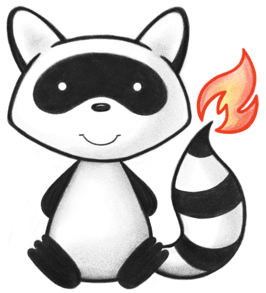
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, products, substances, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. The report also includes non-clinical context such as batch analysis and stability reporting of products and substances. 052 */ 053@ResourceDef(name="DiagnosticReport", profile="http://hl7.org/fhir/StructureDefinition/DiagnosticReport") 054public class DiagnosticReport extends DomainResource { 055 056 public enum DiagnosticReportStatus { 057 /** 058 * The existence of the report is registered, but there is nothing yet available. 059 */ 060 REGISTERED, 061 /** 062 * This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified. 063 */ 064 PARTIAL, 065 /** 066 * Verified early results are available, but not all results are final. 067 */ 068 PRELIMINARY, 069 /** 070 * Prior to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a non-finalized (e.g., preliminary) report that has been issued. 071 */ 072 MODIFIED, 073 /** 074 * The report is complete and verified by an authorized person. 075 */ 076 FINAL, 077 /** 078 * Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued. 079 */ 080 AMENDED, 081 /** 082 * Subsequent to being final, the report has been modified to correct an error in the report or referenced results. 083 */ 084 CORRECTED, 085 /** 086 * Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged. 087 */ 088 APPENDED, 089 /** 090 * The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\"). 091 */ 092 CANCELLED, 093 /** 094 * The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 095 */ 096 ENTEREDINERROR, 097 /** 098 * The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 099 */ 100 UNKNOWN, 101 /** 102 * added to help the parsers with the generic types 103 */ 104 NULL; 105 public static DiagnosticReportStatus fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("registered".equals(codeString)) 109 return REGISTERED; 110 if ("partial".equals(codeString)) 111 return PARTIAL; 112 if ("preliminary".equals(codeString)) 113 return PRELIMINARY; 114 if ("modified".equals(codeString)) 115 return MODIFIED; 116 if ("final".equals(codeString)) 117 return FINAL; 118 if ("amended".equals(codeString)) 119 return AMENDED; 120 if ("corrected".equals(codeString)) 121 return CORRECTED; 122 if ("appended".equals(codeString)) 123 return APPENDED; 124 if ("cancelled".equals(codeString)) 125 return CANCELLED; 126 if ("entered-in-error".equals(codeString)) 127 return ENTEREDINERROR; 128 if ("unknown".equals(codeString)) 129 return UNKNOWN; 130 if (Configuration.isAcceptInvalidEnums()) 131 return null; 132 else 133 throw new FHIRException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 134 } 135 public String toCode() { 136 switch (this) { 137 case REGISTERED: return "registered"; 138 case PARTIAL: return "partial"; 139 case PRELIMINARY: return "preliminary"; 140 case MODIFIED: return "modified"; 141 case FINAL: return "final"; 142 case AMENDED: return "amended"; 143 case CORRECTED: return "corrected"; 144 case APPENDED: return "appended"; 145 case CANCELLED: return "cancelled"; 146 case ENTEREDINERROR: return "entered-in-error"; 147 case UNKNOWN: return "unknown"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 public String getSystem() { 153 switch (this) { 154 case REGISTERED: return "http://hl7.org/fhir/diagnostic-report-status"; 155 case PARTIAL: return "http://hl7.org/fhir/diagnostic-report-status"; 156 case PRELIMINARY: return "http://hl7.org/fhir/diagnostic-report-status"; 157 case MODIFIED: return "http://hl7.org/fhir/diagnostic-report-status"; 158 case FINAL: return "http://hl7.org/fhir/diagnostic-report-status"; 159 case AMENDED: return "http://hl7.org/fhir/diagnostic-report-status"; 160 case CORRECTED: return "http://hl7.org/fhir/diagnostic-report-status"; 161 case APPENDED: return "http://hl7.org/fhir/diagnostic-report-status"; 162 case CANCELLED: return "http://hl7.org/fhir/diagnostic-report-status"; 163 case ENTEREDINERROR: return "http://hl7.org/fhir/diagnostic-report-status"; 164 case UNKNOWN: return "http://hl7.org/fhir/diagnostic-report-status"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 public String getDefinition() { 170 switch (this) { 171 case REGISTERED: return "The existence of the report is registered, but there is nothing yet available."; 172 case PARTIAL: return "This is a partial (e.g. initial, interim or preliminary) report: data in the report may be incomplete or unverified."; 173 case PRELIMINARY: return "Verified early results are available, but not all results are final."; 174 case MODIFIED: return "Prior to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a non-finalized (e.g., preliminary) report that has been issued."; 175 case FINAL: return "The report is complete and verified by an authorized person."; 176 case AMENDED: return "Subsequent to being final, the report has been modified. This includes any change in the results, diagnosis, narrative text, or other content of a report that has been issued."; 177 case CORRECTED: return "Subsequent to being final, the report has been modified to correct an error in the report or referenced results."; 178 case APPENDED: return "Subsequent to being final, the report has been modified by adding new content. The existing content is unchanged."; 179 case CANCELLED: return "The report is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 180 case ENTEREDINERROR: return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 181 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case REGISTERED: return "Registered"; 189 case PARTIAL: return "Partial"; 190 case PRELIMINARY: return "Preliminary"; 191 case MODIFIED: return "Modified"; 192 case FINAL: return "Final"; 193 case AMENDED: return "Amended"; 194 case CORRECTED: return "Corrected"; 195 case APPENDED: return "Appended"; 196 case CANCELLED: return "Cancelled"; 197 case ENTEREDINERROR: return "Entered in Error"; 198 case UNKNOWN: return "Unknown"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 } 204 205 public static class DiagnosticReportStatusEnumFactory implements EnumFactory<DiagnosticReportStatus> { 206 public DiagnosticReportStatus fromCode(String codeString) throws IllegalArgumentException { 207 if (codeString == null || "".equals(codeString)) 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("registered".equals(codeString)) 211 return DiagnosticReportStatus.REGISTERED; 212 if ("partial".equals(codeString)) 213 return DiagnosticReportStatus.PARTIAL; 214 if ("preliminary".equals(codeString)) 215 return DiagnosticReportStatus.PRELIMINARY; 216 if ("modified".equals(codeString)) 217 return DiagnosticReportStatus.MODIFIED; 218 if ("final".equals(codeString)) 219 return DiagnosticReportStatus.FINAL; 220 if ("amended".equals(codeString)) 221 return DiagnosticReportStatus.AMENDED; 222 if ("corrected".equals(codeString)) 223 return DiagnosticReportStatus.CORRECTED; 224 if ("appended".equals(codeString)) 225 return DiagnosticReportStatus.APPENDED; 226 if ("cancelled".equals(codeString)) 227 return DiagnosticReportStatus.CANCELLED; 228 if ("entered-in-error".equals(codeString)) 229 return DiagnosticReportStatus.ENTEREDINERROR; 230 if ("unknown".equals(codeString)) 231 return DiagnosticReportStatus.UNKNOWN; 232 throw new IllegalArgumentException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 233 } 234 public Enumeration<DiagnosticReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.NULL, code); 239 String codeString = ((PrimitiveType) code).asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.NULL, code); 242 if ("registered".equals(codeString)) 243 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.REGISTERED, code); 244 if ("partial".equals(codeString)) 245 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PARTIAL, code); 246 if ("preliminary".equals(codeString)) 247 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.PRELIMINARY, code); 248 if ("modified".equals(codeString)) 249 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.MODIFIED, code); 250 if ("final".equals(codeString)) 251 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.FINAL, code); 252 if ("amended".equals(codeString)) 253 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.AMENDED, code); 254 if ("corrected".equals(codeString)) 255 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CORRECTED, code); 256 if ("appended".equals(codeString)) 257 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.APPENDED, code); 258 if ("cancelled".equals(codeString)) 259 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.CANCELLED, code); 260 if ("entered-in-error".equals(codeString)) 261 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.ENTEREDINERROR, code); 262 if ("unknown".equals(codeString)) 263 return new Enumeration<DiagnosticReportStatus>(this, DiagnosticReportStatus.UNKNOWN, code); 264 throw new FHIRException("Unknown DiagnosticReportStatus code '"+codeString+"'"); 265 } 266 public String toCode(DiagnosticReportStatus code) { 267 if (code == DiagnosticReportStatus.NULL) 268 return null; 269 if (code == DiagnosticReportStatus.REGISTERED) 270 return "registered"; 271 if (code == DiagnosticReportStatus.PARTIAL) 272 return "partial"; 273 if (code == DiagnosticReportStatus.PRELIMINARY) 274 return "preliminary"; 275 if (code == DiagnosticReportStatus.MODIFIED) 276 return "modified"; 277 if (code == DiagnosticReportStatus.FINAL) 278 return "final"; 279 if (code == DiagnosticReportStatus.AMENDED) 280 return "amended"; 281 if (code == DiagnosticReportStatus.CORRECTED) 282 return "corrected"; 283 if (code == DiagnosticReportStatus.APPENDED) 284 return "appended"; 285 if (code == DiagnosticReportStatus.CANCELLED) 286 return "cancelled"; 287 if (code == DiagnosticReportStatus.ENTEREDINERROR) 288 return "entered-in-error"; 289 if (code == DiagnosticReportStatus.UNKNOWN) 290 return "unknown"; 291 return "?"; 292 } 293 public String toSystem(DiagnosticReportStatus code) { 294 return code.getSystem(); 295 } 296 } 297 298 @Block() 299 public static class DiagnosticReportSupportingInfoComponent extends BackboneElement implements IBaseBackboneElement { 300 /** 301 * The code value for the role of the supporting information in the diagnostic report. 302 */ 303 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 304 @Description(shortDefinition="Supporting information role code", formalDefinition="The code value for the role of the supporting information in the diagnostic report." ) 305 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0936") 306 protected CodeableConcept type; 307 308 /** 309 * The reference for the supporting information in the diagnostic report. 310 */ 311 @Child(name = "reference", type = {Procedure.class, Observation.class, DiagnosticReport.class, Citation.class}, order=2, min=1, max=1, modifier=false, summary=false) 312 @Description(shortDefinition="Supporting information reference", formalDefinition="The reference for the supporting information in the diagnostic report." ) 313 protected Reference reference; 314 315 private static final long serialVersionUID = 492391425L; 316 317 /** 318 * Constructor 319 */ 320 public DiagnosticReportSupportingInfoComponent() { 321 super(); 322 } 323 324 /** 325 * Constructor 326 */ 327 public DiagnosticReportSupportingInfoComponent(CodeableConcept type, Reference reference) { 328 super(); 329 this.setType(type); 330 this.setReference(reference); 331 } 332 333 /** 334 * @return {@link #type} (The code value for the role of the supporting information in the diagnostic report.) 335 */ 336 public CodeableConcept getType() { 337 if (this.type == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create DiagnosticReportSupportingInfoComponent.type"); 340 else if (Configuration.doAutoCreate()) 341 this.type = new CodeableConcept(); // cc 342 return this.type; 343 } 344 345 public boolean hasType() { 346 return this.type != null && !this.type.isEmpty(); 347 } 348 349 /** 350 * @param value {@link #type} (The code value for the role of the supporting information in the diagnostic report.) 351 */ 352 public DiagnosticReportSupportingInfoComponent setType(CodeableConcept value) { 353 this.type = value; 354 return this; 355 } 356 357 /** 358 * @return {@link #reference} (The reference for the supporting information in the diagnostic report.) 359 */ 360 public Reference getReference() { 361 if (this.reference == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create DiagnosticReportSupportingInfoComponent.reference"); 364 else if (Configuration.doAutoCreate()) 365 this.reference = new Reference(); // cc 366 return this.reference; 367 } 368 369 public boolean hasReference() { 370 return this.reference != null && !this.reference.isEmpty(); 371 } 372 373 /** 374 * @param value {@link #reference} (The reference for the supporting information in the diagnostic report.) 375 */ 376 public DiagnosticReportSupportingInfoComponent setReference(Reference value) { 377 this.reference = value; 378 return this; 379 } 380 381 protected void listChildren(List<Property> children) { 382 super.listChildren(children); 383 children.add(new Property("type", "CodeableConcept", "The code value for the role of the supporting information in the diagnostic report.", 0, 1, type)); 384 children.add(new Property("reference", "Reference(Procedure|Observation|DiagnosticReport|Citation)", "The reference for the supporting information in the diagnostic report.", 0, 1, reference)); 385 } 386 387 @Override 388 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 389 switch (_hash) { 390 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The code value for the role of the supporting information in the diagnostic report.", 0, 1, type); 391 case -925155509: /*reference*/ return new Property("reference", "Reference(Procedure|Observation|DiagnosticReport|Citation)", "The reference for the supporting information in the diagnostic report.", 0, 1, reference); 392 default: return super.getNamedProperty(_hash, _name, _checkValid); 393 } 394 395 } 396 397 @Override 398 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 399 switch (hash) { 400 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 401 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 402 default: return super.getProperty(hash, name, checkValid); 403 } 404 405 } 406 407 @Override 408 public Base setProperty(int hash, String name, Base value) throws FHIRException { 409 switch (hash) { 410 case 3575610: // type 411 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 412 return value; 413 case -925155509: // reference 414 this.reference = TypeConvertor.castToReference(value); // Reference 415 return value; 416 default: return super.setProperty(hash, name, value); 417 } 418 419 } 420 421 @Override 422 public Base setProperty(String name, Base value) throws FHIRException { 423 if (name.equals("type")) { 424 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 425 } else if (name.equals("reference")) { 426 this.reference = TypeConvertor.castToReference(value); // Reference 427 } else 428 return super.setProperty(name, value); 429 return value; 430 } 431 432 @Override 433 public void removeChild(String name, Base value) throws FHIRException { 434 if (name.equals("type")) { 435 this.type = null; 436 } else if (name.equals("reference")) { 437 this.reference = null; 438 } else 439 super.removeChild(name, value); 440 441 } 442 443 @Override 444 public Base makeProperty(int hash, String name) throws FHIRException { 445 switch (hash) { 446 case 3575610: return getType(); 447 case -925155509: return getReference(); 448 default: return super.makeProperty(hash, name); 449 } 450 451 } 452 453 @Override 454 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 455 switch (hash) { 456 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 457 case -925155509: /*reference*/ return new String[] {"Reference"}; 458 default: return super.getTypesForProperty(hash, name); 459 } 460 461 } 462 463 @Override 464 public Base addChild(String name) throws FHIRException { 465 if (name.equals("type")) { 466 this.type = new CodeableConcept(); 467 return this.type; 468 } 469 else if (name.equals("reference")) { 470 this.reference = new Reference(); 471 return this.reference; 472 } 473 else 474 return super.addChild(name); 475 } 476 477 public DiagnosticReportSupportingInfoComponent copy() { 478 DiagnosticReportSupportingInfoComponent dst = new DiagnosticReportSupportingInfoComponent(); 479 copyValues(dst); 480 return dst; 481 } 482 483 public void copyValues(DiagnosticReportSupportingInfoComponent dst) { 484 super.copyValues(dst); 485 dst.type = type == null ? null : type.copy(); 486 dst.reference = reference == null ? null : reference.copy(); 487 } 488 489 @Override 490 public boolean equalsDeep(Base other_) { 491 if (!super.equalsDeep(other_)) 492 return false; 493 if (!(other_ instanceof DiagnosticReportSupportingInfoComponent)) 494 return false; 495 DiagnosticReportSupportingInfoComponent o = (DiagnosticReportSupportingInfoComponent) other_; 496 return compareDeep(type, o.type, true) && compareDeep(reference, o.reference, true); 497 } 498 499 @Override 500 public boolean equalsShallow(Base other_) { 501 if (!super.equalsShallow(other_)) 502 return false; 503 if (!