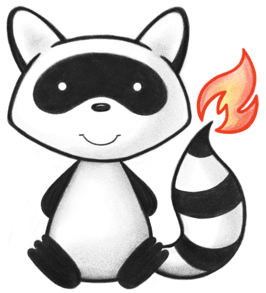
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 052 */ 053@ResourceDef(name="EnrollmentRequest", profile="http://hl7.org/fhir/StructureDefinition/EnrollmentRequest") 054public class EnrollmentRequest extends DomainResource { 055 056 /** 057 * The Response business identifier. 058 */ 059 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 060 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 061 protected List<Identifier> identifier; 062 063 /** 064 * The status of the resource instance. 065 */ 066 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 067 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 069 protected Enumeration<FinancialResourceStatusCodes> status; 070 071 /** 072 * The date when this resource was created. 073 */ 074 @Child(name = "created", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 075 @Description(shortDefinition="Creation date", formalDefinition="The date when this resource was created." ) 076 protected DateTimeType created; 077 078 /** 079 * The Insurer who is target of the request. 080 */ 081 @Child(name = "insurer", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 082 @Description(shortDefinition="Target", formalDefinition="The Insurer who is target of the request." ) 083 protected Reference insurer; 084 085 /** 086 * The practitioner who is responsible for the services rendered to the patient. 087 */ 088 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 089 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 090 protected Reference provider; 091 092 /** 093 * Patient Resource. 094 */ 095 @Child(name = "candidate", type = {Patient.class}, order=5, min=0, max=1, modifier=false, summary=false) 096 @Description(shortDefinition="The subject to be enrolled", formalDefinition="Patient Resource." ) 097 protected Reference candidate; 098 099 /** 100 * Reference to the program or plan identification, underwriter or payor. 101 */ 102 @Child(name = "coverage", type = {Coverage.class}, order=6, min=0, max=1, modifier=false, summary=false) 103 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the program or plan identification, underwriter or payor." ) 104 protected Reference coverage; 105 106 private static final long serialVersionUID = 1033959125L; 107 108 /** 109 * Constructor 110 */ 111 public EnrollmentRequest() { 112 super(); 113 } 114 115 /** 116 * @return {@link #identifier} (The Response business identifier.) 117 */ 118 public List<Identifier> getIdentifier() { 119 if (this.identifier == null) 120 this.identifier = new ArrayList<Identifier>(); 121 return this.identifier; 122 } 123 124 /** 125 * @return Returns a reference to <code>this</code> for easy method chaining 126 */ 127 public EnrollmentRequest setIdentifier(List<Identifier> theIdentifier) { 128 this.identifier = theIdentifier; 129 return this; 130 } 131 132 public boolean hasIdentifier() { 133 if (this.identifier == null) 134 return false; 135 for (Identifier item : this.identifier) 136 if (!item.isEmpty()) 137 return true; 138 return false; 139 } 140 141 public Identifier addIdentifier() { //3 142 Identifier t = new Identifier(); 143 if (this.identifier == null) 144 this.identifier = new ArrayList<Identifier>(); 145 this.identifier.add(t); 146 return t; 147 } 148 149 public EnrollmentRequest addIdentifier(Identifier t) { //3 150 if (t == null) 151 return this; 152 if (this.identifier == null) 153 this.identifier = new ArrayList<Identifier>(); 154 this.identifier.add(t); 155 return this; 156 } 157 158 /** 159 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 160 */ 161 public Identifier getIdentifierFirstRep() { 162 if (getIdentifier().isEmpty()) { 163 addIdentifier(); 164 } 165 return getIdentifier().get(0); 166 } 167 168 /** 169 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 170 */ 171 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 172 if (this.status == null) 173 if (Configuration.errorOnAutoCreate()) 174 throw new Error("Attempt to auto-create EnrollmentRequest.status"); 175 else if (Configuration.doAutoCreate()) 176 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 177 return this.status; 178 } 179 180 public boolean hasStatusElement() { 181 return this.status != null && !this.status.isEmpty(); 182 } 183 184 public boolean hasStatus() { 185 return this.status != null && !this.status.isEmpty(); 186 } 187 188 /** 189 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 190 */ 191 public EnrollmentRequest setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 192 this.status = value; 193 return this; 194 } 195 196 /** 197 * @return The status of the resource instance. 198 */ 199 public FinancialResourceStatusCodes getStatus() { 200 return this.status == null ? null : this.status.getValue(); 201 } 202 203 /** 204 * @param value The status of the resource instance. 