk class of the device model.", 0, 1, type); 1900 case 1864993522: /*justification*/ return new Property("justification", "RelatedArtifact", "Further information qualifying this classification of the device model.", 0, java.lang.Integer.MAX_VALUE, justification); 1901 default: return super.getNamedProperty(_hash, _name, _checkValid); 1902 } 1903 1904 } 1905 1906 @Override 1907 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1908 switch (hash) { 1909 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1910 case 1864993522: /*justification*/ return this.justification == null ? new Base[0] : this.justification.toArray(new Base[this.justification.size()]); // RelatedArtifact 1911 default: return super.getProperty(hash, name, checkValid); 1912 } 1913 1914 } 1915 1916 @Override 1917 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1918 switch (hash) { 1919 case 3575610: // type 1920 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1921 return value; 1922 case 1864993522: // justification 1923 this.getJustification().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 1924 return value; 1925 default: return super.setProperty(hash, name, value); 1926 } 1927 1928 } 1929 1930 @Override 1931 public Base setProperty(String name, Base value) throws FHIRException { 1932 if (name.equals("type")) { 1933 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1934 } else if (name.equals("justification")) { 1935 this.getJustification().add(TypeConvertor.castToRelatedArtifact(value)); 1936 } else 1937 return super.setProperty(name, value); 1938 return value; 1939 } 1940 1941 @Override 1942 public void removeChild(String name, Base value) throws FHIRException { 1943 if (name.equals("type")) { 1944 this.type = null; 1945 } else if (name.equals("justification")) { 1946 this.getJustification().remove(value); 1947 } else 1948 super.removeChild(name, value); 1949 1950 } 1951 1952 @Override 1953 public Base makeProperty(int hash, String name) throws FHIRException { 1954 switch (hash) { 1955 case 3575610: return getType(); 1956 case 1864993522: return addJustification(); 1957 default: return super.makeProperty(hash, name); 1958 } 1959 1960 } 1961 1962 @Override 1963 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1964 switch (hash) { 1965 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1966 case 1864993522: /*justification*/ return new String[] {"RelatedArtifact"}; 1967 default: return super.getTypesForProperty(hash, name); 1968 } 1969 1970 } 1971 1972 @Override 1973 public Base addChild(String name) throws FHIRException { 1974 if (name.equals("type")) { 1975 this.type = new CodeableConcept(); 1976 return this.type; 1977 } 1978 else if (name.equals("justification")) { 1979 return addJustification(); 1980 } 1981 else 1982 return super.addChild(name); 1983 } 1984 1985 public DeviceDefinitionClassificationComponent copy() { 1986 DeviceDefinitionClassificationComponent dst = new DeviceDefinitionClassificationComponent(); 1987 copyValues(dst); 1988 return dst; 1989 } 1990 1991 public void copyValues(DeviceDefinitionClassificationComponent dst) { 1992 super.copyValues(dst); 1993 dst.type = type == null ? null : type.copy(); 1994 if (justification != null) { 1995 dst.justification = new ArrayList<RelatedArtifact>(); 1996 for (RelatedArtifact i : justification) 1997 dst.justification.add(i.copy()); 1998 }; 1999 } 2000 2001 @Override 2002 public boolean equalsDeep(Base other_) { 2003 if (!super.equalsDeep(other_)) 2004 return false; 2005 if (!(other_ instanceof DeviceDefinitionClassificationComponent)) 2006 return false; 2007 DeviceDefinitionClassificationComponent o = (DeviceDefinitionClassificationComponent) other_; 2008 return compareDeep(type, o.type, true) && compareDeep(justification, o.justification, true); 2009 } 2010 2011 @Override 2012 public boolean equalsShallow(Base other_) { 2013 if (!super.equalsShallow(other_)) 2014 return false; 2015 if (!(other_ instanceof DeviceDefinitionClassificationComponent)) 2016 return false; 2017 DeviceDefinitionClassificationComponent o = (DeviceDefinitionClassificationComponent) other_; 2018 return true; 2019 } 2020 2021 public boolean isEmpty() { 2022 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, justification); 2023 } 2024 2025 public String fhirType() { 2026 return "DeviceDefinition.classification"; 2027 2028 } 2029 2030 } 2031 2032 @Block() 2033 public static class DeviceDefinitionConformsToComponent extends BackboneElement implements IBaseBackboneElement { 2034 /** 2035 * Describes the type of the standard, specification, or formal guidance. 2036 */ 2037 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 2038 @Description(shortDefinition="Describes the common type of the standard, specification, or formal guidance", formalDefinition="Describes the type of the standard, specification, or formal guidance." ) 2039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-specification-category") 2040 protected CodeableConcept category; 2041 2042 /** 2043 * Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres. 2044 */ 2045 @Child(name = "specification", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 2046 @Description(shortDefinition="Identifies the standard, specification, or formal guidance that the device adheres to the Device Specification type", formalDefinition="Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres." ) 2047 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-specification-type") 2048 protected CodeableConcept specification; 2049 2050 /** 2051 * Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label. 2052 */ 2053 @Child(name = "version", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2054 @Description(shortDefinition="The specific form or variant of the standard, specification or formal guidance", formalDefinition="Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label." ) 2055 protected List<StringType> version; 2056 2057 /** 2058 * Standard, regulation, certification, or guidance website, document, or other publication, or similar, supporting the conformance. 2059 */ 2060 @Child(name = "source", type = {RelatedArtifact.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2061 @Description(shortDefinition="Standard, regulation, certification, or guidance website, document, or other publication, or similar, supporting the conformance", formalDefinition="Standard, regulation, certification, or guidance website, document, or other publication, or similar, supporting the conformance." ) 2062 protected List<RelatedArtifact> source; 2063 2064 private static final long serialVersionUID = -370906560L; 2065 2066 /** 2067 * Constructor 2068 */ 2069 public DeviceDefinitionConformsToComponent() { 2070 super(); 2071 } 2072 2073 /** 2074 * Constructor 2075 */ 2076 public DeviceDefinitionConformsToComponent(CodeableConcept specification) { 2077 super(); 2078 this.setSpecification(specification); 2079 } 2080 2081 /** 2082 * @return {@link #category} (Describes the type of the standard, specification, or formal guidance.) 2083 */ 2084 public CodeableConcept getCategory() { 2085 if (this.category == null) 2086 if (Configuration.errorOnAutoCreate()) 2087 throw new Error("Attempt to auto-create DeviceDefinitionConformsToComponent.category"); 2088 else if (Configuration.doAutoCreate()) 2089 this.category = new CodeableConcept(); // cc 2090 return this.category; 2091 } 2092 2093 public boolean hasCategory() { 2094 return this.category != null && !this.category.isEmpty(); 2095 } 2096 2097 /** 2098 * @param value {@link #category} (Describes the type of the standard, specification, or formal guidance.) 2099 */ 2100 public DeviceDefinitionConformsToComponent setCategory(CodeableConcept value) { 2101 this.category = value; 2102 return this; 2103 } 2104 2105 /** 2106 * @return {@link #specification} (Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.) 2107 */ 2108 public CodeableConcept getSpecification() { 2109 if (this.specification == null) 2110 if (Configuration.errorOnAutoCreate()) 2111 throw new Error("Attempt to auto-create DeviceDefinitionConformsToComponent.specification"); 2112 else if (Configuration.doAutoCreate()) 2113 this.specification = new CodeableConcept(); // cc 2114 return this.specification; 2115 } 2116 2117 public boolean hasSpecification() { 2118 return this.specification != null && !this.specification.isEmpty(); 2119 } 2120 2121 /** 2122 * @param value {@link #specification} (Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.) 2123 */ 2124 public DeviceDefinitionConformsToComponent setSpecification(CodeableConcept value) { 2125 this.specification = value; 2126 return this; 2127 } 2128 2129 /** 2130 * @return {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.) 2131 */ 2132 public List<StringType> getVersion() { 2133 if (this.version == null) 2134 this.version = new ArrayList<StringType>(); 2135 return this.version; 2136 } 2137 2138 /** 2139 * @return Returns a reference to <code>this</code> for easy method chaining 2140 */ 2141 public DeviceDefinitionConformsToComponent setVersion(List<StringType> theVersion) { 2142 this.version = theVersion; 2143 return this; 2144 } 2145 2146 public boolean hasVersion() { 2147 if (this.version == null) 2148 return false; 2149 for (StringType item : this.version) 2150 if (!item.isEmpty()) 2151 return true; 2152 return false; 2153 } 2154 2155 /** 2156 * @return {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.) 2157 */ 2158 public StringType addVersionElement() {//2 2159 StringType t = new StringType(); 2160 if (this.version == null) 2161 this.version = new ArrayList<StringType>(); 2162 this.version.add(t); 2163 return t; 2164 } 2165 2166 /** 2167 * @param value {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.) 2168 */ 2169 public DeviceDefinitionConformsToComponent addVersion(String value) { //1 2170 StringType t = new StringType(); 2171 t.setValue(value); 2172 if (this.version == null) 2173 this.version = new ArrayList<StringType>(); 2174 this.version.add(t); 2175 return this; 2176 } 2177 2178 /** 2179 * @param value {@link #version} (Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.) 2180 */ 2181 public boolean hasVersion(String value) { 2182 if (this.version == null) 2183 return false; 2184 for (StringType v : this.version) 2185 if (v.getValue().equals(value)) // string 2186 return true; 2187 return false; 2188 } 2189 2190 /** 2191 * @return {@link #source} (Standard, regulation, certification, or guidance website, document, or other publication, or similar, supporting the conformance.) 2192 */ 2193 public List<RelatedArtifact> getSource() { 2194 if (this.source == null) 2195 this.source = new ArrayList<RelatedArtifact>(); 2196 return this.source; 2197 } 2198 2199 /** 2200 * @return Returns a reference to <code>this</code> for easy method chaining 2201 */ 2202 public DeviceDefinitionConformsToComponent setSource(List<RelatedArtifact> theSource) { 2203 this.source = theSource; 2204 return this; 2205 } 2206 2207 public boolean hasSource() { 2208 if (this.source == null) 2209 return false; 2210 for (RelatedArtifact item : this.source) 2211 if (!item.isEmpty()) 2212 return true; 2213 return false; 2214 } 2215 2216 public RelatedArtifact addSource() { //3 2217 RelatedArtifact t = new RelatedArtifact(); 2218 if (this.source == null) 2219 this.source = new ArrayList<RelatedArtifact>(); 2220 this.source.add(t); 2221 return t; 2222 } 2223 2224 public DeviceDefinitionConformsToComponent addSource(RelatedArtifact t) { //3 2225 if (t == null) 2226 return this; 2227 if (this.source == null) 2228 this.source = new ArrayList<RelatedArtifact>(); 2229 this.source.add(t); 2230 return this; 2231 } 2232 2233 /** 2234 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 2235 */ 2236 public RelatedArtifact getSourceFirstRep() { 2237 if (getSource().isEmpty()) { 2238 addSource(); 2239 } 2240 return getSource().get(0); 2241 } 2242 2243 protected void listChildren(List<Property> children) { 2244 super.listChildren(children); 2245 children.add(new Property("category", "CodeableConcept", "Describes the type of the standard, specification, or formal guidance.", 0, 1, category)); 2246 children.add(new Property("specification", "CodeableConcept", "Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.", 0, 1, specification)); 2247 children.add(new Property("version", "string", "Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.", 0, java.lang.Integer.MAX_VALUE, version)); 2248 children.add(new Property("source", "RelatedArtifact", "Standard, regulation, certification, or guidance website, document, or other publication, or similar, supporting the conformance.", 0, java.lang.Integer.MAX_VALUE, source)); 2249 } 2250 2251 @Override 2252 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2253 switch (_hash) { 2254 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Describes the type of the standard, specification, or formal guidance.", 0, 1, category); 2255 case 1307197699: /*specification*/ return new Property("specification", "CodeableConcept", "Code that identifies the specific standard, specification, protocol, formal guidance, regulation, legislation, or certification scheme to which the device adheres.", 0, 1, specification); 2256 case 351608024: /*version*/ return new Property("version", "string", "Identifies the specific form or variant of the standard, specification, or formal guidance. This may be a 'version number', release, document edition, publication year, or other label.", 0, java.lang.Integer.MAX_VALUE, version); 2257 case -896505829: /*source*/ return new Property("source", "RelatedArtifact", "Standard, regulation, certification, or guidance website, document, or other publication, or similar, supporting the conformance.", 0, java.lang.Integer.MAX_VALUE, source); 2258 default: return super.getNamedProperty(_hash, _name, _checkValid); 2259 } 2260 2261 } 2262 2263 @Override 2264 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2265 switch (hash) { 2266 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2267 case 1307197699: /*specification*/ return this.specification == null ? new Base[0] : new Base[] {this.specification}; // CodeableConcept 2268 case 351608024: /*version*/ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // StringType 2269 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // RelatedArtifact 2270 default: return super.getProperty(hash, name, checkValid); 2271 } 2272 2273 } 2274 2275 @Override 2276 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2277 switch (hash) { 2278 case 50511102: // category 2279 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2280 return value; 2281 case 1307197699: // specification 2282 this.specification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2283 return value; 2284 case 351608024: // version 2285 this.getVersion().add(TypeConvertor.castToString(value)); // StringType 2286 return value; 2287 case -896505829: // source 2288 this.getSource().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 2289 return value; 2290 default: return super.setProperty(hash, name, value); 2291 } 2292 2293 } 2294 2295 @Override 2296 public Base setProperty(String name, Base value) throws FHIRException { 2297 if (name.equals("category")) { 2298 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2299 } else if (name.equals("specification")) { 2300 this.specification = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2301 } else if (name.equals("version")) { 2302 this.getVersion().add(TypeConvertor.castToString(value)); 2303 } else if (name.equals("source")) { 2304 this.getSource().add(TypeConvertor.castToRelatedArtifact(value)); 2305 } else 2306 return super.setProperty(name, value); 2307 return value; 2308 } 2309 2310 @Override 2311 public void removeChild(String name, Base value) throws FHIRException { 2312 if (name.equals("category")) { 2313 this.category = null; 2314 } else if (name.equals("specification")) { 2315 this.specification = null; 2316 } else if (name.equals("version")) { 2317 this.getVersion().remove(value); 2318 } else if (name.equals("source")) { 2319 this.getSource().remove(value); 2320 } else 2321 super.removeChild(name, value); 2322 2323 } 2324 2325 @Override 2326 public Base makeProperty(int hash, String name) throws FHIRException { 2327 switch (hash) { 2328 case 50511102: return getCategory(); 2329 case 1307197699: return getSpecification(); 2330 case 351608024: return addVersionElement(); 2331 case -896505829: return addSource(); 2332 default: return super.makeProperty(hash, name); 2333 } 2334 2335 } 2336 2337 @Override 2338 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2339 switch (hash) { 2340 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2341 case 1307197699: /*specification*/ return new String[] {"CodeableConcept"}; 2342 case 351608024: /*version*/ return new String[] {"string"}; 2343 case -896505829: /*source*/ return new String[] {"RelatedArtifact"}; 2344 default: return super.getTypesForProperty(hash, name); 2345 } 2346 2347 } 2348 2349 @Override 2350 public Base addChild(String name) throws FHIRException { 2351 if (name.equals("category")) { 2352 this.category = new CodeableConcept(); 2353 return this.category; 2354 } 2355 else if (name.equals("specification")) { 2356 this.specification = new CodeableConcept(); 2357 return this.specification; 2358 } 2359 else if (name.equals("version")) { 2360 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.conformsTo.version"); 2361 } 2362 else if (name.equals("source")) { 2363 return addSource(); 2364 } 2365 else 2366 return super.addChild(name); 2367 } 2368 2369 public DeviceDefinitionConformsToComponent copy() { 2370 DeviceDefinitionConformsToComponent dst = new DeviceDefinitionConformsToComponent(); 2371 copyValues(dst); 2372 return dst; 2373 } 2374 2375 public void copyValues(DeviceDefinitionConformsToComponent dst) { 2376 super.copyValues(dst); 2377 dst.category = category == null ? null : category.copy(); 2378 dst.specification = specification == null ? null : specification.copy(); 2379 if (version != null) { 2380 dst.version = new ArrayList<StringType>(); 2381 for (StringType i : version) 2382 dst.version.add(i.copy()); 2383 }; 2384 if (source != null) { 2385 dst.source = new ArrayList<RelatedArtifact>(); 2386 for (RelatedArtifact i : source) 2387 dst.source.add(i.copy()); 2388 }; 2389 } 2390 2391 @Override 2392 public boolean equalsDeep(Base other_) { 2393 if (!super.equalsDeep(other_)) 2394 return false; 2395 if (!(other_ instanceof DeviceDefinitionConformsToComponent)) 2396 return false; 2397 DeviceDefinitionConformsToComponent o = (DeviceDefinitionConformsToComponent) other_; 2398 return compareDeep(category, o.category, true) && compareDeep(specification, o.specification, true) 2399 && compareDeep(version, o.version, true) && compareDeep(source, o.source, true); 2400 } 2401 2402 @Override 2403 public boolean equalsShallow(Base other_) { 2404 if (!super.equalsShallow(other_)) 2405 return false; 2406 if (!(other_ instanceof DeviceDefinitionConformsToComponent)) 2407 return false; 2408 DeviceDefinitionConformsToComponent o = (DeviceDefinitionConformsToComponent) other_; 2409 return compareValues(version, o.version, true); 2410 } 2411 2412 public boolean isEmpty() { 2413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, specification, version 2414 , source); 2415 } 2416 2417 public String fhirType() { 2418 return "DeviceDefinition.conformsTo"; 2419 2420 } 2421 2422 } 2423 2424 @Block() 2425 public static class DeviceDefinitionHasPartComponent extends BackboneElement implements IBaseBackboneElement { 2426 /** 2427 * Reference to the device that is part of the current device. 2428 */ 2429 @Child(name = "reference", type = {DeviceDefinition.class}, order=1, min=1, max=1, modifier=false, summary=true) 2430 @Description(shortDefinition="Reference to the part", formalDefinition="Reference to the device that is part of the current device." ) 2431 protected Reference reference; 2432 2433 /** 2434 * Number of instances of the component device in the current device. 2435 */ 2436 @Child(name = "count", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2437 @Description(shortDefinition="Number of occurrences of the part", formalDefinition="Number of instances of the component device in the current device." ) 2438 protected IntegerType count; 2439 2440 private static final long serialVersionUID = -1166127369L; 2441 2442 /** 2443 * Constructor 2444 */ 2445 public DeviceDefinitionHasPartComponent() { 2446 super(); 2447 } 2448 2449 /** 2450 * Constructor 2451 */ 2452 public DeviceDefinitionHasPartComponent(Reference reference) { 2453 super(); 2454 this.setReference(reference); 2455 } 2456 2457 /** 2458 * @return {@link #reference} (Reference to the device that is part of the current device.) 2459 */ 2460 public Reference getReference() { 2461 if (this.reference == null) 2462 if (Configuration.errorOnAutoCreate()) 2463 throw new Error("Attempt to auto-create DeviceDefinitionHasPartComponent.reference"); 2464 else if (Configuration.doAutoCreate()) 2465 this.reference = new Reference(); // cc 2466 return this.reference; 2467 } 2468 2469 public boolean hasReference() { 2470 return this.reference != null && !this.reference.isEmpty(); 2471 } 2472 2473 /** 2474 * @param value {@link #reference} (Reference to the device that is part of the current device.) 2475 */ 2476 public DeviceDefinitionHasPartComponent setReference(Reference value) { 2477 this.reference = value; 2478 return this; 2479 } 2480 2481 /** 2482 * @return {@link #count} (Number of instances of the component device in the current device.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 2483 */ 2484 public IntegerType getCountElement() { 2485 if (this.count == null) 2486 if (Configuration.errorOnAutoCreate()) 2487 throw new Error("Attempt to auto-create DeviceDefinitionHasPartComponent.count"); 2488 else if (Configuration.doAutoCreate()) 2489 this.count = new IntegerType(); // bb 2490 return this.count; 2491 } 2492 2493 public boolean hasCountElement() { 2494 return this.count != null && !this.count.isEmpty(); 2495 } 2496 2497 public boolean hasCount() { 2498 return this.count != null && !this.count.isEmpty(); 2499 } 2500 2501 /** 2502 * @param value {@link #count} (Number of instances of the component device in the current device.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 2503 */ 2504 public DeviceDefinitionHasPartComponent setCountElement(IntegerType value) { 2505 this.count = value; 2506 return this; 2507 } 2508 2509 /** 2510 * @return Number of instances of the component device in the current device. 2511 */ 2512 public int getCount() { 2513 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 2514 } 2515 2516 /** 2517 * @param value Number of instances of the component device in the current device. 2518 */ 2519 public DeviceDefinitionHasPartComponent setCount(int value) { 2520 if (this.count == null) 2521 this.count = new IntegerType(); 2522 this.count.setValue(value); 2523 return this; 2524 } 2525 2526 protected void listChildren(List<Property> children) { 2527 super.listChildren(children); 2528 children.add(new Property("reference", "Reference(DeviceDefinition)", "Reference to the device that is part of the current device.", 0, 1, reference)); 2529 children.add(new Property("count", "integer", "Number of instances of the component device in the current device.", 0, 1, count)); 2530 } 2531 2532 @Override 2533 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2534 switch (_hash) { 2535 case -925155509: /*reference*/ return new Property("reference", "Reference(DeviceDefinition)", "Reference to the device that is part of the current device.", 0, 1, reference); 2536 case 94851343: /*count*/ return new Property("count", "integer", "Number of instances of the component device in the current device.", 0, 1, count); 2537 default: return super.getNamedProperty(_hash, _name, _checkValid); 2538 } 2539 2540 } 2541 2542 @Override 2543 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2544 switch (hash) { 2545 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2546 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // IntegerType 2547 default: return super.getProperty(hash, name, checkValid); 2548 } 2549 2550 } 2551 2552 @Override 2553 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2554 switch (hash) { 2555 case -925155509: // reference 2556 this.reference = TypeConvertor.castToReference(value); // Reference 2557 return value; 2558 case 94851343: // count 2559 this.count = TypeConvertor.castToInteger(value); // IntegerType 2560 return value; 2561 default: return super.setProperty(hash, name, value); 2562 } 2563 2564 } 2565 2566 @Override 2567 public Base setProperty(String name, Base value) throws FHIRException { 2568 if (name.equals("reference")) { 2569 this.reference = TypeConvertor.castToReference(value); // Reference 2570 } else if (name.equals("count")) { 2571 this.count = TypeConvertor.castToInteger(value); // IntegerType 2572 } else 2573 return super.setProperty(name, value); 2574 return value; 2575 } 2576 2577 @Override 2578 public void removeChild(String name, Base value) throws FHIRException { 2579 if (name.equals("reference")) { 2580 this.reference = null; 2581 } else if (name.equals("count")) { 2582 this.count = null; 2583 } else 2584 super.removeChild(name, value); 2585 2586 } 2587 2588 @Override 2589 public Base makeProperty(int hash, String name) throws FHIRException { 2590 switch (hash) { 2591 case -925155509: return getReference(); 2592 case 94851343: return getCountElement(); 2593 default: return super.makeProperty(hash, name); 2594 } 2595 2596 } 2597 2598 @Override 2599 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2600 switch (hash) { 2601 case -925155509: /*reference*/ return new String[] {"Reference"}; 2602 case 94851343: /*count*/ return new String[] {"integer"}; 2603 default: return super.getTypesForProperty(hash, name); 2604 } 2605 2606 } 2607 2608 @Override 2609 public Base addChild(String name) throws FHIRException { 2610 if (name.equals("reference")) { 2611 this.reference = new Reference(); 2612 return this.reference; 2613 } 2614 else if (name.equals("count")) { 2615 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.hasPart.count"); 2616 } 2617 else 2618 return super.addChild(name); 2619 } 2620 2621 public DeviceDefinitionHasPartComponent copy() { 2622 DeviceDefinitionHasPartComponent dst = new DeviceDefinitionHasPartComponent(); 2623 copyValues(dst); 2624 return dst; 2625 } 2626 2627 public void copyValues(DeviceDefinitionHasPartComponent dst) { 2628 super.copyValues(dst); 2629 dst.reference = reference == null ? null : reference.copy(); 2630 dst.count = count == null ? null : count.copy(); 2631 } 2632 2633 @Override 2634 public boolean equalsDeep(Base other_) { 2635 if (!super.equalsDeep(other_)) 2636 return false; 2637 if (!(other_ instanceof DeviceDefinitionHasPartComponent)) 2638 return false; 2639 DeviceDefinitionHasPartComponent o = (DeviceDefinitionHasPartComponent) other_; 2640 return compareDeep(reference, o.reference, true) && compareDeep(count, o.count, true); 2641 } 2642 2643 @Override 2644 public boolean equalsShallow(Base other_) { 2645 if (!super.equalsShallow(other_)) 2646 return false; 2647 if (!(other_ instanceof DeviceDefinitionHasPartComponent)) 2648 return false; 2649 DeviceDefinitionHasPartComponent o = (DeviceDefinitionHasPartComponent) other_; 2650 return compareValues(count, o.count, true); 2651 } 2652 2653 public boolean isEmpty() { 2654 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, count); 2655 } 2656 2657 public String fhirType() { 2658 return "DeviceDefinition.hasPart"; 2659 2660 } 2661 2662 } 2663 2664 @Block() 2665 public static class DeviceDefinitionPackagingComponent extends BackboneElement implements IBaseBackboneElement { 2666 /** 2667 * The business identifier of the packaged medication. 2668 */ 2669 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 2670 @Description(shortDefinition="Business identifier of the packaged medication", formalDefinition="The business identifier of the packaged medication." ) 2671 protected Identifier identifier; 2672 2673 /** 2674 * A code that defines the specific type of packaging. 2675 */ 2676 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2677 @Description(shortDefinition="A code that defines the specific type of packaging", formalDefinition="A code that defines the specific type of packaging." ) 2678 protected CodeableConcept type; 2679 2680 /** 2681 * The number of items contained in the package (devices or sub-packages). 2682 */ 2683 @Child(name = "count", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2684 @Description(shortDefinition="The number of items contained in the package (devices or sub-packages)", formalDefinition="The number of items contained in the package (devices or sub-packages)." ) 2685 protected IntegerType count; 2686 2687 /** 2688 * An organization that distributes the packaged device. 2689 */ 2690 @Child(name = "distributor", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2691 @Description(shortDefinition="An organization that distributes the packaged device", formalDefinition="An organization that distributes the packaged device." ) 2692 protected List<PackagingDistributorComponent> distributor; 2693 2694 /** 2695 * Unique Device Identifier (UDI) Barcode string on the packaging. 2696 */ 2697 @Child(name = "udiDeviceIdentifier", type = {DeviceDefinitionUdiDeviceIdentifierComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2698 @Description(shortDefinition="Unique Device Identifier (UDI) Barcode string on the packaging", formalDefinition="Unique Device Identifier (UDI) Barcode string on the packaging." ) 2699 protected List<DeviceDefinitionUdiDeviceIdentifierComponent> udiDeviceIdentifier; 2700 2701 /** 2702 * Allows packages within packages. 2703 */ 2704 @Child(name = "packaging", type = {DeviceDefinitionPackagingComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2705 @Description(shortDefinition="Allows packages within packages", formalDefinition="Allows packages within packages." ) 2706 protected List<DeviceDefinitionPackagingComponent> packaging; 2707 2708 private static final long serialVersionUID = 1022373074L; 2709 2710 /** 2711 * Constructor 2712 */ 2713 public DeviceDefinitionPackagingComponent() { 2714 super(); 2715 } 2716 2717 /** 2718 * @return {@link #identifier} (The business identifier of the packaged medication.) 2719 */ 2720 public Identifier getIdentifier() { 2721 if (this.identifier == null) 2722 if (Configuration.errorOnAutoCreate()) 2723 throw new Error("Attempt to auto-create DeviceDefinitionPackagingComponent.identifier"); 2724 else if (Configuration.doAutoCreate()) 2725 this.identifier = new Identifier(); // cc 2726 return this.identifier; 2727 } 2728 2729 public boolean hasIdentifier() { 2730 return this.identifier != null && !this.identifier.isEmpty(); 2731 } 2732 2733 /** 2734 * @param value {@link #identifier} (The business identifier of the packaged medication.) 2735 */ 2736 public DeviceDefinitionPackagingComponent setIdentifier(Identifier value) { 2737 this.identifier = value; 2738 return this; 2739 } 2740 2741 /** 2742 * @return {@link #type} (A code that defines the specific type of packaging.) 2743 */ 2744 public CodeableConcept getType() { 2745 if (this.type == null) 2746 if (Configuration.errorOnAutoCreate()) 2747 throw new Error("Attempt to auto-create DeviceDefinitionPackagingComponent.type"); 2748 else if (Configuration.doAutoCreate()) 2749 this.type = new CodeableConcept(); // cc 2750 return this.type; 2751 } 2752 2753 public boolean hasType() { 2754 return this.type != null && !this.type.isEmpty(); 2755 } 2756 2757 /** 2758 * @param value {@link #type} (A code that defines the specific type of packaging.) 2759 */ 2760 public DeviceDefinitionPackagingComponent setType(CodeableConcept value) { 2761 this.type = value; 2762 return this; 2763 } 2764 2765 /** 2766 * @return {@link #count} (The number of items contained in the package (devices or sub-packages).). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 2767 */ 2768 public IntegerType getCountElement() { 2769 if (this.count == null) 2770 if (Configuration.errorOnAutoCreate()) 2771 throw new Error("Attempt to auto-create DeviceDefinitionPackagingComponent.count"); 2772 else if (Configuration.doAutoCreate()) 2773 this.count = new IntegerType(); // bb 2774 return this.count; 2775 } 2776 2777 public boolean hasCountElement() { 2778 return this.count != null && !this.count.isEmpty(); 2779 } 2780 2781 public boolean hasCount() { 2782 return this.count != null && !this.count.isEmpty(); 2783 } 2784 2785 /** 2786 * @param value {@link #count} (The number of items contained in the package (devices or sub-packages).). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 2787 */ 2788 public DeviceDefinitionPackagingComponent setCountElement(IntegerType value) { 2789 this.count = value; 2790 return this; 2791 } 2792 2793 /** 2794 * @return The number of items contained in the package (devices or sub-packages). 2795 */ 2796 public int getCount() { 2797 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 2798 } 2799 2800 /** 2801 * @param value The number of items contained in the package (devices or sub-packages). 2802 */ 2803 public DeviceDefinitionPackagingComponent setCount(int value) { 2804 if (this.count == null) 2805 this.count = new IntegerType(); 2806 this.count.setValue(value); 2807 return this; 2808 } 2809 2810 /** 2811 * @return {@link #distributor} (An organization that distributes the packaged device.) 2812 */ 2813 public List<PackagingDistributorComponent> getDistributor() { 2814 if (this.distributor == null) 2815 this.distributor = new ArrayList<PackagingDistributorComponent>(); 2816 return this.distributor; 2817 } 2818 2819 /** 2820 * @return Returns a reference to <code>this</code> for easy method chaining 2821 */ 2822 public DeviceDefinitionPackagingComponent setDistributor(List<PackagingDistributorComponent> theDistributor) { 2823 this.distributor = theDistributor; 2824 return this; 2825 } 2826 2827 public boolean hasDistributor() { 2828 if (this.distributor == null) 2829 return false; 2830 for (PackagingDistributorComponent item : this.distributor) 2831 if (!item.isEmpty()) 2832 return true; 2833 return false; 2834 } 2835 2836 public PackagingDistributorComponent addDistributor() { //3 2837 PackagingDistributorComponent t = new PackagingDistributorComponent(); 2838 if (this.distributor == null) 2839 this.distributor = new ArrayList<PackagingDistributorComponent>(); 2840 this.distributor.add(t); 2841 return t; 2842 } 2843 2844 public DeviceDefinitionPackagingComponent addDistributor(PackagingDistributorComponent t) { //3 2845 if (t == null) 2846 return this; 2847 if (this.distributor == null) 2848 this.distributor = new ArrayList<PackagingDistributorComponent>(); 2849 this.distributor.add(t); 2850 return this; 2851 } 2852 2853 /** 2854 * @return The first repetition of repeating field {@link #distributor}, creating it if it does not already exist {3} 2855 */ 2856 public PackagingDistributorComponent getDistributorFirstRep() { 2857 if (getDistributor().isEmpty()) { 2858 addDistributor(); 2859 } 2860 return getDistributor().get(0); 2861 } 2862 2863 /** 2864 * @return {@link #udiDeviceIdentifier} (Unique Device Identifier (UDI) Barcode string on the packaging.) 2865 */ 2866 public List<DeviceDefinitionUdiDeviceIdentifierComponent> getUdiDeviceIdentifier() { 2867 if (this.udiDeviceIdentifier == null) 2868 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 2869 return this.udiDeviceIdentifier; 2870 } 2871 2872 /** 2873 * @return Returns a reference to <code>this</code> for easy method chaining 2874 */ 2875 public DeviceDefinitionPackagingComponent setUdiDeviceIdentifier(List<DeviceDefinitionUdiDeviceIdentifierComponent> theUdiDeviceIdentifier) { 2876 this.udiDeviceIdentifier = theUdiDeviceIdentifier; 2877 return this; 2878 } 2879 2880 public boolean hasUdiDeviceIdentifier() { 2881 if (this.udiDeviceIdentifier == null) 2882 return false; 2883 for (DeviceDefinitionUdiDeviceIdentifierComponent item : this.udiDeviceIdentifier) 2884 if (!item.isEmpty()) 2885 return true; 2886 return false; 2887 } 2888 2889 public DeviceDefinitionUdiDeviceIdentifierComponent addUdiDeviceIdentifier() { //3 2890 DeviceDefinitionUdiDeviceIdentifierComponent t = new DeviceDefinitionUdiDeviceIdentifierComponent(); 2891 if (this.udiDeviceIdentifier == null) 2892 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 2893 this.udiDeviceIdentifier.add(t); 2894 return t; 2895 } 2896 2897 public DeviceDefinitionPackagingComponent addUdiDeviceIdentifier(DeviceDefinitionUdiDeviceIdentifierComponent t) { //3 2898 if (t == null) 2899 return this; 2900 if (this.udiDeviceIdentifier == null) 2901 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 2902 this.udiDeviceIdentifier.add(t); 2903 return this; 2904 } 2905 2906 /** 2907 * @return The first repetition of repeating field {@link #udiDeviceIdentifier}, creating it if it does not already exist {3} 2908 */ 2909 public DeviceDefinitionUdiDeviceIdentifierComponent getUdiDeviceIdentifierFirstRep() { 2910 if (getUdiDeviceIdentifier().isEmpty()) { 2911 addUdiDeviceIdentifier(); 2912 } 2913 return getUdiDeviceIdentifier().get(0); 2914 } 2915 2916 /** 2917 * @return {@link #packaging} (Allows packages within packages.) 2918 */ 2919 public List<DeviceDefinitionPackagingComponent> getPackaging() { 2920 if (this.packaging == null) 2921 this.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 2922 return this.packaging; 2923 } 2924 2925 /** 2926 * @return Returns a reference to <code>this</code> for easy method chaining 2927 */ 2928 public DeviceDefinitionPackagingComponent setPackaging(List<DeviceDefinitionPackagingComponent> thePackaging) { 2929 this.packaging = thePackaging; 2930 return this; 2931 } 2932 2933 public boolean hasPackaging() { 2934 if (this.packaging == null) 2935 return false; 2936 for (DeviceDefinitionPackagingComponent item : this.packaging) 2937 if (!item.isEmpty()) 2938 return true; 2939 return false; 2940 } 2941 2942 public DeviceDefinitionPackagingComponent addPackaging() { //3 2943 DeviceDefinitionPackagingComponent t = new DeviceDefinitionPackagingComponent(); 2944 if (this.packaging == null) 2945 this.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 2946 this.packaging.add(t); 2947 return t; 2948 } 2949 2950 public DeviceDefinitionPackagingComponent addPackaging(DeviceDefinitionPackagingComponent t) { //3 2951 if (t == null) 2952 return this; 2953 if (this.packaging == null) 2954 this.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 2955 this.packaging.add(t); 2956 return this; 2957 } 2958 2959 /** 2960 * @return The first repetition of repeating field {@link #packaging}, creating it if it does not already exist {3} 2961 */ 2962 public DeviceDefinitionPackagingComponent getPackagingFirstRep() { 2963 if (getPackaging().isEmpty()) { 2964 addPackaging(); 2965 } 2966 return getPackaging().get(0); 2967 } 2968 2969 protected void listChildren(List<Property> children) { 2970 super.listChildren(children); 2971 children.add(new Property("identifier", "Identifier", "The business identifier of the packaged medication.", 0, 1, identifier)); 2972 children.add(new Property("type", "CodeableConcept", "A code that defines the specific type of packaging.", 0, 1, type)); 2973 children.add(new Property("count", "integer", "The number of items contained in the package (devices or sub-packages).", 0, 1, count)); 2974 children.add(new Property("distributor", "", "An organization that distributes the packaged device.", 0, java.lang.Integer.MAX_VALUE, distributor)); 2975 children.add(new Property("udiDeviceIdentifier", "@DeviceDefinition.udiDeviceIdentifier", "Unique Device Identifier (UDI) Barcode string on the packaging.", 0, java.lang.Integer.MAX_VALUE, udiDeviceIdentifier)); 2976 children.add(new Property("packaging", "@DeviceDefinition.packaging", "Allows packages within packages.", 0, java.lang.Integer.MAX_VALUE, packaging)); 2977 } 2978 2979 @Override 2980 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2981 switch (_hash) { 2982 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The business identifier of the packaged medication.", 0, 1, identifier); 2983 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code that defines the specific type of packaging.", 0, 1, type); 2984 case 94851343: /*count*/ return new Property("count", "integer", "The number of items contained in the package (devices or sub-packages).", 0, 1, count); 2985 case 1334482919: /*distributor*/ return new Property("distributor", "", "An organization that distributes the packaged device.", 0, java.lang.Integer.MAX_VALUE, distributor); 2986 case -99121287: /*udiDeviceIdentifier*/ return new Property("udiDeviceIdentifier", "@DeviceDefinition.udiDeviceIdentifier", "Unique Device Identifier (UDI) Barcode string on the packaging.", 0, java.lang.Integer.MAX_VALUE, udiDeviceIdentifier); 2987 case 1802065795: /*packaging*/ return new Property("packaging", "@DeviceDefinition.packaging", "Allows packages within packages.", 0, java.lang.Integer.MAX_VALUE, packaging); 2988 default: return super.getNamedProperty(_hash, _name, _checkValid); 2989 } 2990 2991 } 2992 2993 @Override 2994 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2995 switch (hash) { 2996 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2997 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2998 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // IntegerType 2999 case 1334482919: /*distributor*/ return this.distributor == null ? new Base[0] : this.distributor.toArray(new Base[this.distributor.size()]); // PackagingDistributorComponent 3000 case -99121287: /*udiDeviceIdentifier*/ return this.udiDeviceIdentifier == null ? new Base[0] : this.udiDeviceIdentifier.toArray(new Base[this.udiDeviceIdentifier.size()]); // DeviceDefinitionUdiDeviceIdentifierComponent 3001 case 1802065795: /*packaging*/ return this.packaging == null ? new Base[0] : this.packaging.toArray(new Base[this.packaging.size()]); // DeviceDefinitionPackagingComponent 3002 default: return super.getProperty(hash, name, checkValid); 3003 } 3004 3005 } 3006 3007 @Override 3008 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3009 switch (hash) { 3010 case -1618432855: // identifier 3011 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3012 return value; 3013 case 3575610: // type 3014 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3015 return value; 3016 case 94851343: // count 3017 this.count = TypeConvertor.castToInteger(value); // IntegerType 3018 return value; 3019 case 1334482919: // distributor 3020 this.getDistributor().add((PackagingDistributorComponent) value); // PackagingDistributorComponent 3021 return value; 3022 case -99121287: // udiDeviceIdentifier 3023 this.getUdiDeviceIdentifier().add((DeviceDefinitionUdiDeviceIdentifierComponent) value); // DeviceDefinitionUdiDeviceIdentifierComponent 3024 return value; 3025 case 1802065795: // packaging 3026 this.getPackaging().add((DeviceDefinitionPackagingComponent) value); // DeviceDefinitionPackagingComponent 3027 return value; 3028 default: return super.setProperty(hash, name, value); 3029 } 3030 3031 } 3032 3033 @Override 3034 public Base setProperty(String name, Base value) throws FHIRException { 3035 if (name.equals("identifier")) { 3036 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3037 } else if (name.equals("type")) { 3038 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3039 } else if (name.equals("count")) { 3040 this.count = TypeConvertor.castToInteger(value); // IntegerType 3041 } else if (name.equals("distributor")) { 3042 this.getDistributor().add((PackagingDistributorComponent) value); 3043 } else if (name.equals("udiDeviceIdentifier")) { 3044 this.getUdiDeviceIdentifier().add((DeviceDefinitionUdiDeviceIdentifierComponent) value); 3045 } else if (name.equals("packaging")) { 3046 this.getPackaging().add((DeviceDefinitionPackagingComponent) value); 3047 } else 3048 return super.setProperty(name, value); 3049 return value; 3050 } 3051 3052 @Override 3053 public void removeChild(String name, Base value) throws FHIRException { 3054 if (name.equals("identifier")) { 3055 this.identifier = null; 3056 } else if (name.equals("type")) { 3057 this.type = null; 3058 } else if (name.equals("count")) { 3059 this.count = null; 3060 } else if (name.equals("distributor")) { 3061 this.getDistributor().remove((PackagingDistributorComponent) value); 3062 } else if (name.equals("udiDeviceIdentifier")) { 3063 this.getUdiDeviceIdentifier().remove((DeviceDefinitionUdiDeviceIdentifierComponent) value); 3064 } else if (name.equals("packaging")) { 3065 this.getPackaging().remove((DeviceDefinitionPackagingComponent) value); 3066 } else 3067 super.removeChild(name, value); 3068 3069 } 3070 3071 @Override 3072 public Base makeProperty(int hash, String name) throws FHIRException { 3073 switch (hash) { 3074 case -1618432855: return getIdentifier(); 3075 case 3575610: return getType(); 3076 case 94851343: return getCountElement(); 3077 case 1334482919: return addDistributor(); 3078 case -99121287: return addUdiDeviceIdentifier(); 3079 case 1802065795: return addPackaging(); 3080 default: return super.makeProperty(hash, name); 3081 } 3082 3083 } 3084 3085 @Override 3086 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3087 switch (hash) { 3088 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3089 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3090 case 94851343: /*count*/ return new String[] {"integer"}; 3091 case 1334482919: /*distributor*/ return new String[] {}; 3092 case -99121287: /*udiDeviceIdentifier*/ return new String[] {"@DeviceDefinition.udiDeviceIdentifier"}; 3093 case 1802065795: /*packaging*/ return new String[] {"@DeviceDefinition.packaging"}; 3094 default: return super.getTypesForProperty(hash, name); 3095 } 3096 3097 } 3098 3099 @Override 3100 public Base addChild(String name) throws FHIRException { 3101 if (name.equals("identifier")) { 3102 this.identifier = new Identifier(); 3103 return this.identifier; 3104 } 3105 else if (name.equals("type")) { 3106 this.type = new CodeableConcept(); 3107 return this.type; 3108 } 3109 else if (name.equals("count")) { 3110 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.packaging.count"); 3111 } 3112 else if (name.equals("distributor")) { 3113 return addDistributor(); 3114 } 3115 else if (name.equals("udiDeviceIdentifier")) { 3116 return addUdiDeviceIdentifier(); 3117 } 3118 else if (name.equals("packaging")) { 3119 return addPackaging(); 3120 } 3121 else 3122 return super.addChild(name); 3123 } 3124 3125 public DeviceDefinitionPackagingComponent copy() { 3126 DeviceDefinitionPackagingComponent dst = new DeviceDefinitionPackagingComponent(); 3127 copyValues(dst); 3128 return dst; 3129 } 3130 3131 public void copyValues(DeviceDefinitionPackagingComponent dst) { 3132 super.copyValues(dst); 3133 dst.identifier = identifier == null ? null : identifier.copy(); 3134 dst.type = type == null ? null : type.copy(); 3135 dst.count = count == null ? null : count.copy(); 3136 if (distributor != null) { 3137 dst.distributor = new ArrayList<PackagingDistributorComponent>(); 3138 for (PackagingDistributorComponent i : distributor) 3139 dst.distributor.add(i.copy()); 3140 }; 3141 if (udiDeviceIdentifier != null) { 3142 dst.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 3143 for (DeviceDefinitionUdiDeviceIdentifierComponent i : udiDeviceIdentifier) 3144 dst.udiDeviceIdentifier.add(i.copy()); 3145 }; 3146 if (packaging != null) { 3147 dst.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 3148 for (DeviceDefinitionPackagingComponent i : packaging) 3149 dst.packaging.add(i.copy()); 3150 }; 3151 } 3152 3153 @Override 3154 public boolean equalsDeep(Base other_) { 3155 if (!super.equalsDeep(other_)) 3156 return false; 3157 if (!(other_ instanceof DeviceDefinitionPackagingComponent)) 3158 return false; 3159 DeviceDefinitionPackagingComponent o = (DeviceDefinitionPackagingComponent) other_; 3160 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(count, o.count, true) 3161 && compareDeep(distributor, o.distributor, true) && compareDeep(udiDeviceIdentifier, o.udiDeviceIdentifier, true) 3162 && compareDeep(packaging, o.packaging, true); 3163 } 3164 3165 @Override 3166 public boolean equalsShallow(Base other_) { 3167 if (!super.equalsShallow(other_)) 3168 return false; 3169 if (!(other_ instanceof DeviceDefinitionPackagingComponent)) 3170 return false; 3171 DeviceDefinitionPackagingComponent o = (DeviceDefinitionPackagingComponent) other_; 3172 return compareValues(count, o.count, true); 3173 } 3174 3175 public boolean isEmpty() { 3176 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, count 3177 , distributor, udiDeviceIdentifier, packaging); 3178 } 3179 3180 public String fhirType() { 3181 return "DeviceDefinition.packaging"; 3182 3183 } 3184 3185 } 3186 3187 @Block() 3188 public static class PackagingDistributorComponent extends BackboneElement implements IBaseBackboneElement { 3189 /** 3190 * Distributor's human-readable name. 3191 */ 3192 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3193 @Description(shortDefinition="Distributor's human-readable name", formalDefinition="Distributor's human-readable name." ) 3194 protected StringType name; 3195 3196 /** 3197 * Distributor as an Organization resource. 3198 */ 3199 @Child(name = "organizationReference", type = {Organization.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3200 @Description(shortDefinition="Distributor as an Organization resource", formalDefinition="Distributor as an Organization resource." ) 3201 protected List<Reference> organizationReference; 3202 3203 private static final long serialVersionUID = 1587433419L; 3204 3205 /** 3206 * Constructor 3207 */ 3208 public PackagingDistributorComponent() { 3209 super(); 3210 } 3211 3212 /** 3213 * @return {@link #name} (Distributor's human-readable name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3214 */ 3215 public StringType getNameElement() { 3216 if (this.name == null) 3217 if (Configuration.errorOnAutoCreate()) 3218 throw new Error("Attempt to auto-create PackagingDistributorComponent.name"); 3219 else if (Configuration.doAutoCreate()) 3220 this.name = new StringType(); // bb 3221 return this.name; 3222 } 3223 3224 public boolean hasNameElement() { 3225 return this.name != null && !this.name.isEmpty(); 3226 } 3227 3228 public boolean hasName() { 3229 return this.name != null && !this.name.isEmpty(); 3230 } 3231 3232 /** 3233 * @param value {@link #name} (Distributor's human-readable name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3234 */ 3235 public PackagingDistributorComponent setNameElement(StringType value) { 3236 this.name = value; 3237 return this; 3238 } 3239 3240 /** 3241 * @return Distributor's human-readable name. 3242 */ 3243 public String getName() { 3244 return this.name == null ? null : this.name.getValue(); 3245 } 3246 3247 /** 3248 * @param value Distributor's human-readable name. 3249 */ 3250 public PackagingDistributorComponent setName(String value) { 3251 if (Utilities.noString(value)) 3252 this.name = null; 3253 else { 3254 if (this.name == null) 3255 this.name = new StringType(); 3256 this.name.setValue(value); 3257 } 3258 return this; 3259 } 3260 3261 /** 3262 * @return {@link #organizationReference} (Distributor as an Organization resource.) 3263 */ 3264 public List<Reference> getOrganizationReference() { 3265 if (this.organizationReference == null) 3266 this.organizationReference = new ArrayList<Reference>(); 3267 return this.organizationReference; 3268 } 3269 3270 /** 3271 * @return Returns a reference to <code>this</code> for easy method chaining 3272 */ 3273 public PackagingDistributorComponent setOrganizationReference(List<Reference> theOrganizationReference) { 3274 this.organizationReference = theOrganizationReference; 3275 return this; 3276 } 3277 3278 public boolean hasOrganizationReference() { 3279 if (this.organizationReference == null) 3280 return false; 3281 for (Reference item : this.organizationReference) 3282 if (!item.isEmpty()) 3283 return true; 3284 return false; 3285 } 3286 3287 public Reference addOrganizationReference() { //3 3288 Reference t = new Reference(); 3289 if (this.organizationReference == null) 3290 this.organizationReference = new ArrayList<Reference>(); 3291 this.organizationReference.add(t); 3292 return t; 3293 } 3294 3295 public PackagingDistributorComponent addOrganizationReference(Reference t) { //3 3296 if (t == null) 3297 return this; 3298 if (this.organizationReference == null) 3299 this.organizationReference = new ArrayList<Reference>(); 3300 this.organizationReference.add(t); 3301 return this; 3302 } 3303 3304 /** 3305 * @return The first repetition of repeating field {@link #organizationReference}, creating it if it does not already exist {3} 3306 */ 3307 public Reference getOrganizationReferenceFirstRep() { 3308 if (getOrganizationReference().isEmpty()) { 3309 addOrganizationReference(); 3310 } 3311 return getOrganizationReference().get(0); 3312 } 3313 3314 protected void listChildren(List<Property> children) { 3315 super.listChildren(children); 3316 children.add(new Property("name", "string", "Distributor's human-readable name.", 0, 1, name)); 3317 children.add(new Property("organizationReference", "Reference(Organization)", "Distributor as an Organization resource.", 0, java.lang.Integer.MAX_VALUE, organizationReference)); 3318 } 3319 3320 @Override 3321 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3322 switch (_hash) { 3323 case 3373707: /*name*/ return new Property("name", "string", "Distributor's human-readable name.", 0, 1, name); 3324 case 1860475736: /*organizationReference*/ return new Property("organizationReference", "Reference(Organization)", "Distributor as an Organization resource.", 0, java.lang.Integer.MAX_VALUE, organizationReference); 3325 default: return super.getNamedProperty(_hash, _name, _checkValid); 3326 } 3327 3328 } 3329 3330 @Override 3331 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3332 switch (hash) { 3333 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3334 case 1860475736: /*organizationReference*/ return this.organizationReference == null ? new Base[0] : this.organizationReference.toArray(new Base[this.organizationReference.size()]); // Reference 3335 default: return super.getProperty(hash, name, checkValid); 3336 } 3337 3338 } 3339 3340 @Override 3341 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3342 switch (hash) { 3343 case 3373707: // name 3344 this.name = TypeConvertor.castToString(value); // StringType 3345 return value; 3346 case 1860475736: // organizationReference 3347 this.getOrganizationReference().add(TypeConvertor.castToReference(value)); // Reference 3348 return value; 3349 default: return super.setProperty(hash, name, value); 3350 } 3351 3352 } 3353 3354 @Override 3355 public Base setProperty(String name, Base value) throws FHIRException { 3356 if (name.equals("name")) { 3357 this.name = TypeConvertor.castToString(value); // StringType 3358 } else if (name.equals("organizationReference")) { 3359 this.getOrganizationReference().add(TypeConvertor.castToReference(value)); 3360 } else 3361 return super.setProperty(name, value); 3362 return value; 3363 } 3364 3365 @Override 3366 public void removeChild(String name, Base value) throws FHIRException { 3367 if (name.equals("name")) { 3368 this.name = null; 3369 } else if (name.equals("organizationReference")) { 3370 this.getOrganizationReference().remove(value); 3371 } else 3372 super.removeChild(name, value); 3373 3374 } 3375 3376 @Override 3377 public Base makeProperty(int hash, String name) throws FHIRException { 3378 switch (hash) { 3379 case 3373707: return getNameElement(); 3380 case 1860475736: return addOrganizationReference(); 3381 default: return super.makeProperty(hash, name); 3382 } 3383 3384 } 3385 3386 @Override 3387 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3388 switch (hash) { 3389 case 3373707: /*name*/ return new String[] {"string"}; 3390 case 1860475736: /*organizationReference*/ return new String[] {"Reference"}; 3391 default: return super.getTypesForProperty(hash, name); 3392 } 3393 3394 } 3395 3396 @Override 3397 public Base addChild(String name) throws FHIRException { 3398 if (name.equals("name")) { 3399 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.packaging.distributor.name"); 3400 } 3401 else if (name.equals("organizationReference")) { 3402 return addOrganizationReference(); 3403 } 3404 else 3405 return super.addChild(name); 3406 } 3407 3408 public PackagingDistributorComponent copy() { 3409 PackagingDistributorComponent dst = new PackagingDistributorComponent(); 3410 copyValues(dst); 3411 return dst; 3412 } 3413 3414 public void copyValues(PackagingDistributorComponent dst) { 3415 super.copyValues(dst); 3416 dst.name = name == null ? null : name.copy(); 3417 if (organizationReference != null) { 3418 dst.organizationReference = new ArrayList<Reference>(); 3419 for (Reference i : organizationReference) 3420 dst.organizationReference.add(i.copy()); 3421 }; 3422 } 3423 3424 @Override 3425 public boolean equalsDeep(Base other_) { 3426 if (!super.equalsDeep(other_)) 3427 return false; 3428 if (!(other_ instanceof PackagingDistributorComponent)) 3429 return false; 3430 PackagingDistributorComponent o = (PackagingDistributorComponent) other_; 3431 return compareDeep(name, o.name, true) && compareDeep(organizationReference, o.organizationReference, true) 3432 ; 3433 } 3434 3435 @Override 3436 public boolean equalsShallow(Base other_) { 3437 if (!super.equalsShallow(other_)) 3438 return false; 3439 if (!(other_ instanceof PackagingDistributorComponent)) 3440 return false; 3441 PackagingDistributorComponent o = (PackagingDistributorComponent) other_; 3442 return compareValues(name, o.name, true); 3443 } 3444 3445 public boolean isEmpty() { 3446 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, organizationReference 3447 ); 3448 } 3449 3450 public String fhirType() { 3451 return "DeviceDefinition.packaging.distributor"; 3452 3453 } 3454 3455 } 3456 3457 @Block() 3458 public static class DeviceDefinitionVersionComponent extends BackboneElement implements IBaseBackboneElement { 3459 /** 3460 * The type of the device version, e.g. manufacturer, approved, internal. 3461 */ 3462 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 3463 @Description(shortDefinition="The type of the device version, e.g. manufacturer, approved, internal", formalDefinition="The type of the device version, e.g. manufacturer, approved, internal." ) 3464 protected CodeableConcept type; 3465 3466 /** 3467 * The hardware or software module of the device to which the version applies. 3468 */ 3469 @Child(name = "component", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 3470 @Description(shortDefinition="The hardware or software module of the device to which the version applies", formalDefinition="The hardware or software module of the device to which the version applies." ) 3471 protected Identifier component; 3472 3473 /** 3474 * The version text. 3475 */ 3476 @Child(name = "value", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=false) 3477 @Description(shortDefinition="The version text", formalDefinition="The version text." ) 3478 protected StringType value; 3479 3480 private static final long serialVersionUID = 645214295L; 3481 3482 /** 3483 * Constructor 3484 */ 3485 public DeviceDefinitionVersionComponent() { 3486 super(); 3487 } 3488 3489 /** 3490 * Constructor 3491 */ 3492 public DeviceDefinitionVersionComponent(String value) { 3493 super(); 3494 this.setValue(value); 3495 } 3496 3497 /** 3498 * @return {@link #type} (The type of the device version, e.g. manufacturer, approved, internal.) 3499 */ 3500 public CodeableConcept getType() { 3501 if (this.type == null) 3502 if (Configuration.errorOnAutoCreate()) 3503 throw new Error("Attempt to auto-create DeviceDefinitionVersionComponent.type"); 3504 else if (Configuration.doAutoCreate()) 3505 this.type = new CodeableConcept(); // cc 3506 return this.type; 3507 } 3508 3509 public boolean hasType() { 3510 return this.type != null && !this.type.isEmpty(); 3511 } 3512 3513 /** 3514 * @param value {@link #type} (The type of the device version, e.g. manufacturer, approved, internal.) 3515 */ 3516 public DeviceDefinitionVersionComponent setType(CodeableConcept value) { 3517 this.type = value; 3518 return this; 3519 } 3520 3521 /** 3522 * @return {@link #component} (The hardware or software module of the device to which the version applies.) 3523 */ 3524 public Identifier getComponent() { 3525 if (this.component == null) 3526 if (Configuration.errorOnAutoCreate()) 3527 throw new Error("Attempt to auto-create DeviceDefinitionVersionComponent.component"); 3528 else if (Configuration.doAutoCreate()) 3529 this.component = new Identifier(); // cc 3530 return this.component; 3531 } 3532 3533 public boolean hasComponent() { 3534 return this.component != null && !this.component.isEmpty(); 3535 } 3536 3537 /** 3538 * @param value {@link #component} (The hardware or software module of the device to which the version applies.) 3539 */ 3540 public DeviceDefinitionVersionComponent setComponent(Identifier value) { 3541 this.component = value; 3542 return this; 3543 } 3544 3545 /** 3546 * @return {@link #value} (The version text.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 3547 */ 3548 public StringType getValueElement() { 3549 if (this.value == null) 3550 if (Configuration.errorOnAutoCreate()) 3551 throw new Error("Attempt to auto-create DeviceDefinitionVersionComponent.value"); 3552 else if (Configuration.doAutoCreate()) 3553 this.value = new StringType(); // bb 3554 return this.value; 3555 } 3556 3557 public boolean hasValueElement() { 3558 return this.value != null && !this.value.isEmpty(); 3559 } 3560 3561 public boolean hasValue() { 3562 return this.value != null && !this.value.isEmpty(); 3563 } 3564 3565 /** 3566 * @param value {@link #value} (The version text.