(other_ instanceof DiagnosticReportSupportingInfoComponent)) 504 return false; 505 DiagnosticReportSupportingInfoComponent o = (DiagnosticReportSupportingInfoComponent) other_; 506 return true; 507 } 508 509 public boolean isEmpty() { 510 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, reference); 511 } 512 513 public String fhirType() { 514 return "DiagnosticReport.supportingInfo"; 515 516 } 517 518 } 519 520 @Block() 521 public static class DiagnosticReportMediaComponent extends BackboneElement implements IBaseBackboneElement { 522 /** 523 * A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features. 524 */ 525 @Child(name = "comment", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 526 @Description(shortDefinition="Comment about the image or data (e.g. explanation)", formalDefinition="A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features." ) 527 protected StringType comment; 528 529 /** 530 * Reference to the image or data source. 531 */ 532 @Child(name = "link", type = {DocumentReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 533 @Description(shortDefinition="Reference to the image or data source", formalDefinition="Reference to the image or data source." ) 534 protected Reference link; 535 536 private static final long serialVersionUID = 1827561947L; 537 538 /** 539 * Constructor 540 */ 541 public DiagnosticReportMediaComponent() { 542 super(); 543 } 544 545 /** 546 * Constructor 547 */ 548 public DiagnosticReportMediaComponent(Reference link) { 549 super(); 550 this.setLink(link); 551 } 552 553 /** 554 * @return {@link #comment} (A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 555 */ 556 public StringType getCommentElement() { 557 if (this.comment == null) 558 if (Configuration.errorOnAutoCreate()) 559 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.comment"); 560 else if (Configuration.doAutoCreate()) 561 this.comment = new StringType(); // bb 562 return this.comment; 563 } 564 565 public boolean hasCommentElement() { 566 return this.comment != null && !this.comment.isEmpty(); 567 } 568 569 public boolean hasComment() { 570 return this.comment != null && !this.comment.isEmpty(); 571 } 572 573 /** 574 * @param value {@link #comment} (A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 575 */ 576 public DiagnosticReportMediaComponent setCommentElement(StringType value) { 577 this.comment = value; 578 return this; 579 } 580 581 /** 582 * @return A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features. 583 */ 584 public String getComment() { 585 return this.comment == null ? null : this.comment.getValue(); 586 } 587 588 /** 589 * @param value A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features. 590 */ 591 public DiagnosticReportMediaComponent setComment(String value) { 592 if (Utilities.noString(value)) 593 this.comment = null; 594 else { 595 if (this.comment == null) 596 this.comment = new StringType(); 597 this.comment.setValue(value); 598 } 599 return this; 600 } 601 602 /** 603 * @return {@link #link} (Reference to the image or data source.) 604 */ 605 public Reference getLink() { 606 if (this.link == null) 607 if (Configuration.errorOnAutoCreate()) 608 throw new Error("Attempt to auto-create DiagnosticReportMediaComponent.link"); 609 else if (Configuration.doAutoCreate()) 610 this.link = new Reference(); // cc 611 return this.link; 612 } 613 614 public boolean hasLink() { 615 return this.link != null && !this.link.isEmpty(); 616 } 617 618 /** 619 * @param value {@link #link} (Reference to the image or data source.) 620 */ 621 public DiagnosticReportMediaComponent setLink(Reference value) { 622 this.link = value; 623 return this; 624 } 625 626 protected void listChildren(List<Property> children) { 627 super.listChildren(children); 628 children.add(new Property("comment", "string", "A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features.", 0, 1, comment)); 629 children.add(new Property("link", "Reference(DocumentReference)", "Reference to the image or data source.", 0, 1, link)); 630 } 631 632 @Override 633 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 634 switch (_hash) { 635 case 950398559: /*comment*/ return new Property("comment", "string", "A comment about the image or data. Typically, this is used to provide an explanation for why the image or data is included, or to draw the viewer's attention to important features.", 0, 1, comment); 636 case 3321850: /*link*/ return new Property("link", "Reference(DocumentReference)", "Reference to the image or data source.", 0, 1, link); 637 default: return super.getNamedProperty(_hash, _name, _checkValid); 638 } 639 640 } 641 642 @Override 643 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 644 switch (hash) { 645 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 646 case 3321850: /*link*/ return this.link == null ? new Base[0] : new Base[] {this.link}; // Reference 647 default: return super.getProperty(hash, name, checkValid); 648 } 649 650 } 651 652 @Override 653 public Base setProperty(int hash, String name, Base value) throws FHIRException { 654 switch (hash) { 655 case 950398559: // comment 656 this.comment = TypeConvertor.castToString(value); // StringType 657 return value; 658 case 3321850: // link 659 this.link = TypeConvertor.castToReference(value); // Reference 660 return value; 661 default: return super.setProperty(hash, name, value); 662 } 663 664 } 665 666 @Override 667 public Base setProperty(String name, Base value) throws FHIRException { 668 if (name.equals("comment")) { 669 this.comment = TypeConvertor.castToString(value); // StringType 670 } else if (name.equals("link")) { 671 this.link = TypeConvertor.castToReference(value); // Reference 672 } else 673 return super.setProperty(name, value); 674 return value; 675 } 676 677 @Override 678 public void removeChild(String name, Base value) throws FHIRException { 679 if (name.equals("comment")) { 680 this.comment = null; 681 } else if (name.equals("link")) { 682 this.link = null; 683 } else 684 super.removeChild(name, value); 685 686 } 687 688 @Override 689 public Base makeProperty(int hash, String name) throws FHIRException { 690 switch (hash) { 691 case 950398559: return getCommentElement(); 692 case 3321850: return getLink(); 693 default: return super.makeProperty(hash, name); 694 } 695 696 } 697 698 @Override 699 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 700 switch (hash) { 701 case 950398559: /*comment*/ return new String[] {"string"}; 702 case 3321850: /*link*/ return new String[] {"Reference"}; 703 default: return super.getTypesForProperty(hash, name); 704 } 705 706 } 707 708 @Override 709 public Base addChild(String name) throws FHIRException { 710 if (name.equals("comment")) { 711 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.media.comment"); 712 } 713 else if (name.equals("link")) { 714 this.link = new Reference(); 715 return this.link; 716 } 717 else 718 return super.addChild(name); 719 } 720 721 public DiagnosticReportMediaComponent copy() { 722 DiagnosticReportMediaComponent dst = new DiagnosticReportMediaComponent(); 723 copyValues(dst); 724 return dst; 725 } 726 727 public void copyValues(DiagnosticReportMediaComponent dst) { 728 super.copyValues(dst); 729 dst.comment = comment == null ? null : comment.copy(); 730 dst.link = link == null ? null : link.copy(); 731 } 732 733 @Override 734 public boolean equalsDeep(Base other_) { 735 if (!super.equalsDeep(other_)) 736 return false; 737 if (!(other_ instanceof DiagnosticReportMediaComponent)) 738 return false; 739 DiagnosticReportMediaComponent o = (DiagnosticReportMediaComponent) other_; 740 return compareDeep(comment, o.comment, true) && compareDeep(link, o.link, true); 741 } 742 743 @Override 744 public boolean equalsShallow(Base other_) { 745 if (!super.equalsShallow(other_)) 746 return false; 747 if (!(other_ instanceof DiagnosticReportMediaComponent)) 748 return false; 749 DiagnosticReportMediaComponent o = (DiagnosticReportMediaComponent) other_; 750 return compareValues(comment, o.comment, true); 751 } 752 753 public boolean isEmpty() { 754 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, link); 755 } 756 757 public String fhirType() { 758 return "DiagnosticReport.media"; 759 760 } 761 762 } 763 764 /** 765 * Identifiers assigned to this report by the performer or other systems. 766 */ 767 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 768 @Description(shortDefinition="Business identifier for report", formalDefinition="Identifiers assigned to this report by the performer or other systems." ) 769 protected List<Identifier> identifier; 770 771 /** 772 * Details concerning a service requested. 773 */ 774 @Child(name = "basedOn", type = {CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 775 @Description(shortDefinition="What was requested", formalDefinition="Details concerning a service requested." ) 776 protected List<Reference> basedOn; 777 778 /** 779 * The status of the diagnostic report. 780 */ 781 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 782 @Description(shortDefinition="registered | partial | preliminary | modified | final | amended | corrected | appended | cancelled | entered-in-error | unknown", formalDefinition="The status of the diagnostic report." ) 783 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnostic-report-status") 784 protected Enumeration<DiagnosticReportStatus> status; 785 786 /** 787 * A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes. 788 */ 789 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 790 @Description(shortDefinition="Service category", formalDefinition="A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes." ) 791 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnostic-service-sections") 792 protected List<CodeableConcept> category; 793 794 /** 795 * A code or name that describes this diagnostic report. 796 */ 797 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 798 @Description(shortDefinition="Name/Code for this diagnostic report", formalDefinition="A code or name that describes this diagnostic report." ) 799 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-codes") 800 protected CodeableConcept code; 801 802 /** 803 * The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources. 804 */ 805 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class, Organization.class, Practitioner.class, Medication.class, Substance.class, BiologicallyDerivedProduct.class}, order=5, min=0, max=1, modifier=false, summary=true) 806 @Description(shortDefinition="The subject of the report - usually, but not always, the patient", formalDefinition="The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources." ) 807 protected Reference subject; 808 809 /** 810 * The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about. 811 */ 812 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 813 @Description(shortDefinition="Health care event when test ordered", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about." ) 814 protected Reference encounter; 815 816 /** 817 * The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself. 818 */ 819 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 820 @Description(shortDefinition="Clinically relevant time/time-period for report", formalDefinition="The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself." ) 821 protected DataType effective; 822 823 /** 824 * The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified. 825 */ 826 @Child(name = "issued", type = {InstantType.class}, order=8, min=0, max=1, modifier=false, summary=true) 827 @Description(shortDefinition="DateTime this version was made", formalDefinition="The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified." ) 828 protected InstantType issued; 829 830 /** 831 * The diagnostic service that is responsible for issuing the report. 832 */ 833 @Child(name = "performer", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 834 @Description(shortDefinition="Responsible Diagnostic Service", formalDefinition="The diagnostic service that is responsible for issuing the report." ) 835 protected List<Reference> performer; 836 837 /** 838 * The practitioner or organization that is responsible for the report's conclusions and interpretations. 839 */ 840 @Child(name = "resultsInterpreter", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 841 @Description(shortDefinition="Primary result interpreter", formalDefinition="The practitioner or organization that is responsible for the report's conclusions and interpretations." ) 842 protected List<Reference> resultsInterpreter; 843 844 /** 845 * Details about the specimens on which this diagnostic report is based. 846 */ 847 @Child(name = "specimen", type = {Specimen.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 848 @Description(shortDefinition="Specimens this report is based on", formalDefinition="Details about the specimens on which this diagnostic report is based." ) 849 protected List<Reference> specimen; 850 851 /** 852 * [Observations](observation.html) that are part of this diagnostic report. 853 */ 854 @Child(name = "result", type = {Observation.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 855 @Description(shortDefinition="Observations", formalDefinition="[Observations](observation.html) that are part of this diagnostic report." ) 856 protected List<Reference> result; 857 858 /** 859 * Comments about the diagnostic report. 860 */ 861 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 862 @Description(shortDefinition="Comments about the diagnostic report", formalDefinition="Comments about the diagnostic report." ) 863 protected List<Annotation> note; 864 865 /** 866 * One or more links to full details of any study performed during the diagnostic investigation. An ImagingStudy might comprise a set of radiologic images obtained via a procedure that are analyzed as a group. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images. A GenomicStudy might comprise one or more analyses, each serving a specific purpose. These analyses may vary in method (e.g., karyotyping, CNV, or SNV detection), performer, software, devices used, or regions targeted. 867 */ 868 @Child(name = "study", type = {GenomicStudy.class, ImagingStudy.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 869 @Description(shortDefinition="Reference to full details of an analysis associated with the diagnostic report", formalDefinition="One or more links to full details of any study performed during the diagnostic investigation. An ImagingStudy might comprise a set of radiologic images obtained via a procedure that are analyzed as a group. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images. A GenomicStudy might comprise one or more analyses, each serving a specific purpose. These analyses may vary in method (e.g., karyotyping, CNV, or SNV detection), performer, software, devices used, or regions targeted." ) 870 protected List<Reference> study; 871 872 /** 873 * This backbone element contains supporting information that was used in the creation of the report not included in the results already included in the report. 874 */ 875 @Child(name = "supportingInfo", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 876 @Description(shortDefinition="Additional information supporting the diagnostic report", formalDefinition="This backbone element contains supporting information that was used in the creation of the report not included in the results already included in the report." ) 877 protected List<DiagnosticReportSupportingInfoComponent> supportingInfo; 878 879 /** 880 * A list of key images or data associated with this report. The images or data are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest). 881 */ 882 @Child(name = "media", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 883 @Description(shortDefinition="Key images or data associated with this report", formalDefinition="A list of key images or data associated with this report. The images or data are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest)." ) 884 protected List<DiagnosticReportMediaComponent> media; 885 886 /** 887 * Reference to a Composition resource instance that provides structure for organizing the contents of the DiagnosticReport. 888 */ 889 @Child(name = "composition", type = {Composition.class}, order=17, min=0, max=1, modifier=false, summary=false) 890 @Description(shortDefinition="Reference to a Composition resource for the DiagnosticReport structure", formalDefinition="Reference to a Composition resource instance that provides structure for organizing the contents of the DiagnosticReport." ) 891 protected Reference composition; 892 893 /** 894 * Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report. 