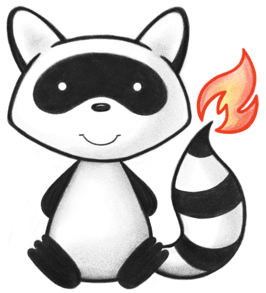
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An interaction between healthcare provider(s), and/or patient(s) for the purpose of providing healthcare service(s) or assessing the health status of patient(s). 052 */ 053@ResourceDef(name="Encounter", profile="http://hl7.org/fhir/StructureDefinition/Encounter") 054public class Encounter extends DomainResource { 055 056 public enum EncounterLocationStatus { 057 /** 058 * The patient is planned to be moved to this location at some point in the future. 059 */ 060 PLANNED, 061 /** 062 * The patient is currently at this location, or was between the period specified.\r\rA system may update these records when the patient leaves the location to either reserved, or completed. 063 */ 064 ACTIVE, 065 /** 066 * This location is held empty for this patient. 067 */ 068 RESERVED, 069 /** 070 * The patient was at this location during the period specified.\r\rNot to be used when the patient is currently at the location. 071 */ 072 COMPLETED, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static EncounterLocationStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("planned".equals(codeString)) 081 return PLANNED; 082 if ("active".equals(codeString)) 083 return ACTIVE; 084 if ("reserved".equals(codeString)) 085 return RESERVED; 086 if ("completed".equals(codeString)) 087 return COMPLETED; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown EncounterLocationStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case PLANNED: return "planned"; 096 case ACTIVE: return "active"; 097 case RESERVED: return "reserved"; 098 case COMPLETED: return "completed"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case PLANNED: return "http://hl7.org/fhir/encounter-location-status"; 106 case ACTIVE: return "http://hl7.org/fhir/encounter-location-status"; 107 case RESERVED: return "http://hl7.org/fhir/encounter-location-status"; 108 case COMPLETED: return "http://hl7.org/fhir/encounter-location-status"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case PLANNED: return "The patient is planned to be moved to this location at some point in the future."; 116 case ACTIVE: return "The patient is currently at this location, or was between the period specified.\r\rA system may update these records when the patient leaves the location to either reserved, or completed."; 117 case RESERVED: return "This location is held empty for this patient."; 118 case COMPLETED: return "The patient was at this location during the period specified.\r\rNot to be used when the patient is currently at the location."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case PLANNED: return "Planned"; 126 case ACTIVE: return "Active"; 127 case RESERVED: return "Reserved"; 128 case COMPLETED: return "Completed"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class EncounterLocationStatusEnumFactory implements EnumFactory<EncounterLocationStatus> { 136 public EncounterLocationStatus fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("planned".equals(codeString)) 141 return EncounterLocationStatus.PLANNED; 142 if ("active".equals(codeString)) 143 return EncounterLocationStatus.ACTIVE; 144 if ("reserved".equals(codeString)) 145 return EncounterLocationStatus.RESERVED; 146 if ("completed".equals(codeString)) 147 return EncounterLocationStatus.COMPLETED; 148 throw new IllegalArgumentException("Unknown EncounterLocationStatus code '"+codeString+"'"); 149 } 150 public Enumeration<EncounterLocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.NULL, code); 158 if ("planned".equals(codeString)) 159 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.PLANNED, code); 160 if ("active".equals(codeString)) 161 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.ACTIVE, code); 162 if ("reserved".equals(codeString)) 163 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.RESERVED, code); 164 if ("completed".equals(codeString)) 165 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.COMPLETED, code); 166 throw new FHIRException("Unknown EncounterLocationStatus code '"+codeString+"'"); 167 } 168 public String toCode(EncounterLocationStatus code) { 169 if (code == EncounterLocationStatus.NULL) 170 return null; 171 if (code == EncounterLocationStatus.PLANNED) 172 return "planned"; 173 if (code == EncounterLocationStatus.ACTIVE) 174 return "active"; 175 if (code == EncounterLocationStatus.RESERVED) 176 return "reserved"; 177 if (code == EncounterLocationStatus.COMPLETED) 178 return "completed"; 179 return "?"; 180 } 181 public String toSystem(EncounterLocationStatus code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class EncounterParticipantComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * Role of participant in encounter. 190 */ 191 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 192 @Description(shortDefinition="Role of participant in encounter", formalDefinition="Role of participant in encounter." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-participant-type") 194 protected List<CodeableConcept> type; 195 196 /** 197 * The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period. 198 */ 199 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="Period of time during the encounter that the participant participated", formalDefinition="The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period." ) 201 protected Period period; 202 203 /** 204 * Person involved in the encounter, the patient/group is also included here to indicate that the patient was actually participating in the encounter. Not including the patient here covers use cases such as a case meeting between practitioners about a patient - non contact times. 205 */ 206 @Child(name = "actor", type = {Patient.class, Group.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, Device.class, HealthcareService.class}, order=3, min=0, max=1, modifier=false, summary=true) 207 @Description(shortDefinition="The individual, device, or service participating in the encounter", formalDefinition="Person involved in the encounter, the patient/group is also included here to indicate that the patient was actually participating in the encounter. Not including the patient here covers use cases such as a case meeting between practitioners about a patient - non contact times." ) 208 protected Reference actor; 209 210 private static final long serialVersionUID = 1982623707L; 211 212 /** 213 * Constructor 214 */ 215 public EncounterParticipantComponent() { 216 super(); 217 } 218 219 /** 220 * @return {@link #type} (Role of participant in encounter.) 221 */ 222 public List<CodeableConcept> getType() { 223 if (this.type == null) 224 this.type = new ArrayList<CodeableConcept>(); 225 return this.type; 226 } 227 228 /** 229 * @return Returns a reference to <code>this</code> for easy method chaining 230 */ 231 public EncounterParticipantComponent setType(List<CodeableConcept> theType) { 232 this.type = theType; 233 return this; 234 } 235 236 public boolean hasType() { 237 if (this.type == null) 238 return false; 239 for (CodeableConcept item : this.type) 240 if (!item.isEmpty()) 241 return true; 242 return false; 243 } 244 245 public CodeableConcept addType() { //3 246 CodeableConcept t = new CodeableConcept(); 247 if (this.type == null) 248 this.type = new ArrayList<CodeableConcept>(); 249 this.type.add(t); 250 return t; 251 } 252 253 public EncounterParticipantComponent addType(CodeableConcept t) { //3 254 if (t == null) 255 return this; 256 if (this.type == null) 257 this.type = new ArrayList<CodeableConcept>(); 258 this.type.add(t); 259 return this; 260 } 261 262 /** 263 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 264 */ 265 public CodeableConcept getTypeFirstRep() { 266 if (getType().isEmpty()) { 267 addType(); 268 } 269 return getType().get(0); 270 } 271 272 /** 273 * @return {@link #period} (The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.) 274 */ 275 public Period getPeriod() { 276 if (this.period == null) 277 if (Configuration.errorOnAutoCreate()) 278 throw new Error("Attempt to auto-create EncounterParticipantComponent.period"); 279 else if (Configuration.doAutoCreate()) 280 this.period = new Period(); // cc 281 return this.period; 282 } 283 284 public boolean hasPeriod() { 285 return this.period != null && !this.period.isEmpty(); 286 } 287 288 /** 289 * @param value {@link #period} (The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.) 290 */ 291 public EncounterParticipantComponent setPeriod(Period value) { 292 this.period = value; 293 return this; 294 } 295 296 /** 297 * @return {@link #actor} (Person involved in the encounter, the patient/group is also included here to indicate that the patient was actually participating in the encounter. Not including the patient here covers use cases such as a case meeting between practitioners about a patient - non contact times.) 298 */ 299 public Reference getActor() { 300 if (this.actor == null) 301 if (Configuration.errorOnAutoCreate()) 302 throw new Error("Attempt to auto-create EncounterParticipantComponent.actor"); 303 else if (Configuration.doAutoCreate()) 304 this.actor = new Reference(); // cc 305 return this.actor; 306 } 307 308 public boolean hasActor() { 309 return this.actor != null && !this.actor.isEmpty(); 310 } 311 312 /** 313 * @param value {@link #actor} (Person involved in the encounter, the patient/group is also included here to indicate that the patient was actually participating in the encounter. Not including the patient here covers use cases such as a case meeting between practitioners about a patient - non contact times.) 314 */ 315 public EncounterParticipantComponent setActor(Reference value) { 316 this.actor = value; 317 return this; 318 } 319 320 protected void listChildren(List<Property> children) { 321 super.listChildren(children); 322 children.add(new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, java.lang.Integer.MAX_VALUE, type)); 323 children.add(new Property("period", "Period", "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 0, 1, period)); 324 children.add(new Property("actor", "Reference(Patient|Group|RelatedPerson|Practitioner|PractitionerRole|Device|HealthcareService)", "Person involved in the encounter, the patient/group is also included here to indicate that the patient was actually participating in the encounter. Not including the patient here covers use cases such as a case meeting between practitioners about a patient - non contact times.", 0, 1, actor)); 325 } 326 327 @Override 328 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 329 switch (_hash) { 330 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, java.lang.Integer.MAX_VALUE, type); 331 case -991726143: /*period*/ return new Property("period", "Period", "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 0, 1, period); 332 case 92645877: /*actor*/ return new Property("actor", "Reference(Patient|Group|RelatedPerson|Practitioner|PractitionerRole|Device|HealthcareService)", "Person involved in the encounter, the patient/group is also included here to indicate that the patient was actually participating in the encounter. Not including the patient here covers use cases such as a case meeting between practitioners about a patient - non contact times.", 0, 1, actor); 333 default: return super.getNamedProperty(_hash, _name, _checkValid); 334 } 335 336 } 337 338 @Override 339 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 340 switch (hash) { 341 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 342 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 343 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 344 default: return super.getProperty(hash, name, checkValid); 345 } 346 347 } 348 349 @Override 350 public Base setProperty(int hash, String name, Base value) throws FHIRException { 351 switch (hash) { 352 case 3575610: // type 353 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 354 return value; 355 case -991726143: // period 356 this.period = TypeConvertor.castToPeriod(value); // Period 357 return value; 358 case 92645877: // actor 359 this.actor = TypeConvertor.castToReference(value); // Reference 360 return value; 361 default: return super.setProperty(hash, name, value); 362 } 363 364 } 365 366 @Override 367 public Base setProperty(String name, Base value) throws FHIRException { 368 if (name.equals("type")) { 369 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 370 } else if (name.equals("period")) { 371 this.period = TypeConvertor.castToPeriod(value); // Period 372 } else if (name.equals("actor")) { 373 this.actor = TypeConvertor.castToReference(value); // Reference 374 } else 375 return super.setProperty(name, value); 376 return value; 377 } 378 379 @Override 380 public void removeChild(String name, Base value) throws FHIRException { 381 if (name.equals("type")) { 382 this.getType().remove(value); 383 } else if (name.equals("period")) { 384 this.period = null; 385 } else if (name.equals("actor")) { 386 this.actor = null; 387 } else 388 super.removeChild(name, value); 389 390 } 391 392 @Override 393 public Base makeProperty(int hash, String name) throws FHIRException { 394 switch (hash) { 395 case 3575610: return addType(); 396 case -991726143: return getPeriod(); 397 case 92645877: return getActor(); 398 default: return super.makeProperty(hash, name); 399 } 400 401 } 402 403 @Override 404 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 405 switch (hash) { 406 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 407 case -991726143: /*period*/ return new String[] {"Period"}; 408 case 92645877: /*actor*/ return new String[] {"Reference"}; 409 default: return super.getTypesForProperty(hash, name); 410 } 411 412 } 413 414 @Override 415 public Base addChild(String name) throws FHIRException { 416 if (name.equals("type")) { 417 return addType(); 418 } 419 else if (name.equals("period")) { 420 this.period = new Period(); 421 return this.period; 422 } 423 else if (name.equals("actor")) { 424 this.actor = new Reference(); 425 return this.actor; 426 } 427 else 428 return super.addChild(name); 429 } 430 431 public EncounterParticipantComponent copy() { 432 EncounterParticipantComponent dst = new EncounterParticipantComponent(); 433 copyValues(dst); 434 return dst; 435 } 436 437 public void copyValues(EncounterParticipantComponent dst) { 438 super.copyValues(dst); 439 if (type != null) { 440 dst.type = new ArrayList<CodeableConcept>(); 441 for (CodeableConcept i : type) 442 dst.type.add(i.copy()); 443 }; 444 dst.period = period == null ? null : period.copy(); 445 dst.actor = actor == null ? null : actor.copy(); 446 } 447 448 @Override 449 public boolean equalsDeep(Base other_) { 450 if (!super.equalsDeep(other_)) 451 return false; 452 if (!(other_ instanceof EncounterParticipantComponent)) 453 return false; 454 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 455 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) && compareDeep(actor, o.actor, true) 456 ; 457 } 458 459 @Override 460 public boolean equalsShallow(Base other_) { 461 if (!super.equalsShallow(other_)) 462 return false; 463 if (!(other_ instanceof EncounterParticipantComponent)) 464 return false; 465 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 466 return true; 467 } 468 469 public boolean isEmpty() { 470 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, actor); 471 } 472 473 public String fhirType() { 474 return "Encounter.participant"; 475 476 } 477 478 } 479 480 @Block() 481 public static class ReasonComponent extends BackboneElement implements IBaseBackboneElement { 482 /** 483 * What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening). 484 */ 485 @Child(name = "use", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 486 @Description(shortDefinition="What the reason value should be used for/as", formalDefinition="What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening)." ) 487 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-reason-use") 488 protected List<CodeableConcept> use; 489 490 /** 491 * Reason the encounter takes place, expressed as a code or a reference to another resource. For admissions, this can be used for a coded admission diagnosis. 492 */ 493 @Child(name = "value", type = {CodeableReference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 494 @Description(shortDefinition="Reason the encounter takes place (core or reference)", formalDefinition="Reason the encounter takes place, expressed as a code or a reference to another resource. For admissions, this can be used for a coded admission diagnosis." ) 495 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-reason") 496 protected List<CodeableReference> value; 497 498 private static final long serialVersionUID = 1305979913L; 499 500 /** 501 * Constructor 502 */ 503 public ReasonComponent() { 504 super(); 505 } 506 507 /** 508 * @return {@link #use} (What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening).) 509 */ 510 public List<CodeableConcept> getUse() { 511 if (this.use == null) 512 this.use = new ArrayList<CodeableConcept>(); 513 return this.use; 514 } 515 516 /** 517 * @return Returns a reference to <code>this</code> for easy method chaining 518 */ 519 public ReasonComponent setUse(List<CodeableConcept> theUse) { 520 this.use = theUse; 521 return this; 522 } 523 524 public boolean hasUse() { 525 if (this.use == null) 526 return false; 527 for (CodeableConcept item : this.use) 528 if (!item.isEmpty()) 529 return true; 530 return false; 531 } 532 533 public CodeableConcept addUse() { //3 534 CodeableConcept t = new CodeableConcept(); 535 if (this.use == null) 536 this.use = new ArrayList<CodeableConcept>(); 537 this.use.add(t); 538 return t; 539 } 540 541 public ReasonComponent addUse(CodeableConcept t) { //3 542 if (t == null) 543 return this; 544 if (this.use == null) 545 this.use = new ArrayList<CodeableConcept>(); 546 this.use.add(t); 547 return this; 548 } 549 550 /** 551 * @return The first repetition of repeating field {@link #use}, creating it if it does not already exist {3} 552 */ 553 public CodeableConcept getUseFirstRep() { 554 if (getUse().isEmpty()) { 555 addUse(); 556 } 557 return getUse().get(0); 558 } 559 560 /** 561 * @return {@link #value} (Reason the encounter takes place, expressed as a code or a reference to another resource. For admissions, this can be used for a coded admission diagnosis.) 562 */ 563 public List<CodeableReference> getValue() { 564 if (this.value == null) 565 this.value = new ArrayList<CodeableReference>(); 566 return this.value; 567 } 568 569 /** 570 * @return Returns a reference to <code>this</code> for easy method chaining 571 */ 572 public ReasonComponent setValue(List<CodeableReference> theValue) { 573 this.value = theValue; 574 return this; 575 } 576 577 public boolean hasValue() { 578 if (this.value == null) 579 return false; 580 for (CodeableReference item : this.value) 581 if (!item.isEmpty()) 582 return true; 583 return false; 584 } 585 586 public CodeableReference addValue() { //3 587 CodeableReference t = new CodeableReference(); 588 if (this.value == null) 589 this.value = new ArrayList<CodeableReference>(); 590 this.value.add(t); 591 return t; 592 } 593 594 public ReasonComponent addValue(CodeableReference t) { //3 595 if (t == null) 596 return this; 597 if (this.value == null) 598 this.value = new ArrayList<CodeableReference>(); 599 this.value.add(t); 600 return this; 601 } 602 603 /** 604 * @return The first repetition of repeating field {@link #value}, creating it if it does not already exist {3} 605 */ 606 public CodeableReference getValueFirstRep() { 607 if (getValue().isEmpty()) { 608 addValue(); 609 } 610 return getValue().get(0); 611 } 612 613 protected void listChildren(List<Property> children) { 614 super.listChildren(children); 615 children.add(new Property("use", "CodeableConcept", "What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening).", 0, java.lang.Integer.MAX_VALUE, use)); 616 children.add(new Property("value", "CodeableReference(Condition|DiagnosticReport|Observation|ImmunizationRecommendation|Procedure)", "Reason the encounter takes place, expressed as a code or a reference to another resource. For admissions, this can be used for a coded admission diagnosis.", 0, java.lang.Integer.MAX_VALUE, value)); 617 } 618 619 @Override 620 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 621 switch (_hash) { 622 case 116103: /*use*/ return new Property("use", "CodeableConcept", "What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening).", 0, java.lang.Integer.MAX_VALUE, use); 623 case 111972721: /*value*/ return new Property("value", "CodeableReference(Condition|DiagnosticReport|Observation|ImmunizationRecommendation|Procedure)", "Reason the encounter takes place, expressed as a code or a reference to another resource. For admissions, this can be used for a coded admission diagnosis.", 0, java.lang.Integer.MAX_VALUE, value); 624 default: return super.getNamedProperty(_hash, _name, _checkValid); 625 } 626 627 } 628 629 @Override 630 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 631 switch (hash) { 632 case 116103: /*use*/ return this.use == null ? new Base[0] : this.use.toArray(new Base[this.use.size()]); // CodeableConcept 633 case 111972721: /*value*/ return this.value == null ? new Base[0] : this.value.toArray(new Base[this.value.size()]); // CodeableReference 634 default: return super.getProperty(hash, name, checkValid); 635 } 636 637 } 638 639 @Override 640 public Base setProperty(int hash, String name, Base value) throws FHIRException { 641 switch (hash) { 642 case 116103: // use 643 this.getUse().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 644 return value; 645 case 111972721: // value 646 this.getValue().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 647 return value; 648 default: return super.setProperty(hash, name, value); 649 } 650 651 } 652 653 @Override 654 public Base setProperty(String name, Base value) throws FHIRException { 655 if (name.equals("use")) { 656 this.getUse().add(TypeConvertor.castToCodeableConcept(value)); 657 } else if (name.equals("value")) { 658 this.getValue().add(TypeConvertor.castToCodeableReference(value)); 659 } else 660 return super.setProperty(name, value); 661 return value; 662 } 663 664 @Override 665 public void removeChild(String name, Base value) throws FHIRException { 666 if (name.equals("use")) { 667 this.getUse().remove(value); 668 } else if (name.equals("value")) { 669 this.getValue().remove(value); 670 } else 671 super.removeChild(name, value); 672 673 } 674 675 @Override 676 public Base makeProperty(int hash, String name) throws FHIRException { 677 switch (hash) { 678 case 116103: return addUse(); 679 case 111972721: return addValue(); 680 default: return super.makeProperty(hash, name); 681 } 682 683 } 684 685 @Override 686 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 687 switch (hash) { 688 case 116103: /*use*/ return new String[] {"CodeableConcept"}; 689 case 111972721: /*value*/ return new String[] {"CodeableReference"}; 690 default: return super.getTypesForProperty(hash, name); 691 } 692 693 } 694 695 @Override 696 public Base addChild(String name) throws FHIRException { 697 if (name.equals("use")) { 698 return addUse(); 699 } 700 else if (name.equals("value")) { 701 return addValue(); 702 } 703 else 704 return super.addChild(name); 705 } 706 707 public ReasonComponent copy() { 708 ReasonComponent dst = new ReasonComponent(); 709 copyValues(dst); 710 return dst; 711 } 712 713 public void copyValues(ReasonComponent dst) { 714 super.copyValues(dst); 715 if (use != null) { 716 dst.use = new ArrayList<CodeableConcept>(); 717 for (CodeableConcept i : use) 718 dst.use.add(i.copy()); 719 }; 720 if (value != null) { 721 dst.value = new ArrayList<CodeableReference>(); 722 for (CodeableReference i : value) 723 dst.value.add(i.copy()); 724 }; 725 } 726 727 @Override 728 public boolean equalsDeep(Base other_) { 729 if (!super.equalsDeep(other_)) 730 return false; 731 if (!(other_ instanceof ReasonComponent)) 732 return false; 733 ReasonComponent o = (ReasonComponent) other_; 734 return compareDeep(use, o.use, true) && compareDeep(value, o.value, true); 735 } 736 737 @Override 738 public boolean equalsShallow(Base other_) { 739 if (!super.equalsShallow(other_)) 740 return false; 741 if (!(other_ instanceof ReasonComponent)) 742 return false; 743 ReasonComponent o = (ReasonComponent) other_; 744 return true; 745 } 746 747 public boolean isEmpty() { 748 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, value); 749 } 750 751 public String fhirType() { 752 return "Encounter.reason"; 753 754 } 755 756 } 757 758 @Block() 759 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 760 /** 761 * The coded diagnosis or a reference to a Condition (with other resources referenced in the evidence.detail), the use property will indicate the purpose of this specific diagnosis. 