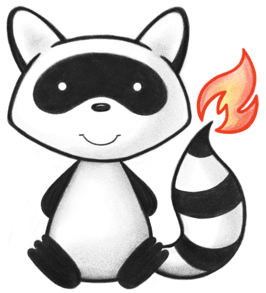
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Dosage Type: Indicates how the medication is/was taken or should be taken by the patient. 050 */ 051@DatatypeDef(name="Dosage") 052public class Dosage extends BackboneType implements ICompositeType { 053 054 @Block() 055 public static class DosageDoseAndRateComponent extends Element implements IBaseDatatypeElement { 056 /** 057 * The kind of dose or rate specified, for example, ordered or calculated. 058 */ 059 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="The kind of dose or rate specified", formalDefinition="The kind of dose or rate specified, for example, ordered or calculated." ) 061 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/dose-rate-type") 062 protected CodeableConcept type; 063 064 /** 065 * Amount of medication per dose. 066 */ 067 @Child(name = "dose", type = {Range.class, Quantity.class}, order=2, min=0, max=1, modifier=false, summary=true) 068 @Description(shortDefinition="Amount of medication per dose", formalDefinition="Amount of medication per dose." ) 069 protected DataType dose; 070 071 /** 072 * Amount of medication per unit of time. 073 */ 074 @Child(name = "rate", type = {Ratio.class, Range.class, Quantity.class}, order=3, min=0, max=1, modifier=false, summary=true) 075 @Description(shortDefinition="Amount of medication per unit of time", formalDefinition="Amount of medication per unit of time." ) 076 protected DataType rate; 077 078 private static final long serialVersionUID = 230646604L; 079 080 /** 081 * Constructor 082 */ 083 public DosageDoseAndRateComponent() { 084 super(); 085 } 086 087 /** 088 * @return {@link #type} (The kind of dose or rate specified, for example, ordered or calculated.) 089 */ 090 public CodeableConcept getType() { 091 if (this.type == null) 092 if (Configuration.errorOnAutoCreate()) 093 throw new Error("Attempt to auto-create DosageDoseAndRateComponent.type"); 094 else if (Configuration.doAutoCreate()) 095 this.type = new CodeableConcept(); // cc 096 return this.type; 097 } 098 099 public boolean hasType() { 100 return this.type != null && !this.type.isEmpty(); 101 } 102 103 /** 104 * @param value {@link #type} (The kind of dose or rate specified, for example, ordered or calculated.) 105 */ 106 public DosageDoseAndRateComponent setType(CodeableConcept value) { 107 this.type = value; 108 return this; 109 } 110 111 /** 112 * @return {@link #dose} (Amount of medication per dose.) 113 */ 114 public DataType getDose() { 115 return this.dose; 116 } 117 118 /** 119 * @return {@link #dose} (Amount of medication per dose.) 120 */ 121 public Range getDoseRange() throws FHIRException { 122 if (this.dose == null) 123 this.dose = new Range(); 124 if (!(this.dose instanceof Range)) 125 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.dose.getClass().getName()+" was encountered"); 126 return (Range) this.dose; 127 } 128 129 public boolean hasDoseRange() { 130 return this.dose instanceof Range; 131 } 132 133 /** 134 * @return {@link #dose} (Amount of medication per dose.) 135 */ 136 public Quantity getDoseQuantity() throws FHIRException { 137 if (this.dose == null) 138 this.dose = new Quantity(); 139 if (!(this.dose instanceof Quantity)) 140 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.dose.getClass().getName()+" was encountered"); 141 return (Quantity) this.dose; 142 } 143 144 public boolean hasDoseQuantity() { 145 return this.dose instanceof Quantity; 146 } 147 148 public boolean hasDose() { 149 return this.dose != null && !this.dose.isEmpty(); 150 } 151 152 /** 153 * @param value {@link #dose} (Amount of medication per dose.) 154 */ 155 public DosageDoseAndRateComponent setDose(DataType value) { 156 if (value != null && !(value instanceof Range || value instanceof Quantity)) 157 throw new FHIRException("Not the right type for Dosage.doseAndRate.dose[x]: "+value.fhirType()); 158 this.dose = value; 159 return this; 160 } 161 162 /** 163 * @return {@link #rate} (Amount of medication per unit of time.) 164 */ 165 public DataType getRate() { 166 return this.rate; 167 } 168 169 /** 170 * @return {@link #rate} (Amount of medication per unit of time.) 171 */ 172 public Ratio getRateRatio() throws FHIRException { 173 if (this.rate == null) 174 this.rate = new Ratio(); 175 if (!(this.rate instanceof Ratio)) 176 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.rate.getClass().getName()+" was encountered"); 177 return (Ratio) this.rate; 178 } 179 180 public boolean hasRateRatio() { 181 return this.rate instanceof Ratio; 182 } 183 184 /** 185 * @return {@link #rate} (Amount of medication per unit of time.) 186 */ 187 public Range getRateRange() throws FHIRException { 188 if (this.rate == null) 189 this.rate = new Range(); 190 if (!(this.rate instanceof Range)) 191 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.rate.getClass().getName()+" was encountered"); 192 return (Range) this.rate; 193 } 194 195 public boolean hasRateRange() { 196 return this.rate instanceof Range; 197 } 198 199 /** 200 * @return {@link #rate} (Amount of medication per unit of time.) 201 */ 202 public Quantity getRateQuantity() throws FHIRException { 203 if (this.rate == null) 204 this.rate = new Quantity(); 205 if (!(this.rate instanceof Quantity)) 206 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.rate.getClass().getName()+" was encountered"); 207 return (Quantity) this.rate; 208 } 209 210 public boolean hasRateQuantity() { 211 return this.rate instanceof Quantity; 212 } 213 214 public boolean hasRate() { 215 return this.rate != null && !this.rate.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #rate} (Amount of medication per unit of time.) 220 */ 221 public DosageDoseAndRateComponent setRate(DataType value) { 222 if (value != null && !(value instanceof Ratio || value instanceof Range || value instanceof Quantity)) 223 throw new FHIRException("Not the right type for Dosage.doseAndRate.rate[x]: "+value.fhirType()); 224 this.rate = value; 225 return this; 226 } 227 228 protected void listChildren(List<Property> children) { 229 super.listChildren(children); 230 children.add(new Property("type", "CodeableConcept", "The kind of dose or rate specified, for example, ordered or calculated.", 0, 1, type)); 231 children.add(new Property("dose[x]", "Range|Quantity", "Amount of medication per dose.", 0, 1, dose)); 232 children.add(new Property("rate[x]", "Ratio|Range|Quantity", "Amount of medication per unit of time.", 0, 1, rate)); 233 } 234 235 @Override 236 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 237 switch (_hash) { 238 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of dose or rate specified, for example, ordered or calculated.", 0, 1, type); 239 case 1843195715: /*dose[x]*/ return new Property("dose[x]", "Range|Quantity", "Amount of medication per dose.", 0, 1, dose); 240 case 3089437: /*dose*/ return new Property("dose[x]", "Range|Quantity", "Amount of medication per dose.", 0, 1, dose); 