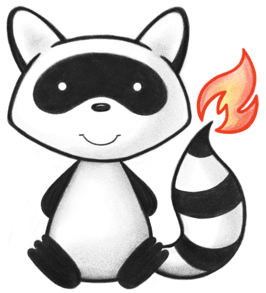
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import org.hl7.fhir.instance.model.api.ICompositeType; 049import org.hl7.fhir.r5.model.Enumerations.BindingStrength; 050import org.hl7.fhir.r5.model.Enumerations.BindingStrengthEnumFactory; 051import org.hl7.fhir.r5.utils.ToolingExtensions; 052import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 053import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 054/** 055 * ElementDefinition Type: Captures constraints on each element within the resource, profile, or extension. 056 */ 057@DatatypeDef(name="ElementDefinition") 058public class ElementDefinition extends BackboneType implements ICompositeType { 059 060 public enum AdditionalBindingPurposeVS { 061 /** 062 * A required binding, for use when the binding strength is 'extensible' or 'preferred' 063 */ 064 MAXIMUM, 065 /** 066 * The minimum allowable value set - any conformant system SHALL support all these codes 067 */ 068 MINIMUM, 069 /** 070 * This value set is used as a required binding (in addition to the base binding (not a replacement), usually in a particular usage context) 071 */ 072 REQUIRED, 073 /** 074 * This value set is used as an extensible binding (in addition to the base binding (not a replacement), usually in a particular usage context) 075 */ 076 EXTENSIBLE, 077 /** 078 * This value set is a candidate to substitute for the overall conformance value set in some situations; usually these are defined in the documentation 079 */ 080 CANDIDATE, 081 /** 082 * New records are required to use this value set, but legacy records may use other codes. The definition of 'new record' is difficult, since systems often create new records based on pre-existing data. Usually 'current' bindings are mandated by an external authority that makes clear rules around this 083 */ 084 CURRENT, 085 /** 086 * This is the value set that is preferred in a given context (documentation should explain why) 087 */ 088 PREFERRED, 089 /** 090 * This value set is provided for user look up in a given context. Typically, these valuesets only include a subset of codes relevant for input in a context 091 */ 092 UI, 093 /** 094 * This value set is a good set of codes to start with when designing your system 095 */ 096 STARTER, 097 /** 098 * This value set is a component of the base value set. Usually this is called out so that documentation can be written about a portion of the value set 099 */ 100 COMPONENT, 101 /** 102 * added to help the parsers with the generic types 103 */ 104 NULL; 105 public static AdditionalBindingPurposeVS fromCode(String codeString) throws FHIRException { 106 if (codeString == null || "".equals(codeString)) 107 return null; 108 if ("maximum".equals(codeString)) 109 return MAXIMUM; 110 if ("minimum".equals(codeString)) 111 return MINIMUM; 112 if ("required".equals(codeString)) 113 return REQUIRED; 114 if ("extensible".equals(codeString)) 115 return EXTENSIBLE; 116 if ("candidate".equals(codeString)) 117 return CANDIDATE; 118 if ("current".equals(codeString)) 119 return CURRENT; 120 if ("preferred".equals(codeString)) 121 return PREFERRED; 122 if ("ui".equals(codeString)) 123 return UI; 124 if ("starter".equals(codeString)) 125 return STARTER; 126 if ("component".equals(codeString)) 127 return COMPONENT; 128 if (Configuration.isAcceptInvalidEnums()) 129 return null; 130 else 131 throw new FHIRException("Unknown AdditionalBindingPurposeVS code '"+codeString+"'"); 132 } 133 public String toCode() { 134 switch (this) { 135 case MAXIMUM: return "maximum"; 136 case MINIMUM: return "minimum"; 137 case REQUIRED: return "required"; 138 case EXTENSIBLE: return "extensible"; 139 case CANDIDATE: return "candidate"; 140 case CURRENT: return "current"; 141 case PREFERRED: return "preferred"; 142 case UI: return "ui"; 143 case STARTER: return "starter"; 144 case COMPONENT: return "component"; 145 case NULL: return null; 146 default: return "?"; 147 } 148 } 149 public String getSystem() { 150 switch (this) { 151 case MAXIMUM: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 152 case MINIMUM: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 153 case REQUIRED: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 154 case EXTENSIBLE: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 155 case CANDIDATE: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 156 case CURRENT: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 157 case PREFERRED: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 158 case UI: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 159 case STARTER: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 160 case COMPONENT: return "http://hl7.org/fhir/CodeSystem/additional-binding-purpose"; 161 case NULL: return null; 162 default: return "?"; 163 } 164 } 165 public String getDefinition() { 166 switch (this) { 167 case MAXIMUM: return "A required binding, for use when the binding strength is 'extensible' or 'preferred'"; 168 case MINIMUM: return "The minimum allowable value set - any conformant system SHALL support all these codes"; 169 case REQUIRED: return "This value set is used as a required binding (in addition to the base binding (not a replacement), usually in a particular usage context)"; 170 case EXTENSIBLE: return "This value set is used as an extensible binding (in addition to the base binding (not a replacement), usually in a particular usage context)"; 171 case CANDIDATE: return "This value set is a candidate to substitute for the overall conformance value set in some situations; usually these are defined in the documentation"; 172 case CURRENT: return "New records are required to use this value set, but legacy records may use other codes. The definition of 'new record' is difficult, since systems often create new records based on pre-existing data. Usually 'current' bindings are mandated by an external authority that makes clear rules around this"; 173 case PREFERRED: return "This is the value set that is preferred in a given context (documentation should explain why)"; 174 case UI: return "This value set is provided for user look up in a given context. Typically, these valuesets only include a subset of codes relevant for input in a context"; 175 case STARTER: return "This value set is a good set of codes to start with when designing your system"; 176 case COMPONENT: return "This value set is a component of the base value set. Usually this is called out so that documentation can be written about a portion of the value set"; 177 case NULL: return null; 178 default: return "?"; 179 } 180 } 181 public String getDisplay() { 182 switch (this) { 183 case MAXIMUM: return "Maximum Binding"; 184 case MINIMUM: return "Minimum Binding"; 185 case REQUIRED: return "Required Binding"; 186 case EXTENSIBLE: return "Conformance Binding"; 187 case CANDIDATE: return "Candidate Binding"; 188 case CURRENT: return "Current Binding"; 189 case PREFERRED: return "Preferred Binding"; 190 case UI: return "UI Suggested Binding"; 191 case STARTER: return "Starter Binding"; 192 case COMPONENT: return "Component Binding"; 193 case NULL: return null; 194 default: return "?"; 195 } 196 } 197 } 198 199 public static class AdditionalBindingPurposeVSEnumFactory implements EnumFactory<AdditionalBindingPurposeVS> { 200 public AdditionalBindingPurposeVS fromCode(String codeString) throws IllegalArgumentException { 201 if (codeString == null || "".equals(codeString)) 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("maximum".equals(codeString)) 205 return AdditionalBindingPurposeVS.MAXIMUM; 206 if ("minimum".equals(codeString)) 207 return AdditionalBindingPurposeVS.MINIMUM; 208 if ("required".equals(codeString)) 209 return AdditionalBindingPurposeVS.REQUIRED; 210 if ("extensible".equals(codeString)) 211 return AdditionalBindingPurposeVS.EXTENSIBLE; 212 if ("candidate".equals(codeString)) 213 return AdditionalBindingPurposeVS.CANDIDATE; 214 if ("current".equals(codeString)) 215 return AdditionalBindingPurposeVS.CURRENT; 216 if ("preferred".equals(codeString)) 217 return AdditionalBindingPurposeVS.PREFERRED; 218 if ("ui".equals(codeString)) 219 return AdditionalBindingPurposeVS.UI; 220 if ("starter".equals(codeString)) 221 return AdditionalBindingPurposeVS.STARTER; 222 if ("component".equals(codeString)) 223 return AdditionalBindingPurposeVS.COMPONENT; 224 throw new IllegalArgumentException("Unknown AdditionalBindingPurposeVS code '"+codeString+"'"); 225 } 226 public Enumeration<AdditionalBindingPurposeVS> fromType(PrimitiveType<?> code) throws FHIRException { 227 if (code == null) 228 return null; 229 if (code.isEmpty()) 230 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.NULL, code); 231 String codeString = ((PrimitiveType) code).asStringValue(); 232 if (codeString == null || "".equals(codeString)) 233 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.NULL, code); 234 if ("maximum".equals(codeString)) 235 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.MAXIMUM, code); 236 if ("minimum".equals(codeString)) 237 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.MINIMUM, code); 238 if ("required".equals(codeString)) 239 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.REQUIRED, code); 240 if ("extensible".equals(codeString)) 241 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.EXTENSIBLE, code); 242 if ("candidate".equals(codeString)) 243 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.CANDIDATE, code); 244 if ("current".equals(codeString)) 245 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.CURRENT, code); 246 if ("preferred".equals(codeString)) 247 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.PREFERRED, code); 248 if ("ui".equals(codeString)) 249 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.UI, code); 250 if ("starter".equals(codeString)) 251 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.STARTER, code); 252 if ("component".equals(codeString)) 253 return new Enumeration<AdditionalBindingPurposeVS>(this, AdditionalBindingPurposeVS.COMPONENT, code); 254 throw new FHIRException("Unknown AdditionalBindingPurposeVS code '"+codeString+"'"); 255 } 256 public String toCode(AdditionalBindingPurposeVS code) { 257 if (code == AdditionalBindingPurposeVS.MAXIMUM) 258 return "maximum"; 259 if (code == AdditionalBindingPurposeVS.MINIMUM) 260 return "minimum"; 261 if (code == AdditionalBindingPurposeVS.REQUIRED) 262 return "required"; 263 if (code == AdditionalBindingPurposeVS.EXTENSIBLE) 264 return "extensible"; 265 if (code == AdditionalBindingPurposeVS.CANDIDATE) 266 return "candidate"; 267 if (code == AdditionalBindingPurposeVS.CURRENT) 268 return "current"; 269 if (code == AdditionalBindingPurposeVS.PREFERRED) 270 return "preferred"; 271 if (code == AdditionalBindingPurposeVS.UI) 272 return "ui"; 273 if (code == AdditionalBindingPurposeVS.STARTER) 274 return "starter"; 275 if (code == AdditionalBindingPurposeVS.COMPONENT) 276 return "component"; 277 return "?"; 278 } 279 public String toSystem(AdditionalBindingPurposeVS code) { 280 return code.getSystem(); 281 } 282 } 283 284 public enum AggregationMode { 285 /** 286 * The reference is a local reference to a contained resource. 287 */ 288 CONTAINED, 289 /** 290 * The reference to a resource that has to be resolved externally to the resource that includes the reference. 291 */ 292 REFERENCED, 293 /** 294 * When the resource is in a Bundle, the resource the reference points to will be found in the same bundle as the resource that includes the reference. 295 */ 296 BUNDLED, 297 /** 298 * added to help the parsers with the generic types 299 */ 300 NULL; 301 public static AggregationMode fromCode(String codeString) throws FHIRException { 302 if (codeString == null || "".equals(codeString)) 303 return null; 304 if ("contained".equals(codeString)) 305 return CONTAINED; 306 if ("referenced".equals(codeString)) 307 return REFERENCED; 308 if ("bundled".equals(codeString)) 309 return BUNDLED; 310 if (Configuration.isAcceptInvalidEnums()) 311 return null; 312 else 313 throw new FHIRException("Unknown AggregationMode code '"+codeString+"'"); 314 } 315 public String toCode() { 316 switch (this) { 317 case CONTAINED: return "contained"; 318 case REFERENCED: return "referenced"; 319 case BUNDLED: return "bundled"; 320 case NULL: return null; 321 default: return "?"; 322 } 323 } 324 public String getSystem() { 325 switch (this) { 326 case CONTAINED: return "http://hl7.org/fhir/resource-aggregation-mode"; 327 case REFERENCED: return "http://hl7.org/fhir/resource-aggregation-mode"; 328 case BUNDLED: return "http://hl7.org/fhir/resource-aggregation-mode"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 public String getDefinition() { 334 switch (this) { 335 case CONTAINED: return "The reference is a local reference to a contained resource."; 336 case REFERENCED: return "The reference to a resource that has to be resolved externally to the resource that includes the reference."; 337 case BUNDLED: return "When the resource is in a Bundle, the resource the reference points to will be found in the same bundle as the resource that includes the reference."; 338 case NULL: return null; 339 default: return "?"; 340 } 341 } 342 public String getDisplay() { 343 switch (this) { 344 case CONTAINED: return "Contained"; 345 case REFERENCED: return "Referenced"; 346 case BUNDLED: return "Bundled"; 347 case NULL: return null; 348 default: return "?"; 349 } 350 } 351 } 352 353 public static class AggregationModeEnumFactory implements EnumFactory<AggregationMode> { 354 public AggregationMode fromCode(String codeString) throws IllegalArgumentException { 355 if (codeString == null || "".equals(codeString)) 356 if (codeString == null || "".equals(codeString)) 357 return null; 358 if ("contained".equals(codeString)) 359 return AggregationMode.CONTAINED; 360 if ("referenced".equals(codeString)) 361 return AggregationMode.REFERENCED; 362 if ("bundled".equals(codeString)) 363 return AggregationMode.BUNDLED; 364 throw new IllegalArgumentException("Unknown AggregationMode code '"+codeString+"'"); 365 } 366 public Enumeration<AggregationMode> fromType(PrimitiveType<?> code) throws FHIRException { 367 if (code == null) 368 return null; 369 if (code.isEmpty()) 370 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 371 String codeString = ((PrimitiveType) code).asStringValue(); 372 if (codeString == null || "".equals(codeString)) 373 return new Enumeration<AggregationMode>(this, AggregationMode.NULL, code); 374 if ("contained".equals(codeString)) 375 return new Enumeration<AggregationMode>(this, AggregationMode.CONTAINED, code); 376 if ("referenced".equals(codeString)) 377 return new Enumeration<AggregationMode>(this, AggregationMode.REFERENCED, code); 378 if ("bundled".equals(codeString)) 379 return new Enumeration<AggregationMode>(this, AggregationMode.BUNDLED, code); 380 throw new FHIRException("Unknown AggregationMode code '"+codeString+"'"); 381 } 382 public String toCode(AggregationMode code) { 383 if (code == AggregationMode.CONTAINED) 384 return "contained"; 385 if (code == AggregationMode.REFERENCED) 386 return "referenced"; 387 if (code == AggregationMode.BUNDLED) 388 return "bundled"; 389 return "?"; 390 } 391 public String toSystem(AggregationMode code) { 392 return code.getSystem(); 393 } 394 } 395 396 public enum ConstraintSeverity { 397 /** 398 * If the constraint is violated, the resource is not conformant. 399 */ 400 ERROR, 401 /** 402 * If the constraint is violated, the resource is conformant, but it is not necessarily following best practice. 403 */ 404 WARNING, 405 /** 406 * added to help the parsers with the generic types 407 */ 408 NULL; 409 public static ConstraintSeverity fromCode(String codeString) throws FHIRException { 410 if (codeString == null || "".equals(codeString)) 411 return null; 412 if ("error".equals(codeString)) 413 return ERROR; 414 if ("warning".equals(codeString)) 415 return WARNING; 416 if (Configuration.isAcceptInvalidEnums()) 417 return null; 418 else 419 throw new FHIRException("Unknown ConstraintSeverity code '"+codeString+"'"); 420 } 421 public String toCode() { 422 switch (this) { 423 case ERROR: return "error"; 424 case WARNING: return "warning"; 425 case NULL: return null; 426 default: return "?"; 427 } 428 } 429 public String getSystem() { 430 switch (this) { 431 case ERROR: return "http://hl7.org/fhir/constraint-severity"; 432 case WARNING: return "http://hl7.org/fhir/constraint-severity"; 433 case NULL: return null; 434 default: return "?"; 435 } 436 } 437 public String getDefinition() { 438 switch (this) { 439 case ERROR: return "If the constraint is violated, the resource is not conformant."; 440 case WARNING: return "If the constraint is violated, the resource is conformant, but it is not necessarily following best practice."; 441 case NULL: return null; 442 default: return "?"; 443 } 444 } 445 public String getDisplay() { 446 switch (this) { 447 case ERROR: return "Error"; 448 case WARNING: return "Warning"; 449 case NULL: return null; 450 default: return "?"; 451 } 452 } 453 } 454 455 public static class ConstraintSeverityEnumFactory implements EnumFactory<ConstraintSeverity> { 456 public ConstraintSeverity fromCode(String codeString) throws IllegalArgumentException { 457 if (codeString == null || "".equals(codeString)) 458 if (codeString == null || "".equals(codeString)) 459 return null; 460 if ("error".equals(codeString)) 461 return ConstraintSeverity.ERROR; 462 if ("warning".equals(codeString)) 463 return ConstraintSeverity.WARNING; 464 throw new IllegalArgumentException("Unknown ConstraintSeverity code '"+codeString+"'"); 465 } 466 public Enumeration<ConstraintSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 467 if (code == null) 468 return null; 469 if (code.isEmpty()) 470 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 471 String codeString = ((PrimitiveType) code).asStringValue(); 472 if (codeString == null || "".equals(codeString)) 473 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.NULL, code); 474 if ("error".equals(codeString)) 475 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.ERROR, code); 476 if ("warning".equals(codeString)) 477 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.WARNING, code); 478 throw new FHIRException("Unknown ConstraintSeverity code '"+codeString+"'"); 479 } 480 public String toCode(ConstraintSeverity code) { 481 if (code == ConstraintSeverity.ERROR) 482 return "error"; 483 if (code == ConstraintSeverity.WARNING) 484 return "warning"; 485 return "?"; 486 } 487 public String toSystem(ConstraintSeverity code) { 488 return code.getSystem(); 489 } 490 } 491 492 public enum DiscriminatorType { 493 /** 494 * The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding. 495 */ 496 VALUE, 497 /** 498 * The slices are differentiated by the presence or absence of the nominated element. There SHALL be no more than two slices. The slices are differentiated by the fact that one must have a max of 0 and the other must have a min of 1 (or more). The order in which the slices are declared doesn't matter. 499 */ 500 EXISTS, 501 /** 502 * The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding. This has the same meaning as 'value' and is deprecated. 503 */ 504 PATTERN, 505 /** 506 * The slices are differentiated by type of the nominated element. 507 */ 508 TYPE, 509 /** 510 * The slices are differentiated by conformance of the nominated element to a specified profile. Note that if the path specifies .resolve() then the profile is the target profile on the reference. In this case, validation by the possible profiles is required to differentiate the slices. 511 */ 512 PROFILE, 513 /** 514 * The slices are differentiated by their index. This is only possible if all but the last slice have min=max cardinality, and the (optional) last slice contains other undifferentiated elements. 515 */ 516 POSITION, 517 /** 518 * added to help the parsers with the generic types 519 */ 520 NULL; 521 public static DiscriminatorType fromCode(String codeString) throws FHIRException { 522 if (codeString == null || "".equals(codeString)) 523 return null; 524 if ("value".equals(codeString)) 525 return VALUE; 526 if ("exists".equals(codeString)) 527 return EXISTS; 528 if ("pattern".equals(codeString)) 529 return PATTERN; 530 if ("type".equals(codeString)) 531 return TYPE; 532 if ("profile".equals(codeString)) 533 return PROFILE; 534 if ("position".equals(codeString)) 535 return POSITION; 536 if (Configuration.isAcceptInvalidEnums()) 537 return null; 538 else 539 throw new FHIRException("Unknown DiscriminatorType code '"+codeString+"'"); 540 } 541 public String toCode() { 542 switch (this) { 543 case VALUE: return "value"; 544 case EXISTS: return "exists"; 545 case PATTERN: return "pattern"; 546 case TYPE: return "type"; 547 case PROFILE: return "profile"; 548 case POSITION: return "position"; 549 case NULL: return null; 550 default: return "?"; 551 } 552 } 553 public String getSystem() { 554 switch (this) { 555 case VALUE: return "http://hl7.org/fhir/discriminator-type"; 556 case EXISTS: return "http://hl7.org/fhir/discriminator-type"; 557 case PATTERN: return "http://hl7.org/fhir/discriminator-type"; 558 case TYPE: return "http://hl7.org/fhir/discriminator-type"; 559 case PROFILE: return "http://hl7.org/fhir/discriminator-type"; 560 case POSITION: return "http://hl7.org/fhir/discriminator-type"; 561 case NULL: return null; 562 default: return "?"; 563 } 564 } 565 public String getDefinition() { 566 switch (this) { 567 case VALUE: return "The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding."; 568 case EXISTS: return "The slices are differentiated by the presence or absence of the nominated element. There SHALL be no more than two slices. The slices are differentiated by the fact that one must have a max of 0 and the other must have a min of 1 (or more). The order in which the slices are declared doesn't matter."; 569 case PATTERN: return "The slices have different values in the nominated element, as determined by the applicable fixed value, pattern, or required ValueSet binding. This has the same meaning as 'value' and is deprecated."; 570 case TYPE: return "The slices are differentiated by type of the nominated element."; 571 case PROFILE: return "The slices are differentiated by conformance of the nominated element to a specified profile. Note that if the path specifies .resolve() then the profile is the target profile on the reference. In this case, validation by the possible profiles is required to differentiate the slices."; 572 case POSITION: return "The slices are differentiated by their index. This is only possible if all but the last slice have min=max cardinality, and the (optional) last slice contains other undifferentiated elements."; 573 case NULL: return null; 574 default: return "?"; 575 } 576 } 577 public String getDisplay() { 578 switch (this) { 579 case VALUE: return "Value"; 580 case EXISTS: return "Exists"; 581 case PATTERN: return "Pattern"; 582 case TYPE: return "Type"; 583 case PROFILE: return "Profile"; 584 case POSITION: return "Position"; 585 case NULL: return null; 586 default: return "?"; 587 } 588 } 589 } 590 591 public static class DiscriminatorTypeEnumFactory implements EnumFactory<DiscriminatorType> { 592 public DiscriminatorType fromCode(String codeString) throws IllegalArgumentException { 593 if (codeString == null || "".equals(codeString)) 594 if (codeString == null || "".equals(codeString)) 595 return null; 596 if ("value".equals(codeString)) 597 return DiscriminatorType.VALUE; 598 if ("exists".equals(codeString)) 599 return DiscriminatorType.EXISTS; 600 if ("pattern".equals(codeString)) 601 return DiscriminatorType.PATTERN; 602 if ("type".equals(codeString)) 603 return DiscriminatorType.TYPE; 604 if ("profile".equals(codeString)) 605 return DiscriminatorType.PROFILE; 606 if ("position".equals(codeString)) 607 return DiscriminatorType.POSITION; 608 throw new IllegalArgumentException("Unknown DiscriminatorType code '"+codeString+"'"); 609 } 610 public Enumeration<DiscriminatorType> fromType(PrimitiveType<?> code) throws FHIRException { 611 if (code == null) 612 return null; 613 if (code.isEmpty()) 614 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 615 String codeString = ((PrimitiveType) code).asStringValue(); 616 if (codeString == null || "".equals(codeString)) 617 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.NULL, code); 618 if ("value".equals(codeString)) 619 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.VALUE, code); 620 if ("exists".equals(codeString)) 621 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.EXISTS, code); 622 if ("pattern".equals(codeString)) 623 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PATTERN, code); 624 if ("type".equals(codeString)) 625 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.TYPE, code); 626 if ("profile".equals(codeString)) 627 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PROFILE, code); 628 if ("position".equals(codeString)) 629 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.POSITION, code); 630 throw new FHIRException("Unknown DiscriminatorType code '"+codeString+"'"); 631 } 632 public String toCode(DiscriminatorType code) { 633 if (code == DiscriminatorType.VALUE) 634 return "value"; 635 if (code == DiscriminatorType.EXISTS) 636 return "exists"; 637 if (code == DiscriminatorType.PATTERN) 638 return "pattern"; 639 if (code == DiscriminatorType.TYPE) 640 return "type"; 641 if (code == DiscriminatorType.PROFILE) 642 return "profile"; 643 if (code == DiscriminatorType.POSITION) 644 return "position"; 645 return "?"; 646 } 647 public String toSystem(DiscriminatorType code) { 648 return code.getSystem(); 649 } 650 } 651 652 public enum PropertyRepresentation { 653 /** 654 * In XML, this property is represented as an attribute not an element. 655 */ 656 XMLATTR, 657 /** 658 * This element is represented using the XML text attribute (primitives only). 659 */ 660 XMLTEXT, 661 /** 662 * The type of this element is indicated using xsi:type. 663 */ 664 TYPEATTR, 665 /** 666 * Use CDA narrative instead of XHTML. 667 */ 668 CDATEXT, 669 /** 670 * The property is represented using XHTML. 671 */ 672 XHTML, 673 /** 674 * added to help the parsers with the generic types 675 */ 676 NULL; 677 public static PropertyRepresentation fromCode(String codeString) throws FHIRException { 678 if (codeString == null || "".equals(codeString)) 679 return null; 680 if ("xmlAttr".equals(codeString)) 681 return XMLATTR; 682 if ("xmlText".equals(codeString)) 683 return XMLTEXT; 684 if ("typeAttr".equals(codeString)) 685 return TYPEATTR; 686 if ("cdaText".equals(codeString)) 687 return CDATEXT; 688 if ("xhtml".equals(codeString)) 689 return XHTML; 690 if (Configuration.isAcceptInvalidEnums()) 691 return null; 692 else 693 throw new FHIRException("Unknown PropertyRepresentation code '"+codeString+"'"); 694 } 695 public String toCode() { 696 switch (this) { 697 case XMLATTR: return "xmlAttr"; 698 case XMLTEXT: return "xmlText"; 699 case TYPEATTR: return "typeAttr"; 700 case CDATEXT: return "cdaText"; 701 case XHTML: return "xhtml"; 702 case NULL: return null; 703 default: return "?"; 704 } 705 } 706 public String getSystem() { 707 switch (this) { 708 case XMLATTR: return "http://hl7.org/fhir/property-representation"; 709 case XMLTEXT: return "http://hl7.org/fhir/property-representation"; 710 case TYPEATTR: return "http://hl7.org/fhir/property-representation"; 711 case CDATEXT: return "http://hl7.org/fhir/property-representation"; 712 case XHTML: return "http://hl7.org/fhir/property-representation"; 713 case NULL: return null; 714 default: return "?"; 715 } 716 } 717 public String getDefinition() { 718 switch (this) { 719 case XMLATTR: return "In XML, this property is represented as an attribute not an element."; 720 case XMLTEXT: return "This element is represented using the XML text attribute (primitives only)."; 721 case TYPEATTR: return "The type of this element is indicated using xsi:type."; 722 case CDATEXT: return "Use CDA narrative instead of XHTML."; 723 case XHTML: return "The property is represented using XHTML."; 724 case NULL: return null; 725 default: return "?"; 726 } 727 } 728 public String getDisplay() { 729 switch (this) { 730 case XMLATTR: return "XML Attribute"; 731 case XMLTEXT: return "XML Text"; 732 case TYPEATTR: return "Type Attribute"; 733 case CDATEXT: return "CDA Text Format"; 734 case XHTML: return "XHTML"; 735 case NULL: return null; 736 default: return "?"; 737 } 738 } 739 } 740 741 public static class PropertyRepresentationEnumFactory implements EnumFactory<PropertyRepresentation> { 742 public PropertyRepresentation fromCode(String codeString) throws IllegalArgumentException { 743 if (codeString == null || "".equals(codeString)) 744 if (codeString == null || "".equals(codeString)) 745 return null; 746 if ("xmlAttr".equals(codeString)) 747 return PropertyRepresentation.XMLATTR; 748 if ("xmlText".equals(codeString)) 749 return PropertyRepresentation.XMLTEXT; 750 if ("typeAttr".equals(codeString)) 751 return PropertyRepresentation.TYPEATTR; 752 if ("cdaText".equals(codeString)) 753 return PropertyRepresentation.CDATEXT; 754 if ("xhtml".equals(codeString)) 755 return PropertyRepresentation.XHTML; 756 throw new IllegalArgumentException("Unknown PropertyRepresentation code '"+codeString+"'"); 757 } 758 public Enumeration<PropertyRepresentation> fromType(PrimitiveType<?> code) throws FHIRException { 759 if (code == null) 760 return null; 761 if (code.isEmpty()) 762 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 763 String codeString = ((PrimitiveType) code).asStringValue(); 764 if (codeString == null || "".equals(codeString)) 765 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.NULL, code); 766 if ("xmlAttr".equals(codeString)) 767 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLATTR, code); 768 if ("xmlText".equals(codeString)) 769 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLTEXT, code); 770 if ("typeAttr".equals(codeString)) 771 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.TYPEATTR, code); 772 if ("cdaText".equals(codeString)) 773 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.CDATEXT, code); 774 if ("xhtml".equals(codeString)) 775 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XHTML, code); 776 throw new FHIRException("Unknown PropertyRepresentation code '"+codeString+"'"); 777 } 778 public String toCode(PropertyRepresentation code) { 779 if (code == PropertyRepresentation.XMLATTR) 780 return "xmlAttr"; 781 if (code == PropertyRepresentation.XMLTEXT) 782 return "xmlText"; 783 if (code == PropertyRepresentation.TYPEATTR) 784 return "typeAttr"; 785 if (code == PropertyRepresentation.CDATEXT) 786 return "cdaText"; 787 if (code == PropertyRepresentation.XHTML) 788 return "xhtml"; 789 return "?"; 790 } 791 public String toSystem(PropertyRepresentation code) { 792 return code.getSystem(); 793 } 794 } 795 796 public enum ReferenceVersionRules { 797 /** 798 * The reference may be either version independent or version specific. 799 */ 800 EITHER, 801 /** 802 * The reference must be version independent. 803 */ 804 INDEPENDENT, 805 /** 806 * The reference must be version specific. 807 */ 808 SPECIFIC, 809 /** 810 * added to help the parsers with the generic types 811 */ 812 NULL; 813 public static ReferenceVersionRules fromCode(String codeString) throws FHIRException { 814 if (codeString == null || "".equals(codeString)) 815 return null; 816 if ("either".equals(codeString)) 817 return EITHER; 818 if ("independent".equals(codeString)) 819 return INDEPENDENT; 820 if ("specific".equals(codeString)) 821 return SPECIFIC; 822 if (Configuration.isAcceptInvalidEnums()) 823 return null; 824 else 825 throw new FHIRException("Unknown ReferenceVersionRules code '"+codeString+"'"); 826 } 827 public String toCode() { 828 switch (this) { 829 case EITHER: return "either"; 830 case INDEPENDENT: return "independent"; 831 case SPECIFIC: return "specific"; 832 case NULL: return null; 833 default: return "?"; 834 } 835 } 836 public String getSystem() { 837 switch (this) { 838 case EITHER: return "http://hl7.org/fhir/reference-version-rules"; 839 case INDEPENDENT: return "http://hl7.org/fhir/reference-version-rules"; 840 case SPECIFIC: return "http://hl7.org/fhir/reference-version-rules"; 841 case NULL: return null; 842 default: return "?"; 843 } 844 } 845 public String getDefinition() { 846 switch (this) { 847 case EITHER: return "The reference may be either version independent or version specific."; 848 case INDEPENDENT: return "The reference must be version independent."; 849 case SPECIFIC: return "The reference must be version specific."; 850 case NULL: return null; 851 default: return "?"; 852 } 853 } 854 public String getDisplay() { 855 switch (this) { 856 case EITHER: return "Either Specific or independent"; 857 case INDEPENDENT: return "Version independent"; 858 case SPECIFIC: return "Version Specific"; 859 case NULL: return null; 860 default: return "?"; 861 } 862 } 863 } 864 865 public static class ReferenceVersionRulesEnumFactory implements EnumFactory<ReferenceVersionRules> { 866 public ReferenceVersionRules fromCode(String codeString) throws IllegalArgumentException { 867 if (codeString == null || "".equals(codeString)) 868 if (codeString == null || "".equals(codeString)) 869 return null; 870 if ("either".equals(codeString)) 871 return ReferenceVersionRules.EITHER; 872 if ("independent".equals(codeString)) 873 return ReferenceVersionRules.INDEPENDENT; 874 if ("specific".equals(codeString)) 875 return ReferenceVersionRules.SPECIFIC; 876 throw new IllegalArgumentException("Unknown ReferenceVersionRules code '"+codeString+"'"); 877 } 878 public Enumeration<ReferenceVersionRules> fromType(PrimitiveType<?> code) throws FHIRException { 879 if (code == null) 880 return null; 881 if (code.isEmpty()) 882 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 883 String codeString = ((PrimitiveType) code).asStringValue(); 884 if (codeString == null || "".equals(codeString)) 885 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.NULL, code); 886 if ("either".equals(codeString)) 887 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.EITHER, code); 888 if ("independent".equals(codeString)) 889 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.INDEPENDENT, code); 890 if ("specific".equals(codeString)) 891 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.SPECIFIC, code); 892 throw new FHIRException("Unknown ReferenceVersionRules code '"+codeString+"'"); 893 } 894 public String toCode(ReferenceVersionRules code) { 895 if (code == ReferenceVersionRules.EITHER) 896 return "either"; 897 if (code == ReferenceVersionRules.INDEPENDENT) 898 return "independent"; 899 if (code == ReferenceVersionRules.SPECIFIC) 900 return "specific"; 901 return "?"; 902 } 903 public String toSystem(ReferenceVersionRules code) { 904 return code.getSystem(); 905 } 906 } 907 908 public enum SlicingRules { 909 /** 910 * No additional content is allowed other than that described by the slices in this profile. 911 */ 912 CLOSED, 913 /** 914 * Additional content is allowed anywhere in the list. 915 */ 916 OPEN, 917 /** 918 * Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required. 919 */ 920 OPENATEND, 921 /** 922 * added to help the parsers with the generic types 923 */ 924 NULL; 925 public static SlicingRules fromCode(String codeString) throws FHIRException { 926 if (codeString == null || "".equals(codeString)) 927 return null; 928 if ("closed".equals(codeString)) 929 return CLOSED; 930 if ("open".equals(codeString)) 931 return OPEN; 932 if ("openAtEnd".equals(codeString)) 933 return OPENATEND; 934 if (Configuration.isAcceptInvalidEnums()) 935 return null; 936 else 937 throw new FHIRException("Unknown SlicingRules code '"+codeString+"'"); 938 } 939 public String toCode() { 940 switch (this) { 941 case CLOSED: return "closed"; 942 case OPEN: return "open"; 943 case OPENATEND: return "openAtEnd"; 944 case NULL: return null; 945 default: return "?"; 946 } 947 } 948 public String getSystem() { 949 switch (this) { 950 case CLOSED: return "http://hl7.org/fhir/resource-slicing-rules"; 951 case OPEN: return "http://hl7.org/fhir/resource-slicing-rules"; 952 case OPENATEND: return "http://hl7.org/fhir/resource-slicing-rules"; 953 case NULL: return null; 954 default: return "?"; 955 } 956 } 957 public String getDefinition() { 958 switch (this) { 959 case CLOSED: return "No additional content is allowed other than that described by the slices in this profile."; 960 case OPEN: return "Additional content is allowed anywhere in the list."; 961 case OPENATEND: return "Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required."; 962 case NULL: return null; 963 default: return "?"; 964 } 965 } 966 public String getDisplay() { 967 switch (this) { 968 case CLOSED: return "Closed"; 969 case OPEN: return "Open"; 970 case OPENATEND: return "Open at End"; 971 case NULL: return null; 972 default: return "?"; 973 } 974 } 975 } 976 977 public static class SlicingRulesEnumFactory implements EnumFactory<SlicingRules> { 978 public SlicingRules fromCode(String codeString) throws IllegalArgumentException { 979 if (codeString == null || "".equals(codeString)) 980 if (codeString == null || "".equals(codeString)) 981 return null; 982 if ("closed".equals(codeString)) 983 return SlicingRules.CLOSED; 984 if ("open".equals(codeString)) 985 return SlicingRules.OPEN; 986 if ("openAtEnd".equals(codeString)) 987 return SlicingRules.OPENATEND; 988 throw new IllegalArgumentException("Unknown SlicingRules code '"+codeString+"'"); 989 } 990 public Enumeration<SlicingRules> fromType(PrimitiveType<?> code) throws FHIRException { 991 if (code == null) 992 return null; 993 if (code.isEmpty()) 994 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 995 String codeString = ((PrimitiveType) code).asStringValue(); 996 if (codeString == null || "".equals(codeString)) 997 return new Enumeration<SlicingRules>(this, SlicingRules.NULL, code); 998 if ("closed".equals(codeString)) 999 return new Enumeration<SlicingRules>(this, SlicingRules.CLOSED, code); 1000 if ("open".equals(codeString)) 1001 return new Enumeration<SlicingRules>(this, SlicingRules.OPEN, code); 1002 if ("openAtEnd".equals(codeString)) 1003 return new Enumeration<SlicingRules>(this, SlicingRules.OPENATEND, code); 1004 throw new FHIRException("Unknown SlicingRules code '"+codeString+"'"); 1005 } 1006 public String toCode(SlicingRules code) { 1007 if (code == SlicingRules.CLOSED) 1008 return "closed"; 1009 if (code == SlicingRules.OPEN) 1010 return "open"; 1011 if (code == SlicingRules.OPENATEND) 1012 return "openAtEnd"; 1013 return "?"; 1014 } 1015 public String toSystem(SlicingRules code) { 1016 return code.getSystem(); 1017 } 1018 } 1019 1020 @Block() 1021 public static class ElementDefinitionSlicingComponent extends Element implements IBaseDatatypeElement { 1022 /** 1023 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices. 1024 */ 1025 @Child(name = "discriminator", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1026 @Description(shortDefinition="Element values that are used to distinguish the slices", formalDefinition="Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices." ) 1027 protected List<ElementDefinitionSlicingDiscriminatorComponent> discriminator; 1028 1029 /** 1030 * A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 1031 */ 1032 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1033 @Description(shortDefinition="Text description of how slicing works (or not)", formalDefinition="A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated." ) 1034 protected StringType description; 1035 1036 /** 1037 * If the matching elements have to occur in the same order as defined in the profile. 1038 */ 1039 @Child(name = "ordered", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1040 @Description(shortDefinition="If elements must be in same order as slices", formalDefinition="If the matching elements have to occur in the same order as defined in the profile." ) 1041 protected BooleanType ordered; 1042 1043 /** 1044 * Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1045 */ 1046 @Child(name = "rules", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1047 @Description(shortDefinition="closed | open | openAtEnd", formalDefinition="Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end." ) 1048 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-slicing-rules") 1049 protected Enumeration<SlicingRules> rules; 1050 1051 private static final long serialVersionUID = -311635839L; 1052 1053 /** 1054 * Constructor 1055 */ 1056 public ElementDefinitionSlicingComponent() { 1057 super(); 1058 } 1059 1060 /** 1061 * Constructor 1062 */ 1063 public ElementDefinitionSlicingComponent(SlicingRules rules) { 1064 super(); 1065 this.setRules(rules); 1066 } 1067 1068 /** 1069 * @return {@link #discriminator} (Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.) 1070 */ 1071 public List<ElementDefinitionSlicingDiscriminatorComponent> getDiscriminator() { 1072 if (this.discriminator == null) 1073 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1074 return this.discriminator; 1075 } 1076 1077 /** 1078 * @return Returns a reference to <code>this</code> for easy method chaining 1079 */ 1080 public ElementDefinitionSlicingComponent setDiscriminator(List<ElementDefinitionSlicingDiscriminatorComponent> theDiscriminator) { 1081 this.discriminator = theDiscriminator; 1082 return this; 1083 } 1084 1085 public boolean hasDiscriminator() { 1086 if (this.discriminator == null) 1087 return false; 1088 for (ElementDefinitionSlicingDiscriminatorComponent item : this.discriminator) 1089 if (!item.isEmpty()) 1090 return true; 1091 return false; 1092 } 1093 1094 public ElementDefinitionSlicingDiscriminatorComponent addDiscriminator() { //3 1095 ElementDefinitionSlicingDiscriminatorComponent t = new ElementDefinitionSlicingDiscriminatorComponent(); 1096 if (this.discriminator == null) 1097 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1098 this.discriminator.add(t); 1099 return t; 1100 } 1101 1102 public ElementDefinitionSlicingComponent addDiscriminator(ElementDefinitionSlicingDiscriminatorComponent t) { //3 1103 if (t == null) 1104 return this; 1105 if (this.discriminator == null) 1106 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1107 this.discriminator.add(t); 1108 return this; 1109 } 1110 1111 /** 1112 * @return The first repetition of repeating field {@link #discriminator}, creating it if it does not already exist {3} 1113 */ 1114 public ElementDefinitionSlicingDiscriminatorComponent getDiscriminatorFirstRep() { 1115 if (getDiscriminator().isEmpty()) { 1116 addDiscriminator(); 1117 } 1118 return getDiscriminator().get(0); 1119 } 1120 1121 /** 1122 * @return {@link #description} (A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1123 */ 1124 public StringType getDescriptionElement() { 1125 if (this.description == null) 1126 if (Configuration.errorOnAutoCreate()) 1127 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.description"); 1128 else if (Configuration.doAutoCreate()) 1129 this.description = new StringType(); // bb 1130 return this.description; 1131 } 1132 1133 public boolean hasDescriptionElement() { 1134 return this.description != null && !this.description.isEmpty(); 1135 } 1136 1137 public boolean hasDescription() { 1138 return this.description != null && !this.description.isEmpty(); 1139 } 1140 1141 /** 1142 * @param value {@link #description} (A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1143 */ 1144 public ElementDefinitionSlicingComponent setDescriptionElement(StringType value) { 1145 this.description = value; 1146 return this; 1147 } 1148 1149 /** 1150 * @return A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 1151 */ 1152 public String getDescription() { 1153 return this.description == null ? null : this.description.getValue(); 1154 } 1155 1156 /** 1157 * @param value A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 1158 */ 1159 public ElementDefinitionSlicingComponent setDescription(String value) { 1160 if (Utilities.noString(value)) 1161 this.description = null; 1162 else { 1163 if (this.description == null) 1164 this.description = new StringType(); 1165 this.description.setValue(value); 1166 } 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #ordered} (If the matching elements have to occur in the same order as defined in the profile.). This is the underlying object with id, value and extensions. The accessor "getOrdered" gives direct access to the value 1172 */ 1173 public BooleanType getOrderedElement() { 1174 if (this.ordered == null) 1175 if (Configuration.errorOnAutoCreate()) 1176 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.ordered"); 1177 else if (Configuration.doAutoCreate()) 1178 this.ordered = new BooleanType(); // bb 1179 return this.ordered; 1180 } 1181 1182 public boolean hasOrderedElement() { 1183 return this.ordered != null && !this.ordered.isEmpty(); 1184 } 1185 1186 public boolean hasOrdered() { 1187 return this.ordered != null && !this.ordered.isEmpty(); 1188 } 1189 1190 /** 1191 * @param value {@link #ordered} (If the matching elements have to occur in the same order as defined in the profile.). This is the underlying object with id, value and extensions. The accessor "getOrdered" gives direct access to the value 1192 */ 1193 public ElementDefinitionSlicingComponent setOrderedElement(BooleanType value) { 1194 this.ordered = value; 1195 return this; 1196 } 1197 1198 /** 1199 * @return If the matching elements have to occur in the same order as defined in the profile. 1200 */ 1201 public boolean getOrdered() { 1202 return this.ordered == null || this.ordered.isEmpty() ? false : this.ordered.getValue(); 1203 } 1204 1205 /** 1206 * @param value If the matching elements have to occur in the same order as defined in the profile. 1207 */ 1208 public ElementDefinitionSlicingComponent setOrdered(boolean value) { 1209 if (this.ordered == null) 1210 this.ordered = new BooleanType(); 1211 this.ordered.setValue(value); 1212 return this; 1213 } 1214 1215 /** 1216 * @return {@link #rules} (Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.). This is the underlying object with id, value and extensions. The accessor "getRules" gives direct access to the value 1217 */ 1218 public Enumeration<SlicingRules> getRulesElement() { 1219 if (this.rules == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.rules"); 1222 else if (Configuration.doAutoCreate()) 1223 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); // bb 1224 return this.rules; 1225 } 1226 1227 public boolean hasRulesElement() { 1228 return this.rules != null && !this.rules.isEmpty(); 1229 } 1230 1231 public boolean hasRules() { 1232 return this.rules != null && !this.rules.isEmpty(); 1233 } 1234 1235 /** 1236 * @param value {@link #rules} (Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.). This is the underlying object with id, value and extensions. The accessor "getRules" gives direct access to the value 1237 */ 1238 public ElementDefinitionSlicingComponent setRulesElement(Enumeration<SlicingRules> value) { 1239 this.rules = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1245 */ 1246 public SlicingRules getRules() { 1247 return this.rules == null ? null : this.rules.getValue(); 1248 } 1249 1250 /** 1251 * @param value Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1252 */ 1253 public ElementDefinitionSlicingComponent setRules(SlicingRules value) { 1254 if (this.rules == null) 1255 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); 1256 this.rules.setValue(value); 1257 return this; 1258 } 1259 1260 protected void listChildren(List<Property> children) { 1261 super.listChildren(children); 1262 children.add(new Property("discriminator", "", "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 0, java.lang.Integer.MAX_VALUE, discriminator)); 1263 children.add(new Property("description", "string", "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 0, 1, description)); 1264 children.add(new Property("ordered", "boolean", "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered)); 1265 children.add(new Property("rules", "code", "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 0, 1, rules)); 1266 } 1267 1268 @Override 1269 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1270 switch (_hash) { 1271 case -1888270692: /*discriminator*/ return new Property("discriminator", "", "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 0, java.lang.Integer.MAX_VALUE, discriminator); 1272 case -1724546052: /*description*/ return new Property("description", "string", "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 0, 1, description); 1273 case -1207109523: /*ordered*/ return new Property("ordered", "boolean", "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered); 1274 case 108873975: /*rules*/ return new Property("rules", "code", "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 0, 1, rules); 1275 default: return super.getNamedProperty(_hash, _name, _checkValid); 1276 } 1277 1278 } 1279 1280 @Override 1281 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1282 switch (hash) { 1283 case -1888270692: /*discriminator*/ return this.discriminator == null ? new Base[0] : this.discriminator.toArray(new Base[this.discriminator.size()]); // ElementDefinitionSlicingDiscriminatorComponent 1284 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1285 case -1207109523: /*ordered*/ return this.ordered == null ? new Base[0] : new Base[] {this.ordered}; // BooleanType 1286 case 108873975: /*rules*/ return this.rules == null ? new Base[0] : new Base[] {this.rules}; // Enumeration<SlicingRules> 1287 default: return super.getProperty(hash, name, checkValid); 1288 } 1289 1290 } 1291 1292 @Override 1293 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1294 switch (hash) { 1295 case -1888270692: // discriminator 1296 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); // ElementDefinitionSlicingDiscriminatorComponent 1297 return value; 1298 case -1724546052: // description 1299 this.description = TypeConvertor.castToString(value); // StringType 1300 return value; 1301 case -1207109523: // ordered 1302 this.ordered = TypeConvertor.castToBoolean(value); // BooleanType 1303 return value; 1304 case 108873975: // rules 1305 value = new SlicingRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1306 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1307 return value; 1308 default: return super.setProperty(hash, name, value); 1309 } 1310 1311 } 1312 1313 @Override 1314 public Base setProperty(String name, Base value) throws FHIRException { 1315 if (name.equals("discriminator")) { 1316 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); 1317 } else if (name.equals("description")) { 1318 this.description = TypeConvertor.castToString(value); // StringType 1319 } else if (name.equals("ordered")) { 1320 this.ordered = TypeConvertor.castToBoolean(value); // BooleanType 1321 } else if (name.equals("rules")) { 1322 value = new SlicingRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1323 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1324 } else 1325 return super.setProperty(name, value); 1326 return value; 1327 } 1328 1329 @Override 1330 public void removeChild(String name, Base value) throws FHIRException { 1331 if (name.equals("discriminator")) { 1332 this.getDiscriminator().remove((ElementDefinitionSlicingDiscriminatorComponent) value); 1333 } else if (name.equals("description")) { 1334 this.description = null; 1335 } else if (name.equals("ordered")) { 1336 this.ordered = null; 1337 } else if (name.equals("rules")) { 1338 value = new SlicingRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1339 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1340 } else 1341 super.removeChild(name, value); 1342 1343 } 1344 1345 @Override 1346 public Base makeProperty(int hash, String name) throws FHIRException { 1347 switch (hash) { 1348 case -1888270692: return addDiscriminator(); 1349 case -1724546052: return getDescriptionElement(); 1350 case -1207109523: return getOrderedElement(); 1351 case 108873975: return getRulesElement(); 1352 default: return super.makeProperty(hash, name); 1353 } 1354 1355 } 1356 1357 @Override 1358 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1359 switch (hash) { 1360 case -1888270692: /*discriminator*/ return new String[] {}; 1361 case -1724546052: /*description*/ return new String[] {"string"}; 1362 case -1207109523: /*ordered*/ return new String[] {"boolean"}; 1363 case 108873975: /*rules*/ return new String[] {"code"}; 1364 default: return super.getTypesForProperty(hash, name); 1365 } 1366 1367 } 1368 1369 @Override 1370 public Base addChild(String name) throws FHIRException { 1371 if (name.equals("discriminator")) { 1372 return addDiscriminator(); 1373 } 1374 else if (name.equals("description")) { 1375 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.description"); 1376 } 1377 else if (name.equals("ordered")) { 1378 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.ordered"); 1379 } 1380 else if (name.equals("rules")) { 1381 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.rules"); 1382 } 1383 else 1384 return super.addChild(name); 1385 } 1386 1387 public ElementDefinitionSlicingComponent copy() { 1388 ElementDefinitionSlicingComponent dst = new ElementDefinitionSlicingComponent(); 1389 copyValues(dst); 1390 return dst; 1391 } 1392 1393 public void copyValues(ElementDefinitionSlicingComponent dst) { 1394 super.copyValues(dst); 1395 if (discriminator != null) { 1396 dst.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1397 for (ElementDefinitionSlicingDiscriminatorComponent i : discriminator) 1398 dst.discriminator.add(i.copy()); 1399 }; 1400 dst.description = description == null ? null : description.copy(); 1401 dst.ordered = ordered == null ? null : ordered.copy(); 1402 dst.rules = rules == null ? null : rules.copy(); 1403 } 1404 1405 @Override 1406 public boolean equalsDeep(Base other_) { 1407 if (!super.equalsDeep(other_)) 1408 return false; 1409 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1410 return false; 1411 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1412 return compareDeep(discriminator, o.discriminator, true) && compareDeep(description, o.description, true) 1413 && compareDeep(ordered, o.ordered, true) && compareDeep(rules, o.rules, true); 1414 } 1415 1416 @Override 1417 public boolean equalsShallow(Base other_) { 1418 if (!super.equalsShallow(other_)) 1419 return false; 1420 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1421 return false; 1422 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1423 return compareValues(description, o.description, true) && compareValues(ordered, o.ordered, true) && compareValues(rules, o.rules, true) 1424 ; 1425 } 1426 1427 public boolean isEmpty() { 1428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(discriminator, description 1429 , ordered, rules); 1430 } 1431 1432 public String fhirType() { 1433 return "ElementDefinition.slicing"; 1434 1435 } 1436 1437 @Override 1438 public String toString() { 1439 return (ordered == null ? "??" : "true".equals(ordered.asStringValue()) ? "ordered" : "unordered")+"/"+ 1440 (rules == null ? "??" : rules.asStringValue())+" "+discriminator.toString(); 1441 } 1442 } 1443 1444 @Block() 1445 public static class ElementDefinitionSlicingDiscriminatorComponent extends Element implements IBaseDatatypeElement { 1446 /** 1447 * How the element value is interpreted when discrimination is evaluated. 1448 */ 1449 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1450 @Description(shortDefinition="value | exists | type | profile | position", formalDefinition="How the element value is interpreted when discrimination is evaluated." ) 1451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/discriminator-type") 1452 protected Enumeration<DiscriminatorType> type; 1453 1454 /** 1455 * A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based. 1456 */ 1457 @Child(name = "path", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1458 @Description(shortDefinition="Path to element value", formalDefinition="A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based." ) 1459 protected StringType path; 1460 1461 private static final long serialVersionUID = 1151159293L; 1462 1463 /** 1464 * Constructor 1465 */ 1466 public ElementDefinitionSlicingDiscriminatorComponent() { 1467 super(); 1468 } 1469 1470 /** 1471 * Constructor 1472 */ 1473 public ElementDefinitionSlicingDiscriminatorComponent(DiscriminatorType type, String path) { 1474 super(); 1475 this.setType(type); 1476 this.setPath(path); 1477 } 1478 1479 /** 1480 * @return {@link #type} (How the element value is interpreted when discrimination is evaluated.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1481 */ 1482 public Enumeration<DiscriminatorType> getTypeElement() { 1483 if (this.type == null) 1484 if (Configuration.errorOnAutoCreate()) 1485 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.type"); 1486 else if (Configuration.doAutoCreate()) 1487 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); // bb 1488 return this.type; 1489 } 1490 1491 public boolean hasTypeElement() { 1492 return this.type != null && !this.type.isEmpty(); 1493 } 1494 1495 public boolean hasType() { 1496 return this.type != null && !this.type.isEmpty(); 1497 } 1498 1499 /** 1500 * @param value {@link #type} (How the element value is interpreted when discrimination is evaluated.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1501 */ 1502 public ElementDefinitionSlicingDiscriminatorComponent setTypeElement(Enumeration<DiscriminatorType> value) { 1503 this.type = value; 1504 return this; 1505 } 1506 1507 /** 1508 * @return How the element value is interpreted when discrimination is evaluated. 1509 */ 1510 public DiscriminatorType getType() { 1511 return this.type == null ? null : this.type.getValue(); 1512 } 1513 1514 /** 1515 * @param value How the element value is interpreted when discrimination is evaluated. 1516 */ 1517 public ElementDefinitionSlicingDiscriminatorComponent setType(DiscriminatorType value) { 1518 if (this.type == null) 1519 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); 1520 this.type.setValue(value); 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #path} (A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1526 */ 1527 public StringType getPathElement() { 1528 if (this.path == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.path"); 1531 else if (Configuration.doAutoCreate()) 1532 this.path = new StringType(); // bb 1533 return this.path; 1534 } 1535 1536 public boolean hasPathElement() { 1537 return this.path != null && !this.path.isEmpty(); 1538 } 1539 1540 public boolean hasPath() { 1541 return this.path != null && !this.path.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #path} (A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1546 */ 1547 public ElementDefinitionSlicingDiscriminatorComponent setPathElement(StringType value) { 1548 this.path = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based. 1554 */ 1555 public String getPath() { 1556 return this.path == null ? null : this.path.getValue(); 1557 } 1558 1559 /** 1560 * @param value A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based. 