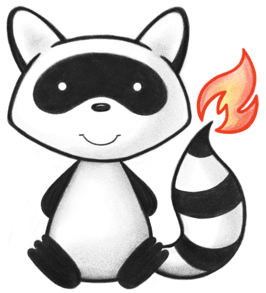
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Represents a request a device to be provided to a specific patient. The device may be an implantable device to be subsequently implanted, or an external assistive device, such as a walker, to be delivered and subsequently be used. 052 */ 053@ResourceDef(name="DeviceRequest", profile="http://hl7.org/fhir/StructureDefinition/DeviceRequest") 054public class DeviceRequest extends DomainResource { 055 056 @Block() 057 public static class DeviceRequestParameterComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * A code or string that identifies the device detail being asserted. 060 */ 061 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Device detail", formalDefinition="A code or string that identifies the device detail being asserted." ) 063 protected CodeableConcept code; 064 065 /** 066 * The value of the device detail. 067 */ 068 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, Range.class, BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="Value of detail", formalDefinition="The value of the device detail." ) 070 protected DataType value; 071 072 private static final long serialVersionUID = -1950789033L; 073 074 /** 075 * Constructor 076 */ 077 public DeviceRequestParameterComponent() { 078 super(); 079 } 080 081 /** 082 * @return {@link #code} (A code or string that identifies the device detail being asserted.) 083 */ 084 public CodeableConcept getCode() { 085 if (this.code == null) 086 if (Configuration.errorOnAutoCreate()) 087 throw new Error("Attempt to auto-create DeviceRequestParameterComponent.code"); 088 else if (Configuration.doAutoCreate()) 089 this.code = new CodeableConcept(); // cc 090 return this.code; 091 } 092 093 public boolean hasCode() { 094 return this.code != null && !this.code.isEmpty(); 095 } 096 097 /** 098 * @param value {@link #code} (A code or string that identifies the device detail being asserted.) 099 */ 100 public DeviceRequestParameterComponent setCode(CodeableConcept value) { 101 this.code = value; 102 return this; 103 } 104 105 /** 106 * @return {@link #value} (The value of the device detail.) 107 */ 108 public DataType getValue() { 109 return this.value; 110 } 111 112 /** 113 * @return {@link #value} (The value of the device detail.) 114 */ 115 public CodeableConcept getValueCodeableConcept() throws FHIRException { 116 if (this.value == null) 117 this.value = new CodeableConcept(); 118 if (!(this.value instanceof CodeableConcept)) 119 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 120 return (CodeableConcept) this.value; 121 } 122 123 public boolean hasValueCodeableConcept() { 124 return this != null && this.value instanceof CodeableConcept; 125 } 126 127 /** 128 * @return {@link #value} (The value of the device detail.) 129 */ 130 public Quantity getValueQuantity() throws FHIRException { 131 if (this.value == null) 132 this.value = new Quantity(); 133 if (!(this.value instanceof Quantity)) 134 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 135 return (Quantity) this.value; 136 } 137 138 public boolean hasValueQuantity() { 139 return this != null && this.value instanceof Quantity; 140 } 141 142 /** 143 * @return {@link #value} (The value of the device detail.) 144 */ 145 public Range getValueRange() throws FHIRException { 146 if (this.value == null) 147 this.value = new Range(); 148 if (!(this.value instanceof Range)) 149 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 150 return (Range) this.value; 151 } 152 153 public boolean hasValueRange() { 154 return this != null && this.value instanceof Range; 155 } 156 157 /** 158 * @return {@link #value} (The value of the device detail.) 159 */ 160 public BooleanType getValueBooleanType() throws FHIRException { 161 if (this.value == null) 162 this.value = new BooleanType(); 163 if (!(this.value instanceof BooleanType)) 164 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 165 return (BooleanType) this.value; 166 } 167 168 public boolean hasValueBooleanType() { 169 return this != null && this.value instanceof BooleanType; 170 } 171 172 public boolean hasValue() { 173 return this.value != null && !this.value.isEmpty(); 174 } 175 176 /** 177 * @param value {@link #value} (The value of the device detail.) 178 */ 179 public DeviceRequestParameterComponent setValue(DataType value) { 180 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range || value instanceof BooleanType)) 181 throw new FHIRException("Not the right type for DeviceRequest.parameter.value[x]: "+value.fhirType()); 182 this.value = value; 183 return this; 184 } 185 186 protected void listChildren(List<Property> children) { 187 super.listChildren(children); 188 children.add(new Property("code", "CodeableConcept", "A code or string that identifies the device detail being asserted.", 0, 1, code)); 189 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 0, 1, value)); 190 } 191 192 @Override 193 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 194 switch (_hash) { 195 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code or string that identifies the device detail being asserted.", 0, 1, code); 196 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 0, 1, value); 197 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 0, 1, value); 198 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the device detail.", 0, 1, value); 199 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the device detail.", 0, 1, value); 200 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the device detail.", 0, 1, value); 201 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the device detail.", 0, 1, value); 202 default: return super.getNamedProperty(_hash, _name, _checkValid); 203 } 204 205 } 206 207 @Override 208 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 209 switch (hash) { 210 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 211 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 212 default: return super.getProperty(hash, name, checkValid); 213 } 214 215 } 216 217 @Override 218 public Base setProperty(int hash, String name, Base value) throws FHIRException { 219 switch (hash) { 220 case 3059181: // code 221 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 222 return value; 223 case 111972721: // value 224 this.value = TypeConvertor.castToType(value); // DataType 225 return value; 226 default: return super.setProperty(hash, name, value); 227 } 228 229 } 230 231 @Override 232 public Base setProperty(String name, Base value) throws FHIRException { 233 if (name.equals("code")) { 234 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 235 } else if (name.equals("value[x]")) { 236 this.value = TypeConvertor.castToType(value); // DataType 237 } else 238 return super.setProperty(name, value); 239 return value; 240 } 241 242 @Override 243 public void removeChild(String name, Base value) throws FHIRException { 244 if (name.equals("code")) { 245 this.code = null; 246 } else if (name.equals("value[x]")) { 247 this.value = null; 248 } else 249 super.removeChild(name, value); 250 251 } 252 253 @Override 254 public Base makeProperty(int hash, String name) throws FHIRException { 255 switch (hash) { 256 case 3059181: return getCode(); 257 case -1410166417: return getValue(); 258 case 111972721: return getValue(); 259 default: return super.makeProperty(hash, name); 260 } 261 262 } 263 264 @Override 265 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 266 switch (hash) { 267 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 268 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "Range", "boolean"}; 269 default: return super.getTypesForProperty(hash, name); 270 } 271 272 } 273 274 @Override 275 public Base addChild(String name) throws FHIRException { 276 if (name.equals("code")) { 277 this.code = new CodeableConcept(); 278 return this.code; 279 } 280 else if (name.equals("valueCodeableConcept")) { 281 this.value = new CodeableConcept(); 282 return this.value; 283 } 284 else if (name.equals("valueQuantity")) { 285 this.value = new Quantity(); 286 return this.value; 287 } 288 else if (name.equals("valueRange")) { 289 this.value = new Range(); 290 return this.value; 291 } 292 else if (name.equals("valueBoolean")) { 293 this.value = new BooleanType(); 294 return this.value; 295 } 296 else 297 return super.addChild(name); 298 } 299 300 public DeviceRequestParameterComponent copy() { 301 DeviceRequestParameterComponent dst = new DeviceRequestParameterComponent(); 302 copyValues(dst); 303 return dst; 304 } 305 306 public void copyValues(DeviceRequestParameterComponent dst) { 307 super.copyValues(dst); 308 dst.code = code == null ? null : code.copy(); 309 dst.value = value == null ? null : value.copy(); 310 } 311 312 @Override 313 public boolean equalsDeep(Base other_) { 314 if (!super.equalsDeep(other_)) 315 return false; 316 if (!(other_ instanceof DeviceRequestParameterComponent)) 317 return false; 318 DeviceRequestParameterComponent o = (DeviceRequestParameterComponent) other_; 319 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 320 } 321 322 @Override 323 public boolean equalsShallow(Base other_) { 324 if (!super.equalsShallow(other_)) 325 return false; 326 if (!(other_ instanceof DeviceRequestParameterComponent)) 327 return false; 328 DeviceRequestParameterComponent o = (DeviceRequestParameterComponent) other_; 329 return true; 330 } 331 332 public boolean isEmpty() { 333 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 334 } 335 336 public String fhirType() { 337 return "DeviceRequest.parameter"; 338 339 } 340 341 } 342 343 /** 344 * Identifiers assigned to this order by the orderer or by the receiver. 345 */ 346 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 347 @Description(shortDefinition="External Request identifier", formalDefinition="Identifiers assigned to this order by the orderer or by the receiver." ) 348 protected List<Identifier> identifier; 349 350 /** 351 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest. 352 */ 353 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 354 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest." ) 355 protected List<CanonicalType> instantiatesCanonical; 356 357 /** 358 * The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest. 359 */ 360 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 361 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest." ) 362 protected List<UriType> instantiatesUri; 363 364 /** 365 * Plan/proposal/order fulfilled by this request. 366 */ 367 @Child(name = "basedOn", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 368 @Description(shortDefinition="What request fulfills", formalDefinition="Plan/proposal/order fulfilled by this request." ) 369 protected List<Reference> basedOn; 370 371 /** 372 * The request takes the place of the referenced completed or terminated request(s). 373 */ 374 @Child(name = "replaces", type = {DeviceRequest.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 375 @Description(shortDefinition="What request replaces", formalDefinition="The request takes the place of the referenced completed or terminated request(s)." ) 376 protected List<Reference> replaces; 377 378 /** 379 * A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time. 380 */ 381 @Child(name = "groupIdentifier", type = {Identifier.class}, order=5, min=0, max=1, modifier=false, summary=true) 382 @Description(shortDefinition="Identifier of composite request", formalDefinition="A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time." ) 383 protected Identifier groupIdentifier; 384 385 /** 386 * The status of the request. 387 */ 388 @Child(name = "status", type = {CodeType.class}, order=6, min=0, max=1, modifier=true, summary=true) 389 @Description(shortDefinition="draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition="The status of the request." ) 390 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 391 protected Enumeration<RequestStatus> status; 392 393 /** 394 * Whether the request is a proposal, plan, an original order or a reflex order. 395 */ 396 @Child(name = "intent", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 397 @Description(shortDefinition="proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Whether the request is a proposal, plan, an original order or a reflex order." ) 398 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 399 protected Enumeration<RequestIntent> intent; 400 401 /** 402 * Indicates how quickly the request should be addressed with respect to other requests. 403 */ 404 @Child(name = "priority", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 405 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the request should be addressed with respect to other requests." ) 406 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 407 protected Enumeration<RequestPriority> priority; 408 409 /** 410 * If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses. 411 */ 412 @Child(name = "doNotPerform", type = {BooleanType.class}, order=9, min=0, max=1, modifier=true, summary=true) 413 @Description(shortDefinition="True if the request is to stop or not to start using the device", formalDefinition="If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses." ) 414 protected BooleanType doNotPerform; 415 416 /** 417 * The details of the device to be used. 418 */ 419 @Child(name = "code", type = {CodeableReference.class}, order=10, min=1, max=1, modifier=false, summary=true) 420 @Description(shortDefinition="Device requested", formalDefinition="The details of the device to be used." ) 421 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/device-type") 422 protected CodeableReference code; 423 424 /** 425 * The number of devices to be provided. 426 */ 427 @Child(name = "quantity", type = {IntegerType.class}, order=11, min=0, max=1, modifier=false, summary=false) 428 @Description(shortDefinition="Quantity of devices to supply", formalDefinition="The number of devices to be provided." ) 429 protected IntegerType quantity; 430 431 /** 432 * Specific parameters for the ordered item. For example, the prism value for lenses. 433 */ 434 @Child(name = "parameter", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 435 @Description(shortDefinition="Device details", formalDefinition="Specific parameters for the ordered item. For example, the prism value for lenses." ) 436 protected List<DeviceRequestParameterComponent> parameter; 437 438 /** 439 * The patient who will use the device. 440 */ 441 @Child(name = "subject", type = {Patient.class, Group.class, Location.class, Device.class}, order=13, min=1, max=1, modifier=false, summary=true) 442 @Description(shortDefinition="Focus of request", formalDefinition="The patient who will use the device." ) 443 protected Reference subject; 444 445 /** 446 * An encounter that provides additional context in which this request is made. 447 */ 448 @Child(name = "encounter", type = {Encounter.class}, order=14, min=0, max=1, modifier=false, summary=true) 449 @Description(shortDefinition="Encounter motivating request", formalDefinition="An encounter that provides additional context in which this request is made." ) 450 protected Reference encounter; 451 452 /** 453 * The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013". 454 */ 455 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=15, min=0, max=1, modifier=false, summary=true) 456 @Description(shortDefinition="Desired time or schedule for use", formalDefinition="The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\"." ) 457 protected DataType occurrence; 458 459 /** 460 * When the request transitioned to being actionable. 