241 case 1775578912: /*doseRange*/ return new Property("dose[x]", "Range", "Amount of medication per dose.", 0, 1, dose); 242 case -2083618872: /*doseQuantity*/ return new Property("dose[x]", "Quantity", "Amount of medication per dose.", 0, 1, dose); 243 case 983460768: /*rate[x]*/ return new Property("rate[x]", "Ratio|Range|Quantity", "Amount of medication per unit of time.", 0, 1, rate); 244 case 3493088: /*rate*/ return new Property("rate[x]", "Ratio|Range|Quantity", "Amount of medication per unit of time.", 0, 1, rate); 245 case 204021515: /*rateRatio*/ return new Property("rate[x]", "Ratio", "Amount of medication per unit of time.", 0, 1, rate); 246 case 204015677: /*rateRange*/ return new Property("rate[x]", "Range", "Amount of medication per unit of time.", 0, 1, rate); 247 case -1085459061: /*rateQuantity*/ return new Property("rate[x]", "Quantity", "Amount of medication per unit of time.", 0, 1, rate); 248 default: return super.getNamedProperty(_hash, _name, _checkValid); 249 } 250 251 } 252 253 @Override 254 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 255 switch (hash) { 256 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 257 case 3089437: /*dose*/ return this.dose == null ? new Base[0] : new Base[] {this.dose}; // DataType 258 case 3493088: /*rate*/ return this.rate == null ? new Base[0] : new Base[] {this.rate}; // DataType 259 default: return super.getProperty(hash, name, checkValid); 260 } 261 262 } 263 264 @Override 265 public Base setProperty(int hash, String name, Base value) throws FHIRException { 266 switch (hash) { 267 case 3575610: // type 268 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 269 return value; 270 case 3089437: // dose 271 this.dose = TypeConvertor.castToType(value); // DataType 272 return value; 273 case 3493088: // rate 274 this.rate = TypeConvertor.castToType(value); // DataType 275 return value; 276 default: return super.setProperty(hash, name, value); 277 } 278 279 } 280 281 @Override 282 public Base setProperty(String name, Base value) throws FHIRException { 283 if (name.equals("type")) { 284 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 285 } else if (name.equals("dose[x]")) { 286 this.dose = TypeConvertor.castToType(value); // DataType 287 } else if (name.equals("rate[x]")) { 288 this.rate = TypeConvertor.castToType(value); // DataType 289 } else 290 return super.setProperty(name, value); 291 return value; 292 } 293 294 @Override 295 public void removeChild(String name, Base value) throws FHIRException { 296 if (name.equals("type")) { 297 this.type = null; 298 } else if (name.equals("dose[x]")) { 299 this.dose = null; 300 } else if (name.equals("rate[x]")) { 301 this.rate = null; 302 } else 303 super.removeChild(name, value); 304 305 } 306 307 @Override 308 public Base makeProperty(int hash, String name) throws FHIRException { 309 switch (hash) { 310 case 3575610: return getType(); 311 case 1843195715: return getDose(); 312 case 3089437: return getDose(); 313 case 983460768: return getRate(); 314 case 3493088: return getRate(); 315 default: return super.makeProperty(hash, name); 316 } 317 318 } 319 320 @Override 321 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 322 switch (hash) { 323 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 324 case 3089437: /*dose*/ return new String[] {"Range", "Quantity"}; 325 case 3493088: /*rate*/ return new String[] {"Ratio", "Range", "Quantity"}; 326 default: return super.getTypesForProperty(hash, name); 327 } 328 329 } 330 331 @Override 332 public Base addChild(String name) throws FHIRException { 333 if (name.equals("type")) { 334 this.type = new CodeableConcept(); 335 return this.type; 336 } 337 else if (name.equals("doseRange")) { 338 this.dose = new Range(); 339 return this.dose; 340 } 341 else if (name.equals("doseQuantity")) { 342 this.dose = new Quantity(); 343 return this.dose; 344 } 345 else if (name.equals("rateRatio")) { 346 this.rate = new Ratio(); 347 return this.rate; 348 } 349 else if (name.equals("rateRange")) { 350 this.rate = new Range(); 351 return this.rate; 352 } 353 else if (name.equals("rateQuantity")) { 354 this.rate = new Quantity(); 355 return this.rate; 356 } 357 else 358 return super.addChild(name); 359 } 360 361 public DosageDoseAndRateComponent copy() { 362 DosageDoseAndRateComponent dst = new DosageDoseAndRateComponent(); 363 copyValues(dst); 364 return dst; 365 } 366 367 public void copyValues(DosageDoseAndRateComponent dst) { 368 super.copyValues(dst); 369 dst.type = type == null ? null : type.copy(); 370 dst.dose = dose == null ? null : dose.copy(); 371 dst.rate = rate == null ? null : rate.copy(); 372 } 373 374 @Override 375 public boolean equalsDeep(Base other_) { 376 if (!super.equalsDeep(other_)) 377 return false; 378 if (!(other_ instanceof DosageDoseAndRateComponent)) 379 return false; 380 DosageDoseAndRateComponent o = (DosageDoseAndRateComponent) other_; 381 return compareDeep(type, o.type, true) && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true) 382 ; 383 } 384 385 @Override 386 public boolean equalsShallow(Base other_) { 387 if (!super.equalsShallow(other_)) 388 return false; 389 if (!(other_ instanceof DosageDoseAndRateComponent)) 390 return false; 391 DosageDoseAndRateComponent o = (DosageDoseAndRateComponent) other_; 392 return true; 393 } 394 395 public boolean isEmpty() { 396 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, dose, rate); 397 } 398 399 public String fhirType() { 400 return "Dosage.doseAndRate"; 401 402 } 403 404 } 405 406 /** 407 * Indicates the order in which the dosage instructions should be applied or interpreted. 408 */ 409 @Child(name = "sequence", type = {IntegerType.class}, order=0, min=0, max=1, modifier=false, summary=true) 410 @Description(shortDefinition="The order of the dosage instructions", formalDefinition="Indicates the order in which the dosage instructions should be applied or interpreted." ) 411 protected IntegerType sequence; 412 413 /** 414 * Free text dosage instructions e.g. SIG. 415 */ 416 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 417 @Description(shortDefinition="Free text dosage instructions e.g. SIG", formalDefinition="Free text dosage instructions e.g. SIG." ) 418 protected StringType text; 419 420 /** 421 * Supplemental instructions to the patient on how to take the medication (e.g. "with meals" or"take half to one hour before food") or warnings for the patient about the medication (e.g. "may cause drowsiness" or "avoid exposure of skin to direct sunlight or sunlamps"). 422 */ 423 @Child(name = "additionalInstruction", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 424 @Description(shortDefinition="Supplemental instruction or warnings to the patient - e.g. \"with meals\", \"may cause drowsiness\"", formalDefinition="Supplemental instructions to the patient on how to take the medication (e.g. \"with meals\" or\"take half to one hour before food\") or warnings for the patient about the medication (e.g. \"may cause drowsiness\" or \"avoid exposure of skin to direct sunlight or sunlamps\")." ) 425 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/additional-instruction-codes") 426 protected List<CodeableConcept> additionalInstruction; 427 428 /** 429 * Instructions in terms that are understood by the patient or consumer. 