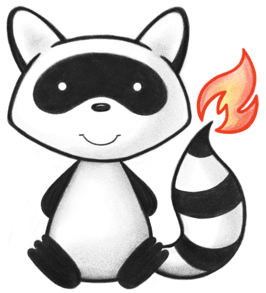
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import org.hl7.fhir.instance.model.api.IBaseElement; 049import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 050import org.hl7.fhir.r5.utils.ToolingExtensions; 051import org.hl7.fhir.utilities.FhirPublication; 052import org.hl7.fhir.utilities.StandardsStatus; 053/** 054 * Element Type: Base definition for all elements in a resource. 055 */ 056@DatatypeDef(name="Element") 057public abstract class Element extends Base implements IBaseHasExtensions, IBaseElement { 058 059 /** 060 * Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces. 061 */ 062 @Child(name = "id", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=false) 063 @Description(shortDefinition="Unique id for inter-element referencing", formalDefinition="Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces." ) 064 protected StringType id; 065 066 /** 067 * May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. 068 */ 069 @Child(name = "extension", type = {Extension.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 070 @Description(shortDefinition="Additional content defined by implementations", formalDefinition="May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension." ) 071 protected List<Extension> extension; 072 073 private static final long serialVersionUID = -1452745816L; 074 075 /** 076 * Constructor 077 */ 078 public Element() { 079 super(); 080 } 081 082 /** 083 * @return {@link #id} (Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 084 */ 085 public StringType getIdElement() { 086 if (this.id == null) 087 if (Configuration.errorOnAutoCreate()) 088 throw new Error("Attempt to auto-create Element.id"); 089 else if (Configuration.doAutoCreate()) 090 this.id = new StringType(); // bb 091 return this.id; 092 } 093 094 public boolean hasIdElement() { 095 return this.id != null && !this.id.isEmpty(); 096 } 097 098 public boolean hasId() { 099 return this.id != null && !this.id.isEmpty(); 100 } 101 102 /** 103 * @param value {@link #id} (Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 104 */ 105 public Element setIdElement(StringType value) { 106 this.id = value; 107 return this; 108 } 109 110 /** 111 * @return Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces. 112 */ 113 public String getId() { 114 return this.id == null ? null : this.id.getValue(); 115 } 116 117 /** 118 * @param value Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces. 119 */ 120 public Element setId(String value) { 121 if (Utilities.noString(value)) 122 this.id = null; 123 else { 124 if (this.id == null) 125 this.id = new StringType(); 126 this.id.setValue(value); 127 } 128 return this; 129 } 130 131 /** 132 * @return {@link #extension} (May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.) 133 */ 134 public List<Extension> getExtension() { 135 if (this.extension == null) 136 this.extension = new ArrayList<Extension>(); 137 return this.extension; 138 } 139 140 /** 141 * @return Returns a reference to <code>this</code> for easy method chaining 142 */ 143 public Element setExtension(List<Extension> theExtension) { 144 this.extension = theExtension; 145 return this; 146 } 147 148 public boolean hasExtension() { 149 if (this.extension == null) 150 return false; 151 for (Extension item : this.extension) 152 if (!item.isEmpty()) 153 return true; 154 return false; 155 } 156 157 public Extension addExtension() { //3 158 Extension t = new Extension(); 159 if (this.extension == null) 160 this.extension = new ArrayList<Extension>(); 161 this.extension.add(t); 162 return t; 163 } 164 165 public Element addExtension(Extension t) { //3 166 if (t == null) 167 return this; 168 if (this.extension == null) 169 this.extension = new ArrayList<Extension>(); 170 this.extension.add(t); 171 return this; 172 } 173 174 /** 175 * @return The first repetition of repeating field {@link #extension}, creating it if it does not already exist {3} 176 */ 177 public Extension getExtensionFirstRep() { 178 if (getExtension().isEmpty()) { 179 addExtension(); 180 } 181 return getExtension().get(0); 182 } 183 184 protected void listChildren(List<Property> children) { 185 super.listChildren(children); 186 children.add(new Property("id", "string", "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 0, 1, id)); 187 children.add(new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension)); 188 } 189 190 @Override 191 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 192 switch (_hash) { 193 case 3355: /*id*/ return new Property("id", "string", "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 0, 1, id); 194 case -612557761: /*extension*/ return new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension); 195 default: return super.getNamedProperty(_hash, _name, _checkValid); 196 } 197 198 } 199 200 @Override 201 