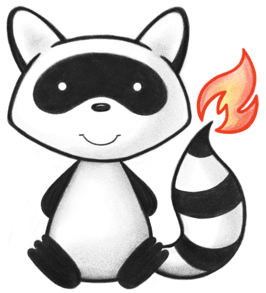
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.r5.model.Enumerations.BindingStrength; 041import org.hl7.fhir.r5.model.Enumerations.BindingStrengthEnumFactory; 042import org.hl7.fhir.r5.utils.ToolingExtensions; 043import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.DatatypeDef; 049import ca.uhn.fhir.model.api.annotation.Description; 050/** 051 * ElementDefinition Type: Captures constraints on each element within the resource, profile, or extension. 052 */ 053@DatatypeDef(name="ElementDefinition") 054public class ElementDefinition extends BackboneType implements ICompositeType { 055 056 public enum AdditionalBindingPurposeVS { 057 /** 058 * A required binding, for use when the binding strength is 'extensible' or 'preferred' 059 */ 060 MAXIMUM, 061 /** 062 * The minimum allowable value set - any conformant system SHALL support all these codes 063 */ 064 MINIMUM, 065 /** 066 * This value set is used as a required binding (in addition to the base binding (not a replacement), usually in a particular usage context) 067 */ 068 REQUIRED, 069 /** 070 * This value set is used as an extensible binding (in addition to the base binding (not a replacement), usually in a particular usage context) 071 */ 072 EXTENSIBLE, 073 /** 074 * This value set is a candidate to substitute for the overall conformance value set in some situations; usually these are defined in the documentation 075 */ 076 CANDIDATE, 077 /** 078 * New records are required to use this value set, but legacy records may use other codes. The definition of 'new record' is difficult, since systems often create new records based on pre-existing data. Usually 'current' bindings are mandated by an external authority that makes clear rules around this 079 */ 080 CURRENT, 081 /** 082 * This is the value set that is preferred in a given context (documentation should explain why) 083 */ 084 PREFERRED, 085 /** 086 * This value set is provided for user look up in a given context. Typically, these valuesets only include a subset of codes relevant for input in a context 087 */ 088 UI, 089 /** 090 * This value set is a good set of codes to start with when designing your system 091 */ 092 STARTER, 093 /** 094 * This value set is a component of the base value set. Usually this is called out so that documentation can be written about a portion of the value set 095 */ 096 COMPONENT, 097 /** 098 * added to help the parsers with the generic types 099 */ 100 NULL; 101 public static AdditionalBindingPurposeVS fromCode(String codeString) throws FHIRException { 102 if (codeString == null || "".equals(codeString)) 103 return null; 104 if ("maximum".equals(codeString)) 105 return MAXIMUM; 106 if ("minimum".equals(codeString)) 107 return MINIMUM; 108 if ("required".equals(codeString)) 109 return REQUIRED; 110 if ("extensible".equals(codeString)) 111 return EXTENSIBLE; 112 if ("candidate".equals(codeString)) 113 return CANDIDATE; 114 if ("current".equals(codeString)) 115 return CURRENT; 116 if ("preferred".equals(codeString)) 117 return PREFERRED; 118 if ("ui".equals(codeString)) 119 return UI; 120 if ("starter".equals(codeString)) 121 return STARTER; 122 if ("component".equals(codeString)) 123 return COMPONENT; 124 if (Configuration.isAcceptInvalidEnums()) 125 return null; 126 else 127 throw new FHIRException("Unknown AdditionalBindingPurposeVS code '"+codeString+"'"); 128 } 129 public String toCode() { 130 switch (this) { 131 case MAXIMUM: return "maximum"; 132 case MINIMUM: return "minimum"; 133 case REQUIRED: return "required"; 134 case EXTENSIBLE: return "extensible"; 135 case CANDIDATE: return "candidate"; 136 case CURRENT: return "current"; 137 case PREFERRED: return "preferred"; 138 case UI: return "ui"; 139 case STARTER: return "starter"; 140 case COMPONENT: return "component"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getSystem() { 146 switch (this) { 147 case MAXIMUM: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 148 case MINIMUM: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 149 case REQUIRED: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 150 case EXTENSIBLE: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 151 case CANDIDATE: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 152 case CURRENT: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 153 case PREFERRED: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 154 case UI: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 155 case STARTER: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 156 case COMPONENT: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 public String getDefinition() { 162 switch (this) { 163 case MAXIMUM: return "A required binding, for use when the binding strength is 'extensible' or 'preferred'"; 164 case MINIMUM: return "The minimum allowable value set - any conformant system SHALL support all these codes"; 165 case REQUIRED: return "This value set is used as a required binding (in addition to the base binding (not a replacement), usually in a particular usage context)"; 166 case EXTENSIBLE: return "This value set is used as an extensible binding (in addition to the base binding (not a replacement), usually in a particular usage context)"; 167 case CANDIDATE: return "This value set is a candidate to substitute for the overall conformance value set in some situations; usually these are defined in the documentation"; 168 case CURRENT: return "New records are required to use this value set, but legacy records may use other codes. The definition of 'new record' is difficult, since systems often create new records based on pre-existing data. Usually 'current' bindings are mandated by an external authority that makes clear rules around this"; 169 case PREFERRED: return "This is the value set that is preferred in a given context (documentation should explain why)"; 170 case UI: return "This value set is provided for user look up in a given context. Typically, these valuesets only include a subset of codes relevant for input in a context"; 171 case STARTER: return "This value set is a good set of codes to start with when designing your system"; 172 case COMPONENT: return "This value set is a component of the base value set. Usually this is called out so that documentation can be written about a portion of the value set"; 173 case NULL: return null; 174 default: return "?"; 175 } 176 } 177 public String getDisplay() { 178 switch (this) { 179 case MAXIMUM: return "Maximum Binding"; 180 case MINIMUM: return "Minimum Binding"; 181 case REQUIRED: return "Required Binding"; 182 case EXTENSIBLE: return "Conformance Binding"; 183 case CANDIDATE: return "Candidate Binding"; 184 case CURRENT: return "Current Binding"; 185 case PREFERRED: return "Preferred Binding"; 186 case UI: return "UI Suggested Binding"; 187 case STARTER: return "Starter Binding"; 188 case COMPONENT: return "Component Binding"; 189 case NULL: return null; 190 default: return "?"; 191 } 192 } 193 } 194 195 public static class AdditionalBindingPurposeVSEnumFactory implements EnumFactory<AdditionalBindingPurposeVS> { 196 public AdditionalBindingPurposeVS fromCode(String codeString) throws IllegalArgumentException { 197 if (codeString == null || "".equals(codeString)) 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("maximum".equals(codeString)) 201 return AdditionalBindingPurposeVS.MAXIMUM; 202 if ("minimum".equals(codeString)) 203 return AdditionalBindingPurposeVS.MINIMUM; 204 if ("required".equals(codeString)) 205 return AdditionalBindingPurposeVS.REQUIRED; 206 if ("extensible".equals(codeString)) 207 return AdditionalBindingPurposeVS.EXTENSIBLE; 208 if ("candidate".equals(codeString)) 209 return AdditionalBindingPurposeVS.CANDIDATE; 210 if ("current".equals(codeString)) 211 return AdditionalBindingPurposeVS.CURRENT; 212 if ("preferred".equals(codeString)) 213 return AdditionalBindingPurposeVS.PREFERRED; 214 if ("ui".equals(codeString)) 215 return AdditionalBindingPurposeVS.UI; 216 if ("starter".equals(codeString)) 217 return AdditionalBindingPurposeVS.STARTER; 218 if ("component".equals(codeString)) 219 return AdditionalBindingPurposeVS.COMPONENT; 220 throw new IllegalArgumentException("Unknown AdditionalBindingPurposeVS code '"+codeString+"'"); 221 } 222 public Enumeration<AdditionalBindingPurposeVS> fromType(PrimitiveType<?> code) throws FHIRException { 223 if (code == null) 224 return null; 225 if (code.isEmpty()) 226 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.NULL, code); 227 String codeString = ((PrimitiveType) code).asStringValue(); 228 if (codeString == null || "".equals(codeString)) 229 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.NULL, code); 230 if ("maximum".equals(codeString)) 231 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.MAXIMUM, code); 232 if ("minimum".equals(codeString)) 233 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.MINIMUM, code); 234 if ("required".equals(codeString)) 235 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.REQUIRED, code); 236 if ("extensible".equals(codeString)) 237 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.EXTENSIBLE, code); 238 if ("candidate".equals(codeString)) 239 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.CANDIDATE, code); 240 if ("current".equals(codeString)) 241 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.CURRENT, code); 242 if ("preferred".equals(codeString)) 243 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.PREFERRED, code); 244 if ("ui".equals(codeString)) 245 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.UI, code); 246 if ("starter".equals(codeString)) 247 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.STARTER, code); 248 if ("component".equals(codeString)) 249 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.COMPONENT, code); 250 throw new FHIRException("Unknown AdditionalBindingPurposeVS code '"+codeString+"'"); 251 } 252 public String toCode(AdditionalBindingPurposeVS code) { 253 if (code == AdditionalBindingPurposeVS.NULL) 254 return null; 255 if (code == AdditionalBindingPurposeVS.MAXIMUM) 256 return "maximum"; 257 if (code == AdditionalBindingPurposeVS.MINIMUM) 258 return "minimum"; 259 if (code == AdditionalBindingPurposeVS.REQUIRED) 260 return "required"; 261 if (code == AdditionalBindingPurposeVS.EXTENSIBLE) 262 return "extensible"; 263 if (code == AdditionalBindingPurposeVS.CANDIDATE) 264 return "candidate"; 265 if (code == AdditionalBindingPurposeVS.CURRENT) 266 return "current"; 267 if (code == AdditionalBindingPurposeVS.PREFERRED) 268 return "preferred"; 269 if (code == AdditionalBindingPurposeVS.UI) 270 return "ui"; 271 if (code == AdditionalBindingPurposeVS.STARTER) 272 return "starter"; 273 if (code == AdditionalBindingPurposeVS.COMPONENT) 274 return "component"; 275 return "?"; 276 } 277 public String toSystem(AdditionalBindingPurposeVS code) { 278 return code.getSystem(); 279 } 280 } 281 282 public enum AggregationMode { 283 /** 284 * The reference is a local reference to a contained resource. 285 */ 286 CONTAINED, 287 /** 288 * The reference to a resource that has to be resolved externally to the resource that includes the reference. 289 */ 290 REFERENCED, 291 /** 292 * When the resource is in a Bundle, the resource the reference points to will be found in the same bundle as the resource that includes the reference. 293 */ 294 BUNDLED, 295 /** 296 * added to help the parsers with the generic types 297 */ 298 NULL; 299 public static AggregationMode fromCode(String codeString) throws FHIRException { 300 if (codeString == null || "".equals(codeString)) 301 return null; 302 if ("contained".equals(codeString)) 303 return CONTAINED; 304 if ("referenced".equals(codeString)) 305 return REFERENCED; 306 if ("bundled".equals(codeString)) 307 return BUNDLED; 308 if (Configuration.isAcceptInvalidEnums()) 309 return null; 310 else 311 throw new FHIRException("Unknown AggregationMode code '"+codeString+"'"); 312 } 313 public String toCode() { 314 switch (this) { 315 case CONTAINED: return "contained"; 316 case REFERENCED: return "referenced"; 317 case BUNDLED: return "bundled"; 318 case NULL: return null; 319 default: return "?"; 320 } 321 } 322 public String getSystem() { 323 switch (this) { 324 case CONTAINED: return "http://hl7.org/fhir/resource-aggregation-mode"; 325 case REFERENCED: return "http://hl7.org/fhir/resource-aggregation-mode"; 326 case BUNDLED: return "http://hl7.org/fhir/resource-aggregation-mode"; 327 case NULL: return null; 328 default: return "?"; 329 } 330 } 331 public String getDefinition() { 332 switch (this) { 333 case CONTAINED: return "The reference is a local reference to a contained resource."; 334 case REFERENCED: return "The reference to a resource that has to be resolved externally to the resource that includes the reference."; 335 case BUNDLED: return "When the resource is in a Bundle, the resource the reference points to will be found in the same bundle as the resource that includes the reference."; 336 case NULL: return null; 337 default: return "?"; 338 } 339 } 340 public String getDisplay() { 341 switch (this) { 342 case CONTAINED: return "Contained"; 343 case REFERENCED: return "Referenced"; 344 case BUNDLED: return "Bundled"; 345 case NULL: return null; 346 default: return "?"; 347 } 348 } 349 } 350 351 public static class AggregationModeEnumFactory implements EnumFactory<AggregationMode> { 352 public AggregationMode fromCode(String codeString) throws IllegalArgumentException { 353 if (codeString == null || "".equals(codeString)) 354 if (codeString == null || "".equals(codeString)) 355 return null; 356 if ("contained".equals(codeString)) 357 return AggregationMode.CONTAINED; 358 if ("referenced".equals(codeString)) 359 return AggregationMode.REFERENCED; 360 if ("bundled".equals(codeString)) 361 return AggregationMode.BUNDLED; 362 throw new IllegalArgumentException("Unknown AggregationMode code '"+codeString+"'"); 363 } 364 public Enumeration<AggregationMode> fromType(PrimitiveType<?> code) throws FHIRException { 365 if (code == null) 366 return null; 367 if (code.isEmpty()) 368 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 369 String codeString = ((PrimitiveType) code).asStringValue(); 370 if (codeString == null || "".equals(codeString)) 371 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 372 if ("contained".equals(codeString)) 373 return new Enumeration<AggregationMode>(this, AggregationMode.CONTAINED, code); 374 if ("referenced".equals(codeString)) 375 return new Enumeration<AggregationMode>(this, AggregationMode.REFERENCED, code); 376 if ("bundled".equals(codeString)) 377 return new Enumeration<AggregationMode>(this, AggregationMode.BUNDLED, code); 378 throw new FHIRException("Unknown AggregationMode code '"+codeString+"'"); 379 } 380 public String toCode(AggregationMode code) { 381 if (code == AggregationMode.NULL) 382 return null; 383 if (code == AggregationMode.CONTAINED) 384 return "contained"; 385 if (code == AggregationMode.REFERENCED) 386 return "referenced"; 387 if (code == AggregationMode.BUNDLED) 388 return "bundled"; 389 return "?"; 390 } 391 public String toSystem(AggregationMode code) { 392 return code.getSystem(); 393 } 394 } 395 396 public enum ConstraintSeverity { 397 /** 398 * If the constraint is violated, the resource is not conformant. 399 */ 400 ERROR, 401 /** 402 * If the constraint is violated, the resource is conformant, but it is not necessarily following best practice. 403 */ 404 WARNING, 405 /** 406 * added to help the parsers with the generic types 407 */ 408 NULL; 409 public static ConstraintSeverity fromCode(String codeString) throws FHIRException { 410 if (codeString == null || "".equals(codeString)) 411 return null; 412 if ("error".equals(codeString)) 413 return ERROR; 414 if ("warning".equals(codeString)) 415 return WARNING; 416 if (Configuration.isAcceptInvalidEnums()) 417 return null; 418 else 419 throw new FHIRException("Unknown ConstraintSeverity code '"+codeString+"'"); 420 } 421 public String toCode() { 422 switch (this) { 423 case ERROR: return "error"; 424 case WARNING: return "warning"; 425 case NULL: return null; 426 default: return "?"; 427 } 428 } 429 public String getSystem() { 430 switch (this) { 431 case ERROR: return "http://hl7.org/fhir/constraint-severity"; 432 case WARNING: return "http://hl7.org/fhir/constraint-severity"; 433 case NULL: return null; 434 default: return "?"; 435 } 436 } 437 public String getDefinition() { 438 switch (this) { 439 case ERROR: return "If the constraint is violated, the resource is not conformant."; 440 case WARNING: return "If the constraint is violated, the resource is conformant, but it is not necessarily following best practice."; 441 case NULL: return null; 442 default: return "?"; 443 } 444 } 445 public String getDisplay() { 446 switch (this) { 447 case ERROR: return "Error"; 448 case WARNING: return "Warning"; 449 case NULL: return null; 450 default: return "?"; 451 } 452 } 453 } 454 455 public static class ConstraintSeverityEnumFactory implements EnumFactory<ConstraintSeverity> { 456 public ConstraintSeverity fromCode(String codeString) throws IllegalArgumentException { 457 if (codeString == null || "".equals(codeString)) 458 if (codeString == null || "".equals(codeString)) 459 return null; 460 if ("error".equals(codeString)) 461 return ConstraintSeverity.ERROR; 462 if ("warning".equals(codeString)) 463 return ConstraintSeverity.WARNING; 464 throw new IllegalArgumentException("Unknown ConstraintSeverity code '"+codeString+"'"); 465 } 466 public Enumeration<ConstraintSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 467 if (code == null) 468 return null; 469 if (code.isEmpty()) 470 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 471 String codeString = ((PrimitiveType) code).asStringValue(); 472 if (codeString == null || "".equals(codeString)) 473 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 474 if ("error".equals(codeString)) 475 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.ERROR, code); 476 if ("warning".equals(codeString)) 477 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.WARNING, code); 478 throw new FHIRException("Unknown ConstraintSeverity code '"+codeString+"'"); 479 } 480 public String toCode(ConstraintSeverity code) { 481 if (code == ConstraintSeverity.NULL) 482 return null; 483 if (code == ConstraintSeverity.ERROR) 484 return "error"; 485 if (code == ConstraintSeverity.WARNING) 486 return "warning"; 487 return "?"; 488 } 489 public String toSystem(ConstraintSeverity code) { 490 return code.getSystem(); 491 } 492 } 493 494 public enum DiscriminatorType { 495 /** 496 * The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding. 497 */ 498 VALUE, 499 /** 500 * The slices are differentiated by the presence or absence of the nominated element. There SHALL be no more than two slices. The slices are differentiated by the fact that one must have a max of 0 and the other must have a min of 1 (or more). The order in which the slices are declared doesn't matter. 501 */ 502 EXISTS, 503 /** 504 * The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding. This has the same meaning as 'value' and is deprecated. 505 */ 506 PATTERN, 507 /** 508 * The slices are differentiated by type of the nominated element. 509 */ 510 TYPE, 511 /** 512 * The slices are differentiated by conformance of the nominated element to a specified profile. Note that if the path specifies .resolve() then the profile is the target profile on the reference. In this case, validation by the possible profiles is required to differentiate the slices. 513 */ 514 PROFILE, 515 /** 516 * The slices are differentiated by their index. This is only possible if all but the last slice have min=max cardinality, and the (optional) last slice contains other undifferentiated elements. 517 */ 518 POSITION, 519 /** 520 * added to help the parsers with the generic types 521 */ 522 NULL; 523 public static DiscriminatorType fromCode(String codeString) throws FHIRException { 524 if (codeString == null || "".equals(codeString)) 525 return null; 526 if ("value".equals(codeString)) 527 return VALUE; 528 if ("exists".equals(codeString)) 529 return EXISTS; 530 if ("pattern".equals(codeString)) 531 return PATTERN; 532 if ("type".equals(codeString)) 533 return TYPE; 534 if ("profile".equals(codeString)) 535 return PROFILE; 536 if ("position".equals(codeString)) 537 return POSITION; 538 if (Configuration.isAcceptInvalidEnums()) 539 return null; 540 else 541 throw new FHIRException("Unknown DiscriminatorType code '"+codeString+"'"); 542 } 543 public String toCode() { 544 switch (this) { 545 case VALUE: return "value"; 546 case EXISTS: return "exists"; 547 case PATTERN: return "pattern"; 548 case TYPE: return "type"; 549 case PROFILE: return "profile"; 550 case POSITION: return "position"; 551 case NULL: return null; 552 default: return "?"; 553 } 554 } 555 public String getSystem() { 556 switch (this) { 557 case VALUE: return "http://hl7.org/fhir/discriminator-type"; 558 case EXISTS: return "http://hl7.org/fhir/discriminator-type"; 559 case PATTERN: return "http://hl7.org/fhir/discriminator-type"; 560 case TYPE: return "http://hl7.org/fhir/discriminator-type"; 561 case PROFILE: return "http://hl7.org/fhir/discriminator-type"; 562 case POSITION: return "http://hl7.org/fhir/discriminator-type"; 563 case NULL: return null; 564 default: return "?"; 565 } 566 } 567 public String getDefinition() { 568 switch (this) { 569 case VALUE: return "The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding."; 570 case EXISTS: return "The slices are differentiated by the presence or absence of the nominated element. There SHALL be no more than two slices. The slices are differentiated by the fact that one must have a max of 0 and the other must have a min of 1 (or more). The order in which the slices are declared doesn't matter."; 571 case PATTERN: return "The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding. This has the same meaning as 'value' and is deprecated."; 572 case TYPE: return "The slices are differentiated by type of the nominated element."; 573 case PROFILE: return "The slices are differentiated by conformance of the nominated element to a specified profile. Note that if the path specifies .resolve() then the profile is the target profile on the reference. In this case, validation by the possible profiles is required to differentiate the slices."; 574 case POSITION: return "The slices are differentiated by their index. This is only possible if all but the last slice have min=max cardinality, and the (optional) last slice contains other undifferentiated elements."; 575 case NULL: return null; 576 default: return "?"; 577 } 578 } 579 public String getDisplay() { 580 switch (this) { 581 case VALUE: return "Value"; 582 case EXISTS: return "Exists"; 583 case PATTERN: return "Pattern"; 584 case TYPE: return "Type"; 585 case PROFILE: return "Profile"; 586 case POSITION: return "Position"; 587 case NULL: return null; 588 default: return "?"; 589 } 590 } 591 } 592 593 public static class DiscriminatorTypeEnumFactory implements EnumFactory<DiscriminatorType> { 594 public DiscriminatorType fromCode(String codeString) throws IllegalArgumentException { 595 if (codeString == null || "".equals(codeString)) 596 if (codeString == null || "".equals(codeString)) 597 return null; 598 if ("value".equals(codeString)) 599 return DiscriminatorType.VALUE; 600 if ("exists".equals(codeString)) 601 return DiscriminatorType.EXISTS; 602 if ("pattern".equals(codeString)) 603 return DiscriminatorType.PATTERN; 604 if ("type".equals(codeString)) 605 return DiscriminatorType.TYPE; 606 if ("profile".equals(codeString)) 607 return DiscriminatorType.PROFILE; 608 if ("position".equals(codeString)) 609 return DiscriminatorType.POSITION; 610 throw new IllegalArgumentException("Unknown DiscriminatorType code '"+codeString+"'"); 611 } 612 public Enumeration<DiscriminatorType> fromType(PrimitiveType<?> code) throws FHIRException { 613 if (code == null) 614 return null; 615 if (code.isEmpty()) 616 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 617 String codeString = ((PrimitiveType) code).asStringValue(); 618 if (codeString == null || "".equals(codeString)) 619 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 620 if ("value".equals(codeString)) 621 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.VALUE, code); 622 if ("exists".equals(codeString)) 623 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.EXISTS, code); 624 if ("pattern".equals(codeString)) 625 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PATTERN, code); 626 if ("type".equals(codeString)) 627 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.TYPE, code); 628 if ("profile".equals(codeString)) 629 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PROFILE, code); 630 if ("position".equals(codeString)) 631 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.POSITION, code); 632 throw new FHIRException("Unknown DiscriminatorType code '"+codeString+"'"); 633 } 634 public String toCode(DiscriminatorType code) { 635 if (code == DiscriminatorType.NULL) 636 return null; 637 if (code == DiscriminatorType.VALUE) 638 return "value"; 639 if (code == DiscriminatorType.EXISTS) 640 return "exists"; 641 if (code == DiscriminatorType.PATTERN) 642 return "pattern"; 643 if (code == DiscriminatorType.TYPE) 644 return "type"; 645 if (code == DiscriminatorType.PROFILE) 646 return "profile"; 647 if (code == DiscriminatorType.POSITION) 648 return "position"; 649 return "?"; 650 } 651 public String toSystem(DiscriminatorType code) { 652 return code.getSystem(); 653 } 654 } 655 656 public enum PropertyRepresentation { 657 /** 658 * In XML, this property is represented as an attribute not an element. 659 */ 660 XMLATTR, 661 /** 662 * This element is represented using the XML text attribute (primitives only). 663 */ 664 XMLTEXT, 665 /** 666 * The type of this element is indicated using xsi:type. 667 */ 668 TYPEATTR, 669 /** 670 * Use CDA narrative instead of XHTML. 671 */ 672 CDATEXT, 673 /** 674 * The property is represented using XHTML. 675 */ 676 XHTML, 677 /** 678 * added to help the parsers with the generic types 679 */ 680 NULL; 681 public static PropertyRepresentation fromCode(String codeString) throws FHIRException { 682 if (codeString == null || "".equals(codeString)) 683 return null; 684 if ("xmlAttr".equals(codeString)) 685 return XMLATTR; 686 if ("xmlText".equals(codeString)) 687 return XMLTEXT; 688 if ("typeAttr".equals(codeString)) 689 return TYPEATTR; 690 if ("cdaText".equals(codeString)) 691 return CDATEXT; 692 if ("xhtml".equals(codeString)) 693 return XHTML; 694 if (Configuration.isAcceptInvalidEnums()) 695 return null; 696 else 697 throw new FHIRException("Unknown PropertyRepresentation code '"+codeString+"'"); 698 } 699 public String toCode() { 700 switch (this) { 701 case XMLATTR: return "xmlAttr"; 702 case XMLTEXT: return "xmlText"; 703 case TYPEATTR: return "typeAttr"; 704 case CDATEXT: return "cdaText"; 705 case XHTML: return "xhtml"; 706 case NULL: return null; 707 default: return "?"; 708 } 709 } 710 public String getSystem() { 711 switch (this) { 712 case XMLATTR: return "http://hl7.org/fhir/property-representation"; 713 case XMLTEXT: return "http://hl7.org/fhir/property-representation"; 714 case TYPEATTR: return "http://hl7.org/fhir/property-representation"; 715 case CDATEXT: return "http://hl7.org/fhir/property-representation"; 716 case XHTML: return "http://hl7.org/fhir/property-representation"; 717 case NULL: return null; 718 default: return "?"; 719 } 720 } 721 public String getDefinition() { 722 switch (this) { 723 case XMLATTR: return "In XML, this property is represented as an attribute not an element."; 724 case XMLTEXT: return "This element is represented using the XML text attribute (primitives only)."; 725 case TYPEATTR: return "The type of this element is indicated using xsi:type."; 726 case CDATEXT: return "Use CDA narrative instead of XHTML."; 727 case XHTML: return "The property is represented using XHTML."; 728 case NULL: return null; 729 default: return "?"; 730 } 731 } 732 public String getDisplay() { 733 switch (this) { 734 case XMLATTR: return "XML Attribute"; 735 case XMLTEXT: return "XML Text"; 736 case TYPEATTR: return "Type Attribute"; 737 case CDATEXT: return "CDA Text Format"; 738 case XHTML: return "XHTML"; 739 case NULL: return null; 740 default: return "?"; 741 } 742 } 743 } 744 745 public static class PropertyRepresentationEnumFactory implements EnumFactory<PropertyRepresentation> { 746 public PropertyRepresentation fromCode(String codeString) throws IllegalArgumentException { 747 if (codeString == null || "".equals(codeString)) 748 if (codeString == null || "".equals(codeString)) 749 return null; 750 if ("xmlAttr".equals(codeString)) 751 return PropertyRepresentation.XMLATTR; 752 if ("xmlText".equals(codeString)) 753 return PropertyRepresentation.XMLTEXT; 754 if ("typeAttr".equals(codeString)) 755 return PropertyRepresentation.TYPEATTR; 756 if ("cdaText".equals(codeString)) 757 return PropertyRepresentation.CDATEXT; 758 if ("xhtml".equals(codeString)) 759 return PropertyRepresentation.XHTML; 760 throw new IllegalArgumentException("Unknown PropertyRepresentation code '"+codeString+"'"); 761 } 762 public Enumeration<PropertyRepresentation> fromType(PrimitiveType<?> code) throws FHIRException { 763 if (code == null) 764 return null; 765 if (code.isEmpty()) 766 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 767 String codeString = ((PrimitiveType) code).asStringValue(); 768 if (codeString == null || "".equals(codeString)) 769 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 770 if ("xmlAttr".equals(codeString)) 771 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLATTR, code); 772 if ("xmlText".equals(codeString)) 773 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLTEXT, code); 774 if ("typeAttr".equals(codeString)) 775 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.TYPEATTR, code); 776 if ("cdaText".equals(codeString)) 777 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.CDATEXT, code); 778 if ("xhtml".equals(codeString)) 779 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XHTML, code); 780 throw new FHIRException("Unknown PropertyRepresentation code '"+codeString+"'"); 781 } 782 public String toCode(PropertyRepresentation code) { 783 if (code == PropertyRepresentation.NULL) 784 return null; 785 if (code == PropertyRepresentation.XMLATTR) 786 return "xmlAttr"; 787 if (code == PropertyRepresentation.XMLTEXT) 788 return "xmlText"; 789 if (code == PropertyRepresentation.TYPEATTR) 790 return "typeAttr"; 791 if (code == PropertyRepresentation.CDATEXT) 792 return "cdaText"; 793 if (code == PropertyRepresentation.XHTML) 794 return "xhtml"; 795 return "?"; 796 } 797 public String toSystem(PropertyRepresentation code) { 798 return code.getSystem(); 799 } 800 } 801 802 public enum ReferenceVersionRules { 803 /** 804 * The reference may be either version independent or version specific. 805 */ 806 EITHER, 807 /** 808 * The reference must be version independent. 809 */ 810 INDEPENDENT, 811 /** 812 * The reference must be version specific. 813 */ 814 SPECIFIC, 815 /** 816 * added to help the parsers with the generic types 817 */ 818 NULL; 819 public static ReferenceVersionRules fromCode(String codeString) throws FHIRException { 820 if (codeString == null || "".equals(codeString)) 821 return null; 822 if ("either".equals(codeString)) 823 return EITHER; 824 if ("independent".equals(codeString)) 825 return INDEPENDENT; 826 if ("specific".equals(codeString)) 827 return SPECIFIC; 828 if (Configuration.isAcceptInvalidEnums()) 829 return null; 830 else 831 throw new FHIRException("Unknown ReferenceVersionRules code '"+codeString+"'"); 832 } 833 public String toCode() { 834 switch (this) { 835 case EITHER: return "either"; 836 case INDEPENDENT: return "independent"; 837 case SPECIFIC: return "specific"; 838 case NULL: return null; 839 default: return "?"; 840 } 841 } 842 public String getSystem() { 843 switch (this) { 844 case EITHER: return "http://hl7.org/fhir/reference-version-rules"; 845 case INDEPENDENT: return "http://hl7.org/fhir/reference-version-rules"; 846 case SPECIFIC: return "http://hl7.org/fhir/reference-version-rules"; 847 case NULL: return null; 848 default: return "?"; 849 } 850 } 851 public String getDefinition() { 852 switch (this) { 853 case EITHER: return "The reference may be either version independent or version specific."; 854 case INDEPENDENT: return "The reference must be version independent."; 855 case SPECIFIC: return "The reference must be version specific."; 856 case NULL: return null; 857 default: return "?"; 858 } 859 } 860 public String getDisplay() { 861 switch (this) { 862 case EITHER: return "Either Specific or independent"; 863 case INDEPENDENT: return "Version independent"; 864 case SPECIFIC: return "Version Specific"; 865 case NULL: return null; 866 default: return "?"; 867 } 868 } 869 } 870 871 public static class ReferenceVersionRulesEnumFactory implements EnumFactory<ReferenceVersionRules> { 872 public ReferenceVersionRules fromCode(String codeString) throws IllegalArgumentException { 873 if (codeString == null || "".equals(codeString)) 874 if (codeString == null || "".equals(codeString)) 875 return null; 876 if ("either".equals(codeString)) 877 return ReferenceVersionRules.EITHER; 878 if ("independent".equals(codeString)) 879 return ReferenceVersionRules.INDEPENDENT; 880 if ("specific".equals(codeString)) 881 return ReferenceVersionRules.SPECIFIC; 882 throw new IllegalArgumentException("Unknown ReferenceVersionRules code '"+codeString+"'"); 883 } 884 public Enumeration<ReferenceVersionRules> fromType(PrimitiveType<?> code) throws FHIRException { 885 if (code == null) 886 return null; 887 if (code.isEmpty()) 888 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 889 String codeString = ((PrimitiveType) code).asStringValue(); 890 if (codeString == null || "".equals(codeString)) 891 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 892 if ("either".equals(codeString)) 893 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.EITHER, code); 894 if ("independent".equals(codeString)) 895 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.INDEPENDENT, code); 896 if ("specific".equals(codeString)) 897 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.SPECIFIC, code); 898 throw new FHIRException("Unknown ReferenceVersionRules code '"+codeString+"'"); 899 } 900 public String toCode(ReferenceVersionRules code) { 901 if (code == ReferenceVersionRules.NULL) 902 return null; 903 if (code == ReferenceVersionRules.EITHER) 904 return "either"; 905 if (code == ReferenceVersionRules.INDEPENDENT) 906 return "independent"; 907 if (code == ReferenceVersionRules.SPECIFIC) 908 return "specific"; 909 return "?"; 910 } 911 public String toSystem(ReferenceVersionRules code) { 912 return code.getSystem(); 913 } 914 } 915 916 public enum SlicingRules { 917 /** 918 * No additional content is allowed other than that described by the slices in this profile. 919 */ 920 CLOSED, 921 /** 922 * Additional content is allowed anywhere in the list. 923 */ 924 OPEN, 925 /** 926 * Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required. 927 */ 928 OPENATEND, 929 /** 930 * added to help the parsers with the generic types 931 */ 932 NULL; 933 public static SlicingRules fromCode(String codeString) throws FHIRException { 934 if (codeString == null || "".equals(codeString)) 935 return null; 936 if ("closed".equals(codeString)) 937 return CLOSED; 938 if ("open".equals(codeString)) 939 return OPEN; 940 if ("openAtEnd".equals(codeString)) 941 return OPENATEND; 942 if (Configuration.isAcceptInvalidEnums()) 943 return null; 944 else 945 throw new FHIRException("Unknown SlicingRules code '"+codeString+"'"); 946 } 947 public String toCode() { 948 switch (this) { 949 case CLOSED: return "closed"; 950 case OPEN: return "open"; 951 case OPENATEND: return "openAtEnd"; 952 case NULL: return null; 953 default: return "?"; 954 } 955 } 956 public String getSystem() { 957 switch (this) { 958 case CLOSED: return "http://hl7.org/fhir/resource-slicing-rules"; 959 case OPEN: return "http://hl7.org/fhir/resource-slicing-rules"; 960 case OPENATEND: return "http://hl7.org/fhir/resource-slicing-rules"; 961 case NULL: return null; 962 default: return "?"; 963 } 964 } 965 public String getDefinition() { 966 switch (this) { 967 case CLOSED: return "No additional content is allowed other than that described by the slices in this profile."; 968 case OPEN: return "Additional content is allowed anywhere in the list."; 969 case OPENATEND: return "Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required."; 970 case NULL: return null; 971 default: return "?"; 972 } 973 } 974 public String getDisplay() { 975 switch (this) { 976 case CLOSED: return "Closed"; 977 case OPEN: return "Open"; 978 case OPENATEND: return "Open at End"; 979 case NULL: return null; 980 default: return "?"; 981 } 982 } 983 } 984 985 public static class SlicingRulesEnumFactory implements EnumFactory<SlicingRules> { 986 public SlicingRules fromCode(String codeString) throws IllegalArgumentException { 987 if (codeString == null || "".equals(codeString)) 988 if (codeString == null || "".equals(codeString)) 989 return null; 990 if ("closed".equals(codeString)) 991 return SlicingRules.CLOSED; 992 if ("open".equals(codeString)) 993 return SlicingRules.OPEN; 994 if ("openAtEnd".equals(codeString)) 995 return SlicingRules.OPENATEND; 996 throw new IllegalArgumentException("Unknown SlicingRules code '"+codeString+"'"); 997 } 998 public Enumeration<SlicingRules> fromType(PrimitiveType<?> code) throws FHIRException { 999 if (code == null) 1000 return null; 1001 if (code.isEmpty()) 1002 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 1003 String codeString = ((PrimitiveType) code).asStringValue(); 1004 if (codeString == null || "".equals(codeString)) 1005 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 1006 if ("closed".equals(codeString)) 1007 return new Enumeration<SlicingRules>(this, SlicingRules.CLOSED, code); 1008 if ("open".equals(codeString)) 1009 return new Enumeration<SlicingRules>(this, SlicingRules.OPEN, code); 1010 if ("openAtEnd".equals(codeString)) 1011 return new Enumeration<SlicingRules>(this, SlicingRules.OPENATEND, code); 1012 throw new FHIRException("Unknown SlicingRules code '"+codeString+"'"); 1013 } 1014 public String toCode(SlicingRules code) { 1015 if (code == SlicingRules.NULL) 1016 return null; 1017 if (code == SlicingRules.CLOSED) 1018 return "closed"; 1019 if (code == SlicingRules.OPEN) 1020 return "open"; 1021 if (code == SlicingRules.OPENATEND) 1022 return "openAtEnd"; 1023 return "?"; 1024 } 1025 public String toSystem(SlicingRules code) { 1026 return code.getSystem(); 1027 } 1028 } 1029 1030 @Block() 1031 public static class ElementDefinitionSlicingComponent extends Element implements IBaseDatatypeElement { 1032 /** 1033 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices. 1034 */ 1035 @Child(name = "discriminator", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1036 @Description(shortDefinition="Element values that are used to distinguish the slices", formalDefinition="Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices." ) 1037 protected List<ElementDefinitionSlicingDiscriminatorComponent> discriminator; 1038 1039 /** 1040 * A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 1041 */ 1042 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1043 @Description(shortDefinition="Text description of how slicing works (or not)", formalDefinition="A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated." ) 1044 protected StringType description; 1045 1046 /** 1047 * If the matching elements have to occur in the same order as defined in the profile. 1048 */ 1049 @Child(name = "ordered", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1050 @Description(shortDefinition="If elements must be in same order as slices", formalDefinition="If the matching elements have to occur in the same order as defined in the profile." ) 1051 protected BooleanType ordered; 1052 1053 /** 1054 * Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1055 */ 1056 @Child(name = "rules", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1057 @Description(shortDefinition="closed | open | openAtEnd", formalDefinition="Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end." ) 1058 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-slicing-rules") 1059 protected Enumeration<SlicingRules> rules; 1060 1061 private static final long serialVersionUID = -311635839L; 1062 1063 /** 1064 * Constructor 1065 */ 1066 public ElementDefinitionSlicingComponent() { 1067 super(); 1068 } 1069 1070 /** 1071 * Constructor 1072 */ 1073 public ElementDefinitionSlicingComponent(SlicingRules rules) { 1074 super(); 1075 this.setRules(rules); 1076 } 1077 1078 /** 1079 * @return {@link #discriminator} (Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.) 1080 */ 1081 public List<ElementDefinitionSlicingDiscriminatorComponent> getDiscriminator() { 1082 if (this.discriminator == null) 1083 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1084 return this.discriminator; 1085 } 1086 1087 /** 1088 * @return Returns a reference to <code>this</code> for easy method chaining 1089 */ 1090 public ElementDefinitionSlicingComponent setDiscriminator(List<ElementDefinitionSlicingDiscriminatorComponent> theDiscriminator) { 1091 this.discriminator = theDiscriminator; 1092 return this; 1093 } 1094 1095 public boolean hasDiscriminator() { 1096 if (this.discriminator == null) 1097 return false; 1098 for (ElementDefinitionSlicingDiscriminatorComponent item : this.discriminator) 1099 if (!item.isEmpty()) 1100 return true; 1101 return false; 1102 } 1103 1104 public ElementDefinitionSlicingDiscriminatorComponent addDiscriminator() { //3 1105 ElementDefinitionSlicingDiscriminatorComponent t = new ElementDefinitionSlicingDiscriminatorComponent(); 1106 if (this.discriminator == null) 1107 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1108 this.discriminator.add(t); 1109 return t; 1110 } 1111 1112 public ElementDefinitionSlicingComponent addDiscriminator(ElementDefinitionSlicingDiscriminatorComponent t) { //3 1113 if (t == null) 1114 return this; 1115 if (this.discriminator == null) 1116 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1117 this.discriminator.add(t); 1118 return this; 1119 } 1120 1121 /** 1122 * @return The first repetition of repeating field {@link #discriminator}, creating it if it does not already exist {3} 1123 */ 1124 public ElementDefinitionSlicingDiscriminatorComponent getDiscriminatorFirstRep() { 1125 if (getDiscriminator().isEmpty()) { 1126 addDiscriminator(); 1127 } 1128 return getDiscriminator().get(0); 1129 } 1130 1131 /** 1132 * @return {@link #description} (A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1133 */ 1134 public StringType getDescriptionElement() { 1135 if (this.description == null) 1136 if (Configuration.errorOnAutoCreate()) 1137 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.description"); 1138 else if (Configuration.doAutoCreate()) 1139 this.description = new StringType(); // bb 1140 return this.description; 1141 } 1142 1143 public boolean hasDescriptionElement() { 1144 return this.description != null && !this.description.isEmpty(); 1145 } 1146 1147 public boolean hasDescription() { 1148 return this.description != null && !this.description.isEmpty(); 1149 } 1150 1151 /** 1152 * @param value {@link #description} (A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1153 */ 1154 public ElementDefinitionSlicingComponent setDescriptionElement(StringType value) { 1155 this.description = value; 1156 return this; 1157 } 1158 1159 /** 1160 * @return A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 1161 */ 1162 public String getDescription() { 1163 return this.description == null ? null : this.description.getValue(); 1164 } 1165 1166 /** 1167 * @param value A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 1168 */ 1169 public ElementDefinitionSlicingComponent setDescription(String value) { 1170 if (Utilities.noString(value)) 1171 this.description = null; 1172 else { 1173 if (this.description == null) 1174 this.description = new StringType(); 1175 this.description.setValue(value); 1176 } 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #ordered} (If the matching elements have to occur in the same order as defined in the profile.). This is the underlying object with id, value and extensions. The accessor "getOrdered" gives direct access to the value 1182 */ 1183 public BooleanType getOrderedElement() { 1184 if (this.ordered == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.ordered"); 1187 else if (Configuration.doAutoCreate()) 1188 this.ordered = new BooleanType(); // bb 1189 return this.ordered; 1190 } 1191 1192 public boolean hasOrderedElement() { 1193 return this.ordered != null && !this.ordered.isEmpty(); 1194 } 1195 1196 public boolean hasOrdered() { 1197 return this.ordered != null && !this.ordered.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #ordered} (If the matching elements have to occur in the same order as defined in the profile.). This is the underlying object with id, value and extensions. The accessor "getOrdered" gives direct access to the value 1202 */ 1203 public ElementDefinitionSlicingComponent setOrderedElement(BooleanType value) { 1204 this.ordered = value; 1205 return this; 1206 } 1207 1208 /** 1209 * @return If the matching elements have to occur in the same order as defined in the profile. 1210 */ 1211 public boolean getOrdered() { 1212 return this.ordered == null || this.ordered.isEmpty() ? false : this.ordered.getValue(); 1213 } 1214 1215 /** 1216 * @param value If the matching elements have to occur in the same order as defined in the profile. 1217 */ 1218 public ElementDefinitionSlicingComponent setOrdered(boolean value) { 1219 if (this.ordered == null) 1220 this.ordered = new BooleanType(); 1221 this.ordered.setValue(value); 1222 return this; 1223 } 1224 1225 /** 1226 * @return {@link #rules} (Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.). This is the underlying object with id, value and extensions. The accessor "getRules" gives direct access to the value 1227 */ 1228 public Enumeration<SlicingRules> getRulesElement() { 1229 if (this.rules == null) 1230 if (Configuration.errorOnAutoCreate()) 1231 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.rules"); 1232 else if (Configuration.doAutoCreate()) 1233 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); // bb 1234 return this.rules; 1235 } 1236 1237 public boolean hasRulesElement() { 1238 return this.rules != null && !this.rules.isEmpty(); 1239 } 1240 1241 public boolean hasRules() { 1242 return this.rules != null && !this.rules.isEmpty(); 1243 } 1244 1245 /** 1246 * @param value {@link #rules} (Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.). This is the underlying object with id, value and extensions. The accessor "getRules" gives direct access to the value 1247 */ 1248 public ElementDefinitionSlicingComponent setRulesElement(Enumeration<SlicingRules> value) { 1249 this.rules = value; 1250 return this; 1251 } 1252 1253 /** 1254 * @return Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1255 */ 1256 public SlicingRules getRules() { 1257 return this.rules == null ? null : this.rules.getValue(); 1258 } 1259 1260 /** 1261 * @param value Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1262 */ 1263 public ElementDefinitionSlicingComponent setRules(SlicingRules value) { 1264 if (this.rules == null) 1265 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); 1266 this.rules.setValue(value); 1267 return this; 1268 } 1269 1270 protected void listChildren(List<Property> children) { 1271 super.listChildren(children); 1272 children.add(new Property("discriminator", "", "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 0, java.lang.Integer.MAX_VALUE, discriminator)); 1273 children.add(new Property("description", "string", "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 0, 1, description)); 1274 children.add(new Property("ordered", "boolean", "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered)); 1275 children.add(new Property("rules", "code", "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 0, 1, rules)); 1276 } 1277 1278 @Override 1279 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1280 switch (_hash) { 1281 case -1888270692: /*discriminator*/ return new Property("discriminator", "", "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 0, java.lang.Integer.MAX_VALUE, discriminator); 1282 case -1724546052: /*description*/ return new Property("description", "string", "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 0, 1, description); 1283 case -1207109523: /*ordered*/ return new Property("ordered", "boolean", "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered); 1284 case 108873975: /*rules*/ return new Property("rules", "code", "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 0, 1, rules); 1285 default: return super.getNamedProperty(_hash, _name, _checkValid); 1286 } 1287 1288 } 1289 1290 @Override 1291 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1292 switch (hash) { 1293 case -1888270692: /*discriminator*/ return this.discriminator == null ? new Base[0] : this.discriminator.toArray(new Base[this.discriminator.size()]); // ElementDefinitionSlicingDiscriminatorComponent 1294 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1295 case -1207109523: /*ordered*/ return this.ordered == null ? new Base[0] : new Base[] {this.ordered}; // BooleanType 1296 case 108873975: /*rules*/ return this.rules == null ? new Base[0] : new Base[] {this.rules}; // Enumeration<SlicingRules> 1297 default: return super.getProperty(hash, name, checkValid); 1298 } 1299 1300 } 1301 1302 @Override 1303 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1304 switch (hash) { 1305 case -1888270692: // discriminator 1306 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); // ElementDefinitionSlicingDiscriminatorComponent 1307 return value; 1308 case -1724546052: // description 1309 this.description = TypeConvertor.castToString(value); // StringType 1310 return value; 1311 case -1207109523: // ordered 1312 this.ordered = TypeConvertor.castToBoolean(value); // BooleanType 1313 return value; 1314 case 108873975: // rules 1315 value = new SlicingRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1316 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1317 return value; 1318 default: return super.setProperty(hash, name, value); 1319 } 1320 1321 } 1322 1323 @Override 1324 public Base setProperty(String name, Base value) throws FHIRException { 1325 if (name.equals("discriminator")) { 1326 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); 1327 } else if (name.equals("description")) { 1328 this.description = TypeConvertor.castToString(value); // StringType 1329 } else if (name.equals("ordered")) { 1330 this.ordered = TypeConvertor.castToBoolean(value); // BooleanType 1331 } else if (name.equals("rules")) { 1332 value = new SlicingRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1333 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1334 } else 1335 return super.setProperty(name, value); 1336 return value; 1337 } 1338 1339 @Override 1340 public void removeChild(String name, Base value) throws FHIRException { 1341 if (name.equals("discriminator")) { 1342 this.getDiscriminator().remove((ElementDefinitionSlicingDiscriminatorComponent) value); 1343 } else if (name.equals("description")) { 1344 this.description = null; 1345 } else if (name.equals("ordered")) { 1346 this.ordered = null; 1347 } else if (name.equals("rules")) { 1348 value = new SlicingRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1349 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1350 } else 1351 super.removeChild(name, value); 1352 1353 } 1354 1355 @Override 1356 public Base makeProperty(int hash, String name) throws FHIRException { 1357 switch (hash) { 1358 case -1888270692: return addDiscriminator(); 1359 case -1724546052: return getDescriptionElement(); 1360 case -1207109523: return getOrderedElement(); 1361 case 108873975: return getRulesElement(); 1362 default: return super.makeProperty(hash, name); 1363 } 1364 1365 } 1366 1367 @Override 1368 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1369 switch (hash) { 1370 case -1888270692: /*discriminator*/ return new String[] {}; 1371 case -1724546052: /*description*/ return new String[] {"string"}; 1372 case -1207109523: /*ordered*/ return new String[] {"boolean"}; 1373 case 108873975: /*rules*/ return new String[] {"code"}; 1374 default: return super.getTypesForProperty(hash, name); 1375 } 1376 1377 } 1378 1379 @Override 1380 public Base addChild(String name) throws FHIRException { 1381 if (name.equals("discriminator")) { 1382 return addDiscriminator(); 1383 } 1384 else if (name.equals("description")) { 1385 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.description"); 1386 } 1387 else if (name.equals("ordered")) { 1388 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.ordered"); 1389 } 1390 else if (name.equals("rules")) { 1391 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.rules"); 1392 } 1393 else 1394 return super.addChild(name); 1395 } 1396 1397 public ElementDefinitionSlicingComponent copy() { 1398 ElementDefinitionSlicingComponent dst = new ElementDefinitionSlicingComponent(); 1399 copyValues(dst); 1400 return dst; 1401 } 1402 1403 public void copyValues(ElementDefinitionSlicingComponent dst) { 1404 super.copyValues(dst); 1405 if (discriminator != null) { 1406 dst.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1407 for (ElementDefinitionSlicingDiscriminatorComponent i : discriminator) 1408 dst.discriminator.add(i.copy()); 1409 }; 1410 dst.description = description == null ? null : description.copy(); 1411 dst.ordered = ordered == null ? null : ordered.copy(); 1412 dst.rules = rules == null ? null : rules.copy(); 1413 } 1414 1415 @Override 1416 public boolean equalsDeep(Base other_) { 1417 if (!super.equalsDeep(other_)) 1418 return false; 1419 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1420 return false; 1421 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1422 return compareDeep(discriminator, o.discriminator, true) && compareDeep(description, o.description, true) 1423 && compareDeep(ordered, o.ordered, true) && compareDeep(rules, o.rules, true); 1424 } 1425 1426 @Override 1427 public boolean equalsShallow(Base other_) { 1428 if (!super.equalsShallow(other_)) 1429 return false; 1430 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1431 return false; 1432 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1433 return compareValues(description, o.description, true) && compareValues(ordered, o.ordered, true) && compareValues(rules, o.rules, true) 1434 ; 1435 } 1436 1437 public boolean isEmpty() { 1438 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(discriminator, description 1439 , ordered, rules); 1440 } 1441 1442 public String fhirType() { 1443 return "ElementDefinition.slicing"; 1444 1445 } 1446 1447 @Override 1448 public String toString() { 1449 return (ordered == null ? "??" : "true".equals(ordered.asStringValue()) ? "ordered" : "unordered")+"/"+ 1450 (rules == null ? "??" : rules.asStringValue())+" "+discriminator.toString(); 1451 } 1452 1453 public String summary() { 1454 StringBuilder b = new StringBuilder(); 1455 if (!hasRulesElement() && !hasOrdered() && !hasDiscriminator()) { 1456 return "(no slicing)"; 1457 } 1458 if (hasRulesElement() || hasOrdered()) { 1459 if (hasRulesElement() && hasOrdered()) { 1460 b.append((getOrdered() ? "orderer" : "unordered")+" and " +getRules().toCode()+", by"); 1461 } else if (hasRules()) { 1462 b.append(getRules().toCode()+", by"); 1463 } else if (getOrdered()) { 1464 b.append("ordered, by"); 1465 } else { 1466 b.append("unordered, by"); 1467 } 1468 } 1469 boolean first = true; 1470 for (ElementDefinitionSlicingDiscriminatorComponent d : getDiscriminator()) { 1471 if (first) { 1472 first = false; 1473 } else { 1474 b.append(","); 1475 } 1476 b.append(" "); 1477 b.append(d.getType().toCode()); 1478 b.append("="); 1479 b.append(d.getPath()); 1480 } 1481 return b.toString(); 1482 } 1483 } 1484 1485 @Block() 1486 public static class ElementDefinitionSlicingDiscriminatorComponent extends Element implements IBaseDatatypeElement { 1487 /** 1488 * How the element value is interpreted when discrimination is evaluated. 1489 */ 1490 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1491 @Description(shortDefinition="value | exists | type | profile | position", formalDefinition="How the element value is interpreted when discrimination is evaluated." ) 1492 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/discriminator-type") 1493 protected Enumeration<DiscriminatorType> type; 1494 1495 /** 1496 * A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based. 1497 */ 1498 @Child(name = "path", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1499 @Description(shortDefinition="Path to element value", formalDefinition="A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based." ) 1500 protected StringType path; 1501 1502 private static final long serialVersionUID = 1151159293L; 1503 1504 /** 1505 * Constructor 1506 */ 1507 public ElementDefinitionSlicingDiscriminatorComponent() { 1508 super(); 1509 } 1510 1511 /** 1512 * Constructor 1513 */ 1514 public ElementDefinitionSlicingDiscriminatorComponent(DiscriminatorType type, String path) { 1515 super(); 1516 this.setType(type); 1517 this.setPath(path); 1518 } 1519 1520 /** 1521 * @return {@link #type} (How the element value is interpreted when discrimination is evaluated.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1522 */ 1523 public Enumeration<DiscriminatorType> getTypeElement() { 1524 if (this.type == null) 1525 if (Configuration.errorOnAutoCreate()) 1526 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.type"); 1527 else if (Configuration.doAutoCreate()) 1528 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); // bb 1529 return this.type; 1530 } 1531 1532 public boolean hasTypeElement() { 1533 return this.type != null && !this.type.isEmpty(); 1534 } 1535 1536 public boolean hasType() { 1537 return this.type != null && !this.type.isEmpty(); 1538 } 1539 1540 /** 1541 * @param value {@link #type} (How the element value is interpreted when discrimination is evaluated.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1542 */ 1543 public ElementDefinitionSlicingDiscriminatorComponent setTypeElement(Enumeration<DiscriminatorType> value) { 1544 this.type = value; 1545 return this; 1546 } 1547 1548 /** 1549 * @return How the element value is interpreted when discrimination is evaluated. 1550 */ 1551 public DiscriminatorType getType() { 1552 return this.type == null ? null : this.type.getValue(); 1553 } 1554 1555 /** 1556 * @param value How the element value is interpreted when discrimination is evaluated. 1557 */ 1558 public ElementDefinitionSlicingDiscriminatorComponent setType(DiscriminatorType value) { 1559 if (this.type == null) 1560 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); 1561 this.type.setValue(value); 1562 return this; 1563 } 1564 1565 /** 1566 * @return {@link #path} (A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1567 */ 1568 public StringType getPathElement() { 1569 if (this.path == null) 1570 if (Configuration.errorOnAutoCreate()) 1571 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.path"); 1572 else if (Configuration.doAutoCreate()) 1573 this.path = new StringType(); // bb 1574 return this.path; 1575 } 1576 1577 public boolean hasPathElement() { 1578 return this.path != null && !this.path.isEmpty(); 1579 } 1580 1581 public boolean hasPath() { 1582 return this.path != null && !this.path.isEmpty(); 1583 } 1584 1585 /** 1586 * @param value {@link #path} (A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1587 */ 1588 public ElementDefinitionSlicingDiscriminatorComponent setPathElement(StringType value) { 1589 this.path = value; 1590 return this; 1591 } 1592 1593 /** 1594 * @return A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based. 1595 */ 1596 public String getPath() { 1597 return this.path == null ? null : this.path.getValue(); 1598 } 1599 1600 /** 1601 * @param value A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based. 