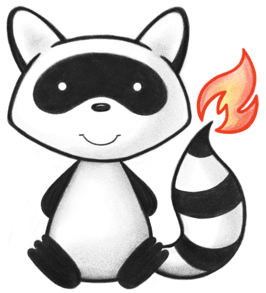
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A reference to a document of any kind for any purpose. While the term ?document? implies a more narrow focus, for this resource this ?document? encompasses *any* serialized object with a mime-type, it includes formal patient-centric documents (CDA), clinical notes, scanned paper, non-patient specific documents like policy text, as well as a photo, video, or audio recording acquired or used in healthcare. The DocumentReference resource provides metadata about the document so that the document can be discovered and managed. The actual content may be inline base64 encoded data or provided by direct reference. 052 */ 053@ResourceDef(name="DocumentReference", profile="http://hl7.org/fhir/StructureDefinition/DocumentReference") 054public class DocumentReference extends DomainResource { 055 056 public enum DocumentReferenceStatus { 057 /** 058 * This is the current reference for this document. 059 */ 060 CURRENT, 061 /** 062 * This reference has been superseded by another reference. 063 */ 064 SUPERSEDED, 065 /** 066 * This reference was created in error. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static DocumentReferenceStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("current".equals(codeString)) 077 return CURRENT; 078 if ("superseded".equals(codeString)) 079 return SUPERSEDED; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case CURRENT: return "current"; 090 case SUPERSEDED: return "superseded"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case CURRENT: return "http://hl7.org/fhir/document-reference-status"; 099 case SUPERSEDED: return "http://hl7.org/fhir/document-reference-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/document-reference-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case CURRENT: return "This is the current reference for this document."; 108 case SUPERSEDED: return "This reference has been superseded by another reference."; 109 case ENTEREDINERROR: return "This reference was created in error."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case CURRENT: return "Current"; 117 case SUPERSEDED: return "Superseded"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class DocumentReferenceStatusEnumFactory implements EnumFactory<DocumentReferenceStatus> { 126 public DocumentReferenceStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("current".equals(codeString)) 131 return DocumentReferenceStatus.CURRENT; 132 if ("superseded".equals(codeString)) 133 return DocumentReferenceStatus.SUPERSEDED; 134 if ("entered-in-error".equals(codeString)) 135 return DocumentReferenceStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 137 } 138 public Enumeration<DocumentReferenceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.NULL, code); 146 if ("current".equals(codeString)) 147 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.CURRENT, code); 148 if ("superseded".equals(codeString)) 149 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.SUPERSEDED, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 153 } 154 public String toCode(DocumentReferenceStatus code) { 155 if (code == DocumentReferenceStatus.NULL) 156 return null; 157 if (code == DocumentReferenceStatus.CURRENT) 158 return "current"; 159 if (code == DocumentReferenceStatus.SUPERSEDED) 160 return "superseded"; 161 if (code == DocumentReferenceStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(DocumentReferenceStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class DocumentReferenceAttesterComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The type of attestation the authenticator offers. 174 */ 175 @Child(name = "mode", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="personal | professional | legal | official", formalDefinition="The type of attestation the authenticator offers." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composition-attestation-mode") 178 protected CodeableConcept mode; 179 180 /** 181 * When the document was attested by the party. 182 */ 183 @Child(name = "time", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 184 @Description(shortDefinition="When the document was attested", formalDefinition="When the document was attested by the party." ) 185 protected DateTimeType time; 186 187 /** 188 * Who attested the document in the specified way. 189 */ 190 @Child(name = "party", type = {Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="Who attested the document", formalDefinition="Who attested the document in the specified way." ) 192 protected Reference party; 193 194 private static final long serialVersionUID = 545132751L; 195 196 /** 197 * Constructor 198 */ 199 public DocumentReferenceAttesterComponent() { 200 super(); 201 } 202 203 /** 204 * Constructor 205 */ 206 public DocumentReferenceAttesterComponent(CodeableConcept mode) { 207 super(); 208 this.setMode(mode); 209 } 210 211 /** 212 * @return {@link #mode} (The type of attestation the authenticator offers.) 213 */ 214 public CodeableConcept getMode() { 215 if (this.mode == null) 216 if (Configuration.errorOnAutoCreate()) 217 throw new Error("Attempt to auto-create DocumentReferenceAttesterComponent.mode"); 218 else if (Configuration.doAutoCreate()) 219 this.mode = new CodeableConcept(); // cc 220 return this.mode; 221 } 222 223 public boolean hasMode() { 224 return this.mode != null && !this.mode.isEmpty(); 225 } 226 227 /** 228 * @param value {@link #mode} (The type of attestation the authenticator offers.) 229 */ 230 public DocumentReferenceAttesterComponent setMode(CodeableConcept value) { 231 this.mode = value; 232 return this; 233 } 234 235 /** 236 * @return {@link #time} (When the document was attested by the party.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 237 */ 238 public DateTimeType getTimeElement() { 239 if (this.time == null) 240 if (Configuration.errorOnAutoCreate()) 241 throw new Error("Attempt to auto-create DocumentReferenceAttesterComponent.time"); 242 else if (Configuration.doAutoCreate()) 243 this.time = new DateTimeType(); // bb 244 return this.time; 245 } 246 247 public boolean hasTimeElement() { 248 return this.time != null && !this.time.isEmpty(); 249 } 250 251 public boolean hasTime() { 252 return this.time != null && !this.time.isEmpty(); 253 } 254 255 /** 256 * @param value {@link #time} (When the document was attested by the party.). This is the underlying object with id, value and extensions. The accessor "getTime" gives direct access to the value 257 */ 258 public DocumentReferenceAttesterComponent setTimeElement(DateTimeType value) { 259 this.time = value; 260 return this; 261 } 262 263 /** 264 * @return When the document was attested by the party. 265 */ 266 public Date getTime() { 267 return this.time == null ? null : this.time.getValue(); 268 } 269 270 /** 271 * @param value When the document was attested by the party. 272 */ 273 public DocumentReferenceAttesterComponent setTime(Date value) { 274 if (value == null) 275 this.time = null; 276 else { 277 if (this.time == null) 278 this.time = new DateTimeType(); 279 this.time.setValue(value); 280 } 281 return this; 282 } 283 284 /** 285 * @return {@link #party} (Who attested the document in the specified way.) 286 */ 287 public Reference getParty() { 288 if (this.party == null) 289 if (Configuration.errorOnAutoCreate()) 290 throw new Error("Attempt to auto-create DocumentReferenceAttesterComponent.party"); 291 else if (Configuration.doAutoCreate()) 292 this.party = new Reference(); // cc 293 return this.party; 294 } 295 296 public boolean hasParty() { 297 return this.party != null && !this.party.isEmpty(); 298 } 299 300 /** 301 * @param value {@link #party} (Who attested the document in the specified way.) 302 */ 303 public DocumentReferenceAttesterComponent setParty(Reference value) { 304 this.party = value; 305 return this; 306 } 307 308 protected void listChildren(List<Property> children) { 309 super.listChildren(children); 310 children.add(new Property("mode", "CodeableConcept", "The type of attestation the authenticator offers.", 0, 1, mode)); 311 children.add(new Property("time", "dateTime", "When the document was attested by the party.", 0, 1, time)); 312 children.add(new Property("party", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Who attested the document in the specified way.", 0, 1, party)); 313 } 314 315 @Override 316 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 317 switch (_hash) { 318 case 3357091: /*mode*/ return new Property("mode", "CodeableConcept", "The type of attestation the authenticator offers.", 0, 1, mode); 319 case 3560141: /*time*/ return new Property("time", "dateTime", "When the document was attested by the party.", 0, 1, time); 320 case 106437350: /*party*/ return new Property("party", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Organization)", "Who attested the document in the specified way.", 0, 1, party); 321 default: return super.getNamedProperty(_hash, _name, _checkValid); 322 } 323 324 } 325 326 @Override 327 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 328 switch (hash) { 329 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // CodeableConcept 330 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // DateTimeType 331 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 332 default: return super.getProperty(hash, name, checkValid); 333 } 334 335 } 336 337 @Override 338 public Base setProperty(int hash, String name, Base value) throws FHIRException { 339 switch (hash) { 340 case 3357091: // mode 341 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 342 return value; 343 case 3560141: // time 344 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 345 return value; 346 case 106437350: // party 347 this.party = TypeConvertor.castToReference(value); // Reference 348 return value; 349 default: return super.setProperty(hash, name, value); 350 } 351 352 } 353 354 @Override 355 public Base setProperty(String name, Base value) throws FHIRException { 356 if (name.equals("mode")) { 357 this.mode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 358 } else if (name.equals("time")) { 359 this.time = TypeConvertor.castToDateTime(value); // DateTimeType 360 } else if (name.equals("party")) { 361 this.party = TypeConvertor.castToReference(value); // Reference 362 } else 363 return super.setProperty(name, value); 364 return value; 365 } 366 367 @Override 368 public void removeChild(String name, Base value) throws FHIRException { 369 if (name.equals("mode")) { 370 this.mode = null; 371 } else if (name.equals("time")) { 372 this.time = null; 373 } else if (name.equals("party")) { 374 this.party = null; 375 } else 376 super.removeChild(name, value); 377 378 } 379 380 @Override 381 public Base makeProperty(int hash, String name) throws FHIRException { 382 switch (hash) { 383 case 3357091: return getMode(); 384 case 3560141: return getTimeElement(); 385 case 106437350: return getParty(); 386 default: return super.makeProperty(hash, name); 387 } 388 389 } 390 391 @Override 392 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 393 switch (hash) { 394 case 3357091: /*mode*/ return new String[] {"CodeableConcept"}; 395 case 3560141: /*time*/ return new String[] {"dateTime"}; 396 case 106437350: /*party*/ return new String[] {"Reference"}; 397 default: return super.getTypesForProperty(hash, name); 398 } 399 400 } 401 402 @Override 403 public Base addChild(String name) throws FHIRException { 404 if (name.equals("mode")) { 405 this.mode = new CodeableConcept(); 406 return this.mode; 407 } 408 else if (name.equals("time")) { 409 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.attester.time"); 410 } 411 else if (name.equals("party")) { 412 this.party = new Reference(); 413 return this.party; 414 } 415 else 416 return super.addChild(name); 417 } 418 419 public DocumentReferenceAttesterComponent copy() { 420 DocumentReferenceAttesterComponent dst = new DocumentReferenceAttesterComponent(); 421 copyValues(dst); 422 return dst; 423 } 424 425 public void copyValues(DocumentReferenceAttesterComponent dst) { 426 super.copyValues(dst); 427 dst.mode = mode == null ? null : mode.copy(); 428 dst.time = time == null ? null : time.copy(); 429 dst.party = party == null ? null : party.copy(); 430 } 431 432 @Override 433 public boolean equalsDeep(Base other_) { 434 if (!super.equalsDeep(other_)) 435 return false; 436 if (!(other_ instanceof DocumentReferenceAttesterComponent)) 437 return false; 438 DocumentReferenceAttesterComponent o = (DocumentReferenceAttesterComponent) other_; 439 return compareDeep(mode, o.mode, true) && compareDeep(time, o.time, true) && compareDeep(party, o.party, true) 440 ; 441 } 442 443 @Override 444 public boolean equalsShallow(Base other_) { 445 if (!super.equalsShallow(other_)) 446 return false; 447 if (!(other_ instanceof DocumentReferenceAttesterComponent)) 448 return false; 449 DocumentReferenceAttesterComponent o = (DocumentReferenceAttesterComponent) other_; 450 return compareValues(time, o.time, true); 451 } 452 453 public boolean isEmpty() { 454 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(mode, time, party); 455 } 456 457 public String fhirType() { 458 return "DocumentReference.attester"; 459 460 } 461 462 } 463 464 @Block() 465 public static class DocumentReferenceRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 466 /** 467 * The type of relationship that this document has with anther document. 468 */ 469 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 470 @Description(shortDefinition="The relationship type with another document", formalDefinition="The type of relationship that this document has with anther document." ) 471 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-relationship-type") 472 protected CodeableConcept code; 473 474 /** 475 * The target document of this relationship. 476 */ 477 @Child(name = "target", type = {DocumentReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 478 @Description(shortDefinition="Target of the relationship", formalDefinition="The target document of this relationship." ) 479 protected Reference target; 480 481 private static final long serialVersionUID = -372012026L; 482 483 /** 484 * Constructor 485 */ 486 public DocumentReferenceRelatesToComponent() { 487 super(); 488 } 489 490 /** 491 * Constructor 492 */ 493 public DocumentReferenceRelatesToComponent(CodeableConcept code, Reference target) { 494 super(); 495 this.setCode(code); 496 this.setTarget(target); 497 } 498 499 /** 500 * @return {@link #code} (The type of relationship that this document has with anther document.) 501 */ 502 public CodeableConcept getCode() { 503 if (this.code == null) 504 if (Configuration.errorOnAutoCreate()) 505 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.code"); 506 else if (Configuration.doAutoCreate()) 507 this.code = new CodeableConcept(); // cc 508 return this.code; 509 } 510 511 public boolean hasCode() { 512 return this.code != null && !this.code.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #code} (The type of relationship that this document has with anther document.) 517 */ 518 public DocumentReferenceRelatesToComponent setCode(CodeableConcept value) { 519 this.code = value; 520 return this; 521 } 522 523 /** 524 * @return {@link #target} (The target document of this relationship.) 525 */ 526 public Reference getTarget() { 527 if (this.target == null) 528 if (Configuration.errorOnAutoCreate()) 529 throw new Error("Attempt to auto-create DocumentReferenceRelatesToComponent.target"); 530 else if (Configuration.doAutoCreate()) 531 this.target = new Reference(); // cc 532 return this.target; 533 } 534 535 public boolean hasTarget() { 536 return this.target != null && !this.target.isEmpty(); 537 } 538 539 /** 540 * @param value {@link #target} (The target document of this relationship.) 541 */ 542 public DocumentReferenceRelatesToComponent setTarget(Reference value) { 543 this.target = value; 544 return this; 545 } 546 547 protected void listChildren(List<Property> children) { 548 super.listChildren(children); 549 children.add(new Property("code", "CodeableConcept", "The type of relationship that this document has with anther document.", 0, 1, code)); 550 children.add(new Property("target", "Reference(DocumentReference)", "The target document of this relationship.", 0, 1, target)); 551 } 552 553 @Override 554 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 555 switch (_hash) { 556 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of relationship that this document has with anther document.", 0, 1, code); 557 case -880905839: /*target*/ return new Property("target", "Reference(DocumentReference)", "The target document of this relationship.", 0, 1, target); 558 default: return super.getNamedProperty(_hash, _name, _checkValid); 559 } 560 561 } 562 563 @Override 564 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 565 switch (hash) { 566 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 567 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Reference 568 default: return super.getProperty(hash, name, checkValid); 569 } 570 571 } 572 573 @Override 574 public Base setProperty(int hash, String name, Base value) throws FHIRException { 575 switch (hash) { 576 case 3059181: // code 577 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 578 return value; 579 case -880905839: // target 580 this.target = TypeConvertor.castToReference(value); // Reference 581 return value; 582 default: return super.setProperty(hash, name, value); 583 } 584 585 } 586 587 @Override 588 public Base setProperty(String name, Base value) throws FHIRException { 589 if (name.equals("code")) { 590 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 591 } else if (name.equals("target")) { 592 this.target = TypeConvertor.castToReference(value); // Reference 593 } else 594 return super.setProperty(name, value); 595 return value; 596 } 597 598 @Override 599 public void removeChild(String name, Base value) throws FHIRException { 600 if (name.equals("code")) { 601 this.code = null; 602 } else if (name.equals("target")) { 603 this.target = null; 604 } else 605 super.removeChild(name, value); 606 607 } 608 609 @Override 610 public Base makeProperty(int hash, String name) throws FHIRException { 611 switch (hash) { 612 case 3059181: return getCode(); 613 case -880905839: return getTarget(); 614 default: return super.makeProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 621 switch (hash) { 622 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 623 case -880905839: /*target*/ return new String[] {"Reference"}; 624 default: return super.getTypesForProperty(hash, name); 625 } 626 627 } 628 629 @Override 630 public Base addChild(String name) throws FHIRException { 631 if (name.equals("code")) { 632 this.code = new CodeableConcept(); 633 return this.code; 634 } 635 else if (name.equals("target")) { 636 this.target = new Reference(); 637 return this.target; 638 } 639 else 640 return super.addChild(name); 641 } 642 643 public DocumentReferenceRelatesToComponent copy() { 644 DocumentReferenceRelatesToComponent dst = new DocumentReferenceRelatesToComponent(); 645 copyValues(dst); 646 return dst; 647 } 648 649 public void copyValues(DocumentReferenceRelatesToComponent dst) { 650 super.copyValues(dst); 651 dst.code = code == null ? null : code.copy(); 652 dst.target = target == null ? null : target.copy(); 653 } 654 655 @Override 656 public boolean equalsDeep(Base other_) { 657 if (!super.equalsDeep(other_)) 658 return false; 659 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 660 return false; 661 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 662 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 663 } 664 665 @Override 666 public boolean equalsShallow(Base other_) { 667 if (!super.equalsShallow(other_)) 668 return false; 669 if (!(other_ instanceof DocumentReferenceRelatesToComponent)) 670 return false; 671 DocumentReferenceRelatesToComponent o = (DocumentReferenceRelatesToComponent) other_; 672 return true; 673 } 674 675 public boolean isEmpty() { 676 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 677 } 678 679 public String fhirType() { 680 return "DocumentReference.relatesTo"; 681 682 } 683 684 } 685 686 @Block() 687 public static class DocumentReferenceContentComponent extends BackboneElement implements IBaseBackboneElement { 688 /** 689 * The document or URL of the document along with critical metadata to prove content has integrity. 