762 */ 763 @Child(name = "condition", type = {CodeableReference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 764 @Description(shortDefinition="The diagnosis relevant to the encounter", formalDefinition="The coded diagnosis or a reference to a Condition (with other resources referenced in the evidence.detail), the use property will indicate the purpose of this specific diagnosis." ) 765 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 766 protected List<CodeableReference> condition; 767 768 /** 769 * Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?). 770 */ 771 @Child(name = "use", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 772 @Description(shortDefinition="Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?)", formalDefinition="Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?)." ) 773 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-diagnosis-use") 774 protected List<CodeableConcept> use; 775 776 private static final long serialVersionUID = 1029565663L; 777 778 /** 779 * Constructor 780 */ 781 public DiagnosisComponent() { 782 super(); 783 } 784 785 /** 786 * @return {@link #condition} (The coded diagnosis or a reference to a Condition (with other resources referenced in the evidence.detail), the use property will indicate the purpose of this specific diagnosis.) 787 */ 788 public List<CodeableReference> getCondition() { 789 if (this.condition == null) 790 this.condition = new ArrayList<CodeableReference>(); 791 return this.condition; 792 } 793 794 /** 795 * @return Returns a reference to <code>this</code> for easy method chaining 796 */ 797 public DiagnosisComponent setCondition(List<CodeableReference> theCondition) { 798 this.condition = theCondition; 799 return this; 800 } 801 802 public boolean hasCondition() { 803 if (this.condition == null) 804 return false; 805 for (CodeableReference item : this.condition) 806 if (!item.isEmpty()) 807 return true; 808 return false; 809 } 810 811 public CodeableReference addCondition() { //3 812 CodeableReference t = new CodeableReference(); 813 if (this.condition == null) 814 this.condition = new ArrayList<CodeableReference>(); 815 this.condition.add(t); 816 return t; 817 } 818 819 public DiagnosisComponent addCondition(CodeableReference t) { //3 820 if (t == null) 821 return this; 822 if (this.condition == null) 823 this.condition = new ArrayList<CodeableReference>(); 824 this.condition.add(t); 825 return this; 826 } 827 828 /** 829 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 830 */ 831 public CodeableReference getConditionFirstRep() { 832 if (getCondition().isEmpty()) { 833 addCondition(); 834 } 835 return getCondition().get(0); 836 } 837 838 /** 839 * @return {@link #use} (Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).) 840 */ 841 public List<CodeableConcept> getUse() { 842 if (this.use == null) 843 this.use = new ArrayList<CodeableConcept>(); 844 return this.use; 845 } 846 847 /** 848 * @return Returns a reference to <code>this</code> for easy method chaining 849 */ 850 public DiagnosisComponent setUse(List<CodeableConcept> theUse) { 851 this.use = theUse; 852 return this; 853 } 854 855 public boolean hasUse() { 856 if (this.use == null) 857 return false; 858 for (CodeableConcept item : this.use) 859 if (!item.isEmpty()) 860 return true; 861 return false; 862 } 863 864 public CodeableConcept addUse() { //3 865 CodeableConcept t = new CodeableConcept(); 866 if (this.use == null) 867 this.use = new ArrayList<CodeableConcept>(); 868 this.use.add(t); 869 return t; 870 } 871 872 public DiagnosisComponent addUse(CodeableConcept t) { //3 873 if (t == null) 874 return this; 875 if (this.use == null) 876 this.use = new ArrayList<CodeableConcept>(); 877 this.use.add(t); 878 return this; 879 } 880 881 /** 882 * @return The first repetition of repeating field {@link #use}, creating it if it does not already exist {3} 883 */ 884 public CodeableConcept getUseFirstRep() { 885 if (getUse().isEmpty()) { 886 addUse(); 887 } 888 return getUse().get(0); 889 } 890 891 protected void listChildren(List<Property> children) { 892 super.listChildren(children); 893 children.add(new Property("condition", "CodeableReference(Condition)", "The coded diagnosis or a reference to a Condition (with other resources referenced in the evidence.detail), the use property will indicate the purpose of this specific diagnosis.", 0, java.lang.Integer.MAX_VALUE, condition)); 894 children.add(new Property("use", "CodeableConcept", "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, java.lang.Integer.MAX_VALUE, use)); 895 } 896 897 @Override 898 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 899 switch (_hash) { 900 case -861311717: /*condition*/ return new Property("condition", "CodeableReference(Condition)", "The coded diagnosis or a reference to a Condition (with other resources referenced in the evidence.detail), the use property will indicate the purpose of this specific diagnosis.", 0, java.lang.Integer.MAX_VALUE, condition); 901 case 116103: /*use*/ return new Property("use", "CodeableConcept", "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, java.lang.Integer.MAX_VALUE, use); 902 default: return super.getNamedProperty(_hash, _name, _checkValid); 903 } 904 905 } 906 907 @Override 908 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 909 switch (hash) { 910 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // CodeableReference 911 case 116103: /*use*/ return this.use == null ? new Base[0] : this.use.toArray(new Base[this.use.size()]); // CodeableConcept 912 default: return super.getProperty(hash, name, checkValid); 913 } 914 915 } 916 917 @Override 918 public Base setProperty(int hash, String name, Base value) throws FHIRException { 919 switch (hash) { 920 case -861311717: // condition 921 this.getCondition().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 922 return value; 923 case 116103: // use 924 this.getUse().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 925 return value; 926 default: return super.setProperty(hash, name, value); 927 } 928 929 } 930 931 @Override 932 public Base setProperty(String name, Base value) throws FHIRException { 933 if (name.equals("condition")) { 934 this.getCondition().add(TypeConvertor.castToCodeableReference(value)); 935 } else if (name.equals("use")) { 936 this.getUse().add(TypeConvertor.castToCodeableConcept(value)); 937 } else 938 return super.setProperty(name, value); 939 return value; 940 } 941 942 @Override 943 public void removeChild(String name, Base value) throws FHIRException { 944 if (name.equals("condition")) { 945 this.getCondition().remove(value); 946 } else if (name.equals("use")) { 947 this.getUse().remove(value); 948 } else 949 super.removeChild(name, value); 950 951 } 952 953 @Override 954 public Base makeProperty(int hash, String name) throws FHIRException { 955 switch (hash) { 956 case -861311717: return addCondition(); 957 case 116103: return addUse(); 958 default: return super.makeProperty(hash, name); 959 } 960 961 } 962 963 @Override 964 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 965 switch (hash) { 966 case -861311717: /*condition*/ return new String[] {"CodeableReference"}; 967 case 116103: /*use*/ return new String[] {"CodeableConcept"}; 968 default: return super.getTypesForProperty(hash, name); 969 } 970 971 } 972 973 @Override 974 public Base addChild(String name) throws FHIRException { 975 if (name.equals("condition")) { 976 return addCondition(); 977 } 978 else if (name.equals("use")) { 979 return addUse(); 980 } 981 else 982 return super.addChild(name); 983 } 984 985 public DiagnosisComponent copy() { 986 DiagnosisComponent dst = new DiagnosisComponent(); 987 copyValues(dst); 988 return dst; 989 } 990 991 public void copyValues(DiagnosisComponent dst) { 992 super.copyValues(dst); 993 if (condition != null) { 994 dst.condition = new ArrayList<CodeableReference>(); 995 for (CodeableReference i : condition) 996 dst.condition.add(i.copy()); 997 }; 998 if (use != null) { 999 dst.use = new ArrayList<CodeableConcept>(); 1000 for (CodeableConcept i : use) 1001 dst.use.add(i.copy()); 1002 }; 1003 } 1004 1005 @Override 1006 public boolean equalsDeep(Base other_) { 1007 if (!super.equalsDeep(other_)) 1008 return false; 1009 if (!(other_ instanceof DiagnosisComponent)) 1010 return false; 1011 DiagnosisComponent o = (DiagnosisComponent) other_; 1012 return compareDeep(condition, o.condition, true) && compareDeep(use, o.use, true); 1013 } 1014 1015 @Override 1016 public boolean equalsShallow(Base other_) { 1017 if (!super.equalsShallow(other_)) 1018 return false; 1019 if (!(other_ instanceof DiagnosisComponent)) 1020 return false; 1021 DiagnosisComponent o = (DiagnosisComponent) other_; 1022 return true; 1023 } 1024 1025 public boolean isEmpty() { 1026 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, use); 1027 } 1028 1029 public String fhirType() { 1030 return "Encounter.diagnosis"; 1031 1032 } 1033 1034 } 1035 1036 @Block() 1037 public static class EncounterAdmissionComponent extends BackboneElement implements IBaseBackboneElement { 1038 /** 1039 * Pre-admission identifier. 1040 */ 1041 @Child(name = "preAdmissionIdentifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 1042 @Description(shortDefinition="Pre-admission identifier", formalDefinition="Pre-admission identifier." ) 1043 protected Identifier preAdmissionIdentifier; 1044 1045 /** 1046 * The location/organization from which the patient came before admission. 1047 */ 1048 @Child(name = "origin", type = {Location.class, Organization.class}, order=2, min=0, max=1, modifier=false, summary=false) 1049 @Description(shortDefinition="The location/organization from which the patient came before admission", formalDefinition="The location/organization from which the patient came before admission." ) 1050 protected Reference origin; 1051 1052 /** 1053 * From where patient was admitted (physician referral, transfer). 1054 */ 1055 @Child(name = "admitSource", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1056 @Description(shortDefinition="From where patient was admitted (physician referral, transfer)", formalDefinition="From where patient was admitted (physician referral, transfer)." ) 1057 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-admit-source") 1058 protected CodeableConcept admitSource; 1059 1060 /** 1061 * Indicates that this encounter is directly related to a prior admission, often because the conditions addressed in the prior admission were not fully addressed. 1062 */ 1063 @Child(name = "reAdmission", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1064 @Description(shortDefinition="Indicates that the patient is being re-admitted", formalDefinition="Indicates that this encounter is directly related to a prior admission, often because the conditions addressed in the prior admission were not fully addressed." ) 1065 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0092") 1066 protected CodeableConcept reAdmission; 1067 1068 /** 1069 * Location/organization to which the patient is discharged. 1070 */ 1071 @Child(name = "destination", type = {Location.class, Organization.class}, order=5, min=0, max=1, modifier=false, summary=false) 1072 @Description(shortDefinition="Location/organization to which the patient is discharged", formalDefinition="Location/organization to which the patient is discharged." ) 1073 protected Reference destination; 1074 1075 /** 1076 * Category or kind of location after discharge. 1077 */ 1078 @Child(name = "dischargeDisposition", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1079 @Description(shortDefinition="Category or kind of location after discharge", formalDefinition="Category or kind of location after discharge." ) 1080 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-discharge-disposition") 1081 protected CodeableConcept dischargeDisposition; 1082 1083 private static final long serialVersionUID = -1702856594L; 1084 1085 /** 1086 * Constructor 1087 */ 1088 public EncounterAdmissionComponent() { 1089 super(); 1090 } 1091 1092 /** 1093 * @return {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1094 */ 1095 public Identifier getPreAdmissionIdentifier() { 1096 if (this.preAdmissionIdentifier == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create EncounterAdmissionComponent.preAdmissionIdentifier"); 1099 else if (Configuration.doAutoCreate()) 1100 this.preAdmissionIdentifier = new Identifier(); // cc 1101 return this.preAdmissionIdentifier; 1102 } 1103 1104 public boolean hasPreAdmissionIdentifier() { 1105 return this.preAdmissionIdentifier != null && !this.preAdmissionIdentifier.isEmpty(); 1106 } 1107 1108 /** 1109 * @param value {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1110 */ 1111 public EncounterAdmissionComponent setPreAdmissionIdentifier(Identifier value) { 1112 this.preAdmissionIdentifier = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #origin} (The location/organization from which the patient came before admission.) 1118 */ 1119 public Reference getOrigin() { 1120 if (this.origin == null) 1121 if (Configuration.errorOnAutoCreate()) 1122 throw new Error("Attempt to auto-create EncounterAdmissionComponent.origin"); 1123 else if (Configuration.doAutoCreate()) 1124 this.origin = new Reference(); // cc 1125 return this.origin; 1126 } 1127 1128 public boolean hasOrigin() { 1129 return this.origin != null && !this.origin.isEmpty(); 1130 } 1131 1132 /** 1133 * @param value {@link #origin} (The location/organization from which the patient came before admission.) 1134 */ 1135 public EncounterAdmissionComponent setOrigin(Reference value) { 1136 this.origin = value; 1137 return this; 1138 } 1139 1140 /** 1141 * @return {@link #admitSource} (From where patient was admitted (physician referral, transfer).) 1142 */ 1143 public CodeableConcept getAdmitSource() { 1144 if (this.admitSource == null) 1145 if (Configuration.errorOnAutoCreate()) 1146 throw new Error("Attempt to auto-create EncounterAdmissionComponent.admitSource"); 1147 else if (Configuration.doAutoCreate()) 1148 this.admitSource = new CodeableConcept(); // cc 1149 return this.admitSource; 1150 } 1151 1152 public boolean hasAdmitSource() { 1153 return this.admitSource != null && !this.admitSource.isEmpty(); 1154 } 1155 1156 /** 1157 * @param value {@link #admitSource} (From where patient was admitted (physician referral, transfer).) 1158 */ 1159 public EncounterAdmissionComponent setAdmitSource(CodeableConcept value) { 1160 this.admitSource = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return {@link #reAdmission} (Indicates that this encounter is directly related to a prior admission, often because the conditions addressed in the prior admission were not fully addressed.) 1166 */ 1167 public CodeableConcept getReAdmission() { 1168 if (this.reAdmission == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create EncounterAdmissionComponent.reAdmission"); 1171 else if (Configuration.doAutoCreate()) 1172 this.reAdmission = new CodeableConcept(); // cc 1173 return this.reAdmission; 1174 } 1175 1176 public boolean hasReAdmission() { 1177 return this.reAdmission != null && !this.reAdmission.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #reAdmission} (Indicates that this encounter is directly related to a prior admission, often because the conditions addressed in the prior admission were not fully addressed.) 1182 */ 1183 public EncounterAdmissionComponent setReAdmission(CodeableConcept value) { 1184 this.reAdmission = value; 1185 return this; 1186 } 1187 1188 /** 1189 * @return {@link #destination} (Location/organization to which the patient is discharged.) 1190 */ 1191 public Reference getDestination() { 1192 if (this.destination == null) 1193 if (Configuration.errorOnAutoCreate()) 1194 throw new Error("Attempt to auto-create EncounterAdmissionComponent.destination"); 1195 else if (Configuration.doAutoCreate()) 1196 this.destination = new Reference(); // cc 1197 return this.destination; 1198 } 1199 1200 public boolean hasDestination() { 1201 return this.destination != null && !this.destination.isEmpty(); 1202 } 1203 1204 /** 1205 * @param value {@link #destination} (Location/organization to which the patient is discharged.) 1206 */ 1207 public EncounterAdmissionComponent setDestination(Reference value) { 1208 this.destination = value; 1209 return this; 1210 } 1211 1212 /** 1213 * @return {@link #dischargeDisposition} (Category or kind of location after discharge.) 1214 */ 1215 public CodeableConcept getDischargeDisposition() { 1216 if (this.dischargeDisposition == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create EncounterAdmissionComponent.dischargeDisposition"); 1219 else if (Configuration.doAutoCreate()) 1220 this.dischargeDisposition = new CodeableConcept(); // cc 1221 return this.dischargeDisposition; 1222 } 1223 1224 public boolean hasDischargeDisposition() { 1225 return this.dischargeDisposition != null && !this.dischargeDisposition.isEmpty(); 1226 } 1227 1228 /** 1229 * @param value {@link #dischargeDisposition} (Category or kind of location after discharge.) 1230 */ 1231 public EncounterAdmissionComponent setDischargeDisposition(CodeableConcept value) { 1232 this.dischargeDisposition = value; 1233 return this; 1234 } 1235 1236 protected void listChildren(List<Property> children) { 1237 super.listChildren(children); 1238 children.add(new Property("preAdmissionIdentifier", "Identifier", "Pre-admission identifier.", 0, 1, preAdmissionIdentifier)); 1239 children.add(new Property("origin", "Reference(Location|Organization)", "The location/organization from which the patient came before admission.", 0, 1, origin)); 1240 children.add(new Property("admitSource", "CodeableConcept", "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource)); 1241 children.add(new Property("reAdmission", "CodeableConcept", "Indicates that this encounter is directly related to a prior admission, often because the conditions addressed in the prior admission were not fully addressed.", 0, 1, reAdmission)); 1242 children.add(new Property("destination", "Reference(Location|Organization)", "Location/organization to which the patient is discharged.", 0, 1, destination)); 1243 children.add(new Property("dischargeDisposition", "CodeableConcept", "Category or kind of location after discharge.", 0, 1, dischargeDisposition)); 1244 } 1245 1246 @Override 1247 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1248 switch (_hash) { 1249 case -965394961: /*preAdmissionIdentifier*/ return new Property("preAdmissionIdentifier", "Identifier", "Pre-admission identifier.", 0, 1, preAdmissionIdentifier); 1250 case -1008619738: /*origin*/ return new Property("origin", "Reference(Location|Organization)", "The location/organization from which the patient came before admission.", 0, 1, origin); 1251 case 538887120: /*admitSource*/ return new Property("admitSource", "CodeableConcept", "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource); 1252 case 669348630: /*reAdmission*/ return new Property("reAdmission", "CodeableConcept", "Indicates that this encounter is directly related to a prior admission, often because the conditions addressed in the prior admission were not fully addressed.", 0, 1, reAdmission); 1253 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location|Organization)", "Location/organization to which the patient is discharged.", 0, 1, destination); 1254 case 528065941: /*dischargeDisposition*/ return new Property("dischargeDisposition", "CodeableConcept", "Category or kind of location after discharge.", 0, 1, dischargeDisposition); 1255 default: return super.getNamedProperty(_hash, _name, _checkValid); 1256 } 1257 1258 } 1259 1260 @Override 1261 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1262 switch (hash) { 1263 case -965394961: /*preAdmissionIdentifier*/ return this.preAdmissionIdentifier == null ? new Base[0] : new Base[] {this.preAdmissionIdentifier}; // Identifier 1264 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : new Base[] {this.origin}; // Reference 1265 case 538887120: /*admitSource*/ return this.admitSource == null ? new Base[0] : new Base[] {this.admitSource}; // CodeableConcept 1266 case 669348630: /*reAdmission*/ return this.reAdmission == null ? new Base[0] : new Base[] {this.reAdmission}; // CodeableConcept 1267 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 1268 case 528065941: /*dischargeDisposition*/ return this.dischargeDisposition == null ? new Base[0] : new Base[] {this.dischargeDisposition}; // CodeableConcept 1269 default: return super.getProperty(hash, name, checkValid); 1270 } 1271 1272 } 1273 1274 @Override 1275 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1276 switch (hash) { 1277 case -965394961: // preAdmissionIdentifier 1278 this.preAdmissionIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 1279 return value; 1280 case -1008619738: // origin 1281 this.origin = TypeConvertor.castToReference(value); // Reference 1282 return value; 1283 case 538887120: // admitSource 1284 this.admitSource = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1285 return value; 1286 case 669348630: // reAdmission 1287 this.reAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1288 return value; 1289 case -1429847026: // destination 1290 this.destination = TypeConvertor.castToReference(value); // Reference 1291 return value; 1292 case 528065941: // dischargeDisposition 1293 this.dischargeDisposition = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1294 return value; 1295 default: return super.setProperty(hash, name, value); 1296 } 1297 1298 } 1299 1300 @Override 1301 public Base setProperty(String name, Base value) throws FHIRException { 1302 if (name.equals("preAdmissionIdentifier")) { 1303 this.preAdmissionIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 1304 } else if (name.equals("origin")) { 1305 this.origin = TypeConvertor.castToReference(value); // Reference 1306 } else if (name.equals("admitSource")) { 1307 this.admitSource = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1308 } else if (name.equals("reAdmission")) { 1309 this.reAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1310 } else if (name.equals("destination")) { 1311 this.destination = TypeConvertor.castToReference(value); // Reference 1312 } else if (name.equals("dischargeDisposition")) { 1313 this.dischargeDisposition = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1314 } else 1315 return super.setProperty(name, value); 1316 return value; 1317 } 1318 1319 @Override 1320 public void removeChild(String name, Base value) throws FHIRException { 1321 if (name.equals("preAdmissionIdentifier")) { 1322 this.preAdmissionIdentifier = null; 1323 } else if (name.equals("origin")) { 1324 this.origin = null; 1325 } else if (name.equals("admitSource")) { 1326 this.admitSource = null; 1327 } else if (name.equals("reAdmission")) { 1328 this.reAdmission = null; 1329 } else if (name.equals("destination")) { 1330 this.destination = null; 1331 } else if (name.equals("dischargeDisposition")) { 1332 this.dischargeDisposition = null; 1333 } else 1334 super.removeChild(name, value); 1335 1336 } 1337 1338 @Override 1339 public Base makeProperty(int hash, String name) throws FHIRException { 1340 switch (hash) { 1341 case -965394961: return getPreAdmissionIdentifier(); 1342 case -1008619738: return getOrigin(); 1343 case 538887120: return getAdmitSource(); 1344 case 669348630: return getReAdmission(); 1345 case -1429847026: return getDestination(); 1346 case 528065941: return getDischargeDisposition(); 1347 default: return super.makeProperty(hash, name); 1348 } 1349 1350 } 1351 1352 @Override 1353 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1354 switch (hash) { 1355 case -965394961: /*preAdmissionIdentifier*/ return new String[] {"Identifier"}; 1356 case -1008619738: /*origin*/ return new String[] {"Reference"}; 1357 case 538887120: /*admitSource*/ return new String[] {"CodeableConcept"}; 1358 case 669348630: /*reAdmission*/ return new String[] {"CodeableConcept"}; 1359 case -1429847026: /*destination*/ return new String[] {"Reference"}; 1360 case 528065941: /*dischargeDisposition*/ return new String[] {"CodeableConcept"}; 1361 default: return super.getTypesForProperty(hash, name); 1362 } 1363 1364 } 1365 1366 @Override 1367 public Base addChild(String name) throws FHIRException { 1368 if (name.equals("preAdmissionIdentifier")) { 1369 this.preAdmissionIdentifier = new Identifier(); 1370 return this.preAdmissionIdentifier; 1371 } 1372 else if (name.equals("origin")) { 1373 this.origin = new Reference(); 1374 return this.origin; 1375 } 1376 else if (name.equals("admitSource")) { 1377 this.admitSource = new CodeableConcept(); 1378 return this.admitSource; 1379 } 1380 else if (name.equals("reAdmission")) { 1381 this.reAdmission = new CodeableConcept(); 1382 return this.reAdmission; 1383 } 1384 else if (name.equals("destination")) { 1385 this.destination = new Reference(); 1386 return this.destination; 1387 } 1388 else if (name.equals("dischargeDisposition")) { 1389 this.dischargeDisposition = new CodeableConcept(); 1390 return this.dischargeDisposition; 1391 } 1392 else 1393 return super.addChild(name); 1394 } 1395 1396 public EncounterAdmissionComponent copy() { 1397 EncounterAdmissionComponent dst = new EncounterAdmissionComponent(); 1398 copyValues(dst); 1399 return dst; 1400 } 1401 1402 public void copyValues(EncounterAdmissionComponent dst) { 1403 super.copyValues(dst); 1404 dst.preAdmissionIdentifier = preAdmissionIdentifier == null ? null : preAdmissionIdentifier.copy(); 1405 dst.origin = origin == null ? null : origin.copy(); 1406 dst.admitSource = admitSource == null ? null : admitSource.copy(); 1407 dst.reAdmission = reAdmission == null ? null : reAdmission.copy(); 1408 dst.destination = destination == null ? null : destination.copy(); 1409 dst.dischargeDisposition = dischargeDisposition == null ? null : dischargeDisposition.copy(); 1410 } 1411 1412 @Override 1413 public boolean equalsDeep(Base other_) { 1414 if (!super.equalsDeep(other_)) 1415 return false; 1416 if (!