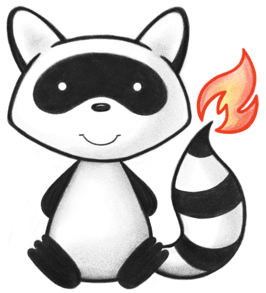
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes a measurement, calculation or setting capability of a device. The DeviceMetric resource is derived from the ISO/IEEE 11073-10201 Domain Information Model standard, but is more widely applicable. 052 */ 053@ResourceDef(name="DeviceMetric", profile="http://hl7.org/fhir/StructureDefinition/DeviceMetric") 054public class DeviceMetric extends DomainResource { 055 056 public enum DeviceMetricCalibrationState { 057 /** 058 * The metric has not been calibrated. 059 */ 060 NOTCALIBRATED, 061 /** 062 * The metric needs to be calibrated. 063 */ 064 CALIBRATIONREQUIRED, 065 /** 066 * The metric has been calibrated. 067 */ 068 CALIBRATED, 069 /** 070 * The state of calibration of this metric is unspecified. 071 */ 072 UNSPECIFIED, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static DeviceMetricCalibrationState fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("not-calibrated".equals(codeString)) 081 return NOTCALIBRATED; 082 if ("calibration-required".equals(codeString)) 083 return CALIBRATIONREQUIRED; 084 if ("calibrated".equals(codeString)) 085 return CALIBRATED; 086 if ("unspecified".equals(codeString)) 087 return UNSPECIFIED; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case NOTCALIBRATED: return "not-calibrated"; 096 case CALIBRATIONREQUIRED: return "calibration-required"; 097 case CALIBRATED: return "calibrated"; 098 case UNSPECIFIED: return "unspecified"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case NOTCALIBRATED: return "http://hl7.org/fhir/metric-calibration-state"; 106 case CALIBRATIONREQUIRED: return "http://hl7.org/fhir/metric-calibration-state"; 107 case CALIBRATED: return "http://hl7.org/fhir/metric-calibration-state"; 108 case UNSPECIFIED: return "http://hl7.org/fhir/metric-calibration-state"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case NOTCALIBRATED: return "The metric has not been calibrated."; 116 case CALIBRATIONREQUIRED: return "The metric needs to be calibrated."; 117 case CALIBRATED: return "The metric has been calibrated."; 118 case UNSPECIFIED: return "The state of calibration of this metric is unspecified."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case NOTCALIBRATED: return "Not Calibrated"; 126 case CALIBRATIONREQUIRED: return "Calibration Required"; 127 case CALIBRATED: return "Calibrated"; 128 case UNSPECIFIED: return "Unspecified"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class DeviceMetricCalibrationStateEnumFactory implements EnumFactory<DeviceMetricCalibrationState> { 136 public DeviceMetricCalibrationState fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("not-calibrated".equals(codeString)) 141 return DeviceMetricCalibrationState.NOTCALIBRATED; 142 if ("calibration-required".equals(codeString)) 143 return DeviceMetricCalibrationState.CALIBRATIONREQUIRED; 144 if ("calibrated".equals(codeString)) 145 return DeviceMetricCalibrationState.CALIBRATED; 146 if ("unspecified".equals(codeString)) 147 return DeviceMetricCalibrationState.UNSPECIFIED; 148 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 149 } 150 public Enumeration<DeviceMetricCalibrationState> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NULL, code); 158 if ("not-calibrated".equals(codeString)) 159 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.NOTCALIBRATED, code); 160 if ("calibration-required".equals(codeString)) 161 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATIONREQUIRED, code); 162 if ("calibrated".equals(codeString)) 163 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.CALIBRATED, code); 164 if ("unspecified".equals(codeString)) 165 return new Enumeration<DeviceMetricCalibrationState>(this, DeviceMetricCalibrationState.UNSPECIFIED, code); 166 throw new FHIRException("Unknown DeviceMetricCalibrationState code '"+codeString+"'"); 167 } 168 public String toCode(DeviceMetricCalibrationState code) { 169 if (code == DeviceMetricCalibrationState.NULL) 170 return null; 171 if (code == DeviceMetricCalibrationState.NOTCALIBRATED) 172 return "not-calibrated"; 173 if (code == DeviceMetricCalibrationState.CALIBRATIONREQUIRED) 174 return "calibration-required"; 175 if (code == DeviceMetricCalibrationState.CALIBRATED) 176 return "calibrated"; 177 if (code == DeviceMetricCalibrationState.UNSPECIFIED) 178 return "unspecified"; 179 return "?"; 180 } 181 public String toSystem(DeviceMetricCalibrationState code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum DeviceMetricCalibrationType { 187 /** 188 * Metric calibration method has not been identified. 189 */ 190 UNSPECIFIED, 191 /** 192 * Offset metric calibration method. 193 */ 194 OFFSET, 195 /** 196 * Gain metric calibration method. 197 */ 198 GAIN, 199 /** 200 * Two-point metric calibration method. 201 */ 202 TWOPOINT, 203 /** 204 * added to help the parsers with the generic types 205 */ 206 NULL; 207 public static DeviceMetricCalibrationType fromCode(String codeString) throws FHIRException { 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("unspecified".equals(codeString)) 211 return UNSPECIFIED; 212 if ("offset".equals(codeString)) 213 return OFFSET; 214 if ("gain".equals(codeString)) 215 return GAIN; 216 if ("two-point".equals(codeString)) 217 return TWOPOINT; 218 if (Configuration.isAcceptInvalidEnums()) 219 return null; 220 else 221 throw new FHIRException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 222 } 223 public String toCode() { 224 switch (this) { 225 case UNSPECIFIED: return "unspecified"; 226 case OFFSET: return "offset"; 227 case GAIN: return "gain"; 228 case TWOPOINT: return "two-point"; 229 case NULL: return null; 230 default: return "?"; 231 } 232 } 233 public String getSystem() { 234 switch (this) { 235 case UNSPECIFIED: return "http://hl7.org/fhir/metric-calibration-type"; 236 case OFFSET: return "http://hl7.org/fhir/metric-calibration-type"; 237 case GAIN: return "http://hl7.org/fhir/metric-calibration-type"; 238 case TWOPOINT: return "http://hl7.org/fhir/metric-calibration-type"; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 public String getDefinition() { 244 switch (this) { 245 case UNSPECIFIED: return "Metric calibration method has not been identified."; 246 case OFFSET: return "Offset metric calibration method."; 247 case GAIN: return "Gain metric calibration method."; 248 case TWOPOINT: return "Two-point metric calibration method."; 249 case NULL: return null; 250 default: return "?"; 251 } 252 } 253 public String getDisplay() { 254 switch (this) { 255 case UNSPECIFIED: return "Unspecified"; 256 case OFFSET: return "Offset"; 257 case GAIN: return "Gain"; 258 case TWOPOINT: return "Two Point"; 259 case NULL: return null; 260 default: return "?"; 261 } 262 } 263 } 264 265 public static class DeviceMetricCalibrationTypeEnumFactory implements EnumFactory<DeviceMetricCalibrationType> { 266 public DeviceMetricCalibrationType fromCode(String codeString) throws IllegalArgumentException { 267 if (codeString == null || "".equals(codeString)) 268 if (codeString == null || "".equals(codeString)) 269 return null; 270 if ("unspecified".equals(codeString)) 271 return DeviceMetricCalibrationType.UNSPECIFIED; 272 if ("offset".equals(codeString)) 273 return DeviceMetricCalibrationType.OFFSET; 274 if ("gain".equals(codeString)) 275 return DeviceMetricCalibrationType.GAIN; 276 if ("two-point".equals(codeString)) 277 return DeviceMetricCalibrationType.TWOPOINT; 278 throw new IllegalArgumentException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 279 } 280 public Enumeration<DeviceMetricCalibrationType> fromType(PrimitiveType<?