690 */ 691 @Child(name = "attachment", type = {Attachment.class}, order=1, min=1, max=1, modifier=false, summary=true) 692 @Description(shortDefinition="Where to access the document", formalDefinition="The document or URL of the document along with critical metadata to prove content has integrity." ) 693 protected Attachment attachment; 694 695 /** 696 * An identifier of the document constraints, encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType. 697 */ 698 @Child(name = "profile", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 699 @Description(shortDefinition="Content profile rules for the document", formalDefinition="An identifier of the document constraints, encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType." ) 700 protected List<DocumentReferenceContentProfileComponent> profile; 701 702 private static final long serialVersionUID = 174089424L; 703 704 /** 705 * Constructor 706 */ 707 public DocumentReferenceContentComponent() { 708 super(); 709 } 710 711 /** 712 * Constructor 713 */ 714 public DocumentReferenceContentComponent(Attachment attachment) { 715 super(); 716 this.setAttachment(attachment); 717 } 718 719 /** 720 * @return {@link #attachment} (The document or URL of the document along with critical metadata to prove content has integrity.) 721 */ 722 public Attachment getAttachment() { 723 if (this.attachment == null) 724 if (Configuration.errorOnAutoCreate()) 725 throw new Error("Attempt to auto-create DocumentReferenceContentComponent.attachment"); 726 else if (Configuration.doAutoCreate()) 727 this.attachment = new Attachment(); // cc 728 return this.attachment; 729 } 730 731 public boolean hasAttachment() { 732 return this.attachment != null && !this.attachment.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #attachment} (The document or URL of the document along with critical metadata to prove content has integrity.) 737 */ 738 public DocumentReferenceContentComponent setAttachment(Attachment value) { 739 this.attachment = value; 740 return this; 741 } 742 743 /** 744 * @return {@link #profile} (An identifier of the document constraints, encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.) 745 */ 746 public List<DocumentReferenceContentProfileComponent> getProfile() { 747 if (this.profile == null) 748 this.profile = new ArrayList<DocumentReferenceContentProfileComponent>(); 749 return this.profile; 750 } 751 752 /** 753 * @return Returns a reference to <code>this</code> for easy method chaining 754 */ 755 public DocumentReferenceContentComponent setProfile(List<DocumentReferenceContentProfileComponent> theProfile) { 756 this.profile = theProfile; 757 return this; 758 } 759 760 public boolean hasProfile() { 761 if (this.profile == null) 762 return false; 763 for (DocumentReferenceContentProfileComponent item : this.profile) 764 if (!item.isEmpty()) 765 return true; 766 return false; 767 } 768 769 public DocumentReferenceContentProfileComponent addProfile() { //3 770 DocumentReferenceContentProfileComponent t = new DocumentReferenceContentProfileComponent(); 771 if (this.profile == null) 772 this.profile = new ArrayList<DocumentReferenceContentProfileComponent>(); 773 this.profile.add(t); 774 return t; 775 } 776 777 public DocumentReferenceContentComponent addProfile(DocumentReferenceContentProfileComponent t) { //3 778 if (t == null) 779 return this; 780 if (this.profile == null) 781 this.profile = new ArrayList<DocumentReferenceContentProfileComponent>(); 782 this.profile.add(t); 783 return this; 784 } 785 786 /** 787 * @return The first repetition of repeating field {@link #profile}, creating it if it does not already exist {3} 788 */ 789 public DocumentReferenceContentProfileComponent getProfileFirstRep() { 790 if (getProfile().isEmpty()) { 791 addProfile(); 792 } 793 return getProfile().get(0); 794 } 795 796 protected void listChildren(List<Property> children) { 797 super.listChildren(children); 798 children.add(new Property("attachment", "Attachment", "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, attachment)); 799 children.add(new Property("profile", "", "An identifier of the document constraints, encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 0, java.lang.Integer.MAX_VALUE, profile)); 800 } 801 802 @Override 803 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 804 switch (_hash) { 805 case -1963501277: /*attachment*/ return new Property("attachment", "Attachment", "The document or URL of the document along with critical metadata to prove content has integrity.", 0, 1, attachment); 806 case -309425751: /*profile*/ return new Property("profile", "", "An identifier of the document constraints, encoding, structure, and template that the document conforms to beyond the base format indicated in the mimeType.", 0, java.lang.Integer.MAX_VALUE, profile); 807 default: return super.getNamedProperty(_hash, _name, _checkValid); 808 } 809 810 } 811 812 @Override 813 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 814 switch (hash) { 815 case -1963501277: /*attachment*/ return this.attachment == null ? new Base[0] : new Base[] {this.attachment}; // Attachment 816 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // DocumentReferenceContentProfileComponent 817 default: return super.getProperty(hash, name, checkValid); 818 } 819 820 } 821 822 @Override 823 public Base setProperty(int hash, String name, Base value) throws FHIRException { 824 switch (hash) { 825 case -1963501277: // attachment 826 this.attachment = TypeConvertor.castToAttachment(value); // Attachment 827 return value; 828 case -309425751: // profile 829 this.getProfile().add((DocumentReferenceContentProfileComponent) value); // DocumentReferenceContentProfileComponent 830 return value; 831 default: return super.setProperty(hash, name, value); 832 } 833 834 } 835 836 @Override 837 public Base setProperty(String name, Base value) throws FHIRException { 838 if (name.equals("attachment")) { 839 this.attachment = TypeConvertor.castToAttachment(value); // Attachment 840 } else if (name.equals("profile")) { 841 this.getProfile().add((DocumentReferenceContentProfileComponent) value); 842 } else 843 return super.setProperty(name, value); 844 return value; 845 } 846 847 @Override 848 public void removeChild(String name, Base value) throws FHIRException { 849 if (name.equals("attachment")) { 850 this.attachment = null; 851 } else if (name.equals("profile")) { 852 this.getProfile().remove((DocumentReferenceContentProfileComponent) value); 853 } else 854 super.removeChild(name, value); 855 856 } 857 858 @Override 859 public Base makeProperty(int hash, String name) throws FHIRException { 860 switch (hash) { 861 case -1963501277: return getAttachment(); 862 case -309425751: return addProfile(); 863 default: return super.makeProperty(hash, name); 864 } 865 866 } 867 868 @Override 869 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 870 switch (hash) { 871 case -1963501277: /*attachment*/ return new String[] {"Attachment"}; 872 case -309425751: /*profile*/ return new String[] {}; 873 default: return super.getTypesForProperty(hash, name); 874 } 875 876 } 877 878 @Override 879 public Base addChild(String name) throws FHIRException { 880 if (name.equals("attachment")) { 881 this.attachment = new Attachment(); 882 return this.attachment; 883 } 884 else if (name.equals("profile")) { 885 return addProfile(); 886 } 887 else 888 return super.addChild(name); 889 } 890 891 public DocumentReferenceContentComponent copy() { 892 DocumentReferenceContentComponent dst = new DocumentReferenceContentComponent(); 893 copyValues(dst); 894 return dst; 895 } 896 897 public void copyValues(DocumentReferenceContentComponent dst) { 898 super.copyValues(dst); 899 dst.attachment = attachment == null ? null : attachment.copy(); 900 if (profile != null) { 901 dst.profile = new ArrayList<DocumentReferenceContentProfileComponent>(); 902 for (DocumentReferenceContentProfileComponent i : profile) 903 dst.profile.add(i.copy()); 904 }; 905 } 906 907 @Override 908 public boolean equalsDeep(Base other_) { 909 if (!super.equalsDeep(other_)) 910 return false; 911 if (!(other_ instanceof DocumentReferenceContentComponent)) 912 return false; 913 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 914 return compareDeep(attachment, o.attachment, true) && compareDeep(profile, o.profile, true); 915 } 916 917 @Override 918 public boolean equalsShallow(Base other_) { 919 if (!super.equalsShallow(other_)) 920 return false; 921 if (!(other_ instanceof DocumentReferenceContentComponent)) 922 return false; 923 DocumentReferenceContentComponent o = (DocumentReferenceContentComponent) other_; 924 return true; 925 } 926 927 public boolean isEmpty() { 928 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(attachment, profile); 929 } 930 931 public String fhirType() { 932 return "DocumentReference.content"; 933 934 } 935 936 } 937 938 @Block() 939 public static class DocumentReferenceContentProfileComponent extends BackboneElement implements IBaseBackboneElement { 940 /** 941 * Code|uri|canonical. 942 */ 943 @Child(name = "value", type = {Coding.class, UriType.class, CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=true) 944 @Description(shortDefinition="Code|uri|canonical", formalDefinition="Code|uri|canonical." ) 945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-HL7FormatCodes") 946 protected DataType value; 947 948 private static final long serialVersionUID = -1135414639L; 949 950 /** 951 * Constructor 952 */ 953 public DocumentReferenceContentProfileComponent() { 954 super(); 955 } 956 957 /** 958 * Constructor 959 */ 960 public DocumentReferenceContentProfileComponent(DataType value) { 961 super(); 962 this.setValue(value); 963 } 964 965 /** 966 * @return {@link #value} (Code|uri|canonical.) 967 */ 968 public DataType getValue() { 969 return this.value; 970 } 971 972 /** 973 * @return {@link #value} (Code|uri|canonical.) 974 */ 975 public Coding getValueCoding() throws FHIRException { 976 if (this.value == null) 977 this.value = new Coding(); 978 if (!(this.value instanceof Coding)) 979 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 980 return (Coding) this.value; 981 } 982 983 public boolean hasValueCoding() { 984 return this != null && this.value instanceof Coding; 985 } 986 987 /** 988 * @return {@link #value} (Code|uri|canonical.) 989 */ 990 public UriType getValueUriType() throws FHIRException { 991 if (this.value == null) 992 this.value = new UriType(); 993 if (!(this.value instanceof UriType)) 994 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 995 return (UriType) this.value; 996 } 997 998 public boolean hasValueUriType() { 999 return this != null && this.value instanceof UriType; 1000 } 1001 1002 /** 1003 * @return {@link #value} (Code|uri|canonical.) 1004 */ 1005 public CanonicalType getValueCanonicalType() throws FHIRException { 1006 if (this.value == null) 1007 this.value = new CanonicalType(); 1008 if (!(this.value instanceof CanonicalType)) 1009 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 1010 return (CanonicalType) this.value; 1011 } 1012 1013 public boolean hasValueCanonicalType() { 1014 return this != null && this.value instanceof CanonicalType; 1015 } 1016 1017 public boolean hasValue() { 1018 return this.value != null && !this.value.isEmpty(); 1019 } 1020 1021 /** 1022 * @param value {@link #value} (Code|uri|canonical.) 1023 */ 1024 public DocumentReferenceContentProfileComponent setValue(DataType value) { 1025 if (value != null && !(value instanceof Coding || value instanceof UriType || value instanceof CanonicalType)) 1026 throw new FHIRException("Not the right type for DocumentReference.content.profile.value[x]: "+value.fhirType()); 1027 this.value = value; 1028 return this; 1029 } 1030 1031 protected void listChildren(List<Property> children) { 1032 super.listChildren(children); 1033 children.add(new Property("value[x]", "Coding|uri|canonical", "Code|uri|canonical.", 0, 1, value)); 1034 } 1035 1036 @Override 1037 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1038 switch (_hash) { 1039 case -1410166417: /*value[x]*/ return new Property("value[x]", "Coding|uri|canonical", "Code|uri|canonical.", 0, 1, value); 1040 case 111972721: /*value*/ return new Property("value[x]", "Coding|uri|canonical", "Code|uri|canonical.", 0, 1, value); 1041 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "Code|uri|canonical.", 0, 1, value); 1042 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "Code|uri|canonical.", 0, 1, value); 1043 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "Code|uri|canonical.", 0, 1, value); 1044 default: return super.getNamedProperty(_hash, _name, _checkValid); 1045 } 1046 1047 } 1048 1049 @Override 1050 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1051 switch (hash) { 1052 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1053 default: return super.getProperty(hash, name, checkValid); 1054 } 1055 1056 } 1057 1058 @Override 1059 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1060 switch (hash) { 1061 case 111972721: // value 1062 this.value = TypeConvertor.castToType(value); // DataType 1063 return value; 1064 default: return super.setProperty(hash, name, value); 1065 } 1066 1067 } 1068 1069 @Override 1070 public Base setProperty(String name, Base value) throws FHIRException { 1071 if (name.equals("value[x]")) { 1072 this.value = TypeConvertor.castToType(value); // DataType 1073 } else 1074 return super.setProperty(name, value); 1075 return value; 1076 } 1077 1078 @Override 1079 public void removeChild(String name, Base value) throws FHIRException { 1080 if (name.equals("value[x]")) { 1081 this.value = null; 1082 } else 1083 super.removeChild(name, value); 1084 1085 } 1086 1087 @Override 1088 public Base makeProperty(int hash, String name) throws FHIRException { 1089 switch (hash) { 1090 case -1410166417: return getValue(); 1091 case 111972721: return getValue(); 1092 default: return super.makeProperty(hash, name); 1093 } 1094 1095 } 1096 1097 @Override 1098 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1099 switch (hash) { 1100 case 111972721: /*value*/ return new String[] {"Coding", "uri", "canonical"}; 1101 default: return super.getTypesForProperty(hash, name); 1102 } 1103 1104 } 1105 1106 @Override 1107 public Base addChild(String name) throws FHIRException { 1108 if (name.equals("valueCoding")) { 1109 this.value = new Coding(); 1110 return this.value; 1111 } 1112 else if (name.equals("valueUri")) { 1113 this.value = new UriType(); 1114 return this.value; 1115 } 1116 else if (name.equals("valueCanonical")) { 1117 this.value = new CanonicalType(); 1118 return this.value; 1119 } 1120 else 1121 return super.addChild(name); 1122 } 1123 1124 public DocumentReferenceContentProfileComponent copy() { 1125 DocumentReferenceContentProfileComponent dst = new DocumentReferenceContentProfileComponent(); 1126 copyValues(dst); 1127 return dst; 1128 } 1129 1130 public void copyValues(DocumentReferenceContentProfileComponent dst) { 1131 super.copyValues(dst); 1132 dst.value = value == null ? null : value.copy(); 1133 } 1134 1135 @Override 1136 public boolean equalsDeep(Base other_) { 1137 if (!super.equalsDeep(other_)) 1138 return false; 1139 if (!(other_ instanceof DocumentReferenceContentProfileComponent)) 1140 return false; 1141 DocumentReferenceContentProfileComponent o = (DocumentReferenceContentProfileComponent) other_; 1142 return compareDeep(value, o.value, true); 1143 } 1144 1145 @Override 1146 public boolean equalsShallow(Base other_) { 1147 if (!super.equalsShallow(other_)) 1148 return false; 1149 if (!(other_ instanceof DocumentReferenceContentProfileComponent)) 1150 return false; 1151 DocumentReferenceContentProfileComponent o = (DocumentReferenceContentProfileComponent) other_; 1152 return true; 1153 } 1154 1155 public boolean isEmpty() { 1156 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 1157 } 1158 1159 public String fhirType() { 1160 return "DocumentReference.content.profile"; 1161 1162 } 1163 1164 } 1165 1166 /** 1167 * Other business identifiers associated with the document, including version independent identifiers. 1168 */ 1169 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1170 @Description(shortDefinition="Business identifiers for the document", formalDefinition="Other business identifiers associated with the document, including version independent identifiers." ) 1171 protected List<Identifier> identifier; 1172 1173 /** 1174 * An explicitly assigned identifer of a variation of the content in the DocumentReference. 1175 */ 1176 @Child(name = "version", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1177 @Description(shortDefinition="An explicitly assigned identifer of a variation of the content in the DocumentReference", formalDefinition="An explicitly assigned identifer of a variation of the content in the DocumentReference." ) 1178 protected StringType version; 1179 1180 /** 1181 * A procedure that is fulfilled in whole or in part by the creation of this media. 1182 */ 1183 @Child(name = "basedOn", type = {Appointment.class, AppointmentResponse.class, CarePlan.class, Claim.class, CommunicationRequest.class, Contract.class, CoverageEligibilityRequest.class, DeviceRequest.class, EnrollmentRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, RequestOrchestration.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1184 @Description(shortDefinition="Procedure that caused this media to be created", formalDefinition="A procedure that is fulfilled in whole or in part by the creation of this media." ) 1185 protected List<Reference> basedOn; 1186 1187 /** 1188 * The status of this document reference. 1189 */ 1190 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 1191 @Description(shortDefinition="current | superseded | entered-in-error", formalDefinition="The status of this document reference." ) 1192 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/document-reference-status") 1193 protected Enumeration<DocumentReferenceStatus> status; 1194 1195 /** 1196 * The status of the underlying document. 1197 */ 1198 @Child(name = "docStatus", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1199 @Description(shortDefinition="registered | partial | preliminary | final | amended | corrected | appended | cancelled | entered-in-error | deprecated | unknown", formalDefinition="The status of the underlying document." ) 1200 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composition-status") 1201 protected Enumeration<CompositionStatus> docStatus; 1202 1203 /** 1204 * Imaging modality used. This may include both acquisition and non-acquisition modalities. 1205 */ 1206 @Child(name = "modality", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1207 @Description(shortDefinition="Imaging modality used", formalDefinition="Imaging modality used. This may include both acquisition and non-acquisition modalities." ) 1208 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_33.html") 1209 protected List<CodeableConcept> modality; 1210 1211 /** 1212 * Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced. 1213 */ 1214 @Child(name = "type", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 1215 @Description(shortDefinition="Kind of document (LOINC if possible)", formalDefinition="Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced." ) 1216 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/doc-typecodes") 1217 protected CodeableConcept type; 1218 1219 /** 1220 * A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type. 1221 */ 1222 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1223 @Description(shortDefinition="Categorization of document", formalDefinition="A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type." ) 1224 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/referenced-item-category") 1225 protected List<CodeableConcept> category; 1226 1227 /** 1228 * Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). 1229 */ 1230 @Child(name = "subject", type = {Reference.class}, order=8, min=0, max=1, modifier=false, summary=true) 1231 @Description(shortDefinition="Who/what is the subject of the document", formalDefinition="Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure)." ) 1232 protected Reference subject; 1233 1234 /** 1235 * Describes the clinical encounter or type of care that the document content is associated with. 1236 */ 1237 @Child(name = "context", type = {Appointment.class, Encounter.class, EpisodeOfCare.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1238 @Description(shortDefinition="Context of the document content", formalDefinition="Describes the clinical encounter or type of care that the document content is associated with." ) 1239 protected List<Reference> context; 1240 1241 /** 1242 * This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a "History and Physical Report" in which the procedure being documented is necessarily a "History and Physical" act. 1243 */ 1244 @Child(name = "event", type = {CodeableReference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1245 @Description(shortDefinition="Main clinical acts documented", formalDefinition="This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act." ) 1246 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActCode") 1247 protected List<CodeableReference> event; 1248 1249 /** 1250 * The anatomic structures included in the document. 