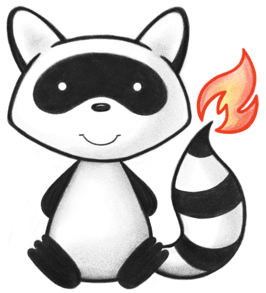
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Indicates that a device is to be or has been dispensed for a named person/patient. This includes a description of the product (supply) provided and the instructions for using the device. 052 */ 053@ResourceDef(name="DeviceDispense", profile="http://hl7.org/fhir/StructureDefinition/DeviceDispense") 054public class DeviceDispense extends DomainResource { 055 056 public enum DeviceDispenseStatusCodes { 057 /** 058 * The core event has not started yet, but some staging activities have begun (e.g. initial preparing of the device. Preparation stages may be tracked e.g. for planning, supply or billing purposes. 059 */ 060 PREPARATION, 061 /** 062 * The dispensed product is ready for pickup. 063 */ 064 INPROGRESS, 065 /** 066 * The dispensed product was not and will never be picked up by the patient. 067 */ 068 CANCELLED, 069 /** 070 * The dispense process is paused while waiting for an external event to reactivate the dispense. For example, new stock has arrived or the prescriber has called. 071 */ 072 ONHOLD, 073 /** 074 * The dispensed product has been picked up. 075 */ 076 COMPLETED, 077 /** 078 * The dispense was entered in error and therefore nullified. 079 */ 080 ENTEREDINERROR, 081 /** 082 * Actions implied by the dispense have been permanently halted, before all of them occurred. 083 */ 084 STOPPED, 085 /** 086 * The dispense was declined and not performed. 087 */ 088 DECLINED, 089 /** 090 * The authoring system does not know which of the status values applies for this dispense. Note: this concept is not to be used for other - one of the listed statuses is presumed to apply, it's just now known which one. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 public static DeviceDispenseStatusCodes fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("preparation".equals(codeString)) 101 return PREPARATION; 102 if ("in-progress".equals(codeString)) 103 return INPROGRESS; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("on-hold".equals(codeString)) 107 return ONHOLD; 108 if ("completed".equals(codeString)) 109 return COMPLETED; 110 if ("entered-in-error".equals(codeString)) 111 return ENTEREDINERROR; 112 if ("stopped".equals(codeString)) 113 return STOPPED; 114 if ("declined".equals(codeString)) 115 return DECLINED; 116 if ("unknown".equals(codeString)) 117 return UNKNOWN; 118 if (Configuration.isAcceptInvalidEnums()) 119 return null; 120 else 121 throw new FHIRException("Unknown DeviceDispenseStatusCodes code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case PREPARATION: return "preparation"; 126 case INPROGRESS: return "in-progress"; 127 case CANCELLED: return "cancelled"; 128 case ONHOLD: return "on-hold"; 129 case COMPLETED: return "completed"; 130 case ENTEREDINERROR: return "entered-in-error"; 131 case STOPPED: return "stopped"; 132 case DECLINED: return "declined"; 133 case UNKNOWN: return "unknown"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getSystem() { 139 switch (this) { 140 case PREPARATION: return "http://hl7.org/fhir/devicedispense-status"; 141 case INPROGRESS: return "http://hl7.org/fhir/devicedispense-status"; 142 case CANCELLED: return "http://hl7.org/fhir/devicedispense-status"; 143 case ONHOLD: return "http://hl7.org/fhir/devicedispense-status"; 144 case COMPLETED: return "http://hl7.org/fhir/devicedispense-status"; 145 case ENTEREDINERROR: return "http://hl7.org/fhir/devicedispense-status"; 146 case STOPPED: return "http://hl7.org/fhir/devicedispense-status"; 147 case DECLINED: return "http://hl7.org/fhir/devicedispense-status"; 148 case UNKNOWN: return "http://hl7.org/fhir/devicedispense-status"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 public String getDefinition() { 154 switch (this) { 155 case PREPARATION: return "The core event has not started yet, but some staging activities have begun (e.g. initial preparing of the device. Preparation stages may be tracked e.g. for planning, supply or billing purposes."; 156 case INPROGRESS: return "The dispensed product is ready for pickup."; 157 case CANCELLED: return "The dispensed product was not and will never be picked up by the patient."; 158 case ONHOLD: return "The dispense process is paused while waiting for an external event to reactivate the dispense. For example, new stock has arrived or the prescriber has called."; 159 case COMPLETED: return "The dispensed product has been picked up."; 160 case ENTEREDINERROR: return "The dispense was entered in error and therefore nullified."; 161 case STOPPED: return "Actions implied by the dispense have been permanently halted, before all of them occurred."; 162 case DECLINED: return "The dispense was declined and not performed."; 163 case UNKNOWN: return "The authoring system does not know which of the status values applies for this dispense. Note: this concept is not to be used for other - one of the listed statuses is presumed to apply, it's just now known which one."; 164 case NULL: return null; 165 default: return "?"; 166 } 167 } 168 public String getDisplay() { 169 switch (this) { 170 case PREPARATION: return "Preparation"; 171 case INPROGRESS: return "In Progress"; 172 case CANCELLED: return "Cancelled"; 173 case ONHOLD: return "On Hold"; 174 case COMPLETED: return "Completed"; 175 case ENTEREDINERROR: return "Entered in Error"; 176 case STOPPED: return "Stopped"; 177 case DECLINED: return "Declined"; 178 case UNKNOWN: return "Unknown"; 179 case NULL: return null; 180 default: return "?"; 181 } 182 } 183 } 184 185 public static class DeviceDispenseStatusCodesEnumFactory implements EnumFactory<DeviceDispenseStatusCodes> { 186 public DeviceDispenseStatusCodes fromCode(String codeString) throws IllegalArgumentException { 187 if (codeString == null || "".equals(codeString)) 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("preparation".equals(codeString)) 191 return DeviceDispenseStatusCodes.PREPARATION; 192 if ("in-progress".equals(codeString)) 193 return DeviceDispenseStatusCodes.INPROGRESS; 194 if ("cancelled".equals(codeString)) 195 return DeviceDispenseStatusCodes.CANCELLED; 196 if ("on-hold".equals(codeString)) 197 return DeviceDispenseStatusCodes.ONHOLD; 198 if ("completed".equals(codeString)) 199 return DeviceDispenseStatusCodes.COMPLETED; 200 if ("entered-in-error".equals(codeString)) 201 return DeviceDispenseStatusCodes.ENTEREDINERROR; 202 if ("stopped".equals(codeString)) 203 return DeviceDispenseStatusCodes.STOPPED; 204 if ("declined".equals(codeString)) 205 return DeviceDispenseStatusCodes.DECLINED; 206 if ("unknown".equals(codeString)) 207 return DeviceDispenseStatusCodes.UNKNOWN; 208 throw new IllegalArgumentException("Unknown DeviceDispenseStatusCodes code '"+codeString+"'"); 209 } 210 public Enumeration<DeviceDispenseStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.NULL, code); 215 String codeString = ((PrimitiveType) code).asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.NULL, code); 218 if ("preparation".equals(codeString)) 219 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.PREPARATION, code); 220 if ("in-progress".equals(codeString)) 221 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.INPROGRESS, code); 222 if ("cancelled".equals(codeString)) 223 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.CANCELLED, code); 224 if ("on-hold".equals(codeString)) 225 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.ONHOLD, code); 226 if ("completed".equals(codeString)) 227 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.COMPLETED, code); 228 if ("entered-in-error".equals(codeString)) 229 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.ENTEREDINERROR, code); 230 if ("stopped".equals(codeString)) 231 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.STOPPED, code); 232 if ("declined".equals(codeString)) 233 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.DECLINED, code); 234 if ("unknown".equals(codeString)) 235 return new Enumeration<DeviceDispenseStatusCodes>(this, DeviceDispenseStatusCodes.UNKNOWN, code); 236 throw new FHIRException("Unknown DeviceDispenseStatusCodes code '"+codeString+"'"); 237 } 238 public String toCode(DeviceDispenseStatusCodes code) { 239 if (code == DeviceDispenseStatusCodes.NULL) 240 return null; 241 if (code == DeviceDispenseStatusCodes.PREPARATION) 242 return "preparation"; 243 if (code == DeviceDispenseStatusCodes.INPROGRESS) 244 return "in-progress"; 245 if (code == DeviceDispenseStatusCodes.CANCELLED) 246 return "cancelled"; 247 if (code == DeviceDispenseStatusCodes.ONHOLD) 248 return "on-hold"; 249 if (code == DeviceDispenseStatusCodes.COMPLETED) 250 return "completed"; 251 if (code == DeviceDispenseStatusCodes.ENTEREDINERROR) 252 return "entered-in-error"; 253 if (code == DeviceDispenseStatusCodes.STOPPED) 254 return "stopped"; 255 if (code == DeviceDispenseStatusCodes.DECLINED) 256 return "declined"; 257 if (code == DeviceDispenseStatusCodes.UNKNOWN) 258 return "unknown"; 259 return "?"; 260 } 261 public String toSystem(DeviceDispenseStatusCodes code) { 262 return code.getSystem(); 263 } 264 } 265 266 @Block() 267 public static class DeviceDispensePerformerComponent extends BackboneElement implements IBaseBackboneElement { 268 /** 269 * Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker. 270 */ 271 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 272 @Description(shortDefinition="Who performed the dispense and what they did", formalDefinition="Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker." ) 273 protected CodeableConcept function; 274 275 /** 276 * The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the device. 277 */ 278 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, Device.class, RelatedPerson.class, CareTeam.class}, order=2, min=1, max=1, modifier=false, summary=false) 279 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the device." ) 280 protected Reference actor; 281 282 private static final long serialVersionUID = -576943815L; 283 284 /** 285 * Constructor 286 */ 287 public DeviceDispensePerformerComponent() { 288 super(); 289 } 290 291 /** 292 * Constructor 293 */ 294 public DeviceDispensePerformerComponent(Reference actor) { 295 super(); 296 this.setActor(actor); 297 } 298 299 /** 300 * @return {@link #function} (Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.) 