430 */ 431 @Child(name = "patientInstruction", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 432 @Description(shortDefinition="Patient or consumer oriented instructions", formalDefinition="Instructions in terms that are understood by the patient or consumer." ) 433 protected StringType patientInstruction; 434 435 /** 436 * When medication should be administered. 437 */ 438 @Child(name = "timing", type = {Timing.class}, order=4, min=0, max=1, modifier=false, summary=true) 439 @Description(shortDefinition="When medication should be administered", formalDefinition="When medication should be administered." ) 440 protected Timing timing; 441 442 /** 443 * Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option). 444 */ 445 @Child(name = "asNeeded", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=true) 446 @Description(shortDefinition="Take \"as needed\"", formalDefinition="Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option)." ) 447 protected BooleanType asNeeded; 448 449 /** 450 * Indicates whether the Medication is only taken based on a precondition for taking the Medication (CodeableConcept). 451 */ 452 @Child(name = "asNeededFor", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 453 @Description(shortDefinition="Take \"as needed\" (for x)", formalDefinition="Indicates whether the Medication is only taken based on a precondition for taking the Medication (CodeableConcept)." ) 454 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 455 protected List<CodeableConcept> asNeededFor; 456 457 /** 458 * Body site to administer to. 459 */ 460 @Child(name = "site", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 461 @Description(shortDefinition="Body site to administer to", formalDefinition="Body site to administer to." ) 462 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/approach-site-codes") 463 protected CodeableConcept site; 464 465 /** 466 * How drug should enter body. 467 */ 468 @Child(name = "route", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 469 @Description(shortDefinition="How drug should enter body", formalDefinition="How drug should enter body." ) 470 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 471 protected CodeableConcept route; 472 473 /** 474 * Technique for administering medication. 475 */ 476 @Child(name = "method", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=true) 477 @Description(shortDefinition="Technique for administering medication", formalDefinition="Technique for administering medication." ) 478 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administration-method-codes") 479 protected CodeableConcept method; 480 481 /** 482 * Depending on the resource,this is the amount of medication administered, to be administered or typical amount to be administered. 483 */ 484 @Child(name = "doseAndRate", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 485 @Description(shortDefinition="Amount of medication administered, to be administered or typical amount to be administered", formalDefinition="Depending on the resource,this is the amount of medication administered, to be administered or typical amount to be administered." ) 486 protected List<DosageDoseAndRateComponent> doseAndRate; 487 488 /** 489 * Upper limit on medication per unit of time. 490 */ 491 @Child(name = "maxDosePerPeriod", type = {Ratio.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 492 @Description(shortDefinition="Upper limit on medication per unit of time", formalDefinition="Upper limit on medication per unit of time." ) 493 protected List<Ratio> maxDosePerPeriod; 494 495 /** 496 * Upper limit on medication per administration. 497 */ 498 @Child(name = "maxDosePerAdministration", type = {Quantity.class}, order=12, min=0, max=1, modifier=false, summary=true) 499 @Description(shortDefinition="Upper limit on medication per administration", formalDefinition="Upper limit on medication per administration." ) 500 protected Quantity maxDosePerAdministration; 501 502 /** 503 * Upper limit on medication per lifetime of the patient. 504 */ 505 @Child(name = "maxDosePerLifetime", type = {Quantity.class}, order=13, min=0, max=1, modifier=false, summary=true) 506 @Description(shortDefinition="Upper limit on medication per lifetime of the patient", formalDefinition="Upper limit on medication per lifetime of the patient." ) 507 protected Quantity maxDosePerLifetime; 508 509 private static final long serialVersionUID = 386091152L; 510 511 /** 512 * Constructor 513 */ 514 public Dosage() { 515 super(); 516 } 517 518 /** 519 * @return {@link #sequence} (Indicates the order in which the dosage instructions should be applied or interpreted.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 520 */ 521 public IntegerType getSequenceElement() { 522 if (this.sequence == null) 523 if (Configuration.errorOnAutoCreate()) 524 throw new Error("Attempt to auto-create Dosage.sequence"); 525 else if (Configuration.doAutoCreate()) 526 this.sequence = new IntegerType(); // bb 527 return this.sequence; 528 } 529 530 public boolean hasSequenceElement() { 531 return this.sequence != null && !this.sequence.isEmpty(); 532 } 533 534 public boolean hasSequence() { 535 return this.sequence != null && !this.sequence.isEmpty(); 536 } 537 538 /** 539 * @param value {@link #sequence} (Indicates the order in which the dosage instructions should be applied or interpreted.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 540 */ 541 public Dosage setSequenceElement(IntegerType value) { 542 this.sequence = value; 543 return this; 544 } 545 546 /** 547 * @return Indicates the order in which the dosage instructions should be applied or interpreted. 548 */ 549 public int getSequence() { 550 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 551 } 552 553 /** 554 * @param value Indicates the order in which the dosage instructions should be applied or interpreted. 555 */ 556 public Dosage setSequence(int value) { 557 if (this.sequence == null) 558 this.sequence = new IntegerType(); 559 this.sequence.setValue(value); 560 return this; 561 } 562 563 /** 564 * @return {@link #text} (Free text dosage instructions e.g. SIG.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 565 */ 566 public StringType getTextElement() { 567 if (this.text == null) 568 if (Configuration.errorOnAutoCreate()) 569 throw new Error("Attempt to auto-create Dosage.text"); 570 else if (Configuration.doAutoCreate()) 571 this.text = new StringType(); // bb 572 return this.text; 573 } 574 575 public boolean hasTextElement() { 576 return this.text != null && !this.text.isEmpty(); 577 } 578 579 public boolean hasText() { 580 return this.text != null && !this.text.isEmpty(); 581 } 582 583 /** 584 * @param value {@link #text} (Free text dosage instructions e.g. SIG.