461 */ 462 @Child(name = "authoredOn", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=true) 463 @Description(shortDefinition="When recorded", formalDefinition="When the request transitioned to being actionable." ) 464 protected DateTimeType authoredOn; 465 466 /** 467 * The individual or entity who initiated the request and has responsibility for its activation. 468 */ 469 @Child(name = "requester", type = {Device.class, Practitioner.class, PractitionerRole.class, Organization.class}, order=17, min=0, max=1, modifier=false, summary=true) 470 @Description(shortDefinition="Who/what submitted the device request", formalDefinition="The individual or entity who initiated the request and has responsibility for its activation." ) 471 protected Reference requester; 472 473 /** 474 * The desired individual or entity to provide the device to the subject of the request (e.g., patient, location). 475 */ 476 @Child(name = "performer", type = {CodeableReference.class}, order=18, min=0, max=1, modifier=false, summary=true) 477 @Description(shortDefinition="Requested Filler", formalDefinition="The desired individual or entity to provide the device to the subject of the request (e.g., patient, location)." ) 478 protected CodeableReference performer; 479 480 /** 481 * Reason or justification for the use of this device. 482 */ 483 @Child(name = "reason", type = {CodeableReference.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 484 @Description(shortDefinition="Coded/Linked Reason for request", formalDefinition="Reason or justification for the use of this device." ) 485 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 486 protected List<CodeableReference> reason; 487 488 /** 489 * This status is to indicate whether the request is a PRN or may be given as needed. 490 */ 491 @Child(name = "asNeeded", type = {BooleanType.class}, order=20, min=0, max=1, modifier=false, summary=false) 492 @Description(shortDefinition="PRN status of request", formalDefinition="This status is to indicate whether the request is a PRN or may be given as needed." ) 493 protected BooleanType asNeeded; 494 495 /** 496 * The reason for using the device. 497 */ 498 @Child(name = "asNeededFor", type = {CodeableConcept.class}, order=21, min=0, max=1, modifier=false, summary=false) 499 @Description(shortDefinition="Device usage reason", formalDefinition="The reason for using the device." ) 500 protected CodeableConcept asNeededFor; 501 502 /** 503 * Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service. 504 */ 505 @Child(name = "insurance", type = {Coverage.class, ClaimResponse.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 506 @Description(shortDefinition="Associated insurance coverage", formalDefinition="Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service." ) 507 protected List<Reference> insurance; 508 509 /** 510 * Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site). 511 */ 512 @Child(name = "supportingInfo", type = {Reference.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 513 @Description(shortDefinition="Additional clinical information", formalDefinition="Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site)." ) 514 protected List<Reference> supportingInfo; 515 516 /** 517 * Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement. 518 */ 519 @Child(name = "note", type = {Annotation.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 520 @Description(shortDefinition="Notes or comments", formalDefinition="Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement." ) 521 protected List<Annotation> note; 522 523 /** 524 * Key events in the history of the request. 525 */ 526 @Child(name = "relevantHistory", type = {Provenance.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 527 @Description(shortDefinition="Request provenance", formalDefinition="Key events in the history of the request." ) 528 protected List<Reference> relevantHistory; 529 530 private static final long serialVersionUID = 1768564436L; 531 532 /** 533 * Constructor 534 */ 535 public DeviceRequest() { 536 super(); 537 } 538 539 /** 540 * Constructor 541 */ 542 public DeviceRequest(RequestIntent intent, CodeableReference code, Reference subject) { 543 super(); 544 this.setIntent(intent); 545 this.setCode(code); 546 this.setSubject(subject); 547 } 548 549 /** 550 * @return {@link #identifier} (Identifiers assigned to this order by the orderer or by the receiver.) 551 */ 552 public List<Identifier> getIdentifier() { 553 if (this.identifier == null) 554 this.identifier = new ArrayList<Identifier>(); 555 return this.identifier; 556 } 557 558 /** 559 * @return Returns a reference to <code>this</code> for easy method chaining 560 */ 561 public DeviceRequest setIdentifier(List<Identifier> theIdentifier) { 562 this.identifier = theIdentifier; 563 return this; 564 } 565 566 public boolean hasIdentifier() { 567 if (this.identifier == null) 568 return false; 569 for (Identifier item : this.identifier) 570 if (!item.isEmpty()) 571 return true; 572 return false; 573 } 574 575 public Identifier addIdentifier() { //3 576 Identifier t = new Identifier(); 577 if (this.identifier == null) 578 this.identifier = new ArrayList<Identifier>(); 579 this.identifier.add(t); 580 return t; 581 } 582 583 public DeviceRequest addIdentifier(Identifier t) { //3 584 if (t == null) 585 return this; 586 if (this.identifier == null) 587 this.identifier = new ArrayList<Identifier>(); 588 this.identifier.add(t); 589 return this; 590 } 591 592 /** 593 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 594 */ 595 public Identifier getIdentifierFirstRep() { 596 if (getIdentifier().isEmpty()) { 597 addIdentifier(); 598 } 599 return getIdentifier().get(0); 600 } 601 602 /** 603 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 604 */ 605 public List<CanonicalType> getInstantiatesCanonical() { 606 if (this.instantiatesCanonical == null) 607 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 608 return this.instantiatesCanonical; 609 } 610 611 /** 612 * @return Returns a reference to <code>this</code> for easy method chaining 613 */ 614 public DeviceRequest setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 615 this.instantiatesCanonical = theInstantiatesCanonical; 616 return this; 617 } 618 619 public boolean hasInstantiatesCanonical() { 620 if (this.instantiatesCanonical == null) 621 return false; 622 for (CanonicalType item : this.instantiatesCanonical) 623 if (!item.isEmpty()) 624 return true; 625 return false; 626 } 627 628 /** 629 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 630 */ 631 public CanonicalType addInstantiatesCanonicalElement() {//2 632 CanonicalType t = new CanonicalType(); 633 if (this.instantiatesCanonical == null) 634 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 635 this.instantiatesCanonical.add(t); 636 return t; 637 } 638 639 /** 640 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 641 */ 642 public DeviceRequest addInstantiatesCanonical(String value) { //1 643 CanonicalType t = new CanonicalType(); 644 t.setValue(value); 645 if (this.instantiatesCanonical == null) 646 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 647 this.instantiatesCanonical.add(t); 648 return this; 649 } 650 651 /** 652 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 653 */ 654 public boolean hasInstantiatesCanonical(String value) { 655 if (this.instantiatesCanonical == null) 656 return false; 657 for (CanonicalType v : this.instantiatesCanonical) 658 if (v.getValue().equals(value)) // canonical 659 return true; 660 return false; 661 } 662 663 /** 664 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 665 */ 666 public List<UriType> getInstantiatesUri() { 667 if (this.instantiatesUri == null) 668 this.instantiatesUri = new ArrayList<UriType>(); 669 return this.instantiatesUri; 670 } 671 672 /** 673 * @return Returns a reference to <code>this</code> for easy method chaining 674 */ 675 public DeviceRequest setInstantiatesUri(List<UriType> theInstantiatesUri) { 676 this.instantiatesUri = theInstantiatesUri; 677 return this; 678 } 679 680 public boolean hasInstantiatesUri() { 681 if (this.instantiatesUri == null) 682 return false; 683 for (UriType item : this.instantiatesUri) 684 if (!item.isEmpty()) 685 return true; 686 return false; 687 } 688 689 /** 690 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 691 */ 692 public UriType addInstantiatesUriElement() {//2 693 UriType t = new UriType(); 694 if (this.instantiatesUri == null) 695 this.instantiatesUri = new ArrayList<UriType>(); 696 this.instantiatesUri.add(t); 697 return t; 698 } 699 700 /** 701 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 702 */ 703 public DeviceRequest addInstantiatesUri(String value) { //1 704 UriType t = new UriType(); 705 t.setValue(value); 706 if (this.instantiatesUri == null) 707 this.instantiatesUri = new ArrayList<UriType>(); 708 this.instantiatesUri.add(t); 709 return this; 710 } 711 712 /** 713 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.) 714 */ 715 public boolean hasInstantiatesUri(String value) { 716 if (this.instantiatesUri == null) 717 return false; 718 for (UriType v : this.instantiatesUri) 719 if (v.getValue().equals(value)) // uri 720 return true; 721 return false; 722 } 723 724 /** 725 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 726 */ 727 public List<Reference> getBasedOn() { 728 if (this.basedOn == null) 729 this.basedOn = new ArrayList<Reference>(); 730 return this.basedOn; 731 } 732 733 /** 734 * @return Returns a reference to <code>this</code> for easy method chaining 735 */ 736 public DeviceRequest setBasedOn(List<Reference> theBasedOn) { 737 this.basedOn = theBasedOn; 738 return this; 739 } 740 741 public boolean hasBasedOn() { 742 if (this.basedOn == null) 743 return false; 744 for (Reference item : this.basedOn) 745 if (!item.isEmpty()) 746 return true; 747 return false; 748 } 749 750 public Reference addBasedOn() { //3 751 Reference t = new Reference(); 752 if (this.basedOn == null) 753 this.basedOn = new ArrayList<Reference>(); 754 this.basedOn.add(t); 755 return t; 756 } 757 758 public DeviceRequest addBasedOn(Reference t) { //3 759 if (t == null) 760 return this; 761 if (this.basedOn == null) 762 this.basedOn = new ArrayList<Reference>(); 763 this.basedOn.add(t); 764 return this; 765 } 766 767 /** 768 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 769 */ 770 public Reference getBasedOnFirstRep() { 771 if (getBasedOn().isEmpty()) { 772 addBasedOn(); 773 } 774 return getBasedOn().get(0); 775 } 776 777 /** 778 * @return {@link #replaces} (The request takes the place of the referenced completed or terminated request(s).) 779 */ 780 public List<Reference> getReplaces() { 781 if (this.replaces == null) 782 this.replaces = new ArrayList<Reference>(); 783 return this.replaces; 784 } 785 786 /** 787 * @return Returns a reference to <code>this</code> for easy method chaining 788 */ 789 public DeviceRequest setReplaces(List<Reference> theReplaces) { 790 this.replaces = theReplaces; 791 return this; 792 } 793 794 public boolean hasReplaces() { 795 if (this.replaces == null) 796 return false; 797 for (Reference item : this.replaces) 798 if (!item.isEmpty()) 799 return true; 800 return false; 801 } 802 803 public Reference addReplaces() { //3 804 Reference t = new Reference(); 805 if (this.replaces == null) 806 this.replaces = new ArrayList<Reference>(); 807 this.replaces.add(t); 808 return t; 809 } 810 811 public DeviceRequest addReplaces(Reference t) { //3 812 if (t == null) 813 return this; 814 if (this.replaces == null) 815 this.replaces = new ArrayList<Reference>(); 816 this.replaces.add(t); 817 return this; 818 } 819 820 /** 821 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist {3} 822 */ 823 public Reference getReplacesFirstRep() { 824 if (getReplaces().isEmpty()) { 825 addReplaces(); 826 } 827 return getReplaces().get(0); 828 } 829 830 /** 831 * @return {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 832 */ 833 public Identifier getGroupIdentifier() { 834 if (this.groupIdentifier == null) 835 if (Configuration.errorOnAutoCreate()) 836 throw new Error("Attempt to auto-create DeviceRequest.groupIdentifier"); 837 else if (Configuration.doAutoCreate()) 838 this.groupIdentifier = new Identifier(); // cc 839 return this.groupIdentifier; 840 } 841 842 public boolean hasGroupIdentifier() { 843 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 844 } 845 846 /** 847 * @param value {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 848 */ 849 public DeviceRequest setGroupIdentifier(Identifier value) { 850 this.groupIdentifier = value; 851 return this; 852 } 853 854 /** 855 * @return {@link #status} (The status of the request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 856 */ 857 public Enumeration<RequestStatus> getStatusElement() { 858 if (this.status == null) 859 if (Configuration.errorOnAutoCreate()) 860 throw new Error("Attempt to auto-create DeviceRequest.status"); 861 else if (Configuration.doAutoCreate()) 862 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 863 return this.status; 864 } 865 866 public boolean hasStatusElement() { 867 return this.status != null && !this.status.isEmpty(); 868 } 869 870 public boolean hasStatus() { 871 return this.status != null && !this.status.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #status} (The status of the request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 876 */ 877 public DeviceRequest setStatusElement(Enumeration<RequestStatus> value) { 878 this.status = value; 879 return this; 880 } 881 882 /** 883 * @return The status of the request. 884 */ 885 public RequestStatus getStatus() { 886 return this.status == null ? null : this.status.getValue(); 887 } 888 889 /** 890 * @param value The status of the request. 891 */ 892 public DeviceRequest setStatus(RequestStatus value) { 893 if (value == null) 894 this.status = null; 895 else { 896 if (this.status == null) 897 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 898 this.status.setValue(value); 899 } 900 return this; 901 } 902 903 /** 904 * @return {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 905 */ 906 public Enumeration<RequestIntent> getIntentElement() { 907 if (this.intent == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create DeviceRequest.intent"); 910 else if (Configuration.doAutoCreate()) 911 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 912 return this.intent; 913 } 914 915 public boolean hasIntentElement() { 916 return this.intent != null && !this.intent.isEmpty(); 917 } 918 919 public boolean hasIntent() { 920 return this.intent != null && !this.intent.isEmpty(); 921 } 922 923 /** 924 * @param value {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 925 */ 926 public DeviceRequest setIntentElement(Enumeration<RequestIntent> value) { 927 this.intent = value; 928 return this; 929 } 930 931 /** 932 * @return Whether the request is a proposal, plan, an original order or a reflex order. 933 */ 934 public RequestIntent getIntent() { 935 return this.intent == null ? null : this.intent.getValue(); 936 } 937 938 /** 939 * @param value Whether the request is a proposal, plan, an original order or a reflex order. 