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 3567 */ 3568 public DeviceDefinitionVersionComponent setValueElement(StringType value) { 3569 this.value = value; 3570 return this; 3571 } 3572 3573 /** 3574 * @return The version text. 3575 */ 3576 public String getValue() { 3577 return this.value == null ? null : this.value.getValue(); 3578 } 3579 3580 /** 3581 * @param value The version text. 3582 */ 3583 public DeviceDefinitionVersionComponent setValue(String value) { 3584 if (this.value == null) 3585 this.value = new StringType(); 3586 this.value.setValue(value); 3587 return this; 3588 } 3589 3590 protected void listChildren(List<Property> children) { 3591 super.listChildren(children); 3592 children.add(new Property("type", "CodeableConcept", "The type of the device version, e.g. manufacturer, approved, internal.", 0, 1, type)); 3593 children.add(new Property("component", "Identifier", "The hardware or software module of the device to which the version applies.", 0, 1, component)); 3594 children.add(new Property("value", "string", "The version text.", 0, 1, value)); 3595 } 3596 3597 @Override 3598 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3599 switch (_hash) { 3600 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the device version, e.g. manufacturer, approved, internal.", 0, 1, type); 3601 case -1399907075: /*component*/ return new Property("component", "Identifier", "The hardware or software module of the device to which the version applies.", 0, 1, component); 3602 case 111972721: /*value*/ return new Property("value", "string", "The version text.", 0, 1, value); 3603 default: return super.getNamedProperty(_hash, _name, _checkValid); 3604 } 3605 3606 } 3607 3608 @Override 3609 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3610 switch (hash) { 3611 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3612 case -1399907075: /*component*/ return this.component == null ? new Base[0] : new Base[] {this.component}; // Identifier 3613 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 3614 default: return super.getProperty(hash, name, checkValid); 3615 } 3616 3617 } 3618 3619 @Override 3620 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3621 switch (hash) { 3622 case 3575610: // type 3623 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3624 return value; 3625 case -1399907075: // component 3626 this.component = TypeConvertor.castToIdentifier(value); // Identifier 3627 return value; 3628 case 111972721: // value 3629 this.value = TypeConvertor.castToString(value); // StringType 3630 return value; 3631 default: return super.setProperty(hash, name, value); 3632 } 3633 3634 } 3635 3636 @Override 3637 public Base setProperty(String name, Base value) throws FHIRException { 3638 if (name.equals("type")) { 3639 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3640 } else if (name.equals("component")) { 3641 this.component = TypeConvertor.castToIdentifier(value); // Identifier 3642 } else if (name.equals("value")) { 3643 this.value = TypeConvertor.castToString(value); // StringType 3644 } else 3645 return super.setProperty(name, value); 3646 return value; 3647 } 3648 3649 @Override 3650 public void removeChild(String name, Base value) throws FHIRException { 3651 if (name.equals("type")) { 3652 this.type = null; 3653 } else if (name.equals("component")) { 3654 this.component = null; 3655 } else if (name.equals("value")) { 3656 this.value = null; 3657 } else 3658 super.removeChild(name, value); 3659 3660 } 3661 3662 @Override 3663 public Base makeProperty(int hash, String name) throws FHIRException { 3664 switch (hash) { 3665 case 3575610: return getType(); 3666 case -1399907075: return getComponent(); 3667 case 111972721: return getValueElement(); 3668 default: return super.makeProperty(hash, name); 3669 } 3670 3671 } 3672 3673 @Override 3674 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3675 switch (hash) { 3676 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3677 case -1399907075: /*component*/ return new String[] {"Identifier"}; 3678 case 111972721: /*value*/ return new String[] {"string"}; 3679 default: return super.getTypesForProperty(hash, name); 3680 } 3681 3682 } 3683 3684 @Override 3685 public Base addChild(String name) throws FHIRException { 3686 if (name.equals("type")) { 3687 this.type = new CodeableConcept(); 3688 return this.type; 3689 } 3690 else if (name.equals("component")) { 3691 this.component = new Identifier(); 3692 return this.component; 3693 } 3694 else if (name.equals("value")) { 3695 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.version.value"); 3696 } 3697 else 3698 return super.addChild(name); 3699 } 3700 3701 public DeviceDefinitionVersionComponent copy() { 3702 DeviceDefinitionVersionComponent dst = new DeviceDefinitionVersionComponent(); 3703 copyValues(dst); 3704 return dst; 3705 } 3706 3707 public void copyValues(DeviceDefinitionVersionComponent dst) { 3708 super.copyValues(dst); 3709 dst.type = type == null ? null : type.copy(); 3710 dst.component = component == null ? null : component.copy(); 3711 dst.value = value == null ? null : value.copy(); 3712 } 3713 3714 @Override 3715 public boolean equalsDeep(Base other_) { 3716 if (!super.equalsDeep(other_)) 3717 return false; 3718 if (!(other_ instanceof DeviceDefinitionVersionComponent)) 3719 return false; 3720 DeviceDefinitionVersionComponent o = (DeviceDefinitionVersionComponent) other_; 3721 return compareDeep(type, o.type, true) && compareDeep(component, o.component, true) && compareDeep(value, o.value, true) 3722 ; 3723 } 3724 3725 @Override 3726 public boolean equalsShallow(Base other_) { 3727 if (!super.equalsShallow(other_)) 3728 return false; 3729 if (!(other_ instanceof DeviceDefinitionVersionComponent)) 3730 return false; 3731 DeviceDefinitionVersionComponent o = (DeviceDefinitionVersionComponent) other_; 3732 return compareValues(value, o.value, true); 3733 } 3734 3735 public boolean isEmpty() { 3736 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, component, value); 3737 } 3738 3739 public String fhirType() { 3740 return "DeviceDefinition.version"; 3741 3742 } 3743 3744 } 3745 3746 @Block() 3747 public static class DeviceDefinitionPropertyComponent extends BackboneElement implements IBaseBackboneElement { 3748 /** 3749 * Code that specifies the property such as a resolution or color being represented. 3750 */ 3751 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 3752 @Description(shortDefinition="Code that specifies the property being represented", formalDefinition="Code that specifies the property such as a resolution or color being represented." ) 3753 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-property-type") 3754 protected CodeableConcept type; 3755 3756 /** 3757 * The value of the property specified by the associated property.type code. 3758 */ 3759 @Child(name = "value", type = {Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Range.class, Attachment.class}, order=2, min=1, max=1, modifier=false, summary=false) 3760 @Description(shortDefinition="Value of the property", formalDefinition="The value of the property specified by the associated property.type code." ) 3761 protected DataType value; 3762 3763 private static final long serialVersionUID = -1659186716L; 3764 3765 /** 3766 * Constructor 3767 */ 3768 public DeviceDefinitionPropertyComponent() { 3769 super(); 3770 } 3771 3772 /** 3773 * Constructor 3774 */ 3775 public DeviceDefinitionPropertyComponent(CodeableConcept type, DataType value) { 3776 super(); 3777 this.setType(type); 3778 this.setValue(value); 3779 } 3780 3781 /** 3782 * @return {@link #type} (Code that specifies the property such as a resolution or color being represented.) 3783 */ 3784 public CodeableConcept getType() { 3785 if (this.type == null) 3786 if (Configuration.errorOnAutoCreate()) 3787 throw new Error("Attempt to auto-create DeviceDefinitionPropertyComponent.type"); 3788 else if (Configuration.doAutoCreate()) 3789 this.type = new CodeableConcept(); // cc 3790 return this.type; 3791 } 3792 3793 public boolean hasType() { 3794 return this.type != null && !this.type.isEmpty(); 3795 } 3796 3797 /** 3798 * @param value {@link #type} (Code that specifies the property such as a resolution or color being represented.) 3799 */ 3800 public DeviceDefinitionPropertyComponent setType(CodeableConcept value) { 3801 this.type = value; 3802 return this; 3803 } 3804 3805 /** 3806 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3807 */ 3808 public DataType getValue() { 3809 return this.value; 3810 } 3811 3812 /** 3813 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3814 */ 3815 public Quantity getValueQuantity() throws FHIRException { 3816 if (this.value == null) 3817 this.value = new Quantity(); 3818 if (!(this.value instanceof Quantity)) 3819 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3820 return (Quantity) this.value; 3821 } 3822 3823 public boolean hasValueQuantity() { 3824 return this != null && this.value instanceof Quantity; 3825 } 3826 3827 /** 3828 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3829 */ 3830 public CodeableConcept getValueCodeableConcept() throws FHIRException { 3831 if (this.value == null) 3832 this.value = new CodeableConcept(); 3833 if (!(this.value instanceof CodeableConcept)) 3834 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 3835 return (CodeableConcept) this.value; 3836 } 3837 3838 public boolean hasValueCodeableConcept() { 3839 return this != null && this.value instanceof CodeableConcept; 3840 } 3841 3842 /** 3843 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3844 */ 3845 public StringType getValueStringType() throws FHIRException { 3846 if (this.value == null) 3847 this.value = new StringType(); 3848 if (!(this.value instanceof StringType)) 3849 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 3850 return (StringType) this.value; 3851 } 3852 3853 public boolean hasValueStringType() { 3854 return this != null && this.value instanceof StringType; 3855 } 3856 3857 /** 3858 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3859 */ 3860 public BooleanType getValueBooleanType() throws FHIRException { 3861 if (this.value == null) 3862 this.value = new BooleanType(); 3863 if (!(this.value instanceof BooleanType)) 3864 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 3865 return (BooleanType) this.value; 3866 } 3867 3868 public boolean hasValueBooleanType() { 3869 return this != null && this.value instanceof BooleanType; 3870 } 3871 3872 /** 3873 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3874 */ 3875 public IntegerType getValueIntegerType() throws FHIRException { 3876 if (this.value == null) 3877 this.value = new IntegerType(); 3878 if (!(this.value instanceof IntegerType)) 3879 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 3880 return (IntegerType) this.value; 3881 } 3882 3883 public boolean hasValueIntegerType() { 3884 return this != null && this.value instanceof IntegerType; 3885 } 3886 3887 /** 3888 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3889 */ 3890 public Range getValueRange() throws FHIRException { 3891 if (this.value == null) 3892 this.value = new Range(); 3893 if (!(this.value instanceof Range)) 3894 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 3895 return (Range) this.value; 3896 } 3897 3898 public boolean hasValueRange() { 3899 return this != null && this.value instanceof Range; 3900 } 3901 3902 /** 3903 * @return {@link #value} (The value of the property specified by the associated property.type code.) 3904 */ 3905 public Attachment getValueAttachment() throws FHIRException { 3906 if (this.value == null) 3907 this.value = new Attachment(); 3908 if (!(this.value instanceof Attachment)) 3909 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 3910 return (Attachment) this.value; 3911 } 3912 3913 public boolean hasValueAttachment() { 3914 return this != null && this.value instanceof Attachment; 3915 } 3916 3917 public boolean hasValue() { 3918 return this.value != null && !this.value.isEmpty(); 3919 } 3920 3921 /** 3922 * @param value {@link #value} (The value of the property specified by the associated property.type code.) 3923 */ 3924 public DeviceDefinitionPropertyComponent setValue(DataType value) { 3925 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range || value instanceof Attachment)) 3926 throw new FHIRException("Not the right type for DeviceDefinition.property.value[x]: "+value.fhirType()); 3927 this.value = value; 3928 return this; 3929 } 3930 3931 protected void listChildren(List<Property> children) { 3932 super.listChildren(children); 3933 children.add(new Property("type", "CodeableConcept", "Code that specifies the property such as a resolution or color being represented.", 0, 1, type)); 3934 children.add(new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value)); 3935 } 3936 3937 @Override 3938 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3939 switch (_hash) { 3940 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Code that specifies the property such as a resolution or color being represented.", 0, 1, type); 3941 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 3942 case 111972721: /*value*/ return new Property("value[x]", "Quantity|CodeableConcept|string|boolean|integer|Range|Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 3943 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the property specified by the associated property.type code.", 0, 1, value); 3944 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the property specified by the associated property.type code.", 0, 1, value); 3945 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the property specified by the associated property.type code.", 0, 1, value); 3946 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the property specified by the associated property.type code.", 0, 1, value); 3947 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the property specified by the associated property.type code.", 0, 1, value); 3948 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the property specified by the associated property.type code.", 0, 1, value); 3949 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The value of the property specified by the associated property.type code.", 0, 1, value); 3950 default: return super.getNamedProperty(_hash, _name, _checkValid); 3951 } 3952 3953 } 3954 3955 @Override 3956 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3957 switch (hash) { 3958 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3959 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3960 default: return super.getProperty(hash, name, checkValid); 3961 } 3962 3963 } 3964 3965 @Override 3966 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3967 switch (hash) { 3968 case 3575610: // type 3969 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3970 return value; 3971 case 111972721: // value 3972 this.value = TypeConvertor.castToType(value); // DataType 3973 return value; 3974 default: return super.setProperty(hash, name, value); 3975 } 3976 3977 } 3978 3979 @Override 3980 public Base setProperty(String name, Base value) throws FHIRException { 3981 if (name.equals("type")) { 3982 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3983 } else if (name.equals("value[x]")) { 3984 this.value = TypeConvertor.castToType(value); // DataType 3985 } else 3986 return super.setProperty(name, value); 3987 return value; 3988 } 3989 3990 @Override 3991 public void removeChild(String name, Base value) throws FHIRException { 3992 if (name.equals("type")) { 3993 this.type = null; 3994 } else if (name.equals("value[x]")) { 3995 this.value = null; 3996 } else 3997 super.removeChild(name, value); 3998 3999 } 4000 4001 @Override 4002 public Base makeProperty(int hash, String name) throws FHIRException { 4003 switch (hash) { 4004 case 3575610: return getType(); 4005 case -1410166417: return getValue(); 4006 case 111972721: return getValue(); 4007 default: return super.makeProperty(hash, name); 4008 } 4009 4010 } 4011 4012 @Override 4013 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4014 switch (hash) { 4015 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4016 case 111972721: /*value*/ return new String[] {"Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Attachment"}; 4017 default: return super.getTypesForProperty(hash, name); 4018 } 4019 4020 } 4021 4022 @Override 4023 public Base addChild(String name) throws FHIRException { 4024 if (name.equals("type")) { 4025 this.type = new CodeableConcept(); 4026 return this.type; 4027 } 4028 else if (name.equals("valueQuantity")) { 4029 this.value = new Quantity(); 4030 return this.value; 4031 } 4032 else if (name.equals("valueCodeableConcept")) { 4033 this.value = new CodeableConcept(); 4034 return this.value; 4035 } 4036 else if (name.equals("valueString")) { 4037 this.value = new StringType(); 4038 return this.value; 4039 } 4040 else if (name.equals("valueBoolean")) { 4041 this.value = new BooleanType(); 4042 return this.value; 4043 } 4044 else if (name.equals("valueInteger")) { 4045 this.value = new IntegerType(); 4046 return this.value; 4047 } 4048 else if (name.equals("valueRange")) { 4049 this.value = new Range(); 4050 return this.value; 4051 } 4052 else if (name.equals("valueAttachment")) { 4053 this.value = new Attachment(); 4054 return this.value; 4055 } 4056 else 4057 return super.addChild(name); 4058 } 4059 4060 public DeviceDefinitionPropertyComponent copy() { 4061 DeviceDefinitionPropertyComponent dst = new DeviceDefinitionPropertyComponent(); 4062 copyValues(dst); 4063 return dst; 4064 } 4065 4066 public void copyValues(DeviceDefinitionPropertyComponent dst) { 4067 super.copyValues(dst); 4068 dst.type = type == null ? null : type.copy(); 4069 dst.value = value == null ? null : value.copy(); 4070 } 4071 4072 @Override 4073 public boolean equalsDeep(Base other_) { 4074 if (!super.equalsDeep(other_)) 4075 return false; 4076 if (!(other_ instanceof DeviceDefinitionPropertyComponent)) 4077 return false; 4078 DeviceDefinitionPropertyComponent o = (DeviceDefinitionPropertyComponent) other_; 4079 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 4080 } 4081 4082 @Override 4083 public boolean equalsShallow(Base other_) { 4084 if (!super.equalsShallow(other_)) 4085 return false; 4086 if (!(other_ instanceof DeviceDefinitionPropertyComponent)) 4087 return false; 4088 DeviceDefinitionPropertyComponent o = (DeviceDefinitionPropertyComponent) other_; 4089 return true; 4090 } 4091 4092 public boolean isEmpty() { 4093 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 4094 } 4095 4096 public String fhirType() { 4097 return "DeviceDefinition.property"; 4098 4099 } 4100 4101 } 4102 4103 @Block() 4104 public static class DeviceDefinitionLinkComponent extends BackboneElement implements IBaseBackboneElement { 4105 /** 4106 * The type indicates the relationship of the related device to the device instance. 4107 */ 4108 @Child(name = "relation", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 4109 @Description(shortDefinition="The type indicates the relationship of the related device to the device instance", formalDefinition="The type indicates the relationship of the related device to the device instance." ) 4110 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicedefinition-relationtype") 4111 protected Coding relation; 4112 4113 /** 4114 * A reference to the linked device. 4115 */ 4116 @Child(name = "relatedDevice", type = {CodeableReference.class}, order=2, min=1, max=1, modifier=false, summary=false) 4117 @Description(shortDefinition="A reference to the linked device", formalDefinition="A reference to the linked device." ) 4118 protected CodeableReference relatedDevice; 4119 4120 private static final long serialVersionUID = 627614461L; 4121 4122 /** 4123 * Constructor 4124 */ 4125 public DeviceDefinitionLinkComponent() { 4126 super(); 4127 } 4128 4129 /** 4130 * Constructor 4131 */ 4132 public DeviceDefinitionLinkComponent(Coding relation, CodeableReference relatedDevice) { 4133 super(); 4134 this.setRelation(relation); 4135 this.setRelatedDevice(relatedDevice); 4136 } 4137 4138 /** 4139 * @return {@link #relation} (The type indicates the relationship of the related device to the device instance.) 4140 */ 4141 public Coding getRelation() { 4142 if (this.relation == null) 4143 if (Configuration.errorOnAutoCreate()) 4144 throw new Error("Attempt to auto-create DeviceDefinitionLinkComponent.relation"); 4145 else if (Configuration.doAutoCreate()) 4146 this.relation = new Coding(); // cc 4147 return this.relation; 4148 } 4149 4150 public boolean hasRelation() { 4151 return this.relation != null && !this.relation.isEmpty(); 4152 } 4153 4154 /** 4155 * @param value {@link #relation} (The type indicates the relationship of the related device to the device instance.) 4156 */ 4157 public DeviceDefinitionLinkComponent setRelation(Coding value) { 4158 this.relation = value; 4159 return this; 4160 } 4161 4162 /** 4163 * @return {@link #relatedDevice} (A reference to the linked device.) 4164 */ 4165 public CodeableReference getRelatedDevice() { 4166 if (this.relatedDevice == null) 4167 if (Configuration.errorOnAutoCreate()) 4168 throw new Error("Attempt to auto-create DeviceDefinitionLinkComponent.relatedDevice"); 4169 else if (Configuration.doAutoCreate()) 4170 this.relatedDevice = new CodeableReference(); // cc 4171 return this.relatedDevice; 4172 } 4173 4174 public boolean hasRelatedDevice() { 4175 return this.relatedDevice != null && !this.relatedDevice.isEmpty(); 4176 } 4177 4178 /** 4179 * @param value {@link #relatedDevice} (A reference to the linked device.) 4180 */ 4181 public DeviceDefinitionLinkComponent setRelatedDevice(CodeableReference value) { 4182 this.relatedDevice = value; 4183 return this; 4184 } 4185 4186 protected void listChildren(List<Property> children) { 4187 super.listChildren(children); 4188 children.add(new Property("relation", "Coding", "The type indicates the relationship of the related device to the device instance.", 0, 1, relation)); 4189 children.add(new Property("relatedDevice", "CodeableReference(DeviceDefinition)", "A reference to the linked device.", 0, 1, relatedDevice)); 4190 } 4191 4192 @Override 4193 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4194 switch (_hash) { 4195 case -554436100: /*relation*/ return new Property("relation", "Coding", "The type indicates the relationship of the related device to the device instance.", 0, 1, relation); 4196 case -296314271: /*relatedDevice*/ return new Property("relatedDevice", "CodeableReference(DeviceDefinition)", "A reference to the linked device.", 0, 1, relatedDevice); 4197 default: return super.getNamedProperty(_hash, _name, _checkValid); 4198 } 4199 4200 } 4201 4202 @Override 4203 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4204 switch (hash) { 4205 case -554436100: /*relation*/ return this.relation == null ? new Base[0] : new Base[] {this.relation}; // Coding 4206 case -296314271: /*relatedDevice*/ return this.relatedDevice == null ? new Base[0] : new Base[] {this.relatedDevice}; // CodeableReference 4207 default: return super.getProperty(hash, name, checkValid); 4208 } 4209 4210 } 4211 4212 @Override 4213 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4214 switch (hash) { 4215 case -554436100: // relation 4216 this.relation = TypeConvertor.castToCoding(value); // Coding 4217 return value; 4218 case -296314271: // relatedDevice 4219 this.relatedDevice = TypeConvertor.castToCodeableReference(value); // CodeableReference 4220 return value; 4221 default: return super.setProperty(hash, name, value); 4222 } 4223 4224 } 4225 4226 @Override 4227 public Base setProperty(String name, Base value) throws FHIRException { 4228 if (name.equals("relation")) { 4229 this.relation = TypeConvertor.castToCoding(value); // Coding 4230 } else if (name.equals("relatedDevice")) { 4231 this.relatedDevice = TypeConvertor.castToCodeableReference(value); // CodeableReference 4232 } else 4233 return super.setProperty(name, value); 4234 return value; 4235 } 4236 4237 @Override 4238 public void removeChild(String name, Base value) throws FHIRException { 4239 if (name.equals("relation")) { 4240 this.relation = null; 4241 } else if (name.equals("relatedDevice")) { 4242 this.relatedDevice = null; 4243 } else 4244 super.removeChild(name, value); 4245 4246 } 4247 4248 @Override 4249 public Base makeProperty(int hash, String name) throws FHIRException { 4250 switch (hash) { 4251 case -554436100: return getRelation(); 4252 case -296314271: return getRelatedDevice(); 4253 default: return super.makeProperty(hash, name); 4254 } 4255 4256 } 4257 4258 @Override 4259 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4260 switch (hash) { 4261 case -554436100: /*relation*/ return new String[] {"Coding"}; 4262 case -296314271: /*relatedDevice*/ return new String[] {"CodeableReference"}; 4263 default: return super.getTypesForProperty(hash, name); 4264 } 4265 4266 } 4267 4268 @Override 4269 public Base addChild(String name) throws FHIRException { 4270 if (name.equals("relation")) { 4271 this.relation = new Coding(); 4272 return this.relation; 4273 } 4274 else if (name.equals("relatedDevice")) { 4275 this.relatedDevice = new CodeableReference(); 4276 return this.relatedDevice; 4277 } 4278 else 4279 return super.addChild(name); 4280 } 4281 4282 public DeviceDefinitionLinkComponent copy() { 4283 DeviceDefinitionLinkComponent dst = new DeviceDefinitionLinkComponent(); 4284 copyValues(dst); 4285 return dst; 4286 } 4287 4288 public void copyValues(DeviceDefinitionLinkComponent dst) { 4289 super.copyValues(dst); 4290 dst.relation = relation == null ? null : relation.copy(); 4291 dst.relatedDevice = relatedDevice == null ? null : relatedDevice.copy(); 4292 } 4293 4294 @Override 4295 public boolean equalsDeep(Base other_) { 4296 if (!super.equalsDeep(other_)) 4297 return false; 4298 if (!(other_ instanceof DeviceDefinitionLinkComponent)) 4299 return false; 4300 DeviceDefinitionLinkComponent o = (DeviceDefinitionLinkComponent) other_; 4301 return compareDeep(relation, o.relation, true) && compareDeep(relatedDevice, o.relatedDevice, true) 4302 ; 4303 } 4304 4305 @Override 4306 public boolean equalsShallow(Base other_) { 4307 if (!super.equalsShallow(other_)) 4308 return false; 4309 if (!(other_ instanceof DeviceDefinitionLinkComponent)) 4310 return false; 4311 DeviceDefinitionLinkComponent o = (DeviceDefinitionLinkComponent) other_; 4312 return true; 4313 } 4314 4315 public boolean isEmpty() { 4316 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(relation, relatedDevice); 4317 } 4318 4319 public String fhirType() { 4320 return "DeviceDefinition.link"; 4321 4322 } 4323 4324 } 4325 4326 @Block() 4327 public static class DeviceDefinitionMaterialComponent extends BackboneElement implements IBaseBackboneElement { 4328 /** 4329 * A substance that the device contains, may contain, or is made of - for example latex - to be used to determine patient compatibility. This is not intended to represent the composition of the device, only the clinically relevant materials. 4330 */ 4331 @Child(name = "substance", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 4332 @Description(shortDefinition="A relevant substance that the device contains, may contain, or is made of", formalDefinition="A substance that the device contains, may contain, or is made of - for example latex - to be used to determine patient compatibility. This is not intended to represent the composition of the device, only the clinically relevant materials." ) 4333 protected CodeableConcept substance; 4334 4335 /** 4336 * Indicates an alternative material of the device. 4337 */ 4338 @Child(name = "alternate", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4339 @Description(shortDefinition="Indicates an alternative material of the device", formalDefinition="Indicates an alternative material of the device." ) 4340 protected BooleanType alternate; 4341 4342 /** 4343 * Whether the substance is a known or suspected allergen. 4344 */ 4345 @Child(name = "allergenicIndicator", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 4346 @Description(shortDefinition="Whether the substance is a known or suspected allergen", formalDefinition="Whether the substance is a known or suspected allergen." ) 4347 protected BooleanType allergenicIndicator; 4348 4349 private static final long serialVersionUID = 1232736508L; 4350 4351 /** 4352 * Constructor 4353 */ 4354 public DeviceDefinitionMaterialComponent() { 4355 super(); 4356 } 4357 4358 /** 4359 * Constructor 4360 */ 4361 public DeviceDefinitionMaterialComponent(CodeableConcept substance) { 4362 super(); 4363 this.setSubstance(substance); 4364 } 4365 4366 /** 4367 * @return {@link #substance} (A substance that the device contains, may contain, or is made of - for example latex - to be used to determine patient compatibility. This is not intended to represent the composition of the device, only the clinically relevant materials.) 4368 */ 4369 public CodeableConcept getSubstance() { 4370 if (this.substance == null) 4371 if (Configuration.errorOnAutoCreate()) 4372 throw new Error("Attempt to auto-create DeviceDefinitionMaterialComponent.substance"); 4373 else if (Configuration.doAutoCreate()) 4374 this.substance = new CodeableConcept(); // cc 4375 return this.substance; 4376 } 4377 4378 public boolean hasSubstance() { 4379 return this.substance != null && !this.substance.isEmpty(); 4380 } 4381 4382 /** 4383 * @param value {@link #substance} (A substance that the device contains, may contain, or is made of - for example latex - to be used to determine patient compatibility. This is not intended to represent the composition of the device, only the clinically relevant materials.) 4384 */ 4385 public DeviceDefinitionMaterialComponent setSubstance(CodeableConcept value) { 4386 this.substance = value; 4387 return this; 4388 } 4389 4390 /** 4391 * @return {@link #alternate} (Indicates an alternative material of the device.). This is the underlying object with id, value and extensions. The accessor "getAlternate" gives direct access to the value 4392 */ 4393 public BooleanType getAlternateElement() { 4394 if (this.alternate == null) 4395 if (Configuration.errorOnAutoCreate()) 4396 throw new Error("Attempt to auto-create DeviceDefinitionMaterialComponent.alternate"); 4397 else if (Configuration.doAutoCreate()) 4398 this.alternate = new BooleanType(); // bb 4399 return this.alternate; 4400 } 4401 4402 public boolean hasAlternateElement() { 4403 return this.alternate != null && !this.alternate.isEmpty(); 4404 } 4405 4406 public boolean hasAlternate() { 4407 return this.alternate != null && !this.alternate.isEmpty(); 4408 } 4409 4410 /** 4411 * @param value {@link #alternate} (Indicates an alternative material of the device.). This is the underlying object with id, value and extensions. The accessor "getAlternate" gives direct access to the value 4412 */ 4413 public DeviceDefinitionMaterialComponent setAlternateElement(BooleanType value) { 4414 this.alternate = value; 4415 return this; 4416 } 4417 4418 /** 4419 * @return Indicates an alternative material of the device. 4420 */ 4421 public boolean getAlternate() { 4422 return this.alternate == null || this.alternate.isEmpty() ? false : this.alternate.getValue(); 4423 } 4424 4425 /** 4426 * @param value Indicates an alternative material of the device. 4427 */ 4428 public DeviceDefinitionMaterialComponent setAlternate(boolean value) { 4429 if (this.alternate == null) 4430 this.alternate = new BooleanType(); 4431 this.alternate.setValue(value); 4432 return this; 4433 } 4434 4435 /** 4436 * @return {@link #allergenicIndicator} (Whether the substance is a known or suspected allergen.). This is the underlying object with id, value and extensions. The accessor "getAllergenicIndicator" gives direct access to the value 4437 */ 4438 public BooleanType getAllergenicIndicatorElement() { 4439 if (this.allergenicIndicator == null) 4440 if (Configuration.errorOnAutoCreate()) 4441 throw new Error("Attempt to auto-create DeviceDefinitionMaterialComponent.allergenicIndicator"); 4442 else if (Configuration.doAutoCreate()) 4443 this.allergenicIndicator = new BooleanType(); // bb 4444 return this.allergenicIndicator; 4445 } 4446 4447 public boolean hasAllergenicIndicatorElement() { 4448 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 4449 } 4450 4451 public boolean hasAllergenicIndicator() { 4452 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 4453 } 4454 4455 /** 4456 * @param value {@link #allergenicIndicator} (Whether the substance is a known or suspected allergen.). This is the underlying object with id, value and extensions. The accessor "getAllergenicIndicator" gives direct access to the value 4457 */ 4458 public DeviceDefinitionMaterialComponent setAllergenicIndicatorElement(BooleanType value) { 4459 this.allergenicIndicator = value; 4460 return this; 4461 } 4462 4463 /** 4464 * @return Whether the substance is a known or suspected allergen. 4465 */ 4466 public boolean getAllergenicIndicator() { 4467 return this.allergenicIndicator == null || this.allergenicIndicator.isEmpty() ? false : this.allergenicIndicator.getValue(); 4468 } 4469 4470 /** 4471 * @param value Whether the substance is a known or suspected allergen. 