1251 */ 1252 @Child(name = "bodySite", type = {CodeableReference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1253 @Description(shortDefinition="Body part included", formalDefinition="The anatomic structures included in the document." ) 1254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1255 protected List<CodeableReference> bodySite; 1256 1257 /** 1258 * The kind of facility where the patient was seen. 1259 */ 1260 @Child(name = "facilityType", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 1261 @Description(shortDefinition="Kind of facility where patient was seen", formalDefinition="The kind of facility where the patient was seen." ) 1262 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-facilitycodes") 1263 protected CodeableConcept facilityType; 1264 1265 /** 1266 * This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty. 1267 */ 1268 @Child(name = "practiceSetting", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 1269 @Description(shortDefinition="Additional details about where the content was created (e.g. clinical specialty)", formalDefinition="This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty." ) 1270 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 1271 protected CodeableConcept practiceSetting; 1272 1273 /** 1274 * The time period over which the service that is described by the document was provided. 1275 */ 1276 @Child(name = "period", type = {Period.class}, order=14, min=0, max=1, modifier=false, summary=true) 1277 @Description(shortDefinition="Time of service that is being documented", formalDefinition="The time period over which the service that is described by the document was provided." ) 1278 protected Period period; 1279 1280 /** 1281 * When the document reference was created. 1282 */ 1283 @Child(name = "date", type = {InstantType.class}, order=15, min=0, max=1, modifier=false, summary=true) 1284 @Description(shortDefinition="When this document reference was created", formalDefinition="When the document reference was created." ) 1285 protected InstantType date; 1286 1287 /** 1288 * Identifies who is responsible for adding the information to the document. 1289 */ 1290 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Organization.class, Device.class, Patient.class, RelatedPerson.class, CareTeam.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1291 @Description(shortDefinition="Who and/or what authored the document", formalDefinition="Identifies who is responsible for adding the information to the document." ) 1292 protected List<Reference> author; 1293 1294 /** 1295 * A participant who has authenticated the accuracy of the document. 1296 */ 1297 @Child(name = "attester", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1298 @Description(shortDefinition="Attests to accuracy of the document", formalDefinition="A participant who has authenticated the accuracy of the document." ) 1299 protected List<DocumentReferenceAttesterComponent> attester; 1300 1301 /** 1302 * Identifies the organization or group who is responsible for ongoing maintenance of and access to the document. 1303 */ 1304 @Child(name = "custodian", type = {Organization.class}, order=18, min=0, max=1, modifier=false, summary=false) 1305 @Description(shortDefinition="Organization which maintains the document", formalDefinition="Identifies the organization or group who is responsible for ongoing maintenance of and access to the document." ) 1306 protected Reference custodian; 1307 1308 /** 1309 * Relationships that this document has with other document references that already exist. 1310 */ 1311 @Child(name = "relatesTo", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1312 @Description(shortDefinition="Relationships to other documents", formalDefinition="Relationships that this document has with other document references that already exist." ) 1313 protected List<DocumentReferenceRelatesToComponent> relatesTo; 1314 1315 /** 1316 * Human-readable description of the source document. 1317 */ 1318 @Child(name = "description", type = {MarkdownType.class}, order=20, min=0, max=1, modifier=false, summary=true) 1319 @Description(shortDefinition="Human-readable description", formalDefinition="Human-readable description of the source document." ) 1320 protected MarkdownType description; 1321 1322 /** 1323 * A set of Security-Tag codes specifying the level of privacy/security of the Document found at DocumentReference.content.attachment.url. Note that DocumentReference.meta.security contains the security labels of the data elements in DocumentReference, while DocumentReference.securityLabel contains the security labels for the document the reference refers to. The distinction recognizes that the document may contain sensitive information, while the DocumentReference is metadata about the document and thus might not be as sensitive as the document. For example: a psychotherapy episode may contain highly sensitive information, while the metadata may simply indicate that some episode happened. 1324 */ 1325 @Child(name = "securityLabel", type = {CodeableConcept.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1326 @Description(shortDefinition="Document security-tags", formalDefinition="A set of Security-Tag codes specifying the level of privacy/security of the Document found at DocumentReference.content.attachment.url. Note that DocumentReference.meta.security contains the security labels of the data elements in DocumentReference, while DocumentReference.securityLabel contains the security labels for the document the reference refers to. The distinction recognizes that the document may contain sensitive information, while the DocumentReference is metadata about the document and thus might not be as sensitive as the document. For example: a psychotherapy episode may contain highly sensitive information, while the metadata may simply indicate that some episode happened." ) 1327 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/security-label-examples") 1328 protected List<CodeableConcept> securityLabel; 1329 1330 /** 1331 * The document and format referenced. If there are multiple content element repetitions, these must all represent the same document in different format, or attachment metadata. 1332 */ 1333 @Child(name = "content", type = {}, order=22, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1334 @Description(shortDefinition="Document referenced", formalDefinition="The document and format referenced. If there are multiple content element repetitions, these must all represent the same document in different format, or attachment metadata." ) 1335 protected List<DocumentReferenceContentComponent> content; 1336 1337 private static final long serialVersionUID = -981268007L; 1338 1339 /** 1340 * Constructor 1341 */ 1342 public DocumentReference() { 1343 super(); 1344 } 1345 1346 /** 1347 * Constructor 1348 */ 1349 public DocumentReference(DocumentReferenceStatus status, DocumentReferenceContentComponent content) { 1350 super(); 1351 this.setStatus(status); 1352 this.addContent(content); 1353 } 1354 1355 /** 1356 * @return {@link #identifier} (Other business identifiers associated with the document, including version independent identifiers.) 1357 */ 1358 public List<Identifier> getIdentifier() { 1359 if (this.identifier == null) 1360 this.identifier = new ArrayList<Identifier>(); 1361 return this.identifier; 1362 } 1363 1364 /** 1365 * @return Returns a reference to <code>this</code> for easy method chaining 1366 */ 1367 public DocumentReference setIdentifier(List<Identifier> theIdentifier) { 1368 this.identifier = theIdentifier; 1369 return this; 1370 } 1371 1372 public boolean hasIdentifier() { 1373 if (this.identifier == null) 1374 return false; 1375 for (Identifier item : this.identifier) 1376 if (!item.isEmpty()) 1377 return true; 1378 return false; 1379 } 1380 1381 public Identifier addIdentifier() { //3 1382 Identifier t = new Identifier(); 1383 if (this.identifier == null) 1384 this.identifier = new ArrayList<Identifier>(); 1385 this.identifier.add(t); 1386 return t; 1387 } 1388 1389 public DocumentReference addIdentifier(Identifier t) { //3 1390 if (t == null) 1391 return this; 1392 if (this.identifier == null) 1393 this.identifier = new ArrayList<Identifier>(); 1394 this.identifier.add(t); 1395 return this; 1396 } 1397 1398 /** 1399 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1400 */ 1401 public Identifier getIdentifierFirstRep() { 1402 if (getIdentifier().isEmpty()) { 1403 addIdentifier(); 1404 } 1405 return getIdentifier().get(0); 1406 } 1407 1408 /** 1409 * @return {@link #version} (An explicitly assigned identifer of a variation of the content in the DocumentReference.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1410 */ 1411 public StringType getVersionElement() { 1412 if (this.version == null) 1413 if (Configuration.errorOnAutoCreate()) 1414 throw new Error("Attempt to auto-create DocumentReference.version"); 1415 else if (Configuration.doAutoCreate()) 1416 this.version = new StringType(); // bb 1417 return this.version; 1418 } 1419 1420 public boolean hasVersionElement() { 1421 return this.version != null && !this.version.isEmpty(); 1422 } 1423 1424 public boolean hasVersion() { 1425 return this.version != null && !this.version.isEmpty(); 1426 } 1427 1428 /** 1429 * @param value {@link #version} (An explicitly assigned identifer of a variation of the content in the DocumentReference.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1430 */ 1431 public DocumentReference setVersionElement(StringType value) { 1432 this.version = value; 1433 return this; 1434 } 1435 1436 /** 1437 * @return An explicitly assigned identifer of a variation of the content in the DocumentReference. 1438 */ 1439 public String getVersion() { 1440 return this.version == null ? null : this.version.getValue(); 1441 } 1442 1443 /** 1444 * @param value An explicitly assigned identifer of a variation of the content in the DocumentReference. 1445 */ 1446 public DocumentReference setVersion(String value) { 1447 if (Utilities.noString(value)) 1448 this.version = null; 1449 else { 1450 if (this.version == null) 1451 this.version = new StringType(); 1452 this.version.setValue(value); 1453 } 1454 return this; 1455 } 1456 1457 /** 1458 * @return {@link #basedOn} (A procedure that is fulfilled in whole or in part by the creation of this media.) 1459 */ 1460 public List<Reference> getBasedOn() { 1461 if (this.basedOn == null) 1462 this.basedOn = new ArrayList<Reference>(); 1463 return this.basedOn; 1464 } 1465 1466 /** 1467 * @return Returns a reference to <code>this</code> for easy method chaining 1468 */ 1469 public DocumentReference setBasedOn(List<Reference> theBasedOn) { 1470 this.basedOn = theBasedOn; 1471 return this; 1472 } 1473 1474 public boolean hasBasedOn() { 1475 if (this.basedOn == null) 1476 return false; 1477 for (Reference item : this.basedOn) 1478 if (!item.isEmpty()) 1479 return true; 1480 return false; 1481 } 1482 1483 public Reference addBasedOn() { //3 1484 Reference t = new Reference(); 1485 if (this.basedOn == null) 1486 this.basedOn = new ArrayList<Reference>(); 1487 this.basedOn.add(t); 1488 return t; 1489 } 1490 1491 public DocumentReference addBasedOn(Reference t) { //3 1492 if (t == null) 1493 return this; 1494 if (this.basedOn == null) 1495 this.basedOn = new ArrayList<Reference>(); 1496 this.basedOn.add(t); 1497 return this; 1498 } 1499 1500 /** 1501 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1502 */ 1503 public Reference getBasedOnFirstRep() { 1504 if (getBasedOn().isEmpty()) { 1505 addBasedOn(); 1506 } 1507 return getBasedOn().get(0); 1508 } 1509 1510 /** 1511 * @return {@link #status} (The status of this document reference.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1512 */ 1513 public Enumeration<DocumentReferenceStatus> getStatusElement() { 1514 if (this.status == null) 1515 if (Configuration.errorOnAutoCreate()) 1516 throw new Error("Attempt to auto-create DocumentReference.status"); 1517 else if (Configuration.doAutoCreate()) 1518 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 1519 return this.status; 1520 } 1521 1522 public boolean hasStatusElement() { 1523 return this.status != null && !this.status.isEmpty(); 1524 } 1525 1526 public boolean hasStatus() { 1527 return this.status != null && !this.status.isEmpty(); 1528 } 1529 1530 /** 1531 * @param value {@link #status} (The status of this document reference.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1532 */ 1533 public DocumentReference setStatusElement(Enumeration<DocumentReferenceStatus> value) { 1534 this.status = value; 1535 return this; 1536 } 1537 1538 /** 1539 * @return The status of this document reference. 1540 */ 1541 public DocumentReferenceStatus getStatus() { 1542 return this.status == null ? null : this.status.getValue(); 1543 } 1544 1545 /** 1546 * @param value The status of this document reference. 1547 */ 1548 public DocumentReference setStatus(DocumentReferenceStatus value) { 1549 if (this.status == null) 1550 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 1551 this.status.setValue(value); 1552 return this; 1553 } 1554 1555 /** 1556 * @return {@link #docStatus} (The status of the underlying document.). This is the underlying object with id, value and extensions. The accessor "getDocStatus" gives direct access to the value 1557 */ 1558 public Enumeration<CompositionStatus> getDocStatusElement() { 1559 if (this.docStatus == null) 1560 if (Configuration.errorOnAutoCreate()) 1561 throw new Error("Attempt to auto-create DocumentReference.docStatus"); 1562 else if (Configuration.doAutoCreate()) 1563 this.docStatus = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); // bb 1564 return this.docStatus; 1565 } 1566 1567 public boolean hasDocStatusElement() { 1568 return this.docStatus != null && !this.docStatus.isEmpty(); 1569 } 1570 1571 public boolean hasDocStatus() { 1572 return this.docStatus != null && !this.docStatus.isEmpty(); 1573 } 1574 1575 /** 1576 * @param value {@link #docStatus} (The status of the underlying document.). This is the underlying object with id, value and extensions. The accessor "getDocStatus" gives direct access to the value 1577 */ 1578 public DocumentReference setDocStatusElement(Enumeration<CompositionStatus> value) { 1579 this.docStatus = value; 1580 return this; 1581 } 1582 1583 /** 1584 * @return The status of the underlying document. 1585 */ 1586 public CompositionStatus getDocStatus() { 1587 return this.docStatus == null ? null : this.docStatus.getValue(); 1588 } 1589 1590 /** 1591 * @param value The status of the underlying document. 1592 */ 1593 public DocumentReference setDocStatus(CompositionStatus value) { 1594 if (value == null) 1595 this.docStatus = null; 1596 else { 1597 if (this.docStatus == null) 1598 this.docStatus = new Enumeration<CompositionStatus>(new CompositionStatusEnumFactory()); 1599 this.docStatus.setValue(value); 1600 } 1601 return this; 1602 } 1603 1604 /** 1605 * @return {@link #modality} (Imaging modality used. This may include both acquisition and non-acquisition modalities.) 1606 */ 1607 public List<CodeableConcept> getModality() { 1608 if (this.modality == null) 1609 this.modality = new ArrayList<CodeableConcept>(); 1610 return this.modality; 1611 } 1612 1613 /** 1614 * @return Returns a reference to <code>this</code> for easy method chaining 1615 */ 1616 public DocumentReference setModality(List<CodeableConcept> theModality) { 1617 this.modality = theModality; 1618 return this; 1619 } 1620 1621 public boolean hasModality() { 1622 if (this.modality == null) 1623 return false; 1624 for (CodeableConcept item : this.modality) 1625 if (!item.isEmpty()) 1626 return true; 1627 return false; 1628 } 1629 1630 public CodeableConcept addModality() { //3 1631 CodeableConcept t = new CodeableConcept(); 1632 if (this.modality == null) 1633 this.modality = new ArrayList<CodeableConcept>(); 1634 this.modality.add(t); 1635 return t; 1636 } 1637 1638 public DocumentReference addModality(CodeableConcept t) { //3 1639 if (t == null) 1640 return this; 1641 if (this.modality == null) 1642 this.modality = new ArrayList<CodeableConcept>(); 1643 this.modality.add(t); 1644 return this; 1645 } 1646 1647 /** 1648 * @return The first repetition of repeating field {@link #modality}, creating it if it does not already exist {3} 1649 */ 1650 public CodeableConcept getModalityFirstRep() { 1651 if (getModality().isEmpty()) { 1652 addModality(); 1653 } 1654 return getModality().get(0); 1655 } 1656 1657 /** 1658 * @return {@link #type} (Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.) 1659 */ 1660 public CodeableConcept getType() { 1661 if (this.type == null) 1662 if (Configuration.errorOnAutoCreate()) 1663 throw new Error("Attempt to auto-create DocumentReference.type"); 1664 else if (Configuration.doAutoCreate()) 1665 this.type = new CodeableConcept(); // cc 1666 return this.type; 1667 } 1668 1669 public boolean hasType() { 1670 return this.type != null && !this.type.isEmpty(); 1671 } 1672 1673 /** 1674 * @param value {@link #type} (Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.) 1675 */ 1676 public DocumentReference setType(CodeableConcept value) { 1677 this.type = value; 1678 return this; 1679 } 1680 1681 /** 1682 * @return {@link #category} (A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.) 1683 */ 1684 public List<CodeableConcept> getCategory() { 1685 if (this.category == null) 1686 this.category = new ArrayList<CodeableConcept>(); 1687 return this.category; 1688 } 1689 1690 /** 1691 * @return Returns a reference to <code>this</code> for easy method chaining 1692 */ 1693 public DocumentReference setCategory(List<CodeableConcept> theCategory) { 1694 this.category = theCategory; 1695 return this; 1696 } 1697 1698 public boolean hasCategory() { 1699 if (this.category == null) 1700 return false; 1701 for (CodeableConcept item : this.category) 1702 if (!item.isEmpty()) 1703 return true; 1704 return false; 1705 } 1706 1707 public CodeableConcept addCategory() { //3 1708 CodeableConcept t = new CodeableConcept(); 1709 if (this.category == null) 1710 this.category = new ArrayList<CodeableConcept>(); 1711 this.category.add(t); 1712 return t; 1713 } 1714 1715 public DocumentReference addCategory(CodeableConcept t) { //3 1716 if (t == null) 1717 return this; 1718 if (this.category == null) 1719 this.category = new ArrayList<CodeableConcept>(); 1720 this.category.add(t); 1721 return this; 1722 } 1723 1724 /** 1725 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1726 */ 1727 public CodeableConcept getCategoryFirstRep() { 1728 if (getCategory().isEmpty()) { 1729 addCategory(); 1730 } 1731 return getCategory().get(0); 1732 } 1733 1734 /** 1735 * @return {@link #subject} (Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).) 1736 */ 1737 public Reference getSubject() { 1738 if (this.subject == null) 1739 if (Configuration.errorOnAutoCreate()) 1740 throw new Error("Attempt to auto-create DocumentReference.subject"); 1741 else if (Configuration.doAutoCreate()) 1742 this.subject = new Reference(); // cc 1743 return this.subject; 1744 } 1745 1746 public boolean hasSubject() { 1747 return this.subject != null && !this.subject.isEmpty(); 1748 } 1749 1750 /** 1751 * @param value {@link #subject} (Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).) 