1602 */ 1603 public ElementDefinitionSlicingDiscriminatorComponent setPath(String value) { 1604 if (this.path == null) 1605 this.path = new StringType(); 1606 this.path.setValue(value); 1607 return this; 1608 } 1609 1610 protected void listChildren(List<Property> children) { 1611 super.listChildren(children); 1612 children.add(new Property("type", "code", "How the element value is interpreted when discrimination is evaluated.", 0, 1, type)); 1613 children.add(new Property("path", "string", "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 0, 1, path)); 1614 } 1615 1616 @Override 1617 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1618 switch (_hash) { 1619 case 3575610: /*type*/ return new Property("type", "code", "How the element value is interpreted when discrimination is evaluated.", 0, 1, type); 1620 case 3433509: /*path*/ return new Property("path", "string", "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 0, 1, path); 1621 default: return super.getNamedProperty(_hash, _name, _checkValid); 1622 } 1623 1624 } 1625 1626 @Override 1627 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1628 switch (hash) { 1629 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DiscriminatorType> 1630 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1631 default: return super.getProperty(hash, name, checkValid); 1632 } 1633 1634 } 1635 1636 @Override 1637 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1638 switch (hash) { 1639 case 3575610: // type 1640 value = new DiscriminatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1641 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1642 return value; 1643 case 3433509: // path 1644 this.path = TypeConvertor.castToString(value); // StringType 1645 return value; 1646 default: return super.setProperty(hash, name, value); 1647 } 1648 1649 } 1650 1651 @Override 1652 public Base setProperty(String name, Base value) throws FHIRException { 1653 if (name.equals("type")) { 1654 value = new DiscriminatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1655 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1656 } else if (name.equals("path")) { 1657 this.path = TypeConvertor.castToString(value); // StringType 1658 } else 1659 return super.setProperty(name, value); 1660 return value; 1661 } 1662 1663 @Override 1664 public void removeChild(String name, Base value) throws FHIRException { 1665 if (name.equals("type")) { 1666 value = new DiscriminatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1667 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1668 } else if (name.equals("path")) { 1669 this.path = null; 1670 } else 1671 super.removeChild(name, value); 1672 1673 } 1674 1675 @Override 1676 public Base makeProperty(int hash, String name) throws FHIRException { 1677 switch (hash) { 1678 case 3575610: return getTypeElement(); 1679 case 3433509: return getPathElement(); 1680 default: return super.makeProperty(hash, name); 1681 } 1682 1683 } 1684 1685 @Override 1686 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1687 switch (hash) { 1688 case 3575610: /*type*/ return new String[] {"code"}; 1689 case 3433509: /*path*/ return new String[] {"string"}; 1690 default: return super.getTypesForProperty(hash, name); 1691 } 1692 1693 } 1694 1695 @Override 1696 public Base addChild(String name) throws FHIRException { 1697 if (name.equals("type")) { 1698 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.discriminator.type"); 1699 } 1700 else if (name.equals("path")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.discriminator.path"); 1702 } 1703 else 1704 return super.addChild(name); 1705 } 1706 1707 public ElementDefinitionSlicingDiscriminatorComponent copy() { 1708 ElementDefinitionSlicingDiscriminatorComponent dst = new ElementDefinitionSlicingDiscriminatorComponent(); 1709 copyValues(dst); 1710 return dst; 1711 } 1712 1713 public void copyValues(ElementDefinitionSlicingDiscriminatorComponent dst) { 1714 super.copyValues(dst); 1715 dst.type = type == null ? null : type.copy(); 1716 dst.path = path == null ? null : path.copy(); 1717 } 1718 1719 @Override 1720 public boolean equalsDeep(Base other_) { 1721 if (!super.equalsDeep(other_)) 1722 return false; 1723 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1724 return false; 1725 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1726 return compareDeep(type, o.type, true) && compareDeep(path, o.path, true); 1727 } 1728 1729 @Override 1730 public boolean equalsShallow(Base other_) { 1731 if (!super.equalsShallow(other_)) 1732 return false; 1733 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1734 return false; 1735 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1736 return compareValues(type, o.type, true) && compareValues(path, o.path, true); 1737 } 1738 1739 public boolean isEmpty() { 1740 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, path); 1741 } 1742 1743 public String fhirType() { 1744 return "ElementDefinition.slicing.discriminator"; 1745 1746 } 1747 1748 @Override 1749 public String toString() { 1750 return (type == null ? "??" : type.getCode()) + "="+(path == null ? "??" : path.asStringValue()); 1751 } 1752 1753 } 1754 1755 @Block() 1756 public static class ElementDefinitionBaseComponent extends Element implements IBaseDatatypeElement { 1757 /** 1758 * The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base. 1759 */ 1760 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1761 @Description(shortDefinition="Path that identifies the base element", formalDefinition="The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base." ) 1762 protected StringType path; 1763 1764 /** 1765 * Minimum cardinality of the base element identified by the path. 1766 */ 1767 @Child(name = "min", type = {UnsignedIntType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1768 @Description(shortDefinition="Min cardinality of the base element", formalDefinition="Minimum cardinality of the base element identified by the path." ) 1769 protected UnsignedIntType min; 1770 1771 /** 1772 * Maximum cardinality of the base element identified by the path. 1773 */ 1774 @Child(name = "max", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1775 @Description(shortDefinition="Max cardinality of the base element", formalDefinition="Maximum cardinality of the base element identified by the path." ) 1776 protected StringType max; 1777 1778 private static final long serialVersionUID = -1412704221L; 1779 1780 /** 1781 * Constructor 1782 */ 1783 public ElementDefinitionBaseComponent() { 1784 super(); 1785 } 1786 1787 /** 1788 * Constructor 1789 */ 1790 public ElementDefinitionBaseComponent(String path, int min, String max) { 1791 super(); 1792 this.setPath(path); 1793 this.setMin(min); 1794 this.setMax(max); 1795 } 1796 1797 /** 1798 * @return {@link #path} (The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1799 */ 1800 public StringType getPathElement() { 1801 if (this.path == null) 1802 if (Configuration.errorOnAutoCreate()) 1803 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.path"); 1804 else if (Configuration.doAutoCreate()) 1805 this.path = new StringType(); // bb 1806 return this.path; 1807 } 1808 1809 public boolean hasPathElement() { 1810 return this.path != null && !this.path.isEmpty(); 1811 } 1812 1813 public boolean hasPath() { 1814 return this.path != null && !this.path.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #path} (The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1819 */ 1820 public ElementDefinitionBaseComponent setPathElement(StringType value) { 1821 this.path = value; 1822 return this; 1823 } 1824 1825 /** 1826 * @return The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base. 1827 */ 1828 public String getPath() { 1829 return this.path == null ? null : this.path.getValue(); 1830 } 1831 1832 /** 1833 * @param value The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base. 1834 */ 1835 public ElementDefinitionBaseComponent setPath(String value) { 1836 if (this.path == null) 1837 this.path = new StringType(); 1838 this.path.setValue(value); 1839 return this; 1840 } 1841 1842 /** 1843 * @return {@link #min} (Minimum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 1844 */ 1845 public UnsignedIntType getMinElement() { 1846 if (this.min == null) 1847 if (Configuration.errorOnAutoCreate()) 1848 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.min"); 1849 else if (Configuration.doAutoCreate()) 1850 this.min = new UnsignedIntType(); // bb 1851 return this.min; 1852 } 1853 1854 public boolean hasMinElement() { 1855 return this.min != null && !this.min.isEmpty(); 1856 } 1857 1858 public boolean hasMin() { 1859 return this.min != null && !this.min.isEmpty(); 1860 } 1861 1862 /** 1863 * @param value {@link #min} (Minimum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 1864 */ 1865 public ElementDefinitionBaseComponent setMinElement(UnsignedIntType value) { 1866 this.min = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return Minimum cardinality of the base element identified by the path. 1872 */ 1873 public int getMin() { 1874 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 1875 } 1876 1877 /** 1878 * @param value Minimum cardinality of the base element identified by the path. 1879 */ 1880 public ElementDefinitionBaseComponent setMin(int value) { 1881 if (this.min == null) 1882 this.min = new UnsignedIntType(); 1883 this.min.setValue(value); 1884 return this; 1885 } 1886 1887 /** 1888 * @return {@link #max} (Maximum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 1889 */ 1890 public StringType getMaxElement() { 1891 if (this.max == null) 1892 if (Configuration.errorOnAutoCreate()) 1893 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.max"); 1894 else if (Configuration.doAutoCreate()) 1895 this.max = new StringType(); // bb 1896 return this.max; 1897 } 1898 1899 public boolean hasMaxElement() { 1900 return this.max != null && !this.max.isEmpty(); 1901 } 1902 1903 public boolean hasMax() { 1904 return this.max != null && !this.max.isEmpty(); 1905 } 1906 1907 /** 1908 * @param value {@link #max} (Maximum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 1909 */ 1910 public ElementDefinitionBaseComponent setMaxElement(StringType value) { 1911 this.max = value; 1912 return this; 1913 } 1914 1915 /** 1916 * @return Maximum cardinality of the base element identified by the path. 1917 */ 1918 public String getMax() { 1919 return this.max == null ? null : this.max.getValue(); 1920 } 1921 1922 /** 1923 * @param value Maximum cardinality of the base element identified by the path. 1924 */ 1925 public ElementDefinitionBaseComponent setMax(String value) { 1926 if (this.max == null) 1927 this.max = new StringType(); 1928 this.max.setValue(value); 1929 return this; 1930 } 1931 1932 protected void listChildren(List<Property> children) { 1933 super.listChildren(children); 1934 children.add(new Property("path", "string", "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.", 0, 1, path)); 1935 children.add(new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 0, 1, min)); 1936 children.add(new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max)); 1937 } 1938 1939 @Override 1940 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1941 switch (_hash) { 1942 case 3433509: /*path*/ return new Property("path", "string", "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.", 0, 1, path); 1943 case 108114: /*min*/ return new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 0, 1, min); 1944 case 107876: /*max*/ return new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max); 1945 default: return super.getNamedProperty(_hash, _name, _checkValid); 1946 } 1947 1948 } 1949 1950 @Override 1951 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1952 switch (hash) { 1953 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1954 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 1955 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 1956 default: return super.getProperty(hash, name, checkValid); 1957 } 1958 1959 } 1960 1961 @Override 1962 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1963 switch (hash) { 1964 case 3433509: // path 1965 this.path = TypeConvertor.castToString(value); // StringType 1966 return value; 1967 case 108114: // min 1968 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1969 return value; 1970 case 107876: // max 1971 this.max = TypeConvertor.castToString(value); // StringType 1972 return value; 1973 default: return super.setProperty(hash, name, value); 1974 } 1975 1976 } 1977 1978 @Override 1979 public Base setProperty(String name, Base value) throws FHIRException { 1980 if (name.equals("path")) { 1981 this.path = TypeConvertor.castToString(value); // StringType 1982 } else if (name.equals("min")) { 1983 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1984 } else if (name.equals("max")) { 1985 this.max = TypeConvertor.castToString(value); // StringType 1986 } else 1987 return super.setProperty(name, value); 1988 return value; 1989 } 1990 1991 @Override 1992 public void removeChild(String name, Base value) throws FHIRException { 1993 if (name.equals("path")) { 1994 this.path = null; 1995 } else if (name.equals("min")) { 1996 this.min = null; 1997 } else if (name.equals("max")) { 1998 this.max = null; 1999 } else 2000 super.removeChild(name, value); 2001 2002 } 2003 2004 @Override 2005 public Base makeProperty(int hash, String name) throws FHIRException { 2006 switch (hash) { 2007 case 3433509: return getPathElement(); 2008 case 108114: return getMinElement(); 2009 case 107876: return getMaxElement(); 2010 default: return super.makeProperty(hash, name); 2011 } 2012 2013 } 2014 2015 @Override 2016 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2017 switch (hash) { 2018 case 3433509: /*path*/ return new String[] {"string"}; 2019 case 108114: /*min*/ return new String[] {"unsignedInt"}; 2020 case 107876: /*max*/ return new String[] {"string"}; 2021 default: return super.getTypesForProperty(hash, name); 2022 } 2023 2024 } 2025 2026 @Override 2027 public Base addChild(String name) throws FHIRException { 2028 if (name.equals("path")) { 2029 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.base.path"); 2030 } 2031 else if (name.equals("min")) { 2032 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.base.min"); 2033 } 2034 else if (name.equals("max")) { 2035 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.base.max"); 2036 } 2037 else 2038 return super.addChild(name); 2039 } 2040 2041 public ElementDefinitionBaseComponent copy() { 2042 ElementDefinitionBaseComponent dst = new ElementDefinitionBaseComponent(); 2043 copyValues(dst); 2044 return dst; 2045 } 2046 2047 public void copyValues(ElementDefinitionBaseComponent dst) { 2048 super.copyValues(dst); 2049 dst.path = path == null ? null : path.copy(); 2050 dst.min = min == null ? null : min.copy(); 2051 dst.max = max == null ? null : max.copy(); 2052 } 2053 2054 @Override 2055 public boolean equalsDeep(Base other_) { 2056 if (!super.equalsDeep(other_)) 2057 return false; 2058 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2059 return false; 2060 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2061 return compareDeep(path, o.path, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 2062 ; 2063 } 2064 2065 @Override 2066 public boolean equalsShallow(Base other_) { 2067 if (!super.equalsShallow(other_)) 2068 return false; 2069 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2070 return false; 2071 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2072 return compareValues(path, o.path, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 2073 ; 2074 } 2075 2076 public boolean isEmpty() { 2077 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, min, max); 2078 } 2079 2080 public String fhirType() { 2081 return "ElementDefinition.base"; 2082 2083 } 2084 2085 } 2086 2087 @Block() 2088 public static class TypeRefComponent extends Element implements IBaseDatatypeElement { 2089 /** 2090 * URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 2091 */ 2092 @Child(name = "code", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2093 @Description(shortDefinition="Data type or Resource (reference to definition)", formalDefinition="URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models." ) 2094 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/elementdefinition-types") 2095 protected UriType code; 2096 2097 /** 2098 * Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide. 2099 */ 2100 @Child(name = "profile", type = {CanonicalType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2101 @Description(shortDefinition="Profiles (StructureDefinition or IG) - one must apply", formalDefinition="Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide." ) 2102 protected List<CanonicalType> profile; 2103 2104 /** 2105 * Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide. 2106 */ 2107 @Child(name = "targetProfile", type = {CanonicalType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2108 @Description(shortDefinition="Profile (StructureDefinition or IG) on the Reference/canonical target - one must apply", formalDefinition="Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide." ) 2109 protected List<CanonicalType> targetProfile; 2110 2111 /** 2112 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2113 */ 2114 @Child(name = "aggregation", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2115 @Description(shortDefinition="contained | referenced | bundled - how aggregated", formalDefinition="If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle." ) 2116 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-aggregation-mode") 2117 protected List<Enumeration<AggregationMode>> aggregation; 2118 2119 /** 2120 * Whether this reference needs to be version specific or version independent, or whether either can be used. 2121 */ 2122 @Child(name = "versioning", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2123 @Description(shortDefinition="either | independent | specific", formalDefinition="Whether this reference needs to be version specific or version independent, or whether either can be used." ) 2124 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reference-version-rules") 2125 protected Enumeration<ReferenceVersionRules> versioning; 2126 2127 private static final long serialVersionUID = 957891653L; 2128 2129 /** 2130 * Constructor 2131 */ 2132 public TypeRefComponent() { 2133 super(); 2134 } 2135 2136 /** 2137 * Constructor 2138 */ 2139 public TypeRefComponent(String code) { 2140 super(); 2141 this.setCode(code); 2142 } 2143 2144 /** 2145 * @return {@link #code} (URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2146 */ 2147 public UriType getCodeElement() { 2148 if (this.code == null) 2149 if (Configuration.errorOnAutoCreate()) 2150 throw new Error("Attempt to auto-create TypeRefComponent.code"); 2151 else if (Configuration.doAutoCreate()) 2152 this.code = new UriType(); // bb 2153 return this.code; 2154 } 2155 2156 public boolean hasCodeElement() { 2157 return this.code != null && !this.code.isEmpty(); 2158 } 2159 2160 public boolean hasCode() { 2161 return this.code != null && !this.code.isEmpty(); 2162 } 2163 2164 /** 2165 * @param value {@link #code} (URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2166 */ 2167 public TypeRefComponent setCodeElement(UriType value) { 2168 this.code = value; 2169 return this; 2170 } 2171 2172 /** 2173 * @return URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 2174 */ 2175 public String getCode() { 2176 return this.code == null ? null : this.code.getValue(); 2177 } 2178 2179 /** 2180 * @param value URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 2181 */ 2182 public TypeRefComponent setCode(String value) { 2183 if (this.code == null) 2184 this.code = new UriType(); 2185 this.code.setValue(value); 2186 return this; 2187 } 2188 2189 /** 2190 * @return {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2191 */ 2192 public List<CanonicalType> getProfile() { 2193 if (this.profile == null) 2194 this.profile = new ArrayList<CanonicalType>(); 2195 return this.profile; 2196 } 2197 2198 /** 2199 * @return Returns a reference to <code>this</code> for easy method chaining 2200 */ 2201 public TypeRefComponent setProfile(List<CanonicalType> theProfile) { 2202 this.profile = theProfile; 2203 return this; 2204 } 2205 2206 public boolean hasProfile() { 2207 if (this.profile == null) 2208 return false; 2209 for (CanonicalType item : this.profile) 2210 if (!item.isEmpty()) 2211 return true; 2212 return false; 2213 } 2214 2215 /** 2216 * @return {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2217 */ 2218 public CanonicalType addProfileElement() {//2 2219 CanonicalType t = new CanonicalType(); 2220 if (this.profile == null) 2221 this.profile = new ArrayList<CanonicalType>(); 2222 this.profile.add(t); 2223 return t; 2224 } 2225 2226 /** 2227 * @param value {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2228 */ 2229 public TypeRefComponent addProfile(String value) { //1 2230 CanonicalType t = new CanonicalType(); 2231 t.setValue(value); 2232 if (this.profile == null) 2233 this.profile = new ArrayList<CanonicalType>(); 2234 this.profile.add(t); 2235 return this; 2236 } 2237 2238 /** 2239 * @param value {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2240 */ 2241 public boolean hasProfile(String value) { 2242 if (this.profile == null) 2243 return false; 2244 for (CanonicalType v : this.profile) 2245 if (v.getValue().equals(value)) // canonical 2246 return true; 2247 return false; 2248 } 2249 2250 /** 2251 * @return {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2252 */ 2253 public List<CanonicalType> getTargetProfile() { 2254 if (this.targetProfile == null) 2255 this.targetProfile = new ArrayList<CanonicalType>(); 2256 return this.targetProfile; 2257 } 2258 2259 /** 2260 * @return Returns a reference to <code>this</code> for easy method chaining 2261 */ 2262 public TypeRefComponent setTargetProfile(List<CanonicalType> theTargetProfile) { 2263 this.targetProfile = theTargetProfile; 2264 return this; 2265 } 2266 2267 public boolean hasTargetProfile() { 2268 if (this.targetProfile == null) 2269 return false; 2270 for (CanonicalType item : this.targetProfile) 2271 if (!item.isEmpty()) 2272 return true; 2273 return false; 2274 } 2275 2276 /** 2277 * @return {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2278 */ 2279 public CanonicalType addTargetProfileElement() {//2 2280 CanonicalType t = new CanonicalType(); 2281 if (this.targetProfile == null) 2282 this.targetProfile = new ArrayList<CanonicalType>(); 2283 this.targetProfile.add(t); 2284 return t; 2285 } 2286 2287 /** 2288 * @param value {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2289 */ 2290 public TypeRefComponent addTargetProfile(String value) { //1 2291 CanonicalType t = new CanonicalType(); 2292 t.setValue(value); 2293 if (this.targetProfile == null) 2294 this.targetProfile = new ArrayList<CanonicalType>(); 2295 this.targetProfile.add(t); 2296 return this; 2297 } 2298 2299 /** 2300 * @param value {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2301 */ 2302 public boolean hasTargetProfile(String value) { 2303 if (this.targetProfile == null) 2304 return false; 2305 for (CanonicalType v : this.targetProfile) 2306 if (v.getValue().equals(value)) // canonical 2307 return true; 2308 return false; 2309 } 2310 2311 /** 2312 * @return {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2313 */ 2314 public List<Enumeration<AggregationMode>> getAggregation() { 2315 if (this.aggregation == null) 2316 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2317 return this.aggregation; 2318 } 2319 2320 /** 2321 * @return Returns a reference to <code>this</code> for easy method chaining 2322 */ 2323 public TypeRefComponent setAggregation(List<Enumeration<AggregationMode>> theAggregation) { 2324 this.aggregation = theAggregation; 2325 return this; 2326 } 2327 2328 public boolean hasAggregation() { 2329 if (this.aggregation == null) 2330 return false; 2331 for (Enumeration<AggregationMode> item : this.aggregation) 2332 if (!item.isEmpty()) 2333 return true; 2334 return false; 2335 } 2336 2337 /** 2338 * @return {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2339 */ 2340 public Enumeration<AggregationMode> addAggregationElement() {//2 2341 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2342 if (this.aggregation == null) 2343 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2344 this.aggregation.add(t); 2345 return t; 2346 } 2347 2348 /** 2349 * @param value {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2350 */ 2351 public TypeRefComponent addAggregation(AggregationMode value) { //1 2352 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2353 t.setValue(value); 2354 if (this.aggregation == null) 2355 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2356 this.aggregation.add(t); 2357 return this; 2358 } 2359 2360 /** 2361 * @param value {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2362 */ 2363 public boolean hasAggregation(AggregationMode value) { 2364 if (this.aggregation == null) 2365 return false; 2366 for (Enumeration<AggregationMode> v : this.aggregation) 2367 if (v.getValue().equals(value)) // code 2368 return true; 2369 return false; 2370 } 2371 2372 /** 2373 * @return {@link #versioning} (Whether this reference needs to be version specific or version independent, or whether either can be used.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 2374 */ 2375 public Enumeration<ReferenceVersionRules> getVersioningElement() { 2376 if (this.versioning == null) 2377 if (Configuration.errorOnAutoCreate()) 2378 throw new Error("Attempt to auto-create TypeRefComponent.versioning"); 2379 else if (Configuration.doAutoCreate()) 2380 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); // bb 2381 return this.versioning; 2382 } 2383 2384 public boolean hasVersioningElement() { 2385 return this.versioning != null && !this.versioning.isEmpty(); 2386 } 2387 2388 public boolean hasVersioning() { 2389 return this.versioning != null && !this.versioning.isEmpty(); 2390 } 2391 2392 /** 2393 * @param value {@link #versioning} (Whether this reference needs to be version specific or version independent, or whether either can be used.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 2394 */ 2395 public TypeRefComponent setVersioningElement(Enumeration<ReferenceVersionRules> value) { 2396 this.versioning = value; 2397 return this; 2398 } 2399 2400 /** 2401 * @return Whether this reference needs to be version specific or version independent, or whether either can be used. 2402 */ 2403 public ReferenceVersionRules getVersioning() { 2404 return this.versioning == null ? null : this.versioning.getValue(); 2405 } 2406 2407 /** 2408 * @param value Whether this reference needs to be version specific or version independent, or whether either can be used. 2409 */ 2410 public TypeRefComponent setVersioning(ReferenceVersionRules value) { 2411 if (value == null) 2412 this.versioning = null; 2413 else { 2414 if (this.versioning == null) 2415 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); 2416 this.versioning.setValue(value); 2417 } 2418 return this; 2419 } 2420 2421 protected void listChildren(List<Property> children) { 2422 super.listChildren(children); 2423 children.add(new Property("code", "uri", "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 0, 1, code)); 2424 children.add(new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, profile)); 2425 children.add(new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, targetProfile)); 2426 children.add(new Property("aggregation", "code", "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 0, java.lang.Integer.MAX_VALUE, aggregation)); 2427 children.add(new Property("versioning", "code", "Whether this reference needs to be version specific or version independent, or whether either can be used.", 0, 1, versioning)); 2428 } 2429 2430 @Override 2431 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2432 switch (_hash) { 2433 case 3059181: /*code*/ return new Property("code", "uri", "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 0, 1, code); 2434 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, profile); 2435 case 1994521304: /*targetProfile*/ return new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, targetProfile); 2436 case 841524962: /*aggregation*/ return new Property("aggregation", "code", "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 0, java.lang.Integer.MAX_VALUE, aggregation); 2437 case -670487542: /*versioning*/ return new Property("versioning", "code", "Whether this reference needs to be version specific or version independent, or whether either can be used.", 0, 1, versioning); 2438 default: return super.getNamedProperty(_hash, _name, _checkValid); 2439 } 2440 2441 } 2442 2443 @Override 2444 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2445 switch (hash) { 2446 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // UriType 2447 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 2448 case 1994521304: /*targetProfile*/ return this.targetProfile == null ? new Base[0] : this.targetProfile.toArray(new Base[this.targetProfile.size()]); // CanonicalType 2449 case 841524962: /*aggregation*/ return this.aggregation == null ? new Base[0] : this.aggregation.toArray(new Base[this.aggregation.size()]); // Enumeration<AggregationMode> 2450 case -670487542: /*versioning*/ return this.versioning == null ? new Base[0] : new Base[] {this.versioning}; // Enumeration<ReferenceVersionRules> 2451 default: return super.getProperty(hash, name, checkValid); 2452 } 2453 2454 } 2455 2456 @Override 2457 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2458 switch (hash) { 2459 case 3059181: // code 2460 this.code = TypeConvertor.castToUri(value); // UriType 2461 return value; 2462 case -309425751: // profile 2463 this.getProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2464 return value; 2465 case 1994521304: // targetProfile 2466 this.getTargetProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2467 return value; 2468 case 841524962: // aggregation 2469 value = new AggregationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2470 this.getAggregation().add((Enumeration) value); // Enumeration<AggregationMode> 2471 return value; 2472 case -670487542: // versioning 2473 value = new ReferenceVersionRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2474 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2475 return value; 2476 default: return super.setProperty(hash, name, value); 2477 } 2478 2479 } 2480 2481 @Override 2482 public Base setProperty(String name, Base value) throws FHIRException { 2483 if (name.equals("code")) { 2484 this.code = TypeConvertor.castToUri(value); // UriType 2485 } else if (name.equals("profile")) { 2486 this.getProfile().add(TypeConvertor.castToCanonical(value)); 2487 } else if (name.equals("targetProfile")) { 2488 this.getTargetProfile().add(TypeConvertor.castToCanonical(value)); 2489 } else if (name.equals("aggregation")) { 2490 value = new AggregationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2491 this.getAggregation().add((Enumeration) value); 2492 } else if (name.equals("versioning")) { 2493 value = new ReferenceVersionRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2494 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2495 } else 2496 return super.setProperty(name, value); 2497 return value; 2498 } 2499 2500 @Override 2501 public void removeChild(String name, Base value) throws FHIRException { 2502 if (name.equals("code")) { 2503 this.code = null; 2504 } else if (name.equals("profile")) { 2505 this.getProfile().remove(value); 2506 } else if (name.equals("targetProfile")) { 2507 this.getTargetProfile().remove(value); 2508 } else if (name.equals("aggregation")) { 2509 value = new AggregationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2510 this.getAggregation().remove((Enumeration) value); 2511 } else if (name.equals("versioning")) { 2512 value = new ReferenceVersionRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2513 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2514 } else 2515 super.removeChild(name, value); 2516 2517 } 2518 2519 @Override 2520 public Base makeProperty(int hash, String name) throws FHIRException { 2521 switch (hash) { 2522 case 3059181: return getCodeElement(); 2523 case -309425751: return addProfileElement(); 2524 case 1994521304: return addTargetProfileElement(); 2525 case 841524962: return addAggregationElement(); 2526 case -670487542: return getVersioningElement(); 2527 default: return super.makeProperty(hash, name); 2528 } 2529 2530 } 2531 2532 @Override 2533 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2534 switch (hash) { 2535 case 3059181: /*code*/ return new String[] {"uri"}; 2536 case -309425751: /*profile*/ return new String[] {"canonical"}; 2537 case 1994521304: /*targetProfile*/ return new String[] {"canonical"}; 2538 case 841524962: /*aggregation*/ return new String[] {"code"}; 2539 case -670487542: /*versioning*/ return new String[] {"code"}; 2540 default: return super.getTypesForProperty(hash, name); 2541 } 2542 2543 } 2544 2545 @Override 2546 public Base addChild(String name) throws FHIRException { 2547 if (name.equals("code")) { 2548 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.code"); 2549 } 2550 else if (name.equals("profile")) { 2551 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.profile"); 2552 } 2553 else if (name.equals("targetProfile")) { 2554 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.targetProfile"); 2555 } 2556 else if (name.equals("aggregation")) { 2557 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.aggregation"); 2558 } 2559 else if (name.equals("versioning")) { 2560 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.versioning"); 2561 } 2562 else 2563 return super.addChild(name); 2564 } 2565 2566 public TypeRefComponent copy() { 2567 TypeRefComponent dst = new TypeRefComponent(); 2568 copyValues(dst); 2569 return dst; 2570 } 2571 2572 public void copyValues(TypeRefComponent dst) { 2573 super.copyValues(dst); 2574 dst.code = code == null ? null : code.copy(); 2575 if (profile != null) { 2576 dst.profile = new ArrayList<CanonicalType>(); 2577 for (CanonicalType i : profile) 2578 dst.profile.add(i.copy()); 2579 }; 2580 if (targetProfile != null) { 2581 dst.targetProfile = new ArrayList<CanonicalType>(); 2582 for (CanonicalType i : targetProfile) 2583 dst.targetProfile.add(i.copy()); 2584 }; 2585 if (aggregation != null) { 2586 dst.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2587 for (Enumeration<AggregationMode> i : aggregation) 2588 dst.aggregation.add(i.copy()); 2589 }; 2590 dst.versioning = versioning == null ? null : versioning.copy(); 2591 } 2592 2593 @Override 2594 public boolean equalsDeep(Base other_) { 2595 if (!super.equalsDeep(other_)) 2596 return false; 2597 if (!(other_ instanceof TypeRefComponent)) 2598 return false; 2599 TypeRefComponent o = (TypeRefComponent) other_; 2600 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(targetProfile, o.targetProfile, true) 2601 && compareDeep(aggregation, o.aggregation, true) && compareDeep(versioning, o.versioning, true) 2602 ; 2603 } 2604 2605 @Override 2606 public boolean equalsShallow(Base other_) { 2607 if (!super.equalsShallow(other_)) 2608 return false; 2609 if (!(other_ instanceof TypeRefComponent)) 2610 return false; 2611 TypeRefComponent o = (TypeRefComponent) other_; 2612 return compareValues(code, o.code, true) && compareValues(profile, o.profile, true) && compareValues(targetProfile, o.targetProfile, true) 2613 && compareValues(aggregation, o.aggregation, true) && compareValues(versioning, o.versioning, true) 2614 ; 2615 } 2616 2617 public boolean isEmpty() { 2618 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, targetProfile 2619 , aggregation, versioning); 2620 } 2621 2622 public String fhirType() { 2623 return "ElementDefinition.type"; 2624 2625 } 2626 2627// added from java-adornments.txt: 2628public boolean hasTarget() { 2629 return Utilities.existsInList(getCode(), "Reference", "canonical", "CodeableReference"); 2630 } 2631 2632 /** 2633 * This code checks for the system prefix and returns the FHIR type 2634 * 2635 * @return 2636 */ 2637 public String getWorkingCode() { 2638 if (hasExtension(ToolingExtensions.EXT_FHIR_TYPE)) 2639 return getExtensionString(ToolingExtensions.EXT_FHIR_TYPE); 2640 if (!hasCodeElement()) 2641 return null; 2642 if (getCodeElement().hasExtension(ToolingExtensions.EXT_XML_TYPE)) { 2643 String s = getCodeElement().getExtensionString(ToolingExtensions.EXT_XML_TYPE); 2644 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date OR xsd:dateTime".equalsIgnoreCase(s)) 2645 return "dateTime"; 2646 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date".equalsIgnoreCase(s)) 2647 return "date"; 2648 if ("xsd:dateTime".equalsIgnoreCase(s)) 2649 return "instant"; 2650 if ("xsd:token".equals(s)) 2651 return "code"; 2652 if ("xsd:boolean".equals(s)) 2653 return "boolean"; 2654 if ("xsd:string".equals(s)) 2655 return "string"; 2656 if ("xsd:time".equals(s)) 2657 return "time"; 2658 if ("xsd:int".equals(s)) 2659 return "integer"; 2660 if ("xsd:decimal OR xsd:double".equals(s)) 2661 return "decimal"; 2662 if ("xsd:decimal".equalsIgnoreCase(s)) 2663 return "decimal"; 2664 if ("xsd:base64Binary".equalsIgnoreCase(s)) 2665 return "base64Binary"; 2666 if ("xsd:positiveInteger".equalsIgnoreCase(s)) 2667 return "positiveInt"; 2668 if ("xsd:nonNegativeInteger".equalsIgnoreCase(s)) 2669 return "unsignedInt"; 2670 if ("xsd:anyURI".equalsIgnoreCase(s)) 2671 return "uri"; 2672 if ("xhtml:div".equalsIgnoreCase(s)) 2673 return "xhtml"; 2674 2675 throw new Error("Unknown xml type '"+s+"'"); 2676 } 2677 return getCode(); 2678 } 2679 2680 @Override 2681 public String toString() { 2682 String res = getCode(); 2683 if (hasProfile()) { 2684 res = res + "{"; 2685 boolean first = true; 2686 for (CanonicalType s : getProfile()) { 2687 if (first) first = false; else res = res + "|"; 2688 res = res + s.getValue(); 2689 } 2690 res = res + "}"; 2691 } 2692 if (hasTargetProfile()) { 2693 res = res + "("; 2694 boolean first = true; 2695 for (CanonicalType s : getTargetProfile()) { 2696 if (first) first = false; else res = res + "|"; 2697 res = res + s.getValue(); 2698 } 2699 res = res + ")"; 2700 } 2701 return res; 2702 } 2703 2704 public String getName() { 2705 return getWorkingCode(); 2706 } 2707 2708 public boolean isResourceReference() { 2709 return "Reference".equals(getCode()) && hasTargetProfile(); 2710 } 2711// end addition 2712 } 2713 2714 @Block() 2715 public static class ElementDefinitionExampleComponent extends Element implements IBaseDatatypeElement { 2716 /** 2717 * Describes the purpose of this example among the set of examples. 2718 */ 2719 @Child(name = "label", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2720 @Description(shortDefinition="Describes the purpose of this example", formalDefinition="Describes the purpose of this example among the set of examples." ) 2721 protected StringType label; 2722 2723 /** 2724 * The actual value for the element, which must be one of the types allowed for this element. 2725 */ 2726 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=2, min=1, max=1, modifier=false, summary=true) 2727 @Description(shortDefinition="Value of Example (one of allowed types)", formalDefinition="The actual value for the element, which must be one of the types allowed for this element." ) 2728 protected DataType value; 2729 2730 private static final long serialVersionUID = 463190922L; 2731 2732 /** 2733 * Constructor 2734 */ 2735 public ElementDefinitionExampleComponent() { 2736 super(); 2737 } 2738 2739 /** 2740 * Constructor 2741 */ 2742 public ElementDefinitionExampleComponent(String label, DataType value) { 2743 super(); 2744 this.setLabel(label); 2745 this.setValue(value); 2746 } 2747 2748 /** 2749 * @return {@link #label} (Describes the purpose of this example among the set of examples.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2750 */ 2751 public StringType getLabelElement() { 2752 if (this.label == null) 2753 if (Configuration.errorOnAutoCreate()) 2754 throw new Error("Attempt to auto-create ElementDefinitionExampleComponent.label"); 2755 else if (Configuration.doAutoCreate()) 2756 this.label = new StringType(); // bb 2757 return this.label; 2758 } 2759 2760 public boolean hasLabelElement() { 2761 return this.label != null && !this.label.isEmpty(); 2762 } 2763 2764 public boolean hasLabel() { 2765 return this.label != null && !this.label.isEmpty(); 2766 } 2767 2768 /** 2769 * @param value {@link #label} (Describes the purpose of this example among the set of examples.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2770 */ 2771 public ElementDefinitionExampleComponent setLabelElement(StringType value) { 2772 this.label = value; 2773 return this; 2774 } 2775 2776 /** 2777 * @return Describes the purpose of this example among the set of examples. 2778 */ 2779 public String getLabel() { 2780 return this.label == null ? null : this.label.getValue(); 2781 } 2782 2783 /** 2784 * @param value Describes the purpose of this example among the set of examples. 2785 */ 2786 public ElementDefinitionExampleComponent setLabel(String value) { 2787 if (this.label == null) 2788 this.label = new StringType(); 2789 this.label.setValue(value); 2790 return this; 2791 } 2792 2793 /** 2794 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2795 */ 2796 public DataType getValue() { 2797 return this.value; 2798 } 2799 2800 /** 2801 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2802 */ 2803 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 2804 if (this.value == null) 2805 this.value = new Base64BinaryType(); 2806 if (!(this.value instanceof Base64BinaryType)) 2807 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 2808 return (Base64BinaryType) this.value; 2809 } 2810 2811 public boolean hasValueBase64BinaryType() { 2812 return this != null && this.value instanceof Base64BinaryType; 2813 } 2814 2815 /** 2816 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2817 */ 2818 public BooleanType getValueBooleanType() throws FHIRException { 2819 if (this.value == null) 2820 this.value = new BooleanType(); 2821 if (!(this.value instanceof BooleanType)) 2822 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2823 return (BooleanType) this.value; 2824 } 2825 2826 public boolean hasValueBooleanType() { 2827 return this != null && this.value instanceof BooleanType; 2828 } 2829 2830 /** 2831 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2832 */ 2833 public CanonicalType getValueCanonicalType() throws FHIRException { 2834 if (this.value == null) 2835 this.value = new CanonicalType(); 2836 if (!(this.value instanceof CanonicalType)) 2837 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2838 return (CanonicalType) this.value; 2839 } 2840 2841 public boolean hasValueCanonicalType() { 2842 return this != null && this.value instanceof CanonicalType; 2843 } 2844 2845 /** 2846 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2847 */ 2848 public CodeType getValueCodeType() throws FHIRException { 2849 if (this.value == null) 2850 this.value = new CodeType(); 2851 if (!(this.value instanceof CodeType)) 2852 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2853 return (CodeType) this.value; 2854 } 2855 2856 public boolean hasValueCodeType() { 2857 return this != null && this.value instanceof CodeType; 2858 } 2859 2860 /** 2861 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2862 */ 2863 public DateType getValueDateType() throws FHIRException { 2864 if (this.value == null) 2865 this.value = new DateType(); 2866 if (!(this.value instanceof DateType)) 2867 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 2868 return (DateType) this.value; 2869 } 2870 2871 public boolean hasValueDateType() { 2872 return this != null && this.value instanceof DateType; 2873 } 2874 2875 /** 2876 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2877 */ 2878 public DateTimeType getValueDateTimeType() throws FHIRException { 2879 if (this.value == null) 2880 this.value = new DateTimeType(); 2881 if (!(this.value instanceof DateTimeType)) 2882 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2883 return (DateTimeType) this.value; 2884 } 2885 2886 public boolean hasValueDateTimeType() { 2887 return this != null && this.value instanceof DateTimeType; 2888 } 2889 2890 /** 2891 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2892 */ 2893 public DecimalType getValueDecimalType() throws FHIRException { 2894 if (this.value == null) 2895 this.value = new DecimalType(); 2896 if (!(this.value instanceof DecimalType)) 2897 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2898 return (DecimalType) this.value; 2899 } 2900 2901 public boolean hasValueDecimalType() { 2902 return this != null && this.value instanceof DecimalType; 2903 } 2904 2905 /** 2906 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2907 */ 2908 public IdType getValueIdType() throws FHIRException { 2909 if (this.value == null) 2910 this.value = new IdType(); 2911 if (!(this.value instanceof IdType)) 2912 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 2913 return (IdType) this.value; 2914 } 2915 2916 public boolean hasValueIdType() { 2917 return this != null && this.value instanceof IdType; 2918 } 2919 2920 /** 2921 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2922 */ 2923 public InstantType getValueInstantType() throws FHIRException { 2924 if (this.value == null) 2925 this.value = new InstantType(); 2926 if (!(this.value instanceof InstantType)) 2927 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 2928 return (InstantType) this.value; 2929 } 2930 2931 public boolean hasValueInstantType() { 2932 return this != null && this.value instanceof InstantType; 2933 } 2934 2935 /** 2936 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2937 */ 2938 public IntegerType getValueIntegerType() throws FHIRException { 2939 if (this.value == null) 2940 this.value = new IntegerType(); 2941 if (!(this.value instanceof IntegerType)) 2942 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2943 return (IntegerType) this.value; 2944 } 2945 2946 public boolean hasValueIntegerType() { 2947 return this != null && this.value instanceof IntegerType; 2948 } 2949 2950 /** 2951 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2952 */ 2953 public Integer64Type getValueInteger64Type() throws FHIRException { 2954 if (this.value == null) 2955 this.value = new Integer64Type(); 2956 if (!(this.value instanceof Integer64Type)) 2957 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 2958 return (Integer64Type) this.value; 2959 } 2960 2961 public boolean hasValueInteger64Type() { 2962 return this != null && this.value instanceof Integer64Type; 2963 } 2964 2965 /** 2966 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2967 */ 2968 public MarkdownType getValueMarkdownType() throws FHIRException { 2969 if (this.value == null) 2970 this.value = new MarkdownType(); 2971 if (!(this.value instanceof MarkdownType)) 2972 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 2973 return (MarkdownType) this.value; 2974 } 2975 2976 public boolean hasValueMarkdownType() { 2977 return this != null && this.value instanceof MarkdownType; 2978 } 2979 2980 /** 2981 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2982 */ 2983 public OidType getValueOidType() throws FHIRException { 2984 if (this.value == null) 2985 this.value = new OidType(); 2986 if (!(this.value instanceof OidType)) 2987 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 2988 return (OidType) this.value; 2989 } 2990 2991 public boolean hasValueOidType() { 2992 return this != null && this.value instanceof OidType; 2993 } 2994 2995 /** 2996 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2997 */ 2998 public PositiveIntType getValuePositiveIntType() throws FHIRException { 2999 if (this.value == null) 3000 this.value = new PositiveIntType(); 3001 if (!(this.value instanceof PositiveIntType)) 3002 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 3003 return (PositiveIntType) this.value; 3004 } 3005 3006 public boolean hasValuePositiveIntType() { 3007 return this != null && this.value instanceof PositiveIntType; 3008 } 3009 3010 /** 3011 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3012 */ 3013 public StringType getValueStringType() throws FHIRException { 3014 if (this.value == null) 3015 this.value = new StringType(); 3016 if (!(this.value instanceof StringType)) 3017 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 3018 return (StringType) this.value; 3019 } 3020 3021 public boolean hasValueStringType() { 3022 return this != null && this.value instanceof StringType; 3023 } 3024 3025 /** 3026 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3027 */ 3028 public TimeType getValueTimeType() throws FHIRException { 3029 if (this.value == null) 3030 this.value = new TimeType(); 3031 if (!(this.value instanceof TimeType)) 3032 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 3033 return (TimeType) this.value; 3034 } 3035 3036 public boolean hasValueTimeType() { 3037 return this != null && this.value instanceof TimeType; 3038 } 3039 3040 /** 3041 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3042 */ 3043 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 3044 if (this.value == null) 3045 this.value = new UnsignedIntType(); 3046 if (!(this.value instanceof UnsignedIntType)) 3047 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 3048 return (UnsignedIntType) this.value; 3049 } 3050 3051 public boolean hasValueUnsignedIntType() { 3052 return this != null && this.value instanceof UnsignedIntType; 3053 } 3054 3055 /** 3056 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3057 */ 3058 public UriType getValueUriType() throws FHIRException { 3059 if (this.value == null) 3060 this.value = new UriType(); 3061 if (!(this.value instanceof UriType)) 3062 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 3063 return (UriType) this.value; 3064 } 3065 3066 public boolean hasValueUriType() { 3067 return this != null && this.value instanceof UriType; 3068 } 3069 3070 /** 3071 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3072 */ 3073 public UrlType getValueUrlType() throws FHIRException { 3074 if (this.value == null) 3075 this.value = new UrlType(); 3076 if (!(this.value instanceof UrlType)) 3077 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 3078 return (UrlType) this.value; 3079 } 3080 3081 public boolean hasValueUrlType() { 3082 return this != null && this.value instanceof UrlType; 3083 } 3084 3085 /** 3086 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3087 */ 3088 public UuidType getValueUuidType() throws FHIRException { 3089 if (this.value == null) 3090 this.value = new UuidType(); 3091 if (!(this.value instanceof UuidType)) 3092 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 3093 return (UuidType) this.value; 3094 } 3095 3096 public boolean hasValueUuidType() { 3097 return this != null && this.value instanceof UuidType; 3098 } 3099 3100 /** 3101 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3102 */ 3103 public Address getValueAddress() throws FHIRException { 3104 if (this.value == null) 3105 this.value = new Address(); 3106 if (!(this.value instanceof Address)) 3107 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 3108 return (Address) this.value; 3109 } 3110 3111 public boolean hasValueAddress() { 3112 return this != null && this.value instanceof Address; 3113 } 3114 3115 /** 3116 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3117 */ 3118 public Age getValueAge() throws FHIRException { 3119 if (this.value == null) 3120 this.value = new Age(); 3121 if (!(this.value instanceof Age)) 3122 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 3123 return (Age) this.value; 3124 } 3125 3126 public boolean hasValueAge() { 3127 return this != null && this.value instanceof Age; 3128 } 3129 3130 /** 3131 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3132 */ 3133 public Annotation getValueAnnotation() throws FHIRException { 3134 if (this.value == null) 3135 this.value = new Annotation(); 3136 if (!(this.value instanceof Annotation)) 3137 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 3138 return (Annotation) this.value; 3139 } 3140 3141 public boolean hasValueAnnotation() { 3142 return this != null && this.value instanceof Annotation; 3143 } 3144 3145 /** 3146 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3147 */ 3148 public Attachment getValueAttachment() throws FHIRException { 3149 if (this.value == null) 3150 this.value = new Attachment(); 3151 if (!(this.value instanceof Attachment)) 3152 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 3153 return (Attachment) this.value; 3154 } 3155 3156 public boolean hasValueAttachment() { 3157 return this != null && this.value instanceof Attachment; 3158 } 3159 3160 /** 3161 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3162 */ 3163 public CodeableConcept getValueCodeableConcept() throws FHIRException { 3164 if (this.value == null) 3165 this.value = new CodeableConcept(); 3166 if (!(this.value instanceof CodeableConcept)) 3167 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 3168 return (CodeableConcept) this.value; 3169 } 3170 3171 public boolean hasValueCodeableConcept() { 3172 return this != null && this.value instanceof CodeableConcept; 3173 } 3174 3175 /** 3176 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3177 */ 3178 public CodeableReference getValueCodeableReference() throws FHIRException { 3179 if (this.value == null) 3180 this.value = new CodeableReference(); 3181 if (!(this.value instanceof CodeableReference)) 3182 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 3183 return (CodeableReference) this.value; 3184 } 3185 3186 public boolean hasValueCodeableReference() { 3187 return this != null && this.value instanceof CodeableReference; 3188 } 3189 3190 /** 3191 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3192 */ 3193 public Coding getValueCoding() throws FHIRException { 3194 if (this.value == null) 3195 this.value = new Coding(); 3196 if (!(this.value instanceof Coding)) 3197 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 3198 return (Coding) this.value; 3199 } 3200 3201 public boolean hasValueCoding() { 3202 return this != null && this.value instanceof Coding; 3203 } 3204 3205 /** 3206 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3207 */ 3208 public ContactPoint getValueContactPoint() throws FHIRException { 3209 if (this.value == null) 3210 this.value = new ContactPoint(); 3211 if (!(this.value instanceof ContactPoint)) 3212 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 3213 return (ContactPoint) this.value; 3214 } 3215 3216 public boolean hasValueContactPoint() { 3217 return this != null && this.value instanceof ContactPoint; 3218 } 3219 3220 /** 3221 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3222 */ 3223 public Count getValueCount() throws FHIRException { 3224 if (this.value == null) 3225 this.value = new Count(); 3226 if (!(this.value instanceof Count)) 3227 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 3228 return (Count) this.value; 3229 } 3230 3231 public boolean hasValueCount() { 3232 return this != null && this.value instanceof Count; 3233 } 3234 3235 /** 3236 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3237 */ 3238 public Distance getValueDistance() throws FHIRException { 3239 if (this.value == null) 3240 this.value = new Distance(); 3241 if (!(this.value instanceof Distance)) 3242 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 3243 return (Distance) this.value; 3244 } 3245 3246 public boolean hasValueDistance() { 3247 return this != null && this.value instanceof Distance; 3248 } 3249 3250 /** 3251 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3252 */ 3253 public Duration getValueDuration() throws FHIRException { 3254 if (this.value == null) 3255 this.value = new Duration(); 3256 if (!(this.value instanceof Duration)) 3257 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 3258 return (Duration) this.value; 3259 } 3260 3261 public boolean hasValueDuration() { 3262 return this != null && this.value instanceof Duration; 3263 } 3264 3265 /** 3266 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3267 */ 3268 public HumanName getValueHumanName() throws FHIRException { 3269 if (this.value == null) 3270 this.value = new HumanName(); 3271 if (!(this.value instanceof HumanName)) 3272 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 3273 return (HumanName) this.value; 3274 } 3275 3276 public boolean hasValueHumanName() { 3277 return this != null && this.value instanceof HumanName; 3278 } 3279 3280 /** 3281 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3282 */ 3283 public Identifier getValueIdentifier() throws FHIRException { 3284 if (this.value == null) 3285 this.value = new Identifier(); 3286 if (!(this.value instanceof Identifier)) 3287 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 3288 return (Identifier) this.value; 3289 } 3290 3291 public boolean hasValueIdentifier() { 3292 return this != null && this.value instanceof Identifier; 3293 } 3294 3295 /** 3296 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3297 */ 3298 public Money getValueMoney() throws FHIRException { 3299 if (this.value == null) 3300 this.value = new Money(); 3301 if (!(this.value instanceof Money)) 3302 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 3303 return (Money) this.value; 3304 } 3305 3306 public boolean hasValueMoney() { 3307 return this != null && this.value instanceof Money; 3308 } 3309 3310 /** 3311 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3312 */ 3313 public Period getValuePeriod() throws FHIRException { 3314 if (this.value == null) 3315 this.value = new Period(); 3316 if (!(this.value instanceof Period)) 3317 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 3318 return (Period) this.value; 3319 } 3320 3321 public boolean hasValuePeriod() { 3322 return this != null && this.value instanceof Period; 3323 } 3324 3325 /** 3326 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3327 */ 3328 public Quantity getValueQuantity() throws FHIRException { 3329 if (this.value == null) 3330 this.value = new Quantity(); 3331 if (!(this.value instanceof Quantity)) 3332 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3333 return (Quantity) this.value; 3334 } 3335 3336 public boolean hasValueQuantity() { 3337 return this != null && this.value instanceof Quantity; 3338 } 3339 3340 /** 3341 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3342 */ 3343 public Range getValueRange() throws FHIRException { 3344 if (this.value == null) 3345 this.value = new Range(); 3346 if (!(this.value instanceof Range)) 3347 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 3348 return (Range) this.value; 3349 } 3350 3351 public boolean hasValueRange() { 3352 return this != null && this.value instanceof Range; 3353 } 3354 3355 /** 3356 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3357 */ 3358 public Ratio getValueRatio() throws FHIRException { 3359 if (this.value == null) 3360 this.value = new Ratio(); 3361 if (!(this.value instanceof Ratio)) 3362 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 3363 return (Ratio) this.value; 3364 } 3365 3366 public boolean hasValueRatio() { 3367 return this != null && this.value instanceof Ratio; 3368 } 3369 3370 /** 3371 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3372 */ 3373 public RatioRange getValueRatioRange() throws FHIRException { 3374 if (this.value == null) 3375 this.value = new RatioRange(); 3376 if (!(this.value instanceof RatioRange)) 3377 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 3378 return (RatioRange) this.value; 3379 } 3380 3381 public boolean hasValueRatioRange() { 3382 return this != null && this.value instanceof RatioRange; 3383 } 3384 3385 /** 3386 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3387 */ 3388 public Reference getValueReference() throws FHIRException { 3389 if (this.value == null) 3390 this.value = new Reference(); 3391 if (!(this.value instanceof Reference)) 3392 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 3393 return (Reference) this.value; 3394 } 3395 3396 public boolean hasValueReference() { 3397 return this != null && this.value instanceof Reference; 3398 } 3399 3400 /** 3401 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3402 */ 3403 public SampledData getValueSampledData() throws FHIRException { 3404 if (this.value == null) 3405 this.value = new SampledData(); 3406 if (!(this.value instanceof SampledData)) 3407 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 3408 return (SampledData) this.value; 3409 } 3410 3411 public boolean hasValueSampledData() { 3412 return this != null && this.value instanceof SampledData; 3413 } 3414 3415 /** 3416 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3417 */ 3418 public Signature getValueSignature() throws FHIRException { 3419 if (this.value == null) 3420 this.value = new Signature(); 3421 if (!(this.value instanceof Signature)) 3422 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 3423 return (Signature) this.value; 3424 } 3425 3426 public boolean hasValueSignature() { 3427 return this != null && this.value instanceof Signature; 3428 } 3429 3430 /** 3431 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3432 */ 3433 public Timing getValueTiming() throws FHIRException { 3434 if (this.value == null) 3435 this.value = new Timing(); 3436 if (!(this.value instanceof Timing)) 3437 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 3438 return (Timing) this.value; 3439 } 3440 3441 public boolean hasValueTiming() { 3442 return this != null && this.value instanceof Timing; 3443 } 3444 3445 /** 3446 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3447 */ 3448 public ContactDetail getValueContactDetail() throws FHIRException { 3449 if (this.value == null) 3450 this.value = new ContactDetail(); 3451 if (!(this.value instanceof ContactDetail)) 3452 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 3453 return (ContactDetail) this.value; 3454 } 3455 3456 public boolean hasValueContactDetail() { 3457 return this != null && this.value instanceof ContactDetail; 3458 } 3459 3460 /** 3461 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3462 */ 3463 public DataRequirement getValueDataRequirement() throws FHIRException { 3464 if (this.value == null) 3465 this.value = new DataRequirement(); 3466 if (!(this.value instanceof DataRequirement)) 3467 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 3468 return (DataRequirement) this.value; 3469 } 3470 3471 public boolean hasValueDataRequirement() { 3472 return this != null && this.value instanceof DataRequirement; 3473 } 3474 3475 /** 3476 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3477 */ 3478 public Expression getValueExpression() throws FHIRException { 3479 if (this.value == null) 3480 this.value = new Expression(); 3481 if (!(this.value instanceof Expression)) 3482 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 3483 return (Expression) this.value; 3484 } 3485 3486 public boolean hasValueExpression() { 3487 return this != null && this.value instanceof Expression; 3488 } 3489 3490 /** 3491 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3492 */ 3493 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 3494 if (this.value == null) 3495 this.value = new ParameterDefinition(); 3496 if (!(this.value instanceof ParameterDefinition)) 3497 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 3498 return (ParameterDefinition) this.value; 3499 } 3500 3501 public boolean hasValueParameterDefinition() { 3502 return this != null && this.value instanceof ParameterDefinition; 3503 } 3504 3505 /** 3506 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3507 */ 3508 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 3509 if (this.value == null) 3510 this.value = new RelatedArtifact(); 3511 if (!(this.value instanceof RelatedArtifact)) 3512 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 3513 return (RelatedArtifact) this.value; 3514 } 3515 3516 public boolean hasValueRelatedArtifact() { 3517 return this != null && this.value instanceof RelatedArtifact; 3518 } 3519 3520 /** 3521 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3522 */ 3523 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 3524 if (this.value == null) 3525 this.value = new TriggerDefinition(); 3526 if (!(this.value instanceof TriggerDefinition)) 3527 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 3528 return (TriggerDefinition) this.value; 3529 } 3530 3531 public boolean hasValueTriggerDefinition() { 3532 return this != null && this.value instanceof TriggerDefinition; 3533 } 3534 3535 /** 3536 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3537 */ 3538 public UsageContext getValueUsageContext() throws FHIRException { 3539 if (this.value == null) 3540 this.value = new UsageContext(); 3541 if (!(this.value instanceof UsageContext)) 3542 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 3543 return (UsageContext) this.value; 3544 } 3545 3546 public boolean hasValueUsageContext() { 3547 return this != null && this.value instanceof UsageContext; 3548 } 3549 3550 /** 3551 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3552 */ 3553 public Availability getValueAvailability() throws FHIRException { 3554 if (this.value == null) 3555 this.value = new Availability(); 3556 if (!(this.value instanceof Availability)) 3557 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 3558 return (Availability) this.value; 3559 } 3560 3561 public boolean hasValueAvailability() { 3562 return this != null && this.value instanceof Availability; 3563 } 3564 3565 /** 3566 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3567 */ 3568 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 3569 if (this.value == null) 3570 this.value = new ExtendedContactDetail(); 3571 if (!