895 */ 896 @Child(name = "conclusion", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 897 @Description(shortDefinition="Clinical conclusion (interpretation) of test results", formalDefinition="Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report." ) 898 protected MarkdownType conclusion; 899 900 /** 901 * One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report. 902 */ 903 @Child(name = "conclusionCode", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 904 @Description(shortDefinition="Codes for the clinical conclusion of test results", formalDefinition="One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report." ) 905 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 906 protected List<CodeableConcept> conclusionCode; 907 908 /** 909 * Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent. 910 */ 911 @Child(name = "presentedForm", type = {Attachment.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 912 @Description(shortDefinition="Entire report as issued", formalDefinition="Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent." ) 913 protected List<Attachment> presentedForm; 914 915 private static final long serialVersionUID = -530178625L; 916 917 /** 918 * Constructor 919 */ 920 public DiagnosticReport() { 921 super(); 922 } 923 924 /** 925 * Constructor 926 */ 927 public DiagnosticReport(DiagnosticReportStatus status, CodeableConcept code) { 928 super(); 929 this.setStatus(status); 930 this.setCode(code); 931 } 932 933 /** 934 * @return {@link #identifier} (Identifiers assigned to this report by the performer or other systems.) 935 */ 936 public List<Identifier> getIdentifier() { 937 if (this.identifier == null) 938 this.identifier = new ArrayList<Identifier>(); 939 return this.identifier; 940 } 941 942 /** 943 * @return Returns a reference to <code>this</code> for easy method chaining 944 */ 945 public DiagnosticReport setIdentifier(List<Identifier> theIdentifier) { 946 this.identifier = theIdentifier; 947 return this; 948 } 949 950 public boolean hasIdentifier() { 951 if (this.identifier == null) 952 return false; 953 for (Identifier item : this.identifier) 954 if (!item.isEmpty()) 955 return true; 956 return false; 957 } 958 959 public Identifier addIdentifier() { //3 960 Identifier t = new Identifier(); 961 if (this.identifier == null) 962 this.identifier = new ArrayList<Identifier>(); 963 this.identifier.add(t); 964 return t; 965 } 966 967 public DiagnosticReport addIdentifier(Identifier t) { //3 968 if (t == null) 969 return this; 970 if (this.identifier == null) 971 this.identifier = new ArrayList<Identifier>(); 972 this.identifier.add(t); 973 return this; 974 } 975 976 /** 977 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 978 */ 979 public Identifier getIdentifierFirstRep() { 980 if (getIdentifier().isEmpty()) { 981 addIdentifier(); 982 } 983 return getIdentifier().get(0); 984 } 985 986 /** 987 * @return {@link #basedOn} (Details concerning a service requested.) 988 */ 989 public List<Reference> getBasedOn() { 990 if (this.basedOn == null) 991 this.basedOn = new ArrayList<Reference>(); 992 return this.basedOn; 993 } 994 995 /** 996 * @return Returns a reference to <code>this</code> for easy method chaining 997 */ 998 public DiagnosticReport setBasedOn(List<Reference> theBasedOn) { 999 this.basedOn = theBasedOn; 1000 return this; 1001 } 1002 1003 public boolean hasBasedOn() { 1004 if (this.basedOn == null) 1005 return false; 1006 for (Reference item : this.basedOn) 1007 if (!item.isEmpty()) 1008 return true; 1009 return false; 1010 } 1011 1012 public Reference addBasedOn() { //3 1013 Reference t = new Reference(); 1014 if (this.basedOn == null) 1015 this.basedOn = new ArrayList<Reference>(); 1016 this.basedOn.add(t); 1017 return t; 1018 } 1019 1020 public DiagnosticReport addBasedOn(Reference t) { //3 1021 if (t == null) 1022 return this; 1023 if (this.basedOn == null) 1024 this.basedOn = new ArrayList<Reference>(); 1025 this.basedOn.add(t); 1026 return this; 1027 } 1028 1029 /** 1030 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1031 */ 1032 public Reference getBasedOnFirstRep() { 1033 if (getBasedOn().isEmpty()) { 1034 addBasedOn(); 1035 } 1036 return getBasedOn().get(0); 1037 } 1038 1039 /** 1040 * @return {@link #status} (The status of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1041 */ 1042 public Enumeration<DiagnosticReportStatus> getStatusElement() { 1043 if (this.status == null) 1044 if (Configuration.errorOnAutoCreate()) 1045 throw new Error("Attempt to auto-create DiagnosticReport.status"); 1046 else if (Configuration.doAutoCreate()) 1047 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); // bb 1048 return this.status; 1049 } 1050 1051 public boolean hasStatusElement() { 1052 return this.status != null && !this.status.isEmpty(); 1053 } 1054 1055 public boolean hasStatus() { 1056 return this.status != null && !this.status.isEmpty(); 1057 } 1058 1059 /** 1060 * @param value {@link #status} (The status of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1061 */ 1062 public DiagnosticReport setStatusElement(Enumeration<DiagnosticReportStatus> value) { 1063 this.status = value; 1064 return this; 1065 } 1066 1067 /** 1068 * @return The status of the diagnostic report. 1069 */ 1070 public DiagnosticReportStatus getStatus() { 1071 return this.status == null ? null : this.status.getValue(); 1072 } 1073 1074 /** 1075 * @param value The status of the diagnostic report. 1076 */ 1077 public DiagnosticReport setStatus(DiagnosticReportStatus value) { 1078 if (this.status == null) 1079 this.status = new Enumeration<DiagnosticReportStatus>(new DiagnosticReportStatusEnumFactory()); 1080 this.status.setValue(value); 1081 return this; 1082 } 1083 1084 /** 1085 * @return {@link #category} (A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.) 1086 */ 1087 public List<CodeableConcept> getCategory() { 1088 if (this.category == null) 1089 this.category = new ArrayList<CodeableConcept>(); 1090 return this.category; 1091 } 1092 1093 /** 1094 * @return Returns a reference to <code>this</code> for easy method chaining 1095 */ 1096 public DiagnosticReport setCategory(List<CodeableConcept> theCategory) { 1097 this.category = theCategory; 1098 return this; 1099 } 1100 1101 public boolean hasCategory() { 1102 if (this.category == null) 1103 return false; 1104 for (CodeableConcept item : this.category) 1105 if (!item.isEmpty()) 1106 return true; 1107 return false; 1108 } 1109 1110 public CodeableConcept addCategory() { //3 1111 CodeableConcept t = new CodeableConcept(); 1112 if (this.category == null) 1113 this.category = new ArrayList<CodeableConcept>(); 1114 this.category.add(t); 1115 return t; 1116 } 1117 1118 public DiagnosticReport addCategory(CodeableConcept t) { //3 1119 if (t == null) 1120 return this; 1121 if (this.category == null) 1122 this.category = new ArrayList<CodeableConcept>(); 1123 this.category.add(t); 1124 return this; 1125 } 1126 1127 /** 1128 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1129 */ 1130 public CodeableConcept getCategoryFirstRep() { 1131 if (getCategory().isEmpty()) { 1132 addCategory(); 1133 } 1134 return getCategory().get(0); 1135 } 1136 1137 /** 1138 * @return {@link #code} (A code or name that describes this diagnostic report.) 1139 */ 1140 public CodeableConcept getCode() { 1141 if (this.code == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create DiagnosticReport.code"); 1144 else if (Configuration.doAutoCreate()) 1145 this.code = new CodeableConcept(); // cc 1146 return this.code; 1147 } 1148 1149 public boolean hasCode() { 1150 return this.code != null && !this.code.isEmpty(); 1151 } 1152 1153 /** 1154 * @param value {@link #code} (A code or name that describes this diagnostic report.) 1155 */ 1156 public DiagnosticReport setCode(CodeableConcept value) { 1157 this.code = value; 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #subject} (The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1163 */ 1164 public Reference getSubject() { 1165 if (this.subject == null) 1166 if (Configuration.errorOnAutoCreate()) 1167 throw new Error("Attempt to auto-create DiagnosticReport.subject"); 1168 else if (Configuration.doAutoCreate()) 1169 this.subject = new Reference(); // cc 1170 return this.subject; 1171 } 1172 1173 public boolean hasSubject() { 1174 return this.subject != null && !this.subject.isEmpty(); 1175 } 1176 1177 /** 1178 * @param value {@link #subject} (The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.) 1179 */ 1180 public DiagnosticReport setSubject(Reference value) { 1181 this.subject = value; 1182 return this; 1183 } 1184 1185 /** 1186 * @return {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.) 1187 */ 1188 public Reference getEncounter() { 1189 if (this.encounter == null) 1190 if (Configuration.errorOnAutoCreate()) 1191 throw new Error("Attempt to auto-create DiagnosticReport.encounter"); 1192 else if (Configuration.doAutoCreate()) 1193 this.encounter = new Reference(); // cc 1194 return this.encounter; 1195 } 1196 1197 public boolean hasEncounter() { 1198 return this.encounter != null && !this.encounter.isEmpty(); 1199 } 1200 1201 /** 1202 * @param value {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.) 1203 */ 1204 public DiagnosticReport setEncounter(Reference value) { 1205 this.encounter = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1211 */ 1212 public DataType getEffective() { 1213 return this.effective; 1214 } 1215 1216 /** 1217 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1218 */ 1219 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1220 if (this.effective == null) 1221 this.effective = new DateTimeType(); 1222 if (!(this.effective instanceof DateTimeType)) 1223 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 1224 return (DateTimeType) this.effective; 1225 } 1226 1227 public boolean hasEffectiveDateTimeType() { 1228 return this != null && this.effective instanceof DateTimeType; 1229 } 1230 1231 /** 1232 * @return {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1233 */ 1234 public Period getEffectivePeriod() throws FHIRException { 1235 if (this.effective == null) 1236 this.effective = new Period(); 1237 if (!(this.effective instanceof Period)) 1238 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 1239 return (Period) this.effective; 1240 } 1241 1242 public boolean hasEffectivePeriod() { 1243 return this != null && this.effective instanceof Period; 1244 } 1245 1246 public boolean hasEffective() { 1247 return this.effective != null && !this.effective.isEmpty(); 1248 } 1249 1250 /** 1251 * @param value {@link #effective} (The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.) 1252 */ 1253 public DiagnosticReport setEffective(DataType value) { 1254 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1255 throw new FHIRException("Not the right type for DiagnosticReport.effective[x]: "+value.fhirType()); 1256 this.effective = value; 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #issued} (The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1262 */ 1263 public InstantType getIssuedElement() { 1264 if (this.issued == null) 1265 if (Configuration.errorOnAutoCreate()) 1266 throw new Error("Attempt to auto-create DiagnosticReport.issued"); 1267 else if (Configuration.doAutoCreate()) 1268 this.issued = new InstantType(); // bb 1269 return this.issued; 1270 } 1271 1272 public boolean hasIssuedElement() { 1273 return this.issued != null && !this.issued.isEmpty(); 1274 } 1275 1276 public boolean hasIssued() { 1277 return this.issued != null && !this.issued.isEmpty(); 1278 } 1279 1280 /** 1281 * @param value {@link #issued} (The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 1282 */ 1283 public DiagnosticReport setIssuedElement(InstantType value) { 1284 this.issued = value; 1285 return this; 1286 } 1287 1288 /** 1289 * @return The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified. 1290 */ 1291 public Date getIssued() { 1292 return this.issued == null ? null : this.issued.getValue(); 1293 } 1294 1295 /** 1296 * @param value The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified. 1297 */ 1298 public DiagnosticReport setIssued(Date value) { 1299 if (value == null) 1300 this.issued = null; 1301 else { 1302 if (this.issued == null) 1303 this.issued = new InstantType(); 1304 this.issued.setValue(value); 1305 } 1306 return this; 1307 } 1308 1309 /** 1310 * @return {@link #performer} (The diagnostic service that is responsible for issuing the report.) 1311 */ 1312 public List<Reference> getPerformer() { 1313 if (this.performer == null) 1314 this.performer = new ArrayList<Reference>(); 1315 return this.performer; 1316 } 1317 1318 /** 1319 * @return Returns a reference to <code>this</code> for easy method chaining 1320 */ 1321 public DiagnosticReport setPerformer(List<Reference> thePerformer) { 1322 this.performer = thePerformer; 1323 return this; 1324 } 1325 1326 public boolean hasPerformer() { 1327 if (this.performer == null) 1328 return false; 1329 for (Reference item : this.performer) 1330 if (!item.isEmpty()) 1331 return true; 1332 return false; 1333 } 1334 1335 public Reference addPerformer() { //3 1336 Reference t = new Reference(); 1337 if (this.performer == null) 1338 this.performer = new ArrayList<Reference>(); 1339 this.performer.add(t); 1340 return t; 1341 } 1342 1343 public DiagnosticReport addPerformer(Reference t) { //3 1344 if (t == null) 1345 return this; 1346 if (this.performer == null) 1347 this.performer = new ArrayList<Reference>(); 1348 this.performer.add(t); 1349 return this; 1350 } 1351 1352 /** 1353 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1354 */ 1355 public Reference getPerformerFirstRep() { 1356 if (getPerformer().isEmpty()) { 1357 addPerformer(); 1358 } 1359 return getPerformer().get(0); 1360 } 1361 1362 /** 1363 * @return {@link #resultsInterpreter} (The practitioner or organization that is responsible for the report's conclusions and interpretations.) 1364 */ 1365 public List<Reference> getResultsInterpreter() { 1366 if (this.resultsInterpreter == null) 1367 this.resultsInterpreter = new ArrayList<Reference>(); 1368 return this.resultsInterpreter; 1369 } 1370 1371 /** 1372 * @return Returns a reference to <code>this</code> for easy method chaining 1373 */ 1374 public DiagnosticReport setResultsInterpreter(List<Reference> theResultsInterpreter) { 1375 this.resultsInterpreter = theResultsInterpreter; 1376 return this; 1377 } 1378 1379 public boolean hasResultsInterpreter() { 1380 if (this.resultsInterpreter == null) 1381 return false; 1382 for (Reference item : this.resultsInterpreter) 1383 if (!item.isEmpty()) 1384 return true; 1385 return false; 1386 } 1387 1388 public Reference addResultsInterpreter() { //3 1389 Reference t = new Reference(); 1390 if (this.resultsInterpreter == null) 1391 this.resultsInterpreter = new ArrayList<Reference>(); 1392 this.resultsInterpreter.add(t); 1393 return t; 1394 } 1395 1396 public DiagnosticReport addResultsInterpreter(Reference t) { //3 1397 if (t == null) 1398 return this; 1399 if (this.resultsInterpreter == null) 1400 this.resultsInterpreter = new ArrayList<Reference>(); 1401 this.resultsInterpreter.add(t); 1402 return this; 1403 } 1404 1405 /** 1406 * @return The first repetition of repeating field {@link #resultsInterpreter}, creating it if it does not already exist {3} 1407 */ 1408 public Reference getResultsInterpreterFirstRep() { 1409 if (getResultsInterpreter().isEmpty()) { 1410 addResultsInterpreter(); 1411 } 1412 return getResultsInterpreter().get(0); 1413 } 1414 1415 /** 1416 * @return {@link #specimen} (Details about the specimens on which this diagnostic report is based.) 1417 */ 1418 public List<Reference> getSpecimen() { 1419 if (this.specimen == null) 1420 this.specimen = new ArrayList<Reference>(); 1421 return this.specimen; 1422 } 1423 1424 /** 1425 * @return Returns a reference to <code>this</code> for easy method chaining 1426 */ 1427 public DiagnosticReport setSpecimen(List<Reference> theSpecimen) { 1428 this.specimen = theSpecimen; 1429 return this; 1430 } 1431 1432 public boolean hasSpecimen() { 1433 if (this.specimen == null) 1434 return false; 1435 for (Reference item : this.specimen) 1436 if (!item.isEmpty()) 1437 return true; 1438 return false; 1439 } 1440 1441 public Reference addSpecimen() { //3 1442 Reference t = new Reference(); 1443 if (this.specimen == null) 1444 this.specimen = new ArrayList<Reference>(); 1445 this.specimen.add(t); 1446 return t; 1447 } 1448 1449 public DiagnosticReport addSpecimen(Reference t) { //3 1450 if (t == null) 1451 return this; 1452 if (this.specimen == null) 1453 this.specimen = new ArrayList<Reference>(); 1454 this.specimen.add(t); 1455 return this; 1456 } 1457 1458 /** 1459 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist {3} 1460 */ 1461 public Reference getSpecimenFirstRep() { 1462 if (getSpecimen().isEmpty()) { 1463 addSpecimen(); 1464 } 1465 return getSpecimen().get(0); 1466 } 1467 1468 /** 1469 * @return {@link #result} ([Observations](observation.html) that are part of this diagnostic report.) 