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 585 */ 586 public Dosage setTextElement(StringType value) { 587 this.text = value; 588 return this; 589 } 590 591 /** 592 * @return Free text dosage instructions e.g. SIG. 593 */ 594 public String getText() { 595 return this.text == null ? null : this.text.getValue(); 596 } 597 598 /** 599 * @param value Free text dosage instructions e.g. SIG. 600 */ 601 public Dosage setText(String value) { 602 if (Utilities.noString(value)) 603 this.text = null; 604 else { 605 if (this.text == null) 606 this.text = new StringType(); 607 this.text.setValue(value); 608 } 609 return this; 610 } 611 612 /** 613 * @return {@link #additionalInstruction} (Supplemental instructions to the patient on how to take the medication (e.g. "with meals" or"take half to one hour before food") or warnings for the patient about the medication (e.g. "may cause drowsiness" or "avoid exposure of skin to direct sunlight or sunlamps").) 614 */ 615 public List<CodeableConcept> getAdditionalInstruction() { 616 if (this.additionalInstruction == null) 617 this.additionalInstruction = new ArrayList<CodeableConcept>(); 618 return this.additionalInstruction; 619 } 620 621 /** 622 * @return Returns a reference to <code>this</code> for easy method chaining 623 */ 624 public Dosage setAdditionalInstruction(List<CodeableConcept> theAdditionalInstruction) { 625 this.additionalInstruction = theAdditionalInstruction; 626 return this; 627 } 628 629 public boolean hasAdditionalInstruction() { 630 if (this.additionalInstruction == null) 631 return false; 632 for (CodeableConcept item : this.additionalInstruction) 633 if (!item.isEmpty()) 634 return true; 635 return false; 636 } 637 638 public CodeableConcept addAdditionalInstruction() { //3 639 CodeableConcept t = new CodeableConcept(); 640 if (this.additionalInstruction == null) 641 this.additionalInstruction = new ArrayList<CodeableConcept>(); 642 this.additionalInstruction.add(t); 643 return t; 644 } 645 646 public Dosage addAdditionalInstruction(CodeableConcept t) { //3 647 if (t == null) 648 return this; 649 if (this.additionalInstruction == null) 650 this.additionalInstruction = new ArrayList<CodeableConcept>(); 651 this.additionalInstruction.add(t); 652 return this; 653 } 654 655 /** 656 * @return The first repetition of repeating field {@link #additionalInstruction}, creating it if it does not already exist {3} 657 */ 658 public CodeableConcept getAdditionalInstructionFirstRep() { 659 if (getAdditionalInstruction().isEmpty()) { 660 addAdditionalInstruction(); 661 } 662 return getAdditionalInstruction().get(0); 663 } 664 665 /** 666 * @return {@link #patientInstruction} (Instructions in terms that are understood by the patient or consumer.). This is the underlying object with id, value and extensions. The accessor "getPatientInstruction" gives direct access to the value 667 */ 668 public StringType getPatientInstructionElement() { 669 if (this.patientInstruction == null) 670 if (Configuration.errorOnAutoCreate()) 671 throw new Error("Attempt to auto-create Dosage.patientInstruction"); 672 else if (Configuration.doAutoCreate()) 673 this.patientInstruction = new StringType(); // bb 674 return this.patientInstruction; 675 } 676 677 public boolean hasPatientInstructionElement() { 678 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 679 } 680 681 public boolean hasPatientInstruction() { 682 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #patientInstruction} (Instructions in terms that are understood by the patient or consumer.). This is the underlying object with id, value and extensions. The accessor "getPatientInstruction" gives direct access to the value 687 */ 688 public Dosage setPatientInstructionElement(StringType value) { 689 this.patientInstruction = value; 690 return this; 691 } 692 693 /** 694 * @return Instructions in terms that are understood by the patient or consumer. 695 */ 696 public String getPatientInstruction() { 697 return this.patientInstruction == null ? null : this.patientInstruction.getValue(); 698 } 699 700 /** 701 * @param value Instructions in terms that are understood by the patient or consumer. 702 */ 703 public Dosage setPatientInstruction(String value) { 704 if (Utilities.noString(value)) 705 this.patientInstruction = null; 706 else { 707 if (this.patientInstruction == null) 708 this.patientInstruction = new StringType(); 709 this.patientInstruction.setValue(value); 710 } 711 return this; 712 } 713 714 /** 715 * @return {@link #timing} (When medication should be administered.) 716 */ 717 public Timing getTiming() { 718 if (this.timing == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create Dosage.timing"); 721 else if (Configuration.doAutoCreate()) 722 this.timing = new Timing(); // cc 723 return this.timing; 724 } 725 726 public boolean hasTiming() { 727 return this.timing != null && !this.timing.isEmpty(); 728 } 729 730 /** 731 * @param value {@link #timing} (When medication should be administered.) 732 */ 733 public Dosage setTiming(Timing value) { 734 this.timing = value; 735 return this; 736 } 737 738 /** 739 * @return {@link #asNeeded} (Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option).). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 740 */ 741 public BooleanType getAsNeededElement() { 742 if (this.asNeeded == null) 743 if (Configuration.errorOnAutoCreate()) 744 throw new Error("Attempt to auto-create Dosage.asNeeded"); 745 else if (Configuration.doAutoCreate()) 746 this.asNeeded = new BooleanType(); // bb 747 return this.asNeeded; 748 } 749 750 public boolean hasAsNeededElement() { 751 return this.asNeeded != null && !this.asNeeded.isEmpty(); 752 } 753 754 public boolean hasAsNeeded() { 755 return this.asNeeded != null && !this.asNeeded.isEmpty(); 756 } 757 758 /** 759 * @param value {@link #asNeeded} (Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option).). This is the underlying object with id, value and extensions. The accessor "getAsNeeded" gives direct access to the value 760 */ 761 public Dosage setAsNeededElement(BooleanType value) { 762 this.asNeeded = value; 763 return this; 764 } 765 766 /** 767 * @return Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option). 768 */ 769 public boolean getAsNeeded() { 770 return this.asNeeded == null || this.asNeeded.isEmpty() ? false : this.asNeeded.getValue(); 771 } 772 773 /** 774 * @param value Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option). 775 */ 776 public Dosage setAsNeeded(boolean value) { 777 if (this.asNeeded == null) 778 this.asNeeded = new BooleanType(); 779 this.asNeeded.setValue(value); 780 return this; 781 } 782 783 /** 784 * @return {@link #asNeededFor} (Indicates whether the Medication is only taken based on a precondition for taking the Medication (CodeableConcept).) 