(other_ instanceof EncounterAdmissionComponent)) 1417 return false; 1418 EncounterAdmissionComponent o = (EncounterAdmissionComponent) other_; 1419 return compareDeep(preAdmissionIdentifier, o.preAdmissionIdentifier, true) && compareDeep(origin, o.origin, true) 1420 && compareDeep(admitSource, o.admitSource, true) && compareDeep(reAdmission, o.reAdmission, true) 1421 && compareDeep(destination, o.destination, true) && compareDeep(dischargeDisposition, o.dischargeDisposition, true) 1422 ; 1423 } 1424 1425 @Override 1426 public boolean equalsShallow(Base other_) { 1427 if (!super.equalsShallow(other_)) 1428 return false; 1429 if (!(other_ instanceof EncounterAdmissionComponent)) 1430 return false; 1431 EncounterAdmissionComponent o = (EncounterAdmissionComponent) other_; 1432 return true; 1433 } 1434 1435 public boolean isEmpty() { 1436 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(preAdmissionIdentifier, origin 1437 , admitSource, reAdmission, destination, dischargeDisposition); 1438 } 1439 1440 public String fhirType() { 1441 return "Encounter.admission"; 1442 1443 } 1444 1445 } 1446 1447 @Block() 1448 public static class EncounterLocationComponent extends BackboneElement implements IBaseBackboneElement { 1449 /** 1450 * The location where the encounter takes place. 1451 */ 1452 @Child(name = "location", type = {Location.class}, order=1, min=1, max=1, modifier=false, summary=false) 1453 @Description(shortDefinition="Location the encounter takes place", formalDefinition="The location where the encounter takes place." ) 1454 protected Reference location; 1455 1456 /** 1457 * The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time. 1458 */ 1459 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1460 @Description(shortDefinition="planned | active | reserved | completed", formalDefinition="The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time." ) 1461 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-location-status") 1462 protected Enumeration<EncounterLocationStatus> status; 1463 1464 /** 1465 * This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query. 1466 */ 1467 @Child(name = "form", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1468 @Description(shortDefinition="The physical type of the location (usually the level in the location hierarchy - bed, room, ward, virtual etc.)", formalDefinition="This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query." ) 1469 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-form") 1470 protected CodeableConcept form; 1471 1472 /** 1473 * Time period during which the patient was present at the location. 1474 */ 1475 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1476 @Description(shortDefinition="Time period during which the patient was present at the location", formalDefinition="Time period during which the patient was present at the location." ) 1477 protected Period period; 1478 1479 private static final long serialVersionUID = -1665957440L; 1480 1481 /** 1482 * Constructor 1483 */ 1484 public EncounterLocationComponent() { 1485 super(); 1486 } 1487 1488 /** 1489 * Constructor 1490 */ 1491 public EncounterLocationComponent(Reference location) { 1492 super(); 1493 this.setLocation(location); 1494 } 1495 1496 /** 1497 * @return {@link #location} (The location where the encounter takes place.) 1498 */ 1499 public Reference getLocation() { 1500 if (this.location == null) 1501 if (Configuration.errorOnAutoCreate()) 1502 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 1503 else if (Configuration.doAutoCreate()) 1504 this.location = new Reference(); // cc 1505 return this.location; 1506 } 1507 1508 public boolean hasLocation() { 1509 return this.location != null && !this.location.isEmpty(); 1510 } 1511 1512 /** 1513 * @param value {@link #location} (The location where the encounter takes place.) 1514 */ 1515 public EncounterLocationComponent setLocation(Reference value) { 1516 this.location = value; 1517 return this; 1518 } 1519 1520 /** 1521 * @return {@link #status} (The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1522 */ 1523 public Enumeration<EncounterLocationStatus> getStatusElement() { 1524 if (this.status == null) 1525 if (Configuration.errorOnAutoCreate()) 1526 throw new Error("Attempt to auto-create EncounterLocationComponent.status"); 1527 else if (Configuration.doAutoCreate()) 1528 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); // bb 1529 return this.status; 1530 } 1531 1532 public boolean hasStatusElement() { 1533 return this.status != null && !this.status.isEmpty(); 1534 } 1535 1536 public boolean hasStatus() { 1537 return this.status != null && !this.status.isEmpty(); 1538 } 1539 1540 /** 1541 * @param value {@link #status} (The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1542 */ 1543 public EncounterLocationComponent setStatusElement(Enumeration<EncounterLocationStatus> value) { 1544 this.status = value; 1545 return this; 1546 } 1547 1548 /** 1549 * @return The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time. 1550 */ 1551 public EncounterLocationStatus getStatus() { 1552 return this.status == null ? null : this.status.getValue(); 1553 } 1554 1555 /** 1556 * @param value The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time. 1557 */ 1558 public EncounterLocationComponent setStatus(EncounterLocationStatus value) { 1559 if (value == null) 1560 this.status = null; 1561 else { 1562 if (this.status == null) 1563 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); 1564 this.status.setValue(value); 1565 } 1566 return this; 1567 } 1568 1569 /** 1570 * @return {@link #form} (This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.) 1571 */ 1572 public CodeableConcept getForm() { 1573 if (this.form == null) 1574 if (Configuration.errorOnAutoCreate()) 1575 throw new Error("Attempt to auto-create EncounterLocationComponent.form"); 1576 else if (Configuration.doAutoCreate()) 1577 this.form = new CodeableConcept(); // cc 1578 return this.form; 1579 } 1580 1581 public boolean hasForm() { 1582 return this.form != null && !this.form.isEmpty(); 1583 } 1584 1585 /** 1586 * @param value {@link #form} (This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.) 1587 */ 1588 public EncounterLocationComponent setForm(CodeableConcept value) { 1589 this.form = value; 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #period} (Time period during which the patient was present at the location.) 1595 */ 1596 public Period getPeriod() { 1597 if (this.period == null) 1598 if (Configuration.errorOnAutoCreate()) 1599 throw new Error("Attempt to auto-create EncounterLocationComponent.period"); 1600 else if (Configuration.doAutoCreate()) 1601 this.period = new Period(); // cc 1602 return this.period; 1603 } 1604 1605 public boolean hasPeriod() { 1606 return this.period != null && !this.period.isEmpty(); 1607 } 1608 1609 /** 1610 * @param value {@link #period} (Time period during which the patient was present at the location.) 1611 */ 1612 public EncounterLocationComponent setPeriod(Period value) { 1613 this.period = value; 1614 return this; 1615 } 1616 1617 protected void listChildren(List<Property> children) { 1618 super.listChildren(children); 1619 children.add(new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 1, location)); 1620 children.add(new Property("status", "code", "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.", 0, 1, status)); 1621 children.add(new Property("form", "CodeableConcept", "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 0, 1, form)); 1622 children.add(new Property("period", "Period", "Time period during which the patient was present at the location.", 0, 1, period)); 1623 } 1624 1625 @Override 1626 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1627 switch (_hash) { 1628 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 1, location); 1629 case -892481550: /*status*/ return new Property("status", "code", "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.", 0, 1, status); 1630 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 0, 1, form); 1631 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which the patient was present at the location.", 0, 1, period); 1632 default: return super.getNamedProperty(_hash, _name, _checkValid); 1633 } 1634 1635 } 1636 1637 @Override 1638 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1639 switch (hash) { 1640 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1641 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EncounterLocationStatus> 1642 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 1643 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1644 default: return super.getProperty(hash, name, checkValid); 1645 } 1646 1647 } 1648 1649 @Override 1650 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1651 switch (hash) { 1652 case 1901043637: // location 1653 this.location = TypeConvertor.castToReference(value); // Reference 1654 return value; 1655 case -892481550: // status 1656 value = new EncounterLocationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1657 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 1658 return value; 1659 case 3148996: // form 1660 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1661 return value; 1662 case -991726143: // period 1663 this.period = TypeConvertor.castToPeriod(value); // Period 1664 return value; 1665 default: return super.setProperty(hash, name, value); 1666 } 1667 1668 } 1669 1670 @Override 1671 public Base setProperty(String name, Base value) throws FHIRException { 1672 if (name.equals("location")) { 1673 this.location = TypeConvertor.castToReference(value); // Reference 1674 } else if (name.equals("status")) { 1675 value = new EncounterLocationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1676 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 1677 } else if (name.equals("form")) { 1678 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1679 } else if (name.equals("period")) { 1680 this.period = TypeConvertor.castToPeriod(value); // Period 1681 } else 1682 return super.setProperty(name, value); 1683 return value; 1684 } 1685 1686 @Override 1687 public void removeChild(String name, Base value) throws FHIRException { 1688 if (name.equals("location")) { 1689 this.location = null; 1690 } else if (name.equals("status")) { 1691 value = new EncounterLocationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1692 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 1693 } else if (name.equals("form")) { 1694 this.form = null; 1695 } else if (name.equals("period")) { 1696 this.period = null; 1697 } else 1698 super.removeChild(name, value); 1699 1700 } 1701 1702 @Override 1703 public Base makeProperty(int hash, String name) throws FHIRException { 1704 switch (hash) { 1705 case 1901043637: return getLocation(); 1706 case -892481550: return getStatusElement(); 1707 case 3148996: return getForm(); 1708 case -991726143: return getPeriod(); 1709 default: return super.makeProperty(hash, name); 1710 } 1711 1712 } 1713 1714 @Override 1715 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1716 switch (hash) { 1717 case 1901043637: /*location*/ return new String[] {"Reference"}; 1718 case -892481550: /*status*/ return new String[] {"code"}; 1719 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 1720 case -991726143: /*period*/ return new String[] {"Period"}; 1721 default: return super.getTypesForProperty(hash, name); 1722 } 1723 1724 } 1725 1726 @Override 1727 public Base addChild(String name) throws FHIRException { 1728 if (name.equals("location")) { 1729 this.location = new Reference(); 1730 return this.location; 1731 } 1732 else if (name.equals("status")) { 1733 throw new FHIRException("Cannot call addChild on a singleton property Encounter.location.status"); 1734 } 1735 else if (name.equals("form")) { 1736 this.form = new CodeableConcept(); 1737 return this.form; 1738 } 1739 else if (name.equals("period")) { 1740 this.period = new Period(); 1741 return this.period; 1742 } 1743 else 1744 return super.addChild(name); 1745 } 1746 1747 public EncounterLocationComponent copy() { 1748 EncounterLocationComponent dst = new EncounterLocationComponent(); 1749 copyValues(dst); 1750 return dst; 1751 } 1752 1753 public void copyValues(EncounterLocationComponent dst) { 1754 super.copyValues(dst); 1755 dst.location = location == null ? null : location.copy(); 1756 dst.status = status == null ? null : status.copy(); 1757 dst.form = form == null ? null : form.copy(); 1758 dst.period = period == null ? null : period.copy(); 1759 } 1760 1761 @Override 1762 public boolean equalsDeep(Base other_) { 1763 if (!super.equalsDeep(other_)) 1764 return false; 1765 if (!(other_ instanceof EncounterLocationComponent)) 1766 return false; 1767 EncounterLocationComponent o = (EncounterLocationComponent) other_; 1768 return compareDeep(location, o.location, true) && compareDeep(status, o.status, true) && compareDeep(form, o.form, true) 1769 && compareDeep(period, o.period, true); 1770 } 1771 1772 @Override 1773 public boolean equalsShallow(Base other_) { 1774 if (!super.equalsShallow(other_)) 1775 return false; 1776 if (!(other_ instanceof EncounterLocationComponent)) 1777 return false; 1778 EncounterLocationComponent o = (EncounterLocationComponent) other_; 1779 return compareValues(status, o.status, true); 1780 } 1781 1782 public boolean isEmpty() { 1783 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(location, status, form, period 1784 ); 1785 } 1786 1787 public String fhirType() { 1788 return "Encounter.location"; 1789 1790 } 1791 1792 } 1793 1794 /** 1795 * Identifier(s) by which this encounter is known. 1796 */ 1797 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1798 @Description(shortDefinition="Identifier(s) by which this encounter is known", formalDefinition="Identifier(s) by which this encounter is known." ) 1799 protected List<Identifier> identifier; 1800 1801 /** 1802 * The current state of the encounter (not the state of the patient within the encounter - that is subjectState). 1803 */ 1804 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1805 @Description(shortDefinition="planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown", formalDefinition="The current state of the encounter (not the state of the patient within the encounter - that is subjectState)." ) 1806 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-status") 1807 protected Enumeration<EncounterStatus> status; 1808 1809 /** 1810 * Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations. 1811 */ 1812 @Child(name = "class", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1813 @Description(shortDefinition="Classification of patient encounter context - e.g. Inpatient, outpatient", formalDefinition="Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations." ) 1814 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/encounter-class") 1815 protected List<CodeableConcept> class_; 1816 1817 /** 1818 * Indicates the urgency of the encounter. 1819 */ 1820 @Child(name = "priority", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1821 @Description(shortDefinition="Indicates the urgency of the encounter", formalDefinition="Indicates the urgency of the encounter." ) 1822 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActPriority") 1823 protected CodeableConcept priority; 1824 1825 /** 1826 * Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation). 1827 */ 1828 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1829 @Description(shortDefinition="Specific type of encounter (e.g. e-mail consultation, surgical day-care, ...)", formalDefinition="Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation)." ) 1830 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-type") 1831 protected List<CodeableConcept> type; 1832 1833 /** 1834 * Broad categorization of the service that is to be provided (e.g. cardiology). 1835 */ 1836 @Child(name = "serviceType", type = {CodeableReference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1837 @Description(shortDefinition="Specific type of service", formalDefinition="Broad categorization of the service that is to be provided (e.g. cardiology)." ) 1838 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 1839 protected List<CodeableReference> serviceType; 1840 1841 /** 1842 * The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam. 1843 */ 1844 @Child(name = "subject", type = {Patient.class, Group.class}, order=6, min=0, max=1, modifier=false, summary=true) 1845 @Description(shortDefinition="The patient or group related to this encounter", formalDefinition="The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam." ) 1846 protected Reference subject; 1847 1848 /** 1849 * The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status. 1850 */ 1851 @Child(name = "subjectStatus", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1852 @Description(shortDefinition="The current status of the subject in relation to the Encounter", formalDefinition="The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status." ) 1853 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-subject-status") 1854 protected CodeableConcept subjectStatus; 1855 1856 /** 1857 * Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years). 1858 */ 1859 @Child(name = "episodeOfCare", type = {EpisodeOfCare.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1860 @Description(shortDefinition="Episode(s) of care that this encounter should be recorded against", formalDefinition="Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years)." ) 1861 protected List<Reference> episodeOfCare; 1862 1863 /** 1864 * The request this encounter satisfies (e.g. incoming referral or procedure request). 1865 */ 1866 @Child(name = "basedOn", type = {CarePlan.class, DeviceRequest.class, MedicationRequest.class, ServiceRequest.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1867 @Description(shortDefinition="The request that initiated this encounter", formalDefinition="The request this encounter satisfies (e.g. incoming referral or procedure request)." ) 1868 protected List<Reference> basedOn; 1869 1870 /** 1871 * The group(s) of individuals, organizations that are allocated to participate in this encounter. The participants backbone will record the actuals of when these individuals participated during the encounter. 1872 */ 1873 @Child(name = "careTeam", type = {CareTeam.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1874 @Description(shortDefinition="The group(s) that are allocated to participate in this encounter", formalDefinition="The group(s) of individuals, organizations that are allocated to participate in this encounter. The participants backbone will record the actuals of when these individuals participated during the encounter." ) 1875 protected List<Reference> careTeam; 1876 1877 /** 1878 * Another Encounter of which this encounter is a part of (administratively or in time). 1879 */ 1880 @Child(name = "partOf", type = {Encounter.class}, order=11, min=0, max=1, modifier=false, summary=false) 1881 @Description(shortDefinition="Another Encounter this encounter is part of", formalDefinition="Another Encounter of which this encounter is a part of (administratively or in time)." ) 1882 protected Reference partOf; 1883 1884 /** 1885 * The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the colonoscopy example on the Encounter examples tab. 1886 */ 1887 @Child(name = "serviceProvider", type = {Organization.class}, order=12, min=0, max=1, modifier=false, summary=false) 1888 @Description(shortDefinition="The organization (facility) responsible for this encounter", formalDefinition="The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the colonoscopy example on the Encounter examples tab." ) 1889 protected Reference serviceProvider; 1890 1891 /** 1892 * The list of people responsible for providing the service. 1893 */ 1894 @Child(name = "participant", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1895 @Description(shortDefinition="List of participants involved in the encounter", formalDefinition="The list of people responsible for providing the service." ) 1896 protected List<EncounterParticipantComponent> participant; 1897 1898 /** 1899 * The appointment that scheduled this encounter. 1900 */ 1901 @Child(name = "appointment", type = {Appointment.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1902 @Description(shortDefinition="The appointment that scheduled this encounter", formalDefinition="The appointment that scheduled this encounter." ) 1903 protected List<Reference> appointment; 1904 1905 /** 1906 * Connection details of a virtual service (e.g. conference call). 1907 */ 1908 @Child(name = "virtualService", type = {VirtualServiceDetail.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1909 @Description(shortDefinition="Connection details of a virtual service (e.g. conference call)", formalDefinition="Connection details of a virtual service (e.g. conference call)." ) 1910 protected List<VirtualServiceDetail> virtualService; 1911 1912 /** 1913 * The actual start and end time of the encounter. 1914 */ 1915 @Child(name = "actualPeriod", type = {Period.class}, order=16, min=0, max=1, modifier=false, summary=false) 1916 @Description(shortDefinition="The actual start and end time of the encounter", formalDefinition="The actual start and end time of the encounter." ) 1917 protected Period actualPeriod; 1918 1919 /** 1920 * The planned start date/time (or admission date) of the encounter. 1921 */ 1922 @Child(name = "plannedStartDate", type = {DateTimeType.class}, order=17, min=0, max=1, modifier=false, summary=false) 1923 @Description(shortDefinition="The planned start date/time (or admission date) of the encounter", formalDefinition="The planned start date/time (or admission date) of the encounter." ) 1924 protected DateTimeType plannedStartDate; 1925 1926 /** 1927 * The planned end date/time (or discharge date) of the encounter. 1928 */ 1929 @Child(name = "plannedEndDate", type = {DateTimeType.class}, order=18, min=0, max=1, modifier=false, summary=false) 1930 @Description(shortDefinition="The planned end date/time (or discharge date) of the encounter", formalDefinition="The planned end date/time (or discharge date) of the encounter." ) 1931 protected DateTimeType plannedEndDate; 1932 1933 /** 1934 * Actual quantity of time the encounter lasted. This excludes the time during leaves of absence. 1935 1936When missing it is the time in between the start and end values. 1937 */ 1938 @Child(name = "length", type = {Duration.class}, order=19, min=0, max=1, modifier=false, summary=false) 1939 @Description(shortDefinition="Actual quantity of time the encounter lasted (less time absent)", formalDefinition="Actual quantity of time the encounter lasted. This excludes the time during leaves of absence.\r\rWhen missing it is the time in between the start and end values." ) 1940 protected Duration length; 1941 1942 /** 1943 * The list of medical reasons that are expected to be addressed during the episode of care. 1944 */ 1945 @Child(name = "reason", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1946 @Description(shortDefinition="The list of medical reasons that are expected to be addressed during the episode of care", formalDefinition="The list of medical reasons that are expected to be addressed during the episode of care." ) 1947 protected List<ReasonComponent> reason; 1948 1949 /** 1950 * The list of diagnosis relevant to this encounter. 1951 */ 1952 @Child(name = "diagnosis", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1953 @Description(shortDefinition="The list of diagnosis relevant to this encounter", formalDefinition="The list of diagnosis relevant to this encounter." ) 1954 protected List<DiagnosisComponent> diagnosis; 1955 1956 /** 1957 * The set of accounts that may be used for billing for this Encounter. 1958 */ 1959 @Child(name = "account", type = {Account.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1960 @Description(shortDefinition="The set of accounts that may be used for billing for this Encounter", formalDefinition="The set of accounts that may be used for billing for this Encounter." ) 1961 protected List<Reference> account; 1962 1963 /** 1964 * Diet preferences reported by the patient. 1965 */ 1966 @Child(name = "dietPreference", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1967 @Description(shortDefinition="Diet preferences reported by the patient", formalDefinition="Diet preferences reported by the patient." ) 1968 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-diet") 1969 protected List<CodeableConcept> dietPreference; 1970 1971 /** 1972 * Any special requests that have been made for this encounter, such as the provision of specific equipment or other things. 