(this.value instanceof ExtendedContactDetail)) 3572 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 3573 return (ExtendedContactDetail) this.value; 3574 } 3575 3576 public boolean hasValueExtendedContactDetail() { 3577 return this != null && this.value instanceof ExtendedContactDetail; 3578 } 3579 3580 /** 3581 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3582 */ 3583 public Dosage getValueDosage() throws FHIRException { 3584 if (this.value == null) 3585 this.value = new Dosage(); 3586 if (!(this.value instanceof Dosage)) 3587 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 3588 return (Dosage) this.value; 3589 } 3590 3591 public boolean hasValueDosage() { 3592 return this != null && this.value instanceof Dosage; 3593 } 3594 3595 /** 3596 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3597 */ 3598 public Meta getValueMeta() throws FHIRException { 3599 if (this.value == null) 3600 this.value = new Meta(); 3601 if (!(this.value instanceof Meta)) 3602 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 3603 return (Meta) this.value; 3604 } 3605 3606 public boolean hasValueMeta() { 3607 return this != null && this.value instanceof Meta; 3608 } 3609 3610 public boolean hasValue() { 3611 return this.value != null && !this.value.isEmpty(); 3612 } 3613 3614 /** 3615 * @param value {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3616 */ 3617 public ElementDefinitionExampleComponent setValue(DataType value) { 3618 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 3619 throw new FHIRException("Not the right type for ElementDefinition.example.value[x]: "+value.fhirType()); 3620 this.value = value; 3621 return this; 3622 } 3623 3624 protected void listChildren(List<Property> children) { 3625 super.listChildren(children); 3626 children.add(new Property("label", "string", "Describes the purpose of this example among the set of examples.", 0, 1, label)); 3627 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value)); 3628 } 3629 3630 @Override 3631 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3632 switch (_hash) { 3633 case 102727412: /*label*/ return new Property("label", "string", "Describes the purpose of this example among the set of examples.", 0, 1, label); 3634 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3635 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3636 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3637 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3638 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3639 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3640 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3641 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3642 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3643 case 231604844: /*valueId*/ return new Property("value[x]", "id", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3644 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3645 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3646 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3647 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3648 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3649 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3650 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3651 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3652 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3653 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3654 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3655 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3656 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3657 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3658 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3659 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3660 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3661 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3662 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3663 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3664 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3665 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3666 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3667 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3668 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3669 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3670 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3671 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3672 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3673 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3674 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3675 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3676 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3677 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3678 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3679 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3680 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3681 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3682 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3683 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3684 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3685 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3686 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3687 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3688 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3689 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3690 default: return super.getNamedProperty(_hash, _name, _checkValid); 3691 } 3692 3693 } 3694 3695 @Override 3696 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3697 switch (hash) { 3698 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 3699 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3700 default: return super.getProperty(hash, name, checkValid); 3701 } 3702 3703 } 3704 3705 @Override 3706 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3707 switch (hash) { 3708 case 102727412: // label 3709 this.label = TypeConvertor.castToString(value); // StringType 3710 return value; 3711 case 111972721: // value 3712 this.value = TypeConvertor.castToType(value); // DataType 3713 return value; 3714 default: return super.setProperty(hash, name, value); 3715 } 3716 3717 } 3718 3719 @Override 3720 public Base setProperty(String name, Base value) throws FHIRException { 3721 if (name.equals("label")) { 3722 this.label = TypeConvertor.castToString(value); // StringType 3723 } else if (name.equals("value[x]")) { 3724 this.value = TypeConvertor.castToType(value); // DataType 3725 } else 3726 return super.setProperty(name, value); 3727 return value; 3728 } 3729 3730 @Override 3731 public void removeChild(String name, Base value) throws FHIRException { 3732 if (name.equals("label")) { 3733 this.label = null; 3734 } else if (name.equals("value[x]")) { 3735 this.value = null; 3736 } else 3737 super.removeChild(name, value); 3738 3739 } 3740 3741 @Override 3742 public Base makeProperty(int hash, String name) throws FHIRException { 3743 switch (hash) { 3744 case 102727412: return getLabelElement(); 3745 case -1410166417: return getValue(); 3746 case 111972721: return getValue(); 3747 default: return super.makeProperty(hash, name); 3748 } 3749 3750 } 3751 3752 @Override 3753 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3754 switch (hash) { 3755 case 102727412: /*label*/ return new String[] {"string"}; 3756 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 3757 default: return super.getTypesForProperty(hash, name); 3758 } 3759 3760 } 3761 3762 @Override 3763 public Base addChild(String name) throws FHIRException { 3764 if (name.equals("label")) { 3765 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.example.label"); 3766 } 3767 else if (name.equals("valueBase64Binary")) { 3768 this.value = new Base64BinaryType(); 3769 return this.value; 3770 } 3771 else if (name.equals("valueBoolean")) { 3772 this.value = new BooleanType(); 3773 return this.value; 3774 } 3775 else if (name.equals("valueCanonical")) { 3776 this.value = new CanonicalType(); 3777 return this.value; 3778 } 3779 else if (name.equals("valueCode")) { 3780 this.value = new CodeType(); 3781 return this.value; 3782 } 3783 else if (name.equals("valueDate")) { 3784 this.value = new DateType(); 3785 return this.value; 3786 } 3787 else if (name.equals("valueDateTime")) { 3788 this.value = new DateTimeType(); 3789 return this.value; 3790 } 3791 else if (name.equals("valueDecimal")) { 3792 this.value = new DecimalType(); 3793 return this.value; 3794 } 3795 else if (name.equals("valueId")) { 3796 this.value = new IdType(); 3797 return this.value; 3798 } 3799 else if (name.equals("valueInstant")) { 3800 this.value = new InstantType(); 3801 return this.value; 3802 } 3803 else if (name.equals("valueInteger")) { 3804 this.value = new IntegerType(); 3805 return this.value; 3806 } 3807 else if (name.equals("valueInteger64")) { 3808 this.value = new Integer64Type(); 3809 return this.value; 3810 } 3811 else if (name.equals("valueMarkdown")) { 3812 this.value = new MarkdownType(); 3813 return this.value; 3814 } 3815 else if (name.equals("valueOid")) { 3816 this.value = new OidType(); 3817 return this.value; 3818 } 3819 else if (name.equals("valuePositiveInt")) { 3820 this.value = new PositiveIntType(); 3821 return this.value; 3822 } 3823 else if (name.equals("valueString")) { 3824 this.value = new StringType(); 3825 return this.value; 3826 } 3827 else if (name.equals("valueTime")) { 3828 this.value = new TimeType(); 3829 return this.value; 3830 } 3831 else if (name.equals("valueUnsignedInt")) { 3832 this.value = new UnsignedIntType(); 3833 return this.value; 3834 } 3835 else if (name.equals("valueUri")) { 3836 this.value = new UriType(); 3837 return this.value; 3838 } 3839 else if (name.equals("valueUrl")) { 3840 this.value = new UrlType(); 3841 return this.value; 3842 } 3843 else if (name.equals("valueUuid")) { 3844 this.value = new UuidType(); 3845 return this.value; 3846 } 3847 else if (name.equals("valueAddress")) { 3848 this.value = new Address(); 3849 return this.value; 3850 } 3851 else if (name.equals("valueAge")) { 3852 this.value = new Age(); 3853 return this.value; 3854 } 3855 else if (name.equals("valueAnnotation")) { 3856 this.value = new Annotation(); 3857 return this.value; 3858 } 3859 else if (name.equals("valueAttachment")) { 3860 this.value = new Attachment(); 3861 return this.value; 3862 } 3863 else if (name.equals("valueCodeableConcept")) { 3864 this.value = new CodeableConcept(); 3865 return this.value; 3866 } 3867 else if (name.equals("valueCodeableReference")) { 3868 this.value = new CodeableReference(); 3869 return this.value; 3870 } 3871 else if (name.equals("valueCoding")) { 3872 this.value = new Coding(); 3873 return this.value; 3874 } 3875 else if (name.equals("valueContactPoint")) { 3876 this.value = new ContactPoint(); 3877 return this.value; 3878 } 3879 else if (name.equals("valueCount")) { 3880 this.value = new Count(); 3881 return this.value; 3882 } 3883 else if (name.equals("valueDistance")) { 3884 this.value = new Distance(); 3885 return this.value; 3886 } 3887 else if (name.equals("valueDuration")) { 3888 this.value = new Duration(); 3889 return this.value; 3890 } 3891 else if (name.equals("valueHumanName")) { 3892 this.value = new HumanName(); 3893 return this.value; 3894 } 3895 else if (name.equals("valueIdentifier")) { 3896 this.value = new Identifier(); 3897 return this.value; 3898 } 3899 else if (name.equals("valueMoney")) { 3900 this.value = new Money(); 3901 return this.value; 3902 } 3903 else if (name.equals("valuePeriod")) { 3904 this.value = new Period(); 3905 return this.value; 3906 } 3907 else if (name.equals("valueQuantity")) { 3908 this.value = new Quantity(); 3909 return this.value; 3910 } 3911 else if (name.equals("valueRange")) { 3912 this.value = new Range(); 3913 return this.value; 3914 } 3915 else if (name.equals("valueRatio")) { 3916 this.value = new Ratio(); 3917 return this.value; 3918 } 3919 else if (name.equals("valueRatioRange")) { 3920 this.value = new RatioRange(); 3921 return this.value; 3922 } 3923 else if (name.equals("valueReference")) { 3924 this.value = new Reference(); 3925 return this.value; 3926 } 3927 else if (name.equals("valueSampledData")) { 3928 this.value = new SampledData(); 3929 return this.value; 3930 } 3931 else if (name.equals("valueSignature")) { 3932 this.value = new Signature(); 3933 return this.value; 3934 } 3935 else if (name.equals("valueTiming")) { 3936 this.value = new Timing(); 3937 return this.value; 3938 } 3939 else if (name.equals("valueContactDetail")) { 3940 this.value = new ContactDetail(); 3941 return this.value; 3942 } 3943 else if (name.equals("valueDataRequirement")) { 3944 this.value = new DataRequirement(); 3945 return this.value; 3946 } 3947 else if (name.equals("valueExpression")) { 3948 this.value = new Expression(); 3949 return this.value; 3950 } 3951 else if (name.equals("valueParameterDefinition")) { 3952 this.value = new ParameterDefinition(); 3953 return this.value; 3954 } 3955 else if (name.equals("valueRelatedArtifact")) { 3956 this.value = new RelatedArtifact(); 3957 return this.value; 3958 } 3959 else if (name.equals("valueTriggerDefinition")) { 3960 this.value = new TriggerDefinition(); 3961 return this.value; 3962 } 3963 else if (name.equals("valueUsageContext")) { 3964 this.value = new UsageContext(); 3965 return this.value; 3966 } 3967 else if (name.equals("valueAvailability")) { 3968 this.value = new Availability(); 3969 return this.value; 3970 } 3971 else if (name.equals("valueExtendedContactDetail")) { 3972 this.value = new ExtendedContactDetail(); 3973 return this.value; 3974 } 3975 else if (name.equals("valueDosage")) { 3976 this.value = new Dosage(); 3977 return this.value; 3978 } 3979 else if (name.equals("valueMeta")) { 3980 this.value = new Meta(); 3981 return this.value; 3982 } 3983 else 3984 return super.addChild(name); 3985 } 3986 3987 public ElementDefinitionExampleComponent copy() { 3988 ElementDefinitionExampleComponent dst = new ElementDefinitionExampleComponent(); 3989 copyValues(dst); 3990 return dst; 3991 } 3992 3993 public void copyValues(ElementDefinitionExampleComponent dst) { 3994 super.copyValues(dst); 3995 dst.label = label == null ? null : label.copy(); 3996 dst.value = value == null ? null : value.copy(); 3997 } 3998 3999 @Override 4000 public boolean equalsDeep(Base other_) { 4001 if (!super.equalsDeep(other_)) 4002 return false; 4003 if (!(other_ instanceof ElementDefinitionExampleComponent)) 4004 return false; 4005 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 4006 return compareDeep(label, o.label, true) && compareDeep(value, o.value, true); 4007 } 4008 4009 @Override 4010 public boolean equalsShallow(Base other_) { 4011 if (!super.equalsShallow(other_)) 4012 return false; 4013 if (!(other_ instanceof ElementDefinitionExampleComponent)) 4014 return false; 4015 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 4016 return compareValues(label, o.label, true); 4017 } 4018 4019 public boolean isEmpty() { 4020 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, value); 4021 } 4022 4023 public String fhirType() { 4024 return "ElementDefinition.example"; 4025 4026 } 4027 4028 } 4029 4030 @Block() 4031 public static class ElementDefinitionConstraintComponent extends Element implements IBaseDatatypeElement { 4032 /** 4033 * Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 4034 */ 4035 @Child(name = "key", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4036 @Description(shortDefinition="Target of 'condition' reference above", formalDefinition="Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality." ) 4037 protected IdType key; 4038 4039 /** 4040 * Description of why this constraint is necessary or appropriate. 4041 */ 4042 @Child(name = "requirements", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4043 @Description(shortDefinition="Why this constraint is necessary or appropriate", formalDefinition="Description of why this constraint is necessary or appropriate." ) 4044 protected MarkdownType requirements; 4045 4046 /** 4047 * Identifies the impact constraint violation has on the conformance of the instance. 4048 */ 4049 @Child(name = "severity", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 4050 @Description(shortDefinition="error | warning", formalDefinition="Identifies the impact constraint violation has on the conformance of the instance." ) 4051 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/constraint-severity") 4052 protected Enumeration<ConstraintSeverity> severity; 4053 4054 /** 4055 * If true, indicates that the warning or best practice guideline should be suppressed. 4056 */ 4057 @Child(name = "suppress", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 4058 @Description(shortDefinition="Suppress warning or hint in profile", formalDefinition="If true, indicates that the warning or best practice guideline should be suppressed." ) 4059 protected BooleanType suppress; 4060 4061 /** 4062 * Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 4063 */ 4064 @Child(name = "human", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=true) 4065 @Description(shortDefinition="Human description of constraint", formalDefinition="Text that can be used to describe the constraint in messages identifying that the constraint has been violated." ) 4066 protected StringType human; 4067 4068 /** 4069 * A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met. 4070 */ 4071 @Child(name = "expression", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 4072 @Description(shortDefinition="FHIRPath expression of constraint", formalDefinition="A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met." ) 4073 protected StringType expression; 4074 4075 /** 4076 * A reference to the original source of the constraint, for traceability purposes. 4077 */ 4078 @Child(name = "source", type = {CanonicalType.class}, order=7, min=0, max=1, modifier=false, summary=true) 4079 @Description(shortDefinition="Reference to original source of constraint", formalDefinition="A reference to the original source of the constraint, for traceability purposes." ) 4080 protected CanonicalType source; 4081 4082 private static final long serialVersionUID = 1642607838L; 4083 4084 /** 4085 * Constructor 4086 */ 4087 public ElementDefinitionConstraintComponent() { 4088 super(); 4089 } 4090 4091 /** 4092 * Constructor 4093 */ 4094 public ElementDefinitionConstraintComponent(String key, ConstraintSeverity severity, String human) { 4095 super(); 4096 this.setKey(key); 4097 this.setSeverity(severity); 4098 this.setHuman(human); 4099 } 4100 4101 /** 4102 * @return {@link #key} (Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 4103 */ 4104 public IdType getKeyElement() { 4105 if (this.key == null) 4106 if (Configuration.errorOnAutoCreate()) 4107 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.key"); 4108 else if (Configuration.doAutoCreate()) 4109 this.key = new IdType(); // bb 4110 return this.key; 4111 } 4112 4113 public boolean hasKeyElement() { 4114 return this.key != null && !this.key.isEmpty(); 4115 } 4116 4117 public boolean hasKey() { 4118 return this.key != null && !this.key.isEmpty(); 4119 } 4120 4121 /** 4122 * @param value {@link #key} (Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 4123 */ 4124 public ElementDefinitionConstraintComponent setKeyElement(IdType value) { 4125 this.key = value; 4126 return this; 4127 } 4128 4129 /** 4130 * @return Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 4131 */ 4132 public String getKey() { 4133 return this.key == null ? null : this.key.getValue(); 4134 } 4135 4136 /** 4137 * @param value Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 4138 */ 4139 public ElementDefinitionConstraintComponent setKey(String value) { 4140 if (this.key == null) 4141 this.key = new IdType(); 4142 this.key.setValue(value); 4143 return this; 4144 } 4145 4146 /** 4147 * @return {@link #requirements} (Description of why this constraint is necessary or appropriate.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 4148 */ 4149 public MarkdownType getRequirementsElement() { 4150 if (this.requirements == null) 4151 if (Configuration.errorOnAutoCreate()) 4152 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.requirements"); 4153 else if (Configuration.doAutoCreate()) 4154 this.requirements = new MarkdownType(); // bb 4155 return this.requirements; 4156 } 4157 4158 public boolean hasRequirementsElement() { 4159 return this.requirements != null && !this.requirements.isEmpty(); 4160 } 4161 4162 public boolean hasRequirements() { 4163 return this.requirements != null && !this.requirements.isEmpty(); 4164 } 4165 4166 /** 4167 * @param value {@link #requirements} (Description of why this constraint is necessary or appropriate.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 4168 */ 4169 public ElementDefinitionConstraintComponent setRequirementsElement(MarkdownType value) { 4170 this.requirements = value; 4171 return this; 4172 } 4173 4174 /** 4175 * @return Description of why this constraint is necessary or appropriate. 4176 */ 4177 public String getRequirements() { 4178 return this.requirements == null ? null : this.requirements.getValue(); 4179 } 4180 4181 /** 4182 * @param value Description of why this constraint is necessary or appropriate. 4183 */ 4184 public ElementDefinitionConstraintComponent setRequirements(String value) { 4185 if (Utilities.noString(value)) 4186 this.requirements = null; 4187 else { 4188 if (this.requirements == null) 4189 this.requirements = new MarkdownType(); 4190 this.requirements.setValue(value); 4191 } 4192 return this; 4193 } 4194 4195 /** 4196 * @return {@link #severity} (Identifies the impact constraint violation has on the conformance of the instance.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 4197 */ 4198 public Enumeration<ConstraintSeverity> getSeverityElement() { 4199 if (this.severity == null) 4200 if (Configuration.errorOnAutoCreate()) 4201 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.severity"); 4202 else if (Configuration.doAutoCreate()) 4203 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); // bb 4204 return this.severity; 4205 } 4206 4207 public boolean hasSeverityElement() { 4208 return this.severity != null && !this.severity.isEmpty(); 4209 } 4210 4211 public boolean hasSeverity() { 4212 return this.severity != null && !this.severity.isEmpty(); 4213 } 4214 4215 /** 4216 * @param value {@link #severity} (Identifies the impact constraint violation has on the conformance of the instance.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 4217 */ 4218 public ElementDefinitionConstraintComponent setSeverityElement(Enumeration<ConstraintSeverity> value) { 4219 this.severity = value; 4220 return this; 4221 } 4222 4223 /** 4224 * @return Identifies the impact constraint violation has on the conformance of the instance. 4225 */ 4226 public ConstraintSeverity getSeverity() { 4227 return this.severity == null ? null : this.severity.getValue(); 4228 } 4229 4230 /** 4231 * @param value Identifies the impact constraint violation has on the conformance of the instance. 4232 */ 4233 public ElementDefinitionConstraintComponent setSeverity(ConstraintSeverity value) { 4234 if (this.severity == null) 4235 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); 4236 this.severity.setValue(value); 4237 return this; 4238 } 4239 4240 /** 4241 * @return {@link #suppress} (If true, indicates that the warning or best practice guideline should be suppressed.). This is the underlying object with id, value and extensions. The accessor "getSuppress" gives direct access to the value 4242 */ 4243 public BooleanType getSuppressElement() { 4244 if (this.suppress == null) 4245 if (Configuration.errorOnAutoCreate()) 4246 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.suppress"); 4247 else if (Configuration.doAutoCreate()) 4248 this.suppress = new BooleanType(); // bb 4249 return this.suppress; 4250 } 4251 4252 public boolean hasSuppressElement() { 4253 return this.suppress != null && !this.suppress.isEmpty(); 4254 } 4255 4256 public boolean hasSuppress() { 4257 return this.suppress != null && !this.suppress.isEmpty(); 4258 } 4259 4260 /** 4261 * @param value {@link #suppress} (If true, indicates that the warning or best practice guideline should be suppressed.). This is the underlying object with id, value and extensions. The accessor "getSuppress" gives direct access to the value 4262 */ 4263 public ElementDefinitionConstraintComponent setSuppressElement(BooleanType value) { 4264 this.suppress = value; 4265 return this; 4266 } 4267 4268 /** 4269 * @return If true, indicates that the warning or best practice guideline should be suppressed. 4270 */ 4271 public boolean getSuppress() { 4272 return this.suppress == null || this.suppress.isEmpty() ? false : this.suppress.getValue(); 4273 } 4274 4275 /** 4276 * @param value If true, indicates that the warning or best practice guideline should be suppressed. 4277 */ 4278 public ElementDefinitionConstraintComponent setSuppress(boolean value) { 4279 if (this.suppress == null) 4280 this.suppress = new BooleanType(); 4281 this.suppress.setValue(value); 4282 return this; 4283 } 4284 4285 /** 4286 * @return {@link #human} (Text that can be used to describe the constraint in messages identifying that the constraint has been violated.). This is the underlying object with id, value and extensions. The accessor "getHuman" gives direct access to the value 4287 */ 4288 public StringType getHumanElement() { 4289 if (this.human == null) 4290 if (Configuration.errorOnAutoCreate()) 4291 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.human"); 4292 else if (Configuration.doAutoCreate()) 4293 this.human = new StringType(); // bb 4294 return this.human; 4295 } 4296 4297 public boolean hasHumanElement() { 4298 return this.human != null && !this.human.isEmpty(); 4299 } 4300 4301 public boolean hasHuman() { 4302 return this.human != null && !this.human.isEmpty(); 4303 } 4304 4305 /** 4306 * @param value {@link #human} (Text that can be used to describe the constraint in messages identifying that the constraint has been violated.). This is the underlying object with id, value and extensions. The accessor "getHuman" gives direct access to the value 4307 */ 4308 public ElementDefinitionConstraintComponent setHumanElement(StringType value) { 4309 this.human = value; 4310 return this; 4311 } 4312 4313 /** 4314 * @return Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 4315 */ 4316 public String getHuman() { 4317 return this.human == null ? null : this.human.getValue(); 4318 } 4319 4320 /** 4321 * @param value Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 4322 */ 4323 public ElementDefinitionConstraintComponent setHuman(String value) { 4324 if (this.human == null) 4325 this.human = new StringType(); 4326 this.human.setValue(value); 4327 return this; 4328 } 4329 4330 /** 4331 * @return {@link #expression} (A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4332 */ 4333 public StringType getExpressionElement() { 4334 if (this.expression == null) 4335 if (Configuration.errorOnAutoCreate()) 4336 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.expression"); 4337 else if (Configuration.doAutoCreate()) 4338 this.expression = new StringType(); // bb 4339 return this.expression; 4340 } 4341 4342 public boolean hasExpressionElement() { 4343 return this.expression != null && !this.expression.isEmpty(); 4344 } 4345 4346 public boolean hasExpression() { 4347 return this.expression != null && !this.expression.isEmpty(); 4348 } 4349 4350 /** 4351 * @param value {@link #expression} (A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4352 */ 4353 public ElementDefinitionConstraintComponent setExpressionElement(StringType value) { 4354 this.expression = value; 4355 return this; 4356 } 4357 4358 /** 4359 * @return A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met. 4360 */ 4361 public String getExpression() { 4362 return this.expression == null ? null : this.expression.getValue(); 4363 } 4364 4365 /** 4366 * @param value A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met. 4367 */ 4368 public ElementDefinitionConstraintComponent setExpression(String value) { 4369 if (Utilities.noString(value)) 4370 this.expression = null; 4371 else { 4372 if (this.expression == null) 4373 this.expression = new StringType(); 4374 this.expression.setValue(value); 4375 } 4376 return this; 4377 } 4378 4379 /** 4380 * @return {@link #source} (A reference to the original source of the constraint, for traceability purposes.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 4381 */ 4382 public CanonicalType getSourceElement() { 4383 if (this.source == null) 4384 if (Configuration.errorOnAutoCreate()) 4385 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.source"); 4386 else if (Configuration.doAutoCreate()) 4387 this.source = new CanonicalType(); // bb 4388 return this.source; 4389 } 4390 4391 public boolean hasSourceElement() { 4392 return this.source != null && !this.source.isEmpty(); 4393 } 4394 4395 public boolean hasSource() { 4396 return this.source != null && !this.source.isEmpty(); 4397 } 4398 4399 /** 4400 * @param value {@link #source} (A reference to the original source of the constraint, for traceability purposes.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 4401 */ 4402 public ElementDefinitionConstraintComponent setSourceElement(CanonicalType value) { 4403 this.source = value; 4404 return this; 4405 } 4406 4407 /** 4408 * @return A reference to the original source of the constraint, for traceability purposes. 4409 */ 4410 public String getSource() { 4411 return this.source == null ? null : this.source.getValue(); 4412 } 4413 4414 /** 4415 * @param value A reference to the original source of the constraint, for traceability purposes. 4416 */ 4417 public ElementDefinitionConstraintComponent setSource(String value) { 4418 if (Utilities.noString(value)) 4419 this.source = null; 4420 else { 4421 if (this.source == null) 4422 this.source = new CanonicalType(); 4423 this.source.setValue(value); 4424 } 4425 return this; 4426 } 4427 4428 protected void listChildren(List<Property> children) { 4429 super.listChildren(children); 4430 children.add(new Property("key", "id", "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 0, 1, key)); 4431 children.add(new Property("requirements", "markdown", "Description of why this constraint is necessary or appropriate.", 0, 1, requirements)); 4432 children.add(new Property("severity", "code", "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity)); 4433 children.add(new Property("suppress", "boolean", "If true, indicates that the warning or best practice guideline should be suppressed.", 0, 1, suppress)); 4434 children.add(new Property("human", "string", "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 0, 1, human)); 4435 children.add(new Property("expression", "string", "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 0, 1, expression)); 4436 children.add(new Property("source", "canonical(StructureDefinition)", "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source)); 4437 } 4438 4439 @Override 4440 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4441 switch (_hash) { 4442 case 106079: /*key*/ return new Property("key", "id", "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 0, 1, key); 4443 case -1619874672: /*requirements*/ return new Property("requirements", "markdown", "Description of why this constraint is necessary or appropriate.", 0, 1, requirements); 4444 case 1478300413: /*severity*/ return new Property("severity", "code", "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity); 4445 case -1663129931: /*suppress*/ return new Property("suppress", "boolean", "If true, indicates that the warning or best practice guideline should be suppressed.", 0, 1, suppress); 4446 case 99639597: /*human*/ return new Property("human", "string", "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 0, 1, human); 4447 case -1795452264: /*expression*/ return new Property("expression", "string", "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 0, 1, expression); 4448 case -896505829: /*source*/ return new Property("source", "canonical(StructureDefinition)", "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source); 4449 default: return super.getNamedProperty(_hash, _name, _checkValid); 4450 } 4451 4452 } 4453 4454 @Override 4455 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4456 switch (hash) { 4457 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // IdType 4458 case -1619874672: /*requirements*/ return this.requirements == null ? new Base[0] : new Base[] {this.requirements}; // MarkdownType 4459 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<ConstraintSeverity> 4460 case -1663129931: /*suppress*/ return this.suppress == null ? new Base[0] : new Base[] {this.suppress}; // BooleanType 4461 case 99639597: /*human*/ return this.human == null ? new Base[0] : new Base[] {this.human}; // StringType 4462 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 4463 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // CanonicalType 4464 default: return super.getProperty(hash, name, checkValid); 4465 } 4466 4467 } 4468 4469 @Override 4470 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4471 switch (hash) { 4472 case 106079: // key 4473 this.key = TypeConvertor.castToId(value); // IdType 4474 return value; 4475 case -1619874672: // requirements 4476 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 4477 return value; 4478 case 1478300413: // severity 4479 value = new ConstraintSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4480 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4481 return value; 4482 case -1663129931: // suppress 4483 this.suppress = TypeConvertor.castToBoolean(value); // BooleanType 4484 return value; 4485 case 99639597: // human 4486 this.human = TypeConvertor.castToString(value); // StringType 4487 return value; 4488 case -1795452264: // expression 4489 this.expression = TypeConvertor.castToString(value); // StringType 4490 return value; 4491 case -896505829: // source 4492 this.source = TypeConvertor.castToCanonical(value); // CanonicalType 4493 return value; 4494 default: return super.setProperty(hash, name, value); 4495 } 4496 4497 } 4498 4499 @Override 4500 public Base setProperty(String name, Base value) throws FHIRException { 4501 if (name.equals("key")) { 4502 this.key = TypeConvertor.castToId(value); // IdType 4503 } else if (name.equals("requirements")) { 4504 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 4505 } else if (name.equals("severity")) { 4506 value = new ConstraintSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4507 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4508 } else if (name.equals("suppress")) { 4509 this.suppress = TypeConvertor.castToBoolean(value); // BooleanType 4510 } else if (name.equals("human")) { 4511 this.human = TypeConvertor.castToString(value); // StringType 4512 } else if (name.equals("expression")) { 4513 this.expression = TypeConvertor.castToString(value); // StringType 4514 } else if (name.equals("source")) { 4515 this.source = TypeConvertor.castToCanonical(value); // CanonicalType 4516 } else 4517 return super.setProperty(name, value); 4518 return value; 4519 } 4520 4521 @Override 4522 public void removeChild(String name, Base value) throws FHIRException { 4523 if (name.equals("key")) { 4524 this.key = null; 4525 } else if (name.equals("requirements")) { 4526 this.requirements = null; 4527 } else if (name.equals("severity")) { 4528 value = new ConstraintSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4529 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4530 } else if (name.equals("suppress")) { 4531 this.suppress = null; 4532 } else if (name.equals("human")) { 4533 this.human = null; 4534 } else if (name.equals("expression")) { 4535 this.expression = null; 4536 } else if (name.equals("source")) { 4537 this.source = null; 4538 } else 4539 super.removeChild(name, value); 4540 4541 } 4542 4543 @Override 4544 public Base makeProperty(int hash, String name) throws FHIRException { 4545 switch (hash) { 4546 case 106079: return getKeyElement(); 4547 case -1619874672: return getRequirementsElement(); 4548 case 1478300413: return getSeverityElement(); 4549 case -1663129931: return getSuppressElement(); 4550 case 99639597: return getHumanElement(); 4551 case -1795452264: return getExpressionElement(); 4552 case -896505829: return getSourceElement(); 4553 default: return super.makeProperty(hash, name); 4554 } 4555 4556 } 4557 4558 @Override 4559 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4560 switch (hash) { 4561 case 106079: /*key*/ return new String[] {"id"}; 4562 case -1619874672: /*requirements*/ return new String[] {"markdown"}; 4563 case 1478300413: /*severity*/ return new String[] {"code"}; 4564 case -1663129931: /*suppress*/ return new String[] {"boolean"}; 4565 case 99639597: /*human*/ return new String[] {"string"}; 4566 case -1795452264: /*expression*/ return new String[] {"string"}; 4567 case -896505829: /*source*/ return new String[] {"canonical"}; 4568 default: return super.getTypesForProperty(hash, name); 4569 } 4570 4571 } 4572 4573 @Override 4574 public Base addChild(String name) throws FHIRException { 4575 if (name.equals("key")) { 4576 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.key"); 4577 } 4578 else if (name.equals("requirements")) { 4579 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.requirements"); 4580 } 4581 else if (name.equals("severity")) { 4582 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.severity"); 4583 } 4584 else if (name.equals("suppress")) { 4585 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.suppress"); 4586 } 4587 else if (name.equals("human")) { 4588 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.human"); 4589 } 4590 else if (name.equals("expression")) { 4591 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.expression"); 4592 } 4593 else if (name.equals("source")) { 4594 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.source"); 4595 } 4596 else 4597 return super.addChild(name); 4598 } 4599 4600 public ElementDefinitionConstraintComponent copy() { 4601 ElementDefinitionConstraintComponent dst = new ElementDefinitionConstraintComponent(); 4602 copyValues(dst); 4603 return dst; 4604 } 4605 4606 public void copyValues(ElementDefinitionConstraintComponent dst) { 4607 super.copyValues(dst); 4608 dst.key = key == null ? null : key.copy(); 4609 dst.requirements = requirements == null ? null : requirements.copy(); 4610 dst.severity = severity == null ? null : severity.copy(); 4611 dst.suppress = suppress == null ? null : suppress.copy(); 4612 dst.human = human == null ? null : human.copy(); 4613 dst.expression = expression == null ? null : expression.copy(); 4614 dst.source = source == null ? null : source.copy(); 4615 } 4616 4617 @Override 4618 public boolean equalsDeep(Base other_) { 4619 if (!super.equalsDeep(other_)) 4620 return false; 4621 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4622 return false; 4623 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4624 return compareDeep(key, o.key, true) && compareDeep(requirements, o.requirements, true) && compareDeep(severity, o.severity, true) 4625 && compareDeep(suppress, o.suppress, true) && compareDeep(human, o.human, true) && compareDeep(expression, o.expression, true) 4626 && compareDeep(source, o.source, true); 4627 } 4628 4629 @Override 4630 public boolean equalsShallow(Base other_) { 4631 if (!super.equalsShallow(other_)) 4632 return false; 4633 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4634 return false; 4635 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4636 return compareValues(key, o.key, true) && compareValues(requirements, o.requirements, true) && compareValues(severity, o.severity, true) 4637 && compareValues(suppress, o.suppress, true) && compareValues(human, o.human, true) && compareValues(expression, o.expression, true) 4638 && compareValues(source, o.source, true); 4639 } 4640 4641 public boolean isEmpty() { 4642 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, requirements, severity 4643 , suppress, human, expression, source); 4644 } 4645 4646 public String fhirType() { 4647 return "ElementDefinition.constraint"; 4648 4649 } 4650 4651 @Override 4652 public String toString() { 4653 return key + ":" + expression + (severity == null ? "("+severity.asStringValue()+")" : ""); 4654 } 4655 4656 } 4657 4658 @Block() 4659 public static class ElementDefinitionBindingComponent extends Element implements IBaseDatatypeElement { 4660 /** 4661 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 4662 */ 4663 @Child(name = "strength", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4664 @Description(shortDefinition="required | extensible | preferred | example", formalDefinition="Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances." ) 4665 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/binding-strength") 4666 protected Enumeration<BindingStrength> strength; 4667 4668 /** 4669 * Describes the intended use of this particular set of codes. 4670 */ 4671 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4672 @Description(shortDefinition="Intended use of codes in the bound value set", formalDefinition="Describes the intended use of this particular set of codes." ) 4673 protected MarkdownType description; 4674 4675 /** 4676 * Refers to the value set that identifies the set of codes the binding refers to. 4677 */ 4678 @Child(name = "valueSet", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4679 @Description(shortDefinition="Source of value set", formalDefinition="Refers to the value set that identifies the set of codes the binding refers to." ) 4680 protected CanonicalType valueSet; 4681 4682 /** 4683 * Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context. 4684 */ 4685 @Child(name = "additional", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4686 @Description(shortDefinition="Additional Bindings - more rules about the binding", formalDefinition="Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context." ) 4687 protected List<ElementDefinitionBindingAdditionalComponent> additional; 4688 4689 private static final long serialVersionUID = 16276611L; 4690 4691 /** 4692 * Constructor 4693 */ 4694 public ElementDefinitionBindingComponent() { 4695 super(); 4696 } 4697 4698 /** 4699 * Constructor 4700 */ 4701 public ElementDefinitionBindingComponent(BindingStrength strength) { 4702 super(); 4703 this.setStrength(strength); 4704 } 4705 4706 /** 4707 * @return {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 4708 */ 4709 public Enumeration<BindingStrength> getStrengthElement() { 4710 if (this.strength == null) 4711 if (Configuration.errorOnAutoCreate()) 4712 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.strength"); 4713 else if (Configuration.doAutoCreate()) 4714 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 4715 return this.strength; 4716 } 4717 4718 public boolean hasStrengthElement() { 4719 return this.strength != null && !this.strength.isEmpty(); 4720 } 4721 4722 public boolean hasStrength() { 4723 return this.strength != null && !this.strength.isEmpty(); 4724 } 4725 4726 /** 4727 * @param value {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 4728 */ 4729 public ElementDefinitionBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 4730 this.strength = value; 4731 return this; 4732 } 4733 4734 /** 4735 * @return Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 4736 */ 4737 public BindingStrength getStrength() { 4738 return this.strength == null ? null : this.strength.getValue(); 4739 } 4740 4741 /** 4742 * @param value Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 4743 */ 4744 public ElementDefinitionBindingComponent setStrength(BindingStrength value) { 4745 if (this.strength == null) 4746 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 4747 this.strength.setValue(value); 4748 return this; 4749 } 4750 4751 /** 4752 * @return {@link #description} (Describes the intended use of this particular set of codes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4753 */ 4754 public MarkdownType getDescriptionElement() { 4755 if (this.description == null) 4756 if (Configuration.errorOnAutoCreate()) 4757 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.description"); 4758 else if (Configuration.doAutoCreate()) 4759 this.description = new MarkdownType(); // bb 4760 return this.description; 4761 } 4762 4763 public boolean hasDescriptionElement() { 4764 return this.description != null && !this.description.isEmpty(); 4765 } 4766 4767 public boolean hasDescription() { 4768 return this.description != null && !this.description.isEmpty(); 4769 } 4770 4771 /** 4772 * @param value {@link #description} (Describes the intended use of this particular set of codes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4773 */ 4774 public ElementDefinitionBindingComponent setDescriptionElement(MarkdownType value) { 4775 this.description = value; 4776 return this; 4777 } 4778 4779 /** 4780 * @return Describes the intended use of this particular set of codes. 4781 */ 4782 public String getDescription() { 4783 return this.description == null ? null : this.description.getValue(); 4784 } 4785 4786 /** 4787 * @param value Describes the intended use of this particular set of codes. 4788 */ 4789 public ElementDefinitionBindingComponent setDescription(String value) { 4790 if (Utilities.noString(value)) 4791 this.description = null; 4792 else { 4793 if (this.description == null) 4794 this.description = new MarkdownType(); 4795 this.description.setValue(value); 4796 } 4797 return this; 4798 } 4799 4800 /** 4801 * @return {@link #valueSet} (Refers to the value set that identifies the set of codes the binding refers to.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4802 */ 4803 public CanonicalType getValueSetElement() { 4804 if (this.valueSet == null) 4805 if (Configuration.errorOnAutoCreate()) 4806 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.valueSet"); 4807 else if (Configuration.doAutoCreate()) 4808 this.valueSet = new CanonicalType(); // bb 4809 return this.valueSet; 4810 } 4811 4812 public boolean hasValueSetElement() { 4813 return this.valueSet != null && !this.valueSet.isEmpty(); 4814 } 4815 4816 public boolean hasValueSet() { 4817 return this.valueSet != null && !this.valueSet.isEmpty(); 4818 } 4819 4820 /** 4821 * @param value {@link #valueSet} (Refers to the value set that identifies the set of codes the binding refers to.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4822 */ 4823 public ElementDefinitionBindingComponent setValueSetElement(CanonicalType value) { 4824 this.valueSet = value; 4825 return this; 4826 } 4827 4828 /** 4829 * @return Refers to the value set that identifies the set of codes the binding refers to. 4830 */ 4831 public String getValueSet() { 4832 return this.valueSet == null ? null : this.valueSet.getValue(); 4833 } 4834 4835 /** 4836 * @param value Refers to the value set that identifies the set of codes the binding refers to. 4837 */ 4838 public ElementDefinitionBindingComponent setValueSet(String value) { 4839 if (Utilities.noString(value)) 4840 this.valueSet = null; 4841 else { 4842 if (this.valueSet == null) 4843 this.valueSet = new CanonicalType(); 4844 this.valueSet.setValue(value); 4845 } 4846 return this; 4847 } 4848 4849 /** 4850 * @return {@link #additional} (Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context.) 4851 */ 4852 public List<ElementDefinitionBindingAdditionalComponent> getAdditional() { 4853 if (this.additional == null) 4854 this.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 4855 return this.additional; 4856 } 4857 4858 /** 4859 * @return Returns a reference to <code>this</code> for easy method chaining 4860 */ 4861 public ElementDefinitionBindingComponent setAdditional(List<ElementDefinitionBindingAdditionalComponent> theAdditional) { 4862 this.additional = theAdditional; 4863 return this; 4864 } 4865 4866 public boolean hasAdditional() { 4867 if (this.additional == null) 4868 return false; 4869 for (ElementDefinitionBindingAdditionalComponent item : this.additional) 4870 if (!item.isEmpty()) 4871 return true; 4872 return false; 4873 } 4874 4875 public ElementDefinitionBindingAdditionalComponent addAdditional() { //3 4876 ElementDefinitionBindingAdditionalComponent t = new ElementDefinitionBindingAdditionalComponent(); 4877 if (this.additional == null) 4878 this.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 4879 this.additional.add(t); 4880 return t; 4881 } 4882 4883 public ElementDefinitionBindingComponent addAdditional(ElementDefinitionBindingAdditionalComponent t) { //3 4884 if (t == null) 4885 return this; 4886 if (this.additional == null) 4887 this.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 4888 this.additional.add(t); 4889 return this; 4890 } 4891 4892 /** 4893 * @return The first repetition of repeating field {@link #additional}, creating it if it does not already exist {3} 4894 */ 4895 public ElementDefinitionBindingAdditionalComponent getAdditionalFirstRep() { 4896 if (getAdditional().isEmpty()) { 4897 addAdditional(); 4898 } 4899 return getAdditional().get(0); 4900 } 4901 4902 protected void listChildren(List<Property> children) { 4903 super.listChildren(children); 4904 children.add(new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength)); 4905 children.add(new Property("description", "markdown", "Describes the intended use of this particular set of codes.", 0, 1, description)); 4906 children.add(new Property("valueSet", "canonical(ValueSet)", "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet)); 4907 children.add(new Property("additional", "", "Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context.", 0, java.lang.Integer.MAX_VALUE, additional)); 4908 } 4909 4910 @Override 4911 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4912 switch (_hash) { 4913 case 1791316033: /*strength*/ return new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength); 4914 case -1724546052: /*description*/ return new Property("description", "markdown", "Describes the intended use of this particular set of codes.", 0, 1, description); 4915 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet); 4916 case -1931413465: /*additional*/ return new Property("additional", "", "Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context.", 0, java.lang.Integer.MAX_VALUE, additional); 4917 default: return super.getNamedProperty(_hash, _name, _checkValid); 4918 } 4919 4920 } 4921 4922 @Override 4923 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4924 switch (hash) { 4925 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // Enumeration<BindingStrength> 4926 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4927 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 4928 case -1931413465: /*additional*/ return this.additional == null ? new Base[0] : this.additional.toArray(new Base[this.additional.size()]); // ElementDefinitionBindingAdditionalComponent 4929 default: return super.getProperty(hash, name, checkValid); 4930 } 4931 4932 } 4933 4934 @Override 4935 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4936 switch (hash) { 4937 case 1791316033: // strength 4938 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 4939 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4940 return value; 4941 case -1724546052: // description 4942 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4943 return value; 4944 case -1410174671: // valueSet 4945 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4946 return value; 4947 case -1931413465: // additional 4948 this.getAdditional().add((ElementDefinitionBindingAdditionalComponent) value); // ElementDefinitionBindingAdditionalComponent 4949 return value; 4950 default: return super.setProperty(hash, name, value); 4951 } 4952 4953 } 4954 4955 @Override 4956 public Base setProperty(String name, Base value) throws FHIRException { 4957 if (name.equals("strength")) { 4958 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 4959 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4960 } else if (name.equals("description")) { 4961 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4962 } else if (name.equals("valueSet")) { 4963 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4964 } else if (name.equals("additional")) { 4965 this.getAdditional().add((ElementDefinitionBindingAdditionalComponent) value); 4966 } else 4967 return super.setProperty(name, value); 4968 return value; 4969 } 4970 4971 @Override 4972 public void removeChild(String name, Base value) throws FHIRException { 4973 if (name.equals("strength")) { 4974 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 4975 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4976 } else if (name.equals("description")) { 4977 this.description = null; 4978 } else if (name.equals("valueSet")) { 4979 this.valueSet = null; 4980 } else if (name.equals("additional")) { 4981 this.getAdditional().remove((ElementDefinitionBindingAdditionalComponent) value); 4982 } else 4983 super.removeChild(name, value); 4984 4985 } 4986 4987 @Override 4988 public Base makeProperty(int hash, String name) throws FHIRException { 4989 switch (hash) { 4990 case 1791316033: return getStrengthElement(); 4991 case -1724546052: return getDescriptionElement(); 4992 case -1410174671: return getValueSetElement(); 4993 case -1931413465: return addAdditional(); 4994 default: return super.makeProperty(hash, name); 4995 } 4996 4997 } 4998 4999 @Override 5000 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5001 switch (hash) { 5002 case 1791316033: /*strength*/ return new String[] {"code"}; 5003 case -1724546052: /*description*/ return new String[] {"markdown"}; 5004 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 5005 case -1931413465: /*additional*/ return new String[] {}; 5006 default: return super.getTypesForProperty(hash, name); 5007 } 5008 5009 } 5010 5011 @Override 5012 public Base addChild(String name) throws FHIRException { 5013 if (name.equals("strength")) { 5014 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.strength"); 5015 } 5016 else if (name.equals("description")) { 5017 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.description"); 5018 } 5019 else if (name.equals("valueSet")) { 5020 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.valueSet"); 5021 } 5022 else if (name.equals("additional")) { 5023 return addAdditional(); 5024 } 5025 else 5026 return super.addChild(name); 5027 } 5028 5029 public ElementDefinitionBindingComponent copy() { 5030 ElementDefinitionBindingComponent dst = new ElementDefinitionBindingComponent(); 5031 copyValues(dst); 5032 return dst; 5033 } 5034 5035 public void copyValues(ElementDefinitionBindingComponent dst) { 5036 super.copyValues(dst); 5037 dst.strength = strength == null ? null : strength.copy(); 5038 dst.description = description == null ? null : description.copy(); 5039 dst.valueSet = valueSet == null ? null : valueSet.copy(); 5040 if (additional != null) { 5041 dst.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 5042 for (ElementDefinitionBindingAdditionalComponent i : additional) 5043 dst.additional.add(i.copy()); 5044 }; 5045 } 5046 5047 @Override 5048 public boolean equalsDeep(Base other_) { 5049 if (!super.equalsDeep(other_)) 5050 return false; 5051 if (!(other_ instanceof ElementDefinitionBindingComponent)) 5052 return false; 5053 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 5054 return compareDeep(strength, o.strength, true) && compareDeep(description, o.description, true) 5055 && compareDeep(valueSet, o.valueSet, true) && compareDeep(additional, o.additional, true); 5056 } 5057 5058 @Override 5059 public boolean equalsShallow(Base other_) { 5060 if (!super.equalsShallow(other_)) 5061 return false; 5062 if (!(other_ instanceof ElementDefinitionBindingComponent)) 5063 return false; 5064 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 5065 return compareValues(strength, o.strength, true) && compareValues(description, o.description, true) 5066 && compareValues(valueSet, o.valueSet, true); 5067 } 5068 5069 public boolean isEmpty() { 5070 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, description, valueSet 5071 , additional); 5072 } 5073 5074 public String fhirType() { 5075 return "ElementDefinition.binding"; 5076 5077 } 5078 5079 public boolean hasAdditional(ElementDefinitionBindingAdditionalComponent ab) { 5080 if (hasAdditional()) { 5081 for (ElementDefinitionBindingAdditionalComponent t : getAdditional()) { 5082 if (Base.compareDeep(t, ab, false)) { 5083 return true; 5084 } 5085 } 5086 } 5087 return false; 5088 } 5089 5090 } 5091 5092 @Block() 5093 public static class ElementDefinitionBindingAdditionalComponent extends Element implements IBaseDatatypeElement { 5094 /** 5095 * The use of this additional binding. 5096 */ 5097 @Child(name = "purpose", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 5098 @Description(shortDefinition="maximum | minimum | required | extensible | candidate | current | preferred | ui | starter | component", formalDefinition="The use of this additional binding." ) 5099 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/additional-binding-purpose") 5100 protected Enumeration<AdditionalBindingPurposeVS> purpose; 5101 5102 /** 5103 * The valueSet that is being bound for the purpose. 5104 */ 5105 @Child(name = "valueSet", type = {CanonicalType.class}, order=2, min=1, max=1, modifier=false, summary=true) 5106 @Description(shortDefinition="The value set for the additional binding", formalDefinition="The valueSet that is being bound for the purpose." ) 5107 protected CanonicalType valueSet; 5108 5109 /** 5110 * Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used. 5111 */ 5112 @Child(name = "documentation", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=true) 5113 @Description(shortDefinition="Documentation of the purpose of use of the binding", formalDefinition="Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used." ) 5114 protected MarkdownType documentation; 5115 5116 /** 5117 * Concise documentation - for summary tables. 5118 */ 5119 @Child(name = "shortDoco", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 5120 @Description(shortDefinition="Concise documentation - for summary tables", formalDefinition="Concise documentation - for summary tables." ) 5121 protected StringType shortDoco; 5122 5123 /** 5124 * Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling. 5125 */ 5126 @Child(name = "usage", type = {UsageContext.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5127 @Description(shortDefinition="Qualifies the usage - jurisdiction, gender, workflow status etc.", formalDefinition="Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling." ) 5128 protected List<UsageContext> usage; 5129 5130 /** 5131 * Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat. 5132 */ 5133 @Child(name = "any", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=true) 5134 @Description(shortDefinition="Whether binding can applies to all repeats, or just one", formalDefinition="Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat." ) 5135 protected BooleanType any; 5136 5137 private static final long serialVersionUID = -1312796441L; 5138 5139 /** 5140 * Constructor 5141 */ 5142 public ElementDefinitionBindingAdditionalComponent() { 5143 super(); 5144 } 5145 5146 /** 5147 * Constructor 5148 */ 5149 public ElementDefinitionBindingAdditionalComponent(AdditionalBindingPurposeVS purpose, String valueSet) { 5150 super(); 5151 this.setPurpose(purpose); 5152 this.setValueSet(valueSet); 5153 } 5154 5155 /** 5156 * @return {@link #purpose} (The use of this additional binding.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5157 */ 5158 public Enumeration<AdditionalBindingPurposeVS> getPurposeElement() { 5159 if (this.purpose == null) 5160 if (Configuration.errorOnAutoCreate()) 5161 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.purpose"); 5162 else if (Configuration.doAutoCreate()) 5163 this.purpose = new Enumeration<AdditionalBindingPurposeVS>(new AdditionalBindingPurposeVSEnumFactory()); // bb 5164 return this.purpose; 5165 } 5166 5167 public boolean hasPurposeElement() { 5168 return this.purpose != null && !this.purpose.isEmpty(); 5169 } 5170 5171 public boolean hasPurpose() { 5172 return this.purpose != null && !this.purpose.isEmpty(); 5173 } 5174 5175 /** 5176 * @param value {@link #purpose} (The use of this additional binding.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5177 */ 5178 public ElementDefinitionBindingAdditionalComponent setPurposeElement(Enumeration<AdditionalBindingPurposeVS> value) { 5179 this.purpose = value; 5180 return this; 5181 } 5182 5183 /** 5184 * @return The use of this additional binding. 5185 */ 5186 public AdditionalBindingPurposeVS getPurpose() { 5187 return this.purpose == null ? null : this.purpose.getValue(); 5188 } 5189 5190 /** 5191 * @param value The use of this additional binding. 5192 */ 5193 public ElementDefinitionBindingAdditionalComponent setPurpose(AdditionalBindingPurposeVS value) { 5194 if (this.purpose == null) 5195 this.purpose = new Enumeration<AdditionalBindingPurposeVS>(new AdditionalBindingPurposeVSEnumFactory()); 5196 this.purpose.setValue(value); 5197 return this; 5198 } 5199 5200 /** 5201 * @return {@link #valueSet} (The valueSet that is being bound for the purpose.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 5202 */ 5203 public CanonicalType getValueSetElement() { 5204 if (this.valueSet == null) 5205 if (Configuration.errorOnAutoCreate()) 5206 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.valueSet"); 5207 else if (Configuration.doAutoCreate()) 5208 this.valueSet = new CanonicalType(); // bb 5209 return this.valueSet; 5210 } 5211 5212 public boolean hasValueSetElement() { 5213 return this.valueSet != null && !this.valueSet.isEmpty(); 5214 } 5215 5216 public boolean hasValueSet() { 5217 return this.valueSet != null && !this.valueSet.isEmpty(); 5218 } 5219 5220 /** 5221 * @param value {@link #valueSet} (The valueSet that is being bound for the purpose.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 5222 */ 5223 public ElementDefinitionBindingAdditionalComponent setValueSetElement(CanonicalType value) { 5224 this.valueSet = value; 5225 return this; 5226 } 5227 5228 /** 5229 * @return The valueSet that is being bound for the purpose. 5230 */ 5231 public String getValueSet() { 5232 return this.valueSet == null ? null : this.valueSet.getValue(); 5233 } 5234 5235 /** 5236 * @param value The valueSet that is being bound for the purpose. 