1752 */ 1753 public DocumentReference setSubject(Reference value) { 1754 this.subject = value; 1755 return this; 1756 } 1757 1758 /** 1759 * @return {@link #context} (Describes the clinical encounter or type of care that the document content is associated with.) 1760 */ 1761 public List<Reference> getContext() { 1762 if (this.context == null) 1763 this.context = new ArrayList<Reference>(); 1764 return this.context; 1765 } 1766 1767 /** 1768 * @return Returns a reference to <code>this</code> for easy method chaining 1769 */ 1770 public DocumentReference setContext(List<Reference> theContext) { 1771 this.context = theContext; 1772 return this; 1773 } 1774 1775 public boolean hasContext() { 1776 if (this.context == null) 1777 return false; 1778 for (Reference item : this.context) 1779 if (!item.isEmpty()) 1780 return true; 1781 return false; 1782 } 1783 1784 public Reference addContext() { //3 1785 Reference t = new Reference(); 1786 if (this.context == null) 1787 this.context = new ArrayList<Reference>(); 1788 this.context.add(t); 1789 return t; 1790 } 1791 1792 public DocumentReference addContext(Reference t) { //3 1793 if (t == null) 1794 return this; 1795 if (this.context == null) 1796 this.context = new ArrayList<Reference>(); 1797 this.context.add(t); 1798 return this; 1799 } 1800 1801 /** 1802 * @return The first repetition of repeating field {@link #context}, creating it if it does not already exist {3} 1803 */ 1804 public Reference getContextFirstRep() { 1805 if (getContext().isEmpty()) { 1806 addContext(); 1807 } 1808 return getContext().get(0); 1809 } 1810 1811 /** 1812 * @return {@link #event} (This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a "History and Physical Report" in which the procedure being documented is necessarily a "History and Physical" act.) 1813 */ 1814 public List<CodeableReference> getEvent() { 1815 if (this.event == null) 1816 this.event = new ArrayList<CodeableReference>(); 1817 return this.event; 1818 } 1819 1820 /** 1821 * @return Returns a reference to <code>this</code> for easy method chaining 1822 */ 1823 public DocumentReference setEvent(List<CodeableReference> theEvent) { 1824 this.event = theEvent; 1825 return this; 1826 } 1827 1828 public boolean hasEvent() { 1829 if (this.event == null) 1830 return false; 1831 for (CodeableReference item : this.event) 1832 if (!item.isEmpty()) 1833 return true; 1834 return false; 1835 } 1836 1837 public CodeableReference addEvent() { //3 1838 CodeableReference t = new CodeableReference(); 1839 if (this.event == null) 1840 this.event = new ArrayList<CodeableReference>(); 1841 this.event.add(t); 1842 return t; 1843 } 1844 1845 public DocumentReference addEvent(CodeableReference t) { //3 1846 if (t == null) 1847 return this; 1848 if (this.event == null) 1849 this.event = new ArrayList<CodeableReference>(); 1850 this.event.add(t); 1851 return this; 1852 } 1853 1854 /** 1855 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 1856 */ 1857 public CodeableReference getEventFirstRep() { 1858 if (getEvent().isEmpty()) { 1859 addEvent(); 1860 } 1861 return getEvent().get(0); 1862 } 1863 1864 /** 1865 * @return {@link #bodySite} (The anatomic structures included in the document.) 1866 */ 1867 public List<CodeableReference> getBodySite() { 1868 if (this.bodySite == null) 1869 this.bodySite = new ArrayList<CodeableReference>(); 1870 return this.bodySite; 1871 } 1872 1873 /** 1874 * @return Returns a reference to <code>this</code> for easy method chaining 1875 */ 1876 public DocumentReference setBodySite(List<CodeableReference> theBodySite) { 1877 this.bodySite = theBodySite; 1878 return this; 1879 } 1880 1881 public boolean hasBodySite() { 1882 if (this.bodySite == null) 1883 return false; 1884 for (CodeableReference item : this.bodySite) 1885 if (!item.isEmpty()) 1886 return true; 1887 return false; 1888 } 1889 1890 public CodeableReference addBodySite() { //3 1891 CodeableReference t = new CodeableReference(); 1892 if (this.bodySite == null) 1893 this.bodySite = new ArrayList<CodeableReference>(); 1894 this.bodySite.add(t); 1895 return t; 1896 } 1897 1898 public DocumentReference addBodySite(CodeableReference t) { //3 1899 if (t == null) 1900 return this; 1901 if (this.bodySite == null) 1902 this.bodySite = new ArrayList<CodeableReference>(); 1903 this.bodySite.add(t); 1904 return this; 1905 } 1906 1907 /** 1908 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 1909 */ 1910 public CodeableReference getBodySiteFirstRep() { 1911 if (getBodySite().isEmpty()) { 1912 addBodySite(); 1913 } 1914 return getBodySite().get(0); 1915 } 1916 1917 /** 1918 * @return {@link #facilityType} (The kind of facility where the patient was seen.) 1919 */ 1920 public CodeableConcept getFacilityType() { 1921 if (this.facilityType == null) 1922 if (Configuration.errorOnAutoCreate()) 1923 throw new Error("Attempt to auto-create DocumentReference.facilityType"); 1924 else if (Configuration.doAutoCreate()) 1925 this.facilityType = new CodeableConcept(); // cc 1926 return this.facilityType; 1927 } 1928 1929 public boolean hasFacilityType() { 1930 return this.facilityType != null && !this.facilityType.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #facilityType} (The kind of facility where the patient was seen.) 1935 */ 1936 public DocumentReference setFacilityType(CodeableConcept value) { 1937 this.facilityType = value; 1938 return this; 1939 } 1940 1941 /** 1942 * @return {@link #practiceSetting} (This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.) 1943 */ 1944 public CodeableConcept getPracticeSetting() { 1945 if (this.practiceSetting == null) 1946 if (Configuration.errorOnAutoCreate()) 1947 throw new Error("Attempt to auto-create DocumentReference.practiceSetting"); 1948 else if (Configuration.doAutoCreate()) 1949 this.practiceSetting = new CodeableConcept(); // cc 1950 return this.practiceSetting; 1951 } 1952 1953 public boolean hasPracticeSetting() { 1954 return this.practiceSetting != null && !this.practiceSetting.isEmpty(); 1955 } 1956 1957 /** 1958 * @param value {@link #practiceSetting} (This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.) 1959 */ 1960 public DocumentReference setPracticeSetting(CodeableConcept value) { 1961 this.practiceSetting = value; 1962 return this; 1963 } 1964 1965 /** 1966 * @return {@link #period} (The time period over which the service that is described by the document was provided.) 1967 */ 1968 public Period getPeriod() { 1969 if (this.period == null) 1970 if (Configuration.errorOnAutoCreate()) 1971 throw new Error("Attempt to auto-create DocumentReference.period"); 1972 else if (Configuration.doAutoCreate()) 1973 this.period = new Period(); // cc 1974 return this.period; 1975 } 1976 1977 public boolean hasPeriod() { 1978 return this.period != null && !this.period.isEmpty(); 1979 } 1980 1981 /** 1982 * @param value {@link #period} (The time period over which the service that is described by the document was provided.) 1983 */ 1984 public DocumentReference setPeriod(Period value) { 1985 this.period = value; 1986 return this; 1987 } 1988 1989 /** 1990 * @return {@link #date} (When the document reference was created.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1991 */ 1992 public InstantType getDateElement() { 1993 if (this.date == null) 1994 if (Configuration.errorOnAutoCreate()) 1995 throw new Error("Attempt to auto-create DocumentReference.date"); 1996 else if (Configuration.doAutoCreate()) 1997 this.date = new InstantType(); // bb 1998 return this.date; 1999 } 2000 2001 public boolean hasDateElement() { 2002 return this.date != null && !this.date.isEmpty(); 2003 } 2004 2005 public boolean hasDate() { 2006 return this.date != null && !this.date.isEmpty(); 2007 } 2008 2009 /** 2010 * @param value {@link #date} (When the document reference was created.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2011 */ 2012 public DocumentReference setDateElement(InstantType value) { 2013 this.date = value; 2014 return this; 2015 } 2016 2017 /** 2018 * @return When the document reference was created. 2019 */ 2020 public Date getDate() { 2021 return this.date == null ? null : this.date.getValue(); 2022 } 2023 2024 /** 2025 * @param value When the document reference was created. 2026 */ 2027 public DocumentReference setDate(Date value) { 2028 if (value == null) 2029 this.date = null; 2030 else { 2031 if (this.date == null) 2032 this.date = new InstantType(); 2033 this.date.setValue(value); 2034 } 2035 return this; 2036 } 2037 2038 /** 2039 * @return {@link #author} (Identifies who is responsible for adding the information to the document.) 2040 */ 2041 public List<Reference> getAuthor() { 2042 if (this.author == null) 2043 this.author = new ArrayList<Reference>(); 2044 return this.author; 2045 } 2046 2047 /** 2048 * @return Returns a reference to <code>this</code> for easy method chaining 2049 */ 2050 public DocumentReference setAuthor(List<Reference> theAuthor) { 2051 this.author = theAuthor; 2052 return this; 2053 } 2054 2055 public boolean hasAuthor() { 2056 if (this.author == null) 2057 return false; 2058 for (Reference item : this.author) 2059 if (!item.isEmpty()) 2060 return true; 2061 return false; 2062 } 2063 2064 public Reference addAuthor() { //3 2065 Reference t = new Reference(); 2066 if (this.author == null) 2067 this.author = new ArrayList<Reference>(); 2068 this.author.add(t); 2069 return t; 2070 } 2071 2072 public DocumentReference addAuthor(Reference t) { //3 2073 if (t == null) 2074 return this; 2075 if (this.author == null) 2076 this.author = new ArrayList<Reference>(); 2077 this.author.add(t); 2078 return this; 2079 } 2080 2081 /** 2082 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 2083 */ 2084 public Reference getAuthorFirstRep() { 2085 if (getAuthor().isEmpty()) { 2086 addAuthor(); 2087 } 2088 return getAuthor().get(0); 2089 } 2090 2091 /** 2092 * @return {@link #attester} (A participant who has authenticated the accuracy of the document.) 2093 */ 2094 public List<DocumentReferenceAttesterComponent> getAttester() { 2095 if (this.attester == null) 2096 this.attester = new ArrayList<DocumentReferenceAttesterComponent>(); 2097 return this.attester; 2098 } 2099 2100 /** 2101 * @return Returns a reference to <code>this</code> for easy method chaining 2102 */ 2103 public DocumentReference setAttester(List<DocumentReferenceAttesterComponent> theAttester) { 2104 this.attester = theAttester; 2105 return this; 2106 } 2107 2108 public boolean hasAttester() { 2109 if (this.attester == null) 2110 return false; 2111 for (DocumentReferenceAttesterComponent item : this.attester) 2112 if (!item.isEmpty()) 2113 return true; 2114 return false; 2115 } 2116 2117 public DocumentReferenceAttesterComponent addAttester() { //3 2118 DocumentReferenceAttesterComponent t = new DocumentReferenceAttesterComponent(); 2119 if (this.attester == null) 2120 this.attester = new ArrayList<DocumentReferenceAttesterComponent>(); 2121 this.attester.add(t); 2122 return t; 2123 } 2124 2125 public DocumentReference addAttester(DocumentReferenceAttesterComponent t) { //3 2126 if (t == null) 2127 return this; 2128 if (this.attester == null) 2129 this.attester = new ArrayList<DocumentReferenceAttesterComponent>(); 2130 this.attester.add(t); 2131 return this; 2132 } 2133 2134 /** 2135 * @return The first repetition of repeating field {@link #attester}, creating it if it does not already exist {3} 2136 */ 2137 public DocumentReferenceAttesterComponent getAttesterFirstRep() { 2138 if (getAttester().isEmpty()) { 2139 addAttester(); 2140 } 2141 return getAttester().get(0); 2142 } 2143 2144 /** 2145 * @return {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.) 2146 */ 2147 public Reference getCustodian() { 2148 if (this.custodian == null) 2149 if (Configuration.errorOnAutoCreate()) 2150 throw new Error("Attempt to auto-create DocumentReference.custodian"); 2151 else if (Configuration.doAutoCreate()) 2152 this.custodian = new Reference(); // cc 2153 return this.custodian; 2154 } 2155 2156 public boolean hasCustodian() { 2157 return this.custodian != null && !this.custodian.isEmpty(); 2158 } 2159 2160 /** 2161 * @param value {@link #custodian} (Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.) 2162 */ 2163 public DocumentReference setCustodian(Reference value) { 2164 this.custodian = value; 2165 return this; 2166 } 2167 2168 /** 2169 * @return {@link #relatesTo} (Relationships that this document has with other document references that already exist.) 2170 */ 2171 public List<DocumentReferenceRelatesToComponent> getRelatesTo() { 2172 if (this.relatesTo == null) 2173 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2174 return this.relatesTo; 2175 } 2176 2177 /** 2178 * @return Returns a reference to <code>this</code> for easy method chaining 2179 */ 2180 public DocumentReference setRelatesTo(List<DocumentReferenceRelatesToComponent> theRelatesTo) { 2181 this.relatesTo = theRelatesTo; 2182 return this; 2183 } 2184 2185 public boolean hasRelatesTo() { 2186 if (this.relatesTo == null) 2187 return false; 2188 for (DocumentReferenceRelatesToComponent item : this.relatesTo) 2189 if (!item.isEmpty()) 2190 return true; 2191 return false; 2192 } 2193 2194 public DocumentReferenceRelatesToComponent addRelatesTo() { //3 2195 DocumentReferenceRelatesToComponent t = new DocumentReferenceRelatesToComponent(); 2196 if (this.relatesTo == null) 2197 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2198 this.relatesTo.add(t); 2199 return t; 2200 } 2201 2202 public DocumentReference addRelatesTo(DocumentReferenceRelatesToComponent t) { //3 2203 if (t == null) 2204 return this; 2205 if (this.relatesTo == null) 2206 this.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2207 this.relatesTo.add(t); 2208 return this; 2209 } 2210 2211 /** 2212 * @return The first repetition of repeating field {@link #relatesTo}, creating it if it does not already exist {3} 2213 */ 2214 public DocumentReferenceRelatesToComponent getRelatesToFirstRep() { 2215 if (getRelatesTo().isEmpty()) { 2216 addRelatesTo(); 2217 } 2218 return getRelatesTo().get(0); 2219 } 2220 2221 /** 2222 * @return {@link #description} (Human-readable description of the source document.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2223 */ 2224 public MarkdownType getDescriptionElement() { 2225 if (this.description == null) 2226 if (Configuration.errorOnAutoCreate()) 2227 throw new Error("Attempt to auto-create DocumentReference.description"); 2228 else if (Configuration.doAutoCreate()) 2229 this.description = new MarkdownType(); // bb 2230 return this.description; 2231 } 2232 2233 public boolean hasDescriptionElement() { 2234 return this.description != null && !this.description.isEmpty(); 2235 } 2236 2237 public boolean hasDescription() { 2238 return this.description != null && !this.description.isEmpty(); 2239 } 2240 2241 /** 2242 * @param value {@link #description} (Human-readable description of the source document.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2243 */ 2244 public DocumentReference setDescriptionElement(MarkdownType value) { 2245 this.description = value; 2246 return this; 2247 } 2248 2249 /** 2250 * @return Human-readable description of the source document. 2251 */ 2252 public String getDescription() { 2253 return this.description == null ? null : this.description.getValue(); 2254 } 2255 2256 /** 2257 * @param value Human-readable description of the source document. 2258 */ 2259 public DocumentReference setDescription(String value) { 2260 if (Utilities.noString(value)) 2261 this.description = null; 2262 else { 2263 if (this.description == null) 2264 this.description = new MarkdownType(); 2265 this.description.setValue(value); 2266 } 2267 return this; 2268 } 2269 2270 /** 2271 * @return {@link #securityLabel} (A set of Security-Tag codes specifying the level of privacy/security of the Document found at DocumentReference.content.attachment.url. Note that DocumentReference.meta.security contains the security labels of the data elements in DocumentReference, while DocumentReference.securityLabel contains the security labels for the document the reference refers to. The distinction recognizes that the document may contain sensitive information, while the DocumentReference is metadata about the document and thus might not be as sensitive as the document. For example: a psychotherapy episode may contain highly sensitive information, while the metadata may simply indicate that some episode happened.) 2272 */ 2273 public List<CodeableConcept> getSecurityLabel() { 2274 if (this.securityLabel == null) 2275 this.securityLabel = new ArrayList<CodeableConcept>(); 2276 return this.securityLabel; 2277 } 2278 2279 /** 2280 * @return Returns a reference to <code>this</code> for easy method chaining 2281 */ 2282 public DocumentReference setSecurityLabel(List<CodeableConcept> theSecurityLabel) { 2283 this.securityLabel = theSecurityLabel; 2284 return this; 2285 } 2286 2287 public boolean hasSecurityLabel() { 2288 if (this.securityLabel == null) 2289 return false; 2290 for (CodeableConcept item : this.securityLabel) 2291 if (!item.isEmpty()) 2292 return true; 2293 return false; 2294 } 2295 2296 public CodeableConcept addSecurityLabel() { //3 2297 CodeableConcept t = new CodeableConcept(); 2298 if (this.securityLabel == null) 2299 this.securityLabel = new ArrayList<CodeableConcept>(); 2300 this.securityLabel.add(t); 2301 return t; 2302 } 2303 2304 public DocumentReference addSecurityLabel(CodeableConcept t) { //3 2305 if (t == null) 2306 return this; 2307 if (this.securityLabel == null) 2308 this.securityLabel = new ArrayList<CodeableConcept>(); 2309 this.securityLabel.add(t); 2310 return this; 2311 } 2312 2313 /** 2314 * @return The first repetition of repeating field {@link #securityLabel}, creating it if it does not already exist {3} 2315 */ 2316 public CodeableConcept getSecurityLabelFirstRep() { 2317 if (getSecurityLabel().isEmpty()) { 2318 addSecurityLabel(); 2319 } 2320 return getSecurityLabel().get(0); 2321 } 2322 2323 /** 2324 * @return {@link #content} (The document and format referenced. If there are multiple content element repetitions, these must all represent the same document in different format, or attachment metadata.) 2325 */ 2326 public List<DocumentReferenceContentComponent> getContent() { 2327 if (this.content == null) 2328 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2329 return this.content; 2330 } 2331 2332 /** 2333 * @return Returns a reference to <code>this</code> for easy method chaining 2334 */ 2335 public DocumentReference setContent(List<DocumentReferenceContentComponent> theContent) { 2336 this.content = theContent; 2337 return this; 2338 } 2339 2340 public boolean hasContent() { 2341 if (this.content == null) 2342 return false; 2343 for (DocumentReferenceContentComponent item : this.content) 2344 if (!item.isEmpty()) 2345 return true; 2346 return false; 2347 } 2348 2349 public DocumentReferenceContentComponent addContent() { //3 2350 DocumentReferenceContentComponent t = new DocumentReferenceContentComponent(); 2351 if (this.content == null) 2352 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2353 this.content.add(t); 2354 return t; 2355 } 2356 2357 public DocumentReference addContent(DocumentReferenceContentComponent t) { //3 2358 if (t == null) 2359 return this; 2360 if (this.content == null) 2361 this.content = new ArrayList<DocumentReferenceContentComponent>(); 2362 this.content.add(t); 2363 return this; 2364 } 2365 2366 /** 2367 * @return The first repetition of repeating field {@link #content}, creating it if it does not already exist {3} 2368 */ 2369 public DocumentReferenceContentComponent getContentFirstRep() { 2370 if (getContent().isEmpty()) { 2371 addContent(); 2372 } 2373 return getContent().get(0); 2374 } 2375 2376 protected void listChildren(List<Property> children) { 2377 super.listChildren(children); 2378 children.add(new Property("identifier", "Identifier", "Other business identifiers associated with the document, including version independent identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2379 children.add(new Property("version", "string", "An explicitly assigned identifer of a variation of the content in the DocumentReference.", 0, 1, version)); 2380 children.add(new Property("basedOn", "Reference(Appointment|AppointmentResponse|CarePlan|Claim|CommunicationRequest|Contract|CoverageEligibilityRequest|DeviceRequest|EnrollmentRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|RequestOrchestration|ServiceRequest|SupplyRequest|VisionPrescription)", "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2381 children.add(new Property("status", "code", "The status of this document reference.", 0, 1, status)); 2382 children.add(new Property("docStatus", "code", "The status of the underlying document.", 0, 1, docStatus)); 2383 children.add(new Property("modality", "CodeableConcept", "Imaging modality used. This may include both acquisition and non-acquisition modalities.", 0, java.lang.Integer.MAX_VALUE, modality)); 2384 children.add(new Property("type", "CodeableConcept", "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 0, 1, type)); 2385 children.add(new Property("category", "CodeableConcept", "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 0, java.lang.Integer.MAX_VALUE, category)); 2386 children.add(new Property("subject", "Reference(Any)", "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 0, 1, subject)); 2387 children.add(new Property("context", "Reference(Appointment|Encounter|EpisodeOfCare)", "Describes the clinical encounter or type of care that the document content is associated with.", 0, java.lang.Integer.MAX_VALUE, context)); 2388 children.add(new Property("event", "CodeableReference", "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 0, java.lang.Integer.MAX_VALUE, event)); 2389 children.add(new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures included in the document.