1470 */ 1471 public List<Reference> getResult() { 1472 if (this.result == null) 1473 this.result = new ArrayList<Reference>(); 1474 return this.result; 1475 } 1476 1477 /** 1478 * @return Returns a reference to <code>this</code> for easy method chaining 1479 */ 1480 public DiagnosticReport setResult(List<Reference> theResult) { 1481 this.result = theResult; 1482 return this; 1483 } 1484 1485 public boolean hasResult() { 1486 if (this.result == null) 1487 return false; 1488 for (Reference item : this.result) 1489 if (!item.isEmpty()) 1490 return true; 1491 return false; 1492 } 1493 1494 public Reference addResult() { //3 1495 Reference t = new Reference(); 1496 if (this.result == null) 1497 this.result = new ArrayList<Reference>(); 1498 this.result.add(t); 1499 return t; 1500 } 1501 1502 public DiagnosticReport addResult(Reference t) { //3 1503 if (t == null) 1504 return this; 1505 if (this.result == null) 1506 this.result = new ArrayList<Reference>(); 1507 this.result.add(t); 1508 return this; 1509 } 1510 1511 /** 1512 * @return The first repetition of repeating field {@link #result}, creating it if it does not already exist {3} 1513 */ 1514 public Reference getResultFirstRep() { 1515 if (getResult().isEmpty()) { 1516 addResult(); 1517 } 1518 return getResult().get(0); 1519 } 1520 1521 /** 1522 * @return {@link #note} (Comments about the diagnostic report.) 1523 */ 1524 public List<Annotation> getNote() { 1525 if (this.note == null) 1526 this.note = new ArrayList<Annotation>(); 1527 return this.note; 1528 } 1529 1530 /** 1531 * @return Returns a reference to <code>this</code> for easy method chaining 1532 */ 1533 public DiagnosticReport setNote(List<Annotation> theNote) { 1534 this.note = theNote; 1535 return this; 1536 } 1537 1538 public boolean hasNote() { 1539 if (this.note == null) 1540 return false; 1541 for (Annotation item : this.note) 1542 if (!item.isEmpty()) 1543 return true; 1544 return false; 1545 } 1546 1547 public Annotation addNote() { //3 1548 Annotation t = new Annotation(); 1549 if (this.note == null) 1550 this.note = new ArrayList<Annotation>(); 1551 this.note.add(t); 1552 return t; 1553 } 1554 1555 public DiagnosticReport addNote(Annotation t) { //3 1556 if (t == null) 1557 return this; 1558 if (this.note == null) 1559 this.note = new ArrayList<Annotation>(); 1560 this.note.add(t); 1561 return this; 1562 } 1563 1564 /** 1565 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1566 */ 1567 public Annotation getNoteFirstRep() { 1568 if (getNote().isEmpty()) { 1569 addNote(); 1570 } 1571 return getNote().get(0); 1572 } 1573 1574 /** 1575 * @return {@link #study} (One or more links to full details of any study performed during the diagnostic investigation. An ImagingStudy might comprise a set of radiologic images obtained via a procedure that are analyzed as a group. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images. A GenomicStudy might comprise one or more analyses, each serving a specific purpose. These analyses may vary in method (e.g., karyotyping, CNV, or SNV detection), performer, software, devices used, or regions targeted.) 1576 */ 1577 public List<Reference> getStudy() { 1578 if (this.study == null) 1579 this.study = new ArrayList<Reference>(); 1580 return this.study; 1581 } 1582 1583 /** 1584 * @return Returns a reference to <code>this</code> for easy method chaining 1585 */ 1586 public DiagnosticReport setStudy(List<Reference> theStudy) { 1587 this.study = theStudy; 1588 return this; 1589 } 1590 1591 public boolean hasStudy() { 1592 if (this.study == null) 1593 return false; 1594 for (Reference item : this.study) 1595 if (!item.isEmpty()) 1596 return true; 1597 return false; 1598 } 1599 1600 public Reference addStudy() { //3 1601 Reference t = new Reference(); 1602 if (this.study == null) 1603 this.study = new ArrayList<Reference>(); 1604 this.study.add(t); 1605 return t; 1606 } 1607 1608 public DiagnosticReport addStudy(Reference t) { //3 1609 if (t == null) 1610 return this; 1611 if (this.study == null) 1612 this.study = new ArrayList<Reference>(); 1613 this.study.add(t); 1614 return this; 1615 } 1616 1617 /** 1618 * @return The first repetition of repeating field {@link #study}, creating it if it does not already exist {3} 1619 */ 1620 public Reference getStudyFirstRep() { 1621 if (getStudy().isEmpty()) { 1622 addStudy(); 1623 } 1624 return getStudy().get(0); 1625 } 1626 1627 /** 1628 * @return {@link #supportingInfo} (This backbone element contains supporting information that was used in the creation of the report not included in the results already included in the report.) 1629 */ 1630 public List<DiagnosticReportSupportingInfoComponent> getSupportingInfo() { 1631 if (this.supportingInfo == null) 1632 this.supportingInfo = new ArrayList<DiagnosticReportSupportingInfoComponent>(); 1633 return this.supportingInfo; 1634 } 1635 1636 /** 1637 * @return Returns a reference to <code>this</code> for easy method chaining 1638 */ 1639 public DiagnosticReport setSupportingInfo(List<DiagnosticReportSupportingInfoComponent> theSupportingInfo) { 1640 this.supportingInfo = theSupportingInfo; 1641 return this; 1642 } 1643 1644 public boolean hasSupportingInfo() { 1645 if (this.supportingInfo == null) 1646 return false; 1647 for (DiagnosticReportSupportingInfoComponent item : this.supportingInfo) 1648 if (!item.isEmpty()) 1649 return true; 1650 return false; 1651 } 1652 1653 public DiagnosticReportSupportingInfoComponent addSupportingInfo() { //3 1654 DiagnosticReportSupportingInfoComponent t = new DiagnosticReportSupportingInfoComponent(); 1655 if (this.supportingInfo == null) 1656 this.supportingInfo = new ArrayList<DiagnosticReportSupportingInfoComponent>(); 1657 this.supportingInfo.add(t); 1658 return t; 1659 } 1660 1661 public DiagnosticReport addSupportingInfo(DiagnosticReportSupportingInfoComponent t) { //3 1662 if (t == null) 1663 return this; 1664 if (this.supportingInfo == null) 1665 this.supportingInfo = new ArrayList<DiagnosticReportSupportingInfoComponent>(); 1666 this.supportingInfo.add(t); 1667 return this; 1668 } 1669 1670 /** 1671 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 1672 */ 1673 public DiagnosticReportSupportingInfoComponent getSupportingInfoFirstRep() { 1674 if (getSupportingInfo().isEmpty()) { 1675 addSupportingInfo(); 1676 } 1677 return getSupportingInfo().get(0); 1678 } 1679 1680 /** 1681 * @return {@link #media} (A list of key images or data associated with this report. The images or data are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).) 1682 */ 1683 public List<DiagnosticReportMediaComponent> getMedia() { 1684 if (this.media == null) 1685 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1686 return this.media; 1687 } 1688 1689 /** 1690 * @return Returns a reference to <code>this</code> for easy method chaining 1691 */ 1692 public DiagnosticReport setMedia(List<DiagnosticReportMediaComponent> theMedia) { 1693 this.media = theMedia; 1694 return this; 1695 } 1696 1697 public boolean hasMedia() { 1698 if (this.media == null) 1699 return false; 1700 for (DiagnosticReportMediaComponent item : this.media) 1701 if (!item.isEmpty()) 1702 return true; 1703 return false; 1704 } 1705 1706 public DiagnosticReportMediaComponent addMedia() { //3 1707 DiagnosticReportMediaComponent t = new DiagnosticReportMediaComponent(); 1708 if (this.media == null) 1709 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1710 this.media.add(t); 1711 return t; 1712 } 1713 1714 public DiagnosticReport addMedia(DiagnosticReportMediaComponent t) { //3 1715 if (t == null) 1716 return this; 1717 if (this.media == null) 1718 this.media = new ArrayList<DiagnosticReportMediaComponent>(); 1719 this.media.add(t); 1720 return this; 1721 } 1722 1723 /** 1724 * @return The first repetition of repeating field {@link #media}, creating it if it does not already exist {3} 1725 */ 1726 public DiagnosticReportMediaComponent getMediaFirstRep() { 1727 if (getMedia().isEmpty()) { 1728 addMedia(); 1729 } 1730 return getMedia().get(0); 1731 } 1732 1733 /** 1734 * @return {@link #composition} (Reference to a Composition resource instance that provides structure for organizing the contents of the DiagnosticReport.) 1735 */ 1736 public Reference getComposition() { 1737 if (this.composition == null) 1738 if (Configuration.errorOnAutoCreate()) 1739 throw new Error("Attempt to auto-create DiagnosticReport.composition"); 1740 else if (Configuration.doAutoCreate()) 1741 this.composition = new Reference(); // cc 1742 return this.composition; 1743 } 1744 1745 public boolean hasComposition() { 1746 return this.composition != null && !this.composition.isEmpty(); 1747 } 1748 1749 /** 1750 * @param value {@link #composition} (Reference to a Composition resource instance that provides structure for organizing the contents of the DiagnosticReport.) 1751 */ 1752 public DiagnosticReport setComposition(Reference value) { 1753 this.composition = value; 1754 return this; 1755 } 1756 1757 /** 1758 * @return {@link #conclusion} (Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getConclusion" gives direct access to the value 1759 */ 1760 public MarkdownType getConclusionElement() { 1761 if (this.conclusion == null) 1762 if (Configuration.errorOnAutoCreate()) 1763 throw new Error("Attempt to auto-create DiagnosticReport.conclusion"); 1764 else if (Configuration.doAutoCreate()) 1765 this.conclusion = new MarkdownType(); // bb 1766 return this.conclusion; 1767 } 1768 1769 public boolean hasConclusionElement() { 1770 return this.conclusion != null && !this.conclusion.isEmpty(); 1771 } 1772 1773 public boolean hasConclusion() { 1774 return this.conclusion != null && !this.conclusion.isEmpty(); 1775 } 1776 1777 /** 1778 * @param value {@link #conclusion} (Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.). This is the underlying object with id, value and extensions. The accessor "getConclusion" gives direct access to the value 1779 */ 1780 public DiagnosticReport setConclusionElement(MarkdownType value) { 1781 this.conclusion = value; 1782 return this; 1783 } 1784 1785 /** 1786 * @return Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report. 1787 */ 1788 public String getConclusion() { 1789 return this.conclusion == null ? null : this.conclusion.getValue(); 1790 } 1791 1792 /** 1793 * @param value Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report. 1794 */ 1795 public DiagnosticReport setConclusion(String value) { 1796 if (Utilities.noString(value)) 1797 this.conclusion = null; 1798 else { 1799 if (this.conclusion == null) 1800 this.conclusion = new MarkdownType(); 1801 this.conclusion.setValue(value); 1802 } 1803 return this; 1804 } 1805 1806 /** 1807 * @return {@link #conclusionCode} (One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.) 1808 */ 1809 public List<CodeableConcept> getConclusionCode() { 1810 if (this.conclusionCode == null) 1811 this.conclusionCode = new ArrayList<CodeableConcept>(); 1812 return this.conclusionCode; 1813 } 1814 1815 /** 1816 * @return Returns a reference to <code>this</code> for easy method chaining 1817 */ 1818 public DiagnosticReport setConclusionCode(List<CodeableConcept> theConclusionCode) { 1819 this.conclusionCode = theConclusionCode; 1820 return this; 1821 } 1822 1823 public boolean hasConclusionCode() { 1824 if (this.conclusionCode == null) 1825 return false; 1826 for (CodeableConcept item : this.conclusionCode) 1827 if (!item.isEmpty()) 1828 return true; 1829 return false; 1830 } 1831 1832 public CodeableConcept addConclusionCode() { //3 1833 CodeableConcept t = new CodeableConcept(); 1834 if (this.conclusionCode == null) 1835 this.conclusionCode = new ArrayList<CodeableConcept>(); 1836 this.conclusionCode.add(t); 1837 return t; 1838 } 1839 1840 public DiagnosticReport addConclusionCode(CodeableConcept t) { //3 1841 if (t == null) 1842 return this; 1843 if (this.conclusionCode == null) 1844 this.conclusionCode = new ArrayList<CodeableConcept>(); 1845 this.conclusionCode.add(t); 1846 return this; 1847 } 1848 1849 /** 1850 * @return The first repetition of repeating field {@link #conclusionCode}, creating it if it does not already exist {3} 1851 */ 1852 public CodeableConcept getConclusionCodeFirstRep() { 1853 if (getConclusionCode().isEmpty()) { 1854 addConclusionCode(); 1855 } 1856 return getConclusionCode().get(0); 1857 } 1858 1859 /** 1860 * @return {@link #presentedForm} (Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.) 1861 */ 1862 public List<Attachment> getPresentedForm() { 1863 if (this.presentedForm == null) 1864 this.presentedForm = new ArrayList<Attachment>(); 1865 return this.presentedForm; 1866 } 1867 1868 /** 1869 * @return Returns a reference to <code>this</code> for easy method chaining 1870 */ 1871 public DiagnosticReport setPresentedForm(List<Attachment> thePresentedForm) { 1872 this.presentedForm = thePresentedForm; 1873 return this; 1874 } 1875 1876 public boolean hasPresentedForm() { 1877 if (this.presentedForm == null) 1878 return false; 1879 for (Attachment item : this.presentedForm) 1880 if (!item.isEmpty()) 1881 return true; 1882 return false; 1883 } 1884 1885 public Attachment addPresentedForm() { //3 1886 Attachment t = new Attachment(); 1887 if (this.presentedForm == null) 1888 this.presentedForm = new ArrayList<Attachment>(); 1889 this.presentedForm.add(t); 1890 return t; 1891 } 1892 1893 public DiagnosticReport addPresentedForm(Attachment t) { //3 1894 if (t == null) 1895 return this; 1896 if (this.presentedForm == null) 1897 this.presentedForm = new ArrayList<Attachment>(); 1898 this.presentedForm.add(t); 1899 return this; 1900 } 1901 1902 /** 1903 * @return The first repetition of repeating field {@link #presentedForm}, creating it if it does not already exist {3} 1904 */ 1905 public Attachment getPresentedFormFirstRep() { 1906 if (getPresentedForm().isEmpty()) { 1907 addPresentedForm(); 1908 } 1909 return getPresentedForm().get(0); 1910 } 1911 1912 protected void listChildren(List<Property> children) { 1913 super.listChildren(children); 1914 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1915 children.add(new Property("basedOn", "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", "Details concerning a service requested.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1916 children.add(new Property("status", "code", "The status of the diagnostic report.", 0, 1, status)); 1917 children.add(new Property("category", "CodeableConcept", "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category)); 1918 children.add(new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code)); 1919 children.add(new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Practitioner|Medication|Substance|BiologicallyDerivedProduct)", "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.", 0, 1, subject)); 1920 children.add(new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.", 0, 1, encounter)); 1921 children.add(new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective)); 1922 children.add(new Property("issued", "instant", "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.", 0, 1, issued)); 1923 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", "The diagnostic service that is responsible for issuing the report.", 0, java.lang.Integer.MAX_VALUE, performer)); 1924 children.add(new Property("resultsInterpreter", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", "The practitioner or organization that is responsible for the report's conclusions and interpretations.", 0, java.lang.Integer.MAX_VALUE, resultsInterpreter)); 1925 children.add(new Property("specimen", "Reference(Specimen)", "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, specimen)); 1926 children.add(new Property("result", "Reference(Observation)", "[Observations](observation.html) that are part of this diagnostic report.", 0, java.lang.Integer.MAX_VALUE, result)); 1927 children.add(new Property("note", "Annotation", "Comments about the diagnostic report.", 0, java.lang.Integer.MAX_VALUE, note)); 1928 children.add(new Property("study", "Reference(GenomicStudy|ImagingStudy)", "One or more links to full details of any study performed during the diagnostic investigation. An ImagingStudy might comprise a set of radiologic images obtained via a procedure that are analyzed as a group. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images. A GenomicStudy might comprise one or more analyses, each serving a specific purpose. These analyses may vary in method (e.g., karyotyping, CNV, or SNV detection), performer, software, devices used, or regions targeted.", 0, java.lang.Integer.MAX_VALUE, study)); 1929 children.add(new Property("supportingInfo", "", "This backbone element contains supporting information that was used in the creation of the report not included in the results already included in the report.