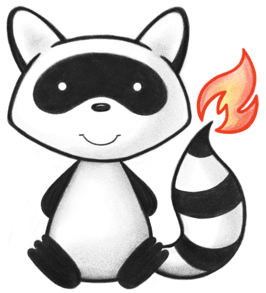
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of significant events/milestones key data throughout the history of an Encounter 052 */ 053@ResourceDef(name="EncounterHistory", profile="http://hl7.org/fhir/StructureDefinition/EncounterHistory") 054public class EncounterHistory extends DomainResource { 055 056 @Block() 057 public static class EncounterHistoryLocationComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The location where the encounter takes place. 060 */ 061 @Child(name = "location", type = {Location.class}, order=1, min=1, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Location the encounter takes place", formalDefinition="The location where the encounter takes place." ) 063 protected Reference location; 064 065 /** 066 * This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query. 067 */ 068 @Child(name = "form", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="The physical type of the location (usually the level in the location hierarchy - bed, room, ward, virtual etc.)", formalDefinition="This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query." ) 070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-form") 071 protected CodeableConcept form; 072 073 private static final long serialVersionUID = -1306742681L; 074 075 /** 076 * Constructor 077 */ 078 public EncounterHistoryLocationComponent() { 079 super(); 080 } 081 082 /** 083 * Constructor 084 */ 085 public EncounterHistoryLocationComponent(Reference location) { 086 super(); 087 this.setLocation(location); 088 } 089 090 /** 091 * @return {@link #location} (The location where the encounter takes place.) 092 */ 093 public Reference getLocation() { 094 if (this.location == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create EncounterHistoryLocationComponent.location"); 097 else if (Configuration.doAutoCreate()) 098 this.location = new Reference(); // cc 099 return this.location; 100 } 101 102 public boolean hasLocation() { 103 return this.location != null && !this.location.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #location} (The location where the encounter takes place.) 108 */ 109 public EncounterHistoryLocationComponent setLocation(Reference value) { 110 this.location = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #form} (This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.) 116 */ 117 public CodeableConcept getForm() { 118 if (this.form == null) 119 if (Configuration.errorOnAutoCreate()) 120 throw new Error("Attempt to auto-create EncounterHistoryLocationComponent.form"); 121 else if (Configuration.doAutoCreate()) 122 this.form = new CodeableConcept(); // cc 123 return this.form; 124 } 125 126 public boolean hasForm() { 127 return this.form != null && !this.form.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #form} (This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.) 132 */ 133 public EncounterHistoryLocationComponent setForm(CodeableConcept value) { 134 this.form = value; 135 return this; 136 } 137 138 protected void listChildren(List<Property> children) { 139 super.listChildren(children); 140 children.add(new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 1, location)); 141 children.add(new Property("form", "CodeableConcept", "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 0, 1, form)); 142 } 143 144 @Override 145 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 146 switch (_hash) { 147 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 1, location); 148 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 0, 1, form); 149 default: return super.getNamedProperty(_hash, _name, _checkValid); 150 } 151 152 } 153 154 @Override 155 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 156 switch (hash) { 157 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 158 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 159 default: return super.getProperty(hash, name, checkValid); 160 } 161 162 } 163 164 @Override 165 public Base setProperty(int hash, String name, Base value) throws FHIRException { 166 switch (hash) { 167 case 1901043637: // location 168 this.location = TypeConvertor.castToReference(value); // Reference 169 return value; 170 case 3148996: // form 171 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 172 return value; 173 default: return super.setProperty(hash, name, value); 174 } 175 176 } 177 178 @Override 179 public Base setProperty(String name, Base value) throws FHIRException { 180 if (name.equals("location")) { 181 this.location = TypeConvertor.castToReference(value); // Reference 182 } else if (name.equals("form")) { 183 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 184 } else 185 return super.setProperty(name, value); 186 return value; 187 } 188 189 @Override 190 public void removeChild(String name, Base value) throws FHIRException { 191 if (name.equals("location")) { 192 this.location = null; 193 } else if (name.equals("form")) { 194 this.form = null; 195 } else 196 super.removeChild(name, value); 197 198 } 199 200 @Override 201 public Base makeProperty(int hash, String name) throws FHIRException { 202 switch (hash) { 203 case 1901043637: return getLocation(); 204 case 3148996: return getForm(); 205 default: return super.makeProperty(hash, name); 206 } 207 208 } 209 210 @Override 211 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 212 switch (hash) { 213 case 1901043637: /*location*/ return new String[] {"Reference"}; 214 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 215 default: return super.getTypesForProperty(hash, name); 216 } 217 218 } 219 220 @Override 221 public Base addChild(String name) throws FHIRException { 222 if (name.equals("location")) { 223 this.location = new Reference(); 224 return this.location; 225 } 226 else if (name.equals("form")) { 227 this.form = new CodeableConcept(); 228 return this.form; 229 } 230 else 231 return super.addChild(name); 232 } 233 234 public EncounterHistoryLocationComponent copy() { 235 EncounterHistoryLocationComponent dst = new EncounterHistoryLocationComponent(); 236 copyValues(dst); 237 return dst; 238 } 239 240 public void copyValues(EncounterHistoryLocationComponent dst) { 241 super.copyValues(dst); 242 dst.location = location == null ? null : location.copy(); 243 dst.form = form == null ? null : form.copy(); 244 } 245 246 @Override 247 public boolean equalsDeep(Base other_) { 248 if (!super.equalsDeep(other_)) 249 return false; 250 if (!(other_ instanceof EncounterHistoryLocationComponent)) 251 return false; 252 EncounterHistoryLocationComponent o = (EncounterHistoryLocationComponent) other_; 253 return compareDeep(location, o.location, true) && compareDeep(form, o.form, true); 254 } 255 256 @Override 257 public boolean equalsShallow(Base other_) { 258 if (!super.equalsShallow(other_)) 259 return false; 260 if (!