1561 */ 1562 public ElementDefinitionSlicingDiscriminatorComponent setPath(String value) { 1563 if (this.path == null) 1564 this.path = new StringType(); 1565 this.path.setValue(value); 1566 return this; 1567 } 1568 1569 protected void listChildren(List<Property> children) { 1570 super.listChildren(children); 1571 children.add(new Property("type", "code", "How the element value is interpreted when discrimination is evaluated.", 0, 1, type)); 1572 children.add(new Property("path", "string", "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 0, 1, path)); 1573 } 1574 1575 @Override 1576 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1577 switch (_hash) { 1578 case 3575610: /*type*/ return new Property("type", "code", "How the element value is interpreted when discrimination is evaluated.", 0, 1, type); 1579 case 3433509: /*path*/ return new Property("path", "string", "A FHIRPath expression, using [the simple subset of FHIRPath](fhirpath.html#simple), that is used to identify the element on which discrimination is based.", 0, 1, path); 1580 default: return super.getNamedProperty(_hash, _name, _checkValid); 1581 } 1582 1583 } 1584 1585 @Override 1586 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1587 switch (hash) { 1588 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DiscriminatorType> 1589 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1590 default: return super.getProperty(hash, name, checkValid); 1591 } 1592 1593 } 1594 1595 @Override 1596 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1597 switch (hash) { 1598 case 3575610: // type 1599 value = new DiscriminatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1600 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1601 return value; 1602 case 3433509: // path 1603 this.path = TypeConvertor.castToString(value); // StringType 1604 return value; 1605 default: return super.setProperty(hash, name, value); 1606 } 1607 1608 } 1609 1610 @Override 1611 public Base setProperty(String name, Base value) throws FHIRException { 1612 if (name.equals("type")) { 1613 value = new DiscriminatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1614 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1615 } else if (name.equals("path")) { 1616 this.path = TypeConvertor.castToString(value); // StringType 1617 } else 1618 return super.setProperty(name, value); 1619 return value; 1620 } 1621 1622 @Override 1623 public void removeChild(String name, Base value) throws FHIRException { 1624 if (name.equals("type")) { 1625 value = new DiscriminatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1626 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1627 } else if (name.equals("path")) { 1628 this.path = null; 1629 } else 1630 super.removeChild(name, value); 1631 1632 } 1633 1634 @Override 1635 public Base makeProperty(int hash, String name) throws FHIRException { 1636 switch (hash) { 1637 case 3575610: return getTypeElement(); 1638 case 3433509: return getPathElement(); 1639 default: return super.makeProperty(hash, name); 1640 } 1641 1642 } 1643 1644 @Override 1645 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1646 switch (hash) { 1647 case 3575610: /*type*/ return new String[] {"code"}; 1648 case 3433509: /*path*/ return new String[] {"string"}; 1649 default: return super.getTypesForProperty(hash, name); 1650 } 1651 1652 } 1653 1654 @Override 1655 public Base addChild(String name) throws FHIRException { 1656 if (name.equals("type")) { 1657 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.discriminator.type"); 1658 } 1659 else if (name.equals("path")) { 1660 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.slicing.discriminator.path"); 1661 } 1662 else 1663 return super.addChild(name); 1664 } 1665 1666 public ElementDefinitionSlicingDiscriminatorComponent copy() { 1667 ElementDefinitionSlicingDiscriminatorComponent dst = new ElementDefinitionSlicingDiscriminatorComponent(); 1668 copyValues(dst); 1669 return dst; 1670 } 1671 1672 public void copyValues(ElementDefinitionSlicingDiscriminatorComponent dst) { 1673 super.copyValues(dst); 1674 dst.type = type == null ? null : type.copy(); 1675 dst.path = path == null ? null : path.copy(); 1676 } 1677 1678 @Override 1679 public boolean equalsDeep(Base other_) { 1680 if (!super.equalsDeep(other_)) 1681 return false; 1682 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1683 return false; 1684 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1685 return compareDeep(type, o.type, true) && compareDeep(path, o.path, true); 1686 } 1687 1688 @Override 1689 public boolean equalsShallow(Base other_) { 1690 if (!super.equalsShallow(other_)) 1691 return false; 1692 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1693 return false; 1694 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1695 return compareValues(type, o.type, true) && compareValues(path, o.path, true); 1696 } 1697 1698 public boolean isEmpty() { 1699 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, path); 1700 } 1701 1702 public String fhirType() { 1703 return "ElementDefinition.slicing.discriminator"; 1704 1705 } 1706 1707 @Override 1708 public String toString() { 1709 return (type == null ? "??" : type.getCode()) + "="+(path == null ? "??" : path.asStringValue()); 1710 } 1711 1712 } 1713 1714 @Block() 1715 public static class ElementDefinitionBaseComponent extends Element implements IBaseDatatypeElement { 1716 /** 1717 * The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base. 1718 */ 1719 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1720 @Description(shortDefinition="Path that identifies the base element", formalDefinition="The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base." ) 1721 protected StringType path; 1722 1723 /** 1724 * Minimum cardinality of the base element identified by the path. 1725 */ 1726 @Child(name = "min", type = {UnsignedIntType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1727 @Description(shortDefinition="Min cardinality of the base element", formalDefinition="Minimum cardinality of the base element identified by the path." ) 1728 protected UnsignedIntType min; 1729 1730 /** 1731 * Maximum cardinality of the base element identified by the path. 1732 */ 1733 @Child(name = "max", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1734 @Description(shortDefinition="Max cardinality of the base element", formalDefinition="Maximum cardinality of the base element identified by the path." ) 1735 protected StringType max; 1736 1737 private static final long serialVersionUID = -1412704221L; 1738 1739 /** 1740 * Constructor 1741 */ 1742 public ElementDefinitionBaseComponent() { 1743 super(); 1744 } 1745 1746 /** 1747 * Constructor 1748 */ 1749 public ElementDefinitionBaseComponent(String path, int min, String max) { 1750 super(); 1751 this.setPath(path); 1752 this.setMin(min); 1753 this.setMax(max); 1754 } 1755 1756 /** 1757 * @return {@link #path} (The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1758 */ 1759 public StringType getPathElement() { 1760 if (this.path == null) 1761 if (Configuration.errorOnAutoCreate()) 1762 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.path"); 1763 else if (Configuration.doAutoCreate()) 1764 this.path = new StringType(); // bb 1765 return this.path; 1766 } 1767 1768 public boolean hasPathElement() { 1769 return this.path != null && !this.path.isEmpty(); 1770 } 1771 1772 public boolean hasPath() { 1773 return this.path != null && !this.path.isEmpty(); 1774 } 1775 1776 /** 1777 * @param value {@link #path} (The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1778 */ 1779 public ElementDefinitionBaseComponent setPathElement(StringType value) { 1780 this.path = value; 1781 return this; 1782 } 1783 1784 /** 1785 * @return The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base. 1786 */ 1787 public String getPath() { 1788 return this.path == null ? null : this.path.getValue(); 1789 } 1790 1791 /** 1792 * @param value The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base. 1793 */ 1794 public ElementDefinitionBaseComponent setPath(String value) { 1795 if (this.path == null) 1796 this.path = new StringType(); 1797 this.path.setValue(value); 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #min} (Minimum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 1803 */ 1804 public UnsignedIntType getMinElement() { 1805 if (this.min == null) 1806 if (Configuration.errorOnAutoCreate()) 1807 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.min"); 1808 else if (Configuration.doAutoCreate()) 1809 this.min = new UnsignedIntType(); // bb 1810 return this.min; 1811 } 1812 1813 public boolean hasMinElement() { 1814 return this.min != null && !this.min.isEmpty(); 1815 } 1816 1817 public boolean hasMin() { 1818 return this.min != null && !this.min.isEmpty(); 1819 } 1820 1821 /** 1822 * @param value {@link #min} (Minimum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 1823 */ 1824 public ElementDefinitionBaseComponent setMinElement(UnsignedIntType value) { 1825 this.min = value; 1826 return this; 1827 } 1828 1829 /** 1830 * @return Minimum cardinality of the base element identified by the path. 1831 */ 1832 public int getMin() { 1833 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 1834 } 1835 1836 /** 1837 * @param value Minimum cardinality of the base element identified by the path. 1838 */ 1839 public ElementDefinitionBaseComponent setMin(int value) { 1840 if (this.min == null) 1841 this.min = new UnsignedIntType(); 1842 this.min.setValue(value); 1843 return this; 1844 } 1845 1846 /** 1847 * @return {@link #max} (Maximum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 1848 */ 1849 public StringType getMaxElement() { 1850 if (this.max == null) 1851 if (Configuration.errorOnAutoCreate()) 1852 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.max"); 1853 else if (Configuration.doAutoCreate()) 1854 this.max = new StringType(); // bb 1855 return this.max; 1856 } 1857 1858 public boolean hasMaxElement() { 1859 return this.max != null && !this.max.isEmpty(); 1860 } 1861 1862 public boolean hasMax() { 1863 return this.max != null && !this.max.isEmpty(); 1864 } 1865 1866 /** 1867 * @param value {@link #max} (Maximum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 1868 */ 1869 public ElementDefinitionBaseComponent setMaxElement(StringType value) { 1870 this.max = value; 1871 return this; 1872 } 1873 1874 /** 1875 * @return Maximum cardinality of the base element identified by the path. 1876 */ 1877 public String getMax() { 1878 return this.max == null ? null : this.max.getValue(); 1879 } 1880 1881 /** 1882 * @param value Maximum cardinality of the base element identified by the path. 1883 */ 1884 public ElementDefinitionBaseComponent setMax(String value) { 1885 if (this.max == null) 1886 this.max = new StringType(); 1887 this.max.setValue(value); 1888 return this; 1889 } 1890 1891 protected void listChildren(List<Property> children) { 1892 super.listChildren(children); 1893 children.add(new Property("path", "string", "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.", 0, 1, path)); 1894 children.add(new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 0, 1, min)); 1895 children.add(new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max)); 1896 } 1897 1898 @Override 1899 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1900 switch (_hash) { 1901 case 3433509: /*path*/ return new Property("path", "string", "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [StructureDefinition](structuredefinition.html#) without a StructureDefinition.base.", 0, 1, path); 1902 case 108114: /*min*/ return new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 0, 1, min); 1903 case 107876: /*max*/ return new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max); 1904 default: return super.getNamedProperty(_hash, _name, _checkValid); 1905 } 1906 1907 } 1908 1909 @Override 1910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1911 switch (hash) { 1912 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1913 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 1914 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 1915 default: return super.getProperty(hash, name, checkValid); 1916 } 1917 1918 } 1919 1920 @Override 1921 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1922 switch (hash) { 1923 case 3433509: // path 1924 this.path = TypeConvertor.castToString(value); // StringType 1925 return value; 1926 case 108114: // min 1927 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1928 return value; 1929 case 107876: // max 1930 this.max = TypeConvertor.castToString(value); // StringType 1931 return value; 1932 default: return super.setProperty(hash, name, value); 1933 } 1934 1935 } 1936 1937 @Override 1938 public Base setProperty(String name, Base value) throws FHIRException { 1939 if (name.equals("path")) { 1940 this.path = TypeConvertor.castToString(value); // StringType 1941 } else if (name.equals("min")) { 1942 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1943 } else if (name.equals("max")) { 1944 this.max = TypeConvertor.castToString(value); // StringType 1945 } else 1946 return super.setProperty(name, value); 1947 return value; 1948 } 1949 1950 @Override 1951 public void removeChild(String name, Base value) throws FHIRException { 1952 if (name.equals("path")) { 1953 this.path = null; 1954 } else if (name.equals("min")) { 1955 this.min = null; 1956 } else if (name.equals("max")) { 1957 this.max = null; 1958 } else 1959 super.removeChild(name, value); 1960 1961 } 1962 1963 @Override 1964 public Base makeProperty(int hash, String name) throws FHIRException { 1965 switch (hash) { 1966 case 3433509: return getPathElement(); 1967 case 108114: return getMinElement(); 1968 case 107876: return getMaxElement(); 1969 default: return super.makeProperty(hash, name); 1970 } 1971 1972 } 1973 1974 @Override 1975 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1976 switch (hash) { 1977 case 3433509: /*path*/ return new String[] {"string"}; 1978 case 108114: /*min*/ return new String[] {"unsignedInt"}; 1979 case 107876: /*max*/ return new String[] {"string"}; 1980 default: return super.getTypesForProperty(hash, name); 1981 } 1982 1983 } 1984 1985 @Override 1986 public Base addChild(String name) throws FHIRException { 1987 if (name.equals("path")) { 1988 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.base.path"); 1989 } 1990 else if (name.equals("min")) { 1991 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.base.min"); 1992 } 1993 else if (name.equals("max")) { 1994 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.base.max"); 1995 } 1996 else 1997 return super.addChild(name); 1998 } 1999 2000 public ElementDefinitionBaseComponent copy() { 2001 ElementDefinitionBaseComponent dst = new ElementDefinitionBaseComponent(); 2002 copyValues(dst); 2003 return dst; 2004 } 2005 2006 public void copyValues(ElementDefinitionBaseComponent dst) { 2007 super.copyValues(dst); 2008 dst.path = path == null ? null : path.copy(); 2009 dst.min = min == null ? null : min.copy(); 2010 dst.max = max == null ? null : max.copy(); 2011 } 2012 2013 @Override 2014 public boolean equalsDeep(Base other_) { 2015 if (!super.equalsDeep(other_)) 2016 return false; 2017 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2018 return false; 2019 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2020 return compareDeep(path, o.path, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 2021 ; 2022 } 2023 2024 @Override 2025 public boolean equalsShallow(Base other_) { 2026 if (!super.equalsShallow(other_)) 2027 return false; 2028 if (!(other_ instanceof ElementDefinitionBaseComponent)) 2029 return false; 2030 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 2031 return compareValues(path, o.path, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 2032 ; 2033 } 2034 2035 public boolean isEmpty() { 2036 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, min, max); 2037 } 2038 2039 public String fhirType() { 2040 return "ElementDefinition.base"; 2041 2042 } 2043 2044 } 2045 2046 @Block() 2047 public static class TypeRefComponent extends Element implements IBaseDatatypeElement { 2048 /** 2049 * URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 2050 */ 2051 @Child(name = "code", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2052 @Description(shortDefinition="Data type or Resource (reference to definition)", formalDefinition="URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models." ) 2053 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/elementdefinition-types") 2054 protected UriType code; 2055 2056 /** 2057 * Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide. 2058 */ 2059 @Child(name = "profile", type = {CanonicalType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2060 @Description(shortDefinition="Profiles (StructureDefinition or IG) - one must apply", formalDefinition="Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide." ) 2061 protected List<CanonicalType> profile; 2062 2063 /** 2064 * Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide. 2065 */ 2066 @Child(name = "targetProfile", type = {CanonicalType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2067 @Description(shortDefinition="Profile (StructureDefinition or IG) on the Reference/canonical target - one must apply", formalDefinition="Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide." ) 2068 protected List<CanonicalType> targetProfile; 2069 2070 /** 2071 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2072 */ 2073 @Child(name = "aggregation", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2074 @Description(shortDefinition="contained | referenced | bundled - how aggregated", formalDefinition="If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle." ) 2075 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-aggregation-mode") 2076 protected List<Enumeration<AggregationMode>> aggregation; 2077 2078 /** 2079 * Whether this reference needs to be version specific or version independent, or whether either can be used. 2080 */ 2081 @Child(name = "versioning", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2082 @Description(shortDefinition="either | independent | specific", formalDefinition="Whether this reference needs to be version specific or version independent, or whether either can be used." ) 2083 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reference-version-rules") 2084 protected Enumeration<ReferenceVersionRules> versioning; 2085 2086 private static final long serialVersionUID = 957891653L; 2087 2088 /** 2089 * Constructor 2090 */ 2091 public TypeRefComponent() { 2092 super(); 2093 } 2094 2095 /** 2096 * Constructor 2097 */ 2098 public TypeRefComponent(String code) { 2099 super(); 2100 this.setCode(code); 2101 } 2102 2103 /** 2104 * @return {@link #code} (URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2105 */ 2106 public UriType getCodeElement() { 2107 if (this.code == null) 2108 if (Configuration.errorOnAutoCreate()) 2109 throw new Error("Attempt to auto-create TypeRefComponent.code"); 2110 else if (Configuration.doAutoCreate()) 2111 this.code = new UriType(); // bb 2112 return this.code; 2113 } 2114 2115 public boolean hasCodeElement() { 2116 return this.code != null && !this.code.isEmpty(); 2117 } 2118 2119 public boolean hasCode() { 2120 return this.code != null && !this.code.isEmpty(); 2121 } 2122 2123 /** 2124 * @param value {@link #code} (URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 2125 */ 2126 public TypeRefComponent setCodeElement(UriType value) { 2127 this.code = value; 2128 return this; 2129 } 2130 2131 /** 2132 * @return URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 2133 */ 2134 public String getCode() { 2135 return this.code == null ? null : this.code.getValue(); 2136 } 2137 2138 /** 2139 * @param value URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 2140 */ 2141 public TypeRefComponent setCode(String value) { 2142 if (this.code == null) 2143 this.code = new UriType(); 2144 this.code.setValue(value); 2145 return this; 2146 } 2147 2148 /** 2149 * @return {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2150 */ 2151 public List<CanonicalType> getProfile() { 2152 if (this.profile == null) 2153 this.profile = new ArrayList<CanonicalType>(); 2154 return this.profile; 2155 } 2156 2157 /** 2158 * @return Returns a reference to <code>this</code> for easy method chaining 2159 */ 2160 public TypeRefComponent setProfile(List<CanonicalType> theProfile) { 2161 this.profile = theProfile; 2162 return this; 2163 } 2164 2165 public boolean hasProfile() { 2166 if (this.profile == null) 2167 return false; 2168 for (CanonicalType item : this.profile) 2169 if (!item.isEmpty()) 2170 return true; 2171 return false; 2172 } 2173 2174 /** 2175 * @return {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2176 */ 2177 public CanonicalType addProfileElement() {//2 2178 CanonicalType t = new CanonicalType(); 2179 if (this.profile == null) 2180 this.profile = new ArrayList<CanonicalType>(); 2181 this.profile.add(t); 2182 return t; 2183 } 2184 2185 /** 2186 * @param value {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2187 */ 2188 public TypeRefComponent addProfile(String value) { //1 2189 CanonicalType t = new CanonicalType(); 2190 t.setValue(value); 2191 if (this.profile == null) 2192 this.profile = new ArrayList<CanonicalType>(); 2193 this.profile.add(t); 2194 return this; 2195 } 2196 2197 /** 2198 * @param value {@link #profile} (Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.) 2199 */ 2200 public boolean hasProfile(String value) { 2201 if (this.profile == null) 2202 return false; 2203 for (CanonicalType v : this.profile) 2204 if (v.getValue().equals(value)) // canonical 2205 return true; 2206 return false; 2207 } 2208 2209 /** 2210 * @return {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2211 */ 2212 public List<CanonicalType> getTargetProfile() { 2213 if (this.targetProfile == null) 2214 this.targetProfile = new ArrayList<CanonicalType>(); 2215 return this.targetProfile; 2216 } 2217 2218 /** 2219 * @return Returns a reference to <code>this</code> for easy method chaining 2220 */ 2221 public TypeRefComponent setTargetProfile(List<CanonicalType> theTargetProfile) { 2222 this.targetProfile = theTargetProfile; 2223 return this; 2224 } 2225 2226 public boolean hasTargetProfile() { 2227 if (this.targetProfile == null) 2228 return false; 2229 for (CanonicalType item : this.targetProfile) 2230 if (!item.isEmpty()) 2231 return true; 2232 return false; 2233 } 2234 2235 /** 2236 * @return {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2237 */ 2238 public CanonicalType addTargetProfileElement() {//2 2239 CanonicalType t = new CanonicalType(); 2240 if (this.targetProfile == null) 2241 this.targetProfile = new ArrayList<CanonicalType>(); 2242 this.targetProfile.add(t); 2243 return t; 2244 } 2245 2246 /** 2247 * @param value {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2248 */ 2249 public TypeRefComponent addTargetProfile(String value) { //1 2250 CanonicalType t = new CanonicalType(); 2251 t.setValue(value); 2252 if (this.targetProfile == null) 2253 this.targetProfile = new ArrayList<CanonicalType>(); 2254 this.targetProfile.add(t); 2255 return this; 2256 } 2257 2258 /** 2259 * @param value {@link #targetProfile} (Used when the type is "Reference" or "canonical", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.) 2260 */ 2261 public boolean hasTargetProfile(String value) { 2262 if (this.targetProfile == null) 2263 return false; 2264 for (CanonicalType v : this.targetProfile) 2265 if (v.getValue().equals(value)) // canonical 2266 return true; 2267 return false; 2268 } 2269 2270 /** 2271 * @return {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2272 */ 2273 public List<Enumeration<AggregationMode>> getAggregation() { 2274 if (this.aggregation == null) 2275 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2276 return this.aggregation; 2277 } 2278 2279 /** 2280 * @return Returns a reference to <code>this</code> for easy method chaining 2281 */ 2282 public TypeRefComponent setAggregation(List<Enumeration<AggregationMode>> theAggregation) { 2283 this.aggregation = theAggregation; 2284 return this; 2285 } 2286 2287 public boolean hasAggregation() { 2288 if (this.aggregation == null) 2289 return false; 2290 for (Enumeration<AggregationMode> item : this.aggregation) 2291 if (!item.isEmpty()) 2292 return true; 2293 return false; 2294 } 2295 2296 /** 2297 * @return {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2298 */ 2299 public Enumeration<AggregationMode> addAggregationElement() {//2 2300 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2301 if (this.aggregation == null) 2302 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2303 this.aggregation.add(t); 2304 return t; 2305 } 2306 2307 /** 2308 * @param value {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2309 */ 2310 public TypeRefComponent addAggregation(AggregationMode value) { //1 2311 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 2312 t.setValue(value); 2313 if (this.aggregation == null) 2314 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2315 this.aggregation.add(t); 2316 return this; 2317 } 2318 2319 /** 2320 * @param value {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2321 */ 2322 public boolean hasAggregation(AggregationMode value) { 2323 if (this.aggregation == null) 2324 return false; 2325 for (Enumeration<AggregationMode> v : this.aggregation) 2326 if (v.getValue().equals(value)) // code 2327 return true; 2328 return false; 2329 } 2330 2331 /** 2332 * @return {@link #versioning} (Whether this reference needs to be version specific or version independent, or whether either can be used.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 2333 */ 2334 public Enumeration<ReferenceVersionRules> getVersioningElement() { 2335 if (this.versioning == null) 2336 if (Configuration.errorOnAutoCreate()) 2337 throw new Error("Attempt to auto-create TypeRefComponent.versioning"); 2338 else if (Configuration.doAutoCreate()) 2339 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); // bb 2340 return this.versioning; 2341 } 2342 2343 public boolean hasVersioningElement() { 2344 return this.versioning != null && !this.versioning.isEmpty(); 2345 } 2346 2347 public boolean hasVersioning() { 2348 return this.versioning != null && !this.versioning.isEmpty(); 2349 } 2350 2351 /** 2352 * @param value {@link #versioning} (Whether this reference needs to be version specific or version independent, or whether either can be used.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 2353 */ 2354 public TypeRefComponent setVersioningElement(Enumeration<ReferenceVersionRules> value) { 2355 this.versioning = value; 2356 return this; 2357 } 2358 2359 /** 2360 * @return Whether this reference needs to be version specific or version independent, or whether either can be used. 2361 */ 2362 public ReferenceVersionRules getVersioning() { 2363 return this.versioning == null ? null : this.versioning.getValue(); 2364 } 2365 2366 /** 2367 * @param value Whether this reference needs to be version specific or version independent, or whether either can be used. 2368 */ 2369 public TypeRefComponent setVersioning(ReferenceVersionRules value) { 2370 if (value == null) 2371 this.versioning = null; 2372 else { 2373 if (this.versioning == null) 2374 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); 2375 this.versioning.setValue(value); 2376 } 2377 return this; 2378 } 2379 2380 protected void listChildren(List<Property> children) { 2381 super.listChildren(children); 2382 children.add(new Property("code", "uri", "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 0, 1, code)); 2383 children.add(new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, profile)); 2384 children.add(new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, targetProfile)); 2385 children.add(new Property("aggregation", "code", "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 0, java.lang.Integer.MAX_VALUE, aggregation)); 2386 children.add(new Property("versioning", "code", "Whether this reference needs to be version specific or version independent, or whether either can be used.", 0, 1, versioning)); 2387 } 2388 2389 @Override 2390 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2391 switch (_hash) { 2392 case 3059181: /*code*/ return new Property("code", "uri", "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 0, 1, code); 2393 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition|ImplementationGuide)", "Identifies a profile structure or implementation Guide that applies to the datatype this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the type SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, profile); 2394 case 1994521304: /*targetProfile*/ return new Property("targetProfile", "canonical(StructureDefinition|ImplementationGuide)", "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this element refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 0, java.lang.Integer.MAX_VALUE, targetProfile); 2395 case 841524962: /*aggregation*/ return new Property("aggregation", "code", "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 0, java.lang.Integer.MAX_VALUE, aggregation); 2396 case -670487542: /*versioning*/ return new Property("versioning", "code", "Whether this reference needs to be version specific or version independent, or whether either can be used.", 0, 1, versioning); 2397 default: return super.getNamedProperty(_hash, _name, _checkValid); 2398 } 2399 2400 } 2401 2402 @Override 2403 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2404 switch (hash) { 2405 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // UriType 2406 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 2407 case 1994521304: /*targetProfile*/ return this.targetProfile == null ? new Base[0] : this.targetProfile.toArray(new Base[this.targetProfile.size()]); // CanonicalType 2408 case 841524962: /*aggregation*/ return this.aggregation == null ? new Base[0] : this.aggregation.toArray(new Base[this.aggregation.size()]); // Enumeration<AggregationMode> 2409 case -670487542: /*versioning*/ return this.versioning == null ? new Base[0] : new Base[] {this.versioning}; // Enumeration<ReferenceVersionRules> 2410 default: return super.getProperty(hash, name, checkValid); 2411 } 2412 2413 } 2414 2415 @Override 2416 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2417 switch (hash) { 2418 case 3059181: // code 2419 this.code = TypeConvertor.castToUri(value); // UriType 2420 return value; 2421 case -309425751: // profile 2422 this.getProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2423 return value; 2424 case 1994521304: // targetProfile 2425 this.getTargetProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2426 return value; 2427 case 841524962: // aggregation 2428 value = new AggregationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2429 this.getAggregation().add((Enumeration) value); // Enumeration<AggregationMode> 2430 return value; 2431 case -670487542: // versioning 2432 value = new ReferenceVersionRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2433 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2434 return value; 2435 default: return super.setProperty(hash, name, value); 2436 } 2437 2438 } 2439 2440 @Override 2441 public Base setProperty(String name, Base value) throws FHIRException { 2442 if (name.equals("code")) { 2443 this.code = TypeConvertor.castToUri(value); // UriType 2444 } else if (name.equals("profile")) { 2445 this.getProfile().add(TypeConvertor.castToCanonical(value)); 2446 } else if (name.equals("targetProfile")) { 2447 this.getTargetProfile().add(TypeConvertor.castToCanonical(value)); 2448 } else if (name.equals("aggregation")) { 2449 value = new AggregationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2450 this.getAggregation().add((Enumeration) value); 2451 } else if (name.equals("versioning")) { 2452 value = new ReferenceVersionRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2453 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2454 } else 2455 return super.setProperty(name, value); 2456 return value; 2457 } 2458 2459 @Override 2460 public void removeChild(String name, Base value) throws FHIRException { 2461 if (name.equals("code")) { 2462 this.code = null; 2463 } else if (name.equals("profile")) { 2464 this.getProfile().remove(value); 2465 } else if (name.equals("targetProfile")) { 2466 this.getTargetProfile().remove(value); 2467 } else if (name.equals("aggregation")) { 2468 value = new AggregationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2469 this.getAggregation().remove((Enumeration) value); 2470 } else if (name.equals("versioning")) { 2471 value = new ReferenceVersionRulesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2472 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2473 } else 2474 super.removeChild(name, value); 2475 2476 } 2477 2478 @Override 2479 public Base makeProperty(int hash, String name) throws FHIRException { 2480 switch (hash) { 2481 case 3059181: return getCodeElement(); 2482 case -309425751: return addProfileElement(); 2483 case 1994521304: return addTargetProfileElement(); 2484 case 841524962: return addAggregationElement(); 2485 case -670487542: return getVersioningElement(); 2486 default: return super.makeProperty(hash, name); 2487 } 2488 2489 } 2490 2491 @Override 2492 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2493 switch (hash) { 2494 case 3059181: /*code*/ return new String[] {"uri"}; 2495 case -309425751: /*profile*/ return new String[] {"canonical"}; 2496 case 1994521304: /*targetProfile*/ return new String[] {"canonical"}; 2497 case 841524962: /*aggregation*/ return new String[] {"code"}; 2498 case -670487542: /*versioning*/ return new String[] {"code"}; 2499 default: return super.getTypesForProperty(hash, name); 2500 } 2501 2502 } 2503 2504 @Override 2505 public Base addChild(String name) throws FHIRException { 2506 if (name.equals("code")) { 2507 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.code"); 2508 } 2509 else if (name.equals("profile")) { 2510 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.profile"); 2511 } 2512 else if (name.equals("targetProfile")) { 2513 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.targetProfile"); 2514 } 2515 else if (name.equals("aggregation")) { 2516 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.aggregation"); 2517 } 2518 else if (name.equals("versioning")) { 2519 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type.versioning"); 2520 } 2521 else 2522 return super.addChild(name); 2523 } 2524 2525 public TypeRefComponent copy() { 2526 TypeRefComponent dst = new TypeRefComponent(); 2527 copyValues(dst); 2528 return dst; 2529 } 2530 2531 public void copyValues(TypeRefComponent dst) { 2532 super.copyValues(dst); 2533 dst.code = code == null ? null : code.copy(); 2534 if (profile != null) { 2535 dst.profile = new ArrayList<CanonicalType>(); 2536 for (CanonicalType i : profile) 2537 dst.profile.add(i.copy()); 2538 }; 2539 if (targetProfile != null) { 2540 dst.targetProfile = new ArrayList<CanonicalType>(); 2541 for (CanonicalType i : targetProfile) 2542 dst.targetProfile.add(i.copy()); 2543 }; 2544 if (aggregation != null) { 2545 dst.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2546 for (Enumeration<AggregationMode> i : aggregation) 2547 dst.aggregation.add(i.copy()); 2548 }; 2549 dst.versioning = versioning == null ? null : versioning.copy(); 2550 } 2551 2552 @Override 2553 public boolean equalsDeep(Base other_) { 2554 if (!super.equalsDeep(other_)) 2555 return false; 2556 if (!(other_ instanceof TypeRefComponent)) 2557 return false; 2558 TypeRefComponent o = (TypeRefComponent) other_; 2559 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(targetProfile, o.targetProfile, true) 2560 && compareDeep(aggregation, o.aggregation, true) && compareDeep(versioning, o.versioning, true) 2561 ; 2562 } 2563 2564 @Override 2565 public boolean equalsShallow(Base other_) { 2566 if (!super.equalsShallow(other_)) 2567 return false; 2568 if (!(other_ instanceof TypeRefComponent)) 2569 return false; 2570 TypeRefComponent o = (TypeRefComponent) other_; 2571 return compareValues(code, o.code, true) && compareValues(profile, o.profile, true) && compareValues(targetProfile, o.targetProfile, true) 2572 && compareValues(aggregation, o.aggregation, true) && compareValues(versioning, o.versioning, true) 2573 ; 2574 } 2575 2576 public boolean isEmpty() { 2577 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, targetProfile 2578 , aggregation, versioning); 2579 } 2580 2581 public String fhirType() { 2582 return "ElementDefinition.type"; 2583 2584 } 2585 2586// added from java-adornments.txt: 2587public boolean hasTarget() { 2588 return Utilities.existsInList(getCode(), "Reference", "canonical", "CodeableReference"); 2589 } 2590 2591 /** 2592 * This code checks for the system prefix and returns the FHIR type 2593 * 2594 * @return 2595 */ 2596 public String getWorkingCode() { 2597 if (hasExtension(ToolingExtensions.EXT_FHIR_TYPE)) 2598 return getExtensionString(ToolingExtensions.EXT_FHIR_TYPE); 2599 if (!hasCodeElement()) 2600 return null; 2601 if (getCodeElement().hasExtension(ToolingExtensions.EXT_XML_TYPE)) { 2602 String s = getCodeElement().getExtensionString(ToolingExtensions.EXT_XML_TYPE); 2603 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date OR xsd:dateTime".equalsIgnoreCase(s)) 2604 return "dateTime"; 2605 if ("xsd:gYear OR xsd:gYearMonth OR xsd:date".equalsIgnoreCase(s)) 2606 return "date"; 2607 if ("xsd:dateTime".equalsIgnoreCase(s)) 2608 return "instant"; 2609 if ("xsd:token".equals(s)) 2610 return "code"; 2611 if ("xsd:boolean".equals(s)) 2612 return "boolean"; 2613 if ("xsd:string".equals(s)) 2614 return "string"; 2615 if ("xsd:time".equals(s)) 2616 return "time"; 2617 if ("xsd:int".equals(s)) 2618 return "integer"; 2619 if ("xsd:decimal OR xsd:double".equals(s)) 2620 return "decimal"; 2621 if ("xsd:decimal".equalsIgnoreCase(s)) 2622 return "decimal"; 2623 if ("xsd:base64Binary".equalsIgnoreCase(s)) 2624 return "base64Binary"; 2625 if ("xsd:positiveInteger".equalsIgnoreCase(s)) 2626 return "positiveInt"; 2627 if ("xsd:nonNegativeInteger".equalsIgnoreCase(s)) 2628 return "unsignedInt"; 2629 if ("xsd:anyURI".equalsIgnoreCase(s)) 2630 return "uri"; 2631 if ("xhtml:div".equalsIgnoreCase(s)) 2632 return "xhtml"; 2633 2634 throw new Error("Unknown xml type '"+s+"'"); 2635 } 2636 return getCode(); 2637 } 2638 2639 @Override 2640 public String toString() { 2641 String res = getCode(); 2642 if (hasProfile()) { 2643 res = res + "{"; 2644 boolean first = true; 2645 for (CanonicalType s : getProfile()) { 2646 if (first) first = false; else res = res + "|"; 2647 res = res + s.getValue(); 2648 } 2649 res = res + "}"; 2650 } 2651 if (hasTargetProfile()) { 2652 res = res + "("; 2653 boolean first = true; 2654 for (CanonicalType s : getTargetProfile()) { 2655 if (first) first = false; else res = res + "|"; 2656 res = res + s.getValue(); 2657 } 2658 res = res + ")"; 2659 } 2660 return res; 2661 } 2662 2663 public String getName() { 2664 return getWorkingCode(); 2665 } 2666 2667 public boolean isResourceReference() { 2668 return "Reference".equals(getCode()) && hasTargetProfile(); 2669 } 2670// end addition 2671 } 2672 2673 @Block() 2674 public static class ElementDefinitionExampleComponent extends Element implements IBaseDatatypeElement { 2675 /** 2676 * Describes the purpose of this example among the set of examples. 2677 */ 2678 @Child(name = "label", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2679 @Description(shortDefinition="Describes the purpose of this example", formalDefinition="Describes the purpose of this example among the set of examples." ) 2680 protected StringType label; 2681 2682 /** 2683 * The actual value for the element, which must be one of the types allowed for this element. 2684 */ 2685 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=2, min=1, max=1, modifier=false, summary=true) 2686 @Description(shortDefinition="Value of Example (one of allowed types)", formalDefinition="The actual value for the element, which must be one of the types allowed for this element." ) 2687 protected DataType value; 2688 2689 private static final long serialVersionUID = 463190922L; 2690 2691 /** 2692 * Constructor 2693 */ 2694 public ElementDefinitionExampleComponent() { 2695 super(); 2696 } 2697 2698 /** 2699 * Constructor 2700 */ 2701 public ElementDefinitionExampleComponent(String label, DataType value) { 2702 super(); 2703 this.setLabel(label); 2704 this.setValue(value); 2705 } 2706 2707 /** 2708 * @return {@link #label} (Describes the purpose of this example among the set of examples.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2709 */ 2710 public StringType getLabelElement() { 2711 if (this.label == null) 2712 if (Configuration.errorOnAutoCreate()) 2713 throw new Error("Attempt to auto-create ElementDefinitionExampleComponent.label"); 2714 else if (Configuration.doAutoCreate()) 2715 this.label = new StringType(); // bb 2716 return this.label; 2717 } 2718 2719 public boolean hasLabelElement() { 2720 return this.label != null && !this.label.isEmpty(); 2721 } 2722 2723 public boolean hasLabel() { 2724 return this.label != null && !this.label.isEmpty(); 2725 } 2726 2727 /** 2728 * @param value {@link #label} (Describes the purpose of this example among the set of examples.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2729 */ 2730 public ElementDefinitionExampleComponent setLabelElement(StringType value) { 2731 this.label = value; 2732 return this; 2733 } 2734 2735 /** 2736 * @return Describes the purpose of this example among the set of examples. 2737 */ 2738 public String getLabel() { 2739 return this.label == null ? null : this.label.getValue(); 2740 } 2741 2742 /** 2743 * @param value Describes the purpose of this example among the set of examples. 2744 */ 2745 public ElementDefinitionExampleComponent setLabel(String value) { 2746 if (this.label == null) 2747 this.label = new StringType(); 2748 this.label.setValue(value); 2749 return this; 2750 } 2751 2752 /** 2753 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2754 */ 2755 public DataType getValue() { 2756 return this.value; 2757 } 2758 2759 /** 2760 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2761 */ 2762 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 2763 if (this.value == null) 2764 this.value = new Base64BinaryType(); 2765 if (!(this.value instanceof Base64BinaryType)) 2766 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 2767 return (Base64BinaryType) this.value; 2768 } 2769 2770 public boolean hasValueBase64BinaryType() { 2771 return this != null && this.value instanceof Base64BinaryType; 2772 } 2773 2774 /** 2775 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2776 */ 2777 public BooleanType getValueBooleanType() throws FHIRException { 2778 if (this.value == null) 2779 this.value = new BooleanType(); 2780 if (!(this.value instanceof BooleanType)) 2781 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2782 return (BooleanType) this.value; 2783 } 2784 2785 public boolean hasValueBooleanType() { 2786 return this != null && this.value instanceof BooleanType; 2787 } 2788 2789 /** 2790 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2791 */ 2792 public CanonicalType getValueCanonicalType() throws FHIRException { 2793 if (this.value == null) 2794 this.value = new CanonicalType(); 2795 if (!(this.value instanceof CanonicalType)) 2796 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2797 return (CanonicalType) this.value; 2798 } 2799 2800 public boolean hasValueCanonicalType() { 2801 return this != null && this.value instanceof CanonicalType; 2802 } 2803 2804 /** 2805 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2806 */ 2807 public CodeType getValueCodeType() throws FHIRException { 2808 if (this.value == null) 2809 this.value = new CodeType(); 2810 if (!(this.value instanceof CodeType)) 2811 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2812 return (CodeType) this.value; 2813 } 2814 2815 public boolean hasValueCodeType() { 2816 return this != null && this.value instanceof CodeType; 2817 } 2818 2819 /** 2820 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2821 */ 2822 public DateType getValueDateType() throws FHIRException { 2823 if (this.value == null) 2824 this.value = new DateType(); 2825 if (!(this.value instanceof DateType)) 2826 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 2827 return (DateType) this.value; 2828 } 2829 2830 public boolean hasValueDateType() { 2831 return this != null && this.value instanceof DateType; 2832 } 2833 2834 /** 2835 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2836 */ 2837 public DateTimeType getValueDateTimeType() throws FHIRException { 2838 if (this.value == null) 2839 this.value = new DateTimeType(); 2840 if (!(this.value instanceof DateTimeType)) 2841 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2842 return (DateTimeType) this.value; 2843 } 2844 2845 public boolean hasValueDateTimeType() { 2846 return this != null && this.value instanceof DateTimeType; 2847 } 2848 2849 /** 2850 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2851 */ 2852 public DecimalType getValueDecimalType() throws FHIRException { 2853 if (this.value == null) 2854 this.value = new DecimalType(); 2855 if (!(this.value instanceof DecimalType)) 2856 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2857 return (DecimalType) this.value; 2858 } 2859 2860 public boolean hasValueDecimalType() { 2861 return this != null && this.value instanceof DecimalType; 2862 } 2863 2864 /** 2865 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2866 */ 2867 public IdType getValueIdType() throws FHIRException { 2868 if (this.value == null) 2869 this.value = new IdType(); 2870 if (!(this.value instanceof IdType)) 2871 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 2872 return (IdType) this.value; 2873 } 2874 2875 public boolean hasValueIdType() { 2876 return this != null && this.value instanceof IdType; 2877 } 2878 2879 /** 2880 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2881 */ 2882 public InstantType getValueInstantType() throws FHIRException { 2883 if (this.value == null) 2884 this.value = new InstantType(); 2885 if (!(this.value instanceof InstantType)) 2886 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 2887 return (InstantType) this.value; 2888 } 2889 2890 public boolean hasValueInstantType() { 2891 return this != null && this.value instanceof InstantType; 2892 } 2893 2894 /** 2895 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2896 */ 2897 public IntegerType getValueIntegerType() throws FHIRException { 2898 if (this.value == null) 2899 this.value = new IntegerType(); 2900 if (!(this.value instanceof IntegerType)) 2901 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2902 return (IntegerType) this.value; 2903 } 2904 2905 public boolean hasValueIntegerType() { 2906 return this != null && this.value instanceof IntegerType; 2907 } 2908 2909 /** 2910 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2911 */ 2912 public Integer64Type getValueInteger64Type() throws FHIRException { 2913 if (this.value == null) 2914 this.value = new Integer64Type(); 2915 if (!(this.value instanceof Integer64Type)) 2916 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 2917 return (Integer64Type) this.value; 2918 } 2919 2920 public boolean hasValueInteger64Type() { 2921 return this != null && this.value instanceof Integer64Type; 2922 } 2923 2924 /** 2925 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2926 */ 2927 public MarkdownType getValueMarkdownType() throws FHIRException { 2928 if (this.value == null) 2929 this.value = new MarkdownType(); 2930 if (!(this.value instanceof MarkdownType)) 2931 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 2932 return (MarkdownType) this.value; 2933 } 2934 2935 public boolean hasValueMarkdownType() { 2936 return this != null && this.value instanceof MarkdownType; 2937 } 2938 2939 /** 2940 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2941 */ 2942 public OidType getValueOidType() throws FHIRException { 2943 if (this.value == null) 2944 this.value = new OidType(); 2945 if (!(this.value instanceof OidType)) 2946 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 2947 return (OidType) this.value; 2948 } 2949 2950 public boolean hasValueOidType() { 2951 return this != null && this.value instanceof OidType; 2952 } 2953 2954 /** 2955 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2956 */ 2957 public PositiveIntType getValuePositiveIntType() throws FHIRException { 2958 if (this.value == null) 2959 this.value = new PositiveIntType(); 2960 if (!(this.value instanceof PositiveIntType)) 2961 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 2962 return (PositiveIntType) this.value; 2963 } 2964 2965 public boolean hasValuePositiveIntType() { 2966 return this != null && this.value instanceof PositiveIntType; 2967 } 2968 2969 /** 2970 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2971 */ 2972 public StringType getValueStringType() throws FHIRException { 2973 if (this.value == null) 2974 this.value = new StringType(); 2975 if (!(this.value instanceof StringType)) 2976 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2977 return (StringType) this.value; 2978 } 2979 2980 public boolean hasValueStringType() { 2981 return this != null && this.value instanceof StringType; 2982 } 2983 2984 /** 2985 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2986 */ 2987 public TimeType getValueTimeType() throws FHIRException { 2988 if (this.value == null) 2989 this.value = new TimeType(); 2990 if (!(this.value instanceof TimeType)) 2991 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2992 return (TimeType) this.value; 2993 } 2994 2995 public boolean hasValueTimeType() { 2996 return this != null && this.value instanceof TimeType; 2997 } 2998 2999 /** 3000 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3001 */ 3002 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 3003 if (this.value == null) 3004 this.value = new UnsignedIntType(); 3005 if (!(this.value instanceof UnsignedIntType)) 3006 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 3007 return (UnsignedIntType) this.value; 3008 } 3009 3010 public boolean hasValueUnsignedIntType() { 3011 return this != null && this.value instanceof UnsignedIntType; 3012 } 3013 3014 /** 3015 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3016 */ 3017 public UriType getValueUriType() throws FHIRException { 3018 if (this.value == null) 3019 this.value = new UriType(); 3020 if (!(this.value instanceof UriType)) 3021 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 3022 return (UriType) this.value; 3023 } 3024 3025 public boolean hasValueUriType() { 3026 return this != null && this.value instanceof UriType; 3027 } 3028 3029 /** 3030 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3031 */ 3032 public UrlType getValueUrlType() throws FHIRException { 3033 if (this.value == null) 3034 this.value = new UrlType(); 3035 if (!(this.value instanceof UrlType)) 3036 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 3037 return (UrlType) this.value; 3038 } 3039 3040 public boolean hasValueUrlType() { 3041 return this != null && this.value instanceof UrlType; 3042 } 3043 3044 /** 3045 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3046 */ 3047 public UuidType getValueUuidType() throws FHIRException { 3048 if (this.value == null) 3049 this.value = new UuidType(); 3050 if (!(this.value instanceof UuidType)) 3051 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 3052 return (UuidType) this.value; 3053 } 3054 3055 public boolean hasValueUuidType() { 3056 return this != null && this.value instanceof UuidType; 3057 } 3058 3059 /** 3060 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3061 */ 3062 public Address getValueAddress() throws FHIRException { 3063 if (this.value == null) 3064 this.value = new Address(); 3065 if (!(this.value instanceof Address)) 3066 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 3067 return (Address) this.value; 3068 } 3069 3070 public boolean hasValueAddress() { 3071 return this != null && this.value instanceof Address; 3072 } 3073 3074 /** 3075 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3076 */ 3077 public Age getValueAge() throws FHIRException { 3078 if (this.value == null) 3079 this.value = new Age(); 3080 if (!(this.value instanceof Age)) 3081 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 3082 return (Age) this.value; 3083 } 3084 3085 public boolean hasValueAge() { 3086 return this != null && this.value instanceof Age; 3087 } 3088 3089 /** 3090 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3091 */ 3092 public Annotation getValueAnnotation() throws FHIRException { 3093 if (this.value == null) 3094 this.value = new Annotation(); 3095 if (!(this.value instanceof Annotation)) 3096 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 3097 return (Annotation) this.value; 3098 } 3099 3100 public boolean hasValueAnnotation() { 3101 return this != null && this.value instanceof Annotation; 3102 } 3103 3104 /** 3105 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3106 */ 3107 public Attachment getValueAttachment() throws FHIRException { 3108 if (this.value == null) 3109 this.value = new Attachment(); 3110 if (!(this.value instanceof Attachment)) 3111 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 3112 return (Attachment) this.value; 3113 } 3114 3115 public boolean hasValueAttachment() { 3116 return this != null && this.value instanceof Attachment; 3117 } 3118 3119 /** 3120 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3121 */ 3122 public CodeableConcept getValueCodeableConcept() throws FHIRException { 3123 if (this.value == null) 3124 this.value = new CodeableConcept(); 3125 if (!(this.value instanceof CodeableConcept)) 3126 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 3127 return (CodeableConcept) this.value; 3128 } 3129 3130 public boolean hasValueCodeableConcept() { 3131 return this != null && this.value instanceof CodeableConcept; 3132 } 3133 3134 /** 3135 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3136 */ 3137 public CodeableReference getValueCodeableReference() throws FHIRException { 3138 if (this.value == null) 3139 this.value = new CodeableReference(); 3140 if (!(this.value instanceof CodeableReference)) 3141 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 3142 return (CodeableReference) this.value; 3143 } 3144 3145 public boolean hasValueCodeableReference() { 3146 return this != null && this.value instanceof CodeableReference; 3147 } 3148 3149 /** 3150 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3151 */ 3152 public Coding getValueCoding() throws FHIRException { 3153 if (this.value == null) 3154 this.value = new Coding(); 3155 if (!(this.value instanceof Coding)) 3156 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 3157 return (Coding) this.value; 3158 } 3159 3160 public boolean hasValueCoding() { 3161 return this != null && this.value instanceof Coding; 3162 } 3163 3164 /** 3165 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3166 */ 3167 public ContactPoint getValueContactPoint() throws FHIRException { 3168 if (this.value == null) 3169 this.value = new ContactPoint(); 3170 if (!(this.value instanceof ContactPoint)) 3171 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 3172 return (ContactPoint) this.value; 3173 } 3174 3175 public boolean hasValueContactPoint() { 3176 return this != null && this.value instanceof ContactPoint; 3177 } 3178 3179 /** 3180 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3181 */ 3182 public Count getValueCount() throws FHIRException { 3183 if (this.value == null) 3184 this.value = new Count(); 3185 if (!(this.value instanceof Count)) 3186 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 3187 return (Count) this.value; 3188 } 3189 3190 public boolean hasValueCount() { 3191 return this != null && this.value instanceof Count; 3192 } 3193 3194 /** 3195 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3196 */ 3197 public Distance getValueDistance() throws FHIRException { 3198 if (this.value == null) 3199 this.value = new Distance(); 3200 if (!(this.value instanceof Distance)) 3201 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 3202 return (Distance) this.value; 3203 } 3204 3205 public boolean hasValueDistance() { 3206 return this != null && this.value instanceof Distance; 3207 } 3208 3209 /** 3210 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3211 */ 3212 public Duration getValueDuration() throws FHIRException { 3213 if (this.value == null) 3214 this.value = new Duration(); 3215 if (!(this.value instanceof Duration)) 3216 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 3217 return (Duration) this.value; 3218 } 3219 3220 public boolean hasValueDuration() { 3221 return this != null && this.value instanceof Duration; 3222 } 3223 3224 /** 3225 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3226 */ 3227 public HumanName getValueHumanName() throws FHIRException { 3228 if (this.value == null) 3229 this.value = new HumanName(); 3230 if (!(this.value instanceof HumanName)) 3231 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 3232 return (HumanName) this.value; 3233 } 3234 3235 public boolean hasValueHumanName() { 3236 return this != null && this.value instanceof HumanName; 3237 } 3238 3239 /** 3240 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3241 */ 3242 public Identifier getValueIdentifier() throws FHIRException { 3243 if (this.value == null) 3244 this.value = new Identifier(); 3245 if (!(this.value instanceof Identifier)) 3246 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 3247 return (Identifier) this.value; 3248 } 3249 3250 public boolean hasValueIdentifier() { 3251 return this != null && this.value instanceof Identifier; 3252 } 3253 3254 /** 3255 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3256 */ 3257 public Money getValueMoney() throws FHIRException { 3258 if (this.value == null) 3259 this.value = new Money(); 3260 if (!(this.value instanceof Money)) 3261 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 3262 return (Money) this.value; 3263 } 3264 3265 public boolean hasValueMoney() { 3266 return this != null && this.value instanceof Money; 3267 } 3268 3269 /** 3270 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3271 */ 3272 public Period getValuePeriod() throws FHIRException { 3273 if (this.value == null) 3274 this.value = new Period(); 3275 if (!(this.value instanceof Period)) 3276 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 3277 return (Period) this.value; 3278 } 3279 3280 public boolean hasValuePeriod() { 3281 return this != null && this.value instanceof Period; 3282 } 3283 3284 /** 3285 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3286 */ 3287 public Quantity getValueQuantity() throws FHIRException { 3288 if (this.value == null) 3289 this.value = new Quantity(); 3290 if (!(this.value instanceof Quantity)) 3291 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3292 return (Quantity) this.value; 3293 } 3294 3295 public boolean hasValueQuantity() { 3296 return this != null && this.value instanceof Quantity; 3297 } 3298 3299 /** 3300 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3301 */ 3302 public Range getValueRange() throws FHIRException { 3303 if (this.value == null) 3304 this.value = new Range(); 3305 if (!(this.value instanceof Range)) 3306 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 3307 return (Range) this.value; 3308 } 3309 3310 public boolean hasValueRange() { 3311 return this != null && this.value instanceof Range; 3312 } 3313 3314 /** 3315 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3316 */ 3317 public Ratio getValueRatio() throws FHIRException { 3318 if (this.value == null) 3319 this.value = new Ratio(); 3320 if (!(this.value instanceof Ratio)) 3321 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 3322 return (Ratio) this.value; 3323 } 3324 3325 public boolean hasValueRatio() { 3326 return this != null && this.value instanceof Ratio; 3327 } 3328 3329 /** 3330 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3331 */ 3332 public RatioRange getValueRatioRange() throws FHIRException { 3333 if (this.value == null) 3334 this.value = new RatioRange(); 3335 if (!(this.value instanceof RatioRange)) 3336 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 3337 return (RatioRange) this.value; 3338 } 3339 3340 public boolean hasValueRatioRange() { 3341 return this != null && this.value instanceof RatioRange; 3342 } 3343 3344 /** 3345 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3346 */ 3347 public Reference getValueReference() throws FHIRException { 3348 if (this.value == null) 3349 this.value = new Reference(); 3350 if (!(this.value instanceof Reference)) 3351 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 3352 return (Reference) this.value; 3353 } 3354 3355 public boolean hasValueReference() { 3356 return this != null && this.value instanceof Reference; 3357 } 3358 3359 /** 3360 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3361 */ 3362 public SampledData getValueSampledData() throws FHIRException { 3363 if (this.value == null) 3364 this.value = new SampledData(); 3365 if (!(this.value instanceof SampledData)) 3366 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 3367 return (SampledData) this.value; 3368 } 3369 3370 public boolean hasValueSampledData() { 3371 return this != null && this.value instanceof SampledData; 3372 } 3373 3374 /** 3375 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3376 */ 3377 public Signature getValueSignature() throws FHIRException { 3378 if (this.value == null) 3379 this.value = new Signature(); 3380 if (!(this.value instanceof Signature)) 3381 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 3382 return (Signature) this.value; 3383 } 3384 3385 public boolean hasValueSignature() { 3386 return this != null && this.value instanceof Signature; 3387 } 3388 3389 /** 3390 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3391 */ 3392 public Timing getValueTiming() throws FHIRException { 3393 if (this.value == null) 3394 this.value = new Timing(); 3395 if (!(this.value instanceof Timing)) 3396 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 3397 return (Timing) this.value; 3398 } 3399 3400 public boolean hasValueTiming() { 3401 return this != null && this.value instanceof Timing; 3402 } 3403 3404 /** 3405 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3406 */ 3407 public ContactDetail getValueContactDetail() throws FHIRException { 3408 if (this.value == null) 3409 this.value = new ContactDetail(); 3410 if (!(this.value instanceof ContactDetail)) 3411 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 3412 return (ContactDetail) this.value; 3413 } 3414 3415 public boolean hasValueContactDetail() { 3416 return this != null && this.value instanceof ContactDetail; 3417 } 3418 3419 /** 3420 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3421 */ 3422 public DataRequirement getValueDataRequirement() throws FHIRException { 3423 if (this.value == null) 3424 this.value = new DataRequirement(); 3425 if (!(this.value instanceof DataRequirement)) 3426 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 3427 return (DataRequirement) this.value; 3428 } 3429 3430 public boolean hasValueDataRequirement() { 3431 return this != null && this.value instanceof DataRequirement; 3432 } 3433 3434 /** 3435 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3436 */ 3437 public Expression getValueExpression() throws FHIRException { 3438 if (this.value == null) 3439 this.value = new Expression(); 3440 if (!(this.value instanceof Expression)) 3441 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 3442 return (Expression) this.value; 3443 } 3444 3445 public boolean hasValueExpression() { 3446 return this != null && this.value instanceof Expression; 3447 } 3448 3449 /** 3450 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3451 */ 3452 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 3453 if (this.value == null) 3454 this.value = new ParameterDefinition(); 3455 if (!(this.value instanceof ParameterDefinition)) 3456 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 3457 return (ParameterDefinition) this.value; 3458 } 3459 3460 public boolean hasValueParameterDefinition() { 3461 return this != null && this.value instanceof ParameterDefinition; 3462 } 3463 3464 /** 3465 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3466 */ 3467 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 3468 if (this.value == null) 3469 this.value = new RelatedArtifact(); 3470 if (!(this.value instanceof RelatedArtifact)) 3471 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 3472 return (RelatedArtifact) this.value; 3473 } 3474 3475 public boolean hasValueRelatedArtifact() { 3476 return this != null && this.value instanceof RelatedArtifact; 3477 } 3478 3479 /** 3480 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3481 */ 3482 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 3483 if (this.value == null) 3484 this.value = new TriggerDefinition(); 3485 if (!(this.value instanceof TriggerDefinition)) 3486 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 3487 return (TriggerDefinition) this.value; 3488 } 3489 3490 public boolean hasValueTriggerDefinition() { 3491 return this != null && this.value instanceof TriggerDefinition; 3492 } 3493 3494 /** 3495 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3496 */ 3497 public UsageContext getValueUsageContext() throws FHIRException { 3498 if (this.value == null) 3499 this.value = new UsageContext(); 3500 if (!(this.value instanceof UsageContext)) 3501 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 3502 return (UsageContext) this.value; 3503 } 3504 3505 public boolean hasValueUsageContext() { 3506 return this != null && this.value instanceof UsageContext; 3507 } 3508 3509 /** 3510 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3511 */ 3512 public Availability getValueAvailability() throws FHIRException { 3513 if (this.value == null) 3514 this.value = new Availability(); 3515 if (!(this.value instanceof Availability)) 3516 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 3517 return (Availability) this.value; 3518 } 3519 3520 public boolean hasValueAvailability() { 3521 return this != null && this.value instanceof Availability; 3522 } 3523 3524 /** 3525 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3526 */ 3527 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 3528 if (this.value == null) 3529 this.value = new ExtendedContactDetail(); 3530 if (!(this.value instanceof ExtendedContactDetail)) 3531 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 3532 return (ExtendedContactDetail) this.value; 3533 } 3534 3535 public boolean hasValueExtendedContactDetail() { 3536 return this != null && this.value instanceof ExtendedContactDetail; 3537 } 3538 3539 /** 3540 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3541 */ 3542 public Dosage getValueDosage() throws FHIRException { 3543 if (this.value == null) 3544 this.value = new Dosage(); 3545 if (!(this.value instanceof Dosage)) 3546 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 3547 return (Dosage) this.value; 3548 } 3549 3550 public boolean hasValueDosage() { 3551 return this != null && this.value instanceof Dosage; 3552 } 3553 3554 /** 3555 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3556 */ 3557 public Meta getValueMeta() throws FHIRException { 3558 if (this.value == null) 3559 this.value = new Meta(); 3560 if (!