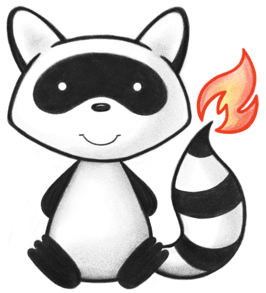
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes a measurement, calculation or setting capability of a device. The DeviceMetric resource is derived from the ISO/IEEE 11073-10201 Domain Information Model standard, but is more widely applicable. 052 */ 053@ResourceDef(name="DeviceMetric", profile="http://hl7.org/fhir/StructureDefinition/DeviceMetric") 054public class DeviceMetric extends DomainResource { 055 056 public enum DeviceMetricCalibrationState { 057 /** 058 * The metric has not been calibrated. 059 */ 060 NOTCALIBRATED, 061 /** 062 * The metric needs to be calibrated. 063 */ 064 CALIBRATIONREQUIRED, 065 /** 066 * The metric has been calibrated. 067 */ 068 CALIBRATED, 069 /** 070 * The state of calibration of this metric is unspecified. 071 */ 072 UNSPECIFIED, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static DeviceMetricCalibrationState fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("not-calibrated".equals(codeString)) 081 return NOTCALIBRATED; 082 if ("calibration-required".equals(codeString)) 083 return CALIBRATIONREQUIRED; 084 if ("calibrated".equals(codeString)) 085 return CALIBRATED; 086 if ("unspecified".equals(codeString)) 087 return UNSPECIFIED; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case NOTCALIBRATED: return "not-calibrated"; 096 case CALIBRATIONREQUIRED: return "calibration-required"; 097 case CALIBRATED: return "calibrated"; 098 case UNSPECIFIED: return "unspecified"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case NOTCALIBRATED: return "http://hl7.org/fhir/metric-calibration-state"; 106 case CALIBRATIONREQUIRED: return "http://hl7.org/fhir/metric-calibration-state"; 107 case CALIBRATED: return "http://hl7.org/fhir/metric-calibration-state"; 108 case UNSPECIFIED: return "http://hl7.org/fhir/metric-calibration-state"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case NOTCALIBRATED: return "The metric has not been calibrated."; 116 case CALIBRATIONREQUIRED: return "The metric needs to be calibrated."; 117 case CALIBRATED: return "The metric has been calibrated."; 118 case UNSPECIFIED: return "The state of calibration of this metric is unspecified."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case NOTCALIBRATED: return "Not Calibrated"; 126 case CALIBRATIONREQUIRED: return "Calibration Required"; 127 case CALIBRATED: return "Calibrated"; 128 case UNSPECIFIED: return "Unspecified"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class DeviceMetricCalibrationStateEnumFactory implements EnumFactory<DeviceMetricCalibrationState> { 136 public DeviceMetricCalibrationState fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("not-calibrated".equals(codeString)) 141 return DeviceMetricCalibrationState.NOTCALIBRATED; 142 if ("calibration-required".equals(codeString)) 143 return DeviceMetricCalibrationState.CALIBRATIONREQUIRED; 144 if ("calibrated".equals(codeString)) 145 return DeviceMetricCalibrationState.CALIBRATED; 146 if ("unspecified".equals(codeString)) 147 return DeviceMetricCalibrationState.UNSPECIFIED; 148 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 149 } 150 public Enumeration<DeviceMetricCalibrationState> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 158 if ("not-calibrated".equals(codeString)) 159 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NOTCALIBRATED, code); 160 if ("calibration-required".equals(codeString)) 161 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATIONREQUIRED, code); 162 if ("calibrated".equals(codeString)) 163 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATED, code); 164 if ("unspecified".equals(codeString)) 165 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.UNSPECIFIED, code); 166 throw new FHIRException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 167 } 168 public String toCode(DeviceMetricCalibrationState code) { 169 if (code == DeviceMetricCalibrationState.NOTCALIBRATED) 170 return "not-calibrated"; 171 if (code == DeviceMetricCalibrationState.CALIBRATIONREQUIRED) 172 return "calibration-required"; 173 if (code == DeviceMetricCalibrationState.CALIBRATED) 174 return "calibrated"; 175 if (code == DeviceMetricCalibrationState.UNSPECIFIED) 176 return "unspecified"; 177 return "?"; 178 } 179 public String toSystem(DeviceMetricCalibrationState code) { 180 return code.getSystem(); 181 } 182 } 183 184 public enum DeviceMetricCalibrationType { 185 /** 186 * Metric calibration method has not been identified. 187 */ 188 UNSPECIFIED, 189 /** 190 * Offset metric calibration method. 191 */ 192 OFFSET, 193 /** 194 * Gain metric calibration method. 195 */ 196 GAIN, 197 /** 198 * Two-point metric calibration method. 199 */ 200 TWOPOINT, 201 /** 202 * added to help the parsers with the generic types 203 */ 204 NULL; 205 public static DeviceMetricCalibrationType fromCode(String codeString) throws FHIRException { 206 if (codeString == null || "".equals(codeString)) 207 return null; 208 if ("unspecified".equals(codeString)) 209 return UNSPECIFIED; 210 if ("offset".equals(codeString)) 211 return OFFSET; 212 if ("gain".equals(codeString)) 213 return GAIN; 214 if ("two-point".equals(codeString)) 215 return TWOPOINT; 216 if (Configuration.isAcceptInvalidEnums()) 217 return null; 218 else 219 throw new FHIRException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 220 } 221 public String toCode() { 222 switch (this) { 223 case UNSPECIFIED: return "unspecified"; 224 case OFFSET: return "offset"; 225 case GAIN: return "gain"; 226 case TWOPOINT: return "two-point"; 227 case NULL: return null; 228 default: return "?"; 229 } 230 } 231 public String getSystem() { 232 switch (this) { 233 case UNSPECIFIED: return "http://hl7.org/fhir/metric-calibration-type"; 234 case OFFSET: return "http://hl7.org/fhir/metric-calibration-type"; 235 case GAIN: return "http://hl7.org/fhir/metric-calibration-type"; 236 case TWOPOINT: return "http://hl7.org/fhir/metric-calibration-type"; 237 case NULL: return null; 238 default: return "?"; 239 } 240 } 241 public String getDefinition() { 242 switch (this) { 243 case UNSPECIFIED: return "Metric calibration method has not been identified."; 244 case OFFSET: return "Offset metric calibration method."; 245 case GAIN: return "Gain metric calibration method."; 246 case TWOPOINT: return "Two-point metric calibration method."; 247 case NULL: return null; 248 default: return "?"; 249 } 250 } 251 public String getDisplay() { 252 switch (this) { 253 case UNSPECIFIED: return "Unspecified"; 254 case OFFSET: return "Offset"; 255 case GAIN: return "Gain"; 256 case TWOPOINT: return "Two Point"; 257 case NULL: return null; 258 default: return "?"; 259 } 260 } 261 } 262 263 public static class DeviceMetricCalibrationTypeEnumFactory implements EnumFactory<DeviceMetricCalibrationType> { 264 public DeviceMetricCalibrationType fromCode(String codeString) throws IllegalArgumentException { 265 if (codeString == null || "".equals(codeString)) 266 if (codeString == null || "".equals(codeString)) 267 return null; 268 if ("unspecified".equals(codeString)) 269 return DeviceMetricCalibrationType.UNSPECIFIED; 270 if ("offset".equals(codeString)) 271 return DeviceMetricCalibrationType.OFFSET; 272 if ("gain".equals(codeString)) 273 return DeviceMetricCalibrationType.GAIN; 274 if ("two-point".equals(codeString)) 275 return DeviceMetricCalibrationType.TWOPOINT; 276 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 277 } 278 public Enumeration<DeviceMetricCalibrationType> fromType(PrimitiveType<?