(other_ instanceof EncounterHistoryLocationComponent)) 261 return false; 262 EncounterHistoryLocationComponent o = (EncounterHistoryLocationComponent) other_; 263 return true; 264 } 265 266 public boolean isEmpty() { 267 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(location, form); 268 } 269 270 public String fhirType() { 271 return "EncounterHistory.location"; 272 273 } 274 275 } 276 277 /** 278 * The Encounter associated with this set of historic values. 279 */ 280 @Child(name = "encounter", type = {Encounter.class}, order=0, min=0, max=1, modifier=false, summary=false) 281 @Description(shortDefinition="The Encounter associated with this set of historic values", formalDefinition="The Encounter associated with this set of historic values." ) 282 protected Reference encounter; 283 284 /** 285 * Identifier(s) by which this encounter is known. 286 */ 287 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 288 @Description(shortDefinition="Identifier(s) by which this encounter is known", formalDefinition="Identifier(s) by which this encounter is known." ) 289 protected List<Identifier> identifier; 290 291 /** 292 * planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown. 293 */ 294 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 295 @Description(shortDefinition="planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown", formalDefinition="planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown." ) 296 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-status") 297 protected Enumeration<EncounterStatus> status; 298 299 /** 300 * Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations. 301 */ 302 @Child(name = "class", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 303 @Description(shortDefinition="Classification of patient encounter", formalDefinition="Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations." ) 304 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActEncounterCode") 305 protected CodeableConcept class_; 306 307 /** 308 * Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation). 309 */ 310 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 311 @Description(shortDefinition="Specific type of encounter", formalDefinition="Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation)." ) 312 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-type") 313 protected List<CodeableConcept> type; 314 315 /** 316 * Broad categorization of the service that is to be provided (e.g. cardiology). 317 */ 318 @Child(name = "serviceType", type = {CodeableReference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 319 @Description(shortDefinition="Specific type of service", formalDefinition="Broad categorization of the service that is to be provided (e.g. cardiology)." ) 320 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 321 protected List<CodeableReference> serviceType; 322 323 /** 324 * The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam. 325 */ 326 @Child(name = "subject", type = {Patient.class, Group.class}, order=6, min=0, max=1, modifier=false, summary=true) 327 @Description(shortDefinition="The patient or group related to this encounter", formalDefinition="The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam." ) 328 protected Reference subject; 329 330 /** 331 * The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status. 332 */ 333 @Child(name = "subjectStatus", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 334 @Description(shortDefinition="The current status of the subject in relation to the Encounter", formalDefinition="The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status." ) 335 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-subject-status") 336 protected CodeableConcept subjectStatus; 337 338 /** 339 * The start and end time associated with this set of values associated with the encounter, may be different to the planned times for various reasons. 340 */ 341 @Child(name = "actualPeriod", type = {Period.class}, order=8, min=0, max=1, modifier=false, summary=false) 342 @Description(shortDefinition="The actual start and end time associated with this set of values associated with the encounter", formalDefinition="The start and end time associated with this set of values associated with the encounter, may be different to the planned times for various reasons." ) 343 protected Period actualPeriod; 344 345 /** 346 * The planned start date/time (or admission date) of the encounter. 347 */ 348 @Child(name = "plannedStartDate", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 349 @Description(shortDefinition="The planned start date/time (or admission date) of the encounter", formalDefinition="The planned start date/time (or admission date) of the encounter." ) 350 protected DateTimeType plannedStartDate; 351 352 /** 353 * The planned end date/time (or discharge date) of the encounter. 354 */ 355 @Child(name = "plannedEndDate", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 356 @Description(shortDefinition="The planned end date/time (or discharge date) of the encounter", formalDefinition="The planned end date/time (or discharge date) of the encounter." ) 357 protected DateTimeType plannedEndDate; 358 359 /** 360 * Actual quantity of time the encounter lasted. This excludes the time during leaves of absence. 361 362When missing it is the time in between the start and end values. 363 */ 364 @Child(name = "length", type = {Duration.class}, order=11, min=0, max=1, modifier=false, summary=false) 365 @Description(shortDefinition="Actual quantity of time the encounter lasted (less time absent)", formalDefinition="Actual quantity of time the encounter lasted. This excludes the time during leaves of absence.\r\rWhen missing it is the time in between the start and end values." ) 366 protected Duration length; 367 368 /** 369 * The location of the patient at this point in the encounter, the multiple cardinality permits de-normalizing the levels of the location hierarchy, such as site/ward/room/bed. 370 */ 371 @Child(name = "location", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 372 @Description(shortDefinition="Location of the patient at this point in the encounter", formalDefinition="The location of the patient at this point in the encounter, the multiple cardinality permits de-normalizing the levels of the location hierarchy, such as site/ward/room/bed." ) 373 protected List<EncounterHistoryLocationComponent> location; 374 375 private static final long serialVersionUID = 775989939L; 376 377 /** 378 * Constructor 379 */ 380 public EncounterHistory() { 381 super(); 382 } 383 384 /** 385 * Constructor 386 */ 387 public EncounterHistory(EncounterStatus status, CodeableConcept class_) { 388 super(); 389 this.setStatus(status); 390 this.setClass_(class_); 391 } 392 393 /** 394 * @return {@link #encounter} (The Encounter associated with this set of historic values.) 