(this.value instanceof Meta)) 3561 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 3562 return (Meta) this.value; 3563 } 3564 3565 public boolean hasValueMeta() { 3566 return this != null && this.value instanceof Meta; 3567 } 3568 3569 public boolean hasValue() { 3570 return this.value != null && !this.value.isEmpty(); 3571 } 3572 3573 /** 3574 * @param value {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 3575 */ 3576 public ElementDefinitionExampleComponent setValue(DataType value) { 3577 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 3578 throw new FHIRException("Not the right type for ElementDefinition.example.value[x]: "+value.fhirType()); 3579 this.value = value; 3580 return this; 3581 } 3582 3583 protected void listChildren(List<Property> children) { 3584 super.listChildren(children); 3585 children.add(new Property("label", "string", "Describes the purpose of this example among the set of examples.", 0, 1, label)); 3586 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value)); 3587 } 3588 3589 @Override 3590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3591 switch (_hash) { 3592 case 102727412: /*label*/ return new Property("label", "string", "Describes the purpose of this example among the set of examples.", 0, 1, label); 3593 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3594 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3595 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3596 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3597 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3598 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3599 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3600 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3601 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3602 case 231604844: /*valueId*/ return new Property("value[x]", "id", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3603 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3604 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3605 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3606 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3607 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3608 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3609 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3610 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3611 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3612 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3613 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3614 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3615 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3616 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3617 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3618 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3619 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3620 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3621 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3622 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3623 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3624 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3625 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3626 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3627 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3628 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3629 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3630 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3631 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3632 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3633 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3634 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3635 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3636 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3637 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3638 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3639 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3640 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3641 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3642 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3643 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3644 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3645 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3646 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3647 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3648 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 3649 default: return super.getNamedProperty(_hash, _name, _checkValid); 3650 } 3651 3652 } 3653 3654 @Override 3655 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3656 switch (hash) { 3657 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 3658 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3659 default: return super.getProperty(hash, name, checkValid); 3660 } 3661 3662 } 3663 3664 @Override 3665 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3666 switch (hash) { 3667 case 102727412: // label 3668 this.label = TypeConvertor.castToString(value); // StringType 3669 return value; 3670 case 111972721: // value 3671 this.value = TypeConvertor.castToType(value); // DataType 3672 return value; 3673 default: return super.setProperty(hash, name, value); 3674 } 3675 3676 } 3677 3678 @Override 3679 public Base setProperty(String name, Base value) throws FHIRException { 3680 if (name.equals("label")) { 3681 this.label = TypeConvertor.castToString(value); // StringType 3682 } else if (name.equals("value[x]")) { 3683 this.value = TypeConvertor.castToType(value); // DataType 3684 } else 3685 return super.setProperty(name, value); 3686 return value; 3687 } 3688 3689 @Override 3690 public void removeChild(String name, Base value) throws FHIRException { 3691 if (name.equals("label")) { 3692 this.label = null; 3693 } else if (name.equals("value[x]")) { 3694 this.value = null; 3695 } else 3696 super.removeChild(name, value); 3697 3698 } 3699 3700 @Override 3701 public Base makeProperty(int hash, String name) throws FHIRException { 3702 switch (hash) { 3703 case 102727412: return getLabelElement(); 3704 case -1410166417: return getValue(); 3705 case 111972721: return getValue(); 3706 default: return super.makeProperty(hash, name); 3707 } 3708 3709 } 3710 3711 @Override 3712 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3713 switch (hash) { 3714 case 102727412: /*label*/ return new String[] {"string"}; 3715 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 3716 default: return super.getTypesForProperty(hash, name); 3717 } 3718 3719 } 3720 3721 @Override 3722 public Base addChild(String name) throws FHIRException { 3723 if (name.equals("label")) { 3724 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.example.label"); 3725 } 3726 else if (name.equals("valueBase64Binary")) { 3727 this.value = new Base64BinaryType(); 3728 return this.value; 3729 } 3730 else if (name.equals("valueBoolean")) { 3731 this.value = new BooleanType(); 3732 return this.value; 3733 } 3734 else if (name.equals("valueCanonical")) { 3735 this.value = new CanonicalType(); 3736 return this.value; 3737 } 3738 else if (name.equals("valueCode")) { 3739 this.value = new CodeType(); 3740 return this.value; 3741 } 3742 else if (name.equals("valueDate")) { 3743 this.value = new DateType(); 3744 return this.value; 3745 } 3746 else if (name.equals("valueDateTime")) { 3747 this.value = new DateTimeType(); 3748 return this.value; 3749 } 3750 else if (name.equals("valueDecimal")) { 3751 this.value = new DecimalType(); 3752 return this.value; 3753 } 3754 else if (name.equals("valueId")) { 3755 this.value = new IdType(); 3756 return this.value; 3757 } 3758 else if (name.equals("valueInstant")) { 3759 this.value = new InstantType(); 3760 return this.value; 3761 } 3762 else if (name.equals("valueInteger")) { 3763 this.value = new IntegerType(); 3764 return this.value; 3765 } 3766 else if (name.equals("valueInteger64")) { 3767 this.value = new Integer64Type(); 3768 return this.value; 3769 } 3770 else if (name.equals("valueMarkdown")) { 3771 this.value = new MarkdownType(); 3772 return this.value; 3773 } 3774 else if (name.equals("valueOid")) { 3775 this.value = new OidType(); 3776 return this.value; 3777 } 3778 else if (name.equals("valuePositiveInt")) { 3779 this.value = new PositiveIntType(); 3780 return this.value; 3781 } 3782 else if (name.equals("valueString")) { 3783 this.value = new StringType(); 3784 return this.value; 3785 } 3786 else if (name.equals("valueTime")) { 3787 this.value = new TimeType(); 3788 return this.value; 3789 } 3790 else if (name.equals("valueUnsignedInt")) { 3791 this.value = new UnsignedIntType(); 3792 return this.value; 3793 } 3794 else if (name.equals("valueUri")) { 3795 this.value = new UriType(); 3796 return this.value; 3797 } 3798 else if (name.equals("valueUrl")) { 3799 this.value = new UrlType(); 3800 return this.value; 3801 } 3802 else if (name.equals("valueUuid")) { 3803 this.value = new UuidType(); 3804 return this.value; 3805 } 3806 else if (name.equals("valueAddress")) { 3807 this.value = new Address(); 3808 return this.value; 3809 } 3810 else if (name.equals("valueAge")) { 3811 this.value = new Age(); 3812 return this.value; 3813 } 3814 else if (name.equals("valueAnnotation")) { 3815 this.value = new Annotation(); 3816 return this.value; 3817 } 3818 else if (name.equals("valueAttachment")) { 3819 this.value = new Attachment(); 3820 return this.value; 3821 } 3822 else if (name.equals("valueCodeableConcept")) { 3823 this.value = new CodeableConcept(); 3824 return this.value; 3825 } 3826 else if (name.equals("valueCodeableReference")) { 3827 this.value = new CodeableReference(); 3828 return this.value; 3829 } 3830 else if (name.equals("valueCoding")) { 3831 this.value = new Coding(); 3832 return this.value; 3833 } 3834 else if (name.equals("valueContactPoint")) { 3835 this.value = new ContactPoint(); 3836 return this.value; 3837 } 3838 else if (name.equals("valueCount")) { 3839 this.value = new Count(); 3840 return this.value; 3841 } 3842 else if (name.equals("valueDistance")) { 3843 this.value = new Distance(); 3844 return this.value; 3845 } 3846 else if (name.equals("valueDuration")) { 3847 this.value = new Duration(); 3848 return this.value; 3849 } 3850 else if (name.equals("valueHumanName")) { 3851 this.value = new HumanName(); 3852 return this.value; 3853 } 3854 else if (name.equals("valueIdentifier")) { 3855 this.value = new Identifier(); 3856 return this.value; 3857 } 3858 else if (name.equals("valueMoney")) { 3859 this.value = new Money(); 3860 return this.value; 3861 } 3862 else if (name.equals("valuePeriod")) { 3863 this.value = new Period(); 3864 return this.value; 3865 } 3866 else if (name.equals("valueQuantity")) { 3867 this.value = new Quantity(); 3868 return this.value; 3869 } 3870 else if (name.equals("valueRange")) { 3871 this.value = new Range(); 3872 return this.value; 3873 } 3874 else if (name.equals("valueRatio")) { 3875 this.value = new Ratio(); 3876 return this.value; 3877 } 3878 else if (name.equals("valueRatioRange")) { 3879 this.value = new RatioRange(); 3880 return this.value; 3881 } 3882 else if (name.equals("valueReference")) { 3883 this.value = new Reference(); 3884 return this.value; 3885 } 3886 else if (name.equals("valueSampledData")) { 3887 this.value = new SampledData(); 3888 return this.value; 3889 } 3890 else if (name.equals("valueSignature")) { 3891 this.value = new Signature(); 3892 return this.value; 3893 } 3894 else if (name.equals("valueTiming")) { 3895 this.value = new Timing(); 3896 return this.value; 3897 } 3898 else if (name.equals("valueContactDetail")) { 3899 this.value = new ContactDetail(); 3900 return this.value; 3901 } 3902 else if (name.equals("valueDataRequirement")) { 3903 this.value = new DataRequirement(); 3904 return this.value; 3905 } 3906 else if (name.equals("valueExpression")) { 3907 this.value = new Expression(); 3908 return this.value; 3909 } 3910 else if (name.equals("valueParameterDefinition")) { 3911 this.value = new ParameterDefinition(); 3912 return this.value; 3913 } 3914 else if (name.equals("valueRelatedArtifact")) { 3915 this.value = new RelatedArtifact(); 3916 return this.value; 3917 } 3918 else if (name.equals("valueTriggerDefinition")) { 3919 this.value = new TriggerDefinition(); 3920 return this.value; 3921 } 3922 else if (name.equals("valueUsageContext")) { 3923 this.value = new UsageContext(); 3924 return this.value; 3925 } 3926 else if (name.equals("valueAvailability")) { 3927 this.value = new Availability(); 3928 return this.value; 3929 } 3930 else if (name.equals("valueExtendedContactDetail")) { 3931 this.value = new ExtendedContactDetail(); 3932 return this.value; 3933 } 3934 else if (name.equals("valueDosage")) { 3935 this.value = new Dosage(); 3936 return this.value; 3937 } 3938 else if (name.equals("valueMeta")) { 3939 this.value = new Meta(); 3940 return this.value; 3941 } 3942 else 3943 return super.addChild(name); 3944 } 3945 3946 public ElementDefinitionExampleComponent copy() { 3947 ElementDefinitionExampleComponent dst = new ElementDefinitionExampleComponent(); 3948 copyValues(dst); 3949 return dst; 3950 } 3951 3952 public void copyValues(ElementDefinitionExampleComponent dst) { 3953 super.copyValues(dst); 3954 dst.label = label == null ? null : label.copy(); 3955 dst.value = value == null ? null : value.copy(); 3956 } 3957 3958 @Override 3959 public boolean equalsDeep(Base other_) { 3960 if (!super.equalsDeep(other_)) 3961 return false; 3962 if (!(other_ instanceof ElementDefinitionExampleComponent)) 3963 return false; 3964 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 3965 return compareDeep(label, o.label, true) && compareDeep(value, o.value, true); 3966 } 3967 3968 @Override 3969 public boolean equalsShallow(Base other_) { 3970 if (!super.equalsShallow(other_)) 3971 return false; 3972 if (!(other_ instanceof ElementDefinitionExampleComponent)) 3973 return false; 3974 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 3975 return compareValues(label, o.label, true); 3976 } 3977 3978 public boolean isEmpty() { 3979 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, value); 3980 } 3981 3982 public String fhirType() { 3983 return "ElementDefinition.example"; 3984 3985 } 3986 3987 } 3988 3989 @Block() 3990 public static class ElementDefinitionConstraintComponent extends Element implements IBaseDatatypeElement { 3991 /** 3992 * Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 3993 */ 3994 @Child(name = "key", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3995 @Description(shortDefinition="Target of 'condition' reference above", formalDefinition="Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality." ) 3996 protected IdType key; 3997 3998 /** 3999 * Description of why this constraint is necessary or appropriate. 4000 */ 4001 @Child(name = "requirements", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4002 @Description(shortDefinition="Why this constraint is necessary or appropriate", formalDefinition="Description of why this constraint is necessary or appropriate." ) 4003 protected MarkdownType requirements; 4004 4005 /** 4006 * Identifies the impact constraint violation has on the conformance of the instance. 4007 */ 4008 @Child(name = "severity", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 4009 @Description(shortDefinition="error | warning", formalDefinition="Identifies the impact constraint violation has on the conformance of the instance." ) 4010 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/constraint-severity") 4011 protected Enumeration<ConstraintSeverity> severity; 4012 4013 /** 4014 * If true, indicates that the warning or best practice guideline should be suppressed. 4015 */ 4016 @Child(name = "suppress", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 4017 @Description(shortDefinition="Suppress warning or hint in profile", formalDefinition="If true, indicates that the warning or best practice guideline should be suppressed." ) 4018 protected BooleanType suppress; 4019 4020 /** 4021 * Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 4022 */ 4023 @Child(name = "human", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=true) 4024 @Description(shortDefinition="Human description of constraint", formalDefinition="Text that can be used to describe the constraint in messages identifying that the constraint has been violated." ) 4025 protected StringType human; 4026 4027 /** 4028 * A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met. 4029 */ 4030 @Child(name = "expression", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 4031 @Description(shortDefinition="FHIRPath expression of constraint", formalDefinition="A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met." ) 4032 protected StringType expression; 4033 4034 /** 4035 * A reference to the original source of the constraint, for traceability purposes. 4036 */ 4037 @Child(name = "source", type = {CanonicalType.class}, order=7, min=0, max=1, modifier=false, summary=true) 4038 @Description(shortDefinition="Reference to original source of constraint", formalDefinition="A reference to the original source of the constraint, for traceability purposes." ) 4039 protected CanonicalType source; 4040 4041 private static final long serialVersionUID = 1642607838L; 4042 4043 /** 4044 * Constructor 4045 */ 4046 public ElementDefinitionConstraintComponent() { 4047 super(); 4048 } 4049 4050 /** 4051 * Constructor 4052 */ 4053 public ElementDefinitionConstraintComponent(String key, ConstraintSeverity severity, String human) { 4054 super(); 4055 this.setKey(key); 4056 this.setSeverity(severity); 4057 this.setHuman(human); 4058 } 4059 4060 /** 4061 * @return {@link #key} (Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 4062 */ 4063 public IdType getKeyElement() { 4064 if (this.key == null) 4065 if (Configuration.errorOnAutoCreate()) 4066 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.key"); 4067 else if (Configuration.doAutoCreate()) 4068 this.key = new IdType(); // bb 4069 return this.key; 4070 } 4071 4072 public boolean hasKeyElement() { 4073 return this.key != null && !this.key.isEmpty(); 4074 } 4075 4076 public boolean hasKey() { 4077 return this.key != null && !this.key.isEmpty(); 4078 } 4079 4080 /** 4081 * @param value {@link #key} (Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 4082 */ 4083 public ElementDefinitionConstraintComponent setKeyElement(IdType value) { 4084 this.key = value; 4085 return this; 4086 } 4087 4088 /** 4089 * @return Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 4090 */ 4091 public String getKey() { 4092 return this.key == null ? null : this.key.getValue(); 4093 } 4094 4095 /** 4096 * @param value Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 4097 */ 4098 public ElementDefinitionConstraintComponent setKey(String value) { 4099 if (this.key == null) 4100 this.key = new IdType(); 4101 this.key.setValue(value); 4102 return this; 4103 } 4104 4105 /** 4106 * @return {@link #requirements} (Description of why this constraint is necessary or appropriate.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 4107 */ 4108 public MarkdownType getRequirementsElement() { 4109 if (this.requirements == null) 4110 if (Configuration.errorOnAutoCreate()) 4111 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.requirements"); 4112 else if (Configuration.doAutoCreate()) 4113 this.requirements = new MarkdownType(); // bb 4114 return this.requirements; 4115 } 4116 4117 public boolean hasRequirementsElement() { 4118 return this.requirements != null && !this.requirements.isEmpty(); 4119 } 4120 4121 public boolean hasRequirements() { 4122 return this.requirements != null && !this.requirements.isEmpty(); 4123 } 4124 4125 /** 4126 * @param value {@link #requirements} (Description of why this constraint is necessary or appropriate.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 4127 */ 4128 public ElementDefinitionConstraintComponent setRequirementsElement(MarkdownType value) { 4129 this.requirements = value; 4130 return this; 4131 } 4132 4133 /** 4134 * @return Description of why this constraint is necessary or appropriate. 4135 */ 4136 public String getRequirements() { 4137 return this.requirements == null ? null : this.requirements.getValue(); 4138 } 4139 4140 /** 4141 * @param value Description of why this constraint is necessary or appropriate. 4142 */ 4143 public ElementDefinitionConstraintComponent setRequirements(String value) { 4144 if (Utilities.noString(value)) 4145 this.requirements = null; 4146 else { 4147 if (this.requirements == null) 4148 this.requirements = new MarkdownType(); 4149 this.requirements.setValue(value); 4150 } 4151 return this; 4152 } 4153 4154 /** 4155 * @return {@link #severity} (Identifies the impact constraint violation has on the conformance of the instance.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 4156 */ 4157 public Enumeration<ConstraintSeverity> getSeverityElement() { 4158 if (this.severity == null) 4159 if (Configuration.errorOnAutoCreate()) 4160 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.severity"); 4161 else if (Configuration.doAutoCreate()) 4162 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); // bb 4163 return this.severity; 4164 } 4165 4166 public boolean hasSeverityElement() { 4167 return this.severity != null && !this.severity.isEmpty(); 4168 } 4169 4170 public boolean hasSeverity() { 4171 return this.severity != null && !this.severity.isEmpty(); 4172 } 4173 4174 /** 4175 * @param value {@link #severity} (Identifies the impact constraint violation has on the conformance of the instance.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 4176 */ 4177 public ElementDefinitionConstraintComponent setSeverityElement(Enumeration<ConstraintSeverity> value) { 4178 this.severity = value; 4179 return this; 4180 } 4181 4182 /** 4183 * @return Identifies the impact constraint violation has on the conformance of the instance. 4184 */ 4185 public ConstraintSeverity getSeverity() { 4186 return this.severity == null ? null : this.severity.getValue(); 4187 } 4188 4189 /** 4190 * @param value Identifies the impact constraint violation has on the conformance of the instance. 4191 */ 4192 public ElementDefinitionConstraintComponent setSeverity(ConstraintSeverity value) { 4193 if (this.severity == null) 4194 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); 4195 this.severity.setValue(value); 4196 return this; 4197 } 4198 4199 /** 4200 * @return {@link #suppress} (If true, indicates that the warning or best practice guideline should be suppressed.). This is the underlying object with id, value and extensions. The accessor "getSuppress" gives direct access to the value 4201 */ 4202 public BooleanType getSuppressElement() { 4203 if (this.suppress == null) 4204 if (Configuration.errorOnAutoCreate()) 4205 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.suppress"); 4206 else if (Configuration.doAutoCreate()) 4207 this.suppress = new BooleanType(); // bb 4208 return this.suppress; 4209 } 4210 4211 public boolean hasSuppressElement() { 4212 return this.suppress != null && !this.suppress.isEmpty(); 4213 } 4214 4215 public boolean hasSuppress() { 4216 return this.suppress != null && !this.suppress.isEmpty(); 4217 } 4218 4219 /** 4220 * @param value {@link #suppress} (If true, indicates that the warning or best practice guideline should be suppressed.). This is the underlying object with id, value and extensions. The accessor "getSuppress" gives direct access to the value 4221 */ 4222 public ElementDefinitionConstraintComponent setSuppressElement(BooleanType value) { 4223 this.suppress = value; 4224 return this; 4225 } 4226 4227 /** 4228 * @return If true, indicates that the warning or best practice guideline should be suppressed. 4229 */ 4230 public boolean getSuppress() { 4231 return this.suppress == null || this.suppress.isEmpty() ? false : this.suppress.getValue(); 4232 } 4233 4234 /** 4235 * @param value If true, indicates that the warning or best practice guideline should be suppressed. 4236 */ 4237 public ElementDefinitionConstraintComponent setSuppress(boolean value) { 4238 if (this.suppress == null) 4239 this.suppress = new BooleanType(); 4240 this.suppress.setValue(value); 4241 return this; 4242 } 4243 4244 /** 4245 * @return {@link #human} (Text that can be used to describe the constraint in messages identifying that the constraint has been violated.). This is the underlying object with id, value and extensions. The accessor "getHuman" gives direct access to the value 4246 */ 4247 public StringType getHumanElement() { 4248 if (this.human == null) 4249 if (Configuration.errorOnAutoCreate()) 4250 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.human"); 4251 else if (Configuration.doAutoCreate()) 4252 this.human = new StringType(); // bb 4253 return this.human; 4254 } 4255 4256 public boolean hasHumanElement() { 4257 return this.human != null && !this.human.isEmpty(); 4258 } 4259 4260 public boolean hasHuman() { 4261 return this.human != null && !this.human.isEmpty(); 4262 } 4263 4264 /** 4265 * @param value {@link #human} (Text that can be used to describe the constraint in messages identifying that the constraint has been violated.). This is the underlying object with id, value and extensions. The accessor "getHuman" gives direct access to the value 4266 */ 4267 public ElementDefinitionConstraintComponent setHumanElement(StringType value) { 4268 this.human = value; 4269 return this; 4270 } 4271 4272 /** 4273 * @return Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 4274 */ 4275 public String getHuman() { 4276 return this.human == null ? null : this.human.getValue(); 4277 } 4278 4279 /** 4280 * @param value Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 4281 */ 4282 public ElementDefinitionConstraintComponent setHuman(String value) { 4283 if (this.human == null) 4284 this.human = new StringType(); 4285 this.human.setValue(value); 4286 return this; 4287 } 4288 4289 /** 4290 * @return {@link #expression} (A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4291 */ 4292 public StringType getExpressionElement() { 4293 if (this.expression == null) 4294 if (Configuration.errorOnAutoCreate()) 4295 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.expression"); 4296 else if (Configuration.doAutoCreate()) 4297 this.expression = new StringType(); // bb 4298 return this.expression; 4299 } 4300 4301 public boolean hasExpressionElement() { 4302 return this.expression != null && !this.expression.isEmpty(); 4303 } 4304 4305 public boolean hasExpression() { 4306 return this.expression != null && !this.expression.isEmpty(); 4307 } 4308 4309 /** 4310 * @param value {@link #expression} (A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4311 */ 4312 public ElementDefinitionConstraintComponent setExpressionElement(StringType value) { 4313 this.expression = value; 4314 return this; 4315 } 4316 4317 /** 4318 * @return A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met. 4319 */ 4320 public String getExpression() { 4321 return this.expression == null ? null : this.expression.getValue(); 4322 } 4323 4324 /** 4325 * @param value A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met. 4326 */ 4327 public ElementDefinitionConstraintComponent setExpression(String value) { 4328 if (Utilities.noString(value)) 4329 this.expression = null; 4330 else { 4331 if (this.expression == null) 4332 this.expression = new StringType(); 4333 this.expression.setValue(value); 4334 } 4335 return this; 4336 } 4337 4338 /** 4339 * @return {@link #source} (A reference to the original source of the constraint, for traceability purposes.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 4340 */ 4341 public CanonicalType getSourceElement() { 4342 if (this.source == null) 4343 if (Configuration.errorOnAutoCreate()) 4344 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.source"); 4345 else if (Configuration.doAutoCreate()) 4346 this.source = new CanonicalType(); // bb 4347 return this.source; 4348 } 4349 4350 public boolean hasSourceElement() { 4351 return this.source != null && !this.source.isEmpty(); 4352 } 4353 4354 public boolean hasSource() { 4355 return this.source != null && !this.source.isEmpty(); 4356 } 4357 4358 /** 4359 * @param value {@link #source} (A reference to the original source of the constraint, for traceability purposes.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 4360 */ 4361 public ElementDefinitionConstraintComponent setSourceElement(CanonicalType value) { 4362 this.source = value; 4363 return this; 4364 } 4365 4366 /** 4367 * @return A reference to the original source of the constraint, for traceability purposes. 4368 */ 4369 public String getSource() { 4370 return this.source == null ? null : this.source.getValue(); 4371 } 4372 4373 /** 4374 * @param value A reference to the original source of the constraint, for traceability purposes. 4375 */ 4376 public ElementDefinitionConstraintComponent setSource(String value) { 4377 if (Utilities.noString(value)) 4378 this.source = null; 4379 else { 4380 if (this.source == null) 4381 this.source = new CanonicalType(); 4382 this.source.setValue(value); 4383 } 4384 return this; 4385 } 4386 4387 protected void listChildren(List<Property> children) { 4388 super.listChildren(children); 4389 children.add(new Property("key", "id", "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 0, 1, key)); 4390 children.add(new Property("requirements", "markdown", "Description of why this constraint is necessary or appropriate.", 0, 1, requirements)); 4391 children.add(new Property("severity", "code", "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity)); 4392 children.add(new Property("suppress", "boolean", "If true, indicates that the warning or best practice guideline should be suppressed.", 0, 1, suppress)); 4393 children.add(new Property("human", "string", "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 0, 1, human)); 4394 children.add(new Property("expression", "string", "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 0, 1, expression)); 4395 children.add(new Property("source", "canonical(StructureDefinition)", "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source)); 4396 } 4397 4398 @Override 4399 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4400 switch (_hash) { 4401 case 106079: /*key*/ return new Property("key", "id", "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 0, 1, key); 4402 case -1619874672: /*requirements*/ return new Property("requirements", "markdown", "Description of why this constraint is necessary or appropriate.", 0, 1, requirements); 4403 case 1478300413: /*severity*/ return new Property("severity", "code", "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity); 4404 case -1663129931: /*suppress*/ return new Property("suppress", "boolean", "If true, indicates that the warning or best practice guideline should be suppressed.", 0, 1, suppress); 4405 case 99639597: /*human*/ return new Property("human", "string", "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 0, 1, human); 4406 case -1795452264: /*expression*/ return new Property("expression", "string", "A [FHIRPath](fhirpath.html) expression of constraint that can be executed to see if this constraint is met.", 0, 1, expression); 4407 case -896505829: /*source*/ return new Property("source", "canonical(StructureDefinition)", "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source); 4408 default: return super.getNamedProperty(_hash, _name, _checkValid); 4409 } 4410 4411 } 4412 4413 @Override 4414 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4415 switch (hash) { 4416 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // IdType 4417 case -1619874672: /*requirements*/ return this.requirements == null ? new Base[0] : new Base[] {this.requirements}; // MarkdownType 4418 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<ConstraintSeverity> 4419 case -1663129931: /*suppress*/ return this.suppress == null ? new Base[0] : new Base[] {this.suppress}; // BooleanType 4420 case 99639597: /*human*/ return this.human == null ? new Base[0] : new Base[] {this.human}; // StringType 4421 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 4422 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // CanonicalType 4423 default: return super.getProperty(hash, name, checkValid); 4424 } 4425 4426 } 4427 4428 @Override 4429 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4430 switch (hash) { 4431 case 106079: // key 4432 this.key = TypeConvertor.castToId(value); // IdType 4433 return value; 4434 case -1619874672: // requirements 4435 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 4436 return value; 4437 case 1478300413: // severity 4438 value = new ConstraintSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4439 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4440 return value; 4441 case -1663129931: // suppress 4442 this.suppress = TypeConvertor.castToBoolean(value); // BooleanType 4443 return value; 4444 case 99639597: // human 4445 this.human = TypeConvertor.castToString(value); // StringType 4446 return value; 4447 case -1795452264: // expression 4448 this.expression = TypeConvertor.castToString(value); // StringType 4449 return value; 4450 case -896505829: // source 4451 this.source = TypeConvertor.castToCanonical(value); // CanonicalType 4452 return value; 4453 default: return super.setProperty(hash, name, value); 4454 } 4455 4456 } 4457 4458 @Override 4459 public Base setProperty(String name, Base value) throws FHIRException { 4460 if (name.equals("key")) { 4461 this.key = TypeConvertor.castToId(value); // IdType 4462 } else if (name.equals("requirements")) { 4463 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 4464 } else if (name.equals("severity")) { 4465 value = new ConstraintSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4466 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4467 } else if (name.equals("suppress")) { 4468 this.suppress = TypeConvertor.castToBoolean(value); // BooleanType 4469 } else if (name.equals("human")) { 4470 this.human = TypeConvertor.castToString(value); // StringType 4471 } else if (name.equals("expression")) { 4472 this.expression = TypeConvertor.castToString(value); // StringType 4473 } else if (name.equals("source")) { 4474 this.source = TypeConvertor.castToCanonical(value); // CanonicalType 4475 } else 4476 return super.setProperty(name, value); 4477 return value; 4478 } 4479 4480 @Override 4481 public void removeChild(String name, Base value) throws FHIRException { 4482 if (name.equals("key")) { 4483 this.key = null; 4484 } else if (name.equals("requirements")) { 4485 this.requirements = null; 4486 } else if (name.equals("severity")) { 4487 value = new ConstraintSeverityEnumFactory().fromType(TypeConvertor.castToCode(value)); 4488 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 4489 } else if (name.equals("suppress")) { 4490 this.suppress = null; 4491 } else if (name.equals("human")) { 4492 this.human = null; 4493 } else if (name.equals("expression")) { 4494 this.expression = null; 4495 } else if (name.equals("source")) { 4496 this.source = null; 4497 } else 4498 super.removeChild(name, value); 4499 4500 } 4501 4502 @Override 4503 public Base makeProperty(int hash, String name) throws FHIRException { 4504 switch (hash) { 4505 case 106079: return getKeyElement(); 4506 case -1619874672: return getRequirementsElement(); 4507 case 1478300413: return getSeverityElement(); 4508 case -1663129931: return getSuppressElement(); 4509 case 99639597: return getHumanElement(); 4510 case -1795452264: return getExpressionElement(); 4511 case -896505829: return getSourceElement(); 4512 default: return super.makeProperty(hash, name); 4513 } 4514 4515 } 4516 4517 @Override 4518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4519 switch (hash) { 4520 case 106079: /*key*/ return new String[] {"id"}; 4521 case -1619874672: /*requirements*/ return new String[] {"markdown"}; 4522 case 1478300413: /*severity*/ return new String[] {"code"}; 4523 case -1663129931: /*suppress*/ return new String[] {"boolean"}; 4524 case 99639597: /*human*/ return new String[] {"string"}; 4525 case -1795452264: /*expression*/ return new String[] {"string"}; 4526 case -896505829: /*source*/ return new String[] {"canonical"}; 4527 default: return super.getTypesForProperty(hash, name); 4528 } 4529 4530 } 4531 4532 @Override 4533 public Base addChild(String name) throws FHIRException { 4534 if (name.equals("key")) { 4535 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.key"); 4536 } 4537 else if (name.equals("requirements")) { 4538 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.requirements"); 4539 } 4540 else if (name.equals("severity")) { 4541 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.severity"); 4542 } 4543 else if (name.equals("suppress")) { 4544 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.suppress"); 4545 } 4546 else if (name.equals("human")) { 4547 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.human"); 4548 } 4549 else if (name.equals("expression")) { 4550 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.expression"); 4551 } 4552 else if (name.equals("source")) { 4553 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.constraint.source"); 4554 } 4555 else 4556 return super.addChild(name); 4557 } 4558 4559 public ElementDefinitionConstraintComponent copy() { 4560 ElementDefinitionConstraintComponent dst = new ElementDefinitionConstraintComponent(); 4561 copyValues(dst); 4562 return dst; 4563 } 4564 4565 public void copyValues(ElementDefinitionConstraintComponent dst) { 4566 super.copyValues(dst); 4567 dst.key = key == null ? null : key.copy(); 4568 dst.requirements = requirements == null ? null : requirements.copy(); 4569 dst.severity = severity == null ? null : severity.copy(); 4570 dst.suppress = suppress == null ? null : suppress.copy(); 4571 dst.human = human == null ? null : human.copy(); 4572 dst.expression = expression == null ? null : expression.copy(); 4573 dst.source = source == null ? null : source.copy(); 4574 } 4575 4576 @Override 4577 public boolean equalsDeep(Base other_) { 4578 if (!super.equalsDeep(other_)) 4579 return false; 4580 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4581 return false; 4582 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4583 return compareDeep(key, o.key, true) && compareDeep(requirements, o.requirements, true) && compareDeep(severity, o.severity, true) 4584 && compareDeep(suppress, o.suppress, true) && compareDeep(human, o.human, true) && compareDeep(expression, o.expression, true) 4585 && compareDeep(source, o.source, true); 4586 } 4587 4588 @Override 4589 public boolean equalsShallow(Base other_) { 4590 if (!super.equalsShallow(other_)) 4591 return false; 4592 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 4593 return false; 4594 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 4595 return compareValues(key, o.key, true) && compareValues(requirements, o.requirements, true) && compareValues(severity, o.severity, true) 4596 && compareValues(suppress, o.suppress, true) && compareValues(human, o.human, true) && compareValues(expression, o.expression, true) 4597 && compareValues(source, o.source, true); 4598 } 4599 4600 public boolean isEmpty() { 4601 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, requirements, severity 4602 , suppress, human, expression, source); 4603 } 4604 4605 public String fhirType() { 4606 return "ElementDefinition.constraint"; 4607 4608 } 4609 4610 @Override 4611 public String toString() { 4612 return key + ":" + expression + (severity == null ? "("+severity.asStringValue()+")" : ""); 4613 } 4614 4615 } 4616 4617 @Block() 4618 public static class ElementDefinitionBindingComponent extends Element implements IBaseDatatypeElement { 4619 /** 4620 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 4621 */ 4622 @Child(name = "strength", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4623 @Description(shortDefinition="required | extensible | preferred | example", formalDefinition="Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances." ) 4624 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/binding-strength") 4625 protected Enumeration<BindingStrength> strength; 4626 4627 /** 4628 * Describes the intended use of this particular set of codes. 4629 */ 4630 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4631 @Description(shortDefinition="Intended use of codes in the bound value set", formalDefinition="Describes the intended use of this particular set of codes." ) 4632 protected MarkdownType description; 4633 4634 /** 4635 * Refers to the value set that identifies the set of codes the binding refers to. 4636 */ 4637 @Child(name = "valueSet", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4638 @Description(shortDefinition="Source of value set", formalDefinition="Refers to the value set that identifies the set of codes the binding refers to." ) 4639 protected CanonicalType valueSet; 4640 4641 /** 4642 * Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context. 4643 */ 4644 @Child(name = "additional", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4645 @Description(shortDefinition="Additional Bindings - more rules about the binding", formalDefinition="Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context." ) 4646 protected List<ElementDefinitionBindingAdditionalComponent> additional; 4647 4648 private static final long serialVersionUID = 16276611L; 4649 4650 /** 4651 * Constructor 4652 */ 4653 public ElementDefinitionBindingComponent() { 4654 super(); 4655 } 4656 4657 /** 4658 * Constructor 4659 */ 4660 public ElementDefinitionBindingComponent(BindingStrength strength) { 4661 super(); 4662 this.setStrength(strength); 4663 } 4664 4665 /** 4666 * @return {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 4667 */ 4668 public Enumeration<BindingStrength> getStrengthElement() { 4669 if (this.strength == null) 4670 if (Configuration.errorOnAutoCreate()) 4671 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.strength"); 4672 else if (Configuration.doAutoCreate()) 4673 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 4674 return this.strength; 4675 } 4676 4677 public boolean hasStrengthElement() { 4678 return this.strength != null && !this.strength.isEmpty(); 4679 } 4680 4681 public boolean hasStrength() { 4682 return this.strength != null && !this.strength.isEmpty(); 4683 } 4684 4685 /** 4686 * @param value {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 4687 */ 4688 public ElementDefinitionBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 4689 this.strength = value; 4690 return this; 4691 } 4692 4693 /** 4694 * @return Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 4695 */ 4696 public BindingStrength getStrength() { 4697 return this.strength == null ? null : this.strength.getValue(); 4698 } 4699 4700 /** 4701 * @param value Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 4702 */ 4703 public ElementDefinitionBindingComponent setStrength(BindingStrength value) { 4704 if (this.strength == null) 4705 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 4706 this.strength.setValue(value); 4707 return this; 4708 } 4709 4710 /** 4711 * @return {@link #description} (Describes the intended use of this particular set of codes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4712 */ 4713 public MarkdownType getDescriptionElement() { 4714 if (this.description == null) 4715 if (Configuration.errorOnAutoCreate()) 4716 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.description"); 4717 else if (Configuration.doAutoCreate()) 4718 this.description = new MarkdownType(); // bb 4719 return this.description; 4720 } 4721 4722 public boolean hasDescriptionElement() { 4723 return this.description != null && !this.description.isEmpty(); 4724 } 4725 4726 public boolean hasDescription() { 4727 return this.description != null && !this.description.isEmpty(); 4728 } 4729 4730 /** 4731 * @param value {@link #description} (Describes the intended use of this particular set of codes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4732 */ 4733 public ElementDefinitionBindingComponent setDescriptionElement(MarkdownType value) { 4734 this.description = value; 4735 return this; 4736 } 4737 4738 /** 4739 * @return Describes the intended use of this particular set of codes. 4740 */ 4741 public String getDescription() { 4742 return this.description == null ? null : this.description.getValue(); 4743 } 4744 4745 /** 4746 * @param value Describes the intended use of this particular set of codes. 4747 */ 4748 public ElementDefinitionBindingComponent setDescription(String value) { 4749 if (Utilities.noString(value)) 4750 this.description = null; 4751 else { 4752 if (this.description == null) 4753 this.description = new MarkdownType(); 4754 this.description.setValue(value); 4755 } 4756 return this; 4757 } 4758 4759 /** 4760 * @return {@link #valueSet} (Refers to the value set that identifies the set of codes the binding refers to.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4761 */ 4762 public CanonicalType getValueSetElement() { 4763 if (this.valueSet == null) 4764 if (Configuration.errorOnAutoCreate()) 4765 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.valueSet"); 4766 else if (Configuration.doAutoCreate()) 4767 this.valueSet = new CanonicalType(); // bb 4768 return this.valueSet; 4769 } 4770 4771 public boolean hasValueSetElement() { 4772 return this.valueSet != null && !this.valueSet.isEmpty(); 4773 } 4774 4775 public boolean hasValueSet() { 4776 return this.valueSet != null && !this.valueSet.isEmpty(); 4777 } 4778 4779 /** 4780 * @param value {@link #valueSet} (Refers to the value set that identifies the set of codes the binding refers to.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 4781 */ 4782 public ElementDefinitionBindingComponent setValueSetElement(CanonicalType value) { 4783 this.valueSet = value; 4784 return this; 4785 } 4786 4787 /** 4788 * @return Refers to the value set that identifies the set of codes the binding refers to. 4789 */ 4790 public String getValueSet() { 4791 return this.valueSet == null ? null : this.valueSet.getValue(); 4792 } 4793 4794 /** 4795 * @param value Refers to the value set that identifies the set of codes the binding refers to. 4796 */ 4797 public ElementDefinitionBindingComponent setValueSet(String value) { 4798 if (Utilities.noString(value)) 4799 this.valueSet = null; 4800 else { 4801 if (this.valueSet == null) 4802 this.valueSet = new CanonicalType(); 4803 this.valueSet.setValue(value); 4804 } 4805 return this; 4806 } 4807 4808 /** 4809 * @return {@link #additional} (Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context.) 4810 */ 4811 public List<ElementDefinitionBindingAdditionalComponent> getAdditional() { 4812 if (this.additional == null) 4813 this.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 4814 return this.additional; 4815 } 4816 4817 /** 4818 * @return Returns a reference to <code>this</code> for easy method chaining 4819 */ 4820 public ElementDefinitionBindingComponent setAdditional(List<ElementDefinitionBindingAdditionalComponent> theAdditional) { 4821 this.additional = theAdditional; 4822 return this; 4823 } 4824 4825 public boolean hasAdditional() { 4826 if (this.additional == null) 4827 return false; 4828 for (ElementDefinitionBindingAdditionalComponent item : this.additional) 4829 if (!item.isEmpty()) 4830 return true; 4831 return false; 4832 } 4833 4834 public ElementDefinitionBindingAdditionalComponent addAdditional() { //3 4835 ElementDefinitionBindingAdditionalComponent t = new ElementDefinitionBindingAdditionalComponent(); 4836 if (this.additional == null) 4837 this.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 4838 this.additional.add(t); 4839 return t; 4840 } 4841 4842 public ElementDefinitionBindingComponent addAdditional(ElementDefinitionBindingAdditionalComponent t) { //3 4843 if (t == null) 4844 return this; 4845 if (this.additional == null) 4846 this.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 4847 this.additional.add(t); 4848 return this; 4849 } 4850 4851 /** 4852 * @return The first repetition of repeating field {@link #additional}, creating it if it does not already exist {3} 4853 */ 4854 public ElementDefinitionBindingAdditionalComponent getAdditionalFirstRep() { 4855 if (getAdditional().isEmpty()) { 4856 addAdditional(); 4857 } 4858 return getAdditional().get(0); 4859 } 4860 4861 protected void listChildren(List<Property> children) { 4862 super.listChildren(children); 4863 children.add(new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength)); 4864 children.add(new Property("description", "markdown", "Describes the intended use of this particular set of codes.", 0, 1, description)); 4865 children.add(new Property("valueSet", "canonical(ValueSet)", "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet)); 4866 children.add(new Property("additional", "", "Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context.", 0, java.lang.Integer.MAX_VALUE, additional)); 4867 } 4868 4869 @Override 4870 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4871 switch (_hash) { 4872 case 1791316033: /*strength*/ return new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength); 4873 case -1724546052: /*description*/ return new Property("description", "markdown", "Describes the intended use of this particular set of codes.", 0, 1, description); 4874 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "Refers to the value set that identifies the set of codes the binding refers to.", 0, 1, valueSet); 4875 case -1931413465: /*additional*/ return new Property("additional", "", "Additional bindings that help applications implementing this element. Additional bindings do not replace the main binding but provide more information and/or context.", 0, java.lang.Integer.MAX_VALUE, additional); 4876 default: return super.getNamedProperty(_hash, _name, _checkValid); 4877 } 4878 4879 } 4880 4881 @Override 4882 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4883 switch (hash) { 4884 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // Enumeration<BindingStrength> 4885 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4886 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 4887 case -1931413465: /*additional*/ return this.additional == null ? new Base[0] : this.additional.toArray(new Base[this.additional.size()]); // ElementDefinitionBindingAdditionalComponent 4888 default: return super.getProperty(hash, name, checkValid); 4889 } 4890 4891 } 4892 4893 @Override 4894 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4895 switch (hash) { 4896 case 1791316033: // strength 4897 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 4898 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4899 return value; 4900 case -1724546052: // description 4901 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4902 return value; 4903 case -1410174671: // valueSet 4904 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4905 return value; 4906 case -1931413465: // additional 4907 this.getAdditional().add((ElementDefinitionBindingAdditionalComponent) value); // ElementDefinitionBindingAdditionalComponent 4908 return value; 4909 default: return super.setProperty(hash, name, value); 4910 } 4911 4912 } 4913 4914 @Override 4915 public Base setProperty(String name, Base value) throws FHIRException { 4916 if (name.equals("strength")) { 4917 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 4918 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4919 } else if (name.equals("description")) { 4920 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4921 } else if (name.equals("valueSet")) { 4922 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 4923 } else if (name.equals("additional")) { 4924 this.getAdditional().add((ElementDefinitionBindingAdditionalComponent) value); 4925 } else 4926 return super.setProperty(name, value); 4927 return value; 4928 } 4929 4930 @Override 4931 public void removeChild(String name, Base value) throws FHIRException { 4932 if (name.equals("strength")) { 4933 value = new BindingStrengthEnumFactory().fromType(TypeConvertor.castToCode(value)); 4934 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 4935 } else if (name.equals("description")) { 4936 this.description = null; 4937 } else if (name.equals("valueSet")) { 4938 this.valueSet = null; 4939 } else if (name.equals("additional")) { 4940 this.getAdditional().remove((ElementDefinitionBindingAdditionalComponent) value); 4941 } else 4942 super.removeChild(name, value); 4943 4944 } 4945 4946 @Override 4947 public Base makeProperty(int hash, String name) throws FHIRException { 4948 switch (hash) { 4949 case 1791316033: return getStrengthElement(); 4950 case -1724546052: return getDescriptionElement(); 4951 case -1410174671: return getValueSetElement(); 4952 case -1931413465: return addAdditional(); 4953 default: return super.makeProperty(hash, name); 4954 } 4955 4956 } 4957 4958 @Override 4959 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4960 switch (hash) { 4961 case 1791316033: /*strength*/ return new String[] {"code"}; 4962 case -1724546052: /*description*/ return new String[] {"markdown"}; 4963 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 4964 case -1931413465: /*additional*/ return new String[] {}; 4965 default: return super.getTypesForProperty(hash, name); 4966 } 4967 4968 } 4969 4970 @Override 4971 public Base addChild(String name) throws FHIRException { 4972 if (name.equals("strength")) { 4973 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.strength"); 4974 } 4975 else if (name.equals("description")) { 4976 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.description"); 4977 } 4978 else if (name.equals("valueSet")) { 4979 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.valueSet"); 4980 } 4981 else if (name.equals("additional")) { 4982 return addAdditional(); 4983 } 4984 else 4985 return super.addChild(name); 4986 } 4987 4988 public ElementDefinitionBindingComponent copy() { 4989 ElementDefinitionBindingComponent dst = new ElementDefinitionBindingComponent(); 4990 copyValues(dst); 4991 return dst; 4992 } 4993 4994 public void copyValues(ElementDefinitionBindingComponent dst) { 4995 super.copyValues(dst); 4996 dst.strength = strength == null ? null : strength.copy(); 4997 dst.description = description == null ? null : description.copy(); 4998 dst.valueSet = valueSet == null ? null : valueSet.copy(); 4999 if (additional != null) { 5000 dst.additional = new ArrayList<ElementDefinitionBindingAdditionalComponent>(); 5001 for (ElementDefinitionBindingAdditionalComponent i : additional) 5002 dst.additional.add(i.copy()); 5003 }; 5004 } 5005 5006 @Override 5007 public boolean equalsDeep(Base other_) { 5008 if (!super.equalsDeep(other_)) 5009 return false; 5010 if (!(other_ instanceof ElementDefinitionBindingComponent)) 5011 return false; 5012 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 5013 return compareDeep(strength, o.strength, true) && compareDeep(description, o.description, true) 5014 && compareDeep(valueSet, o.valueSet, true) && compareDeep(additional, o.additional, true); 5015 } 5016 5017 @Override 5018 public boolean equalsShallow(Base other_) { 5019 if (!super.equalsShallow(other_)) 5020 return false; 5021 if (!(other_ instanceof ElementDefinitionBindingComponent)) 5022 return false; 5023 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 5024 return compareValues(strength, o.strength, true) && compareValues(description, o.description, true) 5025 && compareValues(valueSet, o.valueSet, true); 5026 } 5027 5028 public boolean isEmpty() { 5029 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, description, valueSet 5030 , additional); 5031 } 5032 5033 public String fhirType() { 5034 return "ElementDefinition.binding"; 5035 5036 } 5037 5038 public boolean hasAdditional(ElementDefinitionBindingAdditionalComponent ab) { 5039 if (hasAdditional()) { 5040 for (ElementDefinitionBindingAdditionalComponent t : getAdditional()) { 5041 if (Base.compareDeep(t, ab, false)) { 5042 return true; 5043 } 5044 } 5045 } 5046 return false; 5047 } 5048 5049 } 5050 5051 @Block() 5052 public static class ElementDefinitionBindingAdditionalComponent extends Element implements IBaseDatatypeElement { 5053 /** 5054 * The use of this additional binding. 5055 */ 5056 @Child(name = "purpose", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 5057 @Description(shortDefinition="maximum | minimum | required | extensible | candidate | current | preferred | ui | starter | component", formalDefinition="The use of this additional binding." ) 5058 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/additional-binding-purpose") 5059 protected Enumeration<AdditionalBindingPurposeVS> purpose; 5060 5061 /** 5062 * The valueSet that is being bound for the purpose. 5063 */ 5064 @Child(name = "valueSet", type = {CanonicalType.class}, order=2, min=1, max=1, modifier=false, summary=true) 5065 @Description(shortDefinition="The value set for the additional binding", formalDefinition="The valueSet that is being bound for the purpose." ) 5066 protected CanonicalType valueSet; 5067 5068 /** 5069 * Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used. 5070 */ 5071 @Child(name = "documentation", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=true) 5072 @Description(shortDefinition="Documentation of the purpose of use of the binding", formalDefinition="Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used." ) 5073 protected MarkdownType documentation; 5074 5075 /** 5076 * Concise documentation - for summary tables. 5077 */ 5078 @Child(name = "shortDoco", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 5079 @Description(shortDefinition="Concise documentation - for summary tables", formalDefinition="Concise documentation - for summary tables." ) 5080 protected StringType shortDoco; 5081 5082 /** 5083 * Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling. 5084 */ 5085 @Child(name = "usage", type = {UsageContext.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5086 @Description(shortDefinition="Qualifies the usage - jurisdiction, gender, workflow status etc.", formalDefinition="Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling." ) 5087 protected List<UsageContext> usage; 5088 5089 /** 5090 * Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat. 5091 */ 5092 @Child(name = "any", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=true) 5093 @Description(shortDefinition="Whether binding can applies to all repeats, or just one", formalDefinition="Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat." ) 5094 protected BooleanType any; 5095 5096 private static final long serialVersionUID = -1312796441L; 5097 5098 /** 5099 * Constructor 5100 */ 5101 public ElementDefinitionBindingAdditionalComponent() { 5102 super(); 5103 } 5104 5105 /** 5106 * Constructor 5107 */ 5108 public ElementDefinitionBindingAdditionalComponent(AdditionalBindingPurposeVS purpose, String valueSet) { 5109 super(); 5110 this.setPurpose(purpose); 5111 this.setValueSet(valueSet); 5112 } 5113 5114 /** 5115 * @return {@link #purpose} (The use of this additional binding.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5116 */ 5117 public Enumeration<AdditionalBindingPurposeVS> getPurposeElement() { 5118 if (this.purpose == null) 5119 if (Configuration.errorOnAutoCreate()) 5120 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.purpose"); 5121 else if (Configuration.doAutoCreate()) 5122 this.purpose = new Enumeration<AdditionalBindingPurposeVS>(new AdditionalBindingPurposeVSEnumFactory()); // bb 5123 return this.purpose; 5124 } 5125 5126 public boolean hasPurposeElement() { 5127 return this.purpose != null && !this.purpose.isEmpty(); 5128 } 5129 5130 public boolean hasPurpose() { 5131 return this.purpose != null && !this.purpose.isEmpty(); 5132 } 5133 5134 /** 5135 * @param value {@link #purpose} (The use of this additional binding.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5136 */ 5137 public ElementDefinitionBindingAdditionalComponent setPurposeElement(Enumeration<AdditionalBindingPurposeVS> value) { 5138 this.purpose = value; 5139 return this; 5140 } 5141 5142 /** 5143 * @return The use of this additional binding. 5144 */ 5145 public AdditionalBindingPurposeVS getPurpose() { 5146 return this.purpose == null ? null : this.purpose.getValue(); 5147 } 5148 5149 /** 5150 * @param value The use of this additional binding. 5151 */ 5152 public ElementDefinitionBindingAdditionalComponent setPurpose(AdditionalBindingPurposeVS value) { 5153 if (this.purpose == null) 5154 this.purpose = new Enumeration<AdditionalBindingPurposeVS>(new AdditionalBindingPurposeVSEnumFactory()); 5155 this.purpose.setValue(value); 5156 return this; 5157 } 5158 5159 /** 5160 * @return {@link #valueSet} (The valueSet that is being bound for the purpose.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 5161 */ 5162 public CanonicalType getValueSetElement() { 5163 if (this.valueSet == null) 5164 if (Configuration.errorOnAutoCreate()) 5165 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.valueSet"); 5166 else if (Configuration.doAutoCreate()) 5167 this.valueSet = new CanonicalType(); // bb 5168 return this.valueSet; 5169 } 5170 5171 public boolean hasValueSetElement() { 5172 return this.valueSet != null && !this.valueSet.isEmpty(); 5173 } 5174 5175 public boolean hasValueSet() { 5176 return this.valueSet != null && !this.valueSet.isEmpty(); 5177 } 5178 5179 /** 5180 * @param value {@link #valueSet} (The valueSet that is being bound for the purpose.). This is the underlying object with id, value and extensions. The accessor "getValueSet" gives direct access to the value 5181 */ 5182 public ElementDefinitionBindingAdditionalComponent setValueSetElement(CanonicalType value) { 5183 this.valueSet = value; 5184 return this; 5185 } 5186 5187 /** 5188 * @return The valueSet that is being bound for the purpose. 5189 */ 5190 public String getValueSet() { 5191 return this.valueSet == null ? null : this.valueSet.getValue(); 5192 } 5193 5194 /** 5195 * @param value The valueSet that is being bound for the purpose. 5196 */ 5197 public ElementDefinitionBindingAdditionalComponent setValueSet(String value) { 5198 if (this.valueSet == null) 5199 this.valueSet = new CanonicalType(); 5200 this.valueSet.setValue(value); 5201 return this; 5202 } 5203 5204 /** 5205 * @return {@link #documentation} (Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5206 */ 5207 public MarkdownType getDocumentationElement() { 5208 if (this.documentation == null) 5209 if (Configuration.errorOnAutoCreate()) 5210 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.documentation"); 5211 else if (Configuration.doAutoCreate()) 5212 this.documentation = new MarkdownType(); // bb 5213 return this.documentation; 5214 } 5215 5216 public boolean hasDocumentationElement() { 5217 return this.documentation != null && !this.documentation.isEmpty(); 5218 } 5219 5220 public boolean hasDocumentation() { 5221 return this.documentation != null && !this.documentation.isEmpty(); 5222 } 5223 5224 /** 5225 * @param value {@link #documentation} (Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 5226 */ 5227 public ElementDefinitionBindingAdditionalComponent setDocumentationElement(MarkdownType value) { 5228 this.documentation = value; 5229 return this; 5230 } 5231 5232 /** 5233 * @return Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used. 5234 */ 5235 public String getDocumentation() { 5236 return this.documentation == null ? null : this.documentation.getValue(); 5237 } 5238 5239 /** 5240 * @param value Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used. 5241 */ 5242 public ElementDefinitionBindingAdditionalComponent setDocumentation(String value) { 5243 if (Utilities.noString(value)) 5244 this.documentation = null; 5245 else { 5246 if (this.documentation == null) 5247 this.documentation = new MarkdownType(); 5248 this.documentation.setValue(value); 5249 } 5250 return this; 5251 } 5252 5253 /** 5254 * @return {@link #shortDoco} (Concise documentation - for summary tables.). This is the underlying object with id, value and extensions. The accessor "getShortDoco" gives direct access to the value 5255 */ 5256 public StringType getShortDocoElement() { 5257 if (this.shortDoco == null) 5258 if (Configuration.errorOnAutoCreate()) 5259 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.shortDoco"); 5260 else if (Configuration.doAutoCreate()) 5261 this.shortDoco = new StringType(); // bb 5262 return this.shortDoco; 5263 } 5264 5265 public boolean hasShortDocoElement() { 5266 return this.shortDoco != null && !this.shortDoco.isEmpty(); 5267 } 5268 5269 public boolean hasShortDoco() { 5270 return this.shortDoco != null && !this.shortDoco.isEmpty(); 5271 } 5272 5273 /** 5274 * @param value {@link #shortDoco} (Concise documentation - for summary tables.). This is the underlying object with id, value and extensions. The accessor "getShortDoco" gives direct access to the value 5275 */ 5276 public ElementDefinitionBindingAdditionalComponent setShortDocoElement(StringType value) { 5277 this.shortDoco = value; 5278 return this; 5279 } 5280 5281 /** 5282 * @return Concise documentation - for summary tables. 