940 */ 941 public DeviceRequest setIntent(RequestIntent value) { 942 if (this.intent == null) 943 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 944 this.intent.setValue(value); 945 return this; 946 } 947 948 /** 949 * @return {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 950 */ 951 public Enumeration<RequestPriority> getPriorityElement() { 952 if (this.priority == null) 953 if (Configuration.errorOnAutoCreate()) 954 throw new Error("Attempt to auto-create DeviceRequest.priority"); 955 else if (Configuration.doAutoCreate()) 956 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 957 return this.priority; 958 } 959 960 public boolean hasPriorityElement() { 961 return this.priority != null && !this.priority.isEmpty(); 962 } 963 964 public boolean hasPriority() { 965 return this.priority != null && !this.priority.isEmpty(); 966 } 967 968 /** 969 * @param value {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 970 */ 971 public DeviceRequest setPriorityElement(Enumeration<RequestPriority> value) { 972 this.priority = value; 973 return this; 974 } 975 976 /** 977 * @return Indicates how quickly the request should be addressed with respect to other requests. 978 */ 979 public RequestPriority getPriority() { 980 return this.priority == null ? null : this.priority.getValue(); 981 } 982 983 /** 984 * @param value Indicates how quickly the request should be addressed with respect to other requests. 985 */ 986 public DeviceRequest setPriority(RequestPriority value) { 987 if (value == null) 988 this.priority = null; 989 else { 990 if (this.priority == null) 991 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 992 this.priority.setValue(value); 993 } 994 return this; 995 } 996 997 /** 998 * @return {@link #doNotPerform} (If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 999 */ 1000 public BooleanType getDoNotPerformElement() { 1001 if (this.doNotPerform == null) 1002 if (Configuration.errorOnAutoCreate()) 1003 throw new Error("Attempt to auto-create DeviceRequest.doNotPerform"); 1004 else if (Configuration.doAutoCreate()) 1005 this.doNotPerform = new BooleanType(); // bb 1006 return this.doNotPerform; 1007 } 1008 1009 public boolean hasDoNotPerformElement() { 1010 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1011 } 1012 1013 public boolean hasDoNotPerform() { 1014 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1015 } 1016 1017 /** 1018 * @param value {@link #doNotPerform} (If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 1019 */ 1020 public DeviceRequest setDoNotPerformElement(BooleanType value) { 1021 this.doNotPerform = value; 1022 return this; 1023 } 1024 1025 /** 1026 * @return If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses. 1027 */ 1028 public boolean getDoNotPerform() { 1029 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1030 } 1031 1032 /** 1033 * @param value If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses. 1034 */ 1035 public DeviceRequest setDoNotPerform(boolean value) { 1036 if (this.doNotPerform == null) 1037 this.doNotPerform = new BooleanType(); 1038 this.doNotPerform.setValue(value); 1039 return this; 1040 } 1041 1042 /** 1043 * @return {@link #code} (The details of the device to be used.) 1044 */ 1045 public CodeableReference getCode() { 1046 if (this.code == null) 1047 if (Configuration.errorOnAutoCreate()) 1048 throw new Error("Attempt to auto-create DeviceRequest.code"); 1049 else if (Configuration.doAutoCreate()) 1050 this.code = new CodeableReference(); // cc 1051 return this.code; 1052 } 1053 1054 public boolean hasCode() { 1055 return this.code != null && !this.code.isEmpty(); 1056 } 1057 1058 /** 1059 * @param value {@link #code} (The details of the device to be used.) 1060 */ 1061 public DeviceRequest setCode(CodeableReference value) { 1062 this.code = value; 1063 return this; 1064 } 1065 1066 /** 1067 * @return {@link #quantity} (The number of devices to be provided.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 1068 */ 1069 public IntegerType getQuantityElement() { 1070 if (this.quantity == null) 1071 if (Configuration.errorOnAutoCreate()) 1072 throw new Error("Attempt to auto-create DeviceRequest.quantity"); 1073 else if (Configuration.doAutoCreate()) 1074 this.quantity = new IntegerType(); // bb 1075 return this.quantity; 1076 } 1077 1078 public boolean hasQuantityElement() { 1079 return this.quantity != null && !this.quantity.isEmpty(); 1080 } 1081 1082 public boolean hasQuantity() { 1083 return this.quantity != null && !this.quantity.isEmpty(); 1084 } 1085 1086 /** 1087 * @param value {@link #quantity} (The number of devices to be provided.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 1088 */ 1089 public DeviceRequest setQuantityElement(IntegerType value) { 1090 this.quantity = value; 1091 return this; 1092 } 1093 1094 /** 1095 * @return The number of devices to be provided. 1096 */ 1097 public int getQuantity() { 1098 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 1099 } 1100 1101 /** 1102 * @param value The number of devices to be provided. 1103 */ 1104 public DeviceRequest setQuantity(int value) { 1105 if (this.quantity == null) 1106 this.quantity = new IntegerType(); 1107 this.quantity.setValue(value); 1108 return this; 1109 } 1110 1111 /** 1112 * @return {@link #parameter} (Specific parameters for the ordered item. For example, the prism value for lenses.) 1113 */ 1114 public List<DeviceRequestParameterComponent> getParameter() { 1115 if (this.parameter == null) 1116 this.parameter = new ArrayList<DeviceRequestParameterComponent>(); 1117 return this.parameter; 1118 } 1119 1120 /** 1121 * @return Returns a reference to <code>this</code> for easy method chaining 1122 */ 1123 public DeviceRequest setParameter(List<DeviceRequestParameterComponent> theParameter) { 1124 this.parameter = theParameter; 1125 return this; 1126 } 1127 1128 public boolean hasParameter() { 1129 if (this.parameter == null) 1130 return false; 1131 for (DeviceRequestParameterComponent item : this.parameter) 1132 if (!item.isEmpty()) 1133 return true; 1134 return false; 1135 } 1136 1137 public DeviceRequestParameterComponent addParameter() { //3 1138 DeviceRequestParameterComponent t = new DeviceRequestParameterComponent(); 1139 if (this.parameter == null) 1140 this.parameter = new ArrayList<DeviceRequestParameterComponent>(); 1141 this.parameter.add(t); 1142 return t; 1143 } 1144 1145 public DeviceRequest addParameter(DeviceRequestParameterComponent t) { //3 1146 if (t == null) 1147 return this; 1148 if (this.parameter == null) 1149 this.parameter = new ArrayList<DeviceRequestParameterComponent>(); 1150 this.parameter.add(t); 1151 return this; 1152 } 1153 1154 /** 1155 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 1156 */ 1157 public DeviceRequestParameterComponent getParameterFirstRep() { 1158 if (getParameter().isEmpty()) { 1159 addParameter(); 1160 } 1161 return getParameter().get(0); 1162 } 1163 1164 /** 1165 * @return {@link #subject} (The patient who will use the device.) 1166 */ 1167 public Reference getSubject() { 1168 if (this.subject == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create DeviceRequest.subject"); 1171 else if (Configuration.doAutoCreate()) 1172 this.subject = new Reference(); // cc 1173 return this.subject; 1174 } 1175 1176 public boolean hasSubject() { 1177 return this.subject != null && !this.subject.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #subject} (The patient who will use the device.) 1182 */ 1183 public DeviceRequest setSubject(Reference value) { 1184 this.subject = value; 1185 return this; 1186 } 1187 1188 /** 1189 * @return {@link #encounter} (An encounter that provides additional context in which this request is made.) 1190 */ 1191 public Reference getEncounter() { 1192 if (this.encounter == null) 1193 if (Configuration.errorOnAutoCreate()) 1194 throw new Error("Attempt to auto-create DeviceRequest.encounter"); 1195 else if (Configuration.doAutoCreate()) 1196 this.encounter = new Reference(); // cc 1197 return this.encounter; 1198 } 1199 1200 public boolean hasEncounter() { 1201 return this.encounter != null && !this.encounter.isEmpty(); 1202 } 1203 1204 /** 1205 * @param value {@link #encounter} (An encounter that provides additional context in which this request is made.) 1206 */ 1207 public DeviceRequest setEncounter(Reference value) { 1208 this.encounter = value; 1209 return this; 1210 } 1211 1212 /** 1213 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1214 */ 1215 public DataType getOccurrence() { 1216 return this.occurrence; 1217 } 1218 1219 /** 1220 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1221 */ 1222 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1223 if (this.occurrence == null) 1224 this.occurrence = new DateTimeType(); 1225 if (!(this.occurrence instanceof DateTimeType)) 1226 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1227 return (DateTimeType) this.occurrence; 1228 } 1229 1230 public boolean hasOccurrenceDateTimeType() { 1231 return this != null && this.occurrence instanceof DateTimeType; 1232 } 1233 1234 /** 1235 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1236 */ 1237 public Period getOccurrencePeriod() throws FHIRException { 1238 if (this.occurrence == null) 1239 this.occurrence = new Period(); 1240 if (!(this.occurrence instanceof Period)) 1241 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1242 return (Period) this.occurrence; 1243 } 1244 1245 public boolean hasOccurrencePeriod() { 1246 return this != null && this.occurrence instanceof Period; 1247 } 1248 1249 /** 1250 * @return {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1251 */ 1252 public Timing getOccurrenceTiming() throws FHIRException { 1253 if (this.occurrence == null) 1254 this.occurrence = new Timing(); 1255 if (!(this.occurrence instanceof Timing)) 1256 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1257 return (Timing) this.occurrence; 1258 } 1259 1260 public boolean hasOccurrenceTiming() { 1261 return this != null && this.occurrence instanceof Timing; 1262 } 1263 1264 public boolean hasOccurrence() { 1265 return this.occurrence != null && !this.occurrence.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #occurrence} (The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. "Every 8 hours"; "Three times a day"; "1/2 an hour before breakfast for 10 days from 23-Dec 2011:"; "15 Oct 2013, 17 Oct 2013 and 1 Nov 2013".) 1270 */ 1271 public DeviceRequest setOccurrence(DataType value) { 1272 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1273 throw new FHIRException("Not the right type for DeviceRequest.occurrence[x]: "+value.fhirType()); 1274 this.occurrence = value; 1275 return this; 1276 } 1277 1278 /** 1279 * @return {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1280 */ 1281 public DateTimeType getAuthoredOnElement() { 1282 if (this.authoredOn == null) 1283 if (Configuration.errorOnAutoCreate()) 1284 throw new Error("Attempt to auto-create DeviceRequest.authoredOn"); 1285 else if (Configuration.doAutoCreate()) 1286 this.authoredOn = new DateTimeType(); // bb 1287 return this.authoredOn; 1288 } 1289 1290 public boolean hasAuthoredOnElement() { 1291 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1292 } 1293 1294 public boolean hasAuthoredOn() { 1295 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1296 } 1297 1298 /** 1299 * @param value {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1300 */ 1301 public DeviceRequest setAuthoredOnElement(DateTimeType value) { 1302 this.authoredOn = value; 1303 return this; 1304 } 1305 1306 /** 1307 * @return When the request transitioned to being actionable. 1308 */ 1309 public Date getAuthoredOn() { 1310 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1311 } 1312 1313 /** 1314 * @param value When the request transitioned to being actionable. 1315 */ 1316 public DeviceRequest setAuthoredOn(Date value) { 1317 if (value == null) 1318 this.authoredOn = null; 1319 else { 1320 if (this.authoredOn == null) 1321 this.authoredOn = new DateTimeType(); 1322 this.authoredOn.setValue(value); 1323 } 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #requester} (The individual or entity who initiated the request and has responsibility for its activation.) 1329 */ 1330 public Reference getRequester() { 1331 if (this.requester == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create DeviceRequest.requester"); 1334 else if (Configuration.doAutoCreate()) 1335 this.requester = new Reference(); // cc 1336 return this.requester; 1337 } 1338 1339 public boolean hasRequester() { 1340 return this.requester != null && !this.requester.isEmpty(); 1341 } 1342 1343 /** 1344 * @param value {@link #requester} (The individual or entity who initiated the request and has responsibility for its activation.) 1345 */ 1346 public DeviceRequest setRequester(Reference value) { 1347 this.requester = value; 1348 return this; 1349 } 1350 1351 /** 1352 * @return {@link #performer} (The desired individual or entity to provide the device to the subject of the request (e.g., patient, location).) 1353 */ 1354 public CodeableReference getPerformer() { 1355 if (this.performer == null) 1356 if (Configuration.errorOnAutoCreate()) 1357 throw new Error("Attempt to auto-create DeviceRequest.performer"); 1358 else if (Configuration.doAutoCreate()) 1359 this.performer = new CodeableReference(); // cc 1360 return this.performer; 1361 } 1362 1363 public boolean hasPerformer() { 1364 return this.performer != null && !this.performer.isEmpty(); 1365 } 1366 1367 /** 1368 * @param value {@link #performer} (The desired individual or entity to provide the device to the subject of the request (e.g., patient, location).) 1369 */ 1370 public DeviceRequest setPerformer(CodeableReference value) { 1371 this.performer = value; 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #reason} (Reason or justification for the use of this device.) 1377 */ 1378 public List<CodeableReference> getReason() { 1379 if (this.reason == null) 1380 this.reason = new ArrayList<CodeableReference>(); 1381 return this.reason; 1382 } 1383 1384 /** 1385 * @return Returns a reference to <code>this</code> for easy method chaining 1386 */ 1387 public DeviceRequest setReason(List<CodeableReference> theReason) { 1388 this.reason = theReason; 1389 return this; 1390 } 1391 1392 public boolean hasReason() { 1393 if (this.reason == null) 1394 return false; 1395 for (CodeableReference item : this.reason) 1396 if (!item.isEmpty()) 1397 return true; 1398 return false; 1399 } 1400 1401 public CodeableReference addReason() { //3 1402 CodeableReference t = new CodeableReference(); 1403 if (this.reason == null) 1404 this.reason = new ArrayList<CodeableReference>(); 1405 this.reason.add(t); 1406 return t; 1407 } 1408 1409 public DeviceRequest addReason(CodeableReference t) { //3 1410 if (t == null) 1411 return this; 1412 if (this.reason == null) 1413 this.reason = new ArrayList<CodeableReference>(); 1414 this.reason.add(t); 1415 return this; 1416 } 1417 1418 /** 1419 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1420 */ 1421 public CodeableReference getReasonFirstRep() { 1422 if (getReason().isEmpty()) { 1423 addReason(); 1424 } 1425 return getReason().get(0); 1426 } 1427 1428 /** 1429 * @return {@link #asNeeded} (This status is to indicate whether the request is a PRN or may be given as needed.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 1430 */ 1431 public BooleanType getAsNeededElement() { 1432 if (this.asNeeded == null) 1433 if (Configuration.errorOnAutoCreate()) 1434 throw new Error("Attempt to auto-create DeviceRequest.asNeeded"); 1435 else if (Configuration.doAutoCreate()) 1436 this.asNeeded = new BooleanType(); // bb 1437 return this.asNeeded; 1438 } 1439 1440 public boolean hasAsNeededElement() { 1441 return this.asNeeded != null && !this.asNeeded.isEmpty(); 1442 } 1443 1444 public boolean hasAsNeeded() { 1445 return this.asNeeded != null && !this.asNeeded.isEmpty(); 1446 } 1447 1448 /** 1449 * @param value {@link #asNeeded} (This status is to indicate whether the request is a PRN or may be given as needed.). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 1450 */ 1451 public DeviceRequest setAsNeededElement(BooleanType value) { 1452 this.asNeeded = value; 1453 return this; 1454 } 1455 1456 /** 1457 * @return This status is to indicate whether the request is a PRN or may be given as needed. 1458 */ 1459 public boolean getAsNeeded() { 1460 return this.asNeeded == null || this.asNeeded.isEmpty() ? false : this.asNeeded.getValue(); 1461 } 1462 1463 /** 1464 * @param value This status is to indicate whether the request is a PRN or may be given as needed. 1465 */ 1466 public DeviceRequest setAsNeeded(boolean value) { 1467 if (this.asNeeded == null) 1468 this.asNeeded = new BooleanType(); 1469 this.asNeeded.setValue(value); 1470 return this; 1471 } 1472 1473 /** 1474 * @return {@link #asNeededFor} (The reason for using the device.) 1475 */ 1476 public CodeableConcept getAsNeededFor() { 1477 if (this.asNeededFor == null) 1478 if (Configuration.errorOnAutoCreate()) 1479 throw new Error("Attempt to auto-create DeviceRequest.asNeededFor"); 1480 else if (Configuration.doAutoCreate()) 1481 this.asNeededFor = new CodeableConcept(); // cc 1482 return this.asNeededFor; 1483 } 1484 1485 public boolean hasAsNeededFor() { 1486 return this.asNeededFor != null && !this.asNeededFor.isEmpty(); 1487 } 1488 1489 /** 1490 * @param value {@link #asNeededFor} (The reason for using the device.) 1491 */ 1492 public DeviceRequest setAsNeededFor(CodeableConcept value) { 1493 this.asNeededFor = value; 1494 return this; 1495 } 1496 1497 /** 1498 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.) 1499 */ 1500 public List<Reference> getInsurance() { 1501 if (this.insurance == null) 1502 this.insurance = new ArrayList<Reference>(); 1503 return this.insurance; 1504 } 1505 1506 /** 1507 * @return Returns a reference to <code>this</code> for easy method chaining 1508 */ 1509 public DeviceRequest setInsurance(List<Reference> theInsurance) { 1510 this.insurance = theInsurance; 1511 return this; 1512 } 1513 1514 public boolean hasInsurance() { 1515 if (this.insurance == null) 1516 return false; 1517 for (Reference item : this.insurance) 1518 if (!item.isEmpty()) 1519 return true; 1520 return false; 1521 } 1522 1523 public Reference addInsurance() { //3 1524 Reference t = new Reference(); 1525 if (this.insurance == null) 1526 this.insurance = new ArrayList<Reference>(); 1527 this.insurance.add(t); 1528 return t; 1529 } 1530 1531 public DeviceRequest addInsurance(Reference t) { //3 1532 if (t == null) 1533 return this; 1534 if (this.insurance == null) 1535 this.insurance = new ArrayList<Reference>(); 1536 this.insurance.add(t); 1537 return this; 1538 } 1539 1540 /** 1541 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 1542 */ 1543 public Reference getInsuranceFirstRep() { 1544 if (getInsurance().isEmpty()) { 1545 addInsurance(); 1546 } 1547 return getInsurance().get(0); 1548 } 1549 1550 /** 1551 * @return {@link #supportingInfo} (Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site).) 1552 */ 1553 public List<Reference> getSupportingInfo() { 1554 if (this.supportingInfo == null) 1555 this.supportingInfo = new ArrayList<Reference>(); 1556 return this.supportingInfo; 1557 } 1558 1559 /** 1560 * @return Returns a reference to <code>this</code> for easy method chaining 1561 */ 1562 public DeviceRequest setSupportingInfo(List<Reference> theSupportingInfo) { 1563 this.supportingInfo = theSupportingInfo; 1564 return this; 1565 } 1566 1567 public boolean hasSupportingInfo() { 1568 if (this.supportingInfo == null) 1569 return false; 1570 for (Reference item : this.supportingInfo) 1571 if (!item.isEmpty()) 1572 return true; 1573 return false; 1574 } 1575 1576 public Reference addSupportingInfo() { //3 1577 Reference t = new Reference(); 1578 if (this.supportingInfo == null) 1579 this.supportingInfo = new ArrayList<Reference>(); 1580 this.supportingInfo.add(t); 1581 return t; 1582 } 1583 1584 public DeviceRequest addSupportingInfo(Reference t) { //3 1585 if (t == null) 1586 return this; 1587 if (this.supportingInfo == null) 1588 this.supportingInfo = new ArrayList<Reference>(); 1589 this.supportingInfo.add(t); 1590 return this; 1591 } 1592 1593 /** 1594 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 1595 */ 1596 public Reference getSupportingInfoFirstRep() { 1597 if (getSupportingInfo().isEmpty()) { 1598 addSupportingInfo(); 1599 } 1600 return getSupportingInfo().get(0); 1601 } 1602 1603 /** 1604 * @return {@link #note} (Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.) 1605 */ 1606 public List<Annotation> getNote() { 1607 if (this.note == null) 1608 this.note = new ArrayList<Annotation>(); 1609 return this.note; 1610 } 1611 1612 /** 1613 * @return Returns a reference to <code>this</code> for easy method chaining 1614 */ 1615 public DeviceRequest setNote(List<Annotation> theNote) { 1616 this.note = theNote; 1617 return this; 1618 } 1619 1620 public boolean hasNote() { 1621 if (this.note == null) 1622 return false; 1623 for (Annotation item : this.note) 1624 if (!item.isEmpty()) 1625 return true; 1626 return false; 1627 } 1628 1629 public Annotation addNote() { //3 1630 Annotation t = new Annotation(); 1631 if (this.note == null) 1632 this.note = new ArrayList<Annotation>(); 1633 this.note.add(t); 1634 return t; 1635 } 1636 1637 public DeviceRequest addNote(Annotation t) { //3 1638 if (t == null) 1639 return this; 1640 if (this.note == null) 1641 this.note = new ArrayList<Annotation>(); 1642 this.note.add(t); 1643 return this; 1644 } 1645 1646 /** 1647 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1648 */ 1649 public Annotation getNoteFirstRep() { 1650 if (getNote().isEmpty()) { 1651 addNote(); 1652 } 1653 return getNote().get(0); 1654 } 1655 1656 /** 1657 * @return {@link #relevantHistory} (Key events in the history of the request.) 1658 */ 1659 public List<Reference> getRelevantHistory() { 1660 if (this.relevantHistory == null) 1661 this.relevantHistory = new ArrayList<Reference>(); 1662 return this.relevantHistory; 1663 } 1664 1665 /** 1666 * @return Returns a reference to <code>this</code> for easy method chaining 1667 */ 1668 public DeviceRequest setRelevantHistory(List<Reference> theRelevantHistory) { 1669 this.relevantHistory = theRelevantHistory; 1670 return this; 1671 } 1672 1673 public boolean hasRelevantHistory() { 1674 if (this.relevantHistory == null) 1675 return false; 1676 for (Reference item : this.relevantHistory) 1677 if (!item.isEmpty()) 1678 return true; 1679 return false; 1680 } 1681 1682 public Reference addRelevantHistory() { //3 1683 Reference t = new Reference(); 1684 if (this.relevantHistory == null) 1685 this.relevantHistory = new ArrayList<Reference>(); 1686 this.relevantHistory.add(t); 1687 return t; 1688 } 1689 1690 public DeviceRequest addRelevantHistory(Reference t) { //3 1691 if (t == null) 1692 return this; 1693 if (this.relevantHistory == null) 1694 this.relevantHistory = new ArrayList<Reference>(); 1695 this.relevantHistory.add(t); 1696 return this; 1697 } 1698 1699 /** 1700 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist {3} 1701 */ 1702 public Reference getRelevantHistoryFirstRep() { 1703 if (getRelevantHistory().isEmpty()) { 1704 addRelevantHistory(); 1705 } 1706 return getRelevantHistory().get(0); 1707 } 1708 1709 protected void listChildren(List<Property> children) { 1710 super.listChildren(children); 1711 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1712 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 1713 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 1714 children.add(new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1715 children.add(new Property("replaces", "Reference(DeviceRequest)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces)); 1716 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier)); 1717 children.add(new Property("status", "code", "The status of the request.", 0, 1, status)); 1718 children.add(new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 1719 children.add(new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority)); 1720 children.add(new Property("doNotPerform", "boolean", "If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses.", 0, 1, doNotPerform)); 1721 children.add(new Property("code", "CodeableReference(Device|DeviceDefinition)", "The details of the device to be used.", 0, 1, code)); 1722 children.add(new Property("quantity", "integer", "The number of devices to be provided.", 0, 1, quantity)); 1723 children.add(new Property("parameter", "", "Specific parameters for the ordered item. For example, the prism value for lenses.", 0, java.lang.Integer.MAX_VALUE, parameter)); 1724 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", "The patient who will use the device.", 0, 1, subject)); 1725 children.add(new Property("encounter", "Reference(Encounter)", "An encounter that provides additional context in which this request is made.", 0, 1, encounter)); 1726 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence)); 1727 children.add(new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 1728 children.add(new Property("requester", "Reference(Device|Practitioner|PractitionerRole|Organization)", "The individual or entity who initiated the request and has responsibility for its activation.", 0, 1, requester)); 1729 children.add(new Property("performer", "CodeableReference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "The desired individual or entity to provide the device to the subject of the request (e.g., patient, location).", 0, 1, performer)); 1730 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reason)); 1731 children.add(new Property("asNeeded", "boolean", "This status is to indicate whether the request is a PRN or may be given as needed.", 0, 1, asNeeded)); 1732 children.add(new Property("asNeededFor", "CodeableConcept", "The reason for using the device.", 0, 1, asNeededFor)); 1733 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance)); 1734 children.add(new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site).", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 1735 children.add(new Property("note", "Annotation", "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note)); 1736 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 1737 } 1738 1739 @Override 1740 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1741 switch (_hash) { 1742 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order by the orderer or by the receiver.", 0, java.lang.Integer.MAX_VALUE, identifier); 1743 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 1744 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this DeviceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 1745 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1746 case -430332865: /*replaces*/ return new Property("replaces", "Reference(DeviceRequest)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces); 1747 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier); 1748 case -892481550: /*status*/ return new Property("status", "code", "The status of the request.", 0, 1, status); 1749 case -1183762788: /*intent*/ return new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 1750 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority); 1751 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "If true, indicates that the provider is asking for the patient to either stop using or to not start using the specified device or category of devices. For example, the patient has undergone surgery and the provider is indicating that the patient should not wear contact lenses.", 0, 1, doNotPerform); 1752 case 3059181: /*code*/ return new Property("code", "CodeableReference(Device|DeviceDefinition)", "The details of the device to be used.", 0, 1, code); 1753 case -1285004149: /*quantity*/ return new Property("quantity", "integer", "The number of devices to be provided.", 0, 1, quantity); 1754 case 1954460585: /*parameter*/ return new Property("parameter", "", "Specific parameters for the ordered item. For example, the prism value for lenses.", 0, java.lang.Integer.MAX_VALUE, parameter); 1755 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Location|Device)", "The patient who will use the device.", 0, 1, subject); 1756 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "An encounter that provides additional context in which this request is made.", 0, 1, encounter); 1757 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1758 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1759 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1760 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1761 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "The timing schedule for the use of the device. The Schedule data type allows many different expressions, for example. \"Every 8 hours\"; \"Three times a day\"; \"1/2 an hour before breakfast for 10 days from 23-Dec 2011:\"; \"15 Oct 2013, 17 Oct 2013 and 1 Nov 2013\".", 0, 1, occurrence); 1762 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn); 1763 case 693933948: /*requester*/ return new Property("requester", "Reference(Device|Practitioner|PractitionerRole|Organization)", "The individual or entity who initiated the request and has responsibility for its activation.", 0, 1, requester); 1764 case 481140686: /*performer*/ return new Property("performer", "CodeableReference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "The desired individual or entity to provide the device to the subject of the request (e.g., patient, location).", 0, 1, performer); 1765 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "Reason or justification for the use of this device.", 0, java.lang.Integer.MAX_VALUE, reason); 1766 case -1432923513: /*asNeeded*/ return new Property("asNeeded", "boolean", "This status is to indicate whether the request is a PRN or may be given as needed.", 0, 1, asNeeded); 1767 case -544350014: /*asNeededFor*/ return new Property("asNeededFor", "CodeableConcept", "The reason for using the device.", 0, 1, asNeededFor); 1768 case 73049818: /*insurance*/ return new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance); 1769 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "Reference(Any)", "Additional clinical information about the patient that may influence the request fulfilment. For example, this may include where on the subject's body the device will be used (i.e. the target site).", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 1770 case 3387378: /*note*/ return new Property("note", "Annotation", "Details about this request that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note); 1771 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 1772 default: return super.getNamedProperty(_hash, _name, _checkValid); 1773 } 1774 1775 } 1776 1777 @Override 1778 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1779 switch (hash) { 1780 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1781 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 1782 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 1783 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1784 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 1785 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 1786 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 1787 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 1788 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1789 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 1790 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableReference 1791 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // IntegerType 1792 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // DeviceRequestParameterComponent 1793 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1794 