395 */ 396 public Reference getEncounter() { 397 if (this.encounter == null) 398 if (Configuration.errorOnAutoCreate()) 399 throw new Error("Attempt to auto-create EncounterHistory.encounter"); 400 else if (Configuration.doAutoCreate()) 401 this.encounter = new Reference(); // cc 402 return this.encounter; 403 } 404 405 public boolean hasEncounter() { 406 return this.encounter != null && !this.encounter.isEmpty(); 407 } 408 409 /** 410 * @param value {@link #encounter} (The Encounter associated with this set of historic values.) 411 */ 412 public EncounterHistory setEncounter(Reference value) { 413 this.encounter = value; 414 return this; 415 } 416 417 /** 418 * @return {@link #identifier} (Identifier(s) by which this encounter is known.) 419 */ 420 public List<Identifier> getIdentifier() { 421 if (this.identifier == null) 422 this.identifier = new ArrayList<Identifier>(); 423 return this.identifier; 424 } 425 426 /** 427 * @return Returns a reference to <code>this</code> for easy method chaining 428 */ 429 public EncounterHistory setIdentifier(List<Identifier> theIdentifier) { 430 this.identifier = theIdentifier; 431 return this; 432 } 433 434 public boolean hasIdentifier() { 435 if (this.identifier == null) 436 return false; 437 for (Identifier item : this.identifier) 438 if (!item.isEmpty()) 439 return true; 440 return false; 441 } 442 443 public Identifier addIdentifier() { //3 444 Identifier t = new Identifier(); 445 if (this.identifier == null) 446 this.identifier = new ArrayList<Identifier>(); 447 this.identifier.add(t); 448 return t; 449 } 450 451 public EncounterHistory addIdentifier(Identifier t) { //3 452 if (t == null) 453 return this; 454 if (this.identifier == null) 455 this.identifier = new ArrayList<Identifier>(); 456 this.identifier.add(t); 457 return this; 458 } 459 460 /** 461 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 462 */ 463 public Identifier getIdentifierFirstRep() { 464 if (getIdentifier().isEmpty()) { 465 addIdentifier(); 466 } 467 return getIdentifier().get(0); 468 } 469 470 /** 471 * @return {@link #status} (planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 472 */ 473 public Enumeration<EncounterStatus> getStatusElement() { 474 if (this.status == null) 475 if (Configuration.errorOnAutoCreate()) 476 throw new Error("Attempt to auto-create EncounterHistory.status"); 477 else if (Configuration.doAutoCreate()) 478 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 479 return this.status; 480 } 481 482 public boolean hasStatusElement() { 483 return this.status != null && !this.status.isEmpty(); 484 } 485 486 public boolean hasStatus() { 487 return this.status != null && !this.status.isEmpty(); 488 } 489 490 /** 491 * @param value {@link #status} (planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 492 */ 493 public EncounterHistory setStatusElement(Enumeration<EncounterStatus> value) { 494 this.status = value; 495 return this; 496 } 497 498 /** 499 * @return planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown. 500 */ 501 public EncounterStatus getStatus() { 502 return this.status == null ? null : this.status.getValue(); 503 } 504 505 /** 506 * @param value planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown. 507 */ 508 public EncounterHistory setStatus(EncounterStatus value) { 509 if (this.status == null) 510 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 511 this.status.setValue(value); 512 return this; 513 } 514 515 /** 516 * @return {@link #class_} (Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.) 517 */ 518 public CodeableConcept getClass_() { 519 if (this.class_ == null) 520 if (Configuration.errorOnAutoCreate()) 521 throw new Error("Attempt to auto-create EncounterHistory.class_"); 522 else if (Configuration.doAutoCreate()) 523 this.class_ = new CodeableConcept(); // cc 524 return this.class_; 525 } 526 527 public boolean hasClass_() { 528 return this.class_ != null && !this.class_.isEmpty(); 529 } 530 531 /** 532 * @param value {@link #class_} (Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.) 533 */ 534 public EncounterHistory setClass_(CodeableConcept value) { 535 this.class_ = value; 536 return this; 537 } 538 539 /** 540 * @return {@link #type} (Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).) 541 */ 542 public List<CodeableConcept> getType() { 543 if (this.type == null) 544 this.type = new ArrayList<CodeableConcept>(); 545 return this.type; 546 } 547 548 /** 549 * @return Returns a reference to <code>this</code> for easy method chaining 550 */ 551 public EncounterHistory setType(List<CodeableConcept> theType) { 552 this.type = theType; 553 return this; 554 } 555 556 public boolean hasType() { 557 if (this.type == null) 558 return false; 559 for (CodeableConcept item : this.type) 560 if (!item.isEmpty()) 561 return true; 562 return false; 563 } 564 565 public CodeableConcept addType() { //3 566 CodeableConcept t = new CodeableConcept(); 567 if (this.type == null) 568 this.type = new ArrayList<CodeableConcept>(); 569 this.type.add(t); 570 return t; 571 } 572 573 public EncounterHistory addType(CodeableConcept t) { //3 574 if (t == null) 575 return this; 576 if (this.type == null) 577 this.type = new ArrayList<CodeableConcept>(); 578 this.type.add(t); 579 return this; 580 } 581 582 /** 583 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 584 */ 585 public CodeableConcept getTypeFirstRep() { 586 if (getType().isEmpty()) { 587 addType(); 588 } 589 return getType().get(0); 590 } 591 592 /** 593 * @return {@link #serviceType} (Broad categorization of the service that is to be provided (e.g. cardiology).) 594 */ 595 public List<CodeableReference> getServiceType() { 596 if (this.serviceType == null) 597 this.serviceType = new ArrayList<CodeableReference>(); 598 return this.serviceType; 599 } 600 601 /** 602 * @return Returns a reference to <code>this</code> for easy method chaining 603 */ 604 public EncounterHistory setServiceType(List<CodeableReference> theServiceType) { 605 this.serviceType = theServiceType; 606 return this; 607 } 608 609 public boolean hasServiceType() { 610 if (this.serviceType == null) 611 return false; 612 for (CodeableReference item : this.serviceType) 613 if (!item.isEmpty()) 614 return true; 615 return false; 616 } 617 618 public CodeableReference addServiceType() { //3 619 CodeableReference t = new CodeableReference(); 620 if (this.serviceType == null) 621 this.serviceType = new ArrayList<CodeableReference>(); 622 this.serviceType.add(t); 623 return t; 624 } 625 626 public EncounterHistory addServiceType(CodeableReference t) { //3 627 if (t == null) 628 return this; 629 if (this.serviceType == null) 630 this.serviceType = new ArrayList<CodeableReference>(); 631 this.serviceType.add(t); 632 return this; 633 } 634 635 /** 636 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist {3} 637 */ 638 public CodeableReference getServiceTypeFirstRep() { 639 if (getServiceType().isEmpty()) { 640 addServiceType(); 641 } 642 return getServiceType().get(0); 643 } 644 645 /** 646 * @return {@link #subject} (The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.) 