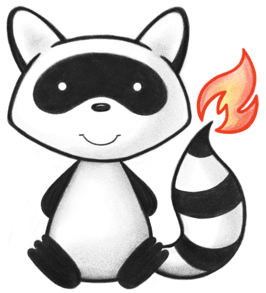
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b, a REST endpoint for another FHIR server, or a s/Mime email address. This may include any security context information. 052 */ 053@ResourceDef(name="Endpoint", profile="http://hl7.org/fhir/StructureDefinition/Endpoint") 054public class Endpoint extends DomainResource { 055 056 public enum EndpointStatus { 057 /** 058 * This endpoint is expected to be active and can be used. 059 */ 060 ACTIVE, 061 /** 062 * This endpoint is temporarily unavailable. 063 */ 064 SUSPENDED, 065 /** 066 * This endpoint has exceeded connectivity thresholds and is considered in an error state and should no longer be attempted to connect to until corrective action is taken. 067 */ 068 ERROR, 069 /** 070 * This endpoint is no longer to be used. 071 */ 072 OFF, 073 /** 074 * This instance should not have been part of this patient's medical record. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static EndpointStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("active".equals(codeString)) 085 return ACTIVE; 086 if ("suspended".equals(codeString)) 087 return SUSPENDED; 088 if ("error".equals(codeString)) 089 return ERROR; 090 if ("off".equals(codeString)) 091 return OFF; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown EndpointStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case ACTIVE: return "active"; 102 case SUSPENDED: return "suspended"; 103 case ERROR: return "error"; 104 case OFF: return "off"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case ACTIVE: return "http://hl7.org/fhir/endpoint-status"; 113 case SUSPENDED: return "http://hl7.org/fhir/endpoint-status"; 114 case ERROR: return "http://hl7.org/fhir/endpoint-status"; 115 case OFF: return "http://hl7.org/fhir/endpoint-status"; 116 case ENTEREDINERROR: return "http://hl7.org/fhir/endpoint-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case ACTIVE: return "This endpoint is expected to be active and can be used."; 124 case SUSPENDED: return "This endpoint is temporarily unavailable."; 125 case ERROR: return "This endpoint has exceeded connectivity thresholds and is considered in an error state and should no longer be attempted to connect to until corrective action is taken."; 126 case OFF: return "This endpoint is no longer to be used."; 127 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case ACTIVE: return "Active"; 135 case SUSPENDED: return "Suspended"; 136 case ERROR: return "Error"; 137 case OFF: return "Off"; 138 case ENTEREDINERROR: return "Entered in error"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class EndpointStatusEnumFactory implements EnumFactory<EndpointStatus> { 146 public EndpointStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("active".equals(codeString)) 151 return EndpointStatus.ACTIVE; 152 if ("suspended".equals(codeString)) 153 return EndpointStatus.SUSPENDED; 154 if ("error".equals(codeString)) 155 return EndpointStatus.ERROR; 156 if ("off".equals(codeString)) 157 return EndpointStatus.OFF; 158 if ("entered-in-error".equals(codeString)) 159 return EndpointStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown EndpointStatus code '"+codeString+"'"); 161 } 162 public Enumeration<EndpointStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<EndpointStatus>(this, EndpointStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<EndpointStatus>(this, EndpointStatus.NULL, code); 170 if ("active".equals(codeString)) 171 return new Enumeration<EndpointStatus>(this, EndpointStatus.ACTIVE, code); 172 if ("suspended".equals(codeString)) 173 return new Enumeration<EndpointStatus>(this, EndpointStatus.SUSPENDED, code); 174 if ("error".equals(codeString)) 175 return new Enumeration<EndpointStatus>(this, EndpointStatus.ERROR, code); 176 if ("off".equals(codeString)) 177 return new Enumeration<EndpointStatus>(this, EndpointStatus.OFF, code); 178 if ("entered-in-error".equals(codeString)) 179 return new Enumeration<EndpointStatus>(this, EndpointStatus.ENTEREDINERROR, code); 180 throw new FHIRException("Unknown EndpointStatus code '"+codeString+"'"); 181 } 182 public String toCode(EndpointStatus code) { 183 if (code == EndpointStatus.NULL) 184 return null; 185 if (code == EndpointStatus.ACTIVE) 186 return "active"; 187 if (code == EndpointStatus.SUSPENDED) 188 return "suspended"; 189 if (code == EndpointStatus.ERROR) 190 return "error"; 191 if (code == EndpointStatus.OFF) 192 return "off"; 193 if (code == EndpointStatus.ENTEREDINERROR) 194 return "entered-in-error"; 195 return "?"; 196 } 197 public String toSystem(EndpointStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class EndpointPayloadComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * The payload type describes the acceptable content that can be communicated on the endpoint. 206 */ 207 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 208 @Description(shortDefinition="The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", formalDefinition="The payload type describes the acceptable content that can be communicated on the endpoint." ) 209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-payload-type") 210 protected List<CodeableConcept> type; 211 212 /** 213 * The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType). 214 */ 215 @Child(name = "mimeType", type = {CodeType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 216 @Description(shortDefinition="Mimetype to send. If not specified, the content could be anything (including no payload, if the connectionType defined this)", formalDefinition="The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType)." ) 217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 218 protected List<CodeType> mimeType; 219 220 private static final long serialVersionUID = -1398955844L; 221 222 /** 223 * Constructor 224 */ 225 public EndpointPayloadComponent() { 226 super(); 227 } 228 229 /** 230 * @return {@link #type} (The payload type describes the acceptable content that can be communicated on the endpoint.) 231 */ 232 public List<CodeableConcept> getType() { 233 if (this.type == null) 234 this.type = new ArrayList<CodeableConcept>(); 235 return this.type; 236 } 237 238 /** 239 * @return Returns a reference to <code>this</code> for easy method chaining 240 */ 241 public EndpointPayloadComponent setType(List<CodeableConcept> theType) { 242 this.type = theType; 243 return this; 244 } 245 246 public boolean hasType() { 247 if (this.type == null) 248 return false; 249 for (CodeableConcept item : this.type) 250 if (!item.isEmpty()) 251 return true; 252 return false; 253 } 254 255 public CodeableConcept addType() { //3 256 CodeableConcept t = new CodeableConcept(); 257 if (this.type == null) 258 this.type = new ArrayList<CodeableConcept>(); 259 this.type.add(t); 260 return t; 261 } 262 263 public EndpointPayloadComponent addType(CodeableConcept t) { //3 264 if (t == null) 265 return this; 266 if (this.type == null) 267 this.type = new ArrayList<CodeableConcept>(); 268 this.type.add(t); 269 return this; 270 } 271 272 /** 273 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 274 */ 275 public CodeableConcept