> code) throws FHIRException { 281 if (code == null) 282 return null; 283 if (code.isEmpty()) 284 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 285 String codeString = ((PrimitiveType) code).asStringValue(); 286 if (codeString == null || "".equals(codeString)) 287 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.NULL, code); 288 if ("unspecified".equals(codeString)) 289 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.UNSPECIFIED, code); 290 if ("offset".equals(codeString)) 291 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.OFFSET, code); 292 if ("gain".equals(codeString)) 293 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.GAIN, code); 294 if ("two-point".equals(codeString)) 295 return new Enumeration<DeviceMetricCalibrationType>(this, DeviceMetricCalibrationType.TWOPOINT, code); 296 throw new FHIRException("Unknown DeviceMetricCalibrationType code '"+codeString+"'"); 297 } 298 public String toCode(DeviceMetricCalibrationType code) { 299 if (code == DeviceMetricCalibrationType.NULL) 300 return null; 301 if (code == DeviceMetricCalibrationType.UNSPECIFIED) 302 return "unspecified"; 303 if (code == DeviceMetricCalibrationType.OFFSET) 304 return "offset"; 305 if (code == DeviceMetricCalibrationType.GAIN) 306 return "gain"; 307 if (code == DeviceMetricCalibrationType.TWOPOINT) 308 return "two-point"; 309 return "?"; 310 } 311 public String toSystem(DeviceMetricCalibrationType code) { 312 return code.getSystem(); 313 } 314 } 315 316 public enum DeviceMetricCategory { 317 /** 318 * Observations generated for this DeviceMetric are measured. 319 */ 320 MEASUREMENT, 321 /** 322 * Observations generated for this DeviceMetric is a setting that will influence the behavior of the Device. 323 */ 324 SETTING, 325 /** 326 * Observations generated for this DeviceMetric are calculated. 327 */ 328 CALCULATION, 329 /** 330 * The category of this DeviceMetric is unspecified. 331 */ 332 UNSPECIFIED, 333 /** 334 * added to help the parsers with the generic types 335 */ 336 NULL; 337 public static DeviceMetricCategory fromCode(String codeString) throws FHIRException { 338 if (codeString == null || "".equals(codeString)) 339 return null; 340 if ("measurement".equals(codeString)) 341 return MEASUREMENT; 342 if ("setting".equals(codeString)) 343 return SETTING; 344 if ("calculation".equals(codeString)) 345 return CALCULATION; 346 if ("unspecified".equals(codeString)) 347 return UNSPECIFIED; 348 if (Configuration.isAcceptInvalidEnums()) 349 return null; 350 else 351 throw new FHIRException("Unknown DeviceMetricCategory code '"+codeString+"'"); 352 } 353 public String toCode() { 354 switch (this) { 355 case MEASUREMENT: return "measurement"; 356 case SETTING: return "setting"; 357 case CALCULATION: return "calculation"; 358 case UNSPECIFIED: return "unspecified"; 359 case NULL: return null; 360 default: return "?"; 361 } 362 } 363 public String getSystem() { 364 switch (this) { 365 case MEASUREMENT: return "http://hl7.org/fhir/metric-category"; 366 case SETTING: return "http://hl7.org/fhir/metric-category"; 367 case CALCULATION: return "http://hl7.org/fhir/metric-category"; 368 case UNSPECIFIED: return "http://hl7.org/fhir/metric-category"; 369 case NULL: return null; 370 default: return "?"; 371 } 372 } 373 public String getDefinition() { 374 switch (this) { 375 case MEASUREMENT: return "Observations generated for this DeviceMetric are measured."; 376 case SETTING: return "Observations generated for this DeviceMetric is a setting that will influence the behavior of the Device."; 377 case CALCULATION: return "Observations generated for this DeviceMetric are calculated."; 378 case UNSPECIFIED: return "The category of this DeviceMetric is unspecified."; 379 case NULL: return null; 380 default: return "?"; 381 } 382 } 383 public String getDisplay() { 384 switch (this) { 385 case MEASUREMENT: return "Measurement"; 386 case SETTING: return "Setting"; 387 case CALCULATION: return "Calculation"; 388 case UNSPECIFIED: return "Unspecified"; 389 case NULL: return null; 390 default: return "?"; 391 } 392 } 393 } 394 395 public static class DeviceMetricCategoryEnumFactory implements EnumFactory<DeviceMetricCategory> { 396 public DeviceMetricCategory fromCode(String codeString) throws IllegalArgumentException { 397 if (codeString == null || "".equals(codeString)) 398 if (codeString == null || "".equals(codeString)) 399 return null; 400 if ("measurement".equals(codeString)) 401 return DeviceMetricCategory.MEASUREMENT; 402 if ("setting".equals(codeString)) 403 return DeviceMetricCategory.SETTING; 404 if ("calculation".equals(codeString)) 405 return DeviceMetricCategory.CALCULATION; 406 if ("unspecified".equals(codeString)) 407 return DeviceMetricCategory.UNSPECIFIED; 408 throw new IllegalArgumentException("Unknown DeviceMetricCategory code '"+codeString+"'"); 409 } 410 public Enumeration<DeviceMetricCategory> fromType(PrimitiveType<?> code) throws FHIRException { 411 if (code == null) 412 return null; 413 if (code.isEmpty()) 414 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 415 String codeString = ((PrimitiveType) code).asStringValue(); 416 if (codeString == null || "".equals(codeString)) 417 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.NULL, code); 418 if ("measurement".equals(codeString)) 419 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.MEASUREMENT, code); 420 if ("setting".equals(codeString)) 421 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.SETTING, code); 422 if ("calculation".equals(codeString)) 423 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.CALCULATION, code); 424 if ("unspecified".equals(codeString)) 425 return new Enumeration<DeviceMetricCategory>(this, DeviceMetricCategory.UNSPECIFIED, code); 426 throw new FHIRException("Unknown DeviceMetricCategory code '"+codeString+"'"); 427 } 428 public String toCode(DeviceMetricCategory code) { 429 if (code == DeviceMetricCategory.NULL) 430 return null; 431 if (code == DeviceMetricCategory.MEASUREMENT) 432 return "measurement"; 433 if (code == DeviceMetricCategory.SETTING) 434 return "setting"; 435 if (code == DeviceMetricCategory.CALCULATION) 436 return "calculation"; 437 if (code == DeviceMetricCategory.UNSPECIFIED) 438 return "unspecified"; 439 return "?"; 440 } 441 public String toSystem(DeviceMetricCategory code) { 442 return code.getSystem(); 443 } 444 } 445 446 public enum DeviceMetricOperationalStatus { 447 /** 448 * The DeviceMetric is operating and will generate Observations. 449 */ 450 ON, 451 /** 452 * The DeviceMetric is not operating. 453 */ 454 OFF, 455 /** 456 * The DeviceMetric is operating, but will not generate any Observations. 457 */ 458 STANDBY, 459 /** 460 * The DeviceMetric was entered in error. 461 */ 462 ENTEREDINERROR, 463 /** 464 * added to help the parsers with the generic types 465 */ 466 NULL; 467 public static DeviceMetricOperationalStatus fromCode(String codeString) throws FHIRException { 468 if (codeString == null || "".equals(codeString)) 469 return null; 470 if ("on".equals(codeString)) 471 return ON; 472 if ("off".equals(codeString)) 473 return OFF; 474 if ("standby".equals(codeString)) 475 return STANDBY; 476 if ("entered-in-error".equals(codeString)) 477 return ENTEREDINERROR; 478 if (Configuration.isAcceptInvalidEnums()) 479 return null; 480 else 481 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 482 } 483 public String toCode() { 484 switch (this) { 485 case ON: return "on"; 486 case OFF: return "off"; 487 case STANDBY: return "standby"; 488 case ENTEREDINERROR: return "entered-in-error"; 489 case NULL: return null; 490 default: return "?"; 491 } 492 } 493 public String getSystem() { 494 switch (this) { 495 case ON: return "http://hl7.org/fhir/metric-operational-status"; 496 case OFF: return "http://hl7.org/fhir/metric-operational-status"; 497 case STANDBY: return "http://hl7.org/fhir/metric-operational-status"; 498 case ENTEREDINERROR: return "http://hl7.org/fhir/metric-operational-status"; 499 case NULL: return null; 500 default: return "?"; 501 } 502 } 503 public String getDefinition() { 504 switch (this) { 505 case ON: return "The DeviceMetric is operating and will generate Observations."; 506 case OFF: return "The DeviceMetric is not operating."; 507 case STANDBY: return "The DeviceMetric is operating, but will not generate any Observations."; 