> code) throws FHIRException { 279 if (code == null) 280 return null; 281 if (code.isEmpty()) 282 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 283 String codeString = ((PrimitiveType) code).asStringValue(); 284 if (codeString == null || "".equals(codeString)) 285 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 286 if ("unspecified".equals(codeString)) 287 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.UNSPECIFIED, code); 288 if ("offset".equals(codeString)) 289 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.OFFSET, code); 290 if ("gain".equals(codeString)) 291 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.GAIN, code); 292 if ("two-point".equals(codeString)) 293 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.TWOPOINT, code); 294 throw new FHIRException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 295 } 296 public String toCode(DeviceMetricCalibrationType code) { 297 if (code == DeviceMetricCalibrationType.UNSPECIFIED) 298 return "unspecified"; 299 if (code == DeviceMetricCalibrationType.OFFSET) 300 return "offset"; 301 if (code == DeviceMetricCalibrationType.GAIN) 302 return "gain"; 303 if (code == DeviceMetricCalibrationType.TWOPOINT) 304 return "two-point"; 305 return "?"; 306 } 307 public String toSystem(DeviceMetricCalibrationType code) { 308 return code.getSystem(); 309 } 310 } 311 312 public enum DeviceMetricCategory { 313 /** 314 * Observations generated for this DeviceMetric are measured. 315 */ 316 MEASUREMENT, 317 /** 318 * Observations generated for this DeviceMetric is a setting that will influence the behavior of the Device. 319 */ 320 SETTING, 321 /** 322 * Observations generated for this DeviceMetric are calculated. 323 */ 324 CALCULATION, 325 /** 326 * The category of this DeviceMetric is unspecified. 327 */ 328 UNSPECIFIED, 329 /** 330 * added to help the parsers with the generic types 331 */ 332 NULL; 333 public static DeviceMetricCategory fromCode(String codeString) throws FHIRException { 334 if (codeString == null || "".equals(codeString)) 335 return null; 336 if ("measurement".equals(codeString)) 337 return MEASUREMENT; 338 if ("setting".equals(codeString)) 339 return SETTING; 340 if ("calculation".equals(codeString)) 341 return CALCULATION; 342 if ("unspecified".equals(codeString)) 343 return UNSPECIFIED; 344 if (Configuration.isAcceptInvalidEnums()) 345 return null; 346 else 347 throw new FHIRException("Unknown DeviceMetricCategory code '"+codeString+"'"); 348 } 349 public String toCode() { 350 switch (this) { 351 case MEASUREMENT: return "measurement"; 352 case SETTING: return "setting"; 353 case CALCULATION: return "calculation"; 354 case UNSPECIFIED: return "unspecified"; 355 case NULL: return null; 356 default: return "?"; 357 } 358 } 359 public String getSystem() { 360 switch (this) { 361 case MEASUREMENT: return "http://hl7.org/fhir/metric-category"; 362 case SETTING: return "http://hl7.org/fhir/metric-category"; 363 case CALCULATION: return "http://hl7.org/fhir/metric-category"; 364 case UNSPECIFIED: return "http://hl7.org/fhir/metric-category"; 365 case NULL: return null; 366 default: return "?"; 367 } 368 } 369 public String getDefinition() { 370 switch (this) { 371 case MEASUREMENT: return "Observations generated for this DeviceMetric are measured."; 372 case SETTING: return "Observations generated for this DeviceMetric is a setting that will influence the behavior of the Device."; 373 case CALCULATION: return "Observations generated for this DeviceMetric are calculated."; 374 case UNSPECIFIED: return "The category of this DeviceMetric is unspecified."; 375 case NULL: return null; 376 default: return "?"; 377 } 378 } 379 public String getDisplay() { 380 switch (this) { 381 case MEASUREMENT: return "Measurement"; 382 case SETTING: return "Setting"; 383 case CALCULATION: return "Calculation"; 384 case UNSPECIFIED: return "Unspecified"; 385 case NULL: return null; 386 default: return "?"; 387 } 388 } 389 } 390 391 public static class DeviceMetricCategoryEnumFactory implements EnumFactory<DeviceMetricCategory> { 392 public DeviceMetricCategory fromCode(String codeString) throws IllegalArgumentException { 393 if (codeString == null || "".equals(codeString)) 394 if (codeString == null || "".equals(codeString)) 395 return null; 396 if ("measurement".equals(codeString)) 397 return DeviceMetricCategory.MEASUREMENT; 398 if ("setting".equals(codeString)) 399 return DeviceMetricCategory.SETTING; 400 if ("calculation".equals(codeString)) 401 return DeviceMetricCategory.CALCULATION; 402 if ("unspecified".equals(codeString)) 403 return DeviceMetricCategory.UNSPECIFIED; 404 throw new IllegalArgumentException("Unknown DeviceMetricCategory code '"+codeString+"'"); 405 } 406 public Enumeration<DeviceMetricCategory> fromType(PrimitiveType<?> code) throws FHIRException { 407 if (code == null) 408 return null; 409 if (code.isEmpty()) 410 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 411 String codeString = ((PrimitiveType) code).asStringValue(); 412 if (codeString == null || "".equals(codeString)) 413 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 414 if ("measurement".equals(codeString)) 415 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.MEASUREMENT, code); 416 if ("setting".equals(codeString)) 417 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.SETTING, code); 418 if ("calculation".equals(codeString)) 419 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.CALCULATION, code); 420 if ("unspecified".equals(codeString)) 421 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.UNSPECIFIED, code); 422 throw new FHIRException("Unknown DeviceMetricCategory code '"+codeString+"'"); 423 } 424 public String toCode(DeviceMetricCategory code) { 425 if (code == DeviceMetricCategory.MEASUREMENT) 426 return "measurement"; 427 if (code == DeviceMetricCategory.SETTING) 428 return "setting"; 429 if (code == DeviceMetricCategory.CALCULATION) 430 return "calculation"; 431 if (code == DeviceMetricCategory.UNSPECIFIED) 432 return "unspecified"; 433 return "?"; 434 } 435 public String toSystem(DeviceMetricCategory code) { 436 return code.getSystem(); 437 } 438 } 439 440 public enum DeviceMetricOperationalStatus { 441 /** 442 * The DeviceMetric is operating and will generate Observations. 443 */ 444 ON, 445 /** 446 * The DeviceMetric is not operating. 447 */ 448 OFF, 449 /** 450 * The DeviceMetric is operating, but will not generate any Observations. 451 */ 452 STANDBY, 453 /** 454 * The DeviceMetric was entered in error. 455 */ 456 ENTEREDINERROR, 457 /** 458 * added to help the parsers with the generic types 459 */ 460 NULL; 461 public static DeviceMetricOperationalStatus fromCode(String codeString) throws FHIRException { 462 if (codeString == null || "".equals(codeString)) 463 return null; 464 if ("on".equals(codeString)) 465 return ON; 466 if ("off".equals(codeString)) 467 return OFF; 468 if ("standby".equals(codeString)) 469 return STANDBY; 470 if ("entered-in-error".equals(codeString)) 471 return ENTEREDINERROR; 472 if (Configuration.isAcceptInvalidEnums()) 473 return null; 474 else 475 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 476 } 477 public String toCode() { 478 switch (this) { 479 case ON: return "on"; 480 case OFF: return "off"; 481 case STANDBY: return "standby"; 482 case ENTEREDINERROR: return "entered-in-error"; 483 case NULL: return null; 484 default: return "?"; 485 } 486 } 487 public String getSystem() { 488 switch (this) { 489 case ON: return "http://hl7.org/fhir/metric-operational-status"; 490 case OFF: return "http://hl7.org/fhir/metric-operational-status"; 491 case STANDBY: return "http://hl7.org/fhir/metric-operational-status"; 492 case ENTEREDINERROR: return "http://hl7.org/fhir/metric-operational-status"; 493 case NULL: return null; 494 default: return "?"; 495 } 496 } 497 public String getDefinition() { 498 switch (this) { 499 case