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 202 switch (hash) { 203 case 3355: /*id*/ return this.id == null ? new Base[0] : new Base[] {this.id}; // StringType 204 case -612557761: /*extension*/ return this.extension == null ? new Base[0] : this.extension.toArray(new Base[this.extension.size()]); // Extension 205 default: return super.getProperty(hash, name, checkValid); 206 } 207 208 } 209 210 @Override 211 public Base setProperty(int hash, String name, Base value) throws FHIRException { 212 switch (hash) { 213 case 3355: // id 214 this.id = TypeConvertor.castToString(value); // StringType 215 return value; 216 case -612557761: // extension 217 this.getExtension().add(TypeConvertor.castToExtension(value)); // Extension 218 return value; 219 default: return super.setProperty(hash, name, value); 220 } 221 222 } 223 224 @Override 225 public Base setProperty(String name, Base value) throws FHIRException { 226 if (name.equals("id")) { 227 this.id = TypeConvertor.castToString(value); // StringType 228 } else if (name.equals("extension")) { 229 this.getExtension().add(TypeConvertor.castToExtension(value)); 230 } else 231 return super.setProperty(name, value); 232 return value; 233 } 234 235 @Override 236 public void removeChild(String name, Base value) throws FHIRException { 237 if (name.equals("id")) { 238 this.id = null; 239 } else if (name.equals("extension")) { 240 this.getExtension().remove(value); 241 } else 242 super.removeChild(name, value); 243 244 } 245 246 @Override 247 public Base makeProperty(int hash, String name) throws FHIRException { 248 switch (hash) { 249 case 3355: return getIdElement(); 250 case -612557761: return addExtension(); 251 default: return super.makeProperty(hash, name); 252 } 253 254 } 255 256 @Override 257 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 258 switch (hash) { 259 case 3355: /*id*/ return new String[] {"string"}; 260 case -612557761: /*extension*/ return new String[] {"Extension"}; 261 default: return super.getTypesForProperty(hash, name); 262 } 263 264 } 265 266 @Override 267 public Base addChild(String name) throws FHIRException { 268 if (name.equals("id")) { 269 throw new FHIRException("Cannot call addChild on a singleton property Element.id"); 270 } 271 else if (name.equals("extension")) { 272 return addExtension(); 273 } 274 else 275 return super.addChild(name); 276 } 277 278 public String fhirType() { 279 return "Element"; 280 281 } 282 283 public abstract Element copy(); 284 285 public void copyValues(Element dst) { 286 super.copyValues(dst); 287 dst.id = id == null ? null : id.copy(); 288 if (extension != null) { 289 dst.extension = new ArrayList<Extension>(); 290 for (Extension i : extension) 291 dst.extension.add(i.copy()); 292 }; 293 } 294 295 @Override 296 public boolean equalsDeep(Base other_) { 297 if (!super.equalsDeep(other_)) 298 return false; 299 if (!(other_ instanceof Element)) 300 return false; 301 Element o = (Element) other_; 302 return compareDeep(id, o.id, true) && compareDeep(extension, o.extension, true); 303 } 304 305 @Override 306 public boolean equalsShallow(Base other_) { 307 if (!super.equalsShallow(other_)) 308 return false; 309 if (!(other_ instanceof Element)) 310 return false; 311 Element o = (Element) other_; 312 return compareValues(id, o.id, true); 313 } 314 315 public boolean isEmpty() { 316 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(id, extension); 317 } 318 319// Manual code (from Configuration.txt): 320 @Override 321 public String getIdBase() { 322 return getId(); 323 } 324 325 @Override 326 public void setIdBase(String value) { 327 setId(value); 328 } 329 330 public void addExtension(String url, DataType value) { 331 if (disallowExtensions) 332 throw new Error("Extensions are not allowed in this context"); 333 Extension ex = new Extension(); 334 ex.setUrl(url); 335 ex.setValue(value); 336 getExtension().add(ex); 337 } 338 339 340 /** 341 * Returns an extension if one (and only one) matches the given URL. 342 * 343 * Note: BackbdoneElements override this to look in matching Modifier Extensions too 344 * 345 * @param theUrl The URL. Must not be blank or null. 346 * @return the matching extension, or null 347 */ 348 public Extension getExtensionByUrl(String theUrl) { 349 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 350 ArrayList<Extension> retVal = new ArrayList<Extension>(); 351 for (Extension next : getExtension()) { 352 if (theUrl.equals(next.getUrl())) { 353 retVal.add(next); 354 } 355 } 356 if (retVal.size() == 0) 357 return null; 358 else { 359 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url "+theUrl+" must have only one match"); 360 return retVal.get(0); 361 } 362 } 363 364 /** 365 * Remove any extensions that match (by given URL). 