508 case ENTEREDINERROR: return "The DeviceMetric was entered in error."; 509 case NULL: return null; 510 default: return "?"; 511 } 512 } 513 public String getDisplay() { 514 switch (this) { 515 case ON: return "On"; 516 case OFF: return "Off"; 517 case STANDBY: return "Standby"; 518 case ENTEREDINERROR: return "Entered In Error"; 519 case NULL: return null; 520 default: return "?"; 521 } 522 } 523 } 524 525 public static class DeviceMetricOperationalStatusEnumFactory implements EnumFactory<DeviceMetricOperationalStatus> { 526 public DeviceMetricOperationalStatus fromCode(String codeString) throws IllegalArgumentException { 527 if (codeString == null || "".equals(codeString)) 528 if (codeString == null || "".equals(codeString)) 529 return null; 530 if ("on".equals(codeString)) 531 return DeviceMetricOperationalStatus.ON; 532 if ("off".equals(codeString)) 533 return DeviceMetricOperationalStatus.OFF; 534 if ("standby".equals(codeString)) 535 return DeviceMetricOperationalStatus.STANDBY; 536 if ("entered-in-error".equals(codeString)) 537 return DeviceMetricOperationalStatus.ENTEREDINERROR; 538 throw new IllegalArgumentException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 539 } 540 public Enumeration<DeviceMetricOperationalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 541 if (code == null) 542 return null; 543 if (code.isEmpty()) 544 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 545 String codeString = ((PrimitiveType) code).asStringValue(); 546 if (codeString == null || "".equals(codeString)) 547 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.NULL, code); 548 if ("on".equals(codeString)) 549 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ON, code); 550 if ("off".equals(codeString)) 551 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.OFF, code); 552 if ("standby".equals(codeString)) 553 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.STANDBY, code); 554 if ("entered-in-error".equals(codeString)) 555 return new Enumeration<DeviceMetricOperationalStatus>(this, DeviceMetricOperationalStatus.ENTEREDINERROR, code); 556 throw new FHIRException("Unknown DeviceMetricOperationalStatus code '"+codeString+"'"); 557 } 558 public String toCode(DeviceMetricOperationalStatus code) { 559 if (code == DeviceMetricOperationalStatus.NULL) 560 return null; 561 if (code == DeviceMetricOperationalStatus.ON) 562 return "on"; 563 if (code == DeviceMetricOperationalStatus.OFF) 564 return "off"; 565 if (code == DeviceMetricOperationalStatus.STANDBY) 566 return "standby"; 567 if (code == DeviceMetricOperationalStatus.ENTEREDINERROR) 568 return "entered-in-error"; 569 return "?"; 570 } 571 public String toSystem(DeviceMetricOperationalStatus code) { 572 return code.getSystem(); 573 } 574 } 575 576 @Block() 577 public static class DeviceMetricCalibrationComponent extends BackboneElement implements IBaseBackboneElement { 578 /** 579 * Describes the type of the calibration method. 580 */ 581 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 582 @Description(shortDefinition="unspecified | offset | gain | two-point", formalDefinition="Describes the type of the calibration method." ) 583 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-calibration-type") 584 protected Enumeration<DeviceMetricCalibrationType> type; 585 586 /** 587 * Describes the state of the calibration. 588 */ 589 @Child(name = "state", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 590 @Description(shortDefinition="not-calibrated | calibration-required | calibrated | unspecified", formalDefinition="Describes the state of the calibration." ) 591 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-calibration-state") 592 protected Enumeration<DeviceMetricCalibrationState> state; 593 594 /** 595 * Describes the time last calibration has been performed. 596 */ 597 @Child(name = "time", type = {InstantType.class}, order=3, min=0, max=1, modifier=false, summary=false) 598 @Description(shortDefinition="Describes the time last calibration has been performed", formalDefinition="Describes the time last calibration has been performed." ) 599 protected InstantType time; 600 601 private static final long serialVersionUID = 1163986578L; 602 603 /** 604 * Constructor 605 */ 606 public DeviceMetricCalibrationComponent() { 607 super(); 608 } 609 610 /** 611 * @return {@link #type} (Describes the type of the calibration method.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 612 */ 613 public Enumeration<DeviceMetricCalibrationType> getTypeElement() { 614 if (this.type == null) 615 if (Configuration.errorOnAutoCreate()) 616 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.type"); 617 else if (Configuration.doAutoCreate()) 618 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); // bb 619 return this.type; 620 } 621 622 public boolean hasTypeElement() { 623 return this.type != null && !this.type.isEmpty(); 624 } 625 626 public boolean hasType() { 627 return this.type != null && !this.type.isEmpty(); 628 } 629 630 /** 631 * @param value {@link #type} (Describes the type of the calibration method.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 632 */ 633 public DeviceMetricCalibrationComponent setTypeElement(Enumeration<DeviceMetricCalibrationType> value) { 634 this.type = value; 635 return this; 636 } 637 638 /** 639 * @return Describes the type of the calibration method. 640 */ 641 public DeviceMetricCalibrationType getType() { 642 return this.type == null ? null : this.type.getValue(); 643 } 644 645 /** 646 * @param value Describes the type of the calibration method. 647 */ 648 public DeviceMetricCalibrationComponent setType(DeviceMetricCalibrationType value) { 649 if (value == null) 650 this.type = null; 651 else { 652 if (this.type == null) 653 this.type = new Enumeration<DeviceMetricCalibrationType>(new DeviceMetricCalibrationTypeEnumFactory()); 654 this.type.setValue(value); 655 } 656 return this; 657 } 658 659 /** 660 * @return {@link #state} (Describes the state of the calibration.). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 661 */ 662 public Enumeration<DeviceMetricCalibrationState> getStateElement() { 663 if (this.state == null) 664 if (Configuration.errorOnAutoCreate()) 665 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.state"); 666 else if (Configuration.doAutoCreate()) 667 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); // bb 668 return this.state; 669 } 670 671 public boolean hasStateElement() { 672 return this.state != null && !this.state.isEmpty(); 673 } 674 675 public boolean hasState() { 676 return this.state != null && !this.state.isEmpty(); 677 } 678 679 /** 680 * @param value {@link #state} (Describes the state of the calibration.). This is the underlying object with id, value and extensions. The accessor "getState" gives direct access to the value 681 */ 682 public DeviceMetricCalibrationComponent setStateElement(Enumeration<DeviceMetricCalibrationState> value) { 683 this.state = value; 684 return this; 685 } 686 687 /** 688 * @return Describes the state of the calibration. 689 */ 690 public DeviceMetricCalibrationState getState() { 691 return this.state == null ? null : this.state.getValue(); 692 } 693 694 /** 695 * @param value Describes the state of the calibration. 696 */ 697 public DeviceMetricCalibrationComponent setState(DeviceMetricCalibrationState value) { 698 if (value == null) 699 this.state = null; 700 else { 701 if (this.state == null) 702 this.state = new Enumeration<DeviceMetricCalibrationState>(new DeviceMetricCalibrationStateEnumFactory()); 703 this.state.setValue(value); 704 } 705 return this; 706 } 707 708 /** 709 * @return {@link #time} (Describes the time last calibration has been performed.