ON: return "The DeviceMetric is operating and will generate Observations."; 500 case OFF: return "The DeviceMetric is not operating."; 501 case STANDBY: return "The DeviceMetric is operating, but will not generate any Observations."; 502 case ENTEREDINERROR: return "The DeviceMetric was entered in error."; 503 case NULL: return null; 504 default: return "?"; 505 } 506 } 507 public String getDisplay() { 508 switch (this) { 509 case ON: return "On"; 510 case OFF: return "Off"; 511 case STANDBY: return "Standby"; 512 case ENTEREDINERROR: return "Entered In Error"; 513 case NULL: return null; 514 default: return "?"; 515 } 516 } 517 } 518 519 public static class DeviceMetricOperationalStatusEnumFactory implements EnumFactory<DeviceMetricOperationalStatus> { 520 public DeviceMetricOperationalStatus fromCode(String codeString) throws IllegalArgumentException { 521 if (codeString == null || "".equals(codeString)) 522 if (codeString == null || "".equals(codeString)) 523 return null; 524 if ("on".equals(codeString)) 525 return DeviceMetricOperationalStatus.ON; 526 if ("off".equals(codeString)) 527 return DeviceMetricOperationalStatus.OFF; 528 if ("standby".equals(codeString)) 529 return DeviceMetricOperationalStatus.STANDBY; 530 if ("entered-in-error".equals(codeString)) 531 return DeviceMetricOperationalStatus.ENTEREDINERROR; 532 throw new IllegalArgumentException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 533 } 534 public Enumeration<DeviceMetricOperationalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 535 if (code == null) 536 return null; 537 if (code.isEmpty()) 538 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 539 String codeString = ((PrimitiveType) code).asStringValue(); 540 if (codeString == null || "".equals(codeString)) 541 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 542 if ("on".equals(codeString)) 543 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ON, code); 544 if ("off".equals(codeString)) 545 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.OFF, code); 546 if ("standby".equals(codeString)) 547 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.STANDBY, code); 548 if ("entered-in-error".equals(codeString)) 549 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ENTEREDINERROR, code); 550 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 551 } 552 public String toCode(DeviceMetricOperationalStatus code) { 553 if (code == DeviceMetricOperationalStatus.ON) 554 return "on"; 555 if (code == DeviceMetricOperationalStatus.OFF) 556 return "off"; 557 if (code == DeviceMetricOperationalStatus.STANDBY) 558 return "standby"; 559 if (code == DeviceMetricOperationalStatus.ENTEREDINERROR) 560 return "entered-in-error"; 561 return "?"; 562 } 563 public String toSystem(DeviceMetricOperationalStatus code) { 564 return code.getSystem(); 565 } 566 } 567 568 @Block() 569 public static class DeviceMetricCalibrationComponent extends BackboneElement implements IBaseBackboneElement { 570 /** 571 * Describes the type of the calibration method. 572 */ 573 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 574 @Description(shortDefinition="unspecified | offset | gain | two-point", formalDefinition="Describes the type of the calibration method." ) 575 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-calibration-type") 576 protected Enumeration<DeviceMetricCalibrationType> type; 577 578 /** 579 * Describes the state of the calibration. 580 */ 581 @Child(name = "state", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 582 @Description(shortDefinition="not-calibrated | calibration-required | calibrated | unspecified", formalDefinition="Describes the state of the calibration." ) 583 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-calibration-state") 584 protected Enumeration<DeviceMetricCalibrationState> state; 585 586 /** 587 * Describes the time last calibration has been performed. 588 */ 589 @Child(name = "time", type = {InstantType.class}, order=3, min=0, max=1, modifier=false, summary=false) 590 @Description(shortDefinition="Describes the time last calibration has been performed", formalDefinition="Describes the time last calibration has been performed." ) 591 protected InstantType time; 592 593 private static final long serialVersionUID = 1163986578L; 594 595 /** 596 * Constructor 597 */ 598 public DeviceMetricCalibrationComponent() { 599 super(); 600 } 601 602 /** 603 * @return {@link #type} (Describes the type of the calibration method.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 604 */ 605 public Enumeration<DeviceMetricCalibrationType> getTypeElement() { 606 if (this.type == null) 607 if (Configuration.errorOnAutoCreate()) 608 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.type"); 609 else if (Configuration.doAutoCreate()) 610 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); // bb 611 return this.type; 612 } 613 614 public boolean hasTypeElement() { 615 return this.type != null && !this.type.isEmpty(); 616 } 617 618 public boolean hasType() { 619 return this.type != null && !this.type.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #type} (Describes the type of the calibration method.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 624 */ 625 public DeviceMetricCalibrationComponent setTypeElement(Enumeration<DeviceMetricCalibrationType> value) { 626 this.type = value; 627 return this; 628 } 629 630 /** 631 * @return Describes the type of the calibration method. 632 */ 633 public DeviceMetricCalibrationType getType() { 634 return this.type == null ? null : this.type.getValue(); 635 } 636 637 /** 638 * @param value Describes the type of the calibration method. 639 */ 640 public DeviceMetricCalibrationComponent setType(DeviceMetricCalibrationType value) { 641 if (value == null) 642 this.type = null; 643 else { 644 if (this.type == null) 645 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); 646 this.type.setValue(value); 647 } 648 return this; 649 } 650 651 /** 652 * @return {@link #state} (Describes the state of the calibration.). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 653 */ 654 public Enumeration<DeviceMetricCalibrationState> getStateElement() { 655 if (this.state == null) 656 if (Configuration.errorOnAutoCreate()) 657 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.state"); 658 else if (Configuration.doAutoCreate()) 659 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); // bb 660 return this.state; 661 } 662 663 public boolean hasStateElement() { 664 return this.state != null && !this.state.isEmpty(); 665 } 666 667 public boolean hasState() { 668 return this.state != null && !this.state.isEmpty(); 669 } 670 671 /** 672 * @param value {@link #state} (Describes the state of the calibration.). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 673 */ 674 public DeviceMetricCalibrationComponent setStateElement(Enumeration<DeviceMetricCalibrationState> value) { 675 this.state = value; 676 return this; 677 } 678 679 /** 680 * @return Describes the state of the calibration. 681 */ 682 public DeviceMetricCalibrationState getState() { 683 return this.state == null ? null : this.state.getValue(); 684 } 685 686 /** 687 * @param value Describes the state of the calibration. 688 */ 689 public DeviceMetricCalibrationComponent setState(DeviceMetricCalibrationState value) { 690 if (value == null) 691 this.state = null; 692 else { 693 if (this.state == null) 694 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); 695 this.state.setValue(value); 696 } 697 return this; 698 } 699 700 /** 701 * @return {@link #time} (Describes the time last calibration has been performed.