647 */ 648 public Reference getSubject() { 649 if (this.subject == null) 650 if (Configuration.errorOnAutoCreate()) 651 throw new Error("Attempt to auto-create EncounterHistory.subject"); 652 else if (Configuration.doAutoCreate()) 653 this.subject = new Reference(); // cc 654 return this.subject; 655 } 656 657 public boolean hasSubject() { 658 return this.subject != null && !this.subject.isEmpty(); 659 } 660 661 /** 662 * @param value {@link #subject} (The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.) 663 */ 664 public EncounterHistory setSubject(Reference value) { 665 this.subject = value; 666 return this; 667 } 668 669 /** 670 * @return {@link #subjectStatus} (The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.) 671 */ 672 public CodeableConcept getSubjectStatus() { 673 if (this.subjectStatus == null) 674 if (Configuration.errorOnAutoCreate()) 675 throw new Error("Attempt to auto-create EncounterHistory.subjectStatus"); 676 else if (Configuration.doAutoCreate()) 677 this.subjectStatus = new CodeableConcept(); // cc 678 return this.subjectStatus; 679 } 680 681 public boolean hasSubjectStatus() { 682 return this.subjectStatus != null && !this.subjectStatus.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #subjectStatus} (The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.) 687 */ 688 public EncounterHistory setSubjectStatus(CodeableConcept value) { 689 this.subjectStatus = value; 690 return this; 691 } 692 693 /** 694 * @return {@link #actualPeriod} (The start and end time associated with this set of values associated with the encounter, may be different to the planned times for various reasons.) 695 */ 696 public Period getActualPeriod() { 697 if (this.actualPeriod == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create EncounterHistory.actualPeriod"); 700 else if (Configuration.doAutoCreate()) 701 this.actualPeriod = new Period(); // cc 702 return this.actualPeriod; 703 } 704 705 public boolean hasActualPeriod() { 706 return this.actualPeriod != null && !this.actualPeriod.isEmpty(); 707 } 708 709 /** 710 * @param value {@link #actualPeriod} (The start and end time associated with this set of values associated with the encounter, may be different to the planned times for various reasons.) 711 */ 712 public EncounterHistory setActualPeriod(Period value) { 713 this.actualPeriod = value; 714 return this; 715 } 716 717 /** 718 * @return {@link #plannedStartDate} (The planned start date/time (or admission date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedStartDate" gives direct access to the value 719 */ 720 public DateTimeType getPlannedStartDateElement() { 721 if (this.plannedStartDate == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create EncounterHistory.plannedStartDate"); 724 else if (Configuration.doAutoCreate()) 725 this.plannedStartDate = new DateTimeType(); // bb 726 return this.plannedStartDate; 727 } 728 729 public boolean hasPlannedStartDateElement() { 730 return this.plannedStartDate != null && !this.plannedStartDate.isEmpty(); 731 } 732 733 public boolean hasPlannedStartDate() { 734 return this.plannedStartDate != null && !this.plannedStartDate.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #plannedStartDate} (The planned start date/time (or admission date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedStartDate" gives direct access to the value 739 */ 740 public EncounterHistory setPlannedStartDateElement(DateTimeType value) { 741 this.plannedStartDate = value; 742 return this; 743 } 744 745 /** 746 * @return The planned start date/time (or admission date) of the encounter. 747 */ 748 public Date getPlannedStartDate() { 749 return this.plannedStartDate == null ? null : this.plannedStartDate.getValue(); 750 } 751 752 /** 753 * @param value The planned start date/time (or admission date) of the encounter. 754 */ 755 public EncounterHistory setPlannedStartDate(Date value) { 756 if (value == null) 757 this.plannedStartDate = null; 758 else { 759 if (this.plannedStartDate == null) 760 this.plannedStartDate = new DateTimeType(); 761 this.plannedStartDate.setValue(value); 762 } 763 return this; 764 } 765 766 /** 767 * @return {@link #plannedEndDate} (The planned end date/time (or discharge date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedEndDate" gives direct access to the value 768 */ 769 public DateTimeType getPlannedEndDateElement() { 770 if (this.plannedEndDate == null) 771 if (Configuration.errorOnAutoCreate()) 772 throw new Error("Attempt to auto-create EncounterHistory.plannedEndDate"); 773 else if (Configuration.doAutoCreate()) 774 this.plannedEndDate = new DateTimeType(); // bb 775 return this.plannedEndDate; 776 } 777 778 public boolean hasPlannedEndDateElement() { 779 return this.plannedEndDate != null && !this.plannedEndDate.isEmpty(); 780 } 781 782 public boolean hasPlannedEndDate() { 783 return this.plannedEndDate != null && !this.plannedEndDate.isEmpty(); 784 } 785 786 /** 787 * @param value {@link #plannedEndDate} (The planned end date/time (or discharge date) of the encounter.). This is the underlying object with id, value and extensions. The accessor "getPlannedEndDate" gives direct access to the value 788 */ 789 public EncounterHistory setPlannedEndDateElement(DateTimeType value) { 790 this.plannedEndDate = value; 791 return this; 792 } 793 794 /** 795 * @return The planned end date/time (or discharge date) of the encounter. 796 */ 797 public Date getPlannedEndDate() { 798 return this.plannedEndDate == null ? null : this.plannedEndDate.getValue(); 799 } 800 801 /** 802 * @param value The planned end date/time (or discharge date) of the encounter. 803 */ 804 public EncounterHistory setPlannedEndDate(Date value) { 805 if (value == null) 806 this.plannedEndDate = null; 807 else { 808 if (this.plannedEndDate == null) 809 this.plannedEndDate = new DateTimeType(); 810 this.plannedEndDate.setValue(value); 811 } 812 return this; 813 } 814 815 /** 816 * @return {@link #length} (Actual quantity of time the encounter lasted. This excludes the time during leaves of absence. 817 818When missing it is the time in between the start and end values.) 819 */ 820 public Duration getLength() { 821 if (this.length == null) 822 if (Configuration.errorOnAutoCreate()) 823 throw new Error("Attempt to auto-create EncounterHistory.length"); 824 else if (Configuration.doAutoCreate()) 825 this.length = new Duration(); // cc 826 return this.length; 827 } 828 829 public boolean hasLength() { 830 return this.length != null && !this.length.isEmpty(); 831 } 832 833 /** 834 * @param value {@link #length} (Actual quantity of time the encounter lasted. This excludes the time during leaves of absence. 835 836When missing it is the time in between the start and end values.) 