getTypeFirstRep() { 276 if (getType().isEmpty()) { 277 addType(); 278 } 279 return getType().get(0); 280 } 281 282 /** 283 * @return {@link #mimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 284 */ 285 public List<CodeType> getMimeType() { 286 if (this.mimeType == null) 287 this.mimeType = new ArrayList<CodeType>(); 288 return this.mimeType; 289 } 290 291 /** 292 * @return Returns a reference to <code>this</code> for easy method chaining 293 */ 294 public EndpointPayloadComponent setMimeType(List<CodeType> theMimeType) { 295 this.mimeType = theMimeType; 296 return this; 297 } 298 299 public boolean hasMimeType() { 300 if (this.mimeType == null) 301 return false; 302 for (CodeType item : this.mimeType) 303 if (!item.isEmpty()) 304 return true; 305 return false; 306 } 307 308 /** 309 * @return {@link #mimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 310 */ 311 public CodeType addMimeTypeElement() {//2 312 CodeType t = new CodeType(); 313 if (this.mimeType == null) 314 this.mimeType = new ArrayList<CodeType>(); 315 this.mimeType.add(t); 316 return t; 317 } 318 319 /** 320 * @param value {@link #mimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 321 */ 322 public EndpointPayloadComponent addMimeType(String value) { //1 323 CodeType t = new CodeType(); 324 t.setValue(value); 325 if (this.mimeType == null) 326 this.mimeType = new ArrayList<CodeType>(); 327 this.mimeType.add(t); 328 return this; 329 } 330 331 /** 332 * @param value {@link #mimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 333 */ 334 public boolean hasMimeType(String value) { 335 if (this.mimeType == null) 336 return false; 337 for (CodeType v : this.mimeType) 338 if (v.getValue().equals(value)) // code 339 return true; 340 return false; 341 } 342 343 protected void listChildren(List<Property> children) { 344 super.listChildren(children); 345 children.add(new Property("type", "CodeableConcept", "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, java.lang.Integer.MAX_VALUE, type)); 346 children.add(new Property("mimeType", "code", "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 0, java.lang.Integer.MAX_VALUE, mimeType)); 347 } 348 349 @Override 350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 351 switch (_hash) { 352 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, java.lang.Integer.MAX_VALUE, type); 353 case -1392120434: /*mimeType*/ return new Property("mimeType", "code", "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 0, java.lang.Integer.MAX_VALUE, mimeType); 354 default: return super.getNamedProperty(_hash, _name, _checkValid); 355 } 356 357 } 358 359 @Override 360 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 361 switch (hash) { 362 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 363 case -1392120434: /*mimeType*/ return this.mimeType == null ? new Base[0] : this.mimeType.toArray(new Base[this.mimeType.size()]); // CodeType 364 default: return super.getProperty(hash, name, checkValid); 365 } 366 367 } 368 369 @Override 370 public Base setProperty(int hash, String name, Base value) throws FHIRException { 371 switch (hash) { 372 case 3575610: // type 373 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 374 return value; 375 case -1392120434: // mimeType 376 this.getMimeType().add(TypeConvertor.castToCode(value)); // CodeType 377 return value; 378 default: return super.setProperty(hash, name, value); 379 } 380 381 } 382 383 @Override 384 public Base setProperty(String name, Base value) throws FHIRException { 385 if (name.equals("type")) { 386 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 387 } else if (name.equals("mimeType")) { 388 this.getMimeType().add(TypeConvertor.castToCode(value)); 389 } else 390 return super.setProperty(name, value); 391 return value; 392 } 393 394 @Override 395 public void removeChild(String name, Base value) throws FHIRException { 396 if (name.equals("type")) { 397 this.getType().remove(value); 398 } else if (name.equals("mimeType")) { 399 this.getMimeType().remove(value); 400 } else 401 super.removeChild(name, value); 402 403 } 404 405 @Override 406 public Base makeProperty(int hash, String name) throws FHIRException { 407 switch (hash) { 408 case 3575610: return addType(); 409 case -1392120434: return addMimeTypeElement(); 410 default: return super.makeProperty(hash, name); 411 } 412 413 } 414 415 @Override 416 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 417 switch (hash) { 418 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 419 case -1392120434: /*mimeType*/ return new String[] {"code"}; 420 default: return super.getTypesForProperty(hash, name); 421 } 422 423 } 424 425 @Override 426 public Base addChild(String name) throws FHIRException { 427 if (name.equals("type")) { 428 return addType(); 429 } 430 else if (name.equals("mimeType")) { 431 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.payload.mimeType"); 432 } 433 else 434 return super.addChild(name); 435 } 436 437 public EndpointPayloadComponent copy() { 438 EndpointPayloadComponent dst = new EndpointPayloadComponent(); 439 copyValues(dst); 440 return dst; 441 } 442 443 public void copyValues(EndpointPayloadComponent dst) { 444 super.copyValues(dst); 445 if (type != null) { 446 dst.type = new ArrayList<CodeableConcept>(); 447 for (CodeableConcept i : type) 448 dst.type.add(i.copy()); 449 }; 450 if (mimeType != null) { 451 dst.mimeType = new ArrayList<CodeType>(); 452 for (CodeType i : mimeType) 453 dst.mimeType.add(i.copy()); 454 }; 455 } 456 457 @Override 458 public boolean equalsDeep(Base other_) { 459 if (!super.equalsDeep(other_)) 460 return false; 461 if (!(other_ instanceof EndpointPayloadComponent)) 462 return false; 463 EndpointPayloadComponent o = (EndpointPayloadComponent) other_; 464 return compareDeep(type, o.type, true) && compareDeep(mimeType, o.mimeType, true); 465 } 466 467 @Override 468 public boolean equalsShallow(Base other_) { 469 if (!super.equalsShallow(other_)) 470 return false; 471 if (!(other_ instanceof EndpointPayloadComponent)) 472 return false; 473 EndpointPayloadComponent o = (EndpointPayloadComponent) other_; 474 return compareValues(mimeType, o.mimeType, true); 475 } 476 477 public boolean isEmpty() { 478 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, mimeType); 479 } 480 481 public String fhirType() { 482 return "Endpoint.payload"; 483 484 } 485 486 } 487 488 /** 489 * Identifier for the organization that is used to identify the endpoint across multiple disparate systems. 490 */ 491 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 492 @Description(shortDefinition="Identifies this endpoint across multiple systems", formalDefinition="Identifier for the organization that is used to identify the endpoint across multiple disparate systems." ) 493 protected List<Identifier> identifier; 494 495 /** 496 * The endpoint status represents the general expected availability of an endpoint. 497 */ 498 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 499 @Description(shortDefinition="active | suspended | error | off | entered-in-error | test", formalDefinition="The endpoint status represents the general expected availability of an endpoint." ) 500 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-status") 501 protected Enumeration<EndpointStatus> status; 502 503 /** 504 * A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook). 