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 710 */ 711 public InstantType getTimeElement() { 712 if (this.time == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create DeviceMetricCalibrationComponent.time"); 715 else if (Configuration.doAutoCreate()) 716 this.time = new InstantType(); // bb 717 return this.time; 718 } 719 720 public boolean hasTimeElement() { 721 return this.time != null && !this.time.isEmpty(); 722 } 723 724 public boolean hasTime() { 725 return this.time != null && !this.time.isEmpty(); 726 } 727 728 /** 729 * @param value {@link #time} (Describes the time last calibration has been performed.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 730 */ 731 public DeviceMetricCalibrationComponent setTimeElement(InstantType value) { 732 this.time = value; 733 return this; 734 } 735 736 /** 737 * @return Describes the time last calibration has been performed. 738 */ 739 public Date getTime() { 740 return this.time == null ? null : this.time.getValue(); 741 } 742 743 /** 744 * @param value Describes the time last calibration has been performed. 745 */ 746 public DeviceMetricCalibrationComponent setTime(Date value) { 747 if (value == null) 748 this.time = null; 749 else { 750 if (this.time == null) 751 this.time = new InstantType(); 752 this.time.setValue(value); 753 } 754 return this; 755 } 756 757 protected void listChildren(List<Property> children) { 758 super.listChildren(children); 759 children.add(new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type)); 760 children.add(new Property("state", "code", "Describes the state of the calibration.", 0, 1, state)); 761 children.add(new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time)); 762 } 763 764 @Override 765 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 766 switch (_hash) { 767 case 3575610: /*type*/ return new Property("type", "code", "Describes the type of the calibration method.", 0, 1, type); 768 case 109757585: /*state*/ return new Property("state", "code", "Describes the state of the calibration.", 0, 1, state); 769 case 3560141: /*time*/ return new Property("time", "instant", "Describes the time last calibration has been performed.", 0, 1, time); 770 default: return super.getNamedProperty(_hash, _name, _checkValid); 771 } 772 773 } 774 775 @Override 776 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 777 switch (hash) { 778 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DeviceMetricCalibrationType> 779 case 109757585: /*state*/ return this.state == null ? new Base[0] : new Base[] {this.state}; // Enumeration<DeviceMetricCalibrationState> 780 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // InstantType 781 default: return super.getProperty(hash, name, checkValid); 782 } 783 784 } 785 786 @Override 787 public Base setProperty(int hash, String name, Base value) throws FHIRException { 788 switch (hash) { 789 case 3575610: // type 790 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 791 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 792 return value; 793 case 109757585: // state 794 value = new DeviceMetricCalibrationStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 795 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 796 return value; 797 case 3560141: // time 798 this.time = TypeConvertor.castToInstant(value); // InstantType 799 return value; 800 default: return super.setProperty(hash, name, value); 801 } 802 803 } 804 805 @Override 806 public Base setProperty(String name, Base value) throws FHIRException { 807 if (name.equals("type")) { 808 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 809 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 810 } else if (name.equals("state")) { 811 value = new DeviceMetricCalibrationStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 812 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 813 } else if (name.equals("time")) { 814 this.time = TypeConvertor.castToInstant(value); // InstantType 815 } else 816 return super.setProperty(name, value); 817 return value; 818 } 819 820 @Override 821 public void removeChild(String name, Base value) throws FHIRException { 822 if (name.equals("type")) { 823 value = new DeviceMetricCalibrationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 824 this.type = (Enumeration) value; // Enumeration<DeviceMetricCalibrationType> 825 } else if (name.equals("state")) { 826 value = new DeviceMetricCalibrationStateEnumFactory().fromType(TypeConvertor.castToCode(value)); 827 this.state = (Enumeration) value; // Enumeration<DeviceMetricCalibrationState> 828 } else if (name.equals("time")) { 829 this.time = null; 830 } else 831 super.removeChild(name, value); 832 833 } 834 835 @Override 836 public Base makeProperty(int hash, String name) throws FHIRException { 837 switch (hash) { 838 case 3575610: return getTypeElement(); 839 case 109757585: return getStateElement(); 840 case 3560141: return getTimeElement(); 841 default: return super.makeProperty(hash, name); 842 } 843 844 } 845 846 @Override 847 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 848 switch (hash) { 849 case 3575610: /*type*/ return new String[] {"code"}; 850 case 109757585: /*state*/ return new String[] {"code"}; 851 case 3560141: /*time*/ return new String[] {"instant"}; 852 default: return super.getTypesForProperty(hash, name); 853 } 854 855 } 856 857 @Override 858 public Base addChild(String name) throws FHIRException { 859 if (name.equals("type")) { 860 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.calibration.type"); 861 } 862 else if (name.equals("state")) { 863 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.calibration.state"); 864 } 865 else if (name.equals("time")) { 866 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.calibration.time"); 867 } 868 else 869 return super.addChild(name); 870 } 871 872 public DeviceMetricCalibrationComponent copy() { 873 DeviceMetricCalibrationComponent dst = new DeviceMetricCalibrationComponent(); 874 copyValues(dst); 875 return dst; 876 } 877 878 public void copyValues(DeviceMetricCalibrationComponent dst) { 879 super.copyValues(dst); 880 dst.type = type == null ? null : type.copy(); 881 dst.state = state == null ? null : state.copy(); 882 dst.time = time == null ? null : time.copy(); 883 } 884 885 @Override 886 public boolean equalsDeep(Base other_) { 887 if (!super.equalsDeep(other_)) 888 return false; 889 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 890 return false; 891 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 892 return compareDeep(type, o.type, true) && compareDeep(state, o.state, true) && compareDeep(time, o.time, true) 893 ; 894 } 895 896 @Override 897 public boolean equalsShallow(Base other_) { 898 if (!super.equalsShallow(other_)) 899 return false; 900 if (!(other_ instanceof DeviceMetricCalibrationComponent)) 901 return false; 902 DeviceMetricCalibrationComponent o = (DeviceMetricCalibrationComponent) other_; 903 return compareValues(type, o.type, true) && compareValues(state, o.state, true) && compareValues(time, o.time, true) 904 ; 905 } 906 907 public boolean isEmpty() { 908 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, state, time); 909 } 910 911 public String fhirType() { 912 return "DeviceMetric.calibration"; 913 914 } 915 916 } 917 918 /** 919 * Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID. 920 */ 921 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 922 @Description(shortDefinition="Instance identifier", formalDefinition="Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID." ) 923 protected List<Identifier> identifier; 924 925 /** 926 * Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc. 927 */ 928 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 929 @Description(shortDefinition="Identity of metric, for example Heart Rate or PEEP Setting", formalDefinition="Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc." ) 930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/devicemetric-type") 931 protected CodeableConcept type; 932 933 /** 934 * Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc. 935 */ 936 @Child(name = "unit", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 937 @Description(shortDefinition="Unit of Measure for the Metric", formalDefinition="Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc." ) 938 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ucum-units") 939 protected CodeableConcept unit; 940 941 /** 942 * Describes the link to the Device. This is also known as a channel device. 