4472 */ 4473 public DeviceDefinitionMaterialComponent setAllergenicIndicator(boolean value) { 4474 if (this.allergenicIndicator == null) 4475 this.allergenicIndicator = new BooleanType(); 4476 this.allergenicIndicator.setValue(value); 4477 return this; 4478 } 4479 4480 protected void listChildren(List<Property> children) { 4481 super.listChildren(children); 4482 children.add(new Property("substance", "CodeableConcept", "A substance that the device contains, may contain, or is made of - for example latex - to be used to determine patient compatibility. This is not intended to represent the composition of the device, only the clinically relevant materials.", 0, 1, substance)); 4483 children.add(new Property("alternate", "boolean", "Indicates an alternative material of the device.", 0, 1, alternate)); 4484 children.add(new Property("allergenicIndicator", "boolean", "Whether the substance is a known or suspected allergen.", 0, 1, allergenicIndicator)); 4485 } 4486 4487 @Override 4488 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4489 switch (_hash) { 4490 case 530040176: /*substance*/ return new Property("substance", "CodeableConcept", "A substance that the device contains, may contain, or is made of - for example latex - to be used to determine patient compatibility. This is not intended to represent the composition of the device, only the clinically relevant materials.", 0, 1, substance); 4491 case -1408024454: /*alternate*/ return new Property("alternate", "boolean", "Indicates an alternative material of the device.", 0, 1, alternate); 4492 case 75406931: /*allergenicIndicator*/ return new Property("allergenicIndicator", "boolean", "Whether the substance is a known or suspected allergen.", 0, 1, allergenicIndicator); 4493 default: return super.getNamedProperty(_hash, _name, _checkValid); 4494 } 4495 4496 } 4497 4498 @Override 4499 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4500 switch (hash) { 4501 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // CodeableConcept 4502 case -1408024454: /*alternate*/ return this.alternate == null ? new Base[0] : new Base[] {this.alternate}; // BooleanType 4503 case 75406931: /*allergenicIndicator*/ return this.allergenicIndicator == null ? new Base[0] : new Base[] {this.allergenicIndicator}; // BooleanType 4504 default: return super.getProperty(hash, name, checkValid); 4505 } 4506 4507 } 4508 4509 @Override 4510 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4511 switch (hash) { 4512 case 530040176: // substance 4513 this.substance = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4514 return value; 4515 case -1408024454: // alternate 4516 this.alternate = TypeConvertor.castToBoolean(value); // BooleanType 4517 return value; 4518 case 75406931: // allergenicIndicator 4519 this.allergenicIndicator = TypeConvertor.castToBoolean(value); // BooleanType 4520 return value; 4521 default: return super.setProperty(hash, name, value); 4522 } 4523 4524 } 4525 4526 @Override 4527 public Base setProperty(String name, Base value) throws FHIRException { 4528 if (name.equals("substance")) { 4529 this.substance = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4530 } else if (name.equals("alternate")) { 4531 this.alternate = TypeConvertor.castToBoolean(value); // BooleanType 4532 } else if (name.equals("allergenicIndicator")) { 4533 this.allergenicIndicator = TypeConvertor.castToBoolean(value); // BooleanType 4534 } else 4535 return super.setProperty(name, value); 4536 return value; 4537 } 4538 4539 @Override 4540 public void removeChild(String name, Base value) throws FHIRException { 4541 if (name.equals("substance")) { 4542 this.substance = null; 4543 } else if (name.equals("alternate")) { 4544 this.alternate = null; 4545 } else if (name.equals("allergenicIndicator")) { 4546 this.allergenicIndicator = null; 4547 } else 4548 super.removeChild(name, value); 4549 4550 } 4551 4552 @Override 4553 public Base makeProperty(int hash, String name) throws FHIRException { 4554 switch (hash) { 4555 case 530040176: return getSubstance(); 4556 case -1408024454: return getAlternateElement(); 4557 case 75406931: return getAllergenicIndicatorElement(); 4558 default: return super.makeProperty(hash, name); 4559 } 4560 4561 } 4562 4563 @Override 4564 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4565 switch (hash) { 4566 case 530040176: /*substance*/ return new String[] {"CodeableConcept"}; 4567 case -1408024454: /*alternate*/ return new String[] {"boolean"}; 4568 case 75406931: /*allergenicIndicator*/ return new String[] {"boolean"}; 4569 default: return super.getTypesForProperty(hash, name); 4570 } 4571 4572 } 4573 4574 @Override 4575 public Base addChild(String name) throws FHIRException { 4576 if (name.equals("substance")) { 4577 this.substance = new CodeableConcept(); 4578 return this.substance; 4579 } 4580 else if (name.equals("alternate")) { 4581 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.material.alternate"); 4582 } 4583 else if (name.equals("allergenicIndicator")) { 4584 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.material.allergenicIndicator"); 4585 } 4586 else 4587 return super.addChild(name); 4588 } 4589 4590 public DeviceDefinitionMaterialComponent copy() { 4591 DeviceDefinitionMaterialComponent dst = new DeviceDefinitionMaterialComponent(); 4592 copyValues(dst); 4593 return dst; 4594 } 4595 4596 public void copyValues(DeviceDefinitionMaterialComponent dst) { 4597 super.copyValues(dst); 4598 dst.substance = substance == null ? null : substance.copy(); 4599 dst.alternate = alternate == null ? null : alternate.copy(); 4600 dst.allergenicIndicator = allergenicIndicator == null ? null : allergenicIndicator.copy(); 4601 } 4602 4603 @Override 4604 public boolean equalsDeep(Base other_) { 4605 if (!super.equalsDeep(other_)) 4606 return false; 4607 if (!(other_ instanceof DeviceDefinitionMaterialComponent)) 4608 return false; 4609 DeviceDefinitionMaterialComponent o = (DeviceDefinitionMaterialComponent) other_; 4610 return compareDeep(substance, o.substance, true) && compareDeep(alternate, o.alternate, true) && compareDeep(allergenicIndicator, o.allergenicIndicator, true) 4611 ; 4612 } 4613 4614 @Override 4615 public boolean equalsShallow(Base other_) { 4616 if (!super.equalsShallow(other_)) 4617 return false; 4618 if (!(other_ instanceof DeviceDefinitionMaterialComponent)) 4619 return false; 4620 DeviceDefinitionMaterialComponent o = (DeviceDefinitionMaterialComponent) other_; 4621 return compareValues(alternate, o.alternate, true) && compareValues(allergenicIndicator, o.allergenicIndicator, true) 4622 ; 4623 } 4624 4625 public boolean isEmpty() { 4626 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, alternate, allergenicIndicator 4627 ); 4628 } 4629 4630 public String fhirType() { 4631 return "DeviceDefinition.material"; 4632 4633 } 4634 4635 } 4636 4637 @Block() 4638 public static class DeviceDefinitionGuidelineComponent extends BackboneElement implements IBaseBackboneElement { 4639 /** 4640 * The circumstances that form the setting for using the device. 4641 */ 4642 @Child(name = "useContext", type = {UsageContext.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4643 @Description(shortDefinition="The circumstances that form the setting for using the device", formalDefinition="The circumstances that form the setting for using the device." ) 4644 protected List<UsageContext> useContext; 4645 4646 /** 4647 * Detailed written and visual directions for the user on how to use the device. 4648 */ 4649 @Child(name = "usageInstruction", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4650 @Description(shortDefinition="Detailed written and visual directions for the user on how to use the device", formalDefinition="Detailed written and visual directions for the user on how to use the device." ) 4651 protected MarkdownType usageInstruction; 4652 4653 /** 4654 * A source of information or reference for this guideline. 4655 */ 4656 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4657 @Description(shortDefinition="A source of information or reference for this guideline", formalDefinition="A source of information or reference for this guideline." ) 4658 protected List<RelatedArtifact> relatedArtifact; 4659 4660 /** 4661 * A clinical condition for which the device was designed to be used. 4662 */ 4663 @Child(name = "indication", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4664 @Description(shortDefinition="A clinical condition for which the device was designed to be used", formalDefinition="A clinical condition for which the device was designed to be used." ) 4665 protected List<CodeableConcept> indication; 4666 4667 /** 4668 * A specific situation when a device should not be used because it may cause harm. 4669 */ 4670 @Child(name = "contraindication", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4671 @Description(shortDefinition="A specific situation when a device should not be used because it may cause harm", formalDefinition="A specific situation when a device should not be used because it may cause harm." ) 4672 protected List<CodeableConcept> contraindication; 4673 4674 /** 4675 * Specific hazard alert information that a user needs to know before using the device. 4676 */ 4677 @Child(name = "warning", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4678 @Description(shortDefinition="Specific hazard alert information that a user needs to know before using the device", formalDefinition="Specific hazard alert information that a user needs to know before using the device." ) 4679 protected List<CodeableConcept> warning; 4680 4681 /** 4682 * A description of the general purpose or medical use of the device or its function. 4683 */ 4684 @Child(name = "intendedUse", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 4685 @Description(shortDefinition="A description of the general purpose or medical use of the device or its function", formalDefinition="A description of the general purpose or medical use of the device or its function." ) 4686 protected StringType intendedUse; 4687 4688 private static final long serialVersionUID = -1323961659L; 4689 4690 /** 4691 * Constructor 4692 */ 4693 public DeviceDefinitionGuidelineComponent() { 4694 super(); 4695 } 4696 4697 /** 4698 * @return {@link #useContext} (The circumstances that form the setting for using the device.) 4699 */ 4700 public List<UsageContext> getUseContext() { 4701 if (this.useContext == null) 4702 this.useContext = new ArrayList<UsageContext>(); 4703 return this.useContext; 4704 } 4705 4706 /** 4707 * @return Returns a reference to <code>this</code> for easy method chaining 4708 */ 4709 public DeviceDefinitionGuidelineComponent setUseContext(List<UsageContext> theUseContext) { 4710 this.useContext = theUseContext; 4711 return this; 4712 } 4713 4714 public boolean hasUseContext() { 4715 if (this.useContext == null) 4716 return false; 4717 for (UsageContext item : this.useContext) 4718 if (!item.isEmpty()) 4719 return true; 4720 return false; 4721 } 4722 4723 public UsageContext addUseContext() { //3 4724 UsageContext t = new UsageContext(); 4725 if (this.useContext == null) 4726 this.useContext = new ArrayList<UsageContext>(); 4727 this.useContext.add(t); 4728 return t; 4729 } 4730 4731 public DeviceDefinitionGuidelineComponent addUseContext(UsageContext t) { //3 4732 if (t == null) 4733 return this; 4734 if (this.useContext == null) 4735 this.useContext = new ArrayList<UsageContext>(); 4736 this.useContext.add(t); 4737 return this; 4738 } 4739 4740 /** 4741 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 4742 */ 4743 public UsageContext getUseContextFirstRep() { 4744 if (getUseContext().isEmpty()) { 4745 addUseContext(); 4746 } 4747 return getUseContext().get(0); 4748 } 4749 4750 /** 4751 * @return {@link #usageInstruction} (Detailed written and visual directions for the user on how to use the device.). This is the underlying object with id, value and extensions. The accessor "getUsageInstruction" gives direct access to the value 4752 */ 4753 public MarkdownType getUsageInstructionElement() { 4754 if (this.usageInstruction == null) 4755 if (Configuration.errorOnAutoCreate()) 4756 throw new Error("Attempt to auto-create DeviceDefinitionGuidelineComponent.usageInstruction"); 4757 else if (Configuration.doAutoCreate()) 4758 this.usageInstruction = new MarkdownType(); // bb 4759 return this.usageInstruction; 4760 } 4761 4762 public boolean hasUsageInstructionElement() { 4763 return this.usageInstruction != null && !this.usageInstruction.isEmpty(); 4764 } 4765 4766 public boolean hasUsageInstruction() { 4767 return this.usageInstruction != null && !this.usageInstruction.isEmpty(); 4768 } 4769 4770 /** 4771 * @param value {@link #usageInstruction} (Detailed written and visual directions for the user on how to use the device.). This is the underlying object with id, value and extensions. The accessor "getUsageInstruction" gives direct access to the value 4772 */ 4773 public DeviceDefinitionGuidelineComponent setUsageInstructionElement(MarkdownType value) { 4774 this.usageInstruction = value; 4775 return this; 4776 } 4777 4778 /** 4779 * @return Detailed written and visual directions for the user on how to use the device. 4780 */ 4781 public String getUsageInstruction() { 4782 return this.usageInstruction == null ? null : this.usageInstruction.getValue(); 4783 } 4784 4785 /** 4786 * @param value Detailed written and visual directions for the user on how to use the device. 4787 */ 4788 public DeviceDefinitionGuidelineComponent setUsageInstruction(String value) { 4789 if (Utilities.noString(value)) 4790 this.usageInstruction = null; 4791 else { 4792 if (this.usageInstruction == null) 4793 this.usageInstruction = new MarkdownType(); 4794 this.usageInstruction.setValue(value); 4795 } 4796 return this; 4797 } 4798 4799 /** 4800 * @return {@link #relatedArtifact} (A source of information or reference for this guideline.) 4801 */ 4802 public List<RelatedArtifact> getRelatedArtifact() { 4803 if (this.relatedArtifact == null) 4804 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4805 return this.relatedArtifact; 4806 } 4807 4808 /** 4809 * @return Returns a reference to <code>this</code> for easy method chaining 4810 */ 4811 public DeviceDefinitionGuidelineComponent setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 4812 this.relatedArtifact = theRelatedArtifact; 4813 return this; 4814 } 4815 4816 public boolean hasRelatedArtifact() { 4817 if (this.relatedArtifact == null) 4818 return false; 4819 for (RelatedArtifact item : this.relatedArtifact) 4820 if (!item.isEmpty()) 4821 return true; 4822 return false; 4823 } 4824 4825 public RelatedArtifact addRelatedArtifact() { //3 4826 RelatedArtifact t = new RelatedArtifact(); 4827 if (this.relatedArtifact == null) 4828 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4829 this.relatedArtifact.add(t); 4830 return t; 4831 } 4832 4833 public DeviceDefinitionGuidelineComponent addRelatedArtifact(RelatedArtifact t) { //3 4834 if (t == null) 4835 return this; 4836 if (this.relatedArtifact == null) 4837 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4838 this.relatedArtifact.add(t); 4839 return this; 4840 } 4841 4842 /** 4843 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 4844 */ 4845 public RelatedArtifact getRelatedArtifactFirstRep() { 4846 if (getRelatedArtifact().isEmpty()) { 4847 addRelatedArtifact(); 4848 } 4849 return getRelatedArtifact().get(0); 4850 } 4851 4852 /** 4853 * @return {@link #indication} (A clinical condition for which the device was designed to be used.) 4854 */ 4855 public List<CodeableConcept> getIndication() { 4856 if (this.indication == null) 4857 this.indication = new ArrayList<CodeableConcept>(); 4858 return this.indication; 4859 } 4860 4861 /** 4862 * @return Returns a reference to <code>this</code> for easy method chaining 4863 */ 4864 public DeviceDefinitionGuidelineComponent setIndication(List<CodeableConcept> theIndication) { 4865 this.indication = theIndication; 4866 return this; 4867 } 4868 4869 public boolean hasIndication() { 4870 if (this.indication == null) 4871 return false; 4872 for (CodeableConcept item : this.indication) 4873 if (!item.isEmpty()) 4874 return true; 4875 return false; 4876 } 4877 4878 public CodeableConcept addIndication() { //3 4879 CodeableConcept t = new CodeableConcept(); 4880 if (this.indication == null) 4881 this.indication = new ArrayList<CodeableConcept>(); 4882 this.indication.add(t); 4883 return t; 4884 } 4885 4886 public DeviceDefinitionGuidelineComponent addIndication(CodeableConcept t) { //3 4887 if (t == null) 4888 return this; 4889 if (this.indication == null) 4890 this.indication = new ArrayList<CodeableConcept>(); 4891 this.indication.add(t); 4892 return this; 4893 } 4894 4895 /** 4896 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist {3} 4897 */ 4898 public CodeableConcept getIndicationFirstRep() { 4899 if (getIndication().isEmpty()) { 4900 addIndication(); 4901 } 4902 return getIndication().get(0); 4903 } 4904 4905 /** 4906 * @return {@link #contraindication} (A specific situation when a device should not be used because it may cause harm.) 4907 */ 4908 public List<CodeableConcept> getContraindication() { 4909 if (this.contraindication == null) 4910 this.contraindication = new ArrayList<CodeableConcept>(); 4911 return this.contraindication; 4912 } 4913 4914 /** 4915 * @return Returns a reference to <code>this</code> for easy method chaining 4916 */ 4917 public DeviceDefinitionGuidelineComponent setContraindication(List<CodeableConcept> theContraindication) { 4918 this.contraindication = theContraindication; 4919 return this; 4920 } 4921 4922 public boolean hasContraindication() { 4923 if (this.contraindication == null) 4924 return false; 4925 for (CodeableConcept item : this.contraindication) 4926 if (!item.isEmpty()) 4927 return true; 4928 return false; 4929 } 4930 4931 public CodeableConcept addContraindication() { //3 4932 CodeableConcept t = new CodeableConcept(); 4933 if (this.contraindication == null) 4934 this.contraindication = new ArrayList<CodeableConcept>(); 4935 this.contraindication.add(t); 4936 return t; 4937 } 4938 4939 public DeviceDefinitionGuidelineComponent addContraindication(CodeableConcept t) { //3 4940 if (t == null) 4941 return this; 4942 if (this.contraindication == null) 4943 this.contraindication = new ArrayList<CodeableConcept>(); 4944 this.contraindication.add(t); 4945 return this; 4946 } 4947 4948 /** 4949 * @return The first repetition of repeating field {@link #contraindication}, creating it if it does not already exist {3} 4950 */ 4951 public CodeableConcept getContraindicationFirstRep() { 4952 if (getContraindication().isEmpty()) { 4953 addContraindication(); 4954 } 4955 return getContraindication().get(0); 4956 } 4957 4958 /** 4959 * @return {@link #warning} (Specific hazard alert information that a user needs to know before using the device.) 4960 */ 4961 public List<CodeableConcept> getWarning() { 4962 if (this.warning == null) 4963 this.warning = new ArrayList<CodeableConcept>(); 4964 return this.warning; 4965 } 4966 4967 /** 4968 * @return Returns a reference to <code>this</code> for easy method chaining 4969 */ 4970 public DeviceDefinitionGuidelineComponent setWarning(List<CodeableConcept> theWarning) { 4971 this.warning = theWarning; 4972 return this; 4973 } 4974 4975 public boolean hasWarning() { 4976 if (this.warning == null) 4977 return false; 4978 for (CodeableConcept item : this.warning) 4979 if (!item.isEmpty()) 4980 return true; 4981 return false; 4982 } 4983 4984 public CodeableConcept addWarning() { //3 4985 CodeableConcept t = new CodeableConcept(); 4986 if (this.warning == null) 4987 this.warning = new ArrayList<CodeableConcept>(); 4988 this.warning.add(t); 4989 return t; 4990 } 4991 4992 public DeviceDefinitionGuidelineComponent addWarning(CodeableConcept t) { //3 4993 if (t == null) 4994 return this; 4995 if (this.warning == null) 4996 this.warning = new ArrayList<CodeableConcept>(); 4997 this.warning.add(t); 4998 return this; 4999 } 5000 5001 /** 5002 * @return The first repetition of repeating field {@link #warning}, creating it if it does not already exist {3} 5003 */ 5004 public CodeableConcept getWarningFirstRep() { 5005 if (getWarning().isEmpty()) { 5006 addWarning(); 5007 } 5008 return getWarning().get(0); 5009 } 5010 5011 /** 5012 * @return {@link #intendedUse} (A description of the general purpose or medical use of the device or its function.). This is the underlying object with id, value and extensions. The accessor "getIntendedUse" gives direct access to the value 5013 */ 5014 public StringType getIntendedUseElement() { 5015 if (this.intendedUse == null) 5016 if (Configuration.errorOnAutoCreate()) 5017 throw new Error("Attempt to auto-create DeviceDefinitionGuidelineComponent.intendedUse"); 5018 else if (Configuration.doAutoCreate()) 5019 this.intendedUse = new StringType(); // bb 5020 return this.intendedUse; 5021 } 5022 5023 public boolean hasIntendedUseElement() { 5024 return this.intendedUse != null && !this.intendedUse.isEmpty(); 5025 } 5026 5027 public boolean hasIntendedUse() { 5028 return this.intendedUse != null && !this.intendedUse.isEmpty(); 5029 } 5030 5031 /** 5032 * @param value {@link #intendedUse} (A description of the general purpose or medical use of the device or its function.). This is the underlying object with id, value and extensions. The accessor "getIntendedUse" gives direct access to the value 5033 */ 5034 public DeviceDefinitionGuidelineComponent setIntendedUseElement(StringType value) { 5035 this.intendedUse = value; 5036 return this; 5037 } 5038 5039 /** 5040 * @return A description of the general purpose or medical use of the device or its function. 5041 */ 5042 public String getIntendedUse() { 5043 return this.intendedUse == null ? null : this.intendedUse.getValue(); 5044 } 5045 5046 /** 5047 * @param value A description of the general purpose or medical use of the device or its function. 5048 */ 5049 public DeviceDefinitionGuidelineComponent setIntendedUse(String value) { 5050 if (Utilities.noString(value)) 5051 this.intendedUse = null; 5052 else { 5053 if (this.intendedUse == null) 5054 this.intendedUse = new StringType(); 5055 this.intendedUse.setValue(value); 5056 } 5057 return this; 5058 } 5059 5060 protected void listChildren(List<Property> children) { 5061 super.listChildren(children); 5062 children.add(new Property("useContext", "UsageContext", "The circumstances that form the setting for using the device.", 0, java.lang.Integer.MAX_VALUE, useContext)); 5063 children.add(new Property("usageInstruction", "markdown", "Detailed written and visual directions for the user on how to use the device.", 0, 1, usageInstruction)); 5064 children.add(new Property("relatedArtifact", "RelatedArtifact", "A source of information or reference for this guideline.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 5065 children.add(new Property("indication", "CodeableConcept", "A clinical condition for which the device was designed to be used.", 0, java.lang.Integer.MAX_VALUE, indication)); 5066 children.add(new Property("contraindication", "CodeableConcept", "A specific situation when a device should not be used because it may cause harm.", 0, java.lang.Integer.MAX_VALUE, contraindication)); 5067 children.add(new Property("warning", "CodeableConcept", "Specific hazard alert information that a user needs to know before using the device.", 0, java.lang.Integer.MAX_VALUE, warning)); 5068 children.add(new Property("intendedUse", "string", "A description of the general purpose or medical use of the device or its function.", 0, 1, intendedUse)); 5069 } 5070 5071 @Override 5072 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5073 switch (_hash) { 5074 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The circumstances that form the setting for using the device.", 0, java.lang.Integer.MAX_VALUE, useContext); 5075 case 2138372141: /*usageInstruction*/ return new Property("usageInstruction", "markdown", "Detailed written and visual directions for the user on how to use the device.", 0, 1, usageInstruction); 5076 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "A source of information or reference for this guideline.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 5077 case -597168804: /*indication*/ return new Property("indication", "CodeableConcept", "A clinical condition for which the device was designed to be used.", 0, java.lang.Integer.MAX_VALUE, indication); 5078 case 107135229: /*contraindication*/ return new Property("contraindication", "CodeableConcept", "A specific situation when a device should not be used because it may cause harm.", 0, java.lang.Integer.MAX_VALUE, contraindication); 5079 case 1124446108: /*warning*/ return new Property("warning", "CodeableConcept", "Specific hazard alert information that a user needs to know before using the device.", 0, java.lang.Integer.MAX_VALUE, warning); 5080 case -1618671268: /*intendedUse*/ return new Property("intendedUse", "string", "A description of the general purpose or medical use of the device or its function.", 0, 1, intendedUse); 5081 default: return super.getNamedProperty(_hash, _name, _checkValid); 5082 } 5083 5084 } 5085 5086 @Override 5087 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5088 switch (hash) { 5089 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5090 case 2138372141: /*usageInstruction*/ return this.usageInstruction == null ? new Base[0] : new Base[] {this.usageInstruction}; // MarkdownType 5091 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5092 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // CodeableConcept 5093 case 107135229: /*contraindication*/ return this.contraindication == null ? new Base[0] : this.contraindication.toArray(new Base[this.contraindication.size()]); // CodeableConcept 5094 case 1124446108: /*warning*/ return this.warning == null ? new Base[0] : this.warning.toArray(new Base[this.warning.size()]); // CodeableConcept 5095 case -1618671268: /*intendedUse*/ return this.intendedUse == null ? new Base[0] : new Base[] {this.intendedUse}; // StringType 5096 default: return super.getProperty(hash, name, checkValid); 5097 } 5098 5099 } 5100 5101 @Override 5102 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5103 switch (hash) { 5104 case -669707736: // useContext 5105 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5106 return value; 5107 case 2138372141: // usageInstruction 5108 this.usageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 5109 return value; 5110 case 666807069: // relatedArtifact 5111 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 5112 return value; 5113 case -597168804: // indication 5114 this.getIndication().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5115 return value; 5116 case 107135229: // contraindication 5117 this.getContraindication().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5118 return value; 5119 case 1124446108: // warning 5120 this.getWarning().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5121 return value; 5122 case -1618671268: // intendedUse 5123 this.intendedUse = TypeConvertor.castToString(value); // StringType 5124 return value; 5125 default: return super.setProperty(hash, name, value); 5126 } 5127 5128 } 5129 5130 @Override 5131 public Base setProperty(String name, Base value) throws FHIRException { 5132 if (name.equals("useContext")) { 5133 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 5134 } else if (name.equals("usageInstruction")) { 5135 this.usageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 5136 } else if (name.equals("relatedArtifact")) { 5137 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 5138 } else if (name.equals("indication")) { 5139 this.getIndication().add(TypeConvertor.castToCodeableConcept(value)); 5140 } else if (name.equals("contraindication")) { 5141 this.getContraindication().add(TypeConvertor.castToCodeableConcept(value)); 5142 } else if (name.equals("warning")) { 5143 this.getWarning().add(TypeConvertor.castToCodeableConcept(value)); 5144 } else if (name.equals("intendedUse")) { 5145 this.intendedUse = TypeConvertor.castToString(value); // StringType 5146 } else 5147 return super.setProperty(name, value); 5148 return value; 5149 } 5150 5151 @Override 5152 public void removeChild(String name, Base value) throws FHIRException { 5153 if (name.equals("useContext")) { 5154 this.getUseContext().remove(value); 5155 } else if (name.equals("usageInstruction")) { 5156 this.usageInstruction = null; 5157 } else if (name.equals("relatedArtifact")) { 5158 this.getRelatedArtifact().remove(value); 5159 } else if (name.equals("indication")) { 5160 this.getIndication().remove(value); 5161 } else if (name.equals("contraindication")) { 5162 this.getContraindication().remove(value); 5163 } else if (name.equals("warning")) { 5164 this.getWarning().remove(value); 5165 } else if (name.equals("intendedUse")) { 5166 this.intendedUse = null; 5167 } else 5168 super.removeChild(name, value); 5169 5170 } 5171 5172 @Override 5173 public Base makeProperty(int hash, String name) throws FHIRException { 5174 switch (hash) { 5175 case -669707736: return addUseContext(); 5176 case 2138372141: return getUsageInstructionElement(); 5177 case 666807069: return addRelatedArtifact(); 5178 case -597168804: return addIndication(); 5179 case 107135229: return addContraindication(); 5180 case 1124446108: return addWarning(); 5181 case -1618671268: return getIntendedUseElement(); 5182 default: return super.makeProperty(hash, name); 5183 } 5184 5185 } 5186 5187 @Override 5188 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5189 switch (hash) { 5190 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5191 case 2138372141: /*usageInstruction*/ return new String[] {"markdown"}; 5192 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 5193 case -597168804: /*indication*/ return new String[] {"CodeableConcept"}; 5194 case 107135229: /*contraindication*/ return new String[] {"CodeableConcept"}; 5195 case 1124446108: /*warning*/ return new String[] {"CodeableConcept"}; 5196 case -1618671268: /*intendedUse*/ return new String[] {"string"}; 5197 default: return super.getTypesForProperty(hash, name); 5198 } 5199 5200 } 5201 5202 @Override 5203 public Base addChild(String name) throws FHIRException { 5204 if (name.equals("useContext")) { 5205 return addUseContext(); 5206 } 5207 else if (name.equals("usageInstruction")) { 5208 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.guideline.usageInstruction"); 5209 } 5210 else if (name.equals("relatedArtifact")) { 5211 return addRelatedArtifact(); 5212 } 5213 else if (name.equals("indication")) { 5214 return addIndication(); 5215 } 5216 else if (name.equals("contraindication")) { 5217 return addContraindication(); 5218 } 5219 else if (name.equals("warning")) { 5220 return addWarning(); 5221 } 5222 else if (name.equals("intendedUse")) { 5223 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.guideline.intendedUse"); 5224 } 5225 else 5226 return super.addChild(name); 5227 } 5228 5229 public DeviceDefinitionGuidelineComponent copy() { 5230 DeviceDefinitionGuidelineComponent dst = new DeviceDefinitionGuidelineComponent(); 5231 copyValues(dst); 5232 return dst; 5233 } 5234 5235 public void copyValues(DeviceDefinitionGuidelineComponent dst) { 5236 super.copyValues(dst); 5237 if (useContext != null) { 5238 dst.useContext = new ArrayList<UsageContext>(); 5239 for (UsageContext i : useContext) 5240 dst.useContext.add(i.copy()); 5241 }; 5242 dst.usageInstruction = usageInstruction == null ? null : usageInstruction.copy(); 5243 if (relatedArtifact != null) { 5244 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5245 for (RelatedArtifact i : relatedArtifact) 5246 dst.relatedArtifact.add(i.copy()); 5247 }; 5248 if (indication != null) { 5249 dst.indication = new ArrayList<CodeableConcept>(); 5250 for (CodeableConcept i : indication) 5251 dst.indication.add(i.copy()); 5252 }; 5253 if (contraindication != null) { 5254 dst.contraindication = new ArrayList<CodeableConcept>(); 5255 for (CodeableConcept i : contraindication) 5256 dst.contraindication.add(i.copy()); 5257 }; 5258 if (warning != null) { 5259 dst.warning = new ArrayList<CodeableConcept>(); 5260 for (CodeableConcept i : warning) 5261 dst.warning.add(i.copy()); 5262 }; 5263 dst.intendedUse = intendedUse == null ? null : intendedUse.copy(); 5264 } 5265 5266 @Override 5267 public boolean equalsDeep(Base other_) { 5268 if (!super.equalsDeep(other_)) 5269 return false; 5270 if (!