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1795 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 1796 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 1797 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 1798 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // CodeableReference 1799 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1800 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // BooleanType 1801 case -544350014: /*asNeededFor*/ return this.asNeededFor == null ? new Base[0] : new Base[] {this.asNeededFor}; // CodeableConcept 1802 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 1803 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 1804 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1805 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 1806 default: return super.getProperty(hash, name, checkValid); 1807 } 1808 1809 } 1810 1811 @Override 1812 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1813 switch (hash) { 1814 case -1618432855: // identifier 1815 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1816 return value; 1817 case 8911915: // instantiatesCanonical 1818 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 1819 return value; 1820 case -1926393373: // instantiatesUri 1821 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 1822 return value; 1823 case -332612366: // basedOn 1824 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1825 return value; 1826 case -430332865: // replaces 1827 this.getReplaces().add(TypeConvertor.castToReference(value)); // Reference 1828 return value; 1829 case -445338488: // groupIdentifier 1830 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 1831 return value; 1832 case -892481550: // status 1833 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1834 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1835 return value; 1836 case -1183762788: // intent 1837 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1838 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 1839 return value; 1840 case -1165461084: // priority 1841 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1842 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1843 return value; 1844 case -1788508167: // doNotPerform 1845 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 1846 return value; 1847 case 3059181: // code 1848 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 1849 return value; 1850 case -1285004149: // quantity 1851 this.quantity = TypeConvertor.castToInteger(value); // IntegerType 1852 return value; 1853 case 1954460585: // parameter 1854 this.getParameter().add((DeviceRequestParameterComponent) value); // DeviceRequestParameterComponent 1855 return value; 1856 case -1867885268: // subject 1857 this.subject = TypeConvertor.castToReference(value); // Reference 1858 return value; 1859 case 1524132147: // encounter 1860 this.encounter = TypeConvertor.castToReference(value); // Reference 1861 return value; 1862 case 1687874001: // occurrence 1863 this.occurrence = TypeConvertor.castToType(value); // DataType 1864 return value; 1865 case -1500852503: // authoredOn 1866 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 1867 return value; 1868 case 693933948: // requester 1869 this.requester = TypeConvertor.castToReference(value); // Reference 1870 return value; 1871 case 481140686: // performer 1872 this.performer = TypeConvertor.castToCodeableReference(value); // CodeableReference 1873 return value; 1874 case -934964668: // reason 1875 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1876 return value; 1877 case -1432923513: // asNeeded 1878 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 1879 return value; 1880 case -544350014: // asNeededFor 1881 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1882 return value; 1883 case 73049818: // insurance 1884 this.getInsurance().add(TypeConvertor.castToReference(value)); // Reference 1885 return value; 1886 case 1922406657: // supportingInfo 1887 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); // Reference 1888 return value; 1889 case 3387378: // note 1890 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1891 return value; 1892 case 1538891575: // relevantHistory 1893 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); // Reference 1894 return value; 1895 default: return super.setProperty(hash, name, value); 1896 } 1897 1898 } 1899 1900 @Override 1901 public Base setProperty(String name, Base value) throws FHIRException { 1902 if (name.equals("identifier")) { 1903 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1904 } else if (name.equals("instantiatesCanonical")) { 1905 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 1906 } else if (name.equals("instantiatesUri")) { 1907 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 1908 } else if (name.equals("basedOn")) { 1909 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1910 } else if (name.equals("replaces")) { 1911 this.getReplaces().add(TypeConvertor.castToReference(value)); 1912 } else if (name.equals("groupIdentifier")) { 1913 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 1914 } else if (name.equals("status")) { 1915 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1916 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1917 } else if (name.equals("intent")) { 1918 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1919 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 1920 } else if (name.equals("priority")) { 1921 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1922 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1923 } else if (name.equals("doNotPerform")) { 1924 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 1925 } else if (name.equals("code")) { 1926 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 1927 } else if (name.equals("quantity")) { 1928 this.quantity = TypeConvertor.castToInteger(value); // IntegerType 1929 } else if (name.equals("parameter")) { 1930 this.getParameter().add((DeviceRequestParameterComponent) value); 1931 } else if (name.equals("subject")) { 1932 this.subject = TypeConvertor.castToReference(value); // Reference 1933 } else if (name.equals("encounter")) { 1934 this.encounter = TypeConvertor.castToReference(value); // Reference 1935 } else if (name.equals("occurrence[x]")) { 1936 this.occurrence = TypeConvertor.castToType(value); // DataType 1937 } else if (name.equals("authoredOn")) { 1938 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 1939 } else if (name.equals("requester")) { 1940 this.requester = TypeConvertor.castToReference(value); // Reference 1941 } else if (name.equals("performer")) { 1942 this.performer = TypeConvertor.castToCodeableReference(value); // CodeableReference 1943 } else if (name.equals("reason")) { 1944 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1945 } else if (name.equals("asNeeded")) { 1946 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 1947 } else if (name.equals("asNeededFor")) { 1948 this.asNeededFor = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1949 } else if (name.equals("insurance")) { 1950 this.getInsurance().add(TypeConvertor.castToReference(value)); 1951 } else if (name.equals("supportingInfo")) { 1952 this.getSupportingInfo().add(TypeConvertor.castToReference(value)); 1953 } else if (name.equals("note")) { 1954 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1955 } else if (name.equals("relevantHistory")) { 1956 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); 1957 } else 1958 return super.setProperty(name, value); 1959 return value; 1960 } 1961 1962 @Override 1963 public void removeChild(String name, Base value) throws FHIRException { 1964 if (name.equals("identifier")) { 1965 this.getIdentifier().remove(value); 1966 } else if (name.equals("instantiatesCanonical")) { 1967 this.getInstantiatesCanonical().remove(value); 1968 } else if (name.equals("instantiatesUri")) { 1969 this.getInstantiatesUri().remove(value); 1970 } else if (name.equals("basedOn")) { 1971 this.getBasedOn().remove(value); 1972 } else if (name.equals("replaces")) { 1973 this.getReplaces().remove(value); 1974 } else if (name.equals("groupIdentifier")) { 1975 this.groupIdentifier = null; 1976 } else if (name.equals("status")) { 1977 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1978 this.status = (Enumeration) value; // Enumeration<RequestStatus> 1979 } else if (name.equals("intent")) { 1980 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1981 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 1982 } else if (name.equals("priority")) { 1983 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1984 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1985 } else if (name.equals("doNotPerform")) { 1986 this.doNotPerform = null; 1987 } else if (name.equals("code")) { 1988 this.code = null; 1989 } else if (name.equals("quantity")) { 1990 this.quantity = null; 1991 } else if (name.equals("parameter")) { 1992 this.getParameter().remove((DeviceRequestParameterComponent) value); 1993 } else if (name.equals("subject")) { 1994 this.subject = null; 1995 } else if (name.equals("encounter")) { 1996 this.encounter = null; 1997 } else if (name.equals("occurrence[x]")) { 1998 this.occurrence = null; 1999 } else if (name.equals("authoredOn")) { 2000 this.authoredOn = null; 2001 } else if (name.equals("requester")) { 2002 this.requester = null; 2003 } else if (name.equals("performer")) { 2004 this.performer = null; 2005 } else if (name.equals("reason")) { 2006 this.getReason().remove(value); 2007 } else if (name.equals("asNeeded")) { 2008 this.asNeeded = null; 2009 } else if (name.equals("asNeededFor")) { 2010 this.asNeededFor = null; 2011 } else if (name.equals("insurance")) { 2012 this.getInsurance().remove(value); 2013 } else if (name.equals("supportingInfo")) { 2014 this.getSupportingInfo().remove(value); 2015 } else if (name.equals("note")) { 2016 this.getNote().remove(value); 2017 } else if (name.equals("relevantHistory")) { 2018 this.getRelevantHistory().remove(value); 2019 } else 2020 super.removeChild(name, value); 2021 2022 } 2023 2024 @Override 2025 public Base makeProperty(int hash, String name) throws FHIRException { 2026 switch (hash) { 2027 case -1618432855: return addIdentifier(); 2028 case 8911915: return addInstantiatesCanonicalElement(); 2029 case -1926393373: return addInstantiatesUriElement(); 2030 case -332612366: return addBasedOn(); 2031 case -430332865: return addReplaces(); 2032 case -445338488: return getGroupIdentifier(); 2033 case -892481550: return getStatusElement(); 2034 case -1183762788: return getIntentElement(); 2035 case -1165461084: return getPriorityElement(); 2036 case -1788508167: return getDoNotPerformElement(); 2037 case 3059181: return getCode(); 2038 case -1285004149: return getQuantityElement(); 2039 case 1954460585: return addParameter(); 2040 case -1867885268: return getSubject(); 2041 case 1524132147: return getEncounter(); 2042 case -2022646513: return getOccurrence(); 2043 case 1687874001: return getOccurrence(); 2044 case -1500852503: return getAuthoredOnElement(); 2045 case 693933948: return getRequester(); 2046 case 481140686: return getPerformer(); 2047 case -934964668: return addReason(); 2048 case -1432923513: return getAsNeededElement(); 2049 case -544350014: return getAsNeededFor(); 2050 case 73049818: return addInsurance(); 2051 case 1922406657: return addSupportingInfo(); 2052 case 3387378: return addNote(); 2053 case 1538891575: return addRelevantHistory(); 2054 default: return super.makeProperty(hash, name); 2055 } 2056 2057 } 2058 2059 @Override 2060 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2061 switch (hash) { 2062 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2063 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 2064 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 2065 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2066 case -430332865: /*replaces*/ return new String[] {"Reference"}; 2067 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 2068 case -892481550: /*status*/ return new String[] {"code"}; 2069 case -1183762788: /*intent*/ return new String[] {"code"}; 2070 case -1165461084: /*priority*/ return new String[] {"code"}; 2071 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 2072 case 3059181: /*code*/ return new String[] {"CodeableReference"}; 2073 case -1285004149: /*quantity*/ return new String[] {"integer"}; 2074 case 1954460585: /*parameter*/ return new String[] {}; 2075 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2076 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2077 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 2078 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 2079 case 693933948: /*requester*/ return new String[] {"Reference"}; 2080 case 481140686: /*performer*/ return new String[] {"CodeableReference"}; 2081 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 2082 case -1432923513: /*asNeeded*/ return new String[] {"boolean"}; 2083 case -544350014: /*asNeededFor*/ return new String[] {"CodeableConcept"}; 2084 case 73049818: /*insurance*/ return new String[] {"Reference"}; 2085 case 1922406657: /*supportingInfo*/ return new String[] {"Reference"}; 2086 case 3387378: /*note*/ return new String[] {"Annotation"}; 2087 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 2088 default: return super.getTypesForProperty(hash, name); 2089 } 2090 2091 } 2092 2093 @Override 2094 public Base addChild(String name) throws FHIRException { 2095 if (name.equals("identifier")) { 2096 return addIdentifier(); 2097 } 2098 else if (name.equals("instantiatesCanonical")) { 2099 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.instantiatesCanonical"); 2100 } 2101 else if (name.equals("instantiatesUri")) { 2102 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.instantiatesUri"); 2103 } 2104 else if (name.equals("basedOn")) { 2105 return addBasedOn(); 2106 } 2107 else if (name.equals("replaces")) { 2108 return addReplaces(); 2109 } 2110 else if (name.equals("groupIdentifier")) { 2111 this.groupIdentifier = new Identifier(); 2112 return this.groupIdentifier; 2113 } 2114 else if (name.equals("status")) { 2115 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.status"); 2116 } 2117 else if (name.equals("intent")) { 2118 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.intent"); 2119 } 2120 else if (name.equals("priority")) { 2121 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.priority"); 2122 } 2123 else if (name.equals("doNotPerform")) { 2124 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.doNotPerform"); 2125 } 2126 else if (name.equals("code")) { 2127 this.code = new CodeableReference(); 2128 return this.code; 2129 } 2130 else if (name.equals("quantity")) { 2131 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.quantity"); 2132 } 2133 else if (name.equals("parameter")) { 2134 return addParameter(); 2135 } 2136 else if (name.equals("subject")) { 2137 this.subject = new Reference(); 2138 return this.subject; 2139 } 2140 else if (name.equals("encounter")) { 2141 this.encounter = new Reference(); 2142 return this.encounter; 2143 } 2144 else if (name.equals("occurrenceDateTime")) { 2145 this.occurrence = new DateTimeType(); 2146 return this.occurrence; 2147 } 2148 else if (name.equals("occurrencePeriod")) { 2149 this.occurrence = new Period(); 2150 return this.occurrence; 2151 } 2152 else if (name.equals("occurrenceTiming")) { 2153 this.occurrence = new Timing(); 2154 return this.occurrence; 2155 } 2156 else if (name.equals("authoredOn")) { 2157 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.authoredOn"); 2158 } 