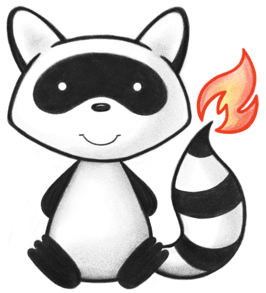
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a device being used by a patient where the record is the result of a report from the patient or a clinician. 052 */ 053@ResourceDef(name="DeviceUsage", profile="http://hl7.org/fhir/StructureDefinition/DeviceUsage") 054public class DeviceUsage extends DomainResource { 055 056 public enum DeviceUsageStatus { 057 /** 058 * The device is still being used. 059 */ 060 ACTIVE, 061 /** 062 * The device is no longer being used. 063 */ 064 COMPLETED, 065 /** 066 * The device was not used. 067 */ 068 NOTDONE, 069 /** 070 * The statement was recorded incorrectly. 071 */ 072 ENTEREDINERROR, 073 /** 074 * The device may be used at some time in the future. 075 */ 076 INTENDED, 077 /** 078 * Actions implied by the statement have been permanently halted, before all of them occurred. 079 */ 080 STOPPED, 081 /** 082 * Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called \"suspended\". 083 */ 084 ONHOLD, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static DeviceUsageStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("completed".equals(codeString)) 095 return COMPLETED; 096 if ("not-done".equals(codeString)) 097 return NOTDONE; 098 if ("entered-in-error".equals(codeString)) 099 return ENTEREDINERROR; 100 if ("intended".equals(codeString)) 101 return INTENDED; 102 if ("stopped".equals(codeString)) 103 return STOPPED; 104 if ("on-hold".equals(codeString)) 105 return ONHOLD; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown DeviceUsageStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case ACTIVE: return "active"; 114 case COMPLETED: return "completed"; 115 case NOTDONE: return "not-done"; 116 case ENTEREDINERROR: return "entered-in-error"; 117 case INTENDED: return "intended"; 118 case STOPPED: return "stopped"; 119 case ONHOLD: return "on-hold"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case ACTIVE: return "http://hl7.org/fhir/deviceusage-status"; 127 case COMPLETED: return "http://hl7.org/fhir/deviceusage-status"; 128 case NOTDONE: return "http://hl7.org/fhir/deviceusage-status"; 129 case ENTEREDINERROR: return "http://hl7.org/fhir/deviceusage-status"; 130 case INTENDED: return "http://hl7.org/fhir/deviceusage-status"; 131 case STOPPED: return "http://hl7.org/fhir/deviceusage-status"; 132 case ONHOLD: return "http://hl7.org/fhir/deviceusage-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case ACTIVE: return "The device is still being used."; 140 case COMPLETED: return "The device is no longer being used."; 141 case NOTDONE: return "The device was not used."; 142 case ENTEREDINERROR: return "The statement was recorded incorrectly."; 143 case INTENDED: return "The device may be used at some time in the future."; 144 case STOPPED: return "Actions implied by the statement have been permanently halted, before all of them occurred."; 145 case ONHOLD: return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case ACTIVE: return "Active"; 153 case COMPLETED: return "Completed"; 154 case NOTDONE: return "Not done"; 155 case ENTEREDINERROR: return "Entered in Error"; 156 case INTENDED: return "Intended"; 157 case STOPPED: return "Stopped"; 158 case ONHOLD: return "On Hold"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class DeviceUsageStatusEnumFactory implements EnumFactory<DeviceUsageStatus> { 166 public DeviceUsageStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("active".equals(codeString)) 171 return DeviceUsageStatus.ACTIVE; 172 if ("completed".equals(codeString)) 173 return DeviceUsageStatus.COMPLETED; 174 if ("not-done".equals(codeString)) 175 return DeviceUsageStatus.NOTDONE; 176 if ("entered-in-error".equals(codeString)) 177 return DeviceUsageStatus.ENTEREDINERROR; 178 if ("intended".equals(codeString)) 179 return DeviceUsageStatus.INTENDED; 180 if ("stopped".equals(codeString)) 181 return DeviceUsageStatus.STOPPED; 182 if ("on-hold".equals(codeString)) 183 return DeviceUsageStatus.ONHOLD; 184 throw new IllegalArgumentException("Unknown DeviceUsageStatus code '"+codeString+"'"); 185 } 186 public Enumeration<DeviceUsageStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.NULL, code); 194 if ("active".equals(codeString)) 195 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.ACTIVE, code); 196 if ("completed".equals(codeString)) 197 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.COMPLETED, code); 198 if ("not-done".equals(codeString)) 199 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.NOTDONE, code); 200 if ("entered-in-error".equals(codeString)) 201 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.ENTEREDINERROR, code); 202 if ("intended".equals(codeString)) 203 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.INTENDED, code); 204 if ("stopped".equals(codeString)) 205 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.STOPPED, code); 206 if ("on-hold".equals(codeString)) 207 return new Enumeration<DeviceUsageStatus>(this, DeviceUsageStatus.ONHOLD, code); 208 throw new FHIRException("Unknown DeviceUsageStatus code '"+codeString+"'"); 209 } 210 public String toCode(DeviceUsageStatus code) { 211 if (code == DeviceUsageStatus.NULL) 212 return null; 213 if (code == DeviceUsageStatus.ACTIVE) 214 return "active"; 215 if (code == DeviceUsageStatus.COMPLETED) 216 return "completed"; 217 if (code == DeviceUsageStatus.NOTDONE) 218 return "not-done"; 219 if (code == DeviceUsageStatus.ENTEREDINERROR) 220 return "entered-in-error"; 221 if (code == DeviceUsageStatus.INTENDED) 222 return "intended"; 223 if (code == DeviceUsageStatus.STOPPED) 224 return "stopped"; 225 if (code == DeviceUsageStatus.ONHOLD) 226 return "on-hold"; 227 return "?"; 228 } 229 public String toSystem(DeviceUsageStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 @Block() 235 public static class DeviceUsageAdherenceComponent extends BackboneElement implements IBaseBackboneElement { 236 /** 237 * Type of adherence. 238 */ 239 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 240 @Description(shortDefinition="always | never | sometimes", formalDefinition="Type of adherence." ) 241 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceusage-adherence-code") 242 protected CodeableConcept code; 243 244 /** 245 * Reason for adherence type. 246 */ 247 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 248 @Description(shortDefinition="lost | stolen | prescribed | broken | burned | forgot", formalDefinition="Reason for adherence type." ) 249 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceusage-adherence-reason") 250 protected List<CodeableConcept> reason; 251 252 private static final long serialVersionUID = -1932336797L; 253 254 /** 255 * Constructor 256 */ 257 public DeviceUsageAdherenceComponent() { 258 super(); 259 } 260 261 /** 262 * Constructor 263 */ 264 public DeviceUsageAdherenceComponent(CodeableConcept code, CodeableConcept reason) { 265 super(); 266 this.setCode(code); 267 this.addReason(reason); 268 } 269 270 /** 271 * @return {@link #code} (Type of adherence.) 272 */ 273 public CodeableConcept getCode() { 274 if (this.code == null) 275 if (Configuration.errorOnAutoCreate()) 276 throw new Error("Attempt to auto-create DeviceUsageAdherenceComponent.code"); 277 else if (Configuration.doAutoCreate()) 278 this.code = new CodeableConcept(); // cc 279 return this.code; 280 } 281 282 public boolean hasCode() { 283 return this.code != null && !this.code.isEmpty(); 284 } 285 286 /** 287 * @param value {@link #code} (Type of adherence.) 288 */ 289 public DeviceUsageAdherenceComponent setCode(CodeableConcept value) { 290 this.code = value; 291 return this; 292 } 293 294 /** 295 * @return {@link #reason} (Reason for adherence type.) 296 */ 297 public List<CodeableConcept> getReason() { 298 if (this.reason == null) 299 this.reason = new ArrayList<CodeableConcept>(); 300 return this.reason; 301 } 302 303 /** 304 * @return Returns a reference to <code>this</code> for easy method chaining 305 */ 306 public DeviceUsageAdherenceComponent setReason(List<CodeableConcept> theReason) { 307 this.reason = theReason; 308 return this; 309 } 310 311 public boolean hasReason() { 312 if (this.reason == null) 313 return false; 314 for (CodeableConcept item : this.reason) 315 if (!item.isEmpty()) 316 return true; 317 return false; 318 } 319 320 public CodeableConcept addReason() { //3 321 CodeableConcept t = new CodeableConcept(); 322 if (this.reason == null) 323 this.reason = new ArrayList<CodeableConcept>(); 324 this.reason.add(t); 325 return t; 326 } 327 328 public DeviceUsageAdherenceComponent addReason(CodeableConcept t) { //3 329 if (t == null) 330 return this; 331 if (this.reason == null) 332 this.reason = new ArrayList<CodeableConcept>(); 333 this.reason.add(t); 334 return this; 335 } 336 337 /** 338 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 339 */ 340 public CodeableConcept getReasonFirstRep() { 341 if (getReason().isEmpty()) { 342 addReason(); 343 } 344 return getReason().get(0); 345 } 346 347 protected void listChildren(List<Property> children) { 348 super.listChildren(children); 349 children.add(new Property("code", "CodeableConcept", "Type of adherence.", 0, 1, code)); 350 children.add(new Property("reason", "CodeableConcept", "Reason for adherence type.", 0, java.lang.Integer.MAX_VALUE, reason)); 351 } 352 353 @Override 354 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 355 switch (_hash) { 356 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Type of adherence.", 0, 1, code); 357 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Reason for adherence type.", 0, java.lang.Integer.MAX_VALUE, reason); 358 default: return super.getNamedProperty(_hash, _name, _checkValid); 359 } 360 361 } 362 363 @Override 364 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 365 switch (hash) { 366 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 367 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 368 default: return super.getProperty(hash, name, checkValid); 369 } 370 371 } 372 373 @Override 374 public Base setProperty(int hash, String name, Base value) throws FHIRException { 375 switch (hash) { 376 case 3059181: // code 377 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 378 return value; 379 case -934964668: // reason 380 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 381 return value; 382 default: return super.setProperty(hash, name, value); 383 } 384 385 } 386 387 @Override 388 public Base setProperty(String name, Base value) throws FHIRException { 389 if (name.equals("code")) { 390 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 391 } else if (name.equals("reason")) { 392 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); 393 } else 394 return super.setProperty(name, value); 395 return value; 396 } 397 398 @Override 399 public void removeChild(String name, Base value) throws FHIRException { 400 if (name.equals("code")) { 401 this.code = null; 402 } else if (name.equals("reason")) { 403 this.getReason().remove(value); 404 } else 405 super.removeChild(name, value); 406 407 } 408 409 @Override 410 public Base makeProperty(int hash, String name) throws FHIRException { 411 switch (hash) { 412 case 3059181: return getCode(); 413 case -934964668: return addReason(); 414 default: return super.makeProperty(hash, name); 415 } 416 417 } 418 419 @Override 420 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 421 switch (hash) { 422 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 423 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 424 default: return super.getTypesForProperty(hash, name); 425 } 426 427 } 428 429 @Override 430 public Base addChild(String name) throws FHIRException { 431 if (name.equals("code")) { 432 this.code = new CodeableConcept(); 433 return this.code; 434 } 435 else if (name.equals("reason")) { 436 return addReason(); 437 } 438 else 439 return super.addChild(name); 440 } 441 442 public DeviceUsageAdherenceComponent copy() { 443 DeviceUsageAdherenceComponent dst = new DeviceUsageAdherenceComponent(); 444 copyValues(dst); 445 return dst; 446 } 447 448 public void copyValues(DeviceUsageAdherenceComponent dst) { 449 super.copyValues(dst); 450 dst.code = code == null ? null : code.copy(); 451 if (reason != null) { 452 dst.reason = new ArrayList<CodeableConcept>(); 453 for (CodeableConcept i : reason) 454 dst.reason.add(i.copy()); 455 }; 456 } 457 458 @Override 459 public boolean equalsDeep(Base other_) { 460 if (!super.equalsDeep(other_)) 461 return false; 462 if (!(other_ instanceof DeviceUsageAdherenceComponent)) 463 return false; 464 DeviceUsageAdherenceComponent o = (DeviceUsageAdherenceComponent) other_; 465 return compareDeep(code, o.code, true) && compareDeep(reason, o.reason, true); 466 } 467 468 @Override 469 public boolean equalsShallow(Base other_) { 470 if (!super.equalsShallow(other_)) 471 return false; 472 if (!(other_ instanceof DeviceUsageAdherenceComponent)) 473 return false; 474 DeviceUsageAdherenceComponent o = (DeviceUsageAdherenceComponent) other_; 475 return true; 476 } 477 478 public boolean isEmpty() { 479 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, reason); 480 } 481 482 public String fhirType() { 483 return "DeviceUsage.adherence"; 484 485 } 486 487 } 488 489 /** 490 * An external identifier for this statement such as an IRI. 491 */ 492 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 493 @Description(shortDefinition="External identifier for this record", formalDefinition="An external identifier for this statement such as an IRI." ) 494 protected List<Identifier> identifier; 495 496 /** 497 * A plan, proposal or order that is fulfilled in whole or in part by this DeviceUsage. 498 */ 499 @Child(name = "basedOn", type = {ServiceRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 500 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this DeviceUsage." ) 501 protected List<Reference> basedOn; 502 503 /** 504 * A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed. 505 */ 506 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 507 @Description(shortDefinition="active | completed | not-done | entered-in-error +", formalDefinition="A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed." ) 508 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceusage-status") 509 protected Enumeration<DeviceUsageStatus> status; 510 511 /** 512 * This attribute indicates a category for the statement - The device statement may be made in an inpatient or outpatient settting (inpatient | outpatient | community | patientspecified). 513 */ 514 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 515 @Description(shortDefinition="The category of the statement - classifying how the statement is made", formalDefinition="This attribute indicates a category for the statement - The device statement may be made in an inpatient or outpatient settting (inpatient | outpatient | community | patientspecified)." ) 516 protected List<CodeableConcept> category; 517 518 /** 519 * The patient who used the device. 520 */ 521 @Child(name = "patient", type = {Patient.class}, order=4, min=1, max=1, modifier=false, summary=true) 522 @Description(shortDefinition="Patient using device", formalDefinition="The patient who used the device." ) 523 protected Reference patient; 524 525 /** 526 * Allows linking the DeviceUsage to the underlying Request, or to other information that supports or is used to derive the DeviceUsage. 527 */ 528 @Child(name = "derivedFrom", type = {ServiceRequest.class, Procedure.class, Claim.class, Observation.class, QuestionnaireResponse.class, DocumentReference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 529 @Description(shortDefinition="Supporting information", formalDefinition="Allows linking the DeviceUsage to the underlying Request, or to other information that supports or is used to derive the DeviceUsage." ) 530 protected List<Reference> derivedFrom; 531 532 /** 533 * The encounter or episode of care that establishes the context for this device use statement. 534 */ 535 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=true) 536 @Description(shortDefinition="The encounter or episode of care that establishes the context for this device use statement", formalDefinition="The encounter or episode of care that establishes the context for this device use statement." ) 537 protected Reference context; 538 539 /** 540 * How often the device was used. 541 */ 542 @Child(name = "timing", type = {Timing.class, Period.class, DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 543 @Description(shortDefinition="How often the device was used", formalDefinition="How often the device was used." ) 544 protected DataType timing; 545 546 /** 547 * The time at which the statement was recorded by informationSource. 548 */ 549 @Child(name = "dateAsserted", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 550 @Description(shortDefinition="When the statement was made (and recorded)", formalDefinition="The time at which the statement was recorded by informationSource." ) 551 protected DateTimeType dateAsserted; 552 553 /** 554 * The status of the device usage, for example always, sometimes, never. This is not the same as the status of the statement. 555 */ 556 @Child(name = "usageStatus", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 557 @Description(shortDefinition="The status of the device usage, for example always, sometimes, never. This is not the same as the status of the statement", formalDefinition="The status of the device usage, for example always, sometimes, never. This is not the same as the status of the statement." ) 558 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/deviceusage-status") 559 protected CodeableConcept usageStatus; 560 561 /** 562 * The reason for asserting the usage status - for example forgot, lost, stolen, broken. 563 */ 564 @Child(name = "usageReason", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 565 @Description(shortDefinition="The reason for asserting the usage status - for example forgot, lost, stolen, broken", formalDefinition="The reason for asserting the usage status - for example forgot, lost, stolen, broken." ) 566 protected List<CodeableConcept> usageReason; 567 568 /** 569 * This indicates how or if the device is being used. 570 */ 571 @Child(name = "adherence", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 572 @Description(shortDefinition="How device is being used", formalDefinition="This indicates how or if the device is being used." ) 573 protected DeviceUsageAdherenceComponent adherence; 574 575 /** 576 * Who reported the device was being used by the patient. 577 */ 578 @Child(name = "informationSource", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Organization.class}, order=12, min=0, max=1, modifier=false, summary=true) 579 @Description(shortDefinition="Who made the statement", formalDefinition="Who reported the device was being used by the patient." ) 580 protected Reference informationSource; 581 582 /** 583 * Code or Reference to device used. 584 */ 585 @Child(name = "device", type = {CodeableReference.class}, order=13, min=1, max=1, modifier=false, summary=true) 586 @Description(shortDefinition="Code or Reference to device used", formalDefinition="Code or Reference to device used." ) 587 protected CodeableReference device; 588 589 /** 590 * Reason or justification for the use of the device. A coded concept, or another resource whose existence justifies this DeviceUsage. 591 */ 592 @Child(name = "reason", type = {CodeableReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 593 @Description(shortDefinition="Why device was used", formalDefinition="Reason or justification for the use of the device. A coded concept, or another resource whose existence justifies this DeviceUsage." ) 594 protected List<CodeableReference> reason; 595 596 /** 597 * Indicates the anotomic location on the subject's body where the device was used ( i.e. the target). 598 */ 599 @Child(name = "bodySite", type = {CodeableReference.class}, order=15, min=0, max=1, modifier=false, summary=true) 600 @Description(shortDefinition="Target body site", formalDefinition="Indicates the anotomic location on the subject's body where the device was used ( i.e. the target)." ) 601 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 602 protected CodeableReference bodySite; 603 604 /** 605 * Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement. 606 */ 607 @Child(name = "note", type = {Annotation.