5237 */ 5238 public ElementDefinitionBindingAdditionalComponent setValueSet(String value) { 5239 if (this.valueSet == null) 5240 this.valueSet = new CanonicalType(); 5241 this.valueSet.setValue(value); 5242 return this; 5243 } 5244 5245 /** 5246 * @return {@link #documentation} (Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5247 */ 5248 public MarkdownType getDocumentationElement() { 5249 if (this.documentation == null) 5250 if (Configuration.errorOnAutoCreate()) 5251 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.documentation"); 5252 else if (Configuration.doAutoCreate()) 5253 this.documentation = new MarkdownType(); // bb 5254 return this.documentation; 5255 } 5256 5257 public boolean hasDocumentationElement() { 5258 return this.documentation != null && !this.documentation.isEmpty(); 5259 } 5260 5261 public boolean hasDocumentation() { 5262 return this.documentation != null && !this.documentation.isEmpty(); 5263 } 5264 5265 /** 5266 * @param value {@link #documentation} (Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5267 */ 5268 public ElementDefinitionBindingAdditionalComponent setDocumentationElement(MarkdownType value) { 5269 this.documentation = value; 5270 return this; 5271 } 5272 5273 /** 5274 * @return Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used. 5275 */ 5276 public String getDocumentation() { 5277 return this.documentation == null ? null : this.documentation.getValue(); 5278 } 5279 5280 /** 5281 * @param value Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used. 5282 */ 5283 public ElementDefinitionBindingAdditionalComponent setDocumentation(String value) { 5284 if (Utilities.noString(value)) 5285 this.documentation = null; 5286 else { 5287 if (this.documentation == null) 5288 this.documentation = new MarkdownType(); 5289 this.documentation.setValue(value); 5290 } 5291 return this; 5292 } 5293 5294 /** 5295 * @return {@link #shortDoco} (Concise documentation - for summary tables.). This is the underlying object with id, value and extensions. The accessor "getShortDoco" gives direct access to the value 5296 */ 5297 public StringType getShortDocoElement() { 5298 if (this.shortDoco == null) 5299 if (Configuration.errorOnAutoCreate()) 5300 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.shortDoco"); 5301 else if (Configuration.doAutoCreate()) 5302 this.shortDoco = new StringType(); // bb 5303 return this.shortDoco; 5304 } 5305 5306 public boolean hasShortDocoElement() { 5307 return this.shortDoco != null && !this.shortDoco.isEmpty(); 5308 } 5309 5310 public boolean hasShortDoco() { 5311 return this.shortDoco != null && !this.shortDoco.isEmpty(); 5312 } 5313 5314 /** 5315 * @param value {@link #shortDoco} (Concise documentation - for summary tables.). This is the underlying object with id, value and extensions. The accessor "getShortDoco" gives direct access to the value 5316 */ 5317 public ElementDefinitionBindingAdditionalComponent setShortDocoElement(StringType value) { 5318 this.shortDoco = value; 5319 return this; 5320 } 5321 5322 /** 5323 * @return Concise documentation - for summary tables. 5324 */ 5325 public String getShortDoco() { 5326 return this.shortDoco == null ? null : this.shortDoco.getValue(); 5327 } 5328 5329 /** 5330 * @param value Concise documentation - for summary tables. 5331 */ 5332 public ElementDefinitionBindingAdditionalComponent setShortDoco(String value) { 5333 if (Utilities.noString(value)) 5334 this.shortDoco = null; 5335 else { 5336 if (this.shortDoco == null) 5337 this.shortDoco = new StringType(); 5338 this.shortDoco.setValue(value); 5339 } 5340 return this; 5341 } 5342 5343 /** 5344 * @return {@link #usage} (Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling.) 5345 */ 5346 public List<UsageContext> getUsage() { 5347 if (this.usage == null) 5348 this.usage = new ArrayList<UsageContext>(); 5349 return this.usage; 5350 } 5351 5352 /** 5353 * @return Returns a reference to <code>this</code> for easy method chaining 5354 */ 5355 public ElementDefinitionBindingAdditionalComponent setUsage(List<UsageContext> theUsage) { 5356 this.usage = theUsage; 5357 return this; 5358 } 5359 5360 public boolean hasUsage() { 5361 if (this.usage == null) 5362 return false; 5363 for (UsageContext item : this.usage) 5364 if (!item.isEmpty()) 5365 return true; 5366 return false; 5367 } 5368 5369 public UsageContext addUsage() { //3 5370 UsageContext t = new UsageContext(); 5371 if (this.usage == null) 5372 this.usage = new ArrayList<UsageContext>(); 5373 this.usage.add(t); 5374 return t; 5375 } 5376 5377 public ElementDefinitionBindingAdditionalComponent addUsage(UsageContext t) { //3 5378 if (t == null) 5379 return this; 5380 if (this.usage == null) 5381 this.usage = new ArrayList<UsageContext>(); 5382 this.usage.add(t); 5383 return this; 5384 } 5385 5386 /** 5387 * @return The first repetition of repeating field {@link #usage}, creating it if it does not already exist {3} 5388 */ 5389 public UsageContext getUsageFirstRep() { 5390 if (getUsage().isEmpty()) { 5391 addUsage(); 5392 } 5393 return getUsage().get(0); 5394 } 5395 5396 /** 5397 * @return {@link #any} (Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.). This is the underlying object with id, value and extensions. The accessor "getAny" gives direct access to the value 5398 */ 5399 public BooleanType getAnyElement() { 5400 if (this.any == null) 5401 if (Configuration.errorOnAutoCreate()) 5402 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.any"); 5403 else if (Configuration.doAutoCreate()) 5404 this.any = new BooleanType(); // bb 5405 return this.any; 5406 } 5407 5408 public boolean hasAnyElement() { 5409 return this.any != null && !this.any.isEmpty(); 5410 } 5411 5412 public boolean hasAny() { 5413 return this.any != null && !this.any.isEmpty(); 5414 } 5415 5416 /** 5417 * @param value {@link #any} (Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.). This is the underlying object with id, value and extensions. The accessor "getAny" gives direct access to the value 5418 */ 5419 public ElementDefinitionBindingAdditionalComponent setAnyElement(BooleanType value) { 5420 this.any = value; 5421 return this; 5422 } 5423 5424 /** 5425 * @return Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat. 5426 */ 5427 public boolean getAny() { 5428 return this.any == null || this.any.isEmpty() ? false : this.any.getValue(); 5429 } 5430 5431 /** 5432 * @param value Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat. 5433 */ 5434 public ElementDefinitionBindingAdditionalComponent setAny(boolean value) { 5435 if (this.any == null) 5436 this.any = new BooleanType(); 5437 this.any.setValue(value); 5438 return this; 5439 } 5440 5441 protected void listChildren(List<Property> children) { 5442 super.listChildren(children); 5443 children.add(new Property("purpose", "code", "The use of this additional binding.", 0, 1, purpose)); 5444 children.add(new Property("valueSet", "canonical(ValueSet)", "The valueSet that is being bound for the purpose.", 0, 1, valueSet)); 5445 children.add(new Property("documentation", "markdown", "Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.", 0, 1, documentation)); 5446 children.add(new Property("shortDoco", "string", "Concise documentation - for summary tables.", 0, 1, shortDoco)); 5447 children.add(new Property("usage", "UsageContext", "Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling.", 0, java.lang.Integer.MAX_VALUE, usage)); 5448 children.add(new Property("any", "boolean", "Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.", 0, 1, any)); 5449 } 5450 5451 @Override 5452 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5453 switch (_hash) { 5454 case -220463842: /*purpose*/ return new Property("purpose", "code", "The use of this additional binding.", 0, 1, purpose); 5455 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "The valueSet that is being bound for the purpose.", 0, 1, valueSet); 5456 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.", 0, 1, documentation); 5457 case -2028503853: /*shortDoco*/ return new Property("shortDoco", "string", "Concise documentation - for summary tables.", 0, 1, shortDoco); 5458 case 111574433: /*usage*/ return new Property("usage", "UsageContext", "Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling.", 0, java.lang.Integer.MAX_VALUE, usage); 5459 case 96748: /*any*/ return new Property("any", "boolean", "Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.", 0, 1, any); 5460 default: return super.getNamedProperty(_hash, _name, _checkValid); 5461 } 5462 5463 } 5464 5465 @Override 5466 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5467 switch (hash) { 5468 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // Enumeration<AdditionalBindingPurposeVS> 5469 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 5470 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 5471 case -2028503853: /*shortDoco*/ return this.shortDoco == null ? new Base[0] : new Base[] {this.shortDoco}; // StringType 5472 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : this.usage.toArray(new Base[this.usage.size()]); // UsageContext 5473 case 96748: /*any*/ return this.any == null ? new Base[0] : new Base[] {this.any}; // BooleanType 5474 default: return super.getProperty(hash, name, checkValid); 5475 } 5476 5477 } 5478 5479 @Override 5480 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5481 switch (hash) { 5482 case -220463842: // purpose 5483 value = new AdditionalBindingPurposeVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 5484 this.purpose = (Enumeration) value; // Enumeration<AdditionalBindingPurposeVS> 5485 return value; 5486 case -1410174671: // valueSet 5487 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 5488 return value; 5489 case 1587405498: // documentation 5490 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5491 return value; 5492 case -2028503853: // shortDoco 5493 this.shortDoco = TypeConvertor.castToString(value); // StringType 5494 return value; 5495 case 111574433: // usage 5496 this.getUsage().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5497 return value; 5498 case 96748: // any 5499 this.any = TypeConvertor.castToBoolean(value); // BooleanType 5500 return value; 5501 default: return super.setProperty(hash, name, value); 5502 } 5503 5504 } 5505 5506 @Override 5507 public Base setProperty(String name, Base value) throws FHIRException { 5508 if (name.equals("purpose")) { 5509 value = new AdditionalBindingPurposeVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 5510 this.purpose = (Enumeration) value; // Enumeration<AdditionalBindingPurposeVS> 5511 } else if (name.equals("valueSet")) { 5512 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 5513 } else if (name.equals("documentation")) { 5514 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5515 } else if (name.equals("shortDoco")) { 5516 this.shortDoco = TypeConvertor.castToString(value); // StringType 5517 } else if (name.equals("usage")) { 5518 this.getUsage().add(TypeConvertor.castToUsageContext(value)); 5519 } else if (name.equals("any")) { 5520 this.any = TypeConvertor.castToBoolean(value); // BooleanType 5521 } else 5522 return super.setProperty(name, value); 5523 return value; 5524 } 5525 5526 @Override 5527 public void removeChild(String name, Base value) throws FHIRException { 5528 if (name.equals("purpose")) { 5529 value = new AdditionalBindingPurposeVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 5530 this.purpose = (Enumeration) value; // Enumeration<AdditionalBindingPurposeVS> 5531 } else if (name.equals("valueSet")) { 5532 this.valueSet = null; 5533 } else if (name.equals("documentation")) { 5534 this.documentation = null; 5535 } else if (name.equals("shortDoco")) { 5536 this.shortDoco = null; 5537 } else if (name.equals("usage")) { 5538 this.getUsage().remove(value); 5539 } else if (name.equals("any")) { 5540 this.any = null; 5541 } else 5542 super.removeChild(name, value); 5543 5544 } 5545 5546 @Override 5547 public Base makeProperty(int hash, String name) throws FHIRException { 5548 switch (hash) { 5549 case -220463842: return getPurposeElement(); 5550 case -1410174671: return getValueSetElement(); 5551 case 1587405498: return getDocumentationElement(); 5552 case -2028503853: return getShortDocoElement(); 5553 case 111574433: return addUsage(); 5554 case 96748: return getAnyElement(); 5555 default: return super.makeProperty(hash, name); 5556 } 5557 5558 } 5559 5560 @Override 5561 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5562 switch (hash) { 5563 case -220463842: /*purpose*/ return new String[] {"code"}; 5564 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 5565 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 5566 case -2028503853: /*shortDoco*/ return new String[] {"string"}; 5567 case 111574433: /*usage*/ return new String[] {"UsageContext"}; 5568 case 96748: /*any*/ return new String[] {"boolean"}; 5569 default: return super.getTypesForProperty(hash, name); 5570 } 5571 5572 } 5573 5574 @Override 5575 public Base addChild(String name) throws FHIRException { 5576 if (name.equals("purpose")) { 5577 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.purpose"); 5578 } 5579 else if (name.equals("valueSet")) { 5580 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.valueSet"); 5581 } 5582 else if (name.equals("documentation")) { 5583 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.documentation"); 5584 } 5585 else if (name.equals("shortDoco")) { 5586 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.shortDoco"); 5587 } 5588 else if (name.equals("usage")) { 5589 return addUsage(); 5590 } 5591 else if (name.equals("any")) { 5592 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.any"); 5593 } 5594 else 5595 return super.addChild(name); 5596 } 5597 5598 public ElementDefinitionBindingAdditionalComponent copy() { 5599 ElementDefinitionBindingAdditionalComponent dst = new ElementDefinitionBindingAdditionalComponent(); 5600 copyValues(dst); 5601 return dst; 5602 } 5603 5604 public void copyValues(ElementDefinitionBindingAdditionalComponent dst) { 5605 super.copyValues(dst); 5606 dst.purpose = purpose == null ? null : purpose.copy(); 5607 dst.valueSet = valueSet == null ? null : valueSet.copy(); 5608 dst.documentation = documentation == null ? null : documentation.copy(); 5609 dst.shortDoco = shortDoco == null ? null : shortDoco.copy(); 5610 if (usage != null) { 5611 dst.usage = new ArrayList<UsageContext>(); 5612 for (UsageContext i : usage) 5613 dst.usage.add(i.copy()); 5614 }; 5615 dst.any = any == null ? null : any.copy(); 5616 } 5617 5618 @Override 5619 public boolean equalsDeep(Base other_) { 5620 if (!super.equalsDeep(other_)) 5621 return false; 5622 if (!(other_ instanceof ElementDefinitionBindingAdditionalComponent)) 5623 return false; 5624 ElementDefinitionBindingAdditionalComponent o = (ElementDefinitionBindingAdditionalComponent) other_; 5625 return compareDeep(purpose, o.purpose, true) && compareDeep(valueSet, o.valueSet, true) && compareDeep(documentation, o.documentation, true) 5626 && compareDeep(shortDoco, o.shortDoco, true) && compareDeep(usage, o.usage, true) && compareDeep(any, o.any, true) 5627 ; 5628 } 5629 5630 @Override 5631 public boolean equalsShallow(Base other_) { 5632 if (!super.equalsShallow(other_)) 5633 return false; 5634 if (!(other_ instanceof ElementDefinitionBindingAdditionalComponent)) 5635 return false; 5636 ElementDefinitionBindingAdditionalComponent o = (ElementDefinitionBindingAdditionalComponent) other_; 5637 return compareValues(purpose, o.purpose, true) && compareValues(valueSet, o.valueSet, true) && compareValues(documentation, o.documentation, true) 5638 && compareValues(shortDoco, o.shortDoco, true) && compareValues(any, o.any, true); 5639 } 5640 5641 public boolean isEmpty() { 5642 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, valueSet, documentation 5643 , shortDoco, usage, any); 5644 } 5645 5646 public String fhirType() { 5647 return "ElementDefinition.binding.additional"; 5648 5649 } 5650 5651 } 5652 5653 @Block() 5654 public static class ElementDefinitionMappingComponent extends Element implements IBaseDatatypeElement { 5655 @Override 5656 public String toString() { 5657 return identity+"=" + map; 5658 } 5659 5660 /** 5661 * An internal reference to the definition of a mapping. 5662 */ 5663 @Child(name = "identity", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 5664 @Description(shortDefinition="Reference to mapping declaration", formalDefinition="An internal reference to the definition of a mapping." ) 5665 protected IdType identity; 5666 5667 /** 5668 * Identifies the computable language in which mapping.map is expressed. 5669 */ 5670 @Child(name = "language", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 5671 @Description(shortDefinition="Computable language of mapping", formalDefinition="Identifies the computable language in which mapping.map is expressed." ) 5672 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 5673 protected CodeType language; 5674 5675 /** 5676 * Expresses what part of the target specification corresponds to this element. 5677 */ 5678 @Child(name = "map", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 5679 @Description(shortDefinition="Details of the mapping", formalDefinition="Expresses what part of the target specification corresponds to this element." ) 5680 protected StringType map; 5681 5682 /** 5683 * Comments that provide information about the mapping or its use. 5684 */ 5685 @Child(name = "comment", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=true) 5686 @Description(shortDefinition="Comments about the mapping or its use", formalDefinition="Comments that provide information about the mapping or its use." ) 5687 protected MarkdownType comment; 5688 5689 private static final long serialVersionUID = -582458727L; 5690 5691 /** 5692 * Constructor 5693 */ 5694 public ElementDefinitionMappingComponent() { 5695 super(); 5696 } 5697 5698 /** 5699 * Constructor 5700 */ 5701 public ElementDefinitionMappingComponent(String identity, String map) { 5702 super(); 5703 this.setIdentity(identity); 5704 this.setMap(map); 5705 } 5706 5707 /** 5708 * @return {@link #identity} (An internal reference to the definition of a mapping.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 5709 */ 5710 public IdType getIdentityElement() { 5711 if (this.identity == null) 5712 if (Configuration.errorOnAutoCreate()) 5713 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.identity"); 5714 else if (Configuration.doAutoCreate()) 5715 this.identity = new IdType(); // bb 5716 return this.identity; 5717 } 5718 5719 public boolean hasIdentityElement() { 5720 return this.identity != null && !this.identity.isEmpty(); 5721 } 5722 5723 public boolean hasIdentity() { 5724 return this.identity != null && !this.identity.isEmpty(); 5725 } 5726 5727 /** 5728 * @param value {@link #identity} (An internal reference to the definition of a mapping.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 5729 */ 5730 public ElementDefinitionMappingComponent setIdentityElement(IdType value) { 5731 this.identity = value; 5732 return this; 5733 } 5734 5735 /** 5736 * @return An internal reference to the definition of a mapping. 5737 */ 5738 public String getIdentity() { 5739 return this.identity == null ? null : this.identity.getValue(); 5740 } 5741 5742 /** 5743 * @param value An internal reference to the definition of a mapping. 5744 */ 5745 public ElementDefinitionMappingComponent setIdentity(String value) { 5746 if (this.identity == null) 5747 this.identity = new IdType(); 5748 this.identity.setValue(value); 5749 return this; 5750 } 5751 5752 /** 5753 * @return {@link #language} (Identifies the computable language in which mapping.map is expressed.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 5754 */ 5755 public CodeType getLanguageElement() { 5756 if (this.language == null) 5757 if (Configuration.errorOnAutoCreate()) 5758 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.language"); 5759 else if (Configuration.doAutoCreate()) 5760 this.language = new CodeType(); // bb 5761 return this.language; 5762 } 5763 5764 public boolean hasLanguageElement() { 5765 return this.language != null && !this.language.isEmpty(); 5766 } 5767 5768 public boolean hasLanguage() { 5769 return this.language != null && !this.language.isEmpty(); 5770 } 5771 5772 /** 5773 * @param value {@link #language} (Identifies the computable language in which mapping.map is expressed.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 5774 */ 5775 public ElementDefinitionMappingComponent setLanguageElement(CodeType value) { 5776 this.language = value; 5777 return this; 5778 } 5779 5780 /** 5781 * @return Identifies the computable language in which mapping.map is expressed. 5782 */ 5783 public String getLanguage() { 5784 return this.language == null ? null : this.language.getValue(); 5785 } 5786 5787 /** 5788 * @param value Identifies the computable language in which mapping.map is expressed. 5789 */ 5790 public ElementDefinitionMappingComponent setLanguage(String value) { 5791 if (Utilities.noString(value)) 5792 this.language = null; 5793 else { 5794 if (this.language == null) 5795 this.language = new CodeType(); 5796 this.language.setValue(value); 5797 } 5798 return this; 5799 } 5800 5801 /** 5802 * @return {@link #map} (Expresses what part of the target specification corresponds to this element.). This is the underlying object with id, value and extensions. The accessor "getMap" gives direct access to the value 5803 */ 5804 public StringType getMapElement() { 5805 if (this.map == null) 5806 if (Configuration.errorOnAutoCreate()) 5807 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.map"); 5808 else if (Configuration.doAutoCreate()) 5809 this.map = new StringType(); // bb 5810 return this.map; 5811 } 5812 5813 public boolean hasMapElement() { 5814 return this.map != null && !this.map.isEmpty(); 5815 } 5816 5817 public boolean hasMap() { 5818 return this.map != null && !this.map.isEmpty(); 5819 } 5820 5821 /** 5822 * @param value {@link #map} (Expresses what part of the target specification corresponds to this element.). This is the underlying object with id, value and extensions. The accessor "getMap" gives direct access to the value 5823 */ 5824 public ElementDefinitionMappingComponent setMapElement(StringType value) { 5825 this.map = value; 5826 return this; 5827 } 5828 5829 /** 5830 * @return Expresses what part of the target specification corresponds to this element. 5831 */ 5832 public String getMap() { 5833 return this.map == null ? null : this.map.getValue(); 5834 } 5835 5836 /** 5837 * @param value Expresses what part of the target specification corresponds to this element. 5838 */ 5839 public ElementDefinitionMappingComponent setMap(String value) { 5840 if (this.map == null) 5841 this.map = new StringType(); 5842 this.map.setValue(value); 5843 return this; 5844 } 5845 5846 /** 5847 * @return {@link #comment} (Comments that provide information about the mapping or its use.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 5848 */ 5849 public MarkdownType getCommentElement() { 5850 if (this.comment == null) 5851 if (Configuration.errorOnAutoCreate()) 5852 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.comment"); 5853 else if (Configuration.doAutoCreate()) 5854 this.comment = new MarkdownType(); // bb 5855 return this.comment; 5856 } 5857 5858 public boolean hasCommentElement() { 5859 return this.comment != null && !this.comment.isEmpty(); 5860 } 5861 5862 public boolean hasComment() { 5863 return this.comment != null && !this.comment.isEmpty(); 5864 } 5865 5866 /** 5867 * @param value {@link #comment} (Comments that provide information about the mapping or its use.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 5868 */ 5869 public ElementDefinitionMappingComponent setCommentElement(MarkdownType value) { 5870 this.comment = value; 5871 return this; 5872 } 5873 5874 /** 5875 * @return Comments that provide information about the mapping or its use. 5876 */ 5877 public String getComment() { 5878 return this.comment == null ? null : this.comment.getValue(); 5879 } 5880 5881 /** 5882 * @param value Comments that provide information about the mapping or its use. 5883 */ 5884 public ElementDefinitionMappingComponent setComment(String value) { 5885 if (Utilities.noString(value)) 5886 this.comment = null; 5887 else { 5888 if (this.comment == null) 5889 this.comment = new MarkdownType(); 5890 this.comment.setValue(value); 5891 } 5892 return this; 5893 } 5894 5895 protected void listChildren(List<Property> children) { 5896 super.listChildren(children); 5897 children.add(new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity)); 5898 children.add(new Property("language", "code", "Identifies the computable language in which mapping.map is expressed.", 0, 1, language)); 5899 children.add(new Property("map", "string", "Expresses what part of the target specification corresponds to this element.", 0, 1, map)); 5900 children.add(new Property("comment", "markdown", "Comments that provide information about the mapping or its use.", 0, 1, comment)); 5901 } 5902 5903 @Override 5904 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5905 switch (_hash) { 5906 case -135761730: /*identity*/ return new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity); 5907 case -1613589672: /*language*/ return new Property("language", "code", "Identifies the computable language in which mapping.map is expressed.", 0, 1, language); 5908 case 107868: /*map*/ return new Property("map", "string", "Expresses what part of the target specification corresponds to this element.", 0, 1, map); 5909 case 950398559: /*comment*/ return new Property("comment", "markdown", "Comments that provide information about the mapping or its use.", 0, 1, comment); 5910 default: return super.getNamedProperty(_hash, _name, _checkValid); 5911 } 5912 5913 } 5914 5915 @Override 5916 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5917 switch (hash) { 5918 case -135761730: /*identity*/ return this.identity == null ? new Base[0] : new Base[] {this.identity}; // IdType 5919 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 5920 case 107868: /*map*/ return this.map == null ? new Base[0] : new Base[] {this.map}; // StringType 5921 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 5922 default: return super.getProperty(hash, name, checkValid); 5923 } 5924 5925 } 5926 5927 @Override 5928 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5929 switch (hash) { 5930 case -135761730: // identity 5931 this.identity = TypeConvertor.castToId(value); // IdType 5932 return value; 5933 case -1613589672: // language 5934 this.language = TypeConvertor.castToCode(value); // CodeType 5935 return value; 5936 case 107868: // map 5937 this.map = TypeConvertor.castToString(value); // StringType 5938 return value; 5939 case 950398559: // comment 5940 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 5941 return value; 5942 default: return super.setProperty(hash, name, value); 5943 } 5944 5945 } 5946 5947 @Override 5948 public Base setProperty(String name, Base value) throws FHIRException { 5949 if (name.equals("identity")) { 5950 this.identity = TypeConvertor.castToId(value); // IdType 5951 } else if (name.equals("language")) { 5952 this.language = TypeConvertor.castToCode(value); // CodeType 5953 } else if (name.equals("map")) { 5954 this.map = TypeConvertor.castToString(value); // StringType 5955 } else if (name.equals("comment")) { 5956 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 5957 } else 5958 return super.setProperty(name, value); 5959 return value; 5960 } 5961 5962 @Override 5963 public void removeChild(String name, Base value) throws FHIRException { 5964 if (name.equals("identity")) { 5965 this.identity = null; 5966 } else if (name.equals("language")) { 5967 this.language = null; 5968 } else if (name.equals("map")) { 5969 this.map = null; 5970 } else if (name.equals("comment")) { 5971 this.comment = null; 5972 } else 5973 super.removeChild(name, value); 5974 5975 } 5976 5977 @Override 5978 public Base makeProperty(int hash, String name) throws FHIRException { 5979 switch (hash) { 5980 case -135761730: return getIdentityElement(); 5981 case -1613589672: return getLanguageElement(); 5982 case 107868: return getMapElement(); 5983 case 950398559: return getCommentElement(); 5984 default: return super.makeProperty(hash, name); 5985 } 5986 5987 } 5988 5989 @Override 5990 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5991 switch (hash) { 5992 case -135761730: /*identity*/ return new String[] {"id"}; 5993 case -1613589672: /*language*/ return new String[] {"code"}; 5994 case 107868: /*map*/ return new String[] {"string"}; 5995 case 950398559: /*comment*/ return new String[] {"markdown"}; 5996 default: return super.getTypesForProperty(hash, name); 5997 } 5998 5999 } 6000 6001 @Override 6002 public Base addChild(String name) throws FHIRException { 6003 if (name.equals("identity")) { 6004 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.identity"); 6005 } 6006 else if (name.equals("language")) { 6007 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.language"); 6008 } 6009 else if (name.equals("map")) { 6010 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.map"); 6011 } 6012 else if (name.equals("comment")) { 6013 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.comment"); 6014 } 6015 else 6016 return super.addChild(name); 6017 } 6018 6019 public ElementDefinitionMappingComponent copy() { 6020 ElementDefinitionMappingComponent dst = new ElementDefinitionMappingComponent(); 6021 copyValues(dst); 6022 return dst; 6023 } 6024 6025 public void copyValues(ElementDefinitionMappingComponent dst) { 6026 super.copyValues(dst); 6027 dst.identity = identity == null ? null : identity.copy(); 6028 dst.language = language == null ? null : language.copy(); 6029 dst.map = map == null ? null : map.copy(); 6030 dst.comment = comment == null ? null : comment.copy(); 6031 } 6032 6033 @Override 6034 public boolean equalsDeep(Base other_) { 6035 if (!super.equalsDeep(other_)) 6036 return false; 6037 if (!(other_ instanceof ElementDefinitionMappingComponent)) 6038 return false; 6039 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 6040 return compareDeep(identity, o.identity, true) && compareDeep(language, o.language, true) && compareDeep(map, o.map, true) 6041 && compareDeep(comment, o.comment, true); 6042 } 6043 6044 @Override 6045 public boolean equalsShallow(Base other_) { 6046 if (!super.equalsShallow(other_)) 6047 return false; 6048 if (!(other_ instanceof ElementDefinitionMappingComponent)) 6049 return false; 6050 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 6051 return compareValues(identity, o.identity, true) && compareValues(language, o.language, true) && compareValues(map, o.map, true) 6052 && compareValues(comment, o.comment, true); 6053 } 6054 6055 public boolean isEmpty() { 6056 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, language, map 6057 , comment); 6058 } 6059 6060 public String fhirType() { 6061 return "ElementDefinition.mapping"; 6062 6063 } 6064 6065 } 6066 6067 /** 6068 * The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 6069 */ 6070 @Child(name = "path", type = {StringType.class}, order=0, min=1, max=1, modifier=false, summary=true) 6071 @Description(shortDefinition="Path of the element in the hierarchy of elements", formalDefinition="The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension." ) 6072 protected StringType path; 6073 6074 /** 6075 * Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used. 6076 */ 6077 @Child(name = "representation", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6078 @Description(shortDefinition="xmlAttr | xmlText | typeAttr | cdaText | xhtml", formalDefinition="Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used." ) 6079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/property-representation") 6080 protected List<Enumeration<PropertyRepresentation>> representation; 6081 6082 /** 6083 * The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 6084 */ 6085 @Child(name = "sliceName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 6086 @Description(shortDefinition="Name for this particular element (in a set of slices)", formalDefinition="The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element." ) 6087 protected StringType sliceName; 6088 6089 /** 6090 * If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName. 6091 */ 6092 @Child(name = "sliceIsConstraining", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 6093 @Description(shortDefinition="If this slice definition constrains an inherited slice definition (or not)", formalDefinition="If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName." ) 6094 protected BooleanType sliceIsConstraining; 6095 6096 /** 6097 * A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 6098 */ 6099 @Child(name = "label", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 6100 @Description(shortDefinition="Name for element to display with or prompt for element", formalDefinition="A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form." ) 6101 protected StringType label; 6102 6103 /** 6104 * A code that has the same meaning as the element in a particular terminology. 6105 */ 6106 @Child(name = "code", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6107 @Description(shortDefinition="Corresponding codes in terminologies", formalDefinition="A code that has the same meaning as the element in a particular terminology." ) 6108 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://loinc.org/vs") 6109 protected List<Coding> code; 6110 6111 /** 6112 * Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set). 6113 */ 6114 @Child(name = "slicing", type = {}, order=6, min=0, max=1, modifier=false, summary=true) 6115 @Description(shortDefinition="This element is sliced - slices follow", formalDefinition="Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set)." ) 6116 protected ElementDefinitionSlicingComponent slicing; 6117 6118 /** 6119 * A concise description of what this element means (e.g. for use in autogenerated summaries). 6120 */ 6121 @Child(name = "short", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 6122 @Description(shortDefinition="Concise definition for space-constrained presentation", formalDefinition="A concise description of what this element means (e.g. for use in autogenerated summaries)." ) 6123 protected StringType short_; 6124 6125 /** 6126 * Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition). 6127 */ 6128 @Child(name = "definition", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=true) 6129 @Description(shortDefinition="Full formal definition as narrative text", formalDefinition="Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition)." ) 6130 protected MarkdownType definition; 6131 6132 /** 6133 * Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment). 6134 */ 6135 @Child(name = "comment", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 6136 @Description(shortDefinition="Comments about the use of this element", formalDefinition="Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment)." ) 6137 protected MarkdownType comment; 6138 6139 /** 6140 * This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 6141 */ 6142 @Child(name = "requirements", type = {MarkdownType.class}, order=10, min=0, max=1, modifier=false, summary=true) 6143 @Description(shortDefinition="Why this resource has been created", formalDefinition="This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element." ) 6144 protected MarkdownType requirements; 6145 6146 /** 6147 * Identifies additional names by which this element might also be known. 6148 */ 6149 @Child(name = "alias", type = {StringType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6150 @Description(shortDefinition="Other names", formalDefinition="Identifies additional names by which this element might also be known." ) 6151 protected List<StringType> alias; 6152 6153 /** 6154 * The minimum number of times this element SHALL appear in the instance. 6155 */ 6156 @Child(name = "min", type = {UnsignedIntType.class}, order=12, min=0, max=1, modifier=false, summary=true) 6157 @Description(shortDefinition="Minimum Cardinality", formalDefinition="The minimum number of times this element SHALL appear in the instance." ) 6158 protected UnsignedIntType min; 6159 6160 /** 6161 * The maximum number of times this element is permitted to appear in the instance. 6162 */ 6163 @Child(name = "max", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=true) 6164 @Description(shortDefinition="Maximum Cardinality (a number or *)", formalDefinition="The maximum number of times this element is permitted to appear in the instance." ) 6165 protected StringType max; 6166 6167 /** 6168 * Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same. 6169 */ 6170 @Child(name = "base", type = {}, order=14, min=0, max=1, modifier=false, summary=true) 6171 @Description(shortDefinition="Base definition information for tools", formalDefinition="Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same." ) 6172 protected ElementDefinitionBaseComponent base; 6173 6174 /** 6175 * Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc. 6176 */ 6177 @Child(name = "contentReference", type = {UriType.class}, order=15, min=0, max=1, modifier=false, summary=true) 6178 @Description(shortDefinition="Reference to definition of content for the element", formalDefinition="Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc." ) 6179 protected UriType contentReference; 6180 6181 /** 6182 * The data type or resource that the value of this element is permitted to be. 6183 */ 6184 @Child(name = "type", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6185 @Description(shortDefinition="Data type and Profile for this element", formalDefinition="The data type or resource that the value of this element is permitted to be." ) 6186 protected List<TypeRefComponent> type; 6187 6188 /** 6189 * The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false'). 6190 */ 6191 @Child(name = "defaultValue", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=17, min=0, max=1, modifier=false, summary=true) 6192 @Description(shortDefinition="Specified value if missing from instance", formalDefinition="The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false')." ) 6193 protected DataType defaultValue; 6194 6195 /** 6196 * The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'). 6197 */ 6198 @Child(name = "meaningWhenMissing", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=true) 6199 @Description(shortDefinition="Implicit meaning when this element is missing", formalDefinition="The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing')." ) 6200 protected MarkdownType meaningWhenMissing; 6201 6202 /** 6203 * If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 6204 */ 6205 @Child(name = "orderMeaning", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=true) 6206 @Description(shortDefinition="What the order of the elements means", formalDefinition="If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning." ) 6207 protected StringType orderMeaning; 6208 6209 /** 6210 * Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing. 6211 */ 6212 @Child(name = "fixed", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=20, min=0, max=1, modifier=false, summary=true) 6213 @Description(shortDefinition="Value must be exactly this", formalDefinition="Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing." ) 6214 protected DataType fixed; 6215 6216 /** 6217 * Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 6218 6219When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 6220 6221When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 6222 6223When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 6224 62251. If primitive: it must match exactly the pattern value 62262. If a complex object: it must match (recursively) the pattern value 62273. If an array: it must match (recursively) the pattern value 6228 6229If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have. 6230 */ 6231 @Child(name = "pattern", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=21, min=0, max=1, modifier=false, summary=true) 6232 @Description(shortDefinition="Value must have at least these property values", formalDefinition="Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have." ) 6233 protected DataType pattern; 6234 6235 /** 6236 * A sample value for this element demonstrating the type of information that would typically be found in the element. 6237 */ 6238 @Child(name = "example", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6239 @Description(shortDefinition="Example value (as defined for type)", formalDefinition="A sample value for this element demonstrating the type of information that would typically be found in the element." ) 6240 protected List<ElementDefinitionExampleComponent> example; 6241 6242 /** 6243 * The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity. 6244 */ 6245 @Child(name = "minValue", type = {DateType.class, DateTimeType.class, InstantType.class, TimeType.class, DecimalType.class, IntegerType.class, Integer64Type.class, PositiveIntType.class, UnsignedIntType.class, Quantity.class}, order=23, min=0, max=1, modifier=false, summary=true) 6246 @Description(shortDefinition="Minimum Allowed Value (for some types)", formalDefinition="The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity." ) 6247 protected DataType minValue; 6248 6249 /** 6250 * The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity. 6251 */ 6252 @Child(name = "maxValue", type = {DateType.class, DateTimeType.class, InstantType.class, TimeType.class, DecimalType.class, IntegerType.class, Integer64Type.class, PositiveIntType.class, UnsignedIntType.class, Quantity.class}, order=24, min=0, max=1, modifier=false, summary=true) 6253 @Description(shortDefinition="Maximum Allowed Value (for some types)", formalDefinition="The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity." ) 6254 protected DataType maxValue; 6255 6256 /** 6257 * Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)). 6258 */ 6259 @Child(name = "maxLength", type = {IntegerType.class}, order=25, min=0, max=1, modifier=false, summary=true) 6260 @Description(shortDefinition="Max length for string type data", formalDefinition="Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html))." ) 6261 protected IntegerType maxLength; 6262 6263 /** 6264 * A reference to an invariant that may make additional statements about the cardinality or value in the instance. 6265 */ 6266 @Child(name = "condition", type = {IdType.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6267 @Description(shortDefinition="Reference to invariant about presence", formalDefinition="A reference to an invariant that may make additional statements about the cardinality or value in the instance." ) 6268 protected List<IdType> condition; 6269 6270 /** 6271 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance. 6272 */ 6273 @Child(name = "constraint", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6274 @Description(shortDefinition="Condition that must evaluate to true", formalDefinition="Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance." ) 6275 protected List<ElementDefinitionConstraintComponent> constraint; 6276 6277 /** 6278 * Specifies for a primitive data type that the value of the data type cannot be replaced by an extension. 6279 */ 6280 @Child(name = "mustHaveValue", type = {BooleanType.class}, order=28, min=0, max=1, modifier=false, summary=true) 6281 @Description(shortDefinition="For primitives, that a value must be present - not replaced by an extension", formalDefinition="Specifies for a primitive data type that the value of the data type cannot be replaced by an extension." ) 6282 protected BooleanType mustHaveValue; 6283 6284 /** 6285 * Specifies a list of extensions that can appear in place of a primitive value. 6286 */ 6287 @Child(name = "valueAlternatives", type = {CanonicalType.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6288 @Description(shortDefinition="Extensions that are allowed to replace a primitive value", formalDefinition="Specifies a list of extensions that can appear in place of a primitive value." ) 6289 protected List<CanonicalType> valueAlternatives; 6290 6291 /** 6292 * If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation. 6293 */ 6294 @Child(name = "mustSupport", type = {BooleanType.class}, order=30, min=0, max=1, modifier=false, summary=true) 6295 @Description(shortDefinition="If the element must be supported (discouraged - see obligations)", formalDefinition="If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation." ) 6296 protected BooleanType mustSupport; 6297 6298 /** 6299 * If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension. 6300 */ 6301 @Child(name = "isModifier", type = {BooleanType.class}, order=31, min=0, max=1, modifier=false, summary=true) 6302 @Description(shortDefinition="If this modifies the meaning of other elements", formalDefinition="If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension." ) 6303 protected BooleanType isModifier; 6304 6305 /** 6306 * Explains how that element affects the interpretation of the resource or element that contains it. 6307 */ 6308 @Child(name = "isModifierReason", type = {StringType.class}, order=32, min=0, max=1, modifier=false, summary=true) 6309 @Description(shortDefinition="Reason that this element is marked as a modifier", formalDefinition="Explains how that element affects the interpretation of the resource or element that contains it." ) 6310 protected StringType isModifierReason; 6311 6312 /** 6313 * Whether the element should be included if a client requests a search with the parameter _summary=true. 6314 */ 6315 @Child(name = "isSummary", type = {BooleanType.class}, order=33, min=0, max=1, modifier=false, summary=true) 6316 @Description(shortDefinition="Include when _summary = true?", formalDefinition="Whether the element should be included if a client requests a search with the parameter _summary=true." ) 6317 protected BooleanType isSummary; 6318 6319 /** 6320 * Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri). 6321 */ 6322 @Child(name = "binding", type = {}, order=34, min=0, max=1, modifier=false, summary=true) 6323 @Description(shortDefinition="ValueSet details if this is coded", formalDefinition="Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri)." ) 6324 protected ElementDefinitionBindingComponent binding; 6325 6326 /** 6327 * Identifies a concept from an external specification that roughly corresponds to this element. 6328 */ 6329 @Child(name = "mapping", type = {}, order=35, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6330 @Description(shortDefinition="Map element to another set of definitions", formalDefinition="Identifies a concept from an external specification that roughly corresponds to this element." ) 6331 protected List<ElementDefinitionMappingComponent> mapping; 6332 6333 private static final long serialVersionUID = -1474105308L; 6334 6335 /** 6336 * Constructor 6337 */ 6338 public ElementDefinition() { 6339 super(); 6340 } 6341 6342 /** 6343 * Constructor 6344 */ 6345 public ElementDefinition(String path) { 6346 super(); 6347 this.setPath(path); 6348 } 6349 6350 /** 6351 * @return {@link #path} (The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 6352 */ 6353 public StringType getPathElement() { 6354 if (this.path == null) 6355 if (Configuration.errorOnAutoCreate()) 6356 throw new Error("Attempt to auto-create ElementDefinition.path"); 6357 else if (Configuration.doAutoCreate()) 6358 this.path = new StringType(); // bb 6359 return this.path; 6360 } 6361 6362 public boolean hasPathElement() { 6363 return this.path != null && !this.path.isEmpty(); 6364 } 6365 6366 public boolean hasPath() { 6367 return this.path != null && !this.path.isEmpty(); 6368 } 6369 6370 /** 6371 * @param value {@link #path} (The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 6372 */ 6373 public ElementDefinition setPathElement(StringType value) { 6374 this.path = value; 6375 return this; 6376 } 6377 6378 /** 6379 * @return The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 6380 */ 6381 public String getPath() { 6382 return this.path == null ? null : this.path.getValue(); 6383 } 6384 6385 /** 6386 * @param value The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 6387 */ 6388 public ElementDefinition setPath(String value) { 6389 if (this.path == null) 6390 this.path = new StringType(); 6391 this.path.setValue(value); 6392 return this; 6393 } 6394 6395 /** 6396 * @return {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6397 */ 6398 public List<Enumeration<PropertyRepresentation>> getRepresentation() { 6399 if (this.representation == null) 6400 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 6401 return this.representation; 6402 } 6403 6404 /** 6405 * @return Returns a reference to <code>this</code> for easy method chaining 6406 */ 6407 public ElementDefinition setRepresentation(List<Enumeration<PropertyRepresentation>> theRepresentation) { 6408 this.representation = theRepresentation; 6409 return this; 6410 } 6411 6412 public boolean hasRepresentation() { 6413 if (this.representation == null) 6414 return false; 6415 for (Enumeration<PropertyRepresentation> item : this.representation) 6416 if (!item.isEmpty()) 6417 return true; 6418 return false; 6419 } 6420 6421 /** 6422 * @return {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6423 */ 6424 public Enumeration<PropertyRepresentation> addRepresentationElement() {//2 6425 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>(new PropertyRepresentationEnumFactory()); 6426 if (this.representation == null) 6427 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 6428 this.representation.add(t); 6429 return t; 6430 } 6431 6432 /** 6433 * @param value {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6434 */ 6435 public ElementDefinition addRepresentation(PropertyRepresentation value) { //1 6436 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>(new PropertyRepresentationEnumFactory()); 6437 t.setValue(value); 6438 if (this.representation == null) 6439 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 6440 this.representation.add(t); 6441 return this; 6442 } 6443 6444 /** 6445 * @param value {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6446 */ 6447 public boolean hasRepresentation(PropertyRepresentation value) { 6448 if (this.representation == null) 6449 return false; 6450 for (Enumeration<PropertyRepresentation> v : this.representation) 6451 if (v.getValue().equals(value)) // code 6452 return true; 6453 return false; 6454 } 6455 6456 /** 6457 * @return {@link #sliceName} (The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 6458 */ 6459 public StringType getSliceNameElement() { 6460 if (this.sliceName == null) 6461 if (Configuration.errorOnAutoCreate()) 6462 throw new Error("Attempt to auto-create ElementDefinition.sliceName"); 6463 else if (Configuration.doAutoCreate()) 6464 this.sliceName = new StringType(); // bb 6465 return this.sliceName; 6466 } 6467 6468 public boolean hasSliceNameElement() { 6469 return this.sliceName != null && !this.sliceName.isEmpty(); 6470 } 6471 6472 public boolean hasSliceName() { 6473 return this.sliceName != null && !this.sliceName.isEmpty(); 6474 } 6475 6476 /** 6477 * @param value {@link #sliceName} (The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 6478 */ 6479 public ElementDefinition setSliceNameElement(StringType value) { 6480 this.sliceName = value; 6481 return this; 6482 } 6483 6484 /** 6485 * @return The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 6486 */ 6487 public String getSliceName() { 6488 return this.sliceName == null ? null : this.sliceName.getValue(); 6489 } 6490 6491 /** 6492 * @param value The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 6493 */ 6494 public ElementDefinition setSliceName(String value) { 6495 if (Utilities.noString(value)) 6496 this.sliceName = null; 6497 else { 6498 if (this.sliceName == null) 6499 this.sliceName = new StringType(); 6500 this.sliceName.setValue(value); 6501 } 6502 return this; 6503 } 6504 6505 /** 6506 * @return {@link #sliceIsConstraining} (If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.). This is the underlying object with id, value and extensions. The accessor "getSliceIsConstraining" gives direct access to the value 6507 */ 6508 public BooleanType getSliceIsConstrainingElement() { 6509 if (this.sliceIsConstraining == null) 6510 if (Configuration.errorOnAutoCreate()) 6511 throw new Error("Attempt to auto-create ElementDefinition.sliceIsConstraining"); 6512 else if (Configuration.doAutoCreate()) 6513 this.sliceIsConstraining = new BooleanType(); // bb 6514 return this.sliceIsConstraining; 6515 } 6516 6517 public boolean hasSliceIsConstrainingElement() { 6518 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 6519 } 6520 6521 public boolean hasSliceIsConstraining() { 6522 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 6523 } 6524 6525 /** 6526 * @param value {@link #sliceIsConstraining} (If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.). This is the underlying object with id, value and extensions. The accessor "getSliceIsConstraining" gives direct access to the value 6527 */ 6528 public ElementDefinition setSliceIsConstrainingElement(BooleanType value) { 6529 this.sliceIsConstraining = value; 6530 return this; 6531 } 6532 6533 /** 6534 * @return If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName. 6535 */ 6536 public boolean getSliceIsConstraining() { 6537 return this.sliceIsConstraining == null || this.sliceIsConstraining.isEmpty() ? false : this.sliceIsConstraining.getValue(); 6538 } 6539 6540 /** 6541 * @param value If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName. 6542 */ 6543 public ElementDefinition setSliceIsConstraining(boolean value) { 6544 if (this.sliceIsConstraining == null) 6545 this.sliceIsConstraining = new BooleanType(); 6546 this.sliceIsConstraining.setValue(value); 6547 return this; 6548 } 6549 6550 /** 6551 * @return {@link #label} (A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6552 */ 6553 public StringType getLabelElement() { 6554 if (this.label == null) 6555 if (Configuration.errorOnAutoCreate()) 6556 throw new Error("Attempt to auto-create ElementDefinition.label"); 6557 else if (Configuration.doAutoCreate()) 6558 this.label = new StringType(); // bb 6559 return this.label; 6560 } 6561 6562 public boolean hasLabelElement() { 6563 return this.label != null && !this.label.isEmpty(); 6564 } 6565 6566 public boolean hasLabel() { 6567 return this.label != null && !this.label.isEmpty(); 6568 } 6569 6570 /** 6571 * @param value {@link #label} (A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6572 */ 6573 public ElementDefinition setLabelElement(StringType value) { 6574 this.label = value; 6575 return this; 6576 } 6577 6578 /** 6579 * @return A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 6580 */ 6581 public String getLabel() { 6582 return this.label == null ? null : this.label.getValue(); 6583 } 6584 6585 /** 6586 * @param value A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 6587 */ 6588 public ElementDefinition setLabel(String value) { 6589 if (Utilities.noString(value)) 6590 this.label = null; 6591 else { 6592 if (this.label == null) 6593 this.label = new StringType(); 6594 this.label.setValue(value); 6595 } 6596 return this; 6597 } 6598 6599 /** 6600 * @return {@link #code} (A code that has the same meaning as the element in a particular terminology.) 6601 */ 6602 public List<Coding> getCode() { 6603 if (this.code == null) 6604 this.code = new ArrayList<Coding>(); 6605 return this.code; 6606 } 6607 6608 /** 6609 * @return Returns a reference to <code>this</code> for easy method chaining 6610 */ 6611 public ElementDefinition setCode(List<Coding> theCode) { 6612 this.code = theCode; 6613 return this; 6614 } 6615 6616 public boolean hasCode() { 6617 if (this.code == null) 6618 return false; 6619 for (Coding item : this.code) 6620 if (!item.isEmpty()) 6621 return true; 6622 return false; 6623 } 6624 6625 public Coding addCode() { //3 6626 Coding t = new Coding(); 6627 if (this.code == null) 6628 this.code = new ArrayList<Coding>(); 6629 this.code.add(t); 6630 return t; 6631 } 6632 6633 public ElementDefinition addCode(Coding t) { //3 6634 if (t == null) 6635 return this; 6636 if (this.code == null) 6637 this.code = new ArrayList<Coding>(); 6638 this.code.add(t); 6639 return this; 6640 } 6641 6642 /** 6643 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 6644 */ 6645 public Coding getCodeFirstRep() { 6646 if (getCode().isEmpty()) { 6647 addCode(); 6648 } 6649 return getCode().get(0); 6650 } 6651 6652 /** 6653 * @return {@link #slicing} (Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).) 6654 */ 6655 public ElementDefinitionSlicingComponent getSlicing() { 6656 if (this.slicing == null) 6657 if (Configuration.errorOnAutoCreate()) 6658 throw new Error("Attempt to auto-create ElementDefinition.slicing"); 6659 else if (Configuration.doAutoCreate()) 6660 this.slicing = new ElementDefinitionSlicingComponent(); // cc 6661 return this.slicing; 6662 } 6663 6664 public boolean hasSlicing() { 6665 return this.slicing != null && !this.slicing.isEmpty(); 6666 } 6667 6668 /** 6669 * @param value {@link #slicing} (Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).) 6670 */ 6671 public ElementDefinition setSlicing(ElementDefinitionSlicingComponent value) { 6672 this.slicing = value; 6673 return this; 6674 } 6675 6676 /** 6677 * @return {@link #short_} (A concise description of what this element means (e.g. for use in autogenerated summaries).). This is the underlying object with id, value and extensions. The accessor "getShort" gives direct access to the value 6678 */ 6679 public StringType getShortElement() { 6680 if (this.short_ == null) 6681 if (Configuration.errorOnAutoCreate()) 6682 throw new Error("Attempt to auto-create ElementDefinition.short_"); 6683 else if (Configuration.doAutoCreate()) 6684 this.short_ = new StringType(); // bb 6685 return this.short_; 6686 } 6687 6688 public boolean hasShortElement() { 6689 return this.short_ != null && !this.short_.isEmpty(); 6690 } 6691 6692 public boolean hasShort() { 6693 return this.short_ != null && !this.short_.isEmpty(); 6694 } 6695 6696 /** 6697 * @param value {@link #short_} (A concise description of what this element means (e.g. for use in autogenerated summaries).). This is the underlying object with id, value and extensions. The accessor "getShort" gives direct access to the value 6698 */ 6699 public ElementDefinition setShortElement(StringType value) { 6700 this.short_ = value; 6701 return this; 6702 } 6703 6704 /** 6705 * @return A concise description of what this element means (e.g. for use in autogenerated summaries). 6706 */ 6707 public String getShort() { 6708 return this.short_ == null ? null : this.short_.getValue(); 6709 } 6710 6711 /** 6712 * @param value A concise description of what this element means (e.g. for use in autogenerated summaries). 6713 */ 6714 public ElementDefinition setShort(String value) { 6715 if (Utilities.noString(value)) 6716 this.short_ = null; 6717 else { 6718 if (this.short_ == null) 6719 this.short_ = new StringType(); 6720 this.short_.setValue(value); 6721 } 6722 return this; 6723 } 6724 6725 /** 6726 * @return {@link #definition} (Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 6727 */ 6728 public MarkdownType getDefinitionElement() { 6729 if (this.definition == null) 6730 if (Configuration.errorOnAutoCreate()) 6731 throw new Error("Attempt to auto-create ElementDefinition.definition"); 6732 else if (Configuration.doAutoCreate()) 6733 this.definition = new MarkdownType(); // bb 6734 return this.definition; 6735 } 6736 6737 public boolean hasDefinitionElement() { 6738 return this.definition != null && !this.definition.isEmpty(); 6739 } 6740 6741 public boolean hasDefinition() { 6742 return this.definition != null && !this.definition.isEmpty(); 6743 } 6744 6745 /** 6746 * @param value {@link #definition} (Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 6747 */ 6748 public ElementDefinition setDefinitionElement(MarkdownType value) { 6749 this.definition = value; 6750 return this; 6751 } 6752 6753 /** 6754 * @return Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition). 6755 */ 6756 public String getDefinition() { 6757 return this.definition == null ? null : this.definition.getValue(); 6758 } 6759 6760 /** 6761 * @param value Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition). 6762 */ 6763 public ElementDefinition setDefinition(String value) { 6764 if (Utilities.noString(value)) 6765 this.definition = null; 6766 else { 6767 if (this.definition == null) 6768 this.definition = new MarkdownType(); 6769 this.definition.setValue(value); 6770 } 6771 return this; 6772 } 6773 6774 /** 6775 * @return {@link #comment} (Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 6776 */ 6777 public MarkdownType getCommentElement() { 6778 if (this.comment == null) 6779 if (Configuration.errorOnAutoCreate()) 6780 throw new Error("Attempt to auto-create ElementDefinition.comment"); 6781 else if (Configuration.doAutoCreate()) 6782 this.comment = new MarkdownType(); // bb 6783 return this.comment; 6784 } 6785 6786 public boolean hasCommentElement() { 6787 return this.comment != null && !this.comment.isEmpty(); 6788 } 6789 6790 public boolean hasComment() { 6791 return this.comment != null && !this.comment.isEmpty(); 6792 } 6793 6794 /** 6795 * @param value {@link #comment} (Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 6796 */ 6797 public ElementDefinition setCommentElement(MarkdownType value) { 6798 this.comment = value; 6799 return this; 6800 } 6801 6802 /** 6803 * @return Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment). 6804 */ 6805 public String getComment() { 6806 return this.comment == null ? null : this.comment.getValue(); 6807 } 6808 6809 /** 6810 * @param value Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment). 6811 */ 6812 public ElementDefinition setComment(String value) { 6813 if (Utilities.noString(value)) 6814 this.comment = null; 6815 else { 6816 if (this.comment == null) 6817 this.comment = new MarkdownType(); 6818 this.comment.setValue(value); 6819 } 6820 return this; 6821 } 6822 6823 /** 6824 * @return {@link #requirements} (This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 6825 */ 6826 public MarkdownType getRequirementsElement() { 6827 if (this.requirements == null) 6828 if (Configuration.errorOnAutoCreate()) 6829 throw new Error("Attempt to auto-create ElementDefinition.requirements"); 6830 else if (Configuration.doAutoCreate()) 6831 this.requirements = new MarkdownType(); // bb 6832 return this.requirements; 6833 } 6834 6835 public boolean hasRequirementsElement() { 6836 return this.requirements != null && !this.requirements.isEmpty(); 6837 } 6838 6839 public boolean hasRequirements() { 6840 return this.requirements != null && !this.requirements.isEmpty(); 6841 } 6842 6843 /** 6844 * @param value {@link #requirements} (This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 6845 */ 6846 public ElementDefinition setRequirementsElement(MarkdownType value) { 6847 this.requirements = value; 6848 return this; 6849 } 6850 6851 /** 6852 * @return This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 6853 */ 6854 public String getRequirements() { 6855 return this.requirements == null ? null : this.requirements.getValue(); 6856 } 6857 6858 /** 6859 * @param value This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 6860 */ 6861 public ElementDefinition setRequirements(String value) { 6862 if (Utilities.noString(value)) 6863 this.requirements = null; 6864 else { 6865 if (this.requirements == null) 6866 this.requirements = new MarkdownType(); 6867 this.requirements.setValue(value); 6868 } 6869 return this; 6870 } 6871 6872 /** 6873 * @return {@link #alias} (Identifies additional names by which this element might also be known.) 