837 */ 838 public EncounterHistory setLength(Duration value) { 839 this.length = value; 840 return this; 841 } 842 843 /** 844 * @return {@link #location} (The location of the patient at this point in the encounter, the multiple cardinality permits de-normalizing the levels of the location hierarchy, such as site/ward/room/bed.) 845 */ 846 public List<EncounterHistoryLocationComponent> getLocation() { 847 if (this.location == null) 848 this.location = new ArrayList<EncounterHistoryLocationComponent>(); 849 return this.location; 850 } 851 852 /** 853 * @return Returns a reference to <code>this</code> for easy method chaining 854 */ 855 public EncounterHistory setLocation(List<EncounterHistoryLocationComponent> theLocation) { 856 this.location = theLocation; 857 return this; 858 } 859 860 public boolean hasLocation() { 861 if (this.location == null) 862 return false; 863 for (EncounterHistoryLocationComponent item : this.location) 864 if (!item.isEmpty()) 865 return true; 866 return false; 867 } 868 869 public EncounterHistoryLocationComponent addLocation() { //3 870 EncounterHistoryLocationComponent t = new EncounterHistoryLocationComponent(); 871 if (this.location == null) 872 this.location = new ArrayList<EncounterHistoryLocationComponent>(); 873 this.location.add(t); 874 return t; 875 } 876 877 public EncounterHistory addLocation(EncounterHistoryLocationComponent t) { //3 878 if (t == null) 879 return this; 880 if (this.location == null) 881 this.location = new ArrayList<EncounterHistoryLocationComponent>(); 882 this.location.add(t); 883 return this; 884 } 885 886 /** 887 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist {3} 888 */ 889 public EncounterHistoryLocationComponent getLocationFirstRep() { 890 if (getLocation().isEmpty()) { 891 addLocation(); 892 } 893 return getLocation().get(0); 894 } 895 896 protected void listChildren(List<Property> children) { 897 super.listChildren(children); 898 children.add(new Property("encounter", "Reference(Encounter)", "The Encounter associated with this set of historic values.", 0, 1, encounter)); 899 children.add(new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier)); 900 children.add(new Property("status", "code", "planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown.", 0, 1, status)); 901 children.add(new Property("class", "CodeableConcept", "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 0, 1, class_)); 902 children.add(new Property("type", "CodeableConcept", "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, java.lang.Integer.MAX_VALUE, type)); 903 children.add(new Property("serviceType", "CodeableReference(HealthcareService)", "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, java.lang.Integer.MAX_VALUE, serviceType)); 904 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.", 0, 1, subject)); 905 children.add(new Property("subjectStatus", "CodeableConcept", "The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.", 0, 1, subjectStatus)); 906 children.add(new Property("actualPeriod", "Period", "The start and end time associated with this set of values associated with the encounter, may be different to the planned times for various reasons.", 0, 1, actualPeriod)); 907 children.add(new Property("plannedStartDate", "dateTime", "The planned start date/time (or admission date) of the encounter.", 0, 1, plannedStartDate)); 908 children.add(new Property("plannedEndDate", "dateTime", "The planned end date/time (or discharge date) of the encounter.", 0, 1, plannedEndDate)); 909 children.add(new Property("length", "Duration", "Actual quantity of time the encounter lasted. This excludes the time during leaves of absence.\r\rWhen missing it is the time in between the start and end values.", 0, 1, length)); 910 children.add(new Property("location", "", "The location of the patient at this point in the encounter, the multiple cardinality permits de-normalizing the levels of the location hierarchy, such as site/ward/room/bed.", 0, java.lang.Integer.MAX_VALUE, location)); 911 } 912 913 @Override 914 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 915 switch (_hash) { 916 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The Encounter associated with this set of historic values.", 0, 1, encounter); 917 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier); 918 case -892481550: /*status*/ return new Property("status", "code", "planned | in-progress | on-hold | discharged | completed | cancelled | discontinued | entered-in-error | unknown.", 0, 1, status); 919 case 94742904: /*class*/ return new Property("class", "CodeableConcept", "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 0, 1, class_); 920 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, java.lang.Integer.MAX_VALUE, type); 921 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableReference(HealthcareService)", "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, java.lang.Integer.MAX_VALUE, serviceType); 922 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group related to this encounter. In some use-cases the patient MAY not be present, such as a case meeting about a patient between several practitioners or a careteam.", 0, 1, subject); 923 case 110854206: /*subjectStatus*/ return new Property("subjectStatus", "CodeableConcept", "The subjectStatus value can be used to track the patient's status within the encounter. It details whether the patient has arrived or departed, has been triaged or is currently in a waiting status.", 0, 1, subjectStatus); 924 case 789194991: /*actualPeriod*/ return new Property("actualPeriod", "Period", "The start and end time associated with this set of values associated with the encounter, may be different to the planned times for various reasons.", 0, 1, actualPeriod); 925 case 460857804: /*plannedStartDate*/ return new Property("plannedStartDate", "dateTime", "The planned start date/time (or admission date) of the encounter.", 0, 1, plannedStartDate); 926 case 1657534661: /*plannedEndDate*/ return new Property("plannedEndDate", "dateTime", "The planned end date/time (or discharge date) of the encounter.", 0, 1, plannedEndDate); 927 case -1106363674: /*length*/ return new Property("length", "Duration", "Actual quantity of time the encounter lasted. This excludes the time during leaves of absence.\r\rWhen missing it is the time in between the start and end values.", 0, 1, length); 928 case 1901043637: /*location*/ return new Property("location", "", "The location of the patient at this point in the encounter, the multiple cardinality permits de-normalizing the levels of the location hierarchy, such as site/ward/room/bed.", 0, java.lang.Integer.MAX_VALUE, location); 929 default: return super.getNamedProperty(_hash, _name, _checkValid); 930 } 931 932 } 933 934 @Override 935 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 936 switch (hash) { 937 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 938 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 939 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EncounterStatus> 940 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // CodeableConcept 941 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 942 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableReference 943 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 944 case 110854206: /*subjectStatus*/ return