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 2390 children.add(new Property("facilityType", "CodeableConcept", "The kind of facility where the patient was seen.", 0, 1, facilityType)); 2391 children.add(new Property("practiceSetting", "CodeableConcept", "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 0, 1, practiceSetting)); 2392 children.add(new Property("period", "Period", "The time period over which the service that is described by the document was provided.", 0, 1, period)); 2393 children.add(new Property("date", "instant", "When the document reference was created.", 0, 1, date)); 2394 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson|CareTeam)", "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, author)); 2395 children.add(new Property("attester", "", "A participant who has authenticated the accuracy of the document.", 0, java.lang.Integer.MAX_VALUE, attester)); 2396 children.add(new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 0, 1, custodian)); 2397 children.add(new Property("relatesTo", "", "Relationships that this document has with other document references that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo)); 2398 children.add(new Property("description", "markdown", "Human-readable description of the source document.", 0, 1, description)); 2399 children.add(new Property("securityLabel", "CodeableConcept", "A set of Security-Tag codes specifying the level of privacy/security of the Document found at DocumentReference.content.attachment.url. Note that DocumentReference.meta.security contains the security labels of the data elements in DocumentReference, while DocumentReference.securityLabel contains the security labels for the document the reference refers to. The distinction recognizes that the document may contain sensitive information, while the DocumentReference is metadata about the document and thus might not be as sensitive as the document. For example: a psychotherapy episode may contain highly sensitive information, while the metadata may simply indicate that some episode happened.", 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2400 children.add(new Property("content", "", "The document and format referenced. If there are multiple content element repetitions, these must all represent the same document in different format, or attachment metadata.", 0, java.lang.Integer.MAX_VALUE, content)); 2401 } 2402 2403 @Override 2404 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2405 switch (_hash) { 2406 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Other business identifiers associated with the document, including version independent identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier); 2407 case 351608024: /*version*/ return new Property("version", "string", "An explicitly assigned identifer of a variation of the content in the DocumentReference.", 0, 1, version); 2408 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Appointment|AppointmentResponse|CarePlan|Claim|CommunicationRequest|Contract|CoverageEligibilityRequest|DeviceRequest|EnrollmentRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|RequestOrchestration|ServiceRequest|SupplyRequest|VisionPrescription)", "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2409 case -892481550: /*status*/ return new Property("status", "code", "The status of this document reference.", 0, 1, status); 2410 case -23496886: /*docStatus*/ return new Property("docStatus", "code", "The status of the underlying document.", 0, 1, docStatus); 2411 case -622722335: /*modality*/ return new Property("modality", "CodeableConcept", "Imaging modality used. This may include both acquisition and non-acquisition modalities.", 0, java.lang.Integer.MAX_VALUE, modality); 2412 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the particular kind of document referenced (e.g. History and Physical, Discharge Summary, Progress Note). This usually equates to the purpose of making the document referenced.", 0, 1, type); 2413 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A categorization for the type of document referenced - helps for indexing and searching. This may be implied by or derived from the code specified in the DocumentReference.type.", 0, java.lang.Integer.MAX_VALUE, category); 2414 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "Who or what the document is about. The document can be about a person, (patient or healthcare practitioner), a device (e.g. a machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure).", 0, 1, subject); 2415 case 951530927: /*context*/ return new Property("context", "Reference(Appointment|Encounter|EpisodeOfCare)", "Describes the clinical encounter or type of care that the document content is associated with.", 0, java.lang.Integer.MAX_VALUE, context); 2416 case 96891546: /*event*/ return new Property("event", "CodeableReference", "This list of codes represents the main clinical acts, such as a colonoscopy or an appendectomy, being documented. In some cases, the event is inherent in the type Code, such as a \"History and Physical Report\" in which the procedure being documented is necessarily a \"History and Physical\" act.", 0, java.lang.Integer.MAX_VALUE, event); 2417 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures included in the document.", 0, java.lang.Integer.MAX_VALUE, bodySite); 2418 case 370698365: /*facilityType*/ return new Property("facilityType", "CodeableConcept", "The kind of facility where the patient was seen.", 0, 1, facilityType); 2419 case 331373717: /*practiceSetting*/ return new Property("practiceSetting", "CodeableConcept", "This property may convey specifics about the practice setting where the content was created, often reflecting the clinical specialty.", 0, 1, practiceSetting); 2420 case -991726143: /*period*/ return new Property("period", "Period", "The time period over which the service that is described by the document was provided.", 0, 1, period); 2421 case 3076014: /*date*/ return new Property("date", "instant", "When the document reference was created.", 0, 1, date); 2422 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson|CareTeam)", "Identifies who is responsible for adding the information to the document.", 0, java.lang.Integer.MAX_VALUE, author); 2423 case 542920370: /*attester*/ return new Property("attester", "", "A participant who has authenticated the accuracy of the document.", 0, java.lang.Integer.MAX_VALUE, attester); 2424 case 1611297262: /*custodian*/ return new Property("custodian", "Reference(Organization)", "Identifies the organization or group who is responsible for ongoing maintenance of and access to the document.", 0, 1, custodian); 2425 case -7765931: /*relatesTo*/ return new Property("relatesTo", "", "Relationships that this document has with other document references that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo); 2426 case -1724546052: /*description*/ return new Property("description", "markdown", "Human-readable description of the source document.", 0, 1, description); 2427 case -722296940: /*securityLabel*/ return new Property("securityLabel", "CodeableConcept", "A set of Security-Tag codes specifying the level of privacy/security of the Document found at DocumentReference.content.attachment.url. Note that DocumentReference.meta.security contains the security labels of the data elements in DocumentReference, while DocumentReference.securityLabel contains the security labels for the document the reference refers to. The distinction recognizes that the document may contain sensitive information, while the DocumentReference is metadata about the document and thus might not be as sensitive as the document. For example: a psychotherapy episode may contain highly sensitive information, while the metadata may simply indicate that some episode happened.", 0, java.lang.Integer.MAX_VALUE, securityLabel); 2428 case 951530617: /*content*/ return new Property("content", "", "The document and format referenced. If there are multiple content element repetitions, these must all represent the same document in different format, or attachment metadata.", 0, java.lang.Integer.MAX_VALUE, content); 2429 default: return super.getNamedProperty(_hash, _name, _checkValid); 2430 } 2431 2432 } 2433 2434 @Override 2435 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2436 switch (hash) { 2437 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2438 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2439 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2440 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<DocumentReferenceStatus> 2441 case -23496886: /*docStatus*/ return this.docStatus == null ? new Base[0] : new Base[] {this.docStatus}; // Enumeration<CompositionStatus> 2442 case -622722335: /*modality*/ return this.modality == null ? new Base[0] : this.modality.toArray(new Base[this.modality.size()]); // CodeableConcept 2443 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2444 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2445 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2446 case 951530927: /*context*/ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // Reference 2447 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // CodeableReference 2448 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableReference 2449 case 370698365: /*facilityType*/ return this.facilityType == null ? new Base[0] : new Base[] {this.facilityType}; // CodeableConcept 2450 case 331373717: /*practiceSetting*/ return this.practiceSetting == null ? new Base[0] : new Base[] {this.practiceSetting}; // CodeableConcept 2451 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 2452 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // InstantType 2453 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2454 case 542920370: /*attester*/ return this.attester == null ? new Base[0] : this.attester.toArray(new Base[this.attester.size()]); // DocumentReferenceAttesterComponent 2455 case 1611297262: /*custodian*/ return this.custodian == null ? new Base[0] : new Base[] {this.custodian}; // Reference 2456 case -7765931: /*relatesTo*/ return this.relatesTo == null ? new Base[0] : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // DocumentReferenceRelatesToComponent 2457 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2458 case -722296940: /*securityLabel*/ return this.securityLabel == null ? new Base[0] : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // CodeableConcept 2459 case 951530617: /*content*/ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // DocumentReferenceContentComponent 2460 default: return super.getProperty(hash, name, checkValid); 2461 } 2462 2463 } 2464 2465 @Override 2466 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2467 switch (hash) { 2468 case -1618432855: // identifier 2469 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2470 return value; 2471 case 351608024: // version 2472 this.version = TypeConvertor.castToString(value); // StringType 2473 return value; 2474 case -332612366: // basedOn 2475 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2476 return value; 2477 case -892481550: // status 2478 value = new DocumentReferenceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2479 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2480 return value; 2481 case -23496886: // docStatus 2482 value = new CompositionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2483 this.docStatus = (Enumeration) value; // Enumeration<CompositionStatus> 2484 return value; 2485 case -622722335: // modality 2486 this.getModality().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2487 return value; 2488 case 3575610: // type 2489 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2490 return value; 2491 case 50511102: // category 2492 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2493 return value; 2494 case -1867885268: // subject 2495 this.subject = TypeConvertor.castToReference(value); // Reference 2496 return value; 2497 case 951530927: // context 2498 this.getContext().add(TypeConvertor.castToReference(value)); // Reference 2499 return value; 2500 case 96891546: // event 2501 this.getEvent().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2502 return value; 2503 case 1702620169: // bodySite 2504 this.getBodySite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2505 return value; 2506 case 370698365: // facilityType 2507 this.facilityType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2508 return value; 2509 case 331373717: // practiceSetting 2510 this.practiceSetting = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2511 return value; 2512 case -991726143: // period 2513 this.period = TypeConvertor.castToPeriod(value); // Period 2514 return value; 2515 case 3076014: // date 2516 this.date = TypeConvertor.castToInstant(value); // InstantType 2517 return value; 2518 case -1406328437: // author 2519 this.getAuthor().add(TypeConvertor.castToReference(value)); // Reference 2520 return value; 2521 case 542920370: // attester 2522 this.getAttester().add((DocumentReferenceAttesterComponent) value); // DocumentReferenceAttesterComponent 2523 return value; 2524 case 1611297262: // custodian 2525 this.custodian = TypeConvertor.castToReference(value); // Reference 2526 return value; 2527 case -7765931: // relatesTo 2528 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); // DocumentReferenceRelatesToComponent 2529 return value; 2530 case -1724546052: // description 2531 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2532 return value; 2533 case -722296940: // securityLabel 2534 this.getSecurityLabel().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2535 return value; 2536 case 951530617: // content 2537 this.getContent().add((DocumentReferenceContentComponent) value); // DocumentReferenceContentComponent 2538 return value; 2539 default: return super.setProperty(hash, name, value); 2540 } 2541 2542 } 2543 2544 @Override 2545 public Base setProperty(String name, Base value) throws FHIRException { 2546 if (name.equals("identifier")) { 2547 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2548 } else if (name.equals("version")) { 2549 this.version = TypeConvertor.castToString(value); // StringType 2550 } else if (name.equals("basedOn")) { 2551 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2552 } else if (name.equals("status")) { 2553 value = new DocumentReferenceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2554 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2555 } else if (name.equals("docStatus")) { 2556 value = new CompositionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2557 this.docStatus = (Enumeration) value; // Enumeration<CompositionStatus> 2558 } else if (name.equals("modality")) { 2559 this.getModality().add(TypeConvertor.castToCodeableConcept(value)); 2560 } else if (name.equals("type")) { 2561 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2562 } else if (name.equals("category")) { 2563 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2564 } else if (name.equals("subject")) { 2565 this.subject = TypeConvertor.castToReference(value); // Reference 2566 } else if (name.equals("context")) { 2567 this.getContext().add(TypeConvertor.castToReference(value)); 2568 } else if (name.equals("event")) { 2569 this.getEvent().add(TypeConvertor.castToCodeableReference(value)); 2570 } else if (name.equals("bodySite")) { 2571 this.getBodySite().add(TypeConvertor.castToCodeableReference(value)); 2572 } else if (name.equals("facilityType")) { 2573 this.facilityType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2574 } else if (name.equals("practiceSetting")) { 2575 this.practiceSetting = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2576 } else if (name.equals("period")) { 2577 this.period = TypeConvertor.castToPeriod(value); // Period 2578 } else if (name.equals("date")) { 2579 this.date = TypeConvertor.castToInstant(value); // InstantType 2580 } else if (name.equals("author")) { 2581 this.getAuthor().add(TypeConvertor.castToReference(value)); 2582 } else if (name.equals("attester")) { 2583 this.getAttester().add((DocumentReferenceAttesterComponent) value); 2584 } else if (name.equals("custodian")) { 2585 this.custodian = TypeConvertor.castToReference(value); // Reference 2586 } else if (name.equals("relatesTo")) { 2587 this.getRelatesTo().add((DocumentReferenceRelatesToComponent) value); 2588 } else if (name.equals("description")) { 2589 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2590 } else if (name.equals("securityLabel")) { 2591 this.getSecurityLabel().add(TypeConvertor.castToCodeableConcept(value)); 2592 } else if (name.equals("content")) { 2593 this.getContent().add((DocumentReferenceContentComponent) value); 2594 } else 2595 return super.setProperty(name, value); 2596 return value; 2597 } 2598 2599 @Override 2600 public void removeChild(String name, Base value) throws FHIRException { 2601 if (name.equals("identifier")) { 2602 this.getIdentifier().remove(value); 2603 } else if (name.equals("version")) { 2604 this.version = null; 2605 } else if (name.equals("basedOn")) { 2606 this.getBasedOn().remove(value); 2607 } else if (name.equals("status")) { 2608 value = new DocumentReferenceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2609 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 2610 } else if (name.equals("docStatus")) { 2611 value = new CompositionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2612 this.docStatus = (Enumeration) value; // Enumeration<CompositionStatus> 2613 } else if (name.equals("modality")) { 2614 this.getModality().remove(value); 2615 } else if (name.equals("type")) { 2616 this.type = null; 2617 } else if (name.equals("category")) { 2618 this.getCategory().remove(value); 2619 } else if (name.equals("subject")) { 2620 this.subject = null; 2621 } else if (name.equals("context")) { 2622 this.getContext().remove(value); 2623 } else if (name.equals("event")) { 2624 this.getEvent().remove(value); 2625 } else if (name.equals("bodySite")) { 2626 this.getBodySite().remove(value); 2627 } else if (name.equals("facilityType")) { 2628 this.facilityType = null; 2629 } else if (name.equals("practiceSetting")) { 2630 this.practiceSetting = null; 2631 } else if (name.equals("period")) { 2632 this.period = null; 2633 } else if (name.equals("date")) { 2634 this.date = null; 2635 } else if (name.equals("author")) { 2636 this.getAuthor().remove(value); 2637 } else if (name.equals("attester")) { 2638 this.getAttester().remove((DocumentReferenceAttesterComponent) value); 2639 } else if (name.equals("custodian")) { 2640 this.custodian = null; 2641 } else if (name.equals("relatesTo")) { 2642 this.getRelatesTo().remove((DocumentReferenceRelatesToComponent) value); 2643 } else if (name.equals("description")) { 2644 this.description = null; 2645 } else if (name.equals("securityLabel")) { 2646 this.getSecurityLabel().remove(value); 2647 } else if (name.equals("content")) { 2648 this.getContent().remove((DocumentReferenceContentComponent) value); 2649 } else 2650 super.removeChild(name, value); 2651 2652 } 2653 2654 @Override 2655 public Base makeProperty(int hash, String name) throws FHIRException { 2656 switch (hash) { 2657 case -1618432855: return addIdentifier(); 2658 case 351608024: return getVersionElement(); 2659 case -332612366: return addBasedOn(); 2660 case -892481550: return getStatusElement(); 2661 case -23496886: return getDocStatusElement(); 2662 case -622722335: return addModality(); 2663 case 3575610: return getType(); 2664 case 50511102: return addCategory(); 2665 case -1867885268: return getSubject(); 2666 case 951530927: return addContext(); 2667 case 96891546: return addEvent(); 2668 case 1702620169: return addBodySite(); 2669 case 370698365: return getFacilityType(); 2670 case 331373717: return getPracticeSetting(); 2671 case -991726143: return getPeriod(); 2672 case 3076014: return getDateElement(); 2673 case -1406328437: return addAuthor(); 2674 case 542920370: return addAttester(); 2675 case 1611297262: return getCustodian(); 2676 case -7765931: return addRelatesTo(); 2677 case -1724546052: return getDescriptionElement(); 2678 case -722296940: return addSecurityLabel(); 2679 case 951530617: return addContent(); 2680 default: return super.makeProperty(hash, name); 2681 } 2682 2683 } 2684 2685 @Override 2686 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2687 switch (hash) { 2688 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2689 case 351608024: /*version*/ return new String[] {"string"}; 2690 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2691 case -892481550: /*status*/ return new String[] {"code"}; 2692 case -23496886: /*docStatus*/ return new String[] {"code"}; 2693 case -622722335: /*modality*/ return new String[] {"CodeableConcept"}; 2694 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2695 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2696 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2697 case 951530927: /*context*/ return new String[] {"Reference"}; 2698 case 96891546: /*event*/ return new String[] {"CodeableReference"}; 2699 case 1702620169: /*bodySite*/ return new String[] {"CodeableReference"}; 2700 case 370698365: /*facilityType*/ return new String[] {"CodeableConcept"}; 2701 case 331373717: /*practiceSetting*/ return new String[] {"CodeableConcept"}; 2702 case -991726143: /*period*/ return new String[] {"Period"}; 2703 case 3076014: /*date*/ return new String[] {"instant"}; 2704 case -1406328437: /*author*/ return new String[] {"Reference"}; 2705 case 542920370: /*attester*/ return new String[] {}; 2706 case 1611297262: /*custodian*/ return new String[] {"Reference"}; 2707 case -7765931: /*relatesTo*/ return new String[] {}; 2708 case -1724546052: /*description*/ return new String[] {"markdown"}; 2709 case -722296940: /*securityLabel*/ return new String[] {"CodeableConcept"}; 2710 case 951530617: /*content*/ return new String[] {}; 2711 default: return super.getTypesForProperty(hash, name); 2712 } 2713 2714 } 2715 2716 @Override 2717 public Base addChild(String name) throws FHIRException { 2718 if (name.equals("identifier")) { 2719 return addIdentifier(); 2720 } 2721 else if (name.equals("version")) { 2722 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.version"); 2723 } 2724 else if (name.equals("basedOn")) { 2725 return addBasedOn(); 2726 } 2727 else if (name.equals("status")) { 2728 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.status"); 2729 } 2730 else if (name.equals("docStatus")) { 2731 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.docStatus"); 2732 } 2733 else if (name.equals("modality")) { 2734 return addModality(); 2735 } 2736 else if (name.equals("type")) { 2737 this.type = new CodeableConcept(); 2738 return this.type; 2739 } 2740 else if (name.equals("category")) { 2741 return addCategory(); 2742 } 2743 else if (name.equals("subject")) { 2744 this.subject = new Reference(); 2745 return this.subject; 2746 } 2747 else if (name.equals("context")) { 2748 return addContext(); 2749 } 2750 else if (name.equals("event")) { 2751 return addEvent(); 2752 } 2753 else if (name.equals("bodySite")) { 2754 return addBodySite(); 2755 } 2756 else if (name.equals("facilityType")) { 2757 this.facilityType = new CodeableConcept(); 2758 return this.facilityType; 2759 } 2760 else if (name.equals("practiceSetting")) { 2761 this.practiceSetting = new CodeableConcept(); 2762 return this.practiceSetting; 2763 } 2764 else if (name.equals("period")) { 2765 this.period = new Period(); 2766 return this.period; 2767 } 2768 else if (name.equals("date")) { 2769 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.date"); 2770 } 2771 else if (name.equals("author")) { 2772 return addAuthor(); 2773 } 2774 else if (name.equals("attester")) { 2775 return addAttester(); 2776 } 2777 else if (name.equals("custodian")) { 2778 this.custodian = new Reference(); 2779 return this.custodian; 2780 } 2781 else if (name.equals("relatesTo")) { 2782 return addRelatesTo(); 2783 } 2784 else if (name.equals("description")) { 2785 throw new FHIRException("Cannot call addChild on a singleton property DocumentReference.description"); 2786 } 2787 else if (name.equals("securityLabel")) { 2788 return addSecurityLabel(); 2789 } 2790 else if (name.equals("content")) { 2791 return addContent(); 2792 } 2793 else 2794 return super.addChild(name); 2795 } 2796 2797 public String fhirType() { 2798 return "DocumentReference"; 2799 2800 } 2801 2802 public DocumentReference copy() { 2803 DocumentReference dst = new DocumentReference(); 2804 copyValues(dst); 2805 return dst; 2806 } 2807 2808 public void copyValues(DocumentReference dst) { 2809 super.copyValues(dst); 2810 if (identifier != null) { 2811 dst.identifier = new ArrayList<Identifier>(); 2812 for (Identifier i : identifier) 2813 dst.identifier.add(i.copy()); 2814 }; 2815 dst.version = version == null ? null : version.copy(); 2816 if (basedOn != null) { 2817 dst.basedOn = new ArrayList<Reference>(); 2818 for (Reference i : basedOn) 2819 dst.basedOn.add(i.copy()); 2820 }; 2821 dst.status = status == null ? null : status.copy(); 2822 dst.docStatus = docStatus == null ? null : docStatus.copy(); 2823 if (modality != null) { 2824 dst.modality = new ArrayList<CodeableConcept>(); 2825 for (CodeableConcept i : modality) 2826 dst.modality.add(i.copy()); 2827 }; 2828 dst.type = type == null ? null : type.copy(); 2829 if (category != null) { 2830 dst.category = new ArrayList<CodeableConcept>(); 2831 for (CodeableConcept i : category) 2832 dst.category.add(i.copy()); 2833 }; 2834 dst.subject = subject == null ? null : subject.copy(); 2835 if (context != null) { 2836 dst.context = new ArrayList<Reference>(); 2837 for (Reference i : context) 2838 dst.context.add(i.copy()); 2839 }; 2840 if (event != null) { 2841 dst.event = new ArrayList<CodeableReference>(); 2842 for (CodeableReference i : event) 2843 dst.event.add(i.copy()); 2844 }; 2845 if (bodySite != null) { 2846 dst.bodySite = new ArrayList<CodeableReference>(); 2847 for (CodeableReference i : bodySite) 2848 dst.bodySite.add(i.copy()); 2849 }; 2850 dst.facilityType = facilityType == null ? null : facilityType.copy(); 2851 dst.practiceSetting = practiceSetting == null ? null : practiceSetting.copy(); 2852 dst.period = period == null ? null : period.copy(); 2853 dst.date = date == null ? null : date.copy(); 2854 if (author != null) { 2855 dst.author = new ArrayList<Reference>(); 2856 for (Reference i : author) 2857 dst.author.add(i.copy()); 2858 }; 2859 if (attester != null) { 2860 dst.attester = new ArrayList<DocumentReferenceAttesterComponent>(); 2861 for (DocumentReferenceAttesterComponent i : attester) 2862 dst.attester.add(i.copy()); 2863 }; 2864 dst.custodian = custodian == null ? null : custodian.copy(); 2865 if (relatesTo != null) { 2866 dst.relatesTo = new ArrayList<DocumentReferenceRelatesToComponent>(); 2867 for (DocumentReferenceRelatesToComponent i : relatesTo) 2868 dst.relatesTo.add(i.copy()); 2869 }; 2870 dst.description = description == null ? null : description.copy(); 2871 if (securityLabel != null) { 2872 dst.securityLabel = new ArrayList<CodeableConcept>(); 2873 for (CodeableConcept i : securityLabel) 2874 dst.securityLabel.add(i.copy()); 2875 }; 2876 if (content != null) { 2877 dst.content = new ArrayList<DocumentReferenceContentComponent>(); 2878 for (DocumentReferenceContentComponent i : content) 2879 dst.content.add(i.copy()); 2880 }; 2881 } 2882 2883 protected DocumentReference typedCopy() { 2884 return copy(); 2885 } 2886 2887 @Override 2888 public boolean equalsDeep(Base other_) { 2889 if (!super.equalsDeep(other_)) 2890 return false; 2891 if (!(other_ instanceof DocumentReference)) 2892 return false; 2893 DocumentReference o = (DocumentReference) other_; 2894 return compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) && compareDeep(basedOn, o.basedOn, true) 2895 && compareDeep(status, o.status, true) && compareDeep(docStatus, o.docStatus, true) && compareDeep(modality, o.modality, true) 2896 && compareDeep(type, o.type, true) && compareDeep(category, o.category, true) && compareDeep(subject, o.subject, true) 2897 && compareDeep(context, o.context, true) && compareDeep(event, o.event, true) && compareDeep(bodySite, o.bodySite, true) 2898 && compareDeep(facilityType, o.facilityType, true) && compareDeep(practiceSetting, o.practiceSetting, true) 2899 && compareDeep(period, o.period, true) && compareDeep(date, o.date, true) && compareDeep(author, o.author, true) 2900 && compareDeep(attester, o.attester, true) && compareDeep(custodian, o.custodian, true) && compareDeep(relatesTo, o.relatesTo, true) 2901 && compareDeep(description, o.description, true) && compareDeep(securityLabel, o.securityLabel, true) 2902 && compareDeep(content, o.content, true); 2903 } 2904 2905 @Override 2906 public boolean equalsShallow(Base other_) { 2907 if (!super.equalsShallow(other_)) 2908 return false; 2909 if (!(other_ instanceof DocumentReference)) 2910 return false; 2911 DocumentReference o = (DocumentReference) other_; 2912 return compareValues(version, o.version, true) && compareValues(status, o.status, true) && compareValues(docStatus, o.docStatus, true) 2913 && compareValues(date, o.date, true) && compareValues(description, o.description, true); 2914 } 2915 2916 public boolean isEmpty() { 2917 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, version, basedOn 2918 , status, docStatus, modality, type, category, subject, context, event, bodySite 2919 , facilityType, practiceSetting, period, date, author, attester, custodian, relatesTo 2920 , description, securityLabel, content); 2921 } 2922 2923 @Override 2924 public ResourceType getResourceType() { 2925 return ResourceType.DocumentReference; 2926 } 2927 2928 /** 2929 * Search parameter: <b>attester</b> 2930 * <p> 2931 * Description: <b>Who attested the document</b><br> 2932 * Type: <b>reference</b><br> 2933 * Path: <b>DocumentReference.attester.party</b><br> 2934 * </p> 2935 */ 2936 @SearchParamDefinition(name="attester", path="DocumentReference.attester.party", description="Who attested the document", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2937 public static final String SP_ATTESTER = "attester"; 2938 /** 2939 * <b>Fluent Client</b> search parameter constant for <b>attester</b> 2940 * <p> 2941 * Description: <b>Who attested the document</b><br> 2942 * Type: <b>reference</b><br> 2943 * Path: <b>DocumentReference.attester.party</b><br> 2944 * </p> 2945 */ 2946 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ATTESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ATTESTER); 2947 2948/** 2949 * Constant for fluent queries to be used to add include statements. Specifies 2950 * the path value of "<b>DocumentReference:attester</b>". 2951 */ 2952 public static final ca.uhn.fhir.model.api.Include INCLUDE_ATTESTER = new ca.uhn.fhir.model.api.Include("DocumentReference:attester").toLocked(); 2953 2954 /** 2955 * Search parameter: <b>author</b> 2956 * <p> 2957 * Description: <b>Who and/or what authored the document</b><br> 2958 * Type: <b>reference</b><br> 2959 * Path: <b>DocumentReference.author</b><br> 2960 * </p> 2961 */ 2962 @SearchParamDefinition(name="author", path="DocumentReference.author", description="Who and/or what authored the document", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2963 public static final String SP_AUTHOR = "author"; 2964 /** 2965 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2966 * <p> 2967 * Description: <b>Who and/or what authored the document</b><br> 2968 * Type: <b>reference</b><br> 2969 * Path: <b>DocumentReference.author</b><br> 2970 * </p> 2971 */ 2972 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 2973 2974/** 2975 * Constant for fluent queries to be used to add include statements. Specifies 2976 * the path value of "<b>DocumentReference:author</b>". 2977 */ 2978 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("DocumentReference:author").toLocked(); 2979 2980 /** 2981 * Search parameter: <b>based-on</b> 2982 * <p> 2983 * Description: <b>Procedure that caused this media to be created</b><br> 2984 * Type: <b>reference</b><br> 2985 * Path: <b>DocumentReference.basedOn</b><br> 2986 * </p> 2987 */ 2988 @SearchParamDefinition(name="based-on", path="DocumentReference.basedOn", description="Procedure that caused this media to be created", type="reference", target={Appointment.class, AppointmentResponse.class, CarePlan.class, Claim.class, CommunicationRequest.class, Contract.class, CoverageEligibilityRequest.class, DeviceRequest.class, EnrollmentRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, RequestOrchestration.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class } ) 2989 public static final String SP_BASED_ON = "based-on"; 2990 /** 2991 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2992 * <p> 2993 * Description: <b>Procedure that caused this media to be created</b><br> 2994 * Type: <b>reference</b><br> 2995 * Path: <b>DocumentReference.basedOn</b><br> 2996 * </p> 2997 */ 2998 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 2999 3000/** 3001 * Constant for fluent queries to be used to add include statements. Specifies 3002 * the path value of "<b>DocumentReference:based-on</b>". 3003 */ 3004 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("DocumentReference:based-on").toLocked(); 3005 3006 /** 3007 * Search parameter: <b>bodysite-reference</b> 3008 * <p> 3009 * Description: <b>The body site studied</b><br> 3010 * Type: <b>reference</b><br> 3011 * Path: <b>DocumentReference.bodySite.reference</b><br> 3012 * </p> 3013 */ 3014 @SearchParamDefinition(name="bodysite-reference", path="DocumentReference.bodySite.reference", description="The body site studied", type="reference", target={BodyStructure.class } ) 3015 public static final String SP_BODYSITE_REFERENCE = "bodysite-reference"; 3016 /** 3017 * <b>Fluent Client</b> search parameter constant for <b>bodysite-reference</b> 3018 * <p> 3019 * Description: <b>The body site studied</b><br> 3020 * Type: <b>reference</b><br> 3021 * Path: <b>DocumentReference.bodySite.reference</b><br> 3022 * </p> 3023 */ 3024 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BODYSITE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BODYSITE_REFERENCE); 3025 3026/** 3027 * Constant for fluent queries to be used to add include statements. Specifies 3028 * the path value of "<b>DocumentReference:bodysite-reference</b>". 3029 */ 3030 public static final ca.uhn.fhir.model.api.Include INCLUDE_BODYSITE_REFERENCE = new ca.uhn.fhir.model.api.Include("DocumentReference:bodysite-reference").toLocked(); 3031 3032 /** 3033 * Search parameter: <b>bodysite</b> 3034 * <p> 3035 * Description: <b>The body site studied</b><br> 3036 * Type: <b>token</b><br> 3037 * Path: <b>DocumentReference.bodySite.concept</b><br> 3038 * </p> 3039 */ 3040 @SearchParamDefinition(name="bodysite", path="DocumentReference.bodySite.concept", description="The body site studied", type="token" ) 3041 public static final String SP_BODYSITE = "bodysite"; 3042 /** 3043 * <b>Fluent Client</b> search parameter constant for <b>bodysite</b> 3044 * <p> 3045 * Description: <b>The body site studied</b><br> 3046 * Type: <b>token</b><br> 3047 * Path: <b>DocumentReference.bodySite.concept</b><br> 3048 * </p> 3049 */ 3050 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODYSITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODYSITE); 3051 3052 /** 3053 * Search parameter: <b>category</b> 3054 * <p> 3055 * Description: <b>Categorization of document</b><br> 3056 * Type: <b>token</b><br> 3057 * Path: <b>DocumentReference.category</b><br> 3058 * </p> 3059 */ 3060 @SearchParamDefinition(name="category", path="DocumentReference.category", description="Categorization of document", type="token" ) 3061 public static final String SP_CATEGORY = "category"; 3062 /** 3063 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3064 * <p> 3065 * Description: <b>Categorization of document</b><br> 3066 * Type: <b>token</b><br> 3067 * Path: <b>DocumentReference.category</b><br> 3068 * </p> 3069 */ 3070 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3071 3072 /** 3073 * Search parameter: <b>contenttype</b> 3074 * <p> 3075 * Description: <b>Mime type of the content, with charset etc.</b><br> 3076 * Type: <b>token</b><br> 3077 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3078 * </p> 3079 */ 3080 @SearchParamDefinition(name="contenttype", path="DocumentReference.content.attachment.contentType", description="Mime type of the content, with charset etc.", type="token" ) 3081 public static final String SP_CONTENTTYPE = "contenttype"; 3082 /** 3083 * <b>Fluent Client</b> search parameter constant for <b>contenttype</b> 3084 * <p> 3085 * Description: <b>Mime type of the content, with charset etc.</b><br> 3086 * Type: <b>token</b><br> 3087 * Path: <b>DocumentReference.content.attachment.contentType</b><br> 3088 * </p> 3089 */ 3090 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENTTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTENTTYPE); 3091 3092 /** 3093 * Search parameter: <b>context</b> 3094 * <p> 3095 * Description: <b>Context of the document content</b><br> 3096 * Type: <b>reference</b><br> 3097 * Path: <b>DocumentReference.context</b><br> 3098 * </p> 3099 */ 3100 @SearchParamDefinition(name="context", path="DocumentReference.context", description="Context of the document content", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Appointment.class, Encounter.class, EpisodeOfCare.class } ) 3101 public static final String SP_CONTEXT = "context"; 3102 /** 3103 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3104 * <p> 3105 * Description: <b>Context of the document content</b><br> 3106 * Type: <b>reference</b><br> 3107 * Path: <b>DocumentReference.context</b><br> 3108 * </p> 3109 */ 3110 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3111 3112/** 3113 * Constant for fluent queries to be used to add include statements. Specifies 3114 * the path value of "<b>DocumentReference:context</b>". 3115 */ 3116 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("DocumentReference:context").toLocked(); 3117 3118 /** 3119 * Search parameter: <b>creation</b> 3120 * <p> 3121 * Description: <b>Date attachment was first created</b><br> 3122 * Type: <b>date</b><br> 3123 * Path: <b>DocumentReference.content.attachment.creation</b><br> 3124 * </p> 3125 */ 3126 @SearchParamDefinition(name="creation", path="DocumentReference.content.attachment.creation", description="Date attachment was first created", type="date" ) 3127 public static final String SP_CREATION = "creation"; 3128 /** 3129 * <b>Fluent Client</b> search parameter constant for <b>creation</b> 3130 * <p> 3131 * Description: <b>Date attachment was first created</b><br> 3132 * Type: <b>date</b><br> 3133 * Path: <b>DocumentReference.content.attachment.creation</b><br> 3134 * </p> 3135 */ 3136 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATION = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATION); 3137 3138 /** 3139 * Search parameter: <b>custodian</b> 3140 * <p> 3141 * Description: <b>Organization which maintains the document</b><br> 3142 * Type: <b>reference</b><br> 3143 * Path: <b>DocumentReference.custodian</b><br> 3144 * </p> 3145 */ 3146 @SearchParamDefinition(name="custodian", path="DocumentReference.custodian", description="Organization which maintains the document", type="reference", target={Organization.class } ) 3147 public static final String SP_CUSTODIAN = "custodian"; 3148 /** 3149 * <b>Fluent Client</b> search parameter constant for <b>custodian</b> 3150 * <p> 3151 * Description: <b>Organization which maintains the document</b><br> 3152 * Type: <b>reference</b><br> 3153 * Path: <b>DocumentReference.custodian</b><br> 3154 * </p> 3155 */ 3156 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CUSTODIAN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CUSTODIAN); 3157 3158/** 3159 * Constant for fluent queries to be used to add include statements. Specifies 3160 * the path value of "<b>DocumentReference:custodian</b>". 