205 */ 206 public EnrollmentRequest setStatus(FinancialResourceStatusCodes value) { 207 if (value == null) 208 this.status = null; 209 else { 210 if (this.status == null) 211 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 212 this.status.setValue(value); 213 } 214 return this; 215 } 216 217 /** 218 * @return {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 219 */ 220 public DateTimeType getCreatedElement() { 221 if (this.created == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create EnrollmentRequest.created"); 224 else if (Configuration.doAutoCreate()) 225 this.created = new DateTimeType(); // bb 226 return this.created; 227 } 228 229 public boolean hasCreatedElement() { 230 return this.created != null && !this.created.isEmpty(); 231 } 232 233 public boolean hasCreated() { 234 return this.created != null && !this.created.isEmpty(); 235 } 236 237 /** 238 * @param value {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 239 */ 240 public EnrollmentRequest setCreatedElement(DateTimeType value) { 241 this.created = value; 242 return this; 243 } 244 245 /** 246 * @return The date when this resource was created. 247 */ 248 public Date getCreated() { 249 return this.created == null ? null : this.created.getValue(); 250 } 251 252 /** 253 * @param value The date when this resource was created. 254 */ 255 public EnrollmentRequest setCreated(Date value) { 256 if (value == null) 257 this.created = null; 258 else { 259 if (this.created == null) 260 this.created = new DateTimeType(); 261 this.created.setValue(value); 262 } 263 return this; 264 } 265 266 /** 267 * @return {@link #insurer} (The Insurer who is target of the request.) 268 */ 269 public Reference getInsurer() { 270 if (this.insurer == null) 271 if (Configuration.errorOnAutoCreate()) 272 throw new Error("Attempt to auto-create EnrollmentRequest.insurer"); 273 else if (Configuration.doAutoCreate()) 274 this.insurer = new Reference(); // cc 275 return this.insurer; 276 } 277 278 public boolean hasInsurer() { 279 return this.insurer != null && !this.insurer.isEmpty(); 280 } 281 282 /** 283 * @param value {@link #insurer} (The Insurer who is target of the request.) 284 */ 285 public EnrollmentRequest setInsurer(Reference value) { 286 this.insurer = value; 287 return this; 288 } 289 290 /** 291 * @return {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 292 */ 293 public Reference getProvider() { 294 if (this.provider == null) 295 if (Configuration.errorOnAutoCreate()) 296 throw new Error("Attempt to auto-create EnrollmentRequest.provider"); 297 else if (Configuration.doAutoCreate()) 298 this.provider = new Reference(); // cc 299 return this.provider; 300 } 301 302 public boolean hasProvider() { 303 return this.provider != null && !this.provider.isEmpty(); 304 } 305 306 /** 307 * @param value {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 308 */ 309 public EnrollmentRequest setProvider(Reference value) { 310 this.provider = value; 311 return this; 312 } 313 314 /** 315 * @return {@link #candidate} (Patient Resource.) 316 */ 317 public Reference getCandidate() { 318 if (this.candidate == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create EnrollmentRequest.candidate"); 321 else if (Configuration.doAutoCreate()) 322 this.candidate = new Reference(); // cc 323 return this.candidate; 324 } 325 326 public boolean hasCandidate() { 327 return this.candidate != null && !this.candidate.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #candidate} (Patient Resource.) 332 */ 333 public EnrollmentRequest setCandidate(Reference value) { 334 this.candidate = value; 335 return this; 336 } 337 338 /** 339 * @return {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 340 */ 341 public Reference getCoverage() { 342 if (this.coverage == null) 343 if (Configuration.errorOnAutoCreate()) 344 throw new Error("Attempt to auto-create EnrollmentRequest.coverage"); 345 else if (Configuration.doAutoCreate()) 346 this.coverage = new Reference(); // cc 347 return this.coverage; 348 } 349 350 public boolean hasCoverage() { 351 return this.coverage != null && !this.coverage.isEmpty(); 352 } 353 354 /** 355 * @param value {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 356 */ 357 public EnrollmentRequest setCoverage(Reference value) { 358 this.coverage = value; 359 return this; 360 } 361 362 protected void listChildren(List<Property> children) { 363 super.listChildren(children); 364 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 365 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 366 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 367 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 368 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider)); 369 children.add(new Property("candidate", "Reference(Patient)", "Patient Resource.", 0, 1, candidate)); 370 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 371 } 372 373 @Override 374 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 375 switch (_hash) { 376 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 377 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 378 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created); 379 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer); 380 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider); 381 case 508663171: /*candidate*/ return new Property("candidate", "Reference(Patient)", "Patient Resource.", 0, 1, candidate); 382 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 383 default: return super.getNamedProperty(_hash, _name, _checkValid); 384 } 385 386 } 387 388 @Override 389 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 390 switch (hash) { 391 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 392 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 