5283 */ 5284 public String getShortDoco() { 5285 return this.shortDoco == null ? null : this.shortDoco.getValue(); 5286 } 5287 5288 /** 5289 * @param value Concise documentation - for summary tables. 5290 */ 5291 public ElementDefinitionBindingAdditionalComponent setShortDoco(String value) { 5292 if (Utilities.noString(value)) 5293 this.shortDoco = null; 5294 else { 5295 if (this.shortDoco == null) 5296 this.shortDoco = new StringType(); 5297 this.shortDoco.setValue(value); 5298 } 5299 return this; 5300 } 5301 5302 /** 5303 * @return {@link #usage} (Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling.) 5304 */ 5305 public List<UsageContext> getUsage() { 5306 if (this.usage == null) 5307 this.usage = new ArrayList<UsageContext>(); 5308 return this.usage; 5309 } 5310 5311 /** 5312 * @return Returns a reference to <code>this</code> for easy method chaining 5313 */ 5314 public ElementDefinitionBindingAdditionalComponent setUsage(List<UsageContext> theUsage) { 5315 this.usage = theUsage; 5316 return this; 5317 } 5318 5319 public boolean hasUsage() { 5320 if (this.usage == null) 5321 return false; 5322 for (UsageContext item : this.usage) 5323 if (!item.isEmpty()) 5324 return true; 5325 return false; 5326 } 5327 5328 public UsageContext addUsage() { //3 5329 UsageContext t = new UsageContext(); 5330 if (this.usage == null) 5331 this.usage = new ArrayList<UsageContext>(); 5332 this.usage.add(t); 5333 return t; 5334 } 5335 5336 public ElementDefinitionBindingAdditionalComponent addUsage(UsageContext t) { //3 5337 if (t == null) 5338 return this; 5339 if (this.usage == null) 5340 this.usage = new ArrayList<UsageContext>(); 5341 this.usage.add(t); 5342 return this; 5343 } 5344 5345 /** 5346 * @return The first repetition of repeating field {@link #usage}, creating it if it does not already exist {3} 5347 */ 5348 public UsageContext getUsageFirstRep() { 5349 if (getUsage().isEmpty()) { 5350 addUsage(); 5351 } 5352 return getUsage().get(0); 5353 } 5354 5355 /** 5356 * @return {@link #any} (Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.). This is the underlying object with id, value and extensions. The accessor "getAny" gives direct access to the value 5357 */ 5358 public BooleanType getAnyElement() { 5359 if (this.any == null) 5360 if (Configuration.errorOnAutoCreate()) 5361 throw new Error("Attempt to auto-create ElementDefinitionBindingAdditionalComponent.any"); 5362 else if (Configuration.doAutoCreate()) 5363 this.any = new BooleanType(); // bb 5364 return this.any; 5365 } 5366 5367 public boolean hasAnyElement() { 5368 return this.any != null && !this.any.isEmpty(); 5369 } 5370 5371 public boolean hasAny() { 5372 return this.any != null && !this.any.isEmpty(); 5373 } 5374 5375 /** 5376 * @param value {@link #any} (Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.). This is the underlying object with id, value and extensions. The accessor "getAny" gives direct access to the value 5377 */ 5378 public ElementDefinitionBindingAdditionalComponent setAnyElement(BooleanType value) { 5379 this.any = value; 5380 return this; 5381 } 5382 5383 /** 5384 * @return Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat. 5385 */ 5386 public boolean getAny() { 5387 return this.any == null || this.any.isEmpty() ? false : this.any.getValue(); 5388 } 5389 5390 /** 5391 * @param value Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat. 5392 */ 5393 public ElementDefinitionBindingAdditionalComponent setAny(boolean value) { 5394 if (this.any == null) 5395 this.any = new BooleanType(); 5396 this.any.setValue(value); 5397 return this; 5398 } 5399 5400 protected void listChildren(List<Property> children) { 5401 super.listChildren(children); 5402 children.add(new Property("purpose", "code", "The use of this additional binding.", 0, 1, purpose)); 5403 children.add(new Property("valueSet", "canonical(ValueSet)", "The valueSet that is being bound for the purpose.", 0, 1, valueSet)); 5404 children.add(new Property("documentation", "markdown", "Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.", 0, 1, documentation)); 5405 children.add(new Property("shortDoco", "string", "Concise documentation - for summary tables.", 0, 1, shortDoco)); 5406 children.add(new Property("usage", "UsageContext", "Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling.", 0, java.lang.Integer.MAX_VALUE, usage)); 5407 children.add(new Property("any", "boolean", "Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.", 0, 1, any)); 5408 } 5409 5410 @Override 5411 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5412 switch (_hash) { 5413 case -220463842: /*purpose*/ return new Property("purpose", "code", "The use of this additional binding.", 0, 1, purpose); 5414 case -1410174671: /*valueSet*/ return new Property("valueSet", "canonical(ValueSet)", "The valueSet that is being bound for the purpose.", 0, 1, valueSet); 5415 case 1587405498: /*documentation*/ return new Property("documentation", "markdown", "Documentation of the purpose of use of the bindingproviding additional information about how it is intended to be used.", 0, 1, documentation); 5416 case -2028503853: /*shortDoco*/ return new Property("shortDoco", "string", "Concise documentation - for summary tables.", 0, 1, shortDoco); 5417 case 111574433: /*usage*/ return new Property("usage", "UsageContext", "Qualifies the usage of the binding. Typically bindings are qualified by jurisdiction, but they may also be qualified by gender, workflow status, clinical domain etc. The information to decide whether a usege context applies is usually outside the resource, determined by context, and this might present challenges for validation tooling.", 0, java.lang.Integer.MAX_VALUE, usage); 5418 case 96748: /*any*/ return new Property("any", "boolean", "Whether the binding applies to all repeats, or just to any one of them. This is only relevant for elements that can repeat.", 0, 1, any); 5419 default: return super.getNamedProperty(_hash, _name, _checkValid); 5420 } 5421 5422 } 5423 5424 @Override 5425 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5426 switch (hash) { 5427 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // Enumeration<AdditionalBindingPurposeVS> 5428 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // CanonicalType 5429 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // MarkdownType 5430 case -2028503853: /*shortDoco*/ return this.shortDoco == null ? new Base[0] : new Base[] {this.shortDoco}; // StringType 5431 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : this.usage.toArray(new Base[this.usage.size()]); // UsageContext 5432 case 96748: /*any*/ return this.any == null ? new Base[0] : new Base[] {this.any}; // BooleanType 5433 default: return super.getProperty(hash, name, checkValid); 5434 } 5435 5436 } 5437 5438 @Override 5439 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5440 switch (hash) { 5441 case -220463842: // purpose 5442 value = new AdditionalBindingPurposeVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 5443 this.purpose = (Enumeration) value; // Enumeration<AdditionalBindingPurposeVS> 5444 return value; 5445 case -1410174671: // valueSet 5446 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 5447 return value; 5448 case 1587405498: // documentation 5449 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5450 return value; 5451 case -2028503853: // shortDoco 5452 this.shortDoco = TypeConvertor.castToString(value); // StringType 5453 return value; 5454 case 111574433: // usage 5455 this.getUsage().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5456 return value; 5457 case 96748: // any 5458 this.any = TypeConvertor.castToBoolean(value); // BooleanType 5459 return value; 5460 default: return super.setProperty(hash, name, value); 5461 } 5462 5463 } 5464 5465 @Override 5466 public Base setProperty(String name, Base value) throws FHIRException { 5467 if (name.equals("purpose")) { 5468 value = new AdditionalBindingPurposeVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 5469 this.purpose = (Enumeration) value; // Enumeration<AdditionalBindingPurposeVS> 5470 } else if (name.equals("valueSet")) { 5471 this.valueSet = TypeConvertor.castToCanonical(value); // CanonicalType 5472 } else if (name.equals("documentation")) { 5473 this.documentation = TypeConvertor.castToMarkdown(value); // MarkdownType 5474 } else if (name.equals("shortDoco")) { 5475 this.shortDoco = TypeConvertor.castToString(value); // StringType 5476 } else if (name.equals("usage")) { 5477 this.getUsage().add(TypeConvertor.castToUsageContext(value)); 5478 } else if (name.equals("any")) { 5479 this.any = TypeConvertor.castToBoolean(value); // BooleanType 5480 } else 5481 return super.setProperty(name, value); 5482 return value; 5483 } 5484 5485 @Override 5486 public void removeChild(String name, Base value) throws FHIRException { 5487 if (name.equals("purpose")) { 5488 value = new AdditionalBindingPurposeVSEnumFactory().fromType(TypeConvertor.castToCode(value)); 5489 this.purpose = (Enumeration) value; // Enumeration<AdditionalBindingPurposeVS> 5490 } else if (name.equals("valueSet")) { 5491 this.valueSet = null; 5492 } else if (name.equals("documentation")) { 5493 this.documentation = null; 5494 } else if (name.equals("shortDoco")) { 5495 this.shortDoco = null; 5496 } else if (name.equals("usage")) { 5497 this.getUsage().remove(value); 5498 } else if (name.equals("any")) { 5499 this.any = null; 5500 } else 5501 super.removeChild(name, value); 5502 5503 } 5504 5505 @Override 5506 public Base makeProperty(int hash, String name) throws FHIRException { 5507 switch (hash) { 5508 case -220463842: return getPurposeElement(); 5509 case -1410174671: return getValueSetElement(); 5510 case 1587405498: return getDocumentationElement(); 5511 case -2028503853: return getShortDocoElement(); 5512 case 111574433: return addUsage(); 5513 case 96748: return getAnyElement(); 5514 default: return super.makeProperty(hash, name); 5515 } 5516 5517 } 5518 5519 @Override 5520 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5521 switch (hash) { 5522 case -220463842: /*purpose*/ return new String[] {"code"}; 5523 case -1410174671: /*valueSet*/ return new String[] {"canonical"}; 5524 case 1587405498: /*documentation*/ return new String[] {"markdown"}; 5525 case -2028503853: /*shortDoco*/ return new String[] {"string"}; 5526 case 111574433: /*usage*/ return new String[] {"UsageContext"}; 5527 case 96748: /*any*/ return new String[] {"boolean"}; 5528 default: return super.getTypesForProperty(hash, name); 5529 } 5530 5531 } 5532 5533 @Override 5534 public Base addChild(String name) throws FHIRException { 5535 if (name.equals("purpose")) { 5536 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.purpose"); 5537 } 5538 else if (name.equals("valueSet")) { 5539 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.valueSet"); 5540 } 5541 else if (name.equals("documentation")) { 5542 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.documentation"); 5543 } 5544 else if (name.equals("shortDoco")) { 5545 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.shortDoco"); 5546 } 5547 else if (name.equals("usage")) { 5548 return addUsage(); 5549 } 5550 else if (name.equals("any")) { 5551 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.binding.additional.any"); 5552 } 5553 else 5554 return super.addChild(name); 5555 } 5556 5557 public ElementDefinitionBindingAdditionalComponent copy() { 5558 ElementDefinitionBindingAdditionalComponent dst = new ElementDefinitionBindingAdditionalComponent(); 5559 copyValues(dst); 5560 return dst; 5561 } 5562 5563 public void copyValues(ElementDefinitionBindingAdditionalComponent dst) { 5564 super.copyValues(dst); 5565 dst.purpose = purpose == null ? null : purpose.copy(); 5566 dst.valueSet = valueSet == null ? null : valueSet.copy(); 5567 dst.documentation = documentation == null ? null : documentation.copy(); 5568 dst.shortDoco = shortDoco == null ? null : shortDoco.copy(); 5569 if (usage != null) { 5570 dst.usage = new ArrayList<UsageContext>(); 5571 for (UsageContext i : usage) 5572 dst.usage.add(i.copy()); 5573 }; 5574 dst.any = any == null ? null : any.copy(); 5575 } 5576 5577 @Override 5578 public boolean equalsDeep(Base other_) { 5579 if (!super.equalsDeep(other_)) 5580 return false; 5581 if (!(other_ instanceof ElementDefinitionBindingAdditionalComponent)) 5582 return false; 5583 ElementDefinitionBindingAdditionalComponent o = (ElementDefinitionBindingAdditionalComponent) other_; 5584 return compareDeep(purpose, o.purpose, true) && compareDeep(valueSet, o.valueSet, true) && compareDeep(documentation, o.documentation, true) 5585 && compareDeep(shortDoco, o.shortDoco, true) && compareDeep(usage, o.usage, true) && compareDeep(any, o.any, true) 5586 ; 5587 } 5588 5589 @Override 5590 public boolean equalsShallow(Base other_) { 5591 if (!super.equalsShallow(other_)) 5592 return false; 5593 if (!(other_ instanceof ElementDefinitionBindingAdditionalComponent)) 5594 return false; 5595 ElementDefinitionBindingAdditionalComponent o = (ElementDefinitionBindingAdditionalComponent) other_; 5596 return compareValues(purpose, o.purpose, true) && compareValues(valueSet, o.valueSet, true) && compareValues(documentation, o.documentation, true) 5597 && compareValues(shortDoco, o.shortDoco, true) && compareValues(any, o.any, true); 5598 } 5599 5600 public boolean isEmpty() { 5601 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, valueSet, documentation 5602 , shortDoco, usage, any); 5603 } 5604 5605 public String fhirType() { 5606 return "ElementDefinition.binding.additional"; 5607 5608 } 5609 5610 } 5611 5612 @Block() 5613 public static class ElementDefinitionMappingComponent extends Element implements IBaseDatatypeElement { 5614 /** 5615 * An internal reference to the definition of a mapping. 5616 */ 5617 @Child(name = "identity", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 5618 @Description(shortDefinition="Reference to mapping declaration", formalDefinition="An internal reference to the definition of a mapping." ) 5619 protected IdType identity; 5620 5621 /** 5622 * Identifies the computable language in which mapping.map is expressed. 5623 */ 5624 @Child(name = "language", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 5625 @Description(shortDefinition="Computable language of mapping", formalDefinition="Identifies the computable language in which mapping.map is expressed." ) 5626 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 5627 protected CodeType language; 5628 5629 /** 5630 * Expresses what part of the target specification corresponds to this element. 5631 */ 5632 @Child(name = "map", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 5633 @Description(shortDefinition="Details of the mapping", formalDefinition="Expresses what part of the target specification corresponds to this element." ) 5634 protected StringType map; 5635 5636 /** 5637 * Comments that provide information about the mapping or its use. 5638 */ 5639 @Child(name = "comment", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=true) 5640 @Description(shortDefinition="Comments about the mapping or its use", formalDefinition="Comments that provide information about the mapping or its use." ) 5641 protected MarkdownType comment; 5642 5643 private static final long serialVersionUID = -582458727L; 5644 5645 /** 5646 * Constructor 5647 */ 5648 public ElementDefinitionMappingComponent() { 5649 super(); 5650 } 5651 5652 /** 5653 * Constructor 5654 */ 5655 public ElementDefinitionMappingComponent(String identity, String map) { 5656 super(); 5657 this.setIdentity(identity); 5658 this.setMap(map); 5659 } 5660 5661 /** 5662 * @return {@link #identity} (An internal reference to the definition of a mapping.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 5663 */ 5664 public IdType getIdentityElement() { 5665 if (this.identity == null) 5666 if (Configuration.errorOnAutoCreate()) 5667 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.identity"); 5668 else if (Configuration.doAutoCreate()) 5669 this.identity = new IdType(); // bb 5670 return this.identity; 5671 } 5672 5673 public boolean hasIdentityElement() { 5674 return this.identity != null && !this.identity.isEmpty(); 5675 } 5676 5677 public boolean hasIdentity() { 5678 return this.identity != null && !this.identity.isEmpty(); 5679 } 5680 5681 /** 5682 * @param value {@link #identity} (An internal reference to the definition of a mapping.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 5683 */ 5684 public ElementDefinitionMappingComponent setIdentityElement(IdType value) { 5685 this.identity = value; 5686 return this; 5687 } 5688 5689 /** 5690 * @return An internal reference to the definition of a mapping. 5691 */ 5692 public String getIdentity() { 5693 return this.identity == null ? null : this.identity.getValue(); 5694 } 5695 5696 /** 5697 * @param value An internal reference to the definition of a mapping. 5698 */ 5699 public ElementDefinitionMappingComponent setIdentity(String value) { 5700 if (this.identity == null) 5701 this.identity = new IdType(); 5702 this.identity.setValue(value); 5703 return this; 5704 } 5705 5706 /** 5707 * @return {@link #language} (Identifies the computable language in which mapping.map is expressed.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 5708 */ 5709 public CodeType getLanguageElement() { 5710 if (this.language == null) 5711 if (Configuration.errorOnAutoCreate()) 5712 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.language"); 5713 else if (Configuration.doAutoCreate()) 5714 this.language = new CodeType(); // bb 5715 return this.language; 5716 } 5717 5718 public boolean hasLanguageElement() { 5719 return this.language != null && !this.language.isEmpty(); 5720 } 5721 5722 public boolean hasLanguage() { 5723 return this.language != null && !this.language.isEmpty(); 5724 } 5725 5726 /** 5727 * @param value {@link #language} (Identifies the computable language in which mapping.map is expressed.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 5728 */ 5729 public ElementDefinitionMappingComponent setLanguageElement(CodeType value) { 5730 this.language = value; 5731 return this; 5732 } 5733 5734 /** 5735 * @return Identifies the computable language in which mapping.map is expressed. 5736 */ 5737 public String getLanguage() { 5738 return this.language == null ? null : this.language.getValue(); 5739 } 5740 5741 /** 5742 * @param value Identifies the computable language in which mapping.map is expressed. 5743 */ 5744 public ElementDefinitionMappingComponent setLanguage(String value) { 5745 if (Utilities.noString(value)) 5746 this.language = null; 5747 else { 5748 if (this.language == null) 5749 this.language = new CodeType(); 5750 this.language.setValue(value); 5751 } 5752 return this; 5753 } 5754 5755 /** 5756 * @return {@link #map} (Expresses what part of the target specification corresponds to this element.). This is the underlying object with id, value and extensions. The accessor "getMap" gives direct access to the value 5757 */ 5758 public StringType getMapElement() { 5759 if (this.map == null) 5760 if (Configuration.errorOnAutoCreate()) 5761 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.map"); 5762 else if (Configuration.doAutoCreate()) 5763 this.map = new StringType(); // bb 5764 return this.map; 5765 } 5766 5767 public boolean hasMapElement() { 5768 return this.map != null && !this.map.isEmpty(); 5769 } 5770 5771 public boolean hasMap() { 5772 return this.map != null && !this.map.isEmpty(); 5773 } 5774 5775 /** 5776 * @param value {@link #map} (Expresses what part of the target specification corresponds to this element.). This is the underlying object with id, value and extensions. The accessor "getMap" gives direct access to the value 5777 */ 5778 public ElementDefinitionMappingComponent setMapElement(StringType value) { 5779 this.map = value; 5780 return this; 5781 } 5782 5783 /** 5784 * @return Expresses what part of the target specification corresponds to this element. 5785 */ 5786 public String getMap() { 5787 return this.map == null ? null : this.map.getValue(); 5788 } 5789 5790 /** 5791 * @param value Expresses what part of the target specification corresponds to this element. 5792 */ 5793 public ElementDefinitionMappingComponent setMap(String value) { 5794 if (this.map == null) 5795 this.map = new StringType(); 5796 this.map.setValue(value); 5797 return this; 5798 } 5799 5800 /** 5801 * @return {@link #comment} (Comments that provide information about the mapping or its use.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 5802 */ 5803 public MarkdownType getCommentElement() { 5804 if (this.comment == null) 5805 if (Configuration.errorOnAutoCreate()) 5806 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.comment"); 5807 else if (Configuration.doAutoCreate()) 5808 this.comment = new MarkdownType(); // bb 5809 return this.comment; 5810 } 5811 5812 public boolean hasCommentElement() { 5813 return this.comment != null && !this.comment.isEmpty(); 5814 } 5815 5816 public boolean hasComment() { 5817 return this.comment != null && !this.comment.isEmpty(); 5818 } 5819 5820 /** 5821 * @param value {@link #comment} (Comments that provide information about the mapping or its use.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 5822 */ 5823 public ElementDefinitionMappingComponent setCommentElement(MarkdownType value) { 5824 this.comment = value; 5825 return this; 5826 } 5827 5828 /** 5829 * @return Comments that provide information about the mapping or its use. 5830 */ 5831 public String getComment() { 5832 return this.comment == null ? null : this.comment.getValue(); 5833 } 5834 5835 /** 5836 * @param value Comments that provide information about the mapping or its use. 5837 */ 5838 public ElementDefinitionMappingComponent setComment(String value) { 5839 if (Utilities.noString(value)) 5840 this.comment = null; 5841 else { 5842 if (this.comment == null) 5843 this.comment = new MarkdownType(); 5844 this.comment.setValue(value); 5845 } 5846 return this; 5847 } 5848 5849 protected void listChildren(List<Property> children) { 5850 super.listChildren(children); 5851 children.add(new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity)); 5852 children.add(new Property("language", "code", "Identifies the computable language in which mapping.map is expressed.", 0, 1, language)); 5853 children.add(new Property("map", "string", "Expresses what part of the target specification corresponds to this element.", 0, 1, map)); 5854 children.add(new Property("comment", "markdown", "Comments that provide information about the mapping or its use.", 0, 1, comment)); 5855 } 5856 5857 @Override 5858 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5859 switch (_hash) { 5860 case -135761730: /*identity*/ return new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity); 5861 case -1613589672: /*language*/ return new Property("language", "code", "Identifies the computable language in which mapping.map is expressed.", 0, 1, language); 5862 case 107868: /*map*/ return new Property("map", "string", "Expresses what part of the target specification corresponds to this element.", 0, 1, map); 5863 case 950398559: /*comment*/ return new Property("comment", "markdown", "Comments that provide information about the mapping or its use.", 0, 1, comment); 5864 default: return super.getNamedProperty(_hash, _name, _checkValid); 5865 } 5866 5867 } 5868 5869 @Override 5870 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5871 switch (hash) { 5872 case -135761730: /*identity*/ return this.identity == null ? new Base[0] : new Base[] {this.identity}; // IdType 5873 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 5874 case 107868: /*map*/ return this.map == null ? new Base[0] : new Base[] {this.map}; // StringType 5875 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 5876 default: return super.getProperty(hash, name, checkValid); 5877 } 5878 5879 } 5880 5881 @Override 5882 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5883 switch (hash) { 5884 case -135761730: // identity 5885 this.identity = TypeConvertor.castToId(value); // IdType 5886 return value; 5887 case -1613589672: // language 5888 this.language = TypeConvertor.castToCode(value); // CodeType 5889 return value; 5890 case 107868: // map 5891 this.map = TypeConvertor.castToString(value); // StringType 5892 return value; 5893 case 950398559: // comment 5894 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 5895 return value; 5896 default: return super.setProperty(hash, name, value); 5897 } 5898 5899 } 5900 5901 @Override 5902 public Base setProperty(String name, Base value) throws FHIRException { 5903 if (name.equals("identity")) { 5904 this.identity = TypeConvertor.castToId(value); // IdType 5905 } else if (name.equals("language")) { 5906 this.language = TypeConvertor.castToCode(value); // CodeType 5907 } else if (name.equals("map")) { 5908 this.map = TypeConvertor.castToString(value); // StringType 5909 } else if (name.equals("comment")) { 5910 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 5911 } else 5912 return super.setProperty(name, value); 5913 return value; 5914 } 5915 5916 @Override 5917 public void removeChild(String name, Base value) throws FHIRException { 5918 if (name.equals("identity")) { 5919 this.identity = null; 5920 } else if (name.equals("language")) { 5921 this.language = null; 5922 } else if (name.equals("map")) { 5923 this.map = null; 5924 } else if (name.equals("comment")) { 5925 this.comment = null; 5926 } else 5927 super.removeChild(name, value); 5928 5929 } 5930 5931 @Override 5932 public Base makeProperty(int hash, String name) throws FHIRException { 5933 switch (hash) { 5934 case -135761730: return getIdentityElement(); 5935 case -1613589672: return getLanguageElement(); 5936 case 107868: return getMapElement(); 5937 case 950398559: return getCommentElement(); 5938 default: return super.makeProperty(hash, name); 5939 } 5940 5941 } 5942 5943 @Override 5944 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5945 switch (hash) { 5946 case -135761730: /*identity*/ return new String[] {"id"}; 5947 case -1613589672: /*language*/ return new String[] {"code"}; 5948 case 107868: /*map*/ return new String[] {"string"}; 5949 case 950398559: /*comment*/ return new String[] {"markdown"}; 5950 default: return super.getTypesForProperty(hash, name); 5951 } 5952 5953 } 5954 5955 @Override 5956 public Base addChild(String name) throws FHIRException { 5957 if (name.equals("identity")) { 5958 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.identity"); 5959 } 5960 else if (name.equals("language")) { 5961 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.language"); 5962 } 5963 else if (name.equals("map")) { 5964 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.map"); 5965 } 5966 else if (name.equals("comment")) { 5967 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mapping.comment"); 5968 } 5969 else 5970 return super.addChild(name); 5971 } 5972 5973 public ElementDefinitionMappingComponent copy() { 5974 ElementDefinitionMappingComponent dst = new ElementDefinitionMappingComponent(); 5975 copyValues(dst); 5976 return dst; 5977 } 5978 5979 public void copyValues(ElementDefinitionMappingComponent dst) { 5980 super.copyValues(dst); 5981 dst.identity = identity == null ? null : identity.copy(); 5982 dst.language = language == null ? null : language.copy(); 5983 dst.map = map == null ? null : map.copy(); 5984 dst.comment = comment == null ? null : comment.copy(); 5985 } 5986 5987 @Override 5988 public boolean equalsDeep(Base other_) { 5989 if (!super.equalsDeep(other_)) 5990 return false; 5991 if (!(other_ instanceof ElementDefinitionMappingComponent)) 5992 return false; 5993 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 5994 return compareDeep(identity, o.identity, true) && compareDeep(language, o.language, true) && compareDeep(map, o.map, true) 5995 && compareDeep(comment, o.comment, true); 5996 } 5997 5998 @Override 5999 public boolean equalsShallow(Base other_) { 6000 if (!super.equalsShallow(other_)) 6001 return false; 6002 if (!(other_ instanceof ElementDefinitionMappingComponent)) 6003 return false; 6004 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 6005 return compareValues(identity, o.identity, true) && compareValues(language, o.language, true) && compareValues(map, o.map, true) 6006 && compareValues(comment, o.comment, true); 6007 } 6008 6009 public boolean isEmpty() { 6010 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, language, map 6011 , comment); 6012 } 6013 6014 public String fhirType() { 6015 return "ElementDefinition.mapping"; 6016 6017 } 6018 6019 } 6020 6021 /** 6022 * The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 6023 */ 6024 @Child(name = "path", type = {StringType.class}, order=0, min=1, max=1, modifier=false, summary=true) 6025 @Description(shortDefinition="Path of the element in the hierarchy of elements", formalDefinition="The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension." ) 6026 protected StringType path; 6027 6028 /** 6029 * Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used. 6030 */ 6031 @Child(name = "representation", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6032 @Description(shortDefinition="xmlAttr | xmlText | typeAttr | cdaText | xhtml", formalDefinition="Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used." ) 6033 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/property-representation") 6034 protected List<Enumeration<PropertyRepresentation>> representation; 6035 6036 /** 6037 * The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 6038 */ 6039 @Child(name = "sliceName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 6040 @Description(shortDefinition="Name for this particular element (in a set of slices)", formalDefinition="The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element." ) 6041 protected StringType sliceName; 6042 6043 /** 6044 * If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName. 6045 */ 6046 @Child(name = "sliceIsConstraining", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 6047 @Description(shortDefinition="If this slice definition constrains an inherited slice definition (or not)", formalDefinition="If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName." ) 6048 protected BooleanType sliceIsConstraining; 6049 6050 /** 6051 * A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 6052 */ 6053 @Child(name = "label", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 6054 @Description(shortDefinition="Name for element to display with or prompt for element", formalDefinition="A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form." ) 6055 protected StringType label; 6056 6057 /** 6058 * A code that has the same meaning as the element in a particular terminology. 6059 */ 6060 @Child(name = "code", type = {Coding.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6061 @Description(shortDefinition="Corresponding codes in terminologies", formalDefinition="A code that has the same meaning as the element in a particular terminology." ) 6062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://loinc.org/vs") 6063 protected List<Coding> code; 6064 6065 /** 6066 * Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set). 6067 */ 6068 @Child(name = "slicing", type = {}, order=6, min=0, max=1, modifier=false, summary=true) 6069 @Description(shortDefinition="This element is sliced - slices follow", formalDefinition="Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set)." ) 6070 protected ElementDefinitionSlicingComponent slicing; 6071 6072 /** 6073 * A concise description of what this element means (e.g. for use in autogenerated summaries). 6074 */ 6075 @Child(name = "short", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 6076 @Description(shortDefinition="Concise definition for space-constrained presentation", formalDefinition="A concise description of what this element means (e.g. for use in autogenerated summaries)." ) 6077 protected StringType short_; 6078 6079 /** 6080 * Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition). 6081 */ 6082 @Child(name = "definition", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=true) 6083 @Description(shortDefinition="Full formal definition as narrative text", formalDefinition="Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition)." ) 6084 protected MarkdownType definition; 6085 6086 /** 6087 * Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment). 6088 */ 6089 @Child(name = "comment", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 6090 @Description(shortDefinition="Comments about the use of this element", formalDefinition="Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment)." ) 6091 protected MarkdownType comment; 6092 6093 /** 6094 * This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 6095 */ 6096 @Child(name = "requirements", type = {MarkdownType.class}, order=10, min=0, max=1, modifier=false, summary=true) 6097 @Description(shortDefinition="Why this resource has been created", formalDefinition="This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element." ) 6098 protected MarkdownType requirements; 6099 6100 /** 6101 * Identifies additional names by which this element might also be known. 6102 */ 6103 @Child(name = "alias", type = {StringType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6104 @Description(shortDefinition="Other names", formalDefinition="Identifies additional names by which this element might also be known." ) 6105 protected List<StringType> alias; 6106 6107 /** 6108 * The minimum number of times this element SHALL appear in the instance. 6109 */ 6110 @Child(name = "min", type = {UnsignedIntType.class}, order=12, min=0, max=1, modifier=false, summary=true) 6111 @Description(shortDefinition="Minimum Cardinality", formalDefinition="The minimum number of times this element SHALL appear in the instance." ) 6112 protected UnsignedIntType min; 6113 6114 /** 6115 * The maximum number of times this element is permitted to appear in the instance. 6116 */ 6117 @Child(name = "max", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=true) 6118 @Description(shortDefinition="Maximum Cardinality (a number or *)", formalDefinition="The maximum number of times this element is permitted to appear in the instance." ) 6119 protected StringType max; 6120 6121 /** 6122 * Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same. 6123 */ 6124 @Child(name = "base", type = {}, order=14, min=0, max=1, modifier=false, summary=true) 6125 @Description(shortDefinition="Base definition information for tools", formalDefinition="Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same." ) 6126 protected ElementDefinitionBaseComponent base; 6127 6128 /** 6129 * Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc. 6130 */ 6131 @Child(name = "contentReference", type = {UriType.class}, order=15, min=0, max=1, modifier=false, summary=true) 6132 @Description(shortDefinition="Reference to definition of content for the element", formalDefinition="Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc." ) 6133 protected UriType contentReference; 6134 6135 /** 6136 * The data type or resource that the value of this element is permitted to be. 6137 */ 6138 @Child(name = "type", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6139 @Description(shortDefinition="Data type and Profile for this element", formalDefinition="The data type or resource that the value of this element is permitted to be." ) 6140 protected List<TypeRefComponent> type; 6141 6142 /** 6143 * The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false'). 6144 */ 6145 @Child(name = "defaultValue", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=17, min=0, max=1, modifier=false, summary=true) 6146 @Description(shortDefinition="Specified value if missing from instance", formalDefinition="The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false')." ) 6147 protected DataType defaultValue; 6148 6149 /** 6150 * The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'). 6151 */ 6152 @Child(name = "meaningWhenMissing", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=true) 6153 @Description(shortDefinition="Implicit meaning when this element is missing", formalDefinition="The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing')." ) 6154 protected MarkdownType meaningWhenMissing; 6155 6156 /** 6157 * If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 6158 */ 6159 @Child(name = "orderMeaning", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=true) 6160 @Description(shortDefinition="What the order of the elements means", formalDefinition="If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning." ) 6161 protected StringType orderMeaning; 6162 6163 /** 6164 * Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing. 6165 */ 6166 @Child(name = "fixed", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=20, min=0, max=1, modifier=false, summary=true) 6167 @Description(shortDefinition="Value must be exactly this", formalDefinition="Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing." ) 6168 protected DataType fixed; 6169 6170 /** 6171 * Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 6172 6173When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 6174 6175When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 6176 6177When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 6178 61791. If primitive: it must match exactly the pattern value 61802. If a complex object: it must match (recursively) the pattern value 61813. If an array: it must match (recursively) the pattern value 6182 6183If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have. 6184 */ 6185 @Child(name = "pattern", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=21, min=0, max=1, modifier=false, summary=true) 6186 @Description(shortDefinition="Value must have at least these property values", formalDefinition="Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have." ) 6187 protected DataType pattern; 6188 6189 /** 6190 * A sample value for this element demonstrating the type of information that would typically be found in the element. 6191 */ 6192 @Child(name = "example", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6193 @Description(shortDefinition="Example value (as defined for type)", formalDefinition="A sample value for this element demonstrating the type of information that would typically be found in the element." ) 6194 protected List<ElementDefinitionExampleComponent> example; 6195 6196 /** 6197 * The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity. 6198 */ 6199 @Child(name = "minValue", type = {DateType.class, DateTimeType.class, InstantType.class, TimeType.class, DecimalType.class, IntegerType.class, Integer64Type.class, PositiveIntType.class, UnsignedIntType.class, Quantity.class}, order=23, min=0, max=1, modifier=false, summary=true) 6200 @Description(shortDefinition="Minimum Allowed Value (for some types)", formalDefinition="The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity." ) 6201 protected DataType minValue; 6202 6203 /** 6204 * The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity. 6205 */ 6206 @Child(name = "maxValue", type = {DateType.class, DateTimeType.class, InstantType.class, TimeType.class, DecimalType.class, IntegerType.class, Integer64Type.class, PositiveIntType.class, UnsignedIntType.class, Quantity.class}, order=24, min=0, max=1, modifier=false, summary=true) 6207 @Description(shortDefinition="Maximum Allowed Value (for some types)", formalDefinition="The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity." ) 6208 protected DataType maxValue; 6209 6210 /** 6211 * Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)). 6212 */ 6213 @Child(name = "maxLength", type = {IntegerType.class}, order=25, min=0, max=1, modifier=false, summary=true) 6214 @Description(shortDefinition="Max length for string type data", formalDefinition="Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html))." ) 6215 protected IntegerType maxLength; 6216 6217 /** 6218 * A reference to an invariant that may make additional statements about the cardinality or value in the instance. 6219 */ 6220 @Child(name = "condition", type = {IdType.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6221 @Description(shortDefinition="Reference to invariant about presence", formalDefinition="A reference to an invariant that may make additional statements about the cardinality or value in the instance." ) 6222 protected List<IdType> condition; 6223 6224 /** 6225 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance. 6226 */ 6227 @Child(name = "constraint", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6228 @Description(shortDefinition="Condition that must evaluate to true", formalDefinition="Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance." ) 6229 protected List<ElementDefinitionConstraintComponent> constraint; 6230 6231 /** 6232 * Specifies for a primitive data type that the value of the data type cannot be replaced by an extension. 6233 */ 6234 @Child(name = "mustHaveValue", type = {BooleanType.class}, order=28, min=0, max=1, modifier=false, summary=true) 6235 @Description(shortDefinition="For primitives, that a value must be present - not replaced by an extension", formalDefinition="Specifies for a primitive data type that the value of the data type cannot be replaced by an extension." ) 6236 protected BooleanType mustHaveValue; 6237 6238 /** 6239 * Specifies a list of extensions that can appear in place of a primitive value. 6240 */ 6241 @Child(name = "valueAlternatives", type = {CanonicalType.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6242 @Description(shortDefinition="Extensions that are allowed to replace a primitive value", formalDefinition="Specifies a list of extensions that can appear in place of a primitive value." ) 6243 protected List<CanonicalType> valueAlternatives; 6244 6245 /** 6246 * If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation. 6247 */ 6248 @Child(name = "mustSupport", type = {BooleanType.class}, order=30, min=0, max=1, modifier=false, summary=true) 6249 @Description(shortDefinition="If the element must be supported (discouraged - see obligations)", formalDefinition="If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation." ) 6250 protected BooleanType mustSupport; 6251 6252 /** 6253 * If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension. 6254 */ 6255 @Child(name = "isModifier", type = {BooleanType.class}, order=31, min=0, max=1, modifier=false, summary=true) 6256 @Description(shortDefinition="If this modifies the meaning of other elements", formalDefinition="If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension." ) 6257 protected BooleanType isModifier; 6258 6259 /** 6260 * Explains how that element affects the interpretation of the resource or element that contains it. 6261 */ 6262 @Child(name = "isModifierReason", type = {StringType.class}, order=32, min=0, max=1, modifier=false, summary=true) 6263 @Description(shortDefinition="Reason that this element is marked as a modifier", formalDefinition="Explains how that element affects the interpretation of the resource or element that contains it." ) 6264 protected StringType isModifierReason; 6265 6266 /** 6267 * Whether the element should be included if a client requests a search with the parameter _summary=true. 6268 */ 6269 @Child(name = "isSummary", type = {BooleanType.class}, order=33, min=0, max=1, modifier=false, summary=true) 6270 @Description(shortDefinition="Include when _summary = true?", formalDefinition="Whether the element should be included if a client requests a search with the parameter _summary=true." ) 6271 protected BooleanType isSummary; 6272 6273 /** 6274 * Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri). 6275 */ 6276 @Child(name = "binding", type = {}, order=34, min=0, max=1, modifier=false, summary=true) 6277 @Description(shortDefinition="ValueSet details if this is coded", formalDefinition="Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri)." ) 6278 protected ElementDefinitionBindingComponent binding; 6279 6280 /** 6281 * Identifies a concept from an external specification that roughly corresponds to this element. 6282 */ 6283 @Child(name = "mapping", type = {}, order=35, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 6284 @Description(shortDefinition="Map element to another set of definitions", formalDefinition="Identifies a concept from an external specification that roughly corresponds to this element." ) 6285 protected List<ElementDefinitionMappingComponent> mapping; 6286 6287 private static final long serialVersionUID = -1474105308L; 6288 6289 /** 6290 * Constructor 6291 */ 6292 public ElementDefinition() { 6293 super(); 6294 } 6295 6296 /** 6297 * Constructor 6298 */ 6299 public ElementDefinition(String path) { 6300 super(); 6301 this.setPath(path); 6302 } 6303 6304 /** 6305 * @return {@link #path} (The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 6306 */ 6307 public StringType getPathElement() { 6308 if (this.path == null) 6309 if (Configuration.errorOnAutoCreate()) 6310 throw new Error("Attempt to auto-create ElementDefinition.path"); 6311 else if (Configuration.doAutoCreate()) 6312 this.path = new StringType(); // bb 6313 return this.path; 6314 } 6315 6316 public boolean hasPathElement() { 6317 return this.path != null && !this.path.isEmpty(); 6318 } 6319 6320 public boolean hasPath() { 6321 return this.path != null && !this.path.isEmpty(); 6322 } 6323 6324 /** 6325 * @param value {@link #path} (The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 6326 */ 6327 public ElementDefinition setPathElement(StringType value) { 6328 this.path = value; 6329 return this; 6330 } 6331 6332 /** 6333 * @return The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 6334 */ 6335 public String getPath() { 6336 return this.path == null ? null : this.path.getValue(); 6337 } 6338 6339 /** 6340 * @param value The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 6341 */ 6342 public ElementDefinition setPath(String value) { 6343 if (this.path == null) 6344 this.path = new StringType(); 6345 this.path.setValue(value); 6346 return this; 6347 } 6348 6349 /** 6350 * @return {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6351 */ 6352 public List<Enumeration<PropertyRepresentation>> getRepresentation() { 6353 if (this.representation == null) 6354 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 6355 return this.representation; 6356 } 6357 6358 /** 6359 * @return Returns a reference to <code>this</code> for easy method chaining 6360 */ 6361 public ElementDefinition setRepresentation(List<Enumeration<PropertyRepresentation>> theRepresentation) { 6362 this.representation = theRepresentation; 6363 return this; 6364 } 6365 6366 public boolean hasRepresentation() { 6367 if (this.representation == null) 6368 return false; 6369 for (Enumeration<PropertyRepresentation> item : this.representation) 6370 if (!item.isEmpty()) 6371 return true; 6372 return false; 6373 } 6374 6375 /** 6376 * @return {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6377 */ 6378 public Enumeration<PropertyRepresentation> addRepresentationElement() {//2 6379 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>(new PropertyRepresentationEnumFactory()); 6380 if (this.representation == null) 6381 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 6382 this.representation.add(t); 6383 return t; 6384 } 6385 6386 /** 6387 * @param value {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6388 */ 6389 public ElementDefinition addRepresentation(PropertyRepresentation value) { //1 6390 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>(new PropertyRepresentationEnumFactory()); 6391 t.setValue(value); 6392 if (this.representation == null) 6393 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 6394 this.representation.add(t); 6395 return this; 6396 } 6397 6398 /** 6399 * @param value {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.) 6400 */ 6401 public boolean hasRepresentation(PropertyRepresentation value) { 6402 if (this.representation == null) 6403 return false; 6404 for (Enumeration<PropertyRepresentation> v : this.representation) 6405 if (v.getValue().equals(value)) // code 6406 return true; 6407 return false; 6408 } 6409 6410 /** 6411 * @return {@link #sliceName} (The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 6412 */ 6413 public StringType getSliceNameElement() { 6414 if (this.sliceName == null) 6415 if (Configuration.errorOnAutoCreate()) 6416 throw new Error("Attempt to auto-create ElementDefinition.sliceName"); 6417 else if (Configuration.doAutoCreate()) 6418 this.sliceName = new StringType(); // bb 6419 return this.sliceName; 6420 } 6421 6422 public boolean hasSliceNameElement() { 6423 return this.sliceName != null && !this.sliceName.isEmpty(); 6424 } 6425 6426 public boolean hasSliceName() { 6427 return this.sliceName != null && !this.sliceName.isEmpty(); 6428 } 6429 6430 /** 6431 * @param value {@link #sliceName} (The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 6432 */ 6433 public ElementDefinition setSliceNameElement(StringType value) { 6434 this.sliceName = value; 6435 return this; 6436 } 6437 6438 /** 6439 * @return The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 6440 */ 6441 public String getSliceName() { 6442 return this.sliceName == null ? null : this.sliceName.getValue(); 6443 } 6444 6445 /** 6446 * @param value The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 6447 */ 6448 public ElementDefinition setSliceName(String value) { 6449 if (Utilities.noString(value)) 6450 this.sliceName = null; 6451 else { 6452 if (this.sliceName == null) 6453 this.sliceName = new StringType(); 6454 this.sliceName.setValue(value); 6455 } 6456 return this; 6457 } 6458 6459 /** 6460 * @return {@link #sliceIsConstraining} (If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.). This is the underlying object with id, value and extensions. The accessor "getSliceIsConstraining" gives direct access to the value 6461 */ 6462 public BooleanType getSliceIsConstrainingElement() { 6463 if (this.sliceIsConstraining == null) 6464 if (Configuration.errorOnAutoCreate()) 6465 throw new Error("Attempt to auto-create ElementDefinition.sliceIsConstraining"); 6466 else if (Configuration.doAutoCreate()) 6467 this.sliceIsConstraining = new BooleanType(); // bb 6468 return this.sliceIsConstraining; 6469 } 6470 6471 public boolean hasSliceIsConstrainingElement() { 6472 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 6473 } 6474 6475 public boolean hasSliceIsConstraining() { 6476 return this.sliceIsConstraining != null && !this.sliceIsConstraining.isEmpty(); 6477 } 6478 6479 /** 6480 * @param value {@link #sliceIsConstraining} (If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.). This is the underlying object with id, value and extensions. The accessor "getSliceIsConstraining" gives direct access to the value 6481 */ 6482 public ElementDefinition setSliceIsConstrainingElement(BooleanType value) { 6483 this.sliceIsConstraining = value; 6484 return this; 6485 } 6486 6487 /** 6488 * @return If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName. 6489 */ 6490 public boolean getSliceIsConstraining() { 6491 return this.sliceIsConstraining == null || this.sliceIsConstraining.isEmpty() ? false : this.sliceIsConstraining.getValue(); 6492 } 6493 6494 /** 6495 * @param value If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName. 6496 */ 6497 public ElementDefinition setSliceIsConstraining(boolean value) { 6498 if (this.sliceIsConstraining == null) 6499 this.sliceIsConstraining = new BooleanType(); 6500 this.sliceIsConstraining.setValue(value); 6501 return this; 6502 } 6503 6504 /** 6505 * @return {@link #label} (A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6506 */ 6507 public StringType getLabelElement() { 6508 if (this.label == null) 6509 if (Configuration.errorOnAutoCreate()) 6510 throw new Error("Attempt to auto-create ElementDefinition.label"); 6511 else if (Configuration.doAutoCreate()) 6512 this.label = new StringType(); // bb 6513 return this.label; 6514 } 6515 6516 public boolean hasLabelElement() { 6517 return this.label != null && !this.label.isEmpty(); 6518 } 6519 6520 public boolean hasLabel() { 6521 return this.label != null && !this.label.isEmpty(); 6522 } 6523 6524 /** 6525 * @param value {@link #label} (A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6526 */ 6527 public ElementDefinition setLabelElement(StringType value) { 6528 this.label = value; 6529 return this; 6530 } 6531 6532 /** 6533 * @return A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 6534 */ 6535 public String getLabel() { 6536 return this.label == null ? null : this.label.getValue(); 6537 } 6538 6539 /** 6540 * @param value A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 6541 */ 6542 public ElementDefinition setLabel(String value) { 6543 if (Utilities.noString(value)) 6544 this.label = null; 6545 else { 6546 if (this.label == null) 6547 this.label = new StringType(); 6548 this.label.setValue(value); 6549 } 6550 return this; 6551 } 6552 6553 /** 6554 * @return {@link #code} (A code that has the same meaning as the element in a particular terminology.) 6555 */ 6556 public List<Coding> getCode() { 6557 if (this.code == null) 6558 this.code = new ArrayList<Coding>(); 6559 return this.code; 6560 } 6561 6562 /** 6563 * @return Returns a reference to <code>this</code> for easy method chaining 6564 */ 6565 public ElementDefinition setCode(List<Coding> theCode) { 6566 this.code = theCode; 6567 return this; 6568 } 6569 6570 public boolean hasCode() { 6571 if (this.code == null) 6572 return false; 6573 for (Coding item : this.code) 6574 if (!item.isEmpty()) 6575 return true; 6576 return false; 6577 } 6578 6579 public Coding addCode() { //3 6580 Coding t = new Coding(); 6581 if (this.code == null) 6582 this.code = new ArrayList<Coding>(); 6583 this.code.add(t); 6584 return t; 6585 } 6586 6587 public ElementDefinition addCode(Coding t) { //3 6588 if (t == null) 6589 return this; 6590 if (this.code == null) 6591 this.code = new ArrayList<Coding>(); 6592 this.code.add(t); 6593 return this; 6594 } 6595 6596 /** 6597 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 6598 */ 6599 public Coding getCodeFirstRep() { 6600 if (getCode().isEmpty()) { 6601 addCode(); 6602 } 6603 return getCode().get(0); 6604 } 6605 6606 /** 6607 * @return {@link #slicing} (Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).) 6608 */ 6609 public ElementDefinitionSlicingComponent getSlicing() { 6610 if (this.slicing == null) 6611 if (Configuration.errorOnAutoCreate()) 6612 throw new Error("Attempt to auto-create ElementDefinition.slicing"); 6613 else if (Configuration.doAutoCreate()) 6614 this.slicing = new ElementDefinitionSlicingComponent(); // cc 6615 return this.slicing; 6616 } 6617 6618 public boolean hasSlicing() { 6619 return this.slicing != null && !this.slicing.isEmpty(); 6620 } 6621 6622 /** 6623 * @param value {@link #slicing} (Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).) 6624 */ 6625 public ElementDefinition setSlicing(ElementDefinitionSlicingComponent value) { 6626 this.slicing = value; 6627 return this; 6628 } 6629 6630 /** 6631 * @return {@link #short_} (A concise description of what this element means (e.g. for use in autogenerated summaries).). This is the underlying object with id, value and extensions. The accessor "getShort" gives direct access to the value 6632 */ 6633 public StringType getShortElement() { 6634 if (this.short_ == null) 6635 if (Configuration.errorOnAutoCreate()) 6636 throw new Error("Attempt to auto-create ElementDefinition.short_"); 6637 else if (Configuration.doAutoCreate()) 6638 this.short_ = new StringType(); // bb 6639 return this.short_; 6640 } 6641 6642 public boolean hasShortElement() { 6643 return this.short_ != null && !this.short_.isEmpty(); 6644 } 6645 6646 public boolean hasShort() { 6647 return this.short_ != null && !this.short_.isEmpty(); 6648 } 6649 6650 /** 6651 * @param value {@link #short_} (A concise description of what this element means (e.g. for use in autogenerated summaries).). This is the underlying object with id, value and extensions. The accessor "getShort" gives direct access to the value 6652 */ 6653 public ElementDefinition setShortElement(StringType value) { 6654 this.short_ = value; 6655 return this; 6656 } 6657 6658 /** 6659 * @return A concise description of what this element means (e.g. for use in autogenerated summaries). 6660 */ 6661 public String getShort() { 6662 return this.short_ == null ? null : this.short_.getValue(); 6663 } 6664 6665 /** 6666 * @param value A concise description of what this element means (e.g. for use in autogenerated summaries). 6667 */ 6668 public ElementDefinition setShort(String value) { 6669 if (Utilities.noString(value)) 6670 this.short_ = null; 6671 else { 6672 if (this.short_ == null) 6673 this.short_ = new StringType(); 6674 this.short_.setValue(value); 6675 } 6676 return this; 6677 } 6678 6679 /** 6680 * @return {@link #definition} (Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 6681 */ 6682 public MarkdownType getDefinitionElement() { 6683 if (this.definition == null) 6684 if (Configuration.errorOnAutoCreate()) 6685 throw new Error("Attempt to auto-create ElementDefinition.definition"); 6686 else if (Configuration.doAutoCreate()) 6687 this.definition = new MarkdownType(); // bb 6688 return this.definition; 6689 } 6690 6691 public boolean hasDefinitionElement() { 6692 return this.definition != null && !this.definition.isEmpty(); 6693 } 6694 6695 public boolean hasDefinition() { 6696 return this.definition != null && !this.definition.isEmpty(); 6697 } 6698 6699 /** 6700 * @param value {@link #definition} (Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 6701 */ 6702 public ElementDefinition setDefinitionElement(MarkdownType value) { 6703 this.definition = value; 6704 return this; 6705 } 6706 6707 /** 6708 * @return Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition). 6709 */ 6710 public String getDefinition() { 6711 return this.definition == null ? null : this.definition.getValue(); 6712 } 6713 6714 /** 6715 * @param value Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition). 6716 */ 6717 public ElementDefinition setDefinition(String value) { 6718 if (Utilities.noString(value)) 6719 this.definition = null; 6720 else { 6721 if (this.definition == null) 6722 this.definition = new MarkdownType(); 6723 this.definition.setValue(value); 6724 } 6725 return this; 6726 } 6727 6728 /** 6729 * @return {@link #comment} (Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 6730 */ 6731 public MarkdownType getCommentElement() { 6732 if (this.comment == null) 6733 if (Configuration.errorOnAutoCreate()) 6734 throw new Error("Attempt to auto-create ElementDefinition.comment"); 6735 else if (Configuration.doAutoCreate()) 6736 this.comment = new MarkdownType(); // bb 6737 return this.comment; 6738 } 6739 6740 public boolean hasCommentElement() { 6741 return this.comment != null && !this.comment.isEmpty(); 6742 } 6743 6744 public boolean hasComment() { 6745 return this.comment != null && !this.comment.isEmpty(); 6746 } 6747 6748 /** 6749 * @param value {@link #comment} (Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 6750 */ 6751 public ElementDefinition setCommentElement(MarkdownType value) { 6752 this.comment = value; 6753 return this; 6754 } 6755 6756 /** 6757 * @return Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment). 6758 */ 6759 public String getComment() { 6760 return this.comment == null ? null : this.comment.getValue(); 6761 } 6762 6763 /** 6764 * @param value Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment). 6765 */ 6766 public ElementDefinition setComment(String value) { 6767 if (Utilities.noString(value)) 6768 this.comment = null; 6769 else { 6770 if (this.comment == null) 6771 this.comment = new MarkdownType(); 6772 this.comment.setValue(value); 6773 } 6774 return this; 6775 } 6776 6777 /** 6778 * @return {@link #requirements} (This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 6779 */ 6780 public MarkdownType getRequirementsElement() { 6781 if (this.requirements == null) 6782 if (Configuration.errorOnAutoCreate()) 6783 throw new Error("Attempt to auto-create ElementDefinition.requirements"); 6784 else if (Configuration.doAutoCreate()) 6785 this.requirements = new MarkdownType(); // bb 6786 return this.requirements; 6787 } 6788 6789 public boolean hasRequirementsElement() { 6790 return this.requirements != null && !this.requirements.isEmpty(); 6791 } 6792 6793 public boolean hasRequirements() { 6794 return this.requirements != null && !this.requirements.isEmpty(); 6795 } 6796 6797 /** 6798 * @param value {@link #requirements} (This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 6799 */ 6800 public ElementDefinition setRequirementsElement(MarkdownType value) { 6801 this.requirements = value; 6802 return this; 6803 } 6804 6805 /** 6806 * @return This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 6807 */ 6808 public String getRequirements() { 6809 return this.requirements == null ? null : this.requirements.getValue(); 6810 } 6811 6812 /** 6813 * @param value This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 6814 */ 6815 public ElementDefinition setRequirements(String value) { 6816 if (Utilities.noString(value)) 6817 this.requirements = null; 6818 else { 6819 if (this.requirements == null) 6820 this.requirements = new MarkdownType(); 6821 this.requirements.setValue(value); 6822 } 6823 return this; 6824 } 6825 6826 /** 6827 * @return {@link #alias} (Identifies additional names by which this element might also be known.) 6828 */ 6829 public List<StringType> getAlias() { 6830 if (this.alias == null) 6831 this.alias = new ArrayList<StringType>(); 6832 return this.alias; 6833 } 6834 6835 /** 6836 * @return Returns a reference to <code>this</code> for easy method chaining 6837 */ 6838 public ElementDefinition setAlias(List<StringType> theAlias) { 6839 this.alias = theAlias; 6840 return this; 6841 } 6842 6843 public boolean hasAlias() { 6844 if (this.alias == null) 6845 return false; 6846 for (StringType item : this.alias) 6847 if (!item.isEmpty()) 6848 return true; 6849 return false; 6850 } 6851 6852 /** 6853 * @return {@link #alias} (Identifies additional names by which this element might also be known.) 6854 */ 6855 public StringType addAliasElement() {//2 6856 StringType t = new StringType(); 6857 if (this.alias == null) 6858 this.alias = new ArrayList<StringType>(); 6859 this.alias.add(t); 6860 return t; 6861 } 6862 6863 /** 6864 * @param value {@link #alias} (Identifies additional names by which this element might also be known.) 