301 */ 302 public CodeableConcept getFunction() { 303 if (this.function == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create DeviceDispensePerformerComponent.function"); 306 else if (Configuration.doAutoCreate()) 307 this.function = new CodeableConcept(); // cc 308 return this.function; 309 } 310 311 public boolean hasFunction() { 312 return this.function != null && !this.function.isEmpty(); 313 } 314 315 /** 316 * @param value {@link #function} (Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.) 317 */ 318 public DeviceDispensePerformerComponent setFunction(CodeableConcept value) { 319 this.function = value; 320 return this; 321 } 322 323 /** 324 * @return {@link #actor} (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the device.) 325 */ 326 public Reference getActor() { 327 if (this.actor == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create DeviceDispensePerformerComponent.actor"); 330 else if (Configuration.doAutoCreate()) 331 this.actor = new Reference(); // cc 332 return this.actor; 333 } 334 335 public boolean hasActor() { 336 return this.actor != null && !this.actor.isEmpty(); 337 } 338 339 /** 340 * @param value {@link #actor} (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the device.) 341 */ 342 public DeviceDispensePerformerComponent setActor(Reference value) { 343 this.actor = value; 344 return this; 345 } 346 347 protected void listChildren(List<Property> children) { 348 super.listChildren(children); 349 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.", 0, 1, function)); 350 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson|CareTeam)", "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the device.", 0, 1, actor)); 351 } 352 353 @Override 354 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 355 switch (_hash) { 356 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.", 0, 1, function); 357 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson|CareTeam)", "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the device.", 0, 1, actor); 358 default: return super.getNamedProperty(_hash, _name, _checkValid); 359 } 360 361 } 362 363 @Override 364 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 365 switch (hash) { 366 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 367 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 368 default: return super.getProperty(hash, name, checkValid); 369 } 370 371 } 372 373 @Override 374 public Base setProperty(int hash, String name, Base value) throws FHIRException { 375 switch (hash) { 376 case 1380938712: // function 377 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 378 return value; 379 case 92645877: // actor 380 this.actor = TypeConvertor.castToReference(value); // Reference 381 return value; 382 default: return super.setProperty(hash, name, value); 383 } 384 385 } 386 387 @Override 388 public Base setProperty(String name, Base value) throws FHIRException { 389 if (name.equals("function")) { 390 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 391 } else if (name.equals("actor")) { 392 this.actor = TypeConvertor.castToReference(value); // Reference 393 } else 394 return super.setProperty(name, value); 395 return value; 396 } 397 398 @Override 399 public void removeChild(String name, Base value) throws FHIRException { 400 if (name.equals("function")) { 401 this.function = null; 402 } else if (name.equals("actor")) { 403 this.actor = null; 404 } else 405 super.removeChild(name, value); 406 407 } 408 409 @Override 410 public Base makeProperty(int hash, String name) throws FHIRException { 411 switch (hash) { 412 case 1380938712: return getFunction(); 413 case 92645877: return getActor(); 414 default: return super.makeProperty(hash, name); 415 } 416 417 } 418 419 @Override 420 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 421 switch (hash) { 422 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 423 case 92645877: /*actor*/ return new String[] {"Reference"}; 424 default: return super.getTypesForProperty(hash, name); 425 } 426 427 } 428 429 @Override 430 public Base addChild(String name) throws FHIRException { 431 if (name.equals("function")) { 432 this.function = new CodeableConcept(); 433 return this.function; 434 } 435 else if (name.equals("actor")) { 436 this.actor = new Reference(); 437 return this.actor; 438 } 439 else 440 return super.addChild(name); 441 } 442 443 public DeviceDispensePerformerComponent copy() { 444 DeviceDispensePerformerComponent dst = new DeviceDispensePerformerComponent(); 445 copyValues(dst); 446 return dst; 447 } 448 449 public void copyValues(DeviceDispensePerformerComponent dst) { 450 super.copyValues(dst); 451 dst.function = function == null ? null : function.copy(); 452 dst.actor = actor == null ? null : actor.copy(); 453 } 454 455 @Override 456 public boolean equalsDeep(Base other_) { 457 if (!super.equalsDeep(other_)) 458 return false; 459 if (!(other_ instanceof DeviceDispensePerformerComponent)) 460 return false; 461 DeviceDispensePerformerComponent o = (DeviceDispensePerformerComponent) other_; 462 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 463 } 464 465 @Override 466 public boolean equalsShallow(Base other_) { 467 if (!super.equalsShallow(other_)) 468 return false; 469 if (!(other_ instanceof DeviceDispensePerformerComponent)) 470 return false; 471 DeviceDispensePerformerComponent o = (DeviceDispensePerformerComponent) other_; 472 return true; 473 } 474 475 public boolean isEmpty() { 476 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 477 } 478 479 public String fhirType() { 480 return "DeviceDispense.performer"; 481 482 } 483 484 } 485 486 /** 487 * Business identifier for this dispensation. 488 */ 489 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 490 @Description(shortDefinition="Business identifier for this dispensation", formalDefinition="Business identifier for this dispensation." ) 491 protected List<Identifier> identifier; 492 493 /** 494 * The order or request that this dispense is fulfilling. 495 */ 496 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 497 @Description(shortDefinition="The order or request that this dispense is fulfilling", formalDefinition="The order or request that this dispense is fulfilling." ) 498 protected List<Reference> basedOn; 499 500 /** 501 * The bigger event that this dispense is a part of. 502 */ 503 @Child(name = "partOf", type = {Procedure.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 504 @Description(shortDefinition="The bigger event that this dispense is a part of", formalDefinition="The bigger event that this dispense is a part of." ) 505 protected List<Reference> partOf; 506 507 /** 508 * A code specifying the state of the set of dispense events. 509 */ 510 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 511 @Description(shortDefinition="preparation | in-progress | cancelled | on-hold | completed | entered-in-error | stopped | declined | unknown", formalDefinition="A code specifying the state of the set of dispense events." ) 512 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicedispense-status") 513 protected Enumeration<DeviceDispenseStatusCodes> status; 514 515 /** 516 * Indicates the reason why a dispense was or was not performed. 517 */ 518 @Child(name = "statusReason", type = {CodeableReference.class}, order=4, min=0, max=1, modifier=false, summary=false) 519 @Description(shortDefinition="Why a dispense was or was not performed", formalDefinition="Indicates the reason why a dispense was or was not performed." ) 520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicedispense-status-reason") 521 protected CodeableReference statusReason; 522 523 /** 524 * Indicates the type of device dispense. 525 */ 526 @Child(name = "category", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 527 @Description(shortDefinition="Type of device dispense", formalDefinition="Indicates the type of device dispense." ) 528 protected List<CodeableConcept> category; 529 530 /** 531 * Identifies the device being dispensed. This is either a link to a resource representing the details of the device or a simple attribute carrying a code that identifies the device from a known list of devices. 532 */ 533 @Child(name = "device", type = {CodeableReference.class}, order=6, min=1, max=1, modifier=false, summary=true) 534 @Description(shortDefinition="What device was supplied", formalDefinition="Identifies the device being dispensed. This is either a link to a resource representing the details of the device or a simple attribute carrying a code that identifies the device from a known list of devices." ) 535 protected CodeableReference device; 536 537 /** 538 * A link to a resource representing the person to whom the device is intended. 539 */ 540 @Child(name = "subject", type = {Patient.class, Practitioner.class}, order=7, min=1, max=1, modifier=false, summary=true) 541 @Description(shortDefinition="Who the dispense is for", formalDefinition="A link to a resource representing the person to whom the device is intended." ) 542 protected Reference subject; 543 544 /** 545 * Identifies the person who picked up the device or the person or location where the device was delivered. This may be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location. 546 */ 547 @Child(name = "receiver", type = {Patient.class, Practitioner.class, RelatedPerson.class, Location.class, PractitionerRole.class}, order=8, min=0, max=1, modifier=false, summary=false) 548 @Description(shortDefinition="Who collected the device or where the medication was delivered", formalDefinition="Identifies the person who picked up the device or the person or location where the device was delivered. This may be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location." ) 549 protected Reference receiver; 550 551 /** 552 * The encounter that establishes the context for this event. 553 */ 554 @Child(name = "encounter", type = {Encounter.class}, order=9, min=0, max=1, modifier=false, summary=false) 555 @Description(shortDefinition="Encounter associated with event", formalDefinition="The encounter that establishes the context for this event." ) 556 protected Reference encounter; 557 558 /** 559 * Additional information that supports the device being dispensed. 