3161 */ 3162 public static final ca.uhn.fhir.model.api.Include INCLUDE_CUSTODIAN = new ca.uhn.fhir.model.api.Include("DocumentReference:custodian").toLocked(); 3163 3164 /** 3165 * Search parameter: <b>description</b> 3166 * <p> 3167 * Description: <b>Human-readable description</b><br> 3168 * Type: <b>string</b><br> 3169 * Path: <b>DocumentReference.description</b><br> 3170 * </p> 3171 */ 3172 @SearchParamDefinition(name="description", path="DocumentReference.description", description="Human-readable description", type="string" ) 3173 public static final String SP_DESCRIPTION = "description"; 3174 /** 3175 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3176 * <p> 3177 * Description: <b>Human-readable description</b><br> 3178 * Type: <b>string</b><br> 3179 * Path: <b>DocumentReference.description</b><br> 3180 * </p> 3181 */ 3182 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3183 3184 /** 3185 * Search parameter: <b>doc-status</b> 3186 * <p> 3187 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 3188 * Type: <b>token</b><br> 3189 * Path: <b>DocumentReference.docStatus</b><br> 3190 * </p> 3191 */ 3192 @SearchParamDefinition(name="doc-status", path="DocumentReference.docStatus", description="preliminary | final | amended | entered-in-error", type="token" ) 3193 public static final String SP_DOC_STATUS = "doc-status"; 3194 /** 3195 * <b>Fluent Client</b> search parameter constant for <b>doc-status</b> 3196 * <p> 3197 * Description: <b>preliminary | final | amended | entered-in-error</b><br> 3198 * Type: <b>token</b><br> 3199 * Path: <b>DocumentReference.docStatus</b><br> 3200 * </p> 3201 */ 3202 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOC_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOC_STATUS); 3203 3204 /** 3205 * Search parameter: <b>event-code</b> 3206 * <p> 3207 * Description: <b>Main clinical acts documented</b><br> 3208 * Type: <b>token</b><br> 3209 * Path: <b>DocumentReference.event.concept</b><br> 3210 * </p> 3211 */ 3212 @SearchParamDefinition(name="event-code", path="DocumentReference.event.concept", description="Main clinical acts documented", type="token" ) 3213 public static final String SP_EVENT_CODE = "event-code"; 3214 /** 3215 * <b>Fluent Client</b> search parameter constant for <b>event-code</b> 3216 * <p> 3217 * Description: <b>Main clinical acts documented</b><br> 3218 * Type: <b>token</b><br> 3219 * Path: <b>DocumentReference.event.concept</b><br> 3220 * </p> 3221 */ 3222 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT_CODE); 3223 3224 /** 3225 * Search parameter: <b>event-reference</b> 3226 * <p> 3227 * Description: <b>Main clinical acts documented</b><br> 3228 * Type: <b>reference</b><br> 3229 * Path: <b>DocumentReference.event.reference</b><br> 3230 * </p> 3231 */ 3232 @SearchParamDefinition(name="event-reference", path="DocumentReference.event.reference", description="Main clinical acts documented", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3233 public static final String SP_EVENT_REFERENCE = "event-reference"; 3234 /** 3235 * <b>Fluent Client</b> search parameter constant for <b>event-reference</b> 3236 * <p> 3237 * Description: <b>Main clinical acts documented</b><br> 3238 * Type: <b>reference</b><br> 3239 * Path: <b>DocumentReference.event.reference</b><br> 3240 * </p> 3241 */ 3242 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EVENT_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EVENT_REFERENCE); 3243 3244/** 3245 * Constant for fluent queries to be used to add include statements. Specifies 3246 * the path value of "<b>DocumentReference:event-reference</b>". 3247 */ 3248 public static final ca.uhn.fhir.model.api.Include INCLUDE_EVENT_REFERENCE = new ca.uhn.fhir.model.api.Include("DocumentReference:event-reference").toLocked(); 3249 3250 /** 3251 * Search parameter: <b>facility</b> 3252 * <p> 3253 * Description: <b>Kind of facility where patient was seen</b><br> 3254 * Type: <b>token</b><br> 3255 * Path: <b>DocumentReference.facilityType</b><br> 3256 * </p> 3257 */ 3258 @SearchParamDefinition(name="facility", path="DocumentReference.facilityType", description="Kind of facility where patient was seen", type="token" ) 3259 public static final String SP_FACILITY = "facility"; 3260 /** 3261 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 3262 * <p> 3263 * Description: <b>Kind of facility where patient was seen</b><br> 3264 * Type: <b>token</b><br> 3265 * Path: <b>DocumentReference.facilityType</b><br> 3266 * </p> 3267 */ 3268 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FACILITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FACILITY); 3269 3270 /** 3271 * Search parameter: <b>format-canonical</b> 3272 * <p> 3273 * Description: <b>Profile canonical content rules for the document</b><br> 3274 * Type: <b>reference</b><br> 3275 * Path: <b>(DocumentReference.content.profile.value.ofType(canonical))</b><br> 3276 * </p> 3277 */ 3278 @SearchParamDefinition(name="format-canonical", path="(DocumentReference.content.profile.value.ofType(canonical))", description="Profile canonical content rules for the document", type="reference", target={ActivityDefinition.class, ActorDefinition.class, CapabilityStatement.class, ChargeItemDefinition.class, Citation.class, CodeSystem.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, ConditionDefinition.class, Contract.class, Device.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, GraphDefinition.class, ImplementationGuide.class, Library.class, Measure.class, MessageDefinition.class, NamingSystem.class, ObservationDefinition.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class, Requirements.class, ResearchStudy.class, SearchParameter.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, SubscriptionTopic.class, TerminologyCapabilities.class, TestPlan.class, TestScript.class, ValueSet.class } ) 3279 public static final String SP_FORMAT_CANONICAL = "format-canonical"; 3280 /** 3281 * <b>Fluent Client</b> search parameter constant for <b>format-canonical</b> 3282 * <p> 3283 * Description: <b>Profile canonical content rules for the document</b><br> 3284 * Type: <b>reference</b><br> 3285 * Path: <b>(DocumentReference.content.profile.value.ofType(canonical))</b><br> 3286 * </p> 3287 */ 3288 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FORMAT_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FORMAT_CANONICAL); 3289 3290/** 3291 * Constant for fluent queries to be used to add include statements. Specifies 3292 * the path value of "<b>DocumentReference:format-canonical</b>". 3293 */ 3294 public static final ca.uhn.fhir.model.api.Include INCLUDE_FORMAT_CANONICAL = new ca.uhn.fhir.model.api.Include("DocumentReference:format-canonical").toLocked(); 3295 3296 /** 3297 * Search parameter: <b>format-code</b> 3298 * <p> 3299 * Description: <b>Format code content rules for the document</b><br> 3300 * Type: <b>token</b><br> 3301 * Path: <b>(DocumentReference.content.profile.value.ofType(Coding))</b><br> 3302 * </p> 3303 */ 3304 @SearchParamDefinition(name="format-code", path="(DocumentReference.content.profile.value.ofType(Coding))", description="Format code content rules for the document", type="token" ) 3305 public static final String SP_FORMAT_CODE = "format-code"; 3306 /** 3307 * <b>Fluent Client</b> search parameter constant for <b>format-code</b> 3308 * <p> 3309 * Description: <b>Format code content rules for the document</b><br> 3310 * Type: <b>token</b><br> 3311 * Path: <b>(DocumentReference.content.profile.value.ofType(Coding))</b><br> 3312 * </p> 3313 */ 3314 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMAT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORMAT_CODE); 3315 3316 /** 3317 * Search parameter: <b>format-uri</b> 3318 * <p> 3319 * Description: <b>Profile URI content rules for the document</b><br> 3320 * Type: <b>uri</b><br> 3321 * Path: <b>(DocumentReference.content.profile.value.ofType(uri))</b><br> 3322 * </p> 3323 */ 3324 @SearchParamDefinition(name="format-uri", path="(DocumentReference.content.profile.value.ofType(uri))", description="Profile URI content rules for the document", type="uri" ) 3325 public static final String SP_FORMAT_URI = "format-uri"; 3326 /** 3327 * <b>Fluent Client</b> search parameter constant for <b>format-uri</b> 3328 * <p> 3329 * Description: <b>Profile URI content rules for the document</b><br> 3330 * Type: <b>uri</b><br> 3331 * Path: <b>(DocumentReference.content.profile.value.ofType(uri))</b><br> 3332 * </p> 3333 */ 3334 public static final ca.uhn.fhir.rest.gclient.UriClientParam FORMAT_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_FORMAT_URI); 3335 3336 /** 3337 * Search parameter: <b>language</b> 3338 * <p> 3339 * Description: <b>Human language of the content (BCP-47)</b><br> 3340 * Type: <b>token</b><br> 3341 * Path: <b>DocumentReference.content.attachment.language</b><br> 3342 * </p> 3343 */ 3344 @SearchParamDefinition(name="language", path="DocumentReference.content.attachment.language", description="Human language of the content (BCP-47)", type="token" ) 3345 public static final String SP_LANGUAGE = "language"; 3346 /** 3347 * <b>Fluent Client</b> search parameter constant for <b>language</b> 3348 * <p> 3349 * Description: <b>Human language of the content (BCP-47)</b><br> 3350 * Type: <b>token</b><br> 3351 * Path: <b>DocumentReference.content.attachment.language</b><br> 3352 * </p> 3353 */ 3354 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LANGUAGE); 3355 3356 /** 3357 * Search parameter: <b>location</b> 3358 * <p> 3359 * Description: <b>Uri where the data can be found</b><br> 3360 * Type: <b>uri</b><br> 3361 * Path: <b>DocumentReference.content.attachment.url</b><br> 3362 * </p> 3363 */ 3364 @SearchParamDefinition(name="location", path="DocumentReference.content.attachment.url", description="Uri where the data can be found", type="uri" ) 3365 public static final String SP_LOCATION = "location"; 3366 /** 3367 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3368 * <p> 3369 * Description: <b>Uri where the data can be found</b><br> 3370 * Type: <b>uri</b><br> 3371 * Path: <b>DocumentReference.content.attachment.url</b><br> 3372 * </p> 3373 */ 3374 public static final ca.uhn.fhir.rest.gclient.UriClientParam LOCATION = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_LOCATION); 3375 3376 /** 3377 * Search parameter: <b>modality</b> 3378 * <p> 3379 * Description: <b>The modality used</b><br> 3380 * Type: <b>token</b><br> 3381 * Path: <b>DocumentReference.modality</b><br> 3382 * </p> 3383 */ 3384 @SearchParamDefinition(name="modality", path="DocumentReference.modality", description="The modality used", type="token" ) 3385 public static final String SP_MODALITY = "modality"; 3386 /** 3387 * <b>Fluent Client</b> search parameter constant for <b>modality</b> 3388 * <p> 3389 * Description: <b>The modality used</b><br> 3390 * Type: <b>token</b><br> 3391 * Path: <b>DocumentReference.modality</b><br> 3392 * </p> 3393 */ 3394 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MODALITY); 3395 3396 /** 3397 * Search parameter: <b>period</b> 3398 * <p> 3399 * Description: <b>Time of service that is being documented</b><br> 3400 * Type: <b>date</b><br> 3401 * Path: <b>DocumentReference.period</b><br> 3402 * </p> 3403 */ 3404 @SearchParamDefinition(name="period", path="DocumentReference.period", description="Time of service that is being documented", type="date" ) 3405 public static final String SP_PERIOD = "period"; 3406 /** 3407 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3408 * <p> 3409 * Description: <b>Time of service that is being documented</b><br> 3410 * Type: <b>date</b><br> 3411 * Path: <b>DocumentReference.period</b><br> 3412 * </p> 3413 */ 3414 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 3415 3416 /** 3417 * Search parameter: <b>relatesto</b> 3418 * <p> 3419 * Description: <b>Target of the relationship</b><br> 3420 * Type: <b>reference</b><br> 3421 * Path: <b>DocumentReference.relatesTo.target</b><br> 3422 * </p> 3423 */ 3424 @SearchParamDefinition(name="relatesto", path="DocumentReference.relatesTo.target", description="Target of the relationship", type="reference", target={DocumentReference.class } ) 3425 public static final String SP_RELATESTO = "relatesto"; 3426 /** 3427 * <b>Fluent Client</b> search parameter constant for <b>relatesto</b> 3428 * <p> 3429 * Description: <b>Target of the relationship</b><br> 3430 * Type: <b>reference</b><br> 3431 * Path: <b>DocumentReference.relatesTo.target</b><br> 3432 * </p> 3433 */ 3434 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATESTO = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RELATESTO); 3435 3436/** 3437 * Constant for fluent queries to be used to add include statements. Specifies 3438 * the path value of "<b>DocumentReference:relatesto</b>". 3439 */ 3440 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATESTO = new ca.uhn.fhir.model.api.Include("DocumentReference:relatesto").toLocked(); 3441 3442 /** 3443 * Search parameter: <b>relation</b> 3444 * <p> 3445 * Description: <b>replaces | transforms | signs | appends</b><br> 3446 * Type: <b>token</b><br> 3447 * Path: <b>DocumentReference.relatesTo.code</b><br> 3448 * </p> 3449 */ 3450 @SearchParamDefinition(name="relation", path="DocumentReference.relatesTo.code", description="replaces | transforms | signs | appends", type="token" ) 3451 public static final String SP_RELATION = "relation"; 3452 /** 3453 * <b>Fluent Client</b> search parameter constant for <b>relation</b> 3454 * <p> 3455 * Description: <b>replaces | transforms | signs | appends</b><br> 3456 * Type: <b>token</b><br> 3457 * Path: <b>DocumentReference.relatesTo.code</b><br> 3458 * </p> 3459 */ 3460 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATION); 3461 3462 /** 3463 * Search parameter: <b>relationship</b> 3464 * <p> 3465 * Description: <b>Combination of relation and relatesTo</b><br> 3466 * Type: <b>composite</b><br> 3467 * Path: <b>DocumentReference.relatesTo</b><br> 3468 * </p> 3469 */ 3470 @SearchParamDefinition(name="relationship", path="DocumentReference.relatesTo", description="Combination of relation and relatesTo", type="composite", compositeOf={"relatesto", "relation"} ) 3471 public static final String SP_RELATIONSHIP = "relationship"; 3472 /** 3473 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 3474 * <p> 3475 * Description: <b>Combination of relation and relatesTo</b><br> 3476 * Type: <b>composite</b><br> 3477 * Path: <b>DocumentReference.relatesTo</b><br> 3478 * </p> 3479 */ 3480 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> RELATIONSHIP = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.ReferenceClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_RELATIONSHIP); 3481 3482 /** 3483 * Search parameter: <b>security-label</b> 3484 * <p> 3485 * Description: <b>Document security-tags</b><br> 3486 * Type: <b>token</b><br> 3487 * Path: <b>DocumentReference.securityLabel</b><br> 3488 * </p> 3489 */ 3490 @SearchParamDefinition(name="security-label", path="DocumentReference.securityLabel", description="Document security-tags", type="token" ) 3491 public static final String SP_SECURITY_LABEL = "security-label"; 3492 /** 3493 * <b>Fluent Client</b> search parameter constant for <b>security-label</b> 3494 * <p> 3495 * Description: <b>Document security-tags</b><br> 3496 * Type: <b>token</b><br> 3497 * Path: <b>DocumentReference.securityLabel</b><br> 3498 * </p> 3499 */ 3500 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SECURITY_LABEL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SECURITY_LABEL); 3501 3502 /** 3503 * Search parameter: <b>setting</b> 3504 * <p> 3505 * Description: <b>Additional details about where the content was created (e.g. clinical specialty)</b><br> 3506 * Type: <b>token</b><br> 3507 * Path: <b>DocumentReference.practiceSetting</b><br> 3508 * </p> 3509 */ 3510 @SearchParamDefinition(name="setting", path="DocumentReference.practiceSetting", description="Additional details about where the content was created (e.g. clinical specialty)", type="token" ) 3511 public static final String SP_SETTING = "setting"; 3512 /** 3513 * <b>Fluent Client</b> search parameter constant for <b>setting</b> 3514 * <p> 3515 * Description: <b>Additional details about where the content was created (e.g. clinical specialty)</b><br> 3516 * Type: <b>token</b><br> 3517 * Path: <b>DocumentReference.practiceSetting</b><br> 3518 * </p> 3519 */ 3520 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SETTING = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SETTING); 3521 3522 /** 3523 * Search parameter: <b>status</b> 3524 * <p> 3525 * Description: <b>current | superseded | entered-in-error</b><br> 3526 * Type: <b>token</b><br> 3527 * Path: <b>DocumentReference.status</b><br> 3528 * </p> 3529 */ 3530 @SearchParamDefinition(name="status", path="DocumentReference.status", description="current | superseded | entered-in-error", type="token" ) 3531 public static final String SP_STATUS = "status"; 3532 /** 3533 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3534 * <p> 3535 * Description: <b>current | superseded | entered-in-error</b><br> 3536 * Type: <b>token</b><br> 3537 * Path: <b>DocumentReference.status</b><br> 3538 * </p> 3539 */ 3540 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3541 3542 /** 3543 * Search parameter: <b>subject</b> 3544 * <p> 3545 * Description: <b>Who/what is the subject of the document</b><br> 3546 * Type: <b>reference</b><br> 3547 * Path: <b>DocumentReference.subject</b><br> 3548 * </p> 3549 */ 3550 @SearchParamDefinition(name="subject", path="DocumentReference.subject", description="Who/what is the subject of the document", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3551 public static final String SP_SUBJECT = "subject"; 3552 /** 3553 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3554 * <p> 3555 * Description: <b>Who/what is the subject of the document</b><br> 3556 * Type: <b>reference</b><br> 3557 * Path: <b>DocumentReference.subject</b><br> 3558 * </p> 3559 */ 3560 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3561 3562/** 3563 * Constant for fluent queries to be used to add include statements. Specifies 3564 * the path value of "<b>DocumentReference:subject</b>". 3565 */ 3566 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("DocumentReference:subject").toLocked(); 3567 3568 /** 3569 * Search parameter: <b>version</b> 3570 * <p> 3571 * Description: <b>The business version identifier</b><br> 3572 * Type: <b>string</b><br> 3573 * Path: <b>DocumentReference.version</b><br> 3574 * </p> 3575 */ 3576 @SearchParamDefinition(name="version", path="DocumentReference.version", description="The business version identifier", type="string" ) 3577 public static final String SP_VERSION = "version"; 3578 /** 3579 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3580 * <p> 3581 * Description: <b>The business version identifier</b><br> 3582 * Type: <b>string</b><br> 3583 * Path: <b>DocumentReference.version</b><br> 3584 * </p> 3585 */ 3586 public static final ca.uhn.fhir.rest.gclient.StringClientParam VERSION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_VERSION); 3587 3588 /** 3589 * Search parameter: <b>date</b> 3590 * <p> 3591 * Description: <b>Multiple Resources: 3592 3593* [AdverseEvent](adverseevent.html): When the event occurred 3594* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3595* [Appointment](appointment.html): Appointment date/time. 3596* [AuditEvent](auditevent.html): Time when the event was recorded 3597* [CarePlan](careplan.html): Time period plan covers 3598* [CareTeam](careteam.html): A date within the coverage time period. 3599* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3600* [Composition](composition.html): Composition editing time 3601* [Consent](consent.html): When consent was agreed to 3602* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3603* [DocumentReference](documentreference.html): When this document reference was created 3604* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3605* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3606* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3607* [Flag](flag.html): Time period when flag is active 3608* [Immunization](immunization.html): Vaccination (non)-Administration Date 3609* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3610* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3611* [Invoice](invoice.html): Invoice date / posting date 3612* [List](list.html): When the list was prepared 3613* [MeasureReport](measurereport.html): The date of the measure report 3614* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3615* [Observation](observation.html): Clinically relevant time/time-period for observation 3616* [Procedure](procedure.html): When the procedure occurred or is occurring 3617* [ResearchSubject](researchsubject.html): Start and end of participation 3618* [RiskAssessment](riskassessment.html): When was assessment made? 3619* [SupplyRequest](supplyrequest.html): When the request was made 3620</b><br> 3621 * Type: <b>date</b><br> 3622 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3623 * </p> 3624 */ 3625 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 3626 public static final String SP_DATE = "date"; 3627 /** 3628 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3629 * <p> 3630 * Description: <b>Multiple Resources: 3631 3632* [AdverseEvent](adverseevent.html): When the event occurred 3633* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3634* [Appointment](appointment.html): Appointment date/time. 3635* [AuditEvent](auditevent.html): Time when the event was recorded 3636* [CarePlan](careplan.html): Time period plan covers 3637* [CareTeam](careteam.html): A date within the coverage time period. 