943 */ 944 @Child(name = "device", type = {Device.class}, order=3, min=1, max=1, modifier=false, summary=true) 945 @Description(shortDefinition="Describes the link to the Device", formalDefinition="Describes the link to the Device. This is also known as a channel device." ) 946 protected Reference device; 947 948 /** 949 * Indicates current operational state of the device. For example: On, Off, Standby, etc. 950 */ 951 @Child(name = "operationalStatus", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 952 @Description(shortDefinition="on | off | standby | entered-in-error", formalDefinition="Indicates current operational state of the device. For example: On, Off, Standby, etc." ) 953 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-operational-status") 954 protected Enumeration<DeviceMetricOperationalStatus> operationalStatus; 955 956 /** 957 * The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta. 958 */ 959 @Child(name = "color", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 960 @Description(shortDefinition="Color name (from CSS4) or #RRGGBB code", formalDefinition="The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta." ) 961 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/color-codes") 962 protected CodeType color; 963 964 /** 965 * Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 966 */ 967 @Child(name = "category", type = {CodeType.class}, order=6, min=1, max=1, modifier=false, summary=true) 968 @Description(shortDefinition="measurement | setting | calculation | unspecified", formalDefinition="Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation." ) 969 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/metric-category") 970 protected Enumeration<DeviceMetricCategory> category; 971 972 /** 973 * The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured. 974 */ 975 @Child(name = "measurementFrequency", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 976 @Description(shortDefinition="Indicates how often the metric is taken or recorded", formalDefinition="The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured." ) 977 protected Quantity measurementFrequency; 978 979 /** 980 * Describes the calibrations that have been performed or that are required to be performed. 981 */ 982 @Child(name = "calibration", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 983 @Description(shortDefinition="Describes the calibrations that have been performed or that are required to be performed", formalDefinition="Describes the calibrations that have been performed or that are required to be performed." ) 984 protected List<DeviceMetricCalibrationComponent> calibration; 985 986 private static final long serialVersionUID = 2109169775L; 987 988 /** 989 * Constructor 990 */ 991 public DeviceMetric() { 992 super(); 993 } 994 995 /** 996 * Constructor 997 */ 998 public DeviceMetric(CodeableConcept type, Reference device, DeviceMetricCategory category) { 999 super(); 1000 this.setType(type); 1001 this.setDevice(device); 1002 this.setCategory(category); 1003 } 1004 1005 /** 1006 * @return {@link #identifier} (Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID.) 1007 */ 1008 public List<Identifier> getIdentifier() { 1009 if (this.identifier == null) 1010 this.identifier = new ArrayList<Identifier>(); 1011 return this.identifier; 1012 } 1013 1014 /** 1015 * @return Returns a reference to <code>this</code> for easy method chaining 1016 */ 1017 public DeviceMetric setIdentifier(List<Identifier> theIdentifier) { 1018 this.identifier = theIdentifier; 1019 return this; 1020 } 1021 1022 public boolean hasIdentifier() { 1023 if (this.identifier == null) 1024 return false; 1025 for (Identifier item : this.identifier) 1026 if (!item.isEmpty()) 1027 return true; 1028 return false; 1029 } 1030 1031 public Identifier addIdentifier() { //3 1032 Identifier t = new Identifier(); 1033 if (this.identifier == null) 1034 this.identifier = new ArrayList<Identifier>(); 1035 this.identifier.add(t); 1036 return t; 1037 } 1038 1039 public DeviceMetric addIdentifier(Identifier t) { //3 1040 if (t == null) 1041 return this; 1042 if (this.identifier == null) 1043 this.identifier = new ArrayList<Identifier>(); 1044 this.identifier.add(t); 1045 return this; 1046 } 1047 1048 /** 1049 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1050 */ 1051 public Identifier getIdentifierFirstRep() { 1052 if (getIdentifier().isEmpty()) { 1053 addIdentifier(); 1054 } 1055 return getIdentifier().get(0); 1056 } 1057 1058 /** 1059 * @return {@link #type} (Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.) 1060 */ 1061 public CodeableConcept getType() { 1062 if (this.type == null) 1063 if (Configuration.errorOnAutoCreate()) 1064 throw new Error("Attempt to auto-create DeviceMetric.type"); 1065 else if (Configuration.doAutoCreate()) 1066 this.type = new CodeableConcept(); // cc 1067 return this.type; 1068 } 1069 1070 public boolean hasType() { 1071 return this.type != null && !this.type.isEmpty(); 1072 } 1073 1074 /** 1075 * @param value {@link #type} (Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.) 1076 */ 1077 public DeviceMetric setType(CodeableConcept value) { 1078 this.type = value; 1079 return this; 1080 } 1081 1082 /** 1083 * @return {@link #unit} (Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.) 1084 */ 1085 public CodeableConcept getUnit() { 1086 if (this.unit == null) 1087 if (Configuration.errorOnAutoCreate()) 1088 throw new Error("Attempt to auto-create DeviceMetric.unit"); 1089 else if (Configuration.doAutoCreate()) 1090 this.unit = new CodeableConcept(); // cc 1091 return this.unit; 1092 } 1093 1094 public boolean hasUnit() { 1095 return this.unit != null && !this.unit.isEmpty(); 1096 } 1097 1098 /** 1099 * @param value {@link #unit} (Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.) 1100 */ 1101 public DeviceMetric setUnit(CodeableConcept value) { 1102 this.unit = value; 1103 return this; 1104 } 1105 1106 /** 1107 * @return {@link #device} (Describes the link to the Device. This is also known as a channel device.) 1108 */ 1109 public Reference getDevice() { 1110 if (this.device == null) 1111 if (Configuration.errorOnAutoCreate()) 1112 throw new Error("Attempt to auto-create DeviceMetric.device"); 1113 else if (Configuration.doAutoCreate()) 1114 this.device = new Reference(); // cc 1115 return this.device; 1116 } 1117 1118 public boolean hasDevice() { 1119 return this.device != null && !this.device.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #device} (Describes the link to the Device. This is also known as a channel device.) 