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 1930 children.add(new Property("media", "", "A list of key images or data associated with this report. The images or data are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 0, java.lang.Integer.MAX_VALUE, media)); 1931 children.add(new Property("composition", "Reference(Composition)", "Reference to a Composition resource instance that provides structure for organizing the contents of the DiagnosticReport.", 0, 1, composition)); 1932 children.add(new Property("conclusion", "markdown", "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.", 0, 1, conclusion)); 1933 children.add(new Property("conclusionCode", "CodeableConcept", "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.", 0, java.lang.Integer.MAX_VALUE, conclusionCode)); 1934 children.add(new Property("presentedForm", "Attachment", "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 0, java.lang.Integer.MAX_VALUE, presentedForm)); 1935 } 1936 1937 @Override 1938 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1939 switch (_hash) { 1940 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this report by the performer or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1941 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", "Details concerning a service requested.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1942 case -892481550: /*status*/ return new Property("status", "code", "The status of the diagnostic report.", 0, 1, status); 1943 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the clinical discipline, department or diagnostic service that created the report (e.g. cardiology, biochemistry, hematology, MRI). This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category); 1944 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code or name that describes this diagnostic report.", 0, 1, code); 1945 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Practitioner|Medication|Substance|BiologicallyDerivedProduct)", "The subject of the report. Usually, but not always, this is a patient. However, diagnostic services also perform analyses on specimens collected from a variety of other sources.", 0, 1, subject); 1946 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) which this DiagnosticReport is about.", 0, 1, encounter); 1947 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1948 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1949 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1950 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "Period", "The time or time-period the observed values are related to. When the subject of the report is a patient, this is usually either the time of the procedure or of specimen collection(s), but very often the source of the date/time is not known, only the date/time itself.", 0, 1, effective); 1951 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time that this version of the report was made available to providers, typically after the report was reviewed and verified.", 0, 1, issued); 1952 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", "The diagnostic service that is responsible for issuing the report.", 0, java.lang.Integer.MAX_VALUE, performer); 1953 case 2134944932: /*resultsInterpreter*/ return new Property("resultsInterpreter", "Reference(Practitioner|PractitionerRole|Organization|CareTeam)", "The practitioner or organization that is responsible for the report's conclusions and interpretations.", 0, java.lang.Integer.MAX_VALUE, resultsInterpreter); 1954 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "Details about the specimens on which this diagnostic report is based.", 0, java.lang.Integer.MAX_VALUE, specimen); 1955 case -934426595: /*result*/ return new Property("result", "Reference(Observation)", "[Observations](observation.html) that are part of this diagnostic report.", 0, java.lang.Integer.MAX_VALUE, result); 1956 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments about the diagnostic report.", 0, java.lang.Integer.MAX_VALUE, note); 1957 case 109776329: /*study*/ return new Property("study", "Reference(GenomicStudy|ImagingStudy)", "One or more links to full details of any study performed during the diagnostic investigation. An ImagingStudy might comprise a set of radiologic images obtained via a procedure that are analyzed as a group. Typically, this is imaging performed by DICOM enabled modalities, but this is not required. A fully enabled PACS viewer can use this information to provide views of the source images. A GenomicStudy might comprise one or more analyses, each serving a specific purpose. These analyses may vary in method (e.g., karyotyping, CNV, or SNV detection), performer, software, devices used, or regions targeted.", 0, java.lang.Integer.MAX_VALUE, study); 1958 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "This backbone element contains supporting information that was used in the creation of the report not included in the results already included in the report.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 1959 case 103772132: /*media*/ return new Property("media", "", "A list of key images or data associated with this report. The images or data are generally created during the diagnostic process, and may be directly of the patient, or of treated specimens (i.e. slides of interest).", 0, java.lang.Integer.MAX_VALUE, media); 1960 case -838923862: /*composition*/ return new Property("composition", "Reference(Composition)", "Reference to a Composition resource instance that provides structure for organizing the contents of the DiagnosticReport.", 0, 1, composition); 1961 case -1731259873: /*conclusion*/ return new Property("conclusion", "markdown", "Concise and clinically contextualized summary conclusion (interpretation/impression) of the diagnostic report.", 0, 1, conclusion); 1962 case -1731523412: /*conclusionCode*/ return new Property("conclusionCode", "CodeableConcept", "One or more codes that represent the summary conclusion (interpretation/impression) of the diagnostic report.", 0, java.lang.Integer.MAX_VALUE, conclusionCode); 1963 case 230090366: /*presentedForm*/ return new Property("presentedForm", "Attachment", "Rich text representation of the entire result as issued by the diagnostic service. Multiple formats are allowed but they SHALL be semantically equivalent.", 0, java.lang.Integer.MAX_VALUE, presentedForm); 1964 default: return super.getNamedProperty(_hash, _name, _checkValid); 1965 } 1966 1967 } 1968 1969 @Override 1970 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1971 switch (hash) { 1972 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1973 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1974 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DiagnosticReportStatus> 1975 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1976 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1977 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1978 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1979 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // DataType 1980 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 1981 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 1982 case 2134944932: /*resultsInterpreter*/ return this.resultsInterpreter == null ? new Base[0] : this.resultsInterpreter.toArray(new Base[this.resultsInterpreter.size()]); // Reference 1983 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 1984 case -934426595: /*result*/ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 1985 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1986 case 109776329: /*study*/ return this.study == null ? new Base[0] : this.study.toArray(new Base[this.study.size()]); // Reference 1987 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // DiagnosticReportSupportingInfoComponent 1988 case 103772132: /*media*/ return this.media == null ? new Base[0] : this.media.toArray(new Base[this.media.size()]); // DiagnosticReportMediaComponent 1989 case -838923862: /*composition*/ return this.composition == null ? new Base[0] : new Base[] {this.composition}; // Reference 1990 case -1731259873: /*conclusion*/ return this.conclusion == null ? new Base[0] : new Base[] {this.conclusion}; // MarkdownType 1991 case -1731523412: /*conclusionCode*/ return this.conclusionCode == null ? new Base[0] : this.conclusionCode.toArray(new Base[this.conclusionCode.size()]); // CodeableConcept 1992 case 230090366: /*presentedForm*/ return this.presentedForm == null ? new Base[0] : this.presentedForm.toArray(new Base[this.presentedForm.size()]); // Attachment 1993 default: return super.getProperty(hash, name, checkValid); 1994 } 1995 1996 } 1997 1998 @Override 1999 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2000 switch (hash) { 2001 case -1618432855: // identifier 2002 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2003 return value; 2004 case -332612366: // basedOn 2005 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2006 return value; 2007 case -892481550: // status 2008 value = new DiagnosticReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2009 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 2010 return value; 2011 case 50511102: // category 2012 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2013 return value; 2014 case 3059181: // code 2015 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2016 return value; 2017 case -1867885268: // subject 2018 this.subject = TypeConvertor.castToReference(value); // Reference 2019 return value; 2020 case 1524132147: // encounter 2021 this.encounter = TypeConvertor.castToReference(value); // Reference 2022 return value; 2023 case -1468651097: // effective 2024 this.effective = TypeConvertor.castToType(value); // DataType 2025 return value; 2026 case -1179159893: // issued 2027 this.issued = TypeConvertor.castToInstant(value); // InstantType 2028 return value; 2029 case 481140686: // performer 2030 this.getPerformer().add(TypeConvertor.castToReference(value)); // Reference 2031 return value; 2032 case 2134944932: // resultsInterpreter 2033 this.getResultsInterpreter().add(TypeConvertor.castToReference(value)); // Reference 2034 return value; 2035 case -2132868344: // specimen 2036 this.getSpecimen().add(TypeConvertor.castToReference(value)); // Reference 2037 return value; 2038 case -934426595: // result 2039 this.getResult().add(TypeConvertor.castToReference(value)); // Reference 2040 return value; 2041 case 3387378: // note 2042 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2043 return value; 2044 case 109776329: // study 2045 this.getStudy().add(TypeConvertor.castToReference(value)); // Reference 2046 return value; 2047 case 1922406657: // supportingInfo 2048 this.getSupportingInfo().add((DiagnosticReportSupportingInfoComponent) value); // DiagnosticReportSupportingInfoComponent 2049 return value; 2050 case 103772132: // media 2051 this.getMedia().add((DiagnosticReportMediaComponent) value); // DiagnosticReportMediaComponent 2052 return value; 2053 case -838923862: // composition 2054 this.composition = TypeConvertor.castToReference(value); // Reference 2055 return value; 2056 case -1731259873: // conclusion 2057 this.conclusion = TypeConvertor.castToMarkdown(value); // MarkdownType 2058 return value; 2059 case -1731523412: // conclusionCode 2060 this.getConclusionCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2061 return value; 2062 case 230090366: // presentedForm 2063 this.getPresentedForm().add(TypeConvertor.castToAttachment(value)); // Attachment 2064 return value; 2065 default: return super.setProperty(hash, name, value); 2066 } 2067 2068 } 2069 2070 @Override 2071 public Base setProperty(String name, Base value) throws FHIRException { 2072 if (name.equals("identifier")) { 2073 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2074 } else if (name.equals("basedOn")) { 2075 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2076 } else if (name.equals("status")) { 2077 value = new DiagnosticReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2078 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 2079 } else if (name.equals("category")) { 2080 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2081 } else if (name.equals("code")) { 2082 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2083 } else if (name.equals("subject")) { 2084 this.subject = TypeConvertor.castToReference(value); // Reference 2085 } else if (name.equals("encounter")) { 2086 this.encounter = TypeConvertor.castToReference(value); // Reference 2087 } else if (name.equals("effective[x]")) { 2088 this.effective = TypeConvertor.castToType(value); // DataType 2089 } else if (name.equals("issued")) { 2090 this.issued = TypeConvertor.castToInstant(value); // InstantType 2091 } else if (name.equals("performer")) { 2092 this.getPerformer().add(TypeConvertor.castToReference(value)); 2093 } else if (name.equals("resultsInterpreter")) { 2094 this.getResultsInterpreter().add(TypeConvertor.castToReference(value)); 2095 } else if (name.equals("specimen")) { 2096 this.getSpecimen().add(TypeConvertor.castToReference(value)); 2097 } else if (name.equals("result")) { 2098 this.getResult().add(TypeConvertor.castToReference(value)); 2099 } else if (name.equals("note")) { 2100 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2101 } else if (name.equals("study")) { 2102 this.getStudy().add(TypeConvertor.castToReference(value)); 2103 } else if (name.equals("supportingInfo")) { 2104 this.getSupportingInfo().add((DiagnosticReportSupportingInfoComponent) value); 2105 } else if (name.equals("media")) { 2106 this.getMedia().add((DiagnosticReportMediaComponent) value); 2107 } else if (name.equals("composition")) { 2108 this.composition = TypeConvertor.castToReference(value); // Reference 2109 } else if (name.equals("conclusion")) { 2110 this.conclusion = TypeConvertor.castToMarkdown(value); // MarkdownType 2111 } else if (name.equals("conclusionCode")) { 2112 this.getConclusionCode().add(TypeConvertor.castToCodeableConcept(value)); 2113 } else if (name.equals("presentedForm")) { 2114 this.getPresentedForm().add(TypeConvertor.castToAttachment(value)); 2115 } else 2116 return super.setProperty(name, value); 2117 return value; 2118 } 2119 2120 @Override 2121 public void removeChild(String name, Base value) throws FHIRException { 2122 if (name.equals("identifier")) { 2123 this.getIdentifier().remove(value); 2124 } else if (name.equals("basedOn")) { 2125 this.getBasedOn().remove(value); 2126 } else if (name.equals("status")) { 2127 value = new DiagnosticReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2128 this.status = (Enumeration) value; // Enumeration<DiagnosticReportStatus> 2129 } else if (name.equals("category")) { 2130 this.getCategory().remove(value); 2131 } else if (name.equals("code")) { 2132 this.code = null; 2133 } else if (name.equals("subject")) { 2134 this.subject = null; 2135 } else if (name.equals("encounter")) { 2136 this.encounter = null; 2137 } else if (name.equals("effective[x]")) { 2138 this.effective = null; 2139 } else if (name.equals("issued")) { 2140 this.issued = null; 2141 } else if (name.equals("performer")) { 2142 this.getPerformer().remove(value); 2143 } else if (name.equals("resultsInterpreter")) { 2144 this.getResultsInterpreter().remove(value); 2145 } else if (name.equals("specimen")) { 2146 this.getSpecimen().remove(value); 2147 } else if (name.equals("result")) { 2148 this.getResult().remove(value); 2149 } else if (name.equals("note")) { 2150 this.getNote().remove(value); 2151 } else if (name.equals("study")) { 2152 this.getStudy().remove(value); 2153 } else if (name.equals("supportingInfo")) { 2154 this.getSupportingInfo().remove((DiagnosticReportSupportingInfoComponent) value); 2155 } else if (name.equals("media")) { 2156 this.getMedia().remove((DiagnosticReportMediaComponent) value); 2157 } else if (name.equals("composition")) { 2158 this.composition = null; 2159 } else if (name.equals("conclusion")) { 2160 this.conclusion = null; 2161 } else if (name.equals("conclusionCode")) { 2162 this.getConclusionCode().remove(value); 2163 } else if (name.equals("presentedForm")) { 2164 this.getPresentedForm().remove(value); 2165 } else 2166 super.removeChild(name, value); 2167 2168 } 2169 2170 @Override 2171 public Base makeProperty(int hash, String name) throws FHIRException { 2172 switch (hash) { 2173 case -1618432855: return addIdentifier(); 2174 case -332612366: return addBasedOn(); 2175 case -892481550: return getStatusElement(); 2176 case 50511102: return addCategory(); 2177 case 3059181: return getCode(); 2178 case -1867885268: return getSubject(); 2179 case 1524132147: return getEncounter(); 2180 case 247104889: return getEffective(); 2181 case -1468651097: return getEffective(); 2182 case -1179159893: return getIssuedElement(); 2183 case 481140686: return addPerformer(); 2184 case 2134944932: return addResultsInterpreter(); 2185 case -2132868344: return addSpecimen(); 2186 case -934426595: return addResult(); 2187 case 3387378: return addNote(); 2188 case 109776329: return addStudy(); 2189 case 1922406657: return addSupportingInfo(); 2190 case 103772132: return addMedia(); 2191 case -838923862: return getComposition(); 2192 case -1731259873: return getConclusionElement(); 2193 case -1731523412: return addConclusionCode(); 2194 case 230090366: return addPresentedForm(); 2195 default: return super.makeProperty(hash, name); 2196 } 2197 2198 } 2199 2200 @Override 2201 