this.subjectStatus == null ? new Base[0] : new Base[] {this.subjectStatus}; // CodeableConcept 945 case 789194991: /*actualPeriod*/ return this.actualPeriod == null ? new Base[0] : new Base[] {this.actualPeriod}; // Period 946 case 460857804: /*plannedStartDate*/ return this.plannedStartDate == null ? new Base[0] : new Base[] {this.plannedStartDate}; // DateTimeType 947 case 1657534661: /*plannedEndDate*/ return this.plannedEndDate == null ? new Base[0] : new Base[] {this.plannedEndDate}; // DateTimeType 948 case -1106363674: /*length*/ return this.length == null ? new Base[0] : new Base[] {this.length}; // Duration 949 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // EncounterHistoryLocationComponent 950 default: return super.getProperty(hash, name, checkValid); 951 } 952 953 } 954 955 @Override 956 public Base setProperty(int hash, String name, Base value) throws FHIRException { 957 switch (hash) { 958 case 1524132147: // encounter 959 this.encounter = TypeConvertor.castToReference(value); // Reference 960 return value; 961 case -1618432855: // identifier 962 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 963 return value; 964 case -892481550: // status 965 value = new EncounterStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 966 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 967 return value; 968 case 94742904: // class 969 this.class_ = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 970 return value; 971 case 3575610: // type 972 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 973 return value; 974 case -1928370289: // serviceType 975 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 976 return value; 977 case -1867885268: // subject 978 this.subject = TypeConvertor.castToReference(value); // Reference 979 return value; 980 case 110854206: // subjectStatus 981 this.subjectStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 982 return value; 983 case 789194991: // actualPeriod 984 this.actualPeriod = TypeConvertor.castToPeriod(value); // Period 985 return value; 986 case 460857804: // plannedStartDate 987 this.plannedStartDate = TypeConvertor.castToDateTime(value); // DateTimeType 988 return value; 989 case 1657534661: // plannedEndDate 990 this.plannedEndDate = TypeConvertor.castToDateTime(value); // DateTimeType 991 return value; 992 case -1106363674: // length 993 this.length = TypeConvertor.castToDuration(value); // Duration 994 return value; 995 case 1901043637: // location 996 this.getLocation().add((EncounterHistoryLocationComponent) value); // EncounterHistoryLocationComponent 997 return value; 998 default: return super.setProperty(hash, name, value); 999 } 1000 1001 } 1002 1003 @Override 1004 public Base setProperty(String name, Base value) throws FHIRException { 1005 if (name.equals("encounter")) { 1006 this.encounter = TypeConvertor.castToReference(value); // Reference 1007 } else if (name.equals("identifier")) { 1008 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1009 } else if (name.equals("status")) { 1010 value = new EncounterStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1011 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 1012 } else if (name.equals("class")) { 1013 this.class_ = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1014 } else if (name.equals("type")) { 1015 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1016 } else if (name.equals("serviceType")) { 1017 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); 1018 } else if (name.equals("subject")) { 1019 this.subject = TypeConvertor.castToReference(value); // Reference 1020 } else if (name.equals("subjectStatus")) { 1021 this.subjectStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1022 } else if (name.equals("actualPeriod")) { 1023 this.actualPeriod = TypeConvertor.castToPeriod(value); // Period 1024 } else if (name.equals("plannedStartDate")) { 1025 this.plannedStartDate = TypeConvertor.castToDateTime(value); // DateTimeType 1026 } else if (name.equals("plannedEndDate")) { 1027 this.plannedEndDate = TypeConvertor.castToDateTime(value); // DateTimeType 1028 } else if (name.equals("length")) { 1029 this.length = TypeConvertor.castToDuration(value); // Duration 1030 } else if (name.equals("location")) { 1031 this.getLocation().add((EncounterHistoryLocationComponent) value); 1032 } else 1033 return super.setProperty(name, value); 1034 return value; 1035 } 1036 1037 @Override 1038 public void removeChild(String name, Base value) throws FHIRException { 1039 if (name.equals("encounter")) { 1040 this.encounter = null; 1041 } else if (name.equals("identifier")) { 1042 this.getIdentifier().remove(value); 1043 } else if (name.equals("status")) { 1044 value = new EncounterStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1045 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 1046 } else if (name.equals("class")) { 1047 this.class_ = null; 1048 } else if (name.equals("type")) { 1049 this.getType().remove(value); 1050 } else if (name.equals("serviceType")) { 1051 this.getServiceType().remove(value); 1052 } else if (name.equals("subject")) { 1053 this.subject = null; 1054 } else if (name.equals("subjectStatus")) { 1055 this.subjectStatus = null; 1056 } else if (name.equals("actualPeriod")) { 1057 this.actualPeriod = null; 1058 } else if (name.equals("plannedStartDate")) { 1059 this.plannedStartDate = null; 1060 } else if (name.equals("plannedEndDate")) { 1061 this.plannedEndDate = null; 1062 } else if (name.equals("length")) { 1063 this.length = null; 1064 } else if (name.equals("location")) { 1065 this.getLocation().remove((EncounterHistoryLocationComponent) value); 1066 } else 1067 super.removeChild(name, value); 1068 1069 } 1070 1071 @Override 1072 public Base makeProperty(int hash, String name) throws FHIRException { 1073 switch (hash) { 1074 case 1524132147: return getEncounter(); 1075 case -1618432855: return addIdentifier(); 1076 case -892481550: return getStatusElement(); 1077 case 94742904: return getClass_(); 1078 case 3575610: return addType(); 1079 case -1928370289: return addServiceType(); 1080 case -1867885268: return getSubject(); 1081 case 110854206: return getSubjectStatus(); 1082 case 789194991: return getActualPeriod(); 1083 case 460857804: return getPlannedStartDateElement(); 1084 case 1657534661: return getPlannedEndDateElement(); 1085 case -1106363674: return getLength(); 1086 case 1901043637: return addLocation(); 1087 default: return super.makeProperty(hash, name); 1088 } 1089 1090 } 1091 1092 @Override 1093 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1094 switch (hash) { 1095 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1096 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1097 case -892481550: /*status*/ return new String[] {"code"}; 1098 case 94742904: /*class*/ return new String[] {"CodeableConcept"}; 1099 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1100 case -1928370289: /*serviceType*/ return new String[] {"CodeableReference"}; 1101 