2159 else if (name.equals("requester")) { 2160 this.requester = new Reference(); 2161 return this.requester; 2162 } 2163 else if (name.equals("performer")) { 2164 this.performer = new CodeableReference(); 2165 return this.performer; 2166 } 2167 else if (name.equals("reason")) { 2168 return addReason(); 2169 } 2170 else if (name.equals("asNeeded")) { 2171 throw new FHIRException("Cannot call addChild on a singleton property DeviceRequest.asNeeded"); 2172 } 2173 else if (name.equals("asNeededFor")) { 2174 this.asNeededFor = new CodeableConcept(); 2175 return this.asNeededFor; 2176 } 2177 else if (name.equals("insurance")) { 2178 return addInsurance(); 2179 } 2180 else if (name.equals("supportingInfo")) { 2181 return addSupportingInfo(); 2182 } 2183 else if (name.equals("note")) { 2184 return addNote(); 2185 } 2186 else if (name.equals("relevantHistory")) { 2187 return addRelevantHistory(); 2188 } 2189 else 2190 return super.addChild(name); 2191 } 2192 2193 public String fhirType() { 2194 return "DeviceRequest"; 2195 2196 } 2197 2198 public DeviceRequest copy() { 2199 DeviceRequest dst = new DeviceRequest(); 2200 copyValues(dst); 2201 return dst; 2202 } 2203 2204 public void copyValues(DeviceRequest dst) { 2205 super.copyValues(dst); 2206 if (identifier != null) { 2207 dst.identifier = new ArrayList<Identifier>(); 2208 for (Identifier i : identifier) 2209 dst.identifier.add(i.copy()); 2210 }; 2211 if (instantiatesCanonical != null) { 2212 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 2213 for (CanonicalType i : instantiatesCanonical) 2214 dst.instantiatesCanonical.add(i.copy()); 2215 }; 2216 if (instantiatesUri != null) { 2217 dst.instantiatesUri = new ArrayList<UriType>(); 2218 for (UriType i : instantiatesUri) 2219 dst.instantiatesUri.add(i.copy()); 2220 }; 2221 if (basedOn != null) { 2222 dst.basedOn = new ArrayList<Reference>(); 2223 for (Reference i : basedOn) 2224 dst.basedOn.add(i.copy()); 2225 }; 2226 if (replaces != null) { 2227 dst.replaces = new ArrayList<Reference>(); 2228 for (Reference i : replaces) 2229 dst.replaces.add(i.copy()); 2230 }; 2231 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2232 dst.status = status == null ? null : status.copy(); 2233 dst.intent = intent == null ? null : intent.copy(); 2234 dst.priority = priority == null ? null : priority.copy(); 2235 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 2236 dst.code = code == null ? null : code.copy(); 2237 dst.quantity = quantity == null ? null : quantity.copy(); 2238 if (parameter != null) { 2239 dst.parameter = new ArrayList<DeviceRequestParameterComponent>(); 2240 for (DeviceRequestParameterComponent i : parameter) 2241 dst.parameter.add(i.copy()); 2242 }; 2243 dst.subject = subject == null ? null : subject.copy(); 2244 dst.encounter = encounter == null ? null : encounter.copy(); 2245 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2246 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2247 dst.requester = requester == null ? null : requester.copy(); 2248 dst.performer = performer == null ? null : performer.copy(); 2249 if (reason != null) { 2250 dst.reason = new ArrayList<CodeableReference>(); 2251 for (CodeableReference i : reason) 2252 dst.reason.add(i.copy()); 2253 }; 2254 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 2255 dst.asNeededFor = asNeededFor == null ? null : asNeededFor.copy(); 2256 if (insurance != null) { 2257 dst.insurance = new ArrayList<Reference>(); 2258 for (Reference i : insurance) 2259 dst.insurance.add(i.copy()); 2260 }; 2261 if (supportingInfo != null) { 2262 dst.supportingInfo = new ArrayList<Reference>(); 2263 for (Reference i : supportingInfo) 2264 dst.supportingInfo.add(i.copy()); 2265 }; 2266 if (note != null) { 2267 dst.note = new ArrayList<Annotation>(); 2268 for (Annotation i : note) 2269 dst.note.add(i.copy()); 2270 }; 2271 if (relevantHistory != null) { 2272 dst.relevantHistory = new ArrayList<Reference>(); 2273 for (Reference i : relevantHistory) 2274 dst.relevantHistory.add(i.copy()); 2275 }; 2276 } 2277 2278 protected DeviceRequest typedCopy() { 2279 return copy(); 2280 } 2281 2282 @Override 2283 public boolean equalsDeep(Base other_) { 2284 if (!super.equalsDeep(other_)) 2285 return false; 2286 if (!(other_ instanceof DeviceRequest)) 2287 return false; 2288 DeviceRequest o = (DeviceRequest) other_; 2289 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 2290 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 2291 && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 2292 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 2293 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(code, o.code, true) && compareDeep(quantity, o.quantity, true) 2294 && compareDeep(parameter, o.parameter, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2295 && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 2296 && compareDeep(performer, o.performer, true) && compareDeep(reason, o.reason, true) && compareDeep(asNeeded, o.asNeeded, true) 2297 && compareDeep(asNeededFor, o.asNeededFor, true) && compareDeep(insurance, o.insurance, true) && compareDeep(supportingInfo, o.supportingInfo, true) 2298 && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true); 2299 } 2300 2301 @Override 2302 public boolean equalsShallow(Base other_) { 2303 if (!super.equalsShallow(other_)) 2304 return false; 2305 if (!(other_ instanceof DeviceRequest)) 2306 return false; 2307 DeviceRequest o = (DeviceRequest) other_; 2308 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 2309 && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 2310 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(quantity, o.quantity, true) && compareValues(authoredOn, o.authoredOn, true) 2311 && compareValues(asNeeded, o.asNeeded, true); 2312 } 2313 2314 public boolean isEmpty() { 2315 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 2316 , instantiatesUri, basedOn, replaces, groupIdentifier, status, intent, priority 2317 , doNotPerform, code, quantity, parameter, subject, encounter, occurrence, authoredOn 2318 , requester, performer, reason, asNeeded, asNeededFor, insurance, supportingInfo 2319 , note, relevantHistory); 2320 } 2321 2322 @Override 2323 public ResourceType getResourceType() { 2324 return ResourceType.DeviceRequest; 2325 } 2326 2327 /** 2328 * Search parameter: <b>authored-on</b> 2329 * <p> 2330 * Description: <b>When the request transitioned to being actionable</b><br> 2331 * Type: <b>date</b><br> 2332 * Path: <b>DeviceRequest.authoredOn</b><br> 2333 * </p> 2334 */ 2335 @SearchParamDefinition(name="authored-on", path="DeviceRequest.authoredOn", description="When the request transitioned to being actionable", type="date" ) 2336 public static final String SP_AUTHORED_ON = "authored-on"; 2337 /** 2338 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 2339 * <p> 2340 * Description: <b>When the request transitioned to being actionable</b><br> 2341 * Type: <b>date</b><br> 2342 * Path: <b>DeviceRequest.authoredOn</b><br> 2343 * </p> 2344 */ 2345 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED_ON); 2346 2347 /** 2348 * Search parameter: <b>based-on</b> 2349 * <p> 2350 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 2351 * Type: <b>reference</b><br> 2352 * Path: <b>DeviceRequest.basedOn</b><br> 2353 * </p> 2354 */ 2355 @SearchParamDefinition(name="based-on", path="DeviceRequest.basedOn", description="Plan/proposal/order fulfilled by this request", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2356 public static final String SP_BASED_ON = "based-on"; 2357 /** 2358 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2359 * <p> 2360 * Description: <b>Plan/proposal/order fulfilled by this request</b><br> 2361 * Type: <b>reference</b><br> 2362 * Path: <b>DeviceRequest.basedOn</b><br> 2363 * </p> 2364 */ 2365 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2366 2367/** 2368 * Constant for fluent queries to be used to add include statements. Specifies 2369 * the path value of "<b>DeviceRequest:based-on</b>". 2370 */ 2371 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("DeviceRequest:based-on").toLocked(); 2372 2373 /** 2374 * Search parameter: <b>device</b> 2375 * <p> 2376 * Description: <b>Reference to resource that is being requested/ordered</b><br> 2377 * Type: <b>reference</b><br> 2378 * Path: <b>DeviceRequest.code.reference</b><br> 2379 * </p> 2380 */ 2381 @SearchParamDefinition(name="device", path="DeviceRequest.code.reference", description="Reference to resource that is being requested/ordered", type="reference", target={Device.class, DeviceDefinition.class } ) 2382 public static final String SP_DEVICE = "device"; 2383 /** 2384 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2385 * <p> 2386 * Description: <b>Reference to resource that is being requested/ordered</b><br> 2387 * Type: <b>reference</b><br> 2388 * Path: <b>DeviceRequest.code.reference</b><br> 2389 * </p> 2390 */ 2391 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 2392 2393/** 2394 * Constant for fluent queries to be used to add include statements. Specifies 2395 * the path value of "<b>DeviceRequest:device</b>". 2396 */ 2397 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("DeviceRequest:device").toLocked(); 2398 2399 /** 2400 * Search parameter: <b>event-date</b> 2401 * <p> 2402 * Description: <b>When service should occur</b><br> 2403 * Type: <b>date</b><br> 2404 * Path: <b>(DeviceRequest.occurrence.ofType(dateTime)) | (DeviceRequest.occurrence.ofType(Period))</b><br> 2405 * </p> 2406 */ 2407 @SearchParamDefinition(name="event-date", path="(DeviceRequest.occurrence.ofType(dateTime)) | (DeviceRequest.occurrence.ofType(Period))", description="When service should occur", type="date" ) 2408 public static final String SP_EVENT_DATE = "event-date"; 2409 /** 2410 * <b>Fluent Client</b> search parameter constant for <b>event-date</b> 2411 * <p> 2412 * Description: <b>When service should occur</b><br> 2413 * Type: <b>date</b><br> 2414 * Path: <b>(DeviceRequest.occurrence.ofType(dateTime)) | (DeviceRequest.occurrence.ofType(Period))</b><br> 2415 * </p> 2416 */ 2417 public static final ca.uhn.fhir.rest.gclient.DateClientParam EVENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EVENT_DATE); 2418 2419 /** 2420 * Search parameter: <b>group-identifier</b> 2421 * <p> 2422 * Description: <b>Composite request this is part of</b><br> 2423 * Type: <b>token</b><br> 2424 * Path: <b>DeviceRequest.groupIdentifier</b><br> 2425 * </p> 2426 */ 2427 @SearchParamDefinition(name="group-identifier", path="DeviceRequest.groupIdentifier", description="Composite request this is part of", type="token" ) 2428 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 2429 /** 2430 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 2431 * <p> 2432 * Description: <b>Composite request this is part of</b><br> 2433 * Type: <b>token</b><br> 2434 * Path: <b>DeviceRequest.groupIdentifier</b><br> 2435 * </p> 2436 */ 2437 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 2438 2439 /** 2440 * Search parameter: <b>instantiates-canonical</b> 2441 * <p> 2442 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2443 * Type: <b>reference</b><br> 2444 * Path: <b>DeviceRequest.instantiatesCanonical</b><br> 2445 * </p> 2446 */ 2447 @SearchParamDefinition(name="instantiates-canonical", path="DeviceRequest.instantiatesCanonical", description="Instantiates FHIR protocol or definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 2448 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 2449 /** 2450 * <b>Fluent Client</b> search parameter constant for <b>instantiates-canonical</b> 2451 * <p> 2452 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2453 * Type: <b>reference</b><br> 2454 * Path: <b>DeviceRequest.instantiatesCanonical</b><br> 2455 * </p> 2456 */ 2457 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSTANTIATES_CANONICAL); 2458 2459/** 2460 * Constant for fluent queries to be used to add include statements. Specifies 2461 * the path value of "<b>DeviceRequest:instantiates-canonical</b>". 2462 */ 2463 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include("DeviceRequest:instantiates-canonical").toLocked(); 2464 2465 /** 2466 * Search parameter: <b>instantiates-uri</b> 2467 * <p> 2468 * Description: <b>Instantiates external protocol or definition</b><br> 2469 * Type: <b>uri</b><br> 2470 * Path: <b>DeviceRequest.instantiatesUri</b><br> 2471 * </p> 2472 */ 2473 @SearchParamDefinition(name="instantiates-uri", path="DeviceRequest.instantiatesUri", description="Instantiates external protocol or definition", type="uri" ) 2474 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 2475 /** 2476 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 2477 * <p> 2478 * Description: <b>Instantiates external protocol or definition</b><br> 2479 * Type: <b>uri</b><br> 2480 * Path: <b>DeviceRequest.instantiatesUri</b><br> 2481 * </p> 2482 */ 2483 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES_URI); 2484 2485 /** 2486 * Search parameter: <b>insurance</b> 2487 * <p> 2488 * Description: <b>Associated insurance coverage</b><br> 2489 * Type: <b>reference</b><br> 2490 * Path: <b>DeviceRequest.insurance</b><br> 2491 * </p> 2492 */ 2493 @SearchParamDefinition(name="insurance", path="DeviceRequest.insurance", description="Associated insurance coverage", type="reference", target={ClaimResponse.class, Coverage.class } ) 2494 public static final String SP_INSURANCE = "insurance"; 2495 /** 2496 * <b>Fluent Client</b> search parameter constant for <b>insurance</b> 2497 * <p> 2498 * Description: <b>Associated insurance coverage</b><br> 2499 * Type: <b>reference</b><br> 2500 * Path: <b>DeviceRequest.insurance</b><br> 2501 * </p> 2502 */ 2503 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURANCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURANCE); 2504 2505/** 2506 * Constant for fluent queries to be used to add include statements. Specifies 2507 * the path value of "<b>DeviceRequest:insurance</b>". 2508 */ 2509 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURANCE = new ca.uhn.fhir.model.api.Include("DeviceRequest:insurance").toLocked(); 2510 2511 /** 2512 * Search parameter: <b>intent</b> 2513 * <p> 2514 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 2515 * Type: <b>token</b><br> 2516 * Path: <b>DeviceRequest.intent</b><br> 2517 * </p> 2518 */ 2519 @SearchParamDefinition(name="intent", path="DeviceRequest.intent", description="proposal | plan | original-order |reflex-order", type="token" ) 2520 public static final String SP_INTENT = "intent"; 2521 /** 2522 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 2523 * <p> 2524 * Description: <b>proposal | plan | original-order |reflex-order</b><br> 2525 * Type: <b>token</b><br> 2526 * Path: <b>DeviceRequest.intent</b><br> 2527 * </p> 2528 */ 2529 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 2530 2531 /** 2532 * Search parameter: <b>performer-code</b> 2533 * <p> 2534 * Description: <b>Desired performer for service</b><br> 2535 * Type: <b>token</b><br> 2536 * Path: <b>DeviceRequest.performer.concept</b><br> 2537 * </p> 2538 */ 2539 @SearchParamDefinition(name="performer-code", path="DeviceRequest.performer.concept", description="Desired performer for service", type="token" ) 2540 public static final String SP_PERFORMER_CODE = "performer-code"; 2541 /** 2542 * <b>Fluent Client</b> search parameter constant for <b>performer-code</b> 2543 * <p> 2544 * Description: <b>Desired performer for service</b><br> 2545 * Type: <b>token</b><br> 2546 * Path: <b>DeviceRequest.performer.concept</b><br> 2547 * </p> 2548 */ 2549 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER_CODE); 2550 2551 /** 2552 * Search parameter: <b>performer</b> 2553 * <p> 2554 * Description: <b>Desired performer for service</b><br> 2555 * Type: <b>reference</b><br> 2556 * Path: <b>DeviceRequest.performer.reference</b><br> 2557 * </p> 2558 */ 2559 @SearchParamDefinition(name="performer", path="DeviceRequest.performer.reference", description="Desired performer for service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2560 public static final String SP_PERFORMER = "performer"; 2561 /** 2562 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2563 * <p> 2564 * Description: <b>Desired performer for service</b><br> 2565 * Type: <b>reference</b><br> 2566 * Path: <b>DeviceRequest.performer.reference</b><br> 2567 * </p> 2568 */ 2569 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2570 2571/** 2572 * Constant for fluent queries to be used to add include statements. Specifies 2573 * the path value of "<b>DeviceRequest:performer</b>". 