560 */ 561 @Child(name = "supportingInformation", type = {Reference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 562 @Description(shortDefinition="Information that supports the dispensing of the device", formalDefinition="Additional information that supports the device being dispensed." ) 563 protected List<Reference> supportingInformation; 564 565 /** 566 * Indicates who or what performed the event. 567 */ 568 @Child(name = "performer", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 569 @Description(shortDefinition="Who performed event", formalDefinition="Indicates who or what performed the event." ) 570 protected List<DeviceDispensePerformerComponent> performer; 571 572 /** 573 * The principal physical location where the dispense was performed. 574 */ 575 @Child(name = "location", type = {Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 576 @Description(shortDefinition="Where the dispense occurred", formalDefinition="The principal physical location where the dispense was performed." ) 577 protected Reference location; 578 579 /** 580 * Indicates the type of dispensing event that is performed. 581 */ 582 @Child(name = "type", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 583 @Description(shortDefinition="Trial fill, partial fill, emergency fill, etc", formalDefinition="Indicates the type of dispensing event that is performed." ) 584 protected CodeableConcept type; 585 586 /** 587 * The number of devices that have been dispensed. 588 */ 589 @Child(name = "quantity", type = {Quantity.class}, order=14, min=0, max=1, modifier=false, summary=false) 590 @Description(shortDefinition="Amount dispensed", formalDefinition="The number of devices that have been dispensed." ) 591 protected Quantity quantity; 592 593 /** 594 * The time when the dispensed product was packaged and reviewed. 595 */ 596 @Child(name = "preparedDate", type = {DateTimeType.class}, order=15, min=0, max=1, modifier=false, summary=true) 597 @Description(shortDefinition="When product was packaged and reviewed", formalDefinition="The time when the dispensed product was packaged and reviewed." ) 598 protected DateTimeType preparedDate; 599 600 /** 601 * The time the dispensed product was made available to the patient or their representative. 602 */ 603 @Child(name = "whenHandedOver", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=false) 604 @Description(shortDefinition="When product was given out", formalDefinition="The time the dispensed product was made available to the patient or their representative." ) 605 protected DateTimeType whenHandedOver; 606 607 /** 608 * Identification of the facility/location where the device was /should be shipped to, as part of the dispense process. 609 */ 610 @Child(name = "destination", type = {Location.class}, order=17, min=0, max=1, modifier=false, summary=false) 611 @Description(shortDefinition="Where the device was sent or should be sent", formalDefinition="Identification of the facility/location where the device was /should be shipped to, as part of the dispense process." ) 612 protected Reference destination; 613 614 /** 615 * Extra information about the dispense that could not be conveyed in the other attributes. 616 */ 617 @Child(name = "note", type = {Annotation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 618 @Description(shortDefinition="Information about the dispense", formalDefinition="Extra information about the dispense that could not be conveyed in the other attributes." ) 619 protected List<Annotation> note; 620 621 /** 622 * The full representation of the instructions. 623 */ 624 @Child(name = "usageInstruction", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 625 @Description(shortDefinition="Full representation of the usage instructions", formalDefinition="The full representation of the instructions." ) 626 protected MarkdownType usageInstruction; 627 628 /** 629 * A summary of the events of interest that have occurred, such as when the dispense was verified. 630 */ 631 @Child(name = "eventHistory", type = {Provenance.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 632 @Description(shortDefinition="A list of relevant lifecycle events", formalDefinition="A summary of the events of interest that have occurred, such as when the dispense was verified." ) 633 protected List<Reference> eventHistory; 634 635 private static final long serialVersionUID = 1740231007L; 636 637 /** 638 * Constructor 639 */ 640 public DeviceDispense() { 641 super(); 642 } 643 644 /** 645 * Constructor 646 */ 647 public DeviceDispense(DeviceDispenseStatusCodes status, CodeableReference device, Reference subject) { 648 super(); 649 this.setStatus(status); 650 this.setDevice(device); 651 this.setSubject(subject); 652 } 653 654 /** 655 * @return {@link #identifier} (Business identifier for this dispensation.) 656 */ 657 public List<Identifier> getIdentifier() { 658 if (this.identifier == null) 659 this.identifier = new ArrayList<Identifier>(); 660 return this.identifier; 661 } 662 663 /** 664 * @return Returns a reference to <code>this</code> for easy method chaining 665 */ 666 public DeviceDispense setIdentifier(List<Identifier> theIdentifier) { 667 this.identifier = theIdentifier; 668 return this; 669 } 670 671 public boolean hasIdentifier() { 672 if (this.identifier == null) 673 return false; 674 for (Identifier item : this.identifier) 675 if (!item.isEmpty()) 676 return true; 677 return false; 678 } 679 680 public Identifier addIdentifier() { //3 681 Identifier t = new Identifier(); 682 if (this.identifier == null) 683 this.identifier = new ArrayList<Identifier>(); 684 this.identifier.add(t); 685 return t; 686 } 687 688 public DeviceDispense addIdentifier(Identifier t) { //3 689 if (t == null) 690 return this; 691 if (this.identifier == null) 692 this.identifier = new ArrayList<Identifier>(); 693 this.identifier.add(t); 694 return this; 695 } 696 697 /** 698 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 699 */ 700 public Identifier getIdentifierFirstRep() { 701 if (getIdentifier().isEmpty()) { 702 addIdentifier(); 703 } 704 return getIdentifier().get(0); 705 } 706 707 /** 708 * @return {@link #basedOn} (The order or request that this dispense is fulfilling.) 709 */ 710 public List<Reference> getBasedOn() { 711 if (this.basedOn == null) 712 this.basedOn = new ArrayList<Reference>(); 713 return this.basedOn; 714 } 715 716 /** 717 * @return Returns a reference to <code>this</code> for easy method chaining 718 */ 719 public DeviceDispense setBasedOn(List<Reference> theBasedOn) { 720 this.basedOn = theBasedOn; 721 return this; 722 } 723 724 public boolean hasBasedOn() { 725 if (this.basedOn == null) 726 return false; 727 for (Reference item : this.basedOn) 728 if (!item.isEmpty()) 729 return true; 730 return false; 731 } 732 733 public Reference addBasedOn() { //3 734 Reference t = new Reference(); 735 if (this.basedOn == null) 736 this.basedOn = new ArrayList<Reference>(); 737 this.basedOn.add(t); 738 return t; 739 } 740 741 public DeviceDispense addBasedOn(Reference t) { //3 742 if (t == null) 743 return this; 744 if (this.basedOn == null) 745 this.basedOn = new ArrayList<Reference>(); 746 this.basedOn.add(t); 747 return this; 748 } 749 750 /** 751 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 752 */ 753 public Reference getBasedOnFirstRep() { 754 if (getBasedOn().isEmpty()) { 755 addBasedOn(); 756 } 757 return getBasedOn().get(0); 758 } 759 760 /** 761 * @return {@link #partOf} (The bigger event that this dispense is a part of.) 762 */ 763 public List<Reference> getPartOf() { 764 if (this.partOf == null) 765 this.partOf = new ArrayList<Reference>(); 766 return this.partOf; 767 } 768 769 /** 770 * @return Returns a reference to <code>this</code> for easy method chaining 771 */ 772 public DeviceDispense setPartOf(List<Reference> thePartOf) { 773 this.partOf = thePartOf; 774 return this; 775 } 776 777 public boolean hasPartOf() { 778 if (this.partOf == null) 779 return false; 780 for (Reference item : this.partOf) 781 if (!item.isEmpty()) 782 return true; 783 return false; 784 } 785 786 public Reference addPartOf() { //3 787 Reference t = new Reference(); 788 if (this.partOf == null) 789 this.partOf = new ArrayList<Reference>(); 790 this.partOf.add(t); 791 return t; 792 } 793 794 public DeviceDispense addPartOf(Reference t) { //3 795 if (t == null) 796 return this; 797 if (this.partOf == null) 798 this.partOf = new ArrayList<Reference>(); 799 this.partOf.add(t); 800 return this; 801 } 802 803 /** 804 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 805 */ 806 public Reference getPartOfFirstRep() { 807 if (getPartOf().isEmpty()) { 808 addPartOf(); 809 } 810 return getPartOf().get(0); 811 } 812 813 /** 814 * @return {@link #status} (A code specifying the state of the set of dispense events.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 815 */ 816 public Enumeration<DeviceDispenseStatusCodes> getStatusElement() { 817 if (this.status == null) 818 if (Configuration.errorOnAutoCreate()) 819 throw new Error("Attempt to auto-create DeviceDispense.status"); 820 else if (Configuration.doAutoCreate()) 821 this.status = new Enumeration<DeviceDispenseStatusCodes>(new DeviceDispenseStatusCodesEnumFactory()); // bb 822 return this.status; 823 } 824 825 public boolean hasStatusElement() { 826 return this.status != null && !this.status.isEmpty(); 827 } 828 829 public boolean hasStatus() { 830 return this.status != null && !this.status.isEmpty(); 831 } 832 833 /** 834 * @param value {@link #status} (A code specifying the state of the set of dispense events.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 835 */ 836 public DeviceDispense setStatusElement(Enumeration<DeviceDispenseStatusCodes> value) { 837 this.status = value; 838 return this; 839 } 840 841 /** 842 * @return A code specifying the state of the set of dispense events. 843 */ 844 public DeviceDispenseStatusCodes getStatus() { 845 return this.status == null ? null : this.status.getValue(); 846 } 847 848 /** 849 * @param value A code specifying the state of the set of dispense events. 