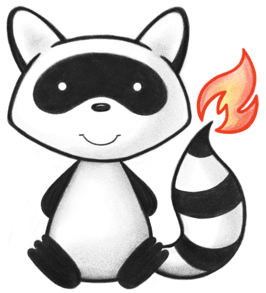
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Collections; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 040import org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions; 041import org.hl7.fhir.instance.model.api.IDomainResource; 042import org.hl7.fhir.r5.utils.ToolingExtensions; 043import org.hl7.fhir.utilities.StandardsStatus; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A resource that includes narrative, extensions, and contained resources. 051 */ 052public abstract class DomainResource extends Resource implements IBaseHasExtensions, IBaseHasModifierExtensions, IDomainResource { 053 054 /** 055 * A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety. 056 */ 057 @Child(name = "text", type = {Narrative.class}, order=0, min=0, max=1, modifier=false, summary=false) 058 @Description(shortDefinition="Text summary of the resource, for human interpretation", formalDefinition="A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety." ) 059 protected Narrative text; 060 061 /** 062 * These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, nor can they have their own independent transaction scope. This is allowed to be a Parameters resource if and only if it is referenced by a resource that provides context/meaning. 063 */ 064 @Child(name = "contained", type = {Resource.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 065 @Description(shortDefinition="Contained, inline Resources", formalDefinition="These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, nor can they have their own independent transaction scope. This is allowed to be a Parameters resource if and only if it is referenced by a resource that provides context/meaning." ) 066 protected List<Resource> contained; 067 068 /** 069 * May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. 070 */ 071 @Child(name = "extension", type = {Extension.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 072 @Description(shortDefinition="Additional content defined by implementations", formalDefinition="May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension." ) 073 protected List<Extension> extension; 074 075 /** 076 * May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 077 078Modifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself). 079 */ 080 @Child(name = "modifierExtension", type = {Extension.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 081 @Description(shortDefinition="Extensions that cannot be ignored", formalDefinition="May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself)." ) 082 protected List<Extension> modifierExtension; 083 084 private static final long serialVersionUID = -970285559L; 085 086 /** 087 * Constructor 088 */ 089 public DomainResource() { 090 super(); 091 } 092 093 /** 094 * @return {@link #text} (A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.) 095 */ 096 public Narrative getText() { 097 if (this.text == null) 098 if (Configuration.errorOnAutoCreate()) 099 throw new Error("Attempt to auto-create DomainResource.text"); 100 else if (Configuration.doAutoCreate()) 101 this.text = new Narrative(); // cc 102 return this.text; 103 } 104 105 public boolean hasText() { 106 return this.text != null && !this.text.isEmpty(); 107 } 108 109 /** 110 * @param value {@link #text} (A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it "clinically safe" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.) 111 */ 112 public DomainResource setText(Narrative value) { 113 this.text = value; 114 return this; 115 } 116 117 /** 118 * @return {@link #contained} (These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, nor can they have their own independent transaction scope. This is allowed to be a Parameters resource if and only if it is referenced by a resource that provides context/meaning.) 119 */ 120 public List<Resource> getContained() { 121 if (this.contained == null) 122 this.contained = new ArrayList<Resource>(); 123 return this.contained; 124 } 125 126 /** 127 * @return Returns a reference to <code>this</code> for easy method chaining 128 */ 129 public DomainResource setContained(List<Resource> theContained) { 130 this.contained = theContained; 131 return this; 132 } 133 134 public boolean hasContained() { 135 if (this.contained == null) 136 return false; 137 for (Resource item : this.contained) 138 if (!item.isEmpty()) 139 return true; 140 return false; 141 } 142 143 public DomainResource addContained(Resource t) { //3 144 if (t == null) 145 return this; 146 if (this.contained == null) 147 this.contained = new ArrayList<Resource>(); 148 this.contained.add(t); 149 return this; 150 } 151 152 /** 153 * @return {@link #extension} (May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.) 