6865 */ 6866 public ElementDefinition addAlias(String value) { //1 6867 StringType t = new StringType(); 6868 t.setValue(value); 6869 if (this.alias == null) 6870 this.alias = new ArrayList<StringType>(); 6871 this.alias.add(t); 6872 return this; 6873 } 6874 6875 /** 6876 * @param value {@link #alias} (Identifies additional names by which this element might also be known.) 6877 */ 6878 public boolean hasAlias(String value) { 6879 if (this.alias == null) 6880 return false; 6881 for (StringType v : this.alias) 6882 if (v.getValue().equals(value)) // string 6883 return true; 6884 return false; 6885 } 6886 6887 /** 6888 * @return {@link #min} (The minimum number of times this element SHALL appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 6889 */ 6890 public UnsignedIntType getMinElement() { 6891 if (this.min == null) 6892 if (Configuration.errorOnAutoCreate()) 6893 throw new Error("Attempt to auto-create ElementDefinition.min"); 6894 else if (Configuration.doAutoCreate()) 6895 this.min = new UnsignedIntType(); // bb 6896 return this.min; 6897 } 6898 6899 public boolean hasMinElement() { 6900 return this.min != null && !this.min.isEmpty(); 6901 } 6902 6903 public boolean hasMin() { 6904 return this.min != null && !this.min.isEmpty(); 6905 } 6906 6907 /** 6908 * @param value {@link #min} (The minimum number of times this element SHALL appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 6909 */ 6910 public ElementDefinition setMinElement(UnsignedIntType value) { 6911 this.min = value; 6912 return this; 6913 } 6914 6915 /** 6916 * @return The minimum number of times this element SHALL appear in the instance. 6917 */ 6918 public int getMin() { 6919 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 6920 } 6921 6922 /** 6923 * @param value The minimum number of times this element SHALL appear in the instance. 6924 */ 6925 public ElementDefinition setMin(int value) { 6926 if (this.min == null) 6927 this.min = new UnsignedIntType(); 6928 this.min.setValue(value); 6929 return this; 6930 } 6931 6932 /** 6933 * @return {@link #max} (The maximum number of times this element is permitted to appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 6934 */ 6935 public StringType getMaxElement() { 6936 if (this.max == null) 6937 if (Configuration.errorOnAutoCreate()) 6938 throw new Error("Attempt to auto-create ElementDefinition.max"); 6939 else if (Configuration.doAutoCreate()) 6940 this.max = new StringType(); // bb 6941 return this.max; 6942 } 6943 6944 public boolean hasMaxElement() { 6945 return this.max != null && !this.max.isEmpty(); 6946 } 6947 6948 public boolean hasMax() { 6949 return this.max != null && !this.max.isEmpty(); 6950 } 6951 6952 /** 6953 * @param value {@link #max} (The maximum number of times this element is permitted to appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 6954 */ 6955 public ElementDefinition setMaxElement(StringType value) { 6956 this.max = value; 6957 return this; 6958 } 6959 6960 /** 6961 * @return The maximum number of times this element is permitted to appear in the instance. 6962 */ 6963 public String getMax() { 6964 return this.max == null ? null : this.max.getValue(); 6965 } 6966 6967 /** 6968 * @param value The maximum number of times this element is permitted to appear in the instance. 6969 */ 6970 public ElementDefinition setMax(String value) { 6971 if (Utilities.noString(value)) 6972 this.max = null; 6973 else { 6974 if (this.max == null) 6975 this.max = new StringType(); 6976 this.max.setValue(value); 6977 } 6978 return this; 6979 } 6980 6981 /** 6982 * @return {@link #base} (Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.) 6983 */ 6984 public ElementDefinitionBaseComponent getBase() { 6985 if (this.base == null) 6986 if (Configuration.errorOnAutoCreate()) 6987 throw new Error("Attempt to auto-create ElementDefinition.base"); 6988 else if (Configuration.doAutoCreate()) 6989 this.base = new ElementDefinitionBaseComponent(); // cc 6990 return this.base; 6991 } 6992 6993 public boolean hasBase() { 6994 return this.base != null && !this.base.isEmpty(); 6995 } 6996 6997 /** 6998 * @param value {@link #base} (Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.) 6999 */ 7000 public ElementDefinition setBase(ElementDefinitionBaseComponent value) { 7001 this.base = value; 7002 return this; 7003 } 7004 7005 /** 7006 * @return {@link #contentReference} (Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.). This is the underlying object with id, value and extensions. The accessor "getContentReference" gives direct access to the value 7007 */ 7008 public UriType getContentReferenceElement() { 7009 if (this.contentReference == null) 7010 if (Configuration.errorOnAutoCreate()) 7011 throw new Error("Attempt to auto-create ElementDefinition.contentReference"); 7012 else if (Configuration.doAutoCreate()) 7013 this.contentReference = new UriType(); // bb 7014 return this.contentReference; 7015 } 7016 7017 public boolean hasContentReferenceElement() { 7018 return this.contentReference != null && !this.contentReference.isEmpty(); 7019 } 7020 7021 public boolean hasContentReference() { 7022 return this.contentReference != null && !this.contentReference.isEmpty(); 7023 } 7024 7025 /** 7026 * @param value {@link #contentReference} (Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.). This is the underlying object with id, value and extensions. The accessor "getContentReference" gives direct access to the value 7027 */ 7028 public ElementDefinition setContentReferenceElement(UriType value) { 7029 this.contentReference = value; 7030 return this; 7031 } 7032 7033 /** 7034 * @return Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc. 7035 */ 7036 public String getContentReference() { 7037 return this.contentReference == null ? null : this.contentReference.getValue(); 7038 } 7039 7040 /** 7041 * @param value Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc. 7042 */ 7043 public ElementDefinition setContentReference(String value) { 7044 if (Utilities.noString(value)) 7045 this.contentReference = null; 7046 else { 7047 if (this.contentReference == null) 7048 this.contentReference = new UriType(); 7049 this.contentReference.setValue(value); 7050 } 7051 return this; 7052 } 7053 7054 /** 7055 * @return {@link #type} (The data type or resource that the value of this element is permitted to be.) 7056 */ 7057 public List<TypeRefComponent> getType() { 7058 if (this.type == null) 7059 this.type = new ArrayList<TypeRefComponent>(); 7060 return this.type; 7061 } 7062 7063 /** 7064 * @return Returns a reference to <code>this</code> for easy method chaining 7065 */ 7066 public ElementDefinition setType(List<TypeRefComponent> theType) { 7067 this.type = theType; 7068 return this; 7069 } 7070 7071 public boolean hasType() { 7072 if (this.type == null) 7073 return false; 7074 for (TypeRefComponent item : this.type) 7075 if (!item.isEmpty()) 7076 return true; 7077 return false; 7078 } 7079 7080 public TypeRefComponent addType() { //3 7081 TypeRefComponent t = new TypeRefComponent(); 7082 if (this.type == null) 7083 this.type = new ArrayList<TypeRefComponent>(); 7084 this.type.add(t); 7085 return t; 7086 } 7087 7088 public ElementDefinition addType(TypeRefComponent t) { //3 7089 if (t == null) 7090 return this; 7091 if (this.type == null) 7092 this.type = new ArrayList<TypeRefComponent>(); 7093 this.type.add(t); 7094 return this; 7095 } 7096 7097 /** 7098 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 7099 */ 7100 public TypeRefComponent getTypeFirstRep() { 7101 if (getType().isEmpty()) { 7102 addType(); 7103 } 7104 return getType().get(0); 7105 } 7106 7107 /** 7108 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7109 */ 7110 public DataType getDefaultValue() { 7111 return this.defaultValue; 7112 } 7113 7114 /** 7115 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7116 */ 7117 public Base64BinaryType getDefaultValueBase64BinaryType() throws FHIRException { 7118 if (this.defaultValue == null) 7119 this.defaultValue = new Base64BinaryType(); 7120 if (!(this.defaultValue instanceof Base64BinaryType)) 7121 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7122 return (Base64BinaryType) this.defaultValue; 7123 } 7124 7125 public boolean hasDefaultValueBase64BinaryType() { 7126 return this != null && this.defaultValue instanceof Base64BinaryType; 7127 } 7128 7129 /** 7130 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7131 */ 7132 public BooleanType getDefaultValueBooleanType() throws FHIRException { 7133 if (this.defaultValue == null) 7134 this.defaultValue = new BooleanType(); 7135 if (!(this.defaultValue instanceof BooleanType)) 7136 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7137 return (BooleanType) this.defaultValue; 7138 } 7139 7140 public boolean hasDefaultValueBooleanType() { 7141 return this != null && this.defaultValue instanceof BooleanType; 7142 } 7143 7144 /** 7145 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7146 */ 7147 public CanonicalType getDefaultValueCanonicalType() throws FHIRException { 7148 if (this.defaultValue == null) 7149 this.defaultValue = new CanonicalType(); 7150 if (!(this.defaultValue instanceof CanonicalType)) 7151 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7152 return (CanonicalType) this.defaultValue; 7153 } 7154 7155 public boolean hasDefaultValueCanonicalType() { 7156 return this != null && this.defaultValue instanceof CanonicalType; 7157 } 7158 7159 /** 7160 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7161 */ 7162 public CodeType getDefaultValueCodeType() throws FHIRException { 7163 if (this.defaultValue == null) 7164 this.defaultValue = new CodeType(); 7165 if (!(this.defaultValue instanceof CodeType)) 7166 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7167 return (CodeType) this.defaultValue; 7168 } 7169 7170 public boolean hasDefaultValueCodeType() { 7171 return this != null && this.defaultValue instanceof CodeType; 7172 } 7173 7174 /** 7175 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7176 */ 7177 public DateType getDefaultValueDateType() throws FHIRException { 7178 if (this.defaultValue == null) 7179 this.defaultValue = new DateType(); 7180 if (!(this.defaultValue instanceof DateType)) 7181 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7182 return (DateType) this.defaultValue; 7183 } 7184 7185 public boolean hasDefaultValueDateType() { 7186 return this != null && this.defaultValue instanceof DateType; 7187 } 7188 7189 /** 7190 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7191 */ 7192 public DateTimeType getDefaultValueDateTimeType() throws FHIRException { 7193 if (this.defaultValue == null) 7194 this.defaultValue = new DateTimeType(); 7195 if (!(this.defaultValue instanceof DateTimeType)) 7196 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7197 return (DateTimeType) this.defaultValue; 7198 } 7199 7200 public boolean hasDefaultValueDateTimeType() { 7201 return this != null && this.defaultValue instanceof DateTimeType; 7202 } 7203 7204 /** 7205 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7206 */ 7207 public DecimalType getDefaultValueDecimalType() throws FHIRException { 7208 if (this.defaultValue == null) 7209 this.defaultValue = new DecimalType(); 7210 if (!(this.defaultValue instanceof DecimalType)) 7211 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7212 return (DecimalType) this.defaultValue; 7213 } 7214 7215 public boolean hasDefaultValueDecimalType() { 7216 return this != null && this.defaultValue instanceof DecimalType; 7217 } 7218 7219 /** 7220 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7221 */ 7222 public IdType getDefaultValueIdType() throws FHIRException { 7223 if (this.defaultValue == null) 7224 this.defaultValue = new IdType(); 7225 if (!(this.defaultValue instanceof IdType)) 7226 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7227 return (IdType) this.defaultValue; 7228 } 7229 7230 public boolean hasDefaultValueIdType() { 7231 return this != null && this.defaultValue instanceof IdType; 7232 } 7233 7234 /** 7235 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7236 */ 7237 public InstantType getDefaultValueInstantType() throws FHIRException { 7238 if (this.defaultValue == null) 7239 this.defaultValue = new InstantType(); 7240 if (!(this.defaultValue instanceof InstantType)) 7241 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7242 return (InstantType) this.defaultValue; 7243 } 7244 7245 public boolean hasDefaultValueInstantType() { 7246 return this != null && this.defaultValue instanceof InstantType; 7247 } 7248 7249 /** 7250 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7251 */ 7252 public IntegerType getDefaultValueIntegerType() throws FHIRException { 7253 if (this.defaultValue == null) 7254 this.defaultValue = new IntegerType(); 7255 if (!(this.defaultValue instanceof IntegerType)) 7256 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7257 return (IntegerType) this.defaultValue; 7258 } 7259 7260 public boolean hasDefaultValueIntegerType() { 7261 return this != null && this.defaultValue instanceof IntegerType; 7262 } 7263 7264 /** 7265 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7266 */ 7267 public Integer64Type getDefaultValueInteger64Type() throws FHIRException { 7268 if (this.defaultValue == null) 7269 this.defaultValue = new Integer64Type(); 7270 if (!(this.defaultValue instanceof Integer64Type)) 7271 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7272 return (Integer64Type) this.defaultValue; 7273 } 7274 7275 public boolean hasDefaultValueInteger64Type() { 7276 return this != null && this.defaultValue instanceof Integer64Type; 7277 } 7278 7279 /** 7280 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7281 */ 7282 public MarkdownType getDefaultValueMarkdownType() throws FHIRException { 7283 if (this.defaultValue == null) 7284 this.defaultValue = new MarkdownType(); 7285 if (!(this.defaultValue instanceof MarkdownType)) 7286 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7287 return (MarkdownType) this.defaultValue; 7288 } 7289 7290 public boolean hasDefaultValueMarkdownType() { 7291 return this != null && this.defaultValue instanceof MarkdownType; 7292 } 7293 7294 /** 7295 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7296 */ 7297 public OidType getDefaultValueOidType() throws FHIRException { 7298 if (this.defaultValue == null) 7299 this.defaultValue = new OidType(); 7300 if (!(this.defaultValue instanceof OidType)) 7301 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7302 return (OidType) this.defaultValue; 7303 } 7304 7305 public boolean hasDefaultValueOidType() { 7306 return this != null && this.defaultValue instanceof OidType; 7307 } 7308 7309 /** 7310 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7311 */ 7312 public PositiveIntType getDefaultValuePositiveIntType() throws FHIRException { 7313 if (this.defaultValue == null) 7314 this.defaultValue = new PositiveIntType(); 7315 if (!(this.defaultValue instanceof PositiveIntType)) 7316 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7317 return (PositiveIntType) this.defaultValue; 7318 } 7319 7320 public boolean hasDefaultValuePositiveIntType() { 7321 return this != null && this.defaultValue instanceof PositiveIntType; 7322 } 7323 7324 /** 7325 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7326 */ 7327 public StringType getDefaultValueStringType() throws FHIRException { 7328 if (this.defaultValue == null) 7329 this.defaultValue = new StringType(); 7330 if (!(this.defaultValue instanceof StringType)) 7331 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7332 return (StringType) this.defaultValue; 7333 } 7334 7335 public boolean hasDefaultValueStringType() { 7336 return this != null && this.defaultValue instanceof StringType; 7337 } 7338 7339 /** 7340 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7341 */ 7342 public TimeType getDefaultValueTimeType() throws FHIRException { 7343 if (this.defaultValue == null) 7344 this.defaultValue = new TimeType(); 7345 if (!(this.defaultValue instanceof TimeType)) 7346 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7347 return (TimeType) this.defaultValue; 7348 } 7349 7350 public boolean hasDefaultValueTimeType() { 7351 return this != null && this.defaultValue instanceof TimeType; 7352 } 7353 7354 /** 7355 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7356 */ 7357 public UnsignedIntType getDefaultValueUnsignedIntType() throws FHIRException { 7358 if (this.defaultValue == null) 7359 this.defaultValue = new UnsignedIntType(); 7360 if (!(this.defaultValue instanceof UnsignedIntType)) 7361 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7362 return (UnsignedIntType) this.defaultValue; 7363 } 7364 7365 public boolean hasDefaultValueUnsignedIntType() { 7366 return this != null && this.defaultValue instanceof UnsignedIntType; 7367 } 7368 7369 /** 7370 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7371 */ 7372 public UriType getDefaultValueUriType() throws FHIRException { 7373 if (this.defaultValue == null) 7374 this.defaultValue = new UriType(); 7375 if (!(this.defaultValue instanceof UriType)) 7376 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7377 return (UriType) this.defaultValue; 7378 } 7379 7380 public boolean hasDefaultValueUriType() { 7381 return this != null && this.defaultValue instanceof UriType; 7382 } 7383 7384 /** 7385 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7386 */ 7387 public UrlType getDefaultValueUrlType() throws FHIRException { 7388 if (this.defaultValue == null) 7389 this.defaultValue = new UrlType(); 7390 if (!(this.defaultValue instanceof UrlType)) 7391 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7392 return (UrlType) this.defaultValue; 7393 } 7394 7395 public boolean hasDefaultValueUrlType() { 7396 return this != null && this.defaultValue instanceof UrlType; 7397 } 7398 7399 /** 7400 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7401 */ 7402 public UuidType getDefaultValueUuidType() throws FHIRException { 7403 if (this.defaultValue == null) 7404 this.defaultValue = new UuidType(); 7405 if (!(this.defaultValue instanceof UuidType)) 7406 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7407 return (UuidType) this.defaultValue; 7408 } 7409 7410 public boolean hasDefaultValueUuidType() { 7411 return this != null && this.defaultValue instanceof UuidType; 7412 } 7413 7414 /** 7415 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7416 */ 7417 public Address getDefaultValueAddress() throws FHIRException { 7418 if (this.defaultValue == null) 7419 this.defaultValue = new Address(); 7420 if (!(this.defaultValue instanceof Address)) 7421 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7422 return (Address) this.defaultValue; 7423 } 7424 7425 public boolean hasDefaultValueAddress() { 7426 return this != null && this.defaultValue instanceof Address; 7427 } 7428 7429 /** 7430 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7431 */ 7432 public Age getDefaultValueAge() throws FHIRException { 7433 if (this.defaultValue == null) 7434 this.defaultValue = new Age(); 7435 if (!(this.defaultValue instanceof Age)) 7436 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7437 return (Age) this.defaultValue; 7438 } 7439 7440 public boolean hasDefaultValueAge() { 7441 return this != null && this.defaultValue instanceof Age; 7442 } 7443 7444 /** 7445 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7446 */ 7447 public Annotation getDefaultValueAnnotation() throws FHIRException { 7448 if (this.defaultValue == null) 7449 this.defaultValue = new Annotation(); 7450 if (!(this.defaultValue instanceof Annotation)) 7451 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7452 return (Annotation) this.defaultValue; 7453 } 7454 7455 public boolean hasDefaultValueAnnotation() { 7456 return this != null && this.defaultValue instanceof Annotation; 7457 } 7458 7459 /** 7460 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7461 */ 7462 public Attachment getDefaultValueAttachment() throws FHIRException { 7463 if (this.defaultValue == null) 7464 this.defaultValue = new Attachment(); 7465 if (!(this.defaultValue instanceof Attachment)) 7466 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7467 return (Attachment) this.defaultValue; 7468 } 7469 7470 public boolean hasDefaultValueAttachment() { 7471 return this != null && this.defaultValue instanceof Attachment; 7472 } 7473 7474 /** 7475 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7476 */ 7477 public CodeableConcept getDefaultValueCodeableConcept() throws FHIRException { 7478 if (this.defaultValue == null) 7479 this.defaultValue = new CodeableConcept(); 7480 if (!(this.defaultValue instanceof CodeableConcept)) 7481 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7482 return (CodeableConcept) this.defaultValue; 7483 } 7484 7485 public boolean hasDefaultValueCodeableConcept() { 7486 return this != null && this.defaultValue instanceof CodeableConcept; 7487 } 7488 7489 /** 7490 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7491 */ 7492 public CodeableReference getDefaultValueCodeableReference() throws FHIRException { 7493 if (this.defaultValue == null) 7494 this.defaultValue = new CodeableReference(); 7495 if (!(this.defaultValue instanceof CodeableReference)) 7496 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7497 return (CodeableReference) this.defaultValue; 7498 } 7499 7500 public boolean hasDefaultValueCodeableReference() { 7501 return this != null && this.defaultValue instanceof CodeableReference; 7502 } 7503 7504 /** 7505 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7506 */ 7507 public Coding getDefaultValueCoding() throws FHIRException { 7508 if (this.defaultValue == null) 7509 this.defaultValue = new Coding(); 7510 if (!(this.defaultValue instanceof Coding)) 7511 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7512 return (Coding) this.defaultValue; 7513 } 7514 7515 public boolean hasDefaultValueCoding() { 7516 return this != null && this.defaultValue instanceof Coding; 7517 } 7518 7519 /** 7520 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7521 */ 7522 public ContactPoint getDefaultValueContactPoint() throws FHIRException { 7523 if (this.defaultValue == null) 7524 this.defaultValue = new ContactPoint(); 7525 if (!(this.defaultValue instanceof ContactPoint)) 7526 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7527 return (ContactPoint) this.defaultValue; 7528 } 7529 7530 public boolean hasDefaultValueContactPoint() { 7531 return this != null && this.defaultValue instanceof ContactPoint; 7532 } 7533 7534 /** 7535 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7536 */ 7537 public Count getDefaultValueCount() throws FHIRException { 7538 if (this.defaultValue == null) 7539 this.defaultValue = new Count(); 7540 if (!(this.defaultValue instanceof Count)) 7541 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7542 return (Count) this.defaultValue; 7543 } 7544 7545 public boolean hasDefaultValueCount() { 7546 return this != null && this.defaultValue instanceof Count; 7547 } 7548 7549 /** 7550 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7551 */ 7552 public Distance getDefaultValueDistance() throws FHIRException { 7553 if (this.defaultValue == null) 7554 this.defaultValue = new Distance(); 7555 if (!(this.defaultValue instanceof Distance)) 7556 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7557 return (Distance) this.defaultValue; 7558 } 7559 7560 public boolean hasDefaultValueDistance() { 7561 return this != null && this.defaultValue instanceof Distance; 7562 } 7563 7564 /** 7565 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7566 */ 7567 public Duration getDefaultValueDuration() throws FHIRException { 7568 if (this.defaultValue == null) 7569 this.defaultValue = new Duration(); 7570 if (!(this.defaultValue instanceof Duration)) 7571 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7572 return (Duration) this.defaultValue; 7573 } 7574 7575 public boolean hasDefaultValueDuration() { 7576 return this != null && this.defaultValue instanceof Duration; 7577 } 7578 7579 /** 7580 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7581 */ 7582 public HumanName getDefaultValueHumanName() throws FHIRException { 7583 if (this.defaultValue == null) 7584 this.defaultValue = new HumanName(); 7585 if (!(this.defaultValue instanceof HumanName)) 7586 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7587 return (HumanName) this.defaultValue; 7588 } 7589 7590 public boolean hasDefaultValueHumanName() { 7591 return this != null && this.defaultValue instanceof HumanName; 7592 } 7593 7594 /** 7595 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7596 */ 7597 public Identifier getDefaultValueIdentifier() throws FHIRException { 7598 if (this.defaultValue == null) 7599 this.defaultValue = new Identifier(); 7600 if (!(this.defaultValue instanceof Identifier)) 7601 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7602 return (Identifier) this.defaultValue; 7603 } 7604 7605 public boolean hasDefaultValueIdentifier() { 7606 return this != null && this.defaultValue instanceof Identifier; 7607 } 7608 7609 /** 7610 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7611 */ 7612 public Money getDefaultValueMoney() throws FHIRException { 7613 if (this.defaultValue == null) 7614 this.defaultValue = new Money(); 7615 if (!(this.defaultValue instanceof Money)) 7616 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7617 return (Money) this.defaultValue; 7618 } 7619 7620 public boolean hasDefaultValueMoney() { 7621 return this != null && this.defaultValue instanceof Money; 7622 } 7623 7624 /** 7625 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7626 */ 7627 public Period getDefaultValuePeriod() throws FHIRException { 7628 if (this.defaultValue == null) 7629 this.defaultValue = new Period(); 7630 if (!(this.defaultValue instanceof Period)) 7631 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7632 return (Period) this.defaultValue; 7633 } 7634 7635 public boolean hasDefaultValuePeriod() { 7636 return this != null && this.defaultValue instanceof Period; 7637 } 7638 7639 /** 7640 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7641 */ 7642 public Quantity getDefaultValueQuantity() throws FHIRException { 7643 if (this.defaultValue == null) 7644 this.defaultValue = new Quantity(); 7645 if (!(this.defaultValue instanceof Quantity)) 7646 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7647 return (Quantity) this.defaultValue; 7648 } 7649 7650 public boolean hasDefaultValueQuantity() { 7651 return this != null && this.defaultValue instanceof Quantity; 7652 } 7653 7654 /** 7655 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7656 */ 7657 public Range getDefaultValueRange() throws FHIRException { 7658 if (this.defaultValue == null) 7659 this.defaultValue = new Range(); 7660 if (!(this.defaultValue instanceof Range)) 7661 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7662 return (Range) this.defaultValue; 7663 } 7664 7665 public boolean hasDefaultValueRange() { 7666 return this != null && this.defaultValue instanceof Range; 7667 } 7668 7669 /** 7670 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7671 */ 7672 public Ratio getDefaultValueRatio() throws FHIRException { 7673 if (this.defaultValue == null) 7674 this.defaultValue = new Ratio(); 7675 if (!(this.defaultValue instanceof Ratio)) 7676 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7677 return (Ratio) this.defaultValue; 7678 } 7679 7680 public boolean hasDefaultValueRatio() { 7681 return this != null && this.defaultValue instanceof Ratio; 7682 } 7683 7684 /** 7685 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7686 */ 7687 public RatioRange getDefaultValueRatioRange() throws FHIRException { 7688 if (this.defaultValue == null) 7689 this.defaultValue = new RatioRange(); 7690 if (!(this.defaultValue instanceof RatioRange)) 7691 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7692 return (RatioRange) this.defaultValue; 7693 } 7694 7695 public boolean hasDefaultValueRatioRange() { 7696 return this != null && this.defaultValue instanceof RatioRange; 7697 } 7698 7699 /** 7700 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7701 */ 7702 public Reference getDefaultValueReference() throws FHIRException { 7703 if (this.defaultValue == null) 7704 this.defaultValue = new Reference(); 7705 if (!(this.defaultValue instanceof Reference)) 7706 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7707 return (Reference) this.defaultValue; 7708 } 7709 7710 public boolean hasDefaultValueReference() { 7711 return this != null && this.defaultValue instanceof Reference; 7712 } 7713 7714 /** 7715 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7716 */ 7717 public SampledData getDefaultValueSampledData() throws FHIRException { 7718 if (this.defaultValue == null) 7719 this.defaultValue = new SampledData(); 7720 if (!(this.defaultValue instanceof SampledData)) 7721 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7722 return (SampledData) this.defaultValue; 7723 } 7724 7725 public boolean hasDefaultValueSampledData() { 7726 return this != null && this.defaultValue instanceof SampledData; 7727 } 7728 7729 /** 7730 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7731 */ 7732 public Signature getDefaultValueSignature() throws FHIRException { 7733 if (this.defaultValue == null) 7734 this.defaultValue = new Signature(); 7735 if (!(this.defaultValue instanceof Signature)) 7736 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7737 return (Signature) this.defaultValue; 7738 } 7739 7740 public boolean hasDefaultValueSignature() { 7741 return this != null && this.defaultValue instanceof Signature; 7742 } 7743 7744 /** 7745 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7746 */ 7747 public Timing getDefaultValueTiming() throws FHIRException { 7748 if (this.defaultValue == null) 7749 this.defaultValue = new Timing(); 7750 if (!(this.defaultValue instanceof Timing)) 7751 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7752 return (Timing) this.defaultValue; 7753 } 7754 7755 public boolean hasDefaultValueTiming() { 7756 return this != null && this.defaultValue instanceof Timing; 7757 } 7758 7759 /** 7760 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7761 */ 7762 public ContactDetail getDefaultValueContactDetail() throws FHIRException { 7763 if (this.defaultValue == null) 7764 this.defaultValue = new ContactDetail(); 7765 if (!(this.defaultValue instanceof ContactDetail)) 7766 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7767 return (ContactDetail) this.defaultValue; 7768 } 7769 7770 public boolean hasDefaultValueContactDetail() { 7771 return this != null && this.defaultValue instanceof ContactDetail; 7772 } 7773 7774 /** 7775 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7776 */ 7777 public DataRequirement getDefaultValueDataRequirement() throws FHIRException { 7778 if (this.defaultValue == null) 7779 this.defaultValue = new DataRequirement(); 7780 if (!(this.defaultValue instanceof DataRequirement)) 7781 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7782 return (DataRequirement) this.defaultValue; 7783 } 7784 7785 public boolean hasDefaultValueDataRequirement() { 7786 return this != null && this.defaultValue instanceof DataRequirement; 7787 } 7788 7789 /** 7790 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7791 */ 7792 public Expression getDefaultValueExpression() throws FHIRException { 7793 if (this.defaultValue == null) 7794 this.defaultValue = new Expression(); 7795 if (!(this.defaultValue instanceof Expression)) 7796 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7797 return (Expression) this.defaultValue; 7798 } 7799 7800 public boolean hasDefaultValueExpression() { 7801 return this != null && this.defaultValue instanceof Expression; 7802 } 7803 7804 /** 7805 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7806 */ 7807 public ParameterDefinition getDefaultValueParameterDefinition() throws FHIRException { 7808 if (this.defaultValue == null) 7809 this.defaultValue = new ParameterDefinition(); 7810 if (!(this.defaultValue instanceof ParameterDefinition)) 7811 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7812 return (ParameterDefinition) this.defaultValue; 7813 } 7814 7815 public boolean hasDefaultValueParameterDefinition() { 7816 return this != null && this.defaultValue instanceof ParameterDefinition; 7817 } 7818 7819 /** 7820 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7821 */ 7822 public RelatedArtifact getDefaultValueRelatedArtifact() throws FHIRException { 7823 if (this.defaultValue == null) 7824 this.defaultValue = new RelatedArtifact(); 7825 if (!(this.defaultValue instanceof RelatedArtifact)) 7826 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7827 return (RelatedArtifact) this.defaultValue; 7828 } 7829 7830 public boolean hasDefaultValueRelatedArtifact() { 7831 return this != null && this.defaultValue instanceof RelatedArtifact; 7832 } 7833 7834 /** 7835 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7836 */ 7837 public TriggerDefinition getDefaultValueTriggerDefinition() throws FHIRException { 7838 if (this.defaultValue == null) 7839 this.defaultValue = new TriggerDefinition(); 7840 if (!(this.defaultValue instanceof TriggerDefinition)) 7841 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7842 return (TriggerDefinition) this.defaultValue; 7843 } 7844 7845 public boolean hasDefaultValueTriggerDefinition() { 7846 return this != null && this.defaultValue instanceof TriggerDefinition; 7847 } 7848 7849 /** 7850 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7851 */ 7852 public UsageContext getDefaultValueUsageContext() throws FHIRException { 7853 if (this.defaultValue == null) 7854 this.defaultValue = new UsageContext(); 7855 if (!(this.defaultValue instanceof UsageContext)) 7856 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7857 return (UsageContext) this.defaultValue; 7858 } 7859 7860 public boolean hasDefaultValueUsageContext() { 7861 return this != null && this.defaultValue instanceof UsageContext; 7862 } 7863 7864 /** 7865 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7866 */ 7867 public Availability getDefaultValueAvailability() throws FHIRException { 7868 if (this.defaultValue == null) 7869 this.defaultValue = new Availability(); 7870 if (!(this.defaultValue instanceof Availability)) 7871 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7872 return (Availability) this.defaultValue; 7873 } 7874 7875 public boolean hasDefaultValueAvailability() { 7876 return this != null && this.defaultValue instanceof Availability; 7877 } 7878 7879 /** 7880 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7881 */ 7882 public ExtendedContactDetail getDefaultValueExtendedContactDetail() throws FHIRException { 7883 if (this.defaultValue == null) 7884 this.defaultValue = new ExtendedContactDetail(); 7885 if (!(this.defaultValue instanceof ExtendedContactDetail)) 7886 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7887 return (ExtendedContactDetail) this.defaultValue; 7888 } 7889 7890 public boolean hasDefaultValueExtendedContactDetail() { 7891 return this != null && this.defaultValue instanceof ExtendedContactDetail; 7892 } 7893 7894 /** 7895 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7896 */ 7897 public Dosage getDefaultValueDosage() throws FHIRException { 7898 if (this.defaultValue == null) 7899 this.defaultValue = new Dosage(); 7900 if (!(this.defaultValue instanceof Dosage)) 7901 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7902 return (Dosage) this.defaultValue; 7903 } 7904 7905 public boolean hasDefaultValueDosage() { 7906 return this != null && this.defaultValue instanceof Dosage; 7907 } 7908 7909 /** 7910 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7911 */ 7912 public Meta getDefaultValueMeta() throws FHIRException { 7913 if (this.defaultValue == null) 7914 this.defaultValue = new Meta(); 7915 if (!(this.defaultValue instanceof Meta)) 7916 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.defaultValue.getClass().getName()+" was encountered"); 7917 return (Meta) this.defaultValue; 7918 } 7919 7920 public boolean hasDefaultValueMeta() { 7921 return this != null && this.defaultValue instanceof Meta; 7922 } 7923 7924 public boolean hasDefaultValue() { 7925 return this.defaultValue != null && !this.defaultValue.isEmpty(); 7926 } 7927 7928 /** 7929 * @param value {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 7930 */ 7931 public ElementDefinition setDefaultValue(DataType value) { 7932 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 7933 throw new FHIRException("Not the right type for ElementDefinition.defaultValue[x]: "+value.fhirType()); 7934 this.defaultValue = value; 7935 return this; 7936 } 7937 7938 /** 7939 * @return {@link #meaningWhenMissing} (The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').). This is the underlying object with id, value and extensions. The accessor "getMeaningWhenMissing" gives direct access to the value 7940 */ 7941 public MarkdownType getMeaningWhenMissingElement() { 7942 if (this.meaningWhenMissing == null) 7943 if (Configuration.errorOnAutoCreate()) 7944 throw new Error("Attempt to auto-create ElementDefinition.meaningWhenMissing"); 7945 else if (Configuration.doAutoCreate()) 7946 this.meaningWhenMissing = new MarkdownType(); // bb 7947 return this.meaningWhenMissing; 7948 } 7949 7950 public boolean hasMeaningWhenMissingElement() { 7951 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 7952 } 7953 7954 public boolean hasMeaningWhenMissing() { 7955 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 7956 } 7957 7958 /** 7959 * @param value {@link #meaningWhenMissing} (The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').). This is the underlying object with id, value and extensions. The accessor "getMeaningWhenMissing" gives direct access to the value 7960 */ 7961 public ElementDefinition setMeaningWhenMissingElement(MarkdownType value) { 7962 this.meaningWhenMissing = value; 7963 return this; 7964 } 7965 7966 /** 7967 * @return The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'). 7968 */ 7969 public String getMeaningWhenMissing() { 7970 return this.meaningWhenMissing == null ? null : this.meaningWhenMissing.getValue(); 7971 } 7972 7973 /** 7974 * @param value The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'). 7975 */ 7976 public ElementDefinition setMeaningWhenMissing(String value) { 7977 if (Utilities.noString(value)) 7978 this.meaningWhenMissing = null; 7979 else { 7980 if (this.meaningWhenMissing == null) 7981 this.meaningWhenMissing = new MarkdownType(); 7982 this.meaningWhenMissing.setValue(value); 7983 } 7984 return this; 7985 } 7986 7987 /** 7988 * @return {@link #orderMeaning} (If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.). This is the underlying object with id, value and extensions. The accessor "getOrderMeaning" gives direct access to the value 7989 */ 7990 public StringType getOrderMeaningElement() { 7991 if (this.orderMeaning == null) 7992 if (Configuration.errorOnAutoCreate()) 7993 throw new Error("Attempt to auto-create ElementDefinition.orderMeaning"); 7994 else if (Configuration.doAutoCreate()) 7995 this.orderMeaning = new StringType(); // bb 7996 return this.orderMeaning; 7997 } 7998 7999 public boolean hasOrderMeaningElement() { 8000 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 8001 } 8002 8003 public boolean hasOrderMeaning() { 8004 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 8005 } 8006 8007 /** 8008 * @param value {@link #orderMeaning} (If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.). This is the underlying object with id, value and extensions. The accessor "getOrderMeaning" gives direct access to the value 8009 */ 8010 public ElementDefinition setOrderMeaningElement(StringType value) { 8011 this.orderMeaning = value; 8012 return this; 8013 } 8014 8015 /** 8016 * @return If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 8017 */ 8018 public String getOrderMeaning() { 8019 return this.orderMeaning == null ? null : this.orderMeaning.getValue(); 8020 } 8021 8022 /** 8023 * @param value If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 8024 */ 8025 public ElementDefinition setOrderMeaning(String value) { 8026 if (Utilities.noString(value)) 8027 this.orderMeaning = null; 8028 else { 8029 if (this.orderMeaning == null) 8030 this.orderMeaning = new StringType(); 8031 this.orderMeaning.setValue(value); 8032 } 8033 return this; 8034 } 8035 8036 /** 8037 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8038 */ 8039 public DataType getFixed() { 8040 return this.fixed; 8041 } 8042 8043 /** 8044 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8045 */ 8046 public Base64BinaryType getFixedBase64BinaryType() throws FHIRException { 8047 if (this.fixed == null) 8048 this.fixed = new Base64BinaryType(); 8049 if (!(this.fixed instanceof Base64BinaryType)) 8050 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8051 return (Base64BinaryType) this.fixed; 8052 } 8053 8054 public boolean hasFixedBase64BinaryType() { 8055 return this != null && this.fixed instanceof Base64BinaryType; 8056 } 8057 8058 /** 8059 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8060 */ 8061 public BooleanType getFixedBooleanType() throws FHIRException { 8062 if (this.fixed == null) 8063 this.fixed = new BooleanType(); 8064 if (!(this.fixed instanceof BooleanType)) 8065 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8066 return (BooleanType) this.fixed; 8067 } 8068 8069 public boolean hasFixedBooleanType() { 8070 return this != null && this.fixed instanceof BooleanType; 8071 } 8072 8073 /** 8074 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8075 */ 8076 public CanonicalType getFixedCanonicalType() throws FHIRException { 8077 if (this.fixed == null) 8078 this.fixed = new CanonicalType(); 8079 if (!(this.fixed instanceof CanonicalType)) 8080 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8081 return (CanonicalType) this.fixed; 8082 } 8083 8084 public boolean hasFixedCanonicalType() { 8085 return this != null && this.fixed instanceof CanonicalType; 8086 } 8087 8088 /** 8089 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8090 */ 8091 public CodeType getFixedCodeType() throws FHIRException { 8092 if (this.fixed == null) 8093 this.fixed = new CodeType(); 8094 if (!(this.fixed instanceof CodeType)) 8095 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8096 return (CodeType) this.fixed; 8097 } 8098 8099 public boolean hasFixedCodeType() { 8100 return this != null && this.fixed instanceof CodeType; 8101 } 8102 8103 /** 8104 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8105 */ 8106 public DateType getFixedDateType() throws FHIRException { 8107 if (this.fixed == null) 8108 this.fixed = new DateType(); 8109 if (!(this.fixed instanceof DateType)) 8110 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8111 return (DateType) this.fixed; 8112 } 8113 8114 public boolean hasFixedDateType() { 8115 return this != null && this.fixed instanceof DateType; 8116 } 8117 8118 /** 8119 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8120 */ 8121 public DateTimeType getFixedDateTimeType() throws FHIRException { 8122 if (this.fixed == null) 8123 this.fixed = new DateTimeType(); 8124 if (!(this.fixed instanceof DateTimeType)) 8125 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8126 return (DateTimeType) this.fixed; 8127 } 8128 8129 public boolean hasFixedDateTimeType() { 8130 return this != null && this.fixed instanceof DateTimeType; 8131 } 8132 8133 /** 8134 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8135 */ 8136 public DecimalType getFixedDecimalType() throws FHIRException { 8137 if (this.fixed == null) 8138 this.fixed = new DecimalType(); 8139 if (!(this.fixed instanceof DecimalType)) 8140 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8141 return (DecimalType) this.fixed; 8142 } 8143 8144 public boolean hasFixedDecimalType() { 8145 return this != null && this.fixed instanceof DecimalType; 8146 } 8147 8148 /** 8149 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8150 */ 8151 public IdType getFixedIdType() throws FHIRException { 8152 if (this.fixed == null) 8153 this.fixed = new IdType(); 8154 if (!(this.fixed instanceof IdType)) 8155 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8156 return (IdType) this.fixed; 8157 } 8158 8159 public boolean hasFixedIdType() { 8160 return this != null && this.fixed instanceof IdType; 8161 } 8162 8163 /** 8164 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8165 */ 8166 public InstantType getFixedInstantType() throws FHIRException { 8167 if (this.fixed == null) 8168 this.fixed = new InstantType(); 8169 if (!(this.fixed instanceof InstantType)) 8170 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8171 return (InstantType) this.fixed; 8172 } 8173 8174 public boolean hasFixedInstantType() { 8175 return this != null && this.fixed instanceof InstantType; 8176 } 8177 8178 /** 8179 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8180 */ 8181 public IntegerType getFixedIntegerType() throws FHIRException { 8182 if (this.fixed == null) 8183 this.fixed = new IntegerType(); 8184 if (!(this.fixed instanceof IntegerType)) 8185 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8186 return (IntegerType) this.fixed; 8187 } 8188 8189 public boolean hasFixedIntegerType() { 8190 return this != null && this.fixed instanceof IntegerType; 8191 } 8192 8193 /** 8194 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8195 */ 8196 public Integer64Type getFixedInteger64Type() throws FHIRException { 8197 if (this.fixed == null) 8198 this.fixed = new Integer64Type(); 8199 if (!(this.fixed instanceof Integer64Type)) 8200 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8201 return (Integer64Type) this.fixed; 8202 } 8203 8204 public boolean hasFixedInteger64Type() { 8205 return this != null && this.fixed instanceof Integer64Type; 8206 } 8207 8208 /** 8209 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8210 */ 8211 public MarkdownType getFixedMarkdownType() throws FHIRException { 8212 if (this.fixed == null) 8213 this.fixed = new MarkdownType(); 8214 if (!(this.fixed instanceof MarkdownType)) 8215 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8216 return (MarkdownType) this.fixed; 8217 } 8218 8219 public boolean hasFixedMarkdownType() { 8220 return this != null && this.fixed instanceof MarkdownType; 8221 } 8222 8223 /** 8224 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8225 */ 8226 public OidType getFixedOidType() throws FHIRException { 8227 if (this.fixed == null) 8228 this.fixed = new OidType(); 8229 if (!(this.fixed instanceof OidType)) 8230 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8231 return (OidType) this.fixed; 8232 } 8233 8234 public boolean hasFixedOidType() { 8235 return this != null && this.fixed instanceof OidType; 8236 } 8237 8238 /** 8239 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8240 */ 8241 public PositiveIntType getFixedPositiveIntType() throws FHIRException { 8242 if (this.fixed == null) 8243 this.fixed = new PositiveIntType(); 8244 if (!(this.fixed instanceof PositiveIntType)) 8245 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8246 return (PositiveIntType) this.fixed; 8247 } 8248 8249 public boolean hasFixedPositiveIntType() { 8250 return this != null && this.fixed instanceof PositiveIntType; 8251 } 8252 8253 /** 8254 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8255 */ 8256 public StringType getFixedStringType() throws FHIRException { 8257 if (this.fixed == null) 8258 this.fixed = new StringType(); 8259 if (!(this.fixed instanceof StringType)) 8260 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8261 return (StringType) this.fixed; 8262 } 8263 8264 public boolean hasFixedStringType() { 8265 return this != null && this.fixed instanceof StringType; 8266 } 8267 8268 /** 8269 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8270 */ 8271 public TimeType getFixedTimeType() throws FHIRException { 8272 if (this.fixed == null) 8273 this.fixed = new TimeType(); 8274 if (!(this.fixed instanceof TimeType)) 8275 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8276 return (TimeType) this.fixed; 8277 } 8278 8279 public boolean hasFixedTimeType() { 8280 return this != null && this.fixed instanceof TimeType; 8281 } 8282 8283 /** 8284 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8285 */ 8286 public UnsignedIntType getFixedUnsignedIntType() throws FHIRException { 8287 if (this.fixed == null) 8288 this.fixed = new UnsignedIntType(); 8289 if (!(this.fixed instanceof UnsignedIntType)) 8290 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8291 return (UnsignedIntType) this.fixed; 8292 } 8293 8294 public boolean hasFixedUnsignedIntType() { 8295 return this != null && this.fixed instanceof UnsignedIntType; 8296 } 8297 8298 /** 8299 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8300 */ 8301 public UriType getFixedUriType() throws FHIRException { 8302 if (this.fixed == null) 8303 this.fixed = new UriType(); 8304 if (!(this.fixed instanceof UriType)) 8305 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8306 return (UriType) this.fixed; 8307 } 8308 8309 public boolean hasFixedUriType() { 8310 return this != null && this.fixed instanceof UriType; 8311 } 8312 8313 /** 8314 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8315 */ 8316 public UrlType getFixedUrlType() throws FHIRException { 8317 if (this.fixed == null) 8318 this.fixed = new UrlType(); 8319 if (!(this.fixed instanceof UrlType)) 8320 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8321 return (UrlType) this.fixed; 8322 } 8323 8324 public boolean hasFixedUrlType() { 8325 return this != null && this.fixed instanceof UrlType; 8326 } 8327 8328 /** 8329 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8330 */ 8331 public UuidType getFixedUuidType() throws FHIRException { 8332 if (this.fixed == null) 8333 this.fixed = new UuidType(); 8334 if (!(this.fixed instanceof UuidType)) 8335 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8336 return (UuidType) this.fixed; 8337 } 8338 8339 public boolean hasFixedUuidType() { 8340 return this != null && this.fixed instanceof UuidType; 8341 } 8342 8343 /** 8344 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8345 */ 8346 public Address getFixedAddress() throws FHIRException { 8347 if (this.fixed == null) 8348 this.fixed = new Address(); 8349 if (!(this.fixed instanceof Address)) 8350 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8351 return (Address) this.fixed; 8352 } 8353 8354 public boolean hasFixedAddress() { 8355 return this != null && this.fixed instanceof Address; 8356 } 8357 8358 /** 8359 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8360 */ 8361 public Age getFixedAge() throws FHIRException { 8362 if (this.fixed == null) 8363 this.fixed = new Age(); 8364 if (!(this.fixed instanceof Age)) 8365 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8366 return (Age) this.fixed; 8367 } 8368 8369 public boolean hasFixedAge() { 8370 return this != null && this.fixed instanceof Age; 8371 } 8372 8373 /** 8374 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8375 */ 8376 public Annotation getFixedAnnotation() throws FHIRException { 8377 if (this.fixed == null) 8378 this.fixed = new Annotation(); 8379 if (!(this.fixed instanceof Annotation)) 8380 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8381 return (Annotation) this.fixed; 8382 } 8383 8384 public boolean hasFixedAnnotation() { 8385 return this != null && this.fixed instanceof Annotation; 8386 } 8387 8388 /** 8389 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8390 */ 8391 public Attachment getFixedAttachment() throws FHIRException { 8392 if (this.fixed == null) 8393 this.fixed = new Attachment(); 8394 if (!(this.fixed instanceof Attachment)) 8395 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8396 return (Attachment) this.fixed; 8397 } 8398 8399 public boolean hasFixedAttachment() { 8400 return this != null && this.fixed instanceof Attachment; 8401 } 8402 8403 /** 8404 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8405 */ 8406 public CodeableConcept getFixedCodeableConcept() throws FHIRException { 8407 if (this.fixed == null) 8408 this.fixed = new CodeableConcept(); 8409 if (!(this.fixed instanceof CodeableConcept)) 8410 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8411 return (CodeableConcept) this.fixed; 8412 } 8413 8414 public boolean hasFixedCodeableConcept() { 8415 return this != null && this.fixed instanceof CodeableConcept; 8416 } 8417 8418 /** 8419 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8420 */ 8421 public CodeableReference getFixedCodeableReference() throws FHIRException { 8422 if (this.fixed == null) 8423 this.fixed = new CodeableReference(); 8424 if (!(this.fixed instanceof CodeableReference)) 8425 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8426 return (CodeableReference) this.fixed; 8427 } 8428 8429 public boolean hasFixedCodeableReference() { 8430 return this != null && this.fixed instanceof CodeableReference; 8431 } 8432 8433 /** 8434 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8435 */ 8436 public Coding getFixedCoding() throws FHIRException { 8437 if (this.fixed == null) 8438 this.fixed = new Coding(); 8439 if (!(this.fixed instanceof Coding)) 8440 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8441 return (Coding) this.fixed; 8442 } 8443 8444 public boolean hasFixedCoding() { 8445 return this != null && this.fixed instanceof Coding; 8446 } 8447 8448 /** 8449 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8450 */ 8451 public ContactPoint getFixedContactPoint() throws FHIRException { 8452 if (this.fixed == null) 8453 this.fixed = new ContactPoint(); 8454 if (!(this.fixed instanceof ContactPoint)) 8455 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8456 return (ContactPoint) this.fixed; 8457 } 8458 8459 public boolean hasFixedContactPoint() { 8460 return this != null && this.fixed instanceof ContactPoint; 8461 } 8462 8463 /** 8464 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8465 */ 8466 public Count getFixedCount() throws FHIRException { 8467 if (this.fixed == null) 8468 this.fixed = new Count(); 8469 if (!(this.fixed instanceof Count)) 8470 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8471 return (Count) this.fixed; 8472 } 8473 8474 public boolean hasFixedCount() { 8475 return this != null && this.fixed instanceof Count; 8476 } 8477 8478 /** 8479 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8480 */ 8481 public Distance getFixedDistance() throws FHIRException { 8482 if (this.fixed == null) 8483 this.fixed = new Distance(); 8484 if (!(this.fixed instanceof Distance)) 8485 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8486 return (Distance) this.fixed; 8487 } 8488 8489 public boolean hasFixedDistance() { 8490 return this != null && this.fixed instanceof Distance; 8491 } 8492 8493 /** 8494 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8495 */ 8496 public Duration getFixedDuration() throws FHIRException { 8497 if (this.fixed == null) 8498 this.fixed = new Duration(); 8499 if (!(this.fixed instanceof Duration)) 8500 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8501 return (Duration) this.fixed; 8502 } 8503 8504 public boolean hasFixedDuration() { 8505 return this != null && this.fixed instanceof Duration; 8506 } 8507 8508 /** 8509 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8510 */ 8511 public HumanName getFixedHumanName() throws FHIRException { 8512 if (this.fixed == null) 8513 this.fixed = new HumanName(); 8514 if (!(this.fixed instanceof HumanName)) 8515 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8516 return (HumanName) this.fixed; 8517 } 8518 8519 public boolean hasFixedHumanName() { 8520 return this != null && this.fixed instanceof HumanName; 8521 } 8522 8523 /** 8524 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8525 */ 8526 public Identifier getFixedIdentifier() throws FHIRException { 8527 if (this.fixed == null) 8528 this.fixed = new Identifier(); 8529 if (!(this.fixed instanceof Identifier)) 8530 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8531 return (Identifier) this.fixed; 8532 } 8533 8534 public boolean hasFixedIdentifier() { 8535 return this != null && this.fixed instanceof Identifier; 8536 } 8537 8538 /** 8539 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8540 */ 8541 public Money getFixedMoney() throws FHIRException { 8542 if (this.fixed == null) 8543 this.fixed = new Money(); 8544 if (!(this.fixed instanceof Money)) 8545 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8546 return (Money) this.fixed; 8547 } 8548 8549 public boolean hasFixedMoney() { 8550 return this != null && this.fixed instanceof Money; 8551 } 8552 8553 /** 8554 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8555 */ 8556 public Period getFixedPeriod() throws FHIRException { 8557 if (this.fixed == null) 8558 this.fixed = new Period(); 8559 if (!(this.fixed instanceof Period)) 8560 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8561 return (Period) this.fixed; 8562 } 8563 8564 public boolean hasFixedPeriod() { 8565 return this != null && this.fixed instanceof Period; 8566 } 8567 8568 /** 8569 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8570 */ 8571 public Quantity getFixedQuantity() throws FHIRException { 8572 if (this.fixed == null) 8573 this.fixed = new Quantity(); 8574 if (!(this.fixed instanceof Quantity)) 8575 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8576 return (Quantity) this.fixed; 8577 } 8578 8579 public boolean hasFixedQuantity() { 8580 return this != null && this.fixed instanceof Quantity; 8581 } 8582 8583 /** 8584 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8585 */ 8586 public Range getFixedRange() throws FHIRException { 8587 if (this.fixed == null) 8588 this.fixed = new Range(); 8589 if (!(this.fixed instanceof Range)) 8590 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8591 return (Range) this.fixed; 8592 } 8593 8594 public boolean hasFixedRange() { 8595 return this != null && this.fixed instanceof Range; 8596 } 8597 8598 /** 8599 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8600 */ 8601 public Ratio getFixedRatio() throws FHIRException { 8602 if (this.fixed == null) 8603 this.fixed = new Ratio(); 8604 if (!(this.fixed instanceof Ratio)) 8605 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8606 return (Ratio) this.fixed; 8607 } 8608 8609 public boolean hasFixedRatio() { 8610 return this != null && this.fixed instanceof Ratio; 8611 } 8612 8613 /** 8614 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8615 */ 8616 public RatioRange getFixedRatioRange() throws FHIRException { 8617 if (this.fixed == null) 8618 this.fixed = new RatioRange(); 8619 if (!(this.fixed instanceof RatioRange)) 8620 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8621 return (RatioRange) this.fixed; 8622 } 8623 8624 public boolean hasFixedRatioRange() { 8625 return this != null && this.fixed instanceof RatioRange; 8626 } 8627 8628 /** 8629 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8630 */ 8631 public Reference getFixedReference() throws FHIRException { 8632 if (this.fixed == null) 8633 this.fixed = new Reference(); 8634 if (!(this.fixed instanceof Reference)) 8635 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8636 return (Reference) this.fixed; 8637 } 8638 8639 public boolean hasFixedReference() { 8640 return this != null && this.fixed instanceof Reference; 8641 } 8642 8643 /** 8644 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8645 */ 8646 public SampledData getFixedSampledData() throws FHIRException { 8647 if (this.fixed == null) 8648 this.fixed = new SampledData(); 8649 if (!(this.fixed instanceof SampledData)) 8650 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8651 return (SampledData) this.fixed; 8652 } 8653 8654 public boolean hasFixedSampledData() { 8655 return this != null && this.fixed instanceof SampledData; 8656 } 8657 8658 /** 8659 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8660 */ 8661 public Signature getFixedSignature() throws FHIRException { 8662 if (this.fixed == null) 8663 this.fixed = new Signature(); 8664 if (!(this.fixed instanceof Signature)) 8665 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8666 return (Signature) this.fixed; 8667 } 8668 8669 public boolean hasFixedSignature() { 8670 return this != null && this.fixed instanceof Signature; 8671 } 8672 8673 /** 8674 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8675 */ 8676 public Timing getFixedTiming() throws FHIRException { 8677 if (this.fixed == null) 8678 this.fixed = new Timing(); 8679 if (!(this.fixed instanceof Timing)) 8680 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8681 return (Timing) this.fixed; 8682 } 8683 8684 public boolean hasFixedTiming() { 8685 return this != null && this.fixed instanceof Timing; 8686 } 8687 8688 /** 8689 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8690 */ 8691 public ContactDetail getFixedContactDetail() throws FHIRException { 8692 if (this.fixed == null) 8693 this.fixed = new ContactDetail(); 8694 if (!(this.fixed instanceof ContactDetail)) 8695 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8696 return (ContactDetail) this.fixed; 8697 } 8698 8699 public boolean hasFixedContactDetail() { 8700 return this != null && this.fixed instanceof ContactDetail; 8701 } 8702 8703 /** 8704 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8705 */ 8706 public DataRequirement getFixedDataRequirement() throws FHIRException { 8707 if (this.fixed == null) 8708 this.fixed = new DataRequirement(); 8709 if (!(this.fixed instanceof DataRequirement)) 8710 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8711 return (DataRequirement) this.fixed; 8712 } 8713 8714 public boolean hasFixedDataRequirement() { 8715 return this != null && this.fixed instanceof DataRequirement; 8716 } 8717 8718 /** 8719 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8720 */ 8721 public Expression getFixedExpression() throws FHIRException { 8722 if (this.fixed == null) 8723 this.fixed = new Expression(); 8724 if (!(this.fixed instanceof Expression)) 8725 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8726 return (Expression) this.fixed; 8727 } 8728 8729 public boolean hasFixedExpression() { 8730 return this != null && this.fixed instanceof Expression; 8731 } 8732 8733 /** 8734 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8735 */ 8736 public ParameterDefinition getFixedParameterDefinition() throws FHIRException { 8737 if (this.fixed == null) 8738 this.fixed = new ParameterDefinition(); 8739 if (!(this.fixed instanceof ParameterDefinition)) 8740 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8741 return (ParameterDefinition) this.fixed; 8742 } 8743 8744 public boolean hasFixedParameterDefinition() { 8745 return this != null && this.fixed instanceof ParameterDefinition; 8746 } 8747 8748 /** 8749 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8750 */ 8751 public RelatedArtifact getFixedRelatedArtifact() throws FHIRException { 8752 if (this.fixed == null) 8753 this.fixed = new RelatedArtifact(); 8754 if (!(this.fixed instanceof RelatedArtifact)) 8755 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8756 return (RelatedArtifact) this.fixed; 8757 } 8758 8759 public boolean hasFixedRelatedArtifact() { 8760 return this != null && this.fixed instanceof RelatedArtifact; 8761 } 8762 8763 /** 8764 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8765 */ 8766 public TriggerDefinition getFixedTriggerDefinition() throws FHIRException { 8767 if (this.fixed == null) 8768 this.fixed = new TriggerDefinition(); 8769 if (!