(other_ instanceof DeviceDefinitionGuidelineComponent)) 5271 return false; 5272 DeviceDefinitionGuidelineComponent o = (DeviceDefinitionGuidelineComponent) other_; 5273 return compareDeep(useContext, o.useContext, true) && compareDeep(usageInstruction, o.usageInstruction, true) 5274 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(indication, o.indication, true) 5275 && compareDeep(contraindication, o.contraindication, true) && compareDeep(warning, o.warning, true) 5276 && compareDeep(intendedUse, o.intendedUse, true); 5277 } 5278 5279 @Override 5280 public boolean equalsShallow(Base other_) { 5281 if (!super.equalsShallow(other_)) 5282 return false; 5283 if (!(other_ instanceof DeviceDefinitionGuidelineComponent)) 5284 return false; 5285 DeviceDefinitionGuidelineComponent o = (DeviceDefinitionGuidelineComponent) other_; 5286 return compareValues(usageInstruction, o.usageInstruction, true) && compareValues(intendedUse, o.intendedUse, true) 5287 ; 5288 } 5289 5290 public boolean isEmpty() { 5291 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(useContext, usageInstruction 5292 , relatedArtifact, indication, contraindication, warning, intendedUse); 5293 } 5294 5295 public String fhirType() { 5296 return "DeviceDefinition.guideline"; 5297 5298 } 5299 5300 } 5301 5302 @Block() 5303 public static class DeviceDefinitionCorrectiveActionComponent extends BackboneElement implements IBaseBackboneElement { 5304 /** 5305 * Whether the last corrective action known for this device was a recall. 5306 */ 5307 @Child(name = "recall", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5308 @Description(shortDefinition="Whether the corrective action was a recall", formalDefinition="Whether the last corrective action known for this device was a recall." ) 5309 protected BooleanType recall; 5310 5311 /** 5312 * The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name. 5313 */ 5314 @Child(name = "scope", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5315 @Description(shortDefinition="model | lot-numbers | serial-numbers", formalDefinition="The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name." ) 5316 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-correctiveactionscope") 5317 protected Enumeration<DeviceCorrectiveActionScope> scope; 5318 5319 /** 5320 * Start and end dates of the corrective action. 5321 */ 5322 @Child(name = "period", type = {Period.class}, order=3, min=1, max=1, modifier=false, summary=false) 5323 @Description(shortDefinition="Start and end dates of the corrective action", formalDefinition="Start and end dates of the corrective action." ) 5324 protected Period period; 5325 5326 private static final long serialVersionUID = -1936691252L; 5327 5328 /** 5329 * Constructor 5330 */ 5331 public DeviceDefinitionCorrectiveActionComponent() { 5332 super(); 5333 } 5334 5335 /** 5336 * Constructor 5337 */ 5338 public DeviceDefinitionCorrectiveActionComponent(boolean recall, Period period) { 5339 super(); 5340 this.setRecall(recall); 5341 this.setPeriod(period); 5342 } 5343 5344 /** 5345 * @return {@link #recall} (Whether the last corrective action known for this device was a recall.). This is the underlying object with id, value and extensions. The accessor "getRecall" gives direct access to the value 5346 */ 5347 public BooleanType getRecallElement() { 5348 if (this.recall == null) 5349 if (Configuration.errorOnAutoCreate()) 5350 throw new Error("Attempt to auto-create DeviceDefinitionCorrectiveActionComponent.recall"); 5351 else if (Configuration.doAutoCreate()) 5352 this.recall = new BooleanType(); // bb 5353 return this.recall; 5354 } 5355 5356 public boolean hasRecallElement() { 5357 return this.recall != null && !this.recall.isEmpty(); 5358 } 5359 5360 public boolean hasRecall() { 5361 return this.recall != null && !this.recall.isEmpty(); 5362 } 5363 5364 /** 5365 * @param value {@link #recall} (Whether the last corrective action known for this device was a recall.). This is the underlying object with id, value and extensions. The accessor "getRecall" gives direct access to the value 5366 */ 5367 public DeviceDefinitionCorrectiveActionComponent setRecallElement(BooleanType value) { 5368 this.recall = value; 5369 return this; 5370 } 5371 5372 /** 5373 * @return Whether the last corrective action known for this device was a recall. 5374 */ 5375 public boolean getRecall() { 5376 return this.recall == null || this.recall.isEmpty() ? false : this.recall.getValue(); 5377 } 5378 5379 /** 5380 * @param value Whether the last corrective action known for this device was a recall. 5381 */ 5382 public DeviceDefinitionCorrectiveActionComponent setRecall(boolean value) { 5383 if (this.recall == null) 5384 this.recall = new BooleanType(); 5385 this.recall.setValue(value); 5386 return this; 5387 } 5388 5389 /** 5390 * @return {@link #scope} (The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name.). This is the underlying object with id, value and extensions. The accessor "getScope" gives direct access to the value 5391 */ 5392 public Enumeration<DeviceCorrectiveActionScope> getScopeElement() { 5393 if (this.scope == null) 5394 if (Configuration.errorOnAutoCreate()) 5395 throw new Error("Attempt to auto-create DeviceDefinitionCorrectiveActionComponent.scope"); 5396 else if (Configuration.doAutoCreate()) 5397 this.scope = new Enumeration<DeviceCorrectiveActionScope>(new DeviceCorrectiveActionScopeEnumFactory()); // bb 5398 return this.scope; 5399 } 5400 5401 public boolean hasScopeElement() { 5402 return this.scope != null && !this.scope.isEmpty(); 5403 } 5404 5405 public boolean hasScope() { 5406 return this.scope != null && !this.scope.isEmpty(); 5407 } 5408 5409 /** 5410 * @param value {@link #scope} (The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name.). This is the underlying object with id, value and extensions. The accessor "getScope" gives direct access to the value 5411 */ 5412 public DeviceDefinitionCorrectiveActionComponent setScopeElement(Enumeration<DeviceCorrectiveActionScope> value) { 5413 this.scope = value; 5414 return this; 5415 } 5416 5417 /** 5418 * @return The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name. 5419 */ 5420 public DeviceCorrectiveActionScope getScope() { 5421 return this.scope == null ? null : this.scope.getValue(); 5422 } 5423 5424 /** 5425 * @param value The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name. 5426 */ 5427 public DeviceDefinitionCorrectiveActionComponent setScope(DeviceCorrectiveActionScope value) { 5428 if (value == null) 5429 this.scope = null; 5430 else { 5431 if (this.scope == null) 5432 this.scope = new Enumeration<DeviceCorrectiveActionScope>(new DeviceCorrectiveActionScopeEnumFactory()); 5433 this.scope.setValue(value); 5434 } 5435 return this; 5436 } 5437 5438 /** 5439 * @return {@link #period} (Start and end dates of the corrective action.) 5440 */ 5441 public Period getPeriod() { 5442 if (this.period == null) 5443 if (Configuration.errorOnAutoCreate()) 5444 throw new Error("Attempt to auto-create DeviceDefinitionCorrectiveActionComponent.period"); 5445 else if (Configuration.doAutoCreate()) 5446 this.period = new Period(); // cc 5447 return this.period; 5448 } 5449 5450 public boolean hasPeriod() { 5451 return this.period != null && !this.period.isEmpty(); 5452 } 5453 5454 /** 5455 * @param value {@link #period} (Start and end dates of the corrective action.) 5456 */ 5457 public DeviceDefinitionCorrectiveActionComponent setPeriod(Period value) { 5458 this.period = value; 5459 return this; 5460 } 5461 5462 protected void listChildren(List<Property> children) { 5463 super.listChildren(children); 5464 children.add(new Property("recall", "boolean", "Whether the last corrective action known for this device was a recall.", 0, 1, recall)); 5465 children.add(new Property("scope", "code", "The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name.", 0, 1, scope)); 5466 children.add(new Property("period", "Period", "Start and end dates of the corrective action.", 0, 1, period)); 5467 } 5468 5469 @Override 5470 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5471 switch (_hash) { 5472 case -934922479: /*recall*/ return new Property("recall", "boolean", "Whether the last corrective action known for this device was a recall.", 0, 1, recall); 5473 case 109264468: /*scope*/ return new Property("scope", "code", "The scope of the corrective action - whether the action targeted all units of a given device model, or only a specific set of batches identified by lot numbers, or individually identified devices identified by the serial name.", 0, 1, scope); 5474 case -991726143: /*period*/ return new Property("period", "Period", "Start and end dates of the corrective action.", 0, 1, period); 5475 default: return super.getNamedProperty(_hash, _name, _checkValid); 5476 } 5477 5478 } 5479 5480 @Override 5481 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5482 switch (hash) { 5483 case -934922479: /*recall*/ return this.recall == null ? new Base[0] : new Base[] {this.recall}; // BooleanType 5484 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : new Base[] {this.scope}; // Enumeration<DeviceCorrectiveActionScope> 5485 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 5486 default: return super.getProperty(hash, name, checkValid); 5487 } 5488 5489 } 5490 5491 @Override 5492 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5493 switch (hash) { 5494 case -934922479: // recall 5495 this.recall = TypeConvertor.castToBoolean(value); // BooleanType 5496 return value; 5497 case 109264468: // scope 5498 value = new DeviceCorrectiveActionScopeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5499 this.scope = (Enumeration) value; // Enumeration<DeviceCorrectiveActionScope> 5500 return value; 5501 case -991726143: // period 5502 this.period = TypeConvertor.castToPeriod(value); // Period 5503 return value; 5504 default: return super.setProperty(hash, name, value); 5505 } 5506 5507 } 5508 5509 @Override 5510 public Base setProperty(String name, Base value) throws FHIRException { 5511 if (name.equals("recall")) { 5512 this.recall = TypeConvertor.castToBoolean(value); // BooleanType 5513 } else if (name.equals("scope")) { 5514 value = new DeviceCorrectiveActionScopeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5515 this.scope = (Enumeration) value; // Enumeration<DeviceCorrectiveActionScope> 5516 } else if (name.equals("period")) { 5517 this.period = TypeConvertor.castToPeriod(value); // Period 5518 } else 5519 return super.setProperty(name, value); 5520 return value; 5521 } 5522 5523 @Override 5524 public void removeChild(String name, Base value) throws FHIRException { 5525 if (name.equals("recall")) { 5526 this.recall = null; 5527 } else if (name.equals("scope")) { 5528 value = new DeviceCorrectiveActionScopeEnumFactory().fromType(TypeConvertor.castToCode(value)); 5529 this.scope = (Enumeration) value; // Enumeration<DeviceCorrectiveActionScope> 5530 } else if (name.equals("period")) { 5531 this.period = null; 5532 } else 5533 super.removeChild(name, value); 5534 5535 } 5536 5537 @Override 5538 public Base makeProperty(int hash, String name) throws FHIRException { 5539 switch (hash) { 5540 case -934922479: return getRecallElement(); 5541 case 109264468: return getScopeElement(); 5542 case -991726143: return getPeriod(); 5543 default: return super.makeProperty(hash, name); 5544 } 5545 5546 } 5547 5548 @Override 5549 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5550 switch (hash) { 5551 case -934922479: /*recall*/ return new String[] {"boolean"}; 5552 case 109264468: /*scope*/ return new String[] {"code"}; 5553 case -991726143: /*period*/ return new String[] {"Period"}; 5554 default: return super.getTypesForProperty(hash, name); 5555 } 5556 5557 } 5558 5559 @Override 5560 public Base addChild(String name) throws FHIRException { 5561 if (name.equals("recall")) { 5562 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.correctiveAction.recall"); 5563 } 5564 else if (name.equals("scope")) { 5565 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.correctiveAction.scope"); 5566 } 5567 else if (name.equals("period")) { 5568 this.period = new Period(); 5569 return this.period; 5570 } 5571 else 5572 return super.addChild(name); 5573 } 5574 5575 public DeviceDefinitionCorrectiveActionComponent copy() { 5576 DeviceDefinitionCorrectiveActionComponent dst = new DeviceDefinitionCorrectiveActionComponent(); 5577 copyValues(dst); 5578 return dst; 5579 } 5580 5581 public void copyValues(DeviceDefinitionCorrectiveActionComponent dst) { 5582 super.copyValues(dst); 5583 dst.recall = recall == null ? null : recall.copy(); 5584 dst.scope = scope == null ? null : scope.copy(); 5585 dst.period = period == null ? null : period.copy(); 5586 } 5587 5588 @Override 5589 public boolean equalsDeep(Base other_) { 5590 if (!super.equalsDeep(other_)) 5591 return false; 5592 if (!(other_ instanceof DeviceDefinitionCorrectiveActionComponent)) 5593 return false; 5594 DeviceDefinitionCorrectiveActionComponent o = (DeviceDefinitionCorrectiveActionComponent) other_; 5595 return compareDeep(recall, o.recall, true) && compareDeep(scope, o.scope, true) && compareDeep(period, o.period, true) 5596 ; 5597 } 5598 5599 @Override 5600 public boolean equalsShallow(Base other_) { 5601 if (!super.equalsShallow(other_)) 5602 return false; 5603 if (!(other_ instanceof DeviceDefinitionCorrectiveActionComponent)) 5604 return false; 5605 DeviceDefinitionCorrectiveActionComponent o = (DeviceDefinitionCorrectiveActionComponent) other_; 5606 return compareValues(recall, o.recall, true) && compareValues(scope, o.scope, true); 5607 } 5608 5609 public boolean isEmpty() { 5610 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(recall, scope, period); 5611 } 5612 5613 public String fhirType() { 5614 return "DeviceDefinition.correctiveAction"; 5615 5616 } 5617 5618 } 5619 5620 @Block() 5621 public static class DeviceDefinitionChargeItemComponent extends BackboneElement implements IBaseBackboneElement { 5622 /** 5623 * The code or reference for the charge item. 5624 */ 5625 @Child(name = "chargeItemCode", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=false) 5626 @Description(shortDefinition="The code or reference for the charge item", formalDefinition="The code or reference for the charge item." ) 5627 protected CodeableReference chargeItemCode; 5628 5629 /** 5630 * Coefficient applicable to the billing code. 5631 */ 5632 @Child(name = "count", type = {Quantity.class}, order=2, min=1, max=1, modifier=false, summary=false) 5633 @Description(shortDefinition="Coefficient applicable to the billing code", formalDefinition="Coefficient applicable to the billing code." ) 5634 protected Quantity count; 5635 5636 /** 5637 * A specific time period in which this charge item applies. 5638 */ 5639 @Child(name = "effectivePeriod", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 5640 @Description(shortDefinition="A specific time period in which this charge item applies", formalDefinition="A specific time period in which this charge item applies." ) 5641 protected Period effectivePeriod; 5642 5643 /** 5644 * The context to which this charge item applies. 5645 */ 5646 @Child(name = "useContext", type = {UsageContext.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5647 @Description(shortDefinition="The context to which this charge item applies", formalDefinition="The context to which this charge item applies." ) 5648 protected List<UsageContext> useContext; 5649 5650 private static final long serialVersionUID = 1312166907L; 5651 5652 /** 5653 * Constructor 5654 */ 5655 public DeviceDefinitionChargeItemComponent() { 5656 super(); 5657 } 5658 5659 /** 5660 * Constructor 5661 */ 5662 public DeviceDefinitionChargeItemComponent(CodeableReference chargeItemCode, Quantity count) { 5663 super(); 5664 this.setChargeItemCode(chargeItemCode); 5665 this.setCount(count); 5666 } 5667 5668 /** 5669 * @return {@link #chargeItemCode} (The code or reference for the charge item.) 5670 */ 5671 public CodeableReference getChargeItemCode() { 5672 if (this.chargeItemCode == null) 5673 if (Configuration.errorOnAutoCreate()) 5674 throw new Error("Attempt to auto-create DeviceDefinitionChargeItemComponent.chargeItemCode"); 5675 else if (Configuration.doAutoCreate()) 5676 this.chargeItemCode = new CodeableReference(); // cc 5677 return this.chargeItemCode; 5678 } 5679 5680 public boolean hasChargeItemCode() { 5681 return this.chargeItemCode != null && !this.chargeItemCode.isEmpty(); 5682 } 5683 5684 /** 5685 * @param value {@link #chargeItemCode} (The code or reference for the charge item.) 5686 */ 5687 public DeviceDefinitionChargeItemComponent setChargeItemCode(CodeableReference value) { 5688 this.chargeItemCode = value; 5689 return this; 5690 } 5691 5692 /** 5693 * @return {@link #count} (Coefficient applicable to the billing code.) 5694 */ 5695 public Quantity getCount() { 5696 if (this.count == null) 5697 if (Configuration.errorOnAutoCreate()) 5698 throw new Error("Attempt to auto-create DeviceDefinitionChargeItemComponent.count"); 5699 else if (Configuration.doAutoCreate()) 5700 this.count = new Quantity(); // cc 5701 return this.count; 5702 } 5703 5704 public boolean hasCount() { 5705 return this.count != null && !this.count.isEmpty(); 5706 } 5707 5708 /** 5709 * @param value {@link #count} (Coefficient applicable to the billing code.) 5710 */ 5711 public DeviceDefinitionChargeItemComponent setCount(Quantity value) { 5712 this.count = value; 5713 return this; 5714 } 5715 5716 /** 5717 * @return {@link #effectivePeriod} (A specific time period in which this charge item applies.) 5718 */ 5719 public Period getEffectivePeriod() { 5720 if (this.effectivePeriod == null) 5721 if (Configuration.errorOnAutoCreate()) 5722 throw new Error("Attempt to auto-create DeviceDefinitionChargeItemComponent.effectivePeriod"); 5723 else if (Configuration.doAutoCreate()) 5724 this.effectivePeriod = new Period(); // cc 5725 return this.effectivePeriod; 5726 } 5727 5728 public boolean hasEffectivePeriod() { 5729 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 5730 } 5731 5732 /** 5733 * @param value {@link #effectivePeriod} (A specific time period in which this charge item applies.) 5734 */ 5735 public DeviceDefinitionChargeItemComponent setEffectivePeriod(Period value) { 5736 this.effectivePeriod = value; 5737 return this; 5738 } 5739 5740 /** 5741 * @return {@link #useContext} (The context to which this charge item applies.) 5742 */ 5743 public List<UsageContext> getUseContext() { 5744 if (this.useContext == null) 5745 this.useContext = new ArrayList<UsageContext>(); 5746 return this.useContext; 5747 } 5748 5749 /** 5750 * @return Returns a reference to <code>this</code> for easy method chaining 5751 */ 5752 public DeviceDefinitionChargeItemComponent setUseContext(List<UsageContext> theUseContext) { 5753 this.useContext = theUseContext; 5754 return this; 5755 } 5756 5757 public boolean hasUseContext() { 5758 if (this.useContext == null) 5759 return false; 5760 for (UsageContext item : this.useContext) 5761 if (!item.isEmpty()) 5762 return true; 5763 return false; 5764 } 5765 5766 public UsageContext addUseContext() { //3 5767 UsageContext t = new UsageContext(); 5768 if (this.useContext == null) 5769 this.useContext = new ArrayList<UsageContext>(); 5770 this.useContext.add(t); 5771 return t; 5772 } 5773 5774 public DeviceDefinitionChargeItemComponent addUseContext(UsageContext t) { //3 5775 if (t == null) 5776 return this; 5777 if (this.useContext == null) 5778 this.useContext = new ArrayList<UsageContext>(); 5779 this.useContext.add(t); 5780 return this; 5781 } 5782 5783 /** 5784 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 5785 */ 5786 public UsageContext getUseContextFirstRep() { 5787 if (getUseContext().isEmpty()) { 5788 addUseContext(); 5789 } 5790 return getUseContext().get(0); 5791 } 5792 5793 protected void listChildren(List<Property> children) { 5794 super.listChildren(children); 5795 children.add(new Property("chargeItemCode", "CodeableReference(ChargeItemDefinition)", "The code or reference for the charge item.", 0, 1, chargeItemCode)); 5796 children.add(new Property("count", "Quantity", "Coefficient applicable to the billing code.", 0, 1, count)); 5797 children.add(new Property("effectivePeriod", "Period", "A specific time period in which this charge item applies.", 0, 1, effectivePeriod)); 5798 children.add(new Property("useContext", "UsageContext", "The context to which this charge item applies.", 0, java.lang.Integer.MAX_VALUE, useContext)); 5799 } 5800 5801 @Override 5802 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5803 switch (_hash) { 5804 case -2001375628: /*chargeItemCode*/ return new Property("chargeItemCode", "CodeableReference(ChargeItemDefinition)", "The code or reference for the charge item.", 0, 1, chargeItemCode); 5805 case 94851343: /*count*/ return new Property("count", "Quantity", "Coefficient applicable to the billing code.", 0, 1, count); 5806 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "A specific time period in which this charge item applies.", 0, 1, effectivePeriod); 5807 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The context to which this charge item applies.", 0, java.lang.Integer.MAX_VALUE, useContext); 5808 default: return super.getNamedProperty(_hash, _name, _checkValid); 5809 } 5810 5811 } 5812 5813 @Override 5814 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5815 switch (hash) { 5816 case -2001375628: /*chargeItemCode*/ return this.chargeItemCode == null ? new Base[0] : new Base[] {this.chargeItemCode}; // CodeableReference 5817 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // Quantity 5818 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 5819 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5820 default: return super.getProperty(hash, name, checkValid); 5821 } 5822 5823 } 5824 5825 @Override 5826 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5827 switch (hash) { 5828 case -2001375628: // chargeItemCode 5829 this.chargeItemCode = TypeConvertor.castToCodeableReference(value); // CodeableReference 5830 return value; 5831 case 94851343: // count 5832 this.count = TypeConvertor.castToQuantity(value); // Quantity 5833 return value; 5834 case -403934648: // effectivePeriod 5835 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 5836 return value; 5837 case -669707736: // useContext 5838 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5839 return value; 5840 default: return super.setProperty(hash, name, value); 5841 } 5842 5843 } 5844 5845 @Override 5846 public Base setProperty(String name, Base value) throws FHIRException { 5847 if (name.equals("chargeItemCode")) { 5848 this.chargeItemCode = TypeConvertor.castToCodeableReference(value); // CodeableReference 5849 } else if (name.equals("count")) { 5850 this.count = TypeConvertor.castToQuantity(value); // Quantity 5851 } else if (name.equals("effectivePeriod")) { 5852 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 5853 } else if (name.equals("useContext")) { 5854 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 5855 } else 5856 return super.setProperty(name, value); 5857 return value; 5858 } 5859 5860 @Override 5861 public void removeChild(String name, Base value) throws FHIRException { 5862 if (name.equals("chargeItemCode")) { 5863 this.chargeItemCode = null; 5864 } else if (name.equals("count")) { 5865 this.count = null; 5866 } else if (name.equals("effectivePeriod")) { 5867 this.effectivePeriod = null; 5868 } else if (name.equals("useContext")) { 5869 this.getUseContext().remove(value); 5870 } else 5871 super.removeChild(name, value); 5872 5873 } 5874 5875 @Override 5876 public Base makeProperty(int hash, String name) throws FHIRException { 5877 switch (hash) { 5878 case -2001375628: return getChargeItemCode(); 5879 case 94851343: return getCount(); 5880 case -403934648: return getEffectivePeriod(); 5881 case -669707736: return addUseContext(); 5882 default: return super.makeProperty(hash, name); 5883 } 5884 5885 } 5886 5887 @Override 5888 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5889 switch (hash) { 5890 case -2001375628: /*chargeItemCode*/ return new String[] {"CodeableReference"}; 5891 case 94851343: /*count*/ return new String[] {"Quantity"}; 5892 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 5893 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5894 default: return super.getTypesForProperty(hash, name); 5895 } 5896 5897 } 5898 5899 @Override 5900 public Base addChild(String name) throws FHIRException { 5901 if (name.equals("chargeItemCode")) { 5902 this.chargeItemCode = new CodeableReference(); 5903 return this.chargeItemCode; 5904 } 5905 else if (name.equals("count")) { 5906 this.count = new Quantity(); 5907 return this.count; 5908 } 5909 else if (name.equals("effectivePeriod")) { 5910 this.effectivePeriod = new Period(); 5911 return this.effectivePeriod; 5912 } 5913 else if (name.equals("useContext")) { 5914 return addUseContext(); 5915 } 5916 else 5917 return super.addChild(name); 5918 } 5919 5920 public DeviceDefinitionChargeItemComponent copy() { 5921 DeviceDefinitionChargeItemComponent dst = new DeviceDefinitionChargeItemComponent(); 5922 copyValues(dst); 5923 return dst; 5924 } 5925 5926 public void copyValues(DeviceDefinitionChargeItemComponent dst) { 5927 super.copyValues(dst); 5928 dst.chargeItemCode = chargeItemCode == null ? null : chargeItemCode.copy(); 5929 dst.count = count == null ? null : count.copy(); 5930 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5931 if (useContext != null) { 5932 dst.useContext = new ArrayList<UsageContext>(); 5933 for (UsageContext i : useContext) 5934 dst.useContext.add(i.copy()); 5935 }; 5936 } 5937 5938 @Override 5939 public boolean equalsDeep(Base other_) { 5940 if (!super.equalsDeep(other_)) 5941 return false; 5942 if (!(other_ instanceof DeviceDefinitionChargeItemComponent)) 5943 return false; 5944 DeviceDefinitionChargeItemComponent o = (DeviceDefinitionChargeItemComponent) other_; 5945 return compareDeep(chargeItemCode, o.chargeItemCode, true) && compareDeep(count, o.count, true) 5946 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(useContext, o.useContext, true) 5947 ; 5948 } 5949 5950 @Override 5951 public boolean equalsShallow(Base other_) { 5952 if (!super.equalsShallow(other_)) 5953 return false; 5954 if (!(other_ instanceof DeviceDefinitionChargeItemComponent)) 5955 return false; 5956 DeviceDefinitionChargeItemComponent o = (DeviceDefinitionChargeItemComponent) other_; 5957 return true; 5958 } 5959 5960 public boolean isEmpty() { 5961 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(chargeItemCode, count, effectivePeriod 5962 , useContext); 5963 } 5964 5965 public String fhirType() { 5966 return "DeviceDefinition.chargeItem"; 5967 5968 } 5969 5970 } 5971 5972 /** 5973 * Additional information to describe the device. 5974 */ 5975 @Child(name = "description", type = {MarkdownType.class}, order=0, min=0, max=1, modifier=false, summary=false) 5976 @Description(shortDefinition="Additional information to describe the device", formalDefinition="Additional information to describe the device." ) 5977 protected MarkdownType description; 5978 5979 /** 5980 * Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID. The identifier is typically valued if the udiDeviceIdentifier, partNumber or modelNumber is not valued and represents a different type of identifier. However, it is permissible to still include those identifiers in DeviceDefinition.identifier with the appropriate identifier.type. 5981 */ 5982 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5983 @Description(shortDefinition="Instance identifier", formalDefinition="Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID. The identifier is typically valued if the udiDeviceIdentifier, partNumber or modelNumber is not valued and represents a different type of identifier. However, it is permissible to still include those identifiers in DeviceDefinition.identifier with the appropriate identifier.type." ) 5984 protected List<Identifier> identifier; 5985 5986 /** 5987 * Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold. 5988 */ 5989 @Child(name = "udiDeviceIdentifier", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5990 @Description(shortDefinition="Unique Device Identifier (UDI) Barcode string", formalDefinition="Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold." ) 5991 protected List<DeviceDefinitionUdiDeviceIdentifierComponent> udiDeviceIdentifier; 5992 5993 /** 5994 * Identifier associated with the regulatory documentation (certificates, technical documentation, post-market surveillance documentation and reports) of a set of device models sharing the same intended purpose, risk class and essential design and manufacturing characteristics. One example is the Basic UDI-DI in Europe. 5995 */ 5996 @Child(name = "regulatoryIdentifier", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5997 @Description(shortDefinition="Regulatory identifier(s) associated with this device", formalDefinition="Identifier associated with the regulatory documentation (certificates, technical documentation, post-market surveillance documentation and reports) of a set of device models sharing the same intended purpose, risk class and essential design and manufacturing characteristics. One example is the Basic UDI-DI in Europe." ) 5998 protected List<DeviceDefinitionRegulatoryIdentifierComponent> regulatoryIdentifier; 5999 6000 /** 6001 * The part number or catalog number of the device. 6002 */ 6003 @Child(name = "partNumber", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 6004 @Description(shortDefinition="The part number or catalog number of the device", formalDefinition="The part number or catalog number of the device." ) 6005 protected StringType partNumber; 6006 6007 /** 6008 * A name of the manufacturer or legal representative e.g. labeler. Whether this is the actual manufacturer or the labeler or responsible depends on implementation and jurisdiction. 6009 */ 6010 @Child(name = "manufacturer", type = {Organization.class}, order=5, min=0, max=1, modifier=false, summary=true) 6011 @Description(shortDefinition="Name of device manufacturer", formalDefinition="A name of the manufacturer or legal representative e.g. labeler. Whether this is the actual manufacturer or the labeler or responsible depends on implementation and jurisdiction." ) 6012 protected Reference manufacturer; 6013 6014 /** 6015 * The name or names of the device as given by the manufacturer. 6016 */ 6017 @Child(name = "deviceName", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6018 @Description(shortDefinition="The name or names of the device as given by the manufacturer", formalDefinition="The name or names of the device as given by the manufacturer." ) 6019 protected List<DeviceDefinitionDeviceNameComponent> deviceName; 6020 6021 /** 6022 * The model number for the device for example as defined by the manufacturer or labeler, or other agency. 6023 */ 6024 @Child(name = "modelNumber", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 6025 @Description(shortDefinition="The catalog or model number for the device for example as defined by the manufacturer", formalDefinition="The model number for the device for example as defined by the manufacturer or labeler, or other agency." ) 6026 protected StringType modelNumber; 6027 6028 /** 6029 * What kind of device or device system this is. 6030 */ 6031 @Child(name = "classification", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6032 @Description(shortDefinition="What kind of device or device system this is", formalDefinition="What kind of device or device system this is." ) 6033 protected List<DeviceDefinitionClassificationComponent> classification; 6034 6035 /** 6036 * Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards. 6037 */ 6038 @Child(name = "conformsTo", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6039 @Description(shortDefinition="Identifies the standards, specifications, or formal guidances for the capabilities supported by the device", formalDefinition="Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards." ) 6040 protected List<DeviceDefinitionConformsToComponent> conformsTo; 6041 6042 /** 6043 * A device that is part (for example a component) of the present device. 