2574 */ 2575 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("DeviceRequest:performer").toLocked(); 2576 2577 /** 2578 * Search parameter: <b>prior-request</b> 2579 * <p> 2580 * Description: <b>Request takes the place of referenced completed or terminated requests</b><br> 2581 * Type: <b>reference</b><br> 2582 * Path: <b>DeviceRequest.replaces</b><br> 2583 * </p> 2584 */ 2585 @SearchParamDefinition(name="prior-request", path="DeviceRequest.replaces", description="Request takes the place of referenced completed or terminated requests", type="reference", target={DeviceRequest.class } ) 2586 public static final String SP_PRIOR_REQUEST = "prior-request"; 2587 /** 2588 * <b>Fluent Client</b> search parameter constant for <b>prior-request</b> 2589 * <p> 2590 * Description: <b>Request takes the place of referenced completed or terminated requests</b><br> 2591 * Type: <b>reference</b><br> 2592 * Path: <b>DeviceRequest.replaces</b><br> 2593 * </p> 2594 */ 2595 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRIOR_REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRIOR_REQUEST); 2596 2597/** 2598 * Constant for fluent queries to be used to add include statements. Specifies 2599 * the path value of "<b>DeviceRequest:prior-request</b>". 2600 */ 2601 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRIOR_REQUEST = new ca.uhn.fhir.model.api.Include("DeviceRequest:prior-request").toLocked(); 2602 2603 /** 2604 * Search parameter: <b>requester</b> 2605 * <p> 2606 * Description: <b>Who/what is requesting service</b><br> 2607 * Type: <b>reference</b><br> 2608 * Path: <b>DeviceRequest.requester</b><br> 2609 * </p> 2610 */ 2611 @SearchParamDefinition(name="requester", path="DeviceRequest.requester", description="Who/what is requesting service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Practitioner.class, PractitionerRole.class } ) 2612 public static final String SP_REQUESTER = "requester"; 2613 /** 2614 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2615 * <p> 2616 * Description: <b>Who/what is requesting service</b><br> 2617 * Type: <b>reference</b><br> 2618 * Path: <b>DeviceRequest.requester</b><br> 2619 * </p> 2620 */ 2621 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 2622 2623/** 2624 * Constant for fluent queries to be used to add include statements. Specifies 2625 * the path value of "<b>DeviceRequest:requester</b>". 2626 */ 2627 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("DeviceRequest:requester").toLocked(); 2628 2629 /** 2630 * Search parameter: <b>status</b> 2631 * <p> 2632 * Description: <b>entered-in-error | draft | active |suspended | completed</b><br> 2633 * Type: <b>token</b><br> 2634 * Path: <b>DeviceRequest.status</b><br> 2635 * </p> 2636 */ 2637 @SearchParamDefinition(name="status", path="DeviceRequest.status", description="entered-in-error | draft | active |suspended | completed", type="token" ) 2638 public static final String SP_STATUS = "status"; 2639 /** 2640 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2641 * <p> 2642 * Description: <b>entered-in-error | draft | active |suspended | completed</b><br> 2643 * Type: <b>token</b><br> 2644 * Path: <b>DeviceRequest.status</b><br> 2645 * </p> 2646 */ 2647 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2648 2649 /** 2650 * Search parameter: <b>subject</b> 2651 * <p> 2652 * Description: <b>Individual the service is ordered for</b><br> 2653 * Type: <b>reference</b><br> 2654 * Path: <b>DeviceRequest.subject</b><br> 2655 * </p> 2656 */ 2657 @SearchParamDefinition(name="subject", path="DeviceRequest.subject", description="Individual the service is ordered for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 2658 public static final String SP_SUBJECT = "subject"; 2659 /** 2660 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2661 * <p> 2662 * Description: <b>Individual the service is ordered for</b><br> 2663 * Type: <b>reference</b><br> 2664 * Path: <b>DeviceRequest.subject</b><br> 2665 * </p> 2666 */ 2667 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2668 2669/** 2670 * Constant for fluent queries to be used to add include statements. Specifies 2671 * the path value of "<b>DeviceRequest:subject</b>". 2672 */ 2673 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DeviceRequest:subject").toLocked(); 2674 2675 /** 2676 * Search parameter: <b>code</b> 2677 * <p> 2678 * Description: <b>Multiple Resources: 2679 2680* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2681* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2682* [AuditEvent](auditevent.html): More specific code for the event 2683* [Basic](basic.html): Kind of Resource 2684* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2685* [Condition](condition.html): Code for the condition 2686* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2687* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2688* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2689* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2690* [ImagingSelection](imagingselection.html): The imaging selection status 2691* [List](list.html): What the purpose of this list is 2692* [Medication](medication.html): Returns medications for a specific code 2693* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2694* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2695* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2696* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2697* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2698* [Observation](observation.html): The code of the observation type 2699* [Procedure](procedure.html): A code to identify a procedure 2700* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2701* [Task](task.html): Search by task code 2702</b><br> 2703 * Type: <b>token</b><br> 2704 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2705 * </p> 2706 */ 2707 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2708 public static final String SP_CODE = "code"; 2709 /** 2710 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2711 * <p> 2712 * Description: <b>Multiple Resources: 2713 2714* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2715* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2716* [AuditEvent](auditevent.html): More specific code for the event 2717* [Basic](basic.html): Kind of Resource 2718* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2719* [Condition](condition.html): Code for the condition 2720* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2721* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2722* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2723* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2724* [ImagingSelection](imagingselection.html): The imaging selection status 2725* [List](list.html): What the purpose of this list is 2726* [Medication](medication.html): Returns medications for a specific code 2727* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2728* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2729* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2730* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2731* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2732* [Observation](observation.html): The code of the observation type 2733* [Procedure](procedure.html): A code to identify a procedure 2734* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2735* [Task](task.html): Search by task code 2736</b><br> 2737 * Type: <b>token</b><br> 2738 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2739 * </p> 2740 */ 2741 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2742 2743 /** 2744 * Search parameter: <b>encounter</b> 2745 * <p> 2746 * Description: <b>Multiple Resources: 2747 2748* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2749* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2750* [ChargeItem](chargeitem.html): Encounter associated with event 2751* [Claim](claim.html): Encounters associated with a billed line item 2752* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2753* [Communication](communication.html): The Encounter during which this Communication was created 2754* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2755* [Composition](composition.html): Context of the Composition 2756* [Condition](condition.html): The Encounter during which this Condition was created 2757* [DeviceRequest](devicerequest.html): Encounter during which request was created 2758* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2759* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2760* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2761* [Flag](flag.html): Alert relevant during encounter 2762* [ImagingStudy](imagingstudy.html): The context of the study 2763* [List](list.html): Context in which list created 2764* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2765* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2766* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2767* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2768* [Observation](observation.html): Encounter related to the observation 2769* [Procedure](procedure.html): The Encounter during which this Procedure was created 2770* [Provenance](provenance.html): Encounter related to the Provenance 2771* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2772* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2773* [RiskAssessment](riskassessment.html): Where was assessment performed? 2774* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2775* [Task](task.html): Search by encounter 2776* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2777</b><br> 2778 * Type: <b>reference</b><br> 2779 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2780 * </p> 2781 */ 2782 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2783 public static final String SP_ENCOUNTER = "encounter"; 2784 /** 2785 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2786 * <p> 2787 * Description: <b>Multiple Resources: 2788 2789* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2790* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2791* [ChargeItem](chargeitem.html): Encounter associated with event 2792* [Claim](claim.html): Encounters associated with a billed line item 2793* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2794* [Communication](communication.html): The Encounter during which this Communication was created 2795* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2796* [Composition](composition.html): Context of the Composition 2797* [Condition](condition.html): The Encounter during which this Condition was created 2798* [DeviceRequest](devicerequest.html): Encounter during which request was created 2799* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2800* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2801* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2802* [Flag](flag.html): Alert relevant during encounter 2803* [ImagingStudy](imagingstudy.html): The context of the study 2804* [List](list.html): Context in which list created 2805* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2806* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2807* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2808* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2809* [Observation](observation.html): Encounter related to the observation 2810* [Procedure](procedure.html): The Encounter during which this Procedure was created 2811* [Provenance](provenance.html): Encounter related to the Provenance 2812* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2813* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2814* [RiskAssessment](riskassessment.html): Where was assessment performed? 2815* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2816* [Task](task.html): Search by encounter 2817* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2818</b><br> 2819 * Type: <b>reference</b><br> 2820 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2821 * </p> 2822 */ 2823 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2824 2825/** 2826 * Constant for fluent queries to be used to add include statements. Specifies 2827 * the path value of "<b>DeviceRequest:encounter</b>". 2828 */ 2829 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("DeviceRequest:encounter").toLocked(); 2830 2831 /** 2832 * Search parameter: <b>identifier</b> 2833 * <p> 2834 * Description: <b>Multiple Resources: 2835 2836* [Account](account.html): Account number 2837* [AdverseEvent](adverseevent.html): Business identifier for the event 2838* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2839* [Appointment](appointment.html): An Identifier of the Appointment 2840* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2841* [Basic](basic.html): Business identifier 2842* [BodyStructure](bodystructure.html): Bodystructure identifier 2843* [CarePlan](careplan.html): External Ids for this plan 2844* [CareTeam](careteam.html): External Ids for this team 2845* [ChargeItem](chargeitem.html): Business Identifier for item 2846* [Claim](claim.html): The primary identifier of the financial resource 2847* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2848* [ClinicalImpression](clinicalimpression.html): Business identifier 2849* [Communication](communication.html): Unique identifier 2850* [CommunicationRequest](communicationrequest.html): Unique identifier 2851* [Composition](composition.html): Version-independent identifier for the Composition 2852* [Condition](condition.html): A unique identifier of the condition record 2853* [Consent](consent.html): Identifier for this record (external references) 2854* [Contract](contract.html): The identity of the contract 2855* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2856* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2857* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2858* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2859* [DeviceRequest](devicerequest.html): Business identifier for request/order 2860* [DeviceUsage](deviceusage.html): Search by identifier 2861* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2862* [DocumentReference](documentreference.html): Identifier of the attachment binary 2863* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2864* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2865* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2866* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2867* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2868* [Flag](flag.html): Business identifier 2869* [Goal](goal.html): External Ids for this goal 2870* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2871* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2872* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2873* [Immunization](immunization.html): Business