(this.fixed instanceof TriggerDefinition)) 8770 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8771 return (TriggerDefinition) this.fixed; 8772 } 8773 8774 public boolean hasFixedTriggerDefinition() { 8775 return this != null && this.fixed instanceof TriggerDefinition; 8776 } 8777 8778 /** 8779 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8780 */ 8781 public UsageContext getFixedUsageContext() throws FHIRException { 8782 if (this.fixed == null) 8783 this.fixed = new UsageContext(); 8784 if (!(this.fixed instanceof UsageContext)) 8785 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8786 return (UsageContext) this.fixed; 8787 } 8788 8789 public boolean hasFixedUsageContext() { 8790 return this != null && this.fixed instanceof UsageContext; 8791 } 8792 8793 /** 8794 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8795 */ 8796 public Availability getFixedAvailability() throws FHIRException { 8797 if (this.fixed == null) 8798 this.fixed = new Availability(); 8799 if (!(this.fixed instanceof Availability)) 8800 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8801 return (Availability) this.fixed; 8802 } 8803 8804 public boolean hasFixedAvailability() { 8805 return this != null && this.fixed instanceof Availability; 8806 } 8807 8808 /** 8809 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8810 */ 8811 public ExtendedContactDetail getFixedExtendedContactDetail() throws FHIRException { 8812 if (this.fixed == null) 8813 this.fixed = new ExtendedContactDetail(); 8814 if (!(this.fixed instanceof ExtendedContactDetail)) 8815 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8816 return (ExtendedContactDetail) this.fixed; 8817 } 8818 8819 public boolean hasFixedExtendedContactDetail() { 8820 return this != null && this.fixed instanceof ExtendedContactDetail; 8821 } 8822 8823 /** 8824 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8825 */ 8826 public Dosage getFixedDosage() throws FHIRException { 8827 if (this.fixed == null) 8828 this.fixed = new Dosage(); 8829 if (!(this.fixed instanceof Dosage)) 8830 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8831 return (Dosage) this.fixed; 8832 } 8833 8834 public boolean hasFixedDosage() { 8835 return this != null && this.fixed instanceof Dosage; 8836 } 8837 8838 /** 8839 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8840 */ 8841 public Meta getFixedMeta() throws FHIRException { 8842 if (this.fixed == null) 8843 this.fixed = new Meta(); 8844 if (!(this.fixed instanceof Meta)) 8845 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.fixed.getClass().getName()+" was encountered"); 8846 return (Meta) this.fixed; 8847 } 8848 8849 public boolean hasFixedMeta() { 8850 return this != null && this.fixed instanceof Meta; 8851 } 8852 8853 public boolean hasFixed() { 8854 return this.fixed != null && !this.fixed.isEmpty(); 8855 } 8856 8857 /** 8858 * @param value {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 8859 */ 8860 public ElementDefinition setFixed(DataType value) { 8861 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 8862 throw new FHIRException("Not the right type for ElementDefinition.fixed[x]: "+value.fhirType()); 8863 this.fixed = value; 8864 return this; 8865 } 8866 8867 /** 8868 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8869 8870When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8871 8872When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8873 8874When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8875 88761. If primitive: it must match exactly the pattern value 88772. If a complex object: it must match (recursively) the pattern value 88783. If an array: it must match (recursively) the pattern value 8879 8880If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8881 */ 8882 public DataType getPattern() { 8883 return this.pattern; 8884 } 8885 8886 /** 8887 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8888 8889When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8890 8891When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8892 8893When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8894 88951. If primitive: it must match exactly the pattern value 88962. If a complex object: it must match (recursively) the pattern value 88973. If an array: it must match (recursively) the pattern value 8898 8899If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8900 */ 8901 public Base64BinaryType getPatternBase64BinaryType() throws FHIRException { 8902 if (this.pattern == null) 8903 this.pattern = new Base64BinaryType(); 8904 if (!(this.pattern instanceof Base64BinaryType)) 8905 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 8906 return (Base64BinaryType) this.pattern; 8907 } 8908 8909 public boolean hasPatternBase64BinaryType() { 8910 return this != null && this.pattern instanceof Base64BinaryType; 8911 } 8912 8913 /** 8914 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8915 8916When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8917 8918When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8919 8920When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8921 89221. If primitive: it must match exactly the pattern value 89232. If a complex object: it must match (recursively) the pattern value 89243. If an array: it must match (recursively) the pattern value 8925 8926If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8927 */ 8928 public BooleanType getPatternBooleanType() throws FHIRException { 8929 if (this.pattern == null) 8930 this.pattern = new BooleanType(); 8931 if (!(this.pattern instanceof BooleanType)) 8932 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 8933 return (BooleanType) this.pattern; 8934 } 8935 8936 public boolean hasPatternBooleanType() { 8937 return this != null && this.pattern instanceof BooleanType; 8938 } 8939 8940 /** 8941 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8942 8943When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8944 8945When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8946 8947When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8948 89491. If primitive: it must match exactly the pattern value 89502. If a complex object: it must match (recursively) the pattern value 89513. If an array: it must match (recursively) the pattern value 8952 8953If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8954 */ 8955 public CanonicalType getPatternCanonicalType() throws FHIRException { 8956 if (this.pattern == null) 8957 this.pattern = new CanonicalType(); 8958 if (!(this.pattern instanceof CanonicalType)) 8959 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 8960 return (CanonicalType) this.pattern; 8961 } 8962 8963 public boolean hasPatternCanonicalType() { 8964 return this != null && this.pattern instanceof CanonicalType; 8965 } 8966 8967 /** 8968 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8969 8970When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8971 8972When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 8973 8974When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 8975 89761. If primitive: it must match exactly the pattern value 89772. If a complex object: it must match (recursively) the pattern value 89783. If an array: it must match (recursively) the pattern value 8979 8980If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 8981 */ 8982 public CodeType getPatternCodeType() throws FHIRException { 8983 if (this.pattern == null) 8984 this.pattern = new CodeType(); 8985 if (!(this.pattern instanceof CodeType)) 8986 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 8987 return (CodeType) this.pattern; 8988 } 8989 8990 public boolean hasPatternCodeType() { 8991 return this != null && this.pattern instanceof CodeType; 8992 } 8993 8994 /** 8995 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 8996 8997When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 8998 8999When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9000 9001When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9002 90031. If primitive: it must match exactly the pattern value 90042. If a complex object: it must match (recursively) the pattern value 90053. If an array: it must match (recursively) the pattern value 9006 9007If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9008 */ 9009 public DateType getPatternDateType() throws FHIRException { 9010 if (this.pattern == null) 9011 this.pattern = new DateType(); 9012 if (!(this.pattern instanceof DateType)) 9013 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9014 return (DateType) this.pattern; 9015 } 9016 9017 public boolean hasPatternDateType() { 9018 return this != null && this.pattern instanceof DateType; 9019 } 9020 9021 /** 9022 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9023 9024When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9025 9026When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9027 9028When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9029 90301. If primitive: it must match exactly the pattern value 90312. If a complex object: it must match (recursively) the pattern value 90323. If an array: it must match (recursively) the pattern value 9033 9034If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9035 */ 9036 public DateTimeType getPatternDateTimeType() throws FHIRException { 9037 if (this.pattern == null) 9038 this.pattern = new DateTimeType(); 9039 if (!(this.pattern instanceof DateTimeType)) 9040 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9041 return (DateTimeType) this.pattern; 9042 } 9043 9044 public boolean hasPatternDateTimeType() { 9045 return this != null && this.pattern instanceof DateTimeType; 9046 } 9047 9048 /** 9049 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9050 9051When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9052 9053When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9054 9055When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9056 90571. If primitive: it must match exactly the pattern value 90582. If a complex object: it must match (recursively) the pattern value 90593. If an array: it must match (recursively) the pattern value 9060 9061If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9062 */ 9063 public DecimalType getPatternDecimalType() throws FHIRException { 9064 if (this.pattern == null) 9065 this.pattern = new DecimalType(); 9066 if (!(this.pattern instanceof DecimalType)) 9067 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9068 return (DecimalType) this.pattern; 9069 } 9070 9071 public boolean hasPatternDecimalType() { 9072 return this != null && this.pattern instanceof DecimalType; 9073 } 9074 9075 /** 9076 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9077 9078When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9079 9080When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9081 9082When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9083 90841. If primitive: it must match exactly the pattern value 90852. If a complex object: it must match (recursively) the pattern value 90863. If an array: it must match (recursively) the pattern value 9087 9088If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9089 */ 9090 public IdType getPatternIdType() throws FHIRException { 9091 if (this.pattern == null) 9092 this.pattern = new IdType(); 9093 if (!(this.pattern instanceof IdType)) 9094 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9095 return (IdType) this.pattern; 9096 } 9097 9098 public boolean hasPatternIdType() { 9099 return this != null && this.pattern instanceof IdType; 9100 } 9101 9102 /** 9103 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9104 9105When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9106 9107When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9108 9109When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9110 91111. If primitive: it must match exactly the pattern value 91122. If a complex object: it must match (recursively) the pattern value 91133. If an array: it must match (recursively) the pattern value 9114 9115If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9116 */ 9117 public InstantType getPatternInstantType() throws FHIRException { 9118 if (this.pattern == null) 9119 this.pattern = new InstantType(); 9120 if (!(this.pattern instanceof InstantType)) 9121 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9122 return (InstantType) this.pattern; 9123 } 9124 9125 public boolean hasPatternInstantType() { 9126 return this != null && this.pattern instanceof InstantType; 9127 } 9128 9129 /** 9130 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9131 9132When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9133 9134When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9135 9136When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9137 91381. If primitive: it must match exactly the pattern value 91392. If a complex object: it must match (recursively) the pattern value 91403. If an array: it must match (recursively) the pattern value 9141 9142If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9143 */ 9144 public IntegerType getPatternIntegerType() throws FHIRException { 9145 if (this.pattern == null) 9146 this.pattern = new IntegerType(); 9147 if (!(this.pattern instanceof IntegerType)) 9148 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9149 return (IntegerType) this.pattern; 9150 } 9151 9152 public boolean hasPatternIntegerType() { 9153 return this != null && this.pattern instanceof IntegerType; 9154 } 9155 9156 /** 9157 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9158 9159When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9160 9161When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9162 9163When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9164 91651. If primitive: it must match exactly the pattern value 91662. If a complex object: it must match (recursively) the pattern value 91673. If an array: it must match (recursively) the pattern value 9168 9169If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9170 */ 9171 public Integer64Type getPatternInteger64Type() throws FHIRException { 9172 if (this.pattern == null) 9173 this.pattern = new Integer64Type(); 9174 if (!(this.pattern instanceof Integer64Type)) 9175 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9176 return (Integer64Type) this.pattern; 9177 } 9178 9179 public boolean hasPatternInteger64Type() { 9180 return this != null && this.pattern instanceof Integer64Type; 9181 } 9182 9183 /** 9184 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9185 9186When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9187 9188When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9189 9190When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9191 91921. If primitive: it must match exactly the pattern value 91932. If a complex object: it must match (recursively) the pattern value 91943. If an array: it must match (recursively) the pattern value 9195 9196If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9197 */ 9198 public MarkdownType getPatternMarkdownType() throws FHIRException { 9199 if (this.pattern == null) 9200 this.pattern = new MarkdownType(); 9201 if (!(this.pattern instanceof MarkdownType)) 9202 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9203 return (MarkdownType) this.pattern; 9204 } 9205 9206 public boolean hasPatternMarkdownType() { 9207 return this != null && this.pattern instanceof MarkdownType; 9208 } 9209 9210 /** 9211 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9212 9213When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9214 9215When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9216 9217When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9218 92191. If primitive: it must match exactly the pattern value 92202. If a complex object: it must match (recursively) the pattern value 92213. If an array: it must match (recursively) the pattern value 9222 9223If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9224 */ 9225 public OidType getPatternOidType() throws FHIRException { 9226 if (this.pattern == null) 9227 this.pattern = new OidType(); 9228 if (!(this.pattern instanceof OidType)) 9229 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9230 return (OidType) this.pattern; 9231 } 9232 9233 public boolean hasPatternOidType() { 9234 return this != null && this.pattern instanceof OidType; 9235 } 9236 9237 /** 9238 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9239 9240When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9241 9242When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9243 9244When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9245 92461. If primitive: it must match exactly the pattern value 92472. If a complex object: it must match (recursively) the pattern value 92483. If an array: it must match (recursively) the pattern value 9249 9250If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9251 */ 9252 public PositiveIntType getPatternPositiveIntType() throws FHIRException { 9253 if (this.pattern == null) 9254 this.pattern = new PositiveIntType(); 9255 if (!(this.pattern instanceof PositiveIntType)) 9256 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9257 return (PositiveIntType) this.pattern; 9258 } 9259 9260 public boolean hasPatternPositiveIntType() { 9261 return this != null && this.pattern instanceof PositiveIntType; 9262 } 9263 9264 /** 9265 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9266 9267When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9268 9269When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9270 9271When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9272 92731. If primitive: it must match exactly the pattern value 92742. If a complex object: it must match (recursively) the pattern value 92753. If an array: it must match (recursively) the pattern value 9276 9277If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9278 */ 9279 public StringType getPatternStringType() throws FHIRException { 9280 if (this.pattern == null) 9281 this.pattern = new StringType(); 9282 if (!(this.pattern instanceof StringType)) 9283 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9284 return (StringType) this.pattern; 9285 } 9286 9287 public boolean hasPatternStringType() { 9288 return this != null && this.pattern instanceof StringType; 9289 } 9290 9291 /** 9292 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9293 9294When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9295 9296When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9297 9298When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9299 93001. If primitive: it must match exactly the pattern value 93012. If a complex object: it must match (recursively) the pattern value 93023. If an array: it must match (recursively) the pattern value 9303 9304If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9305 */ 9306 public TimeType getPatternTimeType() throws FHIRException { 9307 if (this.pattern == null) 9308 this.pattern = new TimeType(); 9309 if (!(this.pattern instanceof TimeType)) 9310 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9311 return (TimeType) this.pattern; 9312 } 9313 9314 public boolean hasPatternTimeType() { 9315 return this != null && this.pattern instanceof TimeType; 9316 } 9317 9318 /** 9319 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9320 9321When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9322 9323When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9324 9325When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9326 93271. If primitive: it must match exactly the pattern value 93282. If a complex object: it must match (recursively) the pattern value 93293. If an array: it must match (recursively) the pattern value 9330 9331If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9332 */ 9333 public UnsignedIntType getPatternUnsignedIntType() throws FHIRException { 9334 if (this.pattern == null) 9335 this.pattern = new UnsignedIntType(); 9336 if (!(this.pattern instanceof UnsignedIntType)) 9337 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9338 return (UnsignedIntType) this.pattern; 9339 } 9340 9341 public boolean hasPatternUnsignedIntType() { 9342 return this != null && this.pattern instanceof UnsignedIntType; 9343 } 9344 9345 /** 9346 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9347 9348When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9349 9350When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9351 9352When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9353 93541. If primitive: it must match exactly the pattern value 93552. If a complex object: it must match (recursively) the pattern value 93563. If an array: it must match (recursively) the pattern value 9357 9358If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9359 */ 9360 public UriType getPatternUriType() throws FHIRException { 9361 if (this.pattern == null) 9362 this.pattern = new UriType(); 9363 if (!(this.pattern instanceof UriType)) 9364 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9365 return (UriType) this.pattern; 9366 } 9367 9368 public boolean hasPatternUriType() { 9369 return this != null && this.pattern instanceof UriType; 9370 } 9371 9372 /** 9373 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9374 9375When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9376 9377When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9378 9379When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9380 93811. If primitive: it must match exactly the pattern value 93822. If a complex object: it must match (recursively) the pattern value 93833. If an array: it must match (recursively) the pattern value 9384 9385If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9386 */ 9387 public UrlType getPatternUrlType() throws FHIRException { 9388 if (this.pattern == null) 9389 this.pattern = new UrlType(); 9390 if (!(this.pattern instanceof UrlType)) 9391 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9392 return (UrlType) this.pattern; 9393 } 9394 9395 public boolean hasPatternUrlType() { 9396 return this != null && this.pattern instanceof UrlType; 9397 } 9398 9399 /** 9400 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9401 9402When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9403 9404When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9405 9406When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9407 94081. If primitive: it must match exactly the pattern value 94092. If a complex object: it must match (recursively) the pattern value 94103. If an array: it must match (recursively) the pattern value 9411 9412If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9413 */ 9414 public UuidType getPatternUuidType() throws FHIRException { 9415 if (this.pattern == null) 9416 this.pattern = new UuidType(); 9417 if (!(this.pattern instanceof UuidType)) 9418 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9419 return (UuidType) this.pattern; 9420 } 9421 9422 public boolean hasPatternUuidType() { 9423 return this != null && this.pattern instanceof UuidType; 9424 } 9425 9426 /** 9427 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9428 9429When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9430 9431When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9432 9433When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9434 94351. If primitive: it must match exactly the pattern value 94362. If a complex object: it must match (recursively) the pattern value 94373. If an array: it must match (recursively) the pattern value 9438 9439If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9440 */ 9441 public Address getPatternAddress() throws FHIRException { 9442 if (this.pattern == null) 9443 this.pattern = new Address(); 9444 if (!(this.pattern instanceof Address)) 9445 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9446 return (Address) this.pattern; 9447 } 9448 9449 public boolean hasPatternAddress() { 9450 return this != null && this.pattern instanceof Address; 9451 } 9452 9453 /** 9454 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9455 9456When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9457 9458When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9459 9460When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9461 94621. If primitive: it must match exactly the pattern value 94632. If a complex object: it must match (recursively) the pattern value 94643. If an array: it must match (recursively) the pattern value 9465 9466If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9467 */ 9468 public Age getPatternAge() throws FHIRException { 9469 if (this.pattern == null) 9470 this.pattern = new Age(); 9471 if (!(this.pattern instanceof Age)) 9472 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9473 return (Age) this.pattern; 9474 } 9475 9476 public boolean hasPatternAge() { 9477 return this != null && this.pattern instanceof Age; 9478 } 9479 9480 /** 9481 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9482 9483When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9484 9485When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9486 9487When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9488 94891. If primitive: it must match exactly the pattern value 94902. If a complex object: it must match (recursively) the pattern value 94913. If an array: it must match (recursively) the pattern value 9492 9493If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9494 */ 9495 public Annotation getPatternAnnotation() throws FHIRException { 9496 if (this.pattern == null) 9497 this.pattern = new Annotation(); 9498 if (!(this.pattern instanceof Annotation)) 9499 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9500 return (Annotation) this.pattern; 9501 } 9502 9503 public boolean hasPatternAnnotation() { 9504 return this != null && this.pattern instanceof Annotation; 9505 } 9506 9507 /** 9508 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9509 9510When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9511 9512When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9513 9514When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9515 95161. If primitive: it must match exactly the pattern value 95172. If a complex object: it must match (recursively) the pattern value 95183. If an array: it must match (recursively) the pattern value 9519 9520If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9521 */ 9522 public Attachment getPatternAttachment() throws FHIRException { 9523 if (this.pattern == null) 9524 this.pattern = new Attachment(); 9525 if (!(this.pattern instanceof Attachment)) 9526 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9527 return (Attachment) this.pattern; 9528 } 9529 9530 public boolean hasPatternAttachment() { 9531 return this != null && this.pattern instanceof Attachment; 9532 } 9533 9534 /** 9535 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9536 9537When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9538 9539When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9540 9541When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9542 95431. If primitive: it must match exactly the pattern value 95442. If a complex object: it must match (recursively) the pattern value 95453. If an array: it must match (recursively) the pattern value 9546 9547If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9548 */ 9549 public CodeableConcept getPatternCodeableConcept() throws FHIRException { 9550 if (this.pattern == null) 9551 this.pattern = new CodeableConcept(); 9552 if (!(this.pattern instanceof CodeableConcept)) 9553 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9554 return (CodeableConcept) this.pattern; 9555 } 9556 9557 public boolean hasPatternCodeableConcept() { 9558 return this != null && this.pattern instanceof CodeableConcept; 9559 } 9560 9561 /** 9562 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9563 9564When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9565 9566When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9567 9568When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9569 95701. If primitive: it must match exactly the pattern value 95712. If a complex object: it must match (recursively) the pattern value 95723. If an array: it must match (recursively) the pattern value 9573 9574If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9575 */ 9576 public CodeableReference getPatternCodeableReference() throws FHIRException { 9577 if (this.pattern == null) 9578 this.pattern = new CodeableReference(); 9579 if (!(this.pattern instanceof CodeableReference)) 9580 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9581 return (CodeableReference) this.pattern; 9582 } 9583 9584 public boolean hasPatternCodeableReference() { 9585 return this != null && this.pattern instanceof CodeableReference; 9586 } 9587 9588 /** 9589 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9590 9591When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9592 9593When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9594 9595When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9596 95971. If primitive: it must match exactly the pattern value 95982. If a complex object: it must match (recursively) the pattern value 95993. If an array: it must match (recursively) the pattern value 9600 9601If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9602 */ 9603 public Coding getPatternCoding() throws FHIRException { 9604 if (this.pattern == null) 9605 this.pattern = new Coding(); 9606 if (!(this.pattern instanceof Coding)) 9607 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9608 return (Coding) this.pattern; 9609 } 9610 9611 public boolean hasPatternCoding() { 9612 return this != null && this.pattern instanceof Coding; 9613 } 9614 9615 /** 9616 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9617 9618When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9619 9620When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9621 9622When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9623 96241. If primitive: it must match exactly the pattern value 96252. If a complex object: it must match (recursively) the pattern value 96263. If an array: it must match (recursively) the pattern value 9627 9628If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9629 */ 9630 public ContactPoint getPatternContactPoint() throws FHIRException { 9631 if (this.pattern == null) 9632 this.pattern = new ContactPoint(); 9633 if (!(this.pattern instanceof ContactPoint)) 9634 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9635 return (ContactPoint) this.pattern; 9636 } 9637 9638 public boolean hasPatternContactPoint() { 9639 return this != null && this.pattern instanceof ContactPoint; 9640 } 9641 9642 /** 9643 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9644 9645When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9646 9647When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9648 9649When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9650 96511. If primitive: it must match exactly the pattern value 96522. If a complex object: it must match (recursively) the pattern value 96533. If an array: it must match (recursively) the pattern value 9654 9655If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9656 */ 9657 public Count getPatternCount() throws FHIRException { 9658 if (this.pattern == null) 9659 this.pattern = new Count(); 9660 if (!(this.pattern instanceof Count)) 9661 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9662 return (Count) this.pattern; 9663 } 9664 9665 public boolean hasPatternCount() { 9666 return this != null && this.pattern instanceof Count; 9667 } 9668 9669 /** 9670 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9671 9672When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9673 9674When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9675 9676When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9677 96781. If primitive: it must match exactly the pattern value 96792. If a complex object: it must match (recursively) the pattern value 96803. If an array: it must match (recursively) the pattern value 9681 9682If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9683 */ 9684 public Distance getPatternDistance() throws FHIRException { 9685 if (this.pattern == null) 9686 this.pattern = new Distance(); 9687 if (!(this.pattern instanceof Distance)) 9688 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9689 return (Distance) this.pattern; 9690 } 9691 9692 public boolean hasPatternDistance() { 9693 return this != null && this.pattern instanceof Distance; 9694 } 9695 9696 /** 9697 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9698 9699When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9700 9701When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9702 9703When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9704 97051. If primitive: it must match exactly the pattern value 97062. If a complex object: it must match (recursively) the pattern value 97073. If an array: it must match (recursively) the pattern value 9708 9709If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9710 */ 9711 public Duration getPatternDuration() throws FHIRException { 9712 if (this.pattern == null) 9713 this.pattern = new Duration(); 9714 if (!(this.pattern instanceof Duration)) 9715 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9716 return (Duration) this.pattern; 9717 } 9718 9719 public boolean hasPatternDuration() { 9720 return this != null && this.pattern instanceof Duration; 9721 } 9722 9723 /** 9724 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9725 9726When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9727 9728When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9729 9730When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9731 97321. If primitive: it must match exactly the pattern value 97332. If a complex object: it must match (recursively) the pattern value 97343. If an array: it must match (recursively) the pattern value 9735 9736If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9737 */ 9738 public HumanName getPatternHumanName() throws FHIRException { 9739 if (this.pattern == null) 9740 this.pattern = new HumanName(); 9741 if (!(this.pattern instanceof HumanName)) 9742 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9743 return (HumanName) this.pattern; 9744 } 9745 9746 public boolean hasPatternHumanName() { 9747 return this != null && this.pattern instanceof HumanName; 9748 } 9749 9750 /** 9751 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9752 9753When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9754 9755When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9756 9757When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9758 97591. If primitive: it must match exactly the pattern value 97602. If a complex object: it must match (recursively) the pattern value 97613. If an array: it must match (recursively) the pattern value 9762 9763If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9764 */ 9765 public Identifier getPatternIdentifier() throws FHIRException { 9766 if (this.pattern == null) 9767 this.pattern = new Identifier(); 9768 if (!(this.pattern instanceof Identifier)) 9769 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9770 return (Identifier) this.pattern; 9771 } 9772 9773 public boolean hasPatternIdentifier() { 9774 return this != null && this.pattern instanceof Identifier; 9775 } 9776 9777 /** 9778 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9779 9780When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9781 9782When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9783 9784When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9785 97861. If primitive: it must match exactly the pattern value 97872. If a complex object: it must match (recursively) the pattern value 97883. If an array: it must match (recursively) the pattern value 9789 9790If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9791 */ 9792 public Money getPatternMoney() throws FHIRException { 9793 if (this.pattern == null) 9794 this.pattern = new Money(); 9795 if (!(this.pattern instanceof Money)) 9796 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9797 return (Money) this.pattern; 9798 } 9799 9800 public boolean hasPatternMoney() { 9801 return this != null && this.pattern instanceof Money; 9802 } 9803 9804 /** 9805 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9806 9807When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9808 9809When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9810 9811When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9812 98131. If primitive: it must match exactly the pattern value 98142. If a complex object: it must match (recursively) the pattern value 98153. If an array: it must match (recursively) the pattern value 9816 9817If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9818 */ 9819 public Period getPatternPeriod() throws FHIRException { 9820 if (this.pattern == null) 9821 this.pattern = new Period(); 9822 if (!(this.pattern instanceof Period)) 9823 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9824 return (Period) this.pattern; 9825 } 9826 9827 public boolean hasPatternPeriod() { 9828 return this != null && this.pattern instanceof Period; 9829 } 9830 9831 /** 9832 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9833 9834When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9835 9836When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9837 9838When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9839 98401. If primitive: it must match exactly the pattern value 98412. If a complex object: it must match (recursively) the pattern value 98423. If an array: it must match (recursively) the pattern value 9843 9844If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9845 */ 9846 public Quantity getPatternQuantity() throws FHIRException { 9847 if (this.pattern == null) 9848 this.pattern = new Quantity(); 9849 if (!(this.pattern instanceof Quantity)) 9850 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9851 return (Quantity) this.pattern; 9852 } 9853 9854 public boolean hasPatternQuantity() { 9855 return this != null && this.pattern instanceof Quantity; 9856 } 9857 9858 /** 9859 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9860 9861When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9862 9863When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9864 9865When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9866 98671. If primitive: it must match exactly the pattern value 98682. If a complex object: it must match (recursively) the pattern value 98693. If an array: it must match (recursively) the pattern value 9870 9871If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9872 */ 9873 public Range getPatternRange() throws FHIRException { 9874 if (this.pattern == null) 9875 this.pattern = new Range(); 9876 if (!(this.pattern instanceof Range)) 9877 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9878 return (Range) this.pattern; 9879 } 9880 9881 public boolean hasPatternRange() { 9882 return this != null && this.pattern instanceof Range; 9883 } 9884 9885 /** 9886 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9887 9888When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9889 9890When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9891 9892When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9893 98941. If primitive: it must match exactly the pattern value 98952. If a complex object: it must match (recursively) the pattern value 98963. If an array: it must match (recursively) the pattern value 9897 9898If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9899 */ 9900 public Ratio getPatternRatio() throws FHIRException { 9901 if (this.pattern == null) 9902 this.pattern = new Ratio(); 9903 if (!(this.pattern instanceof Ratio)) 9904 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9905 return (Ratio) this.pattern; 9906 } 9907 9908 public boolean hasPatternRatio() { 9909 return this != null && this.pattern instanceof Ratio; 9910 } 9911 9912 /** 9913 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9914 9915When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9916 9917When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9918 9919When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9920 99211. If primitive: it must match exactly the pattern value 99222. If a complex object: it must match (recursively) the pattern value 99233. If an array: it must match (recursively) the pattern value 9924 9925If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9926 */ 9927 public RatioRange getPatternRatioRange() throws FHIRException { 9928 if (this.pattern == null) 9929 this.pattern = new RatioRange(); 9930 if (!(this.pattern instanceof RatioRange)) 9931 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9932 return (RatioRange) this.pattern; 9933 } 9934 9935 public boolean hasPatternRatioRange() { 9936 return this != null && this.pattern instanceof RatioRange; 9937 } 9938 9939 /** 9940 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9941 9942When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9943 9944When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9945 9946When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9947 99481. If primitive: it must match exactly the pattern value 99492. If a complex object: it must match (recursively) the pattern value 99503. If an array: it must match (recursively) the pattern value 9951 9952If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9953 */ 9954 public Reference getPatternReference() throws FHIRException { 9955 if (this.pattern == null) 9956 this.pattern = new Reference(); 9957 if (!(this.pattern instanceof Reference)) 9958 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9959 return (Reference) this.pattern; 9960 } 9961 9962 public boolean hasPatternReference() { 9963 return this != null && this.pattern instanceof Reference; 9964 } 9965 9966 /** 9967 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9968 9969When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9970 9971When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9972 9973When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 9974 99751. If primitive: it must match exactly the pattern value 99762. If a complex object: it must match (recursively) the pattern value 99773. If an array: it must match (recursively) the pattern value 9978 9979If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 9980 */ 9981 public SampledData getPatternSampledData() throws FHIRException { 9982 if (this.pattern == null) 9983 this.pattern = new SampledData(); 9984 if (!(this.pattern instanceof SampledData)) 9985 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.pattern.getClass().getName()+" was encountered"); 9986 return (SampledData) this.pattern; 9987 } 9988 9989 public boolean hasPatternSampledData() { 9990 return this != null && this.pattern instanceof SampledData; 9991 } 9992 9993 /** 9994 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 9995 9996When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 9997 9998When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 9999 10000When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10001 100021. If primitive: it must match exactly the pattern value 100032. If a complex object: it must match (recursively) the pattern value 100043. If an array: it must match (recursively) the pattern value 10005 10006If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10007 */ 10008 public Signature getPatternSignature() throws FHIRException { 10009 if (this.pattern == null) 10010 this.pattern = new Signature(); 10011 if (!(this.pattern instanceof Signature)) 10012 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10013 return (Signature) this.pattern; 10014 } 10015 10016 public boolean hasPatternSignature() { 10017 return this != null && this.pattern instanceof Signature; 10018 } 10019 10020 /** 10021 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10022 10023When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10024 10025When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10026 10027When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10028 100291. If primitive: it must match exactly the pattern value 100302. If a complex object: it must match (recursively) the pattern value 100313. If an array: it must match (recursively) the pattern value 10032 10033If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10034 */ 10035 public Timing getPatternTiming() throws FHIRException { 10036 if (this.pattern == null) 10037 this.pattern = new Timing(); 10038 if (!(this.pattern instanceof Timing)) 10039 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10040 return (Timing) this.pattern; 10041 } 10042 10043 public boolean hasPatternTiming() { 10044 return this != null && this.pattern instanceof Timing; 10045 } 10046 10047 /** 10048 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10049 10050When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10051 10052When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10053 10054When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10055 100561. If primitive: it must match exactly the pattern value 100572. If a complex object: it must match (recursively) the pattern value 100583. If an array: it must match (recursively) the pattern value 10059 10060If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10061 */ 10062 public ContactDetail getPatternContactDetail() throws FHIRException { 10063 if (this.pattern == null) 10064 this.pattern = new ContactDetail(); 10065 if (!(this.pattern instanceof ContactDetail)) 10066 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10067 return (ContactDetail) this.pattern; 10068 } 10069 10070 public boolean hasPatternContactDetail() { 10071 return this != null && this.pattern instanceof ContactDetail; 10072 } 10073 10074 /** 10075 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10076 10077When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10078 10079When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10080 10081When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10082 100831. If primitive: it must match exactly the pattern value 100842. If a complex object: it must match (recursively) the pattern value 100853. If an array: it must match (recursively) the pattern value 10086 10087If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10088 */ 10089 public DataRequirement getPatternDataRequirement() throws FHIRException { 10090 if (this.pattern == null) 10091 this.pattern = new DataRequirement(); 10092 if (!(this.pattern instanceof DataRequirement)) 10093 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10094 return (DataRequirement) this.pattern; 10095 } 10096 10097 public boolean hasPatternDataRequirement() { 10098 return this != null && this.pattern instanceof DataRequirement; 10099 } 10100 10101 /** 10102 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10103 10104When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10105 10106When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10107 10108When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10109 101101. If primitive: it must match exactly the pattern value 101112. If a complex object: it must match (recursively) the pattern value 101123. If an array: it must match (recursively) the pattern value 10113 10114If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10115 */ 10116 public Expression getPatternExpression() throws FHIRException { 10117 if (this.pattern == null) 10118 this.pattern = new Expression(); 10119 if (!(this.pattern instanceof Expression)) 10120 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10121 return (Expression) this.pattern; 10122 } 10123 10124 public boolean hasPatternExpression() { 10125 return this != null && this.pattern instanceof Expression; 10126 } 10127 10128 /** 10129 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10130 10131When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10132 10133When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10134 10135When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10136 101371. If primitive: it must match exactly the pattern value 101382. If a complex object: it must match (recursively) the pattern value 101393. If an array: it must match (recursively) the pattern value 10140 10141If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10142 */ 10143 public ParameterDefinition getPatternParameterDefinition() throws FHIRException { 10144 if (this.pattern == null) 10145 this.pattern = new ParameterDefinition(); 10146 if (!(this.pattern instanceof ParameterDefinition)) 10147 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10148 return (ParameterDefinition) this.pattern; 10149 } 10150 10151 public boolean hasPatternParameterDefinition() { 10152 return this != null && this.pattern instanceof ParameterDefinition; 10153 } 10154 10155 /** 10156 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10157 10158When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10159 10160When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10161 10162When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10163 101641. If primitive: it must match exactly the pattern value 101652. If a complex object: it must match (recursively) the pattern value 101663. If an array: it must match (recursively) the pattern value 10167 10168If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10169 */ 10170 public RelatedArtifact getPatternRelatedArtifact() throws FHIRException { 10171 if (this.pattern == null) 10172 this.pattern = new RelatedArtifact(); 10173 if (!(this.pattern instanceof RelatedArtifact)) 10174 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10175 return (RelatedArtifact) this.pattern; 10176 } 10177 10178 public boolean hasPatternRelatedArtifact() { 10179 return this != null && this.pattern instanceof RelatedArtifact; 10180 } 10181 10182 /** 10183 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10184 10185When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10186 10187When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10188 10189When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10190 101911. If primitive: it must match exactly the pattern value 101922. If a complex object: it must match (recursively) the pattern value 101933. If an array: it must match (recursively) the pattern value 10194 10195If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10196 */ 10197 public TriggerDefinition getPatternTriggerDefinition() throws FHIRException { 10198 if (this.pattern == null) 10199 this.pattern = new TriggerDefinition(); 10200 if (!(this.pattern instanceof TriggerDefinition)) 10201 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10202 return (TriggerDefinition) this.pattern; 10203 } 10204 10205 public boolean hasPatternTriggerDefinition() { 10206 return this != null && this.pattern instanceof TriggerDefinition; 10207 } 10208 10209 /** 10210 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10211 10212When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10213 10214When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10215 10216When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10217 102181. If primitive: it must match exactly the pattern value 102192. If a complex object: it must match (recursively) the pattern value 102203. If an array: it must match (recursively) the pattern value 10221 10222If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10223 */ 10224 public UsageContext getPatternUsageContext() throws FHIRException { 10225 if (this.pattern == null) 10226 this.pattern = new UsageContext(); 10227 if (!(this.pattern instanceof UsageContext)) 10228 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10229 return (UsageContext) this.pattern; 10230 } 10231 10232 public boolean hasPatternUsageContext() { 10233 return this != null && this.pattern instanceof UsageContext; 10234 } 10235 10236 /** 10237 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10238 10239When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10240 10241When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10242 10243When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10244 102451. If primitive: it must match exactly the pattern value 102462. If a complex object: it must match (recursively) the pattern value 102473. If an array: it must match (recursively) the pattern value 10248 10249If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10250 */ 10251 public Availability getPatternAvailability() throws FHIRException { 10252 if (this.pattern == null) 10253 this.pattern = new Availability(); 10254 if (!(this.pattern instanceof Availability)) 10255 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10256 return (Availability) this.pattern; 10257 } 10258 10259 public boolean hasPatternAvailability() { 10260 return this != null && this.pattern instanceof Availability; 10261 } 10262 10263 /** 10264 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10265 10266When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10267 10268When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10269 10270When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10271 102721. If primitive: it must match exactly the pattern value 102732. If a complex object: it must match (recursively) the pattern value 102743. If an array: it must match (recursively) the pattern value 10275 10276If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10277 */ 10278 public ExtendedContactDetail getPatternExtendedContactDetail() throws FHIRException { 10279 if (this.pattern == null) 10280 this.pattern = new ExtendedContactDetail(); 10281 if (!(this.pattern instanceof ExtendedContactDetail)) 10282 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10283 return (ExtendedContactDetail) this.pattern; 10284 } 10285 10286 public boolean hasPatternExtendedContactDetail() { 10287 return this != null && this.pattern instanceof ExtendedContactDetail; 10288 } 10289 10290 /** 10291 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10292 10293When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10294 10295When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10296 10297When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10298 102991. If primitive: it must match exactly the pattern value 103002. If a complex object: it must match (recursively) the pattern value 103013. If an array: it must match (recursively) the pattern value 10302 10303If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10304 */ 10305 public Dosage getPatternDosage() throws FHIRException { 10306 if (this.pattern == null) 10307 this.pattern = new Dosage(); 10308 if (!(this.pattern instanceof Dosage)) 10309 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10310 return (Dosage) this.pattern; 10311 } 10312 10313 public boolean hasPatternDosage() { 10314 return this != null && this.pattern instanceof Dosage; 10315 } 10316 10317 /** 10318 * @return {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10319 10320When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10321 10322When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10323 10324When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10325 103261. If primitive: it must match exactly the pattern value 103272. If a complex object: it must match (recursively) the pattern value 103283. If an array: it must match (recursively) the pattern value 10329 10330If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10331 */ 10332 public Meta getPatternMeta() throws FHIRException { 10333 if (this.pattern == null) 10334 this.pattern = new Meta(); 10335 if (!(this.pattern instanceof Meta)) 10336 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.pattern.getClass().getName()+" was encountered"); 10337 return (Meta) this.pattern; 10338 } 10339 10340 public boolean hasPatternMeta() { 10341 return this != null && this.pattern instanceof Meta; 10342 } 10343 10344 public boolean hasPattern() { 10345 return this.pattern != null && !this.pattern.isEmpty(); 10346 } 10347 10348 /** 10349 * @param value {@link #pattern} (Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. 10350 10351When pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly. 10352 10353When an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array. 10354 10355When pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e., 10356 103571. If primitive: it must match exactly the pattern value 103582. If a complex object: it must match (recursively) the pattern value 103593. If an array: it must match (recursively) the pattern value 10360 10361If a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.) 10362 */ 10363 public ElementDefinition setPattern(DataType value) { 10364 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 10365 throw new FHIRException("Not the right type for ElementDefinition.pattern[x]: "+value.fhirType()); 10366 this.pattern = value; 10367 return this; 10368 } 10369 10370 /** 10371 * @return {@link #example} (A sample value for this element demonstrating the type of information that would typically be found in the element.) 10372 */ 10373 public List<ElementDefinitionExampleComponent> getExample() { 10374 if (this.example == null) 10375 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 10376 return this.example; 10377 } 10378 10379 /** 10380 * @return Returns a reference to <code>this</code> for easy method chaining 10381 */ 10382 public ElementDefinition setExample(List<ElementDefinitionExampleComponent> theExample) { 10383 this.example = theExample; 10384 return this; 10385 } 10386 10387 public boolean hasExample() { 10388 if (this.example == null) 10389 return false; 10390 for (ElementDefinitionExampleComponent item : this.example) 10391 if (!item.isEmpty()) 10392 return true; 10393 return false; 10394 } 10395 10396 public ElementDefinitionExampleComponent addExample() { //3 10397 ElementDefinitionExampleComponent t = new ElementDefinitionExampleComponent(); 10398 if (this.example == null) 10399 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 10400 this.example.add(t); 10401 return t; 10402 } 10403 10404 public ElementDefinition addExample(ElementDefinitionExampleComponent t) { //3 10405 if (t == null) 10406 return this; 10407 if (this.example == null) 10408 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 10409 this.example.add(t); 10410 return this; 10411 } 10412 10413 /** 10414 * @return The first repetition of repeating field {@link #example}, creating it if it does not already exist {3} 10415 */ 10416 public ElementDefinitionExampleComponent getExampleFirstRep() { 10417 if (getExample().isEmpty()) { 10418 addExample(); 10419 } 10420 return getExample().get(0); 10421 } 10422 10423 /** 10424 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10425 */ 10426 public DataType getMinValue() { 10427 return this.minValue; 10428 } 10429 10430 /** 10431 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10432 */ 10433 public DateType getMinValueDateType() throws FHIRException { 10434 if (this.minValue == null) 10435 this.minValue = new DateType(); 10436 if (!(this.minValue instanceof DateType)) 10437 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10438 return (DateType) this.minValue; 10439 } 10440 10441 public boolean hasMinValueDateType() { 10442 return this != null && this.minValue instanceof DateType; 10443 } 10444 10445 /** 10446 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10447 */ 10448 public DateTimeType getMinValueDateTimeType() throws FHIRException { 10449 if (this.minValue == null) 10450 this.minValue = new DateTimeType(); 10451 if (!(this.minValue instanceof DateTimeType)) 10452 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10453 return (DateTimeType) this.minValue; 10454 } 10455 10456 public boolean hasMinValueDateTimeType() { 10457 return this != null && this.minValue instanceof DateTimeType; 10458 } 10459 10460 /** 10461 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10462 */ 10463 public InstantType getMinValueInstantType() throws FHIRException { 10464 if (this.minValue == null) 10465 this.minValue = new InstantType(); 10466 if (!(this.minValue instanceof InstantType)) 10467 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10468 return (InstantType) this.minValue; 10469 } 10470 10471 public boolean hasMinValueInstantType() { 10472 return this != null && this.minValue instanceof InstantType; 10473 } 10474 10475 /** 10476 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10477 */ 10478 public TimeType getMinValueTimeType() throws FHIRException { 10479 if (this.minValue == null) 10480 this.minValue = new TimeType(); 10481 if (!(this.minValue instanceof TimeType)) 10482 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10483 return (TimeType) this.minValue; 10484 } 10485 10486 public boolean hasMinValueTimeType() { 10487 return this != null && this.minValue instanceof TimeType; 10488 } 10489 10490 /** 10491 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10492 */ 10493 public DecimalType getMinValueDecimalType() throws FHIRException { 10494 if (this.minValue == null) 10495 this.minValue = new DecimalType(); 10496 if (!(this.minValue instanceof DecimalType)) 10497 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10498 return (DecimalType) this.minValue; 10499 } 10500 10501 public boolean hasMinValueDecimalType() { 10502 return this != null && this.minValue instanceof DecimalType; 10503 } 10504 10505 /** 10506 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10507 */ 10508 public IntegerType getMinValueIntegerType() throws FHIRException { 10509 if (this.minValue == null) 10510 this.minValue = new IntegerType(); 10511 if (!(this.minValue instanceof IntegerType)) 10512 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10513 return (IntegerType) this.minValue; 10514 } 10515 10516 public boolean hasMinValueIntegerType() { 10517 return this != null && this.minValue instanceof IntegerType; 10518 } 10519 10520 /** 10521 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10522 */ 10523 public Integer64Type getMinValueInteger64Type() throws FHIRException { 10524 if (this.minValue == null) 10525 this.minValue = new Integer64Type(); 10526 if (!(this.minValue instanceof Integer64Type)) 10527 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10528 return (Integer64Type) this.minValue; 10529 } 10530 10531 public boolean hasMinValueInteger64Type() { 10532 return this != null && this.minValue instanceof Integer64Type; 10533 } 10534 10535 /** 10536 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10537 */ 10538 public PositiveIntType getMinValuePositiveIntType() throws FHIRException { 10539 if (this.minValue == null) 10540 this.minValue = new PositiveIntType(); 10541 if (!(this.minValue instanceof PositiveIntType)) 10542 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10543 return (PositiveIntType) this.minValue; 10544 } 10545 10546 public boolean hasMinValuePositiveIntType() { 10547 return this != null && this.minValue instanceof PositiveIntType; 10548 } 10549 10550 /** 10551 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10552 */ 10553 public UnsignedIntType getMinValueUnsignedIntType() throws FHIRException { 10554 if (this.minValue == null) 10555 this.minValue = new UnsignedIntType(); 10556 if (!(this.minValue instanceof UnsignedIntType)) 10557 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10558 return (UnsignedIntType) this.minValue; 10559 } 10560 10561 public boolean hasMinValueUnsignedIntType() { 10562 return this != null && this.minValue instanceof UnsignedIntType; 10563 } 10564 10565 /** 10566 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10567 */ 10568 public Quantity getMinValueQuantity() throws FHIRException { 10569 if (this.minValue == null) 10570 this.minValue = new Quantity(); 10571 if (!