1124 */ 1125 public DeviceMetric setDevice(Reference value) { 1126 this.device = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #operationalStatus} (Indicates current operational state of the device. For example: On, Off, Standby, etc.). This is the underlying object with id, value and extensions. The accessor "getOperationalStatus" gives direct access to the value 1132 */ 1133 public Enumeration<DeviceMetricOperationalStatus> getOperationalStatusElement() { 1134 if (this.operationalStatus == null) 1135 if (Configuration.errorOnAutoCreate()) 1136 throw new Error("Attempt to auto-create DeviceMetric.operationalStatus"); 1137 else if (Configuration.doAutoCreate()) 1138 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>(new DeviceMetricOperationalStatusEnumFactory()); // bb 1139 return this.operationalStatus; 1140 } 1141 1142 public boolean hasOperationalStatusElement() { 1143 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1144 } 1145 1146 public boolean hasOperationalStatus() { 1147 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1148 } 1149 1150 /** 1151 * @param value {@link #operationalStatus} (Indicates current operational state of the device. For example: On, Off, Standby, etc.). This is the underlying object with id, value and extensions. The accessor "getOperationalStatus" gives direct access to the value 1152 */ 1153 public DeviceMetric setOperationalStatusElement(Enumeration<DeviceMetricOperationalStatus> value) { 1154 this.operationalStatus = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return Indicates current operational state of the device. For example: On, Off, Standby, etc. 1160 */ 1161 public DeviceMetricOperationalStatus getOperationalStatus() { 1162 return this.operationalStatus == null ? null : this.operationalStatus.getValue(); 1163 } 1164 1165 /** 1166 * @param value Indicates current operational state of the device. For example: On, Off, Standby, etc. 1167 */ 1168 public DeviceMetric setOperationalStatus(DeviceMetricOperationalStatus value) { 1169 if (value == null) 1170 this.operationalStatus = null; 1171 else { 1172 if (this.operationalStatus == null) 1173 this.operationalStatus = new Enumeration<DeviceMetricOperationalStatus>(new DeviceMetricOperationalStatusEnumFactory()); 1174 this.operationalStatus.setValue(value); 1175 } 1176 return this; 1177 } 1178 1179 /** 1180 * @return {@link #color} (The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1181 */ 1182 public CodeType getColorElement() { 1183 if (this.color == null) 1184 if (Configuration.errorOnAutoCreate()) 1185 throw new Error("Attempt to auto-create DeviceMetric.color"); 1186 else if (Configuration.doAutoCreate()) 1187 this.color = new CodeType(); // bb 1188 return this.color; 1189 } 1190 1191 public boolean hasColorElement() { 1192 return this.color != null && !this.color.isEmpty(); 1193 } 1194 1195 public boolean hasColor() { 1196 return this.color != null && !this.color.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #color} (The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1201 */ 1202 public DeviceMetric setColorElement(CodeType value) { 1203 this.color = value; 1204 return this; 1205 } 1206 1207 /** 1208 * @return The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta. 1209 */ 1210 public String getColor() { 1211 return this.color == null ? null : this.color.getValue(); 1212 } 1213 1214 /** 1215 * @param value The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta. 1216 */ 1217 public DeviceMetric setColor(String value) { 1218 if (Utilities.noString(value)) 1219 this.color = null; 1220 else { 1221 if (this.color == null) 1222 this.color = new CodeType(); 1223 this.color.setValue(value); 1224 } 1225 return this; 1226 } 1227 1228 /** 1229 * @return {@link #category} (Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1230 */ 1231 public Enumeration<DeviceMetricCategory> getCategoryElement() { 1232 if (this.category == null) 1233 if (Configuration.errorOnAutoCreate()) 1234 throw new Error("Attempt to auto-create DeviceMetric.category"); 1235 else if (Configuration.doAutoCreate()) 1236 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); // bb 1237 return this.category; 1238 } 1239 1240 public boolean hasCategoryElement() { 1241 return this.category != null && !this.category.isEmpty(); 1242 } 1243 1244 public boolean hasCategory() { 1245 return this.category != null && !this.category.isEmpty(); 1246 } 1247 1248 /** 1249 * @param value {@link #category} (Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1250 */ 1251 public DeviceMetric setCategoryElement(Enumeration<DeviceMetricCategory> value) { 1252 this.category = value; 1253 return this; 1254 } 1255 1256 /** 1257 * @return Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 1258 */ 1259 public DeviceMetricCategory getCategory() { 1260 return this.category == null ? null : this.category.getValue(); 1261 } 1262 1263 /** 1264 * @param value Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation. 1265 */ 1266 public DeviceMetric setCategory(DeviceMetricCategory value) { 1267 if (this.category == null) 1268 this.category = new Enumeration<DeviceMetricCategory>(new DeviceMetricCategoryEnumFactory()); 1269 this.category.setValue(value); 1270 return this; 1271 } 1272 1273 /** 1274 * @return {@link #measurementFrequency} (The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.) 1275 */ 1276 public Quantity getMeasurementFrequency() { 1277 if (this.measurementFrequency == null) 1278 if (Configuration.errorOnAutoCreate()) 1279 throw new Error("Attempt to auto-create DeviceMetric.measurementFrequency"); 1280 else if (Configuration.doAutoCreate()) 1281 this.measurementFrequency = new Quantity(); // cc 1282 return this.measurementFrequency; 1283 } 1284 1285 public boolean hasMeasurementFrequency() { 1286 return this.measurementFrequency != null && !this.measurementFrequency.isEmpty(); 1287 } 1288 1289 /** 1290 * @param value {@link #measurementFrequency} (The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.) 1291 */ 1292 public DeviceMetric setMeasurementFrequency(Quantity value) { 1293 this.measurementFrequency = value; 1294 return this; 1295 } 1296 1297 /** 1298 * @return {@link #calibration} (Describes the calibrations that have been performed or that are required to be performed.) 1299 */ 1300 public List<DeviceMetricCalibrationComponent> getCalibration() { 1301 if (this.calibration == null) 1302 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1303 return this.calibration; 1304 } 1305 1306 /** 1307 * @return Returns a reference to <code>this</code> for easy method chaining 1308 */ 1309 public DeviceMetric setCalibration(List<DeviceMetricCalibrationComponent> theCalibration) { 1310 this.calibration = theCalibration; 1311 return this; 1312 } 1313 1314 public boolean hasCalibration() { 1315 if (this.calibration == null) 1316 return false; 1317 for (DeviceMetricCalibrationComponent item : this.calibration) 1318 if (!item.isEmpty()) 1319 return true; 1320 return false; 1321 } 1322 1323 public DeviceMetricCalibrationComponent addCalibration() { //3 1324 DeviceMetricCalibrationComponent t = new DeviceMetricCalibrationComponent(); 1325 if (this.calibration == null) 1326 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1327 this.calibration.add(t); 1328 return t; 1329 } 1330 1331 public DeviceMetric addCalibration(DeviceMetricCalibrationComponent t) { //3 1332 if (t == null) 1333 return this; 1334 if (this.calibration == null) 1335 this.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1336 this.calibration.add(t); 1337 return this; 1338 } 1339 1340 /** 1341 * @return The first repetition of repeating field {@link #calibration}, creating it if it does not already exist {3} 1342 */ 1343 public DeviceMetricCalibrationComponent getCalibrationFirstRep() { 1344 if (getCalibration().isEmpty()) { 1345 addCalibration(); 1346 } 1347 return getCalibration().get(0); 1348 } 1349 1350 protected void listChildren(List<Property> children) { 1351 super.listChildren(children); 1352 children.add(new Property("identifier", "Identifier", "Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1353 children.add(new Property("type", "CodeableConcept", "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type)); 1354 children.add(new Property("unit", "CodeableConcept", "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 0, 1, unit)); 1355 children.add(new Property("device", "Reference(Device)", "Describes the link to the Device. This is also known as a channel device.", 0, 1, device)); 1356 children.add(new Property("operationalStatus", "code", "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, operationalStatus)); 1357 children.add(new Property("color", "code", "The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.", 0, 1, color)); 1358 children.add(new Property("category", "code", "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 0, 1, category)); 1359 children.add(new Property("measurementFrequency", "Quantity", "The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.", 0, 1, measurementFrequency)); 1360 children.add(new