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2202 switch (hash) { 2203 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2204 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2205 case -892481550: /*status*/ return new String[] {"code"}; 2206 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2207 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2208 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2209 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2210 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 2211 case -1179159893: /*issued*/ return new String[] {"instant"}; 2212 case 481140686: /*performer*/ return new String[] {"Reference"}; 2213 case 2134944932: /*resultsInterpreter*/ return new String[] {"Reference"}; 2214 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 2215 case -934426595: /*result*/ return new String[] {"Reference"}; 2216 case 3387378: /*note*/ return new String[] {"Annotation"}; 2217 case 109776329: /*study*/ return new String[] {"Reference"}; 2218 case 1922406657: /*supportingInfo*/ return new String[] {}; 2219 case 103772132: /*media*/ return new String[] {}; 2220 case -838923862: /*composition*/ return new String[] {"Reference"}; 2221 case -1731259873: /*conclusion*/ return new String[] {"markdown"}; 2222 case -1731523412: /*conclusionCode*/ return new String[] {"CodeableConcept"}; 2223 case 230090366: /*presentedForm*/ return new String[] {"Attachment"}; 2224 default: return super.getTypesForProperty(hash, name); 2225 } 2226 2227 } 2228 2229 @Override 2230 public Base addChild(String name) throws FHIRException { 2231 if (name.equals("identifier")) { 2232 return addIdentifier(); 2233 } 2234 else if (name.equals("basedOn")) { 2235 return addBasedOn(); 2236 } 2237 else if (name.equals("status")) { 2238 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.status"); 2239 } 2240 else if (name.equals("category")) { 2241 return addCategory(); 2242 } 2243 else if (name.equals("code")) { 2244 this.code = new CodeableConcept(); 2245 return this.code; 2246 } 2247 else if (name.equals("subject")) { 2248 this.subject = new Reference(); 2249 return this.subject; 2250 } 2251 else if (name.equals("encounter")) { 2252 this.encounter = new Reference(); 2253 return this.encounter; 2254 } 2255 else if (name.equals("effectiveDateTime")) { 2256 this.effective = new DateTimeType(); 2257 return this.effective; 2258 } 2259 else if (name.equals("effectivePeriod")) { 2260 this.effective = new Period(); 2261 return this.effective; 2262 } 2263 else if (name.equals("issued")) { 2264 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.issued"); 2265 } 2266 else if (name.equals("performer")) { 2267 return addPerformer(); 2268 } 2269 else if (name.equals("resultsInterpreter")) { 2270 return addResultsInterpreter(); 2271 } 2272 else if (name.equals("specimen")) { 2273 return addSpecimen(); 2274 } 2275 else if (name.equals("result")) { 2276 return addResult(); 2277 } 2278 else if (name.equals("note")) { 2279 return addNote(); 2280 } 2281 else if (name.equals("study")) { 2282 return addStudy(); 2283 } 2284 else if (name.equals("supportingInfo")) { 2285 return addSupportingInfo(); 2286 } 2287 else if (name.equals("media")) { 2288 return addMedia(); 2289 } 2290 else if (name.equals("composition")) { 2291 this.composition = new Reference(); 2292 return this.composition; 2293 } 2294 else if (name.equals("conclusion")) { 2295 throw new FHIRException("Cannot call addChild on a singleton property DiagnosticReport.conclusion"); 2296 } 2297 else if (name.equals("conclusionCode")) { 2298 return addConclusionCode(); 2299 } 2300 else if (name.equals("presentedForm")) { 2301 return addPresentedForm(); 2302 } 2303 else 2304 return super.addChild(name); 2305 } 2306 2307 public String fhirType() { 2308 return "DiagnosticReport"; 2309 2310 } 2311 2312 public DiagnosticReport copy() { 2313 DiagnosticReport dst = new DiagnosticReport(); 2314 copyValues(dst); 2315 return dst; 2316 } 2317 2318 public void copyValues(DiagnosticReport dst) { 2319 super.copyValues(dst); 2320 if (identifier != null) { 2321 dst.identifier = new ArrayList<Identifier>(); 2322 for (Identifier i : identifier) 2323 dst.identifier.add(i.copy()); 2324 }; 2325 if (basedOn != null) { 2326 dst.basedOn = new ArrayList<Reference>(); 2327 for (Reference i : basedOn) 2328 dst.basedOn.add(i.copy()); 2329 }; 2330 dst.status = status == null ? null : status.copy(); 2331 if (category != null) { 2332 dst.category = new ArrayList<CodeableConcept>(); 2333 for (CodeableConcept i : category) 2334 dst.category.add(i.copy()); 2335 }; 2336 dst.code = code == null ? null : code.copy(); 2337 dst.subject = subject == null ? null : subject.copy(); 2338 dst.encounter = encounter == null ? null : encounter.copy(); 2339 dst.effective = effective == null ? null : effective.copy(); 2340 dst.issued = issued == null ? null : issued.copy(); 2341 if (performer != null) { 2342 dst.performer = new ArrayList<Reference>(); 2343 for (Reference i : performer) 2344 dst.performer.add(i.copy()); 2345 }; 2346 if (resultsInterpreter != null) { 2347 dst.resultsInterpreter = new ArrayList<Reference>(); 2348 for (Reference i : resultsInterpreter) 2349 dst.resultsInterpreter.add(i.copy()); 2350 }; 2351 if (specimen != null) { 2352 dst.specimen = new ArrayList<Reference>(); 2353 for (Reference i : specimen) 2354 dst.specimen.add(i.copy()); 2355 }; 2356 if (result != null) { 2357 dst.result = new ArrayList<Reference>(); 2358 for (Reference i : result) 2359 dst.result.add(i.copy()); 2360 }; 2361 if (note != null) { 2362 dst.note = new ArrayList<Annotation>(); 2363 for (Annotation i : note) 2364 dst.note.add(i.copy()); 2365 }; 2366 if (study != null) { 2367 dst.study = new ArrayList<Reference>(); 2368 for (Reference i : study) 2369 dst.study.add(i.copy()); 2370 }; 2371 if (supportingInfo != null) { 2372 dst.supportingInfo = new ArrayList<DiagnosticReportSupportingInfoComponent>(); 2373 for (DiagnosticReportSupportingInfoComponent i : supportingInfo) 2374 dst.supportingInfo.add(i.copy()); 2375 }; 2376 if (media != null) { 2377 dst.media = new ArrayList<DiagnosticReportMediaComponent>(); 2378 for (DiagnosticReportMediaComponent i : media) 2379 dst.media.add(i.copy()); 2380 }; 2381 dst.composition = composition == null ? null : composition.copy(); 2382 dst.conclusion = conclusion == null ? null : conclusion.copy(); 2383 if (conclusionCode != null) { 2384 dst.conclusionCode = new ArrayList<CodeableConcept>(); 2385 for (CodeableConcept i : conclusionCode) 2386 dst.conclusionCode.add(i.copy()); 2387 }; 2388 if (presentedForm != null) { 2389 dst.presentedForm = new ArrayList<Attachment>(); 2390 for (Attachment i : presentedForm) 2391 dst.presentedForm.add(i.copy()); 2392 }; 2393 } 2394 2395 protected DiagnosticReport typedCopy() { 2396 return copy(); 2397 } 2398 2399 @Override 2400 public boolean equalsDeep(Base other_) { 2401 if (!super.equalsDeep(other_)) 2402 return false; 2403 if (!(other_ instanceof DiagnosticReport)) 2404 return false; 2405 DiagnosticReport o = (DiagnosticReport) other_; 2406 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(status, o.status, true) 2407 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 2408 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) && compareDeep(issued, o.issued, true) 2409 && compareDeep(performer, o.performer, true) && compareDeep(resultsInterpreter, o.resultsInterpreter, true) 2410 && compareDeep(specimen, o.specimen, true) && compareDeep(result, o.result, true) && compareDeep(note, o.note, true) 2411 && compareDeep(study, o.study, true) && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(media, o.media, true) 2412 && compareDeep(composition, o.composition, true) && compareDeep(conclusion, o.conclusion, true) 2413 && compareDeep(conclusionCode, o.conclusionCode, true) && compareDeep(presentedForm, o.presentedForm, true) 2414 ; 2415 } 2416 2417 @Override 2418 public boolean equalsShallow(Base other_) { 2419 if (!super.equalsShallow(other_)) 2420 return false; 2421 if (!(other_ instanceof DiagnosticReport)) 2422 return false; 2423 DiagnosticReport o = (DiagnosticReport) other_; 2424 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) && compareValues(conclusion, o.conclusion, true) 2425 ; 2426 } 2427 2428 public boolean isEmpty() { 2429 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status 2430 , category, code, subject, encounter, effective, issued, performer, resultsInterpreter 2431 , specimen, result, note, study, supportingInfo, media, composition, conclusion 2432 , conclusionCode, presentedForm); 2433 } 2434 2435 @Override 2436 public ResourceType getResourceType() { 2437 return ResourceType.DiagnosticReport; 2438 } 2439 2440 /** 2441 * Search parameter: <b>based-on</b> 2442 * <p> 2443 * Description: <b>Reference to the service request.</b><br> 2444 * Type: <b>reference</b><br> 2445 * Path: <b>DiagnosticReport.basedOn</b><br> 2446 * </p> 2447 */ 2448 @SearchParamDefinition(name="based-on", path="DiagnosticReport.basedOn", description="Reference to the service request.", type="reference", target={CarePlan.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class } ) 2449 public static final String SP_BASED_ON = "based-on"; 2450 /** 2451 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2452 * <p> 2453 * Description: <b>Reference to the service request.</b><br> 2454 * Type: <b>reference</b><br> 2455 * Path: <b>DiagnosticReport.basedOn</b><br> 2456 * </p> 2457 */ 2458 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2459 2460/** 2461 * Constant for fluent queries to be used to add include statements. Specifies 2462 * the path value of "<b>DiagnosticReport:based-on</b>". 2463 */ 2464 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("DiagnosticReport:based-on").toLocked(); 2465 2466 /** 2467 * Search parameter: <b>category</b> 2468 * <p> 2469 * Description: <b>Which diagnostic discipline/department created the report</b><br> 2470 * Type: <b>token</b><br> 2471 * Path: <b>DiagnosticReport.category</b><br> 2472 * </p> 2473 */ 2474 @SearchParamDefinition(name="category", path="DiagnosticReport.category", description="Which diagnostic discipline/department created the report", type="token" ) 2475 public static final String SP_CATEGORY = "category"; 2476 /** 2477 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2478 * <p> 2479 * Description: <b>Which diagnostic discipline/department created the report</b><br> 2480 * Type: <b>token</b><br> 2481 * Path: <b>DiagnosticReport.category</b><br> 2482 * </p> 2483 */ 2484 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2485 2486 /** 2487 * Search parameter: <b>conclusion</b> 2488 * <p> 2489 * Description: <b>A coded conclusion (interpretation/impression) on the report</b><br> 2490 * Type: <b>token</b><br> 2491 * Path: <b>DiagnosticReport.conclusionCode</b><br> 2492 * </p> 2493 */ 2494 @SearchParamDefinition(name="conclusion", path="DiagnosticReport.conclusionCode", description="A coded conclusion (interpretation/impression) on the report", type="token" ) 2495 public static final String SP_CONCLUSION = "conclusion"; 2496 /** 2497 * <b>Fluent Client</b> search parameter constant for <b>conclusion</b> 2498 * <p> 2499 * Description: <b>A coded conclusion (interpretation/impression) on the report</b><br> 2500 * Type: <b>token</b><br> 2501 * Path: <b>DiagnosticReport.conclusionCode</b><br> 2502 * </p> 2503 */ 2504 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONCLUSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONCLUSION); 2505 2506 /** 2507 * Search parameter: <b>issued</b> 2508 * <p> 2509 * Description: <b>When the report was issued</b><br> 2510 * Type: <b>date</b><br> 2511 * Path: <b>DiagnosticReport.issued</b><br> 2512 * </p> 2513 */ 2514 @SearchParamDefinition(name="issued", path="DiagnosticReport.issued", description="When the report was issued", type="date" ) 2515 public static final String SP_ISSUED = "issued"; 2516 /** 2517 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 2518 * <p> 2519 * Description: <b>When the report was issued</b><br> 2520 * Type: <b>date</b><br> 2521 * Path: <b>DiagnosticReport.issued</b><br> 2522 * </p> 2523 */ 2524 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 2525 2526 /** 2527 * Search parameter: <b>media</b> 2528 * <p> 2529 * Description: <b>A reference to the image source.</b><br> 2530 * Type: <b>reference</b><br> 2531 * Path: <b>DiagnosticReport.media.link</b><br> 2532 * </p> 2533 */ 2534 @SearchParamDefinition(name="media", path="DiagnosticReport.media.link", description="A reference to the image source.", type="reference", target={DocumentReference.class } ) 2535 public static final String SP_MEDIA = "media"; 2536 /** 2537 * <b>Fluent Client</b> search parameter constant for <b>media</b> 2538 * <p> 2539 * Description: <b>A reference to the image source.</b><br> 2540 * Type: <b>reference</b><br> 2541 * Path: <b>DiagnosticReport.media.link</b><br> 2542 * </p> 2543 */ 2544 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDIA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDIA); 2545 2546/** 2547 * Constant for fluent queries to be used to add include statements. Specifies 2548 * the path value of "<b>DiagnosticReport:media</b>". 2549 */ 2550 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDIA = new ca.uhn.fhir.model.api.Include("DiagnosticReport:media").toLocked(); 2551 2552 /** 2553 * Search parameter: <b>performer</b> 2554 * <p> 2555 * Description: <b>Who is responsible for the report</b><br> 2556 * Type: <b>reference</b><br> 2557 * Path: <b>DiagnosticReport.performer</b><br> 2558 * </p> 2559 */ 2560 @SearchParamDefinition(name="performer", path="DiagnosticReport.performer", description="Who is responsible for the report", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={CareTeam.class, Organization.class, Practitioner.class, PractitionerRole.class } ) 2561 public static final String SP_PERFORMER = "performer"; 2562 /** 2563 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2564 * <p> 2565 * Description: <b>Who is responsible for the report</b><br> 2566 * Type: <b>reference</b><br> 2567 * Path: <b>DiagnosticReport.performer</b><br> 2568 * </p> 2569 */ 2570 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2571 2572/** 2573 * Constant for fluent queries to be used to add include statements. Specifies 2574 * the path value of "<b>DiagnosticReport:performer</b>". 2575 */ 2576 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("DiagnosticReport:performer").toLocked(); 2577 2578 /** 2579 * Search parameter: <b>result</b> 2580 * <p> 2581 * Description: <b>Link to an atomic result (observation resource)</b><br> 2582 * Type: <b>reference</b><br> 2583 * Path: <b>DiagnosticReport.result</b><br> 2584 * </p> 2585 */ 2586 @SearchParamDefinition(name="result", path="DiagnosticReport.result", description="Link to an atomic result (observation resource)", type="reference", target={Observation.class } ) 2587 public static final String SP_RESULT = "result"; 2588 /** 2589 * <b>Fluent Client</b> search parameter constant for <b>result</b> 2590 * <p> 2591 * Description: <b>Link to an atomic result (observation resource)</b><br> 2592 * Type: <b>reference</b><br> 2593 * Path: <b>DiagnosticReport.result</b><br> 2594 * </p> 2595 */ 2596 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESULT); 2597 2598/** 2599 * Constant for fluent queries to be used to add include statements. Specifies 2600 * the path value of "<b>DiagnosticReport:result</b>". 2601 */ 2602 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:result").toLocked(); 2603 2604 /** 2605 * Search parameter: <b>results-interpreter</b> 2606 * <p> 2607 * Description: <b>Who was the source of the report</b><br> 2608 * Type: <b>reference</b><br> 2609 * Path: <b>DiagnosticReport.resultsInterpreter</b><br> 2610 * </p> 2611 */ 2612 @SearchParamDefinition(name="results-interpreter", path="DiagnosticReport.resultsInterpreter", description="Who was the source of the report", type="reference", target={CareTeam.class, Organization.class, Practitioner.class, PractitionerRole.class } ) 2613 public static final String SP_RESULTS_INTERPRETER = "results-interpreter"; 2614 /** 2615 * <b>Fluent Client</b> search parameter constant for <b>results-interpreter</b> 2616 * <p> 2617 * Description: <b>Who was the source of the report</b><br> 2618 * Type: <b>reference</b><br> 2619 * Path: <b>DiagnosticReport.resultsInterpreter</b><br> 2620 * </p> 2621 */ 2622 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESULTS_INTERPRETER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESULTS_INTERPRETER); 2623 2624/** 2625 * Constant for fluent queries to be used to add include statements. Specifies 2626 * the path value of "<b>DiagnosticReport:results-interpreter</b>". 