785 */ 786 public List<CodeableConcept> getAsNeededFor() { 787 if (this.asNeededFor == null) 788 this.asNeededFor = new ArrayList<CodeableConcept>(); 789 return this.asNeededFor; 790 } 791 792 /** 793 * @return Returns a reference to <code>this</code> for easy method chaining 794 */ 795 public Dosage setAsNeededFor(List<CodeableConcept> theAsNeededFor) { 796 this.asNeededFor = theAsNeededFor; 797 return this; 798 } 799 800 public boolean hasAsNeededFor() { 801 if (this.asNeededFor == null) 802 return false; 803 for (CodeableConcept item : this.asNeededFor) 804 if (!item.isEmpty()) 805 return true; 806 return false; 807 } 808 809 public CodeableConcept addAsNeededFor() { //3 810 CodeableConcept t = new CodeableConcept(); 811 if (this.asNeededFor == null) 812 this.asNeededFor = new ArrayList<CodeableConcept>(); 813 this.asNeededFor.add(t); 814 return t; 815 } 816 817 public Dosage addAsNeededFor(CodeableConcept t) { //3 818 if (t == null) 819 return this; 820 if (this.asNeededFor == null) 821 this.asNeededFor = new ArrayList<CodeableConcept>(); 822 this.asNeededFor.add(t); 823 return this; 824 } 825 826 /** 827 * @return The first repetition of repeating field {@link #asNeededFor}, creating it if it does not already exist {3} 828 */ 829 public CodeableConcept getAsNeededForFirstRep() { 830 if (getAsNeededFor().isEmpty()) { 831 addAsNeededFor(); 832 } 833 return getAsNeededFor().get(0); 834 } 835 836 /** 837 * @return {@link #site} (Body site to administer to.) 838 */ 839 public CodeableConcept getSite() { 840 if (this.site == null) 841 if (Configuration.errorOnAutoCreate()) 842 throw new Error("Attempt to auto-create Dosage.site"); 843 else if (Configuration.doAutoCreate()) 844 this.site = new CodeableConcept(); // cc 845 return this.site; 846 } 847 848 public boolean hasSite() { 849 return this.site != null && !this.site.isEmpty(); 850 } 851 852 /** 853 * @param value {@link #site} (Body site to administer to.) 854 */ 855 public Dosage setSite(CodeableConcept value) { 856 this.site = value; 857 return this; 858 } 859 860 /** 861 * @return {@link #route} (How drug should enter body.) 862 */ 863 public CodeableConcept getRoute() { 864 if (this.route == null) 865 if (Configuration.errorOnAutoCreate()) 866 throw new Error("Attempt to auto-create Dosage.route"); 867 else if (Configuration.doAutoCreate()) 868 this.route = new CodeableConcept(); // cc 869 return this.route; 870 } 871 872 public boolean hasRoute() { 873 return this.route != null && !this.route.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #route} (How drug should enter body.) 878 */ 879 public Dosage setRoute(CodeableConcept value) { 880 this.route = value; 881 return this; 882 } 883 884 /** 885 * @return {@link #method} (Technique for administering medication.) 886 */ 887 public CodeableConcept getMethod() { 888 if (this.method == null) 889 if (Configuration.errorOnAutoCreate()) 890 throw new Error("Attempt to auto-create Dosage.method"); 891 else if (Configuration.doAutoCreate()) 892 this.method = new CodeableConcept(); // cc 893 return this.method; 894 } 895 896 public boolean hasMethod() { 897 return this.method != null && !this.method.isEmpty(); 898 } 899 900 /** 901 * @param value {@link #method} (Technique for administering medication.) 902 */ 903 public Dosage setMethod(CodeableConcept value) { 904 this.method = value; 905 return this; 906 } 907 908 /** 909 * @return {@link #doseAndRate} (Depending on the resource,this is the amount of medication administered, to be administered or typical amount to be administered.) 910 */ 911 public List<DosageDoseAndRateComponent> getDoseAndRate() { 912 if (this.doseAndRate == null) 913 this.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 914 return this.doseAndRate; 915 } 916 917 /** 918 * @return Returns a reference to <code>this</code> for easy method chaining 919 */ 920 public Dosage setDoseAndRate(List<DosageDoseAndRateComponent> theDoseAndRate) { 921 this.doseAndRate = theDoseAndRate; 922 return this; 923 } 924 925 public boolean hasDoseAndRate() { 926 if (this.doseAndRate == null) 927 return false; 928 for (DosageDoseAndRateComponent item : this.doseAndRate) 929 if (!item.isEmpty()) 930 return true; 931 return false; 932 } 933 934 public DosageDoseAndRateComponent addDoseAndRate() { //3 935 DosageDoseAndRateComponent t = new DosageDoseAndRateComponent(); 936 if (this.doseAndRate == null) 937 this.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 938 this.doseAndRate.add(t); 939 return t; 940 } 941 942 public Dosage addDoseAndRate(DosageDoseAndRateComponent t) { //3 943 if (t == null) 944 return this; 945 if (this.doseAndRate == null) 946 this.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 947 this.doseAndRate.add(t); 948 return this; 949 } 950 951 /** 952 * @return The first repetition of repeating field {@link #doseAndRate}, creating it if it does not already exist {3} 953 */ 954 public DosageDoseAndRateComponent getDoseAndRateFirstRep() { 955 if (getDoseAndRate().isEmpty()) { 956 addDoseAndRate(); 957 } 958 return getDoseAndRate().get(0); 959 } 960 961 /** 962 * @return {@link #maxDosePerPeriod} (Upper limit on medication per unit of time.) 963 */ 964 public List<Ratio> getMaxDosePerPeriod() { 965 if (this.maxDosePerPeriod == null) 966 this.maxDosePerPeriod = new ArrayList<Ratio>(); 967 return this.maxDosePerPeriod; 968 } 969 970 /** 971 * @return Returns a reference to <code>this</code> for easy method chaining 972 */ 973 public Dosage setMaxDosePerPeriod(List<Ratio> theMaxDosePerPeriod) { 974 this.maxDosePerPeriod = theMaxDosePerPeriod; 975 return this; 976 } 977 978 public boolean hasMaxDosePerPeriod() { 979 if (this.maxDosePerPeriod == null) 980 return false; 981 for (Ratio item : this.maxDosePerPeriod) 982 if (!item.isEmpty()) 983 return true; 984 return false; 985 } 986 987 public Ratio addMaxDosePerPeriod() { //3 988 Ratio t = new Ratio(); 989 if (this.maxDosePerPeriod == null) 990 this.maxDosePerPeriod = new ArrayList<Ratio>(); 991 this.maxDosePerPeriod.add(t); 992 return t; 993 } 994 995 public Dosage addMaxDosePerPeriod(Ratio t) { //3 996 if (t == null) 997 return this; 998 if (this.maxDosePerPeriod == null) 999 this.maxDosePerPeriod = new ArrayList<Ratio>(); 1000 this.maxDosePerPeriod.add(t); 1001 return this; 1002 } 1003 1004 /** 1005 * @return The first repetition of repeating field {@link #maxDosePerPeriod}, creating it if it does not already exist {3} 1006 */ 1007 public Ratio getMaxDosePerPeriodFirstRep() { 1008 if (getMaxDosePerPeriod().isEmpty()) { 1009 addMaxDosePerPeriod(); 1010 } 1011 return getMaxDosePerPeriod().get(0); 1012 } 1013 1014 /** 1015 * @return {@link #maxDosePerAdministration} (Upper limit on medication per administration.) 