Property("calibration", "", "Describes the calibrations that have been performed or that are required to be performed.", 0, java.lang.Integer.MAX_VALUE, calibration)); 1361 } 1362 1363 @Override 1364 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1365 switch (_hash) { 1366 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Instance identifiers assigned to a device, by the device or gateway software, manufacturers, other organizations or owners. For example, handle ID.", 0, java.lang.Integer.MAX_VALUE, identifier); 1367 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Describes the type of the metric. For example: Heart Rate, PEEP Setting, etc.", 0, 1, type); 1368 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Describes the unit that an observed value determined for this metric will have. For example: Percent, Seconds, etc.", 0, 1, unit); 1369 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "Describes the link to the Device. This is also known as a channel device.", 0, 1, device); 1370 case -2103166364: /*operationalStatus*/ return new Property("operationalStatus", "code", "Indicates current operational state of the device. For example: On, Off, Standby, etc.", 0, 1, operationalStatus); 1371 case 94842723: /*color*/ return new Property("color", "code", "The preferred color associated with the metric (e.g., display color). This is often used to aid clinicians to track and identify parameter types by color. In practice, consider a Patient Monitor that has ECG/HR and Pleth; the metrics are displayed in different characteristic colors, such as HR in blue, BP in green, and PR and SpO2 in magenta.", 0, 1, color); 1372 case 50511102: /*category*/ return new Property("category", "code", "Indicates the category of the observation generation process. A DeviceMetric can be for example a setting, measurement, or calculation.", 0, 1, category); 1373 case 1766341888: /*measurementFrequency*/ return new Property("measurementFrequency", "Quantity", "The frequency at which the metric is taken or recorded. Devices measure metrics at a wide range of frequencies; for example, an ECG might sample measurements in the millisecond range, while an NIBP might trigger only once an hour. Less often, the measurementFrequency may be based on a unit other than time, such as distance (e.g. for a measuring wheel). The update period may be different than the measurement frequency, if the device does not update the published observed value with the same frequency as it was measured.", 0, 1, measurementFrequency); 1374 case 1421318634: /*calibration*/ return new Property("calibration", "", "Describes the calibrations that have been performed or that are required to be performed.", 0, java.lang.Integer.MAX_VALUE, calibration); 1375 default: return super.getNamedProperty(_hash, _name, _checkValid); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1382 switch (hash) { 1383 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1384 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1385 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 1386 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 1387 case -2103166364: /*operationalStatus*/ return this.operationalStatus == null ? new Base[0] : new Base[] {this.operationalStatus}; // Enumeration<DeviceMetricOperationalStatus> 1388 case 94842723: /*color*/ return this.color == null ? new Base[0] : new Base[] {this.color}; // CodeType 1389 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<DeviceMetricCategory> 1390 case 1766341888: /*measurementFrequency*/ return this.measurementFrequency == null ? new Base[0] : new Base[] {this.measurementFrequency}; // Quantity 1391 case 1421318634: /*calibration*/ return this.calibration == null ? new Base[0] : this.calibration.toArray(new Base[this.calibration.size()]); // DeviceMetricCalibrationComponent 1392 default: return super.getProperty(hash, name, checkValid); 1393 } 1394 1395 } 1396 1397 @Override 1398 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1399 switch (hash) { 1400 case -1618432855: // identifier 1401 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1402 return value; 1403 case 3575610: // type 1404 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1405 return value; 1406 case 3594628: // unit 1407 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1408 return value; 1409 case -1335157162: // device 1410 this.device = TypeConvertor.castToReference(value); // Reference 1411 return value; 1412 case -2103166364: // operationalStatus 1413 value = new DeviceMetricOperationalStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1414 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1415 return value; 1416 case 94842723: // color 1417 this.color = TypeConvertor.castToCode(value); // CodeType 1418 return value; 1419 case 50511102: // category 1420 value = new DeviceMetricCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1421 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1422 return value; 1423 case 1766341888: // measurementFrequency 1424 this.measurementFrequency = TypeConvertor.castToQuantity(value); // Quantity 1425 return value; 1426 case 1421318634: // calibration 1427 this.getCalibration().add((DeviceMetricCalibrationComponent) value); // DeviceMetricCalibrationComponent 1428 return value; 1429 default: return super.setProperty(hash, name, value); 1430 } 1431 1432 } 1433 1434 @Override 1435 public Base setProperty(String name, Base value) throws FHIRException { 1436 if (name.equals("identifier")) { 1437 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1438 } else if (name.equals("type")) { 1439 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1440 } else if (name.equals("unit")) { 1441 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1442 } else if (name.equals("device")) { 1443 this.device = TypeConvertor.castToReference(value); // Reference 1444 } else if (name.equals("operationalStatus")) { 1445 value = new DeviceMetricOperationalStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1446 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1447 } else if (name.equals("color")) { 1448 this.color = TypeConvertor.castToCode(value); // CodeType 1449 } else if (name.equals("category")) { 1450 value = new DeviceMetricCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1451 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1452 } else if (name.equals("measurementFrequency")) { 1453 this.measurementFrequency = TypeConvertor.castToQuantity(value); // Quantity 1454 } else if (name.equals("calibration")) { 1455 this.getCalibration().add((DeviceMetricCalibrationComponent) value); 1456 } else 1457 return super.setProperty(name, value); 1458 return value; 1459 } 1460 1461 @Override 1462 public void removeChild(String name, Base value) throws FHIRException { 1463 if (name.equals("identifier")) { 1464 this.getIdentifier().remove(value); 1465 } else if (name.equals("type")) { 1466 this.type = null; 1467 } else if (name.equals("unit")) { 1468 this.unit = null; 1469 } else if (name.equals("device")) { 1470 this.device = null; 1471 } else if (name.equals("operationalStatus")) { 1472 value = new DeviceMetricOperationalStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1473 this.operationalStatus = (Enumeration) value; // Enumeration<DeviceMetricOperationalStatus> 1474 } else if (name.equals("color")) { 1475 this.color = null; 1476 } else if (name.equals("category")) { 1477 value = new DeviceMetricCategoryEnumFactory().fromType(TypeConvertor.castToCode(value)); 1478 this.category = (Enumeration) value; // Enumeration<DeviceMetricCategory> 1479 } else if (name.equals("measurementFrequency")) { 1480 this.measurementFrequency = null; 1481 } else if (name.equals("calibration")) { 1482 this.getCalibration().remove((DeviceMetricCalibrationComponent) value); 1483 } else 1484 super.removeChild(name, value); 1485 1486 } 1487 1488 @Override 1489 public Base makeProperty(int hash, String name) throws FHIRException { 1490 