6874 */ 6875 public List<StringType> getAlias() { 6876 if (this.alias == null) 6877 this.alias = new ArrayList<StringType>(); 6878 return this.alias; 6879 } 6880 6881 /** 6882 * @return Returns a reference to <code>this</code> for easy method chaining 6883 */ 6884 public ElementDefinition setAlias(List<StringType> theAlias) { 6885 this.alias = theAlias; 6886 return this; 6887 } 6888 6889 public boolean hasAlias() { 6890 if (this.alias == null) 6891 return false; 6892 for (StringType item : this.alias) 6893 if (!item.isEmpty()) 6894 return true; 6895 return false; 6896 } 6897 6898 /** 6899 * @return {@link #alias} (Identifies additional names by which this element might also be known.) 6900 */ 6901 public StringType addAliasElement() {//2 6902 StringType t = new StringType(); 6903 if (this.alias == null) 6904 this.alias = new ArrayList<StringType>(); 6905 this.alias.add(t); 6906 return t; 6907 } 6908 6909 /** 6910 * @param value {@link #alias} (Identifies additional names by which this element might also be known.) 6911 */ 6912 public ElementDefinition addAlias(String value) { //1 6913 StringType t = new StringType(); 6914 t.setValue(value); 6915 if (this.alias == null) 6916 this.alias = new ArrayList<StringType>(); 6917 this.alias.add(t); 6918 return this; 6919 } 6920 6921 /** 6922 * @param value {@link #alias} (Identifies additional names by which this element might also be known.) 6923 */ 6924 public boolean hasAlias(String value) { 6925 if (this.alias == null) 6926 return false; 6927 for (StringType v : this.alias) 6928 if (v.getValue().equals(value)) // string 6929 return true; 6930 return false; 6931 } 6932 6933 /** 6934 * @return {@link #min} (The minimum number of times this element SHALL appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 6935 */ 6936 public UnsignedIntType getMinElement() { 6937 if (this.min == null) 6938 if (Configuration.errorOnAutoCreate()) 6939 throw new Error("Attempt to auto-create ElementDefinition.min"); 6940 else if (Configuration.doAutoCreate()) 6941 this.min = new UnsignedIntType(); // bb 6942 return this.min; 6943 } 6944 6945 public boolean hasMinElement() { 6946 return this.min != null && !this.min.isEmpty(); 6947 } 6948 6949 public boolean hasMin() { 6950 return this.min != null && !this.min.isEmpty(); 6951 } 6952 6953 /** 6954 * @param value {@link #min} (The minimum number of times this element SHALL appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 6955 */ 6956 public ElementDefinition setMinElement(UnsignedIntType value) { 6957 this.min = value; 6958 return this; 6959 } 6960 6961 /** 6962 * @return The minimum number of times this element SHALL appear in the instance. 6963 */ 6964 public int getMin() { 6965 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 6966 } 6967 6968 /** 6969 * @param value The minimum number of times this element SHALL appear in the instance. 6970 */ 6971 public ElementDefinition setMin(int value) { 6972 if (this.min == null) 6973 this.min = new UnsignedIntType(); 6974 this.min.setValue(value); 6975 return this; 6976 } 6977 6978 /** 6979 * @return {@link #max} (The maximum number of times this element is permitted to appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 6980 */ 6981 public StringType getMaxElement() { 6982 if (this.max == null) 6983 if (Configuration.errorOnAutoCreate()) 6984 throw new Error("Attempt to auto-create ElementDefinition.max"); 6985 else if (Configuration.doAutoCreate()) 6986 this.max = new StringType(); // bb 6987 return this.max; 6988 } 6989 6990 public boolean hasMaxElement() { 6991 return this.max != null && !this.max.isEmpty(); 6992 } 6993 6994 public boolean hasMax() { 6995 return this.max != null && !this.max.isEmpty(); 6996 } 6997 6998 /** 6999 * @param value {@link #max} (The maximum number of times this element is permitted to appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 7000 */ 7001 public ElementDefinition setMaxElement(StringType value) { 7002 this.max = value; 7003 return this; 7004 } 7005 7006 /** 7007 * @return The maximum number of times this element is permitted to appear in the instance. 7008 */ 7009 public String getMax() { 7010 return this.max == null ? null : this.max.getValue(); 7011 } 7012 7013 /** 7014 * @param value The maximum number of times this element is permitted to appear in the instance. 7015 */ 7016 public ElementDefinition setMax(String value) { 7017 if (Utilities.noString(value)) 7018 this.max = null; 7019 else { 7020 if (this.max == null) 7021 this.max = new StringType(); 7022 this.max.setValue(value); 7023 } 7024 return this; 7025 } 7026 7027 /** 7028 * @return {@link #base} (Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.) 7029 */ 7030 public ElementDefinitionBaseComponent getBase() { 7031 if (this.base == null) 7032 if (Configuration.errorOnAutoCreate()) 7033 throw new Error("Attempt to auto-create ElementDefinition.base"); 7034 else if (Configuration.doAutoCreate()) 7035 this.base = new ElementDefinitionBaseComponent(); // cc 7036 return this.base; 7037 } 7038 7039 public boolean hasBase() { 7040 return this.base != null && !this.base.isEmpty(); 7041 } 7042 7043 /** 7044 * @param value {@link #base} (Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.) 7045 */ 7046 public ElementDefinition setBase(ElementDefinitionBaseComponent value) { 7047 this.base = value; 7048 return this; 7049 } 7050 7051 /** 7052 * @return {@link #contentReference} (Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.). This is the underlying object with id, value and extensions. The accessor "getContentReference" gives direct access to the value 7053 */ 7054 public UriType getContentReferenceElement() { 7055 if (this.contentReference == null) 7056 if (Configuration.errorOnAutoCreate()) 7057 throw new Error("Attempt to auto-create ElementDefinition.contentReference"); 7058 else if (Configuration.doAutoCreate()) 7059 this.contentReference = new UriType(); // bb 7060 return this.contentReference; 7061 } 7062 7063 public boolean hasContentReferenceElement() { 7064 return this.contentReference != null && !this.contentReference.isEmpty(); 7065 } 7066 7067 public boolean hasContentReference() { 7068 return this.contentReference != null && !this.contentReference.isEmpty(); 7069 } 7070 7071 /** 7072 * @param value {@link #contentReference} (Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.). This is the underlying object with id, value and extensions. The accessor "getContentReference" gives direct access to the value 7073 */ 7074 public ElementDefinition setContentReferenceElement(UriType value) { 7075 this.contentReference = value; 7076 return this; 7077 } 7078 7079 /** 7080 * @return Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc. 7081 */ 7082 public String getContentReference() { 7083 return this.contentReference == null ? null : this.contentReference.getValue(); 7084 } 7085 7086 /** 7087 * @param value Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc. 7088 */ 7089 public ElementDefinition setContentReference(String value) { 7090 if (Utilities.noString(value)) 7091 this.contentReference = null; 7092 else { 7093 if (this.contentReference == null) 7094 this.contentReference = new UriType(); 7095 this.contentReference.setValue(value); 7096 } 7097 return this; 7098 } 7099 7100 /** 7101 * @return {@link #type} (The data type or resource that the value of this element is permitted to be.) 7102 */ 7103 public List<TypeRefComponent> getType() { 7104 if (this.type == null) 7105 this.type = new ArrayList<TypeRefComponent>(); 7106 return this.type; 7107 } 7108 7109 /** 7110 * @return Returns a reference to <code>this</code> for easy method chaining 7111 */ 7112 public ElementDefinition setType(List<TypeRefComponent> theType) { 7113 this.type = theType; 7114 return this; 7115 } 7116 7117 public boolean hasType() { 7118 if (this.type == null) 7119 return false; 7120 for (TypeRefComponent item : this.type) 7121 if (!item.isEmpty()) 7122 return true; 7123 return false; 7124 } 7125 7126 public TypeRefComponent addType() { //3 7127 TypeRefComponent t = new TypeRefComponent(); 7128 if (this.type == null) 7129 this.type = new ArrayList<TypeRefComponent>(); 7130 this.type.add(t); 7131 return t; 7132 } 7133 7134 public ElementDefinition addType(TypeRefComponent t) { //3 7135 if (t == null) 7136 return this; 7137 if (this.type == null) 7138 this.type = new ArrayList<TypeRefComponent>(); 7139 this.type.add(t); 7140 return this; 7141 } 7142 7143 /** 7144 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 7145 */ 7146 public TypeRefComponent getTypeFirstRep() { 7147 if (getType().isEmpty()) { 7148 addType(); 7149 } 7150 return getType().get(0); 7151 } 7152 7153 /** 7154 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7155 */ 7156 public DataType getDefaultValue() { 7157 return this.defaultValue; 7158 } 7159 7160 /** 7161 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7162 */ 7163 public Base64BinaryType getDefaultValueBase64BinaryType() throws FHIRException { 7164 if (this.defaultValue == null) 7165 this.defaultValue = new Base64BinaryType(); 7166 if (!(this.defaultValue instanceof Base64BinaryType)) 7167 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7168 return (Base64BinaryType) this.defaultValue; 7169 } 7170 7171 public boolean hasDefaultValueBase64BinaryType() { 7172 return this != null && this.defaultValue instanceof Base64BinaryType; 7173 } 7174 7175 /** 7176 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7177 */ 7178 public BooleanType getDefaultValueBooleanType() throws FHIRException { 7179 if (this.defaultValue == null) 7180 this.defaultValue = new BooleanType(); 7181 if (!(this.defaultValue instanceof BooleanType)) 7182 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7183 return (BooleanType) this.defaultValue; 7184 } 7185 7186 public boolean hasDefaultValueBooleanType() { 7187 return this != null && this.defaultValue instanceof BooleanType; 7188 } 7189 7190 /** 7191 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7192 */ 7193 public CanonicalType getDefaultValueCanonicalType() throws FHIRException { 7194 if (this.defaultValue == null) 7195 this.defaultValue = new CanonicalType(); 7196 if (!(this.defaultValue instanceof CanonicalType)) 7197 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7198 return (CanonicalType) this.defaultValue; 7199 } 7200 7201 public boolean hasDefaultValueCanonicalType() { 7202 return this != null && this.defaultValue instanceof CanonicalType; 7203 } 7204 7205 /** 7206 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7207 */ 7208 public CodeType getDefaultValueCodeType() throws FHIRException { 7209 if (this.defaultValue == null) 7210 this.defaultValue = new CodeType(); 7211 if (!(this.defaultValue instanceof CodeType)) 7212 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7213 return (CodeType) this.defaultValue; 7214 } 7215 7216 public boolean hasDefaultValueCodeType() { 7217 return this != null && this.defaultValue instanceof CodeType; 7218 } 7219 7220 /** 7221 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7222 */ 7223 public DateType getDefaultValueDateType() throws FHIRException { 7224 if (this.defaultValue == null) 7225 this.defaultValue = new DateType(); 7226 if (!(this.defaultValue instanceof DateType)) 7227 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7228 return (DateType) this.defaultValue; 7229 } 7230 7231 public boolean hasDefaultValueDateType() { 7232 return this != null && this.defaultValue instanceof DateType; 7233 } 7234 7235 /** 7236 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7237 */ 7238 public DateTimeType getDefaultValueDateTimeType() throws FHIRException { 7239 if (this.defaultValue == null) 7240 this.defaultValue = new DateTimeType(); 7241 if (!(this.defaultValue instanceof DateTimeType)) 7242 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7243 return (DateTimeType) this.defaultValue; 7244 } 7245 7246 public boolean hasDefaultValueDateTimeType() { 7247 return this != null && this.defaultValue instanceof DateTimeType; 7248 } 7249 7250 /** 7251 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7252 */ 7253 public DecimalType getDefaultValueDecimalType() throws FHIRException { 7254 if (this.defaultValue == null) 7255 this.defaultValue = new DecimalType(); 7256 if (!(this.defaultValue instanceof DecimalType)) 7257 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7258 return (DecimalType) this.defaultValue; 7259 } 7260 7261 public boolean hasDefaultValueDecimalType() { 7262 return this != null && this.defaultValue instanceof DecimalType; 7263 } 7264 7265 /** 7266 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7267 */ 7268 public IdType getDefaultValueIdType() throws FHIRException { 7269 if (this.defaultValue == null) 7270 this.defaultValue = new IdType(); 7271 if (!(this.defaultValue instanceof IdType)) 7272 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7273 return (IdType) this.defaultValue; 7274 } 7275 7276 public boolean hasDefaultValueIdType() { 7277 return this != null && this.defaultValue instanceof IdType; 7278 } 7279 7280 /** 7281 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7282 */ 7283 public InstantType getDefaultValueInstantType() throws FHIRException { 7284 if (this.defaultValue == null) 7285 this.defaultValue = new InstantType(); 7286 if (!(this.defaultValue instanceof InstantType)) 7287 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7288 return (InstantType) this.defaultValue; 7289 } 7290 7291 public boolean hasDefaultValueInstantType() { 7292 return this != null && this.defaultValue instanceof InstantType; 7293 } 7294 7295 /** 7296 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7297 */ 7298 public IntegerType getDefaultValueIntegerType() throws FHIRException { 7299 if (this.defaultValue == null) 7300 this.defaultValue = new IntegerType(); 7301 if (!(this.defaultValue instanceof IntegerType)) 7302 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7303 return (IntegerType) this.defaultValue; 7304 } 7305 7306 public boolean hasDefaultValueIntegerType() { 7307 return this != null && this.defaultValue instanceof IntegerType; 7308 } 7309 7310 /** 7311 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7312 */ 7313 public Integer64Type getDefaultValueInteger64Type() throws FHIRException { 7314 if (this.defaultValue == null) 7315 this.defaultValue = new Integer64Type(); 7316 if (!(this.defaultValue instanceof Integer64Type)) 7317 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7318 return (Integer64Type) this.defaultValue; 7319 } 7320 7321 public boolean hasDefaultValueInteger64Type() { 7322 return this != null && this.defaultValue instanceof Integer64Type; 7323 } 7324 7325 /** 7326 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7327 */ 7328 public MarkdownType getDefaultValueMarkdownType() throws FHIRException { 7329 if (this.defaultValue == null) 7330 this.defaultValue = new MarkdownType(); 7331 if (!(this.defaultValue instanceof MarkdownType)) 7332 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7333 return (MarkdownType) this.defaultValue; 7334 } 7335 7336 public boolean hasDefaultValueMarkdownType() { 7337 return this != null && this.defaultValue instanceof MarkdownType; 7338 } 7339 7340 /** 7341 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7342 */ 7343 public OidType getDefaultValueOidType() throws FHIRException { 7344 if (this.defaultValue == null) 7345 this.defaultValue = new OidType(); 7346 if (!(this.defaultValue instanceof OidType)) 7347 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7348 return (OidType) this.defaultValue; 7349 } 7350 7351 public boolean hasDefaultValueOidType() { 7352 return this != null && this.defaultValue instanceof OidType; 7353 } 7354 7355 /** 7356 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7357 */ 7358 public PositiveIntType getDefaultValuePositiveIntType() throws FHIRException { 7359 if (this.defaultValue == null) 7360 this.defaultValue = new PositiveIntType(); 7361 if (!(this.defaultValue instanceof PositiveIntType)) 7362 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7363 return (PositiveIntType) this.defaultValue; 7364 } 7365 7366 public boolean hasDefaultValuePositiveIntType() { 7367 return this != null && this.defaultValue instanceof PositiveIntType; 7368 } 7369 7370 /** 7371 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7372 */ 7373 public StringType getDefaultValueStringType() throws FHIRException { 7374 if (this.defaultValue == null) 7375 this.defaultValue = new StringType(); 7376 if (!(this.defaultValue instanceof StringType)) 7377 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7378 return (StringType) this.defaultValue; 7379 } 7380 7381 public boolean hasDefaultValueStringType() { 7382 return this != null && this.defaultValue instanceof StringType; 7383 } 7384 7385 /** 7386 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7387 */ 7388 public TimeType getDefaultValueTimeType() throws FHIRException { 7389 if (this.defaultValue == null) 7390 this.defaultValue = new TimeType(); 7391 if (!(this.defaultValue instanceof TimeType)) 7392 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7393 return (TimeType) this.defaultValue; 7394 } 7395 7396 public boolean hasDefaultValueTimeType() { 7397 return this != null && this.defaultValue instanceof TimeType; 7398 } 7399 7400 /** 7401 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7402 */ 7403 public UnsignedIntType getDefaultValueUnsignedIntType() throws FHIRException { 7404 if (this.defaultValue == null) 7405 this.defaultValue = new UnsignedIntType(); 7406 if (!(this.defaultValue instanceof UnsignedIntType)) 7407 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7408 return (UnsignedIntType) this.defaultValue; 7409 } 7410 7411 public boolean hasDefaultValueUnsignedIntType() { 7412 return this != null && this.defaultValue instanceof UnsignedIntType; 7413 } 7414 7415 /** 7416 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7417 */ 7418 public UriType getDefaultValueUriType() throws FHIRException { 7419 if (this.defaultValue == null) 7420 this.defaultValue = new UriType(); 7421 if (!(this.defaultValue instanceof UriType)) 7422 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7423 return (UriType) this.defaultValue; 7424 } 7425 7426 public boolean hasDefaultValueUriType() { 7427 return this != null && this.defaultValue instanceof UriType; 7428 } 7429 7430 /** 7431 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7432 */ 7433 public UrlType getDefaultValueUrlType() throws FHIRException { 7434 if (this.defaultValue == null) 7435 this.defaultValue = new UrlType(); 7436 if (!(this.defaultValue instanceof UrlType)) 7437 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7438 return (UrlType) this.defaultValue; 7439 } 7440 7441 public boolean hasDefaultValueUrlType() { 7442 return this != null && this.defaultValue instanceof UrlType; 7443 } 7444 7445 /** 7446 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7447 */ 7448 public UuidType getDefaultValueUuidType() throws FHIRException { 7449 if (this.defaultValue == null) 7450 this.defaultValue = new UuidType(); 7451 if (!(this.defaultValue instanceof UuidType)) 7452 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7453 return (UuidType) this.defaultValue; 7454 } 7455 7456 public boolean hasDefaultValueUuidType() { 7457 return this != null && this.defaultValue instanceof UuidType; 7458 } 7459 7460 /** 7461 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7462 */ 7463 public Address getDefaultValueAddress() throws FHIRException { 7464 if (this.defaultValue == null) 7465 this.defaultValue = new Address(); 7466 if (!(this.defaultValue instanceof Address)) 7467 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7468 return (Address) this.defaultValue; 7469 } 7470 7471 public boolean hasDefaultValueAddress() { 7472 return this != null && this.defaultValue instanceof Address; 7473 } 7474 7475 /** 7476 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7477 */ 7478 public Age getDefaultValueAge() throws FHIRException { 7479 if (this.defaultValue == null) 7480 this.defaultValue = new Age(); 7481 if (!(this.defaultValue instanceof Age)) 7482 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7483 return (Age) this.defaultValue; 7484 } 7485 7486 public boolean hasDefaultValueAge() { 7487 return this != null && this.defaultValue instanceof Age; 7488 } 7489 7490 /** 7491 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7492 */ 7493 public Annotation getDefaultValueAnnotation() throws FHIRException { 7494 if (this.defaultValue == null) 7495 this.defaultValue = new Annotation(); 7496 if (!(this.defaultValue instanceof Annotation)) 7497 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7498 return (Annotation) this.defaultValue; 7499 } 7500 7501 public boolean hasDefaultValueAnnotation() { 7502 return this != null && this.defaultValue instanceof Annotation; 7503 } 7504 7505 /** 7506 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7507 */ 7508 public Attachment getDefaultValueAttachment() throws FHIRException { 7509 if (this.defaultValue == null) 7510 this.defaultValue = new Attachment(); 7511 if (!(this.defaultValue instanceof Attachment)) 7512 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7513 return (Attachment) this.defaultValue; 7514 } 7515 7516 public boolean hasDefaultValueAttachment() { 7517 return this != null && this.defaultValue instanceof Attachment; 7518 } 7519 7520 /** 7521 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7522 */ 7523 public CodeableConcept getDefaultValueCodeableConcept() throws FHIRException { 7524 if (this.defaultValue == null) 7525 this.defaultValue = new CodeableConcept(); 7526 if (!(this.defaultValue instanceof CodeableConcept)) 7527 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7528 return (CodeableConcept) this.defaultValue; 7529 } 7530 7531 public boolean hasDefaultValueCodeableConcept() { 7532 return this != null && this.defaultValue instanceof CodeableConcept; 7533 } 7534 7535 /** 7536 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7537 */ 7538 public CodeableReference getDefaultValueCodeableReference() throws FHIRException { 7539 if (this.defaultValue == null) 7540 this.defaultValue = new CodeableReference(); 7541 if (!(this.defaultValue instanceof CodeableReference)) 7542 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7543 return (CodeableReference) this.defaultValue; 7544 } 7545 7546 public boolean hasDefaultValueCodeableReference() { 7547 return this != null && this.defaultValue instanceof CodeableReference; 7548 } 7549 7550 /** 7551 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7552 */ 7553 public Coding getDefaultValueCoding() throws FHIRException { 7554 if (this.defaultValue == null) 7555 this.defaultValue = new Coding(); 7556 if (!(this.defaultValue instanceof Coding)) 7557 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7558 return (Coding) this.defaultValue; 7559 } 7560 7561 public boolean hasDefaultValueCoding() { 7562 return this != null && this.defaultValue instanceof Coding; 7563 } 7564 7565 /** 7566 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7567 */ 7568 public ContactPoint getDefaultValueContactPoint() throws FHIRException { 7569 if (this.defaultValue == null) 7570 this.defaultValue = new ContactPoint(); 7571 if (!(this.defaultValue instanceof ContactPoint)) 7572 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7573 return (ContactPoint) this.defaultValue; 7574 } 7575 7576 public boolean hasDefaultValueContactPoint() { 7577 return this != null && this.defaultValue instanceof ContactPoint; 7578 } 7579 7580 /** 7581 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7582 */ 7583 public Count getDefaultValueCount() throws FHIRException { 7584 if (this.defaultValue == null) 7585 this.defaultValue = new Count(); 7586 if (!(this.defaultValue instanceof Count)) 7587 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7588 return (Count) this.defaultValue; 7589 } 7590 7591 public boolean hasDefaultValueCount() { 7592 return this != null && this.defaultValue instanceof Count; 7593 } 7594 7595 /** 7596 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7597 */ 7598 public Distance getDefaultValueDistance() throws FHIRException { 7599 if (this.defaultValue == null) 7600 this.defaultValue = new Distance(); 7601 if (!(this.defaultValue instanceof Distance)) 7602 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7603 return (Distance) this.defaultValue; 7604 } 7605 7606 public boolean hasDefaultValueDistance() { 7607 return this != null && this.defaultValue instanceof Distance; 7608 } 7609 7610 /** 7611 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7612 */ 7613 public Duration getDefaultValueDuration() throws FHIRException { 7614 if (this.defaultValue == null) 7615 this.defaultValue = new Duration(); 7616 if (!(this.defaultValue instanceof Duration)) 7617 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7618 return (Duration) this.defaultValue; 7619 } 7620 7621 public boolean hasDefaultValueDuration() { 7622 return this != null && this.defaultValue instanceof Duration; 7623 } 7624 7625 /** 7626 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7627 */ 7628 public HumanName getDefaultValueHumanName() throws FHIRException { 7629 if (this.defaultValue == null) 7630 this.defaultValue = new HumanName(); 7631 if (!(this.defaultValue instanceof HumanName)) 7632 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7633 return (HumanName) this.defaultValue; 7634 } 7635 7636 public boolean hasDefaultValueHumanName() { 7637 return this != null && this.defaultValue instanceof HumanName; 7638 } 7639 7640 /** 7641 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7642 */ 7643 public Identifier getDefaultValueIdentifier() throws FHIRException { 7644 if (this.defaultValue == null) 7645 this.defaultValue = new Identifier(); 7646 if (!(this.defaultValue instanceof Identifier)) 7647 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7648 return (Identifier) this.defaultValue; 7649 } 7650 7651 public boolean hasDefaultValueIdentifier() { 7652 return this != null && this.defaultValue instanceof Identifier; 7653 } 7654 7655 /** 7656 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7657 */ 7658 public Money getDefaultValueMoney() throws FHIRException { 7659 if (this.defaultValue == null) 7660 this.defaultValue = new Money(); 7661 if (!(this.defaultValue instanceof Money)) 7662 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7663 return (Money) this.defaultValue; 7664 } 7665 7666 public boolean hasDefaultValueMoney() { 7667 return this != null && this.defaultValue instanceof Money; 7668 } 7669 7670 /** 7671 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7672 */ 7673 public Period getDefaultValuePeriod() throws FHIRException { 7674 if (this.defaultValue == null) 7675 this.defaultValue = new Period(); 7676 if (!(this.defaultValue instanceof Period)) 7677 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7678 return (Period) this.defaultValue; 7679 } 7680 7681 public boolean hasDefaultValuePeriod() { 7682 return this != null && this.defaultValue instanceof Period; 7683 } 7684 7685 /** 7686 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7687 */ 7688 public Quantity getDefaultValueQuantity() throws FHIRException { 7689 if (this.defaultValue == null) 7690 this.defaultValue = new Quantity(); 7691 if (!(this.defaultValue instanceof Quantity)) 7692 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7693 return (Quantity) this.defaultValue; 7694 } 7695 7696 public boolean hasDefaultValueQuantity() { 7697 return this != null && this.defaultValue instanceof Quantity; 7698 } 7699 7700 /** 7701 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7702 */ 7703 public Range getDefaultValueRange() throws FHIRException { 7704 if (this.defaultValue == null) 7705 this.defaultValue = new Range(); 7706 if (!(this.defaultValue instanceof Range)) 7707 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7708 return (Range) this.defaultValue; 7709 } 7710 7711 public boolean hasDefaultValueRange() { 7712 return this != null && this.defaultValue instanceof Range; 7713 } 7714 7715 /** 7716 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7717 */ 7718 public Ratio getDefaultValueRatio() throws FHIRException { 7719 if (this.defaultValue == null) 7720 this.defaultValue = new Ratio(); 7721 if (!(this.defaultValue instanceof Ratio)) 7722 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7723 return (Ratio) this.defaultValue; 7724 } 7725 7726 public boolean hasDefaultValueRatio() { 7727 return this != null && this.defaultValue instanceof Ratio; 7728 } 7729 7730 /** 7731 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7732 */ 7733 public RatioRange getDefaultValueRatioRange() throws FHIRException { 7734 if (this.defaultValue == null) 7735 this.defaultValue = new RatioRange(); 7736 if (!(this.defaultValue instanceof RatioRange)) 7737 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7738 return (RatioRange) this.defaultValue; 7739 } 7740 7741 public boolean hasDefaultValueRatioRange() { 7742 return this != null && this.defaultValue instanceof RatioRange; 7743 } 7744 7745 /** 7746 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7747 */ 7748 public Reference getDefaultValueReference() throws FHIRException { 7749 if (this.defaultValue == null) 7750 this.defaultValue = new Reference(); 7751 if (!(this.defaultValue instanceof Reference)) 7752 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7753 return (Reference) this.defaultValue; 7754 } 7755 7756 public boolean hasDefaultValueReference() { 7757 return this != null && this.defaultValue instanceof Reference; 7758 } 7759 7760 /** 7761 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7762 */ 7763 public SampledData getDefaultValueSampledData() throws FHIRException { 7764 if (this.defaultValue == null) 7765 this.defaultValue = new SampledData(); 7766 if (!(this.defaultValue instanceof SampledData)) 7767 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7768 return (SampledData) this.defaultValue; 7769 } 7770 7771 public boolean hasDefaultValueSampledData() { 7772 return this != null && this.defaultValue instanceof SampledData; 7773 } 7774 7775 /** 7776 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7777 */ 7778 public Signature getDefaultValueSignature() throws FHIRException { 7779 if (this.defaultValue == null) 7780 this.defaultValue = new Signature(); 7781 if (!(this.defaultValue instanceof Signature)) 7782 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7783 return (Signature) this.defaultValue; 7784 } 7785 7786 public boolean hasDefaultValueSignature() { 7787 return this != null && this.defaultValue instanceof Signature; 7788 } 7789 7790 /** 7791 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7792 */ 7793 public Timing getDefaultValueTiming() throws FHIRException { 7794 if (this.defaultValue == null) 7795 this.defaultValue = new Timing(); 7796 if (!(this.defaultValue instanceof Timing)) 7797 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7798 return (Timing) this.defaultValue; 7799 } 7800 7801 public boolean hasDefaultValueTiming() { 7802 return this != null && this.defaultValue instanceof Timing; 7803 } 7804 7805 /** 7806 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7807 */ 7808 public ContactDetail getDefaultValueContactDetail() throws FHIRException { 7809 if (this.defaultValue == null) 7810 this.defaultValue = new ContactDetail(); 7811 if (!(this.defaultValue instanceof ContactDetail)) 7812 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7813 return (ContactDetail) this.defaultValue; 7814 } 7815 7816 public boolean hasDefaultValueContactDetail() { 7817 return this != null && this.defaultValue instanceof ContactDetail; 7818 } 7819 7820 /** 7821 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7822 */ 7823 public DataRequirement getDefaultValueDataRequirement() throws FHIRException { 7824 if (this.defaultValue == null) 7825 this.defaultValue = new DataRequirement(); 7826 if (!(this.defaultValue instanceof DataRequirement)) 7827 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7828 return (DataRequirement) this.defaultValue; 7829 } 7830 7831 public boolean hasDefaultValueDataRequirement() { 7832 return this != null && this.defaultValue instanceof DataRequirement; 7833 } 7834 7835 /** 7836 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7837 */ 7838 public Expression getDefaultValueExpression() throws FHIRException { 7839 if (this.defaultValue == null) 7840 this.defaultValue = new Expression(); 7841 if (!(this.defaultValue instanceof Expression)) 7842 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7843 return (Expression) this.defaultValue; 7844 } 7845 7846 public boolean hasDefaultValueExpression() { 7847 return this != null && this.defaultValue instanceof Expression; 7848 } 7849 7850 /** 7851 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7852 */ 7853 public ParameterDefinition getDefaultValueParameterDefinition() throws FHIRException { 7854 if (this.defaultValue == null) 7855 this.defaultValue = new ParameterDefinition(); 7856 if (!(this.defaultValue instanceof ParameterDefinition)) 7857 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7858 return (ParameterDefinition) this.defaultValue; 7859 } 7860 7861 public boolean hasDefaultValueParameterDefinition() { 7862 return this != null && this.defaultValue instanceof ParameterDefinition; 7863 } 7864 7865 /** 7866 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7867 */ 7868 public RelatedArtifact getDefaultValueRelatedArtifact() throws FHIRException { 7869 if (this.defaultValue == null) 7870 this.defaultValue = new RelatedArtifact(); 7871 if (!(this.defaultValue instanceof RelatedArtifact)) 7872 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7873 return (RelatedArtifact) this.defaultValue; 7874 } 7875 7876 public boolean hasDefaultValueRelatedArtifact() { 7877 return this != null && this.defaultValue instanceof RelatedArtifact; 7878 } 7879 7880 /** 7881 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7882 */ 7883 public TriggerDefinition getDefaultValueTriggerDefinition() throws FHIRException { 7884 if (this.defaultValue == null) 7885 this.defaultValue = new TriggerDefinition(); 7886 if (!(this.defaultValue instanceof TriggerDefinition)) 7887 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7888 return (TriggerDefinition) this.defaultValue; 7889 } 7890 7891 public boolean hasDefaultValueTriggerDefinition() { 7892 return this != null && this.defaultValue instanceof TriggerDefinition; 7893 } 7894 7895 /** 7896 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7897 */ 7898 public UsageContext getDefaultValueUsageContext() throws FHIRException { 7899 if (this.defaultValue == null) 7900 this.defaultValue = new UsageContext(); 7901 if (!(this.defaultValue instanceof UsageContext)) 7902 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7903 return (UsageContext) this.defaultValue; 7904 } 7905 7906 public boolean hasDefaultValueUsageContext() { 7907 return this != null && this.defaultValue instanceof UsageContext; 7908 } 7909 7910 /** 7911 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7912 */ 7913 public Availability getDefaultValueAvailability() throws FHIRException { 7914 if (this.defaultValue == null) 7915 this.defaultValue = new Availability(); 7916 if (!(this.defaultValue instanceof Availability)) 7917 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7918 return (Availability) this.defaultValue; 7919 } 7920 7921 public boolean hasDefaultValueAvailability() { 7922 return this != null && this.defaultValue instanceof Availability; 7923 } 7924 7925 /** 7926 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7927 */ 7928 public ExtendedContactDetail getDefaultValueExtendedContactDetail() throws FHIRException { 7929 if (this.defaultValue == null) 7930 this.defaultValue = new ExtendedContactDetail(); 7931 if (!(this.defaultValue instanceof ExtendedContactDetail)) 7932 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7933 return (ExtendedContactDetail) this.defaultValue; 7934 } 7935 7936 public boolean hasDefaultValueExtendedContactDetail() { 7937 return this != null && this.defaultValue instanceof ExtendedContactDetail; 7938 } 7939 7940 /** 7941 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7942 */ 7943 public Dosage getDefaultValueDosage() throws FHIRException { 7944 if (this.defaultValue == null) 7945 this.defaultValue = new Dosage(); 7946 if (!(this.defaultValue instanceof Dosage)) 7947 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7948 return (Dosage) this.defaultValue; 7949 } 7950 7951 public boolean hasDefaultValueDosage() { 7952 return this != null && this.defaultValue instanceof Dosage; 7953 } 7954 7955 /** 7956 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7957 */ 7958 public Meta getDefaultValueMeta() throws FHIRException { 7959 if (this.defaultValue == null) 7960 this.defaultValue = new Meta(); 7961 if (!(this.defaultValue instanceof Meta)) 7962 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7963 return (Meta) this.defaultValue; 7964 } 7965 7966 public boolean hasDefaultValueMeta() { 7967 return this != null && this.defaultValue instanceof Meta; 7968 } 7969 7970 public boolean hasDefaultValue() { 7971 return this.defaultValue != null && !this.defaultValue.isEmpty(); 7972 } 7973 7974 /** 7975 * @param value {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7976 */ 7977 public ElementDefinition setDefaultValue(DataType value) { 7978 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 7979 throw new FHIRException("Not the right type for ElementDefinition.defaultValue[x]: "+value.fhirType()); 7980 this.defaultValue = value; 7981 return this; 7982 } 7983 7984 /** 7985 * @return {@link #meaningWhenMissing} (The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').). This is the underlying object with id, value and extensions. The accessor "getMeaningWhenMissing" gives direct access to the value 7986 */ 7987 public MarkdownType getMeaningWhenMissingElement() { 7988 if (this.meaningWhenMissing == null) 7989 if (Configuration.errorOnAutoCreate()) 7990 throw new Error("Attempt to auto-create ElementDefinition.meaningWhenMissing"); 7991 else if (Configuration.doAutoCreate()) 7992 this.meaningWhenMissing = new MarkdownType(); // bb 7993 return this.meaningWhenMissing; 7994 } 7995 7996 public boolean hasMeaningWhenMissingElement() { 7997 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 7998 } 7999 8000 public boolean hasMeaningWhenMissing() { 8001 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 8002 } 8003 8004 /** 8005 * @param value {@link #meaningWhenMissing} (The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').). This is the underlying object with id, value and extensions. The accessor "getMeaningWhenMissing" gives direct access to the value 8006 */ 8007 public ElementDefinition setMeaningWhenMissingElement(MarkdownType value) { 8008 this.meaningWhenMissing = value; 8009 return this; 8010 } 8011 8012 /** 8013 * @return The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'). 8014 */ 8015 public String getMeaningWhenMissing() { 8016 return this.meaningWhenMissing == null ? null : this.meaningWhenMissing.getValue(); 8017 } 8018 8019 /** 8020 * @param value The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'). 8021 */ 8022 public ElementDefinition setMeaningWhenMissing(String value) { 8023 if (Utilities.noString(value)) 8024 this.meaningWhenMissing = null; 8025 else { 8026 if (this.meaningWhenMissing == null) 8027 this.meaningWhenMissing = new MarkdownType(); 8028 this.meaningWhenMissing.setValue(value); 8029 } 8030 return this; 8031 } 8032 8033 /** 8034 * @return {@link #orderMeaning} (If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.). This is the underlying object with id, value and extensions. The accessor "getOrderMeaning" gives direct access to the value 8035 */ 8036 public StringType getOrderMeaningElement() { 8037 if (this.orderMeaning == null) 8038 if (Configuration.errorOnAutoCreate()) 8039 throw new Error("Attempt to auto-create ElementDefinition.orderMeaning"); 8040 else if (Configuration.doAutoCreate()) 8041 this.orderMeaning = new StringType(); // bb 8042 return this.orderMeaning; 8043 } 8044 8045 public boolean hasOrderMeaningElement() { 8046 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 8047 } 8048 8049 public boolean hasOrderMeaning() { 8050 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 8051 } 8052 8053 /** 8054 * @param value {@link #orderMeaning} (If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.). This is the underlying object with id, value and extensions. The accessor "getOrderMeaning" gives direct access to the value 8055 */ 8056 public ElementDefinition setOrderMeaningElement(StringType value) { 8057 this.orderMeaning = value; 8058 return this; 8059 } 8060 8061 /** 8062 * @return If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 8063 */ 8064 public String getOrderMeaning() { 8065 return this.orderMeaning == null ? null : this.orderMeaning.getValue(); 8066 } 8067 8068 /** 8069 * @param value If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 8070 */ 8071 public ElementDefinition setOrderMeaning(String value) { 8072 if (Utilities.noString(value)) 8073 this.orderMeaning = null; 8074 else { 8075 if (this.orderMeaning == null) 8076 this.orderMeaning = new StringType(); 8077 this.orderMeaning.setValue(value); 8078 } 8079 return this; 8080 } 8081 8082 /** 8083 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8084 */ 8085 public DataType getFixed() { 8086 return this.fixed; 8087 } 8088 8089 /** 8090 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8091 */ 8092 public Base64BinaryType getFixedBase64BinaryType() throws FHIRException { 8093 if (this.fixed == null) 8094 this.fixed = new Base64BinaryType(); 8095 if (!(this.fixed instanceof Base64BinaryType)) 8096 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8097 return (Base64BinaryType) this.fixed; 8098 } 8099 8100 public boolean hasFixedBase64BinaryType() { 8101 return this != null && this.fixed instanceof Base64BinaryType; 8102 } 8103 8104 /** 8105 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8106 */ 8107 public BooleanType getFixedBooleanType() throws FHIRException { 8108 if (this.fixed == null) 8109 this.fixed = new BooleanType(); 8110 if (!(this.fixed instanceof BooleanType)) 8111 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8112 return (BooleanType) this.fixed; 8113 } 8114 8115 public boolean hasFixedBooleanType() { 8116 return this != null && this.fixed instanceof BooleanType; 8117 } 8118 8119 /** 8120 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8121 */ 8122 public CanonicalType getFixedCanonicalType() throws FHIRException { 8123 if (this.fixed == null) 8124 this.fixed = new CanonicalType(); 8125 if (!(this.fixed instanceof CanonicalType)) 8126 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8127 return (CanonicalType) this.fixed; 8128 } 8129 8130 public boolean hasFixedCanonicalType() { 8131 return this != null && this.fixed instanceof CanonicalType; 8132 } 8133 8134 /** 8135 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8136 */ 8137 public CodeType getFixedCodeType() throws FHIRException { 8138 if (this.fixed == null) 8139 this.fixed = new CodeType(); 8140 if (!(this.fixed instanceof CodeType)) 8141 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8142 return (CodeType) this.fixed; 8143 } 8144 8145 public boolean hasFixedCodeType() { 8146 return this != null && this.fixed instanceof CodeType; 8147 } 8148 8149 /** 8150 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8151 */ 8152 public DateType getFixedDateType() throws FHIRException { 8153 if (this.fixed == null) 8154 this.fixed = new DateType(); 8155 if (!(this.fixed instanceof DateType)) 8156 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8157 return (DateType) this.fixed; 8158 } 8159 8160 public boolean hasFixedDateType() { 8161 return this != null && this.fixed instanceof DateType; 8162 } 8163 8164 /** 8165 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8166 */ 8167 public DateTimeType getFixedDateTimeType() throws FHIRException { 8168 if (this.fixed == null) 8169 this.fixed = new DateTimeType(); 8170 if (!(this.fixed instanceof DateTimeType)) 8171 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8172 return (DateTimeType) this.fixed; 8173 } 8174 8175 public boolean hasFixedDateTimeType() { 8176 return this != null && this.fixed instanceof DateTimeType; 8177 } 8178 8179 /** 8180 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8181 */ 8182 public DecimalType getFixedDecimalType() throws FHIRException { 8183 if (this.fixed == null) 8184 this.fixed = new DecimalType(); 8185 if (!(this.fixed instanceof DecimalType)) 8186 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8187 return (DecimalType) this.fixed; 8188 } 8189 8190 public boolean hasFixedDecimalType() { 8191 return this != null && this.fixed instanceof DecimalType; 8192 } 8193 8194 /** 8195 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8196 */ 8197 public IdType getFixedIdType() throws FHIRException { 8198 if (this.fixed == null) 8199 this.fixed = new IdType(); 8200 if (!(this.fixed instanceof IdType)) 8201 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8202 return (IdType) this.fixed; 8203 } 8204 8205 public boolean hasFixedIdType() { 8206 return this != null && this.fixed instanceof IdType; 8207 } 8208 8209 /** 8210 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8211 */ 8212 public InstantType getFixedInstantType() throws FHIRException { 8213 if (this.fixed == null) 8214 this.fixed = new InstantType(); 8215 if (!(this.fixed instanceof InstantType)) 8216 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8217 return (InstantType) this.fixed; 8218 } 8219 8220 public boolean hasFixedInstantType() { 8221 return this != null && this.fixed instanceof InstantType; 8222 } 8223 8224 /** 8225 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8226 */ 8227 public IntegerType getFixedIntegerType() throws FHIRException { 8228 if (this.fixed == null) 8229 this.fixed = new IntegerType(); 8230 if (!(this.fixed instanceof IntegerType)) 8231 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8232 return (IntegerType) this.fixed; 8233 } 8234 8235 public boolean hasFixedIntegerType() { 8236 return this != null && this.fixed instanceof IntegerType; 8237 } 8238 8239 /** 8240 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8241 */ 8242 public Integer64Type getFixedInteger64Type() throws FHIRException { 8243 if (this.fixed == null) 8244 this.fixed = new Integer64Type(); 8245 if (!(this.fixed instanceof Integer64Type)) 8246 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8247 return (Integer64Type) this.fixed; 8248 } 8249 8250 public boolean hasFixedInteger64Type() { 8251 return this != null && this.fixed instanceof Integer64Type; 8252 } 8253 8254 /** 8255 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8256 */ 8257 public MarkdownType getFixedMarkdownType() throws FHIRException { 8258 if (this.fixed == null) 8259 this.fixed = new MarkdownType(); 8260 if (!(this.fixed instanceof MarkdownType)) 8261 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8262 return (MarkdownType) this.fixed; 8263 } 8264 8265 public boolean hasFixedMarkdownType() { 8266 return this != null && this.fixed instanceof MarkdownType; 8267 } 8268 8269 /** 8270 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8271 */ 8272 public OidType getFixedOidType() throws FHIRException { 8273 if (this.fixed == null) 8274 this.fixed = new OidType(); 8275 if (!(this.fixed instanceof OidType)) 8276 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8277 return (OidType) this.fixed; 8278 } 8279 8280 public boolean hasFixedOidType() { 8281 return this != null && this.fixed instanceof OidType; 8282 } 8283 8284 /** 8285 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8286 */ 8287 public PositiveIntType getFixedPositiveIntType() throws FHIRException { 8288 if (this.fixed == null) 8289 this.fixed = new PositiveIntType(); 8290 if (!(this.fixed instanceof PositiveIntType)) 8291 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8292 return (PositiveIntType) this.fixed; 8293 } 8294 8295 public boolean hasFixedPositiveIntType() { 8296 return this != null && this.fixed instanceof PositiveIntType; 8297 } 8298 8299 /** 8300 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8301 */ 8302 public StringType getFixedStringType() throws FHIRException { 8303 if (this.fixed == null) 8304 this.fixed = new StringType(); 8305 if (!(this.fixed instanceof StringType)) 8306 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8307 return (StringType) this.fixed; 8308 } 8309 8310 public boolean hasFixedStringType() { 8311 return this != null && this.fixed instanceof StringType; 8312 } 8313 8314 /** 8315 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8316 */ 8317 public TimeType getFixedTimeType() throws FHIRException { 8318 if (this.fixed == null) 8319 this.fixed = new TimeType(); 8320 if (!(this.fixed instanceof TimeType)) 8321 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8322 return (TimeType) this.fixed; 8323 } 8324 8325 public boolean hasFixedTimeType() { 8326 return this != null && this.fixed instanceof TimeType; 8327 } 8328 8329 /** 8330 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8331 */ 8332 public UnsignedIntType getFixedUnsignedIntType() throws FHIRException { 8333 if (this.fixed == null) 8334 this.fixed = new UnsignedIntType(); 8335 if (!(this.fixed instanceof UnsignedIntType)) 8336 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8337 return (UnsignedIntType) this.fixed; 8338 } 8339 8340 public boolean hasFixedUnsignedIntType() { 8341 return this != null && this.fixed instanceof UnsignedIntType; 8342 } 8343 8344 /** 8345 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8346 */ 8347 public UriType getFixedUriType() throws FHIRException { 8348 if (this.fixed == null) 8349 this.fixed = new UriType(); 8350 if (!(this.fixed instanceof UriType)) 8351 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8352 return (UriType) this.fixed; 8353 } 8354 8355 public boolean hasFixedUriType() { 8356 return this != null && this.fixed instanceof UriType; 8357 } 8358 8359 /** 8360 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8361 */ 8362 public UrlType getFixedUrlType() throws FHIRException { 8363 if (this.fixed == null) 8364 this.fixed = new UrlType(); 8365 if (!(this.fixed instanceof UrlType)) 8366 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8367 return (UrlType) this.fixed; 8368 } 8369 8370 public boolean hasFixedUrlType() { 8371 return this != null && this.fixed instanceof UrlType; 8372 } 8373 8374 /** 8375 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8376 */ 8377 public UuidType getFixedUuidType() throws FHIRException { 8378 if (this.fixed == null) 8379 this.fixed = new UuidType(); 8380 if (!(this.fixed instanceof UuidType)) 8381 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8382 return (UuidType) this.fixed; 8383 } 8384 8385 public boolean hasFixedUuidType() { 8386 return this != null && this.fixed instanceof UuidType; 8387 } 8388 8389 /** 8390 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8391 */ 8392 public Address getFixedAddress() throws FHIRException { 8393 if (this.fixed == null) 8394 this.fixed = new Address(); 8395 if (!(this.fixed instanceof Address)) 8396 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8397 return (Address) this.fixed; 8398 } 8399 8400 public boolean hasFixedAddress() { 8401 return this != null && this.fixed instanceof Address; 8402 } 8403 8404 /** 8405 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8406 */ 8407 public Age getFixedAge() throws FHIRException { 8408 if (this.fixed == null) 8409 this.fixed = new Age(); 8410 if (!(this.fixed instanceof Age)) 8411 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8412 return (Age) this.fixed; 8413 } 8414 8415 public boolean hasFixedAge() { 8416 return this != null && this.fixed instanceof Age; 8417 } 8418 8419 /** 8420 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8421 */ 8422 public Annotation getFixedAnnotation() throws FHIRException { 8423 if (this.fixed == null) 8424 this.fixed = new Annotation(); 8425 if (!(this.fixed instanceof Annotation)) 8426 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8427 return (Annotation) this.fixed; 8428 } 8429 8430 public boolean hasFixedAnnotation() { 8431 return this != null && this.fixed instanceof Annotation; 8432 } 8433 8434 /** 8435 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8436 */ 8437 public Attachment getFixedAttachment() throws FHIRException { 8438 if (this.fixed == null) 8439 this.fixed = new Attachment(); 8440 if (!(this.fixed instanceof Attachment)) 8441 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8442 return (Attachment) this.fixed; 8443 } 8444 8445 public boolean hasFixedAttachment() { 8446 return this != null && this.fixed instanceof Attachment; 8447 } 8448 8449 /** 8450 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8451 */ 8452 public CodeableConcept getFixedCodeableConcept() throws FHIRException { 8453 if (this.fixed == null) 8454 this.fixed = new CodeableConcept(); 8455 if (!(this.fixed instanceof CodeableConcept)) 8456 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8457 return (CodeableConcept) this.fixed; 8458 } 8459 8460 public boolean hasFixedCodeableConcept() { 8461 return this != null && this.fixed instanceof CodeableConcept; 8462 } 8463 8464 /** 8465 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8466 */ 8467 public CodeableReference getFixedCodeableReference() throws FHIRException { 8468 if (this.fixed == null) 8469 this.fixed = new CodeableReference(); 8470 if (!(this.fixed instanceof CodeableReference)) 8471 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8472 return (CodeableReference) this.fixed; 8473 } 8474 8475 public boolean hasFixedCodeableReference() { 8476 return this != null && this.fixed instanceof CodeableReference; 8477 } 8478 8479 /** 8480 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8481 */ 8482 public Coding getFixedCoding() throws FHIRException { 8483 if (this.fixed == null) 8484 this.fixed = new Coding(); 8485 if (!(this.fixed instanceof Coding)) 8486 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8487 return (Coding) this.fixed; 8488 } 8489 8490 public boolean hasFixedCoding() { 8491 return this != null && this.fixed instanceof Coding; 8492 } 8493 8494 /** 8495 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8496 */ 8497 public ContactPoint getFixedContactPoint() throws FHIRException { 8498 if (this.fixed == null) 8499 this.fixed = new ContactPoint(); 8500 if (!(this.fixed instanceof ContactPoint)) 8501 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8502 return (ContactPoint) this.fixed; 8503 } 8504 8505 public boolean hasFixedContactPoint() { 8506 return this != null && this.fixed instanceof ContactPoint; 8507 } 8508 8509 /** 8510 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8511 */ 8512 public Count getFixedCount() throws FHIRException { 8513 if (this.fixed == null) 8514 this.fixed = new Count(); 8515 if (!(this.fixed instanceof Count)) 8516 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8517 return (Count) this.fixed; 8518 } 8519 8520 public boolean hasFixedCount() { 8521 return this != null && this.fixed instanceof Count; 8522 } 8523 8524 /** 8525 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8526 */ 8527 public Distance getFixedDistance() throws FHIRException { 8528 if (this.fixed == null) 8529 this.fixed = new Distance(); 8530 if (!(this.fixed instanceof Distance)) 8531 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8532 return (Distance) this.fixed; 8533 } 8534 8535 public boolean hasFixedDistance() { 8536 return this != null && this.fixed instanceof Distance; 8537 } 8538 8539 /** 8540 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8541 */ 8542 public Duration getFixedDuration() throws FHIRException { 8543 if (this.fixed == null) 8544 this.fixed = new Duration(); 8545 if (!(this.fixed instanceof Duration)) 8546 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8547 return (Duration) this.fixed; 8548 } 8549 8550 public boolean hasFixedDuration() { 8551 return this != null && this.fixed instanceof Duration; 8552 } 8553 8554 /** 8555 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8556 */ 8557 public HumanName getFixedHumanName() throws FHIRException { 8558 if (this.fixed == null) 8559 this.fixed = new HumanName(); 8560 if (!(this.fixed instanceof HumanName)) 8561 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8562 return (HumanName) this.fixed; 8563 } 8564 8565 public boolean hasFixedHumanName() { 8566 return this != null && this.fixed instanceof HumanName; 8567 } 8568 8569 /** 8570 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8571 */ 8572 public Identifier getFixedIdentifier() throws FHIRException { 8573 if (this.fixed == null) 8574 this.fixed = new Identifier(); 8575 if (!(this.fixed instanceof Identifier)) 8576 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8577 return (Identifier) this.fixed; 8578 } 8579 8580 public boolean hasFixedIdentifier() { 8581 return this != null && this.fixed instanceof Identifier; 8582 } 8583 8584 /** 8585 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8586 */ 8587 public Money getFixedMoney() throws FHIRException { 8588 if (this.fixed == null) 8589 this.fixed = new Money(); 8590 if (!(this.fixed instanceof Money)) 8591 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8592 return (Money) this.fixed; 8593 } 8594 8595 public boolean hasFixedMoney() { 8596 return this != null && this.fixed instanceof Money; 8597 } 8598 8599 /** 8600 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8601 */ 8602 public Period getFixedPeriod() throws FHIRException { 8603 if (this.fixed == null) 8604 this.fixed = new Period(); 8605 if (!(this.fixed instanceof Period)) 8606 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8607 return (Period) this.fixed; 8608 } 8609 8610 public boolean hasFixedPeriod() { 8611 return this != null && this.fixed instanceof Period; 8612 } 8613 8614 /** 8615 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8616 */ 8617 public Quantity getFixedQuantity() throws FHIRException { 8618 if (this.fixed == null) 8619 this.fixed = new Quantity(); 8620 if (!(this.fixed instanceof Quantity)) 8621 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8622 return (Quantity) this.fixed; 8623 } 8624 8625 public boolean hasFixedQuantity() { 8626 return this != null && this.fixed instanceof Quantity; 8627 } 8628 8629 /** 8630 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8631 */ 8632 public Range getFixedRange() throws FHIRException { 8633 if (this.fixed == null) 8634 this.fixed = new Range(); 8635 if (!(this.fixed instanceof Range)) 8636 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8637 return (Range) this.fixed; 8638 } 8639 8640 public boolean hasFixedRange() { 8641 return this != null && this.fixed instanceof Range; 8642 } 8643 8644 /** 8645 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8646 */ 8647 public Ratio getFixedRatio() throws FHIRException { 8648 if (this.fixed == null) 8649 this.fixed = new Ratio(); 8650 if (!(this.fixed instanceof Ratio)) 8651 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8652 return (Ratio) this.fixed; 8653 } 8654 8655 public boolean hasFixedRatio() { 8656 return this != null && this.fixed instanceof Ratio; 8657 } 8658 8659 /** 8660 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8661 */ 8662 public RatioRange getFixedRatioRange() throws FHIRException { 8663 if (this.fixed == null) 8664 this.fixed = new RatioRange(); 8665 if (!(this.fixed instanceof RatioRange)) 8666 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8667 return (RatioRange) this.fixed; 8668 } 8669 8670 public boolean hasFixedRatioRange() { 8671 return this != null && this.fixed instanceof RatioRange; 8672 } 8673 8674 /** 8675 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8676 */ 8677 public Reference getFixedReference() throws FHIRException { 8678 if (this.fixed == null) 8679 this.fixed = new Reference(); 8680 if (!(this.fixed instanceof Reference)) 8681 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8682 return (Reference) this.fixed; 8683 } 8684 8685 public boolean hasFixedReference() { 8686 return this != null && this.fixed instanceof Reference; 8687 } 8688 8689 /** 8690 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8691 */ 8692 public SampledData getFixedSampledData() throws FHIRException { 8693 if (this.fixed == null) 8694 this.fixed = new SampledData(); 8695 if (!(this.fixed instanceof SampledData)) 8696 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8697 return (SampledData) this.fixed; 8698 } 8699 8700 public boolean hasFixedSampledData() { 8701 return this != null && this.fixed instanceof SampledData; 8702 } 8703 8704 /** 8705 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8706 */ 8707 public Signature getFixedSignature() throws FHIRException { 8708 if (this.fixed == null) 8709 this.fixed = new Signature(); 8710 if (!(this.fixed instanceof Signature)) 8711 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8712 return (Signature) this.fixed; 8713 } 8714 8715 public boolean hasFixedSignature() { 8716 return this != null && this.fixed instanceof Signature; 8717 } 8718 8719 /** 8720 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8721 */ 8722 public Timing getFixedTiming() throws FHIRException { 8723 if (this.fixed == null) 8724 this.fixed = new Timing(); 8725 if (!(this.fixed instanceof Timing)) 8726 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8727 return (Timing) this.fixed; 8728 } 8729 8730 public boolean hasFixedTiming() { 8731 return this != null && this.fixed instanceof Timing; 8732 } 8733 8734 /** 8735 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8736 */ 8737 public ContactDetail getFixedContactDetail() throws FHIRException { 8738 if (this.fixed == null) 8739 this.fixed = new ContactDetail(); 8740 if (!(this.fixed instanceof ContactDetail)) 8741 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8742 return (ContactDetail) this.fixed; 8743 } 8744 8745 public boolean hasFixedContactDetail() { 8746 return this != null && this.fixed instanceof ContactDetail; 8747 } 8748 8749 /** 8750 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8751 */ 8752 public DataRequirement getFixedDataRequirement() throws FHIRException { 8753 if (this.fixed == null) 8754 this.fixed = new DataRequirement(); 8755 if (!(this.fixed instanceof DataRequirement)) 8756 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8757 return (DataRequirement) this.fixed; 8758 } 8759 8760 public boolean hasFixedDataRequirement() { 8761 return this != null && this.fixed instanceof DataRequirement; 8762 } 8763 8764 /** 8765 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8766 */ 8767 public Expression getFixedExpression() throws FHIRException { 8768 if (this.fixed == null) 8769 this.fixed = new Expression(); 8770 if (!