1973 */ 1974 @Child(name = "specialArrangement", type = {CodeableConcept.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1975 @Description(shortDefinition="Wheelchair, translator, stretcher, etc", formalDefinition="Any special requests that have been made for this encounter, such as the provision of specific equipment or other things." ) 1976 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-special-arrangements") 1977 protected List<CodeableConcept> specialArrangement; 1978 1979 /** 1980 * Special courtesies that may be provided to the patient during the encounter (VIP, board member, professional courtesy). 1981 */ 1982 @Child(name = "specialCourtesy", type = {CodeableConcept.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1983 @Description(shortDefinition="Special courtesies (VIP, board member)", formalDefinition="Special courtesies that may be provided to the patient during the encounter (VIP, board member, professional courtesy)." ) 1984 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-special-courtesy") 1985 protected List<CodeableConcept> specialCourtesy; 1986 1987 /** 1988 * Details about the stay during which a healthcare service is provided. 1989 1990This does not describe the event of admitting the patient, but rather any information that is relevant from the time of admittance until the time of discharge. 1991 */ 1992 @Child(name = "admission", type = {}, order=26, min=0, max=1, modifier=false, summary=false) 1993 @Description(shortDefinition="Details about the admission to a healthcare service", formalDefinition="Details about the stay during which a healthcare service is provided.\r\rThis does not describe the event of admitting the patient, but rather any information that is relevant from the time of admittance until the time of discharge." ) 1994 protected EncounterAdmissionComponent admission; 1995 1996 /** 1997 * List of locations where the patient has been during this encounter. 1998 */ 1999 @Child(name = "location", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2000 @Description(shortDefinition="List of locations where the patient has been", formalDefinition="List of locations where the patient has been during this encounter." ) 2001 protected List<EncounterLocationComponent> location; 2002 2003 private static final long serialVersionUID = -1336316477L; 2004 2005 /** 2006 * Constructor 2007 */ 2008 public Encounter() { 2009 super(); 2010 } 2011 2012 /** 2013 * Constructor 2014 */ 2015 public Encounter(EncounterStatus status) { 2016 super(); 2017 this.setStatus(status); 2018 } 2019 2020 /** 2021 * @return {@link #identifier} (Identifier(s) by which this encounter is known.) 2022 */ 2023 public List<Identifier> getIdentifier() { 2024 if (this.identifier == null) 2025 this.identifier = new ArrayList<Identifier>(); 2026 return this.identifier; 2027 } 2028 2029 /** 2030 * @return Returns a reference to <code>this</code> for easy method chaining 2031 */ 2032 public Encounter setIdentifier(List<Identifier> theIdentifier) { 2033 this.identifier = theIdentifier; 2034 return this; 2035 } 2036 2037 public boolean hasIdentifier() { 2038 if (this.identifier == null) 2039 return false; 2040 for (Identifier item : this.identifier) 2041 if (!item.isEmpty()) 2042 return true; 2043 return false; 2044 } 2045 2046 public Identifier addIdentifier() { //3 2047 Identifier t = new Identifier(); 2048 if (this.identifier == null) 2049 this.identifier = new ArrayList<Identifier>(); 2050 this.identifier.add(t); 2051 return t; 2052 } 2053 2054 public Encounter addIdentifier(Identifier t) { //3 2055 if (t == null) 2056 return this; 2057 if (this.identifier == null) 2058 this.identifier = new ArrayList<Identifier>(); 2059 this.identifier.add(t); 2060 return this; 2061 } 2062 2063 /** 2064 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2065 */ 2066 public Identifier getIdentifierFirstRep() { 2067 if (getIdentifier().isEmpty()) { 2068 addIdentifier(); 2069 } 2070 return getIdentifier().get(0); 2071 } 2072 2073 /** 2074 * @return {@link #status} (The current state of the encounter (not the state of the patient within the encounter - that is subjectState).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2075 */ 2076 public Enumeration<EncounterStatus> getStatusElement() { 2077 if (this.status == null) 2078 if (Configuration.errorOnAutoCreate()) 2079 throw new Error("Attempt to auto-create Encounter.status"); 2080 else if (Configuration.doAutoCreate()) 2081 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 2082 return this.status; 2083 } 2084 2085 public boolean hasStatusElement() { 2086 return this.status != null && !this.status.isEmpty(); 2087 } 2088 2089 public boolean hasStatus() { 2090 return this.status != null && !this.status.isEmpty(); 2091 } 2092 2093 /** 2094 * @param value {@link #status} (The current state of the encounter (not the state of the patient within the encounter - that is subjectState).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2095 */ 2096 public Encounter setStatusElement(Enumeration<EncounterStatus> value) { 2097 this.status = value; 2098 return this; 2099 } 2100 2101 /** 2102 * @return The current state of the encounter (not the state of the patient within the encounter - that is subjectState). 2103 */ 2104 public EncounterStatus getStatus() { 2105 return this.status == null ? null : this.status.getValue(); 2106 } 2107 2108 /** 2109 * @param value The current state of the encounter (not the state of the patient within the encounter - that is subjectState). 2110 */ 2111 public Encounter setStatus(EncounterStatus value) { 2112 if (this.status == null) 2113 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 2114 this.status.setValue(value); 2115 return this; 2116 } 2117 2118 /** 2119 * @return {@link #class_} (Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.) 2120 */ 2121 public List<CodeableConcept> getClass_() { 2122 if (this.class_ == null) 2123 this.class_ = new ArrayList<CodeableConcept>(); 2124 return this.class_; 2125 } 2126 2127 /** 2128 * @return Returns a reference to <code>this</code> for easy method chaining 2129 */ 2130 public Encounter setClass_(List<CodeableConcept> theClass_) { 2131 this.class_ = theClass_; 2132 return this; 2133 } 2134 2135 public boolean hasClass_() { 2136 if (this.class_ == null) 2137 return false; 2138 for (CodeableConcept item : this.class_) 2139 if (!item.isEmpty()) 2140 return true; 2141 return false; 2142 } 2143 2144 public CodeableConcept addClass_() { //3 2145 CodeableConcept t = new CodeableConcept(); 2146 if (this.class_ == null) 2147 this.class_ = new ArrayList<CodeableConcept>(); 2148 this.class_.add(t); 2149 return t; 2150 } 2151 2152 public Encounter addClass_(CodeableConcept t) { //3 2153 if (t == null) 2154 return this; 2155 if (this.class_ == null) 2156 this.class_ = new ArrayList<CodeableConcept>(); 2157 this.class_.add(t); 2158 return this; 2159 } 2160 2161 /** 2162 * @return The first repetition of repeating field {@link #class_}, creating it if it does not already exist {3} 2163 */ 2164 public CodeableConcept getClass_FirstRep() { 2165 if (getClass_().isEmpty()) { 2166 addClass_(); 2167 } 2168 return getClass_().get(0); 2169 } 2170 2171 /** 2172 * @return {@link #priority} (Indicates the urgency of the encounter.) 2173 */ 2174 public CodeableConcept getPriority() { 2175 if (this.priority == null) 2176 if (Configuration.errorOnAutoCreate()) 2177 throw new Error("Attempt to auto-create Encounter.priority"); 2178 else if (Configuration.doAutoCreate()) 2179 this.priority = new CodeableConcept(); // cc 2180 return this.priority; 2181 } 2182 2183 public boolean hasPriority() { 2184 return this.priority != null && !this.priority.isEmpty(); 2185 } 2186 2187 /** 2188 * @param value {@link #priority} (Indicates the urgency of the encounter.) 2189 */ 2190 public Encounter setPriority(CodeableConcept value) { 2191 this.priority = value; 2192 return this; 2193 } 2194 2195 /** 2196 * @return {@link #type} (Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).) 2197 */ 2198 public List<CodeableConcept> getType() { 2199 if (this.type == null) 2200 this.type = new ArrayList<CodeableConcept>(); 2201 return this.type; 2202 } 2203 2204 /** 2205 * @return Returns a reference to <code>this</code> for easy method chaining 2206 */ 2207 public Encounter setType(List<CodeableConcept> theType) { 2208 this.type = theType; 2209 return this; 2210 } 2211 2212 public boolean hasType() { 2213 if (this.type == null) 2214 return false; 2215 for (CodeableConcept item : this.type) 2216 if (!item.isEmpty()) 2217 return true; 2218 return false; 2219 } 2220 2221 public CodeableConcept addType() { //3 2222 CodeableConcept t = new CodeableConcept(); 2223 if (this.type == null) 2224 this.type = new ArrayList<CodeableConcept>(); 2225 this.type.add(t); 2226 return t; 2227 } 2228 2229 public Encounter addType(CodeableConcept t) { //3 2230 if (t == null) 2231 return this; 2232 if (this.type == null) 2233 this.type = new ArrayList<CodeableConcept>(); 2234 this.type.add(t); 2235 return this; 2236 } 2237 2238 /** 2239 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2240 */ 2241 public CodeableConcept getTypeFirstRep() { 2242 if (getType().isEmpty()) { 2243 addType(); 2244 } 2245 return getType().get(0); 2246 } 2247 2248 /** 2249 * @return {@link #serviceType} (Broad categorization of the service that is to be provided (e.g. cardiology).) 2250 */ 2251 public List<CodeableReference> getServiceType() { 2252 if (this.serviceType == null) 2253 this.serviceType = new ArrayList<CodeableReference>(); 2254 return this.serviceType; 2255 } 2256 2257 /** 2258 * @return Returns a reference to <code>this</code> for easy method chaining 2259 */ 2260 public Encounter setServiceType(List<CodeableReference> theServiceType) { 2261 this.serviceType = theServiceType; 2262 return this; 2263 } 2264 2265 public boolean hasServiceType() { 2266 if (this.serviceType == null) 2267 return false; 2268 for (CodeableReference item : this.serviceType) 2269 if (!item.isEmpty()) 2270 return true; 2271 return false; 2272 } 2273 2274 public CodeableReference addServiceType() { //3 2275 CodeableReference t = new CodeableReference(); 2276 if (this.serviceType == null) 2277 this.serviceType = new ArrayList<CodeableReference>(); 2278 this.serviceType.add(t); 2279 return t; 2280 } 2281 2282 public Encounter addServiceType(CodeableReference t) { //3 2283 if (t == null) 2284 return this; 2285 if (this.serviceType == null) 2286 this.serviceType = new ArrayList<CodeableReference>(); 2287 this.serviceType.add(t); 2288 return this; 2289 } 2290 2291 /** 2292 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist {3} 2293 */ 2294 public CodeableReference getServiceTypeFirstRep() { 2295 if (getServiceType().isEmpty()) { 2296 addServiceType(); 2297 } 2298 return getServiceType().get(0); 2299 } 2300 2301 /** 2302 * @return {@link #subject} (The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.) 2303 */ 2304 public Reference getSubject() { 2305 if (this.subject == null) 2306 if (Configuration.errorOnAutoCreate()) 2307 throw new Error("Attempt to auto-create Encounter.subject"); 2308 else if (Configuration.doAutoCreate()) 2309 this.subject = new Reference(); // cc 2310 return this.subject; 2311 } 2312 2313 public boolean hasSubject() { 2314 return this.subject != null && !this.subject.isEmpty(); 2315 } 2316 2317 /** 2318 * @param value {@link #subject} (The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.) 2319 */ 2320 public Encounter setSubject(Reference value) { 2321 this.subject = value; 2322 return this; 2323 } 2324 2325 /** 2326 * @return {@link #subjectStatus} (The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.) 2327 */ 2328 public CodeableConcept getSubjectStatus() { 2329 if (this.subjectStatus == null) 2330 if (Configuration.errorOnAutoCreate()) 2331 throw new Error("Attempt to auto-create Encounter.subjectStatus"); 2332 else if (Configuration.doAutoCreate()) 2333 this.subjectStatus = new CodeableConcept(); // cc 2334 return this.subjectStatus; 2335 } 2336 2337 public boolean hasSubjectStatus() { 2338 return this.subjectStatus != null && !this.subjectStatus.isEmpty(); 2339 } 2340 2341 /** 2342 * @param value {@link #subjectStatus} (The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.) 2343 */ 2344 public Encounter setSubjectStatus(CodeableConcept value) { 2345 this.subjectStatus = value; 2346 return this; 2347 } 2348 2349 /** 2350 * @return {@link #episodeOfCare} (Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).) 2351 */ 2352 public List<Reference> getEpisodeOfCare() { 2353 if (this.episodeOfCare == null) 2354 this.episodeOfCare = new ArrayList<Reference>(); 2355 return this.episodeOfCare; 2356 } 2357 2358 /** 2359 * @return Returns a reference to <code>this</code> for easy method chaining 2360 */ 2361 public Encounter setEpisodeOfCare(List<Reference> theEpisodeOfCare) { 2362 this.episodeOfCare = theEpisodeOfCare; 2363 return this; 2364 } 2365 2366 public boolean hasEpisodeOfCare() { 2367 if (this.episodeOfCare == null) 2368 return false; 2369 for (Reference item : this.episodeOfCare) 2370 if (!item.isEmpty()) 2371 return true; 2372 return false; 2373 } 2374 2375 public Reference addEpisodeOfCare() { //3 2376 Reference t = new Reference(); 2377 if (this.episodeOfCare == null) 2378 this.episodeOfCare = new ArrayList<Reference>(); 2379 this.episodeOfCare.add(t); 2380 return t; 2381 } 2382 2383 public Encounter addEpisodeOfCare(Reference t) { //3 2384 if (t == null) 2385 return this; 2386 if (this.episodeOfCare == null) 2387 this.episodeOfCare = new ArrayList<Reference>(); 2388 this.episodeOfCare.add(t); 2389 return this; 2390 } 2391 2392 /** 2393 * @return The first repetition of repeating field {@link #episodeOfCare}, creating it if it does not already exist {3} 2394 */ 2395 public Reference getEpisodeOfCareFirstRep() { 2396 if (getEpisodeOfCare().isEmpty()) { 2397 addEpisodeOfCare(); 2398 } 2399 return getEpisodeOfCare().get(0); 2400 } 2401 2402 /** 2403 * @return {@link #basedOn} (The request this encounter satisfies (e.g. incoming referral or procedure request).) 2404 */ 2405 public List<Reference> getBasedOn() { 2406 if (this.basedOn == null) 2407 this.basedOn = new ArrayList<Reference>(); 2408 return this.basedOn; 2409 } 2410 2411 /** 2412 * @return Returns a reference to <code>this</code> for easy method chaining 2413 */ 2414 public Encounter setBasedOn(List<Reference> theBasedOn) { 2415 this.basedOn = theBasedOn; 2416 return this; 2417 } 2418 2419 public boolean hasBasedOn() { 2420 if (this.basedOn == null) 2421 return false; 2422 for (Reference item : this.basedOn) 2423 if (!item.isEmpty()) 2424 return true; 2425 return false; 2426 } 2427 2428 public Reference addBasedOn() { //3 2429 Reference t = new Reference(); 2430 if (this.basedOn == null) 2431 this.basedOn = new ArrayList<Reference>(); 2432 this.basedOn.add(t); 2433 return t; 2434 } 2435 2436 public Encounter addBasedOn(Reference t) { //3 2437 if (t == null) 2438 return this; 2439 if (this.basedOn == null) 2440 this.basedOn = new ArrayList<Reference>(); 2441 this.basedOn.add(t); 2442 return this; 2443 } 2444 2445 /** 2446 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 2447 */ 2448 public Reference getBasedOnFirstRep() { 2449 if (getBasedOn().isEmpty()) { 2450 addBasedOn(); 2451 } 2452 return getBasedOn().get(0); 2453 } 2454 2455 /** 2456 * @return {@link #careTeam} (The group(s) of individuals, organizations that are allocated to participate in this encounter. The participants backbone will record the actuals of when these individuals participated during the encounter.) 2457 */ 2458 public List<Reference> getCareTeam() { 2459 if (this.careTeam == null) 2460 this.careTeam = new ArrayList<Reference>(); 2461 return this.careTeam; 2462 } 2463 2464 /** 2465 * @return Returns a reference to <code>this</code> for easy method chaining 2466 */ 2467 public Encounter setCareTeam(List<Reference> theCareTeam) { 2468 this.careTeam = theCareTeam; 2469 return this; 2470 } 2471 2472 public boolean hasCareTeam() { 2473 if (this.careTeam == null) 2474 return false; 2475 for (Reference item : this.careTeam) 2476 if (!item.isEmpty()) 2477 return true; 2478 return false; 2479 } 2480 2481 public Reference addCareTeam() { //3 2482 Reference t = new Reference(); 2483 if (this.careTeam == null) 2484 this.careTeam = new ArrayList<Reference>(); 2485 this.careTeam.add(t); 2486 return t; 2487 } 2488 2489 public Encounter addCareTeam(Reference t) { //3 2490 if (t == null) 2491 return this; 2492 if (this.careTeam == null) 2493 this.careTeam = new ArrayList<Reference>(); 2494 this.careTeam.add(t); 2495 return this; 2496 } 2497 2498 /** 2499 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist {3} 2500 */ 2501 public Reference getCareTeamFirstRep() { 2502 if (getCareTeam().isEmpty()) { 2503 addCareTeam(); 2504 } 2505 return getCareTeam().get(0); 2506 } 2507 2508 /** 2509 * @return {@link #partOf} (Another Encounter of which this encounter is a part of (administratively or in time).) 2510 */ 2511 public Reference getPartOf() { 2512 if (this.partOf == null) 2513 if (Configuration.errorOnAutoCreate()) 2514 throw new Error("Attempt to auto-create Encounter.partOf"); 2515 else if (Configuration.doAutoCreate()) 2516 this.partOf = new Reference(); // cc 2517 return this.partOf; 2518 } 2519 2520 public boolean hasPartOf() { 2521 return this.partOf != null && !this.partOf.isEmpty(); 2522 } 2523 2524 /** 2525 * @param value {@link #partOf} (Another Encounter of which this encounter is a part of (administratively or in time).) 2526 */ 2527 public Encounter setPartOf(Reference value) { 2528 this.partOf = value; 2529 return this; 2530 } 2531 2532 /** 2533 * @return {@link #serviceProvider} (The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the colonoscopy example on the Encounter examples tab.) 2534 */ 2535 public Reference getServiceProvider() { 2536 if (this.serviceProvider == null) 2537 if (Configuration.errorOnAutoCreate()) 2538 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 2539 else if (Configuration.doAutoCreate()) 2540 this.serviceProvider = new Reference(); // cc 2541 return this.serviceProvider; 2542 } 2543 2544 public boolean hasServiceProvider() { 2545 return this.serviceProvider != null && !this.serviceProvider.isEmpty(); 2546 } 2547 2548 /** 2549 * @param value {@link #serviceProvider} (The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the colonoscopy example on the Encounter examples tab.) 2550 */ 2551 public Encounter setServiceProvider(Reference value) { 2552 this.serviceProvider = value; 2553 return this; 2554 } 2555 2556 /** 2557 * @return {@link #participant} (The list of people responsible for providing the service.) 2558 */ 2559 public List<EncounterParticipantComponent> getParticipant() { 2560 if (this.participant == null) 2561 this.participant = new ArrayList<EncounterParticipantComponent>(); 2562 return this.participant; 2563 } 2564 2565 /** 2566 * @return Returns a reference to <code>this</code> for easy method chaining 2567 */ 2568 public Encounter setParticipant(List<EncounterParticipantComponent> theParticipant) { 2569 this.participant = theParticipant; 2570 return this; 2571 } 2572 2573 public boolean hasParticipant() { 2574 if (this.participant == null) 2575 return false; 2576 for (EncounterParticipantComponent item : this.participant) 2577 if (!item.isEmpty()) 2578 return true; 2579 return false; 2580 } 2581 2582 public EncounterParticipantComponent addParticipant() { //3 2583 EncounterParticipantComponent t = new EncounterParticipantComponent(); 2584 if (this.participant == null) 2585 this.participant = new ArrayList<EncounterParticipantComponent>(); 2586 this.participant.add(t); 2587 return t; 2588 } 2589 2590 public Encounter addParticipant(EncounterParticipantComponent t) { //3 2591 if (t == null) 2592 return this; 2593 if (this.participant == null) 2594 this.participant = new ArrayList<EncounterParticipantComponent>(); 2595 this.participant.add(t); 2596 return this; 2597 } 2598 2599 /** 2600 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 2601 */ 2602 public EncounterParticipantComponent getParticipantFirstRep() { 2603 if (getParticipant().isEmpty()) { 2604 addParticipant(); 2605 } 2606 return getParticipant().get(0); 2607 } 2608 2609 /** 2610 * @return {@link #appointment} (The appointment that scheduled this encounter.) 2611 */ 2612 public List<Reference> getAppointment() { 2613 if (this.appointment == null) 2614 this.appointment = new ArrayList<Reference>(); 2615 return this.appointment; 2616 } 2617 2618 /** 2619 * @return Returns a reference to <code>this</code> for easy method chaining 2620 */ 2621 public Encounter setAppointment(List<Reference> theAppointment) { 2622 this.appointment = theAppointment; 2623 return this; 2624 } 2625 2626 public boolean hasAppointment() { 2627 if (this.appointment == null) 2628 return false; 2629 for (Reference item : this.appointment) 2630 if (!item.isEmpty()) 2631 return true; 2632 return false; 2633 } 2634 2635 public Reference addAppointment() { //3 2636 Reference t = new Reference(); 2637 if (this.appointment == null) 2638 this.appointment = new ArrayList<Reference>(); 2639 this.appointment.add(t); 2640 return t; 2641 } 2642 2643 public Encounter addAppointment(Reference t) { //3 2644 if (t == null) 2645 return this; 2646 if (this.appointment == null) 2647 this.appointment = new ArrayList<Reference>(); 2648 this.appointment.add(t); 2649 return this; 2650 } 2651 2652 /** 2653 * @return The first repetition of repeating field {@link #appointment}, creating it if it does not already exist {3} 2654 */ 2655 public Reference getAppointmentFirstRep() { 2656 if (getAppointment().isEmpty()) { 2657 addAppointment(); 2658 } 2659 return getAppointment().get(0); 2660 } 2661 2662 /** 2663 * @return {@link #virtualService} (Connection details of a virtual service (e.g. conference call).) 2664 */ 2665 public List<VirtualServiceDetail> getVirtualService() { 2666 if (this.virtualService == null) 2667 this.virtualService = new ArrayList<VirtualServiceDetail>(); 2668 return this.virtualService; 2669 } 2670 2671 /** 2672 * @return Returns a reference to <code>this</code> for easy method chaining 2673 */ 2674 public Encounter setVirtualService(List<VirtualServiceDetail> theVirtualService) { 2675 this.virtualService = theVirtualService; 2676 return this; 2677 } 2678 2679 public boolean hasVirtualService() { 2680 if (this.virtualService == null) 2681 return false; 2682 for (VirtualServiceDetail item : this.virtualService) 2683 if (!item.isEmpty()) 2684 return true; 2685 return false; 2686 } 2687 2688 public VirtualServiceDetail addVirtualService() { //3 2689 VirtualServiceDetail t = new VirtualServiceDetail(); 2690 if (this.virtualService == null) 2691 this.virtualService = new ArrayList<VirtualServiceDetail>(); 2692 this.virtualService.add(t); 2693 return t; 2694 } 2695 2696 public Encounter addVirtualService(VirtualServiceDetail t) { //3 2697 if (t == null) 2698 return this; 2699 if (this.virtualService == null) 2700 this.virtualService = new ArrayList<VirtualServiceDetail>(); 2701 this.virtualService.add(t); 2702 return this; 2703 } 2704 2705 /** 2706 * @return The first repetition of repeating field {@link #virtualService}, creating it if it does not already exist {3} 2707 */ 2708 public VirtualServiceDetail getVirtualServiceFirstRep() { 2709 if (getVirtualService().isEmpty()) { 2710 addVirtualService(); 2711 } 2712 return getVirtualService().get(0); 2713 } 2714 2715 /** 2716 * @return {@link #actualPeriod} (The actual start and end time of the encounter.) 2717 */ 2718 public Period getActualPeriod() { 2719 if (this.actualPeriod == null) 2720 if (Configuration.errorOnAutoCreate()) 2721 throw new Error("Attempt to auto-create Encounter.actualPeriod"); 2722 else if (Configuration.doAutoCreate()) 2723 this.actualPeriod = new Period(); // cc 2724 return this.actualPeriod; 2725 } 2726 2727 public boolean hasActualPeriod() { 2728 return this.actualPeriod != null && !this.actualPeriod.isEmpty(); 2729 } 2730 2731 /** 2732 * @param value {@link #actualPeriod} (The actual start and end time of the encounter.) 2733 */ 2734 public Encounter setActualPeriod(Period value) { 2735 this.actualPeriod = value; 2736 return this; 2737 } 2738 2739 /** 2740 * @return {@link #plannedStartDate} (The planned start date/time (or admission date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedStartDate" gives direct access to the value 2741 */ 2742 public DateTimeType getPlannedStartDateElement() { 2743 if (this.plannedStartDate == null) 2744 if (Configuration.errorOnAutoCreate()) 2745 throw new Error("Attempt to auto-create Encounter.plannedStartDate"); 2746 else if (Configuration.doAutoCreate()) 2747 this.plannedStartDate = new DateTimeType(); // bb 2748 return this.plannedStartDate; 2749 } 2750 2751 public boolean hasPlannedStartDateElement() { 2752 return this.plannedStartDate != null && !this.plannedStartDate.isEmpty(); 2753 } 2754 2755 public boolean hasPlannedStartDate() { 2756 return this.plannedStartDate != null && !this.plannedStartDate.isEmpty(); 2757 } 2758 2759 /** 2760 * @param value {@link #plannedStartDate} (The planned start date/time (or admission date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedStartDate" gives direct access to the value 2761 */ 2762 public Encounter setPlannedStartDateElement(DateTimeType value) { 2763 this.plannedStartDate = value; 2764 return this; 2765 } 2766 2767 /** 2768 * @return The planned start date/time (or admission date) of the encounter. 