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 702 */ 703 public InstantType getTimeElement() { 704 if (this.time == null) 705 if (Configuration.errorOnAutoCreate()) 706 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.time"); 707 else if (Configuration.doAutoCreate()) 708 this.time = new InstantType(); // bb 709 return this.time; 710 } 711 712 public boolean hasTimeElement() { 713 return this.time != null && !this.time.isEmpty(); 714 } 715 716 public boolean hasTime() { 717 return this.time != null && !this.time.isEmpty(); 718 } 719 720 /** 721 * @param value {@link #time} (Describes the time last calibration has been performed.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 722 */ 723 public DeviceMetricCalibrationComponent setTimeElement(InstantType value) { 724 this.time = value; 725 return this; 726 } 727 728 /** 729 * @return Describes the time last calibration has been performed. 730 */ 731 public Date getTime() { 732 return this.time == null ? null : this.time.getValue(); 733 } 734 735 /** 736 * @param value Describes the time last calibration has been performed. 737 */ 738 public DeviceMetricCalibrationComponent setTime(Date value) { 739 if (value == null) 740 this.time = null; 741 else { 742 if (this.time == null) 743 this.time = new InstantType(); 744 this.time.setValue(value); 745 } 746 return this; 747 } 748 749 protected void listChildren(List<Property> children) { 750 super.listChildren(children); 751 children.add(new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type)); 752 children.add(new Property("state", "code", "Describes the state of the calibration.", 0, 1, state)); 753 children.add(new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time)); 754 } 755 756 @Override 757 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 758 switch (_hash) { 759 case 3575610: /*type*/ return new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type); 760 case 109757585: /*state*/ return new Property("state", "code", "Describes the state of the calibration.", 0, 1, state); 761 case 3560141: /*time*/ return new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time); 762 default: return super.getNamedProperty(_hash, _name, _checkValid); 763 } 764 765 } 766 767 @Override 768 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 769 switch (hash) { 770 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DeviceMetricCalibrationType> 771 case 109757585: /*state*/ return this.state == null ? new Base[0] : new Base[] {this.state}; // Enumeration<DeviceMetricCalibrationState> 772 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // InstantType 773 default: return super.getProperty(hash, name, checkValid); 774 } 775 776 } 777 778 @Override 779 public Base setProperty(int hash, String name, Base value) throws FHIRException { 780 switch (hash) { 781 case 3575610: // type 782 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 783 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 784 return value; 785 case 109757585: // state 786 value = new DeviceMetricCalibrationStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 787 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 788 return value; 789 case 3560141: // time 790 this.time = TypeConvertor.castToInstant(value); // InstantType 791 return value; 792 default: return super.setProperty(hash, name, value); 793 } 794 795 } 796 797 @Override 798 public Base setProperty(String name, Base value) throws FHIRException { 799 if (name.equals("type")) { 800 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 801 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 802 } else if (name.equals("state")) { 803 value = new DeviceMetricCalibrationStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 804 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 805 } else if (name.equals("time")) { 806 this.time = TypeConvertor.castToInstant(value); // InstantType 807 } else 808 return super.setProperty(name, value); 809 return value; 810 } 811 812 @Override 813 public void removeChild(String name, Base value) throws FHIRException { 814 if (name.equals("type")) { 815 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 816 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 817 } else if (name.equals("state")) { 818 value = new DeviceMetricCalibrationStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 819 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 820 } else if (name.equals("time")) { 821 this.time = null; 822 } else 823 super.removeChild(name, value); 824 825 } 826 827 @Override 828 public Base makeProperty(int hash, String name) throws FHIRException { 829 switch (hash) { 830 case 3575610: return getTypeElement(); 831 case 109757585: return getStateElement(); 832 case 3560141: return getTimeElement(); 833 default: return super.makeProperty(hash, name); 834 } 835 836 } 837 838 @Override 839 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 840 switch (hash) { 841 case 3575610: /*type*/ return new String[] {"code"}; 842 case 109757585: /*state*/ return new String[] {"code"}; 843 case 3560141: /*time*/ return new String[] {"instant"}; 844 default: return super.getTypesForProperty(hash, name); 845 } 846 847 } 848 849 @Override 850 public Base addChild(String name) throws FHIRException { 851 if (name.equals("type")) { 852 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.calibration.type"); 853 } 854 else if (name.equals("state")) { 855 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.calibration.state"); 856 } 857 else if (name.equals("time")) { 858 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.calibration.time"); 859 } 860 else 861 return super.addChild(name); 862 } 863 864 public DeviceMetricCalibrationComponent copy() { 865 DeviceMetricCalibrationComponent dst = new DeviceMetricCalibrationComponent(); 866 copyValues(dst); 867 return dst; 868 } 869 870 public void copyValues(DeviceMetricCalibrationComponent dst) { 871 super.copyValues(dst); 872 dst.type = type == null ? null : type.copy(); 873 dst.state = state == null ? null : state.copy(); 874 dst.time = time == null ? null : time.copy(); 875 } 876 877 @Override 878 public boolean equalsDeep(Base other_) { 879 if (!super.equalsDeep(other_)) 880 return false; 881 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 882 return false; 883 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 884 return compareDeep(type, o.type, true) && compareDeep(state, o.state, true) && compareDeep(time, o.time, true) 885 ; 886 } 887 888 @Override 889 public boolean equalsShallow(Base other_) { 890 if (!super.equalsShallow(other_)) 891 return false; 892 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 893 return false; 894 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 895 return compareValues(type, o.type, true) && compareValues(state, o.state, true) && compareValues(time, o.time, true) 896 ; 897 } 898 899 public boolean isEmpty() { 900 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, state, time); 901 } 902 903 public String fhirType() { 904 return "DeviceMetric.calibration"; 905 906 } 907 908 } 909 910 /** 911 * Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID. 912 */ 913 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 914 @Description(shortDefinition="Instance identifier", formalDefinition="Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID." ) 915 protected List<Identifier> identifier; 916 917 /** 918 * Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc. 919 */ 920 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 921 @Description(shortDefinition="Identity of metric, for example Heart Rate or PEEP Setting", formalDefinition="Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc." ) 922 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicemetric-type") 923 protected CodeableConcept type; 924 925 /** 926 * Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc. 927 */ 928 @Child(name = "unit", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 929 @Description(shortDefinition="Unit of Measure for the Metric", formalDefinition="Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc." ) 930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ucum-units") 931 protected CodeableConcept unit; 932 933 /** 934 * Describes the link to the Device. This is also known as a channel device. 