1016 */ 1017 public Quantity getMaxDosePerAdministration() { 1018 if (this.maxDosePerAdministration == null) 1019 if (Configuration.errorOnAutoCreate()) 1020 throw new Error("Attempt to auto-create Dosage.maxDosePerAdministration"); 1021 else if (Configuration.doAutoCreate()) 1022 this.maxDosePerAdministration = new Quantity(); // cc 1023 return this.maxDosePerAdministration; 1024 } 1025 1026 public boolean hasMaxDosePerAdministration() { 1027 return this.maxDosePerAdministration != null && !this.maxDosePerAdministration.isEmpty(); 1028 } 1029 1030 /** 1031 * @param value {@link #maxDosePerAdministration} (Upper limit on medication per administration.) 1032 */ 1033 public Dosage setMaxDosePerAdministration(Quantity value) { 1034 this.maxDosePerAdministration = value; 1035 return this; 1036 } 1037 1038 /** 1039 * @return {@link #maxDosePerLifetime} (Upper limit on medication per lifetime of the patient.) 1040 */ 1041 public Quantity getMaxDosePerLifetime() { 1042 if (this.maxDosePerLifetime == null) 1043 if (Configuration.errorOnAutoCreate()) 1044 throw new Error("Attempt to auto-create Dosage.maxDosePerLifetime"); 1045 else if (Configuration.doAutoCreate()) 1046 this.maxDosePerLifetime = new Quantity(); // cc 1047 return this.maxDosePerLifetime; 1048 } 1049 1050 public boolean hasMaxDosePerLifetime() { 1051 return this.maxDosePerLifetime != null && !this.maxDosePerLifetime.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #maxDosePerLifetime} (Upper limit on medication per lifetime of the patient.) 1056 */ 1057 public Dosage setMaxDosePerLifetime(Quantity value) { 1058 this.maxDosePerLifetime = value; 1059 return this; 1060 } 1061 1062 protected void listChildren(List<Property> children) { 1063 super.listChildren(children); 1064 children.add(new Property("sequence", "integer", "Indicates the order in which the dosage instructions should be applied or interpreted.", 0, 1, sequence)); 1065 children.add(new Property("text", "string", "Free text dosage instructions e.g. SIG.", 0, 1, text)); 1066 children.add(new Property("additionalInstruction", "CodeableConcept", "Supplemental instructions to the patient on how to take the medication (e.g. \"with meals\" or\"take half to one hour before food\") or warnings for the patient about the medication (e.g. \"may cause drowsiness\" or \"avoid exposure of skin to direct sunlight or sunlamps\").", 0, java.lang.Integer.MAX_VALUE, additionalInstruction)); 1067 children.add(new Property("patientInstruction", "string", "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction)); 1068 children.add(new Property("timing", "Timing", "When medication should be administered.", 0, 1, timing)); 1069 children.add(new Property("asNeeded", "boolean", "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option).", 0, 1, asNeeded)); 1070 children.add(new Property("asNeededFor", "CodeableConcept", "Indicates whether the Medication is only taken based on a precondition for taking the Medication (CodeableConcept).", 0, java.lang.Integer.MAX_VALUE, asNeededFor)); 1071 children.add(new Property("site", "CodeableConcept", "Body site to administer to.", 0, 1, site)); 1072 children.add(new Property("route", "CodeableConcept", "How drug should enter body.", 0, 1, route)); 1073 children.add(new Property("method", "CodeableConcept", "Technique for administering medication.", 0, 1, method)); 1074 children.add(new Property("doseAndRate", "", "Depending on the resource,this is the amount of medication administered, to be administered or typical amount to be administered.", 0, java.lang.Integer.MAX_VALUE, doseAndRate)); 1075 children.add(new Property("maxDosePerPeriod", "Ratio", "Upper limit on medication per unit of time.", 0, java.lang.Integer.MAX_VALUE, maxDosePerPeriod)); 1076 children.add(new Property("maxDosePerAdministration", "Quantity", "Upper limit on medication per administration.", 0, 1, maxDosePerAdministration)); 1077 children.add(new Property("maxDosePerLifetime", "Quantity", "Upper limit on medication per lifetime of the patient.", 0, 1, maxDosePerLifetime)); 1078 } 1079 1080 @Override 1081 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1082 switch (_hash) { 1083 case 1349547969: /*sequence*/ return new Property("sequence", "integer", "Indicates the order in which the dosage instructions should be applied or interpreted.", 0, 1, sequence); 1084 case 3556653: /*text*/ return new Property("text", "string", "Free text dosage instructions e.g. SIG.", 0, 1, text); 1085 case 1623641575: /*additionalInstruction*/ return new Property("additionalInstruction", "CodeableConcept", "Supplemental instructions to the patient on how to take the medication (e.g. \"with meals\" or\"take half to one hour before food\") or warnings for the patient about the medication (e.g. \"may cause drowsiness\" or \"avoid exposure of skin to direct sunlight or sunlamps\").", 0, java.lang.Integer.MAX_VALUE, additionalInstruction); 1086 case 737543241: /*patientInstruction*/ return new Property("patientInstruction", "string", "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction); 1087 case -873664438: /*timing*/ return new Property("timing", "Timing", "When medication should be administered.", 0, 1, timing); 1088 case -1432923513: /*asNeeded*/ return new Property("asNeeded", "boolean", "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option).", 0, 1, asNeeded); 1089 case -544350014: /*asNeededFor*/ return new Property("asNeededFor", "CodeableConcept", "Indicates whether the Medication is only taken based on a precondition for taking the Medication (CodeableConcept).", 0, java.lang.Integer.MAX_VALUE, asNeededFor); 1090 case 3530567: /*site*/ return new Property("site", "CodeableConcept", "Body site to administer to.", 0, 1, site); 1091 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "How drug should enter body.", 0, 1, route); 1092 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Technique for administering medication.", 0, 1, method); 1093 case -611024774: /*doseAndRate*/ return new Property("doseAndRate", "", "Depending on the resource,this is the amount of medication administered, to be administered or typical amount to be administered.", 0, java.lang.Integer.MAX_VALUE, doseAndRate); 1094 case 1506263709: /*maxDosePerPeriod*/ return new Property("maxDosePerPeriod", "Ratio", "Upper limit on medication per unit of time.", 0, java.lang.Integer.MAX_VALUE, maxDosePerPeriod); 1095 case 2004889914: /*maxDosePerAdministration*/ return new Property("maxDosePerAdministration", "Quantity", "Upper limit on medication per administration.", 0, 1, maxDosePerAdministration); 1096 case 642099621: /*maxDosePerLifetime*/ return new Property("maxDosePerLifetime", "Quantity", "Upper limit on medication per lifetime of the patient.", 0, 1, maxDosePerLifetime); 1097 default: return super.getNamedProperty(_hash, _name, _checkValid); 1098 } 1099 1100 } 1101 1102 @Override 1103 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1104 switch (hash) { 1105 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // IntegerType 1106 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 1107 case 1623641575: /*additionalInstruction*/ return this.additionalInstruction == null ? new Base[0] : this.additionalInstruction.toArray(new Base[this.additionalInstruction.size()]); // CodeableConcept 