2769 */ 2770 public Date getPlannedStartDate() { 2771 return this.plannedStartDate == null ? null : this.plannedStartDate.getValue(); 2772 } 2773 2774 /** 2775 * @param value The planned start date/time (or admission date) of the encounter. 2776 */ 2777 public Encounter setPlannedStartDate(Date value) { 2778 if (value == null) 2779 this.plannedStartDate = null; 2780 else { 2781 if (this.plannedStartDate == null) 2782 this.plannedStartDate = new DateTimeType(); 2783 this.plannedStartDate.setValue(value); 2784 } 2785 return this; 2786 } 2787 2788 /** 2789 * @return {@link #plannedEndDate} (The planned end date/time (or discharge date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedEndDate" gives direct access to the value 2790 */ 2791 public DateTimeType getPlannedEndDateElement() { 2792 if (this.plannedEndDate == null) 2793 if (Configuration.errorOnAutoCreate()) 2794 throw new Error("Attempt to auto-create Encounter.plannedEndDate"); 2795 else if (Configuration.doAutoCreate()) 2796 this.plannedEndDate = new DateTimeType(); // bb 2797 return this.plannedEndDate; 2798 } 2799 2800 public boolean hasPlannedEndDateElement() { 2801 return this.plannedEndDate != null && !this.plannedEndDate.isEmpty(); 2802 } 2803 2804 public boolean hasPlannedEndDate() { 2805 return this.plannedEndDate != null && !this.plannedEndDate.isEmpty(); 2806 } 2807 2808 /** 2809 * @param value {@link #plannedEndDate} (The planned end date/time (or discharge date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedEndDate" gives direct access to the value 2810 */ 2811 public Encounter setPlannedEndDateElement(DateTimeType value) { 2812 this.plannedEndDate = value; 2813 return this; 2814 } 2815 2816 /** 2817 * @return The planned end date/time (or discharge date) of the encounter. 2818 */ 2819 public Date getPlannedEndDate() { 2820 return this.plannedEndDate == null ? null : this.plannedEndDate.getValue(); 2821 } 2822 2823 /** 2824 * @param value The planned end date/time (or discharge date) of the encounter. 2825 */ 2826 public Encounter setPlannedEndDate(Date value) { 2827 if (value == null) 2828 this.plannedEndDate = null; 2829 else { 2830 if (this.plannedEndDate == null) 2831 this.plannedEndDate = new DateTimeType(); 2832 this.plannedEndDate.setValue(value); 2833 } 2834 return this; 2835 } 2836 2837 /** 2838 * @return {@link #length} (Actual quantity of time the encounter lasted. This excludes the time during leaves of absence. 2839 2840When missing it is the time in between the start and end values.) 2841 */ 2842 public Duration getLength() { 2843 if (this.length == null) 2844 if (Configuration.errorOnAutoCreate()) 2845 throw new Error("Attempt to auto-create Encounter.length"); 2846 else if (Configuration.doAutoCreate()) 2847 this.length = new Duration(); // cc 2848 return this.length; 2849 } 2850 2851 public boolean hasLength() { 2852 return this.length != null && !this.length.isEmpty(); 2853 } 2854 2855 /** 2856 * @param value {@link #length} (Actual quantity of time the encounter lasted. This excludes the time during leaves of absence. 2857 2858When missing it is the time in between the start and end values.) 2859 */ 2860 public Encounter setLength(Duration value) { 2861 this.length = value; 2862 return this; 2863 } 2864 2865 /** 2866 * @return {@link #reason} (The list of medical reasons that are expected to be addressed during the episode of care.) 2867 */ 2868 public List<ReasonComponent> getReason() { 2869 if (this.reason == null) 2870 this.reason = new ArrayList<ReasonComponent>(); 2871 return this.reason; 2872 } 2873 2874 /** 2875 * @return Returns a reference to <code>this</code> for easy method chaining 2876 */ 2877 public Encounter setReason(List<ReasonComponent> theReason) { 2878 this.reason = theReason; 2879 return this; 2880 } 2881 2882 public boolean hasReason() { 2883 if (this.reason == null) 2884 return false; 2885 for (ReasonComponent item : this.reason) 2886 if (!item.isEmpty()) 2887 return true; 2888 return false; 2889 } 2890 2891 public ReasonComponent addReason() { //3 2892 ReasonComponent t = new ReasonComponent(); 2893 if (this.reason == null) 2894 this.reason = new ArrayList<ReasonComponent>(); 2895 this.reason.add(t); 2896 return t; 2897 } 2898 2899 public Encounter addReason(ReasonComponent t) { //3 2900 if (t == null) 2901 return this; 2902 if (this.reason == null) 2903 this.reason = new ArrayList<ReasonComponent>(); 2904 this.reason.add(t); 2905 return this; 2906 } 2907 2908 /** 2909 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2910 */ 2911 public ReasonComponent getReasonFirstRep() { 2912 if (getReason().isEmpty()) { 2913 addReason(); 2914 } 2915 return getReason().get(0); 2916 } 2917 2918 /** 2919 * @return {@link #diagnosis} (The list of diagnosis relevant to this encounter.) 2920 */ 2921 public List<DiagnosisComponent> getDiagnosis() { 2922 if (this.diagnosis == null) 2923 this.diagnosis = new ArrayList<DiagnosisComponent>(); 2924 return this.diagnosis; 2925 } 2926 2927 /** 2928 * @return Returns a reference to <code>this</code> for easy method chaining 2929 */ 2930 public Encounter setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 2931 this.diagnosis = theDiagnosis; 2932 return this; 2933 } 2934 2935 public boolean hasDiagnosis() { 2936 if (this.diagnosis == null) 2937 return false; 2938 for (DiagnosisComponent item : this.diagnosis) 2939 if (!item.isEmpty()) 2940 return true; 2941 return false; 2942 } 2943 2944 public DiagnosisComponent addDiagnosis() { //3 2945 DiagnosisComponent t = new DiagnosisComponent(); 2946 if (this.diagnosis == null) 2947 this.diagnosis = new ArrayList<DiagnosisComponent>(); 2948 this.diagnosis.add(t); 2949 return t; 2950 } 2951 2952 public Encounter addDiagnosis(DiagnosisComponent t) { //3 2953 if (t == null) 2954 return this; 2955 if (this.diagnosis == null) 2956 this.diagnosis = new ArrayList<DiagnosisComponent>(); 2957 this.diagnosis.add(t); 2958 return this; 2959 } 2960 2961 /** 2962 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 2963 */ 2964 public DiagnosisComponent getDiagnosisFirstRep() { 2965 if (getDiagnosis().isEmpty()) { 2966 addDiagnosis(); 2967 } 2968 return getDiagnosis().get(0); 2969 } 2970 2971 /** 2972 * @return {@link #account} (The set of accounts that may be used for billing for this Encounter.) 2973 */ 2974 public List<Reference> getAccount() { 2975 if (this.account == null) 2976 this.account = new ArrayList<Reference>(); 2977 return this.account; 2978 } 2979 2980 /** 2981 * @return Returns a reference to <code>this</code> for easy method chaining 2982 */ 2983 public Encounter setAccount(List<Reference> theAccount) { 2984 this.account = theAccount; 2985 return this; 2986 } 2987 2988 public boolean hasAccount() { 2989 if (this.account == null) 2990 return false; 2991 for (Reference item : this.account) 2992 if (!item.isEmpty()) 2993 return true; 2994 return false; 2995 } 2996 2997 public Reference addAccount() { //3 2998 Reference t = new Reference(); 2999 if (this.account == null) 3000 this.account = new ArrayList<Reference>(); 3001 this.account.add(t); 3002 return t; 3003 } 3004 3005 public Encounter addAccount(Reference t) { //3 3006 if (t == null) 3007 return this; 3008 if (this.account == null) 3009 this.account = new ArrayList<Reference>(); 3010 this.account.add(t); 3011 return this; 3012 } 3013 3014 /** 3015 * @return The first repetition of repeating field {@link #account}, creating it if it does not already exist {3} 3016 */ 3017 public Reference getAccountFirstRep() { 3018 if (getAccount().isEmpty()) { 3019 addAccount(); 3020 } 3021 return getAccount().get(0); 3022 } 3023 3024 /** 3025 * @return {@link #dietPreference} (Diet preferences reported by the patient.) 3026 */ 3027 public List<CodeableConcept> getDietPreference() { 3028 if (this.dietPreference == null) 3029 this.dietPreference = new ArrayList<CodeableConcept>(); 3030 return this.dietPreference; 3031 } 3032 3033 /** 3034 * @return Returns a reference to <code>this</code> for easy method chaining 3035 */ 3036 public Encounter setDietPreference(List<CodeableConcept> theDietPreference) { 3037 this.dietPreference = theDietPreference; 3038 return this; 3039 } 3040 3041 public boolean hasDietPreference() { 3042 if (this.dietPreference == null) 3043 return false; 3044 for (CodeableConcept item : this.dietPreference) 3045 if (!item.isEmpty()) 3046 return true; 3047 return false; 3048 } 3049 3050 public CodeableConcept addDietPreference() { //3 3051 CodeableConcept t = new CodeableConcept(); 3052 if (this.dietPreference == null) 3053 this.dietPreference = new ArrayList<CodeableConcept>(); 3054 this.dietPreference.add(t); 3055 return t; 3056 } 3057 3058 public Encounter addDietPreference(CodeableConcept t) { //3 3059 if (t == null) 3060 return this; 3061 if (this.dietPreference == null) 3062 this.dietPreference = new ArrayList<CodeableConcept>(); 3063 this.dietPreference.add(t); 3064 return this; 3065 } 3066 3067 /** 3068 * @return The first repetition of repeating field {@link #dietPreference}, creating it if it does not already exist {3} 3069 */ 3070 public CodeableConcept getDietPreferenceFirstRep() { 3071 if (getDietPreference().isEmpty()) { 3072 addDietPreference(); 3073 } 3074 return getDietPreference().get(0); 3075 } 3076 3077 /** 3078 * @return {@link #specialArrangement} (Any special requests that have been made for this encounter, such as the provision of specific equipment or other things.) 3079 */ 3080 public List<CodeableConcept> getSpecialArrangement() { 3081 if (this.specialArrangement == null) 3082 this.specialArrangement = new ArrayList<CodeableConcept>(); 3083 return this.specialArrangement; 3084 } 3085 3086 /** 3087 * @return Returns a reference to <code>this</code> for easy method chaining 3088 */ 3089 public Encounter setSpecialArrangement(List<CodeableConcept> theSpecialArrangement) { 3090 this.specialArrangement = theSpecialArrangement; 3091 return this; 3092 } 3093 3094 public boolean hasSpecialArrangement() { 3095 if (this.specialArrangement == null) 3096 return false; 3097 for (CodeableConcept item : this.specialArrangement) 3098 if (!item.isEmpty()) 3099 return true; 3100 return false; 3101 } 3102 3103 public CodeableConcept addSpecialArrangement() { //3 3104 CodeableConcept t = new CodeableConcept(); 3105 if (this.specialArrangement == null) 3106 this.specialArrangement = new ArrayList<CodeableConcept>(); 3107 this.specialArrangement.add(t); 3108 return t; 3109 } 3110 3111 public Encounter addSpecialArrangement(CodeableConcept t) { //3 3112 if (t == null) 3113 return this; 3114 if (this.specialArrangement == null) 3115 this.specialArrangement = new ArrayList<CodeableConcept>(); 3116 this.specialArrangement.add(t); 3117 return this; 3118 } 3119 3120 /** 3121 * @return The first repetition of repeating field {@link #specialArrangement}, creating it if it does not already exist {3} 3122 */ 3123 public CodeableConcept getSpecialArrangementFirstRep() { 3124 if (getSpecialArrangement().isEmpty()) { 3125 addSpecialArrangement(); 3126 } 3127 return getSpecialArrangement().get(0); 3128 } 3129 3130 /** 3131 * @return {@link #specialCourtesy} (Special courtesies that may be provided to the patient during the encounter (VIP, board member, professional courtesy).) 3132 */ 3133 public List<CodeableConcept> getSpecialCourtesy() { 3134 if (this.specialCourtesy == null) 3135 this.specialCourtesy = new ArrayList<CodeableConcept>(); 3136 return this.specialCourtesy; 3137 } 3138 3139 /** 3140 * @return Returns a reference to <code>this</code> for easy method chaining 3141 */ 3142 public Encounter setSpecialCourtesy(List<CodeableConcept> theSpecialCourtesy) { 3143 this.specialCourtesy = theSpecialCourtesy; 3144 return this; 3145 } 3146 3147 public boolean hasSpecialCourtesy() { 3148 if (this.specialCourtesy == null) 3149 return false; 3150 for (CodeableConcept item : this.specialCourtesy) 3151 if (!item.isEmpty()) 3152 return true; 3153 return false; 3154 } 3155 3156 public CodeableConcept addSpecialCourtesy() { //3 3157 CodeableConcept t = new CodeableConcept(); 3158 if (this.specialCourtesy == null) 3159 this.specialCourtesy = new ArrayList<CodeableConcept>(); 3160 this.specialCourtesy.add(t); 3161 return t; 3162 } 3163 3164 public Encounter addSpecialCourtesy(CodeableConcept t) { //3 3165 if (t == null) 3166 return this; 3167 if (this.specialCourtesy == null) 3168 this.specialCourtesy = new ArrayList<CodeableConcept>(); 3169 this.specialCourtesy.add(t); 3170 return this; 3171 } 3172 3173 /** 3174 * @return The first repetition of repeating field {@link #specialCourtesy}, creating it if it does not already exist {3} 3175 */ 3176 public CodeableConcept getSpecialCourtesyFirstRep() { 3177 if (getSpecialCourtesy().isEmpty()) { 3178 addSpecialCourtesy(); 3179 } 3180 return getSpecialCourtesy().get(0); 3181 } 3182 3183 /** 3184 * @return {@link #admission} (Details about the stay during which a healthcare service is provided. 3185 3186This does not describe the event of admitting the patient, but rather any information that is relevant from the time of admittance until the time of discharge.) 3187 */ 3188 public EncounterAdmissionComponent getAdmission() { 3189 if (this.admission == null) 3190 if (Configuration.errorOnAutoCreate()) 3191 throw new Error("Attempt to auto-create Encounter.admission"); 3192 else if (Configuration.doAutoCreate()) 3193 this.admission = new EncounterAdmissionComponent(); // cc 3194 return this.admission; 3195 } 3196 3197 public boolean hasAdmission() { 3198 return this.admission != null && !this.admission.isEmpty(); 3199 } 3200 3201 /** 3202 * @param value {@link #admission} (Details about the stay during which a healthcare service is provided. 3203 3204This does not describe the event of admitting the patient, but rather any information that is relevant from the time of admittance until the time of discharge.) 3205 */ 3206 public Encounter setAdmission(EncounterAdmissionComponent value) { 3207 this.admission = value; 3208 return this; 3209 } 3210 3211 /** 3212 * @return {@link #location} (List of locations where the patient has been during this encounter.) 3213 */ 3214 public List<EncounterLocationComponent> getLocation() { 3215 if (this.location == null) 3216 this.location = new ArrayList<EncounterLocationComponent>(); 3217 return this.location; 3218 } 3219 3220 /** 3221 * @return Returns a reference to <code>this</code> for easy method chaining 3222 */ 3223 public Encounter setLocation(List<EncounterLocationComponent> theLocation) { 3224 this.location = theLocation; 3225 return this; 3226 } 3227 3228 public boolean hasLocation() { 3229 if (this.location == null) 3230 return false; 3231 for (EncounterLocationComponent item : this.location) 3232 if (!item.isEmpty()) 3233 return true; 3234 return false; 3235 } 3236 3237 public EncounterLocationComponent addLocation() { //3 3238 EncounterLocationComponent t = new EncounterLocationComponent(); 3239 if (this.location == null) 3240 this.location = new ArrayList<EncounterLocationComponent>(); 3241 this.location.add(t); 3242 return t; 3243 } 3244 3245 public Encounter addLocation(EncounterLocationComponent t) { //3 3246 if (t == null) 3247 return this; 3248 if (this.location == null) 3249 this.location = new ArrayList<EncounterLocationComponent>(); 3250 this.location.add(t); 3251 return this; 3252 } 3253 3254 /** 3255 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist {3} 3256 */ 3257 public EncounterLocationComponent getLocationFirstRep() { 3258 if (getLocation().isEmpty()) { 3259 addLocation(); 3260 } 3261 return getLocation().get(0); 3262 } 3263 3264 protected void listChildren(List<Property> children) { 3265 super.listChildren(children); 3266 children.add(new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3267 children.add(new Property("status", "code", "The current state of the encounter (not the state of the patient within the encounter - that is subjectState).", 0, 1, status)); 3268 children.add(new Property("class", "CodeableConcept", "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 0, java.lang.Integer.MAX_VALUE, class_)); 3269 children.add(new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, priority)); 3270 children.add(new Property("type", "CodeableConcept", "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, java.lang.Integer.MAX_VALUE, type)); 3271 children.add(new Property("serviceType", "CodeableReference(HealthcareService)", "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, java.lang.Integer.MAX_VALUE, serviceType)); 3272 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.", 0, 1, subject)); 3273 children.add(new Property("subjectStatus", "CodeableConcept", "The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.", 0, 1, subjectStatus)); 3274 children.add(new Property("episodeOfCare", "Reference(EpisodeOfCare)", "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 0, java.lang.Integer.MAX_VALUE, episodeOfCare)); 3275 children.add(new Property("basedOn", "Reference(CarePlan|DeviceRequest|MedicationRequest|ServiceRequest)", "The request this encounter satisfies (e.g. incoming referral or procedure request).", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3276 children.add(new Property("careTeam", "Reference(CareTeam)", "The group(s) of individuals, organizations that are allocated to participate in this encounter. The participants backbone will record the actuals of when these individuals participated during the encounter.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 3277 children.add(new Property("partOf", "Reference(Encounter)", "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf)); 3278 children.add(new Property("serviceProvider", "Reference(Organization)", "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the colonoscopy example on the Encounter examples tab.", 0, 1, serviceProvider)); 3279 children.add(new Property("participant", "", "The list of people responsible for providing the service.", 0, java.lang.Integer.MAX_VALUE, participant)); 3280 children.add(new Property("appointment", "Reference(Appointment)", "The appointment that scheduled this encounter.", 0, java.lang.Integer.MAX_VALUE, appointment)); 3281 children.add(new Property("virtualService", "VirtualServiceDetail", "Connection details of a virtual service (e.g. conference call).", 0, java.lang.Integer.MAX_VALUE, virtualService)); 3282 children.add(new Property("actualPeriod", "Period", "The actual start and end time of the encounter.", 0, 1, actualPeriod)); 3283 children.add(new Property("plannedStartDate", "dateTime", "The planned start date/time (or admission date) of the encounter.", 0, 1, plannedStartDate)); 3284 children.add(new Property("plannedEndDate", "dateTime", "The planned end date/time (or discharge date) of the encounter.", 0, 1, plannedEndDate)); 3285 children.add(new Property("length", "Duration", "Actual quantity of time the encounter lasted. This excludes the time during leaves of absence.\r\rWhen missing it is the time in between the start and end values.", 0, 1, length)); 3286 children.add(new Property("reason", "", "The list of medical reasons that are expected to be addressed during the episode of care.", 0, java.lang.Integer.MAX_VALUE, reason)); 3287 children.add(new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 3288 children.add(new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, account)); 3289 children.add(new Property("dietPreference", "CodeableConcept", "Diet preferences reported by the patient.", 0, java.lang.Integer.MAX_VALUE, dietPreference)); 3290 children.add(new Property("specialArrangement", "CodeableConcept", "Any special requests that have been made for this encounter, such as the provision of specific equipment or other things.", 0, java.lang.Integer.MAX_VALUE, specialArrangement)); 3291 children.add(new Property("specialCourtesy", "CodeableConcept", "Special courtesies that may be provided to the patient during the encounter (VIP, board member, professional courtesy).", 0, java.lang.Integer.MAX_VALUE, specialCourtesy)); 3292 children.add(new Property("admission", "", "Details about the stay during which a healthcare service is provided.\r\rThis does not describe the event of admitting the patient, but rather any information that is relevant from the time of admittance until the time of discharge.", 0, 1, admission)); 3293 children.add(new Property("location", "", "List of locations where the patient has been during this encounter.", 0, java.lang.Integer.MAX_VALUE, location)); 3294 } 3295 3296 @Override 3297 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3298 switch (_hash) { 3299 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier); 3300 case -892481550: /*status*/ return new Property("status", "code", "The current state of the encounter (not the state of the patient within the encounter - that is subjectState).", 0, 1, status); 3301 case 94742904: /*class*/ return new Property("class", "CodeableConcept", "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 0, java.lang.Integer.MAX_VALUE, class_); 3302 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, priority); 3303 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, java.lang.Integer.MAX_VALUE, type); 3304 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableReference(HealthcareService)", "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, java.lang.Integer.MAX_VALUE, serviceType); 3305 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.", 0, 1, subject); 3306 case 110854206: /*subjectStatus*/ return new Property("subjectStatus", "CodeableConcept", "The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.", 0, 1, subjectStatus); 3307 case -1892140189: /*episodeOfCare*/ return new Property("episodeOfCare", "Reference(EpisodeOfCare)", "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 0, java.lang.Integer.MAX_VALUE, episodeOfCare); 3308 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|DeviceRequest|MedicationRequest|ServiceRequest)", "The request this encounter satisfies (e.g. incoming referral or procedure request).", 0, java.lang.Integer.MAX_VALUE, basedOn); 3309 case -7323378: /*careTeam*/ return new Property("careTeam", "Reference(CareTeam)", "The group(s) of individuals, organizations that are allocated to participate in this encounter. The participants backbone will record the actuals of when these individuals participated during the encounter.", 0, java.lang.Integer.MAX_VALUE, careTeam); 3310 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Encounter)", "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf); 3311 case 243182534: /*serviceProvider*/ return new Property("serviceProvider", "Reference(Organization)", "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the colonoscopy example on the Encounter examples tab.", 0, 1, serviceProvider); 3312 case 767422259: /*participant*/ return new Property("participant", "", "The list of people responsible for providing the service.", 0, java.lang.Integer.MAX_VALUE, participant); 3313 case -1474995297: /*appointment*/ return new Property("appointment", "Reference(Appointment)", "The appointment that scheduled this encounter.", 0, java.lang.Integer.MAX_VALUE, appointment); 3314 case 1420774698: /*virtualService*/ return new Property("virtualService", "VirtualServiceDetail", "Connection details of a virtual service (e.g. conference call).", 0, java.lang.Integer.MAX_VALUE, virtualService); 3315 case 789194991: /*actualPeriod*/ return new Property("actualPeriod", "Period", "The actual start and end time of the encounter.", 0, 1, actualPeriod); 3316 case 460857804: /*plannedStartDate*/ return new Property("plannedStartDate", "dateTime", "The planned start date/time (or admission date) of the encounter.", 0, 1, plannedStartDate); 3317 case 1657534661: /*plannedEndDate*/ return new Property("plannedEndDate", "dateTime", "The planned end date/time (or discharge date) of the encounter.", 0, 1, plannedEndDate); 3318 case -1106363674: /*length*/ return new Property("length", "Duration", "Actual quantity of time the encounter lasted. This excludes the time during leaves of absence.