505 */ 506 @Child(name = "connectionType", type = {CodeableConcept.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 507 @Description(shortDefinition="Protocol/Profile/Standard to be used with this endpoint connection", formalDefinition="A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook)." ) 508 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-connection-type") 509 protected List<CodeableConcept> connectionType; 510 511 /** 512 * A friendly name that this endpoint can be referred to with. 513 */ 514 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 515 @Description(shortDefinition="A name that this endpoint can be identified by", formalDefinition="A friendly name that this endpoint can be referred to with." ) 516 protected StringType name; 517 518 /** 519 * The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose). 520 */ 521 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 522 @Description(shortDefinition="Additional details about the endpoint that could be displayed as further information to identify the description beyond its name", formalDefinition="The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose)." ) 523 protected StringType description; 524 525 /** 526 * The type of environment(s) exposed at this endpoint (dev, prod, test, etc.). 527 */ 528 @Child(name = "environmentType", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 529 @Description(shortDefinition="The type of environment(s) exposed at this endpoint", formalDefinition="The type of environment(s) exposed at this endpoint (dev, prod, test, etc.)." ) 530 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-environment") 531 protected List<CodeableConcept> environmentType; 532 533 /** 534 * The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data). 535 */ 536 @Child(name = "managingOrganization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=true) 537 @Description(shortDefinition="Organization that manages this endpoint (might not be the organization that exposes the endpoint)", formalDefinition="The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data)." ) 538 protected Reference managingOrganization; 539 540 /** 541 * Contact details for a human to contact about the endpoint. The primary use of this for system administrator troubleshooting. 542 */ 543 @Child(name = "contact", type = {ContactPoint.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 544 @Description(shortDefinition="Contact details for source (e.g. troubleshooting)", formalDefinition="Contact details for a human to contact about the endpoint. The primary use of this for system administrator troubleshooting." ) 545 protected List<ContactPoint> contact; 546 547 /** 548 * The interval during which the endpoint is expected to be operational. 549 */ 550 @Child(name = "period", type = {Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 551 @Description(shortDefinition="Interval the endpoint is expected to be operational", formalDefinition="The interval during which the endpoint is expected to be operational." ) 552 protected Period period; 553 554 /** 555 * The set of payloads that are provided/available at this endpoint. 556 */ 557 @Child(name = "payload", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 558 @Description(shortDefinition="Set of payloads that are provided by this endpoint", formalDefinition="The set of payloads that are provided/available at this endpoint." ) 559 protected List<EndpointPayloadComponent> payload; 560 561 /** 562 * The uri that describes the actual end-point to connect to. 563 */ 564 @Child(name = "address", type = {UrlType.class}, order=10, min=1, max=1, modifier=false, summary=true) 565 @Description(shortDefinition="The technical base address for connecting to this endpoint", formalDefinition="The uri that describes the actual end-point to connect to." ) 566 protected UrlType address; 567 568 /** 569 * Additional headers / information to send as part of the notification. 570 */ 571 @Child(name = "header", type = {StringType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 572 @Description(shortDefinition="Usage depends on the channel type", formalDefinition="Additional headers / information to send as part of the notification." ) 573 protected List<StringType> header; 574 575 private static final long serialVersionUID = 1633700267L; 576 577 /** 578 * Constructor 579 */ 580 public Endpoint() { 581 super(); 582 } 583 584 /** 585 * Constructor 586 */ 587 public Endpoint(EndpointStatus status, CodeableConcept connectionType, String address) { 588 super(); 589 this.setStatus(status); 590 this.addConnectionType(connectionType); 591 this.setAddress(address); 592 } 593 594 /** 595 * @return {@link #identifier} (Identifier for the organization that is used to identify the endpoint across multiple disparate systems.) 596 */ 597 public List<Identifier> getIdentifier() { 598 if (this.identifier == null) 599 this.identifier = new ArrayList<Identifier>(); 600 return this.identifier; 601 } 602 603 /** 604 * @return Returns a reference to <code>this</code> for easy method chaining 605 */ 606 public Endpoint setIdentifier(List<Identifier> theIdentifier) { 607 this.identifier = theIdentifier; 608 return this; 609 } 610 611 public boolean hasIdentifier() { 612 if (this.identifier == null) 613 return false; 614 for (Identifier item : this.identifier) 615 if (!item.isEmpty()) 616 return true; 617 return false; 618 } 619 620 public Identifier addIdentifier() { //3 621 Identifier t = new Identifier(); 622 if (this.identifier == null) 623 this.identifier = new ArrayList<Identifier>(); 624 this.identifier.add(t); 625 return t; 626 } 627 628 public Endpoint addIdentifier(Identifier t) { //3 629 if (t == null) 630 return this; 631 if (this.identifier == null) 632 this.identifier = new ArrayList<Identifier>(); 633 this.identifier.add(t); 634 return this; 635 } 636 637 /** 638 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 639 */ 640 public Identifier getIdentifierFirstRep() { 641 if (getIdentifier().isEmpty()) { 642 addIdentifier(); 643 } 644 return getIdentifier().get(0); 645 } 646 647 /** 648 * @return {@link #status} (The endpoint status represents the general expected availability of an endpoint.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 649 */ 650 public Enumeration<EndpointStatus> getStatusElement() { 651 if (this.status == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create Endpoint.status"); 654 else if (Configuration.doAutoCreate()) 655 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); // bb 656 return this.status; 657 } 658 659 public boolean hasStatusElement() { 660 return this.status != null && !this.status.isEmpty(); 661 } 662 663 public boolean hasStatus() { 664 return this.status != null && !this.status.isEmpty(); 665 } 666 667 /** 668 * @param value {@link #status} (The endpoint status represents the general expected availability of an endpoint.