154 */ 155 public List<Extension> getExtension() { 156 if (this.extension == null) 157 this.extension = new ArrayList<Extension>(); 158 return this.extension; 159 } 160 161 /** 162 * @return Returns a reference to <code>this</code> for easy method chaining 163 */ 164 public DomainResource setExtension(List<Extension> theExtension) { 165 this.extension = theExtension; 166 return this; 167 } 168 169 public boolean hasExtension() { 170 if (this.extension == null) 171 return false; 172 for (Extension item : this.extension) 173 if (!item.isEmpty()) 174 return true; 175 return false; 176 } 177 178 public Extension addExtension() { //3 179 Extension t = new Extension(); 180 if (this.extension == null) 181 this.extension = new ArrayList<Extension>(); 182 this.extension.add(t); 183 return t; 184 } 185 186 public DomainResource addExtension(Extension t) { //3 187 if (t == null) 188 return this; 189 if (this.extension == null) 190 this.extension = new ArrayList<Extension>(); 191 this.extension.add(t); 192 return this; 193 } 194 195 /** 196 * @return {@link #modifierExtension} (May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 197 198Modifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).) 199 */ 200 public List<Extension> getModifierExtension() { 201 if (this.modifierExtension == null) 202 this.modifierExtension = new ArrayList<Extension>(); 203 return this.modifierExtension; 204 } 205 206 /** 207 * @return Returns a reference to <code>this</code> for easy method chaining 208 */ 209 public DomainResource setModifierExtension(List<Extension> theModifierExtension) { 210 this.modifierExtension = theModifierExtension; 211 return this; 212 } 213 214 public boolean hasModifierExtension() { 215 if (this.modifierExtension == null) 216 return false; 217 for (Extension item : this.modifierExtension) 218 if (!item.isEmpty()) 219 return true; 220 return false; 221 } 222 223 public Extension addModifierExtension() { //3 224 Extension t = new Extension(); 225 if (this.modifierExtension == null) 226 this.modifierExtension = new ArrayList<Extension>(); 227 this.modifierExtension.add(t); 228 return t; 229 } 230 231 public DomainResource addModifierExtension(Extension t) { //3 232 if (t == null) 233 return this; 234 if (this.modifierExtension == null) 235 this.modifierExtension = new ArrayList<Extension>(); 236 this.modifierExtension.add(t); 237 return this; 238 } 239 240 protected void listChildren(List<Property> children) { 241 super.listChildren(children); 242 children.add(new Property("text", "Narrative", "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 0, 1, text)); 243 children.add(new Property("contained", "Resource", "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, nor can they have their own independent transaction scope. This is allowed to be a Parameters resource if and only if it is referenced by a resource that provides context/meaning.", 0, java.lang.Integer.MAX_VALUE, contained)); 244 children.add(new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension)); 245 children.add(new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 246 } 247 248 @Override 249 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 250 switch (_hash) { 251 case 3556653: /*text*/ return new Property("text", "Narrative", "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 0, 1, text); 252 case -410956685: /*contained*/ return new Property("contained", "Resource", "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, nor can they have their own independent transaction scope. This is allowed to be a Parameters resource if and only if it is referenced by a resource that provides context/meaning.", 0, java.lang.Integer.MAX_VALUE, contained); 253 case -612557761: /*extension*/ return new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension); 254 case -298878168: /*modifierExtension*/ return new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 0, java.lang.Integer.MAX_VALUE, modifierExtension); 255 default: return super.getNamedProperty(_hash, _name, _checkValid); 256 } 257 258 } 259 260 @Override 261 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 262 switch (hash) { 263 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // Narrative 264 case -410956685: /*contained*/ return this.contained == null ? new Base[0] : this.contained.toArray(new Base[this.contained.size()]); // Resource 265 case -612557761: /*extension*/ return