identifier 2874* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2875* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2876* [Invoice](invoice.html): Business Identifier for item 2877* [List](list.html): Business identifier 2878* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2879* [Medication](medication.html): Returns medications with this external identifier 2880* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2881* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2882* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2883* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2884* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2885* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2886* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2887* [Observation](observation.html): The unique id for a particular observation 2888* [Person](person.html): A person Identifier 2889* [Procedure](procedure.html): A unique identifier for a procedure 2890* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2891* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2892* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2893* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2894* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2895* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2896* [Specimen](specimen.html): The unique identifier associated with the specimen 2897* [SupplyDelivery](supplydelivery.html): External identifier 2898* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2899* [Task](task.html): Search for a task instance by its business identifier 2900* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2901</b><br> 2902 * Type: <b>token</b><br> 2903 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2904 * </p> 2905 */ 2906 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2907 public static final String SP_IDENTIFIER = "identifier"; 2908 /** 2909 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2910 * <p> 2911 * Description: <b>Multiple Resources: 2912 2913* [Account](account.html): Account number 2914* [AdverseEvent](adverseevent.html): Business identifier for the event 2915* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2916* [Appointment](appointment.html): An Identifier of the Appointment 2917* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2918* [Basic](basic.html): Business identifier 2919* [BodyStructure](bodystructure.html): Bodystructure identifier 2920* [CarePlan](careplan.html): External Ids for this plan 2921* [CareTeam](careteam.html): External Ids for this team 2922* [ChargeItem](chargeitem.html): Business Identifier for item 2923* [Claim](claim.html): The primary identifier of the financial resource 2924* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2925* [ClinicalImpression](clinicalimpression.html): Business identifier 2926* [Communication](communication.html): Unique identifier 2927* [CommunicationRequest](communicationrequest.html): Unique identifier 2928* [Composition](composition.html): Version-independent identifier for the Composition 2929* [Condition](condition.html): A unique identifier of the condition record 2930* [Consent](consent.html): Identifier for this record (external references) 2931* [Contract](contract.html): The identity of the contract 2932* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2933* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2934* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2935* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2936* [DeviceRequest](devicerequest.html): Business identifier for request/order 2937* [DeviceUsage](deviceusage.html): Search by identifier 2938* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2939* [DocumentReference](documentreference.html): Identifier of the attachment binary 2940* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2941* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2942* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2943* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2944* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2945* [Flag](flag.html): Business identifier 2946* [Goal](goal.html): External Ids for this goal 2947* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2948* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2949* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2950* [Immunization](immunization.html): Business identifier 2951* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2952* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2953* [Invoice](invoice.html): Business Identifier for item 2954* [List](list.html): Business identifier 2955* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2956* [Medication](medication.html): Returns medications with this external identifier 2957* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2958* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2959* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2960* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2961* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2962* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2963* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2964* [Observation](observation.html): The unique id for a particular observation 2965* [Person](person.html): A person Identifier 2966* [Procedure](procedure.html): A unique identifier for a procedure 2967* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2968* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2969* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2970* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2971* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2972* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2973* [Specimen](specimen.html): The unique identifier associated with the specimen 2974* [SupplyDelivery](supplydelivery.html): External identifier 2975* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2976* [Task](task.html): Search for a task instance by its business identifier 2977* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2978</b><br> 2979 * Type: <b>token</b><br> 2980 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2981 * </p> 2982 */ 2983 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2984 2985 /** 2986 * Search parameter: <b>patient</b> 2987 * <p> 2988 * Description: <b>Multiple Resources: 2989 2990* [Account](account.html): The entity that caused the expenses 2991* [AdverseEvent](adverseevent.html): Subject impacted by event 2992* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2993* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2994* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2995* [AuditEvent](auditevent.html): Where the activity involved patient data 2996* [Basic](basic.html): Identifies the focus of this resource 2997* [BodyStructure](bodystructure.html): Who this is about 2998* [CarePlan](careplan.html): Who the care plan is for 2999* [CareTeam](careteam.html): Who care team is for 3000* [ChargeItem](chargeitem.html): Individual service was done for/to 3001* [Claim](claim.html): Patient receiving the products or services 3002* [ClaimResponse](claimresponse.html): The subject of care 3003* [ClinicalImpression](clinicalimpression.html): Patient assessed 3004* [Communication](communication.html): Focus of message 3005* [CommunicationRequest](communicationrequest.html): Focus of message 3006* [Composition](composition.html): Who and/or what the composition is about 3007* [Condition](condition.html): Who has the condition? 3008* [Consent](consent.html): Who the consent applies to 3009* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3010* [Coverage](coverage.html): Retrieve coverages for a patient 3011* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3012* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3013* [DetectedIssue](detectedissue.html): Associated patient 3014* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3015* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3016* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3017* [DocumentReference](documentreference.html): Who/what is the subject of the document 3018* [Encounter](encounter.html): The patient present at the encounter 3019* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3020* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3021* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3022* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3023* [Flag](flag.html): The identity of a subject to list flags for 3024* [Goal](goal.html): Who this goal is intended for 3025* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3026* [ImagingSelection](imagingselection.html): Who the study is about 3027* [ImagingStudy](imagingstudy.html): Who the study is about 3028* [Immunization](immunization.html): The patient for the vaccination record 3029* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3030* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3031* [Invoice](invoice.html): Recipient(s) of goods and services 3032* [List](list.html): If all resources have the same subject 3033* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3034* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3035* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3036* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3037* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3038* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3039* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3040* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3041* [Observation](observation.html): The subject that the observation is about (if patient) 3042* [Person](person.html): The Person links to this Patient 3043* [Procedure](procedure.html): Search by subject - a patient 3044* [Provenance](provenance.html): Where the activity involved patient data 3045* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3046* [RelatedPerson](relatedperson.html): The patient this related person is related to 3047* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3048* [ResearchSubject](researchsubject.html): Who or what is part of study 3049* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3050* [ServiceRequest](servicerequest.html): Search by subject - a patient 3051* [Specimen](specimen.html): The patient the specimen comes from 3052* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3053* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3054* [Task](task.html): Search by patient 3055* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3056</b><br> 3057 * Type: <b>reference</b><br> 3058 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3059 * </p> 3060 */ 3061 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3062 public static final String SP_PATIENT = "patient"; 3063 /** 3064 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3065 * <p> 3066 * Description: <b>Multiple Resources: 3067 3068* [Account](account.html): The entity that caused the expenses 3069* [AdverseEvent](adverseevent.html): Subject impacted by event 3070* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3071* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3072* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3073* [AuditEvent](auditevent.html): Where the activity involved patient data 3074* [Basic](basic.html): Identifies the focus of this resource 3075* [BodyStructure](bodystructure.html): Who this is about 3076* [CarePlan](careplan.html): Who the care plan is for 3077* [CareTeam](careteam.html): Who care team is for 3078* [ChargeItem](chargeitem.html): Individual service was done for/to 3079* [Claim](claim.html): Patient receiving the products or services 3080* [ClaimResponse](claimresponse.html): The subject of care 3081* [ClinicalImpression](clinicalimpression.html): Patient assessed 3082* [Communication](communication.html): Focus of message 3083* [CommunicationRequest](communicationrequest.html): Focus of message 3084* [Composition](composition.html): Who and/or what the composition is about 3085* [Condition](condition.html): Who has the condition? 3086* [Consent](consent.html): Who the consent applies to 3087* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3088* [Coverage](coverage.html): Retrieve coverages for a patient 3089* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3090* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3091* [DetectedIssue](detectedissue.html): Associated patient 3092* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3093* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3094* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3095* [DocumentReference](documentreference.html): Who/what is the subject of the document 3096* [Encounter](encounter.html): The patient present at the encounter 3097* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3098* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3099* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3100* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3101* [Flag](flag.html): The identity of a subject to list flags for 3102* [Goal](goal.html): Who this goal is intended for 3103* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3104* [ImagingSelection](imagingselection.html): Who the study is about 3105* [ImagingStudy](imagingstudy.html): Who the study is about 3106* [Immunization](immunization.html): The patient for the vaccination record 3107* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3108* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3109* [Invoice](invoice.html): Recipient(s) of goods and services 3110* [List](list.html): If all resources have the same subject 3111* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3112* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3113* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3114* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3115* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3116* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3117* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3118* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3119* [Observation](observation.html): The subject that the observation is about (if patient) 3120* [Person](person.html): The Person links to this Patient 3121* [Procedure](procedure.html): Search by subject - a patient 3122* [Provenance](provenance.html): Where the activity involved patient data 3123* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3124* [RelatedPerson](relatedperson.html): The patient this related person is related to 3125* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3126* [ResearchSubject](researchsubject.html): Who or what is part of study 3127* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3128* [ServiceRequest](servicerequest.html): Search by subject - a patient 3129* [Specimen](specimen.html): The patient the specimen comes from 3130* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3131* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3132* [Task](task.html): Search by patient 3133* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3134</b><br> 3135 * Type: <b>reference</b><br> 3136 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3137 * </p> 3138 */ 3139 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3140 3141/** 3142 * Constant for fluent queries to be used to add include statements. Specifies 3143 * the path value of "<b>DeviceRequest:patient</b>". 3144 */ 3145 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceRequest:patient").toLocked(); 3146 3147 3148} 3149