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 669 */ 670 public Endpoint setStatusElement(Enumeration<EndpointStatus> value) { 671 this.status = value; 672 return this; 673 } 674 675 /** 676 * @return The endpoint status represents the general expected availability of an endpoint. 677 */ 678 public EndpointStatus getStatus() { 679 return this.status == null ? null : this.status.getValue(); 680 } 681 682 /** 683 * @param value The endpoint status represents the general expected availability of an endpoint. 684 */ 685 public Endpoint setStatus(EndpointStatus value) { 686 if (this.status == null) 687 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); 688 this.status.setValue(value); 689 return this; 690 } 691 692 /** 693 * @return {@link #connectionType} (A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).) 694 */ 695 public List<CodeableConcept> getConnectionType() { 696 if (this.connectionType == null) 697 this.connectionType = new ArrayList<CodeableConcept>(); 698 return this.connectionType; 699 } 700 701 /** 702 * @return Returns a reference to <code>this</code> for easy method chaining 703 */ 704 public Endpoint setConnectionType(List<CodeableConcept> theConnectionType) { 705 this.connectionType = theConnectionType; 706 return this; 707 } 708 709 public boolean hasConnectionType() { 710 if (this.connectionType == null) 711 return false; 712 for (CodeableConcept item : this.connectionType) 713 if (!item.isEmpty()) 714 return true; 715 return false; 716 } 717 718 public CodeableConcept addConnectionType() { //3 719 CodeableConcept t = new CodeableConcept(); 720 if (this.connectionType == null) 721 this.connectionType = new ArrayList<CodeableConcept>(); 722 this.connectionType.add(t); 723 return t; 724 } 725 726 public Endpoint addConnectionType(CodeableConcept t) { //3 727 if (t == null) 728 return this; 729 if (this.connectionType == null) 730 this.connectionType = new ArrayList<CodeableConcept>(); 731 this.connectionType.add(t); 732 return this; 733 } 734 735 /** 736 * @return The first repetition of repeating field {@link #connectionType}, creating it if it does not already exist {3} 737 */ 738 public CodeableConcept getConnectionTypeFirstRep() { 739 if (getConnectionType().isEmpty()) { 740 addConnectionType(); 741 } 742 return getConnectionType().get(0); 743 } 744 745 /** 746 * @return {@link #name} (A friendly name that this endpoint can be referred to with.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 747 */ 748 public StringType getNameElement() { 749 if (this.name == null) 750 if (Configuration.errorOnAutoCreate()) 751 throw new Error("Attempt to auto-create Endpoint.name"); 752 else if (Configuration.doAutoCreate()) 753 this.name = new StringType(); // bb 754 return this.name; 755 } 756 757 public boolean hasNameElement() { 758 return this.name != null && !this.name.isEmpty(); 759 } 760 761 public boolean hasName() { 762 return this.name != null && !this.name.isEmpty(); 763 } 764 765 /** 766 * @param value {@link #name} (A friendly name that this endpoint can be referred to with.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 767 */ 768 public Endpoint setNameElement(StringType value) { 769 this.name = value; 770 return this; 771 } 772 773 /** 774 * @return A friendly name that this endpoint can be referred to with. 775 */ 776 public String getName() { 777 return this.name == null ? null : this.name.getValue(); 778 } 779 780 /** 781 * @param value A friendly name that this endpoint can be referred to with. 782 */ 783 public Endpoint setName(String value) { 784 if (Utilities.noString(value)) 785 this.name = null; 786 else { 787 if (this.name == null) 788 this.name = new StringType(); 789 this.name.setValue(value); 790 } 791 return this; 792 } 793 794 /** 795 * @return {@link #description} (The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 796 */ 797 public StringType getDescriptionElement() { 798 if (this.description == null) 799 if (Configuration.errorOnAutoCreate()) 800 throw new Error("Attempt to auto-create Endpoint.description"); 801 else if (Configuration.doAutoCreate()) 802 this.description = new StringType(); // bb 803 return this.description; 804 } 805 806 public boolean hasDescriptionElement() { 807 return this.description != null && !this.description.isEmpty(); 808 } 809 810 public boolean hasDescription() { 811 return this.description != null && !this.description.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #description} (The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 816 */ 817 public Endpoint setDescriptionElement(StringType value) { 818 this.description = value; 819 return this; 820 } 821 822 /** 823 * @return The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose). 824 */ 825 public String getDescription() { 826 return this.description == null ? null : this.description.getValue(); 827 } 828 829 /** 830 * @param value The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose). 831 */ 832 public Endpoint setDescription(String value) { 833 if (Utilities.noString(value)) 834 this.description = null; 835 else { 836 if (this.description == null) 837 this.description = new StringType(); 838 this.description.setValue(value); 839 } 840 return this; 841 } 842 843 /** 844 * @return {@link #environmentType} (The type of environment(s) exposed at this endpoint (dev, prod, test, etc.).) 845 */ 846 public List<CodeableConcept> getEnvironmentType() { 847 if (this.environmentType == null) 848 this.environmentType = new ArrayList<CodeableConcept>(); 849 return this.environmentType; 850 } 851 852 /** 853 * @return Returns a reference to <code>this</code> for easy method chaining 854 */ 855 public Endpoint setEnvironmentType(List<CodeableConcept> theEnvironmentType) { 856 this.environmentType = theEnvironmentType; 857 return this; 858 } 859 860 public boolean hasEnvironmentType() { 861 if (this.environmentType == null) 862 return false; 863 for (CodeableConcept item : this.environmentType) 864 if (!item.isEmpty()) 865 return true; 866 return false; 867 } 868 869 public CodeableConcept addEnvironmentType() { //3 870 CodeableConcept t = new CodeableConcept(); 871 if (this.environmentType == null) 872 this.environmentType = new ArrayList<CodeableConcept>(); 873 this.environmentType.add(t); 874 return t; 875 } 876 877 public Endpoint addEnvironmentType(CodeableConcept t) { //3 878 if (t == null) 879 return this; 880 if (this.environmentType == null) 881 this.environmentType = new ArrayList<CodeableConcept>(); 882 this.environmentType.add(t); 883 return this; 884 } 885 886 /** 887 * @return The first repetition of repeating field {@link #environmentType}, creating it if it does not already exist {3} 888 */ 889 public CodeableConcept getEnvironmentTypeFirstRep() { 890 if (getEnvironmentType().isEmpty()) { 891 addEnvironmentType(); 892 } 893 return getEnvironmentType().get(0); 894 } 895 896 /** 897 * @return {@link #managingOrganization} (The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data).) 