850 */ 851 public DeviceDispense setStatus(DeviceDispenseStatusCodes value) { 852 if (this.status == null) 853 this.status = new Enumeration<DeviceDispenseStatusCodes>(new DeviceDispenseStatusCodesEnumFactory()); 854 this.status.setValue(value); 855 return this; 856 } 857 858 /** 859 * @return {@link #statusReason} (Indicates the reason why a dispense was or was not performed.) 860 */ 861 public CodeableReference getStatusReason() { 862 if (this.statusReason == null) 863 if (Configuration.errorOnAutoCreate()) 864 throw new Error("Attempt to auto-create DeviceDispense.statusReason"); 865 else if (Configuration.doAutoCreate()) 866 this.statusReason = new CodeableReference(); // cc 867 return this.statusReason; 868 } 869 870 public boolean hasStatusReason() { 871 return this.statusReason != null && !this.statusReason.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #statusReason} (Indicates the reason why a dispense was or was not performed.) 876 */ 877 public DeviceDispense setStatusReason(CodeableReference value) { 878 this.statusReason = value; 879 return this; 880 } 881 882 /** 883 * @return {@link #category} (Indicates the type of device dispense.) 884 */ 885 public List<CodeableConcept> getCategory() { 886 if (this.category == null) 887 this.category = new ArrayList<CodeableConcept>(); 888 return this.category; 889 } 890 891 /** 892 * @return Returns a reference to <code>this</code> for easy method chaining 893 */ 894 public DeviceDispense setCategory(List<CodeableConcept> theCategory) { 895 this.category = theCategory; 896 return this; 897 } 898 899 public boolean hasCategory() { 900 if (this.category == null) 901 return false; 902 for (CodeableConcept item : this.category) 903 if (!item.isEmpty()) 904 return true; 905 return false; 906 } 907 908 public CodeableConcept addCategory() { //3 909 CodeableConcept t = new CodeableConcept(); 910 if (this.category == null) 911 this.category = new ArrayList<CodeableConcept>(); 912 this.category.add(t); 913 return t; 914 } 915 916 public DeviceDispense addCategory(CodeableConcept t) { //3 917 if (t == null) 918 return this; 919 if (this.category == null) 920 this.category = new ArrayList<CodeableConcept>(); 921 this.category.add(t); 922 return this; 923 } 924 925 /** 926 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 927 */ 928 public CodeableConcept getCategoryFirstRep() { 929 if (getCategory().isEmpty()) { 930 addCategory(); 931 } 932 return getCategory().get(0); 933 } 934 935 /** 936 * @return {@link #device} (Identifies the device being dispensed. This is either a link to a resource representing the details of the device or a simple attribute carrying a code that identifies the device from a known list of devices.) 937 */ 938 public CodeableReference getDevice() { 939 if (this.device == null) 940 if (Configuration.errorOnAutoCreate()) 941 throw new Error("Attempt to auto-create DeviceDispense.device"); 942 else if (Configuration.doAutoCreate()) 943 this.device = new CodeableReference(); // cc 944 return this.device; 945 } 946 947 public boolean hasDevice() { 948 return this.device != null && !this.device.isEmpty(); 949 } 950 951 /** 952 * @param value {@link #device} (Identifies the device being dispensed. This is either a link to a resource representing the details of the device or a simple attribute carrying a code that identifies the device from a known list of devices.) 953 */ 954 public DeviceDispense setDevice(CodeableReference value) { 955 this.device = value; 956 return this; 957 } 958 959 /** 960 * @return {@link #subject} (A link to a resource representing the person to whom the device is intended.) 961 */ 962 public Reference getSubject() { 963 if (this.subject == null) 964 if (Configuration.errorOnAutoCreate()) 965 throw new Error("Attempt to auto-create DeviceDispense.subject"); 966 else if (Configuration.doAutoCreate()) 967 this.subject = new Reference(); // cc 968 return this.subject; 969 } 970 971 public boolean hasSubject() { 972 return this.subject != null && !this.subject.isEmpty(); 973 } 974 975 /** 976 * @param value {@link #subject} (A link to a resource representing the person to whom the device is intended.) 977 */ 978 public DeviceDispense setSubject(Reference value) { 979 this.subject = value; 980 return this; 981 } 982 983 /** 984 * @return {@link #receiver} (Identifies the person who picked up the device or the person or location where the device was delivered. This may be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location.) 985 */ 986 public Reference getReceiver() { 987 if (this.receiver == null) 988 if (Configuration.errorOnAutoCreate()) 989 throw new Error("Attempt to auto-create DeviceDispense.receiver"); 990 else if (Configuration.doAutoCreate()) 991 this.receiver = new Reference(); // cc 992 return this.receiver; 993 } 994 995 public boolean hasReceiver() { 996 return this.receiver != null && !this.receiver.isEmpty(); 997 } 998 999 /** 1000 * @param value {@link #receiver} (Identifies the person who picked up the device or the person or location where the device was delivered. This may be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location.) 1001 */ 1002 public DeviceDispense setReceiver(Reference value) { 1003 this.receiver = value; 1004 return this; 1005 } 1006 1007 /** 1008 * @return {@link #encounter} (The encounter that establishes the context for this event.) 1009 */ 1010 public Reference getEncounter() { 1011 if (this.encounter == null) 1012 if (Configuration.errorOnAutoCreate()) 1013 throw new Error("Attempt to auto-create DeviceDispense.encounter"); 1014 else if (Configuration.doAutoCreate()) 1015 this.encounter = new Reference(); // cc 1016 return this.encounter; 1017 } 1018 1019 public boolean hasEncounter() { 1020 return this.encounter != null && !this.encounter.isEmpty(); 1021 } 1022 1023 /** 1024 * @param value {@link #encounter} (The encounter that establishes the context for this event.) 1025 */ 1026 public DeviceDispense setEncounter(Reference value) { 1027 this.encounter = value; 1028 return this; 1029 } 1030 1031 /** 1032 * @return {@link #supportingInformation} (Additional information that supports the device being dispensed.) 1033 */ 1034 public List<Reference> getSupportingInformation() { 1035 if (this.supportingInformation == null) 1036 this.supportingInformation = new ArrayList<Reference>(); 1037 return this.supportingInformation; 1038 } 1039 1040 /** 1041 * @return Returns a reference to <code>this</code> for easy method chaining 1042 */ 1043 public DeviceDispense setSupportingInformation(List<Reference> theSupportingInformation) { 1044 this.supportingInformation = theSupportingInformation; 1045 return this; 1046 } 1047 1048 public boolean hasSupportingInformation() { 1049 if (this.supportingInformation == null) 1050 return false; 1051 for (Reference item : this.supportingInformation) 1052 if (!item.isEmpty()) 1053 return true; 1054 return false; 1055 } 1056 1057 public Reference addSupportingInformation() { //3 1058 Reference t = new Reference(); 1059 if (this.supportingInformation == null) 1060 this.supportingInformation = new ArrayList<Reference>(); 1061 this.supportingInformation.add(t); 1062 return t; 1063 } 1064 1065 public DeviceDispense addSupportingInformation(Reference t) { //3 1066 if (t == null) 1067 return this; 1068 if (this.supportingInformation == null) 1069 this.supportingInformation = new ArrayList<Reference>(); 1070 this.supportingInformation.add(t); 1071 return this; 1072 } 1073 1074 /** 1075 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 1076 */ 1077 public Reference getSupportingInformationFirstRep() { 1078 if (getSupportingInformation().isEmpty()) { 1079 addSupportingInformation(); 1080 } 1081 return getSupportingInformation().get(0); 1082 } 1083 1084 /** 1085 * @return {@link #performer} (Indicates who or what performed the event.) 1086 */ 1087 public List<DeviceDispensePerformerComponent> getPerformer() { 1088 if (this.performer == null) 1089 this.performer = new ArrayList<DeviceDispensePerformerComponent>(); 1090 return this.performer; 1091 } 1092 1093 /** 1094 * @return Returns a reference to <code>this</code> for easy method chaining 1095 */ 1096 public DeviceDispense setPerformer(List<DeviceDispensePerformerComponent> thePerformer) { 1097 this.performer = thePerformer; 1098 return this; 1099 } 1100 1101 public boolean hasPerformer() { 1102 if (this.performer == null) 1103 return false; 1104 for (DeviceDispensePerformerComponent item : this.performer) 1105 if (!item.isEmpty()) 1106 return true; 1107 return false; 1108 } 1109 1110 public DeviceDispensePerformerComponent addPerformer() { //3 1111 DeviceDispensePerformerComponent t = new DeviceDispensePerformerComponent(); 1112 if (this.performer == null) 1113 this.performer = new ArrayList<DeviceDispensePerformerComponent>(); 1114 this.performer.add(t); 1115 return t; 1116 } 1117 1118 public DeviceDispense addPerformer(DeviceDispensePerformerComponent t) { //3 1119 if (t == null) 1120 return this; 1121 if (this.performer == null) 1122 this.performer = new ArrayList<DeviceDispensePerformerComponent>(); 1123 this.performer.add(t); 1124 return this; 1125 } 1126 1127 /** 1128 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1129 */ 1130 public DeviceDispensePerformerComponent getPerformerFirstRep() { 1131 if (getPerformer().isEmpty()) { 1132 addPerformer(); 1133 } 1134 return getPerformer().get(0); 1135 } 1136 1137 /** 1138 * @return {@link #location} (The principal physical location where the dispense was performed.) 1139 */ 1140 public Reference getLocation() { 1141 if (this.location == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create DeviceDispense.location"); 1144 else if (Configuration.doAutoCreate()) 1145 this.location = new Reference(); // cc 1146 return this.location; 1147 } 1148 1149 public boolean hasLocation() { 1150 return this.location != null && !this.location.isEmpty(); 1151 } 1152 1153 /** 1154 * @param value {@link #location} (The principal physical location where the dispense was performed.) 1155 */ 1156 public DeviceDispense setLocation(Reference value) { 1157 this.location = value; 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #type} (Indicates the type of dispensing event that is performed.) 