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 608 @Description(shortDefinition="Addition details (comments, instructions)", formalDefinition="Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement." ) 609 protected List<Annotation> note; 610 611 private static final long serialVersionUID = -10803928L; 612 613 /** 614 * Constructor 615 */ 616 public DeviceUsage() { 617 super(); 618 } 619 620 /** 621 * Constructor 622 */ 623 public DeviceUsage(DeviceUsageStatus status, Reference patient, CodeableReference device) { 624 super(); 625 this.setStatus(status); 626 this.setPatient(patient); 627 this.setDevice(device); 628 } 629 630 /** 631 * @return {@link #identifier} (An external identifier for this statement such as an IRI.) 632 */ 633 public List<Identifier> getIdentifier() { 634 if (this.identifier == null) 635 this.identifier = new ArrayList<Identifier>(); 636 return this.identifier; 637 } 638 639 /** 640 * @return Returns a reference to <code>this</code> for easy method chaining 641 */ 642 public DeviceUsage setIdentifier(List<Identifier> theIdentifier) { 643 this.identifier = theIdentifier; 644 return this; 645 } 646 647 public boolean hasIdentifier() { 648 if (this.identifier == null) 649 return false; 650 for (Identifier item : this.identifier) 651 if (!item.isEmpty()) 652 return true; 653 return false; 654 } 655 656 public Identifier addIdentifier() { //3 657 Identifier t = new Identifier(); 658 if (this.identifier == null) 659 this.identifier = new ArrayList<Identifier>(); 660 this.identifier.add(t); 661 return t; 662 } 663 664 public DeviceUsage addIdentifier(Identifier t) { //3 665 if (t == null) 666 return this; 667 if (this.identifier == null) 668 this.identifier = new ArrayList<Identifier>(); 669 this.identifier.add(t); 670 return this; 671 } 672 673 /** 674 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 675 */ 676 public Identifier getIdentifierFirstRep() { 677 if (getIdentifier().isEmpty()) { 678 addIdentifier(); 679 } 680 return getIdentifier().get(0); 681 } 682 683 /** 684 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this DeviceUsage.) 685 */ 686 public List<Reference> getBasedOn() { 687 if (this.basedOn == null) 688 this.basedOn = new ArrayList<Reference>(); 689 return this.basedOn; 690 } 691 692 /** 693 * @return Returns a reference to <code>this</code> for easy method chaining 694 */ 695 public DeviceUsage setBasedOn(List<Reference> theBasedOn) { 696 this.basedOn = theBasedOn; 697 return this; 698 } 699 700 public boolean hasBasedOn() { 701 if (this.basedOn == null) 702 return false; 703 for (Reference item : this.basedOn) 704 if (!item.isEmpty()) 705 return true; 706 return false; 707 } 708 709 public Reference addBasedOn() { //3 710 Reference t = new Reference(); 711 if (this.basedOn == null) 712 this.basedOn = new ArrayList<Reference>(); 713 this.basedOn.add(t); 714 return t; 715 } 716 717 public DeviceUsage addBasedOn(Reference t) { //3 718 if (t == null) 719 return this; 720 if (this.basedOn == null) 721 this.basedOn = new ArrayList<Reference>(); 722 this.basedOn.add(t); 723 return this; 724 } 725 726 /** 727 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 728 */ 729 public Reference getBasedOnFirstRep() { 730 if (getBasedOn().isEmpty()) { 731 addBasedOn(); 732 } 733 return getBasedOn().get(0); 734 } 735 736 /** 737 * @return {@link #status} (A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 738 */ 739 public Enumeration<DeviceUsageStatus> getStatusElement() { 740 if (this.status == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create DeviceUsage.status"); 743 else if (Configuration.doAutoCreate()) 744 this.status = new Enumeration<DeviceUsageStatus>(new DeviceUsageStatusEnumFactory()); // bb 745 return this.status; 746 } 747 748 public boolean hasStatusElement() { 749 return this.status != null && !this.status.isEmpty(); 750 } 751 752 public boolean hasStatus() { 753 return this.status != null && !this.status.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #status} (A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 758 */ 759 public DeviceUsage setStatusElement(Enumeration<DeviceUsageStatus> value) { 760 this.status = value; 761 return this; 762 } 763 764 /** 765 * @return A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed. 766 */ 767 public DeviceUsageStatus getStatus() { 768 return this.status == null ? null : this.status.getValue(); 769 } 770 771 /** 772 * @param value A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed. 773 */ 774 public DeviceUsage setStatus(DeviceUsageStatus value) { 775 if (this.status == null) 776 this.status = new Enumeration<DeviceUsageStatus>(new DeviceUsageStatusEnumFactory()); 777 this.status.setValue(value); 778 return this; 779 } 780 781 /** 782 * @return {@link #category} (This attribute indicates a category for the statement - The device statement may be made in an inpatient or outpatient settting (inpatient | outpatient | community | patientspecified).) 783 */ 784 public List<CodeableConcept> getCategory() { 785 if (this.category == null) 786 this.category = new ArrayList<CodeableConcept>(); 787 return this.category; 788 } 789 790 /** 791 * @return Returns a reference to <code>this</code> for easy method chaining 792 */ 793 public DeviceUsage setCategory(List<CodeableConcept> theCategory) { 794 this.category = theCategory; 795 return this; 796 } 797 798 public boolean hasCategory() { 799 if (this.category == null) 800 return false; 801 for (CodeableConcept item : this.category) 802 if (!item.isEmpty()) 803 return true; 804 return false; 805 } 806 807 public CodeableConcept addCategory() { //3 808 CodeableConcept t = new CodeableConcept(); 809 if (this.category == null) 810 this.category = new ArrayList<CodeableConcept>(); 811 this.category.add(t); 812 return t; 813 } 814 815 public DeviceUsage addCategory(CodeableConcept t) { //3 816 if (t == null) 817 return this; 818 if (this.category == null) 819 this.category = new ArrayList<CodeableConcept>(); 820 this.category.add(t); 821 return this; 822 } 823 824 /** 825 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 826 */ 827 public CodeableConcept getCategoryFirstRep() { 828 if (getCategory().isEmpty()) { 829 addCategory(); 830 } 831 return getCategory().get(0); 832 } 833 834 /** 835 * @return {@link #patient} (The patient who used the device.) 836 */ 837 public Reference getPatient() { 838 if (this.patient == null) 839 if (Configuration.errorOnAutoCreate()) 840 throw new Error("Attempt to auto-create DeviceUsage.patient"); 841 else if (Configuration.doAutoCreate()) 842 this.patient = new Reference(); // cc 843 return this.patient; 844 } 845 846 public boolean hasPatient() { 847 return this.patient != null && !this.patient.isEmpty(); 848 } 849 850 /** 851 * @param value {@link #patient} (The patient who used the device.) 852 */ 853 public DeviceUsage setPatient(Reference value) { 854 this.patient = value; 855 return this; 856 } 857 858 /** 859 * @return {@link #derivedFrom} (Allows linking the DeviceUsage to the underlying Request, or to other information that supports or is used to derive the DeviceUsage.) 860 */ 861 public List<Reference> getDerivedFrom() { 862 if (this.derivedFrom == null) 863 this.derivedFrom = new ArrayList<Reference>(); 864 return this.derivedFrom; 865 } 866 867 /** 868 * @return Returns a reference to <code>this</code> for easy method chaining 869 */ 870 public DeviceUsage setDerivedFrom(List<Reference> theDerivedFrom) { 871 this.derivedFrom = theDerivedFrom; 872 return this; 873 } 874 875 public boolean hasDerivedFrom() { 876 if (this.derivedFrom == null) 877 return false; 878 for (Reference item : this.derivedFrom) 879 if (!item.isEmpty()) 880 return true; 881 return false; 882 } 883 884 public Reference addDerivedFrom() { //3 885 Reference t = new Reference(); 886 if (this.derivedFrom == null) 887 this.derivedFrom = new ArrayList<Reference>(); 888 this.derivedFrom.add(t); 889 return t; 890 } 891 892 public DeviceUsage addDerivedFrom(Reference t) { //3 893 if (t == null) 894 return this; 895 if (this.derivedFrom == null) 896 this.derivedFrom = new ArrayList<Reference>(); 897 this.derivedFrom.add(t); 898 return this; 899 } 900 901 /** 902 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 903 */ 904 public Reference getDerivedFromFirstRep() { 905 if (getDerivedFrom().isEmpty()) { 906 addDerivedFrom(); 907 } 908 return getDerivedFrom().get(0); 909 } 910 911 /** 912 * @return {@link #context} (The encounter or episode of care that establishes the context for this device use statement.) 913 */ 914 public Reference getContext() { 915 if (this.context == null) 916 if (Configuration.errorOnAutoCreate()) 917 throw new Error("Attempt to auto-create DeviceUsage.context"); 918 else if (Configuration.doAutoCreate()) 919 this.context = new Reference(); // cc 920 return this.context; 921 } 922 923 public boolean hasContext() { 924 return this.context != null && !this.context.isEmpty(); 925 } 926 927 /** 928 * @param value {@link #context} (The encounter or episode of care that establishes the context for this device use statement.) 929 */ 930 public DeviceUsage setContext(Reference value) { 931 this.context = value; 932 return this; 933 } 934 935 /** 936 * @return {@link #timing} (How often the device was used.) 937 */ 938 public DataType getTiming() { 939 return this.timing; 940 } 941 942 /** 943 * @return {@link #timing} (How often the device was used.) 944 */ 945 public Timing getTimingTiming() throws FHIRException { 946 if (this.timing == null) 947 this.timing = new Timing(); 948 if (!(this.timing instanceof Timing)) 949 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 950 return (Timing) this.timing; 951 } 952 953 public boolean hasTimingTiming() { 954 return this != null && this.timing instanceof Timing; 955 } 956 957 /** 958 * @return {@link #timing} (How often the device was used.) 959 */ 960 public Period getTimingPeriod() throws FHIRException { 961 if (this.timing == null) 962 this.timing = new Period(); 963 if (!(this.timing instanceof Period)) 964 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 965 return (Period) this.timing; 966 } 967 968 public boolean hasTimingPeriod() { 969 return this != null && this.timing instanceof Period; 970 } 971 972 /** 973 * @return {@link #timing} (How often the device was used.) 974 */ 975 public DateTimeType getTimingDateTimeType() throws FHIRException { 976 if (this.timing == null) 977 this.timing = new DateTimeType(); 978 if (!(this.timing instanceof DateTimeType)) 979 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 980 return (DateTimeType) this.timing; 981 } 982 983 public boolean hasTimingDateTimeType() { 984 return this != null && this.timing instanceof DateTimeType; 985 } 986 987 public boolean hasTiming() { 988 return this.timing != null && !this.timing.isEmpty(); 989 } 990 991 /** 992 * @param value {@link #timing} (How often the device was used.) 993 */ 994 public DeviceUsage setTiming(DataType value) { 995 if (value != null && !