2627 */ 2628 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESULTS_INTERPRETER = new ca.uhn.fhir.model.api.Include("DiagnosticReport:results-interpreter").toLocked(); 2629 2630 /** 2631 * Search parameter: <b>specimen</b> 2632 * <p> 2633 * Description: <b>The specimen details</b><br> 2634 * Type: <b>reference</b><br> 2635 * Path: <b>DiagnosticReport.specimen</b><br> 2636 * </p> 2637 */ 2638 @SearchParamDefinition(name="specimen", path="DiagnosticReport.specimen", description="The specimen details", type="reference", target={Specimen.class } ) 2639 public static final String SP_SPECIMEN = "specimen"; 2640 /** 2641 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 2642 * <p> 2643 * Description: <b>The specimen details</b><br> 2644 * Type: <b>reference</b><br> 2645 * Path: <b>DiagnosticReport.specimen</b><br> 2646 * </p> 2647 */ 2648 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 2649 2650/** 2651 * Constant for fluent queries to be used to add include statements. Specifies 2652 * the path value of "<b>DiagnosticReport:specimen</b>". 2653 */ 2654 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("DiagnosticReport:specimen").toLocked(); 2655 2656 /** 2657 * Search parameter: <b>status</b> 2658 * <p> 2659 * Description: <b>The status of the report</b><br> 2660 * Type: <b>token</b><br> 2661 * Path: <b>DiagnosticReport.status</b><br> 2662 * </p> 2663 */ 2664 @SearchParamDefinition(name="status", path="DiagnosticReport.status", description="The status of the report", type="token" ) 2665 public static final String SP_STATUS = "status"; 2666 /** 2667 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2668 * <p> 2669 * Description: <b>The status of the report</b><br> 2670 * Type: <b>token</b><br> 2671 * Path: <b>DiagnosticReport.status</b><br> 2672 * </p> 2673 */ 2674 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2675 2676 /** 2677 * Search parameter: <b>study</b> 2678 * <p> 2679 * Description: <b>Studies associated with the diagnostic report</b><br> 2680 * Type: <b>reference</b><br> 2681 * Path: <b>DiagnosticReport.study</b><br> 2682 * </p> 2683 */ 2684 @SearchParamDefinition(name="study", path="DiagnosticReport.study", description="Studies associated with the diagnostic report", type="reference", target={GenomicStudy.class, ImagingStudy.class } ) 2685 public static final String SP_STUDY = "study"; 2686 /** 2687 * <b>Fluent Client</b> search parameter constant for <b>study</b> 2688 * <p> 2689 * Description: <b>Studies associated with the diagnostic report</b><br> 2690 * Type: <b>reference</b><br> 2691 * Path: <b>DiagnosticReport.study</b><br> 2692 * </p> 2693 */ 2694 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_STUDY); 2695 2696/** 2697 * Constant for fluent queries to be used to add include statements. Specifies 2698 * the path value of "<b>DiagnosticReport:study</b>". 2699 */ 2700 public static final ca.uhn.fhir.model.api.Include INCLUDE_STUDY = new ca.uhn.fhir.model.api.Include("DiagnosticReport:study").toLocked(); 2701 2702 /** 2703 * Search parameter: <b>subject</b> 2704 * <p> 2705 * Description: <b>The subject of the report</b><br> 2706 * Type: <b>reference</b><br> 2707 * Path: <b>DiagnosticReport.subject</b><br> 2708 * </p> 2709 */ 2710 @SearchParamDefinition(name="subject", path="DiagnosticReport.subject", description="The subject of the report", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={BiologicallyDerivedProduct.class, Device.class, Group.class, Location.class, Medication.class, Organization.class, Patient.class, Practitioner.class, Substance.class } ) 2711 public static final String SP_SUBJECT = "subject"; 2712 /** 2713 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2714 * <p> 2715 * Description: <b>The subject of the report</b><br> 2716 * Type: <b>reference</b><br> 2717 * Path: <b>DiagnosticReport.subject</b><br> 2718 * </p> 2719 */ 2720 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2721 2722/** 2723 * Constant for fluent queries to be used to add include statements. Specifies 2724 * the path value of "<b>DiagnosticReport:subject</b>". 2725 */ 2726 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:subject").toLocked(); 2727 2728 /** 2729 * Search parameter: <b>code</b> 2730 * <p> 2731 * Description: <b>Multiple Resources: 2732 2733* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2734* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2735* [AuditEvent](auditevent.html): More specific code for the event 2736* [Basic](basic.html): Kind of Resource 2737* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2738* [Condition](condition.html): Code for the condition 2739* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2740* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2741* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2742* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2743* [ImagingSelection](imagingselection.html): The imaging selection status 2744* [List](list.html): What the purpose of this list is 2745* [Medication](medication.html): Returns medications for a specific code 2746* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2747* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2748* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2749* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2750* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2751* [Observation](observation.html): The code of the observation type 2752* [Procedure](procedure.html): A code to identify a procedure 2753* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2754* [Task](task.html): Search by task code 2755</b><br> 2756 * Type: <b>token</b><br> 2757 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2758 * </p> 2759 */ 2760 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2761 public static final String SP_CODE = "code"; 2762 /** 2763 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2764 * <p> 2765 * Description: <b>Multiple Resources: 2766 2767* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2768* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2769* [AuditEvent](auditevent.html): More specific code for the event 2770* [Basic](basic.html): Kind of Resource 2771* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2772* [Condition](condition.html): Code for the condition 2773* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2774* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2775* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2776* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2777* [ImagingSelection](imagingselection.html): The imaging selection status 2778* [List](list.html): What the purpose of this list is 2779* [Medication](medication.html): Returns medications for a specific code 2780* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2781* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2782* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2783* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2784* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2785* [Observation](observation.html): The code of the observation type 2786* [Procedure](procedure.html): A code to identify a procedure 2787* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2788* [Task](task.html): Search by task code 2789</b><br> 2790 * Type: <b>token</b><br> 2791 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2792 * </p> 2793 */ 2794 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2795 2796 /** 2797 * Search parameter: <b>date</b> 2798 * <p> 2799 * Description: <b>Multiple Resources: 2800 2801* [AdverseEvent](adverseevent.html): When the event occurred 2802* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2803* [Appointment](appointment.html): Appointment date/time. 2804* [AuditEvent](auditevent.html): Time when the event was recorded 2805* [CarePlan](careplan.html): Time period plan covers 2806* [CareTeam](careteam.html): A date within the coverage time period. 2807* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2808* [Composition](composition.html): Composition editing time 2809* [Consent](consent.html): When consent was agreed to 2810* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2811* [DocumentReference](documentreference.html): When this document reference was created 2812* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2813* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2814* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2815* [Flag](flag.html): Time period when flag is active 2816* [Immunization](immunization.html): Vaccination (non)-Administration Date 2817* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2818* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2819* [Invoice](invoice.html): Invoice date / posting date 2820* [List](list.html): When the list was prepared 2821* [MeasureReport](measurereport.html): The date of the measure report 2822* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2823* [Observation](observation.html): Clinically relevant time/time-period for observation 2824* [Procedure](procedure.html): When the procedure occurred or is occurring 2825* [ResearchSubject](researchsubject.html): Start and end of participation 2826* [RiskAssessment](riskassessment.html): When was assessment made? 2827* [SupplyRequest](supplyrequest.html): When the request was made 2828</b><br> 2829 * Type: <b>date</b><br> 2830 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2831 * </p> 2832 */ 2833 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 2834 public static final String SP_DATE = "date"; 2835 /** 2836 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2837 * <p> 2838 * Description: <b>Multiple Resources: 2839 2840* [AdverseEvent](adverseevent.html): When the event occurred 2841* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2842* [Appointment](appointment.html): Appointment date/time. 2843* [AuditEvent](auditevent.html): Time when the event was recorded 2844* [CarePlan](careplan.html): Time period plan covers 2845* [CareTeam](careteam.html): A date within the coverage time period. 2846* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2847* [Composition](composition.html): Composition editing time 2848* [Consent](consent.html): When consent was agreed to 2849* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2850* [DocumentReference](documentreference.html): When this document reference was created 2851* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2852* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2853* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2854* [Flag](flag.html): Time period when flag is active 2855* [Immunization](immunization.html): Vaccination (non)-Administration Date 2856* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2857* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2858* [Invoice](invoice.html): Invoice date / posting date 2859* [List](list.html): When the list was prepared 2860* [MeasureReport](measurereport.html): The date of the measure report 2861* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2862* [Observation](observation.html): Clinically relevant time/time-period for observation 2863* [Procedure](procedure.html): When the procedure occurred or is occurring 2864* [ResearchSubject](researchsubject.html): Start and end of participation 2865* [RiskAssessment](riskassessment.html): When was assessment made? 2866* [SupplyRequest](supplyrequest.html): When the request was made 2867</b><br> 2868 * Type: <b>date</b><br> 2869 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2870 * </p> 2871 */ 2872 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2873 2874 /** 2875 * Search parameter: <b>encounter</b> 2876 * <p> 2877 * Description: <b>Multiple Resources: 2878 2879* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2880* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2881* [ChargeItem](chargeitem.html): Encounter associated with event 2882* [Claim](claim.html): Encounters associated with a billed line item 2883* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2884* [Communication](communication.html): The Encounter during which this Communication was created 2885* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2886* [Composition](composition.html): Context of the Composition 2887* [Condition](condition.html): The Encounter during which this Condition was created 2888* [DeviceRequest](devicerequest.html): Encounter during which request was created 2889* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2890* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2891* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2892* [Flag](flag.html): Alert relevant during encounter 2893* [ImagingStudy](imagingstudy.html): The context of the study 2894* [List](list.html): Context in which list created 2895* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2896* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2897* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2898* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2899* [Observation](observation.html): Encounter related to the observation 2900* [Procedure](procedure.html): The Encounter during which this Procedure was created 2901* [Provenance](provenance.html): Encounter related to the Provenance 2902* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2903* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2904* [RiskAssessment](riskassessment.html): Where was assessment performed? 2905* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2906* [Task](task.html): Search by encounter 2907* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2908</b><br> 2909 * Type: <b>reference</b><br> 2910 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2911 * </p> 2912 */ 2913 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 2914 public static final String SP_ENCOUNTER = "encounter"; 2915 /** 2916 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2917 * <p> 2918 * Description: <b>Multiple Resources: 2919 2920* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2921* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2922* [ChargeItem](chargeitem.html): Encounter associated with event 2923* [Claim](claim.html): Encounters associated with a billed line item 2924* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2925* [Communication](communication.html): The Encounter during which this Communication was created 2926* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2927* [Composition](composition.html): Context of the Composition 2928* [Condition](condition.html): The Encounter during which this Condition was created 2929* [DeviceRequest](devicerequest.html): Encounter during which request was created 2930* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2931* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2932* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2933* [Flag](flag.html): Alert relevant during encounter 2934* [ImagingStudy](imagingstudy.html): The context of the study 2935* [List](list.html): Context in which list created 2936* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2937* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2938* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2939* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2940* [Observation](observation.html): Encounter related to the observation 2941* [Procedure](procedure.html): The Encounter during which this Procedure was created 2942* [Provenance](provenance.html): Encounter related to the Provenance 2943* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2944* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2945* [RiskAssessment](riskassessment.html): Where was assessment performed? 2946* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2947* [Task](task.html): Search by encounter 2948* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2949</b><br> 2950 * Type: <b>reference</b><br> 2951 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2952 * </p> 2953 */ 2954 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2955 2956/** 2957 * Constant for fluent queries to be used to add include statements. Specifies 2958 * the path value of "<b>DiagnosticReport:encounter</b>". 