1163 */ 1164 public CodeableConcept getType() { 1165 if (this.type == null) 1166 if (Configuration.errorOnAutoCreate()) 1167 throw new Error("Attempt to auto-create DeviceDispense.type"); 1168 else if (Configuration.doAutoCreate()) 1169 this.type = new CodeableConcept(); // cc 1170 return this.type; 1171 } 1172 1173 public boolean hasType() { 1174 return this.type != null && !this.type.isEmpty(); 1175 } 1176 1177 /** 1178 * @param value {@link #type} (Indicates the type of dispensing event that is performed.) 1179 */ 1180 public DeviceDispense setType(CodeableConcept value) { 1181 this.type = value; 1182 return this; 1183 } 1184 1185 /** 1186 * @return {@link #quantity} (The number of devices that have been dispensed.) 1187 */ 1188 public Quantity getQuantity() { 1189 if (this.quantity == null) 1190 if (Configuration.errorOnAutoCreate()) 1191 throw new Error("Attempt to auto-create DeviceDispense.quantity"); 1192 else if (Configuration.doAutoCreate()) 1193 this.quantity = new Quantity(); // cc 1194 return this.quantity; 1195 } 1196 1197 public boolean hasQuantity() { 1198 return this.quantity != null && !this.quantity.isEmpty(); 1199 } 1200 1201 /** 1202 * @param value {@link #quantity} (The number of devices that have been dispensed.) 1203 */ 1204 public DeviceDispense setQuantity(Quantity value) { 1205 this.quantity = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return {@link #preparedDate} (The time when the dispensed product was packaged and reviewed.). This is the underlying object with id, value and extensions. The accessor "getPreparedDate" gives direct access to the value 1211 */ 1212 public DateTimeType getPreparedDateElement() { 1213 if (this.preparedDate == null) 1214 if (Configuration.errorOnAutoCreate()) 1215 throw new Error("Attempt to auto-create DeviceDispense.preparedDate"); 1216 else if (Configuration.doAutoCreate()) 1217 this.preparedDate = new DateTimeType(); // bb 1218 return this.preparedDate; 1219 } 1220 1221 public boolean hasPreparedDateElement() { 1222 return this.preparedDate != null && !this.preparedDate.isEmpty(); 1223 } 1224 1225 public boolean hasPreparedDate() { 1226 return this.preparedDate != null && !this.preparedDate.isEmpty(); 1227 } 1228 1229 /** 1230 * @param value {@link #preparedDate} (The time when the dispensed product was packaged and reviewed.). This is the underlying object with id, value and extensions. The accessor "getPreparedDate" gives direct access to the value 1231 */ 1232 public DeviceDispense setPreparedDateElement(DateTimeType value) { 1233 this.preparedDate = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return The time when the dispensed product was packaged and reviewed. 1239 */ 1240 public Date getPreparedDate() { 1241 return this.preparedDate == null ? null : this.preparedDate.getValue(); 1242 } 1243 1244 /** 1245 * @param value The time when the dispensed product was packaged and reviewed. 1246 */ 1247 public DeviceDispense setPreparedDate(Date value) { 1248 if (value == null) 1249 this.preparedDate = null; 1250 else { 1251 if (this.preparedDate == null) 1252 this.preparedDate = new DateTimeType(); 1253 this.preparedDate.setValue(value); 1254 } 1255 return this; 1256 } 1257 1258 /** 1259 * @return {@link #whenHandedOver} (The time the dispensed product was made available to the patient or their representative.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1260 */ 1261 public DateTimeType getWhenHandedOverElement() { 1262 if (this.whenHandedOver == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create DeviceDispense.whenHandedOver"); 1265 else if (Configuration.doAutoCreate()) 1266 this.whenHandedOver = new DateTimeType(); // bb 1267 return this.whenHandedOver; 1268 } 1269 1270 public boolean hasWhenHandedOverElement() { 1271 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1272 } 1273 1274 public boolean hasWhenHandedOver() { 1275 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #whenHandedOver} (The time the dispensed product was made available to the patient or their representative.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1280 */ 1281 public DeviceDispense setWhenHandedOverElement(DateTimeType value) { 1282 this.whenHandedOver = value; 1283 return this; 1284 } 1285 1286 /** 1287 * @return The time the dispensed product was made available to the patient or their representative. 1288 */ 1289 public Date getWhenHandedOver() { 1290 return this.whenHandedOver == null ? null : this.whenHandedOver.getValue(); 1291 } 1292 1293 /** 1294 * @param value The time the dispensed product was made available to the patient or their representative. 1295 */ 1296 public DeviceDispense setWhenHandedOver(Date value) { 1297 if (value == null) 1298 this.whenHandedOver = null; 1299 else { 1300 if (this.whenHandedOver == null) 1301 this.whenHandedOver = new DateTimeType(); 1302 this.whenHandedOver.setValue(value); 1303 } 1304 return this; 1305 } 1306 1307 /** 1308 * @return {@link #destination} (Identification of the facility/location where the device was /should be shipped to, as part of the dispense process.) 1309 */ 1310 public Reference getDestination() { 1311 if (this.destination == null) 1312 if (Configuration.errorOnAutoCreate()) 1313 throw new Error("Attempt to auto-create DeviceDispense.destination"); 1314 else if (Configuration.doAutoCreate()) 1315 this.destination = new Reference(); // cc 1316 return this.destination; 1317 } 1318 1319 public boolean hasDestination() { 1320 return this.destination != null && !this.destination.isEmpty(); 1321 } 1322 1323 /** 1324 * @param value {@link #destination} (Identification of the facility/location where the device was /should be shipped to, as part of the dispense process.) 1325 */ 1326 public DeviceDispense setDestination(Reference value) { 1327 this.destination = value; 1328 return this; 1329 } 1330 1331 /** 1332 * @return {@link #note} (Extra information about the dispense that could not be conveyed in the other attributes.) 1333 */ 1334 public List<Annotation> getNote() { 1335 if (this.note == null) 1336 this.note = new ArrayList<Annotation>(); 1337 return this.note; 1338 } 1339 1340 /** 1341 * @return Returns a reference to <code>this</code> for easy method chaining 1342 */ 1343 public DeviceDispense setNote(List<Annotation> theNote) { 1344 this.note = theNote; 1345 return this; 1346 } 1347 1348 public boolean hasNote() { 1349 if (this.note == null) 1350 return false; 1351 for (Annotation item : this.note) 1352 if (!item.isEmpty()) 1353 return true; 1354 return false; 1355 } 1356 1357 public Annotation addNote() { //3 1358 Annotation t = new Annotation(); 1359 if (this.note == null) 1360 this.note = new ArrayList<Annotation>(); 1361 this.note.add(t); 1362 return t; 1363 } 1364 1365 public DeviceDispense addNote(Annotation t) { //3 1366 if (t == null) 1367 return this; 1368 if (this.note == null) 1369 this.note = new ArrayList<Annotation>(); 1370 this.note.add(t); 1371 return this; 1372 } 1373 1374 /** 1375 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1376 */ 1377 public Annotation getNoteFirstRep() { 1378 if (getNote().isEmpty()) { 1379 addNote(); 1380 } 1381 return getNote().get(0); 1382 } 1383 1384 /** 1385 * @return {@link #usageInstruction} (The full representation of the instructions.). This is the underlying object with id, value and extensions. The accessor "getUsageInstruction" gives direct access to the value 1386 */ 1387 public MarkdownType getUsageInstructionElement() { 1388 if (this.usageInstruction == null) 1389 if (Configuration.errorOnAutoCreate()) 1390 throw new Error("Attempt to auto-create DeviceDispense.usageInstruction"); 1391 else if (Configuration.doAutoCreate()) 1392 this.usageInstruction = new MarkdownType(); // bb 1393 return this.usageInstruction; 1394 } 1395 1396 public boolean hasUsageInstructionElement() { 1397 return this.usageInstruction != null && !this.usageInstruction.isEmpty(); 1398 } 1399 1400 public boolean hasUsageInstruction() { 1401 return this.usageInstruction != null && !this.usageInstruction.isEmpty(); 1402 } 1403 1404 /** 1405 * @param value {@link #usageInstruction} (The full representation of the instructions.). This is the underlying object with id, value and extensions. The accessor "getUsageInstruction" gives direct access to the value 1406 */ 1407 public DeviceDispense setUsageInstructionElement(MarkdownType value) { 1408 this.usageInstruction = value; 1409 return this; 1410 } 1411 1412 /** 1413 * @return The full representation of the instructions. 1414 */ 1415 public String getUsageInstruction() { 1416 return this.usageInstruction == null ? null : this.usageInstruction.getValue(); 1417 } 1418 1419 /** 1420 * @param value The full representation of the instructions. 1421 */ 1422 public DeviceDispense setUsageInstruction(String value) { 1423 if (Utilities.noString(value)) 1424 this.usageInstruction = null; 1425 else { 1426 if (this.usageInstruction == null) 1427 this.usageInstruction = new MarkdownType(); 1428 this.usageInstruction.setValue(value); 1429 } 1430 return this; 1431 } 1432 1433 /** 1434 * @return {@link #eventHistory} (A summary of the events of interest that have occurred, such as when the dispense was verified.) 1435 */ 1436 public List<Reference> getEventHistory() { 1437 if (this.eventHistory == null) 1438 this.eventHistory = new ArrayList<Reference>(); 1439 return this.eventHistory; 1440 } 1441 1442 /** 1443 * @return Returns a reference to <code>this</code> for easy method chaining 1444 */ 1445 public DeviceDispense setEventHistory(List<Reference> theEventHistory) { 1446 this.eventHistory = theEventHistory; 1447 return this; 1448 } 1449 1450 public boolean hasEventHistory() { 1451 if (this.eventHistory == null) 1452 return false; 1453 for (Reference item : this.eventHistory) 1454 if (!item.isEmpty()) 1455 return true; 1456 return false; 1457 } 1458 1459 public Reference addEventHistory() { //3 1460 Reference t = new Reference(); 1461 if (this.eventHistory == null) 1462 this.eventHistory = new ArrayList<Reference>(); 1463 this.eventHistory.add(t); 1464 return t; 1465 } 1466 1467 public DeviceDispense addEventHistory(Reference t) { //3 1468 if (t == null) 1469 return this; 1470 if (this.eventHistory == null) 1471 this.eventHistory = new ArrayList<Reference>(); 1472 this.eventHistory.add(t); 1473 return this; 1474 } 1475 1476 /** 1477 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist {3} 1478 */ 1479 public Reference getEventHistoryFirstRep() { 1480 if (getEventHistory().isEmpty()) { 1481 addEventHistory(); 1482 } 1483 return getEventHistory().get(0); 1484 } 1485 1486 protected void listChildren(List<Property> children) { 1487 super.listChildren(children); 1488 children.add(new Property("identifier", "Identifier", "Business identifier for this dispensation.