\r\rWhen missing it is the time in between the start and end values.", 0, 1, length); 3319 case -934964668: /*reason*/ return new Property("reason", "", "The list of medical reasons that are expected to be addressed during the episode of care.", 0, java.lang.Integer.MAX_VALUE, reason); 3320 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 3321 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, account); 3322 case -1360641041: /*dietPreference*/ return new Property("dietPreference", "CodeableConcept", "Diet preferences reported by the patient.", 0, java.lang.Integer.MAX_VALUE, dietPreference); 3323 case 47410321: /*specialArrangement*/ return new Property("specialArrangement", "CodeableConcept", "Any special requests that have been made for this encounter, such as the provision of specific equipment or other things.", 0, java.lang.Integer.MAX_VALUE, specialArrangement); 3324 case 1583588345: /*specialCourtesy*/ return new Property("specialCourtesy", "CodeableConcept", "Special courtesies that may be provided to the patient during the encounter (VIP, board member, professional courtesy).", 0, java.lang.Integer.MAX_VALUE, specialCourtesy); 3325 case 27400201: /*admission*/ return new Property("admission", "", "Details about the stay during which a healthcare service is provided.\r\rThis does not describe the event of admitting the patient, but rather any information that is relevant from the time of admittance until the time of discharge.", 0, 1, admission); 3326 case 1901043637: /*location*/ return new Property("location", "", "List of locations where the patient has been during this encounter.", 0, java.lang.Integer.MAX_VALUE, location); 3327 default: return super.getNamedProperty(_hash, _name, _checkValid); 3328 } 3329 3330 } 3331 3332 @Override 3333 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3334 switch (hash) { 3335 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3336 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EncounterStatus> 3337 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // CodeableConcept 3338 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 3339 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3340 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableReference 3341 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3342 case 110854206: /*subjectStatus*/ return this.subjectStatus == null ? new Base[0] : new Base[] {this.subjectStatus}; // CodeableConcept 3343 case -1892140189: /*episodeOfCare*/ return this.episodeOfCare == null ? new Base[0] : this.episodeOfCare.toArray(new Base[this.episodeOfCare.size()]); // Reference 3344 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3345 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // Reference 3346 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : new Base[] {this.partOf}; // Reference 3347 case 243182534: /*serviceProvider*/ return this.serviceProvider == null ? new Base[0] : new Base[] {this.serviceProvider}; // Reference 3348 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // EncounterParticipantComponent 3349 case -1474995297: /*appointment*/ return this.appointment == null ? new Base[0] : this.appointment.toArray(new Base[this.appointment.size()]); // Reference 3350 case 1420774698: /*virtualService*/ return this.virtualService == null ? new Base[0] : this.virtualService.toArray(new Base[this.virtualService.size()]); // VirtualServiceDetail 3351 case 789194991: /*actualPeriod*/ return this.actualPeriod == null ? new Base[0] : new Base[] {this.actualPeriod}; // Period 3352 case 460857804: /*plannedStartDate*/ return this.plannedStartDate == null ? new Base[0] : new Base[] {this.plannedStartDate}; // DateTimeType 3353 case 1657534661: /*plannedEndDate*/ return this.plannedEndDate == null ? new Base[0] : new Base[] {this.plannedEndDate}; // DateTimeType 3354 case -1106363674: /*length*/ return this.length == null ? new Base[0] : new Base[] {this.length}; // Duration 3355 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // ReasonComponent 3356 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 3357 case -1177318867: /*account*/ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 3358 case -1360641041: /*dietPreference*/ return this.dietPreference == null ? new Base[0] : this.dietPreference.toArray(new Base[this.dietPreference.size()]); // CodeableConcept 3359 case 47410321: /*specialArrangement*/ return this.specialArrangement == null ? new Base[0] : this.specialArrangement.toArray(new Base[this.specialArrangement.size()]); // CodeableConcept 3360 case 1583588345: /*specialCourtesy*/ return this.specialCourtesy == null ? new Base[0] : this.specialCourtesy.toArray(new Base[this.specialCourtesy.size()]); // CodeableConcept 3361 case 27400201: /*admission*/ return this.admission == null ? new Base[0] : new Base[] {this.admission}; // EncounterAdmissionComponent 3362 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // EncounterLocationComponent 3363 default: return super.getProperty(hash, name, checkValid); 3364 } 3365 3366 } 3367 3368 @Override 3369 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3370 switch (hash) { 3371 case -1618432855: // identifier 3372 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3373 return value; 3374 case -892481550: // status 3375 value = new EncounterStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3376 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 3377 return value; 3378 case 94742904: // class 3379 this.getClass_().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3380 return value; 3381 case -1165461084: // priority 3382 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3383 return value; 3384 case 3575610: // type 3385 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3386 return value; 3387 case -1928370289: // serviceType 3388 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 3389 return value; 3390 case -1867885268: // subject 3391 this.subject = TypeConvertor.castToReference(value); // Reference 3392 return value; 3393 case 110854206: // subjectStatus 3394 this.subjectStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3395 return value; 3396 case -1892140189: // episodeOfCare 3397 this.getEpisodeOfCare().add(TypeConvertor.castToReference(value)); // Reference 3398 return value; 3399 case -332612366: // basedOn 3400 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 3401 return value; 3402 case -7323378: // careTeam 3403 this.getCareTeam().add(TypeConvertor.castToReference(value)); // Reference 3404 return value; 3405 case -995410646: // partOf 3406 this.partOf = TypeConvertor.castToReference(value); // Reference 3407 return value; 3408 case 243182534: // serviceProvider 3409 this.serviceProvider = TypeConvertor.castToReference(value); // Reference 3410 return value; 3411 case 767422259: // participant 3412 this.getParticipant().add((EncounterParticipantComponent) value); // EncounterParticipantComponent 3413 return value; 3414 case -1474995297: // appointment 3415 this.getAppointment().add(TypeConvertor.castToReference(value)); // Reference 3416 return value; 3417 case 1420774698: // virtualService 3418 this.getVirtualService().add(TypeConvertor.castToVirtualServiceDetail(value)); // VirtualServiceDetail 3419 return value; 3420 case 789194991: // actualPeriod 3421 this.actualPeriod = TypeConvertor.castToPeriod(value); // Period 3422 return value; 3423 case 460857804: // plannedStartDate 3424 this.plannedStartDate = TypeConvertor.castToDateTime(value); // DateTimeType 3425 return value; 3426 case 1657534661: // plannedEndDate 3427 this.plannedEndDate = TypeConvertor.castToDateTime(value); // DateTimeType 3428 return value; 3429 case -1106363674: // length 3430 this.length = TypeConvertor.castToDuration(value); // Duration 3431 return value; 3432 case -934964668: // reason 3433 this.getReason().add((ReasonComponent) value); // ReasonComponent 3434 return value; 3435 case 1196993265: // diagnosis 3436 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 3437 return value; 3438 case -1177318867: // account 3439 this.getAccount().add(TypeConvertor.castToReference(value)); // Reference 3440 return value; 3441 case -1360641041: // dietPreference 3442 this.getDietPreference().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3443 return value; 3444 case 47410321: // specialArrangement 3445 this.getSpecialArrangement().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3446 return value; 3447 case 1583588345: // specialCourtesy 3448 this.getSpecialCourtesy().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3449 return value; 3450 case 27400201: // admission 3451 this.admission = (EncounterAdmissionComponent) value; // EncounterAdmissionComponent 3452 return value; 3453 case 1901043637: // location 3454 this.getLocation().add((EncounterLocationComponent) value); // EncounterLocationComponent 3455 return value; 3456 default: return super.setProperty(hash, name, value); 3457 } 3458 3459 } 3460 3461 @Override 3462 public Base setProperty(String name, Base value) throws FHIRException { 3463 if (name.equals("identifier")) { 3464 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3465 } else if (name.equals("status")) { 3466 value = new EncounterStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3467 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 3468 } else if (name.equals("class")) { 3469 this.getClass_().add(TypeConvertor.castToCodeableConcept(value)); 3470 } else if (name.equals("priority")) { 3471 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3472 } else if (name.equals("type")) { 3473 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 3474 } else if (name.equals("serviceType")) { 3475 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); 3476 } else if (name.equals("subject")) { 3477 this.subject = TypeConvertor.castToReference(value); // Reference 3478 } else if (name.equals("subjectStatus")) { 3479 this.subjectStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3480 } else if (name.equals("episodeOfCare")) { 3481 this.getEpisodeOfCare().add(TypeConvertor.castToReference(value)); 3482 } else if (name.equals("basedOn")) { 3483 this.getBasedOn().add(TypeConvertor.castToReference(value)); 3484 } else if (name.equals("careTeam")) { 3485 this.getCareTeam().add(TypeConvertor.castToReference(value)); 3486 } else if (name.equals("partOf")) { 3487 this.partOf = TypeConvertor.castToReference(value); // Reference 3488 } else if (name.equals("serviceProvider")) { 3489 this.serviceProvider = TypeConvertor.castToReference(value); // Reference 3490 } else if (name.equals("participant")) { 3491 this.getParticipant().add((EncounterParticipantComponent) value); 3492 } else if (name.equals("appointment")) { 3493 this.getAppointment().add(TypeConvertor.castToReference(value)); 3494 } else if (name.equals("virtualService")) { 3495 this.getVirtualService().add(TypeConvertor.castToVirtualServiceDetail(value)); 3496 } else if (name.equals("actualPeriod")) { 3497 this.actualPeriod = TypeConvertor.castToPeriod(value); // Period 3498 } else if (name.equals("plannedStartDate")) { 3499 this.plannedStartDate = TypeConvertor.castToDateTime(value); // DateTimeType 3500 } else if (name.equals("plannedEndDate")) { 3501 this.plannedEndDate = TypeConvertor.castToDateTime(value); // DateTimeType 3502 } else if (name.equals("length")) { 3503 this.length = TypeConvertor.castToDuration(value); // Duration 3504 } else if (name.equals("reason")) { 3505 this.getReason().add((ReasonComponent) value); 3506 } else if (name.equals("diagnosis")) { 3507 this.getDiagnosis().add((DiagnosisComponent) value); 3508 } else if (name.equals("account")) { 3509 this.getAccount().add(TypeConvertor.castToReference(value)); 3510 } else if (name.equals("dietPreference")) { 3511 this.getDietPreference().add(TypeConvertor.castToCodeableConcept(value)); 3512 } else if (name.equals("specialArrangement")) { 3513 this.getSpecialArrangement().add(TypeConvertor.castToCodeableConcept(value)); 3514 } else if (name.equals("specialCourtesy")) { 3515 this.getSpecialCourtesy().add(TypeConvertor.castToCodeableConcept(value)); 3516 } else if (name.equals("admission")) { 3517 this.admission = (EncounterAdmissionComponent) value; // EncounterAdmissionComponent 3518 } else if (name.equals("location")) { 3519 this.getLocation().add((EncounterLocationComponent) value); 3520 } else 3521 return super.setProperty(name, value); 3522 return value; 3523 } 3524 3525 @Override 3526 public void removeChild(String name, Base value) throws FHIRException { 3527 if (name.equals("identifier")) { 3528 this.getIdentifier().remove(value); 3529 } else if (name.equals("status")) { 3530 value = new EncounterStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3531 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 3532 } else if (name.equals("class")) { 3533 this.getClass_().remove(value); 3534 } else if (name.equals("priority")) { 3535 this.priority = null; 3536 } else if (name.equals("type")) { 3537 this.getType().remove(value); 3538 } else if (name.equals("serviceType")) { 3539 this.getServiceType().remove(value); 3540 } else if (name.equals("subject")) { 3541 this.subject = null; 3542 } else if (name.equals("subjectStatus")) { 3543 this.subjectStatus = null; 3544 } else if (name.equals("episodeOfCare")) { 3545 this.getEpisodeOfCare().remove(value); 3546 } else if (name.equals("basedOn")) { 3547 this.getBasedOn().remove(value); 3548 } else if (name.equals("careTeam")) { 3549 this.getCareTeam().remove(value); 3550 } else if (name.equals("partOf")) { 3551 this.partOf = null; 3552 } else if (name.equals("serviceProvider")) { 3553 this.serviceProvider = null; 3554 } else if (name.equals("participant")) { 3555 this.getParticipant().remove((EncounterParticipantComponent) value); 3556 } else if (name.equals("appointment")) { 3557 this.getAppointment().remove(value); 3558 } else if (name.equals("virtualService")) { 3559 this.getVirtualService().remove(value); 3560 } else if (name.equals("actualPeriod")) { 3561 this.actualPeriod = null; 3562 } else if (name.equals("plannedStartDate")) { 3563 this.plannedStartDate = null; 3564 } else if (name.equals("plannedEndDate")) { 3565 this.plannedEndDate = null; 3566 } else if (name.equals("length")) { 3567 this.length = null; 3568 } else if (name.equals("reason")) { 3569 this.getReason().remove((ReasonComponent) value); 3570 } else if (name.equals("diagnosis")) { 3571 this.getDiagnosis().remove((DiagnosisComponent) value); 3572 } else if (name.equals("account")) { 3573 this.getAccount().remove(value); 3574 } else if (name.equals("dietPreference")) { 3575 this.getDietPreference().remove(value); 3576 } else if (name.equals("specialArrangement")) { 3577 this.getSpecialArrangement().remove(value); 3578 } else if (name.equals("specialCourtesy")) { 3579 this.getSpecialCourtesy().remove(value); 3580 } else if (name.equals("admission")) { 3581 this.admission = (EncounterAdmissionComponent) value; // EncounterAdmissionComponent 3582 } else if (name.equals("location")) { 3583 this.getLocation().remove((EncounterLocationComponent) value); 3584 } else 3585 super.removeChild(name, value); 3586 3587 } 3588 3589 @Override 3590 public Base makeProperty(int hash, String name) throws FHIRException { 3591 switch (hash) { 3592 case -1618432855: return addIdentifier(); 3593 case -892481550: return getStatusElement(); 3594 case 94742904: return addClass_(); 3595 case -1165461084: return getPriority(); 3596 case 3575610: return addType(); 3597 case -1928370289: return addServiceType(); 3598 case -1867885268: return getSubject(); 3599 case 110854206: return getSubjectStatus(); 3600 case -1892140189: return addEpisodeOfCare(); 3601 case -332612366: return addBasedOn(); 3602 case -7323378: return addCareTeam(); 3603 case -995410646: return getPartOf(); 3604 case 243182534: return getServiceProvider(); 3605 case 767422259: return addParticipant(); 3606 case -1474995297: return addAppointment(); 3607 case 1420774698: return addVirtualService(); 3608 case 789194991: return getActualPeriod(); 3609 case 460857804: return getPlannedStartDateElement(); 3610 case 1657534661: return getPlannedEndDateElement(); 3611 case -1106363674: return getLength(); 3612 case -934964668: return addReason(); 3613 case 1196993265: return addDiagnosis(); 3614 case -1177318867: return addAccount(); 3615 case -1360641041: return addDietPreference(); 3616 case 47410321: return addSpecialArrangement(); 3617 case 1583588345: return addSpecialCourtesy(); 3618 case 27400201: return getAdmission(); 3619 case 1901043637: return addLocation(); 3620 default: return super.makeProperty(hash, name); 3621 } 3622 3623 } 3624 3625 @Override 3626 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3627 switch (hash) { 3628 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3629 case -892481550: /*status*/ return new String[] {"code"}; 3630 case 94742904: /*class*/ return new String[] {"CodeableConcept"}; 3631 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 3632 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3633 case -1928370289: /*serviceType*/ return new String[] {"CodeableReference"}; 3634 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3635 case 110854206: /*subjectStatus*/ return new String[] {"CodeableConcept"}; 3636 case -1892140189: /*episodeOfCare*/ return new String[] {"Reference"}; 3637 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3638 case -7323378: /*careTeam*/ return new String[] {"Reference"}; 3639 case -995410646: /*partOf*/ return new String[] {"Reference"}; 3640 case 243182534: /*serviceProvider*/ return new String[] {"Reference"}; 3641 case 767422259: /*participant*/ return new String[] {}; 3642 case -1474995297: /*appointment*/ return new String[] {"Reference"}; 3643 case 1420774698: /*virtualService*/ return new String[] {"VirtualServiceDetail"}; 3644 case 789194991: /*actualPeriod*/ return new String[] {"Period"}; 3645 case 460857804: /*plannedStartDate*/ return new String[] {"dateTime"}; 3646 case 1657534661: /*plannedEndDate*/ return new String[] {"dateTime"}; 3647 case -1106363674: /*length*/ return new String[] {"Duration"}; 3648 case -934964668: /*reason*/ return new String[] {}; 3649 case 1196993265: /*diagnosis*/ return new String[] {}; 3650 case -1177318867: /*account*/ return new String[] {"Reference"}; 3651 case -1360641041: /*dietPreference*/ return new String[] {"CodeableConcept"}; 3652 case 47410321: /*specialArrangement*/ return new String[] {"CodeableConcept"}; 3653 case 1583588345: /*specialCourtesy*/ return new String[] {"CodeableConcept"}; 3654 case 27400201: /*admission*/ return new String[] {}; 3655 case 1901043637: /*location*/ return new String[] {}; 3656 default: return super.getTypesForProperty(hash, name); 3657 } 3658 3659 } 3660 3661 @Override 3662 public Base addChild(String name) throws FHIRException { 3663 if (name.equals("identifier")) { 3664 return addIdentifier(); 3665 } 3666 else if (name.equals("status")) { 3667 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 3668 } 3669 else if (name.equals("class")) { 3670 return addClass_(); 3671 } 3672 else if (name.equals("priority")) { 3673 this.priority = new CodeableConcept(); 3674 return this.priority; 3675 } 3676 else if (name.equals("type")) { 3677 return addType(); 3678 } 3679 else if (name.equals("serviceType")) { 3680 return addServiceType(); 3681 } 3682 else if (name.equals("subject")) { 3683 this.subject = new Reference(); 3684 return this.subject; 3685 } 3686 else if (name.equals("subjectStatus")) { 3687 this.subjectStatus = new CodeableConcept(); 3688 return this.subjectStatus; 3689 } 3690 else if (name.equals("episodeOfCare")) { 3691 return addEpisodeOfCare(); 3692 } 3693 else if (name.equals("basedOn")) { 3694 return addBasedOn(); 3695 } 3696 else if (name.equals("careTeam")) { 3697 return addCareTeam(); 3698 } 3699 else if (name.equals("partOf")) { 3700 this.partOf = new Reference(); 3701 return this.partOf; 3702 } 3703 else if (name.equals("serviceProvider")) { 3704 this.serviceProvider = new Reference(); 3705 return this.serviceProvider; 3706 } 3707 else if (name.equals("participant")) { 3708 return addParticipant(); 3709 } 3710 else if (name.equals("appointment")) { 3711 return addAppointment(); 3712 } 3713 else if (name.equals("virtualService")) { 3714 return addVirtualService(); 3715 } 3716 else if (name.equals("actualPeriod")) { 3717 this.actualPeriod = new Period(); 3718 return this.actualPeriod; 3719 } 3720 else if (name.equals("plannedStartDate")) { 3721 throw new FHIRException("Cannot call addChild on a singleton property Encounter.plannedStartDate"); 3722 } 3723 else if (name.equals("plannedEndDate")) { 3724 throw new FHIRException("Cannot call addChild on a singleton property Encounter.plannedEndDate"); 3725 } 3726 else if (name.equals("length")) { 3727 this.length = new Duration(); 3728 return this.length; 3729 } 3730 else if (name.equals("reason")) { 3731 return addReason(); 3732 } 3733 else if (name.equals("diagnosis")) { 3734 return addDiagnosis(); 3735 } 3736 else if (name.equals("account")) { 3737 return addAccount(); 3738 } 3739 else if (name.equals("dietPreference")) { 3740 return addDietPreference(); 3741 } 3742 else if (name.equals("specialArrangement")) { 3743 return addSpecialArrangement(); 3744 } 3745 else if (name.equals("specialCourtesy")) { 3746 return addSpecialCourtesy(); 3747 } 3748 else if (name.equals("admission")) { 3749 this.admission = new EncounterAdmissionComponent(); 3750 return this.admission; 3751 } 3752 else if (name.equals("location")) { 3753 return addLocation(); 3754 } 3755 else 3756 return super.addChild(name); 3757 } 3758 3759 public String fhirType() { 3760 return "Encounter"; 3761 3762 } 3763 3764 public Encounter copy() { 3765 Encounter dst = new Encounter(); 3766 copyValues(dst); 3767 return dst; 3768 } 3769 3770 public void copyValues(Encounter dst) { 3771 super.copyValues(dst); 3772 if (identifier != null) { 3773 dst.identifier = new ArrayList<Identifier>(); 3774 for (Identifier i : identifier) 3775 dst.identifier.add(i.copy()); 3776 }; 3777 dst.status = status == null ? null : status.copy(); 3778 if (class_ != null) { 3779 dst.class_ = new ArrayList<CodeableConcept>(); 3780 for (CodeableConcept i : class_) 3781 dst.class_.add(i.copy()); 3782 }; 3783 dst.priority = priority == null ? null : priority.copy(); 3784 if (type != null) { 3785 dst.type = new ArrayList<CodeableConcept>(); 3786 for (CodeableConcept i : type) 3787 dst.type.add(i.copy()); 3788 }; 3789 if (serviceType != null) { 3790 dst.serviceType = new ArrayList<CodeableReference>(); 3791 for (CodeableReference i : serviceType) 3792 dst.serviceType.add(i.copy()); 3793 }; 3794 dst.subject = subject == null ? null : subject.copy(); 3795 dst.subjectStatus = subjectStatus == null ? null : subjectStatus.copy(); 3796 if (episodeOfCare != null) { 3797 dst.episodeOfCare = new ArrayList<Reference>(); 3798 for (Reference i : episodeOfCare) 3799 dst.episodeOfCare.add(i.copy()); 3800 }; 3801 if (basedOn != null) { 3802 dst.basedOn = new ArrayList<Reference>(); 3803 for (Reference i : basedOn) 3804 dst.basedOn.add(i.copy()); 3805 }; 3806 if (careTeam != null) { 3807 dst.careTeam = new ArrayList<Reference>(); 3808 for (Reference i : careTeam) 3809 dst.careTeam.add(i.copy()); 3810 }; 3811 dst.partOf = partOf == null ? null : partOf.copy(); 3812 dst.serviceProvider = serviceProvider == null ? null : serviceProvider.copy(); 3813 if (participant != null) { 3814 dst.participant = new ArrayList<EncounterParticipantComponent>(); 3815 for (EncounterParticipantComponent i : participant) 3816 dst.participant.add(i.copy()); 3817 }; 3818 if (appointment != null) { 3819 dst.appointment = new ArrayList<Reference>(); 3820 for (Reference i : appointment) 3821 dst.appointment.add(i.copy()); 3822 }; 3823 if (virtualService != null) { 3824 dst.virtualService = new ArrayList<VirtualServiceDetail>(); 3825 for (VirtualServiceDetail i : virtualService) 3826 dst.virtualService.add(i.copy()); 3827 }; 3828 dst.actualPeriod = actualPeriod == null ? null : actualPeriod.copy(); 3829 dst.plannedStartDate = plannedStartDate == null ? null : plannedStartDate.copy(); 3830 dst.plannedEndDate = plannedEndDate == null ? null : plannedEndDate.copy(); 3831 dst.length = length == null ? null : length.copy(); 3832 if (reason != null) { 3833 dst.reason = new ArrayList<ReasonComponent>(); 3834 for (ReasonComponent i : reason) 3835 dst.reason.add(i.copy()); 3836 }; 3837 if (diagnosis != null) { 3838 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 3839 for (DiagnosisComponent i : diagnosis) 3840 dst.diagnosis.add(i.copy()); 3841 }; 3842 if (account != null) { 3843 dst.account = new ArrayList<Reference>(); 3844 for (Reference i : account) 3845 dst.account.add(i.copy()); 3846 }; 3847 if (dietPreference != null) { 3848 dst.dietPreference = new ArrayList<CodeableConcept>(); 3849 for (CodeableConcept i : dietPreference) 3850 dst.dietPreference.add(i.copy()); 3851 }; 3852 if (specialArrangement != null) { 3853 dst.specialArrangement = new ArrayList<CodeableConcept>(); 3854 for (CodeableConcept i : specialArrangement) 3855 dst.specialArrangement.add(i.copy()); 3856 }; 3857 if (specialCourtesy != null) { 3858 dst.specialCourtesy = new ArrayList<CodeableConcept>(); 3859 for (CodeableConcept i : specialCourtesy) 3860 dst.specialCourtesy.add(i.copy()); 3861 }; 3862 dst.admission = admission == null ? null : admission.copy(); 3863 if (location != null) { 3864 dst.location = new ArrayList<EncounterLocationComponent>(); 3865 for (EncounterLocationComponent i : location) 3866 dst.location.add(i.copy()); 3867 }; 3868 } 3869 3870 protected Encounter typedCopy() { 3871 return copy(); 3872 } 3873 3874 @Override 3875 public boolean equalsDeep(Base other_) { 3876 if (!super.equalsDeep(other_)) 3877 return false; 3878 if (!