898 */ 899 public Reference getManagingOrganization() { 900 if (this.managingOrganization == null) 901 if (Configuration.errorOnAutoCreate()) 902 throw new Error("Attempt to auto-create Endpoint.managingOrganization"); 903 else if (Configuration.doAutoCreate()) 904 this.managingOrganization = new Reference(); // cc 905 return this.managingOrganization; 906 } 907 908 public boolean hasManagingOrganization() { 909 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 910 } 911 912 /** 913 * @param value {@link #managingOrganization} (The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data).) 914 */ 915 public Endpoint setManagingOrganization(Reference value) { 916 this.managingOrganization = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #contact} (Contact details for a human to contact about the endpoint. The primary use of this for system administrator troubleshooting.) 922 */ 923 public List<ContactPoint> getContact() { 924 if (this.contact == null) 925 this.contact = new ArrayList<ContactPoint>(); 926 return this.contact; 927 } 928 929 /** 930 * @return Returns a reference to <code>this</code> for easy method chaining 931 */ 932 public Endpoint setContact(List<ContactPoint> theContact) { 933 this.contact = theContact; 934 return this; 935 } 936 937 public boolean hasContact() { 938 if (this.contact == null) 939 return false; 940 for (ContactPoint item : this.contact) 941 if (!item.isEmpty()) 942 return true; 943 return false; 944 } 945 946 public ContactPoint addContact() { //3 947 ContactPoint t = new ContactPoint(); 948 if (this.contact == null) 949 this.contact = new ArrayList<ContactPoint>(); 950 this.contact.add(t); 951 return t; 952 } 953 954 public Endpoint addContact(ContactPoint t) { //3 955 if (t == null) 956 return this; 957 if (this.contact == null) 958 this.contact = new ArrayList<ContactPoint>(); 959 this.contact.add(t); 960 return this; 961 } 962 963 /** 964 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 965 */ 966 public ContactPoint getContactFirstRep() { 967 if (getContact().isEmpty()) { 968 addContact(); 969 } 970 return getContact().get(0); 971 } 972 973 /** 974 * @return {@link #period} (The interval during which the endpoint is expected to be operational.) 975 */ 976 public Period getPeriod() { 977 if (this.period == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create Endpoint.period"); 980 else if (Configuration.doAutoCreate()) 981 this.period = new Period(); // cc 982 return this.period; 983 } 984 985 public boolean hasPeriod() { 986 return this.period != null && !this.period.isEmpty(); 987 } 988 989 /** 990 * @param value {@link #period} (The interval during which the endpoint is expected to be operational.) 991 */ 992 public Endpoint setPeriod(Period value) { 993 this.period = value; 994 return this; 995 } 996 997 /** 998 * @return {@link #payload} (The set of payloads that are provided/available at this endpoint.) 999 */ 1000 public List<EndpointPayloadComponent> getPayload() { 1001 if (this.payload == null) 1002 this.payload = new ArrayList<EndpointPayloadComponent>(); 1003 return this.payload; 1004 } 1005 1006 /** 1007 * @return Returns a reference to <code>this</code> for easy method chaining 1008 */ 1009 public Endpoint setPayload(List<EndpointPayloadComponent> thePayload) { 1010 this.payload = thePayload; 1011 return this; 1012 } 1013 1014 public boolean hasPayload() { 1015 if (this.payload == null) 1016 return false; 1017 for (EndpointPayloadComponent item : this.payload) 1018 if (!item.isEmpty()) 1019 return true; 1020 return false; 1021 } 1022 1023 public EndpointPayloadComponent addPayload() { //3 1024 EndpointPayloadComponent t = new EndpointPayloadComponent(); 1025 if (this.payload == null) 1026 this.payload = new ArrayList<EndpointPayloadComponent>(); 1027 this.payload.add(t); 1028 return t; 1029 } 1030 1031 public Endpoint addPayload(EndpointPayloadComponent t) { //3 1032 if (t == null) 1033 return this; 1034 if (this.payload == null) 1035 this.payload = new ArrayList<EndpointPayloadComponent>(); 1036 this.payload.add(t); 1037 return this; 1038 } 1039 1040 /** 1041 * @return The first repetition of repeating field {@link #payload}, creating it if it does not already exist {3} 1042 */ 1043 public EndpointPayloadComponent getPayloadFirstRep() { 1044 if (getPayload().isEmpty()) { 1045 addPayload(); 1046 } 1047 return getPayload().get(0); 1048 } 1049 1050 /** 1051 * @return {@link #address} (The uri that describes the actual end-point to connect to.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 1052 */ 1053 public UrlType getAddressElement() { 1054 if (this.address == null) 1055 if (Configuration.errorOnAutoCreate()) 1056 throw new Error("Attempt to auto-create Endpoint.address"); 1057 else if (Configuration.doAutoCreate()) 1058 this.address = new UrlType(); // bb 1059 return this.address; 1060 } 1061 1062 public boolean hasAddressElement() { 1063 return this.address != null && !this.address.isEmpty(); 1064 } 1065 1066 public boolean hasAddress() { 1067 return this.address != null && !this.address.isEmpty(); 1068 } 1069 1070 /** 1071 * @param value {@link #address} (The uri that describes the actual end-point to connect to.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 1072 */ 1073 public Endpoint setAddressElement(UrlType value) { 1074 this.address = value; 1075 return this; 1076 } 1077 1078 /** 1079 * @return The uri that describes the actual end-point to connect to. 1080 */ 1081 public String getAddress() { 1082 return this.address == null ? null : this.address.getValue(); 1083 } 1084 1085 /** 1086 * @param value The uri that describes the actual end-point to connect to. 1087 */ 1088 public Endpoint setAddress(String value) { 1089 if (this.address == null) 1090 this.address = new UrlType(); 1091 this.address.setValue(value); 1092 return this; 1093 } 1094 1095 /** 1096 * @return {@link #header} (Additional headers / information to send as part of the notification.) 1097 */ 1098 public List<StringType> getHeader() { 1099 if (this.header == null) 1100 this.header = new ArrayList<StringType>(); 1101 return this.header; 1102 } 1103 1104 /** 1105 * @return Returns a reference to <code>this</code> for easy method chaining 1106 */ 1107 public Endpoint setHeader(List<StringType> theHeader) { 1108 this.header = theHeader; 1109 return this; 1110 } 1111 1112 public boolean hasHeader() { 1113 if (this.header == null) 1114 return false; 1115 for (StringType item : this.header) 1116 if (!item.isEmpty()) 1117 return true; 1118 return false; 1119 } 1120 1121 /** 1122 * @return {@link #header} (Additional headers / information to send as part of the notification.) 