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1489 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest)", "The order or request that this dispense is fulfilling.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1490 children.add(new Property("partOf", "Reference(Procedure)", "The bigger event that this dispense is a part of.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1491 children.add(new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 1, status)); 1492 children.add(new Property("statusReason", "CodeableReference(DetectedIssue)", "Indicates the reason why a dispense was or was not performed.", 0, 1, statusReason)); 1493 children.add(new Property("category", "CodeableConcept", "Indicates the type of device dispense.", 0, java.lang.Integer.MAX_VALUE, category)); 1494 children.add(new Property("device", "CodeableReference(Device|DeviceDefinition)", "Identifies the device being dispensed. This is either a link to a resource representing the details of the device or a simple attribute carrying a code that identifies the device from a known list of devices.", 0, 1, device)); 1495 children.add(new Property("subject", "Reference(Patient|Practitioner)", "A link to a resource representing the person to whom the device is intended.", 0, 1, subject)); 1496 children.add(new Property("receiver", "Reference(Patient|Practitioner|RelatedPerson|Location|PractitionerRole)", "Identifies the person who picked up the device or the person or location where the device was delivered. This may be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location.", 0, 1, receiver)); 1497 children.add(new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this event.", 0, 1, encounter)); 1498 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information that supports the device being dispensed.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 1499 children.add(new Property("performer", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, performer)); 1500 children.add(new Property("location", "Reference(Location)", "The principal physical location where the dispense was performed.", 0, 1, location)); 1501 children.add(new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed.", 0, 1, type)); 1502 children.add(new Property("quantity", "Quantity", "The number of devices that have been dispensed.", 0, 1, quantity)); 1503 children.add(new Property("preparedDate", "dateTime", "The time when the dispensed product was packaged and reviewed.", 0, 1, preparedDate)); 1504 children.add(new Property("whenHandedOver", "dateTime", "The time the dispensed product was made available to the patient or their representative.", 0, 1, whenHandedOver)); 1505 children.add(new Property("destination", "Reference(Location)", "Identification of the facility/location where the device was /should be shipped to, as part of the dispense process.", 0, 1, destination)); 1506 children.add(new Property("note", "Annotation", "Extra information about the dispense that could not be conveyed in the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 1507 children.add(new Property("usageInstruction", "markdown", "The full representation of the instructions.", 0, 1, usageInstruction)); 1508 children.add(new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 1509 } 1510 1511 @Override 1512 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1513 switch (_hash) { 1514 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for this dispensation.", 0, java.lang.Integer.MAX_VALUE, identifier); 1515 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest)", "The order or request that this dispense is fulfilling.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1516 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure)", "The bigger event that this dispense is a part of.", 0, java.lang.Integer.MAX_VALUE, partOf); 1517 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 1, status); 1518 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableReference(DetectedIssue)", "Indicates the reason why a dispense was or was not performed.", 0, 1, statusReason); 1519 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates the type of device dispense.", 0, java.lang.Integer.MAX_VALUE, category); 1520 case -1335157162: /*device*/ return new Property("device", "CodeableReference(Device|DeviceDefinition)", "Identifies the device being dispensed. This is either a link to a resource representing the details of the device or a simple attribute carrying a code that identifies the device from a known list of devices.", 0, 1, device); 1521 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Practitioner)", "A link to a resource representing the person to whom the device is intended.", 0, 1, subject); 1522 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Patient|Practitioner|RelatedPerson|Location|PractitionerRole)", "Identifies the person who picked up the device or the person or location where the device was delivered. This may be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location.", 0, 1, receiver); 1523 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this event.", 0, 1, encounter); 1524 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information that supports the device being dispensed.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 1525 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, performer); 1526 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The principal physical location where the dispense was performed.", 0, 1, location); 1527 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed.", 0, 1, type); 1528 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of devices that have been dispensed.", 0, 1, quantity); 1529 case -2024959605: /*preparedDate*/ return new Property("preparedDate", "dateTime", "The time when the dispensed product was packaged and reviewed.", 0, 1, preparedDate); 1530 case -940241380: /*whenHandedOver*/ return new Property("whenHandedOver", "dateTime", "The time the dispensed product was made available to the patient or their representative.", 0, 1, whenHandedOver); 1531 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Identification of the facility/location where the device was /should be shipped to, as part of the dispense process.", 0, 1, destination); 1532 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the dispense that could not be conveyed in the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 1533 case 2138372141: /*usageInstruction*/ return new Property("usageInstruction", "markdown", "The full representation of the instructions.", 0, 1, usageInstruction); 1534 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 1535 default: return super.getNamedProperty(_hash, _name, _checkValid); 1536 } 1537 1538 } 1539 1540 @Override 1541 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1542 switch (hash) { 1543 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1544 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1545 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1546 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DeviceDispenseStatusCodes> 1547 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableReference 1548 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1549 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // CodeableReference 1550 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1551 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : new Base[] {this.receiver}; // Reference 1552 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1553 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 1554 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // DeviceDispensePerformerComponent 1555 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1556 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1557 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1558 case -2024959605: /*preparedDate*/ return this.preparedDate == null ? new Base[0] : new Base[] {this.preparedDate}; // DateTimeType 1559 case -940241380: /*whenHandedOver*/ return this.whenHandedOver == null ? new Base[0] : new Base[] {this.whenHandedOver}; // DateTimeType 1560 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 1561 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1562 case 2138372141: /*usageInstruction*/ return this.usageInstruction == null ? new Base[0] : new Base[] {this.usageInstruction}; // MarkdownType 1563 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 1564 default: return super.getProperty(hash, name, checkValid); 1565 } 1566 1567 } 1568 1569 @Override 1570 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1571 switch (hash) { 1572 case -1618432855: // identifier 1573 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1574 return value; 1575 case -332612366: // basedOn 1576 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1577 return value; 1578 case -995410646: // partOf 1579 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1580 return value; 1581 case -892481550: // status 1582 value = new DeviceDispenseStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1583 this.status = (Enumeration) value; // Enumeration<DeviceDispenseStatusCodes> 1584 return value; 1585 case 2051346646: // statusReason 1586 this.statusReason = TypeConvertor.castToCodeableReference(value); // CodeableReference 1587 return value; 1588 case 50511102: // category 1589 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1590 return value; 1591 case -1335157162: // device 1592 this.device = TypeConvertor.castToCodeableReference(value); // CodeableReference 1593 return value; 1594 case -1867885268: // subject 1595 this.subject = TypeConvertor.castToReference(value); // Reference 