366 * 367 * Note: BackbdoneElements override this to remove from Modifier Extensions too 368 * 369 * @param theUrl The URL. Must not be blank or null. 370 */ 371 public void removeExtension(String theUrl) { 372 for (int i = getExtension().size()-1; i >= 0; i--) { 373 if (theUrl.equals(getExtension().get(i).getUrl())) 374 getExtension().remove(i); 375 } 376 } 377 378 /** 379 * This is used in the FHIRPath engine to record that no extensions are allowed for this item in the context in which it is used. 380 * todo: enforce this.... 381 */ 382 private boolean disallowExtensions; 383 384 public boolean isDisallowExtensions() { 385 return disallowExtensions; 386 } 387 388 public Element setDisallowExtensions(boolean disallowExtensions) { 389 this.disallowExtensions = disallowExtensions; 390 return this; 391 } 392 393 public Element noExtensions() { 394 this.disallowExtensions = true; 395 return this; 396 } 397 398 /** 399 * Returns an unmodifiable list containing all extensions on this element which 400 * match the given URL. 401 * 402 * Note: BackbdoneElements override this to add matching Modifier Extensions too 403 * 404 * @param theUrl The URL. Must not be blank or null. 405 * @return an unmodifiable list containing all extensions on this element which match the given URL 406 */ 407 public List<Extension> getExtensionsByUrl(String theUrl) { 408 if (theUrl == null) { 409 throw new NullPointerException("theUrl must not be null"); 410 } else if (theUrl.length() == 0) { 411 throw new IllegalArgumentException("theUrl must not be empty"); 412 } 413 ArrayList<Extension> retVal = new ArrayList<>(); 414 for (Extension next : getExtension()) { 415 if (theUrl.equals(next.getUrl())) { 416 retVal.add(next); 417 } 418 } 419 return java.util.Collections.unmodifiableList(retVal); 420 } 421 422 public List<Extension> getExtensionsByUrl(String... theUrls) { 423 424 ArrayList<Extension> retVal = new ArrayList<>(); 425 for (Extension next : getExtension()) { 426 if (Utilities.existsInList(next.getUrl(), theUrls)) { 427 retVal.add(next); 428 } 429 } 430 return java.util.Collections.unmodifiableList(retVal); 431 } 432 433 434 public boolean hasExtension(String... theUrls) { 435 for (Extension next : getExtension()) { 436 if (Utilities.existsInList(next.getUrl(), theUrls)) { 437 return true; 438 } 439 } 440 return false; 441 } 442 443 444 /** 445 * Returns an true if this element has an extension that matchs the given URL. 446 * 447 * Note: BackbdoneElements override this to check Modifier Extensions too 448 * 449 * @param theUrl The URL. Must not be blank or null. 450 */ 451 public boolean hasExtension(String theUrl) { 452 if (extension == null || extension.size() == 0) { 453 return false; 454 } 455 456 for (Extension ext : extension) { 457 if (theUrl.equals(ext.getUrl())) { 458 return true; 459 } 460 } 461 462 return false; 463 } 464 465 /** 466 * Returns the value as a string if this element has only one extension that matches the given URL, and that can be converted to a string. 467 * 468 * Note: BackbdoneElements override this to check Modifier Extensions too 469 * 470 * @param theUrl The URL. Must not be blank or null. 471 */ 472 public String getExtensionString(String theUrl) throws FHIRException { 473 List<Extension> ext = getExtensionsByUrl(theUrl); 474 if (ext.isEmpty()) 475 return null; 476 if (ext.size() > 1) 477 throw new FHIRException("Multiple matching extensions found for extension '"+theUrl+"'"); 478 if (!ext.get(0).hasValue()) 479 return null; 480 if (!ext.get(0).getValue().isPrimitive()) 481 throw new FHIRException("Extension '"+theUrl+"' could not be converted to a string"); 482 return ext.get(0).getValue().primitiveValue(); 483 } 484 485 public String getExtensionString(String... theUrls) throws FHIRException { 486 for (String url : theUrls) { 487 if (hasExtension(url)) { 488 return getExtensionString(url); 489 } 490 } 491 return null; 492 } 493 494 495 public StandardsStatus getStandardsStatus() { 496 return ToolingExtensions.getStandardsStatus(this); 497 } 498 499 public void setStandardsStatus(StandardsStatus status) { 500 ToolingExtensions.setStandardsStatus(this, status, null); 501 } 502 503 public boolean hasExtension(Extension ext) { 504 if (hasExtension()) { 505 for (Extension t : getExtension()) { 506 if (Base.compareDeep(t, ext, false)) { 507 return true; 508 } 509 } 510 } 511 return false; 512 } 513 514 public void copyExtensions(org.hl7.fhir.r5.model.Element src, String... urls) { 515 for (Extension e : src.getExtension()) { 516 if (Utilities.existsInList(e.getUrl(), urls)) { 517 addExtension(e.copy()); 518 } 519 } 520 } 521 522 public void copyNewExtensions(org.hl7.fhir.r5.model.Element src, String... urls) { 523 for (Extension e : src.getExtension()) { 524 if (Utilities.existsInList(e.getUrl(), urls) && !!hasExtension(e.getUrl())) { 525 addExtension(e.copy()); 526 } 527 } 528 } 529 530 531 public FhirPublication getFHIRPublicationVersion() { 532 return FhirPublication.R5; 533 } 534// end addition 535 536} 537