(this.fixed instanceof Expression)) 8771 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8772 return (Expression) this.fixed; 8773 } 8774 8775 public boolean hasFixedExpression() { 8776 return this != null && this.fixed instanceof Expression; 8777 } 8778 8779 /** 8780 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8781 */ 8782 public ParameterDefinition getFixedParameterDefinition() throws FHIRException { 8783 if (this.fixed == null) 8784 this.fixed = new ParameterDefinition(); 8785 if (!(this.fixed instanceof ParameterDefinition)) 8786 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8787 return (ParameterDefinition) this.fixed; 8788 } 8789 8790 public boolean hasFixedParameterDefinition() { 8791 return this != null && this.fixed instanceof ParameterDefinition; 8792 } 8793 8794 /** 8795 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8796 */ 8797 public RelatedArtifact getFixedRelatedArtifact() throws FHIRException { 8798 if (this.fixed == null) 8799 this.fixed = new RelatedArtifact(); 8800 if (!(this.fixed instanceof RelatedArtifact)) 8801 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8802 return (RelatedArtifact) this.fixed; 8803 } 8804 8805 public boolean hasFixedRelatedArtifact() { 8806 return this != null && this.fixed instanceof RelatedArtifact; 8807 } 8808 8809 /** 8810 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8811 */ 8812 public TriggerDefinition getFixedTriggerDefinition() throws FHIRException { 8813 if (this.fixed == null) 8814 this.fixed = new TriggerDefinition(); 8815 if (!(this.fixed instanceof TriggerDefinition)) 8816 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8817 return (TriggerDefinition) this.fixed; 8818 } 8819 8820 public boolean hasFixedTriggerDefinition() { 8821 return this != null && this.fixed instanceof TriggerDefinition; 8822 } 8823 8824 /** 8825 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8826 */ 8827 public UsageContext getFixedUsageContext() throws FHIRException { 8828 if (this.fixed == null) 8829 this.fixed = new UsageContext(); 8830 if (!(this.fixed instanceof UsageContext)) 8831 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8832 return (UsageContext) this.fixed; 8833 } 8834 8835 public boolean hasFixedUsageContext() { 8836 return this != null && this.fixed instanceof UsageContext; 8837 } 8838 8839 /** 8840 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8841 */ 8842 public Availability getFixedAvailability() throws FHIRException { 8843 if (this.fixed == null) 8844 this.fixed = new Availability(); 8845 if (!(this.fixed instanceof Availability)) 8846 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8847 return (Availability) this.fixed; 8848 } 8849 8850 public boolean hasFixedAvailability() { 8851 return this != null && this.fixed instanceof Availability; 8852 } 8853 8854 /** 8855 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8856 */ 8857 public ExtendedContactDetail getFixedExtendedContactDetail() throws FHIRException { 8858 if (this.fixed == null) 8859 this.fixed = new ExtendedContactDetail(); 8860 if (!(this.fixed instanceof ExtendedContactDetail)) 8861 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8862 return (ExtendedContactDetail) this.fixed; 8863 } 8864 8865 public boolean hasFixedExtendedContactDetail() { 8866 return this != null && this.fixed instanceof ExtendedContactDetail; 8867 } 8868 8869 /** 8870 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8871 */ 8872 public Dosage getFixedDosage() throws FHIRException { 8873 if (this.fixed == null) 8874 this.fixed = new Dosage(); 8875 if (!(this.fixed instanceof Dosage)) 8876 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8877 return (Dosage) this.fixed; 8878 } 8879 8880 public boolean hasFixedDosage() { 8881 return this != null && this.fixed instanceof Dosage; 8882 } 8883 8884 /** 8885 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8886 */ 8887 public Meta getFixedMeta() throws FHIRException { 8888 if (this.fixed == null) 8889 this.fixed = new Meta(); 8890 if (!(this.fixed instanceof Meta)) 8891 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8892 return (Meta) this.fixed; 8893 } 8894 8895 public boolean hasFixedMeta() { 8896 return this != null && this.fixed instanceof Meta; 8897 } 8898 8899 public boolean hasFixed() { 8900 return this.fixed != null && !this.fixed.isEmpty(); 8901 } 8902 8903 /** 8904 * @param value {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8905 */ 8906 public ElementDefinition setFixed(DataType value) { 8907 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 8908 throw new FHIRException("Not the right type for ElementDefinition.fixed[x]: "+value.fhirType()); 8909 this.fixed = value; 8910 return this; 8911 } 8912 8913 /** 8914 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8915 8916When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8917 8918When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8919 8920When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8921 89221. If primitive: it must match exactly the pattern value 89232. If a complex object: it must match (recursively) the pattern value 89243. If an array: it must match (recursively) the pattern value 8925 8926If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8927 */ 8928 public DataType getPattern() { 8929 return this.pattern; 8930 } 8931 8932 /** 8933 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8934 8935When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8936 8937When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8938 8939When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8940 89411. If primitive: it must match exactly the pattern value 89422. If a complex object: it must match (recursively) the pattern value 89433. If an array: it must match (recursively) the pattern value 8944 8945If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8946 */ 8947 public Base64BinaryType getPatternBase64BinaryType() throws FHIRException { 8948 if (this.pattern == null) 8949 this.pattern = new Base64BinaryType(); 8950 if (!(this.pattern instanceof Base64BinaryType)) 8951 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 8952 return (Base64BinaryType) this.pattern; 8953 } 8954 8955 public boolean hasPatternBase64BinaryType() { 8956 return this != null && this.pattern instanceof Base64BinaryType; 8957 } 8958 8959 /** 8960 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8961 8962When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8963 8964When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8965 8966When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8967 89681. If primitive: it must match exactly the pattern value 89692. If a complex object: it must match (recursively) the pattern value 89703. If an array: it must match (recursively) the pattern value 8971 8972If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8973 */ 8974 public BooleanType getPatternBooleanType() throws FHIRException { 8975 if (this.pattern == null) 8976 this.pattern = new BooleanType(); 8977 if (!(this.pattern instanceof BooleanType)) 8978 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 8979 return (BooleanType) this.pattern; 8980 } 8981 8982 public boolean hasPatternBooleanType() { 8983 return this != null && this.pattern instanceof BooleanType; 8984 } 8985 8986 /** 8987 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8988 8989When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8990 8991When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8992 8993When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8994 89951. If primitive: it must match exactly the pattern value 89962. If a complex object: it must match (recursively) the pattern value 89973. If an array: it must match (recursively) the pattern value 8998 8999If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9000 */ 9001 public CanonicalType getPatternCanonicalType() throws FHIRException { 9002 if (this.pattern == null) 9003 this.pattern = new CanonicalType(); 9004 if (!(this.pattern instanceof CanonicalType)) 9005 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9006 return (CanonicalType) this.pattern; 9007 } 9008 9009 public boolean hasPatternCanonicalType() { 9010 return this != null && this.pattern instanceof CanonicalType; 9011 } 9012 9013 /** 9014 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9015 9016When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9017 9018When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9019 9020When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9021 90221. If primitive: it must match exactly the pattern value 90232. If a complex object: it must match (recursively) the pattern value 90243. If an array: it must match (recursively) the pattern value 9025 9026If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9027 */ 9028 public CodeType getPatternCodeType() throws FHIRException { 9029 if (this.pattern == null) 9030 this.pattern = new CodeType(); 9031 if (!(this.pattern instanceof CodeType)) 9032 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9033 return (CodeType) this.pattern; 9034 } 9035 9036 public boolean hasPatternCodeType() { 9037 return this != null && this.pattern instanceof CodeType; 9038 } 9039 9040 /** 9041 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9042 9043When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9044 9045When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9046 9047When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9048 90491. If primitive: it must match exactly the pattern value 90502. If a complex object: it must match (recursively) the pattern value 90513. If an array: it must match (recursively) the pattern value 9052 9053If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9054 */ 9055 public DateType getPatternDateType() throws FHIRException { 9056 if (this.pattern == null) 9057 this.pattern = new DateType(); 9058 if (!(this.pattern instanceof DateType)) 9059 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9060 return (DateType) this.pattern; 9061 } 9062 9063 public boolean hasPatternDateType() { 9064 return this != null && this.pattern instanceof DateType; 9065 } 9066 9067 /** 9068 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9069 9070When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9071 9072When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9073 9074When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9075 90761. If primitive: it must match exactly the pattern value 90772. If a complex object: it must match (recursively) the pattern value 90783. If an array: it must match (recursively) the pattern value 9079 9080If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9081 */ 9082 public DateTimeType getPatternDateTimeType() throws FHIRException { 9083 if (this.pattern == null) 9084 this.pattern = new DateTimeType(); 9085 if (!(this.pattern instanceof DateTimeType)) 9086 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9087 return (DateTimeType) this.pattern; 9088 } 9089 9090 public boolean hasPatternDateTimeType() { 9091 return this != null && this.pattern instanceof DateTimeType; 9092 } 9093 9094 /** 9095 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9096 9097When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9098 9099When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9100 9101When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9102 91031. If primitive: it must match exactly the pattern value 91042. If a complex object: it must match (recursively) the pattern value 91053. If an array: it must match (recursively) the pattern value 9106 9107If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9108 */ 9109 public DecimalType getPatternDecimalType() throws FHIRException { 9110 if (this.pattern == null) 9111 this.pattern = new DecimalType(); 9112 if (!(this.pattern instanceof DecimalType)) 9113 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9114 return (DecimalType) this.pattern; 9115 } 9116 9117 public boolean hasPatternDecimalType() { 9118 return this != null && this.pattern instanceof DecimalType; 9119 } 9120 9121 /** 9122 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9123 9124When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9125 9126When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9127 9128When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9129 91301. If primitive: it must match exactly the pattern value 91312. If a complex object: it must match (recursively) the pattern value 91323. If an array: it must match (recursively) the pattern value 9133 9134If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9135 */ 9136 public IdType getPatternIdType() throws FHIRException { 9137 if (this.pattern == null) 9138 this.pattern = new IdType(); 9139 if (!(this.pattern instanceof IdType)) 9140 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9141 return (IdType) this.pattern; 9142 } 9143 9144 public boolean hasPatternIdType() { 9145 return this != null && this.pattern instanceof IdType; 9146 } 9147 9148 /** 9149 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9150 9151When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9152 9153When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9154 9155When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9156 91571. If primitive: it must match exactly the pattern value 91582. If a complex object: it must match (recursively) the pattern value 91593. If an array: it must match (recursively) the pattern value 9160 9161If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9162 */ 9163 public InstantType getPatternInstantType() throws FHIRException { 9164 if (this.pattern == null) 9165 this.pattern = new InstantType(); 9166 if (!(this.pattern instanceof InstantType)) 9167 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9168 return (InstantType) this.pattern; 9169 } 9170 9171 public boolean hasPatternInstantType() { 9172 return this != null && this.pattern instanceof InstantType; 9173 } 9174 9175 /** 9176 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9177 9178When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9179 9180When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9181 9182When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9183 91841. If primitive: it must match exactly the pattern value 91852. If a complex object: it must match (recursively) the pattern value 91863. If an array: it must match (recursively) the pattern value 9187 9188If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9189 */ 9190 public IntegerType getPatternIntegerType() throws FHIRException { 9191 if (this.pattern == null) 9192 this.pattern = new IntegerType(); 9193 if (!(this.pattern instanceof IntegerType)) 9194 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9195 return (IntegerType) this.pattern; 9196 } 9197 9198 public boolean hasPatternIntegerType() { 9199 return this != null && this.pattern instanceof IntegerType; 9200 } 9201 9202 /** 9203 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9204 9205When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9206 9207When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9208 9209When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9210 92111. If primitive: it must match exactly the pattern value 92122. If a complex object: it must match (recursively) the pattern value 92133. If an array: it must match (recursively) the pattern value 9214 9215If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9216 */ 9217 public Integer64Type getPatternInteger64Type() throws FHIRException { 9218 if (this.pattern == null) 9219 this.pattern = new Integer64Type(); 9220 if (!(this.pattern instanceof Integer64Type)) 9221 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9222 return (Integer64Type) this.pattern; 9223 } 9224 9225 public boolean hasPatternInteger64Type() { 9226 return this != null && this.pattern instanceof Integer64Type; 9227 } 9228 9229 /** 9230 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9231 9232When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9233 9234When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9235 9236When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9237 92381. If primitive: it must match exactly the pattern value 92392. If a complex object: it must match (recursively) the pattern value 92403. If an array: it must match (recursively) the pattern value 9241 9242If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9243 */ 9244 public MarkdownType getPatternMarkdownType() throws FHIRException { 9245 if (this.pattern == null) 9246 this.pattern = new MarkdownType(); 9247 if (!(this.pattern instanceof MarkdownType)) 9248 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9249 return (MarkdownType) this.pattern; 9250 } 9251 9252 public boolean hasPatternMarkdownType() { 9253 return this != null && this.pattern instanceof MarkdownType; 9254 } 9255 9256 /** 9257 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9258 9259When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9260 9261When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9262 9263When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9264 92651. If primitive: it must match exactly the pattern value 92662. If a complex object: it must match (recursively) the pattern value 92673. If an array: it must match (recursively) the pattern value 9268 9269If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9270 */ 9271 public OidType getPatternOidType() throws FHIRException { 9272 if (this.pattern == null) 9273 this.pattern = new OidType(); 9274 if (!(this.pattern instanceof OidType)) 9275 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9276 return (OidType) this.pattern; 9277 } 9278 9279 public boolean hasPatternOidType() { 9280 return this != null && this.pattern instanceof OidType; 9281 } 9282 9283 /** 9284 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9285 9286When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9287 9288When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9289 9290When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9291 92921. If primitive: it must match exactly the pattern value 92932. If a complex object: it must match (recursively) the pattern value 92943. If an array: it must match (recursively) the pattern value 9295 9296If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9297 */ 9298 public PositiveIntType getPatternPositiveIntType() throws FHIRException { 9299 if (this.pattern == null) 9300 this.pattern = new PositiveIntType(); 9301 if (!(this.pattern instanceof PositiveIntType)) 9302 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9303 return (PositiveIntType) this.pattern; 9304 } 9305 9306 public boolean hasPatternPositiveIntType() { 9307 return this != null && this.pattern instanceof PositiveIntType; 9308 } 9309 9310 /** 9311 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9312 9313When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9314 9315When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9316 9317When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9318 93191. If primitive: it must match exactly the pattern value 93202. If a complex object: it must match (recursively) the pattern value 93213. If an array: it must match (recursively) the pattern value 9322 9323If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9324 */ 9325 public StringType getPatternStringType() throws FHIRException { 9326 if (this.pattern == null) 9327 this.pattern = new StringType(); 9328 if (!(this.pattern instanceof StringType)) 9329 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9330 return (StringType) this.pattern; 9331 } 9332 9333 public boolean hasPatternStringType() { 9334 return this != null && this.pattern instanceof StringType; 9335 } 9336 9337 /** 9338 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9339 9340When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9341 9342When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9343 9344When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9345 93461. If primitive: it must match exactly the pattern value 93472. If a complex object: it must match (recursively) the pattern value 93483. If an array: it must match (recursively) the pattern value 9349 9350If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9351 */ 9352 public TimeType getPatternTimeType() throws FHIRException { 9353 if (this.pattern == null) 9354 this.pattern = new TimeType(); 9355 if (!(this.pattern instanceof TimeType)) 9356 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9357 return (TimeType) this.pattern; 9358 } 9359 9360 public boolean hasPatternTimeType() { 9361 return this != null && this.pattern instanceof TimeType; 9362 } 9363 9364 /** 9365 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9366 9367When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9368 9369When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9370 9371When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9372 93731. If primitive: it must match exactly the pattern value 93742. If a complex object: it must match (recursively) the pattern value 93753. If an array: it must match (recursively) the pattern value 9376 9377If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9378 */ 9379 public UnsignedIntType getPatternUnsignedIntType() throws FHIRException { 9380 if (this.pattern == null) 9381 this.pattern = new UnsignedIntType(); 9382 if (!(this.pattern instanceof UnsignedIntType)) 9383 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9384 return (UnsignedIntType) this.pattern; 9385 } 9386 9387 public boolean hasPatternUnsignedIntType() { 9388 return this != null && this.pattern instanceof UnsignedIntType; 9389 } 9390 9391 /** 9392 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9393 9394When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9395 9396When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9397 9398When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9399 94001. If primitive: it must match exactly the pattern value 94012. If a complex object: it must match (recursively) the pattern value 94023. If an array: it must match (recursively) the pattern value 9403 9404If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9405 */ 9406 public UriType getPatternUriType() throws FHIRException { 9407 if (this.pattern == null) 9408 this.pattern = new UriType(); 9409 if (!(this.pattern instanceof UriType)) 9410 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9411 return (UriType) this.pattern; 9412 } 9413 9414 public boolean hasPatternUriType() { 9415 return this != null && this.pattern instanceof UriType; 9416 } 9417 9418 /** 9419 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9420 9421When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9422 9423When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9424 9425When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9426 94271. If primitive: it must match exactly the pattern value 94282. If a complex object: it must match (recursively) the pattern value 94293. If an array: it must match (recursively) the pattern value 9430 9431If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9432 */ 9433 public UrlType getPatternUrlType() throws FHIRException { 9434 if (this.pattern == null) 9435 this.pattern = new UrlType(); 9436 if (!(this.pattern instanceof UrlType)) 9437 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9438 return (UrlType) this.pattern; 9439 } 9440 9441 public boolean hasPatternUrlType() { 9442 return this != null && this.pattern instanceof UrlType; 9443 } 9444 9445 /** 9446 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9447 9448When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9449 9450When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9451 9452When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9453 94541. If primitive: it must match exactly the pattern value 94552. If a complex object: it must match (recursively) the pattern value 94563. If an array: it must match (recursively) the pattern value 9457 9458If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9459 */ 9460 public UuidType getPatternUuidType() throws FHIRException { 9461 if (this.pattern == null) 9462 this.pattern = new UuidType(); 9463 if (!(this.pattern instanceof UuidType)) 9464 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9465 return (UuidType) this.pattern; 9466 } 9467 9468 public boolean hasPatternUuidType() { 9469 return this != null && this.pattern instanceof UuidType; 9470 } 9471 9472 /** 9473 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9474 9475When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9476 9477When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9478 9479When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9480 94811. If primitive: it must match exactly the pattern value 94822. If a complex object: it must match (recursively) the pattern value 94833. If an array: it must match (recursively) the pattern value 9484 9485If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9486 */ 9487 public Address getPatternAddress() throws FHIRException { 9488 if (this.pattern == null) 9489 this.pattern = new Address(); 9490 if (!(this.pattern instanceof Address)) 9491 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9492 return (Address) this.pattern; 9493 } 9494 9495 public boolean hasPatternAddress() { 9496 return this != null && this.pattern instanceof Address; 9497 } 9498 9499 /** 9500 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9501 9502When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9503 9504When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9505 9506When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9507 95081. If primitive: it must match exactly the pattern value 95092. If a complex object: it must match (recursively) the pattern value 95103. If an array: it must match (recursively) the pattern value 9511 9512If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9513 */ 9514 public Age getPatternAge() throws FHIRException { 9515 if (this.pattern == null) 9516 this.pattern = new Age(); 9517 if (!(this.pattern instanceof Age)) 9518 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9519 return (Age) this.pattern; 9520 } 9521 9522 public boolean hasPatternAge() { 9523 return this != null && this.pattern instanceof Age; 9524 } 9525 9526 /** 9527 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9528 9529When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9530 9531When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9532 9533When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9534 95351. If primitive: it must match exactly the pattern value 95362. If a complex object: it must match (recursively) the pattern value 95373. If an array: it must match (recursively) the pattern value 9538 9539If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9540 */ 9541 public Annotation getPatternAnnotation() throws FHIRException { 9542 if (this.pattern == null) 9543 this.pattern = new Annotation(); 9544 if (!(this.pattern instanceof Annotation)) 9545 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9546 return (Annotation) this.pattern; 9547 } 9548 9549 public boolean hasPatternAnnotation() { 9550 return this != null && this.pattern instanceof Annotation; 9551 } 9552 9553 /** 9554 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9555 9556When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9557 9558When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9559 9560When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9561 95621. If primitive: it must match exactly the pattern value 95632. If a complex object: it must match (recursively) the pattern value 95643. If an array: it must match (recursively) the pattern value 9565 9566If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9567 */ 9568 public Attachment getPatternAttachment() throws FHIRException { 9569 if (this.pattern == null) 9570 this.pattern = new Attachment(); 9571 if (!(this.pattern instanceof Attachment)) 9572 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9573 return (Attachment) this.pattern; 9574 } 9575 9576 public boolean hasPatternAttachment() { 9577 return this != null && this.pattern instanceof Attachment; 9578 } 9579 9580 /** 9581 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9582 9583When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9584 9585When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9586 9587When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9588 95891. If primitive: it must match exactly the pattern value 95902. If a complex object: it must match (recursively) the pattern value 95913. If an array: it must match (recursively) the pattern value 9592 9593If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9594 */ 9595 public CodeableConcept getPatternCodeableConcept() throws FHIRException { 9596 if (this.pattern == null) 9597 this.pattern = new CodeableConcept(); 9598 if (!(this.pattern instanceof CodeableConcept)) 9599 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9600 return (CodeableConcept) this.pattern; 9601 } 9602 9603 public boolean hasPatternCodeableConcept() { 9604 return this != null && this.pattern instanceof CodeableConcept; 9605 } 9606 9607 /** 9608 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9609 9610When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9611 9612When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9613 9614When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9615 96161. If primitive: it must match exactly the pattern value 96172. If a complex object: it must match (recursively) the pattern value 96183. If an array: it must match (recursively) the pattern value 9619 9620If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9621 */ 9622 public CodeableReference getPatternCodeableReference() throws FHIRException { 9623 if (this.pattern == null) 9624 this.pattern = new CodeableReference(); 9625 if (!(this.pattern instanceof CodeableReference)) 9626 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9627 return (CodeableReference) this.pattern; 9628 } 9629 9630 public boolean hasPatternCodeableReference() { 9631 return this != null && this.pattern instanceof CodeableReference; 9632 } 9633 9634 /** 9635 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9636 9637When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9638 9639When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9640 9641When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9642 96431. If primitive: it must match exactly the pattern value 96442. If a complex object: it must match (recursively) the pattern value 96453. If an array: it must match (recursively) the pattern value 9646 9647If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9648 */ 9649 public Coding getPatternCoding() throws FHIRException { 9650 if (this.pattern == null) 9651 this.pattern = new Coding(); 9652 if (!(this.pattern instanceof Coding)) 9653 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9654 return (Coding) this.pattern; 9655 } 9656 9657 public boolean hasPatternCoding() { 9658 return this != null && this.pattern instanceof Coding; 9659 } 9660 9661 /** 9662 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9663 9664When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9665 9666When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9667 9668When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9669 96701. If primitive: it must match exactly the pattern value 96712. If a complex object: it must match (recursively) the pattern value 96723. If an array: it must match (recursively) the pattern value 9673 9674If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9675 */ 9676 public ContactPoint getPatternContactPoint() throws FHIRException { 9677 if (this.pattern == null) 9678 this.pattern = new ContactPoint(); 9679 if (!(this.pattern instanceof ContactPoint)) 9680 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9681 return (ContactPoint) this.pattern; 9682 } 9683 9684 public boolean hasPatternContactPoint() { 9685 return this != null && this.pattern instanceof ContactPoint; 9686 } 9687 9688 /** 9689 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9690 9691When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9692 9693When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9694 9695When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9696 96971. If primitive: it must match exactly the pattern value 96982. If a complex object: it must match (recursively) the pattern value 96993. If an array: it must match (recursively) the pattern value 9700 9701If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9702 */ 9703 public Count getPatternCount() throws FHIRException { 9704 if (this.pattern == null) 9705 this.pattern = new Count(); 9706 if (!(this.pattern instanceof Count)) 9707 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9708 return (Count) this.pattern; 9709 } 9710 9711 public boolean hasPatternCount() { 9712 return this != null && this.pattern instanceof Count; 9713 } 9714 9715 /** 9716 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9717 9718When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9719 9720When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9721 9722When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9723 97241. If primitive: it must match exactly the pattern value 97252. If a complex object: it must match (recursively) the pattern value 97263. If an array: it must match (recursively) the pattern value 9727 9728If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9729 */ 9730 public Distance getPatternDistance() throws FHIRException { 9731 if (this.pattern == null) 9732 this.pattern = new Distance(); 9733 if (!(this.pattern instanceof Distance)) 9734 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9735 return (Distance) this.pattern; 9736 } 9737 9738 public boolean hasPatternDistance() { 9739 return this != null && this.pattern instanceof Distance; 9740 } 9741 9742 /** 9743 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9744 9745When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9746 9747When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9748 9749When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9750 97511. If primitive: it must match exactly the pattern value 97522. If a complex object: it must match (recursively) the pattern value 97533. If an array: it must match (recursively) the pattern value 9754 9755If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9756 */ 9757 public Duration getPatternDuration() throws FHIRException { 9758 if (this.pattern == null) 9759 this.pattern = new Duration(); 9760 if (!(this.pattern instanceof Duration)) 9761 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9762 return (Duration) this.pattern; 9763 } 9764 9765 public boolean hasPatternDuration() { 9766 return this != null && this.pattern instanceof Duration; 9767 } 9768 9769 /** 9770 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9771 9772When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9773 9774When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9775 9776When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9777 97781. If primitive: it must match exactly the pattern value 97792. If a complex object: it must match (recursively) the pattern value 97803. If an array: it must match (recursively) the pattern value 9781 9782If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9783 */ 9784 public HumanName getPatternHumanName() throws FHIRException { 9785 if (this.pattern == null) 9786 this.pattern = new HumanName(); 9787 if (!(this.pattern instanceof HumanName)) 9788 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9789 return (HumanName) this.pattern; 9790 } 9791 9792 public boolean hasPatternHumanName() { 9793 return this != null && this.pattern instanceof HumanName; 9794 } 9795 9796 /** 9797 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9798 9799When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9800 9801When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9802 9803When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9804 98051. If primitive: it must match exactly the pattern value 98062. If a complex object: it must match (recursively) the pattern value 98073. If an array: it must match (recursively) the pattern value 9808 9809If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9810 */ 9811 public Identifier getPatternIdentifier() throws FHIRException { 9812 if (this.pattern == null) 9813 this.pattern = new Identifier(); 9814 if (!(this.pattern instanceof Identifier)) 9815 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9816 return (Identifier) this.pattern; 9817 } 9818 9819 public boolean hasPatternIdentifier() { 9820 return this != null && this.pattern instanceof Identifier; 9821 } 9822 9823 /** 9824 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9825 9826When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9827 9828When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9829 9830When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9831 98321. If primitive: it must match exactly the pattern value 98332. If a complex object: it must match (recursively) the pattern value 98343. If an array: it must match (recursively) the pattern value 9835 9836If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9837 */ 9838 public Money getPatternMoney() throws FHIRException { 9839 if (this.pattern == null) 9840 this.pattern = new Money(); 9841 if (!(this.pattern instanceof Money)) 9842 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9843 return (Money) this.pattern; 9844 } 9845 9846 public boolean hasPatternMoney() { 9847 return this != null && this.pattern instanceof Money; 9848 } 9849 9850 /** 9851 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9852 9853When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9854 9855When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9856 9857When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9858 98591. If primitive: it must match exactly the pattern value 98602. If a complex object: it must match (recursively) the pattern value 98613. If an array: it must match (recursively) the pattern value 9862 9863If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9864 */ 9865 public Period getPatternPeriod() throws FHIRException { 9866 if (this.pattern == null) 9867 this.pattern = new Period(); 9868 if (!(this.pattern instanceof Period)) 9869 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9870 return (Period) this.pattern; 9871 } 9872 9873 public boolean hasPatternPeriod() { 9874 return this != null && this.pattern instanceof Period; 9875 } 9876 9877 /** 9878 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9879 9880When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9881 9882When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9883 9884When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9885 98861. If primitive: it must match exactly the pattern value 98872. If a complex object: it must match (recursively) the pattern value 98883. If an array: it must match (recursively) the pattern value 9889 9890If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9891 */ 9892 public Quantity getPatternQuantity() throws FHIRException { 9893 if (this.pattern == null) 9894 this.pattern = new Quantity(); 9895 if (!(this.pattern instanceof Quantity)) 9896 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9897 return (Quantity) this.pattern; 9898 } 9899 9900 public boolean hasPatternQuantity() { 9901 return this != null && this.pattern instanceof Quantity; 9902 } 9903 9904 /** 9905 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9906 9907When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9908 9909When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9910 9911When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9912 99131. If primitive: it must match exactly the pattern value 99142. If a complex object: it must match (recursively) the pattern value 99153. If an array: it must match (recursively) the pattern value 9916 9917If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9918 */ 9919 public Range getPatternRange() throws FHIRException { 9920 if (this.pattern == null) 9921 this.pattern = new Range(); 9922 if (!(this.pattern instanceof Range)) 9923 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9924 return (Range) this.pattern; 9925 } 9926 9927 public boolean hasPatternRange() { 9928 return this != null && this.pattern instanceof Range; 9929 } 9930 9931 /** 9932 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9933 9934When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9935 9936When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9937 9938When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9939 99401. If primitive: it must match exactly the pattern value 99412. If a complex object: it must match (recursively) the pattern value 99423. If an array: it must match (recursively) the pattern value 9943 9944If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9945 */ 9946 public Ratio getPatternRatio() throws FHIRException { 9947 if (this.pattern == null) 9948 this.pattern = new Ratio(); 9949 if (!(this.pattern instanceof Ratio)) 9950 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9951 return (Ratio) this.pattern; 9952 } 9953 9954 public boolean hasPatternRatio() { 9955 return this != null && this.pattern instanceof Ratio; 9956 } 9957 9958 /** 9959 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9960 9961When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9962 9963When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9964 9965When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9966 99671. If primitive: it must match exactly the pattern value 99682. If a complex object: it must match (recursively) the pattern value 99693. If an array: it must match (recursively) the pattern value 9970 9971If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9972 */ 9973 public RatioRange getPatternRatioRange() throws FHIRException { 9974 if (this.pattern == null) 9975 this.pattern = new RatioRange(); 9976 if (!(this.pattern instanceof RatioRange)) 9977 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9978 return (RatioRange) this.pattern; 9979 } 9980 9981 public boolean hasPatternRatioRange() { 9982 return this != null && this.pattern instanceof RatioRange; 9983 } 9984 9985 /** 9986 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9987 9988When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9989 9990When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9991 9992When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9993 99941. If primitive: it must match exactly the pattern value 99952. If a complex object: it must match (recursively) the pattern value 99963. If an array: it must match (recursively) the pattern value 9997 9998If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9999 */ 10000 public Reference getPatternReference() throws FHIRException { 10001 if (this.pattern == null) 10002 this.pattern = new Reference(); 10003 if (!(this.pattern instanceof Reference)) 10004 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10005 return (Reference) this.pattern; 10006 } 10007 10008 public boolean hasPatternReference() { 10009 return this != null && this.pattern instanceof Reference; 10010 } 10011 10012 /** 10013 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10014 10015When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10016 10017When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10018 10019When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10020 100211. If primitive: it must match exactly the pattern value 100222. If a complex object: it must match (recursively) the pattern value 100233. If an array: it must match (recursively) the pattern value 10024 10025If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10026 */ 10027 public SampledData getPatternSampledData() throws FHIRException { 10028 if (this.pattern == null) 10029 this.pattern = new SampledData(); 10030 if (!(this.pattern instanceof SampledData)) 10031 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10032 return (SampledData) this.pattern; 10033 } 10034 10035 public boolean hasPatternSampledData() { 10036 return this != null && this.pattern instanceof SampledData; 10037 } 10038 10039 /** 10040 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10041 10042When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10043 10044When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10045 10046When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10047 100481. If primitive: it must match exactly the pattern value 100492. If a complex object: it must match (recursively) the pattern value 100503. If an array: it must match (recursively) the pattern value 10051 10052If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10053 */ 10054 public Signature getPatternSignature() throws FHIRException { 10055 if (this.pattern == null) 10056 this.pattern = new Signature(); 10057 if (!(this.pattern instanceof Signature)) 10058 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10059 return (Signature) this.pattern; 10060 } 10061 10062 public boolean hasPatternSignature() { 10063 return this != null && this.pattern instanceof Signature; 10064 } 10065 10066 /** 10067 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10068 10069When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10070 10071When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10072 10073When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10074 100751. If primitive: it must match exactly the pattern value 100762. If a complex object: it must match (recursively) the pattern value 100773. If an array: it must match (recursively) the pattern value 10078 10079If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10080 */ 10081 public Timing getPatternTiming() throws FHIRException { 10082 if (this.pattern == null) 10083 this.pattern = new Timing(); 10084 if (!(this.pattern instanceof Timing)) 10085 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10086 return (Timing) this.pattern; 10087 } 10088 10089 public boolean hasPatternTiming() { 10090 return this != null && this.pattern instanceof Timing; 10091 } 10092 10093 /** 10094 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10095 10096When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10097 10098When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10099 10100When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10101 101021. If primitive: it must match exactly the pattern value 101032. If a complex object: it must match (recursively) the pattern value 101043. If an array: it must match (recursively) the pattern value 10105 10106If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10107 */ 10108 public ContactDetail getPatternContactDetail() throws FHIRException { 10109 if (this.pattern == null) 10110 this.pattern = new ContactDetail(); 10111 if (!(this.pattern instanceof ContactDetail)) 10112 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10113 return (ContactDetail) this.pattern; 10114 } 10115 10116 public boolean hasPatternContactDetail() { 10117 return this != null && this.pattern instanceof ContactDetail; 10118 } 10119 10120 /** 10121 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10122 10123When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10124 10125When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10126 10127When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10128 101291. If primitive: it must match exactly the pattern value 101302. If a complex object: it must match (recursively) the pattern value 101313. If an array: it must match (recursively) the pattern value 10132 10133If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10134 */ 10135 public DataRequirement getPatternDataRequirement() throws FHIRException { 10136 if (this.pattern == null) 10137 this.pattern = new DataRequirement(); 10138 if (!(this.pattern instanceof DataRequirement)) 10139 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10140 return (DataRequirement) this.pattern; 10141 } 10142 10143 public boolean hasPatternDataRequirement() { 10144 return this != null && this.pattern instanceof DataRequirement; 10145 } 10146 10147 /** 10148 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10149 10150When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10151 10152When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10153 10154When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10155 101561. If primitive: it must match exactly the pattern value 101572. If a complex object: it must match (recursively) the pattern value 101583. If an array: it must match (recursively) the pattern value 10159 10160If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10161 */ 10162 public Expression getPatternExpression() throws FHIRException { 10163 if (this.pattern == null) 10164 this.pattern = new Expression(); 10165 if (!(this.pattern instanceof Expression)) 10166 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10167 return (Expression) this.pattern; 10168 } 10169 10170 public boolean hasPatternExpression() { 10171 return this != null && this.pattern instanceof Expression; 10172 } 10173 10174 /** 10175 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10176 10177When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10178 10179When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10180 10181When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10182 101831. If primitive: it must match exactly the pattern value 101842. If a complex object: it must match (recursively) the pattern value 101853. If an array: it must match (recursively) the pattern value 10186 10187If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10188 */ 10189 public ParameterDefinition getPatternParameterDefinition() throws FHIRException { 10190 if (this.pattern == null) 10191 this.pattern = new ParameterDefinition(); 10192 if (!(this.pattern instanceof ParameterDefinition)) 10193 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10194 return (ParameterDefinition) this.pattern; 10195 } 10196 10197 public boolean hasPatternParameterDefinition() { 10198 return this != null && this.pattern instanceof ParameterDefinition; 10199 } 10200 10201 /** 10202 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10203 10204When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10205 10206When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10207 10208When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10209 102101. If primitive: it must match exactly the pattern value 102112. If a complex object: it must match (recursively) the pattern value 102123. If an array: it must match (recursively) the pattern value 10213 10214If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10215 */ 10216 public RelatedArtifact getPatternRelatedArtifact() throws FHIRException { 10217 if (this.pattern == null) 10218 this.pattern = new RelatedArtifact(); 10219 if (!(this.pattern instanceof RelatedArtifact)) 10220 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10221 return (RelatedArtifact) this.pattern; 10222 } 10223 10224 public boolean hasPatternRelatedArtifact() { 10225 return this != null && this.pattern instanceof RelatedArtifact; 10226 } 10227 10228 /** 10229 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10230 10231When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10232 10233When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10234 10235When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10236 102371. If primitive: it must match exactly the pattern value 102382. If a complex object: it must match (recursively) the pattern value 102393. If an array: it must match (recursively) the pattern value 10240 10241If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10242 */ 10243 public TriggerDefinition getPatternTriggerDefinition() throws FHIRException { 10244 if (this.pattern == null) 10245 this.pattern = new TriggerDefinition(); 10246 if (!(this.pattern instanceof TriggerDefinition)) 10247 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10248 return (TriggerDefinition) this.pattern; 10249 } 10250 10251 public boolean hasPatternTriggerDefinition() { 10252 return this != null && this.pattern instanceof TriggerDefinition; 10253 } 10254 10255 /** 10256 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10257 10258When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10259 10260When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10261 10262When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10263 102641. If primitive: it must match exactly the pattern value 102652. If a complex object: it must match (recursively) the pattern value 102663. If an array: it must match (recursively) the pattern value 10267 10268If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10269 */ 10270 public UsageContext getPatternUsageContext() throws FHIRException { 10271 if (this.pattern == null) 10272 this.pattern = new UsageContext(); 10273 if (!(this.pattern instanceof UsageContext)) 10274 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10275 return (UsageContext) this.pattern; 10276 } 10277 10278 public boolean hasPatternUsageContext() { 10279 return this != null && this.pattern instanceof UsageContext; 10280 } 10281 10282 /** 10283 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10284 10285When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10286 10287When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10288 10289When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10290 102911. If primitive: it must match exactly the pattern value 102922. If a complex object: it must match (recursively) the pattern value 102933. If an array: it must match (recursively) the pattern value 10294 10295If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10296 */ 10297 public Availability getPatternAvailability() throws FHIRException { 10298 if (this.pattern == null) 10299 this.pattern = new Availability(); 10300 if (!(this.pattern instanceof Availability)) 10301 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10302 return (Availability) this.pattern; 10303 } 10304 10305 public boolean hasPatternAvailability() { 10306 return this != null && this.pattern instanceof Availability; 10307 } 10308 10309 /** 10310 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10311 10312When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10313 10314When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10315 10316When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10317 103181. If primitive: it must match exactly the pattern value 103192. If a complex object: it must match (recursively) the pattern value 103203. If an array: it must match (recursively) the pattern value 10321 10322If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10323 */ 10324 public ExtendedContactDetail getPatternExtendedContactDetail() throws FHIRException { 10325 if (this.pattern == null) 10326 this.pattern = new ExtendedContactDetail(); 10327 if (!(this.pattern instanceof ExtendedContactDetail)) 10328 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10329 return (ExtendedContactDetail) this.pattern; 10330 } 10331 10332 public boolean hasPatternExtendedContactDetail() { 10333 return this != null && this.pattern instanceof ExtendedContactDetail; 10334 } 10335 10336 /** 10337 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10338 10339When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10340 10341When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10342 10343When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10344 103451. If primitive: it must match exactly the pattern value 103462. If a complex object: it must match (recursively) the pattern value 103473. If an array: it must match (recursively) the pattern value 10348 10349If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10350 */ 10351 public Dosage getPatternDosage() throws FHIRException { 10352 if (this.pattern == null) 10353 this.pattern = new Dosage(); 10354 if (!(this.pattern instanceof Dosage)) 10355 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10356 return (Dosage) this.pattern; 10357 } 10358 10359 public boolean hasPatternDosage() { 10360 return this != null && this.pattern instanceof Dosage; 10361 } 10362 10363 /** 10364 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10365 10366When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10367 10368When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10369 10370When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10371 103721. If primitive: it must match exactly the pattern value 103732. If a complex object: it must match (recursively) the pattern value 103743. If an array: it must match (recursively) the pattern value 10375 10376If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10377 */ 10378 public Meta getPatternMeta() throws FHIRException { 10379 if (this.pattern == null) 10380 this.pattern = new Meta(); 10381 if (!(this.pattern instanceof Meta)) 10382 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10383 return (Meta) this.pattern; 10384 } 10385 10386 public boolean hasPatternMeta() { 10387 return this != null && this.pattern instanceof Meta; 10388 } 10389 10390 public boolean hasPattern() { 10391 return this.pattern != null && !this.pattern.isEmpty(); 10392 } 10393 10394 /** 10395 * @param value {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10396 10397When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10398 10399When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10400 10401When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10402 104031. If primitive: it must match exactly the pattern value 104042. If a complex object: it must match (recursively) the pattern value 104053. If an array: it must match (recursively) the pattern value 10406 10407If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10408 */ 10409 public ElementDefinition setPattern(DataType value) { 10410 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 10411 throw new FHIRException("Not the right type for ElementDefinition.pattern[x]: "+value.fhirType()); 10412 this.pattern = value; 10413 return this; 10414 } 10415 10416 /** 10417 * @return {@link #example} (A sample value for this element demonstrating the type of information that would typically be found in the element.) 10418 */ 10419 public List<ElementDefinitionExampleComponent> getExample() { 10420 if (this.example == null) 10421 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 10422 return this.example; 10423 } 10424 10425 /** 10426 * @return Returns a reference to <code>this</code> for easy method chaining 10427 */ 10428 public ElementDefinition setExample(List<ElementDefinitionExampleComponent> theExample) { 10429 this.example = theExample; 10430 return this; 10431 } 10432 10433 public boolean hasExample() { 10434 if (this.example == null) 10435 return false; 10436 for (ElementDefinitionExampleComponent item : this.example) 10437 if (!item.isEmpty()) 10438 return true; 10439 return false; 10440 } 10441 10442 public ElementDefinitionExampleComponent addExample() { //3 10443 ElementDefinitionExampleComponent t = new ElementDefinitionExampleComponent(); 10444 if (this.example == null) 10445 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 10446 this.example.add(t); 10447 return t; 10448 } 10449 10450 public ElementDefinition addExample(ElementDefinitionExampleComponent t) { //3 10451 if (t == null) 10452 return this; 10453 if (this.example == null) 10454 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 10455 this.example.add(t); 10456 return this; 10457 } 10458 10459 /** 10460 * @return The first repetition of repeating field {@link #example}, creating it if it does not already exist {3} 10461 */ 10462 public ElementDefinitionExampleComponent getExampleFirstRep() { 10463 if (getExample().isEmpty()) { 10464 addExample(); 10465 } 10466 return getExample().get(0); 10467 } 10468 10469 /** 10470 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10471 */ 10472 public DataType getMinValue() { 10473 return this.minValue; 10474 } 10475 10476 /** 10477 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10478 */ 10479 public DateType getMinValueDateType() throws FHIRException { 10480 if (this.minValue == null) 10481 this.minValue = new DateType(); 10482 if (!(this.minValue instanceof DateType)) 10483 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10484 return (DateType) this.minValue; 10485 } 10486 10487 public boolean hasMinValueDateType() { 10488 return this != null && this.minValue instanceof DateType; 10489 } 10490 10491 /** 10492 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10493 */ 10494 public DateTimeType getMinValueDateTimeType() throws FHIRException { 10495 if (this.minValue == null) 10496 this.minValue = new DateTimeType(); 10497 if (!(this.minValue instanceof DateTimeType)) 10498 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10499 return (DateTimeType) this.minValue; 10500 } 10501 10502 public boolean hasMinValueDateTimeType() { 10503 return this != null && this.minValue instanceof DateTimeType; 10504 } 10505 10506 /** 10507 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10508 */ 10509 public InstantType getMinValueInstantType() throws FHIRException { 10510 if (this.minValue == null) 10511 this.minValue = new InstantType(); 10512 if (!(this.minValue instanceof InstantType)) 10513 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10514 return (InstantType) this.minValue; 10515 } 10516 10517 public boolean hasMinValueInstantType() { 10518 return this != null && this.minValue instanceof InstantType; 10519 } 10520 10521 /** 10522 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10523 */ 10524 public TimeType getMinValueTimeType() throws FHIRException { 10525 if (this.minValue == null) 10526 this.minValue = new TimeType(); 10527 if (!(this.minValue instanceof TimeType)) 10528 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10529 return (TimeType) this.minValue; 10530 } 10531 10532 public boolean hasMinValueTimeType() { 10533 return this != null && this.minValue instanceof TimeType; 10534 } 10535 10536 /** 10537 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10538 */ 10539 public DecimalType getMinValueDecimalType() throws FHIRException { 10540 if (this.minValue == null) 10541 this.minValue = new DecimalType(); 10542 if (!(this.minValue instanceof DecimalType)) 10543 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10544 return (DecimalType) this.minValue; 10545 } 10546 10547 public boolean hasMinValueDecimalType() { 10548 return this != null && this.minValue instanceof DecimalType; 10549 } 10550 10551 /** 10552 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10553 */ 10554 public IntegerType getMinValueIntegerType() throws FHIRException { 10555 if (this.minValue == null) 10556 this.minValue = new IntegerType(); 10557 if (!