1123 */ 1124 public StringType addHeaderElement() {//2 1125 StringType t = new StringType(); 1126 if (this.header == null) 1127 this.header = new ArrayList<StringType>(); 1128 this.header.add(t); 1129 return t; 1130 } 1131 1132 /** 1133 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 1134 */ 1135 public Endpoint addHeader(String value) { //1 1136 StringType t = new StringType(); 1137 t.setValue(value); 1138 if (this.header == null) 1139 this.header = new ArrayList<StringType>(); 1140 this.header.add(t); 1141 return this; 1142 } 1143 1144 /** 1145 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 1146 */ 1147 public boolean hasHeader(String value) { 1148 if (this.header == null) 1149 return false; 1150 for (StringType v : this.header) 1151 if (v.getValue().equals(value)) // string 1152 return true; 1153 return false; 1154 } 1155 1156 protected void listChildren(List<Property> children) { 1157 super.listChildren(children); 1158 children.add(new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1159 children.add(new Property("status", "code", "The endpoint status represents the general expected availability of an endpoint.", 0, 1, status)); 1160 children.add(new Property("connectionType", "CodeableConcept", "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 0, java.lang.Integer.MAX_VALUE, connectionType)); 1161 children.add(new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 1, name)); 1162 children.add(new Property("description", "string", "The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose).", 0, 1, description)); 1163 children.add(new Property("environmentType", "CodeableConcept", "The type of environment(s) exposed at this endpoint (dev, prod, test, etc.).", 0, java.lang.Integer.MAX_VALUE, environmentType)); 1164 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data).", 0, 1, managingOrganization)); 1165 children.add(new Property("contact", "ContactPoint", "Contact details for a human to contact about the endpoint. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact)); 1166 children.add(new Property("period", "Period", "The interval during which the endpoint is expected to be operational.", 0, 1, period)); 1167 children.add(new Property("payload", "", "The set of payloads that are provided/available at this endpoint.", 0, java.lang.Integer.MAX_VALUE, payload)); 1168 children.add(new Property("address", "url", "The uri that describes the actual end-point to connect to.", 0, 1, address)); 1169 children.add(new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header)); 1170 } 1171 1172 @Override 1173 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1174 switch (_hash) { 1175 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1176 case -892481550: /*status*/ return new Property("status", "code", "The endpoint status represents the general expected availability of an endpoint.", 0, 1, status); 1177 case 1270211384: /*connectionType*/ return new Property("connectionType", "CodeableConcept", "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 0, java.lang.Integer.MAX_VALUE, connectionType); 1178 case 3373707: /*name*/ return new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 1, name); 1179 case -1724546052: /*description*/ return new Property("description", "string", "The description of the endpoint and what it is for (typically used as supplemental information in an endpoint directory describing its usage/purpose).", 0, 1, description); 1180 case 1680602093: /*environmentType*/ return new Property("environmentType", "CodeableConcept", "The type of environment(s) exposed at this endpoint (dev, prod, test, etc.).", 0, java.lang.Integer.MAX_VALUE, environmentType); 1181 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization that manages this endpoint (even if technically another organization is hosting this in the cloud, it is the organization associated with the data).", 0, 1, managingOrganization); 1182 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for a human to contact about the endpoint. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact); 1183 case -991726143: /*period*/ return new Property("period", "Period", "The interval during which the endpoint is expected to be operational.", 0, 1, period); 1184 case -786701938: /*payload*/ return new Property("payload", "", "The set of payloads that are provided/available at this endpoint.", 0, java.lang.Integer.MAX_VALUE, payload); 1185 case -1147692044: /*address*/ return new Property("address", "url", "The uri that describes the actual end-point to connect to.", 0, 1, address); 1186 case -1221270899: /*header*/ return new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header); 1187 default: return super.getNamedProperty(_hash, _name, _checkValid); 1188 } 1189 1190 } 1191 1192 @Override 1193 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1194 switch (hash) { 1195 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1196 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EndpointStatus> 1197 case 1270211384: /*connectionType*/ return this.connectionType == null ? new Base[0] : this.connectionType.toArray(new Base[this.connectionType.size()]); // CodeableConcept 1198 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1199 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1200 case 1680602093: /*environmentType*/ return this.environmentType == null ? new Base[0] : this.environmentType.toArray(new Base[this.environmentType.size()]); // CodeableConcept 1201 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 1202 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 1203 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1204 case -786701938: /*payload*/ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // EndpointPayloadComponent 1205 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // UrlType 1206 case -1221270899: /*header*/ return this.header == null ? new Base[0] : this.header.toArray(new Base[this.header.size()]); // StringType 1207 default: return super.getProperty(hash, name, checkValid); 1208 } 1209 1210 } 1211 1212 @Override 1213 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1214 switch (hash) { 1215 case -1618432855: // identifier 1216 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1217 return value; 1218 case -892481550: // status 1219 value = new EndpointStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1220 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 1221 return value; 1222 case 1270211384: // connectionType 1223 this.getConnectionType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1224 return value; 1225 case 3373707: // name 1226 this.name = TypeConvertor.castToString(value); // StringType 1227 return value; 1228 case -1724546052: // description 1229 this.description = TypeConvertor.castToString(value); // StringType 1230 return value; 1231 case 1680602093: // environmentType 1232 this.getEnvironmentType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1233 return value; 1234 case -2058947787: // managingOrganization 1235 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1236 return value; 1237 case 951526432: // contact 1238 this.getContact().