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1102 case 110854206: /*subjectStatus*/ return new String[] {"CodeableConcept"}; 1103 case 789194991: /*actualPeriod*/ return new String[] {"Period"}; 1104 case 460857804: /*plannedStartDate*/ return new String[] {"dateTime"}; 1105 case 1657534661: /*plannedEndDate*/ return new String[] {"dateTime"}; 1106 case -1106363674: /*length*/ return new String[] {"Duration"}; 1107 case 1901043637: /*location*/ return new String[] {}; 1108 default: return super.getTypesForProperty(hash, name); 1109 } 1110 1111 } 1112 1113 @Override 1114 public Base addChild(String name) throws FHIRException { 1115 if (name.equals("encounter")) { 1116 this.encounter = new Reference(); 1117 return this.encounter; 1118 } 1119 else if (name.equals("identifier")) { 1120 return addIdentifier(); 1121 } 1122 else if (name.equals("status")) { 1123 throw new FHIRException("Cannot call addChild on a singleton property EncounterHistory.status"); 1124 } 1125 else if (name.equals("class")) { 1126 this.class_ = new CodeableConcept(); 1127 return this.class_; 1128 } 1129 else if (name.equals("type")) { 1130 return addType(); 1131 } 1132 else if (name.equals("serviceType")) { 1133 return addServiceType(); 1134 } 1135 else if (name.equals("subject")) { 1136 this.subject = new Reference(); 1137 return this.subject; 1138 } 1139 else if (name.equals("subjectStatus")) { 1140 this.subjectStatus = new CodeableConcept(); 1141 return this.subjectStatus; 1142 } 1143 else if (name.equals("actualPeriod")) { 1144 this.actualPeriod = new Period(); 1145 return this.actualPeriod; 1146 } 1147 else if (name.equals("plannedStartDate")) { 1148 throw new FHIRException("Cannot call addChild on a singleton property EncounterHistory.plannedStartDate"); 1149 } 1150 else if (name.equals("plannedEndDate")) { 1151 throw new FHIRException("Cannot call addChild on a singleton property EncounterHistory.plannedEndDate"); 1152 } 1153 else if (name.equals("length")) { 1154 this.length = new Duration(); 1155 return this.length; 1156 } 1157 else if (name.equals("location")) { 1158 return addLocation(); 1159 } 1160 else 1161 return super.addChild(name); 1162 } 1163 1164 public String fhirType() { 1165 return "EncounterHistory"; 1166 1167 } 1168 1169 public EncounterHistory copy() { 1170 EncounterHistory dst = new EncounterHistory(); 1171 copyValues(dst); 1172 return dst; 1173 } 1174 1175 public void copyValues(EncounterHistory dst) { 1176 super.copyValues(dst); 1177 dst.encounter = encounter == null ? null : encounter.copy(); 1178 if (identifier != null) { 1179 dst.identifier = new ArrayList<Identifier>(); 1180 for (Identifier i : identifier) 1181 dst.identifier.add(i.copy()); 1182 }; 1183 dst.status = status == null ? null : status.copy(); 1184 dst.class_ = class_ == null ? null : class_.copy(); 1185 if (type != null) { 1186 dst.type = new ArrayList<CodeableConcept>(); 1187 for (CodeableConcept i : type) 1188 dst.type.add(i.copy()); 1189 }; 1190 if (serviceType != null) { 1191 dst.serviceType = new ArrayList<CodeableReference>(); 1192 for (CodeableReference i : serviceType) 1193 dst.serviceType.add(i.copy()); 1194 }; 1195 dst.subject = subject == null ? null : subject.copy(); 1196 dst.subjectStatus = subjectStatus == null ? null : subjectStatus.copy(); 1197 dst.actualPeriod = actualPeriod == null ? null : actualPeriod.copy(); 1198 dst.plannedStartDate = plannedStartDate == null ? null : plannedStartDate.copy(); 1199 dst.plannedEndDate = plannedEndDate == null ? null : plannedEndDate.copy(); 1200 dst.length = length == null ? null : length.copy(); 1201 if (location != null) { 1202 dst.location = new ArrayList<EncounterHistoryLocationComponent>(); 1203 for (EncounterHistoryLocationComponent i : location) 1204 dst.location.add(i.copy()); 1205 }; 1206 } 1207 1208 protected EncounterHistory typedCopy() { 1209 return copy(); 1210 } 1211 1212 @Override 1213 public boolean equalsDeep(Base other_) { 1214 if (!super.equalsDeep(other_)) 1215 return false; 1216 if (!(other_ instanceof EncounterHistory)) 1217 return false; 1218 EncounterHistory o = (EncounterHistory) other_; 1219 return compareDeep(encounter, o.encounter, true) && compareDeep(identifier, o.identifier, true) 1220 && compareDeep(status, o.status, true) && compareDeep(class_, o.class_, true) && compareDeep(type, o.type, true) 1221 && compareDeep(serviceType, o.serviceType, true) && compareDeep(subject, o.subject, true) && compareDeep(subjectStatus, o.subjectStatus, true) 1222 && compareDeep(actualPeriod, o.actualPeriod, true) && compareDeep(plannedStartDate, o.plannedStartDate, true) 1223 && compareDeep(plannedEndDate, o.plannedEndDate, true) && compareDeep(length, o.length, true) && compareDeep(location, o.location, true) 1224 ; 1225 } 1226 1227 @Override 1228 public boolean equalsShallow(Base other_) { 1229 if (!super.equalsShallow(other_)) 1230 return false; 1231 if (!(other_ instanceof EncounterHistory)) 1232 return false; 1233 EncounterHistory o = (EncounterHistory) other_; 1234 return compareValues(status, o.status, true) && compareValues(plannedStartDate, o.plannedStartDate, true) 1235 && compareValues(plannedEndDate, o.plannedEndDate, true); 1236 } 1237 1238 public boolean isEmpty() { 1239 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(encounter, identifier, status 1240 , class_, type, serviceType, subject, subjectStatus, actualPeriod, plannedStartDate 1241 , plannedEndDate, length, location); 1242 } 1243 1244 @Override 1245 public ResourceType getResourceType() { 1246 return ResourceType.EncounterHistory; 1247 } 1248 1249 /** 1250 * Search parameter: <b>identifier</b> 1251 * <p> 1252 * Description: <b>Identifier(s) by which this encounter is known</b><br> 1253 * Type: <b>token</b><br> 1254 * Path: <b>EncounterHistory.identifier</b><br> 1255 * </p> 1256 */ 1257 @SearchParamDefinition(name="identifier", path="EncounterHistory.identifier", description="Identifier(s) by which this encounter is known", type="token" ) 1258 public static final String SP_IDENTIFIER = "identifier"; 1259 /** 1260 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1261 * <p> 1262 * Description: <b>Identifier(s) by which this encounter is known</b><br> 1263 * Type: <b>token</b><br> 1264 * Path: <b>EncounterHistory.identifier</b><br> 1265 * </p> 1266 */ 1267 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1268 1269 /** 1270 * Search parameter: <b>patient</b> 1271 * <p> 1272 * Description: <b>The patient present at the encounter</b><br> 1273 * Type: <b>reference</b><br> 1274 * Path: <b>EncounterHistory.subject.where(resolve() is Patient)</b><br> 1275 * </p> 1276 */ 1277 @SearchParamDefinition(name="patient", path="EncounterHistory.subject.where(resolve() is Patient)", description="The patient present at the encounter", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1278 public static final String SP_PATIENT = "patient"; 1279 /** 1280 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1281 * <p> 1282 * Description: <b>The patient present at the encounter</b><br> 1283 * Type: <b>reference</b><br> 1284 * Path: <b>EncounterHistory.subject.where(resolve() is Patient)</b><br> 1285 * </p> 1286 */ 1287 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1288 1289/** 1290 * Constant for fluent queries to be used to add include statements. Specifies 1291 * the path value of "<b>EncounterHistory:patient</b>". 