6044 */ 6045 @Child(name = "hasPart", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6046 @Description(shortDefinition="A device, part of the current one", formalDefinition="A device that is part (for example a component) of the present device." ) 6047 protected List<DeviceDefinitionHasPartComponent> hasPart; 6048 6049 /** 6050 * Information about the packaging of the device, i.e. how the device is packaged. 6051 */ 6052 @Child(name = "packaging", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6053 @Description(shortDefinition="Information about the packaging of the device, i.e. how the device is packaged", formalDefinition="Information about the packaging of the device, i.e. how the device is packaged." ) 6054 protected List<DeviceDefinitionPackagingComponent> packaging; 6055 6056 /** 6057 * The version of the device or software. 6058 */ 6059 @Child(name = "version", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6060 @Description(shortDefinition="The version of the device or software", formalDefinition="The version of the device or software." ) 6061 protected List<DeviceDefinitionVersionComponent> version; 6062 6063 /** 6064 * Safety characteristics of the device. 6065 */ 6066 @Child(name = "safety", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6067 @Description(shortDefinition="Safety characteristics of the device", formalDefinition="Safety characteristics of the device." ) 6068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-safety") 6069 protected List<CodeableConcept> safety; 6070 6071 /** 6072 * Shelf Life and storage information. 6073 */ 6074 @Child(name = "shelfLifeStorage", type = {ProductShelfLife.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6075 @Description(shortDefinition="Shelf Life and storage information", formalDefinition="Shelf Life and storage information." ) 6076 protected List<ProductShelfLife> shelfLifeStorage; 6077 6078 /** 6079 * Language code for the human-readable text strings produced by the device (all supported). 6080 */ 6081 @Child(name = "languageCode", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6082 @Description(shortDefinition="Language code for the human-readable text strings produced by the device (all supported)", formalDefinition="Language code for the human-readable text strings produced by the device (all supported)." ) 6083 protected List<CodeableConcept> languageCode; 6084 6085 /** 6086 * Static or essentially fixed characteristics or features of this kind of device that are otherwise not captured in more specific attributes, e.g., time or timing attributes, resolution, accuracy, and physical attributes. 6087 */ 6088 @Child(name = "property", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6089 @Description(shortDefinition="Inherent, essentially fixed, characteristics of this kind of device, e.g., time properties, size, etc", formalDefinition="Static or essentially fixed characteristics or features of this kind of device that are otherwise not captured in more specific attributes, e.g., time or timing attributes, resolution, accuracy, and physical attributes." ) 6090 protected List<DeviceDefinitionPropertyComponent> property; 6091 6092 /** 6093 * An organization that is responsible for the provision and ongoing maintenance of the device. 6094 */ 6095 @Child(name = "owner", type = {Organization.class}, order=17, min=0, max=1, modifier=false, summary=false) 6096 @Description(shortDefinition="Organization responsible for device", formalDefinition="An organization that is responsible for the provision and ongoing maintenance of the device." ) 6097 protected Reference owner; 6098 6099 /** 6100 * Contact details for an organization or a particular human that is responsible for the device. 6101 */ 6102 @Child(name = "contact", type = {ContactPoint.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6103 @Description(shortDefinition="Details for human/organization for support", formalDefinition="Contact details for an organization or a particular human that is responsible for the device." ) 6104 protected List<ContactPoint> contact; 6105 6106 /** 6107 * An associated device, attached to, used with, communicating with or linking a previous or new device model to the focal device. 6108 */ 6109 @Child(name = "link", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6110 @Description(shortDefinition="An associated device, attached to, used with, communicating with or linking a previous or new device model to the focal device", formalDefinition="An associated device, attached to, used with, communicating with or linking a previous or new device model to the focal device." ) 6111 protected List<DeviceDefinitionLinkComponent> link; 6112 6113 /** 6114 * Descriptive information, usage information or implantation information that is not captured in an existing element. 6115 */ 6116 @Child(name = "note", type = {Annotation.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6117 @Description(shortDefinition="Device notes and comments", formalDefinition="Descriptive information, usage information or implantation information that is not captured in an existing element." ) 6118 protected List<Annotation> note; 6119 6120 /** 6121 * A substance used to create the material(s) of which the device is made. 6122 */ 6123 @Child(name = "material", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6124 @Description(shortDefinition="A substance used to create the material(s) of which the device is made", formalDefinition="A substance used to create the material(s) of which the device is made." ) 6125 protected List<DeviceDefinitionMaterialComponent> material; 6126 6127 /** 6128 * Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label. 6129 */ 6130 @Child(name = "productionIdentifierInUDI", type = {CodeType.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6131 @Description(shortDefinition="lot-number | manufactured-date | serial-number | expiration-date | biological-source | software-version", formalDefinition="Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label." ) 6132 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-productidentifierinudi") 6133 protected List<Enumeration<DeviceProductionIdentifierInUDI>> productionIdentifierInUDI; 6134 6135 /** 6136 * Information aimed at providing directions for the usage of this model of device. 6137 */ 6138 @Child(name = "guideline", type = {}, order=23, min=0, max=1, modifier=false, summary=false) 6139 @Description(shortDefinition="Information aimed at providing directions for the usage of this model of device", formalDefinition="Information aimed at providing directions for the usage of this model of device." ) 6140 protected DeviceDefinitionGuidelineComponent guideline; 6141 6142 /** 6143 * Tracking of latest field safety corrective action. 6144 */ 6145 @Child(name = "correctiveAction", type = {}, order=24, min=0, max=1, modifier=false, summary=false) 6146 @Description(shortDefinition="Tracking of latest field safety corrective action", formalDefinition="Tracking of latest field safety corrective action." ) 6147 protected DeviceDefinitionCorrectiveActionComponent correctiveAction; 6148 6149 /** 6150 * Billing code or reference associated with the device. 6151 */ 6152 @Child(name = "chargeItem", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6153 @Description(shortDefinition="Billing code or reference associated with the device", formalDefinition="Billing code or reference associated with the device." ) 6154 protected List<DeviceDefinitionChargeItemComponent> chargeItem; 6155 6156 private static final long serialVersionUID = -260935704L; 6157 6158 /** 6159 * Constructor 6160 */ 6161 public DeviceDefinition() { 6162 super(); 6163 } 6164 6165 /** 6166 * @return {@link #description} (Additional information to describe the device.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6167 */ 6168 public MarkdownType getDescriptionElement() { 6169 if (this.description == null) 6170 if (Configuration.errorOnAutoCreate()) 6171 throw new Error("Attempt to auto-create DeviceDefinition.description"); 6172 else if (Configuration.doAutoCreate()) 6173 this.description = new MarkdownType(); // bb 6174 return this.description; 6175 } 6176 6177 public boolean hasDescriptionElement() { 6178 return this.description != null && !this.description.isEmpty(); 6179 } 6180 6181 public boolean hasDescription() { 6182 return this.description != null && !this.description.isEmpty(); 6183 } 6184 6185 /** 6186 * @param value {@link #description} (Additional information to describe the device.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6187 */ 6188 public DeviceDefinition setDescriptionElement(MarkdownType value) { 6189 this.description = value; 6190 return this; 6191 } 6192 6193 /** 6194 * @return Additional information to describe the device. 6195 */ 6196 public String getDescription() { 6197 return this.description == null ? null : this.description.getValue(); 6198 } 6199 6200 /** 6201 * @param value Additional information to describe the device. 6202 */ 6203 public DeviceDefinition setDescription(String value) { 6204 if (Utilities.noString(value)) 6205 this.description = null; 6206 else { 6207 if (this.description == null) 6208 this.description = new MarkdownType(); 6209 this.description.setValue(value); 6210 } 6211 return this; 6212 } 6213 6214 /** 6215 * @return {@link #identifier} (Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID. The identifier is typically valued if the udiDeviceIdentifier, partNumber or modelNumber is not valued and represents a different type of identifier. However, it is permissible to still include those identifiers in DeviceDefinition.identifier with the appropriate identifier.type.) 6216 */ 6217 public List<Identifier> getIdentifier() { 6218 if (this.identifier == null) 6219 this.identifier = new ArrayList<Identifier>(); 6220 return this.identifier; 6221 } 6222 6223 /** 6224 * @return Returns a reference to <code>this</code> for easy method chaining 6225 */ 6226 public DeviceDefinition setIdentifier(List<Identifier> theIdentifier) { 6227 this.identifier = theIdentifier; 6228 return this; 6229 } 6230 6231 public boolean hasIdentifier() { 6232 if (this.identifier == null) 6233 return false; 6234 for (Identifier item : this.identifier) 6235 if (!item.isEmpty()) 6236 return true; 6237 return false; 6238 } 6239 6240 public Identifier addIdentifier() { //3 6241 Identifier t = new Identifier(); 6242 if (this.identifier == null) 6243 this.identifier = new ArrayList<Identifier>(); 6244 this.identifier.add(t); 6245 return t; 6246 } 6247 6248 public DeviceDefinition addIdentifier(Identifier t) { //3 6249 if (t == null) 6250 return this; 6251 if (this.identifier == null) 6252 this.identifier = new ArrayList<Identifier>(); 6253 this.identifier.add(t); 6254 return this; 6255 } 6256 6257 /** 6258 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 6259 */ 6260 public Identifier getIdentifierFirstRep() { 6261 if (getIdentifier().isEmpty()) { 6262 addIdentifier(); 6263 } 6264 return getIdentifier().get(0); 6265 } 6266 6267 /** 6268 * @return {@link #udiDeviceIdentifier} (Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.) 6269 */ 6270 public List<DeviceDefinitionUdiDeviceIdentifierComponent> getUdiDeviceIdentifier() { 6271 if (this.udiDeviceIdentifier == null) 6272 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 6273 return this.udiDeviceIdentifier; 6274 } 6275 6276 /** 6277 * @return Returns a reference to <code>this</code> for easy method chaining 6278 */ 6279 public DeviceDefinition setUdiDeviceIdentifier(List<DeviceDefinitionUdiDeviceIdentifierComponent> theUdiDeviceIdentifier) { 6280 this.udiDeviceIdentifier = theUdiDeviceIdentifier; 6281 return this; 6282 } 6283 6284 public boolean hasUdiDeviceIdentifier() { 6285 if (this.udiDeviceIdentifier == null) 6286 return false; 6287 for (DeviceDefinitionUdiDeviceIdentifierComponent item : this.udiDeviceIdentifier) 6288 if (!item.isEmpty()) 6289 return true; 6290 return false; 6291 } 6292 6293 public DeviceDefinitionUdiDeviceIdentifierComponent addUdiDeviceIdentifier() { //3 6294 DeviceDefinitionUdiDeviceIdentifierComponent t = new DeviceDefinitionUdiDeviceIdentifierComponent(); 6295 if (this.udiDeviceIdentifier == null) 6296 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 6297 this.udiDeviceIdentifier.add(t); 6298 return t; 6299 } 6300 6301 public DeviceDefinition addUdiDeviceIdentifier(DeviceDefinitionUdiDeviceIdentifierComponent t) { //3 6302 if (t == null) 6303 return this; 6304 if (this.udiDeviceIdentifier == null) 6305 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 6306 this.udiDeviceIdentifier.add(t); 6307 return this; 6308 } 6309 6310 /** 6311 * @return The first repetition of repeating field {@link #udiDeviceIdentifier}, creating it if it does not already exist {3} 6312 */ 6313 public DeviceDefinitionUdiDeviceIdentifierComponent getUdiDeviceIdentifierFirstRep() { 6314 if (getUdiDeviceIdentifier().isEmpty()) { 6315 addUdiDeviceIdentifier(); 6316 } 6317 return getUdiDeviceIdentifier().get(0); 6318 } 6319 6320 /** 6321 * @return {@link #regulatoryIdentifier} (Identifier associated with the regulatory documentation (certificates, technical documentation, post-market surveillance documentation and reports) of a set of device models sharing the same intended purpose, risk class and essential design and manufacturing characteristics. One example is the Basic UDI-DI in Europe.) 6322 */ 6323 public List<DeviceDefinitionRegulatoryIdentifierComponent> getRegulatoryIdentifier() { 6324 if (this.regulatoryIdentifier == null) 6325 this.regulatoryIdentifier = new ArrayList<DeviceDefinitionRegulatoryIdentifierComponent>(); 6326 return this.regulatoryIdentifier; 6327 } 6328 6329 /** 6330 * @return Returns a reference to <code>this</code> for easy method chaining 6331 */ 6332 public DeviceDefinition setRegulatoryIdentifier(List<DeviceDefinitionRegulatoryIdentifierComponent> theRegulatoryIdentifier) { 6333 this.regulatoryIdentifier = theRegulatoryIdentifier; 6334 return this; 6335 } 6336 6337 public boolean hasRegulatoryIdentifier() { 6338 if (this.regulatoryIdentifier == null) 6339 return false; 6340 for (DeviceDefinitionRegulatoryIdentifierComponent item : this.regulatoryIdentifier) 6341 if (!item.isEmpty()) 6342 return true; 6343 return false; 6344 } 6345 6346 public DeviceDefinitionRegulatoryIdentifierComponent addRegulatoryIdentifier() { //3 6347 DeviceDefinitionRegulatoryIdentifierComponent t = new DeviceDefinitionRegulatoryIdentifierComponent(); 6348 if (this.regulatoryIdentifier == null) 6349 this.regulatoryIdentifier = new ArrayList<DeviceDefinitionRegulatoryIdentifierComponent>(); 6350 this.regulatoryIdentifier.add(t); 6351 return t; 6352 } 6353 6354 public DeviceDefinition addRegulatoryIdentifier(DeviceDefinitionRegulatoryIdentifierComponent t) { //3 6355 if (t == null) 6356 return this; 6357 if (this.regulatoryIdentifier == null) 6358 this.regulatoryIdentifier = new ArrayList<DeviceDefinitionRegulatoryIdentifierComponent>(); 6359 this.regulatoryIdentifier.add(t); 6360 return this; 6361 } 6362 6363 /** 6364 * @return The first repetition of repeating field {@link #regulatoryIdentifier}, creating it if it does not already exist {3} 6365 */ 6366 public DeviceDefinitionRegulatoryIdentifierComponent getRegulatoryIdentifierFirstRep() { 6367 if (getRegulatoryIdentifier().isEmpty()) { 6368 addRegulatoryIdentifier(); 6369 } 6370 return getRegulatoryIdentifier().get(0); 6371 } 6372 6373 /** 6374 * @return {@link #partNumber} (The part number or catalog number of the device.). This is the underlying object with id, value and extensions. The accessor "getPartNumber" gives direct access to the value 6375 */ 6376 public StringType getPartNumberElement() { 6377 if (this.partNumber == null) 6378 if (Configuration.errorOnAutoCreate()) 6379 throw new Error("Attempt to auto-create DeviceDefinition.partNumber"); 6380 else if (Configuration.doAutoCreate()) 6381 this.partNumber = new StringType(); // bb 6382 return this.partNumber; 6383 } 6384 6385 public boolean hasPartNumberElement() { 6386 return this.partNumber != null && !this.partNumber.isEmpty(); 6387 } 6388 6389 public boolean hasPartNumber() { 6390 return this.partNumber != null && !this.partNumber.isEmpty(); 6391 } 6392 6393 /** 6394 * @param value {@link #partNumber} (The part number or catalog number of the device.). This is the underlying object with id, value and extensions. The accessor "getPartNumber" gives direct access to the value 6395 */ 6396 public DeviceDefinition setPartNumberElement(StringType value) { 6397 this.partNumber = value; 6398 return this; 6399 } 6400 6401 /** 6402 * @return The part number or catalog number of the device. 6403 */ 6404 public String getPartNumber() { 6405 return this.partNumber == null ? null : this.partNumber.getValue(); 6406 } 6407 6408 /** 6409 * @param value The part number or catalog number of the device. 6410 */ 6411 public DeviceDefinition setPartNumber(String value) { 6412 if (Utilities.noString(value)) 6413 this.partNumber = null; 6414 else { 6415 if (this.partNumber == null) 6416 this.partNumber = new StringType(); 6417 this.partNumber.setValue(value); 6418 } 6419 return this; 6420 } 6421 6422 /** 6423 * @return {@link #manufacturer} (A name of the manufacturer or legal representative e.g. labeler. Whether this is the actual manufacturer or the labeler or responsible depends on implementation and jurisdiction.) 6424 */ 6425 public Reference getManufacturer() { 6426 if (this.manufacturer == null) 6427 if (Configuration.errorOnAutoCreate()) 6428 throw new Error("Attempt to auto-create DeviceDefinition.manufacturer"); 6429 else if (Configuration.doAutoCreate()) 6430 this.manufacturer = new Reference(); // cc 6431 return this.manufacturer; 6432 } 6433 6434 public boolean hasManufacturer() { 6435 return this.manufacturer != null && !this.manufacturer.isEmpty(); 6436 } 6437 6438 /** 6439 * @param value {@link #manufacturer} (A name of the manufacturer or legal representative e.g. labeler. Whether this is the actual manufacturer or the labeler or responsible depends on implementation and jurisdiction.) 6440 */ 6441 public DeviceDefinition setManufacturer(Reference value) { 6442 this.manufacturer = value; 6443 return this; 6444 } 6445 6446 /** 6447 * @return {@link #deviceName} (The name or names of the device as given by the manufacturer.) 6448 */ 6449 public List<DeviceDefinitionDeviceNameComponent> getDeviceName() { 6450 if (this.deviceName == null) 6451 this.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 6452 return this.deviceName; 6453 } 6454 6455 /** 6456 * @return Returns a reference to <code>this</code> for easy method chaining 6457 */ 6458 public DeviceDefinition setDeviceName(List<DeviceDefinitionDeviceNameComponent> theDeviceName) { 6459 this.deviceName = theDeviceName; 6460 return this; 6461 } 6462 6463 public boolean hasDeviceName() { 6464 if (this.deviceName == null) 6465 return false; 6466 for (DeviceDefinitionDeviceNameComponent item : this.deviceName) 6467 if (!item.isEmpty()) 6468 return true; 6469 return false; 6470 } 6471 6472 public DeviceDefinitionDeviceNameComponent addDeviceName() { //3 6473 DeviceDefinitionDeviceNameComponent t = new DeviceDefinitionDeviceNameComponent(); 6474 if (this.deviceName == null) 6475 this.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 6476 this.deviceName.add(t); 6477 return t; 6478 } 6479 6480 public DeviceDefinition addDeviceName(DeviceDefinitionDeviceNameComponent t) { //3 6481 if (t == null) 6482 return this; 6483 if (this.deviceName == null) 6484 this.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 6485 this.deviceName.add(t); 6486 return this; 6487 } 6488 6489 /** 6490 * @return The first repetition of repeating field {@link #deviceName}, creating it if it does not already exist {3} 6491 */ 6492 public DeviceDefinitionDeviceNameComponent getDeviceNameFirstRep() { 6493 if (getDeviceName().isEmpty()) { 6494 addDeviceName(); 6495 } 6496 return getDeviceName().get(0); 6497 } 6498 6499 /** 6500 * @return {@link #modelNumber} (The model number for the device for example as defined by the manufacturer or labeler, or other agency.). This is the underlying object with id, value and extensions. The accessor "getModelNumber" gives direct access to the value 6501 */ 6502 public StringType getModelNumberElement() { 6503 if (this.modelNumber == null) 6504 if (Configuration.errorOnAutoCreate()) 6505 throw new Error("Attempt to auto-create DeviceDefinition.modelNumber"); 6506 else if (Configuration.doAutoCreate()) 6507 this.modelNumber = new StringType(); // bb 6508 return this.modelNumber; 6509 } 6510 6511 public boolean hasModelNumberElement() { 6512 return this.modelNumber != null && !this.modelNumber.isEmpty(); 6513 } 6514 6515 public boolean hasModelNumber() { 6516 return this.modelNumber != null && !this.modelNumber.isEmpty(); 6517 } 6518 6519 /** 6520 * @param value {@link #modelNumber} (The model number for the device for example as defined by the manufacturer or labeler, or other agency.). This is the underlying object with id, value and extensions. The accessor "getModelNumber" gives direct access to the value 6521 */ 6522 public DeviceDefinition setModelNumberElement(StringType value) { 6523 this.modelNumber = value; 6524 return this; 6525 } 6526 6527 /** 6528 * @return The model number for the device for example as defined by the manufacturer or labeler, or other agency. 6529 */ 6530 public String getModelNumber() { 6531 return this.modelNumber == null ? null : this.modelNumber.getValue(); 6532 } 6533 6534 /** 6535 * @param value The model number for the device for example as defined by the manufacturer or labeler, or other agency. 6536 */ 6537 public DeviceDefinition setModelNumber(String value) { 6538 if (Utilities.noString(value)) 6539 this.modelNumber = null; 6540 else { 6541 if (this.modelNumber == null) 6542 this.modelNumber = new StringType(); 6543 this.modelNumber.setValue(value); 6544 } 6545 return this; 6546 } 6547 6548 /** 6549 * @return {@link #classification} (What kind of device or device system this is.) 6550 */ 6551 public List<DeviceDefinitionClassificationComponent> getClassification() { 6552 if (this.classification == null) 6553 this.classification = new ArrayList<DeviceDefinitionClassificationComponent>(); 6554 return this.classification; 6555 } 6556 6557 /** 6558 * @return Returns a reference to <code>this</code> for easy method chaining 6559 */ 6560 public DeviceDefinition setClassification(List<DeviceDefinitionClassificationComponent> theClassification) { 6561 this.classification = theClassification; 6562 return this; 6563 } 6564 6565 public boolean hasClassification() { 6566 if (this.classification == null) 6567 return false; 6568 for (DeviceDefinitionClassificationComponent item : this.classification) 6569 if (!item.isEmpty()) 6570 return true; 6571 return false; 6572 } 6573 6574 public DeviceDefinitionClassificationComponent addClassification() { //3 6575 DeviceDefinitionClassificationComponent t = new DeviceDefinitionClassificationComponent(); 6576 if (this.classification == null) 6577 this.classification = new ArrayList<DeviceDefinitionClassificationComponent>(); 6578 this.classification.add(t); 6579 return t; 6580 } 6581 6582 public DeviceDefinition addClassification(DeviceDefinitionClassificationComponent t) { //3 6583 if (t == null) 6584 return this; 6585 if (this.classification == null) 6586 this.classification = new ArrayList<DeviceDefinitionClassificationComponent>(); 6587 this.classification.add(t); 6588 return this; 6589 } 6590 6591 /** 6592 * @return The first repetition of repeating field {@link #classification}, creating it if it does not already exist {3} 6593 */ 6594 public DeviceDefinitionClassificationComponent getClassificationFirstRep() { 6595 if (getClassification().isEmpty()) { 6596 addClassification(); 6597 } 6598 return getClassification().get(0); 6599 } 6600 6601 /** 6602 * @return {@link #conformsTo} (Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.) 6603 */ 6604 public List<DeviceDefinitionConformsToComponent> getConformsTo() { 6605 if (this.conformsTo == null) 6606 this.conformsTo = new ArrayList<DeviceDefinitionConformsToComponent>(); 6607 return this.conformsTo; 6608 } 6609 6610 /** 6611 * @return Returns a reference to <code>this</code> for easy method chaining 6612 */ 6613 public DeviceDefinition setConformsTo(List<DeviceDefinitionConformsToComponent> theConformsTo) { 6614 this.conformsTo = theConformsTo; 6615 return this; 6616 } 6617 6618 public boolean hasConformsTo() { 6619 if (this.conformsTo == null) 6620 return false; 6621 for (DeviceDefinitionConformsToComponent item : this.conformsTo) 6622 if (!item.isEmpty()) 6623 return true; 6624 return false; 6625 } 6626 6627 public DeviceDefinitionConformsToComponent addConformsTo() { //3 6628 DeviceDefinitionConformsToComponent t = new DeviceDefinitionConformsToComponent(); 6629 if (this.conformsTo == null) 6630 this.conformsTo = new ArrayList<DeviceDefinitionConformsToComponent>(); 6631 this.conformsTo.add(t); 6632 return t; 6633 } 6634 6635 public DeviceDefinition addConformsTo(DeviceDefinitionConformsToComponent t) { //3 6636 if (t == null) 6637 return this; 6638 if (this.conformsTo == null) 6639 this.conformsTo = new ArrayList<DeviceDefinitionConformsToComponent>(); 6640 this.conformsTo.add(t); 6641 return this; 6642 } 6643 6644 /** 6645 * @return The first repetition of repeating field {@link #conformsTo}, creating it if it does not already exist {3} 6646 */ 6647 public DeviceDefinitionConformsToComponent getConformsToFirstRep() { 6648 if (getConformsTo().isEmpty()) { 6649 addConformsTo(); 6650 } 6651 return getConformsTo().get(0); 6652 } 6653 6654 /** 6655 * @return {@link #hasPart} (A device that is part (for example a component) of the present device.) 6656 */ 6657 public List<DeviceDefinitionHasPartComponent> getHasPart() { 6658 if (this.hasPart == null) 6659 this.hasPart = new ArrayList<DeviceDefinitionHasPartComponent>(); 6660 return this.hasPart; 6661 } 6662 6663 /** 6664 * @return Returns a reference to <code>this</code> for easy method chaining 6665 */ 6666 public DeviceDefinition setHasPart(List<DeviceDefinitionHasPartComponent> theHasPart) { 6667 this.hasPart = theHasPart; 6668 return this; 6669 } 6670 6671 public boolean hasHasPart() { 6672 if (this.hasPart == null) 6673 return false; 6674 for (DeviceDefinitionHasPartComponent item : this.hasPart) 6675 if (!item.isEmpty()) 6676 return true; 6677 return false; 6678 } 6679 6680 public DeviceDefinitionHasPartComponent addHasPart() { //3 6681 DeviceDefinitionHasPartComponent t = new DeviceDefinitionHasPartComponent(); 6682 if (this.hasPart == null) 6683 this.hasPart = new ArrayList<DeviceDefinitionHasPartComponent>(); 6684 this.hasPart.add(t); 6685 return t; 6686 } 6687 6688 public DeviceDefinition addHasPart(DeviceDefinitionHasPartComponent t) { //3 6689 if (t == null) 6690 return this; 6691 if (this.hasPart == null) 6692 this.hasPart = new ArrayList<DeviceDefinitionHasPartComponent>(); 6693 this.hasPart.add(t); 6694 return this; 6695 } 6696 6697 /** 6698 * @return The first repetition of repeating field {@link #hasPart}, creating it if it does not already exist {3} 6699 */ 6700 public DeviceDefinitionHasPartComponent getHasPartFirstRep() { 6701 if (getHasPart().isEmpty()) { 6702 addHasPart(); 6703 } 6704 return getHasPart().get(0); 6705 } 6706 6707 /** 6708 * @return {@link #packaging} (Information about the packaging of the device, i.e. how the device is packaged.) 6709 */ 6710 public List<DeviceDefinitionPackagingComponent> getPackaging() { 6711 if (this.packaging == null) 6712 this.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 6713 return this.packaging; 6714 } 6715 6716 /** 6717 * @return Returns a reference to <code>this</code> for easy method chaining 6718 */ 6719 public DeviceDefinition setPackaging(List<DeviceDefinitionPackagingComponent> thePackaging) { 6720 this.packaging = thePackaging; 6721 return this; 6722 } 6723 6724 public boolean hasPackaging() { 6725 if (this.packaging == null) 6726 return false; 6727 for (DeviceDefinitionPackagingComponent item : this.packaging) 6728 if (!item.isEmpty()) 6729 return true; 6730 return false; 6731 } 6732 6733 public DeviceDefinitionPackagingComponent addPackaging() { //3 6734 DeviceDefinitionPackagingComponent t = new DeviceDefinitionPackagingComponent(); 6735 if (this.packaging == null) 6736 this.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 6737 this.packaging.add(t); 6738 return t; 6739 } 6740 6741 public DeviceDefinition addPackaging(DeviceDefinitionPackagingComponent t) { //3 6742 if (t == null) 6743 return this; 6744 if (this.packaging == null) 6745 this.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 6746 this.packaging.add(t); 6747 return this; 6748 } 6749 6750 /** 6751 * @return The first repetition of repeating field {@link #packaging}, creating it if it does not already exist {3} 6752 */ 6753 public DeviceDefinitionPackagingComponent getPackagingFirstRep() { 6754 if (getPackaging().isEmpty()) { 6755 addPackaging(); 6756 } 6757 return getPackaging().get(0); 6758 } 6759 6760 /** 6761 * @return {@link #version} (The version of the device or software.) 6762 */ 6763 public List<DeviceDefinitionVersionComponent> getVersion() { 6764 if (this.version == null) 6765 this.version = new ArrayList<DeviceDefinitionVersionComponent>(); 6766 return this.version; 6767 } 6768 6769 /** 6770 * @return Returns a reference to <code>this</code> for easy method chaining 6771 */ 6772 public DeviceDefinition setVersion(List<DeviceDefinitionVersionComponent> theVersion) { 6773 this.version = theVersion; 6774 return this; 6775 } 6776 6777 public boolean hasVersion() { 6778 if (this.version == null) 6779 return false; 6780 for (DeviceDefinitionVersionComponent item : this.version) 6781 if (!item.isEmpty()) 6782 return true; 6783 return false; 6784 } 6785 6786 public DeviceDefinitionVersionComponent addVersion() { //3 6787 DeviceDefinitionVersionComponent t = new DeviceDefinitionVersionComponent(); 6788 if (this.version == null) 6789 this.version = new ArrayList<DeviceDefinitionVersionComponent>(); 6790 this.version.add(t); 6791 return t; 6792 } 6793 6794 public DeviceDefinition addVersion(DeviceDefinitionVersionComponent t) { //3 6795 if (t == null) 6796 return this; 6797 if (this.version == null) 6798 this.version = new ArrayList<DeviceDefinitionVersionComponent>(); 6799 this.version.add(t); 6800 return this; 6801 } 6802 6803 /** 6804 * @return The first repetition of repeating field {@link #version}, creating it if it does not already exist {3} 6805 */ 6806 public DeviceDefinitionVersionComponent getVersionFirstRep() { 6807 if (getVersion().isEmpty()) { 6808 addVersion(); 6809 } 6810 return getVersion().get(0); 6811 } 6812 6813 /** 6814 * @return {@link #safety} (Safety characteristics of the device.) 