(value instanceof Timing || value instanceof Period || value instanceof DateTimeType)) 996 throw new FHIRException("Not the right type for DeviceUsage.timing[x]: "+value.fhirType()); 997 this.timing = value; 998 return this; 999 } 1000 1001 /** 1002 * @return {@link #dateAsserted} (The time at which the statement was recorded by informationSource.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 1003 */ 1004 public DateTimeType getDateAssertedElement() { 1005 if (this.dateAsserted == null) 1006 if (Configuration.errorOnAutoCreate()) 1007 throw new Error("Attempt to auto-create DeviceUsage.dateAsserted"); 1008 else if (Configuration.doAutoCreate()) 1009 this.dateAsserted = new DateTimeType(); // bb 1010 return this.dateAsserted; 1011 } 1012 1013 public boolean hasDateAssertedElement() { 1014 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 1015 } 1016 1017 public boolean hasDateAsserted() { 1018 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 1019 } 1020 1021 /** 1022 * @param value {@link #dateAsserted} (The time at which the statement was recorded by informationSource.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 1023 */ 1024 public DeviceUsage setDateAssertedElement(DateTimeType value) { 1025 this.dateAsserted = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return The time at which the statement was recorded by informationSource. 1031 */ 1032 public Date getDateAsserted() { 1033 return this.dateAsserted == null ? null : this.dateAsserted.getValue(); 1034 } 1035 1036 /** 1037 * @param value The time at which the statement was recorded by informationSource. 1038 */ 1039 public DeviceUsage setDateAsserted(Date value) { 1040 if (value == null) 1041 this.dateAsserted = null; 1042 else { 1043 if (this.dateAsserted == null) 1044 this.dateAsserted = new DateTimeType(); 1045 this.dateAsserted.setValue(value); 1046 } 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #usageStatus} (The status of the device usage, for example always, sometimes, never. This is not the same as the status of the statement.) 1052 */ 1053 public CodeableConcept getUsageStatus() { 1054 if (this.usageStatus == null) 1055 if (Configuration.errorOnAutoCreate()) 1056 throw new Error("Attempt to auto-create DeviceUsage.usageStatus"); 1057 else if (Configuration.doAutoCreate()) 1058 this.usageStatus = new CodeableConcept(); // cc 1059 return this.usageStatus; 1060 } 1061 1062 public boolean hasUsageStatus() { 1063 return this.usageStatus != null && !this.usageStatus.isEmpty(); 1064 } 1065 1066 /** 1067 * @param value {@link #usageStatus} (The status of the device usage, for example always, sometimes, never. This is not the same as the status of the statement.) 1068 */ 1069 public DeviceUsage setUsageStatus(CodeableConcept value) { 1070 this.usageStatus = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return {@link #usageReason} (The reason for asserting the usage status - for example forgot, lost, stolen, broken.) 1076 */ 1077 public List<CodeableConcept> getUsageReason() { 1078 if (this.usageReason == null) 1079 this.usageReason = new ArrayList<CodeableConcept>(); 1080 return this.usageReason; 1081 } 1082 1083 /** 1084 * @return Returns a reference to <code>this</code> for easy method chaining 1085 */ 1086 public DeviceUsage setUsageReason(List<CodeableConcept> theUsageReason) { 1087 this.usageReason = theUsageReason; 1088 return this; 1089 } 1090 1091 public boolean hasUsageReason() { 1092 if (this.usageReason == null) 1093 return false; 1094 for (CodeableConcept item : this.usageReason) 1095 if (!item.isEmpty()) 1096 return true; 1097 return false; 1098 } 1099 1100 public CodeableConcept addUsageReason() { //3 1101 CodeableConcept t = new CodeableConcept(); 1102 if (this.usageReason == null) 1103 this.usageReason = new ArrayList<CodeableConcept>(); 1104 this.usageReason.add(t); 1105 return t; 1106 } 1107 1108 public DeviceUsage addUsageReason(CodeableConcept t) { //3 1109 if (t == null) 1110 return this; 1111 if (this.usageReason == null) 1112 this.usageReason = new ArrayList<CodeableConcept>(); 1113 this.usageReason.add(t); 1114 return this; 1115 } 1116 1117 /** 1118 * @return The first repetition of repeating field {@link #usageReason}, creating it if it does not already exist {3} 1119 */ 1120 public CodeableConcept getUsageReasonFirstRep() { 1121 if (getUsageReason().isEmpty()) { 1122 addUsageReason(); 1123 } 1124 return getUsageReason().get(0); 1125 } 1126 1127 /** 1128 * @return {@link #adherence} (This indicates how or if the device is being used.) 1129 */ 1130 public DeviceUsageAdherenceComponent getAdherence() { 1131 if (this.adherence == null) 1132 if (Configuration.errorOnAutoCreate()) 1133 throw new Error("Attempt to auto-create DeviceUsage.adherence"); 1134 else if (Configuration.doAutoCreate()) 1135 this.adherence = new DeviceUsageAdherenceComponent(); // cc 1136 return this.adherence; 1137 } 1138 1139 public boolean hasAdherence() { 1140 return this.adherence != null && !this.adherence.isEmpty(); 1141 } 1142 1143 /** 1144 * @param value {@link #adherence} (This indicates how or if the device is being used.) 1145 */ 1146 public DeviceUsage setAdherence(DeviceUsageAdherenceComponent value) { 1147 this.adherence = value; 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #informationSource} (Who reported the device was being used by the patient.) 1153 */ 1154 public Reference getInformationSource() { 1155 if (this.informationSource == null) 1156 if (Configuration.errorOnAutoCreate()) 1157 throw new Error("Attempt to auto-create DeviceUsage.informationSource"); 1158 else if (Configuration.doAutoCreate()) 1159 this.informationSource = new Reference(); // cc 1160 return this.informationSource; 1161 } 1162 1163 public boolean hasInformationSource() { 1164 return this.informationSource != null && !this.informationSource.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #informationSource} (Who reported the device was being used by the patient.) 1169 */ 1170 public DeviceUsage setInformationSource(Reference value) { 1171 this.informationSource = value; 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #device} (Code or Reference to device used.) 1177 */ 1178 public CodeableReference getDevice() { 1179 if (this.device == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create DeviceUsage.device"); 1182 else if (Configuration.doAutoCreate()) 1183 this.device = new CodeableReference(); // cc 1184 return this.device; 1185 } 1186 1187 public boolean hasDevice() { 1188 return this.device != null && !this.device.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #device} (Code or Reference to device used.) 1193 */ 1194 public DeviceUsage setDevice(CodeableReference value) { 1195 this.device = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #reason} (Reason or justification for the use of the device. A coded concept, or another resource whose existence justifies this DeviceUsage.) 1201 */ 1202 public List<CodeableReference> getReason() { 1203 if (this.reason == null) 1204 this.reason = new ArrayList<CodeableReference>(); 1205 return this.reason; 1206 } 1207 1208 /** 1209 * @return Returns a reference to <code>this</code> for easy method chaining 1210 */ 1211 public DeviceUsage setReason(List<CodeableReference> theReason) { 1212 this.reason = theReason; 1213 return this; 1214 } 1215 1216 public boolean hasReason() { 1217 if (this.reason == null) 1218 return false; 1219 for (CodeableReference item : this.reason) 1220 if (!item.isEmpty()) 1221 return true; 1222 return false; 1223 } 1224 1225 public CodeableReference addReason() { //3 1226 CodeableReference t = new CodeableReference(); 1227 if (this.reason == null) 1228 this.reason = new ArrayList<CodeableReference>(); 1229 this.reason.add(t); 1230 return t; 1231 } 1232 1233 public DeviceUsage addReason(CodeableReference t) { //3 1234 if (t == null) 1235 return this; 1236 if (this.reason == null) 1237 this.reason = new ArrayList<CodeableReference>(); 1238 this.reason.add(t); 1239 return this; 1240 } 1241 1242 /** 1243 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1244 */ 1245 public CodeableReference getReasonFirstRep() { 1246 if (getReason().isEmpty()) { 1247 addReason(); 1248 } 1249 return getReason().get(0); 1250 } 1251 1252 /** 1253 * @return {@link #bodySite} (Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).) 1254 */ 1255 public CodeableReference getBodySite() { 1256 if (this.bodySite == null) 1257 if (Configuration.errorOnAutoCreate()) 1258 throw new Error("Attempt to auto-create DeviceUsage.bodySite"); 1259 else if (Configuration.doAutoCreate()) 1260 this.bodySite = new CodeableReference(); // cc 1261 return this.bodySite; 1262 } 1263 1264 public boolean hasBodySite() { 1265 return this.bodySite != null && !this.bodySite.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #bodySite} (Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).) 1270 */ 1271 public DeviceUsage setBodySite(CodeableReference value) { 1272 this.bodySite = value; 1273 return this; 1274 } 1275 1276 /** 1277 * @return {@link #note} (Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.) 1278 */ 1279 public List<Annotation> getNote() { 1280 if (this.note == null) 1281 this.note = new ArrayList<Annotation>(); 1282 return this.note; 1283 } 1284 1285 /** 1286 * @return Returns a reference to <code>this</code> for easy method chaining 1287 */ 1288 public DeviceUsage setNote(List<Annotation> theNote) { 1289 this.note = theNote; 1290 return this; 1291 } 1292 1293 public boolean hasNote() { 1294 if (this.note == null) 1295 return false; 1296 for (Annotation item : this.note) 1297 if (!item.isEmpty()) 1298 return true; 1299 return false; 1300 } 1301 1302 public Annotation addNote() { //3 1303 Annotation t = new Annotation(); 1304 if (this.note == null) 1305 this.note = new ArrayList<Annotation>(); 1306 this.note.add(t); 1307 return t; 1308 } 1309 1310 public DeviceUsage addNote(Annotation t) { //3 1311 if (t == null) 1312 return this; 1313 if (this.note == null) 1314 this.note = new ArrayList<Annotation>(); 1315 this.note.add(t); 1316 return this; 1317 } 1318 1319 /** 1320 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1321 */ 1322 public Annotation getNoteFirstRep() { 1323 if (getNote().isEmpty()) { 1324 addNote(); 1325 } 1326 return getNote().get(0); 1327 } 1328 1329 protected void listChildren(List<Property> children) { 1330 super.listChildren(children); 1331 children.add(new Property("identifier", "Identifier", "An external identifier for this statement such as an IRI.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1332 children.add(new Property("basedOn", "Reference(ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUsage.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1333 children.add(new Property("status", "code", "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 0, 1, status)); 1334 children.add(new Property("category", "CodeableConcept", "This attribute indicates a category for the statement - The device statement may be made in an inpatient or outpatient settting (inpatient | outpatient | community | patientspecified).", 0, java.lang.Integer.MAX_VALUE, category)); 1335 children.add(new Property("patient", "Reference(Patient)", "The patient who used the device.", 0, 1, patient)); 1336 children.add(new Property("derivedFrom", "Reference(ServiceRequest|Procedure|Claim|Observation|QuestionnaireResponse|DocumentReference)", "Allows linking the DeviceUsage to the underlying Request, or to other information that supports or is used to derive the DeviceUsage.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1337 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this device use statement.", 0, 1, context)); 1338 children.add(new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing)); 1339 children.add(new Property("dateAsserted", "dateTime", "The time at which the statement was recorded by informationSource.", 0, 1, dateAsserted)); 1340 children.add(new Property("usageStatus", "CodeableConcept", "The status of the device usage, for example always, sometimes, never. This is not the same as the status of the statement.", 0, 1, usageStatus)); 1341 children.add(new Property("usageReason", "CodeableConcept", "The reason for asserting the usage status - for example forgot, lost, stolen, broken.", 0, java.lang.Integer.MAX_VALUE, usageReason)); 1342 children.add(new Property("adherence", "", "This indicates how or if the device is being used.", 0, 1, adherence)); 1343 children.add(new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "Who reported the device was being used by the patient.", 0, 1, informationSource)); 1344 children.add(new Property("device", "CodeableReference(Device|DeviceDefinition)", "Code or Reference to device used.", 0, 1, device)); 1345 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference|Procedure)", "Reason or justification for the use of the device. A coded concept, or another resource whose existence justifies this DeviceUsage.", 0, java.lang.Integer.MAX_VALUE, reason)); 1346 children.add(new Property("bodySite", "CodeableReference(BodyStructure)", "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).", 0, 1, bodySite)); 1347 children.add(new Property("note", "Annotation", "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note)); 1348 } 1349 1350 @Override 1351 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1352 switch (_hash) { 1353 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An external identifier for this statement such as an IRI.", 0, java.lang.Integer.MAX_VALUE, identifier); 1354 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(ServiceRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUsage.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1355 case -892481550: /*status*/ return new Property("status", "code", "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 0, 1, status); 1356 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "This attribute indicates a category for the statement - The device statement may be made in an inpatient or outpatient settting (inpatient | outpatient | community | patientspecified).", 0, java.lang.Integer.MAX_VALUE, category); 1357 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who used the device.", 0, 1, patient); 1358 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(ServiceRequest|Procedure|Claim|Observation|QuestionnaireResponse|DocumentReference)", "Allows linking the DeviceUsage to the underlying Request, or to other information that supports or is used to derive the DeviceUsage.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1359 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this device use statement.", 0, 1, context); 1360 case 164632566: /*timing[x]*/ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing); 1361 case -873664438: /*timing*/ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing); 1362 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing", "How often the device was used.", 0, 1, timing); 1363 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Period", "How often the device was used.", 0, 1, timing); 1364 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "dateTime", "How often the device was used.", 0, 1, timing); 1365 case -1980855245: /*dateAsserted*/ return new Property("dateAsserted", "dateTime", "The time at which the statement was recorded by informationSource.", 0, 1, dateAsserted); 1366 case 907197683: /*usageStatus*/ return new Property("usageStatus", "CodeableConcept", "The status of the device usage, for example always, sometimes, never. This is not the same as the status of the statement.", 0, 1, usageStatus); 1367 case 864714565: /*usageReason*/ return new Property("usageReason", "CodeableConcept", "The reason for asserting the usage status - for example forgot, lost, stolen, broken.", 0, java.lang.Integer.MAX_VALUE, usageReason); 1368 case -231003683: /*adherence*/ return new Property("adherence", "", "This indicates how or if the device is being used.", 0, 1, adherence); 1369 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "Who reported the device was being used by the patient.", 0, 1, informationSource); 1370 case -1335157162: /*device*/ return new Property("device", "CodeableReference(Device|DeviceDefinition)", "Code or Reference to device used.", 0, 1, device); 1371 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference|Procedure)", "Reason or justification for the use of the device. A coded concept, or another resource whose existence justifies this DeviceUsage.", 0, java.lang.Integer.MAX_VALUE, reason); 1372 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableReference(BodyStructure)", "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).", 0, 1, bodySite); 1373 case 3387378: /*note*/ return new Property("note", "Annotation", "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 0, java.lang.Integer.MAX_VALUE, note); 1374 default: return super.getNamedProperty(_hash, _name, _checkValid); 1375 } 1376 1377 } 1378 1379 @Override 1380 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1381 switch (hash) { 1382 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1383 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1384 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DeviceUsageStatus> 1385 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1386 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1387 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1388 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1389 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 1390 case -1980855245: /*dateAsserted*/ return this.dateAsserted == null ? new Base[0] : new Base[] {this.dateAsserted}; // DateTimeType 1391 case 907197683: /*usageStatus*/ return this.usageStatus == null ? new Base[0] : new Base[] {this.usageStatus}; // CodeableConcept 1392 case 864714565: /*usageReason*/ return this.usageReason == null ? new Base[0] : this.usageReason.toArray(new Base[this.usageReason.size()]); // CodeableConcept 1393 case -231003683: /*adherence*/ return this.adherence == null ? new Base[0] : new Base[] {this.adherence}; // DeviceUsageAdherenceComponent 1394 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : new Base[] {this.informationSource}; // Reference 1395 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // CodeableReference 1396 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1397 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableReference 1398 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1399 default: return super.getProperty(hash, name, checkValid); 1400 } 1401 1402 } 1403 1404 @Override 1405 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1406 switch (hash) { 1407 case -1618432855: // identifier 1408 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1409 return value; 1410 case -332612366: // basedOn 1411 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1412 return value; 1413 case -892481550: // status 1414 value = new DeviceUsageStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1415 this.status = (Enumeration) value; // Enumeration<DeviceUsageStatus> 1416 return value; 1417 case 50511102: // category 1418 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1419 return value; 1420 case -791418107: // patient 1421 this.patient = TypeConvertor.castToReference(value); // Reference 1422 return value; 1423 case 1077922663: // derivedFrom 1424 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 1425 return value; 1426 case 951530927: // context 1427 this.context = TypeConvertor.castToReference(value); // Reference 1428 return value; 1429 case -873664438: // timing 1430 this.timing = TypeConvertor.castToType(value); // DataType 1431 return value; 1432 case -1980855245: // dateAsserted 1433 this.dateAsserted = TypeConvertor.castToDateTime(value); // DateTimeType 1434 return value; 1435 case 907197683: // usageStatus 1436 this.usageStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1437 return value; 1438 case 864714565: // usageReason 1439 this.getUsageReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1440 return value; 1441 case -231003683: // adherence 1442 this.adherence = (DeviceUsageAdherenceComponent) value; // DeviceUsageAdherenceComponent 1443 return value; 1444 case -2123220889: // informationSource 1445 this.informationSource = TypeConvertor.castToReference(value); // Reference 1446 return value; 1447 case -1335157162: // device 1448 this.device = TypeConvertor.castToCodeableReference(value); // CodeableReference 1449 return value; 1450 case -934964668: // reason 1451 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1452 return value; 1453 case 1702620169: // bodySite 1454 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 1455 return value; 1456 case 3387378: // note 1457 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1458 return value; 1459 default: return super.setProperty(hash, name, value); 1460 } 1461 1462 } 1463 1464 @Override 1465 public Base setProperty(String name, Base value) throws FHIRException { 1466 if (name.equals("identifier")) { 1467 