3638* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3639* [Composition](composition.html): Composition editing time 3640* [Consent](consent.html): When consent was agreed to 3641* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3642* [DocumentReference](documentreference.html): When this document reference was created 3643* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3644* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3645* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3646* [Flag](flag.html): Time period when flag is active 3647* [Immunization](immunization.html): Vaccination (non)-Administration Date 3648* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3649* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3650* [Invoice](invoice.html): Invoice date / posting date 3651* [List](list.html): When the list was prepared 3652* [MeasureReport](measurereport.html): The date of the measure report 3653* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3654* [Observation](observation.html): Clinically relevant time/time-period for observation 3655* [Procedure](procedure.html): When the procedure occurred or is occurring 3656* [ResearchSubject](researchsubject.html): Start and end of participation 3657* [RiskAssessment](riskassessment.html): When was assessment made? 3658* [SupplyRequest](supplyrequest.html): When the request was made 3659</b><br> 3660 * Type: <b>date</b><br> 3661 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3662 * </p> 3663 */ 3664 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3665 3666 /** 3667 * Search parameter: <b>identifier</b> 3668 * <p> 3669 * Description: <b>Multiple Resources: 3670 3671* [Account](account.html): Account number 3672* [AdverseEvent](adverseevent.html): Business identifier for the event 3673* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3674* [Appointment](appointment.html): An Identifier of the Appointment 3675* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3676* [Basic](basic.html): Business identifier 3677* [BodyStructure](bodystructure.html): Bodystructure identifier 3678* [CarePlan](careplan.html): External Ids for this plan 3679* [CareTeam](careteam.html): External Ids for this team 3680* [ChargeItem](chargeitem.html): Business Identifier for item 3681* [Claim](claim.html): The primary identifier of the financial resource 3682* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3683* [ClinicalImpression](clinicalimpression.html): Business identifier 3684* [Communication](communication.html): Unique identifier 3685* [CommunicationRequest](communicationrequest.html): Unique identifier 3686* [Composition](composition.html): Version-independent identifier for the Composition 3687* [Condition](condition.html): A unique identifier of the condition record 3688* [Consent](consent.html): Identifier for this record (external references) 3689* [Contract](contract.html): The identity of the contract 3690* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3691* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3692* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3693* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3694* [DeviceRequest](devicerequest.html): Business identifier for request/order 3695* [DeviceUsage](deviceusage.html): Search by identifier 3696* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3697* [DocumentReference](documentreference.html): Identifier of the attachment binary 3698* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3699* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3700* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3701* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3702* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3703* [Flag](flag.html): Business identifier 3704* [Goal](goal.html): External Ids for this goal 3705* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3706* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3707* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3708* [Immunization](immunization.html): Business identifier 3709* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3710* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3711* [Invoice](invoice.html): Business Identifier for item 3712* [List](list.html): Business identifier 3713* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3714* [Medication](medication.html): Returns medications with this external identifier 3715* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3716* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3717* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3718* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3719* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3720* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3721* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3722* [Observation](observation.html): The unique id for a particular observation 3723* [Person](person.html): A person Identifier 3724* [Procedure](procedure.html): A unique identifier for a procedure 3725* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3726* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3727* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3728* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3729* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3730* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3731* [Specimen](specimen.html): The unique identifier associated with the specimen 3732* [SupplyDelivery](supplydelivery.html): External identifier 3733* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3734* [Task](task.html): Search for a task instance by its business identifier 3735* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3736</b><br> 3737 * Type: <b>token</b><br> 3738 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3739 * </p> 3740 */ 3741 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3742 public static final String SP_IDENTIFIER = "identifier"; 3743 /** 3744 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3745 * <p> 3746 * Description: <b>Multiple Resources: 3747 3748* [Account](account.html): Account number 3749* [AdverseEvent](adverseevent.html): Business identifier for the event 3750* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3751* [Appointment](appointment.html): An Identifier of the Appointment 3752* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3753* [Basic](basic.html): Business identifier 3754* [BodyStructure](bodystructure.html): Bodystructure identifier 3755* [CarePlan](careplan.html): External Ids for this plan 3756* [CareTeam](careteam.html): External Ids for this team 3757* [ChargeItem](chargeitem.html): Business Identifier for item 3758* [Claim](claim.html): The primary identifier of the financial resource 3759* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3760* [ClinicalImpression](clinicalimpression.html): Business identifier 3761* [Communication](communication.html): Unique identifier 3762* [CommunicationRequest](communicationrequest.html): Unique identifier 3763* [Composition](composition.html): Version-independent identifier for the Composition 3764* [Condition](condition.html): A unique identifier of the condition record 3765* [Consent](consent.html): Identifier for this record (external references) 3766* [Contract](contract.html): The identity of the contract 3767* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3768* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3769* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3770* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3771* [DeviceRequest](devicerequest.html): Business identifier for request/order 3772* [DeviceUsage](deviceusage.html): Search by identifier 3773* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3774* [DocumentReference](documentreference.html): Identifier of the attachment binary 3775* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3776* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3777* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3778* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3779* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3780* [Flag](flag.html): Business identifier 3781* [Goal](goal.html): External Ids for this goal 3782* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3783* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3784* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3785* [Immunization](immunization.html): Business identifier 3786* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3787* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3788* [Invoice](invoice.html): Business Identifier for item 3789* [List](list.html): Business identifier 3790* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3791* [Medication](medication.html): Returns medications with this external identifier 3792* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3793* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3794* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3795* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3796* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3797* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3798* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3799* [Observation](observation.html): The unique id for a particular observation 3800* [Person](person.html): A person Identifier 3801* [Procedure](procedure.html): A unique identifier for a procedure 3802* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3803* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3804* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3805* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3806* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3807* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3808* [Specimen](specimen.html): The unique identifier associated with the specimen 3809* [SupplyDelivery](supplydelivery.html): External identifier 3810* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3811* [Task](task.html): Search for a task instance by its business identifier 3812* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3813</b><br> 3814 * Type: <b>token</b><br> 3815 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3816 * </p> 3817 */ 3818 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3819 3820 /** 3821 * Search parameter: <b>patient</b> 3822 * <p> 3823 * Description: <b>Multiple Resources: 3824 3825* [Account](account.html): The entity that caused the expenses 3826* [AdverseEvent](adverseevent.html): Subject impacted by event 3827* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3828* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3829* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3830* [AuditEvent](auditevent.html): Where the activity involved patient data 3831* [Basic](basic.html): Identifies the focus of this resource 3832* [BodyStructure](bodystructure.html): Who this is about 3833* [CarePlan](careplan.html): Who the care plan is for 3834* [CareTeam](careteam.html): Who care team is for 3835* [ChargeItem](chargeitem.html): Individual service was done for/to 3836* [Claim](claim.html): Patient receiving the products or services 3837* [ClaimResponse](claimresponse.html): The subject of care 3838* [ClinicalImpression](clinicalimpression.html): Patient assessed 3839* [Communication](communication.html): Focus of message 3840* [CommunicationRequest](communicationrequest.html): Focus of message 3841* [Composition](composition.html): Who and/or what the composition is about 3842* [Condition](condition.html): Who has the condition? 3843* [Consent](consent.html): Who the consent applies to 3844* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3845* [Coverage](coverage.html): Retrieve coverages for a patient 3846* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3847* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3848* [DetectedIssue](detectedissue.html): Associated patient 3849* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3850* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3851* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3852* [DocumentReference](documentreference.html): Who/what is the subject of the document 3853* [Encounter](encounter.html): The patient present at the encounter 3854* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3855* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3856* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3857* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3858* [Flag](flag.html): The identity of a subject to list flags for 3859* [Goal](goal.html): Who this goal is intended for 3860* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3861* [ImagingSelection](imagingselection.html): Who the study is about 3862* [ImagingStudy](imagingstudy.html): Who the study is about 3863* [Immunization](immunization.html): The patient for the vaccination record 3864* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3865* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3866* [Invoice](invoice.html): Recipient(s) of goods and services 3867* [List](list.html): If all resources have the same subject 3868* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3869* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3870* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3871* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3872* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3873* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3874* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3875* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3876* [Observation](observation.html): The subject that the observation is about (if patient) 3877* [Person](person.html): The Person links to this Patient 3878* [Procedure](procedure.html): Search by subject - a patient 3879* [Provenance](provenance.html): Where the activity involved patient data 3880* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3881* [RelatedPerson](relatedperson.html): The patient this related person is related to 3882* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3883* [ResearchSubject](researchsubject.html): Who or what is part of study 3884* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3885* [ServiceRequest](servicerequest.html): Search by subject - a patient 3886* [Specimen](specimen.html): The patient the specimen comes from 3887* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3888* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3889* [Task](task.html): Search by patient 3890* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3891</b><br> 3892 * Type: <b>reference</b><br> 3893 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3894 * </p> 3895 */ 3896 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3897 public static final String SP_PATIENT = "patient"; 3898 /** 3899 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3900 * <p> 3901 * Description: <b>Multiple Resources: 3902 3903* [Account](account.html): The entity that caused the expenses 3904* [AdverseEvent](adverseevent.html): Subject impacted by event 3905* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3906* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3907* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3908* [AuditEvent](auditevent.html): Where the activity involved patient data 3909* [Basic](basic.html): Identifies the focus of this resource 3910* [BodyStructure](bodystructure.html): Who this is about 3911* [CarePlan](careplan.html): Who the care plan is for 3912* [CareTeam](careteam.html): Who care team is for 3913* [ChargeItem](chargeitem.html): Individual service was done for/to 3914* [Claim](claim.html): Patient receiving the products or services 3915* [ClaimResponse](claimresponse.html): The subject of care 3916* [ClinicalImpression](clinicalimpression.html): Patient assessed 3917* [Communication](communication.html): Focus of message 3918* [CommunicationRequest](communicationrequest.html): Focus of message 3919* [Composition](composition.html): Who and/or what the composition is about 3920* [Condition](condition.html): Who has the condition? 3921* [Consent](consent.html): Who the consent applies to 3922* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3923* [Coverage](coverage.html): Retrieve coverages for a patient 3924* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3925* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3926* [DetectedIssue](detectedissue.html): Associated patient 3927* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3928* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3929* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3930* [DocumentReference](documentreference.html): Who/what is the subject of the document 3931* [Encounter](encounter.html): The patient present at the encounter 3932* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3933* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3934* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3935* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3936* [Flag](flag.html): The identity of a subject to list flags for 3937* [Goal](goal.html): Who this goal is intended for 3938* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3939* [ImagingSelection](imagingselection.html): Who the study is about 3940* [ImagingStudy](imagingstudy.html): Who the study is about 3941* [Immunization](immunization.html): The patient for the vaccination record 3942* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3943* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3944* [Invoice](invoice.html): Recipient(s) of goods and services 3945* [List](list.html): If all resources have the same subject 3946* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3947* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3948* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3949* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3950* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3951* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3952* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3953* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3954* [Observation](observation.html): The subject that the observation is about (if patient) 3955* [Person](person.html): The Person links to this Patient 3956* [Procedure](procedure.html): Search by subject - a patient 3957* [Provenance](provenance.html): Where the activity involved patient data 3958* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3959* [RelatedPerson](relatedperson.html): The patient this related person is related to 3960* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3961* [ResearchSubject](researchsubject.html): Who or what is part of study 3962* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3963* [ServiceRequest](servicerequest.html): Search by subject - a patient 3964* [Specimen](specimen.html): The patient the specimen comes from 3965* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3966* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3967* [Task](task.html): Search by patient 3968* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3969</b><br> 3970 * Type: <b>reference</b><br> 3971 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3972 * </p> 3973 */ 3974 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3975 3976/** 3977 * Constant for fluent queries to be used to add include statements. Specifies 3978 * the path value of "<b>DocumentReference:patient</b>". 3979 */ 3980 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("DocumentReference:patient").toLocked(); 3981 3982 /** 3983 * Search parameter: <b>type</b> 3984 * <p> 3985 * Description: <b>Multiple Resources: 3986 3987* [Account](account.html): E.g. patient, expense, depreciation 3988* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3989* [Composition](composition.html): Kind of composition (LOINC if possible) 3990* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3991* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3992* [Encounter](encounter.html): Specific type of encounter 3993* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3994* [Invoice](invoice.html): Type of Invoice 3995* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3996* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3997* [Specimen](specimen.html): The specimen type 3998</b><br> 3999 * Type: <b>token</b><br> 4000 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 4001 * </p> 4002 */ 4003 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 4004 public static final String SP_TYPE = "type"; 4005 /** 4006 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4007 * <p> 4008 * Description: <b>Multiple Resources: 4009 4010* [Account](account.html): E.g. patient, expense, depreciation 4011* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 4012* [Composition](composition.html): Kind of composition (LOINC if possible) 4013* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 4014* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 4015* [Encounter](encounter.html): Specific type of encounter 4016* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 4017* [Invoice](invoice.html): Type of Invoice 4018* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 4019* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 4020* [Specimen](specimen.html): The specimen type 4021</b><br> 4022 * Type: <b>token</b><br> 4023 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 4024 * </p> 4025 */ 4026 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4027 4028 4029} 4030