935 */ 936 @Child(name = "device", type = {Device.class}, order=3, min=1, max=1, modifier=false, summary=true) 937 @Description(shortDefinition="Describes the link to the Device", formalDefinition="Describes the link to the Device. This is also known as a channel device." ) 938 protected Reference device; 939 940 /** 941 * Indicates current operational state of the device. For example: On, Off, Standby, etc. 942 */ 943 @Child(name = "operationalStatus", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 944 @Description(shortDefinition="on | off | standby | entered-in-error", formalDefinition="Indicates current operational state of the device. For example: On, Off, Standby, etc." ) 945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-operational-status") 946 protected Enumeration<DeviceMetricOperationalStatus> operationalStatus; 947 948 /** 949 * The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta. 950 */ 951 @Child(name = "color", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 952 @Description(shortDefinition="Color name (from CSS4) or #RRGGBB code", formalDefinition="The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta." ) 953 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/color-codes") 954 protected CodeType color; 955 956 /** 957 * Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 958 */ 959 @Child(name = "category", type = {CodeType.class}, order=6, min=1, max=1, modifier=false, summary=true) 960 @Description(shortDefinition="measurement | setting | calculation | unspecified", formalDefinition="Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation." ) 961 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-category") 962 protected Enumeration<DeviceMetricCategory> category; 963 964 /** 965 * The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured. 966 */ 967 @Child(name = "measurementFrequency", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 968 @Description(shortDefinition="Indicates how often the metric is taken or recorded", formalDefinition="The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured." ) 969 protected Quantity measurementFrequency; 970 971 /** 972 * Describes the calibrations that have been performed or that are required to be performed. 973 */ 974 @Child(name = "calibration", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 975 @Description(shortDefinition="Describes the calibrations that have been performed or that are required to be performed", formalDefinition="Describes the calibrations that have been performed or that are required to be performed." ) 976 protected List<DeviceMetricCalibrationComponent> calibration; 977 978 private static final long serialVersionUID = 2109169775L; 979 980 /** 981 * Constructor 982 */ 983 public DeviceMetric() { 984 super(); 985 } 986 987 /** 988 * Constructor 989 */ 990 public DeviceMetric(CodeableConcept type, Reference device, DeviceMetricCategory category) { 991 super(); 992 this.setType(type); 993 this.setDevice(device); 994 this.setCategory(category); 995 } 996 997 /** 998 * @return {@link #identifier} (Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID.) 999 */ 1000 public List<Identifier> getIdentifier() { 1001 if (this.identifier == null) 1002 this.identifier = new ArrayList<Identifier>(); 1003 return this.identifier; 1004 } 1005 1006 /** 1007 * @return Returns a reference to <code>this</code> for easy method chaining 1008 */ 1009 public DeviceMetric setIdentifier(List<Identifier> theIdentifier) { 1010 this.identifier = theIdentifier; 1011 return this; 1012 } 1013 1014 public boolean hasIdentifier() { 1015 if (this.identifier == null) 1016 return false; 1017 for (Identifier item : this.identifier) 1018 if (!item.isEmpty()) 1019 return true; 1020 return false; 1021 } 1022 1023 public Identifier addIdentifier() { //3 1024 Identifier t = new Identifier(); 1025 if (this.identifier == null) 1026 this.identifier = new ArrayList<Identifier>(); 1027 this.identifier.add(t); 1028 return t; 1029 } 1030 1031 public DeviceMetric addIdentifier(Identifier t) { //3 1032 if (t == null) 1033 return this; 1034 if (this.identifier == null) 1035 this.identifier = new ArrayList<Identifier>(); 1036 this.identifier.add(t); 1037 return this; 1038 } 1039 1040 /** 1041 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1042 */ 1043 public Identifier getIdentifierFirstRep() { 1044 if (getIdentifier().isEmpty()) { 1045 addIdentifier(); 1046 } 1047 return getIdentifier().get(0); 1048 } 1049 1050 /** 1051 * @return {@link #type} (Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.) 1052 */ 1053 public CodeableConcept getType() { 1054 if (this.type == null) 1055 if (Configuration.errorOnAutoCreate()) 1056 throw new Error("Attempt to auto-create DeviceMetric.type"); 1057 else if (Configuration.doAutoCreate()) 1058 this.type = new CodeableConcept(); // cc 1059 return this.type; 1060 } 1061 1062 public boolean hasType() { 1063 return this.type != null && !this.type.isEmpty(); 1064 } 1065 1066 /** 1067 * @param value {@link #type} (Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.) 1068 */ 1069 public DeviceMetric setType(CodeableConcept value) { 1070 this.type = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return {@link #unit} (Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.) 1076 */ 1077 public CodeableConcept getUnit() { 1078 if (this.unit == null) 1079 if (Configuration.errorOnAutoCreate()) 1080 throw new Error("Attempt to auto-create DeviceMetric.unit"); 1081 else if (Configuration.doAutoCreate()) 1082 this.unit = new CodeableConcept(); // cc 1083 return this.unit; 1084 } 1085 1086 public boolean hasUnit() { 1087 return this.unit != null && !this.unit.isEmpty(); 1088 } 1089 1090 /** 1091 * @param value {@link #unit} (Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.) 1092 */ 1093 public DeviceMetric setUnit(CodeableConcept value) { 1094 this.unit = value; 1095 return this; 1096 } 1097 1098 /** 1099 * @return {@link #device} (Describes the link to the Device. This is also known as a channel device.) 1100 */ 1101 public Reference getDevice() { 1102 if (this.device == null) 1103 if (Configuration.errorOnAutoCreate()) 1104 throw new Error("Attempt to auto-create DeviceMetric.device"); 1105 else if (Configuration.doAutoCreate()) 1106 this.device = new Reference(); // cc 1107 return this.device; 1108 } 1109 1110 public boolean hasDevice() { 1111 return this.device != null && !this.device.isEmpty(); 1112 } 1113 1114 /** 1115 * @param value {@link #device} (Describes the link to the Device. This is also known as a channel device.) 