(other_ instanceof Encounter)) 3879 return false; 3880 Encounter o = (Encounter) other_; 3881 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(class_, o.class_, true) 3882 && compareDeep(priority, o.priority, true) && compareDeep(type, o.type, true) && compareDeep(serviceType, o.serviceType, true) 3883 && compareDeep(subject, o.subject, true) && compareDeep(subjectStatus, o.subjectStatus, true) && compareDeep(episodeOfCare, o.episodeOfCare, true) 3884 && compareDeep(basedOn, o.basedOn, true) && compareDeep(careTeam, o.careTeam, true) && compareDeep(partOf, o.partOf, true) 3885 && compareDeep(serviceProvider, o.serviceProvider, true) && compareDeep(participant, o.participant, true) 3886 && compareDeep(appointment, o.appointment, true) && compareDeep(virtualService, o.virtualService, true) 3887 && compareDeep(actualPeriod, o.actualPeriod, true) && compareDeep(plannedStartDate, o.plannedStartDate, true) 3888 && compareDeep(plannedEndDate, o.plannedEndDate, true) && compareDeep(length, o.length, true) && compareDeep(reason, o.reason, true) 3889 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(account, o.account, true) && compareDeep(dietPreference, o.dietPreference, true) 3890 && compareDeep(specialArrangement, o.specialArrangement, true) && compareDeep(specialCourtesy, o.specialCourtesy, true) 3891 && compareDeep(admission, o.admission, true) && compareDeep(location, o.location, true); 3892 } 3893 3894 @Override 3895 public boolean equalsShallow(Base other_) { 3896 if (!super.equalsShallow(other_)) 3897 return false; 3898 if (!(other_ instanceof Encounter)) 3899 return false; 3900 Encounter o = (Encounter) other_; 3901 return compareValues(status, o.status, true) && compareValues(plannedStartDate, o.plannedStartDate, true) 3902 && compareValues(plannedEndDate, o.plannedEndDate, true); 3903 } 3904 3905 public boolean isEmpty() { 3906 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, class_ 3907 , priority, type, serviceType, subject, subjectStatus, episodeOfCare, basedOn 3908 , careTeam, partOf, serviceProvider, participant, appointment, virtualService, actualPeriod 3909 , plannedStartDate, plannedEndDate, length, reason, diagnosis, account, dietPreference 3910 , specialArrangement, specialCourtesy, admission, location); 3911 } 3912 3913 @Override 3914 public ResourceType getResourceType() { 3915 return ResourceType.Encounter; 3916 } 3917 3918 /** 3919 * Search parameter: <b>account</b> 3920 * <p> 3921 * Description: <b>The set of accounts that may be used for billing for this Encounter</b><br> 3922 * Type: <b>reference</b><br> 3923 * Path: <b>Encounter.account</b><br> 3924 * </p> 3925 */ 3926 @SearchParamDefinition(name="account", path="Encounter.account", description="The set of accounts that may be used for billing for this Encounter", type="reference", target={Account.class } ) 3927 public static final String SP_ACCOUNT = "account"; 3928 /** 3929 * <b>Fluent Client</b> search parameter constant for <b>account</b> 3930 * <p> 3931 * Description: <b>The set of accounts that may be used for billing for this Encounter</b><br> 3932 * Type: <b>reference</b><br> 3933 * Path: <b>Encounter.account</b><br> 3934 * </p> 3935 */ 3936 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACCOUNT); 3937 3938/** 3939 * Constant for fluent queries to be used to add include statements. Specifies 3940 * the path value of "<b>Encounter:account</b>". 3941 */ 3942 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include("Encounter:account").toLocked(); 3943 3944 /** 3945 * Search parameter: <b>appointment</b> 3946 * <p> 3947 * Description: <b>The appointment that scheduled this encounter</b><br> 3948 * Type: <b>reference</b><br> 3949 * Path: <b>Encounter.appointment</b><br> 3950 * </p> 3951 */ 3952 @SearchParamDefinition(name="appointment", path="Encounter.appointment", description="The appointment that scheduled this encounter", type="reference", target={Appointment.class } ) 3953 public static final String SP_APPOINTMENT = "appointment"; 3954 /** 3955 * <b>Fluent Client</b> search parameter constant for <b>appointment</b> 3956 * <p> 3957 * Description: <b>The appointment that scheduled this encounter</b><br> 3958 * Type: <b>reference</b><br> 3959 * Path: <b>Encounter.appointment</b><br> 3960 * </p> 3961 */ 3962 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam APPOINTMENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_APPOINTMENT); 3963 3964/** 3965 * Constant for fluent queries to be used to add include statements. Specifies 3966 * the path value of "<b>Encounter:appointment</b>". 3967 */ 3968 public static final ca.uhn.fhir.model.api.Include INCLUDE_APPOINTMENT = new ca.uhn.fhir.model.api.Include("Encounter:appointment").toLocked(); 3969 3970 /** 3971 * Search parameter: <b>based-on</b> 3972 * <p> 3973 * Description: <b>The ServiceRequest that initiated this encounter</b><br> 3974 * Type: <b>reference</b><br> 3975 * Path: <b>Encounter.basedOn</b><br> 3976 * </p> 3977 */ 3978 @SearchParamDefinition(name="based-on", path="Encounter.basedOn", description="The ServiceRequest that initiated this encounter", type="reference", target={CarePlan.class, DeviceRequest.class, MedicationRequest.class, ServiceRequest.class } ) 3979 public static final String SP_BASED_ON = "based-on"; 3980 /** 3981 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3982 * <p> 3983 * Description: <b>The ServiceRequest that initiated this encounter</b><br> 3984 * Type: <b>reference</b><br> 3985 * Path: <b>Encounter.basedOn</b><br> 3986 * </p> 3987 */ 3988 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3989 3990/** 3991 * Constant for fluent queries to be used to add include statements. Specifies 3992 * the path value of "<b>Encounter:based-on</b>". 3993 */ 3994 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Encounter:based-on").toLocked(); 3995 3996 /** 3997 * Search parameter: <b>careteam</b> 3998 * <p> 3999 * Description: <b>Careteam allocated to participate in the encounter</b><br> 4000 * Type: <b>reference</b><br> 4001 * Path: <b>Encounter.careTeam</b><br> 4002 * </p> 4003 */ 4004 @SearchParamDefinition(name="careteam", path="Encounter.careTeam", description="Careteam allocated to participate in the encounter", type="reference", target={CareTeam.class } ) 4005 public static final String SP_CARETEAM = "careteam"; 4006 /** 4007 * <b>Fluent Client</b> search parameter constant for <b>careteam</b> 4008 * <p> 4009 * Description: <b>Careteam allocated to participate in the encounter</b><br> 4010 * Type: <b>reference</b><br> 4011 * Path: <b>Encounter.careTeam</b><br> 4012 * </p> 4013 */ 4014 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARETEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARETEAM); 4015 4016/** 4017 * Constant for fluent queries to be used to add include statements. Specifies 4018 * the path value of "<b>Encounter:careteam</b>". 4019 */ 4020 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARETEAM = new ca.uhn.fhir.model.api.Include("Encounter:careteam").toLocked(); 4021 4022 /** 4023 * Search parameter: <b>class</b> 4024 * <p> 4025 * Description: <b>Classification of patient encounter</b><br> 4026 * Type: <b>token</b><br> 4027 * Path: <b>Encounter.class</b><br> 4028 * </p> 4029 */ 4030 @SearchParamDefinition(name="class", path="Encounter.class", description="Classification of patient encounter", type="token" ) 4031 public static final String SP_CLASS = "class"; 4032 /** 4033 * <b>Fluent Client</b> search parameter constant for <b>class</b> 4034 * <p> 4035 * Description: <b>Classification of patient encounter</b><br> 4036 * Type: <b>token</b><br> 4037 * Path: <b>Encounter.class</b><br> 4038 * </p> 4039 */ 4040 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASS); 4041 4042 /** 4043 * Search parameter: <b>date-start</b> 4044 * <p> 4045 * Description: <b>The actual start date of the Encounter</b><br> 4046 * Type: <b>date</b><br> 4047 * Path: <b>Encounter.actualPeriod.start</b><br> 4048 * </p> 4049 */ 4050 @SearchParamDefinition(name="date-start", path="Encounter.actualPeriod.start", description="The actual start date of the Encounter", type="date" ) 4051 public static final String SP_DATE_START = "date-start"; 4052 /** 4053 * <b>Fluent Client</b> search parameter constant for <b>date-start</b> 4054 * <p> 4055 * Description: <b>The actual start date of the Encounter</b><br> 4056 * Type: <b>date</b><br> 4057 * Path: <b>Encounter.actualPeriod.start</b><br> 4058 * </p> 4059 */ 4060 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE_START = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE_START); 4061 4062 /** 4063 * Search parameter: <b>diagnosis-code</b> 4064 * <p> 4065 * Description: <b>The diagnosis or procedure relevant to the encounter (coded)</b><br> 4066 * Type: <b>token</b><br> 4067 * Path: <b>Encounter.diagnosis.condition.concept</b><br> 4068 * </p> 4069 */ 4070 @SearchParamDefinition(name="diagnosis-code", path="Encounter.diagnosis.condition.concept", description="The diagnosis or procedure relevant to the encounter (coded)", type="token" ) 4071 public static final String SP_DIAGNOSIS_CODE = "diagnosis-code"; 4072 /** 4073 * <b>Fluent Client</b> search parameter constant for <b>diagnosis-code</b> 4074 * <p> 4075 * Description: <b>The diagnosis or procedure relevant to the encounter (coded)</b><br> 4076 * Type: <b>token</b><br> 4077 * Path: <b>Encounter.diagnosis.condition.concept</b><br> 4078 * </p> 4079 */ 4080 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DIAGNOSIS_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DIAGNOSIS_CODE); 4081 4082 /** 4083 * Search parameter: <b>diagnosis-reference</b> 4084 * <p> 4085 * Description: <b>The diagnosis or procedure relevant to the encounter (resource reference)</b><br> 4086 * Type: <b>reference</b><br> 4087 * Path: <b>Encounter.diagnosis.condition.reference</b><br> 4088 * </p> 4089 */ 4090 @SearchParamDefinition(name="diagnosis-reference", path="Encounter.diagnosis.condition.reference", description="The diagnosis or procedure relevant to the encounter (resource reference)", type="reference", target={Condition.class } ) 4091 public static final String SP_DIAGNOSIS_REFERENCE = "diagnosis-reference"; 4092 /** 4093 * <b>Fluent Client</b> search parameter constant for <b>diagnosis-reference</b> 4094 * <p> 4095 * Description: <b>The diagnosis or procedure relevant to the encounter (resource reference)</b><br> 4096 * Type: <b>reference</b><br> 4097 * Path: <b>Encounter.diagnosis.condition.reference</b><br> 4098 * </p> 4099 */ 4100 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DIAGNOSIS_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DIAGNOSIS_REFERENCE); 4101 4102/** 4103 * Constant for fluent queries to be used to add include statements. Specifies 4104 * the path value of "<b>Encounter:diagnosis-reference</b>". 4105 */ 4106 public static final ca.uhn.fhir.model.api.Include INCLUDE_DIAGNOSIS_REFERENCE = new ca.uhn.fhir.model.api.Include("Encounter:diagnosis-reference").toLocked(); 4107 4108 /** 4109 * Search parameter: <b>end-date</b> 4110 * <p> 4111 * Description: <b>The actual end date of the Encounter</b><br> 4112 * Type: <b>date</b><br> 4113 * Path: <b>Encounter.actualPeriod.end</b><br> 4114 * </p> 4115 */ 4116 @SearchParamDefinition(name="end-date", path="Encounter.actualPeriod.end", description="The actual end date of the Encounter", type="date" ) 4117 public static final String SP_END_DATE = "end-date"; 4118 /** 4119 * <b>Fluent Client</b> search parameter constant for <b>end-date</b> 4120 * <p> 4121 * Description: <b>The actual end date of the Encounter</b><br> 4122 * Type: <b>date</b><br> 4123 * Path: <b>Encounter.actualPeriod.end</b><br> 4124 * </p> 4125 */ 4126 public static final ca.uhn.fhir.rest.gclient.DateClientParam END_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_END_DATE); 4127 4128 /** 4129 * Search parameter: <b>episode-of-care</b> 4130 * <p> 4131 * Description: <b>Episode(s) of care that this encounter should be recorded against</b><br> 4132 * Type: <b>reference</b><br> 4133 * Path: <b>Encounter.episodeOfCare</b><br> 4134 * </p> 4135 */ 4136 @SearchParamDefinition(name="episode-of-care", path="Encounter.episodeOfCare", description="Episode(s) of care that this encounter should be recorded against", type="reference", target={EpisodeOfCare.class } ) 4137 public static final String SP_EPISODE_OF_CARE = "episode-of-care"; 4138 /** 4139 * <b>Fluent Client</b> search parameter constant for <b>episode-of-care</b> 4140 * <p> 4141 * Description: <b>Episode(s) of care that this encounter should be recorded against</b><br> 4142 * Type: <b>reference</b><br> 4143 * Path: <b>Encounter.episodeOfCare</b><br> 4144 * </p> 4145 */ 4146 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EPISODE_OF_CARE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EPISODE_OF_CARE); 4147 4148/** 4149 * Constant for fluent queries to be used to add include statements. Specifies 4150 * the path value of "<b>Encounter:episode-of-care</b>". 4151 */ 4152 public static final ca.uhn.fhir.model.api.Include INCLUDE_EPISODE_OF_CARE = new ca.uhn.fhir.model.api.Include("Encounter:episode-of-care").toLocked(); 4153 4154 /** 4155 * Search parameter: <b>length</b> 4156 * <p> 4157 * Description: <b>Length of encounter in days</b><br> 4158 * Type: <b>quantity</b><br> 4159 * Path: <b>Encounter.length</b><br> 4160 * </p> 4161 */ 4162 @SearchParamDefinition(name="length", path="Encounter.length", description="Length of encounter in days", type="quantity" ) 4163 public static final String SP_LENGTH = "length"; 4164 /** 4165 * <b>Fluent Client</b> search parameter constant for <b>length</b> 4166 * <p> 4167 * Description: <b>Length of encounter in days</b><br> 4168 * Type: <b>quantity</b><br> 4169 * Path: <b>Encounter.length</b><br> 4170 * </p> 4171 */ 4172 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam LENGTH = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_LENGTH); 4173 4174 /** 4175 * Search parameter: <b>location</b> 4176 * <p> 4177 * Description: <b>Location the encounter takes place</b><br> 4178 * Type: <b>reference</b><br> 4179 * Path: <b>Encounter.location.location</b><br> 4180 * </p> 4181 */ 4182 @SearchParamDefinition(name="location", path="Encounter.location.location", description="Location the encounter takes place", type="reference", target={Location.class } ) 4183 public static final String SP_LOCATION = "location"; 4184 /** 4185 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4186 * <p> 4187 * Description: <b>Location the encounter takes place</b><br> 4188 * Type: <b>reference</b><br> 4189 * Path: <b>Encounter.location.location</b><br> 4190 * </p> 4191 */ 4192 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 4193 4194/** 4195 * Constant for fluent queries to be used to add include statements. Specifies 4196 * the path value of "<b>Encounter:location</b>". 4197 */ 4198 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Encounter:location").toLocked(); 4199 4200 /** 4201 * Search parameter: <b>part-of</b> 4202 * <p> 4203 * Description: <b>Another Encounter this encounter is part of</b><br> 4204 * Type: <b>reference</b><br> 4205 * Path: <b>Encounter.partOf</b><br> 4206 * </p> 4207 */ 4208 @SearchParamDefinition(name="part-of", path="Encounter.partOf", description="Another Encounter this encounter is part of", type="reference", target={Encounter.class } ) 4209 public static final String SP_PART_OF = "part-of"; 4210 /** 4211 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4212 * <p> 4213 * Description: <b>Another Encounter this encounter is part of</b><br> 4214 * Type: <b>reference</b><br> 4215 * Path: <b>Encounter.partOf</b><br> 4216 * </p> 4217 */ 4218 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 4219 4220/** 4221 * Constant for fluent queries to be used to add include statements. Specifies 4222 * the path value of "<b>Encounter:part-of</b>". 4223 */ 4224 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Encounter:part-of").toLocked(); 4225 4226 /** 4227 * Search parameter: <b>participant-type</b> 4228 * <p> 4229 * Description: <b>Role of participant in encounter</b><br> 4230 * Type: <b>token</b><br> 4231 * Path: <b>Encounter.participant.type</b><br> 4232 * </p> 4233 */ 4234 @SearchParamDefinition(name="participant-type", path="Encounter.participant.type", description="Role of participant in encounter", type="token" ) 4235 public static final String SP_PARTICIPANT_TYPE = "participant-type"; 4236 /** 4237 * <b>Fluent Client</b> search parameter constant for <b>participant-type</b> 4238 * <p> 4239 * Description: <b>Role of participant in encounter</b><br> 4240 * Type: <b>token</b><br> 4241 * Path: <b>Encounter.participant.type</b><br> 4242 * </p> 4243 */ 4244 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PARTICIPANT_TYPE); 4245 4246 /** 4247 * Search parameter: <b>participant</b> 4248 * <p> 4249 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4250 * Type: <b>reference</b><br> 4251 * Path: <b>Encounter.participant.actor</b><br> 4252 * </p> 4253 */ 4254 @SearchParamDefinition(name="participant", path="Encounter.participant.actor", description="Persons involved in the encounter other than the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Device.class, Group.class, HealthcareService.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 4255 public static final String SP_PARTICIPANT = "participant"; 4256 /** 4257 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 4258 * <p> 4259 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4260 * Type: <b>reference</b><br> 4261 * Path: <b>Encounter.participant.actor</b><br> 4262 * </p> 4263 */ 4264 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 4265 4266/** 4267 * Constant for fluent queries to be used to add include statements. Specifies 4268 * the path value of "<b>Encounter:participant</b>". 4269 */ 4270 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("Encounter:participant").toLocked(); 4271 4272 /** 4273 * Search parameter: <b>practitioner</b> 4274 * <p> 4275 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4276 * Type: <b>reference</b><br> 4277 * Path: <b>Encounter.participant.actor.where(resolve() is Practitioner)</b><br> 4278 * </p> 4279 */ 4280 @SearchParamDefinition(name="practitioner", path="Encounter.participant.actor.where(resolve() is Practitioner)", description="Persons involved in the encounter other than the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class } ) 4281 public static final String SP_PRACTITIONER = "practitioner"; 4282 /** 4283 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 4284 * <p> 4285 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4286 * Type: <b>reference</b><br> 4287 * Path: <b>Encounter.participant.actor.where(resolve() is Practitioner)</b><br> 4288 * </p> 4289 */ 4290 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 4291 4292/** 4293 * Constant for fluent queries to be used to add include statements. Specifies 4294 * the path value of "<b>Encounter:practitioner</b>". 4295 */ 4296 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Encounter:practitioner").toLocked(); 4297 4298 /** 4299 * Search parameter: <b>reason-code</b> 4300 * <p> 4301 * Description: <b>Reference to a concept (coded)</b><br> 4302 * Type: <b>token</b><br> 4303 * Path: <b>Encounter.reason.value.concept</b><br> 4304 * </p> 4305 */ 4306 @SearchParamDefinition(name="reason-code", path="Encounter.reason.value.concept", description="Reference to a concept (coded)", type="token" ) 4307 public static final String SP_REASON_CODE = "reason-code"; 4308 /** 4309 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 4310 * <p> 4311 * Description: <b>Reference to a concept (coded)</b><br> 4312 * Type: <b>token</b><br> 4313 * Path: <b>Encounter.reason.value.concept</b><br> 4314 * </p> 4315 */ 4316 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_CODE); 4317 4318 /** 4319 * Search parameter: <b>reason-reference</b> 4320 * <p> 4321 * Description: <b>Reference to a resource (resource reference)</b><br> 4322 * Type: <b>reference</b><br> 4323 * Path: <b>Encounter.reason.value.reference</b><br> 4324 * </p> 4325 */ 4326 @SearchParamDefinition(name="reason-reference", path="Encounter.reason.value.reference", description="Reference to a resource (resource reference)", type="reference", target={Condition.class, DiagnosticReport.class, ImmunizationRecommendation.class, Observation.class, Procedure.class } ) 4327 public static final String SP_REASON_REFERENCE = "reason-reference"; 4328 /** 4329 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 4330 * <p> 4331 * Description: <b>Reference to a resource (resource reference)</b><br> 4332 * Type: <b>reference</b><br> 4333 * Path: <b>Encounter.reason.value.reference</b><br> 4334 * </p> 4335 */ 4336 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REASON_REFERENCE); 4337 4338/** 4339 * Constant for fluent queries to be used to add include statements. Specifies 4340 * the path value of "<b>Encounter:reason-reference</b>". 4341 */ 4342 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include("Encounter:reason-reference").toLocked(); 4343 4344 /** 4345 * Search parameter: <b>service-provider</b> 4346 * <p> 4347 * Description: <b>The organization (facility) responsible for this encounter</b><br> 4348 * Type: <b>reference</b><br> 4349 * Path: <b>Encounter.serviceProvider</b><br> 4350 * </p> 4351 */ 4352 @SearchParamDefinition(name="service-provider", path="Encounter.serviceProvider", description="The organization (facility) responsible for this encounter", type="reference", target={Organization.class } ) 4353 public static final String SP_SERVICE_PROVIDER = "service-provider"; 4354 /** 4355 * <b>Fluent Client</b> search parameter constant for <b>service-provider</b> 4356 * <p> 4357 * Description: <b>The organization (facility) responsible for this encounter</b><br> 4358 * Type: <b>reference</b><br> 4359 * Path: <b>Encounter.serviceProvider</b><br> 4360 * </p> 4361 */ 4362 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE_PROVIDER); 4363 4364/** 4365 * Constant for fluent queries to be used to add include statements. Specifies 4366 * the path value of "<b>Encounter:service-provider</b>". 4367 */ 4368 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE_PROVIDER = new ca.uhn.fhir.model.api.Include("Encounter:service-provider").toLocked(); 4369 4370 /** 4371 * Search parameter: <b>special-arrangement</b> 4372 * <p> 4373 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 4374 * Type: <b>token</b><br> 4375 * Path: <b>Encounter.specialArrangement</b><br> 4376 * </p> 4377 */ 4378 @SearchParamDefinition(name="special-arrangement", path="Encounter.specialArrangement", description="Wheelchair, translator, stretcher, etc.", type="token" ) 4379 public static final String SP_SPECIAL_ARRANGEMENT = "special-arrangement"; 4380 /** 4381 * <b>Fluent Client</b> search parameter constant for <b>special-arrangement</b> 4382 * <p> 4383 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 4384 * Type: <b>token</b><br> 4385 * Path: <b>Encounter.specialArrangement</b><br> 4386 * </p> 4387 */ 4388 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIAL_ARRANGEMENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIAL_ARRANGEMENT); 4389 4390 /** 4391 * Search parameter: <b>status</b> 4392 * <p> 4393 * Description: <b>planned | in-progress | on-hold | completed | cancelled | entered-in-error | unknown</b><br> 4394 * Type: <b>token</b><br> 4395 * Path: <b>Encounter.status</b><br> 4396 * </p> 4397 */ 4398 @SearchParamDefinition(name="status", path="Encounter.status", description="planned | in-progress | on-hold | completed | cancelled | entered-in-error | unknown", type="token" ) 4399 public static final String SP_STATUS = "status"; 4400 /** 4401 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4402 * <p> 4403 * Description: <b>planned | in-progress | on-hold | completed | cancelled | entered-in-error | unknown</b><br> 4404 * Type: <b>token</b><br> 4405 * Path: <b>Encounter.status</b><br> 4406 * </p> 4407 */ 4408 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4409 4410 /** 4411 * Search parameter: <b>subject-status</b> 4412 * <p> 4413 * Description: <b>The current status of the subject in relation to the Encounter</b><br> 4414 * Type: <b>token</b><br> 4415 * Path: <b>Encounter.subjectStatus</b><br> 4416 * </p> 4417 */ 4418 @SearchParamDefinition(name="subject-status", path="Encounter.subjectStatus", description="The current status of the subject in relation to the Encounter", type="token" ) 4419 public static final String SP_SUBJECT_STATUS = "subject-status"; 4420 /** 4421 * <b>Fluent Client</b> search parameter constant for <b>subject-status</b> 4422 * <p> 4423 * Description: <b>The current status of the subject in relation to the Encounter</b><br> 4424 * Type: <b>token</b><br> 4425 * Path: <b>Encounter.subjectStatus</b><br> 4426 * </p> 4427 */ 4428 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBJECT_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUBJECT_STATUS); 4429 4430 /** 4431 * Search parameter: <b>subject</b> 4432 * <p> 4433 * Description: <b>The patient or group present at the encounter</b><br> 4434 * Type: <b>reference</b><br> 4435 * Path: <b>Encounter.subject</b><br> 4436 * </p> 4437 */ 4438 @SearchParamDefinition(name="subject", path="Encounter.subject", description="The patient or group present at the encounter", type="reference", target={Group.class, Patient.class } ) 4439 public static final String SP_SUBJECT = "subject"; 4440 /** 4441 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4442 * <p> 4443 * Description: <b>The patient or group present at the encounter</b><br> 4444 * Type: <b>reference</b><br> 4445 * Path: <b>Encounter.subject</b><br> 4446 * </p> 4447 */ 4448 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4449 4450/** 4451 * Constant for fluent queries to be used to add include statements. Specifies 4452 * the path value of "<b>Encounter:subject</b>". 