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 1239 return value; 1240 case -991726143: // period 1241 this.period = TypeConvertor.castToPeriod(value); // Period 1242 return value; 1243 case -786701938: // payload 1244 this.getPayload().add((EndpointPayloadComponent) value); // EndpointPayloadComponent 1245 return value; 1246 case -1147692044: // address 1247 this.address = TypeConvertor.castToUrl(value); // UrlType 1248 return value; 1249 case -1221270899: // header 1250 this.getHeader().add(TypeConvertor.castToString(value)); // StringType 1251 return value; 1252 default: return super.setProperty(hash, name, value); 1253 } 1254 1255 } 1256 1257 @Override 1258 public Base setProperty(String name, Base value) throws FHIRException { 1259 if (name.equals("identifier")) { 1260 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1261 } else if (name.equals("status")) { 1262 value = new EndpointStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1263 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 1264 } else if (name.equals("connectionType")) { 1265 this.getConnectionType().add(TypeConvertor.castToCodeableConcept(value)); 1266 } else if (name.equals("name")) { 1267 this.name = TypeConvertor.castToString(value); // StringType 1268 } else if (name.equals("description")) { 1269 this.description = TypeConvertor.castToString(value); // StringType 1270 } else if (name.equals("environmentType")) { 1271 this.getEnvironmentType().add(TypeConvertor.castToCodeableConcept(value)); 1272 } else if (name.equals("managingOrganization")) { 1273 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1274 } else if (name.equals("contact")) { 1275 this.getContact().add(TypeConvertor.castToContactPoint(value)); 1276 } else if (name.equals("period")) { 1277 this.period = TypeConvertor.castToPeriod(value); // Period 1278 } else if (name.equals("payload")) { 1279 this.getPayload().add((EndpointPayloadComponent) value); 1280 } else if (name.equals("address")) { 1281 this.address = TypeConvertor.castToUrl(value); // UrlType 1282 } else if (name.equals("header")) { 1283 this.getHeader().add(TypeConvertor.castToString(value)); 1284 } else 1285 return super.setProperty(name, value); 1286 return value; 1287 } 1288 1289 @Override 1290 public void removeChild(String name, Base value) throws FHIRException { 1291 if (name.equals("identifier")) { 1292 this.getIdentifier().remove(value); 1293 } else if (name.equals("status")) { 1294 value = new EndpointStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1295 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 1296 } else if (name.equals("connectionType")) { 1297 this.getConnectionType().remove(value); 1298 } else if (name.equals("name")) { 1299 this.name = null; 1300 } else if (name.equals("description")) { 1301 this.description = null; 1302 } else if (name.equals("environmentType")) { 1303 this.getEnvironmentType().remove(value); 1304 } else if (name.equals("managingOrganization")) { 1305 this.managingOrganization = null; 1306 } else if (name.equals("contact")) { 1307 this.getContact().remove(value); 1308 } else if (name.equals("period")) { 1309 this.period = null; 1310 } else if (name.equals("payload")) { 1311 this.getPayload().remove((EndpointPayloadComponent) value); 1312 } else if (name.equals("address")) { 1313 this.address = null; 1314 } else if (name.equals("header")) { 1315 this.getHeader().remove(value); 1316 } else 1317 super.removeChild(name, value); 1318 1319 } 1320 1321 @Override 1322 public Base makeProperty(int hash, String name) throws FHIRException { 1323 switch (hash) { 1324 case -1618432855: return addIdentifier(); 1325 case -892481550: return getStatusElement(); 1326 case 1270211384: return addConnectionType(); 1327 case 3373707: return getNameElement(); 1328 case -1724546052: return getDescriptionElement(); 1329 case 1680602093: return addEnvironmentType(); 1330 case -2058947787: return getManagingOrganization(); 1331 case 951526432: return addContact(); 1332 case -991726143: return getPeriod(); 1333 case -786701938: return addPayload(); 1334 case -1147692044: return getAddressElement(); 1335 case -1221270899: return addHeaderElement(); 1336 default: return super.makeProperty(hash, name); 1337 } 1338 1339 } 1340 1341 @Override 1342 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1343 switch (hash) { 1344 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1345 case -892481550: /*status*/ return new String[] {"code"}; 1346 case 1270211384: /*connectionType*/ return new String[] {"CodeableConcept"}; 1347 case 3373707: /*name*/ return new String[] {"string"}; 1348 case -1724546052: /*description*/ return new String[] {"string"}; 1349 case 1680602093: /*environmentType*/ return new String[] {"CodeableConcept"}; 1350 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1351 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 1352 case -991726143: /*period*/ return new String[] {"Period"}; 1353 case -786701938: /*payload*/ return new String[] {}; 1354 case -1147692044: /*address*/ return new String[] {"url"}; 1355 case -1221270899: /*header*/ return new String[] {"string"}; 1356 default: return super.getTypesForProperty(hash, name); 1357 } 1358 1359 } 1360 1361 @Override 1362 public Base addChild(String name) throws FHIRException { 1363 if (name.equals("identifier")) { 1364 return addIdentifier(); 1365 } 1366 else if (name.equals("status")) { 1367 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.status"); 1368 } 1369 else if (name.equals("connectionType")) { 1370 return addConnectionType(); 1371 } 1372 else if (name.equals("name")) { 1373 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.name"); 1374 } 1375 else if (name.equals("description")) { 1376 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.description"); 1377 } 1378 else if (name.equals("environmentType")) { 1379 return addEnvironmentType(); 1380 } 1381 else if (name.equals("managingOrganization")) { 1382 this.managingOrganization = new Reference(); 1383 return this.managingOrganization; 1384 } 1385 else if (name.equals("contact")) { 1386 return addContact(); 1387 } 1388 else if (name.equals("period")) { 1389 this.period = new Period(); 1390 return this.period; 1391 } 1392 else if (name.equals("payload")) { 1393 return addPayload(); 1394 } 1395 else if (name.equals("address")) { 1396 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.address"); 1397 } 1398 else if (name.equals("header")) { 1399 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.header"); 1400 } 1401 else 1402 return super.addChild(name); 1403 } 1404 1405 public String fhirType() { 1406 return "Endpoint"; 1407 1408 } 1409 1410 public Endpoint copy() { 1411 Endpoint dst = new Endpoint(); 1412 copyValues(dst); 1413 return dst; 1414 } 1415 1416 public void copyValues(Endpoint dst) { 1417 super.copyValues(dst); 1418 if (identifier != null) { 1419 dst.identifier = new ArrayList<Identifier>(); 1420 for (Identifier i : identifier) 1421 dst.identifier.add(i.copy()); 1422 }; 1423 dst.status = status == null ? null : status.copy(); 1424 if (connectionType != null) { 1425 dst.connectionType = new ArrayList<CodeableConcept>(); 1426 for (CodeableConcept i : connectionType) 1427 dst.connectionType.add(i.copy()); 1428 }; 1429 dst.name = name == null ? null : name.copy(); 1430 dst.description = description == null ? null : description.copy(); 1431 if (environmentType != null) { 1432 dst.environmentType = new ArrayList<CodeableConcept>(); 1433 for (CodeableConcept i : environmentType) 1434 dst.environmentType.add(i.copy()); 1435 }; 1436 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1437 if (contact != null) { 1438 dst.contact = new ArrayList<ContactPoint>(); 1439 for (ContactPoint i : contact) 1440 dst.contact.add(i.copy()); 1441 }; 1442 dst.period = period == null ? null : period.copy(); 1443 if (payload != null) { 1444 dst.payload = new ArrayList<EndpointPayloadComponent>(); 1445 for (EndpointPayloadComponent i : payload) 1446 dst.payload.add(i.copy()); 1447 }; 1448 dst.address = address == null ? null : address.copy(); 1449 if (header != null) { 1450 dst.header = new ArrayList<StringType>(); 1451 for (StringType i : header) 1452 dst.header.add(i.copy()); 1453 }; 1454 } 1455 1456 protected Endpoint typedCopy() { 1457 return copy(); 1458 } 1459 1460 @Override 1461 public boolean equalsDeep(Base other_) { 1462 if (!super.equalsDeep(other_)) 1463 return false; 1464 if (!