393 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 394 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 395 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 396 case 508663171: /*candidate*/ return this.candidate == null ? new Base[0] : new Base[] {this.candidate}; // Reference 397 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 398 default: return super.getProperty(hash, name, checkValid); 399 } 400 401 } 402 403 @Override 404 public Base setProperty(int hash, String name, Base value) throws FHIRException { 405 switch (hash) { 406 case -1618432855: // identifier 407 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 408 return value; 409 case -892481550: // status 410 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 411 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 412 return value; 413 case 1028554472: // created 414 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 415 return value; 416 case 1957615864: // insurer 417 this.insurer = TypeConvertor.castToReference(value); // Reference 418 return value; 419 case -987494927: // provider 420 this.provider = TypeConvertor.castToReference(value); // Reference 421 return value; 422 case 508663171: // candidate 423 this.candidate = TypeConvertor.castToReference(value); // Reference 424 return value; 425 case -351767064: // coverage 426 this.coverage = TypeConvertor.castToReference(value); // Reference 427 return value; 428 default: return super.setProperty(hash, name, value); 429 } 430 431 } 432 433 @Override 434 public Base setProperty(String name, Base value) throws FHIRException { 435 if (name.equals("identifier")) { 436 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 437 } else if (name.equals("status")) { 438 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 439 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 440 } else if (name.equals("created")) { 441 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 442 } else if (name.equals("insurer")) { 443 this.insurer = TypeConvertor.castToReference(value); // Reference 444 } else if (name.equals("provider")) { 445 this.provider = TypeConvertor.castToReference(value); // Reference 446 } else if (name.equals("candidate")) { 447 this.candidate = TypeConvertor.castToReference(value); // Reference 448 } else if (name.equals("coverage")) { 449 this.coverage = TypeConvertor.castToReference(value); // Reference 450 } else 451 return super.setProperty(name, value); 452 return value; 453 } 454 455 @Override 456 public Base makeProperty(int hash, String name) throws FHIRException { 457 switch (hash) { 458 case -1618432855: return addIdentifier(); 459 case -892481550: return getStatusElement(); 460 case 1028554472: return getCreatedElement(); 461 case 1957615864: return getInsurer(); 462 case -987494927: return getProvider(); 463 case 508663171: return getCandidate(); 464 case -351767064: return getCoverage(); 465 default: return super.makeProperty(hash, name); 466 } 467 468 } 469 470 @Override 471 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 472 switch (hash) { 473 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 474 case -892481550: /*status*/ return new String[] {"code"}; 475 case 1028554472: /*created*/ return new String[] {"dateTime"}; 476 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 477 case -987494927: /*provider*/ return new String[] {"Reference"}; 478 case 508663171: /*candidate*/ return new String[] {"Reference"}; 479 case -351767064: /*coverage*/ return new String[] {"Reference"}; 480 default: return super.getTypesForProperty(hash, name); 481 } 482 483 } 484 485 @Override 486 public Base addChild(String name) throws FHIRException { 487 if (name.equals("identifier")) { 488 return addIdentifier(); 489 } 490 else if (name.equals("status")) { 491 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentRequest.status"); 492 } 493 else if (name.equals("created")) { 494 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentRequest.created"); 495 } 496 else if (name.equals("insurer")) { 497 this.insurer = new Reference(); 498 return this.insurer; 499 } 500 else if (name.equals("provider")) { 501 this.provider = new Reference(); 502 return this.provider; 503 } 504 else if (name.equals("candidate")) { 505 this.candidate = new Reference(); 506 return this.candidate; 507 } 508 else if (name.equals("coverage")) { 509 this.coverage = new Reference(); 510 return this.coverage; 511 } 512 else 513 return super.addChild(name); 514 } 515 516 public String fhirType() { 517 return "EnrollmentRequest"; 518 519 } 520 521 public EnrollmentRequest copy() { 522 EnrollmentRequest dst = new EnrollmentRequest(); 523 copyValues(dst); 524 return dst; 525 } 526 527 public void copyValues(EnrollmentRequest dst) { 528 super.copyValues(dst); 529 if (identifier != null) { 530 dst.identifier = new ArrayList<Identifier>(); 531 for (Identifier i : identifier) 532 dst.identifier.add(i.copy()); 533 }; 534 dst.status = status == null ? null : status.copy(); 535 dst.created = created == null ? null : created.copy(); 536 dst.insurer = insurer == null ? null : insurer.copy(); 537 dst.provider = provider == null ? null : provider.copy(); 538 dst.candidate = candidate == null ? null : candidate.copy(); 539 dst.coverage = coverage == null ? null : coverage.copy(); 540 } 541 542 protected EnrollmentRequest typedCopy() { 543 return copy(); 544 } 545 546 @Override 547 public boolean equalsDeep(Base other_) { 548 if (!super.equalsDeep(other_)) 549 return false; 550 if (!