1596 return value; 1597 case -808719889: // receiver 1598 this.receiver = TypeConvertor.castToReference(value); // Reference 1599 return value; 1600 case 1524132147: // encounter 1601 this.encounter = TypeConvertor.castToReference(value); // Reference 1602 return value; 1603 case -1248768647: // supportingInformation 1604 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 1605 return value; 1606 case 481140686: // performer 1607 this.getPerformer().add((DeviceDispensePerformerComponent) value); // DeviceDispensePerformerComponent 1608 return value; 1609 case 1901043637: // location 1610 this.location = TypeConvertor.castToReference(value); // Reference 1611 return value; 1612 case 3575610: // type 1613 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1614 return value; 1615 case -1285004149: // quantity 1616 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1617 return value; 1618 case -2024959605: // preparedDate 1619 this.preparedDate = TypeConvertor.castToDateTime(value); // DateTimeType 1620 return value; 1621 case -940241380: // whenHandedOver 1622 this.whenHandedOver = TypeConvertor.castToDateTime(value); // DateTimeType 1623 return value; 1624 case -1429847026: // destination 1625 this.destination = TypeConvertor.castToReference(value); // Reference 1626 return value; 1627 case 3387378: // note 1628 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1629 return value; 1630 case 2138372141: // usageInstruction 1631 this.usageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 1632 return value; 1633 case 1835190426: // eventHistory 1634 this.getEventHistory().add(TypeConvertor.castToReference(value)); // Reference 1635 return value; 1636 default: return super.setProperty(hash, name, value); 1637 } 1638 1639 } 1640 1641 @Override 1642 public Base setProperty(String name, Base value) throws FHIRException { 1643 if (name.equals("identifier")) { 1644 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1645 } else if (name.equals("basedOn")) { 1646 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1647 } else if (name.equals("partOf")) { 1648 this.getPartOf().add(TypeConvertor.castToReference(value)); 1649 } else if (name.equals("status")) { 1650 value = new DeviceDispenseStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1651 this.status = (Enumeration) value; // Enumeration<DeviceDispenseStatusCodes> 1652 } else if (name.equals("statusReason")) { 1653 this.statusReason = TypeConvertor.castToCodeableReference(value); // CodeableReference 1654 } else if (name.equals("category")) { 1655 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1656 } else if (name.equals("device")) { 1657 this.device = TypeConvertor.castToCodeableReference(value); // CodeableReference 1658 } else if (name.equals("subject")) { 1659 this.subject = TypeConvertor.castToReference(value); // Reference 1660 } else if (name.equals("receiver")) { 1661 this.receiver = TypeConvertor.castToReference(value); // Reference 1662 } else if (name.equals("encounter")) { 1663 this.encounter = TypeConvertor.castToReference(value); // Reference 1664 } else if (name.equals("supportingInformation")) { 1665 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 1666 } else if (name.equals("performer")) { 1667 this.getPerformer().add((DeviceDispensePerformerComponent) value); 1668 } else if (name.equals("location")) { 1669 this.location = TypeConvertor.castToReference(value); // Reference 1670 } else if (name.equals("type")) { 1671 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1672 } else if (name.equals("quantity")) { 1673 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1674 } else if (name.equals("preparedDate")) { 1675 this.preparedDate = TypeConvertor.castToDateTime(value); // DateTimeType 1676 } else if (name.equals("whenHandedOver")) { 1677 this.whenHandedOver = TypeConvertor.castToDateTime(value); // DateTimeType 1678 } else if (name.equals("destination")) { 1679 this.destination = TypeConvertor.castToReference(value); // Reference 1680 } else if (name.equals("note")) { 1681 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1682 } else if (name.equals("usageInstruction")) { 1683 this.usageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 1684 } else if (name.equals("eventHistory")) { 1685 this.getEventHistory().add(TypeConvertor.castToReference(value)); 1686 } else 1687 return super.setProperty(name, value); 1688 return value; 1689 } 1690 1691 @Override 1692 public void removeChild(String name, Base value) throws FHIRException { 1693 if (name.equals("identifier")) { 1694 this.getIdentifier().remove(value); 1695 } else if (name.equals("basedOn")) { 1696 this.getBasedOn().remove(value); 1697 } else if (name.equals("partOf")) { 1698 this.getPartOf().remove(value); 1699 } else if (name.equals("status")) { 1700 value = new DeviceDispenseStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1701 this.status = (Enumeration) value; // Enumeration<DeviceDispenseStatusCodes> 1702 } else if (name.equals("statusReason")) { 1703 this.statusReason = null; 1704 } else if (name.equals("category")) { 1705 this.getCategory().remove(value); 1706 } else if (name.equals("device")) { 1707 this.device = null; 1708 } else if (name.equals("subject")) { 1709 this.subject = null; 1710 } else if (name.equals("receiver")) { 1711 this.receiver = null; 1712 } else if (name.equals("encounter")) { 1713 this.encounter = null; 1714 } else if (name.equals("supportingInformation")) { 1715 this.getSupportingInformation().remove(value); 1716 } else if (name.equals("performer")) { 1717 this.getPerformer().remove((DeviceDispensePerformerComponent) value); 1718 } else if (name.equals("location")) { 1719 this.location = null; 1720 } else if (name.equals("type")) { 1721 this.type = null; 1722 } else if (name.equals("quantity")) { 1723 this.quantity = null; 1724 } else if (name.equals("preparedDate")) { 1725 this.preparedDate = null; 1726 } else if (name.equals("whenHandedOver")) { 1727 this.whenHandedOver = null; 1728 } else if (name.equals("destination")) { 1729 this.destination = null; 1730 } else if (name.equals("note")) { 1731 this.getNote().remove(value); 1732 } else if (name.equals("usageInstruction")) { 1733 this.usageInstruction = null; 1734 } else if (name.equals("eventHistory")) { 1735 this.getEventHistory().remove(value); 1736 } else 1737 super.removeChild(name, value); 1738 1739 } 1740 1741 @Override 1742 public Base makeProperty(int hash, String name) throws FHIRException { 1743 switch (hash) { 1744 case -1618432855: return addIdentifier(); 1745 case -332612366: return addBasedOn(); 1746 case -995410646: return addPartOf(); 1747 case -892481550: return getStatusElement(); 1748 case 2051346646: return getStatusReason(); 1749 case 50511102: return addCategory(); 1750 case -1335157162: return getDevice(); 1751 case -1867885268: return getSubject(); 1752 case -808719889: return getReceiver(); 1753 case 1524132147: return getEncounter(); 1754 case -1248768647: return addSupportingInformation(); 1755 case 481140686: return addPerformer(); 1756 case 1901043637: return getLocation(); 1757 case 3575610: return getType(); 1758 case -1285004149: return getQuantity(); 1759 case -2024959605: return getPreparedDateElement(); 1760 case -940241380: return getWhenHandedOverElement(); 1761 case -1429847026: return getDestination(); 1762 case 3387378: return addNote(); 1763 case 2138372141: return getUsageInstructionElement(); 1764 case 1835190426: return addEventHistory(); 1765 default: return super.makeProperty(hash, name); 1766 } 1767 1768 } 1769 1770 @Override 1771 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1772 switch (hash) { 1773 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1774 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1775 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1776 case -892481550: /*status*/ return new String[] {"code"}; 1777 case 2051346646: /*statusReason*/ return new String[] {"CodeableReference"}; 1778 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1779 case -1335157162: /*device*/ return new String[] {"CodeableReference"}; 1780 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1781 case -808719889: /*receiver*/ return new String[] {"Reference"}; 1782 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1783 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 1784 case 481140686: /*performer*/ return new String[] {}; 1785 case 1901043637: /*location*/ return new String[] {"Reference"}; 1786 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1787 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1788 case -2024959605: /*preparedDate*/ return new String[] {"dateTime"}; 1789 case -940241380: /*whenHandedOver*/ return new String[] {"dateTime"}; 1790 case -1429847026: /*destination*/ return new String[] {"Reference"}; 1791 case 3387378: /*note*/ return new String[] {"Annotation"}; 1792 case 2138372141: /*usageInstruction*/ return new String[] {"markdown"}; 1793 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 1794 default: return super.getTypesForProperty(hash, name); 1795 } 1796 1797 } 1798 1799 @Override 1800 public Base addChild(String name) throws FHIRException { 1801 if (name.equals("identifier")) { 1802 return addIdentifier(); 1803 } 1804 else if (name.equals("basedOn")) { 1805 return addBasedOn(); 1806 } 1807 else if (name.equals("partOf")) { 1808 return addPartOf(); 1809 } 1810 else if (name.equals("status")) { 1811 throw new FHIRException("Cannot call addChild on a singleton property DeviceDispense.status"); 1812 } 1813 else if (name.equals("statusReason")) { 1814 this.statusReason = new CodeableReference(); 1815 return this.statusReason; 1816 } 1817 else if (name.equals("category")) { 1818 return addCategory(); 1819 } 1820 else if (name.equals("device")) { 1821 this.device = new CodeableReference(); 1822 return this.device; 1823 } 1824 else if (name.equals("subject")) { 1825 this.subject = new Reference(); 1826 return this.subject; 1827 } 1828 else if (name.equals("receiver")) { 1829 this.receiver = new Reference(); 1830 return this.receiver; 1831 } 1832 else if (name.equals("encounter")) { 1833 this.encounter = new Reference(); 1834 return this.encounter; 1835 } 1836 else if (name.equals("supportingInformation")) { 