1116 */ 1117 public DeviceMetric setDevice(Reference value) { 1118 this.device = value; 1119 return this; 1120 } 1121 1122 /** 1123 * @return {@link #operationalStatus} (Indicates current operational state of the device. For example: On, Off, Standby, etc.). This is the underlying object with id, value and extensions. The accessor "getOperationalStatus" gives direct access to the value 1124 */ 1125 public Enumeration<DeviceMetricOperationalStatus> getOperationalStatusElement() { 1126 if (this.operationalStatus == null) 1127 if (Configuration.errorOnAutoCreate()) 1128 throw new Error("Attempt to auto-create DeviceMetric.operationalStatus"); 1129 else if (Configuration.doAutoCreate()) 1130 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>(new DeviceMetricOperationalStatusEnumFactory()); // bb 1131 return this.operationalStatus; 1132 } 1133 1134 public boolean hasOperationalStatusElement() { 1135 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1136 } 1137 1138 public boolean hasOperationalStatus() { 1139 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1140 } 1141 1142 /** 1143 * @param value {@link #operationalStatus} (Indicates current operational state of the device. For example: On, Off, Standby, etc.). This is the underlying object with id, value and extensions. The accessor "getOperationalStatus" gives direct access to the value 1144 */ 1145 public DeviceMetric setOperationalStatusElement(Enumeration<DeviceMetricOperationalStatus> value) { 1146 this.operationalStatus = value; 1147 return this; 1148 } 1149 1150 /** 1151 * @return Indicates current operational state of the device. For example: On, Off, Standby, etc. 1152 */ 1153 public DeviceMetricOperationalStatus getOperationalStatus() { 1154 return this.operationalStatus == null ? null : this.operationalStatus.getValue(); 1155 } 1156 1157 /** 1158 * @param value Indicates current operational state of the device. For example: On, Off, Standby, etc. 1159 */ 1160 public DeviceMetric setOperationalStatus(DeviceMetricOperationalStatus value) { 1161 if (value == null) 1162 this.operationalStatus = null; 1163 else { 1164 if (this.operationalStatus == null) 1165 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>(new DeviceMetricOperationalStatusEnumFactory()); 1166 this.operationalStatus.setValue(value); 1167 } 1168 return this; 1169 } 1170 1171 /** 1172 * @return {@link #color} (The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1173 */ 1174 public CodeType getColorElement() { 1175 if (this.color == null) 1176 if (Configuration.errorOnAutoCreate()) 1177 throw new Error("Attempt to auto-create DeviceMetric.color"); 1178 else if (Configuration.doAutoCreate()) 1179 this.color = new CodeType(); // bb 1180 return this.color; 1181 } 1182 1183 public boolean hasColorElement() { 1184 return this.color != null && !this.color.isEmpty(); 1185 } 1186 1187 public boolean hasColor() { 1188 return this.color != null && !this.color.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #color} (The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1193 */ 1194 public DeviceMetric setColorElement(CodeType value) { 1195 this.color = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta. 1201 */ 1202 public String getColor() { 1203 return this.color == null ? null : this.color.getValue(); 1204 } 1205 1206 /** 1207 * @param value The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta. 1208 */ 1209 public DeviceMetric setColor(String value) { 1210 if (Utilities.noString(value)) 1211 this.color = null; 1212 else { 1213 if (this.color == null) 1214 this.color = new CodeType(); 1215 this.color.setValue(value); 1216 } 1217 return this; 1218 } 1219 1220 /** 1221 * @return {@link #category} (Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1222 */ 1223 public Enumeration<DeviceMetricCategory> getCategoryElement() { 1224 if (this.category == null) 1225 if (Configuration.errorOnAutoCreate()) 1226 throw new Error("Attempt to auto-create DeviceMetric.category"); 1227 else if (Configuration.doAutoCreate()) 1228 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); // bb 1229 return this.category; 1230 } 1231 1232 public boolean hasCategoryElement() { 1233 return this.category != null && !this.category.isEmpty(); 1234 } 1235 1236 public boolean hasCategory() { 1237 return this.category != null && !this.category.isEmpty(); 1238 } 1239 1240 /** 1241 * @param value {@link #category} (Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1242 */ 1243 public DeviceMetric setCategoryElement(Enumeration<DeviceMetricCategory> value) { 1244 this.category = value; 1245 return this; 1246 } 1247 1248 /** 1249 * @return Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 1250 */ 1251 public DeviceMetricCategory getCategory() { 1252 return this.category == null ? null : this.category.getValue(); 1253 } 1254 1255 /** 1256 * @param value Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 1257 */ 1258 public DeviceMetric setCategory(DeviceMetricCategory value) { 1259 if (this.category == null) 1260 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); 1261 this.category.setValue(value); 1262 return this; 1263 } 1264 1265 /** 1266 * @return {@link #measurementFrequency} (The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.) 1267 */ 1268 public Quantity getMeasurementFrequency() { 1269 if (this.measurementFrequency == null) 1270 if (Configuration.errorOnAutoCreate()) 1271 throw new Error("Attempt to auto-create DeviceMetric.measurementFrequency"); 1272 else if (Configuration.doAutoCreate()) 1273 this.measurementFrequency = new Quantity(); // cc 1274 return this.measurementFrequency; 1275 } 1276 1277 public boolean hasMeasurementFrequency() { 1278 return this.measurementFrequency != null && !this.measurementFrequency.isEmpty(); 1279 } 1280 1281 /** 1282 * @param value {@link #measurementFrequency} (The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.) 1283 */ 1284 public DeviceMetric setMeasurementFrequency(Quantity value) { 1285 this.measurementFrequency = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return {@link #calibration} (Describes the calibrations that have been performed or that are required to be performed.) 1291 */ 1292 public List<DeviceMetricCalibrationComponent> getCalibration() { 1293 if (this.calibration == null) 1294 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1295 return this.calibration; 1296 } 1297 1298 /** 1299 * @return Returns a reference to <code>this</code> for easy method chaining 1300 */ 1301 public DeviceMetric setCalibration(List<DeviceMetricCalibrationComponent> theCalibration) { 1302 this.calibration = theCalibration; 1303 return this; 1304 } 1305 1306 public boolean hasCalibration() { 1307 if (this.calibration == null) 1308 return false; 1309 for (DeviceMetricCalibrationComponent item : this.calibration) 1310 if (!item.isEmpty()) 1311 return true; 1312 return false; 1313 } 1314 1315 public DeviceMetricCalibrationComponent addCalibration() { //3 1316 DeviceMetricCalibrationComponent t = new DeviceMetricCalibrationComponent(); 1317 if (this.calibration == null) 1318 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1319 this.calibration.add(t); 1320 return t; 1321 } 1322 1323 public DeviceMetric addCalibration(DeviceMetricCalibrationComponent t) { //3 1324 if (t == null) 1325 return this; 1326 if (this.calibration == null) 1327 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1328 this.calibration.add(t); 1329 return this; 1330 } 1331 1332 /** 1333 * @return The first repetition of repeating field {@link #calibration}, creating it if it does not already exist {3} 1334 */ 1335 public DeviceMetricCalibrationComponent getCalibrationFirstRep() { 1336 if (getCalibration().isEmpty()) { 1337 addCalibration(); 1338 } 1339 return getCalibration().get(0); 1340 } 1341 1342 protected void listChildren(List<Property> children) { 1343 super.listChildren(children); 1344 children.add(new Property("identifier", "Identifier", "Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1345 children.add(new Property("type", "CodeableConcept", "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type)); 1346 children.add(new Property("unit", "CodeableConcept", "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 0, 1, unit)); 