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1468 } else if (name.equals("basedOn")) { 1469 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1470 } else if (name.equals("status")) { 1471 value = new DeviceUsageStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1472 this.status = (Enumeration) value; // Enumeration<DeviceUsageStatus> 1473 } else if (name.equals("category")) { 1474 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1475 } else if (name.equals("patient")) { 1476 this.patient = TypeConvertor.castToReference(value); // Reference 1477 } else if (name.equals("derivedFrom")) { 1478 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 1479 } else if (name.equals("context")) { 1480 this.context = TypeConvertor.castToReference(value); // Reference 1481 } else if (name.equals("timing[x]")) { 1482 this.timing = TypeConvertor.castToType(value); // DataType 1483 } else if (name.equals("dateAsserted")) { 1484 this.dateAsserted = TypeConvertor.castToDateTime(value); // DateTimeType 1485 } else if (name.equals("usageStatus")) { 1486 this.usageStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1487 } else if (name.equals("usageReason")) { 1488 this.getUsageReason().add(TypeConvertor.castToCodeableConcept(value)); 1489 } else if (name.equals("adherence")) { 1490 this.adherence = (DeviceUsageAdherenceComponent) value; // DeviceUsageAdherenceComponent 1491 } else if (name.equals("informationSource")) { 1492 this.informationSource = TypeConvertor.castToReference(value); // Reference 1493 } else if (name.equals("device")) { 1494 this.device = TypeConvertor.castToCodeableReference(value); // CodeableReference 1495 } else if (name.equals("reason")) { 1496 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1497 } else if (name.equals("bodySite")) { 1498 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 1499 } else if (name.equals("note")) { 1500 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1501 } else 1502 return super.setProperty(name, value); 1503 return value; 1504 } 1505 1506 @Override 1507 public void removeChild(String name, Base value) throws FHIRException { 1508 if (name.equals("identifier")) { 1509 this.getIdentifier().remove(value); 1510 } else if (name.equals("basedOn")) { 1511 this.getBasedOn().remove(value); 1512 } else if (name.equals("status")) { 1513 value = new DeviceUsageStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1514 this.status = (Enumeration) value; // Enumeration<DeviceUsageStatus> 1515 } else if (name.equals("category")) { 1516 this.getCategory().remove(value); 1517 } else if (name.equals("patient")) { 1518 this.patient = null; 1519 } else if (name.equals("derivedFrom")) { 1520 this.getDerivedFrom().remove(value); 1521 } else if (name.equals("context")) { 1522 this.context = null; 1523 } else if (name.equals("timing[x]")) { 1524 this.timing = null; 1525 } else if (name.equals("dateAsserted")) { 1526 this.dateAsserted = null; 1527 } else if (name.equals("usageStatus")) { 1528 this.usageStatus = null; 1529 } else if (name.equals("usageReason")) { 1530 this.getUsageReason().remove(value); 1531 } else if (name.equals("adherence")) { 1532 this.adherence = (DeviceUsageAdherenceComponent) value; // DeviceUsageAdherenceComponent 1533 } else if (name.equals("informationSource")) { 1534 this.informationSource = null; 1535 } else if (name.equals("device")) { 1536 this.device = null; 1537 } else if (name.equals("reason")) { 1538 this.getReason().remove(value); 1539 } else if (name.equals("bodySite")) { 1540 this.bodySite = null; 1541 } else if (name.equals("note")) { 1542 this.getNote().remove(value); 1543 } else 1544 super.removeChild(name, value); 1545 1546 } 1547 1548 @Override 1549 public Base makeProperty(int hash, String name) throws FHIRException { 1550 switch (hash) { 1551 case -1618432855: return addIdentifier(); 1552 case -332612366: return addBasedOn(); 1553 case -892481550: return getStatusElement(); 1554 case 50511102: return addCategory(); 1555 case -791418107: return getPatient(); 1556 case 1077922663: return addDerivedFrom(); 1557 case 951530927: return getContext(); 1558 case 164632566: return getTiming(); 1559 case -873664438: return getTiming(); 1560 case -1980855245: return getDateAssertedElement(); 1561 case 907197683: return getUsageStatus(); 1562 case 864714565: return addUsageReason(); 1563 case -231003683: return getAdherence(); 1564 case -2123220889: return getInformationSource(); 1565 case -1335157162: return getDevice(); 1566 case -934964668: return addReason(); 1567 case 1702620169: return getBodySite(); 1568 case 3387378: return addNote(); 1569 default: return super.makeProperty(hash, name); 1570 } 1571 1572 } 1573 1574 @Override 1575 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1576 switch (hash) { 1577 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1578 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1579 case -892481550: /*status*/ return new String[] {"code"}; 1580 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1581 case -791418107: /*patient*/ return new String[] {"Reference"}; 1582 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 1583 case 951530927: /*context*/ return new String[] {"Reference"}; 1584 case -873664438: /*timing*/ return new String[] {"Timing", "Period", "dateTime"}; 1585 case -1980855245: /*dateAsserted*/ return new String[] {"dateTime"}; 1586 case 907197683: /*usageStatus*/ return new String[] {"CodeableConcept"}; 1587 case 864714565: /*usageReason*/ return new String[] {"CodeableConcept"}; 1588 case -231003683: /*adherence*/ return new String[] {}; 1589 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 1590 case -1335157162: /*device*/ return new String[] {"CodeableReference"}; 1591 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1592 case 1702620169: /*bodySite*/ return new String[] {"CodeableReference"}; 1593 case 3387378: /*note*/ return new String[] {"Annotation"}; 1594 default: return super.getTypesForProperty(hash, name); 1595 } 1596 1597 } 1598 1599 @Override 1600 public Base addChild(String name) throws FHIRException { 1601 if (name.equals("identifier")) { 1602 return addIdentifier(); 1603 } 1604 else if (name.equals("basedOn")) { 1605 return addBasedOn(); 1606 } 1607 else if (name.equals("status")) { 1608 throw new FHIRException("Cannot call addChild on a singleton property DeviceUsage.status"); 1609 } 1610 else if (name.equals("category")) { 1611 return addCategory(); 1612 } 1613 else if (name.equals("patient")) { 1614 this.patient = new Reference(); 1615 return this.patient; 1616 } 1617 else if (name.equals("derivedFrom")) { 1618 return addDerivedFrom(); 1619 } 1620 else if (name.equals("context")) { 1621 this.context = new Reference(); 1622 return this.context; 1623 } 1624 else if (name.equals("timingTiming")) { 1625 this.timing = new Timing(); 1626 return this.timing; 1627 } 1628 else if (name.equals("timingPeriod")) { 1629 this.timing = new Period(); 1630 return this.timing; 1631 } 1632 else if (name.equals("timingDateTime")) { 1633 this.timing = new DateTimeType(); 1634 return this.timing; 1635 } 1636 else if (name.equals("dateAsserted")) { 1637 throw new FHIRException("Cannot call addChild on a singleton property DeviceUsage.dateAsserted"); 1638 } 1639 else if (name.equals("usageStatus")) { 1640 this.usageStatus = new CodeableConcept(); 1641 return this.usageStatus; 1642 } 1643 else if (name.equals("usageReason")) { 1644 return addUsageReason(); 1645 } 1646 else if (name.equals("adherence")) { 1647 this.adherence = new DeviceUsageAdherenceComponent(); 1648 return this.adherence; 1649 } 1650 else if (name.equals("informationSource")) { 1651 this.informationSource = new Reference(); 1652 return this.informationSource; 1653 } 1654 else if (name.equals("device")) { 1655 this.device = new CodeableReference(); 1656 return this.device; 1657 } 1658 else if (name.equals("reason")) { 1659 return addReason(); 1660 } 1661 else if (name.equals("bodySite")) { 1662 this.bodySite = new CodeableReference(); 1663 return this.bodySite; 1664 } 1665 else if (name.equals("note")) { 1666 return addNote(); 1667 } 1668 else 1669 return super.addChild(name); 1670 } 1671 1672 public String fhirType() { 1673 return "DeviceUsage"; 1674 1675 } 1676 1677 public DeviceUsage copy() { 1678 DeviceUsage dst = new DeviceUsage(); 1679 copyValues(dst); 1680 return dst; 1681 } 1682 1683 public void copyValues(DeviceUsage dst) { 1684 super.copyValues(dst); 1685 if (identifier != null) { 1686 dst.identifier = new ArrayList<Identifier>(); 1687 for (Identifier i : identifier) 1688 dst.identifier.add(i.copy()); 1689 }; 1690 if (basedOn != null) { 1691 dst.basedOn = new ArrayList<Reference>(); 1692 for (Reference i : basedOn) 1693 dst.basedOn.add(i.copy()); 1694 }; 1695 dst.status = status == null ? null : status.copy(); 1696 if (category != null) { 1697 dst.category = new ArrayList<CodeableConcept>(); 1698 for (CodeableConcept i : category) 1699 dst.category.add(i.copy()); 1700 }; 1701 dst.patient = patient == null ? null : patient.copy(); 1702 if (derivedFrom != null) { 1703 dst.derivedFrom = new ArrayList<Reference>(); 1704 for (Reference i : derivedFrom) 1705 dst.derivedFrom.add(i.copy()); 1706 }; 1707 dst.context = context == null ? null : context.copy(); 1708 dst.timing = timing == null ? null : timing.copy(); 1709 dst.dateAsserted = dateAsserted == null ? null : dateAsserted.copy(); 1710 dst.usageStatus = usageStatus == null ? null : usageStatus.copy(); 1711 if (usageReason != null) { 1712 dst.usageReason = new ArrayList<CodeableConcept>(); 1713 for (CodeableConcept i : usageReason) 1714 dst.usageReason.add(i.copy()); 1715 }; 1716 dst.adherence = adherence == null ? null : adherence.copy(); 1717 dst.informationSource = informationSource == null ? null : informationSource.copy(); 1718 dst.device = device == null ? null : device.copy(); 1719 if (reason != null) { 1720 dst.reason = new ArrayList<CodeableReference>(); 1721 for (CodeableReference i : reason) 1722 dst.reason.add(i.copy()); 1723 }; 1724 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1725 if (note != null) { 1726 dst.note = new ArrayList<Annotation>(); 1727 for (Annotation i : note) 1728 dst.note.add(i.copy()); 1729 }; 1730 } 1731 1732 protected DeviceUsage typedCopy() { 1733 return copy(); 1734 } 1735 1736 @Override 1737 public boolean equalsDeep(Base other_) { 1738 if (!super.equalsDeep(other_)) 1739 return false; 1740 if (!