1837 return addSupportingInformation(); 1838 } 1839 else if (name.equals("performer")) { 1840 return addPerformer(); 1841 } 1842 else if (name.equals("location")) { 1843 this.location = new Reference(); 1844 return this.location; 1845 } 1846 else if (name.equals("type")) { 1847 this.type = new CodeableConcept(); 1848 return this.type; 1849 } 1850 else if (name.equals("quantity")) { 1851 this.quantity = new Quantity(); 1852 return this.quantity; 1853 } 1854 else if (name.equals("preparedDate")) { 1855 throw new FHIRException("Cannot call addChild on a singleton property DeviceDispense.preparedDate"); 1856 } 1857 else if (name.equals("whenHandedOver")) { 1858 throw new FHIRException("Cannot call addChild on a singleton property DeviceDispense.whenHandedOver"); 1859 } 1860 else if (name.equals("destination")) { 1861 this.destination = new Reference(); 1862 return this.destination; 1863 } 1864 else if (name.equals("note")) { 1865 return addNote(); 1866 } 1867 else if (name.equals("usageInstruction")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property DeviceDispense.usageInstruction"); 1869 } 1870 else if (name.equals("eventHistory")) { 1871 return addEventHistory(); 1872 } 1873 else 1874 return super.addChild(name); 1875 } 1876 1877 public String fhirType() { 1878 return "DeviceDispense"; 1879 1880 } 1881 1882 public DeviceDispense copy() { 1883 DeviceDispense dst = new DeviceDispense(); 1884 copyValues(dst); 1885 return dst; 1886 } 1887 1888 public void copyValues(DeviceDispense dst) { 1889 super.copyValues(dst); 1890 if (identifier != null) { 1891 dst.identifier = new ArrayList<Identifier>(); 1892 for (Identifier i : identifier) 1893 dst.identifier.add(i.copy()); 1894 }; 1895 if (basedOn != null) { 1896 dst.basedOn = new ArrayList<Reference>(); 1897 for (Reference i : basedOn) 1898 dst.basedOn.add(i.copy()); 1899 }; 1900 if (partOf != null) { 1901 dst.partOf = new ArrayList<Reference>(); 1902 for (Reference i : partOf) 1903 dst.partOf.add(i.copy()); 1904 }; 1905 dst.status = status == null ? null : status.copy(); 1906 dst.statusReason = statusReason == null ? null : statusReason.copy(); 1907 if (category != null) { 1908 dst.category = new ArrayList<CodeableConcept>(); 1909 for (CodeableConcept i : category) 1910 dst.category.add(i.copy()); 1911 }; 1912 dst.device = device == null ? null : device.copy(); 1913 dst.subject = subject == null ? null : subject.copy(); 1914 dst.receiver = receiver == null ? null : receiver.copy(); 1915 dst.encounter = encounter == null ? null : encounter.copy(); 1916 if (supportingInformation != null) { 1917 dst.supportingInformation = new ArrayList<Reference>(); 1918 for (Reference i : supportingInformation) 1919 dst.supportingInformation.add(i.copy()); 1920 }; 1921 if (performer != null) { 1922 dst.performer = new ArrayList<DeviceDispensePerformerComponent>(); 1923 for (DeviceDispensePerformerComponent i : performer) 1924 dst.performer.add(i.copy()); 1925 }; 1926 dst.location = location == null ? null : location.copy(); 1927 dst.type = type == null ? null : type.copy(); 1928 dst.quantity = quantity == null ? null : quantity.copy(); 1929 dst.preparedDate = preparedDate == null ? null : preparedDate.copy(); 1930 dst.whenHandedOver = whenHandedOver == null ? null : whenHandedOver.copy(); 1931 dst.destination = destination == null ? null : destination.copy(); 1932 if (note != null) { 1933 dst.note = new ArrayList<Annotation>(); 1934 for (Annotation i : note) 1935 dst.note.add(i.copy()); 1936 }; 1937 dst.usageInstruction = usageInstruction == null ? null : usageInstruction.copy(); 1938 if (eventHistory != null) { 1939 dst.eventHistory = new ArrayList<Reference>(); 1940 for (Reference i : eventHistory) 1941 dst.eventHistory.add(i.copy()); 1942 }; 1943 } 1944 1945 protected DeviceDispense typedCopy() { 1946 return copy(); 1947 } 1948 1949 @Override 1950 public boolean equalsDeep(Base other_) { 1951 if (!super.equalsDeep(other_)) 1952 return false; 1953 if (!(other_ instanceof DeviceDispense)) 1954 return false; 1955 DeviceDispense o = (DeviceDispense) other_; 1956 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1957 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) && compareDeep(category, o.category, true) 1958 && compareDeep(device, o.device, true) && compareDeep(subject, o.subject, true) && compareDeep(receiver, o.receiver, true) 1959 && compareDeep(encounter, o.encounter, true) && compareDeep(supportingInformation, o.supportingInformation, true) 1960 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) && compareDeep(type, o.type, true) 1961 && compareDeep(quantity, o.quantity, true) && compareDeep(preparedDate, o.preparedDate, true) && compareDeep(whenHandedOver, o.whenHandedOver, true) 1962 && compareDeep(destination, o.destination, true) && compareDeep(note, o.note, true) && compareDeep(usageInstruction, o.usageInstruction, true) 1963 && compareDeep(eventHistory, o.eventHistory, true); 1964 } 1965 1966 @Override 1967 public boolean equalsShallow(Base other_) { 1968 if (!super.equalsShallow(other_)) 1969 return false; 1970 if (!(other_ instanceof DeviceDispense)) 1971 return false; 1972 DeviceDispense o = (DeviceDispense) other_; 1973 return compareValues(status, o.status, true) && compareValues(preparedDate, o.preparedDate, true) && compareValues(whenHandedOver, o.whenHandedOver, true) 1974 && compareValues(usageInstruction, o.usageInstruction, true); 1975 } 1976 1977 public boolean isEmpty() { 1978 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1979 , status, statusReason, category, device, subject, receiver, encounter, supportingInformation 1980 , performer, location, type, quantity, preparedDate, whenHandedOver, destination 1981 , note, usageInstruction, eventHistory); 1982 } 1983 1984 @Override 1985 public ResourceType getResourceType() { 1986 return ResourceType.DeviceDispense; 1987 } 1988 1989 /** 1990 * Search parameter: <b>code</b> 1991 * <p> 1992 * Description: <b>Search for devices that match this code</b><br> 1993 * Type: <b>token</b><br> 1994 * Path: <b>DeviceDispense.device.concept</b><br> 1995 * </p> 1996 */ 1997 @SearchParamDefinition(name="code", path="DeviceDispense.device.concept", description="Search for devices that match this code", type="token" ) 1998 public static final String SP_CODE = "code"; 1999 /** 2000 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2001 * <p> 2002 * Description: <b>Search for devices that match this code</b><br> 2003 * Type: <b>token</b><br> 2004 * Path: <b>DeviceDispense.device.concept</b><br> 2005 * </p> 2006 */ 2007 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2008 2009 /** 2010 * Search parameter: <b>identifier</b> 2011 * <p> 2012 * Description: <b>The identifier of the dispense</b><br> 2013 * Type: <b>token</b><br> 2014 * Path: <b>DeviceDispense.identifier</b><br> 2015 * </p> 2016 */ 2017 @SearchParamDefinition(name="identifier", path="DeviceDispense.identifier", description="The identifier of the dispense", type="token" ) 2018 public static final String SP_IDENTIFIER = "identifier"; 2019 /** 2020 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2021 * <p> 2022 * Description: <b>The identifier of the dispense</b><br> 2023 * Type: <b>token</b><br> 2024 * Path: <b>DeviceDispense.identifier</b><br> 2025 * </p> 2026 */ 2027 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2028 2029 /** 2030 * Search parameter: <b>patient</b> 2031 * <p> 2032 * Description: <b>Returns device dispenses for a specific patient</b><br> 2033 * Type: <b>reference</b><br> 2034 * Path: <b>DeviceDispense.subject.where(resolve() is Patient)</b><br> 2035 * </p> 2036 */ 2037 @SearchParamDefinition(name="patient", path="DeviceDispense.subject.where(resolve() is Patient)", description="Returns device dispenses for a specific patient", type="reference", target={Patient.class } ) 2038 public static final String SP_PATIENT = "patient"; 2039 /** 2040 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2041 * <p> 2042 * Description: <b>Returns device dispenses for a specific patient</b><br> 2043 * Type: <b>reference</b><br> 2044 * Path: <b>DeviceDispense.subject.where(resolve() is Patient)</b><br> 2045 * </p> 2046 */ 2047 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2048 2049/** 2050 * Constant for fluent queries to be used to add include statements. Specifies 2051 * the path value of "<b>DeviceDispense:patient</b>". 2052 */ 2053 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceDispense:patient").toLocked(); 2054 2055 /** 2056 * Search parameter: <b>status</b> 2057 * <p> 2058 * Description: <b>The status of the dispense</b><br> 2059 * Type: <b>token</b><br> 2060 * Path: <b>DeviceDispense.status</b><br> 2061 * </p> 2062 */ 2063 @SearchParamDefinition(name="status", path="DeviceDispense.status", description="The status of the dispense", type="token" ) 2064 public static final String SP_STATUS = "status"; 2065 /** 2066 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2067 * <p> 2068 * Description: <b>The status of the dispense</b><br> 2069 * Type: <b>token</b><br> 2070 * Path: <b>DeviceDispense.status</b><br> 2071 * </p> 2072 */ 2073 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2074 2075 /** 2076 * Search parameter: <b>subject</b> 2077 * <p> 2078 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 2079 * Type: <b>reference</b><br> 2080 * Path: <b>DeviceDispense.subject</b><br> 2081 * </p> 2082 */ 2083 @SearchParamDefinition(name="subject", path="DeviceDispense.subject", description="The identity of a patient for whom to list dispenses", type="reference", target={Patient.class, Practitioner.class } ) 2084 public static final String SP_SUBJECT = "subject"; 2085 /** 2086 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2087 * <p> 2088 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 2089 * Type: <b>reference</b><br> 2090 * Path: <b>DeviceDispense.subject</b><br> 2091 * </p> 2092 */ 2093 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2094 2095/** 2096 * Constant for fluent queries to be used to add include statements. Specifies 2097 * the path value of "<b>DeviceDispense:subject</b>". 2098 */ 2099 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DeviceDispense:subject").toLocked(); 2100 2101 2102} 2103