this.extension == null ? new Base[0] : this.extension.toArray(new Base[this.extension.size()]); // Extension 266 case -298878168: /*modifierExtension*/ return this.modifierExtension == null ? new Base[0] : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 267 default: return super.getProperty(hash, name, checkValid); 268 } 269 270 } 271 272 @Override 273 public Base setProperty(int hash, String name, Base value) throws FHIRException { 274 switch (hash) { 275 case 3556653: // text 276 this.text = TypeConvertor.castToNarrative(value); // Narrative 277 return value; 278 case -410956685: // contained 279 this.getContained().add(TypeConvertor.castToResource(value)); // Resource 280 return value; 281 case -612557761: // extension 282 this.getExtension().add(TypeConvertor.castToExtension(value)); // Extension 283 return value; 284 case -298878168: // modifierExtension 285 this.getModifierExtension().add(TypeConvertor.castToExtension(value)); // Extension 286 return value; 287 default: return super.setProperty(hash, name, value); 288 } 289 290 } 291 292 @Override 293 public Base setProperty(String name, Base value) throws FHIRException { 294 if (name.equals("text")) { 295 this.text = TypeConvertor.castToNarrative(value); // Narrative 296 } else if (name.equals("contained")) { 297 this.getContained().add(TypeConvertor.castToResource(value)); 298 } else if (name.equals("extension")) { 299 this.getExtension().add(TypeConvertor.castToExtension(value)); 300 } else if (name.equals("modifierExtension")) { 301 this.getModifierExtension().add(TypeConvertor.castToExtension(value)); 302 } else 303 return super.setProperty(name, value); 304 return value; 305 } 306 307 @Override 308 public void removeChild(String name, Base value) throws FHIRException { 309 if (name.equals("text")) { 310 this.text = null; 311 } else if (name.equals("contained")) { 312 this.getContained().remove(value); 313 } else if (name.equals("extension")) { 314 this.getExtension().remove(value); 315 } else if (name.equals("modifierExtension")) { 316 this.getModifierExtension().remove(value); 317 } else 318 super.removeChild(name, value); 319 320 } 321 322 @Override 323 public Base makeProperty(int hash, String name) throws FHIRException { 324 switch (hash) { 325 case 3556653: return getText(); 326 case -410956685: throw new FHIRException("Cannot make property contained as it is not a complex type"); // Resource 327 case -612557761: return addExtension(); 328 case -298878168: return addModifierExtension(); 329 default: return super.makeProperty(hash, name); 330 } 331 332 } 333 334 @Override 335 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 336 switch (hash) { 337 case 3556653: /*text*/ return new String[] {"Narrative"}; 338 case -410956685: /*contained*/ return new String[] {"Resource"}; 339 case -612557761: /*extension*/ return new String[] {"Extension"}; 340 case -298878168: /*modifierExtension*/ return new String[] {"Extension"}; 341 default: return super.getTypesForProperty(hash, name); 342 } 343 344 } 345 346 @Override 347 public Base addChild(String name) throws FHIRException { 348 if (name.equals("text")) { 349 this.text = new Narrative(); 350 return this.text; 351 } 352 else if (name.equals("contained")) { 353 throw new FHIRException("Cannot call addChild on an abstract type DomainResource.contained"); 354 } 355 else if (name.equals("extension")) { 356 return addExtension(); 357 } 358 else if (name.equals("modifierExtension")) { 359 return addModifierExtension(); 360 } 361 else 362 return super.addChild(name); 363 } 364 365 public String fhirType() { 366 return "DomainResource"; 367 368 } 369 370 public abstract DomainResource copy(); 371 372 public void copyValues(DomainResource dst) { 373 super.copyValues(dst); 374 dst.text = text == null ? null : text.copy(); 375 if (contained != null) { 376 dst.contained = new ArrayList<Resource>(); 377 for (Resource i : contained) 378 dst.contained.add(i.copy()); 379 }; 380 if (extension != null) { 381 dst.extension = new ArrayList<Extension>(); 382 for (Extension i : extension) 383 dst.extension.add(i.copy()); 384 }; 385 if (modifierExtension != null) { 386 dst.modifierExtension = new ArrayList<Extension>(); 387 for (Extension i : modifierExtension) 388 dst.modifierExtension.add(i.copy()); 389 }; 390 } 391 392 @Override 393 public boolean equalsDeep(Base other_) { 394 if (!super.equalsDeep(other_)) 395 return false; 396 if (!