1347 children.add(new Property("device", "Reference(Device)", "Describes the link to the Device. This is also known as a channel device.", 0, 1, device)); 1348 children.add(new Property("operationalStatus", "code", "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, operationalStatus)); 1349 children.add(new Property("color", "code", "The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.", 0, 1, color)); 1350 children.add(new Property("category", "code", "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 0, 1, category)); 1351 children.add(new Property("measurementFrequency", "Quantity", "The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.", 0, 1, measurementFrequency)); 1352 children.add(new Property("calibration", "", "Describes the calibrations that have been performed or that are required to be performed.", 0, java.lang.Integer.MAX_VALUE, calibration)); 1353 } 1354 1355 @Override 1356 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1357 switch (_hash) { 1358 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID.", 0, java.lang.Integer.MAX_VALUE, identifier); 1359 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type); 1360 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 0, 1, unit); 1361 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "Describes the link to the Device. This is also known as a channel device.", 0, 1, device); 1362 case -2103166364: /*operationalStatus*/ return new Property("operationalStatus", "code", "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, operationalStatus); 1363 case 94842723: /*color*/ return new Property("color", "code", "The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.", 0, 1, color); 1364 case 50511102: /*category*/ return new Property("category", "code", "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 0, 1, category); 1365 case 1766341888: /*measurementFrequency*/ return new Property("measurementFrequency", "Quantity", "The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.", 0, 1, measurementFrequency); 1366 case 1421318634: /*calibration*/ return new Property("calibration", "", "Describes the calibrations that have been performed or that are required to be performed.", 0, java.lang.Integer.MAX_VALUE, calibration); 1367 default: return super.getNamedProperty(_hash, _name, _checkValid); 1368 } 1369 1370 } 1371 1372 @Override 1373 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1374 switch (hash) { 1375 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1376 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1377 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 1378 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 1379 case -2103166364: /*operationalStatus*/ return this.operationalStatus == null ? new Base[0] : new Base[] {this.operationalStatus}; // Enumeration<DeviceMetricOperationalStatus> 1380 case 94842723: /*color*/ return this.color == null ? new Base[0] : new Base[] {this.color}; // CodeType 1381 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<DeviceMetricCategory> 1382 case 1766341888: /*measurementFrequency*/ return this.measurementFrequency == null ? new Base[0] : new Base[] {this.measurementFrequency}; // Quantity 1383 case 1421318634: /*calibration*/ return this.calibration == null ? new Base[0] : this.calibration.toArray(new Base[this.calibration.size()]); // DeviceMetricCalibrationComponent 1384 default: return super.getProperty(hash, name, checkValid); 1385 } 1386 1387 } 1388 1389 @Override 1390 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1391 switch (hash) { 1392 case -1618432855: // identifier 1393 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1394 return value; 1395 case 3575610: // type 1396 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1397 return value; 1398 case 3594628: // unit 1399 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1400 return value; 1401 case -1335157162: // device 1402 this.device = TypeConvertor.castToReference(value); // Reference 1403 return value; 1404 case -2103166364: // operationalStatus 1405 value = new DeviceMetricOperationalStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1406 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1407 return value; 1408 case 94842723: // color 1409 this.color = TypeConvertor.castToCode(value); // CodeType 1410 return value; 1411 case 50511102: // category 1412 value = new DeviceMetricCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1413 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1414 return value; 1415 case 1766341888: // measurementFrequency 1416 this.measurementFrequency = TypeConvertor.castToQuantity(value); // Quantity 1417 return value; 1418 case 1421318634: // calibration 1419 this.getCalibration().add((DeviceMetricCalibrationComponent) value); // DeviceMetricCalibrationComponent 1420 return value; 1421 default: return super.setProperty(hash, name, value); 1422 } 1423 1424 } 1425 1426 @Override 1427 public Base setProperty(String name, Base value) throws FHIRException { 1428 if (name.equals("identifier")) { 1429 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1430 } else if (name.equals("type")) { 1431 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1432 } else if (name.equals("unit")) { 1433 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1434 } else if (name.equals("device")) { 1435 this.device = TypeConvertor.castToReference(value); // Reference 1436 } else if (name.equals("operationalStatus")) { 1437 value = new DeviceMetricOperationalStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1438 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1439 } else if (name.equals("color")) { 1440 this.color = TypeConvertor.castToCode(value); // CodeType 1441 } else if (name.equals("category")) { 1442 value = new DeviceMetricCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1443 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1444 } else if (name.equals("measurementFrequency")) { 1445 this.measurementFrequency = TypeConvertor.castToQuantity(value); // Quantity 1446 } else if (name.equals("calibration")) { 1447 this.getCalibration().add((DeviceMetricCalibrationComponent) value); 1448 } else 1449 return super.setProperty(name, value); 1450 return value; 1451 } 1452 1453 @Override 1454 public void removeChild(String name, Base value) throws FHIRException { 1455 if (name.equals("identifier")) { 1456 this.getIdentifier().remove(value); 1457 } else if (name.equals("type")) { 1458 this.type = null; 1459 } else if (name.equals("unit")) { 1460 this.unit = null; 1461 } else if (name.equals("device")) { 1462 this.device = null; 1463 } else if (name.equals("operationalStatus")) { 1464 value = new DeviceMetricOperationalStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1465 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1466 } else if (name.equals("color")) { 1467 this.color = null; 1468 } else if (name.equals("category")) { 1469 value = new DeviceMetricCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1470 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1471 } else if (name.equals("measurementFrequency")) { 1472 this.measurementFrequency = null; 1473 } else if (name.equals("calibration")) { 1474 this.getCalibration().remove((DeviceMetricCalibrationComponent) value); 1475 } else 1476 super.removeChild(name, value); 1477 1478 } 1479 1480 @Override 1481 public Base makeProperty(int hash, String name) throws FHIRException { 1482 switch (hash) { 1483 case -1618432855: return addIdentifier(); 1484 case 3575610: return getType(); 1485 case 3594628: return getUnit(); 1486 case -1335157162: return getDevice(); 1487 case -2103166364: return getOperationalStatusElement(); 