(this.minValue instanceof IntegerType)) 10558 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10559 return (IntegerType) this.minValue; 10560 } 10561 10562 public boolean hasMinValueIntegerType() { 10563 return this != null && this.minValue instanceof IntegerType; 10564 } 10565 10566 /** 10567 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10568 */ 10569 public Integer64Type getMinValueInteger64Type() throws FHIRException { 10570 if (this.minValue == null) 10571 this.minValue = new Integer64Type(); 10572 if (!(this.minValue instanceof Integer64Type)) 10573 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10574 return (Integer64Type) this.minValue; 10575 } 10576 10577 public boolean hasMinValueInteger64Type() { 10578 return this != null && this.minValue instanceof Integer64Type; 10579 } 10580 10581 /** 10582 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10583 */ 10584 public PositiveIntType getMinValuePositiveIntType() throws FHIRException { 10585 if (this.minValue == null) 10586 this.minValue = new PositiveIntType(); 10587 if (!(this.minValue instanceof PositiveIntType)) 10588 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10589 return (PositiveIntType) this.minValue; 10590 } 10591 10592 public boolean hasMinValuePositiveIntType() { 10593 return this != null && this.minValue instanceof PositiveIntType; 10594 } 10595 10596 /** 10597 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10598 */ 10599 public UnsignedIntType getMinValueUnsignedIntType() throws FHIRException { 10600 if (this.minValue == null) 10601 this.minValue = new UnsignedIntType(); 10602 if (!(this.minValue instanceof UnsignedIntType)) 10603 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10604 return (UnsignedIntType) this.minValue; 10605 } 10606 10607 public boolean hasMinValueUnsignedIntType() { 10608 return this != null && this.minValue instanceof UnsignedIntType; 10609 } 10610 10611 /** 10612 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10613 */ 10614 public Quantity getMinValueQuantity() throws FHIRException { 10615 if (this.minValue == null) 10616 this.minValue = new Quantity(); 10617 if (!(this.minValue instanceof Quantity)) 10618 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10619 return (Quantity) this.minValue; 10620 } 10621 10622 public boolean hasMinValueQuantity() { 10623 return this != null && this.minValue instanceof Quantity; 10624 } 10625 10626 public boolean hasMinValue() { 10627 return this.minValue != null && !this.minValue.isEmpty(); 10628 } 10629 10630 /** 10631 * @param value {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10632 */ 10633 public ElementDefinition setMinValue(DataType value) { 10634 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 10635 throw new FHIRException("Not the right type for ElementDefinition.minValue[x]: "+value.fhirType()); 10636 this.minValue = value; 10637 return this; 10638 } 10639 10640 /** 10641 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10642 */ 10643 public DataType getMaxValue() { 10644 return this.maxValue; 10645 } 10646 10647 /** 10648 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10649 */ 10650 public DateType getMaxValueDateType() throws FHIRException { 10651 if (this.maxValue == null) 10652 this.maxValue = new DateType(); 10653 if (!(this.maxValue instanceof DateType)) 10654 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10655 return (DateType) this.maxValue; 10656 } 10657 10658 public boolean hasMaxValueDateType() { 10659 return this != null && this.maxValue instanceof DateType; 10660 } 10661 10662 /** 10663 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10664 */ 10665 public DateTimeType getMaxValueDateTimeType() throws FHIRException { 10666 if (this.maxValue == null) 10667 this.maxValue = new DateTimeType(); 10668 if (!(this.maxValue instanceof DateTimeType)) 10669 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10670 return (DateTimeType) this.maxValue; 10671 } 10672 10673 public boolean hasMaxValueDateTimeType() { 10674 return this != null && this.maxValue instanceof DateTimeType; 10675 } 10676 10677 /** 10678 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10679 */ 10680 public InstantType getMaxValueInstantType() throws FHIRException { 10681 if (this.maxValue == null) 10682 this.maxValue = new InstantType(); 10683 if (!(this.maxValue instanceof InstantType)) 10684 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10685 return (InstantType) this.maxValue; 10686 } 10687 10688 public boolean hasMaxValueInstantType() { 10689 return this != null && this.maxValue instanceof InstantType; 10690 } 10691 10692 /** 10693 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10694 */ 10695 public TimeType getMaxValueTimeType() throws FHIRException { 10696 if (this.maxValue == null) 10697 this.maxValue = new TimeType(); 10698 if (!(this.maxValue instanceof TimeType)) 10699 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10700 return (TimeType) this.maxValue; 10701 } 10702 10703 public boolean hasMaxValueTimeType() { 10704 return this != null && this.maxValue instanceof TimeType; 10705 } 10706 10707 /** 10708 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10709 */ 10710 public DecimalType getMaxValueDecimalType() throws FHIRException { 10711 if (this.maxValue == null) 10712 this.maxValue = new DecimalType(); 10713 if (!(this.maxValue instanceof DecimalType)) 10714 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10715 return (DecimalType) this.maxValue; 10716 } 10717 10718 public boolean hasMaxValueDecimalType() { 10719 return this != null && this.maxValue instanceof DecimalType; 10720 } 10721 10722 /** 10723 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10724 */ 10725 public IntegerType getMaxValueIntegerType() throws FHIRException { 10726 if (this.maxValue == null) 10727 this.maxValue = new IntegerType(); 10728 if (!(this.maxValue instanceof IntegerType)) 10729 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10730 return (IntegerType) this.maxValue; 10731 } 10732 10733 public boolean hasMaxValueIntegerType() { 10734 return this != null && this.maxValue instanceof IntegerType; 10735 } 10736 10737 /** 10738 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10739 */ 10740 public Integer64Type getMaxValueInteger64Type() throws FHIRException { 10741 if (this.maxValue == null) 10742 this.maxValue = new Integer64Type(); 10743 if (!(this.maxValue instanceof Integer64Type)) 10744 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10745 return (Integer64Type) this.maxValue; 10746 } 10747 10748 public boolean hasMaxValueInteger64Type() { 10749 return this != null && this.maxValue instanceof Integer64Type; 10750 } 10751 10752 /** 10753 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10754 */ 10755 public PositiveIntType getMaxValuePositiveIntType() throws FHIRException { 10756 if (this.maxValue == null) 10757 this.maxValue = new PositiveIntType(); 10758 if (!(this.maxValue instanceof PositiveIntType)) 10759 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10760 return (PositiveIntType) this.maxValue; 10761 } 10762 10763 public boolean hasMaxValuePositiveIntType() { 10764 return this != null && this.maxValue instanceof PositiveIntType; 10765 } 10766 10767 /** 10768 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10769 */ 10770 public UnsignedIntType getMaxValueUnsignedIntType() throws FHIRException { 10771 if (this.maxValue == null) 10772 this.maxValue = new UnsignedIntType(); 10773 if (!(this.maxValue instanceof UnsignedIntType)) 10774 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10775 return (UnsignedIntType) this.maxValue; 10776 } 10777 10778 public boolean hasMaxValueUnsignedIntType() { 10779 return this != null && this.maxValue instanceof UnsignedIntType; 10780 } 10781 10782 /** 10783 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10784 */ 10785 public Quantity getMaxValueQuantity() throws FHIRException { 10786 if (this.maxValue == null) 10787 this.maxValue = new Quantity(); 10788 if (!(this.maxValue instanceof Quantity)) 10789 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10790 return (Quantity) this.maxValue; 10791 } 10792 10793 public boolean hasMaxValueQuantity() { 10794 return this != null && this.maxValue instanceof Quantity; 10795 } 10796 10797 public boolean hasMaxValue() { 10798 return this.maxValue != null && !this.maxValue.isEmpty(); 10799 } 10800 10801 /** 10802 * @param value {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10803 */ 10804 public ElementDefinition setMaxValue(DataType value) { 10805 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 10806 throw new FHIRException("Not the right type for ElementDefinition.maxValue[x]: "+value.fhirType()); 10807 this.maxValue = value; 10808 return this; 10809 } 10810 10811 /** 10812 * @return {@link #maxLength} (Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 10813 */ 10814 public IntegerType getMaxLengthElement() { 10815 if (this.maxLength == null) 10816 if (Configuration.errorOnAutoCreate()) 10817 throw new Error("Attempt to auto-create ElementDefinition.maxLength"); 10818 else if (Configuration.doAutoCreate()) 10819 this.maxLength = new IntegerType(); // bb 10820 return this.maxLength; 10821 } 10822 10823 public boolean hasMaxLengthElement() { 10824 return this.maxLength != null && !this.maxLength.isEmpty(); 10825 } 10826 10827 public boolean hasMaxLength() { 10828 return this.maxLength != null && !this.maxLength.isEmpty(); 10829 } 10830 10831 /** 10832 * @param value {@link #maxLength} (Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 10833 */ 10834 public ElementDefinition setMaxLengthElement(IntegerType value) { 10835 this.maxLength = value; 10836 return this; 10837 } 10838 10839 /** 10840 * @return Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)). 10841 */ 10842 public int getMaxLength() { 10843 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 10844 } 10845 10846 /** 10847 * @param value Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)). 10848 */ 10849 public ElementDefinition setMaxLength(int value) { 10850 if (this.maxLength == null) 10851 this.maxLength = new IntegerType(); 10852 this.maxLength.setValue(value); 10853 return this; 10854 } 10855 10856 /** 10857 * @return {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10858 */ 10859 public List<IdType> getCondition() { 10860 if (this.condition == null) 10861 this.condition = new ArrayList<IdType>(); 10862 return this.condition; 10863 } 10864 10865 /** 10866 * @return Returns a reference to <code>this</code> for easy method chaining 10867 */ 10868 public ElementDefinition setCondition(List<IdType> theCondition) { 10869 this.condition = theCondition; 10870 return this; 10871 } 10872 10873 public boolean hasCondition() { 10874 if (this.condition == null) 10875 return false; 10876 for (IdType item : this.condition) 10877 if (!item.isEmpty()) 10878 return true; 10879 return false; 10880 } 10881 10882 /** 10883 * @return {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10884 */ 10885 public IdType addConditionElement() {//2 10886 IdType t = new IdType(); 10887 if (this.condition == null) 10888 this.condition = new ArrayList<IdType>(); 10889 this.condition.add(t); 10890 return t; 10891 } 10892 10893 /** 10894 * @param value {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10895 */ 10896 public ElementDefinition addCondition(String value) { //1 10897 IdType t = new IdType(); 10898 t.setValue(value); 10899 if (this.condition == null) 10900 this.condition = new ArrayList<IdType>(); 10901 this.condition.add(t); 10902 return this; 10903 } 10904 10905 /** 10906 * @param value {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10907 */ 10908 public boolean hasCondition(String value) { 10909 if (this.condition == null) 10910 return false; 10911 for (IdType v : this.condition) 10912 if (v.getValue().equals(value)) // id 10913 return true; 10914 return false; 10915 } 10916 10917 /** 10918 * @return {@link #constraint} (Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.) 10919 */ 10920 public List<ElementDefinitionConstraintComponent> getConstraint() { 10921 if (this.constraint == null) 10922 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 10923 return this.constraint; 10924 } 10925 10926 /** 10927 * @return Returns a reference to <code>this</code> for easy method chaining 10928 */ 10929 public ElementDefinition setConstraint(List<ElementDefinitionConstraintComponent> theConstraint) { 10930 this.constraint = theConstraint; 10931 return this; 10932 } 10933 10934 public boolean hasConstraint() { 10935 if (this.constraint == null) 10936 return false; 10937 for (ElementDefinitionConstraintComponent item : this.constraint) 10938 if (!item.isEmpty()) 10939 return true; 10940 return false; 10941 } 10942 10943 public ElementDefinitionConstraintComponent addConstraint() { //3 10944 ElementDefinitionConstraintComponent t = new ElementDefinitionConstraintComponent(); 10945 if (this.constraint == null) 10946 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 10947 this.constraint.add(t); 10948 return t; 10949 } 10950 10951 public ElementDefinition addConstraint(ElementDefinitionConstraintComponent t) { //3 10952 if (t == null) 10953 return this; 10954 if (this.constraint == null) 10955 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 10956 this.constraint.add(t); 10957 return this; 10958 } 10959 10960 /** 10961 * @return The first repetition of repeating field {@link #constraint}, creating it if it does not already exist {3} 10962 */ 10963 public ElementDefinitionConstraintComponent getConstraintFirstRep() { 10964 if (getConstraint().isEmpty()) { 10965 addConstraint(); 10966 } 10967 return getConstraint().get(0); 10968 } 10969 10970 /** 10971 * @return {@link #mustHaveValue} (Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.). This is the underlying object with id, value and extensions. The accessor "getMustHaveValue" gives direct access to the value 10972 */ 10973 public BooleanType getMustHaveValueElement() { 10974 if (this.mustHaveValue == null) 10975 if (Configuration.errorOnAutoCreate()) 10976 throw new Error("Attempt to auto-create ElementDefinition.mustHaveValue"); 10977 else if (Configuration.doAutoCreate()) 10978 this.mustHaveValue = new BooleanType(); // bb 10979 return this.mustHaveValue; 10980 } 10981 10982 public boolean hasMustHaveValueElement() { 10983 return this.mustHaveValue != null && !this.mustHaveValue.isEmpty(); 10984 } 10985 10986 public boolean hasMustHaveValue() { 10987 return this.mustHaveValue != null && !this.mustHaveValue.isEmpty(); 10988 } 10989 10990 /** 10991 * @param value {@link #mustHaveValue} (Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.). This is the underlying object with id, value and extensions. The accessor "getMustHaveValue" gives direct access to the value 10992 */ 10993 public ElementDefinition setMustHaveValueElement(BooleanType value) { 10994 this.mustHaveValue = value; 10995 return this; 10996 } 10997 10998 /** 10999 * @return Specifies for a primitive data type that the value of the data type cannot be replaced by an extension. 11000 */ 11001 public boolean getMustHaveValue() { 11002 return this.mustHaveValue == null || this.mustHaveValue.isEmpty() ? false : this.mustHaveValue.getValue(); 11003 } 11004 11005 /** 11006 * @param value Specifies for a primitive data type that the value of the data type cannot be replaced by an extension. 11007 */ 11008 public ElementDefinition setMustHaveValue(boolean value) { 11009 if (this.mustHaveValue == null) 11010 this.mustHaveValue = new BooleanType(); 11011 this.mustHaveValue.setValue(value); 11012 return this; 11013 } 11014 11015 /** 11016 * @return {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 11017 */ 11018 public List<CanonicalType> getValueAlternatives() { 11019 if (this.valueAlternatives == null) 11020 this.valueAlternatives = new ArrayList<CanonicalType>(); 11021 return this.valueAlternatives; 11022 } 11023 11024 /** 11025 * @return Returns a reference to <code>this</code> for easy method chaining 11026 */ 11027 public ElementDefinition setValueAlternatives(List<CanonicalType> theValueAlternatives) { 11028 this.valueAlternatives = theValueAlternatives; 11029 return this; 11030 } 11031 11032 public boolean hasValueAlternatives() { 11033 if (this.valueAlternatives == null) 11034 return false; 11035 for (CanonicalType item : this.valueAlternatives) 11036 if (!item.isEmpty()) 11037 return true; 11038 return false; 11039 } 11040 11041 /** 11042 * @return {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 11043 */ 11044 public CanonicalType addValueAlternativesElement() {//2 11045 CanonicalType t = new CanonicalType(); 11046 if (this.valueAlternatives == null) 11047 this.valueAlternatives = new ArrayList<CanonicalType>(); 11048 this.valueAlternatives.add(t); 11049 return t; 11050 } 11051 11052 /** 11053 * @param value {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 11054 */ 11055 public ElementDefinition addValueAlternatives(String value) { //1 11056 CanonicalType t = new CanonicalType(); 11057 t.setValue(value); 11058 if (this.valueAlternatives == null) 11059 this.valueAlternatives = new ArrayList<CanonicalType>(); 11060 this.valueAlternatives.add(t); 11061 return this; 11062 } 11063 11064 /** 11065 * @param value {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 11066 */ 11067 public boolean hasValueAlternatives(String value) { 11068 if (this.valueAlternatives == null) 11069 return false; 11070 for (CanonicalType v : this.valueAlternatives) 11071 if (v.getValue().equals(value)) // canonical 11072 return true; 11073 return false; 11074 } 11075 11076 /** 11077 * @return {@link #mustSupport} (If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.). This is the underlying object with id, value and extensions. The accessor "getMustSupport" gives direct access to the value 11078 */ 11079 public BooleanType getMustSupportElement() { 11080 if (this.mustSupport == null) 11081 if (Configuration.errorOnAutoCreate()) 11082 throw new Error("Attempt to auto-create ElementDefinition.mustSupport"); 11083 else if (Configuration.doAutoCreate()) 11084 this.mustSupport = new BooleanType(); // bb 11085 return this.mustSupport; 11086 } 11087 11088 public boolean hasMustSupportElement() { 11089 return this.mustSupport != null && !this.mustSupport.isEmpty(); 11090 } 11091 11092 public boolean hasMustSupport() { 11093 return this.mustSupport != null && !this.mustSupport.isEmpty(); 11094 } 11095 11096 /** 11097 * @param value {@link #mustSupport} (If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.). This is the underlying object with id, value and extensions. The accessor "getMustSupport" gives direct access to the value 11098 */ 11099 public ElementDefinition setMustSupportElement(BooleanType value) { 11100 this.mustSupport = value; 11101 return this; 11102 } 11103 11104 /** 11105 * @return If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation. 11106 */ 11107 public boolean getMustSupport() { 11108 return this.mustSupport == null || this.mustSupport.isEmpty() ? false : this.mustSupport.getValue(); 11109 } 11110 11111 /** 11112 * @param value If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation. 11113 */ 11114 public ElementDefinition setMustSupport(boolean value) { 11115 if (this.mustSupport == null) 11116 this.mustSupport = new BooleanType(); 11117 this.mustSupport.setValue(value); 11118 return this; 11119 } 11120 11121 /** 11122 * @return {@link #isModifier} (If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.). This is the underlying object with id, value and extensions. The accessor "getIsModifier" gives direct access to the value 11123 */ 11124 public BooleanType getIsModifierElement() { 11125 if (this.isModifier == null) 11126 if (Configuration.errorOnAutoCreate()) 11127 throw new Error("Attempt to auto-create ElementDefinition.isModifier"); 11128 else if (Configuration.doAutoCreate()) 11129 this.isModifier = new BooleanType(); // bb 11130 return this.isModifier; 11131 } 11132 11133 public boolean hasIsModifierElement() { 11134 return this.isModifier != null && !this.isModifier.isEmpty(); 11135 } 11136 11137 public boolean hasIsModifier() { 11138 return this.isModifier != null && !this.isModifier.isEmpty(); 11139 } 11140 11141 /** 11142 * @param value {@link #isModifier} (If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.). This is the underlying object with id, value and extensions. The accessor "getIsModifier" gives direct access to the value 11143 */ 11144 public ElementDefinition setIsModifierElement(BooleanType value) { 11145 this.isModifier = value; 11146 return this; 11147 } 11148 11149 /** 11150 * @return If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension. 11151 */ 11152 public boolean getIsModifier() { 11153 return this.isModifier == null || this.isModifier.isEmpty() ? false : this.isModifier.getValue(); 11154 } 11155 11156 /** 11157 * @param value If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension. 11158 */ 11159 public ElementDefinition setIsModifier(boolean value) { 11160 if (this.isModifier == null) 11161 this.isModifier = new BooleanType(); 11162 this.isModifier.setValue(value); 11163 return this; 11164 } 11165 11166 /** 11167 * @return {@link #isModifierReason} (Explains how that element affects the interpretation of the resource or element that contains it.). This is the underlying object with id, value and extensions. The accessor "getIsModifierReason" gives direct access to the value 11168 */ 11169 public StringType getIsModifierReasonElement() { 11170 if (this.isModifierReason == null) 11171 if (Configuration.errorOnAutoCreate()) 11172 throw new Error("Attempt to auto-create ElementDefinition.isModifierReason"); 11173 else if (Configuration.doAutoCreate()) 11174 this.isModifierReason = new StringType(); // bb 11175 return this.isModifierReason; 11176 } 11177 11178 public boolean hasIsModifierReasonElement() { 11179 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 11180 } 11181 11182 public boolean hasIsModifierReason() { 11183 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 11184 } 11185 11186 /** 11187 * @param value {@link #isModifierReason} (Explains how that element affects the interpretation of the resource or element that contains it.). This is the underlying object with id, value and extensions. The accessor "getIsModifierReason" gives direct access to the value 11188 */ 11189 public ElementDefinition setIsModifierReasonElement(StringType value) { 11190 this.isModifierReason = value; 11191 return this; 11192 } 11193 11194 /** 11195 * @return Explains how that element affects the interpretation of the resource or element that contains it. 11196 */ 11197 public String getIsModifierReason() { 11198 return this.isModifierReason == null ? null : this.isModifierReason.getValue(); 11199 } 11200 11201 /** 11202 * @param value Explains how that element affects the interpretation of the resource or element that contains it. 11203 */ 11204 public ElementDefinition setIsModifierReason(String value) { 11205 if (Utilities.noString(value)) 11206 this.isModifierReason = null; 11207 else { 11208 if (this.isModifierReason == null) 11209 this.isModifierReason = new StringType(); 11210 this.isModifierReason.setValue(value); 11211 } 11212 return this; 11213 } 11214 11215 /** 11216 * @return {@link #isSummary} (Whether the element should be included if a client requests a search with the parameter _summary=true.). This is the underlying object with id, value and extensions. The accessor "getIsSummary" gives direct access to the value 11217 */ 11218 public BooleanType getIsSummaryElement() { 11219 if (this.isSummary == null) 11220 if (Configuration.errorOnAutoCreate()) 11221 throw new Error("Attempt to auto-create ElementDefinition.isSummary"); 11222 else if (Configuration.doAutoCreate()) 11223 this.isSummary = new BooleanType(); // bb 11224 return this.isSummary; 11225 } 11226 11227 public boolean hasIsSummaryElement() { 11228 return this.isSummary != null && !this.isSummary.isEmpty(); 11229 } 11230 11231 public boolean hasIsSummary() { 11232 return this.isSummary != null && !this.isSummary.isEmpty(); 11233 } 11234 11235 /** 11236 * @param value {@link #isSummary} (Whether the element should be included if a client requests a search with the parameter _summary=true.). This is the underlying object with id, value and extensions. The accessor "getIsSummary" gives direct access to the value 11237 */ 11238 public ElementDefinition setIsSummaryElement(BooleanType value) { 11239 this.isSummary = value; 11240 return this; 11241 } 11242 11243 /** 11244 * @return Whether the element should be included if a client requests a search with the parameter _summary=true. 11245 */ 11246 public boolean getIsSummary() { 11247 return this.isSummary == null || this.isSummary.isEmpty() ? false : this.isSummary.getValue(); 11248 } 11249 11250 /** 11251 * @param value Whether the element should be included if a client requests a search with the parameter _summary=true. 11252 */ 11253 public ElementDefinition setIsSummary(boolean value) { 11254 if (this.isSummary == null) 11255 this.isSummary = new BooleanType(); 11256 this.isSummary.setValue(value); 11257 return this; 11258 } 11259 11260 /** 11261 * @return {@link #binding} (Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).) 11262 */ 11263 public ElementDefinitionBindingComponent getBinding() { 11264 if (this.binding == null) 11265 if (Configuration.errorOnAutoCreate()) 11266 throw new Error("Attempt to auto-create ElementDefinition.binding"); 11267 else if (Configuration.doAutoCreate()) 11268 this.binding = new ElementDefinitionBindingComponent(); // cc 11269 return this.binding; 11270 } 11271 11272 public boolean hasBinding() { 11273 return this.binding != null && !this.binding.isEmpty(); 11274 } 11275 11276 /** 11277 * @param value {@link #binding} (Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).) 11278 */ 11279 public ElementDefinition setBinding(ElementDefinitionBindingComponent value) { 11280 this.binding = value; 11281 return this; 11282 } 11283 11284 /** 11285 * @return {@link #mapping} (Identifies a concept from an external specification that roughly corresponds to this element.) 11286 */ 11287 public List<ElementDefinitionMappingComponent> getMapping() { 11288 if (this.mapping == null) 11289 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 11290 return this.mapping; 11291 } 11292 11293 /** 11294 * @return Returns a reference to <code>this</code> for easy method chaining 11295 */ 11296 public ElementDefinition setMapping(List<ElementDefinitionMappingComponent> theMapping) { 11297 this.mapping = theMapping; 11298 return this; 11299 } 11300 11301 public boolean hasMapping() { 11302 if (this.mapping == null) 11303 return false; 11304 for (ElementDefinitionMappingComponent item : this.mapping) 11305 if (!item.isEmpty()) 11306 return true; 11307 return false; 11308 } 11309 11310 public ElementDefinitionMappingComponent addMapping() { //3 11311 ElementDefinitionMappingComponent t = new ElementDefinitionMappingComponent(); 11312 if (this.mapping == null) 11313 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 11314 this.mapping.add(t); 11315 return t; 11316 } 11317 11318 public ElementDefinition addMapping(ElementDefinitionMappingComponent t) { //3 11319 if (t == null) 11320 return this; 11321 if (this.mapping == null) 11322 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 11323 this.mapping.add(t); 11324 return this; 11325 } 11326 11327 /** 11328 * @return The first repetition of repeating field {@link #mapping}, creating it if it does not already exist {3} 11329 */ 11330 public ElementDefinitionMappingComponent getMappingFirstRep() { 11331 if (getMapping().isEmpty()) { 11332 addMapping(); 11333 } 11334 return getMapping().get(0); 11335 } 11336 11337 protected void listChildren(List<Property> children) { 11338 super.listChildren(children); 11339 children.add(new Property("path", "string", "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 0, 1, path)); 11340 children.add(new Property("representation", "code", "Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.", 0, java.lang.Integer.MAX_VALUE, representation)); 11341 children.add(new Property("sliceName", "string", "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 0, 1, sliceName)); 11342 children.add(new Property("sliceIsConstraining", "boolean", "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 0, 1, sliceIsConstraining)); 11343 children.add(new Property("label", "string", "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 0, 1, label)); 11344 children.add(new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 0, java.lang.Integer.MAX_VALUE, code)); 11345 children.add(new Property("slicing", "", "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 0, 1, slicing)); 11346 children.add(new Property("short", "string", "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_)); 11347 children.add(new Property("definition", "markdown", "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 0, 1, definition)); 11348 children.add(new Property("comment", "markdown", "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 0, 1, comment)); 11349 children.add(new Property("requirements", "markdown", "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 0, 1, requirements)); 11350 children.add(new Property("alias", "string", "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, alias)); 11351 children.add(new Property("min", "unsignedInt", "The minimum number of times this element SHALL appear in the instance.", 0, 1, min)); 11352 children.add(new Property("max", "string", "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max)); 11353 children.add(new Property("base", "", "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 0, 1, base)); 11354 children.add(new Property("contentReference", "uri", "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 0, 1, contentReference)); 11355 children.add(new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, java.lang.Integer.MAX_VALUE, type)); 11356 children.add(new Property("defaultValue[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue)); 11357 children.add(new Property("meaningWhenMissing", "markdown", "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 0, 1, meaningWhenMissing)); 11358 children.add(new Property("orderMeaning", "string", "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 0, 1, orderMeaning)); 11359 children.add(new Property("fixed[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed)); 11360 children.add(new Property("pattern[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern)); 11361 children.add(new Property("example", "", "A sample value for this element demonstrating the type of information that would typically be found in the element.", 0, java.lang.Integer.MAX_VALUE, example)); 11362 children.add(new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue)); 11363 children.add(new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue)); 11364 children.add(new Property("maxLength", "integer", "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).", 0, 1, maxLength)); 11365 children.add(new Property("condition", "id", "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 0, java.lang.Integer.MAX_VALUE, condition)); 11366 children.add(new Property("constraint", "", "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 0, java.lang.Integer.MAX_VALUE, constraint)); 11367 children.add(new Property("mustHaveValue", "boolean", "Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.", 0, 1, mustHaveValue)); 11368 children.add(new Property("valueAlternatives", "canonical(StructureDefinition)", "Specifies a list of extensions that can appear in place of a primitive value.", 0, java.lang.Integer.MAX_VALUE, valueAlternatives)); 11369 children.add(new Property("mustSupport", "boolean", "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 0, 1, mustSupport)); 11370 children.add(new Property("isModifier", "boolean", "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.", 0, 1, isModifier)); 11371 children.add(new Property("isModifierReason", "string", "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, isModifierReason)); 11372 children.add(new Property("isSummary", "boolean", "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, isSummary)); 11373 children.add(new Property("binding", "", "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 0, 1, binding)); 11374 children.add(new Property("mapping", "", "Identifies a concept from an external specification that roughly corresponds to this element.", 0, java.lang.Integer.MAX_VALUE, mapping)); 11375 } 11376 11377 @Override 11378 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11379 switch (_hash) { 11380 case 3433509: /*path*/ return new Property("path", "string", "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 0, 1, path); 11381 case -671065907: /*representation*/ return new Property("representation", "code", "Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.", 0, java.lang.Integer.MAX_VALUE, representation); 11382 case -825289923: /*sliceName*/ return new Property("sliceName", "string", "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 0, 1, sliceName); 11383 case 333040519: /*sliceIsConstraining*/ return new Property("sliceIsConstraining", "boolean", "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 0, 1, sliceIsConstraining); 11384 case 102727412: /*label*/ return new Property("label", "string", "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 0, 1, label); 11385 case 3059181: /*code*/ return new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 0, java.lang.Integer.MAX_VALUE, code); 11386 case -2119287345: /*slicing*/ return new Property("slicing", "", "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 0, 1, slicing); 11387 case 109413500: /*short*/ return new Property("short", "string", "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_); 11388 case -1014418093: /*definition*/ return new Property("definition", "markdown", "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 0, 1, definition); 11389 case 950398559: /*comment*/ return new Property("comment", "markdown", "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 0, 1, comment); 11390 case -1619874672: /*requirements*/ return new Property("requirements", "markdown", "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 0, 1, requirements); 11391 case 92902992: /*alias*/ return new Property("alias", "string", "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, alias); 11392 case 108114: /*min*/ return new Property("min", "unsignedInt", "The minimum number of times this element SHALL appear in the instance.", 0, 1, min); 11393 case 107876: /*max*/ return new Property("max", "string", "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max); 11394 case 3016401: /*base*/ return new Property("base", "", "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 0, 1, base); 11395 case 1193747154: /*contentReference*/ return new Property("contentReference", "uri", "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 0, 1, contentReference); 11396 case 3575610: /*type*/ return new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, java.lang.Integer.MAX_VALUE, type); 11397 case 587922128: /*defaultValue[x]*/ return new Property("defaultValue[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11398 case -659125328: /*defaultValue*/ return new Property("defaultValue[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11399 case 1470297600: /*defaultValueBase64Binary*/ return new Property("defaultValue[x]", "base64Binary", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11400 case 600437336: /*defaultValueBoolean*/ return new Property("defaultValue[x]", "boolean", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11401 case 264593188: /*defaultValueCanonical*/ return new Property("defaultValue[x]", "canonical", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11402 case 1044993469: /*defaultValueCode*/ return new Property("defaultValue[x]", "code", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11403 case 1045010302: /*defaultValueDate*/ return new Property("defaultValue[x]", "date", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11404 case 1220374379: /*defaultValueDateTime*/ return new Property("defaultValue[x]", "dateTime", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11405 case 2077989249: /*defaultValueDecimal*/ return new Property("defaultValue[x]", "decimal", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11406 case -2059245333: /*defaultValueId*/ return new Property("defaultValue[x]", "id", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11407 case -1801671663: /*defaultValueInstant*/ return new Property("defaultValue[x]", "instant", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11408 case -1801189522: /*defaultValueInteger*/ return new Property("defaultValue[x]", "integer", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11409 case -71308628: /*defaultValueInteger64*/ return new Property("defaultValue[x]", "integer64", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11410 case -325436225: /*defaultValueMarkdown*/ return new Property("defaultValue[x]", "markdown", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11411 case 587910138: /*defaultValueOid*/ return new Property("defaultValue[x]", "oid", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11412 case -737344154: /*defaultValuePositiveInt*/ return new Property("defaultValue[x]", "positiveInt", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11413 case -320515103: /*defaultValueString*/ return new Property("defaultValue[x]", "string", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11414 case 1045494429: /*defaultValueTime*/ return new Property("defaultValue[x]", "time", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11415 case 539117290: /*defaultValueUnsignedInt*/ return new Property("defaultValue[x]", "unsignedInt", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11416 case 587916188: /*defaultValueUri*/ return new Property("defaultValue[x]", "uri", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11417 case 587916191: /*defaultValueUrl*/ return new Property("defaultValue[x]", "url", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11418 case 1045535627: /*defaultValueUuid*/ return new Property("defaultValue[x]", "uuid", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11419 case -611966428: /*defaultValueAddress*/ return new Property("defaultValue[x]", "Address", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11420 case 587896623: /*defaultValueAge*/ return new Property("defaultValue[x]", "Age", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11421 case -1851689217: /*defaultValueAnnotation*/ return new Property("defaultValue[x]", "Annotation", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11422 case 2034820339: /*defaultValueAttachment*/ return new Property("defaultValue[x]", "Attachment", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11423 case -410434095: /*defaultValueCodeableConcept*/ return new Property("defaultValue[x]", "CodeableConcept", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11424 case 678417524: /*defaultValueCodeableReference*/ return new Property("defaultValue[x]", "CodeableReference", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11425 case -783616198: /*defaultValueCoding*/ return new Property("defaultValue[x]", "Coding", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11426 case -344740576: /*defaultValueContactPoint*/ return new Property("defaultValue[x]", "ContactPoint", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11427 case -1964924097: /*defaultValueCount*/ return new Property("defaultValue[x]", "Count", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11428 case -283915323: /*defaultValueDistance*/ return new Property("defaultValue[x]", "Distance", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11429 case 1730579812: /*defaultValueDuration*/ return new Property("defaultValue[x]", "Duration", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11430 case -975393912: /*defaultValueHumanName*/ return new Property("defaultValue[x]", "HumanName", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11431 case -1915078535: /*defaultValueIdentifier*/ return new Property("defaultValue[x]", "Identifier", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11432 case -1955695888: /*defaultValueMoney*/ return new Property("defaultValue[x]", "Money", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11433 case -420255343: /*defaultValuePeriod*/ return new Property("defaultValue[x]", "Period", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11434 case -1857379237: /*defaultValueQuantity*/ return new Property("defaultValue[x]", "Quantity", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11435 case -1951495315: /*defaultValueRange*/ return new Property("defaultValue[x]", "Range", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11436 case -1951489477: /*defaultValueRatio*/ return new Property("defaultValue[x]", "Ratio", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11437 case 1803932610: /*defaultValueRatioRange*/ return new Property("defaultValue[x]", "RatioRange", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11438 case -1488914053: /*defaultValueReference*/ return new Property("defaultValue[x]", "Reference", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11439 case -449641228: /*defaultValueSampledData*/ return new Property("defaultValue[x]", "SampledData", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11440 case 509825768: /*defaultValueSignature*/ return new Property("defaultValue[x]", "Signature", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11441 case -302193638: /*defaultValueTiming*/ return new Property("defaultValue[x]", "Timing", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11442 case 1845473985: /*defaultValueContactDetail*/ return new Property("defaultValue[x]", "ContactDetail", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11443 case 375217257: /*defaultValueDataRequirement*/ return new Property("defaultValue[x]", "DataRequirement", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11444 case -2092097944: /*defaultValueExpression*/ return new Property("defaultValue[x]", "Expression", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11445 case -701053940: /*defaultValueParameterDefinition*/ return new Property("defaultValue[x]", "ParameterDefinition", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11446 case 412877133: /*defaultValueRelatedArtifact*/ return new Property("defaultValue[x]", "RelatedArtifact", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11447 case 1913203547: /*defaultValueTriggerDefinition*/ return new Property("defaultValue[x]", "TriggerDefinition", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11448 case -701644642: /*defaultValueUsageContext*/ return new Property("defaultValue[x]", "UsageContext", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11449 case 388885803: /*defaultValueAvailability*/ return new Property("defaultValue[x]", "Availability", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11450 case 1398098440: /*defaultValueExtendedContactDetail*/ return new Property("defaultValue[x]", "ExtendedContactDetail", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11451 case -754548089: /*defaultValueDosage*/ return new Property("defaultValue[x]", "Dosage", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11452 case 1045282261: /*defaultValueMeta*/ return new Property("defaultValue[x]", "Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11453 case 1857257103: /*meaningWhenMissing*/ return new Property("meaningWhenMissing", "markdown", "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 0, 1, meaningWhenMissing); 11454 case 1828196047: /*orderMeaning*/ return new Property("orderMeaning", "string", "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 0, 1, orderMeaning); 11455 case -391522164: /*fixed[x]*/ return new Property("fixed[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11456 case 97445748: /*fixed*/ return new Property("fixed[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11457 case -799290428: /*fixedBase64Binary*/ return new Property("fixed[x]", "base64Binary", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11458 case 520851988: /*fixedBoolean*/ return new Property("fixed[x]", "boolean", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11459 case 1092485088: /*fixedCanonical*/ return new Property("fixed[x]", "canonical", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11460 case 746991489: /*fixedCode*/ return new Property("fixed[x]", "code", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11461 case 747008322: /*fixedDate*/ return new Property("fixed[x]", "date", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11462 case -1246771409: /*fixedDateTime*/ return new Property("fixed[x]", "dateTime", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11463 case 1998403901: /*fixedDecimal*/ return new Property("fixed[x]", "decimal", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11464 case -843914321: /*fixedId*/ return new Property("fixed[x]", "id", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11465 case -1881257011: /*fixedInstant*/ return new Property("fixed[x]", "instant", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11466 case -1880774870: /*fixedInteger*/ return new Property("fixed[x]", "integer", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11467 case 756583272: /*fixedInteger64*/ return new Property("fixed[x]", "integer64", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11468 case 1502385283: /*fixedMarkdown*/ return new Property("fixed[x]", "markdown", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11469 case -391534154: /*fixedOid*/ return new Property("fixed[x]", "oid", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11470 case 297821986: /*fixedPositiveInt*/ return new Property("fixed[x]", "positiveInt", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11471 case 1062390949: /*fixedString*/ return new Property("fixed[x]", "string", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11472 case 747492449: /*fixedTime*/ return new Property("fixed[x]", "time", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11473 case 1574283430: /*fixedUnsignedInt*/ return new Property("fixed[x]", "unsignedInt", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11474 case -391528104: /*fixedUri*/ return new Property("fixed[x]", "uri", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11475 case -391528101: /*fixedUrl*/ return new Property("fixed[x]", "url", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11476 case 747533647: /*fixedUuid*/ return new Property("fixed[x]", "uuid", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11477 case -691551776: /*fixedAddress*/ return new Property("fixed[x]", "Address", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11478 case -391547669: /*fixedAge*/ return new Property("fixed[x]", "Age", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11479 case -1956844093: /*fixedAnnotation*/ return new Property("fixed[x]", "Annotation", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11480 case 1929665463: /*fixedAttachment*/ return new Property("fixed[x]", "Attachment", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11481 case 1962764685: /*fixedCodeableConcept*/ return new Property("fixed[x]", "CodeableConcept", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11482 case 694810928: /*fixedCodeableReference*/ return new Property("fixed[x]", "CodeableReference", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11483 case 599289854: /*fixedCoding*/ return new Property("fixed[x]", "Coding", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11484 case 1680638692: /*fixedContactPoint*/ return new Property("fixed[x]", "ContactPoint", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11485 case 1681916411: /*fixedCount*/ return new Property("fixed[x]", "Count", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11486 case 1543906185: /*fixedDistance*/ return new Property("fixed[x]", "Distance", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11487 case -736565976: /*fixedDuration*/ return new Property("fixed[x]", "Duration", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11488 case -147502012: /*fixedHumanName*/ return new Property("fixed[x]", "HumanName", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11489 case -2020233411: /*fixedIdentifier*/ return new Property("fixed[x]", "Identifier", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11490 case 1691144620: /*fixedMoney*/ return new Property("fixed[x]", "Money", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11491 case 962650709: /*fixedPeriod*/ return new Property("fixed[x]", "Period", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11492 case -29557729: /*fixedQuantity*/ return new Property("fixed[x]", "Quantity", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11493 case 1695345193: /*fixedRange*/ return new Property("fixed[x]", "Range", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11494 case 1695351031: /*fixedRatio*/ return new Property("fixed[x]", "Ratio", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11495 case 1698777734: /*fixedRatioRange*/ return new Property("fixed[x]", "RatioRange", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11496 case -661022153: /*fixedReference*/ return new Property("fixed[x]", "Reference", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11497 case 585524912: /*fixedSampledData*/ return new Property("fixed[x]", "SampledData", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11498 case 1337717668: /*fixedSignature*/ return new Property("fixed[x]", "Signature", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11499 case 1080712414: /*fixedTiming*/ return new Property("fixed[x]", "Timing", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11500 case 207721853: /*fixedContactDetail*/ return new Property("fixed[x]", "ContactDetail", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11501 case -1546551259: /*fixedDataRequirement*/ return new Property("fixed[x]", "DataRequirement", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11502 case 2097714476: /*fixedExpression*/ return new Property("fixed[x]", "Expression", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11503 case -2126861880: /*fixedParameterDefinition*/ return new Property("fixed[x]", "ParameterDefinition", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11504 case -1508891383: /*fixedRelatedArtifact*/ return new Property("fixed[x]", "RelatedArtifact", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11505 case 1929596951: /*fixedTriggerDefinition*/ return new Property("fixed[x]", "TriggerDefinition", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11506 case 1323734626: /*fixedUsageContext*/ return new Property("fixed[x]", "UsageContext", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11507 case -1880702225: /*fixedAvailability*/ return new Property("fixed[x]", "Availability", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11508 case 1291235524: /*fixedExtendedContactDetail*/ return new Property("fixed[x]", "ExtendedContactDetail", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11509 case 628357963: /*fixedDosage*/ return new Property("fixed[x]", "Dosage", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11510 case 747280281: /*fixedMeta*/ return new Property("fixed[x]", "Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11511 case -885125392: /*pattern[x]*/ return new Property("pattern[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11512 case -791090288: /*pattern*/ return new Property("pattern[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11513 case 2127857120: /*patternBase64Binary*/ return new Property("pattern[x]", "base64Binary", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11514 case -1776945544: /*patternBoolean*/ return new Property("pattern[x]", "boolean", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11515 case 522246980: /*patternCanonical*/ return new Property("pattern[x]", "canonical", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11516 case -1669806691: /*patternCode*/ return new Property("pattern[x]", "code", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11517 case -1669789858: /*patternDate*/ return new Property("pattern[x]", "date", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11518 case 535949131: /*patternDateTime*/ return new Property("pattern[x]", "dateTime", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11519 case -299393631: /*patternDecimal*/ return new Property("pattern[x]", "decimal", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11520 case -28553013: /*patternId*/ return new Property("pattern[x]", "id", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11521 case 115912753: /*patternInstant*/ return new Property("pattern[x]", "instant", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11522 case 116394894: /*patternInteger*/ return new Property("pattern[x]", "integer", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11523 case 186345164: /*patternInteger64*/ return new Property("pattern[x]", "integer64", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11524 case -1009861473: /*patternMarkdown*/ return new Property("pattern[x]", "markdown", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11525 case -885137382: /*patternOid*/ return new Property("pattern[x]", "oid", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11526 case 2054814086: /*patternPositiveInt*/ return new Property("pattern[x]", "positiveInt", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11527 case 2096647105: /*patternString*/ return new Property("pattern[x]", "string", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11528 case -1669305731: /*patternTime*/ return new Property("pattern[x]", "time", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11529 case -963691766: /*patternUnsignedInt*/ return new Property("pattern[x]", "unsignedInt", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11530 case -885131332: /*patternUri*/ return new Property("pattern[x]", "uri", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11531 case -885131329: /*patternUrl*/ return new Property("pattern[x]", "url", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11532 case -1669264533: /*patternUuid*/ return new Property("pattern[x]", "uuid", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11533 case 1305617988: /*patternAddress*/ return new Property("pattern[x]", "Address", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11534 case -885150897: /*patternAge*/ return new Property("pattern[x]", "Age", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11535 case 1840611039: /*patternAnnotation*/ return new Property("pattern[x]", "Annotation", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11536 case 1432153299: /*patternAttachment*/ return new Property("pattern[x]", "Attachment", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11537 case -400610831: /*patternCodeableConcept*/ return new Property("pattern[x]", "CodeableConcept", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11538 case 1528639636: /*patternCodeableReference*/ return new Property("pattern[x]", "CodeableReference", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11539 case 1633546010: /*patternCoding*/ return new Property("pattern[x]", "Coding", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11540 case 312818944: /*patternContactPoint*/ return new Property("pattern[x]", "ContactPoint", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11541 case -224383137: /*patternCount*/ return new Property("pattern[x]", "Count", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11542 case -968340571: /*patternDistance*/ return new Property("pattern[x]", "Distance", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11543 case 1046154564: /*patternDuration*/ return new Property("pattern[x]", "Duration", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11544 case -717740120: /*patternHumanName*/ return new Property("pattern[x]", "HumanName", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11545 case 1777221721: /*patternIdentifier*/ return new Property("pattern[x]", "Identifier", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11546 case -215154928: /*patternMoney*/ return new Property("pattern[x]", "Money", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11547 case 1996906865: /*patternPeriod*/ return new Property("pattern[x]", "Period", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11548 case 1753162811: /*patternQuantity*/ return new Property("pattern[x]", "Quantity", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11549 case -210954355: /*patternRange*/ return new Property("pattern[x]", "Range", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11550 case -210948517: /*patternRatio*/ return new Property("pattern[x]", "Ratio", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11551 case 1201265570: /*patternRatioRange*/ return new Property("pattern[x]", "RatioRange", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11552 case -1231260261: /*patternReference*/ return new Property("pattern[x]", "Reference", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11553 case -1952450284: /*patternSampledData*/ return new Property("pattern[x]", "SampledData", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11554 case 767479560: /*patternSignature*/ return new Property("pattern[x]", "Signature", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11555 case 2114968570: /*patternTiming*/ return new Property("pattern[x]", "Timing", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11556 case 754982625: /*patternContactDetail*/ return new Property("pattern[x]", "ContactDetail", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11557 case 385040521: /*patternDataRequirement*/ return new Property("pattern[x]", "DataRequirement", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11558 case 1600202312: /*patternExpression*/ return new Property("pattern[x]", "Expression", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11559 case 318609452: /*patternParameterDefinition*/ return new Property("pattern[x]", "ParameterDefinition", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11560 case 422700397: /*patternRelatedArtifact*/ return new Property("pattern[x]", "RelatedArtifact", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11561 case -1531541637: /*patternTriggerDefinition*/ return new Property("pattern[x]", "TriggerDefinition", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11562 case -44085122: /*patternUsageContext*/ return new Property("pattern[x]", "UsageContext", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11563 case 1046445323: /*patternAvailability*/ return new Property("pattern[x]", "Availability", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11564 case 2042074664: /*patternExtendedContactDetail*/ return new Property("pattern[x]", "ExtendedContactDetail", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11565 case 1662614119: /*patternDosage*/ return new Property("pattern[x]", "Dosage", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11566 case -1669517899: /*patternMeta*/ return new Property("pattern[x]", "Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11567 case -1322970774: /*example*/ return new Property("example", "", "A sample value for this element demonstrating the type of information that would typically be found in the element.", 0, java.lang.Integer.MAX_VALUE, example); 11568 case -55301663: /*minValue[x]*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11569 case -1376969153: /*minValue*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11570 case -1715058035: /*minValueDate*/ return new Property("minValue[x]", "date", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11571 case 1635517178: /*minValueDateTime*/ return new Property("minValue[x]", "dateTime", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11572 case 151382690: /*minValueInstant*/ return new Property("minValue[x]", "instant", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11573 case -1714573908: /*minValueTime*/ return new Property("minValue[x]", "time", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11574 case -263923694: /*minValueDecimal*/ return new Property("minValue[x]", "decimal", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11575 case 151864831: /*minValueInteger*/ return new Property("minValue[x]", "integer", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11576 case -86783747: /*minValueInteger64*/ return new Property("minValue[x]", "integer64", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11577 case 1570935671: /*minValuePositiveInt*/ return new Property("minValue[x]", "positiveInt", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11578 case -1447570181: /*minValueUnsignedInt*/ return new Property("minValue[x]", "unsignedInt", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11579 case -1442236438: /*minValueQuantity*/ return new Property("minValue[x]", "Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11580 case 622130931: /*maxValue[x]*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11581 case 399227501: /*maxValue*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11582 case 2105483195: /*maxValueDate*/ return new Property("maxValue[x]", "date", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11583 case 1699385640: /*maxValueDateTime*/ return new Property("maxValue[x]", "dateTime", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11584 case 1261821620: /*maxValueInstant*/ return new Property("maxValue[x]", "instant", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11585 case 2105967322: /*maxValueTime*/ return new Property("maxValue[x]", "time", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11586 case 846515236: /*maxValueDecimal*/ return new Property("maxValue[x]", "decimal", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11587 case 1262303761: /*maxValueInteger*/ return new Property("maxValue[x]", "integer", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11588 case 1893138575: /*maxValueInteger64*/ return new Property("maxValue[x]", "integer64", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11589 case 1605774985: /*maxValuePositiveInt*/ return new Property("maxValue[x]", "positiveInt", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11590 case -1412730867: /*maxValueUnsignedInt*/ return new Property("maxValue[x]", "unsignedInt", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11591 case -1378367976: /*maxValueQuantity*/ return new Property("maxValue[x]", "Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11592 case -791400086: /*maxLength*/ return new Property("maxLength", "integer", "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).", 0, 1, maxLength); 11593 case -861311717: /*condition*/ return new Property("condition", "id", "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 