switch (hash) { 1491 case -1618432855: return addIdentifier(); 1492 case 3575610: return getType(); 1493 case 3594628: return getUnit(); 1494 case -1335157162: return getDevice(); 1495 case -2103166364: return getOperationalStatusElement(); 1496 case 94842723: return getColorElement(); 1497 case 50511102: return getCategoryElement(); 1498 case 1766341888: return getMeasurementFrequency(); 1499 case 1421318634: return addCalibration(); 1500 default: return super.makeProperty(hash, name); 1501 } 1502 1503 } 1504 1505 @Override 1506 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1507 switch (hash) { 1508 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1509 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1510 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 1511 case -1335157162: /*device*/ return new String[] {"Reference"}; 1512 case -2103166364: /*operationalStatus*/ return new String[] {"code"}; 1513 case 94842723: /*color*/ return new String[] {"code"}; 1514 case 50511102: /*category*/ return new String[] {"code"}; 1515 case 1766341888: /*measurementFrequency*/ return new String[] {"Quantity"}; 1516 case 1421318634: /*calibration*/ return new String[] {}; 1517 default: return super.getTypesForProperty(hash, name); 1518 } 1519 1520 } 1521 1522 @Override 1523 public Base addChild(String name) throws FHIRException { 1524 if (name.equals("identifier")) { 1525 return addIdentifier(); 1526 } 1527 else if (name.equals("type")) { 1528 this.type = new CodeableConcept(); 1529 return this.type; 1530 } 1531 else if (name.equals("unit")) { 1532 this.unit = new CodeableConcept(); 1533 return this.unit; 1534 } 1535 else if (name.equals("device")) { 1536 this.device = new Reference(); 1537 return this.device; 1538 } 1539 else if (name.equals("operationalStatus")) { 1540 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.operationalStatus"); 1541 } 1542 else if (name.equals("color")) { 1543 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.color"); 1544 } 1545 else if (name.equals("category")) { 1546 throw new FHIRException("Cannot call addChild on a singleton property DeviceMetric.category"); 1547 } 1548 else if (name.equals("measurementFrequency")) { 1549 this.measurementFrequency = new Quantity(); 1550 return this.measurementFrequency; 1551 } 1552 else if (name.equals("calibration")) { 1553 return addCalibration(); 1554 } 1555 else 1556 return super.addChild(name); 1557 } 1558 1559 public String fhirType() { 1560 return "DeviceMetric"; 1561 1562 } 1563 1564 public DeviceMetric copy() { 1565 DeviceMetric dst = new DeviceMetric(); 1566 copyValues(dst); 1567 return dst; 1568 } 1569 1570 public void copyValues(DeviceMetric dst) { 1571 super.copyValues(dst); 1572 if (identifier != null) { 1573 dst.identifier = new ArrayList<Identifier>(); 1574 for (Identifier i : identifier) 1575 dst.identifier.add(i.copy()); 1576 }; 1577 dst.type = type == null ? null : type.copy(); 1578 dst.unit = unit == null ? null : unit.copy(); 1579 dst.device = device == null ? null : device.copy(); 1580 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 1581 dst.color = color == null ? null : color.copy(); 1582 dst.category = category == null ? null : category.copy(); 1583 dst.measurementFrequency = measurementFrequency == null ? null : measurementFrequency.copy(); 1584 if (calibration != null) { 1585 dst.calibration = new ArrayList<DeviceMetricCalibrationComponent>(); 1586 for (DeviceMetricCalibrationComponent i : calibration) 1587 dst.calibration.add(i.copy()); 1588 }; 1589 } 1590 1591 protected DeviceMetric typedCopy() { 1592 return copy(); 1593 } 1594 1595 @Override 1596 public boolean equalsDeep(Base other_) { 1597 if (!super.equalsDeep(other_)) 1598 return false; 1599 if (!(other_ instanceof DeviceMetric)) 1600 return false; 1601 DeviceMetric o = (DeviceMetric) other_; 1602 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(unit, o.unit, true) 1603 && compareDeep(device, o.device, true) && compareDeep(operationalStatus, o.operationalStatus, true) 1604 && compareDeep(color, o.color, true) && compareDeep(category, o.category, true) && compareDeep(measurementFrequency, o.measurementFrequency, true) 1605 && compareDeep(calibration, o.calibration, true); 1606 } 1607 1608 @Override 1609 public boolean equalsShallow(Base other_) { 1610 if (!super.equalsShallow(other_)) 1611 return false; 1612 if (!(other_ instanceof DeviceMetric)) 1613 return false; 1614 DeviceMetric o = (DeviceMetric) other_; 1615 return compareValues(operationalStatus, o.operationalStatus, true) && compareValues(color, o.color, true) 1616 && compareValues(category, o.category, true); 1617 } 1618 1619 public boolean isEmpty() { 1620 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, unit, device 1621 , operationalStatus, color, category, measurementFrequency, calibration); 1622 } 1623 1624 @Override 1625 public ResourceType getResourceType() { 1626 return ResourceType.DeviceMetric; 1627 } 1628 1629 /** 1630 * Search parameter: <b>category</b> 1631 * <p> 1632 * Description: <b>The category of the metric</b><br> 1633 * Type: <b>token</b><br> 1634 * Path: <b>DeviceMetric.category</b><br> 1635 * </p> 1636 */ 1637 @SearchParamDefinition(name="category", path="DeviceMetric.category", description="The category of the metric", type="token" ) 1638 public static final String SP_CATEGORY = "category"; 1639 /** 1640 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1641 * <p> 1642 * Description: <b>The category of the metric</b><br> 1643 * Type: <b>token</b><br> 1644 * Path: <b>DeviceMetric.category</b><br> 1645 * </p> 1646 */ 1647 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1648 1649 /** 1650 * Search parameter: <b>device</b> 1651 * <p> 1652 * Description: <b>The device resource</b><br> 1653 * Type: <b>reference</b><br> 1654 * Path: <b>DeviceMetric.device</b><br> 1655 * </p> 1656 */ 1657 @SearchParamDefinition(name="device", path="DeviceMetric.device", description="The device resource", type="reference", target={Device.class } ) 1658 public static final String SP_DEVICE = "device"; 1659 /** 1660 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1661 * <p> 1662 * Description: <b>The device resource</b><br> 1663 * Type: <b>reference</b><br> 1664 * Path: <b>DeviceMetric.device</b><br> 1665 * </p> 1666 */ 1667 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 1668 1669/** 1670 * Constant for fluent queries to be used to add include statements. Specifies 1671 * the path value of "<b>DeviceMetric:device</b>". 1672 */ 1673 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceMetric:device").toLocked(); 1674 1675 /** 1676 * Search parameter: <b>identifier</b> 1677 * <p> 1678 * Description: <b>The identifier of the metric</b><br> 1679 * Type: <b>token</b><br> 1680 * Path: <b>DeviceMetric.identifier</b><br> 1681 * </p> 1682 */ 1683 @SearchParamDefinition(name="identifier", path="DeviceMetric.identifier", description="The identifier of the metric", type="token" ) 1684 public static final String SP_IDENTIFIER = "identifier"; 1685 /** 1686 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1687 * <p> 1688 * Description: <b>The identifier of the metric</b><br> 1689 * Type: <b>token</b><br> 1690 * Path: <b>DeviceMetric.identifier</b><br> 1691 * </p> 1692 */ 1693 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1694 1695 /** 1696 * Search parameter: <b>type</b> 1697 * <p> 1698 * Description: <b>The type of metric</b><br> 1699 * Type: <b>token</b><br> 1700 * Path: <b>DeviceMetric.type</b><br> 1701 * </p> 1702 */ 1703 @SearchParamDefinition(name="type", path="DeviceMetric.type", description="The type of metric", type="token" ) 1704 public static final String SP_TYPE = "type"; 1705 /** 1706 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1707 * <p> 1708 * Description: <b>The type of metric</b><br> 1709 * Type: <b>token</b><br> 1710 * Path: <b>DeviceMetric.type</b><br> 1711 * </p> 1712 */ 1713 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1714 1715 1716} 1717