1108 case 737543241: /*patientInstruction*/ return this.patientInstruction == null ? new Base[0] : new Base[] {this.patientInstruction}; // StringType 1109 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Timing 1110 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // BooleanType 1111 case -544350014: /*asNeededFor*/ return this.asNeededFor == null ? new Base[0] : this.asNeededFor.toArray(new Base[this.asNeededFor.size()]); // CodeableConcept 1112 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // CodeableConcept 1113 case 108704329: /*route*/ return this.route == null ? new Base[0] : new Base[] {this.route}; // CodeableConcept 1114 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 1115 case -611024774: /*doseAndRate*/ return this.doseAndRate == null ? new Base[0] : this.doseAndRate.toArray(new Base[this.doseAndRate.size()]); // DosageDoseAndRateComponent 1116 case 1506263709: /*maxDosePerPeriod*/ return this.maxDosePerPeriod == null ? new Base[0] : this.maxDosePerPeriod.toArray(new Base[this.maxDosePerPeriod.size()]); // Ratio 1117 case 2004889914: /*maxDosePerAdministration*/ return this.maxDosePerAdministration == null ? new Base[0] : new Base[] {this.maxDosePerAdministration}; // Quantity 1118 case 642099621: /*maxDosePerLifetime*/ return this.maxDosePerLifetime == null ? new Base[0] : new Base[] {this.maxDosePerLifetime}; // Quantity 1119 default: return super.getProperty(hash, name, checkValid); 1120 } 1121 1122 } 1123 1124 @Override 1125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1126 switch (hash) { 1127 case 1349547969: // sequence 1128 this.sequence = TypeConvertor.castToInteger(value); // IntegerType 1129 return value; 1130 case 3556653: // text 1131 this.text = TypeConvertor.castToString(value); // StringType 1132 return value; 1133 case 1623641575: // additionalInstruction 1134 this.getAdditionalInstruction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1135 return value; 1136 case 737543241: // patientInstruction 1137 this.patientInstruction = TypeConvertor.castToString(value); // StringType 1138 return value; 1139 case -873664438: // timing 1140 this.timing = TypeConvertor.castToTiming(value); // Timing 1141 return value; 1142 case -1432923513: // asNeeded 1143 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 1144 return value; 1145 case -544350014: // asNeededFor 1146 this.getAsNeededFor().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1147 return value; 1148 case 3530567: // site 1149 this.site = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1150 return value; 1151 case 108704329: // route 1152 this.route = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1153 return value; 1154 case -1077554975: // method 1155 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1156 return value; 1157 case -611024774: // doseAndRate 1158 this.getDoseAndRate().add((DosageDoseAndRateComponent) value); // DosageDoseAndRateComponent 1159 return value; 1160 case 1506263709: // maxDosePerPeriod 1161 this.getMaxDosePerPeriod().add(TypeConvertor.castToRatio(value)); // Ratio 1162 return value; 1163 case 2004889914: // maxDosePerAdministration 1164 this.maxDosePerAdministration = TypeConvertor.castToQuantity(value); // Quantity 1165 return value; 1166 case 642099621: // maxDosePerLifetime 1167 this.maxDosePerLifetime = TypeConvertor.castToQuantity(value); // Quantity 1168 return value; 1169 default: return super.setProperty(hash, name, value); 1170 } 1171 1172 } 1173 1174 @Override 1175 public Base setProperty(String name, Base value) throws FHIRException { 1176 if (name.equals("sequence")) { 1177 this.sequence = TypeConvertor.castToInteger(value); // IntegerType 1178 } else if (name.equals("text")) { 1179 this.text = TypeConvertor.castToString(value); // StringType 1180 } else if (name.equals("additionalInstruction")) { 1181 this.getAdditionalInstruction().add(TypeConvertor.castToCodeableConcept(value)); 1182 } else if (name.equals("patientInstruction")) { 1183 this.patientInstruction = TypeConvertor.castToString(value); // StringType 1184 } else if (name.equals("timing")) { 1185 this.timing = TypeConvertor.castToTiming(value); // Timing 1186 } else if (name.equals("asNeeded")) { 1187 this.asNeeded = TypeConvertor.castToBoolean(value); // BooleanType 1188 } else if (name.equals("asNeededFor")) { 1189 this.getAsNeededFor().add(TypeConvertor.castToCodeableConcept(value)); 1190 } else if (name.equals("site")) { 1191 this.site = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1192 } else if (name.equals("route")) { 1193 this.route = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1194 } else if (name.equals("method")) { 1195 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1196 } else if (name.equals("doseAndRate")) { 1197 this.getDoseAndRate().add((DosageDoseAndRateComponent) value); 1198 } else if (name.equals("maxDosePerPeriod")) { 1199 this.getMaxDosePerPeriod().add(TypeConvertor.castToRatio(value)); 1200 } else if (name.equals("maxDosePerAdministration")) { 1201 this.maxDosePerAdministration = TypeConvertor.castToQuantity(value); // Quantity 1202 } else if (name.equals("maxDosePerLifetime")) { 1203 this.maxDosePerLifetime = TypeConvertor.castToQuantity(value); // Quantity 1204 } else 1205 return super.setProperty(name, value); 1206 return value; 1207 } 1208 1209 @Override 1210 public void removeChild(String name, Base value) throws FHIRException { 1211 if (name.equals("sequence")) { 1212 this.sequence = null; 1213 } else if (name.equals("text")) { 1214 this.text = null; 1215 } else if (name.equals("additionalInstruction")) { 1216 this.getAdditionalInstruction().remove(value); 1217 } else if (name.equals("patientInstruction")) { 1218 this.patientInstruction = null; 1219 } else if (name.equals("timing")) { 1220 this.timing = null; 1221 } else if (name.equals("asNeeded")) { 1222 this.asNeeded = null; 1223 } else if (name.equals("asNeededFor")) { 1224 this.getAsNeededFor().remove(value); 1225 } else if (name.equals("site")) { 1226 this.site = null; 1227 } else if (name.equals("route")) { 1228 this.route = null; 1229 } else if (name.equals("method")) { 1230 this.method = null; 1231 } else if (name.equals("doseAndRate")) { 1232 this.getDoseAndRate().remove((DosageDoseAndRateComponent) value); 1233 } else if (name.equals("maxDosePerPeriod")) { 1234 this.getMaxDosePerPeriod().remove(value); 1235 } else if (name.equals("maxDosePerAdministration")) { 1236 this.maxDosePerAdministration = null; 1237 } else if (name.equals("maxDosePerLifetime")) { 1238 this.maxDosePerLifetime = null; 1239 } else 1240 super.removeChild(name, value); 1241 1242 } 1243 1244 @Override 1245 public Base makeProperty(int hash, String name) throws FHIRException { 1246 switch (hash) { 1247 case 1349547969: return getSequenceElement(); 1248 case 3556653: return getTextElement(); 1249 case 1623641575: return addAdditionalInstruction(); 1250 case 737543241: return getPatientInstructionElement(); 1251 case -873664438: return getTiming(); 1252 case -1432923513: return getAsNeededElement(); 1253 case -544350014: return addAsNeededFor(); 1254 case 3530567: return getSite(); 1255 case 108704329: return getRoute(); 1256 case -1077554975: return getMethod(); 1257 case -611024774: return addDoseAndRate(); 1258 case 1506263709: return addMaxDosePerPeriod(); 1259 case 2004889914: return getMaxDosePerAdministration(); 1260 case 642099621: return getMaxDosePerLifetime(); 1261 default: return super.makeProperty(hash, name); 1262 } 1263 1264 } 1265 1266 @Override 1267 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1268 switch (hash) { 1269 case 1349547969: /*sequence*/ return new String[] {"integer"}; 1270 case 3556653: /*text*/ return new String[] {"string"}; 1271 case 1623641575: /*additionalInstruction*/ return new String[] {"CodeableConcept"}; 1272 case 737543241: /*patientInstruction*/ return new String[] {"string"}; 1273 case -873664438: /*timing*/ return new String[] {"Timing"}; 1274 case -1432923513: /*asNeeded*/ return new String[] {"boolean"}; 1275 case -544350014: /*asNeededFor*/ return new String[] {"CodeableConcept"}; 1276 case 3530567: /*site*/ return new String[] {"CodeableConcept"}; 1277 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 1278 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1279 case -611024774: /*doseAndRate*/ return new String[] {}; 1280 case 1506263709: /*maxDosePerPeriod*/ return new String[] {"Ratio"}; 1281 case 2004889914: /*maxDosePerAdministration*/ return new String[] {"Quantity"}; 1282 case 642099621: /*maxDosePerLifetime*/ return new String[] {"Quantity"}; 1283 default: return super.getTypesForProperty(hash, name); 1284 } 1285 1286 } 1287 1288 @Override 1289 public Base addChild(String name) throws FHIRException { 1290 if (name.equals("sequence")) { 1291 throw new FHIRException("Cannot call addChild on a singleton property Dosage.sequence"); 1292 } 1293 else if (name.equals("text")) { 1294 throw new FHIRException("Cannot call addChild on a singleton property Dosage.text"); 1295 } 1296 else if (name.equals("additionalInstruction")) { 1297 return addAdditionalInstruction(); 1298 } 1299 else if (name.equals("patientInstruction")) { 1300 throw new FHIRException("Cannot call addChild on a singleton property Dosage.patientInstruction"); 1301 } 1302 else if (name.equals("timing")) { 1303 this.timing = new Timing(); 1304 return this.timing; 1305 } 1306 else if (name.equals("asNeeded")) { 1307 throw new FHIRException("Cannot call addChild on a singleton property Dosage.asNeeded"); 1308 } 1309 else if (name.equals("asNeededFor")) { 1310 return addAsNeededFor(); 1311 } 1312 else if (name.equals("site")) { 1313 this.site = new CodeableConcept(); 1314 return this.site; 1315 } 1316 else if (name.equals("route")) { 1317 this.route = new CodeableConcept(); 1318 return this.route; 1319 } 1320 else if (name.equals("method")) { 1321 this.method = new CodeableConcept(); 1322 return this.method; 1323 } 1324 else if (name.equals("doseAndRate")) { 1325 return addDoseAndRate(); 1326 } 1327 else if (name.equals("maxDosePerPeriod")) { 1328 return addMaxDosePerPeriod(); 1329 } 1330 else if (name.equals("maxDosePerAdministration")) { 1331 this.maxDosePerAdministration = new Quantity(); 1332 return this.maxDosePerAdministration; 1333 } 1334 else if (name.equals("maxDosePerLifetime")) { 1335 this.maxDosePerLifetime = new Quantity(); 1336 return this.maxDosePerLifetime; 1337 } 1338 else 1339 return super.addChild(name); 1340 } 1341 1342 public String fhirType() { 1343 return "Dosage"; 1344 1345 } 1346 1347 public Dosage copy() { 1348 Dosage dst = new Dosage(); 1349 copyValues(dst); 1350 return dst; 1351 } 1352 1353 public void copyValues(Dosage dst) { 1354 super.copyValues(dst); 1355 dst.sequence = sequence == null ? null : sequence.copy(); 1356 dst.text = text == null ? null : text.copy(); 1357 if (additionalInstruction != null) { 1358 dst.additionalInstruction = new ArrayList<CodeableConcept>(); 1359 for (CodeableConcept i : additionalInstruction) 1360 dst.additionalInstruction.add(i.copy()); 1361 }; 1362 dst.patientInstruction = patientInstruction == null ? null : patientInstruction.copy(); 1363 dst.timing = timing == null ? null : timing.copy(); 1364 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 1365 if (asNeededFor != null) { 1366 dst.asNeededFor = new ArrayList<CodeableConcept>(); 1367 for (CodeableConcept i : asNeededFor) 1368 dst.asNeededFor.add(i.copy()); 1369 }; 1370 dst.site = site == null ? null : site.copy(); 1371 dst.route = route == null ? null : route.copy(); 1372 dst.method = method == null ? null : method.copy(); 1373 if (doseAndRate != null) { 1374 dst.doseAndRate = new ArrayList<DosageDoseAndRateComponent>(); 1375 for (DosageDoseAndRateComponent i : doseAndRate) 1376 dst.doseAndRate.add(i.copy()); 1377 }; 1378 if (maxDosePerPeriod != null) { 1379 dst.maxDosePerPeriod = new ArrayList<Ratio>(); 1380 for (Ratio i : maxDosePerPeriod) 1381 dst.maxDosePerPeriod.add(i.copy()); 1382 }; 1383 dst.maxDosePerAdministration = maxDosePerAdministration == null ? null : maxDosePerAdministration.copy(); 1384 dst.maxDosePerLifetime = maxDosePerLifetime == null ? null : maxDosePerLifetime.copy(); 1385 } 1386 1387 protected Dosage typedCopy() { 1388 return copy(); 1389 } 1390 1391 @Override 1392 public boolean equalsDeep(Base other_) { 1393 if (!super.equalsDeep(other_)) 1394 return false; 1395 if (!(other_ instanceof Dosage)) 1396 return false; 1397 Dosage o = (Dosage) other_; 1398 return compareDeep(sequence, o.sequence, true) && compareDeep(text, o.text, true) && compareDeep(additionalInstruction, o.additionalInstruction, true) 1399 && compareDeep(patientInstruction, o.patientInstruction, true) && compareDeep(timing, o.timing, true) 1400 && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(asNeededFor, o.asNeededFor, true) && compareDeep(site, o.site, true) 1401 && compareDeep(route, o.route, true) && compareDeep(method, o.method, true) && compareDeep(doseAndRate, o.doseAndRate, true) 1402 && compareDeep(maxDosePerPeriod, o.maxDosePerPeriod, true) && compareDeep(maxDosePerAdministration, o.maxDosePerAdministration, true) 1403 && compareDeep(maxDosePerLifetime, o.maxDosePerLifetime, true); 1404 } 1405 1406 @Override 1407 public boolean equalsShallow(Base other_) { 1408 if (!super.equalsShallow(other_)) 1409 return false; 1410 if (!(other_ instanceof Dosage)) 1411 return false; 1412 Dosage o = (Dosage) other_; 1413 return compareValues(sequence, o.sequence, true) && compareValues(text, o.text, true) && compareValues(patientInstruction, o.patientInstruction, true) 1414 && compareValues(asNeeded, o.asNeeded, true); 1415 } 1416 1417 public boolean isEmpty() { 1418 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, text, additionalInstruction 1419 , patientInstruction, timing, asNeeded, asNeededFor, site, route, method, doseAndRate 1420 , maxDosePerPeriod, maxDosePerAdministration, maxDosePerLifetime); 1421 } 1422 1423 1424} 1425