(this.minValue instanceof Quantity)) 10572 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.minValue.getClass().getName()+" was encountered"); 10573 return (Quantity) this.minValue; 10574 } 10575 10576 public boolean hasMinValueQuantity() { 10577 return this != null && this.minValue instanceof Quantity; 10578 } 10579 10580 public boolean hasMinValue() { 10581 return this.minValue != null && !this.minValue.isEmpty(); 10582 } 10583 10584 /** 10585 * @param value {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10586 */ 10587 public ElementDefinition setMinValue(DataType value) { 10588 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 10589 throw new FHIRException("Not the right type for ElementDefinition.minValue[x]: "+value.fhirType()); 10590 this.minValue = value; 10591 return this; 10592 } 10593 10594 /** 10595 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10596 */ 10597 public DataType getMaxValue() { 10598 return this.maxValue; 10599 } 10600 10601 /** 10602 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10603 */ 10604 public DateType getMaxValueDateType() throws FHIRException { 10605 if (this.maxValue == null) 10606 this.maxValue = new DateType(); 10607 if (!(this.maxValue instanceof DateType)) 10608 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10609 return (DateType) this.maxValue; 10610 } 10611 10612 public boolean hasMaxValueDateType() { 10613 return this != null && this.maxValue instanceof DateType; 10614 } 10615 10616 /** 10617 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10618 */ 10619 public DateTimeType getMaxValueDateTimeType() throws FHIRException { 10620 if (this.maxValue == null) 10621 this.maxValue = new DateTimeType(); 10622 if (!(this.maxValue instanceof DateTimeType)) 10623 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10624 return (DateTimeType) this.maxValue; 10625 } 10626 10627 public boolean hasMaxValueDateTimeType() { 10628 return this != null && this.maxValue instanceof DateTimeType; 10629 } 10630 10631 /** 10632 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10633 */ 10634 public InstantType getMaxValueInstantType() throws FHIRException { 10635 if (this.maxValue == null) 10636 this.maxValue = new InstantType(); 10637 if (!(this.maxValue instanceof InstantType)) 10638 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10639 return (InstantType) this.maxValue; 10640 } 10641 10642 public boolean hasMaxValueInstantType() { 10643 return this != null && this.maxValue instanceof InstantType; 10644 } 10645 10646 /** 10647 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10648 */ 10649 public TimeType getMaxValueTimeType() throws FHIRException { 10650 if (this.maxValue == null) 10651 this.maxValue = new TimeType(); 10652 if (!(this.maxValue instanceof TimeType)) 10653 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10654 return (TimeType) this.maxValue; 10655 } 10656 10657 public boolean hasMaxValueTimeType() { 10658 return this != null && this.maxValue instanceof TimeType; 10659 } 10660 10661 /** 10662 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10663 */ 10664 public DecimalType getMaxValueDecimalType() throws FHIRException { 10665 if (this.maxValue == null) 10666 this.maxValue = new DecimalType(); 10667 if (!(this.maxValue instanceof DecimalType)) 10668 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10669 return (DecimalType) this.maxValue; 10670 } 10671 10672 public boolean hasMaxValueDecimalType() { 10673 return this != null && this.maxValue instanceof DecimalType; 10674 } 10675 10676 /** 10677 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10678 */ 10679 public IntegerType getMaxValueIntegerType() throws FHIRException { 10680 if (this.maxValue == null) 10681 this.maxValue = new IntegerType(); 10682 if (!(this.maxValue instanceof IntegerType)) 10683 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10684 return (IntegerType) this.maxValue; 10685 } 10686 10687 public boolean hasMaxValueIntegerType() { 10688 return this != null && this.maxValue instanceof IntegerType; 10689 } 10690 10691 /** 10692 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10693 */ 10694 public Integer64Type getMaxValueInteger64Type() throws FHIRException { 10695 if (this.maxValue == null) 10696 this.maxValue = new Integer64Type(); 10697 if (!(this.maxValue instanceof Integer64Type)) 10698 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10699 return (Integer64Type) this.maxValue; 10700 } 10701 10702 public boolean hasMaxValueInteger64Type() { 10703 return this != null && this.maxValue instanceof Integer64Type; 10704 } 10705 10706 /** 10707 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10708 */ 10709 public PositiveIntType getMaxValuePositiveIntType() throws FHIRException { 10710 if (this.maxValue == null) 10711 this.maxValue = new PositiveIntType(); 10712 if (!(this.maxValue instanceof PositiveIntType)) 10713 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10714 return (PositiveIntType) this.maxValue; 10715 } 10716 10717 public boolean hasMaxValuePositiveIntType() { 10718 return this != null && this.maxValue instanceof PositiveIntType; 10719 } 10720 10721 /** 10722 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10723 */ 10724 public UnsignedIntType getMaxValueUnsignedIntType() throws FHIRException { 10725 if (this.maxValue == null) 10726 this.maxValue = new UnsignedIntType(); 10727 if (!(this.maxValue instanceof UnsignedIntType)) 10728 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10729 return (UnsignedIntType) this.maxValue; 10730 } 10731 10732 public boolean hasMaxValueUnsignedIntType() { 10733 return this != null && this.maxValue instanceof UnsignedIntType; 10734 } 10735 10736 /** 10737 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10738 */ 10739 public Quantity getMaxValueQuantity() throws FHIRException { 10740 if (this.maxValue == null) 10741 this.maxValue = new Quantity(); 10742 if (!(this.maxValue instanceof Quantity)) 10743 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 10744 return (Quantity) this.maxValue; 10745 } 10746 10747 public boolean hasMaxValueQuantity() { 10748 return this != null && this.maxValue instanceof Quantity; 10749 } 10750 10751 public boolean hasMaxValue() { 10752 return this.maxValue != null && !this.maxValue.isEmpty(); 10753 } 10754 10755 /** 10756 * @param value {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 10757 */ 10758 public ElementDefinition setMaxValue(DataType value) { 10759 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 10760 throw new FHIRException("Not the right type for ElementDefinition.maxValue[x]: "+value.fhirType()); 10761 this.maxValue = value; 10762 return this; 10763 } 10764 10765 /** 10766 * @return {@link #maxLength} (Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 10767 */ 10768 public IntegerType getMaxLengthElement() { 10769 if (this.maxLength == null) 10770 if (Configuration.errorOnAutoCreate()) 10771 throw new Error("Attempt to auto-create ElementDefinition.maxLength"); 10772 else if (Configuration.doAutoCreate()) 10773 this.maxLength = new IntegerType(); // bb 10774 return this.maxLength; 10775 } 10776 10777 public boolean hasMaxLengthElement() { 10778 return this.maxLength != null && !this.maxLength.isEmpty(); 10779 } 10780 10781 public boolean hasMaxLength() { 10782 return this.maxLength != null && !this.maxLength.isEmpty(); 10783 } 10784 10785 /** 10786 * @param value {@link #maxLength} (Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 10787 */ 10788 public ElementDefinition setMaxLengthElement(IntegerType value) { 10789 this.maxLength = value; 10790 return this; 10791 } 10792 10793 /** 10794 * @return Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)). 10795 */ 10796 public int getMaxLength() { 10797 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 10798 } 10799 10800 /** 10801 * @param value Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)). 10802 */ 10803 public ElementDefinition setMaxLength(int value) { 10804 if (this.maxLength == null) 10805 this.maxLength = new IntegerType(); 10806 this.maxLength.setValue(value); 10807 return this; 10808 } 10809 10810 /** 10811 * @return {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10812 */ 10813 public List<IdType> getCondition() { 10814 if (this.condition == null) 10815 this.condition = new ArrayList<IdType>(); 10816 return this.condition; 10817 } 10818 10819 /** 10820 * @return Returns a reference to <code>this</code> for easy method chaining 10821 */ 10822 public ElementDefinition setCondition(List<IdType> theCondition) { 10823 this.condition = theCondition; 10824 return this; 10825 } 10826 10827 public boolean hasCondition() { 10828 if (this.condition == null) 10829 return false; 10830 for (IdType item : this.condition) 10831 if (!item.isEmpty()) 10832 return true; 10833 return false; 10834 } 10835 10836 /** 10837 * @return {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10838 */ 10839 public IdType addConditionElement() {//2 10840 IdType t = new IdType(); 10841 if (this.condition == null) 10842 this.condition = new ArrayList<IdType>(); 10843 this.condition.add(t); 10844 return t; 10845 } 10846 10847 /** 10848 * @param value {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10849 */ 10850 public ElementDefinition addCondition(String value) { //1 10851 IdType t = new IdType(); 10852 t.setValue(value); 10853 if (this.condition == null) 10854 this.condition = new ArrayList<IdType>(); 10855 this.condition.add(t); 10856 return this; 10857 } 10858 10859 /** 10860 * @param value {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 10861 */ 10862 public boolean hasCondition(String value) { 10863 if (this.condition == null) 10864 return false; 10865 for (IdType v : this.condition) 10866 if (v.getValue().equals(value)) // id 10867 return true; 10868 return false; 10869 } 10870 10871 /** 10872 * @return {@link #constraint} (Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.) 10873 */ 10874 public List<ElementDefinitionConstraintComponent> getConstraint() { 10875 if (this.constraint == null) 10876 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 10877 return this.constraint; 10878 } 10879 10880 /** 10881 * @return Returns a reference to <code>this</code> for easy method chaining 10882 */ 10883 public ElementDefinition setConstraint(List<ElementDefinitionConstraintComponent> theConstraint) { 10884 this.constraint = theConstraint; 10885 return this; 10886 } 10887 10888 public boolean hasConstraint() { 10889 if (this.constraint == null) 10890 return false; 10891 for (ElementDefinitionConstraintComponent item : this.constraint) 10892 if (!item.isEmpty()) 10893 return true; 10894 return false; 10895 } 10896 10897 public ElementDefinitionConstraintComponent addConstraint() { //3 10898 ElementDefinitionConstraintComponent t = new ElementDefinitionConstraintComponent(); 10899 if (this.constraint == null) 10900 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 10901 this.constraint.add(t); 10902 return t; 10903 } 10904 10905 public ElementDefinition addConstraint(ElementDefinitionConstraintComponent t) { //3 10906 if (t == null) 10907 return this; 10908 if (this.constraint == null) 10909 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 10910 this.constraint.add(t); 10911 return this; 10912 } 10913 10914 /** 10915 * @return The first repetition of repeating field {@link #constraint}, creating it if it does not already exist {3} 10916 */ 10917 public ElementDefinitionConstraintComponent getConstraintFirstRep() { 10918 if (getConstraint().isEmpty()) { 10919 addConstraint(); 10920 } 10921 return getConstraint().get(0); 10922 } 10923 10924 /** 10925 * @return {@link #mustHaveValue} (Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.). This is the underlying object with id, value and extensions. The accessor "getMustHaveValue" gives direct access to the value 10926 */ 10927 public BooleanType getMustHaveValueElement() { 10928 if (this.mustHaveValue == null) 10929 if (Configuration.errorOnAutoCreate()) 10930 throw new Error("Attempt to auto-create ElementDefinition.mustHaveValue"); 10931 else if (Configuration.doAutoCreate()) 10932 this.mustHaveValue = new BooleanType(); // bb 10933 return this.mustHaveValue; 10934 } 10935 10936 public boolean hasMustHaveValueElement() { 10937 return this.mustHaveValue != null && !this.mustHaveValue.isEmpty(); 10938 } 10939 10940 public boolean hasMustHaveValue() { 10941 return this.mustHaveValue != null && !this.mustHaveValue.isEmpty(); 10942 } 10943 10944 /** 10945 * @param value {@link #mustHaveValue} (Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.). This is the underlying object with id, value and extensions. The accessor "getMustHaveValue" gives direct access to the value 10946 */ 10947 public ElementDefinition setMustHaveValueElement(BooleanType value) { 10948 this.mustHaveValue = value; 10949 return this; 10950 } 10951 10952 /** 10953 * @return Specifies for a primitive data type that the value of the data type cannot be replaced by an extension. 10954 */ 10955 public boolean getMustHaveValue() { 10956 return this.mustHaveValue == null || this.mustHaveValue.isEmpty() ? false : this.mustHaveValue.getValue(); 10957 } 10958 10959 /** 10960 * @param value Specifies for a primitive data type that the value of the data type cannot be replaced by an extension. 10961 */ 10962 public ElementDefinition setMustHaveValue(boolean value) { 10963 if (this.mustHaveValue == null) 10964 this.mustHaveValue = new BooleanType(); 10965 this.mustHaveValue.setValue(value); 10966 return this; 10967 } 10968 10969 /** 10970 * @return {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 10971 */ 10972 public List<CanonicalType> getValueAlternatives() { 10973 if (this.valueAlternatives == null) 10974 this.valueAlternatives = new ArrayList<CanonicalType>(); 10975 return this.valueAlternatives; 10976 } 10977 10978 /** 10979 * @return Returns a reference to <code>this</code> for easy method chaining 10980 */ 10981 public ElementDefinition setValueAlternatives(List<CanonicalType> theValueAlternatives) { 10982 this.valueAlternatives = theValueAlternatives; 10983 return this; 10984 } 10985 10986 public boolean hasValueAlternatives() { 10987 if (this.valueAlternatives == null) 10988 return false; 10989 for (CanonicalType item : this.valueAlternatives) 10990 if (!item.isEmpty()) 10991 return true; 10992 return false; 10993 } 10994 10995 /** 10996 * @return {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 10997 */ 10998 public CanonicalType addValueAlternativesElement() {//2 10999 CanonicalType t = new CanonicalType(); 11000 if (this.valueAlternatives == null) 11001 this.valueAlternatives = new ArrayList<CanonicalType>(); 11002 this.valueAlternatives.add(t); 11003 return t; 11004 } 11005 11006 /** 11007 * @param value {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 11008 */ 11009 public ElementDefinition addValueAlternatives(String value) { //1 11010 CanonicalType t = new CanonicalType(); 11011 t.setValue(value); 11012 if (this.valueAlternatives == null) 11013 this.valueAlternatives = new ArrayList<CanonicalType>(); 11014 this.valueAlternatives.add(t); 11015 return this; 11016 } 11017 11018 /** 11019 * @param value {@link #valueAlternatives} (Specifies a list of extensions that can appear in place of a primitive value.) 11020 */ 11021 public boolean hasValueAlternatives(String value) { 11022 if (this.valueAlternatives == null) 11023 return false; 11024 for (CanonicalType v : this.valueAlternatives) 11025 if (v.getValue().equals(value)) // canonical 11026 return true; 11027 return false; 11028 } 11029 11030 /** 11031 * @return {@link #mustSupport} (If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.). This is the underlying object with id, value and extensions. The accessor "getMustSupport" gives direct access to the value 11032 */ 11033 public BooleanType getMustSupportElement() { 11034 if (this.mustSupport == null) 11035 if (Configuration.errorOnAutoCreate()) 11036 throw new Error("Attempt to auto-create ElementDefinition.mustSupport"); 11037 else if (Configuration.doAutoCreate()) 11038 this.mustSupport = new BooleanType(); // bb 11039 return this.mustSupport; 11040 } 11041 11042 public boolean hasMustSupportElement() { 11043 return this.mustSupport != null && !this.mustSupport.isEmpty(); 11044 } 11045 11046 public boolean hasMustSupport() { 11047 return this.mustSupport != null && !this.mustSupport.isEmpty(); 11048 } 11049 11050 /** 11051 * @param value {@link #mustSupport} (If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.). This is the underlying object with id, value and extensions. The accessor "getMustSupport" gives direct access to the value 11052 */ 11053 public ElementDefinition setMustSupportElement(BooleanType value) { 11054 this.mustSupport = value; 11055 return this; 11056 } 11057 11058 /** 11059 * @return If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation. 11060 */ 11061 public boolean getMustSupport() { 11062 return this.mustSupport == null || this.mustSupport.isEmpty() ? false : this.mustSupport.getValue(); 11063 } 11064 11065 /** 11066 * @param value If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation. 11067 */ 11068 public ElementDefinition setMustSupport(boolean value) { 11069 if (this.mustSupport == null) 11070 this.mustSupport = new BooleanType(); 11071 this.mustSupport.setValue(value); 11072 return this; 11073 } 11074 11075 /** 11076 * @return {@link #isModifier} (If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.). This is the underlying object with id, value and extensions. The accessor "getIsModifier" gives direct access to the value 11077 */ 11078 public BooleanType getIsModifierElement() { 11079 if (this.isModifier == null) 11080 if (Configuration.errorOnAutoCreate()) 11081 throw new Error("Attempt to auto-create ElementDefinition.isModifier"); 11082 else if (Configuration.doAutoCreate()) 11083 this.isModifier = new BooleanType(); // bb 11084 return this.isModifier; 11085 } 11086 11087 public boolean hasIsModifierElement() { 11088 return this.isModifier != null && !this.isModifier.isEmpty(); 11089 } 11090 11091 public boolean hasIsModifier() { 11092 return this.isModifier != null && !this.isModifier.isEmpty(); 11093 } 11094 11095 /** 11096 * @param value {@link #isModifier} (If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.). This is the underlying object with id, value and extensions. The accessor "getIsModifier" gives direct access to the value 11097 */ 11098 public ElementDefinition setIsModifierElement(BooleanType value) { 11099 this.isModifier = value; 11100 return this; 11101 } 11102 11103 /** 11104 * @return If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension. 11105 */ 11106 public boolean getIsModifier() { 11107 return this.isModifier == null || this.isModifier.isEmpty() ? false : this.isModifier.getValue(); 11108 } 11109 11110 /** 11111 * @param value If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension. 11112 */ 11113 public ElementDefinition setIsModifier(boolean value) { 11114 if (this.isModifier == null) 11115 this.isModifier = new BooleanType(); 11116 this.isModifier.setValue(value); 11117 return this; 11118 } 11119 11120 /** 11121 * @return {@link #isModifierReason} (Explains how that element affects the interpretation of the resource or element that contains it.). This is the underlying object with id, value and extensions. The accessor "getIsModifierReason" gives direct access to the value 11122 */ 11123 public StringType getIsModifierReasonElement() { 11124 if (this.isModifierReason == null) 11125 if (Configuration.errorOnAutoCreate()) 11126 throw new Error("Attempt to auto-create ElementDefinition.isModifierReason"); 11127 else if (Configuration.doAutoCreate()) 11128 this.isModifierReason = new StringType(); // bb 11129 return this.isModifierReason; 11130 } 11131 11132 public boolean hasIsModifierReasonElement() { 11133 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 11134 } 11135 11136 public boolean hasIsModifierReason() { 11137 return this.isModifierReason != null && !this.isModifierReason.isEmpty(); 11138 } 11139 11140 /** 11141 * @param value {@link #isModifierReason} (Explains how that element affects the interpretation of the resource or element that contains it.). This is the underlying object with id, value and extensions. The accessor "getIsModifierReason" gives direct access to the value 11142 */ 11143 public ElementDefinition setIsModifierReasonElement(StringType value) { 11144 this.isModifierReason = value; 11145 return this; 11146 } 11147 11148 /** 11149 * @return Explains how that element affects the interpretation of the resource or element that contains it. 11150 */ 11151 public String getIsModifierReason() { 11152 return this.isModifierReason == null ? null : this.isModifierReason.getValue(); 11153 } 11154 11155 /** 11156 * @param value Explains how that element affects the interpretation of the resource or element that contains it. 11157 */ 11158 public ElementDefinition setIsModifierReason(String value) { 11159 if (Utilities.noString(value)) 11160 this.isModifierReason = null; 11161 else { 11162 if (this.isModifierReason == null) 11163 this.isModifierReason = new StringType(); 11164 this.isModifierReason.setValue(value); 11165 } 11166 return this; 11167 } 11168 11169 /** 11170 * @return {@link #isSummary} (Whether the element should be included if a client requests a search with the parameter _summary=true.). This is the underlying object with id, value and extensions. The accessor "getIsSummary" gives direct access to the value 11171 */ 11172 public BooleanType getIsSummaryElement() { 11173 if (this.isSummary == null) 11174 if (Configuration.errorOnAutoCreate()) 11175 throw new Error("Attempt to auto-create ElementDefinition.isSummary"); 11176 else if (Configuration.doAutoCreate()) 11177 this.isSummary = new BooleanType(); // bb 11178 return this.isSummary; 11179 } 11180 11181 public boolean hasIsSummaryElement() { 11182 return this.isSummary != null && !this.isSummary.isEmpty(); 11183 } 11184 11185 public boolean hasIsSummary() { 11186 return this.isSummary != null && !this.isSummary.isEmpty(); 11187 } 11188 11189 /** 11190 * @param value {@link #isSummary} (Whether the element should be included if a client requests a search with the parameter _summary=true.). This is the underlying object with id, value and extensions. The accessor "getIsSummary" gives direct access to the value 11191 */ 11192 public ElementDefinition setIsSummaryElement(BooleanType value) { 11193 this.isSummary = value; 11194 return this; 11195 } 11196 11197 /** 11198 * @return Whether the element should be included if a client requests a search with the parameter _summary=true. 11199 */ 11200 public boolean getIsSummary() { 11201 return this.isSummary == null || this.isSummary.isEmpty() ? false : this.isSummary.getValue(); 11202 } 11203 11204 /** 11205 * @param value Whether the element should be included if a client requests a search with the parameter _summary=true. 11206 */ 11207 public ElementDefinition setIsSummary(boolean value) { 11208 if (this.isSummary == null) 11209 this.isSummary = new BooleanType(); 11210 this.isSummary.setValue(value); 11211 return this; 11212 } 11213 11214 /** 11215 * @return {@link #binding} (Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).) 11216 */ 11217 public ElementDefinitionBindingComponent getBinding() { 11218 if (this.binding == null) 11219 if (Configuration.errorOnAutoCreate()) 11220 throw new Error("Attempt to auto-create ElementDefinition.binding"); 11221 else if (Configuration.doAutoCreate()) 11222 this.binding = new ElementDefinitionBindingComponent(); // cc 11223 return this.binding; 11224 } 11225 11226 public boolean hasBinding() { 11227 return this.binding != null && !this.binding.isEmpty(); 11228 } 11229 11230 /** 11231 * @param value {@link #binding} (Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).) 11232 */ 11233 public ElementDefinition setBinding(ElementDefinitionBindingComponent value) { 11234 this.binding = value; 11235 return this; 11236 } 11237 11238 /** 11239 * @return {@link #mapping} (Identifies a concept from an external specification that roughly corresponds to this element.) 11240 */ 11241 public List<ElementDefinitionMappingComponent> getMapping() { 11242 if (this.mapping == null) 11243 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 11244 return this.mapping; 11245 } 11246 11247 /** 11248 * @return Returns a reference to <code>this</code> for easy method chaining 11249 */ 11250 public ElementDefinition setMapping(List<ElementDefinitionMappingComponent> theMapping) { 11251 this.mapping = theMapping; 11252 return this; 11253 } 11254 11255 public boolean hasMapping() { 11256 if (this.mapping == null) 11257 return false; 11258 for (ElementDefinitionMappingComponent item : this.mapping) 11259 if (!item.isEmpty()) 11260 return true; 11261 return false; 11262 } 11263 11264 public ElementDefinitionMappingComponent addMapping() { //3 11265 ElementDefinitionMappingComponent t = new ElementDefinitionMappingComponent(); 11266 if (this.mapping == null) 11267 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 11268 this.mapping.add(t); 11269 return t; 11270 } 11271 11272 public ElementDefinition addMapping(ElementDefinitionMappingComponent t) { //3 11273 if (t == null) 11274 return this; 11275 if (this.mapping == null) 11276 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 11277 this.mapping.add(t); 11278 return this; 11279 } 11280 11281 /** 11282 * @return The first repetition of repeating field {@link #mapping}, creating it if it does not already exist {3} 11283 */ 11284 public ElementDefinitionMappingComponent getMappingFirstRep() { 11285 if (getMapping().isEmpty()) { 11286 addMapping(); 11287 } 11288 return getMapping().get(0); 11289 } 11290 11291 protected void listChildren(List<Property> children) { 11292 super.listChildren(children); 11293 children.add(new Property("path", "string", "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 0, 1, path)); 11294 children.add(new Property("representation", "code", "Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.", 0, java.lang.Integer.MAX_VALUE, representation)); 11295 children.add(new Property("sliceName", "string", "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 0, 1, sliceName)); 11296 children.add(new Property("sliceIsConstraining", "boolean", "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 0, 1, sliceIsConstraining)); 11297 children.add(new Property("label", "string", "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 0, 1, label)); 11298 children.add(new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 0, java.lang.Integer.MAX_VALUE, code)); 11299 children.add(new Property("slicing", "", "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 0, 1, slicing)); 11300 children.add(new Property("short", "string", "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_)); 11301 children.add(new Property("definition", "markdown", "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 0, 1, definition)); 11302 children.add(new Property("comment", "markdown", "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 0, 1, comment)); 11303 children.add(new Property("requirements", "markdown", "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 0, 1, requirements)); 11304 children.add(new Property("alias", "string", "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, alias)); 11305 children.add(new Property("min", "unsignedInt", "The minimum number of times this element SHALL appear in the instance.", 0, 1, min)); 11306 children.add(new Property("max", "string", "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max)); 11307 children.add(new Property("base", "", "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 0, 1, base)); 11308 children.add(new Property("contentReference", "uri", "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 0, 1, contentReference)); 11309 children.add(new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, java.lang.Integer.MAX_VALUE, type)); 11310 children.add(new Property("defaultValue[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue)); 11311 children.add(new Property("meaningWhenMissing", "markdown", "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 0, 1, meaningWhenMissing)); 11312 children.add(new Property("orderMeaning", "string", "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 0, 1, orderMeaning)); 11313 children.add(new Property("fixed[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed)); 11314 children.add(new Property("pattern[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern)); 11315 children.add(new Property("example", "", "A sample value for this element demonstrating the type of information that would typically be found in the element.", 0, java.lang.Integer.MAX_VALUE, example)); 11316 children.add(new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue)); 11317 children.add(new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue)); 11318 children.add(new Property("maxLength", "integer", "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).", 0, 1, maxLength)); 11319 children.add(new Property("condition", "id", "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 0, java.lang.Integer.MAX_VALUE, condition)); 11320 children.add(new Property("constraint", "", "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 0, java.lang.Integer.MAX_VALUE, constraint)); 11321 children.add(new Property("mustHaveValue", "boolean", "Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.", 0, 1, mustHaveValue)); 11322 children.add(new Property("valueAlternatives", "canonical(StructureDefinition)", "Specifies a list of extensions that can appear in place of a primitive value.", 0, java.lang.Integer.MAX_VALUE, valueAlternatives)); 11323 children.add(new Property("mustSupport", "boolean", "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 0, 1, mustSupport)); 11324 children.add(new Property("isModifier", "boolean", "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.", 0, 1, isModifier)); 11325 children.add(new Property("isModifierReason", "string", "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, isModifierReason)); 11326 children.add(new Property("isSummary", "boolean", "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, isSummary)); 11327 children.add(new Property("binding", "", "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 0, 1, binding)); 11328 children.add(new Property("mapping", "", "Identifies a concept from an external specification that roughly corresponds to this element.", 0, java.lang.Integer.MAX_VALUE, mapping)); 11329 } 11330 11331 @Override 11332 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11333 switch (_hash) { 11334 case 3433509: /*path*/ return new Property("path", "string", "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 0, 1, path); 11335 case -671065907: /*representation*/ return new Property("representation", "code", "Codes that define how this element is represented in instances, when the deviation varies from the normal case. No extensions are allowed on elements with a representation of 'xmlAttr', no matter what FHIR serialization format is used.", 0, java.lang.Integer.MAX_VALUE, representation); 11336 case -825289923: /*sliceName*/ return new Property("sliceName", "string", "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 0, 1, sliceName); 11337 case 333040519: /*sliceIsConstraining*/ return new Property("sliceIsConstraining", "boolean", "If true, indicates that this slice definition is constraining a slice definition with the same name in an inherited profile. If false, the slice is not overriding any slice in an inherited profile. If missing, the slice might or might not be overriding a slice in an inherited profile, depending on the sliceName.", 0, 1, sliceIsConstraining); 11338 case 102727412: /*label*/ return new Property("label", "string", "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 0, 1, label); 11339 case 3059181: /*code*/ return new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 0, java.lang.Integer.MAX_VALUE, code); 11340 case -2119287345: /*slicing*/ return new Property("slicing", "", "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 0, 1, slicing); 11341 case 109413500: /*short*/ return new Property("short", "string", "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_); 11342 case -1014418093: /*definition*/ return new Property("definition", "markdown", "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. (Note: The text you are reading is specified in ElementDefinition.definition).", 0, 1, definition); 11343 case 950398559: /*comment*/ return new Property("comment", "markdown", "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. (Note: The text you are reading is specified in ElementDefinition.comment).", 0, 1, comment); 11344 case -1619874672: /*requirements*/ return new Property("requirements", "markdown", "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 0, 1, requirements); 11345 case 92902992: /*alias*/ return new Property("alias", "string", "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, alias); 11346 case 108114: /*min*/ return new Property("min", "unsignedInt", "The minimum number of times this element SHALL appear in the instance.", 0, 1, min); 11347 case 107876: /*max*/ return new Property("max", "string", "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max); 11348 case 3016401: /*base*/ return new Property("base", "", "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. When the element definition is not the original definition of an element - e.g. either in a constraint on another type, or for elements from a super type in a snap shot - then the information in provided in the element definition may be different to the base definition. On the original definition of the element, it will be same.", 0, 1, base); 11349 case 1193747154: /*contentReference*/ return new Property("contentReference", "uri", "Identifies an element defined elsewhere in the definition whose content rules should be applied to the current element. ContentReferences bring across all the rules that are in the ElementDefinition for the element, including definitions, cardinality constraints, bindings, invariants etc.", 0, 1, contentReference); 11350 case 3575610: /*type*/ return new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, java.lang.Integer.MAX_VALUE, type); 11351 case 587922128: /*defaultValue[x]*/ return new Property("defaultValue[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11352 case -659125328: /*defaultValue*/ return new Property("defaultValue[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11353 case 1470297600: /*defaultValueBase64Binary*/ return new Property("defaultValue[x]", "base64Binary", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11354 case 600437336: /*defaultValueBoolean*/ return new Property("defaultValue[x]", "boolean", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11355 case 264593188: /*defaultValueCanonical*/ return new Property("defaultValue[x]", "canonical", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11356 case 1044993469: /*defaultValueCode*/ return new Property("defaultValue[x]", "code", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11357 case 1045010302: /*defaultValueDate*/ return new Property("defaultValue[x]", "date", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11358 case 1220374379: /*defaultValueDateTime*/ return new Property("defaultValue[x]", "dateTime", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11359 case 2077989249: /*defaultValueDecimal*/ return new Property("defaultValue[x]", "decimal", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11360 case -2059245333: /*defaultValueId*/ return new Property("defaultValue[x]", "id", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11361 case -1801671663: /*defaultValueInstant*/ return new Property("defaultValue[x]", "instant", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11362 case -1801189522: /*defaultValueInteger*/ return new Property("defaultValue[x]", "integer", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11363 case -71308628: /*defaultValueInteger64*/ return new Property("defaultValue[x]", "integer64", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11364 case -325436225: /*defaultValueMarkdown*/ return new Property("defaultValue[x]", "markdown", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11365 case 587910138: /*defaultValueOid*/ return new Property("defaultValue[x]", "oid", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11366 case -737344154: /*defaultValuePositiveInt*/ return new Property("defaultValue[x]", "positiveInt", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11367 case -320515103: /*defaultValueString*/ return new Property("defaultValue[x]", "string", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11368 case 1045494429: /*defaultValueTime*/ return new Property("defaultValue[x]", "time", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11369 case 539117290: /*defaultValueUnsignedInt*/ return new Property("defaultValue[x]", "unsignedInt", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11370 case 587916188: /*defaultValueUri*/ return new Property("defaultValue[x]", "uri", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11371 case 587916191: /*defaultValueUrl*/ return new Property("defaultValue[x]", "url", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11372 case 1045535627: /*defaultValueUuid*/ return new Property("defaultValue[x]", "uuid", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11373 case -611966428: /*defaultValueAddress*/ return new Property("defaultValue[x]", "Address", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11374 case 587896623: /*defaultValueAge*/ return new Property("defaultValue[x]", "Age", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11375 case -1851689217: /*defaultValueAnnotation*/ return new Property("defaultValue[x]", "Annotation", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11376 case 2034820339: /*defaultValueAttachment*/ return new Property("defaultValue[x]", "Attachment", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11377 case -410434095: /*defaultValueCodeableConcept*/ return new Property("defaultValue[x]", "CodeableConcept", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11378 case 678417524: /*defaultValueCodeableReference*/ return new Property("defaultValue[x]", "CodeableReference", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11379 case -783616198: /*defaultValueCoding*/ return new Property("defaultValue[x]", "Coding", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11380 case -344740576: /*defaultValueContactPoint*/ return new Property("defaultValue[x]", "ContactPoint", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11381 case -1964924097: /*defaultValueCount*/ return new Property("defaultValue[x]", "Count", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11382 case -283915323: /*defaultValueDistance*/ return new Property("defaultValue[x]", "Distance", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11383 case 1730579812: /*defaultValueDuration*/ return new Property("defaultValue[x]", "Duration", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11384 case -975393912: /*defaultValueHumanName*/ return new Property("defaultValue[x]", "HumanName", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11385 case -1915078535: /*defaultValueIdentifier*/ return new Property("defaultValue[x]", "Identifier", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11386 case -1955695888: /*defaultValueMoney*/ return new Property("defaultValue[x]", "Money", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11387 case -420255343: /*defaultValuePeriod*/ return new Property("defaultValue[x]", "Period", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11388 case -1857379237: /*defaultValueQuantity*/ return new Property("defaultValue[x]", "Quantity", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11389 case -1951495315: /*defaultValueRange*/ return new Property("defaultValue[x]", "Range", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11390 case -1951489477: /*defaultValueRatio*/ return new Property("defaultValue[x]", "Ratio", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11391 case 1803932610: /*defaultValueRatioRange*/ return new Property("defaultValue[x]", "RatioRange", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11392 case -1488914053: /*defaultValueReference*/ return new Property("defaultValue[x]", "Reference", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11393 case -449641228: /*defaultValueSampledData*/ return new Property("defaultValue[x]", "SampledData", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11394 case 509825768: /*defaultValueSignature*/ return new Property("defaultValue[x]", "Signature", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11395 case -302193638: /*defaultValueTiming*/ return new Property("defaultValue[x]", "Timing", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11396 case 1845473985: /*defaultValueContactDetail*/ return new Property("defaultValue[x]", "ContactDetail", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11397 case 375217257: /*defaultValueDataRequirement*/ return new Property("defaultValue[x]", "DataRequirement", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11398 case -2092097944: /*defaultValueExpression*/ return new Property("defaultValue[x]", "Expression", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11399 case -701053940: /*defaultValueParameterDefinition*/ return new Property("defaultValue[x]", "ParameterDefinition", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11400 case 412877133: /*defaultValueRelatedArtifact*/ return new Property("defaultValue[x]", "RelatedArtifact", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11401 case 1913203547: /*defaultValueTriggerDefinition*/ return new Property("defaultValue[x]", "TriggerDefinition", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11402 case -701644642: /*defaultValueUsageContext*/ return new Property("defaultValue[x]", "UsageContext", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11403 case 388885803: /*defaultValueAvailability*/ return new Property("defaultValue[x]", "Availability", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11404 case 1398098440: /*defaultValueExtendedContactDetail*/ return new Property("defaultValue[x]", "ExtendedContactDetail", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11405 case -754548089: /*defaultValueDosage*/ return new Property("defaultValue[x]", "Dosage", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11406 case 1045282261: /*defaultValueMeta*/ return new Property("defaultValue[x]", "Meta", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 11407 case 1857257103: /*meaningWhenMissing*/ return new Property("meaningWhenMissing", "markdown", "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing').", 0, 1, meaningWhenMissing); 11408 case 1828196047: /*orderMeaning*/ return new Property("orderMeaning", "string", "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 0, 1, orderMeaning); 11409 case -391522164: /*fixed[x]*/ return new Property("fixed[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11410 case 97445748: /*fixed*/ return new Property("fixed[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11411 case -799290428: /*fixedBase64Binary*/ return new Property("fixed[x]", "base64Binary", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11412 case 520851988: /*fixedBoolean*/ return new Property("fixed[x]", "boolean", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11413 case 1092485088: /*fixedCanonical*/ return new Property("fixed[x]", "canonical", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11414 case 746991489: /*fixedCode*/ return new Property("fixed[x]", "code", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11415 case 747008322: /*fixedDate*/ return new Property("fixed[x]", "date", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11416 case -1246771409: /*fixedDateTime*/ return new Property("fixed[x]", "dateTime", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11417 case 1998403901: /*fixedDecimal*/ return new Property("fixed[x]", "decimal", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11418 case -843914321: /*fixedId*/ return new Property("fixed[x]", "id", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11419 case -1881257011: /*fixedInstant*/ return new Property("fixed[x]", "instant", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11420 case -1880774870: /*fixedInteger*/ return new Property("fixed[x]", "integer", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11421 case 756583272: /*fixedInteger64*/ return new Property("fixed[x]", "integer64", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11422 case 1502385283: /*fixedMarkdown*/ return new Property("fixed[x]", "markdown", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11423 case -391534154: /*fixedOid*/ return new Property("fixed[x]", "oid", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11424 case 297821986: /*fixedPositiveInt*/ return new Property("fixed[x]", "positiveInt", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11425 case 1062390949: /*fixedString*/ return new Property("fixed[x]", "string", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11426 case 747492449: /*fixedTime*/ return new Property("fixed[x]", "time", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11427 case 1574283430: /*fixedUnsignedInt*/ return new Property("fixed[x]", "unsignedInt", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11428 case -391528104: /*fixedUri*/ return new Property("fixed[x]", "uri", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11429 case -391528101: /*fixedUrl*/ return new Property("fixed[x]", "url", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11430 case 747533647: /*fixedUuid*/ return new Property("fixed[x]", "uuid", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11431 case -691551776: /*fixedAddress*/ return new Property("fixed[x]", "Address", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11432 case -391547669: /*fixedAge*/ return new Property("fixed[x]", "Age", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11433 case -1956844093: /*fixedAnnotation*/ return new Property("fixed[x]", "Annotation", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11434 case 1929665463: /*fixedAttachment*/ return new Property("fixed[x]", "Attachment", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11435 case 1962764685: /*fixedCodeableConcept*/ return new Property("fixed[x]", "CodeableConcept", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11436 case 694810928: /*fixedCodeableReference*/ return new Property("fixed[x]", "CodeableReference", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11437 case 599289854: /*fixedCoding*/ return new Property("fixed[x]", "Coding", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11438 case 1680638692: /*fixedContactPoint*/ return new Property("fixed[x]", "ContactPoint", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11439 case 1681916411: /*fixedCount*/ return new Property("fixed[x]", "Count", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11440 case 1543906185: /*fixedDistance*/ return new Property("fixed[x]", "Distance", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11441 case -736565976: /*fixedDuration*/ return new Property("fixed[x]", "Duration", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11442 case -147502012: /*fixedHumanName*/ return new Property("fixed[x]", "HumanName", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11443 case -2020233411: /*fixedIdentifier*/ return new Property("fixed[x]", "Identifier", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11444 case 1691144620: /*fixedMoney*/ return new Property("fixed[x]", "Money", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11445 case 962650709: /*fixedPeriod*/ return new Property("fixed[x]", "Period", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11446 case -29557729: /*fixedQuantity*/ return new Property("fixed[x]", "Quantity", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11447 case 1695345193: /*fixedRange*/ return new Property("fixed[x]", "Range", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11448 case 1695351031: /*fixedRatio*/ return new Property("fixed[x]", "Ratio", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11449 case 1698777734: /*fixedRatioRange*/ return new Property("fixed[x]", "RatioRange", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11450 case -661022153: /*fixedReference*/ return new Property("fixed[x]", "Reference", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11451 case 585524912: /*fixedSampledData*/ return new Property("fixed[x]", "SampledData", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11452 case 1337717668: /*fixedSignature*/ return new Property("fixed[x]", "Signature", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11453 case 1080712414: /*fixedTiming*/ return new Property("fixed[x]", "Timing", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11454 case 207721853: /*fixedContactDetail*/ return new Property("fixed[x]", "ContactDetail", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11455 case -1546551259: /*fixedDataRequirement*/ return new Property("fixed[x]", "DataRequirement", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11456 case 2097714476: /*fixedExpression*/ return new Property("fixed[x]", "Expression", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11457 case -2126861880: /*fixedParameterDefinition*/ return new Property("fixed[x]", "ParameterDefinition", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11458 case -1508891383: /*fixedRelatedArtifact*/ return new Property("fixed[x]", "RelatedArtifact", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11459 case 1929596951: /*fixedTriggerDefinition*/ return new Property("fixed[x]", "TriggerDefinition", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11460 case 1323734626: /*fixedUsageContext*/ return new Property("fixed[x]", "UsageContext", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11461 case -1880702225: /*fixedAvailability*/ return new Property("fixed[x]", "Availability", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11462 case 1291235524: /*fixedExtendedContactDetail*/ return new Property("fixed[x]", "ExtendedContactDetail", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11463 case 628357963: /*fixedDosage*/ return new Property("fixed[x]", "Dosage", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11464 case 747280281: /*fixedMeta*/ return new Property("fixed[x]", "Meta", "Specifies a value that SHALL be exactly the value for this element in the instance, if present. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 11465 case -885125392: /*pattern[x]*/ return new Property("pattern[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11466 case -791090288: /*pattern*/ return new Property("pattern[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11467 case 2127857120: /*patternBase64Binary*/ return new Property("pattern[x]", "base64Binary", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11468 case -1776945544: /*patternBoolean*/ return new Property("pattern[x]", "boolean", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11469 case 522246980: /*patternCanonical*/ return new Property("pattern[x]", "canonical", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11470 case -1669806691: /*patternCode*/ return new Property("pattern[x]", "code", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11471 case -1669789858: /*patternDate*/ return new Property("pattern[x]", "date", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11472 case 535949131: /*patternDateTime*/ return new Property("pattern[x]", "dateTime", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11473 case -299393631: /*patternDecimal*/ return new Property("pattern[x]", "decimal", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11474 case -28553013: /*patternId*/ return new Property("pattern[x]", "id", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11475 case 115912753: /*patternInstant*/ return new Property("pattern[x]", "instant", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11476 case 116394894: /*patternInteger*/ return new Property("pattern[x]", "integer", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11477 case 186345164: /*patternInteger64*/ return new Property("pattern[x]", "integer64", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11478 case -1009861473: /*patternMarkdown*/ return new Property("pattern[x]", "markdown", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11479 case -885137382: /*patternOid*/ return new Property("pattern[x]", "oid", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11480 case 2054814086: /*patternPositiveInt*/ return new Property("pattern[x]", "positiveInt", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11481 case 2096647105: /*patternString*/ return new Property("pattern[x]", "string", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11482 case -1669305731: /*patternTime*/ return new Property("pattern[x]", "time", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11483 case -963691766: /*patternUnsignedInt*/ return new Property("pattern[x]", "unsignedInt", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11484 case -885131332: /*patternUri*/ return new Property("pattern[x]", "uri", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11485 case -885131329: /*patternUrl*/ return new Property("pattern[x]", "url", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11486 case -1669264533: /*patternUuid*/ return new Property("pattern[x]", "uuid", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11487 case 1305617988: /*patternAddress*/ return new Property("pattern[x]", "Address", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11488 case -885150897: /*patternAge*/ return new Property("pattern[x]", "Age", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11489 case 1840611039: /*patternAnnotation*/ return new Property("pattern[x]", "Annotation", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11490 case 1432153299: /*patternAttachment*/ return new Property("pattern[x]", "Attachment", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11491 case -400610831: /*patternCodeableConcept*/ return new Property("pattern[x]", "CodeableConcept", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11492 case 1528639636: /*patternCodeableReference*/ return new Property("pattern[x]", "CodeableReference", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11493 case 1633546010: /*patternCoding*/ return new Property("pattern[x]", "Coding", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11494 case 312818944: /*patternContactPoint*/ return new Property("pattern[x]", "ContactPoint", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11495 case -224383137: /*patternCount*/ return new Property("pattern[x]", "Count", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11496 case -968340571: /*patternDistance*/ return new Property("pattern[x]", "Distance", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11497 case 1046154564: /*patternDuration*/ return new Property("pattern[x]", "Duration", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11498 case -717740120: /*patternHumanName*/ return new Property("pattern[x]", "HumanName", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11499 case 1777221721: /*patternIdentifier*/ return new Property("pattern[x]", "Identifier", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11500 case -215154928: /*patternMoney*/ return new Property("pattern[x]", "Money", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11501 case 1996906865: /*patternPeriod*/ return new Property("pattern[x]", "Period", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11502 case 1753162811: /*patternQuantity*/ return new Property("pattern[x]", "Quantity", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11503 case -210954355: /*patternRange*/ return new Property("pattern[x]", "Range", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11504 case -210948517: /*patternRatio*/ return new Property("pattern[x]", "Ratio", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11505 case 1201265570: /*patternRatioRange*/ return new Property("pattern[x]", "RatioRange", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11506 case -1231260261: /*patternReference*/ return new Property("pattern[x]", "Reference", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11507 case -1952450284: /*patternSampledData*/ return new Property("pattern[x]", "SampledData", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11508 case 767479560: /*patternSignature*/ return new Property("pattern[x]", "Signature", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11509 case 2114968570: /*patternTiming*/ return new Property("pattern[x]", "Timing", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11510 case 754982625: /*patternContactDetail*/ return new Property("pattern[x]", "ContactDetail", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11511 case 385040521: /*patternDataRequirement*/ return new Property("pattern[x]", "DataRequirement", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11512 case 1600202312: /*patternExpression*/ return new Property("pattern[x]", "Expression", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11513 case 318609452: /*patternParameterDefinition*/ return new Property("pattern[x]", "ParameterDefinition", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11514 case 422700397: /*patternRelatedArtifact*/ return new Property("pattern[x]", "RelatedArtifact", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11515 case -1531541637: /*patternTriggerDefinition*/ return new Property("pattern[x]", "TriggerDefinition", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11516 case -44085122: /*patternUsageContext*/ return new Property("pattern[x]", "UsageContext", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11517 case 1046445323: /*patternAvailability*/ return new Property("pattern[x]", "Availability", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11518 case 2042074664: /*patternExtendedContactDetail*/ return new Property("pattern[x]", "ExtendedContactDetail", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11519 case 1662614119: /*patternDosage*/ return new Property("pattern[x]", "Dosage", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11520 case -1669517899: /*patternMeta*/ return new Property("pattern[x]", "Meta", "Specifies a value that each occurrence of the element in the instance SHALL follow - that is, any value in the pattern must be found in the instance, if the element has a value. Other additional values may be found too. This is effectively constraint by example. \n\nWhen pattern[x] is used to constrain a primitive, it means that the value provided in the pattern[x] must match the instance value exactly.\n\nWhen an element within a pattern[x] is used to constrain an array, it means that each element provided in the pattern[x] must (recursively) match at least one element from the instance array.