4453 */ 4454 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Encounter:subject").toLocked(); 4455 4456 /** 4457 * Search parameter: <b>date</b> 4458 * <p> 4459 * Description: <b>Multiple Resources: 4460 4461* [AdverseEvent](adverseevent.html): When the event occurred 4462* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4463* [Appointment](appointment.html): Appointment date/time. 4464* [AuditEvent](auditevent.html): Time when the event was recorded 4465* [CarePlan](careplan.html): Time period plan covers 4466* [CareTeam](careteam.html): A date within the coverage time period. 4467* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4468* [Composition](composition.html): Composition editing time 4469* [Consent](consent.html): When consent was agreed to 4470* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4471* [DocumentReference](documentreference.html): When this document reference was created 4472* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4473* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4474* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4475* [Flag](flag.html): Time period when flag is active 4476* [Immunization](immunization.html): Vaccination (non)-Administration Date 4477* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4478* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4479* [Invoice](invoice.html): Invoice date / posting date 4480* [List](list.html): When the list was prepared 4481* [MeasureReport](measurereport.html): The date of the measure report 4482* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4483* [Observation](observation.html): Clinically relevant time/time-period for observation 4484* [Procedure](procedure.html): When the procedure occurred or is occurring 4485* [ResearchSubject](researchsubject.html): Start and end of participation 4486* [RiskAssessment](riskassessment.html): When was assessment made? 4487* [SupplyRequest](supplyrequest.html): When the request was made 4488</b><br> 4489 * Type: <b>date</b><br> 4490 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4491 * </p> 4492 */ 4493 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4494 public static final String SP_DATE = "date"; 4495 /** 4496 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4497 * <p> 4498 * Description: <b>Multiple Resources: 4499 4500* [AdverseEvent](adverseevent.html): When the event occurred 4501* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4502* [Appointment](appointment.html): Appointment date/time. 4503* [AuditEvent](auditevent.html): Time when the event was recorded 4504* [CarePlan](careplan.html): Time period plan covers 4505* [CareTeam](careteam.html): A date within the coverage time period. 4506* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4507* [Composition](composition.html): Composition editing time 4508* [Consent](consent.html): When consent was agreed to 4509* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4510* [DocumentReference](documentreference.html): When this document reference was created 4511* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4512* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4513* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4514* [Flag](flag.html): Time period when flag is active 4515* [Immunization](immunization.html): Vaccination (non)-Administration Date 4516* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4517* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4518* [Invoice](invoice.html): Invoice date / posting date 4519* [List](list.html): When the list was prepared 4520* [MeasureReport](measurereport.html): The date of the measure report 4521* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4522* [Observation](observation.html): Clinically relevant time/time-period for observation 4523* [Procedure](procedure.html): When the procedure occurred or is occurring 4524* [ResearchSubject](researchsubject.html): Start and end of participation 4525* [RiskAssessment](riskassessment.html): When was assessment made? 4526* [SupplyRequest](supplyrequest.html): When the request was made 4527</b><br> 4528 * Type: <b>date</b><br> 4529 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4530 * </p> 4531 */ 4532 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4533 4534 /** 4535 * Search parameter: <b>identifier</b> 4536 * <p> 4537 * Description: <b>Multiple Resources: 4538 4539* [Account](account.html): Account number 4540* [AdverseEvent](adverseevent.html): Business identifier for the event 4541* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4542* [Appointment](appointment.html): An Identifier of the Appointment 4543* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4544* [Basic](basic.html): Business identifier 4545* [BodyStructure](bodystructure.html): Bodystructure identifier 4546* [CarePlan](careplan.html): External Ids for this plan 4547* [CareTeam](careteam.html): External Ids for this team 4548* [ChargeItem](chargeitem.html): Business Identifier for item 4549* [Claim](claim.html): The primary identifier of the financial resource 4550* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4551* [ClinicalImpression](clinicalimpression.html): Business identifier 4552* [Communication](communication.html): Unique identifier 4553* [CommunicationRequest](communicationrequest.html): Unique identifier 4554* [Composition](composition.html): Version-independent identifier for the Composition 4555* [Condition](condition.html): A unique identifier of the condition record 4556* [Consent](consent.html): Identifier for this record (external references) 4557* [Contract](contract.html): The identity of the contract 4558* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4559* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4560* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4561* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4562* [DeviceRequest](devicerequest.html): Business identifier for request/order 4563* [DeviceUsage](deviceusage.html): Search by identifier 4564* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4565* [DocumentReference](documentreference.html): Identifier of the attachment binary 4566* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4567* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4568* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4569* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4570* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4571* [Flag](flag.html): Business identifier 4572* [Goal](goal.html): External Ids for this goal 4573* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4574* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4575* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4576* [Immunization](immunization.html): Business identifier 4577* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4578* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4579* [Invoice](invoice.html): Business Identifier for item 4580* [List](list.html): Business identifier 4581* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4582* [Medication](medication.html): Returns medications with this external identifier 4583* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4584* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4585* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4586* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4587* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4588* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4589* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4590* [Observation](observation.html): The unique id for a particular observation 4591* [Person](person.html): A person Identifier 4592* [Procedure](procedure.html): A unique identifier for a procedure 4593* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4594* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4595* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4596* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4597* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4598* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4599* [Specimen](specimen.html): The unique identifier associated with the specimen 4600* [SupplyDelivery](supplydelivery.html): External identifier 4601* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4602* [Task](task.html): Search for a task instance by its business identifier 4603* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4604</b><br> 4605 * Type: <b>token</b><br> 4606 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4607 * </p> 4608 */ 4609 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4610 public static final String SP_IDENTIFIER = "identifier"; 4611 /** 4612 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4613 * <p> 4614 * Description: <b>Multiple Resources: 4615 4616* [Account](account.html): Account number 4617* [AdverseEvent](adverseevent.html): Business identifier for the event 4618* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4619* [Appointment](appointment.html): An Identifier of the Appointment 4620* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4621* [Basic](basic.html): Business identifier 4622* [BodyStructure](bodystructure.html): Bodystructure identifier 4623* [CarePlan](careplan.html): External Ids for this plan 4624* [CareTeam](careteam.html): External Ids for this team 4625* [ChargeItem](chargeitem.html): Business Identifier for item 4626* [Claim](claim.html): The primary identifier of the financial resource 4627* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4628* [ClinicalImpression](clinicalimpression.html): Business identifier 4629* [Communication](communication.html): Unique identifier 4630* [CommunicationRequest](communicationrequest.html): Unique identifier 4631* [Composition](composition.html): Version-independent identifier for the Composition 4632* [Condition](condition.html): A unique identifier of the condition record 4633* [Consent](consent.html): Identifier for this record (external references) 4634* [Contract](contract.html): The identity of the contract 4635* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4636* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4637* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4638* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4639* [DeviceRequest](devicerequest.html): Business identifier for request/order 4640* [DeviceUsage](deviceusage.html): Search by identifier 4641* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4642* [DocumentReference](documentreference.html): Identifier of the attachment binary 4643* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4644* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4645* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4646* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4647* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4648* [Flag](flag.html): Business identifier 4649* [Goal](goal.html): External Ids for this goal 4650* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4651* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4652* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4653* [Immunization](immunization.html): Business identifier 4654* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4655* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4656* [Invoice](invoice.html): Business Identifier for item 4657* [List](list.html): Business identifier 4658* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4659* [Medication](medication.html): Returns medications with this external identifier 4660* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4661* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4662* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4663* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4664* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4665* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4666* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4667* [Observation](observation.html): The unique id for a particular observation 4668* [Person](person.html): A person Identifier 4669* [Procedure](procedure.html): A unique identifier for a procedure 4670* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4671* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4672* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4673* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4674* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4675* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4676* [Specimen](specimen.html): The unique identifier associated with the specimen 4677* [SupplyDelivery](supplydelivery.html): External identifier 4678* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4679* [Task](task.html): Search for a task instance by its business identifier 4680* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4681</b><br> 4682 * Type: <b>token</b><br> 4683 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4684 * </p> 4685 */ 4686 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4687 4688 /** 4689 * Search parameter: <b>patient</b> 4690 * <p> 4691 * Description: <b>Multiple Resources: 4692 4693* [Account](account.html): The entity that caused the expenses 4694* [AdverseEvent](adverseevent.html): Subject impacted by event 4695* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4696* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4697* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4698* [AuditEvent](auditevent.html): Where the activity involved patient data 4699* [Basic](basic.html): Identifies the focus of this resource 4700* [BodyStructure](bodystructure.html): Who this is about 4701* [CarePlan](careplan.html): Who the care plan is for 4702* [CareTeam](careteam.html): Who care team is for 4703* [ChargeItem](chargeitem.html): Individual service was done for/to 4704* [Claim](claim.html): Patient receiving the products or services 4705* [ClaimResponse](claimresponse.html): The subject of care 4706* [ClinicalImpression](clinicalimpression.html): Patient assessed 4707* [Communication](communication.html): Focus of message 4708* [CommunicationRequest](communicationrequest.html): Focus of message 4709* [Composition](composition.html): Who and/or what the composition is about 4710* [Condition](condition.html): Who has the condition? 4711* [Consent](consent.html): Who the consent applies to 4712* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4713* [Coverage](coverage.html): Retrieve coverages for a patient 4714* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4715* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4716* [DetectedIssue](detectedissue.html): Associated patient 4717* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4718* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4719* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4720* [DocumentReference](documentreference.html): Who/what is the subject of the document 4721* [Encounter](encounter.html): The patient present at the encounter 4722* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4723* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4724* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4725* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4726* [Flag](flag.html): The identity of a subject to list flags for 4727* [Goal](goal.html): Who this goal is intended for 4728* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4729* [ImagingSelection](imagingselection.html): Who the study is about 4730* [ImagingStudy](imagingstudy.html): Who the study is about 4731* [Immunization](immunization.html): The patient for the vaccination record 4732* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4733* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4734* [Invoice](invoice.html): Recipient(s) of goods and services 4735* [List](list.html): If all resources have the same subject 4736* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4737* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4738* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4739* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4740* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4741* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4742* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4743* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4744* [Observation](observation.html): The subject that the observation is about (if patient) 4745* [Person](person.html): The Person links to this Patient 4746* [Procedure](procedure.html): Search by subject - a patient 4747* [Provenance](provenance.html): Where the activity involved patient data 4748* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4749* [RelatedPerson](relatedperson.html): The patient this related person is related to 4750* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4751* [ResearchSubject](researchsubject.html): Who or what is part of study 4752* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4753* [ServiceRequest](servicerequest.html): Search by subject - a patient 4754* [Specimen](specimen.html): The patient the specimen comes from 4755* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4756* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4757* [Task](task.html): Search by patient 4758* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4759</b><br> 4760 * Type: <b>reference</b><br> 4761 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4762 * </p> 4763 */ 4764 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 4765 public static final String SP_PATIENT = "patient"; 4766 /** 4767 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4768 * <p> 4769 * Description: <b>Multiple Resources: 4770 4771* [Account](account.html): The entity that caused the expenses 4772* [AdverseEvent](adverseevent.html): Subject impacted by event 4773* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4774* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4775* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4776* [AuditEvent](auditevent.html): Where the activity involved patient data 4777* [Basic](basic.html): Identifies the focus of this resource 4778* [BodyStructure](bodystructure.html): Who this is about 4779* [CarePlan](careplan.html): Who the care plan is for 4780* [CareTeam](careteam.html): Who care team is for 4781* [ChargeItem](chargeitem.html): Individual service was done for/to 4782* [Claim](claim.html): Patient receiving the products or services 4783* [ClaimResponse](claimresponse.html): The subject of care 4784* [ClinicalImpression](clinicalimpression.html): Patient assessed 4785* [Communication](communication.html): Focus of message 4786* [CommunicationRequest](communicationrequest.html): Focus of message 4787* [Composition](composition.html): Who and/or what the composition is about 4788* [Condition](condition.html): Who has the condition? 4789* [Consent](consent.html): Who the consent applies to 4790* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4791* [Coverage](coverage.html): Retrieve coverages for a patient 4792* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4793* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4794* [DetectedIssue](detectedissue.html): Associated patient 4795* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4796* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4797* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4798* [DocumentReference](documentreference.html): Who/what is the subject of the document 4799* [Encounter](encounter.html): The patient present at the encounter 4800* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4801* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4802* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4803* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4804* [Flag](flag.html): The identity of a subject to list flags for 4805* [Goal](goal.html): Who this goal is intended for 4806* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4807* [ImagingSelection](imagingselection.html): Who the study is about 4808* [ImagingStudy](imagingstudy.html): Who the study is about 4809* [Immunization](immunization.html): The patient for the vaccination record 4810* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4811* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4812* [Invoice](invoice.html): Recipient(s) of goods and services 4813* [List](list.html): If all resources have the same subject 4814* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4815* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4816* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4817* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4818* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4819* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4820* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4821* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4822* [Observation](observation.html): The subject that the observation is about (if patient) 4823* [Person](person.html): The Person links to this Patient 4824* [Procedure](procedure.html): Search by subject - a patient 4825* [Provenance](provenance.html): Where the activity involved patient data 4826* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4827* [RelatedPerson](relatedperson.html): The patient this related person is related to 4828* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4829* [ResearchSubject](researchsubject.html): Who or what is part of study 4830* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4831* [ServiceRequest](servicerequest.html): Search by subject - a patient 4832* [Specimen](specimen.html): The patient the specimen comes from 4833* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4834* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4835* [Task](task.html): Search by patient 4836* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4837</b><br> 4838 * Type: <b>reference</b><br> 4839 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4840 * </p> 4841 */ 4842 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4843 4844/** 4845 * Constant for fluent queries to be used to add include statements. Specifies 4846 * the path value of "<b>Encounter:patient</b>". 4847 */ 4848 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Encounter:patient").toLocked(); 4849 4850 /** 4851 * Search parameter: <b>type</b> 4852 * <p> 4853 * Description: <b>Multiple Resources: 4854 4855* [Account](account.html): E.g. patient, expense, depreciation 4856* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 4857* [Composition](composition.html): Kind of composition (LOINC if possible) 4858* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 4859* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 4860* [Encounter](encounter.html): Specific type of encounter 4861* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 4862* [Invoice](invoice.html): Type of Invoice 4863* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 4864* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 4865* [Specimen](specimen.html): The specimen type 4866</b><br> 4867 * Type: <b>token</b><br> 4868 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 4869 * </p> 4870 */ 4871 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 4872 public static final String SP_TYPE = "type"; 4873 /** 4874 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4875 * <p> 4876 * Description: <b>Multiple Resources: 4877 4878* [Account](account.html): E.g. patient, expense, depreciation 4879* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 4880* [Composition](composition.html): Kind of composition (LOINC if possible) 4881* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 4882* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 4883* [Encounter](encounter.html): Specific type of encounter 4884* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 4885* [Invoice](invoice.html): Type of Invoice 4886* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 4887* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 4888* [Specimen](specimen.html): The specimen type 4889</b><br> 4890 * Type: <b>token</b><br> 4891 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 4892 * </p> 4893 */ 4894 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4895 4896 4897} 4898