(other_ instanceof DeviceUsage)) 1741 return false; 1742 DeviceUsage o = (DeviceUsage) other_; 1743 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(status, o.status, true) 1744 && compareDeep(category, o.category, true) && compareDeep(patient, o.patient, true) && compareDeep(derivedFrom, o.derivedFrom, true) 1745 && compareDeep(context, o.context, true) && compareDeep(timing, o.timing, true) && compareDeep(dateAsserted, o.dateAsserted, true) 1746 && compareDeep(usageStatus, o.usageStatus, true) && compareDeep(usageReason, o.usageReason, true) 1747 && compareDeep(adherence, o.adherence, true) && compareDeep(informationSource, o.informationSource, true) 1748 && compareDeep(device, o.device, true) && compareDeep(reason, o.reason, true) && compareDeep(bodySite, o.bodySite, true) 1749 && compareDeep(note, o.note, true); 1750 } 1751 1752 @Override 1753 public boolean equalsShallow(Base other_) { 1754 if (!super.equalsShallow(other_)) 1755 return false; 1756 if (!(other_ instanceof DeviceUsage)) 1757 return false; 1758 DeviceUsage o = (DeviceUsage) other_; 1759 return compareValues(status, o.status, true) && compareValues(dateAsserted, o.dateAsserted, true); 1760 } 1761 1762 public boolean isEmpty() { 1763 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status 1764 , category, patient, derivedFrom, context, timing, dateAsserted, usageStatus, usageReason 1765 , adherence, informationSource, device, reason, bodySite, note); 1766 } 1767 1768 @Override 1769 public ResourceType getResourceType() { 1770 return ResourceType.DeviceUsage; 1771 } 1772 1773 /** 1774 * Search parameter: <b>device</b> 1775 * <p> 1776 * Description: <b>Search by device</b><br> 1777 * Type: <b>token</b><br> 1778 * Path: <b>DeviceUsage.device.concept</b><br> 1779 * </p> 1780 */ 1781 @SearchParamDefinition(name="device", path="DeviceUsage.device.concept", description="Search by device", type="token" ) 1782 public static final String SP_DEVICE = "device"; 1783 /** 1784 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1785 * <p> 1786 * Description: <b>Search by device</b><br> 1787 * Type: <b>token</b><br> 1788 * Path: <b>DeviceUsage.device.concept</b><br> 1789 * </p> 1790 */ 1791 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DEVICE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DEVICE); 1792 1793 /** 1794 * Search parameter: <b>status</b> 1795 * <p> 1796 * Description: <b>The status of the device usage</b><br> 1797 * Type: <b>token</b><br> 1798 * Path: <b>DeviceUsage.status</b><br> 1799 * </p> 1800 */ 1801 @SearchParamDefinition(name="status", path="DeviceUsage.status", description="The status of the device usage", type="token" ) 1802 public static final String SP_STATUS = "status"; 1803 /** 1804 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1805 * <p> 1806 * Description: <b>The status of the device usage</b><br> 1807 * Type: <b>token</b><br> 1808 * Path: <b>DeviceUsage.status</b><br> 1809 * </p> 1810 */ 1811 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1812 1813 /** 1814 * Search parameter: <b>identifier</b> 1815 * <p> 1816 * Description: <b>Multiple Resources: 1817 1818* [Account](account.html): Account number 1819* [AdverseEvent](adverseevent.html): Business identifier for the event 1820* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1821* [Appointment](appointment.html): An Identifier of the Appointment 1822* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1823* [Basic](basic.html): Business identifier 1824* [BodyStructure](bodystructure.html): Bodystructure identifier 1825* [CarePlan](careplan.html): External Ids for this plan 1826* [CareTeam](careteam.html): External Ids for this team 1827* [ChargeItem](chargeitem.html): Business Identifier for item 1828* [Claim](claim.html): The primary identifier of the financial resource 1829* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1830* [ClinicalImpression](clinicalimpression.html): Business identifier 1831* [Communication](communication.html): Unique identifier 1832* [CommunicationRequest](communicationrequest.html): Unique identifier 1833* [Composition](composition.html): Version-independent identifier for the Composition 1834* [Condition](condition.html): A unique identifier of the condition record 1835* [Consent](consent.html): Identifier for this record (external references) 1836* [Contract](contract.html): The identity of the contract 1837* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1838* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1839* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1840* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1841* [DeviceRequest](devicerequest.html): Business identifier for request/order 1842* [DeviceUsage](deviceusage.html): Search by identifier 1843* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1844* [DocumentReference](documentreference.html): Identifier of the attachment binary 1845* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1846* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1847* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1848* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1849* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1850* [Flag](flag.html): Business identifier 1851* [Goal](goal.html): External Ids for this goal 1852* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1853* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1854* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1855* [Immunization](immunization.html): Business identifier 1856* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1857* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1858* [Invoice](invoice.html): Business Identifier for item 1859* [List](list.html): Business identifier 1860* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1861* [Medication](medication.html): Returns medications with this external identifier 1862* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1863* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1864* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1865* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1866* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1867* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1868* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1869* [Observation](observation.html): The unique id for a particular observation 1870* [Person](person.html): A person Identifier 1871* [Procedure](procedure.html): A unique identifier for a procedure 1872* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1873* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1874* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1875* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1876* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1877* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1878* [Specimen](specimen.html): The unique identifier associated with the specimen 1879* [SupplyDelivery](supplydelivery.html): External identifier 1880* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1881* [Task](task.html): Search for a task instance by its business identifier 1882* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1883</b><br> 1884 * Type: <b>token</b><br> 1885 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1886 * </p> 1887 */ 1888 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1889 public static final String SP_IDENTIFIER = "identifier"; 1890 /** 1891 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1892 * <p> 1893 * Description: <b>Multiple Resources: 1894 1895* [Account](account.html): Account number 1896* [AdverseEvent](adverseevent.html): Business identifier for the event 1897* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1898* [Appointment](appointment.html): An Identifier of the Appointment 1899* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1900* [Basic](basic.html): Business identifier 1901* [BodyStructure](bodystructure.html): Bodystructure identifier 1902* [CarePlan](careplan.html): External Ids for this plan 1903* [CareTeam](careteam.html): External Ids for this team 1904* [ChargeItem](chargeitem.html): Business Identifier for item 1905* [Claim](claim.html): The primary identifier of the financial resource 1906* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1907* [ClinicalImpression](clinicalimpression.html): Business identifier 1908* [Communication](communication.html): Unique identifier 1909* [CommunicationRequest](communicationrequest.html): Unique identifier 1910* [Composition](composition.html): Version-independent identifier for the Composition 1911* [Condition](condition.html): A unique identifier of the condition record 1912* [Consent](consent.html): Identifier for this record (external references) 1913* [Contract](contract.html): The identity of the contract 1914* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1915* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1916* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1917* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1918* [DeviceRequest](devicerequest.html): Business identifier for request/order 1919* [DeviceUsage](deviceusage.html): Search by identifier 1920* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1921* [DocumentReference](documentreference.html): Identifier of the attachment binary 1922* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1923* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1924* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1925* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1926* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1927* [Flag](flag.html): Business identifier 1928* [Goal](goal.html): External Ids for this goal 1929* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1930* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1931* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1932* [Immunization](immunization.html): Business identifier 1933* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1934* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1935* [Invoice](invoice.html): Business Identifier for item 1936* [List](list.html): Business identifier 1937* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1938* [Medication](medication.html): Returns medications with this external identifier 1939* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1940* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1941* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1942* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1943* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1944* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1945* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1946* [Observation](observation.html): The