0, java.lang.Integer.MAX_VALUE, condition); 11594 case -190376483: /*constraint*/ return new Property("constraint", "", "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 0, java.lang.Integer.MAX_VALUE, constraint); 11595 case -923694880: /*mustHaveValue*/ return new Property("mustHaveValue", "boolean", "Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.", 0, 1, mustHaveValue); 11596 case -2124672393: /*valueAlternatives*/ return new Property("valueAlternatives", "canonical(StructureDefinition)", "Specifies a list of extensions that can appear in place of a primitive value.", 0, java.lang.Integer.MAX_VALUE, valueAlternatives); 11597 case -1402857082: /*mustSupport*/ return new Property("mustSupport", "boolean", "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 0, 1, mustSupport); 11598 case -1408783839: /*isModifier*/ return new Property("isModifier", "boolean", "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.", 0, 1, isModifier); 11599 case -1854387259: /*isModifierReason*/ return new Property("isModifierReason", "string", "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, isModifierReason); 11600 case 1857548060: /*isSummary*/ return new Property("isSummary", "boolean", "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, isSummary); 11601 case -108220795: /*binding*/ return new Property("binding", "", "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 0, 1, binding); 11602 case 837556430: /*mapping*/ return new Property("mapping", "", "Identifies a concept from an external specification that roughly corresponds to this element.", 0, java.lang.Integer.MAX_VALUE, mapping); 11603 default: return super.getNamedProperty(_hash, _name, _checkValid); 11604 } 11605 11606 } 11607 11608 @Override 11609 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11610 switch (hash) { 11611 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 11612 case -671065907: /*representation*/ return this.representation == null ? new Base[0] : this.representation.toArray(new Base[this.representation.size()]); // Enumeration<PropertyRepresentation> 11613 case -825289923: /*sliceName*/ return this.sliceName == null ? new Base[0] : new Base[] {this.sliceName}; // StringType 11614 case 333040519: /*sliceIsConstraining*/ return this.sliceIsConstraining == null ? new Base[0] : new Base[] {this.sliceIsConstraining}; // BooleanType 11615 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 11616 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 11617 case -2119287345: /*slicing*/ return this.slicing == null ? new Base[0] : new Base[] {this.slicing}; // ElementDefinitionSlicingComponent 11618 case 109413500: /*short*/ return this.short_ == null ? new Base[0] : new Base[] {this.short_}; // StringType 11619 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // MarkdownType 11620 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 11621 case -1619874672: /*requirements*/ return this.requirements == null ? new Base[0] : new Base[] {this.requirements}; // MarkdownType 11622 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 11623 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 11624 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 11625 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // ElementDefinitionBaseComponent 11626 case 1193747154: /*contentReference*/ return this.contentReference == null ? new Base[0] : new Base[] {this.contentReference}; // UriType 11627 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // TypeRefComponent 11628 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // DataType 11629 case 1857257103: /*meaningWhenMissing*/ return this.meaningWhenMissing == null ? new Base[0] : new Base[] {this.meaningWhenMissing}; // MarkdownType 11630 case 1828196047: /*orderMeaning*/ return this.orderMeaning == null ? new Base[0] : new Base[] {this.orderMeaning}; // StringType 11631 case 97445748: /*fixed*/ return this.fixed == null ? new Base[0] : new Base[] {this.fixed}; // DataType 11632 case -791090288: /*pattern*/ return this.pattern == null ? new Base[0] : new Base[] {this.pattern}; // DataType 11633 case -1322970774: /*example*/ return this.example == null ? new Base[0] : this.example.toArray(new Base[this.example.size()]); // ElementDefinitionExampleComponent 11634 case -1376969153: /*minValue*/ return this.minValue == null ? new Base[0] : new Base[] {this.minValue}; // DataType 11635 case 399227501: /*maxValue*/ return this.maxValue == null ? new Base[0] : new Base[] {this.maxValue}; // DataType 11636 case -791400086: /*maxLength*/ return this.maxLength == null ? new Base[0] : new Base[] {this.maxLength}; // IntegerType 11637 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // IdType 11638 case -190376483: /*constraint*/ return this.constraint == null ? new Base[0] : this.constraint.toArray(new Base[this.constraint.size()]); // ElementDefinitionConstraintComponent 11639 case -923694880: /*mustHaveValue*/ return this.mustHaveValue == null ? new Base[0] : new Base[] {this.mustHaveValue}; // BooleanType 11640 case -2124672393: /*valueAlternatives*/ return this.valueAlternatives == null ? new Base[0] : this.valueAlternatives.toArray(new Base[this.valueAlternatives.size()]); // CanonicalType 11641 case -1402857082: /*mustSupport*/ return this.mustSupport == null ? new Base[0] : new Base[] {this.mustSupport}; // BooleanType 11642 case -1408783839: /*isModifier*/ return this.isModifier == null ? new Base[0] : new Base[] {this.isModifier}; // BooleanType 11643 case -1854387259: /*isModifierReason*/ return this.isModifierReason == null ? new Base[0] : new Base[] {this.isModifierReason}; // StringType 11644 case 1857548060: /*isSummary*/ return this.isSummary == null ? new Base[0] : new Base[] {this.isSummary}; // BooleanType 11645 case -108220795: /*binding*/ return this.binding == null ? new Base[0] : new Base[] {this.binding}; // ElementDefinitionBindingComponent 11646 case 837556430: /*mapping*/ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // ElementDefinitionMappingComponent 11647 default: return super.getProperty(hash, name, checkValid); 11648 } 11649 11650 } 11651 11652 @Override 11653 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11654 switch (hash) { 11655 case 3433509: // path 11656 this.path = TypeConvertor.castToString(value); // StringType 11657 return value; 11658 case -671065907: // representation 11659 value = new PropertyRepresentationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11660 this.getRepresentation().add((Enumeration) value); // Enumeration<PropertyRepresentation> 11661 return value; 11662 case -825289923: // sliceName 11663 this.sliceName = TypeConvertor.castToString(value); // StringType 11664 return value; 11665 case 333040519: // sliceIsConstraining 11666 this.sliceIsConstraining = TypeConvertor.castToBoolean(value); // BooleanType 11667 return value; 11668 case 102727412: // label 11669 this.label = TypeConvertor.castToString(value); // StringType 11670 return value; 11671 case 3059181: // code 11672 this.getCode().add(TypeConvertor.castToCoding(value)); // Coding 11673 return value; 11674 case -2119287345: // slicing 11675 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 11676 return value; 11677 case 109413500: // short 11678 this.short_ = TypeConvertor.castToString(value); // StringType 11679 return value; 11680 case -1014418093: // definition 11681 this.definition = TypeConvertor.castToMarkdown(value); // MarkdownType 11682 return value; 11683 case 950398559: // comment 11684 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 11685 return value; 11686 case -1619874672: // requirements 11687 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 11688 return value; 11689 case 92902992: // alias 11690 this.getAlias().add(TypeConvertor.castToString(value)); // StringType 11691 return value; 11692 case 108114: // min 11693 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 11694 return value; 11695 case 107876: // max 11696 this.max = TypeConvertor.castToString(value); // StringType 11697 return value; 11698 case 3016401: // base 11699 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 11700 return value; 11701 case 1193747154: // contentReference 11702 this.contentReference = TypeConvertor.castToUri(value); // UriType 11703 return value; 11704 case 3575610: // type 11705 this.getType().add((TypeRefComponent) value); // TypeRefComponent 11706 return value; 11707 case -659125328: // defaultValue 11708 this.defaultValue = TypeConvertor.castToType(value); // DataType 11709 return value; 11710 case 1857257103: // meaningWhenMissing 11711 this.meaningWhenMissing = TypeConvertor.castToMarkdown(value); // MarkdownType 11712 return value; 11713 case 1828196047: // orderMeaning 11714 this.orderMeaning = TypeConvertor.castToString(value); // StringType 11715 return value; 11716 case 97445748: // fixed 11717 this.fixed = TypeConvertor.castToType(value); // DataType 11718 return value; 11719 case -791090288: // pattern 11720 this.pattern = TypeConvertor.castToType(value); // DataType 11721 return value; 11722 case -1322970774: // example 11723 this.getExample().add((ElementDefinitionExampleComponent) value); // ElementDefinitionExampleComponent 11724 return value; 11725 case -1376969153: // minValue 11726 this.minValue = TypeConvertor.castToType(value); // DataType 11727 return value; 11728 case 399227501: // maxValue 11729 this.maxValue = TypeConvertor.castToType(value); // DataType 11730 return value; 11731 case -791400086: // maxLength 11732 this.maxLength = TypeConvertor.castToInteger(value); // IntegerType 11733 return value; 11734 case -861311717: // condition 11735 this.getCondition().add(TypeConvertor.castToId(value)); // IdType 11736 return value; 11737 case -190376483: // constraint 11738 this.getConstraint().add((ElementDefinitionConstraintComponent) value); // ElementDefinitionConstraintComponent 11739 return value; 11740 case -923694880: // mustHaveValue 11741 this.mustHaveValue = TypeConvertor.castToBoolean(value); // BooleanType 11742 return value; 11743 case -2124672393: // valueAlternatives 11744 this.getValueAlternatives().add(TypeConvertor.castToCanonical(value)); // CanonicalType 11745 return value; 11746 case -1402857082: // mustSupport 11747 this.mustSupport = TypeConvertor.castToBoolean(value); // BooleanType 11748 return value; 11749 case -1408783839: // isModifier 11750 this.isModifier = TypeConvertor.castToBoolean(value); // BooleanType 11751 return value; 11752 case -1854387259: // isModifierReason 11753 this.isModifierReason = TypeConvertor.castToString(value); // StringType 11754 return value; 11755 case 1857548060: // isSummary 11756 this.isSummary = TypeConvertor.castToBoolean(value); // BooleanType 11757 return value; 11758 case -108220795: // binding 11759 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 11760 return value; 11761 case 837556430: // mapping 11762 this.getMapping().add((ElementDefinitionMappingComponent) value); // ElementDefinitionMappingComponent 11763 return value; 11764 default: return super.setProperty(hash, name, value); 11765 } 11766 11767 } 11768 11769 @Override 11770 public Base setProperty(String name, Base value) throws FHIRException { 11771 if (name.equals("path")) { 11772 this.path = TypeConvertor.castToString(value); // StringType 11773 } else if (name.equals("representation")) { 11774 value = new PropertyRepresentationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11775 this.getRepresentation().add((Enumeration) value); 11776 } else if (name.equals("sliceName")) { 11777 this.sliceName = TypeConvertor.castToString(value); // StringType 11778 } else if (name.equals("sliceIsConstraining")) { 11779 this.sliceIsConstraining = TypeConvertor.castToBoolean(value); // BooleanType 11780 } else if (name.equals("label")) { 11781 this.label = TypeConvertor.castToString(value); // StringType 11782 } else if (name.equals("code")) { 11783 this.getCode().add(TypeConvertor.castToCoding(value)); 11784 } else if (name.equals("slicing")) { 11785 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 11786 } else if (name.equals("short")) { 11787 this.short_ = TypeConvertor.castToString(value); // StringType 11788 } else if (name.equals("definition")) { 11789 this.definition = TypeConvertor.castToMarkdown(value); // MarkdownType 11790 } else if (name.equals("comment")) { 11791 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 11792 } else if (name.equals("requirements")) { 11793 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 11794 } else if (name.equals("alias")) { 11795 this.getAlias().add(TypeConvertor.castToString(value)); 11796 } else if (name.equals("min")) { 11797 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 11798 } else if (name.equals("max")) { 11799 this.max = TypeConvertor.castToString(value); // StringType 11800 } else if (name.equals("base")) { 11801 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 11802 } else if (name.equals("contentReference")) { 11803 this.contentReference = TypeConvertor.castToUri(value); // UriType 11804 } else if (name.equals("type")) { 11805 this.getType().add((TypeRefComponent) value); 11806 } else if (name.equals("defaultValue[x]")) { 11807 this.defaultValue = TypeConvertor.castToType(value); // DataType 11808 } else if (name.equals("meaningWhenMissing")) { 11809 this.meaningWhenMissing = TypeConvertor.castToMarkdown(value); // MarkdownType 11810 } else if (name.equals("orderMeaning")) { 11811 this.orderMeaning = TypeConvertor.castToString(value); // StringType 11812 } else if (name.equals("fixed[x]")) { 11813 this.fixed = TypeConvertor.castToType(value); // DataType 11814 } else if (name.equals("pattern[x]")) { 11815 this.pattern = TypeConvertor.castToType(value); // DataType 11816 } else if (name.equals("example")) { 11817 this.getExample().add((ElementDefinitionExampleComponent) value); 11818 } else if (name.equals("minValue[x]")) { 11819 this.minValue = TypeConvertor.castToType(value); // DataType 11820 } else if (name.equals("maxValue[x]")) { 11821 this.maxValue = TypeConvertor.castToType(value); // DataType 11822 } else if (name.equals("maxLength")) { 11823 this.maxLength = TypeConvertor.castToInteger(value); // IntegerType 11824 } else if (name.equals("condition")) { 11825 this.getCondition().add(TypeConvertor.castToId(value)); 11826 } else if (name.equals("constraint")) { 11827 this.getConstraint().add((ElementDefinitionConstraintComponent) value); 11828 } else if (name.equals("mustHaveValue")) { 11829 this.mustHaveValue = TypeConvertor.castToBoolean(value); // BooleanType 11830 } else if (name.equals("valueAlternatives")) { 11831 this.getValueAlternatives().add(TypeConvertor.castToCanonical(value)); 11832 } else if (name.equals("mustSupport")) { 11833 this.mustSupport = TypeConvertor.castToBoolean(value); // BooleanType 11834 } else if (name.equals("isModifier")) { 11835 this.isModifier = TypeConvertor.castToBoolean(value); // BooleanType 11836 } else if (name.equals("isModifierReason")) { 11837 this.isModifierReason = TypeConvertor.castToString(value); // StringType 11838 } else if (name.equals("isSummary")) { 11839 this.isSummary = TypeConvertor.castToBoolean(value); // BooleanType 11840 } else if (name.equals("binding")) { 11841 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 11842 } else if (name.equals("mapping")) { 11843 this.getMapping().add((ElementDefinitionMappingComponent) value); 11844 } else 11845 return super.setProperty(name, value); 11846 return value; 11847 } 11848 11849 @Override 11850 public void removeChild(String name, Base value) throws FHIRException { 11851 if (name.equals("path")) { 11852 this.path = null; 11853 } else if (name.equals("representation")) { 11854 value = new PropertyRepresentationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11855 this.getRepresentation().remove((Enumeration) value); 11856 } else if (name.equals("sliceName")) { 11857 this.sliceName = null; 11858 } else if (name.equals("sliceIsConstraining")) { 11859 this.sliceIsConstraining = null; 11860 } else if (name.equals("label")) { 11861 this.label = null; 11862 } else if (name.equals("code")) { 11863 this.getCode().remove(value); 11864 } else if (name.equals("slicing")) { 11865 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 11866 } else if (name.equals("short")) { 11867 this.short_ = null; 11868 } else if (name.equals("definition")) { 11869 this.definition = null; 11870 } else if (name.equals("comment")) { 11871 this.comment = null; 11872 } else if (name.equals("requirements")) { 11873 this.requirements = null; 11874 } else if (name.equals("alias")) { 11875 this.getAlias().remove(value); 11876 } else if (name.equals("min")) { 11877 this.min = null; 11878 } else if (name.equals("max")) { 11879 this.max = null; 11880 } else if (name.equals("base")) { 11881 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 11882 } else if (name.equals("contentReference")) { 11883 this.contentReference = null; 11884 } else if (name.equals("type")) { 11885 this.getType().remove((TypeRefComponent) value); 11886 } else if (name.equals("defaultValue[x]")) { 11887 this.defaultValue = null; 11888 } else if (name.equals("meaningWhenMissing")) { 11889 this.meaningWhenMissing = null; 11890 } else if (name.equals("orderMeaning")) { 11891 this.orderMeaning = null; 11892 } else if (name.equals("fixed[x]")) { 11893 this.fixed = null; 11894 } else if (name.equals("pattern[x]")) { 11895 this.pattern = null; 11896 } else if (name.equals("example")) { 11897 this.getExample().remove((ElementDefinitionExampleComponent) value); 11898 } else if (name.equals("minValue[x]")) { 11899 this.minValue = null; 11900 } else if (name.equals("maxValue[x]")) { 11901 this.maxValue = null; 11902 } else if (name.equals("maxLength")) { 11903 this.maxLength = null; 11904 } else if (name.equals("condition")) { 11905 this.getCondition().remove(value); 11906 } else if (name.equals("constraint")) { 11907 this.getConstraint().remove((ElementDefinitionConstraintComponent) value); 11908 } else if (name.equals("mustHaveValue")) { 11909 this.mustHaveValue = null; 11910 } else if (name.equals("valueAlternatives")) { 11911 this.getValueAlternatives().remove(value); 11912 } else if (name.equals("mustSupport")) { 11913 this.mustSupport = null; 11914 } else if (name.equals("isModifier")) { 11915 this.isModifier = null; 11916 } else if (name.equals("isModifierReason")) { 11917 this.isModifierReason = null; 11918 } else if (name.equals("isSummary")) { 11919 this.isSummary = null; 11920 } else if (name.equals("binding")) { 11921 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 11922 } else if (name.equals("mapping")) { 11923 this.getMapping().remove((ElementDefinitionMappingComponent) value); 11924 } else 11925 super.removeChild(name, value); 11926 11927 } 11928 11929 @Override 11930 public Base makeProperty(int hash, String name) throws FHIRException { 11931 switch (hash) { 11932 case 3433509: return getPathElement(); 11933 case -671065907: return addRepresentationElement(); 11934 case -825289923: return getSliceNameElement(); 11935 case 333040519: return getSliceIsConstrainingElement(); 11936 case 102727412: return getLabelElement(); 11937 case 3059181: return addCode(); 11938 case -2119287345: return getSlicing(); 11939 case 109413500: return getShortElement(); 11940 case -1014418093: return getDefinitionElement(); 11941 case 950398559: return getCommentElement(); 11942 case -1619874672: return getRequirementsElement(); 11943 case 92902992: return addAliasElement(); 11944 case 108114: return getMinElement(); 11945 case 107876: return getMaxElement(); 11946 case 3016401: return getBase(); 11947 case 1193747154: return getContentReferenceElement(); 11948 case 3575610: return addType(); 11949 case 587922128: return getDefaultValue(); 11950 case -659125328: return getDefaultValue(); 11951 case 1857257103: return getMeaningWhenMissingElement(); 11952 case 1828196047: return getOrderMeaningElement(); 11953 case -391522164: return getFixed(); 11954 case 97445748: return getFixed(); 11955 case -885125392: return getPattern(); 11956 case -791090288: return getPattern(); 11957 case -1322970774: return addExample(); 11958 case -55301663: return getMinValue(); 11959 case -1376969153: return getMinValue(); 11960 case 622130931: return getMaxValue(); 11961 case 399227501: return getMaxValue(); 11962 case -791400086: return getMaxLengthElement(); 11963 case -861311717: return addConditionElement(); 11964 case -190376483: return addConstraint(); 11965 case -923694880: return getMustHaveValueElement(); 11966 case -2124672393: return addValueAlternativesElement(); 11967 case -1402857082: return getMustSupportElement(); 11968 case -1408783839: return getIsModifierElement(); 11969 case -1854387259: return getIsModifierReasonElement(); 11970 case 1857548060: return getIsSummaryElement(); 11971 case -108220795: return getBinding(); 11972 case 837556430: return addMapping(); 11973 default: return super.makeProperty(hash, name); 11974 } 11975 11976 } 11977 11978 @Override 11979 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11980 switch (hash) { 11981 case 3433509: /*path*/ return new String[] {"string"}; 11982 case -671065907: /*representation*/ return new String[] {"code"}; 11983 case -825289923: /*sliceName*/ return new String[] {"string"}; 11984 case 333040519: /*sliceIsConstraining*/ return new String[] {"boolean"}; 11985 case 102727412: /*label*/ return new String[] {"string"}; 11986 case 3059181: /*code*/ return new String[] {"Coding"}; 11987 case -2119287345: /*slicing*/ return new String[] {}; 11988 case 109413500: /*short*/ return new String[] {"string"}; 11989 case -1014418093: /*definition*/ return new String[] {"markdown"}; 11990 case 950398559: /*comment*/ return new String[] {"markdown"}; 11991 case -1619874672: /*requirements*/ return new String[] {"markdown"}; 11992 case 92902992: /*alias*/ return new String[] {"string"}; 11993 case 108114: /*min*/ return new String[] {"unsignedInt"}; 11994 case 107876: /*max*/ return new String[] {"string"}; 11995 case 3016401: /*base*/ return new String[] {}; 11996 case 1193747154: /*contentReference*/ return new String[] {"uri"}; 11997 case 3575610: /*type*/ return new String[] {}; 11998 case -659125328: /*defaultValue*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 11999 case 1857257103: /*meaningWhenMissing*/ return new String[] {"markdown"}; 12000 case 1828196047: /*orderMeaning*/ return new String[] {"string"}; 12001 case 97445748: /*fixed*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 12002 case -791090288: /*pattern*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 12003 case -1322970774: /*example*/ return new String[] {}; 12004 case -1376969153: /*minValue*/ return new String[] {"date", "dateTime", "instant", "time", "decimal", "integer", "integer64", "positiveInt", "unsignedInt", "Quantity"}; 12005 case 399227501: /*maxValue*/ return new String[] {"date", "dateTime", "instant", "time", "decimal", "integer", "integer64", "positiveInt", "unsignedInt", "Quantity"}; 12006 case -791400086: /*maxLength*/ return new String[] {"integer"}; 12007 case -861311717: /*condition*/ return new String[] {"id"}; 12008 case -190376483: /*constraint*/ return new String[] {}; 12009 case -923694880: /*mustHaveValue*/ return new String[] {"boolean"}; 12010 case -2124672393: /*valueAlternatives*/ return new String[] {"canonical"}; 12011 case -1402857082: /*mustSupport*/ return new String[] {"boolean"}; 12012 case -1408783839: /*isModifier*/ return new String[] {"boolean"}; 12013 case -1854387259: /*isModifierReason*/ return new String[] {"string"}; 12014 case 1857548060: /*isSummary*/ return new String[] {"boolean"}; 12015 case -108220795: /*binding*/ return new String[] {}; 12016 case 837556430: /*mapping*/ return new String[] {}; 12017 default: return super.getTypesForProperty(hash, name); 12018 } 12019 12020 } 12021 12022 @Override 12023 public Base addChild(String name) throws FHIRException { 12024 if (name.equals("path")) { 12025 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 12026 } 12027 else if (name.equals("representation")) { 12028 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.representation"); 12029 } 12030 else if (name.equals("sliceName")) { 12031 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceName"); 12032 } 12033 else if (name.equals("sliceIsConstraining")) { 12034 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceIsConstraining"); 12035 } 12036 else if (name.equals("label")) { 12037 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 12038 } 12039 else if (name.equals("code")) { 12040 return addCode(); 12041 } 12042 else if (name.equals("slicing")) { 12043 this.slicing = new ElementDefinitionSlicingComponent(); 12044 return this.slicing; 12045 } 12046 else if (name.equals("short")) { 12047 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.short"); 12048 } 12049 else if (name.equals("definition")) { 12050 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.definition"); 12051 } 12052 else if (name.equals("comment")) { 12053 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 12054 } 12055 else if (name.equals("requirements")) { 12056 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 12057 } 12058 else if (name.equals("alias")) { 12059 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.alias"); 12060 } 12061 else if (name.equals("min")) { 12062 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 12063 } 12064 else if (name.equals("max")) { 12065 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 12066 } 12067 else if (name.equals("base")) { 12068 this.base = new ElementDefinitionBaseComponent(); 12069 return this.base; 12070 } 12071 else if (name.equals("contentReference")) { 12072 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.contentReference"); 12073 } 12074 else if (name.equals("type")) { 12075 return addType(); 12076 } 12077 else if (name.equals("defaultValueBase64Binary")) { 12078 this.defaultValue = new Base64BinaryType(); 12079 return this.defaultValue; 12080 } 12081 else if (name.equals("defaultValueBoolean")) { 12082 this.defaultValue = new BooleanType(); 12083 return this.defaultValue; 12084 } 12085 else if (name.equals("defaultValueCanonical")) { 12086 this.defaultValue = new CanonicalType(); 12087 return this.defaultValue; 12088 } 12089 else if (name.equals("defaultValueCode")) { 12090 this.defaultValue = new CodeType(); 12091 return this.defaultValue; 12092 } 12093 else if (name.equals("defaultValueDate")) { 12094 this.defaultValue = new DateType(); 12095 return this.defaultValue; 12096 } 12097 else if (name.equals("defaultValueDateTime")) { 12098 this.defaultValue = new DateTimeType(); 12099 return this.defaultValue; 12100 } 12101 else if (name.equals("defaultValueDecimal")) { 12102 this.defaultValue = new DecimalType(); 12103 return this.defaultValue; 12104 } 12105 else if (name.equals("defaultValueId")) { 12106 this.defaultValue = new IdType(); 12107 return this.defaultValue; 12108 } 12109 else if (name.equals("defaultValueInstant")) { 12110 this.defaultValue = new InstantType(); 12111 return this.defaultValue; 12112 } 12113 else if (name.equals("defaultValueInteger")) { 12114 this.defaultValue = new IntegerType(); 12115 return this.defaultValue; 12116 } 12117 else if (name.equals("defaultValueInteger64")) { 12118 this.defaultValue = new Integer64Type(); 12119 return this.defaultValue; 12120 } 12121 else if (name.equals("defaultValueMarkdown")) { 12122 this.defaultValue = new MarkdownType(); 12123 return this.defaultValue; 12124 } 12125 else if (name.equals("defaultValueOid")) { 12126 this.defaultValue = new OidType(); 12127 return this.defaultValue; 12128 } 12129 else if (name.equals("defaultValuePositiveInt")) { 12130 this.defaultValue = new PositiveIntType(); 12131 return this.defaultValue; 12132 } 12133 else if (name.equals("defaultValueString")) { 12134 this.defaultValue = new StringType(); 12135 return this.defaultValue; 12136 } 12137 else if (name.equals("defaultValueTime")) { 12138 this.defaultValue = new TimeType(); 12139 return this.defaultValue; 12140 } 12141 else if (name.equals("defaultValueUnsignedInt")) { 12142 this.defaultValue = new UnsignedIntType(); 12143 return this.defaultValue; 12144 } 12145 else if (name.equals("defaultValueUri")) { 12146 this.defaultValue = new UriType(); 12147 return this.defaultValue; 12148 } 12149 else if (name.equals("defaultValueUrl")) { 12150 this.defaultValue = new UrlType(); 12151 return this.defaultValue; 12152 } 12153 else if (name.equals("defaultValueUuid")) { 12154 this.defaultValue = new UuidType(); 12155 return this.defaultValue; 12156 } 12157 else if (name.equals("defaultValueAddress")) { 12158 this.defaultValue = new Address(); 12159 return this.defaultValue; 12160 } 12161 else if (name.equals("defaultValueAge")) { 12162 this.defaultValue = new Age(); 12163 return this.defaultValue; 12164 } 12165 else if (name.equals("defaultValueAnnotation")) { 12166 this.defaultValue = new Annotation(); 12167 return this.defaultValue; 12168 } 12169 else if (name.equals("defaultValueAttachment")) { 12170 this.defaultValue = new Attachment(); 12171 return this.defaultValue; 12172 } 12173 else if (name.equals("defaultValueCodeableConcept")) { 12174 this.defaultValue = new CodeableConcept(); 12175 return this.defaultValue; 12176 } 12177 else if (name.equals("defaultValueCodeableReference")) { 12178 this.defaultValue = new CodeableReference(); 12179 return this.defaultValue; 12180 } 12181 else if (name.equals("defaultValueCoding")) { 12182 this.defaultValue = new Coding(); 12183 return this.defaultValue; 12184 } 12185 else if (name.equals("defaultValueContactPoint")) { 12186 this.defaultValue = new ContactPoint(); 12187 return this.defaultValue; 12188 } 12189 else if (name.equals("defaultValueCount")) { 12190 this.defaultValue = new Count(); 12191 return this.defaultValue; 12192 } 12193 else if (name.equals("defaultValueDistance")) { 12194 this.defaultValue = new Distance(); 12195 return this.defaultValue; 12196 } 12197 else if (name.equals("defaultValueDuration")) { 12198 this.defaultValue = new Duration(); 12199 return this.defaultValue; 12200 } 12201 else if (name.equals("defaultValueHumanName")) { 12202 this.defaultValue = new HumanName(); 12203 return this.defaultValue; 12204 } 12205 else if (name.equals("defaultValueIdentifier")) { 12206 this.defaultValue = new Identifier(); 12207 return this.defaultValue; 12208 } 12209 else if (name.equals("defaultValueMoney")) { 12210 this.defaultValue = new Money(); 12211 return this.defaultValue; 12212 } 12213 else if (name.equals("defaultValuePeriod")) { 12214 this.defaultValue = new Period(); 12215 return this.defaultValue; 12216 } 12217 else if (name.equals("defaultValueQuantity")) { 12218 this.defaultValue = new Quantity(); 12219 return this.defaultValue; 12220 } 12221 else if (name.equals("defaultValueRange")) { 12222 this.defaultValue = new Range(); 12223 return this.defaultValue; 12224 } 12225 else if (name.equals("defaultValueRatio")) { 12226 this.defaultValue = new Ratio(); 12227 return this.defaultValue; 12228 } 12229 else if (name.equals("defaultValueRatioRange")) { 12230 this.defaultValue = new RatioRange(); 12231 return this.defaultValue; 12232 } 12233 else if (name.equals("defaultValueReference")) { 12234 this.defaultValue = new Reference(); 12235 return this.defaultValue; 12236 } 12237 else if (name.equals("defaultValueSampledData")) { 12238 this.defaultValue = new SampledData(); 12239 return this.defaultValue; 12240 } 12241 else if (name.equals("defaultValueSignature")) { 12242 this.defaultValue = new Signature(); 12243 return this.defaultValue; 12244 } 12245 else if (name.equals("defaultValueTiming")) { 12246 this.defaultValue = new Timing(); 12247 return this.defaultValue; 12248 } 12249 else if (name.equals("defaultValueContactDetail")) { 12250 this.defaultValue = new ContactDetail(); 12251 return this.defaultValue; 12252 } 12253 else if (name.equals("defaultValueDataRequirement")) { 12254 this.defaultValue = new DataRequirement(); 12255 return this.defaultValue; 12256 } 12257 else if (name.equals("defaultValueExpression")) { 12258 this.defaultValue = new Expression(); 12259 return this.defaultValue; 12260 } 12261 else if (name.equals("defaultValueParameterDefinition")) { 12262 this.defaultValue = new ParameterDefinition(); 12263 return this.defaultValue; 12264 } 12265 else if (name.equals("defaultValueRelatedArtifact")) { 12266 this.defaultValue = new RelatedArtifact(); 12267 return this.defaultValue; 12268 } 12269 else if (name.equals("defaultValueTriggerDefinition")) { 12270 this.defaultValue = new TriggerDefinition(); 12271 return this.defaultValue; 12272 } 12273 else if (name.equals("defaultValueUsageContext")) { 12274 this.defaultValue = new UsageContext(); 12275 return this.defaultValue; 12276 } 12277 else if (name.equals("defaultValueAvailability")) { 12278 this.defaultValue = new Availability(); 12279 return this.defaultValue; 12280 } 12281 else if (name.equals("defaultValueExtendedContactDetail")) { 12282 this.defaultValue = new ExtendedContactDetail(); 12283 return this.defaultValue; 12284 } 12285 else if (name.equals("defaultValueDosage")) { 12286 this.defaultValue = new Dosage(); 12287 return this.defaultValue; 12288 } 12289 else if (name.equals("defaultValueMeta")) { 12290 this.defaultValue = new Meta(); 12291 return this.defaultValue; 12292 } 12293 else if (name.equals("meaningWhenMissing")) { 12294 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.meaningWhenMissing"); 12295 } 12296 else if (name.equals("orderMeaning")) { 12297 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.orderMeaning"); 12298 } 12299 else if (name.equals("fixedBase64Binary")) { 12300 this.fixed = new Base64BinaryType(); 12301 return this.fixed; 12302 } 12303 else if (name.equals("fixedBoolean")) { 12304 this.fixed = new BooleanType(); 12305 return this.fixed; 12306 } 12307 else if (name.equals("fixedCanonical")) { 12308 this.fixed = new CanonicalType(); 12309 return this.fixed; 12310 } 12311 else if (name.equals("fixedCode")) { 12312 this.fixed = new CodeType(); 12313 return this.fixed; 12314 } 12315 else if (name.equals("fixedDate")) { 12316 this.fixed = new DateType(); 12317 return this.fixed; 12318 } 12319 else if (name.equals("fixedDateTime")) { 12320 this.fixed = new DateTimeType(); 12321 return this.fixed; 12322 } 12323 else if (name.equals("fixedDecimal")) { 12324 this.fixed = new DecimalType(); 12325 return this.fixed; 12326 } 12327 else if (name.equals("fixedId")) { 12328 this.fixed = new IdType(); 12329 return this.fixed; 12330 } 12331 else if (name.equals("fixedInstant")) { 12332 this.fixed = new InstantType(); 12333 return this.fixed; 12334 } 12335 else if (name.equals("fixedInteger")) { 12336 this.fixed = new IntegerType(); 12337 return this.fixed; 12338 } 12339 else if (name.equals("fixedInteger64")) { 12340 this.fixed = new Integer64Type(); 12341 return this.fixed; 12342 } 12343 else if (name.equals("fixedMarkdown")) { 12344 this.fixed = new MarkdownType(); 12345 return this.fixed; 12346 } 12347 else if (name.equals("fixedOid")) { 12348 this.fixed = new OidType(); 12349 return this.fixed; 12350 } 12351 else if (name.equals("fixedPositiveInt")) { 12352 this.fixed = new PositiveIntType(); 12353 return this.fixed; 12354 } 12355 else if (name.equals("fixedString")) { 12356 this.fixed = new StringType(); 12357 return this.fixed; 12358 } 12359 else if (name.equals("fixedTime")) { 12360 this.fixed = new TimeType(); 12361 return this.fixed; 12362 } 12363 else if (name.equals("fixedUnsignedInt")) { 12364 this.fixed = new UnsignedIntType(); 12365 return this.fixed; 12366 } 12367 else if (name.equals("fixedUri")) { 12368 this.fixed = new UriType(); 12369 return this.fixed; 12370 } 12371 else if (name.equals("fixedUrl")) { 12372 this.fixed = new UrlType(); 12373 return this.fixed; 12374 } 12375 else if (name.equals("fixedUuid")) { 12376 this.fixed = new UuidType(); 12377 return this.fixed; 12378 } 12379 else if (name.equals("fixedAddress")) { 12380 this.fixed = new Address(); 12381 return this.fixed; 12382 } 12383 else if (name.equals("fixedAge")) { 12384 this.fixed = new Age(); 12385 return this.fixed; 12386 } 12387 else if (name.equals("fixedAnnotation")) { 12388 this.fixed = new Annotation(); 12389 return this.fixed; 12390 } 12391 else if (name.equals("fixedAttachment")) { 12392 this.fixed = new Attachment(); 12393 return this.fixed; 12394 } 12395 else if (name.equals("fixedCodeableConcept")) { 12396 this.fixed = new CodeableConcept(); 12397 return this.fixed; 12398 } 12399 else if (name.equals("fixedCodeableReference")) { 12400 this.fixed = new CodeableReference(); 12401 return this.fixed; 12402 } 12403 else if (name.equals("fixedCoding")) { 12404 this.fixed = new Coding(); 12405 return this.fixed; 12406 } 12407 else if (name.equals("fixedContactPoint")) { 12408 this.fixed = new ContactPoint(); 12409 return this.fixed; 12410 } 12411 else if (name.equals("fixedCount")) { 12412 this.fixed = new Count(); 12413 return this.fixed; 12414 } 12415 else if (name.equals("fixedDistance")) { 12416 this.fixed = new Distance(); 12417 return this.fixed; 12418 } 12419 else if (name.equals("fixedDuration")) { 12420 this.fixed = new Duration(); 12421 return this.fixed; 12422 } 12423 else if (name.equals("fixedHumanName")) { 12424 this.fixed = new HumanName(); 12425 return this.fixed; 12426 } 12427 else if (name.equals("fixedIdentifier")) { 12428 this.fixed = new Identifier(); 12429 return this.fixed; 12430 } 12431 else if (name.equals("fixedMoney")) { 12432 this.fixed = new Money(); 12433 return this.fixed; 12434 } 12435 else if (name.equals("fixedPeriod")) { 12436 this.fixed = new Period(); 12437 return this.fixed; 12438 } 12439 else if (name.equals("fixedQuantity")) { 12440 this.fixed = new Quantity(); 12441 return this.fixed; 12442 } 12443 else if (name.equals("fixedRange")) { 12444 this.fixed = new Range(); 12445 return this.fixed; 12446 } 12447 else if (name.equals("fixedRatio")) { 12448 this.fixed = new Ratio(); 12449 return this.fixed; 12450 } 12451 else if (name.equals("fixedRatioRange")) { 12452 this.fixed = new RatioRange(); 12453 return this.fixed; 12454 } 12455 else if (name.equals("fixedReference")) { 12456 this.fixed = new Reference(); 12457 return this.fixed; 12458 } 12459 else if (name.equals("fixedSampledData")) { 12460 this.fixed = new SampledData(); 12461 return this.fixed; 12462 } 12463 else if (name.equals("fixedSignature")) { 12464 this.fixed = new Signature(); 12465 return this.fixed; 12466 } 12467 else if (name.equals("fixedTiming")) { 12468 this.fixed = new Timing(); 12469 return this.fixed; 12470 } 12471 else if (name.equals("fixedContactDetail")) { 12472 this.fixed = new ContactDetail(); 12473 return this.fixed; 12474 } 12475 else if (name.equals("fixedDataRequirement")) { 12476 this.fixed = new DataRequirement(); 12477 return this.fixed; 12478 } 12479 else if (name.equals("fixedExpression")) { 12480 this.fixed = new Expression(); 12481 return this.fixed; 12482 } 12483 else if (name.equals("fixedParameterDefinition")) { 12484 this.fixed = new ParameterDefinition(); 12485 return this.fixed; 12486 } 12487 else if (name.equals("fixedRelatedArtifact")) { 12488 this.fixed = new RelatedArtifact(); 12489 return this.fixed; 12490 } 12491 else if (name.equals("fixedTriggerDefinition")) { 12492 this.fixed = new TriggerDefinition(); 12493 return this.fixed; 12494 } 12495 else if (name.equals("fixedUsageContext")) { 12496 this.fixed = new UsageContext(); 12497 return this.fixed; 12498 } 12499 else if (name.equals("fixedAvailability")) { 12500 this.fixed = new Availability(); 12501 return this.fixed; 12502 } 12503 else if (name.equals("fixedExtendedContactDetail")) { 12504 this.fixed = new ExtendedContactDetail(); 12505 return this.fixed; 12506 } 12507 else if (name.equals("fixedDosage")) { 12508 this.fixed = new Dosage(); 12509 return this.fixed; 12510 } 12511 else if (name.equals("fixedMeta")) { 12512 this.fixed = new Meta(); 12513 return this.fixed; 12514 } 12515 else if (name.equals("patternBase64Binary")) { 12516 this.pattern = new Base64BinaryType(); 12517 return this.pattern; 12518 } 12519 else if (name.equals("patternBoolean")) { 12520 this.pattern = new BooleanType(); 12521 return this.pattern; 12522 } 12523 else if (name.equals("patternCanonical")) { 12524 this.pattern = new CanonicalType(); 12525 return this.pattern; 12526 } 12527 else if (name.equals("patternCode")) { 12528 this.pattern = new CodeType(); 12529 return this.pattern; 12530 } 12531 else if (name.equals("patternDate")) { 12532 this.pattern = new DateType(); 12533 return this.pattern; 12534 } 12535 else if (name.equals("patternDateTime")) { 12536 this.pattern = new DateTimeType(); 12537 return this.pattern; 12538 } 12539 else if (name.equals("patternDecimal")) { 12540 this.pattern = new DecimalType(); 12541 return this.pattern; 12542 } 12543 else if (name.equals("patternId")) { 12544 this.pattern = new IdType(); 12545 return this.pattern; 12546 } 12547 else if (name.equals("patternInstant")) { 12548 this.pattern = new InstantType(); 12549 return this.pattern; 12550 } 12551 else if (name.equals("patternInteger")) { 12552 this.pattern = new IntegerType(); 12553 return this.pattern; 12554 } 12555 else if (name.equals("patternInteger64")) { 12556 this.pattern = new Integer64Type(); 12557 return this.pattern; 12558 } 12559 else if (name.equals("patternMarkdown")) { 12560 this.pattern = new MarkdownType(); 12561 return this.pattern; 12562 } 12563 else if (name.equals("patternOid")) { 12564 this.pattern = new OidType(); 12565 return this.pattern; 12566 } 12567 else if (name.equals("patternPositiveInt")) { 12568 this.pattern = new PositiveIntType(); 12569 return this.pattern; 12570 } 12571 else if (name.equals("patternString")) { 12572 this.pattern = new StringType(); 12573 return this.pattern; 12574 } 12575 else if (name.equals("patternTime")) { 12576 this.pattern = new TimeType(); 12577 return this.pattern; 12578 } 12579 else if (name.equals("patternUnsignedInt")) { 12580 this.pattern = new UnsignedIntType(); 12581 return this.pattern; 12582 } 12583 else if (name.equals("patternUri")) { 12584 this.pattern = new UriType(); 12585 return this.pattern; 12586 } 12587 else if (name.equals("patternUrl")) { 12588 this.pattern = new UrlType(); 12589 return this.pattern; 12590 } 12591 else if (name.equals("patternUuid")) { 12592 this.pattern = new UuidType(); 12593 return this.pattern; 12594 } 12595 else if (name.equals("patternAddress")) { 12596 this.pattern = new Address(); 12597 return this.pattern; 12598 } 12599 else if (name.equals("patternAge")) { 12600 this.pattern = new Age(); 12601 return this.pattern; 12602 } 12603 else if (name.equals("patternAnnotation")) { 12604 this.pattern = new Annotation(); 12605 return this.pattern; 12606 } 12607 else if (name.equals("patternAttachment")) { 12608 this.pattern = new Attachment(); 12609 return this.pattern; 12610 } 12611 else if (name.equals("patternCodeableConcept")) { 12612 this.pattern = new CodeableConcept(); 12613 return this.pattern; 12614 } 12615 else if (name.equals("patternCodeableReference")) { 12616 this.pattern = new CodeableReference(); 12617 return this.pattern; 12618 } 12619 else if (name.equals("patternCoding")) { 12620 this.pattern = new Coding(); 12621 return this.pattern; 12622 } 12623 else if (name.equals("patternContactPoint")) { 12624 this.pattern = new ContactPoint(); 12625 return this.pattern; 12626 } 12627 else if (name.equals("patternCount")) { 12628 this.pattern = new Count(); 12629 return this.pattern; 12630 } 12631 else if (name.equals("patternDistance")) { 12632 this.pattern = new Distance(); 12633 return this.pattern; 12634 } 12635 else if (name.equals("patternDuration")) { 12636 this.pattern = new Duration(); 12637 return this.pattern; 12638 } 12639 else if (name.equals("patternHumanName")) { 12640 this.pattern = new HumanName(); 12641 return this.pattern; 12642 } 12643 else if (name.equals("patternIdentifier")) { 12644 this.pattern = new Identifier(); 12645 return this.pattern; 12646 } 12647 else if (name.equals("patternMoney")) { 12648 this.pattern = new Money(); 12649 return this.pattern; 12650 } 12651 else if (name.equals("patternPeriod")) { 12652 this.pattern = new Period(); 12653 return this.pattern; 12654 } 12655 else if (name.equals("patternQuantity")) { 12656 this.pattern = new Quantity(); 12657 return this.pattern; 12658 } 12659 else if (name.equals("patternRange")) { 12660 this.pattern = new Range(); 12661 return this.pattern; 12662 } 12663 else if (name.equals("patternRatio")) { 12664 this.pattern = new Ratio(); 12665 return this.pattern; 12666 } 12667 else if (name.equals("patternRatioRange")) { 12668 this.pattern = new RatioRange(); 12669 return this.pattern; 12670 } 12671 else if (name.equals("patternReference")) { 12672 this.pattern = new Reference(); 12673 return this.pattern; 12674 } 12675 else if (name.equals("patternSampledData")) { 12676 this.pattern = new SampledData(); 12677 return this.pattern; 12678 } 12679 else if (name.equals("patternSignature")) { 12680 this.pattern = new Signature(); 12681 return this.pattern; 12682 } 12683 else if (name.equals("patternTiming")) { 12684 this.pattern = new Timing(); 12685 return this.pattern; 12686 } 12687 else if (name.equals("patternContactDetail")) { 12688 this.pattern = new ContactDetail(); 12689 return this.pattern; 12690 } 12691 else if (name.equals("patternDataRequirement")) { 12692 this.pattern = new DataRequirement(); 12693 return this.pattern; 12694 } 12695 else if (name.equals("patternExpression")) { 12696 this.pattern = new Expression(); 12697 return this.pattern; 12698 } 12699 else if (name.equals("patternParameterDefinition")) { 12700 this.pattern = new ParameterDefinition(); 12701 return this.pattern; 12702 } 12703 else if (name.equals("patternRelatedArtifact")) { 12704 this.pattern = new RelatedArtifact(); 12705 return this.pattern; 12706 } 12707 else if (name.equals("patternTriggerDefinition")) { 12708 this.pattern = new TriggerDefinition(); 12709 return this.pattern; 12710 } 12711 else if (name.equals("patternUsageContext")) { 12712 this.pattern = new UsageContext(); 12713 return this.pattern; 12714 } 12715 else if (name.equals("patternAvailability")) { 12716 this.pattern = new Availability(); 12717 return this.pattern; 12718 } 12719 else if (name.equals("patternExtendedContactDetail")) { 12720 this.pattern = new ExtendedContactDetail(); 12721 return this.pattern; 12722 } 12723 else if (name.equals("patternDosage")) { 12724 this.pattern = new Dosage(); 12725 return this.pattern; 12726 } 12727 else if (name.equals("patternMeta")) { 12728 this.pattern = new Meta(); 12729 return this.pattern; 12730 } 12731 else if (name.equals("example")) { 12732 return addExample(); 12733 } 12734 else if (name.equals("minValueDate")) { 12735 this.minValue = new DateType(); 12736 return this.minValue; 12737 } 12738 else if (name.equals("minValueDateTime")) { 12739 this.minValue = new DateTimeType(); 12740 return this.minValue; 12741 } 12742 else if (name.equals("minValueInstant")) { 12743 this.minValue = new InstantType(); 12744 return this.minValue; 12745 } 12746 else if (name.equals("minValueTime")) { 12747 this.minValue = new TimeType(); 12748 return this.minValue; 12749 } 12750 else if (name.equals("minValueDecimal")) { 12751 this.minValue = new DecimalType(); 12752 return this.minValue; 12753 } 12754 else if (name.equals("minValueInteger")) { 12755 this.minValue = new IntegerType(); 12756 return this.minValue; 12757 } 12758 else if (name.equals("minValueInteger64")) { 12759 this.minValue = new Integer64Type(); 12760 return this.minValue; 12761 } 12762 else if (name.equals("minValuePositiveInt")) { 12763 this.minValue = new PositiveIntType(); 12764 return this.minValue; 12765 } 12766 else if (name.equals("minValueUnsignedInt")) { 12767 this.minValue = new UnsignedIntType(); 12768 return this.minValue; 12769 } 12770 else if (name.equals("minValueQuantity")) { 12771 this.minValue = new Quantity(); 12772 return this.minValue; 12773 } 12774 else if (name.equals("maxValueDate")) { 12775 this.maxValue = new DateType(); 12776 return this.maxValue; 12777 } 12778 else if (name.equals("maxValueDateTime")) { 12779 this.maxValue = new DateTimeType(); 12780 return this.maxValue; 12781 } 12782 else if (name.equals("maxValueInstant")) { 12783 this.maxValue = new InstantType(); 12784 return this.maxValue; 12785 } 12786 else if (name.equals("maxValueTime")) { 12787 this.maxValue = new TimeType(); 12788 return this.maxValue; 12789 } 12790 else if (name.equals("maxValueDecimal")) { 12791 this.maxValue = new DecimalType(); 12792 return this.maxValue; 12793 } 12794 else if (name.equals("maxValueInteger")) { 12795 this.maxValue = new IntegerType(); 12796 return this.maxValue; 12797 } 12798 else if (name.equals("maxValueInteger64")) { 12799 this.maxValue = new Integer64Type(); 12800 return this.maxValue; 12801 } 12802 else if (name.equals("maxValuePositiveInt")) { 12803 this.maxValue = new PositiveIntType(); 12804 return this.maxValue; 12805 } 12806 else if (name.equals("maxValueUnsignedInt")) { 12807 this.maxValue = new UnsignedIntType(); 12808 return this.maxValue; 12809 } 12810 else if (name.equals("maxValueQuantity")) { 12811 this.maxValue = new Quantity(); 12812 return this.maxValue; 12813 } 12814 else if (name.equals("maxLength")) { 12815 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.maxLength"); 12816 } 12817 else if (name.equals("condition")) { 12818 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.condition"); 12819 } 12820 else if (name.equals("constraint")) { 12821 return addConstraint(); 12822 } 12823 else if (name.equals("mustHaveValue")) { 12824 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustHaveValue"); 12825 } 12826 else if (name.equals("valueAlternatives")) { 12827 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.valueAlternatives"); 12828 } 12829 else if (name.equals("mustSupport")) { 12830 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustSupport"); 12831 } 12832 else if (name.equals("isModifier")) { 12833 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifier"); 12834 } 12835 else if (name.equals("isModifierReason")) { 12836 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifierReason"); 12837 } 12838 else if (name.equals("isSummary")) { 12839 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isSummary"); 12840 } 12841 else if (name.equals("binding")) { 12842 this.binding = new ElementDefinitionBindingComponent(); 12843 return this.binding; 12844 } 12845 else if (name.equals("mapping")) { 12846 return addMapping(); 12847 } 12848 else 12849 return super.addChild(name); 12850 } 12851 12852 public String fhirType() { 12853 return "ElementDefinition"; 12854 12855 } 12856 12857 public ElementDefinition copy() { 12858 ElementDefinition dst = new ElementDefinition(); 12859 copyValues(dst); 12860 return dst; 12861 } 12862 12863 public void copyValues(ElementDefinition dst) { 12864 super.copyValues(dst); 12865 dst.path = path == null ? null : path.copy(); 12866 if (representation != null) { 12867 dst.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 12868 for (Enumeration<PropertyRepresentation> i : representation) 12869 dst.representation.add(i.copy()); 12870 }; 12871 dst.sliceName = sliceName == null ? null : sliceName.copy(); 12872 dst.sliceIsConstraining = sliceIsConstraining == null ? null : sliceIsConstraining.copy(); 12873 dst.label = label == null ? null : label.copy(); 12874 if (code != null) { 12875 dst.code = new ArrayList<Coding>(); 12876 for (Coding i : code) 12877 dst.code.add(i.copy()); 12878 }; 12879 dst.slicing = slicing == null ? null : slicing.copy(); 12880 dst.short_ = short_ == null ? null : short_.copy(); 12881 dst.definition = definition == null ? null : definition.copy(); 12882 dst.comment = comment == null ? null : comment.copy(); 12883 dst.requirements = requirements == null ? null : requirements.copy(); 12884 if (alias != null) { 12885 dst.alias = new ArrayList<StringType>(); 12886 for (StringType i : alias) 12887 dst.alias.add(i.copy()); 12888 }; 12889 dst.min = min == null ? null : min.copy(); 12890 dst.max = max == null ? null : max.copy(); 12891 dst.base = base == null ? null : base.copy(); 12892 dst.contentReference = contentReference == null ? null : contentReference.copy(); 12893 if (type != null) { 12894 dst.type = new ArrayList<TypeRefComponent>(); 12895 for (TypeRefComponent i : type) 12896 dst.type.add(i.copy()); 12897 }; 12898 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 12899 dst.meaningWhenMissing = meaningWhenMissing == null ? null : meaningWhenMissing.copy(); 12900 dst.orderMeaning = orderMeaning == null ? null : orderMeaning.copy(); 12901 dst.fixed = fixed == null ? null : fixed.copy(); 12902 dst.pattern = pattern == null ? null : pattern.copy(); 12903 if (example != null) { 12904 dst.example = new ArrayList<ElementDefinitionExampleComponent>(); 12905 for (ElementDefinitionExampleComponent i : example) 12906 dst.example.add(i.copy()); 12907 }; 12908 dst.minValue = minValue == null ? null : minValue.copy(); 12909 dst.maxValue = maxValue == null ? null : maxValue.copy(); 12910 dst.maxLength = maxLength == null ? null : maxLength.copy(); 12911 if (condition != null) { 12912 dst.condition = new ArrayList<IdType>(); 12913 for (IdType i : condition) 12914 dst.condition.add(i.copy()); 12915 }; 12916 if (constraint != null) { 12917 dst.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 12918 for (ElementDefinitionConstraintComponent i : constraint) 12919 dst.constraint.add(i.copy()); 12920 }; 12921 dst.mustHaveValue = mustHaveValue == null ? null : mustHaveValue.copy(); 12922 if (valueAlternatives != null) { 12923 dst.valueAlternatives = new ArrayList<CanonicalType>(); 12924 for (CanonicalType i : valueAlternatives) 12925 dst.valueAlternatives.add(i.copy()); 12926 }; 12927 dst.mustSupport = mustSupport == null ? null : mustSupport.copy(); 12928 dst.isModifier = isModifier == null ? null : isModifier.copy(); 12929 dst.isModifierReason = isModifierReason == null ? null : isModifierReason.copy(); 12930 dst.isSummary = isSummary == null ? null : isSummary.copy(); 12931 dst.binding = binding == null ? null : binding.copy(); 12932 if (mapping != null) { 12933 dst.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 12934 for (ElementDefinitionMappingComponent i : mapping) 12935 dst.mapping.add(i.copy()); 12936 }; 12937 } 12938 12939 protected ElementDefinition typedCopy() { 12940 return copy(); 12941 } 12942 12943 @Override 12944 public boolean equalsDeep(Base other_) { 12945 if (!super.equalsDeep(other_)) 12946 return false; 12947 if (!(other_ instanceof ElementDefinition)) 12948 return false; 12949 ElementDefinition o = (ElementDefinition) other_; 12950 return compareDeep(path, o.path, true) && compareDeep(representation, o.representation, true) && compareDeep(sliceName, o.sliceName, true) 12951 && compareDeep(sliceIsConstraining, o.sliceIsConstraining, true) && compareDeep(label, o.label, true) 12952 && compareDeep(code, o.code, true) && compareDeep(slicing, o.slicing, true) && compareDeep(short_, o.short_, true) 12953 && compareDeep(definition, o.definition, true) && compareDeep(comment, o.comment, true) && compareDeep(requirements, o.requirements, true) 12954 && compareDeep(alias, o.alias, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 12955 && compareDeep(base, o.base, true) && compareDeep(contentReference, o.contentReference, true) && compareDeep(type, o.type, true) 12956 && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(meaningWhenMissing, o.meaningWhenMissing, true) 12957 && compareDeep(orderMeaning, o.orderMeaning, true) && compareDeep(fixed, o.fixed, true) && compareDeep(pattern, o.pattern, true) 12958 && compareDeep(example, o.example, true) && compareDeep(minValue, o.minValue, true) && compareDeep(maxValue, o.maxValue, true) 12959 && compareDeep(maxLength, o.maxLength, true) && compareDeep(condition, o.condition, true) && compareDeep(constraint, o.constraint, true) 12960 && compareDeep(mustHaveValue, o.mustHaveValue, true) && compareDeep(valueAlternatives, o.valueAlternatives, true) 12961 && compareDeep(mustSupport, o.mustSupport, true) && compareDeep(isModifier, o.isModifier, true) 12962 && compareDeep(isModifierReason, o.isModifierReason, true) && compareDeep(isSummary, o.isSummary, true) 12963 && compareDeep(binding, o.binding, true) && compareDeep(mapping, o.mapping, true); 12964 } 12965 12966 @Override 12967 public boolean equalsShallow(Base other_) { 12968 if (!super.equalsShallow(other_)) 12969 return false; 12970 if (!(other_ instanceof ElementDefinition)) 12971 return false; 12972 ElementDefinition o = (ElementDefinition) other_; 12973 return compareValues(path, o.path, true) && compareValues(representation, o.representation, true) && compareValues(sliceName, o.sliceName, true) 12974 && compareValues(sliceIsConstraining, o.sliceIsConstraining, true) && compareValues(label, o.label, true) 12975 && compareValues(short_, o.short_, true) && compareValues(definition, o.definition, true) && compareValues(comment, o.comment, true) 12976 && compareValues(requirements, o.requirements, true) && compareValues(alias, o.alias, true) && compareValues(min, o.min, true) 12977 && compareValues(max, o.max, true) && compareValues(contentReference, o.contentReference, true) && compareValues(meaningWhenMissing, o.meaningWhenMissing, true) 12978 && compareValues(orderMeaning, o.orderMeaning, true) && compareValues(maxLength, o.maxLength, true) 12979 && compareValues(condition, o.condition, true) && compareValues(mustHaveValue, o.mustHaveValue, true) 12980 && compareValues(valueAlternatives, o.valueAlternatives, true) && compareValues(mustSupport, o.mustSupport, true) 12981 && compareValues(isModifier, o.isModifier, true) && compareValues(isModifierReason, o.isModifierReason, true) 12982 && compareValues(isSummary, o.isSummary, true); 12983 } 12984 12985 public boolean isEmpty() { 12986 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, representation, sliceName 12987 , sliceIsConstraining, label, code, slicing, short_, definition, comment, requirements 12988 , alias, min, max, base, contentReference, type, defaultValue, meaningWhenMissing 12989 , orderMeaning, fixed, pattern, example, minValue, maxValue, maxLength, condition 12990 , constraint, mustHaveValue, valueAlternatives, mustSupport, isModifier, isModifierReason 12991 , isSummary, binding, mapping); 12992 } 12993 12994// Manual code (from Configuration.txt): 12995 12996 public String toString() { 12997 if (hasId()) 12998 return getId(); 12999 if (hasSliceName()) 13000 return getPath()+":"+getSliceName(); 13001 else 13002 return getPath(); 13003 } 13004 13005 public void makeBase(String path, int min, String max) { 13006 ElementDefinitionBaseComponent self = getBase(); 13007 self.setPath(path); 13008 self.setMin(min); 13009 self.setMax(max); 13010 } 13011 13012 public void makeBase() { 13013 ElementDefinitionBaseComponent self = getBase(); 13014 self.setPath(getPath()); 13015 self.setMin(getMin()); 13016 self.setMax(getMax()); 13017 } 13018 13019 13020 public String typeSummary() { 13021 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder(); 13022 for (TypeRefComponent tr : getType()) { 13023 if (tr.hasCode()) 13024 b.append(tr.getWorkingCode()); 13025 } 13026 return b.toString(); 13027 } 13028 13029 public List<String> typeList() { 13030 List<String> res = new ArrayList<>(); 13031 for (TypeRefComponent tr : getType()) { 13032 if (tr.hasCode()) 13033 res.add(tr.getWorkingCode()); 13034 } 13035 return res; 13036 } 13037 13038 public String typeSummaryVB() { 13039 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder("|"); 13040 for (TypeRefComponent tr : getType()) { 13041 if (tr.hasCode()) 13042 b.append(tr.getWorkingCode()); 13043 } 13044 return b.toString().replace(" ", ""); 13045 } 13046 13047 public TypeRefComponent getType(String code) { 13048 for (TypeRefComponent tr : getType()) 13049 if (tr.getCode().equals(code)) 13050 return tr; 13051 TypeRefComponent tr = new TypeRefComponent(); 13052 tr.setCode(code); 13053 type.add(tr); 13054 return tr; 13055 } 13056 13057 public static final boolean NOT_MODIFIER = false; 13058 public static final boolean NOT_IN_SUMMARY = false; 13059 public static final boolean IS_MODIFIER = true; 13060 public static final boolean IS_IN_SUMMARY = true; 13061 public ElementDefinition(boolean defaults, boolean modifier, boolean inSummary) { 13062 super(); 13063 if (defaults) { 13064 setIsModifier(modifier); 13065 setIsSummary(inSummary); 13066 } 13067 } 13068 13069 public String present() { 13070 return hasId() ? getId() : getPath(); 13071 } 13072 13073 public boolean hasCondition(IdType id) { 13074 for (IdType c : getCondition()) { 13075 if (c.primitiveValue().equals(id.primitiveValue())) 13076 return true; 13077 } 13078 return false; 13079 } 13080 13081 public boolean hasConstraint(String key) { 13082 for (ElementDefinitionConstraintComponent c : getConstraint()) { 13083 if (c.getKey().equals(key)) 13084 return true; 13085 } 13086 return false; 13087 } 13088 13089 public boolean hasCode(Coding c) { 13090 for (Coding t : getCode()) { 13091 if (t.getSystem().equals(c.getSystem()) && t.getCode().equals(c.getCode())) 13092 return true; 13093 } 13094 return false; 13095 } 13096 13097 public boolean isChoice() { 13098 return getPath().endsWith("[x]"); 13099 } 13100 13101 public String getName() { 13102 return hasPath() ? getPath().contains(".") ? getPath().substring(getPath().lastIndexOf(".")+1) : getPath() : null; 13103 } 13104 13105 public String getNameBase() { 13106 return getName().replace("[x]", ""); 13107 } 13108 13109 public boolean unbounded() { 13110 if (getMax() == null) { 13111 throw new Error("No max on "+getPath()); 13112 } 13113 return getMax().equals("*") || Integer.parseInt(getMax()) > 1; 13114 } 13115 13116 public boolean isMandatory() { 13117 return getMin() > 0; 13118 } 13119 13120 public boolean isInlineType() { 13121 return getType().size() == 1 && Utilities.existsInList(getType().get(0).getCode(), "Element", "BackboneElement"); 13122 } 13123 13124 13125 public boolean prohibited() { 13126 return "0".equals(getMax()); 13127 } 13128 13129 public boolean hasFixedOrPattern() { 13130 return hasFixed() || hasPattern(); 13131 } 13132 13133 public DataType getFixedOrPattern() { 13134 return hasFixed() ? getFixed() : getPattern(); 13135 } 13136 13137 public boolean isProhibited() { 13138 return "0".equals(getMax()); 13139 } 13140 13141 public boolean isRequired() { 13142 return getMin() == 1; 13143 } 13144 13145 public CanonicalType addValueAlternative(CanonicalType t) { 13146 if (this.valueAlternatives == null) 13147 this.valueAlternatives = new ArrayList<CanonicalType>(); 13148 this.valueAlternatives.add(t); 13149 return t; 13150 } 13151 13152 public boolean repeats() { 13153 return !Utilities.existsInList(getMax(), "0", "1"); 13154 } 13155 13156 public String getIdOrPath() { 13157 return hasId() ? getId() : getPath(); 13158 } 13159 13160 public int getMaxAsInt() { 13161 return "*".equals(getMax()) ? Integer.MAX_VALUE : Integer.parseInt(getMax()); 13162 } 13163 13164 public TypeRefComponent getByType(String code) { 13165 for (TypeRefComponent tr : getType()) { 13166 if (tr.getWorkingCode().equals(code)) { 13167 return tr; 13168 } 13169 } 13170 return null; 13171 } 13172 13173// end addition 13174 13175} 13176