1292 */ 1293 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("EncounterHistory:patient").toLocked(); 1294 1295 /** 1296 * Search parameter: <b>status</b> 1297 * <p> 1298 * Description: <b>Status of the Encounter history entry</b><br> 1299 * Type: <b>token</b><br> 1300 * Path: <b>EncounterHistory.status</b><br> 1301 * </p> 1302 */ 1303 @SearchParamDefinition(name="status", path="EncounterHistory.status", description="Status of the Encounter history entry", type="token" ) 1304 public static final String SP_STATUS = "status"; 1305 /** 1306 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1307 * <p> 1308 * Description: <b>Status of the Encounter history entry</b><br> 1309 * Type: <b>token</b><br> 1310 * Path: <b>EncounterHistory.status</b><br> 1311 * </p> 1312 */ 1313 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1314 1315 /** 1316 * Search parameter: <b>subject</b> 1317 * <p> 1318 * Description: <b>The patient or group present at the encounter</b><br> 1319 * Type: <b>reference</b><br> 1320 * Path: <b>EncounterHistory.subject</b><br> 1321 * </p> 1322 */ 1323 @SearchParamDefinition(name="subject", path="EncounterHistory.subject", description="The patient or group present at the encounter", type="reference", target={Group.class, Patient.class } ) 1324 public static final String SP_SUBJECT = "subject"; 1325 /** 1326 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1327 * <p> 1328 * Description: <b>The patient or group present at the encounter</b><br> 1329 * Type: <b>reference</b><br> 1330 * Path: <b>EncounterHistory.subject</b><br> 1331 * </p> 1332 */ 1333 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1334 1335/** 1336 * Constant for fluent queries to be used to add include statements. Specifies 1337 * the path value of "<b>EncounterHistory:subject</b>". 1338 */ 1339 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("EncounterHistory:subject").toLocked(); 1340 1341 /** 1342 * Search parameter: <b>encounter</b> 1343 * <p> 1344 * Description: <b>Multiple Resources: 1345 1346* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1347* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1348* [ChargeItem](chargeitem.html): Encounter associated with event 1349* [Claim](claim.html): Encounters associated with a billed line item 1350* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1351* [Communication](communication.html): The Encounter during which this Communication was created 1352* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1353* [Composition](composition.html): Context of the Composition 1354* [Condition](condition.html): The Encounter during which this Condition was created 1355* [DeviceRequest](devicerequest.html): Encounter during which request was created 1356* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1357* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1358* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1359* [Flag](flag.html): Alert relevant during encounter 1360* [ImagingStudy](imagingstudy.html): The context of the study 1361* [List](list.html): Context in which list created 1362* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1363* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1364* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1365* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1366* [Observation](observation.html): Encounter related to the observation 1367* [Procedure](procedure.html): The Encounter during which this Procedure was created 1368* [Provenance](provenance.html): Encounter related to the Provenance 1369* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1370* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1371* [RiskAssessment](riskassessment.html): Where was assessment performed? 1372* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1373* [Task](task.html): Search by encounter 1374* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1375</b><br> 1376 * Type: <b>reference</b><br> 1377 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1378 * </p> 1379 */ 1380 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 1381 public static final String SP_ENCOUNTER = "encounter"; 1382 /** 1383 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1384 * <p> 1385 * Description: <b>Multiple Resources: 1386 1387* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1388* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1389* [ChargeItem](chargeitem.html): Encounter associated with event 1390* [Claim](claim.html): Encounters associated with a billed line item 1391* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1392* [Communication](communication.html): The Encounter during which this Communication was created 1393* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1394* [Composition](composition.html): Context of the Composition 1395* [Condition](condition.html): The Encounter during which this Condition was created 1396* [DeviceRequest](devicerequest.html): Encounter during which request was created 1397* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1398* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1399* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1400* [Flag](flag.html): Alert relevant during encounter 1401* [ImagingStudy](imagingstudy.html): The context of the study 1402* [List](list.html): Context in which list created 1403* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1404* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1405* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1406* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1407* [Observation](observation.html): Encounter related to the observation 1408* [Procedure](procedure.html): The Encounter during which this Procedure was created 1409* [Provenance](provenance.html): Encounter related to the Provenance 1410* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1411* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1412* [RiskAssessment](riskassessment.html): Where was assessment performed? 1413* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1414* [Task](task.html): Search by encounter 1415* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1416</b><br> 1417 * Type: <b>reference</b><br> 1418 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1419 * </p> 1420 */ 1421 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 1422 1423/** 1424 * Constant for fluent queries to be used to add include statements. Specifies 1425 * the path value of "<b>EncounterHistory:encounter</b>". 1426 */ 1427 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("EncounterHistory:encounter").toLocked(); 1428 1429 1430} 1431