1488 case 94842723: return getColorElement(); 1489 case 50511102: return getCategoryElement(); 1490 case 1766341888: return getMeasurementFrequency(); 1491 case 1421318634: return addCalibration(); 1492 default: return super.makeProperty(hash, name); 1493 } 1494 1495 } 1496 1497 @Override 1498 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1499 switch (hash) { 1500 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1501 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1502 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 1503 case -1335157162: /*device*/ return new String[] {"Reference"}; 1504 case -2103166364: /*operationalStatus*/ return new String[] {"code"}; 1505 case 94842723: /*color*/ return new String[] {"code"}; 1506 case 50511102: /*category*/ return new String[] {"code"}; 1507 case 1766341888: /*measurementFrequency*/ return new String[] {"Quantity"}; 1508 case 1421318634: /*calibration*/ return new String[] {}; 1509 default: return super.getTypesForProperty(hash, name); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base addChild(String name) throws FHIRException { 1516 if (name.equals("identifier")) { 1517 return addIdentifier(); 1518 } 1519 else if (name.equals("type")) { 1520 this.type = new CodeableConcept(); 1521 return this.type; 1522 } 1523 else if (name.equals("unit")) { 1524 this.unit = new CodeableConcept(); 1525 return this.unit; 1526 } 1527 else if (name.equals("device")) { 1528 this.device = new Reference(); 1529 return this.device; 1530 } 1531 else if (name.equals("operationalStatus")) { 1532 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.operationalStatus"); 1533 } 1534 else if (name.equals("color")) { 1535 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.color"); 1536 } 1537 else if (name.equals("category")) { 1538 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.category"); 1539 } 1540 else if (name.equals("measurementFrequency")) { 1541 this.measurementFrequency = new Quantity(); 1542 return this.measurementFrequency; 1543 } 1544 else if (name.equals("calibration")) { 1545 return addCalibration(); 1546 } 1547 else 1548 return super.addChild(name); 1549 } 1550 1551 public String fhirType() { 1552 return "DeviceMetric"; 1553 1554 } 1555 1556 public DeviceMetric copy() { 1557 DeviceMetric dst = new DeviceMetric(); 1558 copyValues(dst); 1559 return dst; 1560 } 1561 1562 public void copyValues(DeviceMetric dst) { 1563 super.copyValues(dst); 1564 if (identifier != null) { 1565 dst.identifier = new ArrayList<Identifier>(); 1566 for (Identifier i : identifier) 1567 dst.identifier.add(i.copy()); 1568 }; 1569 dst.type = type == null ? null : type.copy(); 1570 dst.unit = unit == null ? null : unit.copy(); 1571 dst.device = device == null ? null : device.copy(); 1572 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 1573 dst.color = color == null ? null : color.copy(); 1574 dst.category = category == null ? null : category.copy(); 1575 dst.measurementFrequency = measurementFrequency == null ? null : measurementFrequency.copy(); 1576 if (calibration != null) { 1577 dst.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1578 for (DeviceMetricCalibrationComponent i : calibration) 1579 dst.calibration.add(i.copy()); 1580 }; 1581 } 1582 1583 protected DeviceMetric typedCopy() { 1584 return copy(); 1585 } 1586 1587 @Override 1588 public boolean equalsDeep(Base other_) { 1589 if (!super.equalsDeep(other_)) 1590 return false; 1591 if (!(other_ instanceof DeviceMetric)) 1592 return false; 1593 DeviceMetric o = (DeviceMetric) other_; 1594 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(unit, o.unit, true) 1595 && compareDeep(device, o.device, true) && compareDeep(operationalStatus, o.operationalStatus, true) 1596 && compareDeep(color, o.color, true) && compareDeep(category, o.category, true) && compareDeep(measurementFrequency, o.measurementFrequency, true) 1597 && compareDeep(calibration, o.calibration, true); 1598 } 1599 1600 @Override 1601 public boolean equalsShallow(Base other_) { 1602 if (!super.equalsShallow(other_)) 1603 return false; 1604 if (!(other_ instanceof DeviceMetric)) 1605 return false; 1606 DeviceMetric o = (DeviceMetric) other_; 1607 return compareValues(operationalStatus, o.operationalStatus, true) && compareValues(color, o.color, true) 1608 && compareValues(category, o.category, true); 1609 } 1610 1611 public boolean isEmpty() { 1612 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, unit, device 1613 , operationalStatus, color, category, measurementFrequency, calibration); 1614 } 1615 1616 @Override 1617 public ResourceType getResourceType() { 1618 return ResourceType.DeviceMetric; 1619 } 1620 1621 /** 1622 * Search parameter: <b>category</b> 1623 * <p> 1624 * Description: <b>The category of the metric</b><br> 1625 * Type: <b>token</b><br> 1626 * Path: <b>DeviceMetric.category</b><br> 1627 * </p> 1628 */ 1629 @SearchParamDefinition(name="category", path="DeviceMetric.category", description="The category of the metric", type="token" ) 1630 public static final String SP_CATEGORY = "category"; 1631 /** 1632 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1633 * <p> 1634 * Description: <b>The category of the metric</b><br> 1635 * Type: <b>token</b><br> 1636 * Path: <b>DeviceMetric.category</b><br> 1637 * </p> 1638 */ 1639 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1640 1641 /** 1642 * Search parameter: <b>device</b> 1643 * <p> 1644 * Description: <b>The device resource</b><br> 1645 * Type: <b>reference</b><br> 1646 * Path: <b>DeviceMetric.device</b><br> 1647 * </p> 1648 */ 1649 @SearchParamDefinition(name="device", path="DeviceMetric.device", description="The device resource", type="reference", target={Device.class } ) 1650 public static final String SP_DEVICE = "device"; 1651 /** 1652 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1653 * <p> 1654 * Description: <b>The device resource</b><br> 1655 * Type: <b>reference</b><br> 1656 * Path: <b>DeviceMetric.device</b><br> 1657 * </p> 1658 */ 1659 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 1660 1661/** 1662 * Constant for fluent queries to be used to add include statements. Specifies 1663 * the path value of "<b>DeviceMetric:device</b>". 1664 */ 1665 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceMetric:device").toLocked(); 1666 1667 /** 1668 * Search parameter: <b>identifier</b> 1669 * <p> 1670 * Description: <b>The identifier of the metric</b><br> 1671 * Type: <b>token</b><br> 1672 * Path: <b>DeviceMetric.identifier</b><br> 1673 * </p> 1674 */ 1675 @SearchParamDefinition(name="identifier", path="DeviceMetric.identifier", description="The identifier of the metric", type="token" ) 1676 public static final String SP_IDENTIFIER = "identifier"; 1677 /** 1678 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1679 * <p> 1680 * Description: <b>The identifier of the metric</b><br> 1681 * Type: <b>token</b><br> 1682 * Path: <b>DeviceMetric.identifier</b><br> 1683 * </p> 1684 */ 1685 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1686 1687 /** 1688 * Search parameter: <b>type</b> 1689 * <p> 1690 * Description: <b>The type of metric</b><br> 1691 * Type: <b>token</b><br> 1692 * Path: <b>DeviceMetric.type</b><br> 1693 * </p> 1694 */ 1695 @SearchParamDefinition(name="type", path="DeviceMetric.type", description="The type of metric", type="token" ) 1696 public static final String SP_TYPE = "type"; 1697 /** 1698 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1699 * <p> 1700 * Description: <b>The type of metric</b><br> 1701 * Type: <b>token</b><br> 1702 * Path: <b>DeviceMetric.type</b><br> 1703 * </p> 1704 */ 1705 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1706 1707 1708} 1709