2959 */ 2960 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("DiagnosticReport:encounter").toLocked(); 2961 2962 /** 2963 * Search parameter: <b>identifier</b> 2964 * <p> 2965 * Description: <b>Multiple Resources: 2966 2967* [Account](account.html): Account number 2968* [AdverseEvent](adverseevent.html): Business identifier for the event 2969* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2970* [Appointment](appointment.html): An Identifier of the Appointment 2971* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2972* [Basic](basic.html): Business identifier 2973* [BodyStructure](bodystructure.html): Bodystructure identifier 2974* [CarePlan](careplan.html): External Ids for this plan 2975* [CareTeam](careteam.html): External Ids for this team 2976* [ChargeItem](chargeitem.html): Business Identifier for item 2977* [Claim](claim.html): The primary identifier of the financial resource 2978* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2979* [ClinicalImpression](clinicalimpression.html): Business identifier 2980* [Communication](communication.html): Unique identifier 2981* [CommunicationRequest](communicationrequest.html): Unique identifier 2982* [Composition](composition.html): Version-independent identifier for the Composition 2983* [Condition](condition.html): A unique identifier of the condition record 2984* [Consent](consent.html): Identifier for this record (external references) 2985* [Contract](contract.html): The identity of the contract 2986* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2987* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2988* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2989* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2990* [DeviceRequest](devicerequest.html): Business identifier for request/order 2991* [DeviceUsage](deviceusage.html): Search by identifier 2992* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2993* [DocumentReference](documentreference.html): Identifier of the attachment binary 2994* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2995* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2996* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2997* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2998* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2999* [Flag](flag.html): Business identifier 3000* [Goal](goal.html): External Ids for this goal 3001* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3002* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3003* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3004* [Immunization](immunization.html): Business identifier 3005* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3006* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3007* [Invoice](invoice.html): Business Identifier for item 3008* [List](list.html): Business identifier 3009* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3010* [Medication](medication.html): Returns medications with this external identifier 3011* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3012* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3013* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3014* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3015* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3016* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3017* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3018* [Observation](observation.html): The unique id for a particular observation 3019* [Person](person.html): A person Identifier 3020* [Procedure](procedure.html): A unique identifier for a procedure 3021* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3022* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3023* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3024* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3025* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3026* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3027* [Specimen](specimen.html): The unique identifier associated with the specimen 3028* [SupplyDelivery](supplydelivery.html): External identifier 3029* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3030* [Task](task.html): Search for a task instance by its business identifier 3031* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3032</b><br> 3033 * Type: <b>token</b><br> 3034 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3035 * </p> 3036 */ 3037 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3038 public static final String SP_IDENTIFIER = "identifier"; 3039 /** 3040 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3041 * <p> 3042 * Description: <b>Multiple Resources: 3043 3044* [Account](account.html): Account number 3045* [AdverseEvent](adverseevent.html): Business identifier for the event 3046* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3047* [Appointment](appointment.html): An Identifier of the Appointment 3048* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3049* [Basic](basic.html): Business identifier 3050* [BodyStructure](bodystructure.html): Bodystructure identifier 3051* [CarePlan](careplan.html): External Ids for this plan 3052* [CareTeam](careteam.html): External Ids for this team 3053* [ChargeItem](chargeitem.html): Business Identifier for item 3054* [Claim](claim.html): The primary identifier of the financial resource 3055* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3056* [ClinicalImpression](clinicalimpression.html): Business identifier 3057* [Communication](communication.html): Unique identifier 3058* [CommunicationRequest](communicationrequest.html): Unique identifier 3059* [Composition](composition.html): Version-independent identifier for the Composition 3060* [Condition](condition.html): A unique identifier of the condition record 3061* [Consent](consent.html): Identifier for this record (external references) 3062* [Contract](contract.html): The identity of the contract 3063* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3064* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3065* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3066* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3067* [DeviceRequest](devicerequest.html): Business identifier for request/order 3068* [DeviceUsage](deviceusage.html): Search by identifier 3069* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3070* [DocumentReference](documentreference.html): Identifier of the attachment binary 3071* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3072* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3073* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3074* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3075* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3076* [Flag](flag.html): Business identifier 3077* [Goal](goal.html): External Ids for this goal 3078* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3079* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3080* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3081* [Immunization](immunization.html): Business identifier 3082* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3083* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3084* [Invoice](invoice.html): Business Identifier for item 3085* [List](list.html): Business identifier 3086* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3087* [Medication](medication.html): Returns medications with this external identifier 3088* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3089* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3090* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3091* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3092* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3093* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3094* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3095* [Observation](observation.html): The unique id for a particular observation 3096* [Person](person.html): A person Identifier 3097* [Procedure](procedure.html): A unique identifier for a procedure 3098* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3099* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3100* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3101* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3102* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3103* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3104* [Specimen](specimen.html): The unique identifier associated with the specimen 3105* [SupplyDelivery](supplydelivery.html): External identifier 3106* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3107* [Task](task.html): Search for a task instance by its business identifier 3108* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3109</b><br> 3110 * Type: <b>token</b><br> 3111 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3112 * </p> 3113 */ 3114 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3115 3116 /** 3117 * Search parameter: <b>patient</b> 3118 * <p> 3119 * Description: <b>Multiple Resources: 3120 3121* [Account](account.html): The entity that caused the expenses 3122* [AdverseEvent](adverseevent.html): Subject impacted by event 3123* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3124* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3125* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3126* [AuditEvent](auditevent.html): Where the activity involved patient data 3127* [Basic](basic.html): Identifies the focus of this resource 3128* [BodyStructure](bodystructure.html): Who this is about 3129* [CarePlan](careplan.html): Who the care plan is for 3130* [CareTeam](careteam.html): Who care team is for 3131* [ChargeItem](chargeitem.html): Individual service was done for/to 3132* [Claim](claim.html): Patient receiving the products or services 3133* [ClaimResponse](claimresponse.html): The subject of care 3134* [ClinicalImpression](clinicalimpression.html): Patient assessed 3135* [Communication](communication.html): Focus of message 3136* [CommunicationRequest](communicationrequest.html): Focus of message 3137* [Composition](composition.html): Who and/or what the composition is about 3138* [Condition](condition.html): Who has the condition? 3139* [Consent](consent.html): Who the consent applies to 3140* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3141* [Coverage](coverage.html): Retrieve coverages for a patient 3142* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3143* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3144* [DetectedIssue](detectedissue.html): Associated patient 3145* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3146* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3147* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3148* [DocumentReference](documentreference.html): Who/what is the subject of the document 3149* [Encounter](encounter.html): The patient present at the encounter 3150* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3151* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3152* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3153* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3154* [Flag](flag.html): The identity of a subject to list flags for 3155* [Goal](goal.html): Who this goal is intended for 3156* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3157* [ImagingSelection](imagingselection.html): Who the study is about 3158* [ImagingStudy](imagingstudy.html): Who the study is about 3159* [Immunization](immunization.html): The patient for the vaccination record 3160* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3161* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3162* [Invoice](invoice.html): Recipient(s) of goods and services 3163* [List](list.html): If all resources have the same subject 3164* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3165* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3166* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3167* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3168* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3169* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3170* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3171* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3172* [Observation](observation.html): The subject that the observation is about (if patient) 3173* [Person](person.html): The Person links to this Patient 3174* [Procedure](procedure.html): Search by subject - a patient 3175* [Provenance](provenance.html): Where the activity involved patient data 3176* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3177* [RelatedPerson](relatedperson.html): The patient this related person is related to 3178* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3179* [ResearchSubject](researchsubject.html): Who or what is part of study 3180* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3181* [ServiceRequest](servicerequest.html): Search by subject - a patient 3182* [Specimen](specimen.html): The patient the specimen comes from 3183* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3184* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3185* [Task](task.html): Search by patient 3186* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3187</b><br> 3188 * Type: <b>reference</b><br> 3189 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3190 * </p> 3191 */ 3192 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3193 public static final String SP_PATIENT = "patient"; 3194 /** 3195 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3196 * <p> 3197 * Description: <b>Multiple Resources: 3198 3199* [Account](account.html): The entity that caused the expenses 3200* [AdverseEvent](adverseevent.html): Subject impacted by event 3201* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3202* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3203* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3204* [AuditEvent](auditevent.html): Where the activity involved patient data 3205* [Basic](basic.html): Identifies the focus of this resource 3206* [BodyStructure](bodystructure.html): Who this is about 3207* [CarePlan](careplan.html): Who the care plan is for 3208* [CareTeam](careteam.html): Who care team is for 3209* [ChargeItem](chargeitem.html): Individual service was done for/to 3210* [Claim](claim.html): Patient receiving the products or services 3211* [ClaimResponse](claimresponse.html): The subject of care 3212* [ClinicalImpression](clinicalimpression.html): Patient assessed 3213* [Communication](communication.html): Focus of message 3214* [CommunicationRequest](communicationrequest.html): Focus of message 3215* [Composition](composition.html): Who and/or what the composition is about 3216* [Condition](condition.html): Who has the condition? 3217* [Consent](consent.html): Who the consent applies to 3218* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3219* [Coverage](coverage.html): Retrieve coverages for a patient 3220* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3221* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3222* [DetectedIssue](detectedissue.html): Associated patient 3223* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3224* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3225* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3226* [DocumentReference](documentreference.html): Who/what is the subject of the document 3227* [Encounter](encounter.html): The patient present at the encounter 3228* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3229* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3230* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3231* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3232* [Flag](flag.html): The identity of a subject to list flags for 3233* [Goal](goal.html): Who this goal is intended for 3234* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3235* [ImagingSelection](imagingselection.html): Who the study is about 3236* [ImagingStudy](imagingstudy.html): Who the study is about 3237* [Immunization](immunization.html): The patient for the vaccination record 3238* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3239* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3240* [Invoice](invoice.html): Recipient(s) of goods and services 3241* [List](list.html): If all resources have the same subject 3242* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3243* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3244* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3245* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3246* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3247* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3248* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3249* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3250* [Observation](observation.html): The subject that the observation is about (if patient) 3251* [Person](person.html): The Person links to this Patient 3252* [Procedure](procedure.html): Search by subject - a patient 3253* [Provenance](provenance.html): Where the activity involved patient data 3254* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3255* [RelatedPerson](relatedperson.html): The patient this related person is related to 3256* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3257* [ResearchSubject](researchsubject.html): Who or what is part of study 3258* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3259* [ServiceRequest](servicerequest.html): Search by subject - a patient 3260* [Specimen](specimen.html): The patient the specimen comes from 3261* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3262* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3263* [Task](task.html): Search by patient 3264* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3265</b><br> 3266 * Type: <b>reference</b><br> 3267 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3268 * </p> 3269 */ 3270 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3271 3272/** 3273 * Constant for fluent queries to be used to add include statements. Specifies 3274 * the path value of "<b>DiagnosticReport:patient</b>". 3275 */ 3276 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DiagnosticReport:patient").toLocked(); 3277 3278 3279} 3280