(other_ instanceof Endpoint)) 1465 return false; 1466 Endpoint o = (Endpoint) other_; 1467 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(connectionType, o.connectionType, true) 1468 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(environmentType, o.environmentType, true) 1469 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(contact, o.contact, true) 1470 && compareDeep(period, o.period, true) && compareDeep(payload, o.payload, true) && compareDeep(address, o.address, true) 1471 && compareDeep(header, o.header, true); 1472 } 1473 1474 @Override 1475 public boolean equalsShallow(Base other_) { 1476 if (!super.equalsShallow(other_)) 1477 return false; 1478 if (!(other_ instanceof Endpoint)) 1479 return false; 1480 Endpoint o = (Endpoint) other_; 1481 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 1482 && compareValues(address, o.address, true) && compareValues(header, o.header, true); 1483 } 1484 1485 public boolean isEmpty() { 1486 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, connectionType 1487 , name, description, environmentType, managingOrganization, contact, period, payload 1488 , address, header); 1489 } 1490 1491 @Override 1492 public ResourceType getResourceType() { 1493 return ResourceType.Endpoint; 1494 } 1495 1496 /** 1497 * Search parameter: <b>connection-type</b> 1498 * <p> 1499 * Description: <b>Protocol/Profile/Standard to be used with this endpoint connection</b><br> 1500 * Type: <b>token</b><br> 1501 * Path: <b>Endpoint.connectionType</b><br> 1502 * </p> 1503 */ 1504 @SearchParamDefinition(name="connection-type", path="Endpoint.connectionType", description="Protocol/Profile/Standard to be used with this endpoint connection", type="token" ) 1505 public static final String SP_CONNECTION_TYPE = "connection-type"; 1506 /** 1507 * <b>Fluent Client</b> search parameter constant for <b>connection-type</b> 1508 * <p> 1509 * Description: <b>Protocol/Profile/Standard to be used with this endpoint connection</b><br> 1510 * Type: <b>token</b><br> 1511 * Path: <b>Endpoint.connectionType</b><br> 1512 * </p> 1513 */ 1514 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONNECTION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONNECTION_TYPE); 1515 1516 /** 1517 * Search parameter: <b>identifier</b> 1518 * <p> 1519 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1520 * Type: <b>token</b><br> 1521 * Path: <b>Endpoint.identifier</b><br> 1522 * </p> 1523 */ 1524 @SearchParamDefinition(name="identifier", path="Endpoint.identifier", description="Identifies this endpoint across multiple systems", type="token" ) 1525 public static final String SP_IDENTIFIER = "identifier"; 1526 /** 1527 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1528 * <p> 1529 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1530 * Type: <b>token</b><br> 1531 * Path: <b>Endpoint.identifier</b><br> 1532 * </p> 1533 */ 1534 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1535 1536 /** 1537 * Search parameter: <b>name</b> 1538 * <p> 1539 * Description: <b>A name that this endpoint can be identified by</b><br> 1540 * Type: <b>string</b><br> 1541 * Path: <b>Endpoint.name</b><br> 1542 * </p> 1543 */ 1544 @SearchParamDefinition(name="name", path="Endpoint.name", description="A name that this endpoint can be identified by", type="string" ) 1545 public static final String SP_NAME = "name"; 1546 /** 1547 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1548 * <p> 1549 * Description: <b>A name that this endpoint can be identified by</b><br> 1550 * Type: <b>string</b><br> 1551 * Path: <b>Endpoint.name</b><br> 1552 * </p> 1553 */ 1554 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1555 1556 /** 1557 * Search parameter: <b>organization</b> 1558 * <p> 1559 * Description: <b>The organization that is managing the endpoint</b><br> 1560 * Type: <b>reference</b><br> 1561 * Path: <b>Endpoint.managingOrganization</b><br> 1562 * </p> 1563 */ 1564 @SearchParamDefinition(name="organization", path="Endpoint.managingOrganization", description="The organization that is managing the endpoint", type="reference", target={Organization.class } ) 1565 public static final String SP_ORGANIZATION = "organization"; 1566 /** 1567 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1568 * <p> 1569 * Description: <b>The organization that is managing the endpoint</b><br> 1570 * Type: <b>reference</b><br> 1571 * Path: <b>Endpoint.managingOrganization</b><br> 1572 * </p> 1573 */ 1574 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1575 1576/** 1577 * Constant for fluent queries to be used to add include statements. Specifies 1578 * the path value of "<b>Endpoint:organization</b>". 1579 */ 1580 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Endpoint:organization").toLocked(); 1581 1582 /** 1583 * Search parameter: <b>payload-type</b> 1584 * <p> 1585 * Description: <b>The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)</b><br> 1586 * Type: <b>token</b><br> 1587 * Path: <b>Endpoint.payload.type</b><br> 1588 * </p> 1589 */ 1590 @SearchParamDefinition(name="payload-type", path="Endpoint.payload.type", description="The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", type="token" ) 1591 public static final String SP_PAYLOAD_TYPE = "payload-type"; 1592 /** 1593 * <b>Fluent Client</b> search parameter constant for <b>payload-type</b> 1594 * <p> 1595 * Description: <b>The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)</b><br> 1596 * Type: <b>token</b><br> 1597 * Path: <b>Endpoint.payload.type</b><br> 1598 * </p> 1599 */ 1600 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYLOAD_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PAYLOAD_TYPE); 1601 1602 /** 1603 * Search parameter: <b>status</b> 1604 * <p> 1605 * Description: <b>The current status of the Endpoint (usually expected to be active)</b><br> 1606 * Type: <b>token</b><br> 1607 * Path: <b>Endpoint.status</b><br> 1608 * </p> 1609 */ 1610 @SearchParamDefinition(name="status", path="Endpoint.status", description="The current status of the Endpoint (usually expected to be active)", type="token" ) 1611 public static final String SP_STATUS = "status"; 1612 /** 1613 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1614 * <p> 1615 * Description: <b>The current status of the Endpoint (usually expected to be active)</b><br> 1616 * Type: <b>token</b><br> 1617 * Path: <b>Endpoint.status</b><br> 1618 * </p> 1619 */ 1620 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1621 1622 1623} 1624