unique id for a particular observation 1947* [Person](person.html): A person Identifier 1948* [Procedure](procedure.html): A unique identifier for a procedure 1949* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1950* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1951* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1952* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1953* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1954* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1955* [Specimen](specimen.html): The unique identifier associated with the specimen 1956* [SupplyDelivery](supplydelivery.html): External identifier 1957* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1958* [Task](task.html): Search for a task instance by its business identifier 1959* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1960</b><br> 1961 * Type: <b>token</b><br> 1962 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1963 * </p> 1964 */ 1965 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1966 1967 /** 1968 * Search parameter: <b>patient</b> 1969 * <p> 1970 * Description: <b>Multiple Resources: 1971 1972* [Account](account.html): The entity that caused the expenses 1973* [AdverseEvent](adverseevent.html): Subject impacted by event 1974* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1975* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1976* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1977* [AuditEvent](auditevent.html): Where the activity involved patient data 1978* [Basic](basic.html): Identifies the focus of this resource 1979* [BodyStructure](bodystructure.html): Who this is about 1980* [CarePlan](careplan.html): Who the care plan is for 1981* [CareTeam](careteam.html): Who care team is for 1982* [ChargeItem](chargeitem.html): Individual service was done for/to 1983* [Claim](claim.html): Patient receiving the products or services 1984* [ClaimResponse](claimresponse.html): The subject of care 1985* [ClinicalImpression](clinicalimpression.html): Patient assessed 1986* [Communication](communication.html): Focus of message 1987* [CommunicationRequest](communicationrequest.html): Focus of message 1988* [Composition](composition.html): Who and/or what the composition is about 1989* [Condition](condition.html): Who has the condition? 1990* [Consent](consent.html): Who the consent applies to 1991* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1992* [Coverage](coverage.html): Retrieve coverages for a patient 1993* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1994* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1995* [DetectedIssue](detectedissue.html): Associated patient 1996* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1997* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1998* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1999* [DocumentReference](documentreference.html): Who/what is the subject of the document 2000* [Encounter](encounter.html): The patient present at the encounter 2001* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2002* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2003* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2004* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2005* [Flag](flag.html): The identity of a subject to list flags for 2006* [Goal](goal.html): Who this goal is intended for 2007* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2008* [ImagingSelection](imagingselection.html): Who the study is about 2009* [ImagingStudy](imagingstudy.html): Who the study is about 2010* [Immunization](immunization.html): The patient for the vaccination record 2011* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2012* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2013* [Invoice](invoice.html): Recipient(s) of goods and services 2014* [List](list.html): If all resources have the same subject 2015* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2016* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2017* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2018* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2019* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2020* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2021* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2022* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2023* [Observation](observation.html): The subject that the observation is about (if patient) 2024* [Person](person.html): The Person links to this Patient 2025* [Procedure](procedure.html): Search by subject - a patient 2026* [Provenance](provenance.html): Where the activity involved patient data 2027* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2028* [RelatedPerson](relatedperson.html): The patient this related person is related to 2029* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2030* [ResearchSubject](researchsubject.html): Who or what is part of study 2031* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2032* [ServiceRequest](servicerequest.html): Search by subject - a patient 2033* [Specimen](specimen.html): The patient the specimen comes from 2034* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2035* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2036* [Task](task.html): Search by patient 2037* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2038</b><br> 2039 * Type: <b>reference</b><br> 2040 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2041 * </p> 2042 */ 2043 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2044 public static final String SP_PATIENT = "patient"; 2045 /** 2046 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2047 * <p> 2048 * Description: <b>Multiple Resources: 2049 2050* [Account](account.html): The entity that caused the expenses 2051* [AdverseEvent](adverseevent.html): Subject impacted by event 2052* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2053* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2054* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2055* [AuditEvent](auditevent.html): Where the activity involved patient data 2056* [Basic](basic.html): Identifies the focus of this resource 2057* [BodyStructure](bodystructure.html): Who this is about 2058* [CarePlan](careplan.html): Who the care plan is for 2059* [CareTeam](careteam.html): Who care team is for 2060* [ChargeItem](chargeitem.html): Individual service was done for/to 2061* [Claim](claim.html): Patient receiving the products or services 2062* [ClaimResponse](claimresponse.html): The subject of care 2063* [ClinicalImpression](clinicalimpression.html): Patient assessed 2064* [Communication](communication.html): Focus of message 2065* [CommunicationRequest](communicationrequest.html): Focus of message 2066* [Composition](composition.html): Who and/or what the composition is about 2067* [Condition](condition.html): Who has the condition? 2068* [Consent](consent.html): Who the consent applies to 2069* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2070* [Coverage](coverage.html): Retrieve coverages for a patient 2071* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2072* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2073* [DetectedIssue](detectedissue.html): Associated patient 2074* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2075* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2076* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2077* [DocumentReference](documentreference.html): Who/what is the subject of the document 2078* [Encounter](encounter.html): The patient present at the encounter 2079* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2080* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2081* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2082* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2083* [Flag](flag.html): The identity of a subject to list flags for 2084* [Goal](goal.html): Who this goal is intended for 2085* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2086* [ImagingSelection](imagingselection.html): Who the study is about 2087* [ImagingStudy](imagingstudy.html): Who the study is about 2088* [Immunization](immunization.html): The patient for the vaccination record 2089* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2090* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2091* [Invoice](invoice.html): Recipient(s) of goods and services 2092* [List](list.html): If all resources have the same subject 2093* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2094* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2095* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2096* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2097* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2098* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2099* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2100* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2101* [Observation](observation.html): The subject that the observation is about (if patient) 2102* [Person](person.html): The Person links to this Patient 2103* [Procedure](procedure.html): Search by subject - a patient 2104* [Provenance](provenance.html): Where the activity involved patient data 2105* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2106* [RelatedPerson](relatedperson.html): The patient this related person is related to 2107* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2108* [ResearchSubject](researchsubject.html): Who or what is part of study 2109* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2110* [ServiceRequest](servicerequest.html): Search by subject - a patient 2111* [Specimen](specimen.html): The patient the specimen comes from 2112* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2113* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2114* [Task](task.html): Search by patient 2115* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2116</b><br> 2117 * Type: <b>reference</b><br> 2118 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2119 * </p> 2120 */ 2121 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2122 2123/** 2124 * Constant for fluent queries to be used to add include statements. Specifies 2125 * the path value of "<b>DeviceUsage:patient</b>". 2126 */ 2127 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DeviceUsage:patient").toLocked(); 2128 2129 2130} 2131