6815 */ 6816 public List<CodeableConcept> getSafety() { 6817 if (this.safety == null) 6818 this.safety = new ArrayList<CodeableConcept>(); 6819 return this.safety; 6820 } 6821 6822 /** 6823 * @return Returns a reference to <code>this</code> for easy method chaining 6824 */ 6825 public DeviceDefinition setSafety(List<CodeableConcept> theSafety) { 6826 this.safety = theSafety; 6827 return this; 6828 } 6829 6830 public boolean hasSafety() { 6831 if (this.safety == null) 6832 return false; 6833 for (CodeableConcept item : this.safety) 6834 if (!item.isEmpty()) 6835 return true; 6836 return false; 6837 } 6838 6839 public CodeableConcept addSafety() { //3 6840 CodeableConcept t = new CodeableConcept(); 6841 if (this.safety == null) 6842 this.safety = new ArrayList<CodeableConcept>(); 6843 this.safety.add(t); 6844 return t; 6845 } 6846 6847 public DeviceDefinition addSafety(CodeableConcept t) { //3 6848 if (t == null) 6849 return this; 6850 if (this.safety == null) 6851 this.safety = new ArrayList<CodeableConcept>(); 6852 this.safety.add(t); 6853 return this; 6854 } 6855 6856 /** 6857 * @return The first repetition of repeating field {@link #safety}, creating it if it does not already exist {3} 6858 */ 6859 public CodeableConcept getSafetyFirstRep() { 6860 if (getSafety().isEmpty()) { 6861 addSafety(); 6862 } 6863 return getSafety().get(0); 6864 } 6865 6866 /** 6867 * @return {@link #shelfLifeStorage} (Shelf Life and storage information.) 6868 */ 6869 public List<ProductShelfLife> getShelfLifeStorage() { 6870 if (this.shelfLifeStorage == null) 6871 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 6872 return this.shelfLifeStorage; 6873 } 6874 6875 /** 6876 * @return Returns a reference to <code>this</code> for easy method chaining 6877 */ 6878 public DeviceDefinition setShelfLifeStorage(List<ProductShelfLife> theShelfLifeStorage) { 6879 this.shelfLifeStorage = theShelfLifeStorage; 6880 return this; 6881 } 6882 6883 public boolean hasShelfLifeStorage() { 6884 if (this.shelfLifeStorage == null) 6885 return false; 6886 for (ProductShelfLife item : this.shelfLifeStorage) 6887 if (!item.isEmpty()) 6888 return true; 6889 return false; 6890 } 6891 6892 public ProductShelfLife addShelfLifeStorage() { //3 6893 ProductShelfLife t = new ProductShelfLife(); 6894 if (this.shelfLifeStorage == null) 6895 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 6896 this.shelfLifeStorage.add(t); 6897 return t; 6898 } 6899 6900 public DeviceDefinition addShelfLifeStorage(ProductShelfLife t) { //3 6901 if (t == null) 6902 return this; 6903 if (this.shelfLifeStorage == null) 6904 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 6905 this.shelfLifeStorage.add(t); 6906 return this; 6907 } 6908 6909 /** 6910 * @return The first repetition of repeating field {@link #shelfLifeStorage}, creating it if it does not already exist {3} 6911 */ 6912 public ProductShelfLife getShelfLifeStorageFirstRep() { 6913 if (getShelfLifeStorage().isEmpty()) { 6914 addShelfLifeStorage(); 6915 } 6916 return getShelfLifeStorage().get(0); 6917 } 6918 6919 /** 6920 * @return {@link #languageCode} (Language code for the human-readable text strings produced by the device (all supported).) 6921 */ 6922 public List<CodeableConcept> getLanguageCode() { 6923 if (this.languageCode == null) 6924 this.languageCode = new ArrayList<CodeableConcept>(); 6925 return this.languageCode; 6926 } 6927 6928 /** 6929 * @return Returns a reference to <code>this</code> for easy method chaining 6930 */ 6931 public DeviceDefinition setLanguageCode(List<CodeableConcept> theLanguageCode) { 6932 this.languageCode = theLanguageCode; 6933 return this; 6934 } 6935 6936 public boolean hasLanguageCode() { 6937 if (this.languageCode == null) 6938 return false; 6939 for (CodeableConcept item : this.languageCode) 6940 if (!item.isEmpty()) 6941 return true; 6942 return false; 6943 } 6944 6945 public CodeableConcept addLanguageCode() { //3 6946 CodeableConcept t = new CodeableConcept(); 6947 if (this.languageCode == null) 6948 this.languageCode = new ArrayList<CodeableConcept>(); 6949 this.languageCode.add(t); 6950 return t; 6951 } 6952 6953 public DeviceDefinition addLanguageCode(CodeableConcept t) { //3 6954 if (t == null) 6955 return this; 6956 if (this.languageCode == null) 6957 this.languageCode = new ArrayList<CodeableConcept>(); 6958 this.languageCode.add(t); 6959 return this; 6960 } 6961 6962 /** 6963 * @return The first repetition of repeating field {@link #languageCode}, creating it if it does not already exist {3} 6964 */ 6965 public CodeableConcept getLanguageCodeFirstRep() { 6966 if (getLanguageCode().isEmpty()) { 6967 addLanguageCode(); 6968 } 6969 return getLanguageCode().get(0); 6970 } 6971 6972 /** 6973 * @return {@link #property} (Static or essentially fixed characteristics or features of this kind of device that are otherwise not captured in more specific attributes, e.g., time or timing attributes, resolution, accuracy, and physical attributes.) 6974 */ 6975 public List<DeviceDefinitionPropertyComponent> getProperty() { 6976 if (this.property == null) 6977 this.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 6978 return this.property; 6979 } 6980 6981 /** 6982 * @return Returns a reference to <code>this</code> for easy method chaining 6983 */ 6984 public DeviceDefinition setProperty(List<DeviceDefinitionPropertyComponent> theProperty) { 6985 this.property = theProperty; 6986 return this; 6987 } 6988 6989 public boolean hasProperty() { 6990 if (this.property == null) 6991 return false; 6992 for (DeviceDefinitionPropertyComponent item : this.property) 6993 if (!item.isEmpty()) 6994 return true; 6995 return false; 6996 } 6997 6998 public DeviceDefinitionPropertyComponent addProperty() { //3 6999 DeviceDefinitionPropertyComponent t = new DeviceDefinitionPropertyComponent(); 7000 if (this.property == null) 7001 this.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 7002 this.property.add(t); 7003 return t; 7004 } 7005 7006 public DeviceDefinition addProperty(DeviceDefinitionPropertyComponent t) { //3 7007 if (t == null) 7008 return this; 7009 if (this.property == null) 7010 this.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 7011 this.property.add(t); 7012 return this; 7013 } 7014 7015 /** 7016 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 7017 */ 7018 public DeviceDefinitionPropertyComponent getPropertyFirstRep() { 7019 if (getProperty().isEmpty()) { 7020 addProperty(); 7021 } 7022 return getProperty().get(0); 7023 } 7024 7025 /** 7026 * @return {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 7027 */ 7028 public Reference getOwner() { 7029 if (this.owner == null) 7030 if (Configuration.errorOnAutoCreate()) 7031 throw new Error("Attempt to auto-create DeviceDefinition.owner"); 7032 else if (Configuration.doAutoCreate()) 7033 this.owner = new Reference(); // cc 7034 return this.owner; 7035 } 7036 7037 public boolean hasOwner() { 7038 return this.owner != null && !this.owner.isEmpty(); 7039 } 7040 7041 /** 7042 * @param value {@link #owner} (An organization that is responsible for the provision and ongoing maintenance of the device.) 7043 */ 7044 public DeviceDefinition setOwner(Reference value) { 7045 this.owner = value; 7046 return this; 7047 } 7048 7049 /** 7050 * @return {@link #contact} (Contact details for an organization or a particular human that is responsible for the device.) 7051 */ 7052 public List<ContactPoint> getContact() { 7053 if (this.contact == null) 7054 this.contact = new ArrayList<ContactPoint>(); 7055 return this.contact; 7056 } 7057 7058 /** 7059 * @return Returns a reference to <code>this</code> for easy method chaining 7060 */ 7061 public DeviceDefinition setContact(List<ContactPoint> theContact) { 7062 this.contact = theContact; 7063 return this; 7064 } 7065 7066 public boolean hasContact() { 7067 if (this.contact == null) 7068 return false; 7069 for (ContactPoint item : this.contact) 7070 if (!item.isEmpty()) 7071 return true; 7072 return false; 7073 } 7074 7075 public ContactPoint addContact() { //3 7076 ContactPoint t = new ContactPoint(); 7077 if (this.contact == null) 7078 this.contact = new ArrayList<ContactPoint>(); 7079 this.contact.add(t); 7080 return t; 7081 } 7082 7083 public DeviceDefinition addContact(ContactPoint t) { //3 7084 if (t == null) 7085 return this; 7086 if (this.contact == null) 7087 this.contact = new ArrayList<ContactPoint>(); 7088 this.contact.add(t); 7089 return this; 7090 } 7091 7092 /** 7093 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 7094 */ 7095 public ContactPoint getContactFirstRep() { 7096 if (getContact().isEmpty()) { 7097 addContact(); 7098 } 7099 return getContact().get(0); 7100 } 7101 7102 /** 7103 * @return {@link #link} (An associated device, attached to, used with, communicating with or linking a previous or new device model to the focal device.) 7104 */ 7105 public List<DeviceDefinitionLinkComponent> getLink() { 7106 if (this.link == null) 7107 this.link = new ArrayList<DeviceDefinitionLinkComponent>(); 7108 return this.link; 7109 } 7110 7111 /** 7112 * @return Returns a reference to <code>this</code> for easy method chaining 7113 */ 7114 public DeviceDefinition setLink(List<DeviceDefinitionLinkComponent> theLink) { 7115 this.link = theLink; 7116 return this; 7117 } 7118 7119 public boolean hasLink() { 7120 if (this.link == null) 7121 return false; 7122 for (DeviceDefinitionLinkComponent item : this.link) 7123 if (!item.isEmpty()) 7124 return true; 7125 return false; 7126 } 7127 7128 public DeviceDefinitionLinkComponent addLink() { //3 7129 DeviceDefinitionLinkComponent t = new DeviceDefinitionLinkComponent(); 7130 if (this.link == null) 7131 this.link = new ArrayList<DeviceDefinitionLinkComponent>(); 7132 this.link.add(t); 7133 return t; 7134 } 7135 7136 public DeviceDefinition addLink(DeviceDefinitionLinkComponent t) { //3 7137 if (t == null) 7138 return this; 7139 if (this.link == null) 7140 this.link = new ArrayList<DeviceDefinitionLinkComponent>(); 7141 this.link.add(t); 7142 return this; 7143 } 7144 7145 /** 7146 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist {3} 7147 */ 7148 public DeviceDefinitionLinkComponent getLinkFirstRep() { 7149 if (getLink().isEmpty()) { 7150 addLink(); 7151 } 7152 return getLink().get(0); 7153 } 7154 7155 /** 7156 * @return {@link #note} (Descriptive information, usage information or implantation information that is not captured in an existing element.) 7157 */ 7158 public List<Annotation> getNote() { 7159 if (this.note == null) 7160 this.note = new ArrayList<Annotation>(); 7161 return this.note; 7162 } 7163 7164 /** 7165 * @return Returns a reference to <code>this</code> for easy method chaining 7166 */ 7167 public DeviceDefinition setNote(List<Annotation> theNote) { 7168 this.note = theNote; 7169 return this; 7170 } 7171 7172 public boolean hasNote() { 7173 if (this.note == null) 7174 return false; 7175 for (Annotation item : this.note) 7176 if (!item.isEmpty()) 7177 return true; 7178 return false; 7179 } 7180 7181 public Annotation addNote() { //3 7182 Annotation t = new Annotation(); 7183 if (this.note == null) 7184 this.note = new ArrayList<Annotation>(); 7185 this.note.add(t); 7186 return t; 7187 } 7188 7189 public DeviceDefinition addNote(Annotation t) { //3 7190 if (t == null) 7191 return this; 7192 if (this.note == null) 7193 this.note = new ArrayList<Annotation>(); 7194 this.note.add(t); 7195 return this; 7196 } 7197 7198 /** 7199 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 7200 */ 7201 public Annotation getNoteFirstRep() { 7202 if (getNote().isEmpty()) { 7203 addNote(); 7204 } 7205 return getNote().get(0); 7206 } 7207 7208 /** 7209 * @return {@link #material} (A substance used to create the material(s) of which the device is made.) 7210 */ 7211 public List<DeviceDefinitionMaterialComponent> getMaterial() { 7212 if (this.material == null) 7213 this.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 7214 return this.material; 7215 } 7216 7217 /** 7218 * @return Returns a reference to <code>this</code> for easy method chaining 7219 */ 7220 public DeviceDefinition setMaterial(List<DeviceDefinitionMaterialComponent> theMaterial) { 7221 this.material = theMaterial; 7222 return this; 7223 } 7224 7225 public boolean hasMaterial() { 7226 if (this.material == null) 7227 return false; 7228 for (DeviceDefinitionMaterialComponent item : this.material) 7229 if (!item.isEmpty()) 7230 return true; 7231 return false; 7232 } 7233 7234 public DeviceDefinitionMaterialComponent addMaterial() { //3 7235 DeviceDefinitionMaterialComponent t = new DeviceDefinitionMaterialComponent(); 7236 if (this.material == null) 7237 this.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 7238 this.material.add(t); 7239 return t; 7240 } 7241 7242 public DeviceDefinition addMaterial(DeviceDefinitionMaterialComponent t) { //3 7243 if (t == null) 7244 return this; 7245 if (this.material == null) 7246 this.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 7247 this.material.add(t); 7248 return this; 7249 } 7250 7251 /** 7252 * @return The first repetition of repeating field {@link #material}, creating it if it does not already exist {3} 7253 */ 7254 public DeviceDefinitionMaterialComponent getMaterialFirstRep() { 7255 if (getMaterial().isEmpty()) { 7256 addMaterial(); 7257 } 7258 return getMaterial().get(0); 7259 } 7260 7261 /** 7262 * @return {@link #productionIdentifierInUDI} (Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label.) 7263 */ 7264 public List<Enumeration<DeviceProductionIdentifierInUDI>> getProductionIdentifierInUDI() { 7265 if (this.productionIdentifierInUDI == null) 7266 this.productionIdentifierInUDI = new ArrayList<Enumeration<DeviceProductionIdentifierInUDI>>(); 7267 return this.productionIdentifierInUDI; 7268 } 7269 7270 /** 7271 * @return Returns a reference to <code>this</code> for easy method chaining 7272 */ 7273 public DeviceDefinition setProductionIdentifierInUDI(List<Enumeration<DeviceProductionIdentifierInUDI>> theProductionIdentifierInUDI) { 7274 this.productionIdentifierInUDI = theProductionIdentifierInUDI; 7275 return this; 7276 } 7277 7278 public boolean hasProductionIdentifierInUDI() { 7279 if (this.productionIdentifierInUDI == null) 7280 return false; 7281 for (Enumeration<DeviceProductionIdentifierInUDI> item : this.productionIdentifierInUDI) 7282 if (!item.isEmpty()) 7283 return true; 7284 return false; 7285 } 7286 7287 /** 7288 * @return {@link #productionIdentifierInUDI} (Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label.) 7289 */ 7290 public Enumeration<DeviceProductionIdentifierInUDI> addProductionIdentifierInUDIElement() {//2 7291 Enumeration<DeviceProductionIdentifierInUDI> t = new Enumeration<DeviceProductionIdentifierInUDI>(new DeviceProductionIdentifierInUDIEnumFactory()); 7292 if (this.productionIdentifierInUDI == null) 7293 this.productionIdentifierInUDI = new ArrayList<Enumeration<DeviceProductionIdentifierInUDI>>(); 7294 this.productionIdentifierInUDI.add(t); 7295 return t; 7296 } 7297 7298 /** 7299 * @param value {@link #productionIdentifierInUDI} (Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label.) 7300 */ 7301 public DeviceDefinition addProductionIdentifierInUDI(DeviceProductionIdentifierInUDI value) { //1 7302 Enumeration<DeviceProductionIdentifierInUDI> t = new Enumeration<DeviceProductionIdentifierInUDI>(new DeviceProductionIdentifierInUDIEnumFactory()); 7303 t.setValue(value); 7304 if (this.productionIdentifierInUDI == null) 7305 this.productionIdentifierInUDI = new ArrayList<Enumeration<DeviceProductionIdentifierInUDI>>(); 7306 this.productionIdentifierInUDI.add(t); 7307 return this; 7308 } 7309 7310 /** 7311 * @param value {@link #productionIdentifierInUDI} (Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label.) 7312 */ 7313 public boolean hasProductionIdentifierInUDI(DeviceProductionIdentifierInUDI value) { 7314 if (this.productionIdentifierInUDI == null) 7315 return false; 7316 for (Enumeration<DeviceProductionIdentifierInUDI> v : this.productionIdentifierInUDI) 7317 if (v.getValue().equals(value)) // code 7318 return true; 7319 return false; 7320 } 7321 7322 /** 7323 * @return {@link #guideline} (Information aimed at providing directions for the usage of this model of device.) 7324 */ 7325 public DeviceDefinitionGuidelineComponent getGuideline() { 7326 if (this.guideline == null) 7327 if (Configuration.errorOnAutoCreate()) 7328 throw new Error("Attempt to auto-create DeviceDefinition.guideline"); 7329 else if (Configuration.doAutoCreate()) 7330 this.guideline = new DeviceDefinitionGuidelineComponent(); // cc 7331 return this.guideline; 7332 } 7333 7334 public boolean hasGuideline() { 7335 return this.guideline != null && !this.guideline.isEmpty(); 7336 } 7337 7338 /** 7339 * @param value {@link #guideline} (Information aimed at providing directions for the usage of this model of device.) 7340 */ 7341 public DeviceDefinition setGuideline(DeviceDefinitionGuidelineComponent value) { 7342 this.guideline = value; 7343 return this; 7344 } 7345 7346 /** 7347 * @return {@link #correctiveAction} (Tracking of latest field safety corrective action.) 7348 */ 7349 public DeviceDefinitionCorrectiveActionComponent getCorrectiveAction() { 7350 if (this.correctiveAction == null) 7351 if (Configuration.errorOnAutoCreate()) 7352 throw new Error("Attempt to auto-create DeviceDefinition.correctiveAction"); 7353 else if (Configuration.doAutoCreate()) 7354 this.correctiveAction = new DeviceDefinitionCorrectiveActionComponent(); // cc 7355 return this.correctiveAction; 7356 } 7357 7358 public boolean hasCorrectiveAction() { 7359 return this.correctiveAction != null && !this.correctiveAction.isEmpty(); 7360 } 7361 7362 /** 7363 * @param value {@link #correctiveAction} (Tracking of latest field safety corrective action.) 7364 */ 7365 public DeviceDefinition setCorrectiveAction(DeviceDefinitionCorrectiveActionComponent value) { 7366 this.correctiveAction = value; 7367 return this; 7368 } 7369 7370 /** 7371 * @return {@link #chargeItem} (Billing code or reference associated with the device.) 7372 */ 7373 public List<DeviceDefinitionChargeItemComponent> getChargeItem() { 7374 if (this.chargeItem == null) 7375 this.chargeItem = new ArrayList<DeviceDefinitionChargeItemComponent>(); 7376 return this.chargeItem; 7377 } 7378 7379 /** 7380 * @return Returns a reference to <code>this</code> for easy method chaining 7381 */ 7382 public DeviceDefinition setChargeItem(List<DeviceDefinitionChargeItemComponent> theChargeItem) { 7383 this.chargeItem = theChargeItem; 7384 return this; 7385 } 7386 7387 public boolean hasChargeItem() { 7388 if (this.chargeItem == null) 7389 return false; 7390 for (DeviceDefinitionChargeItemComponent item : this.chargeItem) 7391 if (!item.isEmpty()) 7392 return true; 7393 return false; 7394 } 7395 7396 public DeviceDefinitionChargeItemComponent addChargeItem() { //3 7397 DeviceDefinitionChargeItemComponent t = new DeviceDefinitionChargeItemComponent(); 7398 if (this.chargeItem == null) 7399 this.chargeItem = new ArrayList<DeviceDefinitionChargeItemComponent>(); 7400 this.chargeItem.add(t); 7401 return t; 7402 } 7403 7404 public DeviceDefinition addChargeItem(DeviceDefinitionChargeItemComponent t) { //3 7405 if (t == null) 7406 return this; 7407 if (this.chargeItem == null) 7408 this.chargeItem = new ArrayList<DeviceDefinitionChargeItemComponent>(); 7409 this.chargeItem.add(t); 7410 return this; 7411 } 7412 7413 /** 7414 * @return The first repetition of repeating field {@link #chargeItem}, creating it if it does not already exist {3} 7415 */ 7416 public DeviceDefinitionChargeItemComponent getChargeItemFirstRep() { 7417 if (getChargeItem().isEmpty()) { 7418 addChargeItem(); 7419 } 7420 return getChargeItem().get(0); 7421 } 7422 7423 protected void listChildren(List<Property> children) { 7424 super.listChildren(children); 7425 children.add(new Property("description", "markdown", "Additional information to describe the device.", 0, 1, description)); 7426 children.add(new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID. The identifier is typically valued if the udiDeviceIdentifier, partNumber or modelNumber is not valued and represents a different type of identifier. However, it is permissible to still include those identifiers in DeviceDefinition.identifier with the appropriate identifier.type.", 0, java.lang.Integer.MAX_VALUE, identifier)); 7427 children.add(new Property("udiDeviceIdentifier", "", "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 0, java.lang.Integer.MAX_VALUE, udiDeviceIdentifier)); 7428 children.add(new Property("regulatoryIdentifier", "", "Identifier associated with the regulatory documentation (certificates, technical documentation, post-market surveillance documentation and reports) of a set of device models sharing the same intended purpose, risk class and essential design and manufacturing characteristics. One example is the Basic UDI-DI in Europe.", 0, java.lang.Integer.MAX_VALUE, regulatoryIdentifier)); 7429 children.add(new Property("partNumber", "string", "The part number or catalog number of the device.", 0, 1, partNumber)); 7430 children.add(new Property("manufacturer", "Reference(Organization)", "A name of the manufacturer or legal representative e.g. labeler. Whether this is the actual manufacturer or the labeler or responsible depends on implementation and jurisdiction.", 0, 1, manufacturer)); 7431 children.add(new Property("deviceName", "", "The name or names of the device as given by the manufacturer.", 0, java.lang.Integer.MAX_VALUE, deviceName)); 7432 children.add(new Property("modelNumber", "string", "The model number for the device for example as defined by the manufacturer or labeler, or other agency.", 0, 1, modelNumber)); 7433 children.add(new Property("classification", "", "What kind of device or device system this is.", 0, java.lang.Integer.MAX_VALUE, classification)); 7434 children.add(new Property("conformsTo", "", "Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.", 0, java.lang.Integer.MAX_VALUE, conformsTo)); 7435 children.add(new Property("hasPart", "", "A device that is part (for example a component) of the present device.", 0, java.lang.Integer.MAX_VALUE, hasPart)); 7436 children.add(new Property("packaging", "", "Information about the packaging of the device, i.e. how the device is packaged.", 0, java.lang.Integer.MAX_VALUE, packaging)); 7437 children.add(new Property("version", "", "The version of the device or software.", 0, java.lang.Integer.MAX_VALUE, version)); 7438 children.add(new Property("safety", "CodeableConcept", "Safety characteristics of the device.", 0, java.lang.Integer.MAX_VALUE, safety)); 7439 children.add(new Property("shelfLifeStorage", "ProductShelfLife", "Shelf Life and storage information.", 0, java.lang.Integer.MAX_VALUE, shelfLifeStorage)); 7440 children.add(new Property("languageCode", "CodeableConcept", "Language code for the human-readable text strings produced by the device (all supported).", 0, java.lang.Integer.MAX_VALUE, languageCode)); 7441 children.add(new Property("property", "", "Static or essentially fixed characteristics or features of this kind of device that are otherwise not captured in more specific attributes, e.g., time or timing attributes, resolution, accuracy, and physical attributes.", 0, java.lang.Integer.MAX_VALUE, property)); 7442 children.add(new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner)); 7443 children.add(new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact)); 7444 children.add(new Property("link", "", "An associated device, attached to, used with, communicating with or linking a previous or new device model to the focal device.", 0, java.lang.Integer.MAX_VALUE, link)); 7445 children.add(new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note)); 7446 children.add(new Property("material", "", "A substance used to create the material(s) of which the device is made.", 0, java.lang.Integer.MAX_VALUE, material)); 7447 children.add(new Property("productionIdentifierInUDI", "code", "Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label.", 0, java.lang.Integer.MAX_VALUE, productionIdentifierInUDI)); 7448 children.add(new Property("guideline", "", "Information aimed at providing directions for the usage of this model of device.", 0, 1, guideline)); 7449 children.add(new Property("correctiveAction", "", "Tracking of latest field safety corrective action.", 0, 1, correctiveAction)); 7450 children.add(new Property("chargeItem", "", "Billing code or reference associated with the device.", 0, java.lang.Integer.MAX_VALUE, chargeItem)); 7451 } 7452 7453 @Override 7454 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7455 switch (_hash) { 7456 case -1724546052: /*description*/ return new Property("description", "markdown", "Additional information to describe the device.", 0, 1, description); 7457 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID. The identifier is typically valued if the udiDeviceIdentifier, partNumber or modelNumber is not valued and represents a different type of identifier. However, it is permissible to still include those identifiers in DeviceDefinition.identifier with the appropriate identifier.type.", 0, java.lang.Integer.MAX_VALUE, identifier); 7458 case -99121287: /*udiDeviceIdentifier*/ return new Property("udiDeviceIdentifier", "", "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 0, java.lang.Integer.MAX_VALUE, udiDeviceIdentifier); 7459 case 455683425: /*regulatoryIdentifier*/ return new Property("regulatoryIdentifier", "", "Identifier associated with the regulatory documentation (certificates, technical documentation, post-market surveillance documentation and reports) of a set of device models sharing the same intended purpose, risk class and essential design and manufacturing characteristics. One example is the Basic UDI-DI in Europe.", 0, java.lang.Integer.MAX_VALUE, regulatoryIdentifier); 7460 case -731502308: /*partNumber*/ return new Property("partNumber", "string", "The part number or catalog number of the device.", 0, 1, partNumber); 7461 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "A name of the manufacturer or legal representative e.g. labeler. Whether this is the actual manufacturer or the labeler or responsible depends on implementation and jurisdiction.", 0, 1, manufacturer); 7462 case 780988929: /*deviceName*/ return new Property("deviceName", "", "The name or names of the device as given by the manufacturer.", 0, java.lang.Integer.MAX_VALUE, deviceName); 7463 case 346619858: /*modelNumber*/ return new Property("modelNumber", "string", "The model number for the device for example as defined by the manufacturer or labeler, or other agency.", 0, 1, modelNumber); 7464 case 382350310: /*classification*/ return new Property("classification", "", "What kind of device or device system this is.", 0, java.lang.Integer.MAX_VALUE, classification); 7465 case 1014198088: /*conformsTo*/ return new Property("conformsTo", "", "Identifies the standards, specifications, or formal guidances for the capabilities supported by the device. The device may be certified as conformant to these specifications e.g., communication, performance, process, measurement, or specialization standards.", 0, java.lang.Integer.MAX_VALUE, conformsTo); 7466 case 696815021: /*hasPart*/ return new Property("hasPart", "", "A device that is part (for example a component) of the present device.", 0, java.lang.Integer.MAX_VALUE, hasPart); 7467 case 1802065795: /*packaging*/ return new Property("packaging", "", "Information about the packaging of the device, i.e. how the device is packaged.", 0, java.lang.Integer.MAX_VALUE, packaging); 7468 case 351608024: /*version*/ return new Property("version", "", "The version of the device or software.", 0, java.lang.Integer.MAX_VALUE, version); 7469 case -909893934: /*safety*/ return new Property("safety", "CodeableConcept", "Safety characteristics of the device.", 0, java.lang.Integer.MAX_VALUE, safety); 7470 case 172049237: /*shelfLifeStorage*/ return new Property("shelfLifeStorage", "ProductShelfLife", "Shelf Life and storage information.", 0, java.lang.Integer.MAX_VALUE, shelfLifeStorage); 7471 case -2092349083: /*languageCode*/ return new Property("languageCode", "CodeableConcept", "Language code for the human-readable text strings produced by the device (all supported).", 0, java.lang.Integer.MAX_VALUE, languageCode); 7472 case -993141291: /*property*/ return new Property("property", "", "Static or essentially fixed characteristics or features of this kind of device that are otherwise not captured in more specific attributes, e.g., time or timing attributes, resolution, accuracy, and physical attributes.", 0, java.lang.Integer.MAX_VALUE, property); 7473 case 106164915: /*owner*/ return new Property("owner", "Reference(Organization)", "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner); 7474 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for an organization or a particular human that is responsible for the device.", 0, java.lang.Integer.MAX_VALUE, contact); 7475 case 3321850: /*link*/ return new Property("link", "", "An associated device, attached to, used with, communicating with or linking a previous or new device model to the focal device.", 0, java.lang.Integer.MAX_VALUE, link); 7476 case 3387378: /*note*/ return new Property("note", "Annotation", "Descriptive information, usage information or implantation information that is not captured in an existing element.", 0, java.lang.Integer.MAX_VALUE, note); 7477 case 299066663: /*material*/ return new Property("material", "", "A substance used to create the material(s) of which the device is made.", 0, java.lang.Integer.MAX_VALUE, material); 7478 case 312405811: /*productionIdentifierInUDI*/ return new Property("productionIdentifierInUDI", "code", "Indicates the production identifier(s) that are expected to appear in the UDI carrier on the device label.", 0, java.lang.Integer.MAX_VALUE, productionIdentifierInUDI); 7479 case -2075718416: /*guideline*/ return new Property("guideline", "", "Information aimed at providing directions for the usage of this model of device.", 0, 1, guideline); 7480 case 1354575876: /*correctiveAction*/ return new Property("correctiveAction", "", "Tracking of latest field safety corrective action.", 0, 1, correctiveAction); 7481 case 1417779175: /*chargeItem*/ return new Property("chargeItem", "", "Billing code or reference associated with the device.", 0, java.lang.Integer.MAX_VALUE, chargeItem); 7482 default: return super.getNamedProperty(_hash, _name, _checkValid); 7483 } 7484 7485 } 7486 7487 @Override 7488 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7489 switch (hash) { 7490 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 7491 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 7492 case -99121287: /*udiDeviceIdentifier*/ return this.udiDeviceIdentifier == null ? new Base[0] : this.udiDeviceIdentifier.toArray(new Base[this.udiDeviceIdentifier.size()]); // DeviceDefinitionUdiDeviceIdentifierComponent 7493 case 455683425: /*regulatoryIdentifier*/ return this.regulatoryIdentifier == null ? new Base[0] : this.regulatoryIdentifier.toArray(new Base[this.regulatoryIdentifier.size()]); // DeviceDefinitionRegulatoryIdentifierComponent 7494 case -731502308: /*partNumber*/ return this.partNumber == null ? new Base[0] : new Base[] {this.partNumber}; // StringType 7495 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // Reference 7496 case 780988929: /*deviceName*/ return this.deviceName == null ? new Base[0] : this.deviceName.toArray(new Base[this.deviceName.size()]); // DeviceDefinitionDeviceNameComponent 7497 case 346619858: /*modelNumber*/ return this.modelNumber == null ? new Base[0] : new Base[] {this.modelNumber}; // StringType 7498 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : this.classification.toArray(new Base[this.classification.size()]); // DeviceDefinitionClassificationComponent 7499 case 1014198088: /*conformsTo*/ return this.conformsTo == null ? new Base[0] : this.conformsTo.toArray(new Base[this.conformsTo.size()]); // DeviceDefinitionConformsToComponent 7500 case 696815021: /*hasPart*/ return this.hasPart == null ? new Base[0] : this.hasPart.toArray(new Base[this.hasPart.size()]); // DeviceDefinitionHasPartComponent 7501 case 1802065795: /*packaging*/ return this.packaging == null ? new Base[0] : this.packaging.toArray(new Base[this.packaging.size()]); // DeviceDefinitionPackagingComponent 7502 case 351608024: /*version*/ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // DeviceDefinitionVersionComponent 7503 case -909893934: /*safety*/ return this.safety == null ? new Base[0] : this.safety.toArray(new Base[this.safety.size()]); // CodeableConcept 7504 case 172049237: /*shelfLifeStorage*/ return this.shelfLifeStorage == null ? new Base[0] : this.shelfLifeStorage.toArray(new Base[this.shelfLifeStorage.size()]); // ProductShelfLife 7505 case -2092349083: /*languageCode*/ return this.languageCode == null ? new Base[0] : this.languageCode.toArray(new Base[this.languageCode.size()]); // CodeableConcept 7506 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // DeviceDefinitionPropertyComponent 7507 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 7508 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 7509 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // DeviceDefinitionLinkComponent 7510 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 7511 case 299066663: /*material*/ return this.material == null ? new Base[0] : this.material.toArray(new Base[this.material.size()]); // DeviceDefinitionMaterialComponent 7512 case 312405811: /*productionIdentifierInUDI*/ return this.productionIdentifierInUDI == null ? new Base[0] : this.productionIdentifierInUDI.toArray(new Base[this.productionIdentifierInUDI.size()]); // Enumeration<DeviceProductionIdentifierInUDI> 7513 case -2075718416: /*guideline*/ return this.guideline == null ? new Base[0] : new Base[] {this.guideline}; // DeviceDefinitionGuidelineComponent 7514 case 1354575876: /*correctiveAction*/ return this.correctiveAction == null ? new Base[0] : new Base[] {this.correctiveAction}; // DeviceDefinitionCorrectiveActionComponent 7515 case 1417779175: /*chargeItem*/ return this.chargeItem == null ? new Base[0] : this.chargeItem.toArray(new Base[this.chargeItem.size()]); // DeviceDefinitionChargeItemComponent 7516 default: return super.getProperty(hash, name, checkValid); 7517 } 7518 7519 } 7520 7521 @Override 7522 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7523 switch (hash) { 7524 case -1724546052: // description 7525 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 7526 return value; 7527 case -1618432855: // identifier 7528 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 7529 return value; 7530 case -99121287: // udiDeviceIdentifier 7531 this.getUdiDeviceIdentifier().add((DeviceDefinitionUdiDeviceIdentifierComponent) value); // DeviceDefinitionUdiDeviceIdentifierComponent 7532 return value; 7533 case 455683425: // regulatoryIdentifier 7534 this.getRegulatoryIdentifier().add((DeviceDefinitionRegulatoryIdentifierComponent) value); // DeviceDefinitionRegulatoryIdentifierComponent 7535 return value; 7536 case -731502308: // partNumber 7537 this.partNumber = TypeConvertor.castToString(value); // StringType 7538 return value; 7539 case -1969347631: // manufacturer 7540 this.manufacturer = TypeConvertor.castToReference(value); // Reference 7541 return value; 7542 case 780988929: // deviceName 7543 this.getDeviceName().add((DeviceDefinitionDeviceNameComponent) value); // DeviceDefinitionDeviceNameComponent 7544 return value; 7545 case 346619858: // modelNumber 7546 this.modelNumber = TypeConvertor.castToString(value); // StringType 7547 return value; 7548 case 382350310: // classification 7549 this.getClassification().add((DeviceDefinitionClassificationComponent) value); // DeviceDefinitionClassificationComponent 7550 return value; 7551 case 1014198088: // conformsTo 7552 this.getConformsTo().add((DeviceDefinitionConformsToComponent) value); // DeviceDefinitionConformsToComponent 7553 return value; 7554 case 696815021: // hasPart 7555 this.getHasPart().add((DeviceDefinitionHasPartComponent) value); // DeviceDefinitionHasPartComponent 7556 return value; 7557 case 1802065795: // packaging 7558 this.getPackaging().add((DeviceDefinitionPackagingComponent) value); // DeviceDefinitionPackagingComponent 7559 return value; 7560 case 351608024: // version 7561 this.getVersion().add((DeviceDefinitionVersionComponent) value); // DeviceDefinitionVersionComponent 7562 return value; 7563 case -909893934: // safety 7564 this.getSafety().