\n\nWhen pattern[x] is used to constrain a complex object, it means that each property in the pattern must be present in the complex object, and its value must recursively match -- i.e.,\n\n1. If primitive: it must match exactly the pattern value\n2. If a complex object: it must match (recursively) the pattern value\n3. If an array: it must match (recursively) the pattern value\n\nIf a pattern[x] is declared on a repeating element, the pattern applies to all repetitions. If the desire is for a pattern to apply to only one element or a subset of elements, slicing must be used. See [Examples of Patterns](elementdefinition-examples.html#pattern-examples) for examples of pattern usage and the effect it will have.", 0, 1, pattern); 11521 case -1322970774: /*example*/ return new Property("example", "", "A sample value for this element demonstrating the type of information that would typically be found in the element.", 0, java.lang.Integer.MAX_VALUE, example); 11522 case -55301663: /*minValue[x]*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11523 case -1376969153: /*minValue*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11524 case -1715058035: /*minValueDate*/ return new Property("minValue[x]", "date", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11525 case 1635517178: /*minValueDateTime*/ return new Property("minValue[x]", "dateTime", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11526 case 151382690: /*minValueInstant*/ return new Property("minValue[x]", "instant", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11527 case -1714573908: /*minValueTime*/ return new Property("minValue[x]", "time", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11528 case -263923694: /*minValueDecimal*/ return new Property("minValue[x]", "decimal", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11529 case 151864831: /*minValueInteger*/ return new Property("minValue[x]", "integer", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11530 case -86783747: /*minValueInteger64*/ return new Property("minValue[x]", "integer64", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11531 case 1570935671: /*minValuePositiveInt*/ return new Property("minValue[x]", "positiveInt", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11532 case -1447570181: /*minValueUnsignedInt*/ return new Property("minValue[x]", "unsignedInt", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11533 case -1442236438: /*minValueQuantity*/ return new Property("minValue[x]", "Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 11534 case 622130931: /*maxValue[x]*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11535 case 399227501: /*maxValue*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|integer64|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11536 case 2105483195: /*maxValueDate*/ return new Property("maxValue[x]", "date", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11537 case 1699385640: /*maxValueDateTime*/ return new Property("maxValue[x]", "dateTime", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11538 case 1261821620: /*maxValueInstant*/ return new Property("maxValue[x]", "instant", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11539 case 2105967322: /*maxValueTime*/ return new Property("maxValue[x]", "time", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11540 case 846515236: /*maxValueDecimal*/ return new Property("maxValue[x]", "decimal", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11541 case 1262303761: /*maxValueInteger*/ return new Property("maxValue[x]", "integer", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11542 case 1893138575: /*maxValueInteger64*/ return new Property("maxValue[x]", "integer64", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11543 case 1605774985: /*maxValuePositiveInt*/ return new Property("maxValue[x]", "positiveInt", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11544 case -1412730867: /*maxValueUnsignedInt*/ return new Property("maxValue[x]", "unsignedInt", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11545 case -1378367976: /*maxValueQuantity*/ return new Property("maxValue[x]", "Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 11546 case -791400086: /*maxLength*/ return new Property("maxLength", "integer", "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. ```maxLength``` SHOULD only be used on primitive data types that have a string representation (see [http://hl7.org/fhir/StructureDefinition/structuredefinition-type-characteristics](https://build.fhir.org/ig/HL7/fhir-extensions/StructureDefinition-structuredefinition-type-characteristics.html)).", 0, 1, maxLength); 11547 case -861311717: /*condition*/ return new Property("condition", "id", "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 0, java.lang.Integer.MAX_VALUE, condition); 11548 case -190376483: /*constraint*/ return new Property("constraint", "", "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 0, java.lang.Integer.MAX_VALUE, constraint); 11549 case -923694880: /*mustHaveValue*/ return new Property("mustHaveValue", "boolean", "Specifies for a primitive data type that the value of the data type cannot be replaced by an extension.", 0, 1, mustHaveValue); 11550 case -2124672393: /*valueAlternatives*/ return new Property("valueAlternatives", "canonical(StructureDefinition)", "Specifies a list of extensions that can appear in place of a primitive value.", 0, java.lang.Integer.MAX_VALUE, valueAlternatives); 11551 case -1402857082: /*mustSupport*/ return new Property("mustSupport", "boolean", "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. Note that this is being phased out and replaced by obligations (see below). If false, the element may be ignored and not supported. If false, whether to populate or use the data element in any way is at the discretion of the implementation.", 0, 1, mustSupport); 11552 case -1408783839: /*isModifier*/ return new Property("isModifier", "boolean", "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. When used on the root element in an extension definition, this indicates whether or not the extension is a modifier extension.", 0, 1, isModifier); 11553 case -1854387259: /*isModifierReason*/ return new Property("isModifierReason", "string", "Explains how that element affects the interpretation of the resource or element that contains it.", 0, 1, isModifierReason); 11554 case 1857548060: /*isSummary*/ return new Property("isSummary", "boolean", "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, isSummary); 11555 case -108220795: /*binding*/ return new Property("binding", "", "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 0, 1, binding); 11556 case 837556430: /*mapping*/ return new Property("mapping", "", "Identifies a concept from an external specification that roughly corresponds to this element.", 0, java.lang.Integer.MAX_VALUE, mapping); 11557 default: return super.getNamedProperty(_hash, _name, _checkValid); 11558 } 11559 11560 } 11561 11562 @Override 11563 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11564 switch (hash) { 11565 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 11566 case -671065907: /*representation*/ return this.representation == null ? new Base[0] : this.representation.toArray(new Base[this.representation.size()]); // Enumeration<PropertyRepresentation> 11567 case -825289923: /*sliceName*/ return this.sliceName == null ? new Base[0] : new Base[] {this.sliceName}; // StringType 11568 case 333040519: /*sliceIsConstraining*/ return this.sliceIsConstraining == null ? new Base[0] : new Base[] {this.sliceIsConstraining}; // BooleanType 11569 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 11570 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 11571 case -2119287345: /*slicing*/ return this.slicing == null ? new Base[0] : new Base[] {this.slicing}; // ElementDefinitionSlicingComponent 11572 case 109413500: /*short*/ return this.short_ == null ? new Base[0] : new Base[] {this.short_}; // StringType 11573 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // MarkdownType 11574 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 11575 case -1619874672: /*requirements*/ return this.requirements == null ? new Base[0] : new Base[] {this.requirements}; // MarkdownType 11576 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 11577 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 11578 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 11579 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // ElementDefinitionBaseComponent 11580 case 1193747154: /*contentReference*/ return this.contentReference == null ? new Base[0] : new Base[] {this.contentReference}; // UriType 11581 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // TypeRefComponent 11582 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // DataType 11583 case 1857257103: /*meaningWhenMissing*/ return this.meaningWhenMissing == null ? new Base[0] : new Base[] {this.meaningWhenMissing}; // MarkdownType 11584 case 1828196047: /*orderMeaning*/ return this.orderMeaning == null ? new Base[0] : new Base[] {this.orderMeaning}; // StringType 11585 case 97445748: /*fixed*/ return this.fixed == null ? new Base[0] : new Base[] {this.fixed}; // DataType 11586 case -791090288: /*pattern*/ return this.pattern == null ? new Base[0] : new Base[] {this.pattern}; // DataType 11587 case -1322970774: /*example*/ return this.example == null ? new Base[0] : this.example.toArray(new Base[this.example.size()]); // ElementDefinitionExampleComponent 11588 case -1376969153: /*minValue*/ return this.minValue == null ? new Base[0] : new Base[] {this.minValue}; // DataType 11589 case 399227501: /*maxValue*/ return this.maxValue == null ? new Base[0] : new Base[] {this.maxValue}; // DataType 11590 case -791400086: /*maxLength*/ return this.maxLength == null ? new Base[0] : new Base[] {this.maxLength}; // IntegerType 11591 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // IdType 11592 case -190376483: /*constraint*/ return this.constraint == null ? new Base[0] : this.constraint.toArray(new Base[this.constraint.size()]); // ElementDefinitionConstraintComponent 11593 case -923694880: /*mustHaveValue*/ return this.mustHaveValue == null ? new Base[0] : new Base[] {this.mustHaveValue}; // BooleanType 11594 case -2124672393: /*valueAlternatives*/ return this.valueAlternatives == null ? new Base[0] : this.valueAlternatives.toArray(new Base[this.valueAlternatives.size()]); // CanonicalType 11595 case -1402857082: /*mustSupport*/ return this.mustSupport == null ? new Base[0] : new Base[] {this.mustSupport}; // BooleanType 11596 case -1408783839: /*isModifier*/ return this.isModifier == null ? new Base[0] : new Base[] {this.isModifier}; // BooleanType 11597 case -1854387259: /*isModifierReason*/ return this.isModifierReason == null ? new Base[0] : new Base[] {this.isModifierReason}; // StringType 11598 case 1857548060: /*isSummary*/ return this.isSummary == null ? new Base[0] : new Base[] {this.isSummary}; // BooleanType 11599 case -108220795: /*binding*/ return this.binding == null ? new Base[0] : new Base[] {this.binding}; // ElementDefinitionBindingComponent 11600 case 837556430: /*mapping*/ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // ElementDefinitionMappingComponent 11601 default: return super.getProperty(hash, name, checkValid); 11602 } 11603 11604 } 11605 11606 @Override 11607 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11608 switch (hash) { 11609 case 3433509: // path 11610 this.path = TypeConvertor.castToString(value); // StringType 11611 return value; 11612 case -671065907: // representation 11613 value = new PropertyRepresentationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11614 this.getRepresentation().add((Enumeration) value); // Enumeration<PropertyRepresentation> 11615 return value; 11616 case -825289923: // sliceName 11617 this.sliceName = TypeConvertor.castToString(value); // StringType 11618 return value; 11619 case 333040519: // sliceIsConstraining 11620 this.sliceIsConstraining = TypeConvertor.castToBoolean(value); // BooleanType 11621 return value; 11622 case 102727412: // label 11623 this.label = TypeConvertor.castToString(value); // StringType 11624 return value; 11625 case 3059181: // code 11626 this.getCode().add(TypeConvertor.castToCoding(value)); // Coding 11627 return value; 11628 case -2119287345: // slicing 11629 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 11630 return value; 11631 case 109413500: // short 11632 this.short_ = TypeConvertor.castToString(value); // StringType 11633 return value; 11634 case -1014418093: // definition 11635 this.definition = TypeConvertor.castToMarkdown(value); // MarkdownType 11636 return value; 11637 case 950398559: // comment 11638 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 11639 return value; 11640 case -1619874672: // requirements 11641 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 11642 return value; 11643 case 92902992: // alias 11644 this.getAlias().add(TypeConvertor.castToString(value)); // StringType 11645 return value; 11646 case 108114: // min 11647 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 11648 return value; 11649 case 107876: // max 11650 this.max = TypeConvertor.castToString(value); // StringType 11651 return value; 11652 case 3016401: // base 11653 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 11654 return value; 11655 case 1193747154: // contentReference 11656 this.contentReference = TypeConvertor.castToUri(value); // UriType 11657 return value; 11658 case 3575610: // type 11659 this.getType().add((TypeRefComponent) value); // TypeRefComponent 11660 return value; 11661 case -659125328: // defaultValue 11662 this.defaultValue = TypeConvertor.castToType(value); // DataType 11663 return value; 11664 case 1857257103: // meaningWhenMissing 11665 this.meaningWhenMissing = TypeConvertor.castToMarkdown(value); // MarkdownType 11666 return value; 11667 case 1828196047: // orderMeaning 11668 this.orderMeaning = TypeConvertor.castToString(value); // StringType 11669 return value; 11670 case 97445748: // fixed 11671 this.fixed = TypeConvertor.castToType(value); // DataType 11672 return value; 11673 case -791090288: // pattern 11674 this.pattern = TypeConvertor.castToType(value); // DataType 11675 return value; 11676 case -1322970774: // example 11677 this.getExample().add((ElementDefinitionExampleComponent) value); // ElementDefinitionExampleComponent 11678 return value; 11679 case -1376969153: // minValue 11680 this.minValue = TypeConvertor.castToType(value); // DataType 11681 return value; 11682 case 399227501: // maxValue 11683 this.maxValue = TypeConvertor.castToType(value); // DataType 11684 return value; 11685 case -791400086: // maxLength 11686 this.maxLength = TypeConvertor.castToInteger(value); // IntegerType 11687 return value; 11688 case -861311717: // condition 11689 this.getCondition().add(TypeConvertor.castToId(value)); // IdType 11690 return value; 11691 case -190376483: // constraint 11692 this.getConstraint().add((ElementDefinitionConstraintComponent) value); // ElementDefinitionConstraintComponent 11693 return value; 11694 case -923694880: // mustHaveValue 11695 this.mustHaveValue = TypeConvertor.castToBoolean(value); // BooleanType 11696 return value; 11697 case -2124672393: // valueAlternatives 11698 this.getValueAlternatives().add(TypeConvertor.castToCanonical(value)); // CanonicalType 11699 return value; 11700 case -1402857082: // mustSupport 11701 this.mustSupport = TypeConvertor.castToBoolean(value); // BooleanType 11702 return value; 11703 case -1408783839: // isModifier 11704 this.isModifier = TypeConvertor.castToBoolean(value); // BooleanType 11705 return value; 11706 case -1854387259: // isModifierReason 11707 this.isModifierReason = TypeConvertor.castToString(value); // StringType 11708 return value; 11709 case 1857548060: // isSummary 11710 this.isSummary = TypeConvertor.castToBoolean(value); // BooleanType 11711 return value; 11712 case -108220795: // binding 11713 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 11714 return value; 11715 case 837556430: // mapping 11716 this.getMapping().add((ElementDefinitionMappingComponent) value); // ElementDefinitionMappingComponent 11717 return value; 11718 default: return super.setProperty(hash, name, value); 11719 } 11720 11721 } 11722 11723 @Override 11724 public Base setProperty(String name, Base value) throws FHIRException { 11725 if (name.equals("path")) { 11726 this.path = TypeConvertor.castToString(value); // StringType 11727 } else if (name.equals("representation")) { 11728 value = new PropertyRepresentationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11729 this.getRepresentation().add((Enumeration) value); 11730 } else if (name.equals("sliceName")) { 11731 this.sliceName = TypeConvertor.castToString(value); // StringType 11732 } else if (name.equals("sliceIsConstraining")) { 11733 this.sliceIsConstraining = TypeConvertor.castToBoolean(value); // BooleanType 11734 } else if (name.equals("label")) { 11735 this.label = TypeConvertor.castToString(value); // StringType 11736 } else if (name.equals("code")) { 11737 this.getCode().add(TypeConvertor.castToCoding(value)); 11738 } else if (name.equals("slicing")) { 11739 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 11740 } else if (name.equals("short")) { 11741 this.short_ = TypeConvertor.castToString(value); // StringType 11742 } else if (name.equals("definition")) { 11743 this.definition = TypeConvertor.castToMarkdown(value); // MarkdownType 11744 } else if (name.equals("comment")) { 11745 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 11746 } else if (name.equals("requirements")) { 11747 this.requirements = TypeConvertor.castToMarkdown(value); // MarkdownType 11748 } else if (name.equals("alias")) { 11749 this.getAlias().add(TypeConvertor.castToString(value)); 11750 } else if (name.equals("min")) { 11751 this.min = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 11752 } else if (name.equals("max")) { 11753 this.max = TypeConvertor.castToString(value); // StringType 11754 } else if (name.equals("base")) { 11755 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 11756 } else if (name.equals("contentReference")) { 11757 this.contentReference = TypeConvertor.castToUri(value); // UriType 11758 } else if (name.equals("type")) { 11759 this.getType().add((TypeRefComponent) value); 11760 } else if (name.equals("defaultValue[x]")) { 11761 this.defaultValue = TypeConvertor.castToType(value); // DataType 11762 } else if (name.equals("meaningWhenMissing")) { 11763 this.meaningWhenMissing = TypeConvertor.castToMarkdown(value); // MarkdownType 11764 } else if (name.equals("orderMeaning")) { 11765 this.orderMeaning = TypeConvertor.castToString(value); // StringType 11766 } else if (name.equals("fixed[x]")) { 11767 this.fixed = TypeConvertor.castToType(value); // DataType 11768 } else if (name.equals("pattern[x]")) { 11769 this.pattern = TypeConvertor.castToType(value); // DataType 11770 } else if (name.equals("example")) { 11771 this.getExample().add((ElementDefinitionExampleComponent) value); 11772 } else if (name.equals("minValue[x]")) { 11773 this.minValue = TypeConvertor.castToType(value); // DataType 11774 } else if (name.equals("maxValue[x]")) { 11775 this.maxValue = TypeConvertor.castToType(value); // DataType 11776 } else if (name.equals("maxLength")) { 11777 this.maxLength = TypeConvertor.castToInteger(value); // IntegerType 11778 } else if (name.equals("condition")) { 11779 this.getCondition().add(TypeConvertor.castToId(value)); 11780 } else if (name.equals("constraint")) { 11781 this.getConstraint().add((ElementDefinitionConstraintComponent) value); 11782 } else if (name.equals("mustHaveValue")) { 11783 this.mustHaveValue = TypeConvertor.castToBoolean(value); // BooleanType 11784 } else if (name.equals("valueAlternatives")) { 11785 this.getValueAlternatives().add(TypeConvertor.castToCanonical(value)); 11786 } else if (name.equals("mustSupport")) { 11787 this.mustSupport = TypeConvertor.castToBoolean(value); // BooleanType 11788 } else if (name.equals("isModifier")) { 11789 this.isModifier = TypeConvertor.castToBoolean(value); // BooleanType 11790 } else if (name.equals("isModifierReason")) { 11791 this.isModifierReason = TypeConvertor.castToString(value); // StringType 11792 } else if (name.equals("isSummary")) { 11793 this.isSummary = TypeConvertor.castToBoolean(value); // BooleanType 11794 } else if (name.equals("binding")) { 11795 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 11796 } else if (name.equals("mapping")) { 11797 this.getMapping().add((ElementDefinitionMappingComponent) value); 11798 } else 11799 return super.setProperty(name, value); 11800 return value; 11801 } 11802 11803 @Override 11804 public void removeChild(String name, Base value) throws FHIRException { 11805 if (name.equals("path")) { 11806 this.path = null; 11807 } else if (name.equals("representation")) { 11808 value = new PropertyRepresentationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11809 this.getRepresentation().remove((Enumeration) value); 11810 } else if (name.equals("sliceName")) { 11811 this.sliceName = null; 11812 } else if (name.equals("sliceIsConstraining")) { 11813 this.sliceIsConstraining = null; 11814 } else if (name.equals("label")) { 11815 this.label = null; 11816 } else if (name.equals("code")) { 11817 this.getCode().remove(value); 11818 } else if (name.equals("slicing")) { 11819 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 11820 } else if (name.equals("short")) { 11821 this.short_ = null; 11822 } else if (name.equals("definition")) { 11823 this.definition = null; 11824 } else if (name.equals("comment")) { 11825 this.comment = null; 11826 } else if (name.equals("requirements")) { 11827 this.requirements = null; 11828 } else if (name.equals("alias")) { 11829 this.getAlias().remove(value); 11830 } else if (name.equals("min")) { 11831 this.min = null; 11832 } else if (name.equals("max")) { 11833 this.max = null; 11834 } else if (name.equals("base")) { 11835 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 11836 } else if (name.equals("contentReference")) { 11837 this.contentReference = null; 11838 } else if (name.equals("type")) { 11839 this.getType().remove((TypeRefComponent) value); 11840 } else if (name.equals("defaultValue[x]")) { 11841 this.defaultValue = null; 11842 } else if (name.equals("meaningWhenMissing")) { 11843 this.meaningWhenMissing = null; 11844 } else if (name.equals("orderMeaning")) { 11845 this.orderMeaning = null; 11846 } else if (name.equals("fixed[x]")) { 11847 this.fixed = null; 11848 } else if (name.equals("pattern[x]")) { 11849 this.pattern = null; 11850 } else if (name.equals("example")) { 11851 this.getExample().remove((ElementDefinitionExampleComponent) value); 11852 } else if (name.equals("minValue[x]")) { 11853 this.minValue = null; 11854 } else if (name.equals("maxValue[x]")) { 11855 this.maxValue = null; 11856 } else if (name.equals("maxLength")) { 11857 this.maxLength = null; 11858 } else if (name.equals("condition")) { 11859 this.getCondition().remove(value); 11860 } else if (name.equals("constraint")) { 11861 this.getConstraint().remove((ElementDefinitionConstraintComponent) value); 11862 } else if (name.equals("mustHaveValue")) { 11863 this.mustHaveValue = null; 11864 } else if (name.equals("valueAlternatives")) { 11865 this.getValueAlternatives().remove(value); 11866 } else if (name.equals("mustSupport")) { 11867 this.mustSupport = null; 11868 } else if (name.equals("isModifier")) { 11869 this.isModifier = null; 11870 } else if (name.equals("isModifierReason")) { 11871 this.isModifierReason = null; 11872 } else if (name.equals("isSummary")) { 11873 this.isSummary = null; 11874 } else if (name.equals("binding")) { 11875 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 11876 } else if (name.equals("mapping")) { 11877 this.getMapping().remove((ElementDefinitionMappingComponent) value); 11878 } else 11879 super.removeChild(name, value); 11880 11881 } 11882 11883 @Override 11884 public Base makeProperty(int hash, String name) throws FHIRException { 11885 switch (hash) { 11886 case 3433509: return getPathElement(); 11887 case -671065907: return addRepresentationElement(); 11888 case -825289923: return getSliceNameElement(); 11889 case 333040519: return getSliceIsConstrainingElement(); 11890 case 102727412: return getLabelElement(); 11891 case 3059181: return addCode(); 11892 case -2119287345: return getSlicing(); 11893 case 109413500: return getShortElement(); 11894 case -1014418093: return getDefinitionElement(); 11895 case 950398559: return getCommentElement(); 11896 case -1619874672: return getRequirementsElement(); 11897 case 92902992: return addAliasElement(); 11898 case 108114: return getMinElement(); 11899 case 107876: return getMaxElement(); 11900 case 3016401: return getBase(); 11901 case 1193747154: return getContentReferenceElement(); 11902 case 3575610: return addType(); 11903 case 587922128: return getDefaultValue(); 11904 case -659125328: return getDefaultValue(); 11905 case 1857257103: return getMeaningWhenMissingElement(); 11906 case 1828196047: return getOrderMeaningElement(); 11907 case -391522164: return getFixed(); 11908 case 97445748: return getFixed(); 11909 case -885125392: return getPattern(); 11910 case -791090288: return getPattern(); 11911 case -1322970774: return addExample(); 11912 case -55301663: return getMinValue(); 11913 case -1376969153: return getMinValue(); 11914 case 622130931: return getMaxValue(); 11915 case 399227501: return getMaxValue(); 11916 case -791400086: return getMaxLengthElement(); 11917 case -861311717: return addConditionElement(); 11918 case -190376483: return addConstraint(); 11919 case -923694880: return getMustHaveValueElement(); 11920 case -2124672393: return addValueAlternativesElement(); 11921 case -1402857082: return getMustSupportElement(); 11922 case -1408783839: return getIsModifierElement(); 11923 case -1854387259: return getIsModifierReasonElement(); 11924 case 1857548060: return getIsSummaryElement(); 11925 case -108220795: return getBinding(); 11926 case 837556430: return addMapping(); 11927 default: return super.makeProperty(hash, name); 11928 } 11929 11930 } 11931 11932 @Override 11933 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11934 switch (hash) { 11935 case 3433509: /*path*/ return new String[] {"string"}; 11936 case -671065907: /*representation*/ return new String[] {"code"}; 11937 case -825289923: /*sliceName*/ return new String[] {"string"}; 11938 case 333040519: /*sliceIsConstraining*/ return new String[] {"boolean"}; 11939 case 102727412: /*label*/ return new String[] {"string"}; 11940 case 3059181: /*code*/ return new String[] {"Coding"}; 11941 case -2119287345: /*slicing*/ return new String[] {}; 11942 case 109413500: /*short*/ return new String[] {"string"}; 11943 case -1014418093: /*definition*/ return new String[] {"markdown"}; 11944 case 950398559: /*comment*/ return new String[] {"markdown"}; 11945 case -1619874672: /*requirements*/ return new String[] {"markdown"}; 11946 case 92902992: /*alias*/ return new String[] {"string"}; 11947 case 108114: /*min*/ return new String[] {"unsignedInt"}; 11948 case 107876: /*max*/ return new String[] {"string"}; 11949 case 3016401: /*base*/ return new String[] {}; 11950 case 1193747154: /*contentReference*/ return new String[] {"uri"}; 11951 case 3575610: /*type*/ return new String[] {}; 11952 case -659125328: /*defaultValue*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 11953 case 1857257103: /*meaningWhenMissing*/ return new String[] {"markdown"}; 11954 case 1828196047: /*orderMeaning*/ return new String[] {"string"}; 11955 case 97445748: /*fixed*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 11956 case -791090288: /*pattern*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 11957 case -1322970774: /*example*/ return new String[] {}; 11958 case -1376969153: /*minValue*/ return new String[] {"date", "dateTime", "instant", "time", "decimal", "integer", "integer64", "positiveInt", "unsignedInt", "Quantity"}; 11959 case 399227501: /*maxValue*/ return new String[] {"date", "dateTime", "instant", "time", "decimal", "integer", "integer64", "positiveInt", "unsignedInt", "Quantity"}; 11960 case -791400086: /*maxLength*/ return new String[] {"integer"}; 11961 case -861311717: /*condition*/ return new String[] {"id"}; 11962 case -190376483: /*constraint*/ return new String[] {}; 11963 case -923694880: /*mustHaveValue*/ return new String[] {"boolean"}; 11964 case -2124672393: /*valueAlternatives*/ return new String[] {"canonical"}; 11965 case -1402857082: /*mustSupport*/ return new String[] {"boolean"}; 11966 case -1408783839: /*isModifier*/ return new String[] {"boolean"}; 11967 case -1854387259: /*isModifierReason*/ return new String[] {"string"}; 11968 case 1857548060: /*isSummary*/ return new String[] {"boolean"}; 11969 case -108220795: /*binding*/ return new String[] {}; 11970 case 837556430: /*mapping*/ return new String[] {}; 11971 default: return super.getTypesForProperty(hash, name); 11972 } 11973 11974 } 11975 11976 @Override 11977 public Base addChild(String name) throws FHIRException { 11978 if (name.equals("path")) { 11979 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 11980 } 11981 else if (name.equals("representation")) { 11982 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.representation"); 11983 } 11984 else if (name.equals("sliceName")) { 11985 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceName"); 11986 } 11987 else if (name.equals("sliceIsConstraining")) { 11988 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceIsConstraining"); 11989 } 11990 else if (name.equals("label")) { 11991 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 11992 } 11993 else if (name.equals("code")) { 11994 return addCode(); 11995 } 11996 else if (name.equals("slicing")) { 11997 this.slicing = new ElementDefinitionSlicingComponent(); 11998 return this.slicing; 11999 } 12000 else if (name.equals("short")) { 12001 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.short"); 12002 } 12003 else if (name.equals("definition")) { 12004 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.definition"); 12005 } 12006 else if (name.equals("comment")) { 12007 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 12008 } 12009 else if (name.equals("requirements")) { 12010 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 12011 } 12012 else if (name.equals("alias")) { 12013 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.alias"); 12014 } 12015 else if (name.equals("min")) { 12016 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 12017 } 12018 else if (name.equals("max")) { 12019 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 12020 } 12021 else if (name.equals("base")) { 12022 this.base = new ElementDefinitionBaseComponent(); 12023 return this.base; 12024 } 12025 else if (name.equals("contentReference")) { 12026 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.contentReference"); 12027 } 12028 else if (name.equals("type")) { 12029 return addType(); 12030 } 12031 else if (name.equals("defaultValueBase64Binary")) { 12032 this.defaultValue = new Base64BinaryType(); 12033 return this.defaultValue; 12034 } 12035 else if (name.equals("defaultValueBoolean")) { 12036 this.defaultValue = new BooleanType(); 12037 return this.defaultValue; 12038 } 12039 else if (name.equals("defaultValueCanonical")) { 12040 this.defaultValue = new CanonicalType(); 12041 return this.defaultValue; 12042 } 12043 else if (name.equals("defaultValueCode")) { 12044 this.defaultValue = new CodeType(); 12045 return this.defaultValue; 12046 } 12047 else if (name.equals("defaultValueDate")) { 12048 this.defaultValue = new DateType(); 12049 return this.defaultValue; 12050 } 12051 else if (name.equals("defaultValueDateTime")) { 12052 this.defaultValue = new DateTimeType(); 12053 return this.defaultValue; 12054 } 12055 else if (name.equals("defaultValueDecimal")) { 12056 this.defaultValue = new DecimalType(); 12057 return this.defaultValue; 12058 } 12059 else if (name.equals("defaultValueId")) { 12060 this.defaultValue = new IdType(); 12061 return this.defaultValue; 12062 } 12063 else if (name.equals("defaultValueInstant")) { 12064 this.defaultValue = new InstantType(); 12065 return this.defaultValue; 12066 } 12067 else if (name.equals("defaultValueInteger")) { 12068 this.defaultValue = new IntegerType(); 12069 return this.defaultValue; 12070 } 12071 else if (name.equals("defaultValueInteger64")) { 12072 this.defaultValue = new Integer64Type(); 12073 return this.defaultValue; 12074 } 12075 else if (name.equals("defaultValueMarkdown")) { 12076 this.defaultValue = new MarkdownType(); 12077 return this.defaultValue; 12078 } 12079 else if (name.equals("defaultValueOid")) { 12080 this.defaultValue = new OidType(); 12081 return this.defaultValue; 12082 } 12083 else if (name.equals("defaultValuePositiveInt")) { 12084 this.defaultValue = new PositiveIntType(); 12085 return this.defaultValue; 12086 } 12087 else if (name.equals("defaultValueString")) { 12088 this.defaultValue = new StringType(); 12089 return this.defaultValue; 12090 } 12091 else if (name.equals("defaultValueTime")) { 12092 this.defaultValue = new TimeType(); 12093 return this.defaultValue; 12094 } 12095 else if (name.equals("defaultValueUnsignedInt")) { 12096 this.defaultValue = new UnsignedIntType(); 12097 return this.defaultValue; 12098 } 12099 else if (name.equals("defaultValueUri")) { 12100 this.defaultValue = new UriType(); 12101 return this.defaultValue; 12102 } 12103 else if (name.equals("defaultValueUrl")) { 12104 this.defaultValue = new UrlType(); 12105 return this.defaultValue; 12106 } 12107 else if (name.equals("defaultValueUuid")) { 12108 this.defaultValue = new UuidType(); 12109 return this.defaultValue; 12110 } 12111 else if (name.equals("defaultValueAddress")) { 12112 this.defaultValue = new Address(); 12113 return this.defaultValue; 12114 } 12115 else if (name.equals("defaultValueAge")) { 12116 this.defaultValue = new Age(); 12117 return this.defaultValue; 12118 } 12119 else if (name.equals("defaultValueAnnotation")) { 12120 this.defaultValue = new Annotation(); 12121 return this.defaultValue; 12122 } 12123 else if (name.equals("defaultValueAttachment")) { 12124 this.defaultValue = new Attachment(); 12125 return this.defaultValue; 12126 } 12127 else if (name.equals("defaultValueCodeableConcept")) { 12128 this.defaultValue = new CodeableConcept(); 12129 return this.defaultValue; 12130 } 12131 else if (name.equals("defaultValueCodeableReference")) { 12132 this.defaultValue = new CodeableReference(); 12133 return this.defaultValue; 12134 } 12135 else if (name.equals("defaultValueCoding")) { 12136 this.defaultValue = new Coding(); 12137 return this.defaultValue; 12138 } 12139 else if (name.equals("defaultValueContactPoint")) { 12140 this.defaultValue = new ContactPoint(); 12141 return this.defaultValue; 12142 } 12143 else if (name.equals("defaultValueCount")) { 12144 this.defaultValue = new Count(); 12145 return this.defaultValue; 12146 } 12147 else if (name.equals("defaultValueDistance")) { 12148 this.defaultValue = new Distance(); 12149 return this.defaultValue; 12150 } 12151 else if (name.equals("defaultValueDuration")) { 12152 this.defaultValue = new Duration(); 12153 return this.defaultValue; 12154 } 12155 else if (name.equals("defaultValueHumanName")) { 12156 this.defaultValue = new HumanName(); 12157 return this.defaultValue; 12158 } 12159 else if (name.equals("defaultValueIdentifier")) { 12160 this.defaultValue = new Identifier(); 12161 return this.defaultValue; 12162 } 12163 else if (name.equals("defaultValueMoney")) { 12164 this.defaultValue = new Money(); 12165 return this.defaultValue; 12166 } 12167 else if (name.equals("defaultValuePeriod")) { 12168 this.defaultValue = new Period(); 12169 return this.defaultValue; 12170 } 12171 else if (name.equals("defaultValueQuantity")) { 12172 this.defaultValue = new Quantity(); 12173 return this.defaultValue; 12174 } 12175 else if (name.equals("defaultValueRange")) { 12176 this.defaultValue = new Range(); 12177 return this.defaultValue; 12178 } 12179 else if (name.equals("defaultValueRatio")) { 12180 this.defaultValue = new Ratio(); 12181 return this.defaultValue; 12182 } 12183 else if (name.equals("defaultValueRatioRange")) { 12184 this.defaultValue = new RatioRange(); 12185 return this.defaultValue; 12186 } 12187 else if (name.equals("defaultValueReference")) { 12188 this.defaultValue = new Reference(); 12189 return this.defaultValue; 12190 } 12191 else if (name.equals("defaultValueSampledData")) { 12192 this.defaultValue = new SampledData(); 12193 return this.defaultValue; 12194 } 12195 else if (name.equals("defaultValueSignature")) { 12196 this.defaultValue = new Signature(); 12197 return this.defaultValue; 12198 } 12199 else if (name.equals("defaultValueTiming")) { 12200 this.defaultValue = new Timing(); 12201 return this.defaultValue; 12202 } 12203 else if (name.equals("defaultValueContactDetail")) { 12204 this.defaultValue = new ContactDetail(); 12205 return this.defaultValue; 12206 } 12207 else if (name.equals("defaultValueDataRequirement")) { 12208 this.defaultValue = new DataRequirement(); 12209 return this.defaultValue; 12210 } 12211 else if (name.equals("defaultValueExpression")) { 12212 this.defaultValue = new Expression(); 12213 return this.defaultValue; 12214 } 12215 else if (name.equals("defaultValueParameterDefinition")) { 12216 this.defaultValue = new ParameterDefinition(); 12217 return this.defaultValue; 12218 } 12219 else if (name.equals("defaultValueRelatedArtifact")) { 12220 this.defaultValue = new RelatedArtifact(); 12221 return this.defaultValue; 12222 } 12223 else if (name.equals("defaultValueTriggerDefinition")) { 12224 this.defaultValue = new TriggerDefinition(); 12225 return this.defaultValue; 12226 } 12227 else if (name.equals("defaultValueUsageContext")) { 12228 this.defaultValue = new UsageContext(); 12229 return this.defaultValue; 12230 } 12231 else if (name.equals("defaultValueAvailability")) { 12232 this.defaultValue = new Availability(); 12233 return this.defaultValue; 12234 } 12235 else if (name.equals("defaultValueExtendedContactDetail")) { 12236 this.defaultValue = new ExtendedContactDetail(); 12237 return this.defaultValue; 12238 } 12239 else if (name.equals("defaultValueDosage")) { 12240 this.defaultValue = new Dosage(); 12241 return this.defaultValue; 12242 } 12243 else if (name.equals("defaultValueMeta")) { 12244 this.defaultValue = new Meta(); 12245 return this.defaultValue; 12246 } 12247 else if (name.equals("meaningWhenMissing")) { 12248 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.meaningWhenMissing"); 12249 } 12250 else if (name.equals("orderMeaning")) { 12251 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.orderMeaning"); 12252 } 12253 else if (name.equals("fixedBase64Binary")) { 12254 this.fixed = new Base64BinaryType(); 12255 return this.fixed; 12256 } 12257 else if (name.equals("fixedBoolean")) { 12258 this.fixed = new BooleanType(); 12259 return this.fixed; 12260 } 12261 else if (name.equals("fixedCanonical")) { 12262 this.fixed = new CanonicalType(); 12263 return this.fixed; 12264 } 12265 else if (name.equals("fixedCode")) { 12266 this.fixed = new CodeType(); 12267 return this.fixed; 12268 } 12269 else if (name.equals("fixedDate")) { 12270 this.fixed = new DateType(); 12271 return this.fixed; 12272 } 12273 else if (name.equals("fixedDateTime")) { 12274 this.fixed = new DateTimeType(); 12275 return this.fixed; 12276 } 12277 else if (name.equals("fixedDecimal")) { 12278 this.fixed = new DecimalType(); 12279 return this.fixed; 12280 } 12281 else if (name.equals("fixedId")) { 12282 this.fixed = new IdType(); 12283 return this.fixed; 12284 } 12285 else if (name.equals("fixedInstant")) { 12286 this.fixed = new InstantType(); 12287 return this.fixed; 12288 } 12289 else if (name.equals("fixedInteger")) { 12290 this.fixed = new IntegerType(); 12291 return this.fixed; 12292 } 12293 else if (name.equals("fixedInteger64")) { 12294 this.fixed = new Integer64Type(); 12295 return this.fixed; 12296 } 12297 else if (name.equals("fixedMarkdown")) { 12298 this.fixed = new MarkdownType(); 12299 return this.fixed; 12300 } 12301 else if (name.equals("fixedOid")) { 12302 this.fixed = new OidType(); 12303 return this.fixed; 12304 } 12305 else if (name.equals("fixedPositiveInt")) { 12306 this.fixed = new PositiveIntType(); 12307 return this.fixed; 12308 } 12309 else if (name.equals("fixedString")) { 12310 this.fixed = new StringType(); 12311 return this.fixed; 12312 } 12313 else if (name.equals("fixedTime")) { 12314 this.fixed = new TimeType(); 12315 return this.fixed; 12316 } 12317 else if (name.equals("fixedUnsignedInt")) { 12318 this.fixed = new UnsignedIntType(); 12319 return this.fixed; 12320 } 12321 else if (name.equals("fixedUri")) { 12322 this.fixed = new UriType(); 12323 return this.fixed; 12324 } 12325 else if (name.equals("fixedUrl")) { 12326 this.fixed = new UrlType(); 12327 return this.fixed; 12328 } 12329 else if (name.equals("fixedUuid")) { 12330 this.fixed = new UuidType(); 12331 return this.fixed; 12332 } 12333 else if (name.equals("fixedAddress")) { 12334 this.fixed = new Address(); 12335 return this.fixed; 12336 } 12337 else if (name.equals("fixedAge")) { 12338 this.fixed = new Age(); 12339 return this.fixed; 12340 } 12341 else if (name.equals("fixedAnnotation")) { 12342 this.fixed = new Annotation(); 12343 return this.fixed; 12344 } 12345 else if (name.equals("fixedAttachment")) { 12346 this.fixed = new Attachment(); 12347 return this.fixed; 12348 } 12349 else if (name.equals("fixedCodeableConcept")) { 12350 this.fixed = new CodeableConcept(); 12351 return this.fixed; 12352 } 12353 else if (name.equals("fixedCodeableReference")) { 12354 this.fixed = new CodeableReference(); 12355 return this.fixed; 12356 } 12357 else if (name.equals("fixedCoding")) { 12358 this.fixed = new Coding(); 12359 return this.fixed; 12360 } 12361 else if (name.equals("fixedContactPoint")) { 12362 this.fixed = new ContactPoint(); 12363 return this.fixed; 12364 } 12365 else if (name.equals("fixedCount")) { 12366 this.fixed = new Count(); 12367 return this.fixed; 12368 } 12369 else if (name.equals("fixedDistance")) { 12370 this.fixed = new Distance(); 12371 return this.fixed; 12372 } 12373 else if (name.equals("fixedDuration")) { 12374 this.fixed = new Duration(); 12375 return this.fixed; 12376 } 12377 else if (name.equals("fixedHumanName")) { 12378 this.fixed = new HumanName(); 12379 return this.fixed; 12380 } 12381 else if (name.equals("fixedIdentifier")) { 12382 this.fixed = new Identifier(); 12383 return this.fixed; 12384 } 12385 else if (name.equals("fixedMoney")) { 12386 this.fixed = new Money(); 12387 return this.fixed; 12388 } 12389 else if (name.equals("fixedPeriod")) { 12390 this.fixed = new Period(); 12391 return this.fixed; 12392 } 12393 else if (name.equals("fixedQuantity")) { 12394 this.fixed = new Quantity(); 12395 return this.fixed; 12396 } 12397 else if (name.equals("fixedRange")) { 12398 this.fixed = new Range(); 12399 return this.fixed; 12400 } 12401 else if (name.equals("fixedRatio")) { 12402 this.fixed = new Ratio(); 12403 return this.fixed; 12404 } 12405 else if (name.equals("fixedRatioRange")) { 12406 this.fixed = new RatioRange(); 12407 return this.fixed; 12408 } 12409 else if (name.equals("fixedReference")) { 12410 this.fixed = new Reference(); 12411 return this.fixed; 12412 } 12413 else if (name.equals("fixedSampledData")) { 12414 this.fixed = new SampledData(); 12415 return this.fixed; 12416 } 12417 else if (name.equals("fixedSignature")) { 12418 this.fixed = new Signature(); 12419 return this.fixed; 12420 } 12421 else if (name.equals("fixedTiming")) { 12422 this.fixed = new Timing(); 12423 return this.fixed; 12424 } 12425 else if (name.equals("fixedContactDetail")) { 12426 this.fixed = new ContactDetail(); 12427 return this.fixed; 12428 } 12429 else if (name.equals("fixedDataRequirement")) { 12430 this.fixed = new DataRequirement(); 12431 return this.fixed; 12432 } 12433 else if (name.equals("fixedExpression")) { 12434 this.fixed = new Expression(); 12435 return this.fixed; 12436 } 12437 else if (name.equals("fixedParameterDefinition")) { 12438 this.fixed = new ParameterDefinition(); 12439 return this.fixed; 12440 } 12441 else if (name.equals("fixedRelatedArtifact")) { 12442 this.fixed = new RelatedArtifact(); 12443 return this.fixed; 12444 } 12445 else if (name.equals("fixedTriggerDefinition")) { 12446 this.fixed = new TriggerDefinition(); 12447 return this.fixed; 12448 } 12449 else if (name.equals("fixedUsageContext")) { 12450 this.fixed = new UsageContext(); 12451 return this.fixed; 12452 } 12453 else if (name.equals("fixedAvailability")) { 12454 this.fixed = new Availability(); 12455 return this.fixed; 12456 } 12457 else if (name.equals("fixedExtendedContactDetail")) { 12458 this.fixed = new ExtendedContactDetail(); 12459 return this.fixed; 12460 } 12461 else if (name.equals("fixedDosage")) { 12462 this.fixed = new Dosage(); 12463 return this.fixed; 12464 } 12465 else if (name.equals("fixedMeta")) { 12466 this.fixed = new Meta(); 12467 return this.fixed; 12468 } 12469 else if (name.equals("patternBase64Binary")) { 12470 this.pattern = new Base64BinaryType(); 12471 return this.pattern; 12472 } 12473 else if (name.equals("patternBoolean")) { 12474 this.pattern = new BooleanType(); 12475 return this.pattern; 12476 } 12477 else if (name.equals("patternCanonical")) { 12478 this.pattern = new CanonicalType(); 12479 return this.pattern; 12480 } 12481 else if (name.equals("patternCode")) { 12482 this.pattern = new CodeType(); 12483 return this.pattern; 12484 } 12485 else if (name.equals("patternDate")) { 12486 this.pattern = new DateType(); 12487 return this.pattern; 12488 } 12489 else if (name.equals("patternDateTime")) { 12490 this.pattern = new DateTimeType(); 12491 return this.pattern; 12492 } 12493 else if (name.equals("patternDecimal")) { 12494 this.pattern = new DecimalType(); 12495 return this.pattern; 12496 } 12497 else if (name.equals("patternId")) { 12498 this.pattern = new IdType(); 12499 return this.pattern; 12500 } 12501 else if (name.equals("patternInstant")) { 12502 this.pattern = new InstantType(); 12503 return this.pattern; 12504 } 12505 else if (name.equals("patternInteger")) { 12506 this.pattern = new IntegerType(); 12507 return this.pattern; 12508 } 12509 else if (name.equals("patternInteger64")) { 12510 this.pattern = new Integer64Type(); 12511 return this.pattern; 12512 } 12513 else if (name.equals("patternMarkdown")) { 12514 this.pattern = new MarkdownType(); 12515 return this.pattern; 12516 } 12517 else if (name.equals("patternOid")) { 12518 this.pattern = new OidType(); 12519 return this.pattern; 12520 } 12521 else if (name.equals("patternPositiveInt")) { 12522 this.pattern = new PositiveIntType(); 12523 return this.pattern; 12524 } 12525 else if (name.equals("patternString")) { 12526 this.pattern = new StringType(); 12527 return this.pattern; 12528 } 12529 else if (name.equals("patternTime")) { 12530 this.pattern = new TimeType(); 12531 return this.pattern; 12532 } 12533 else if (name.equals("patternUnsignedInt")) { 12534 this.pattern = new UnsignedIntType(); 12535 return this.pattern; 12536 } 12537 else if (name.equals("patternUri")) { 12538 this.pattern = new UriType(); 12539 return this.pattern; 12540 } 12541 else if (name.equals("patternUrl")) { 12542 this.pattern = new UrlType(); 12543 return this.pattern; 12544 } 12545 else if (name.equals("patternUuid")) { 12546 this.pattern = new UuidType(); 12547 return this.pattern; 12548 } 12549 else if (name.equals("patternAddress")) { 12550 this.pattern = new Address(); 12551 return this.pattern; 12552 } 12553 else if (name.equals("patternAge")) { 12554 this.pattern = new Age(); 12555 return this.pattern; 12556 } 12557 else if (name.equals("patternAnnotation")) { 12558 this.pattern = new Annotation(); 12559 return this.pattern; 12560 } 12561 else if (name.equals("patternAttachment")) { 12562 this.pattern = new Attachment(); 12563 return this.pattern; 12564 } 12565 else if (name.equals("patternCodeableConcept")) { 12566 this.pattern = new CodeableConcept(); 12567 return this.pattern; 12568 } 12569 else if (name.equals("patternCodeableReference")) { 12570 this.pattern = new CodeableReference(); 12571 return this.pattern; 12572 } 12573 else if (name.equals("patternCoding")) { 12574 this.pattern = new Coding(); 12575 return this.pattern; 12576 } 12577 else if (name.equals("patternContactPoint")) { 12578 this.pattern = new ContactPoint(); 12579 return this.pattern; 12580 } 12581 else if (name.equals("patternCount")) { 12582 this.pattern = new Count(); 12583 return this.pattern; 12584 } 12585 else if (name.equals("patternDistance")) { 12586 this.pattern = new Distance(); 12587 return this.pattern; 12588 } 12589 else if (name.equals("patternDuration")) { 12590 this.pattern = new Duration(); 12591 return this.pattern; 12592 } 12593 else if (name.equals("patternHumanName")) { 12594 this.pattern = new HumanName(); 12595 return this.pattern; 12596 } 12597 else if (name.equals("patternIdentifier")) { 12598 this.pattern = new Identifier(); 12599 return this.pattern; 12600 } 12601 else if (name.equals("patternMoney")) { 12602 this.pattern = new Money(); 12603 return this.pattern; 12604 } 12605 else if (name.equals("patternPeriod")) { 12606 this.pattern = new Period(); 12607 return this.pattern; 12608 } 12609 else if (name.equals("patternQuantity")) { 12610 this.pattern = new Quantity(); 12611 return this.pattern; 12612 } 12613 else if (name.equals("patternRange")) { 12614 this.pattern = new Range(); 12615 return this.pattern; 12616 } 12617 else if (name.equals("patternRatio")) { 12618 this.pattern = new Ratio(); 12619 return this.pattern; 12620 } 12621 else if (name.equals("patternRatioRange")) { 12622 this.pattern = new RatioRange(); 12623 return this.pattern; 12624 } 12625 else if (name.equals("patternReference")) { 12626 this.pattern = new Reference(); 12627 return this.pattern; 12628 } 12629 else if (name.equals("patternSampledData")) { 12630 this.pattern = new SampledData(); 12631 return this.pattern; 12632 } 12633 else if (name.equals("patternSignature")) { 12634 this.pattern = new Signature(); 12635 return this.pattern; 12636 } 12637 else if (name.equals("patternTiming")) { 12638 this.pattern = new Timing(); 12639 return this.pattern; 12640 } 12641 else if (name.equals("patternContactDetail")) { 12642 this.pattern = new ContactDetail(); 12643 return this.pattern; 12644 } 12645 else if (name.equals("patternDataRequirement")) { 12646 this.pattern = new DataRequirement(); 12647 return this.pattern; 12648 } 12649 else if (name.equals("patternExpression")) { 12650 this.pattern = new Expression(); 12651 return this.pattern; 12652 } 12653 else if (name.equals("patternParameterDefinition")) { 12654 this.pattern = new ParameterDefinition(); 12655 return this.pattern; 12656 } 12657 else if (name.equals("patternRelatedArtifact")) { 12658 this.pattern = new RelatedArtifact(); 12659 return this.pattern; 12660 } 12661 else if (name.equals("patternTriggerDefinition")) { 12662 this.pattern = new TriggerDefinition(); 12663 return this.pattern; 12664 } 12665 else if (name.equals("patternUsageContext")) { 12666 this.pattern = new UsageContext(); 12667 return this.pattern; 12668 } 12669 else if (name.equals("patternAvailability")) { 12670 this.pattern = new Availability(); 12671 return this.pattern; 12672 } 12673 else if (name.equals("patternExtendedContactDetail")) { 12674 this.pattern = new ExtendedContactDetail(); 12675 return this.pattern; 12676 } 12677 else if (name.equals("patternDosage")) { 12678 this.pattern = new Dosage(); 12679 return this.pattern; 12680 } 12681 else if (name.equals("patternMeta")) { 12682 this.pattern = new Meta(); 12683 return this.pattern; 12684 } 12685 else if (name.equals("example")) { 12686 return addExample(); 12687 } 12688 else if (name.equals("minValueDate")) { 12689 this.minValue = new DateType(); 12690 return this.minValue; 12691 } 12692 else if (name.equals("minValueDateTime")) { 12693 this.minValue = new DateTimeType(); 12694 return this.minValue; 12695 } 12696 else if (name.equals("minValueInstant")) { 12697 this.minValue = new InstantType(); 12698 return this.minValue; 12699 } 12700 else if (name.equals("minValueTime")) { 12701 this.minValue = new TimeType(); 12702 return this.minValue; 12703 } 12704 else if (name.equals("minValueDecimal")) { 12705 this.minValue = new DecimalType(); 12706 return this.minValue; 12707 } 12708 else if (name.equals("minValueInteger")) { 12709 this.minValue = new IntegerType(); 12710 return this.minValue; 12711 } 12712 else if (name.equals("minValueInteger64")) { 12713 this.minValue = new Integer64Type(); 12714 return this.minValue; 12715 } 12716 else if (name.equals("minValuePositiveInt")) { 12717 this.minValue = new PositiveIntType(); 12718 return this.minValue; 12719 } 12720 else if (name.equals("minValueUnsignedInt")) { 12721 this.minValue = new UnsignedIntType(); 12722 return this.minValue; 12723 } 12724 else if (name.equals("minValueQuantity")) { 12725 this.minValue = new Quantity(); 12726 return this.minValue; 12727 } 12728 else if (name.equals("maxValueDate")) { 12729 this.maxValue = new DateType(); 12730 return this.maxValue; 12731 } 12732 else if (name.equals("maxValueDateTime")) { 12733 this.maxValue = new DateTimeType(); 12734 return this.maxValue; 12735 } 12736 else if (name.equals("maxValueInstant")) { 12737 this.maxValue = new InstantType(); 12738 return this.maxValue; 12739 } 12740 else if (name.equals("maxValueTime")) { 12741 this.maxValue = new TimeType(); 12742 return this.maxValue; 12743 } 12744 else if (name.equals("maxValueDecimal")) { 12745 this.maxValue = new DecimalType(); 12746 return this.maxValue; 12747 } 12748 else if (name.equals("maxValueInteger")) { 12749 this.maxValue = new IntegerType(); 12750 return this.maxValue; 12751 } 12752 else if (name.equals("maxValueInteger64")) { 12753 this.maxValue = new Integer64Type(); 12754 return this.maxValue; 12755 } 12756 else if (name.equals("maxValuePositiveInt")) { 12757 this.maxValue = new PositiveIntType(); 12758 return this.maxValue; 12759 } 12760 else if (name.equals("maxValueUnsignedInt")) { 12761 this.maxValue = new UnsignedIntType(); 12762 return this.maxValue; 12763 } 12764 else if (name.equals("maxValueQuantity")) { 12765 this.maxValue = new Quantity(); 12766 return this.maxValue; 12767 } 12768 else if (name.equals("maxLength")) { 12769 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.maxLength"); 12770 } 12771 else if (name.equals("condition")) { 12772 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.condition"); 12773 } 12774 else if (name.equals("constraint")) { 12775 return addConstraint(); 12776 } 12777 else if (name.equals("mustHaveValue")) { 12778 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustHaveValue"); 12779 } 12780 else if (name.equals("valueAlternatives")) { 12781 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.valueAlternatives"); 12782 } 12783 else if (name.equals("mustSupport")) { 12784 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustSupport"); 12785 } 12786 else if (name.equals("isModifier")) { 12787 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifier"); 12788 } 12789 else if (name.equals("isModifierReason")) { 12790 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifierReason"); 12791 } 12792 else if (name.equals("isSummary")) { 12793 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isSummary"); 12794 } 12795 else if (name.equals("binding")) { 12796 this.binding = new ElementDefinitionBindingComponent(); 12797 return this.binding; 12798 } 12799 else if (name.equals("mapping")) { 12800 return addMapping(); 12801 } 12802 else 12803 return super.addChild(name); 12804 } 12805 12806 public String fhirType() { 12807 return "ElementDefinition"; 12808 12809 } 12810 12811 public ElementDefinition copy() { 12812 ElementDefinition dst = new ElementDefinition(); 12813 copyValues(dst); 12814 return dst; 12815 } 12816 12817 public void copyValues(ElementDefinition dst) { 12818 super.copyValues(dst); 12819 dst.path = path == null ? null : path.copy(); 12820 if (representation != null) { 12821 dst.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 12822 for (Enumeration<PropertyRepresentation> i : representation) 12823 dst.representation.add(i.copy()); 12824 }; 12825 dst.sliceName = sliceName == null ? null : sliceName.copy(); 12826 dst.sliceIsConstraining = sliceIsConstraining == null ? null : sliceIsConstraining.copy(); 12827 dst.label = label == null ? null : label.copy(); 12828 if (code != null) { 12829 dst.code = new ArrayList<Coding>(); 12830 for (Coding i : code) 12831 dst.code.add(i.copy()); 12832 }; 12833 dst.slicing = slicing == null ? null : slicing.copy(); 12834 dst.short_ = short_ == null ? null : short_.copy(); 12835 dst.definition = definition == null ? null : definition.copy(); 12836 dst.comment = comment == null ? null : comment.copy(); 12837 dst.requirements = requirements == null ? null : requirements.copy(); 12838 if (alias != null) { 12839 dst.alias = new ArrayList<StringType>(); 12840 for (StringType i : alias) 12841 dst.alias.add(i.copy()); 12842 }; 12843 dst.min = min == null ? null : min.copy(); 12844 dst.max = max == null ? null : max.copy(); 12845 dst.base = base == null ? null : base.copy(); 12846 dst.contentReference = contentReference == null ? null : contentReference.copy(); 12847 if (type != null) { 12848 dst.type = new ArrayList<TypeRefComponent>(); 12849 for (TypeRefComponent i : type) 12850 dst.type.add(i.copy()); 12851 }; 12852 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 12853 dst.meaningWhenMissing = meaningWhenMissing == null ? null : meaningWhenMissing.copy(); 12854 dst.orderMeaning = orderMeaning == null ? null : orderMeaning.copy(); 12855 dst.fixed = fixed == null ? null : fixed.copy(); 12856 dst.pattern = pattern == null ? null : pattern.copy(); 12857 if (example != null) { 12858 dst.example = new ArrayList<ElementDefinitionExampleComponent>(); 12859 for (ElementDefinitionExampleComponent i : example) 12860 dst.example.add(i.copy()); 12861 }; 12862 dst.minValue = minValue == null ? null : minValue.copy(); 12863 dst.maxValue = maxValue == null ? null : maxValue.copy(); 12864 dst.maxLength = maxLength == null ? null : maxLength.copy(); 12865 if (condition != null) { 12866 dst.condition = new ArrayList<IdType>(); 12867 for (IdType i : condition) 12868 dst.condition.add(i.copy()); 12869 }; 12870 if (constraint != null) { 12871 dst.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 12872 for (ElementDefinitionConstraintComponent i : constraint) 12873 dst.constraint.add(i.copy()); 12874 }; 12875 dst.mustHaveValue = mustHaveValue == null ? null : mustHaveValue.copy(); 12876 if (valueAlternatives != null) { 12877 dst.valueAlternatives = new ArrayList<CanonicalType>(); 12878 for (CanonicalType i : valueAlternatives) 12879 dst.valueAlternatives.add(i.copy()); 12880 }; 12881 dst.mustSupport = mustSupport == null ? null : mustSupport.copy(); 12882 dst.isModifier = isModifier == null ? null : isModifier.copy(); 12883 dst.isModifierReason = isModifierReason == null ? null : isModifierReason.copy(); 12884 dst.isSummary = isSummary == null ? null : isSummary.copy(); 12885 dst.binding = binding == null ? null : binding.copy(); 12886 if (mapping != null) { 12887 dst.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 12888 for (ElementDefinitionMappingComponent i : mapping) 12889 dst.mapping.add(i.copy()); 12890 }; 12891 } 12892 12893 protected ElementDefinition typedCopy() { 12894 return copy(); 12895 } 12896 12897 @Override 12898 public boolean equalsDeep(Base other_) { 12899 if (!super.equalsDeep(other_)) 12900 return false; 12901 if (!(other_ instanceof ElementDefinition)) 12902 return false; 12903 ElementDefinition o = (ElementDefinition) other_; 12904 return compareDeep(path, o.path, true) && compareDeep(representation, o.representation, true) && compareDeep(sliceName, o.sliceName, true) 12905 && compareDeep(sliceIsConstraining, o.sliceIsConstraining, true) && compareDeep(label, o.label, true) 12906 && compareDeep(code, o.code, true) && compareDeep(slicing, o.slicing, true) && compareDeep(short_, o.short_, true) 12907 && compareDeep(definition, o.definition, true) && compareDeep(comment, o.comment, true) && compareDeep(requirements, o.requirements, true) 12908 && compareDeep(alias, o.alias, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 12909 && compareDeep(base, o.base, true) && compareDeep(contentReference, o.contentReference, true) && compareDeep(type, o.type, true) 12910 && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(meaningWhenMissing, o.meaningWhenMissing, true) 12911 && compareDeep(orderMeaning, o.orderMeaning, true) && compareDeep(fixed, o.fixed, true) && compareDeep(pattern, o.pattern, true) 12912 && compareDeep(example, o.example, true) && compareDeep(minValue, o.minValue, true) && compareDeep(maxValue, o.maxValue, true) 12913 && compareDeep(maxLength, o.maxLength, true) && compareDeep(condition, o.condition, true) && compareDeep(constraint, o.constraint, true) 12914 && compareDeep(mustHaveValue, o.mustHaveValue, true) && compareDeep(valueAlternatives, o.valueAlternatives, true) 12915 && compareDeep(mustSupport, o.mustSupport, true) && compareDeep(isModifier, o.isModifier, true) 12916 && compareDeep(isModifierReason, o.isModifierReason, true) && compareDeep(isSummary, o.isSummary, true) 12917 && compareDeep(binding, o.binding, true) && compareDeep(mapping, o.mapping, true); 12918 } 12919 12920 @Override 12921 public boolean equalsShallow(Base other_) { 12922 if (!super.equalsShallow(other_)) 12923 return false; 12924 if (!(other_ instanceof ElementDefinition)) 12925 return false; 12926 ElementDefinition o = (ElementDefinition) other_; 12927 return compareValues(path, o.path, true) && compareValues(representation, o.representation, true) && compareValues(sliceName, o.sliceName, true) 12928 && compareValues(sliceIsConstraining, o.sliceIsConstraining, true) && compareValues(label, o.label, true) 12929 && compareValues(short_, o.short_, true) && compareValues(definition, o.definition, true) && compareValues(comment, o.comment, true) 12930 && compareValues(requirements, o.requirements, true) && compareValues(alias, o.alias, true) && compareValues(min, o.min, true) 12931 && compareValues(max, o.max, true) && compareValues(contentReference, o.contentReference, true) && compareValues(meaningWhenMissing, o.meaningWhenMissing, true) 12932 && compareValues(orderMeaning, o.orderMeaning, true) && compareValues(maxLength, o.maxLength, true) 12933 && compareValues(condition, o.condition, true) && compareValues(mustHaveValue, o.mustHaveValue, true) 12934 && compareValues(valueAlternatives, o.valueAlternatives, true) && compareValues(mustSupport, o.mustSupport, true) 12935 && compareValues(isModifier, o.isModifier, true) && compareValues(isModifierReason, o.isModifierReason, true) 12936 && compareValues(isSummary, o.isSummary, true); 12937 } 12938 12939 public boolean isEmpty() { 12940 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, representation, sliceName 12941 , sliceIsConstraining, label, code, slicing, short_, definition, comment, requirements 12942 , alias, min, max, base, contentReference, type, defaultValue, meaningWhenMissing 12943 , orderMeaning, fixed, pattern, example, minValue, maxValue, maxLength, condition 12944 , constraint, mustHaveValue, valueAlternatives, mustSupport, isModifier, isModifierReason 12945 , isSummary, binding, mapping); 12946 } 12947 12948// Manual code (from Configuration.txt): 12949 12950 public String toString() { 12951 if (hasId()) 12952 return getId(); 12953 if (hasSliceName()) 12954 return getPath()+":"+getSliceName(); 12955 else 12956 return getPath(); 12957 } 12958 12959 public void makeBase(String path, int min, String max) { 12960 ElementDefinitionBaseComponent self = getBase(); 12961 self.setPath(path); 12962 self.setMin(min); 12963 self.setMax(max); 12964 } 12965 12966 public void makeBase() { 12967 ElementDefinitionBaseComponent self = getBase(); 12968 self.setPath(getPath()); 12969 self.setMin(getMin()); 12970 self.setMax(getMax()); 12971 } 12972 12973 12974 public String typeSummary() { 12975 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder(); 12976 for (TypeRefComponent tr : getType()) { 12977 if (tr.hasCode()) 12978 b.append(tr.getWorkingCode()); 12979 } 12980 return b.toString(); 12981 } 12982 12983 public List<String> typeList() { 12984 List<String> res = new ArrayList<>(); 12985 for (TypeRefComponent tr : getType()) { 12986 if (tr.hasCode()) 12987 res.add(tr.getWorkingCode()); 12988 } 12989 return res; 12990 } 12991 12992 public String typeSummaryVB() { 12993 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder("|"); 12994 for (TypeRefComponent tr : getType()) { 12995 if (tr.hasCode()) 12996 b.append(tr.getWorkingCode()); 12997 } 12998 return b.toString().replace(" ", ""); 12999 } 13000 13001 public TypeRefComponent getType(String code) { 13002 for (TypeRefComponent tr : getType()) 13003 if (tr.getCode().equals(code)) 13004 return tr; 13005 TypeRefComponent tr = new TypeRefComponent(); 13006 tr.setCode(code); 13007 type.add(tr); 13008 return tr; 13009 } 13010 13011 public static final boolean NOT_MODIFIER = false; 13012 public static final boolean NOT_IN_SUMMARY = false; 13013 public static final boolean IS_MODIFIER = true; 13014 public static final boolean IS_IN_SUMMARY = true; 13015 public ElementDefinition(boolean defaults, boolean modifier, boolean inSummary) { 13016 super(); 13017 if (defaults) { 13018 setIsModifier(modifier); 13019 setIsSummary(inSummary); 13020 } 13021 } 13022 13023 public String present() { 13024 return hasId() ? getId() : getPath(); 13025 } 13026 13027 public boolean hasCondition(IdType id) { 13028 for (IdType c : getCondition()) { 13029 if (c.primitiveValue().equals(id.primitiveValue())) 13030 return true; 13031 } 13032 return false; 13033 } 13034 13035 public boolean hasConstraint(String key) { 13036 for (ElementDefinitionConstraintComponent c : getConstraint()) { 13037 if (c.getKey().equals(key)) 13038 return true; 13039 } 13040 return false; 13041 } 13042 13043 public boolean hasCode(Coding c) { 13044 for (Coding t : getCode()) { 13045 if (t.getSystem().equals(c.getSystem()) && t.getCode().equals(c.getCode())) 13046 return true; 13047 } 13048 return false; 13049 } 13050 13051 public boolean isChoice() { 13052 return getPath().endsWith("[x]"); 13053 } 13054 13055 public String getName() { 13056 return hasPath() ? getPath().contains(".") ? getPath().substring(getPath().lastIndexOf(".")+1) : getPath() : null; 13057 } 13058 13059 public String getNameBase() { 13060 return getName().replace("[x]", ""); 13061 } 13062 13063 public boolean unbounded() { 13064 return getMax().equals("*") || Integer.parseInt(getMax()) > 1; 13065 } 13066 13067 public boolean isMandatory() { 13068 return getMin() > 0; 13069 } 13070 13071 public boolean isInlineType() { 13072 return getType().size() == 1 && Utilities.existsInList(getType().get(0).getCode(), "Element", "BackboneElement"); 13073 } 13074 13075 13076 public boolean prohibited() { 13077 return "0".equals(getMax()); 13078 } 13079 13080 public boolean hasFixedOrPattern() { 13081 return hasFixed() || hasPattern(); 13082 } 13083 13084 public DataType getFixedOrPattern() { 13085 return hasFixed() ? getFixed() : getPattern(); 13086 } 13087 13088 public boolean isProhibited() { 13089 return "0".equals(getMax()); 13090 } 13091 13092 public boolean isRequired() { 13093 return getMin() == 1; 13094 } 13095 13096 public CanonicalType addValueAlternative(CanonicalType t) { 13097 if (this.valueAlternatives == null) 13098 this.valueAlternatives = new ArrayList<CanonicalType>(); 13099 this.valueAlternatives.add(t); 13100 return t; 13101 } 13102 13103 public boolean repeats() { 13104 return !Utilities.existsInList(getMax(), "0", "1"); 13105 } 13106 13107// end addition 13108 13109} 13110