(other_ instanceof DomainResource)) 397 return false; 398 DomainResource o = (DomainResource) other_; 399 return compareDeep(text, o.text, true) && compareDeep(contained, o.contained, true) && compareDeep(extension, o.extension, true) 400 && compareDeep(modifierExtension, o.modifierExtension, true); 401 } 402 403 @Override 404 public boolean equalsShallow(Base other_) { 405 if (!super.equalsShallow(other_)) 406 return false; 407 if (!(other_ instanceof DomainResource)) 408 return false; 409 DomainResource o = (DomainResource) other_; 410 return true; 411 } 412 413 public boolean isEmpty() { 414 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, contained, extension 415 , modifierExtension); 416 } 417 418 /** 419 * Search parameter: <b>_text</b> 420 * <p> 421 * Description: <b>Search on the narrative of the resource</b><br> 422 * Type: <b>special</b><br> 423 * Path: <b>null</b><br> 424 * </p> 425 */ 426 @SearchParamDefinition(name="_text", path="", description="Search on the narrative of the resource", type="special" ) 427 public static final String SP_TEXT = "_text"; 428 /** 429 * <b>Fluent Client</b> search parameter constant for <b>_text</b> 430 * <p> 431 * Description: <b>Search on the narrative of the resource</b><br> 432 * Type: <b>special</b><br> 433 * Path: <b>null</b><br> 434 * </p> 435 */ 436 public static final ca.uhn.fhir.rest.gclient.SpecialClientParam TEXT = new ca.uhn.fhir.rest.gclient.SpecialClientParam(SP_TEXT); 437 438// Manual code (from Configuration.txt): 439public void checkNoModifiers(String noun, String verb) throws FHIRException { 440 if (hasModifierExtension()) { 441 throw new FHIRException("Found unknown Modifier Exceptions on "+noun+" doing "+verb); 442 } 443 444 } 445 446 public void addExtension(String url, DataType value) { 447 Extension ex = new Extension(); 448 ex.setUrl(url); 449 ex.setValue(value); 450 getExtension().add(ex); 451 } 452 453 public boolean hasExtension(String... theUrls) { 454 for (Extension next : getExtension()) { 455 if (Utilities.existsInList(next.getUrl(), theUrls)) { 456 return true; 457 } 458 } 459 return false; 460 } 461 462 public boolean hasExtension(String url) { 463 for (Extension e : getExtension()) 464 if (url.equals(e.getUrl())) 465 return true; 466 return false; 467 } 468 469 public Extension getExtensionByUrl(String theUrl) { 470 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 471 ArrayList<Extension> retVal = new ArrayList<Extension>(); 472 for (Extension next : getExtension()) { 473 if (theUrl.equals(next.getUrl())) { 474 retVal.add(next); 475 } 476 } 477 if (retVal.size() == 0) 478 return null; 479 else { 480 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url "+theUrl+" must have only one match"); 481 return retVal.get(0); 482 } 483 } 484 485 public Resource getContained(String ref) { 486 if (ref == null) 487 return null; 488 489 if (ref.startsWith("#")) 490 ref = ref.substring(1); 491 for (Resource r : getContained()) { 492 if (r.getId().equals(ref)) 493 return r; 494 } 495 return null; 496 } 497 498 /** 499 * Returns a list of extensions from this element which have the given URL. Note that 500 * this list may not be modified (you can not add or remove elements from it) 501 */ 502 public List<Extension> getExtensionsByUrl(String theUrl) { 503 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 504 ArrayList<Extension> retVal = new ArrayList<Extension>(); 505 for (Extension next : getExtension()) { 506 if (theUrl.equals(next.getUrl())) { 507 retVal.add(next); 508 } 509 } 510 return Collections.unmodifiableList(retVal); 511 } 512 513 514 public List<Extension> getExtensionsByUrl(String... theUrls) { 515 516 ArrayList<Extension> retVal = new ArrayList<>(); 517 for (Extension next : getExtension()) { 518 if (Utilities.existsInList(next.getUrl(), theUrls)) { 519 retVal.add(next); 520 } 521 } 522 return java.util.Collections.unmodifiableList(retVal); 523 } 524 525 /** 526 * Returns a list of modifier extensions from this element which have the given URL. Note that 527 * this list may not be modified (you can not add or remove elements from it) 528 */ 529 public List<Extension> getModifierExtensionsByUrl(String theUrl) { 530 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 531 ArrayList<Extension> retVal = new ArrayList<Extension>(); 532 for (Extension next : getModifierExtension()) { 533 if (theUrl.equals(next.getUrl())) { 534 retVal.add(next); 535 } 536 } 537 return Collections.unmodifiableList(retVal); 538 } 539 540 541 public StandardsStatus getStandardsStatus() { 542 return ToolingExtensions.getStandardsStatus(this); 543 } 544 545 public void setStandardsStatus(StandardsStatus status) { 546 ToolingExtensions.setStandardsStatus(this, status, null); 547 } 548 549 550// end addition 551 552} 553