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7565 return value; 7566 case 172049237: // shelfLifeStorage 7567 this.getShelfLifeStorage().add(TypeConvertor.castToProductShelfLife(value)); // ProductShelfLife 7568 return value; 7569 case -2092349083: // languageCode 7570 this.getLanguageCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7571 return value; 7572 case -993141291: // property 7573 this.getProperty().add((DeviceDefinitionPropertyComponent) value); // DeviceDefinitionPropertyComponent 7574 return value; 7575 case 106164915: // owner 7576 this.owner = TypeConvertor.castToReference(value); // Reference 7577 return value; 7578 case 951526432: // contact 7579 this.getContact().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 7580 return value; 7581 case 3321850: // link 7582 this.getLink().add((DeviceDefinitionLinkComponent) value); // DeviceDefinitionLinkComponent 7583 return value; 7584 case 3387378: // note 7585 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 7586 return value; 7587 case 299066663: // material 7588 this.getMaterial().add((DeviceDefinitionMaterialComponent) value); // DeviceDefinitionMaterialComponent 7589 return value; 7590 case 312405811: // productionIdentifierInUDI 7591 value = new DeviceProductionIdentifierInUDIEnumFactory().fromType(TypeConvertor.castToCode(value)); 7592 this.getProductionIdentifierInUDI().add((Enumeration) value); // Enumeration<DeviceProductionIdentifierInUDI> 7593 return value; 7594 case -2075718416: // guideline 7595 this.guideline = (DeviceDefinitionGuidelineComponent) value; // DeviceDefinitionGuidelineComponent 7596 return value; 7597 case 1354575876: // correctiveAction 7598 this.correctiveAction = (DeviceDefinitionCorrectiveActionComponent) value; // DeviceDefinitionCorrectiveActionComponent 7599 return value; 7600 case 1417779175: // chargeItem 7601 this.getChargeItem().add((DeviceDefinitionChargeItemComponent) value); // DeviceDefinitionChargeItemComponent 7602 return value; 7603 default: return super.setProperty(hash, name, value); 7604 } 7605 7606 } 7607 7608 @Override 7609 public Base setProperty(String name, Base value) throws FHIRException { 7610 if (name.equals("description")) { 7611 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 7612 } else if (name.equals("identifier")) { 7613 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 7614 } else if (name.equals("udiDeviceIdentifier")) { 7615 this.getUdiDeviceIdentifier().add((DeviceDefinitionUdiDeviceIdentifierComponent) value); 7616 } else if (name.equals("regulatoryIdentifier")) { 7617 this.getRegulatoryIdentifier().add((DeviceDefinitionRegulatoryIdentifierComponent) value); 7618 } else if (name.equals("partNumber")) { 7619 this.partNumber = TypeConvertor.castToString(value); // StringType 7620 } else if (name.equals("manufacturer")) { 7621 this.manufacturer = TypeConvertor.castToReference(value); // Reference 7622 } else if (name.equals("deviceName")) { 7623 this.getDeviceName().add((DeviceDefinitionDeviceNameComponent) value); 7624 } else if (name.equals("modelNumber")) { 7625 this.modelNumber = TypeConvertor.castToString(value); // StringType 7626 } else if (name.equals("classification")) { 7627 this.getClassification().add((DeviceDefinitionClassificationComponent) value); 7628 } else if (name.equals("conformsTo")) { 7629 this.getConformsTo().add((DeviceDefinitionConformsToComponent) value); 7630 } else if (name.equals("hasPart")) { 7631 this.getHasPart().add((DeviceDefinitionHasPartComponent) value); 7632 } else if (name.equals("packaging")) { 7633 this.getPackaging().add((DeviceDefinitionPackagingComponent) value); 7634 } else if (name.equals("version")) { 7635 this.getVersion().add((DeviceDefinitionVersionComponent) value); 7636 } else if (name.equals("safety")) { 7637 this.getSafety().add(TypeConvertor.castToCodeableConcept(value)); 7638 } else if (name.equals("shelfLifeStorage")) { 7639 this.getShelfLifeStorage().add(TypeConvertor.castToProductShelfLife(value)); 7640 } else if (name.equals("languageCode")) { 7641 this.getLanguageCode().add(TypeConvertor.castToCodeableConcept(value)); 7642 } else if (name.equals("property")) { 7643 this.getProperty().add((DeviceDefinitionPropertyComponent) value); 7644 } else if (name.equals("owner")) { 7645 this.owner = TypeConvertor.castToReference(value); // Reference 7646 } else if (name.equals("contact")) { 7647 this.getContact().add(TypeConvertor.castToContactPoint(value)); 7648 } else if (name.equals("link")) { 7649 this.getLink().add((DeviceDefinitionLinkComponent) value); 7650 } else if (name.equals("note")) { 7651 this.getNote().add(TypeConvertor.castToAnnotation(value)); 7652 } else if (name.equals("material")) { 7653 this.getMaterial().add((DeviceDefinitionMaterialComponent) value); 7654 } else if (name.equals("productionIdentifierInUDI")) { 7655 value = new DeviceProductionIdentifierInUDIEnumFactory().fromType(TypeConvertor.castToCode(value)); 7656 this.getProductionIdentifierInUDI().add((Enumeration) value); 7657 } else if (name.equals("guideline")) { 7658 this.guideline = (DeviceDefinitionGuidelineComponent) value; // DeviceDefinitionGuidelineComponent 7659 } else if (name.equals("correctiveAction")) { 7660 this.correctiveAction = (DeviceDefinitionCorrectiveActionComponent) value; // DeviceDefinitionCorrectiveActionComponent 7661 } else if (name.equals("chargeItem")) { 7662 this.getChargeItem().add((DeviceDefinitionChargeItemComponent) value); 7663 } else 7664 return super.setProperty(name, value); 7665 return value; 7666 } 7667 7668 @Override 7669 public void removeChild(String name, Base value) throws FHIRException { 7670 if (name.equals("description")) { 7671 this.description = null; 7672 } else if (name.equals("identifier")) { 7673 this.getIdentifier().remove(value); 7674 } else if (name.equals("udiDeviceIdentifier")) { 7675 this.getUdiDeviceIdentifier().remove((DeviceDefinitionUdiDeviceIdentifierComponent) value); 7676 } else if (name.equals("regulatoryIdentifier")) { 7677 this.getRegulatoryIdentifier().remove((DeviceDefinitionRegulatoryIdentifierComponent) value); 7678 } else if (name.equals("partNumber")) { 7679 this.partNumber = null; 7680 } else if (name.equals("manufacturer")) { 7681 this.manufacturer = null; 7682 } else if (name.equals("deviceName")) { 7683 this.getDeviceName().remove((DeviceDefinitionDeviceNameComponent) value); 7684 } else if (name.equals("modelNumber")) { 7685 this.modelNumber = null; 7686 } else if (name.equals("classification")) { 7687 this.getClassification().remove((DeviceDefinitionClassificationComponent) value); 7688 } else if (name.equals("conformsTo")) { 7689 this.getConformsTo().remove((DeviceDefinitionConformsToComponent) value); 7690 } else if (name.equals("hasPart")) { 7691 this.getHasPart().remove((DeviceDefinitionHasPartComponent) value); 7692 } else if (name.equals("packaging")) { 7693 this.getPackaging().remove((DeviceDefinitionPackagingComponent) value); 7694 } else if (name.equals("version")) { 7695 this.getVersion().remove((DeviceDefinitionVersionComponent) value); 7696 } else if (name.equals("safety")) { 7697 this.getSafety().remove(value); 7698 } else if (name.equals("shelfLifeStorage")) { 7699 this.getShelfLifeStorage().remove(value); 7700 } else if (name.equals("languageCode")) { 7701 this.getLanguageCode().remove(value); 7702 } else if (name.equals("property")) { 7703 this.getProperty().remove((DeviceDefinitionPropertyComponent) value); 7704 } else if (name.equals("owner")) { 7705 this.owner = null; 7706 } else if (name.equals("contact")) { 7707 this.getContact().remove(value); 7708 } else if (name.equals("link")) { 7709 this.getLink().remove((DeviceDefinitionLinkComponent) value); 7710 } else if (name.equals("note")) { 7711 this.getNote().remove(value); 7712 } else if (name.equals("material")) { 7713 this.getMaterial().remove((DeviceDefinitionMaterialComponent) value); 7714 } else if (name.equals("productionIdentifierInUDI")) { 7715 value = new DeviceProductionIdentifierInUDIEnumFactory().fromType(TypeConvertor.castToCode(value)); 7716 this.getProductionIdentifierInUDI().remove((Enumeration) value); 7717 } else if (name.equals("guideline")) { 7718 this.guideline = (DeviceDefinitionGuidelineComponent) value; // DeviceDefinitionGuidelineComponent 7719 } else if (name.equals("correctiveAction")) { 7720 this.correctiveAction = (DeviceDefinitionCorrectiveActionComponent) value; // DeviceDefinitionCorrectiveActionComponent 7721 } else if (name.equals("chargeItem")) { 7722 this.getChargeItem().remove((DeviceDefinitionChargeItemComponent) value); 7723 } else 7724 super.removeChild(name, value); 7725 7726 } 7727 7728 @Override 7729 public Base makeProperty(int hash, String name) throws FHIRException { 7730 switch (hash) { 7731 case -1724546052: return getDescriptionElement(); 7732 case -1618432855: return addIdentifier(); 7733 case -99121287: return addUdiDeviceIdentifier(); 7734 case 455683425: return addRegulatoryIdentifier(); 7735 case -731502308: return getPartNumberElement(); 7736 case -1969347631: return getManufacturer(); 7737 case 780988929: return addDeviceName(); 7738 case 346619858: return getModelNumberElement(); 7739 case 382350310: return addClassification(); 7740 case 1014198088: return addConformsTo(); 7741 case 696815021: return addHasPart(); 7742 case 1802065795: return addPackaging(); 7743 case 351608024: return addVersion(); 7744 case -909893934: return addSafety(); 7745 case 172049237: return addShelfLifeStorage(); 7746 case -2092349083: return addLanguageCode(); 7747 case -993141291: return addProperty(); 7748 case 106164915: return getOwner(); 7749 case 951526432: return addContact(); 7750 case 3321850: return addLink(); 7751 case 3387378: return addNote(); 7752 case 299066663: return addMaterial(); 7753 case 312405811: return addProductionIdentifierInUDIElement(); 7754 case -2075718416: return getGuideline(); 7755 case 1354575876: return getCorrectiveAction(); 7756 case 1417779175: return addChargeItem(); 7757 default: return super.makeProperty(hash, name); 7758 } 7759 7760 } 7761 7762 @Override 7763 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7764 switch (hash) { 7765 case -1724546052: /*description*/ return new String[] {"markdown"}; 7766 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 7767 case -99121287: /*udiDeviceIdentifier*/ return new String[] {}; 7768 case 455683425: /*regulatoryIdentifier*/ return new String[] {}; 7769 case -731502308: /*partNumber*/ return new String[] {"string"}; 7770 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 7771 case 780988929: /*deviceName*/ return new String[] {}; 7772 case 346619858: /*modelNumber*/ return new String[] {"string"}; 7773 case 382350310: /*classification*/ return new String[] {}; 7774 case 1014198088: /*conformsTo*/ return new String[] {}; 7775 case 696815021: /*hasPart*/ return new String[] {}; 7776 case 1802065795: /*packaging*/ return new String[] {}; 7777 case 351608024: /*version*/ return new String[] {}; 7778 case -909893934: /*safety*/ return new String[] {"CodeableConcept"}; 7779 case 172049237: /*shelfLifeStorage*/ return new String[] {"ProductShelfLife"}; 7780 case -2092349083: /*languageCode*/ return new String[] {"CodeableConcept"}; 7781 case -993141291: /*property*/ return new String[] {}; 7782 case 106164915: /*owner*/ return new String[] {"Reference"}; 7783 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 7784 case 3321850: /*link*/ return new String[] {}; 7785 case 3387378: /*note*/ return new String[] {"Annotation"}; 7786 case 299066663: /*material*/ return new String[] {}; 7787 case 312405811: /*productionIdentifierInUDI*/ return new String[] {"code"}; 7788 case -2075718416: /*guideline*/ return new String[] {}; 7789 case 1354575876: /*correctiveAction*/ return new String[] {}; 7790 case 1417779175: /*chargeItem*/ return new String[] {}; 7791 default: return super.getTypesForProperty(hash, name); 7792 } 7793 7794 } 7795 7796 @Override 7797 public Base addChild(String name) throws FHIRException { 7798 if (name.equals("description")) { 7799 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.description"); 7800 } 7801 else if (name.equals("identifier")) { 7802 return addIdentifier(); 7803 } 7804 else if (name.equals("udiDeviceIdentifier")) { 7805 return addUdiDeviceIdentifier(); 7806 } 7807 else if (name.equals("regulatoryIdentifier")) { 7808 return addRegulatoryIdentifier(); 7809 } 7810 else if (name.equals("partNumber")) { 7811 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.partNumber"); 7812 } 7813 else if (name.equals("manufacturer")) { 7814 this.manufacturer = new Reference(); 7815 return this.manufacturer; 7816 } 7817 else if (name.equals("deviceName")) { 7818 return addDeviceName(); 7819 } 7820 else if (name.equals("modelNumber")) { 7821 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.modelNumber"); 7822 } 7823 else if (name.equals("classification")) { 7824 return addClassification(); 7825 } 7826 else if (name.equals("conformsTo")) { 7827 return addConformsTo(); 7828 } 7829 else if (name.equals("hasPart")) { 7830 return addHasPart(); 7831 } 7832 else if (name.equals("packaging")) { 7833 return addPackaging(); 7834 } 7835 else if (name.equals("version")) { 7836 return addVersion(); 7837 } 7838 else if (name.equals("safety")) { 7839 return addSafety(); 7840 } 7841 else if (name.equals("shelfLifeStorage")) { 7842 return addShelfLifeStorage(); 7843 } 7844 else if (name.equals("languageCode")) { 7845 return addLanguageCode(); 7846 } 7847 else if (name.equals("property")) { 7848 return addProperty(); 7849 } 7850 else if (name.equals("owner")) { 7851 this.owner = new Reference(); 7852 return this.owner; 7853 } 7854 else if (name.equals("contact")) { 7855 return addContact(); 7856 } 7857 else if (name.equals("link")) { 7858 return addLink(); 7859 } 7860 else if (name.equals("note")) { 7861 return addNote(); 7862 } 7863 else if (name.equals("material")) { 7864 return addMaterial(); 7865 } 7866 else if (name.equals("productionIdentifierInUDI")) { 7867 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.productionIdentifierInUDI"); 7868 } 7869 else if (name.equals("guideline")) { 7870 this.guideline = new DeviceDefinitionGuidelineComponent(); 7871 return this.guideline; 7872 } 7873 else if (name.equals("correctiveAction")) { 7874 this.correctiveAction = new DeviceDefinitionCorrectiveActionComponent(); 7875 return this.correctiveAction; 7876 } 7877 else if (name.equals("chargeItem")) { 7878 return addChargeItem(); 7879 } 7880 else 7881 return super.addChild(name); 7882 } 7883 7884 public String fhirType() { 7885 return "DeviceDefinition"; 7886 7887 } 7888 7889 public DeviceDefinition copy() { 7890 DeviceDefinition dst = new DeviceDefinition(); 7891 copyValues(dst); 7892 return dst; 7893 } 7894 7895 public void copyValues(DeviceDefinition dst) { 7896 super.copyValues(dst); 7897 dst.description = description == null ? null : description.copy(); 7898 if (identifier != null) { 7899 dst.identifier = new ArrayList<Identifier>(); 7900 for (Identifier i : identifier) 7901 dst.identifier.add(i.copy()); 7902 }; 7903 if (udiDeviceIdentifier != null) { 7904 dst.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 7905 for (DeviceDefinitionUdiDeviceIdentifierComponent i : udiDeviceIdentifier) 7906 dst.udiDeviceIdentifier.add(i.copy()); 7907 }; 7908 if (regulatoryIdentifier != null) { 7909 dst.regulatoryIdentifier = new ArrayList<DeviceDefinitionRegulatoryIdentifierComponent>(); 7910 for (DeviceDefinitionRegulatoryIdentifierComponent i : regulatoryIdentifier) 7911 dst.regulatoryIdentifier.add(i.copy()); 7912 }; 7913 dst.partNumber = partNumber == null ? null : partNumber.copy(); 7914 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 7915 if (deviceName != null) { 7916 dst.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 7917 for (DeviceDefinitionDeviceNameComponent i : deviceName) 7918 dst.deviceName.add(i.copy()); 7919 }; 7920 dst.modelNumber = modelNumber == null ? null : modelNumber.copy(); 7921 if (classification != null) { 7922 dst.classification = new ArrayList<DeviceDefinitionClassificationComponent>(); 7923 for (DeviceDefinitionClassificationComponent i : classification) 7924 dst.classification.add(i.copy()); 7925 }; 7926 if (conformsTo != null) { 7927 dst.conformsTo = new ArrayList<DeviceDefinitionConformsToComponent>(); 7928 for (DeviceDefinitionConformsToComponent i : conformsTo) 7929 dst.conformsTo.add(i.copy()); 7930 }; 7931 if (hasPart != null) { 7932 dst.hasPart = new ArrayList<DeviceDefinitionHasPartComponent>(); 7933 for (DeviceDefinitionHasPartComponent i : hasPart) 7934 dst.hasPart.add(i.copy()); 7935 }; 7936 if (packaging != null) { 7937 dst.packaging = new ArrayList<DeviceDefinitionPackagingComponent>(); 7938 for (DeviceDefinitionPackagingComponent i : packaging) 7939 dst.packaging.add(i.copy()); 7940 }; 7941 if (version != null) { 7942 dst.version = new ArrayList<DeviceDefinitionVersionComponent>(); 7943 for (DeviceDefinitionVersionComponent i : version) 7944 dst.version.add(i.copy()); 7945 }; 7946 if (safety != null) { 7947 dst.safety = new ArrayList<CodeableConcept>(); 7948 for (CodeableConcept i : safety) 7949 dst.safety.add(i.copy()); 7950 }; 7951 if (shelfLifeStorage != null) { 7952 dst.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 7953 for (ProductShelfLife i : shelfLifeStorage) 7954 dst.shelfLifeStorage.add(i.copy()); 7955 }; 7956 if (languageCode != null) { 7957 dst.languageCode = new ArrayList<CodeableConcept>(); 7958 for (CodeableConcept i : languageCode) 7959 dst.languageCode.add(i.copy()); 7960 }; 7961 if (property != null) { 7962 dst.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 7963 for (DeviceDefinitionPropertyComponent i : property) 7964 dst.property.add(i.copy()); 7965 }; 7966 dst.owner = owner == null ? null : owner.copy(); 7967 if (contact != null) { 7968 dst.contact = new ArrayList<ContactPoint>(); 7969 for (ContactPoint i : contact) 7970 dst.contact.add(i.copy()); 7971 }; 7972 if (link != null) { 7973 dst.link = new ArrayList<DeviceDefinitionLinkComponent>(); 7974 for (DeviceDefinitionLinkComponent i : link) 7975 dst.link.add(i.copy()); 7976 }; 7977 if (note != null) { 7978 dst.note = new ArrayList<Annotation>(); 7979 for (Annotation i : note) 7980 dst.note.add(i.copy()); 7981 }; 7982 if (material != null) { 7983 dst.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 7984 for (DeviceDefinitionMaterialComponent i : material) 7985 dst.material.add(i.copy()); 7986 }; 7987 if (productionIdentifierInUDI != null) { 7988 dst.productionIdentifierInUDI = new ArrayList<Enumeration<DeviceProductionIdentifierInUDI>>(); 7989 for (Enumeration<DeviceProductionIdentifierInUDI> i : productionIdentifierInUDI) 7990 dst.productionIdentifierInUDI.add(i.copy()); 7991 }; 7992 dst.guideline = guideline == null ? null : guideline.copy(); 7993 dst.correctiveAction = correctiveAction == null ? null : correctiveAction.copy(); 7994 if (chargeItem != null) { 7995 dst.chargeItem = new ArrayList<DeviceDefinitionChargeItemComponent>(); 7996 for (DeviceDefinitionChargeItemComponent i : chargeItem) 7997 dst.chargeItem.add(i.copy()); 7998 }; 7999 } 8000 8001 protected DeviceDefinition typedCopy() { 8002 return copy(); 8003 } 8004 8005 @Override 8006 public boolean equalsDeep(Base other_) { 8007 if (!super.equalsDeep(other_)) 8008 return false; 8009 if (!(other_ instanceof DeviceDefinition)) 8010 return false; 8011 DeviceDefinition o = (DeviceDefinition) other_; 8012 return compareDeep(description, o.description, true) && compareDeep(identifier, o.identifier, true) 8013 && compareDeep(udiDeviceIdentifier, o.udiDeviceIdentifier, true) && compareDeep(regulatoryIdentifier, o.regulatoryIdentifier, true) 8014 && compareDeep(partNumber, o.partNumber, true) && compareDeep(manufacturer, o.manufacturer, true) 8015 && compareDeep(deviceName, o.deviceName, true) && compareDeep(modelNumber, o.modelNumber, true) 8016 && compareDeep(classification, o.classification, true) && compareDeep(conformsTo, o.conformsTo, true) 8017 && compareDeep(hasPart, o.hasPart, true) && compareDeep(packaging, o.packaging, true) && compareDeep(version, o.version, true) 8018 && compareDeep(safety, o.safety, true) && compareDeep(shelfLifeStorage, o.shelfLifeStorage, true) 8019 && compareDeep(languageCode, o.languageCode, true) && compareDeep(property, o.property, true) && compareDeep(owner, o.owner, true) 8020 && compareDeep(contact, o.contact, true) && compareDeep(link, o.link, true) && compareDeep(note, o.note, true) 8021 && compareDeep(material, o.material, true) && compareDeep(productionIdentifierInUDI, o.productionIdentifierInUDI, true) 8022 && compareDeep(guideline, o.guideline, true) && compareDeep(correctiveAction, o.correctiveAction, true) 8023 && compareDeep(chargeItem, o.chargeItem, true); 8024 } 8025 8026 @Override 8027 public boolean equalsShallow(Base other_) { 8028 if (!super.equalsShallow(other_)) 8029 return false; 8030 if (!(other_ instanceof DeviceDefinition)) 8031 return false; 8032 DeviceDefinition o = (DeviceDefinition) other_; 8033 return compareValues(description, o.description, true) && compareValues(partNumber, o.partNumber, true) 8034 && compareValues(modelNumber, o.modelNumber, true) && compareValues(productionIdentifierInUDI, o.productionIdentifierInUDI, true) 8035 ; 8036 } 8037 8038 public boolean isEmpty() { 8039 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, identifier, udiDeviceIdentifier 8040 , regulatoryIdentifier, partNumber, manufacturer, deviceName, modelNumber, classification 8041 , conformsTo, hasPart, packaging, version, safety, shelfLifeStorage, languageCode 8042 , property, owner, contact, link, note, material, productionIdentifierInUDI, guideline 8043 , correctiveAction, chargeItem); 8044 } 8045 8046 @Override 8047 public ResourceType getResourceType() { 8048 return ResourceType.DeviceDefinition; 8049 } 8050 8051 /** 8052 * Search parameter: <b>device-name</b> 8053 * <p> 8054 * Description: <b>A server defined search that may match any of the string fields in DeviceDefinition.name or DeviceDefinition.classification.type - the latter to search for 'generic' devices.</b><br> 8055 * Type: <b>string</b><br> 8056 * Path: <b>DeviceDefinition.deviceName.name | DeviceDefinition.classification.type.coding.display | DeviceDefinition.classification.type.text</b><br> 8057 * </p> 8058 */ 8059 @SearchParamDefinition(name="device-name", path="DeviceDefinition.deviceName.name | DeviceDefinition.classification.type.coding.display | DeviceDefinition.classification.type.text", description="A server defined search that may match any of the string fields in DeviceDefinition.name or DeviceDefinition.classification.type - the latter to search for 'generic' devices.", type="string" ) 8060 public static final String SP_DEVICE_NAME = "device-name"; 8061 /** 8062 * <b>Fluent Client</b> search parameter constant for <b>device-name</b> 8063 * <p> 8064 * Description: <b>A server defined search that may match any of the string fields in DeviceDefinition.name or DeviceDefinition.classification.type - the latter to search for 'generic' devices.</b><br> 8065 * Type: <b>string</b><br> 8066 * Path: <b>DeviceDefinition.deviceName.name | DeviceDefinition.classification.type.coding.display | DeviceDefinition.classification.type.text</b><br> 8067 * </p> 8068 */ 8069 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEVICE_NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DEVICE_NAME); 8070 8071 /** 8072 * Search parameter: <b>identifier</b> 8073 * <p> 8074 * Description: <b>The identifier of the component</b><br> 8075 * Type: <b>token</b><br> 8076 * Path: <b>DeviceDefinition.identifier</b><br> 8077 * </p> 8078 */ 8079 @SearchParamDefinition(name="identifier", path="DeviceDefinition.identifier", description="The identifier of the component", type="token" ) 8080 public static final String SP_IDENTIFIER = "identifier"; 8081 /** 8082 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 8083 * <p> 8084 * Description: <b>The identifier of the component</b><br> 8085 * Type: <b>token</b><br> 8086 * Path: <b>DeviceDefinition.identifier</b><br> 8087 * </p> 8088 */ 8089 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 8090 8091 /** 8092 * Search parameter: <b>manufacturer</b> 8093 * <p> 8094 * Description: <b>The manufacturer of the device</b><br> 8095 * Type: <b>reference</b><br> 8096 * Path: <b>DeviceDefinition.manufacturer</b><br> 8097 * </p> 8098 */ 8099 @SearchParamDefinition(name="manufacturer", path="DeviceDefinition.manufacturer", description="The manufacturer of the device", type="reference", target={Organization.class } ) 8100 public static final String SP_MANUFACTURER = "manufacturer"; 8101 /** 8102 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 8103 * <p> 8104 * Description: <b>The manufacturer of the device</b><br> 8105 * Type: <b>reference</b><br> 8106 * Path: <b>DeviceDefinition.manufacturer</b><br> 8107 * </p> 8108 */ 8109 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURER); 8110 8111/** 8112 * Constant for fluent queries to be used to add include statements. Specifies 8113 * the path value of "<b>DeviceDefinition:manufacturer</b>". 8114 */ 8115 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include("DeviceDefinition:manufacturer").toLocked(); 8116 8117 /** 8118 * Search parameter: <b>organization</b> 8119 * <p> 8120 * Description: <b>The organization responsible for the device</b><br> 8121 * Type: <b>reference</b><br> 8122 * Path: <b>DeviceDefinition.owner</b><br> 8123 * </p> 8124 */ 8125 @SearchParamDefinition(name="organization", path="DeviceDefinition.owner", description="The organization responsible for the device", type="reference", target={Organization.class } ) 8126 public static final String SP_ORGANIZATION = "organization"; 8127 /** 8128 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 8129 * <p> 8130 * Description: <b>The organization responsible for the device</b><br> 8131 * Type: <b>reference</b><br> 8132 * Path: <b>DeviceDefinition.owner</b><br> 8133 * </p> 8134 */ 8135 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 8136 8137/** 8138 * Constant for fluent queries to be used to add include statements. Specifies 8139 * the path value of "<b>DeviceDefinition:organization</b>". 8140 */ 8141 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("DeviceDefinition:organization").toLocked(); 8142 8143 /** 8144 * Search parameter: <b>specification</b> 8145 * <p> 8146 * Description: <b>The specification that the device conforms to</b><br> 8147 * Type: <b>token</b><br> 8148 * Path: <b>DeviceDefinition.conformsTo.specification</b><br> 8149 * </p> 8150 */ 8151 @SearchParamDefinition(name="specification", path="DeviceDefinition.conformsTo.specification", description="The specification that the device conforms to", type="token" ) 8152 public static final String SP_SPECIFICATION = "specification"; 8153 /** 8154 * <b>Fluent Client</b> search parameter constant for <b>specification</b> 8155 * <p> 8156 * Description: <b>The specification that the device conforms to</b><br> 8157 * Type: <b>token</b><br> 8158 * Path: <b>DeviceDefinition.conformsTo.specification</b><br> 8159 * </p> 8160 */ 8161 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIFICATION); 8162 8163 /** 8164 * Search parameter: <b>type</b> 8165 * <p> 8166 * Description: <b>The device type</b><br> 8167 * Type: <b>token</b><br> 8168 * Path: <b>DeviceDefinition.conformsTo.category</b><br> 8169 * </p> 8170 */ 8171 @SearchParamDefinition(name="type", path="DeviceDefinition.conformsTo.category", description="The device type", type="token" ) 8172 public static final String SP_TYPE = "type"; 8173 /** 8174 * <b>Fluent Client</b> search parameter constant for <b>type</b> 8175 * <p> 8176 * Description: <b>The device type</b><br> 8177 * Type: <b>token</b><br> 8178 * Path: <b>DeviceDefinition.conformsTo.category</b><br> 8179 * </p> 8180 */ 8181 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 8182 8183 8184} 8185