(other_ instanceof EnrollmentRequest)) 551 return false; 552 EnrollmentRequest o = (EnrollmentRequest) other_; 553 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(created, o.created, true) 554 && compareDeep(insurer, o.insurer, true) && compareDeep(provider, o.provider, true) && compareDeep(candidate, o.candidate, true) 555 && compareDeep(coverage, o.coverage, true); 556 } 557 558 @Override 559 public boolean equalsShallow(Base other_) { 560 if (!super.equalsShallow(other_)) 561 return false; 562 if (!(other_ instanceof EnrollmentRequest)) 563 return false; 564 EnrollmentRequest o = (EnrollmentRequest) other_; 565 return compareValues(status, o.status, true) && compareValues(created, o.created, true); 566 } 567 568 public boolean isEmpty() { 569 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created 570 , insurer, provider, candidate, coverage); 571 } 572 573 @Override 574 public ResourceType getResourceType() { 575 return ResourceType.EnrollmentRequest; 576 } 577 578 /** 579 * Search parameter: <b>status</b> 580 * <p> 581 * Description: <b>The status of the enrollment</b><br> 582 * Type: <b>token</b><br> 583 * Path: <b>EnrollmentRequest.status</b><br> 584 * </p> 585 */ 586 @SearchParamDefinition(name="status", path="EnrollmentRequest.status", description="The status of the enrollment", type="token" ) 587 public static final String SP_STATUS = "status"; 588 /** 589 * <b>Fluent Client</b> search parameter constant for <b>status</b> 590 * <p> 591 * Description: <b>The status of the enrollment</b><br> 592 * Type: <b>token</b><br> 593 * Path: <b>EnrollmentRequest.status</b><br> 594 * </p> 595 */ 596 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 597 598 /** 599 * Search parameter: <b>subject</b> 600 * <p> 601 * Description: <b>The party to be enrolled</b><br> 602 * Type: <b>reference</b><br> 603 * Path: <b>EnrollmentRequest.candidate</b><br> 604 * </p> 605 */ 606 @SearchParamDefinition(name="subject", path="EnrollmentRequest.candidate", description="The party to be enrolled", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 607 public static final String SP_SUBJECT = "subject"; 608 /** 609 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 610 * <p> 611 * Description: <b>The party to be enrolled</b><br> 612 * Type: <b>reference</b><br> 613 * Path: <b>EnrollmentRequest.candidate</b><br> 614 * </p> 615 */ 616 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 617 618/** 619 * Constant for fluent queries to be used to add include statements. Specifies 620 * the path value of "<b>EnrollmentRequest:subject</b>". 621 */ 622 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("EnrollmentRequest:subject").toLocked(); 623 624 /** 625 * Search parameter: <b>identifier</b> 626 * <p> 627 * Description: <b>Multiple Resources: 628 629* [Account](account.html): Account number 630* [AdverseEvent](adverseevent.html): Business identifier for the event 631* [AllergyIntolerance](allergyintolerance.html): External ids for this item 632* [Appointment](appointment.html): An Identifier of the Appointment 633* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 634* [Basic](basic.html): Business identifier 635* [BodyStructure](bodystructure.html): Bodystructure identifier 636* [CarePlan](careplan.html): External Ids for this plan 637* [CareTeam](careteam.html): External Ids for this team 638* [ChargeItem](chargeitem.html): Business Identifier for item 639* [Claim](claim.html): The primary identifier of the financial resource 640* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 641* [ClinicalImpression](clinicalimpression.html): Business identifier 642* [Communication](communication.html): Unique identifier 643* [CommunicationRequest](communicationrequest.html): Unique identifier 644* [Composition](composition.html): Version-independent identifier for the Composition 645* [Condition](condition.html): A unique identifier of the condition record 646* [Consent](consent.html): Identifier for this record (external references) 647* [Contract](contract.html): The identity of the contract 648* [Coverage](coverage.html): The primary identifier of the insured and the coverage 649* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 650* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 651* [DetectedIssue](detectedissue.html): Unique id for the detected issue 652* [DeviceRequest](devicerequest.html): Business identifier for request/order 653* [DeviceUsage](deviceusage.html): Search by identifier 654* [DiagnosticReport](diagnosticreport.html): An identifier for the report 655* [DocumentReference](documentreference.html): Identifier of the attachment binary 656* [Encounter](encounter.html): Identifier(s) by which this encounter is known 657* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 658* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 659* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 660* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 661* [Flag](flag.html): Business identifier 662* [Goal](goal.html): External Ids for this goal 663* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 664* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 665* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 666* [Immunization](immunization.html): Business identifier 667* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 668* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 669* [Invoice](invoice.html): Business Identifier for item 670* [List](list.html): Business identifier 671* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 672* [Medication](medication.html): Returns medications with this external identifier 673* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 674* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 675* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 676* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 677* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 678* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 679* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 680* [Observation](observation.html): The unique id for a particular observation 681* [Person](person.html): A person Identifier 682* [Procedure](procedure.html): A unique identifier for a procedure 683* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 684* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 685* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 686* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 687* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 688* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 689* [Specimen](specimen.html): The unique identifier associated with the specimen 690* [SupplyDelivery](supplydelivery.html): External identifier 691* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 692* [Task](task.html): Search for a task instance by its business identifier 693* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 694</b><br> 695 * Type: <b>token</b><br> 696 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 697 * </p> 698 */ 699 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 700 public static final String SP_IDENTIFIER = "identifier"; 701 /** 702 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 703 * <p> 704 * Description: <b>Multiple Resources: 705 706* [Account](account.html): Account number 707* [AdverseEvent](adverseevent.html): Business identifier for the event 708* [AllergyIntolerance](allergyintolerance.html): External ids for this item 709* [Appointment](appointment.html): An Identifier of the Appointment 710* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 711* [Basic](basic.html): Business identifier 712* [BodyStructure](bodystructure.html): Bodystructure identifier 713* [CarePlan](careplan.html): External Ids for this plan 714* [CareTeam](careteam.html): External Ids for this team 715* [ChargeItem](chargeitem.html): Business Identifier for item 716* [Claim](claim.html): The primary identifier of the financial resource 717* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 718* [ClinicalImpression](clinicalimpression.html): Business identifier 719* [Communication](communication.html): Unique identifier 720* [CommunicationRequest](communicationrequest.html): Unique identifier 721* [Composition](composition.html): Version-independent identifier for the Composition 722* [Condition](condition.html): A unique identifier of the condition record 723* [Consent](consent.html): Identifier for this record (external references) 724* [Contract](contract.html): The identity of the contract 725* [Coverage](coverage.html): The primary identifier of the insured and the coverage 726* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 727* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 728* [DetectedIssue](detectedissue.html): Unique id for the detected issue 729* [DeviceRequest](devicerequest.html): Business identifier for request/order 730* [DeviceUsage](deviceusage.html): Search by identifier 731* [DiagnosticReport](diagnosticreport.html): An identifier for the report 732* [DocumentReference](documentreference.html): Identifier of the attachment binary 733* [Encounter](encounter.html): Identifier(s) by which this encounter is known 734* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 735* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 736* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 737* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 738* [Flag](flag.html): Business identifier 739* [Goal](goal.html): External Ids for this goal 740* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 741* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 742* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 743* [Immunization](immunization.html): Business identifier 744* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 745* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 746* [Invoice](invoice.html): Business Identifier for item 747* [List](list.html): Business identifier 748* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 749* [Medication](medication.html): Returns medications with this external identifier 750* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 751* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 752* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 753* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 754* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 755* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 756* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 757* [Observation](observation.html): The unique id for a particular observation 758* [Person](person.html): A person Identifier 759* [Procedure](procedure.html): A unique identifier for a procedure 760* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 761* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 762* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 763* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 764* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 765* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 766* [Specimen](specimen.html): The unique identifier associated with the specimen 767* [SupplyDelivery](supplydelivery.html): External identifier 768* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 769* [Task](task.html): Search for a task instance by its business identifier 770* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 771</b><br> 772 * Type: <b>token</b><br> 773 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 774 * </p> 775 */ 776 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 777 778 /** 779 * Search parameter: <b>patient</b> 780 * <p> 781 * Description: <b>Multiple Resources: 782 783* [Account](account.html): The entity that caused the expenses 784* [AdverseEvent](adverseevent.html): Subject impacted by event 785* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 786* [Appointment](appointment.html): One of the individuals of the appointment is this patient 787* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 788* [AuditEvent](auditevent.html): Where the activity involved patient data 789* [Basic](basic.html): Identifies the focus of this resource 790* [BodyStructure](bodystructure.html): Who this is about 791* [CarePlan](careplan.html): Who the care plan is for 792* [CareTeam](careteam.html): Who care team is for 793* [ChargeItem](chargeitem.html): Individual service was done for/to 794* [Claim](claim.html): Patient receiving the products or services 795* [ClaimResponse](claimresponse.html): The subject of care 796* [ClinicalImpression](clinicalimpression.html): Patient assessed 797* [Communication](communication.html): Focus of message 798* [CommunicationRequest](communicationrequest.html): Focus of message 799* [Composition](composition.html): Who and/or what the composition is about 800* [Condition](condition.html): Who has the condition? 801* [Consent](consent.html): Who the consent applies to 802* [Contract](contract.html): The identity of the subject of the contract (if a patient) 803* [Coverage](coverage.html): Retrieve coverages for a patient 804* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 805* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 806* [DetectedIssue](detectedissue.html): Associated patient 807* [DeviceRequest](devicerequest.html): Individual the service is ordered for 808* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 809* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 810* [DocumentReference](documentreference.html): Who/what is the subject of the document 811* [Encounter](encounter.html): The patient present at the encounter 812* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 813* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 814* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 815* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 816* [Flag](flag.html): The identity of a subject to list flags for 817* [Goal](goal.html): Who this goal is intended for 818* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 819* [ImagingSelection](imagingselection.html): Who the study is about 820* [ImagingStudy](imagingstudy.html): Who the study is about 821* [Immunization](immunization.html): The patient for the vaccination record 822* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 823* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 824* [Invoice](invoice.html): Recipient(s) of goods and services 825* [List](list.html): If all resources have the same subject 826* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 827* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 828* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 829* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 830* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 831* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 832* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 833* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 834* [Observation](observation.html): The subject that the observation is about (if patient) 835* [Person](person.html): The Person links to this Patient 836* [Procedure](procedure.html): Search by subject - a patient 837* [Provenance](provenance.html): Where the activity involved patient data 838* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 839* [RelatedPerson](relatedperson.html): The patient this related person is related to 840* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 841* [ResearchSubject](researchsubject.html): Who or what is part of study 842* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 843* [ServiceRequest](servicerequest.html): Search by subject - a patient 844* [Specimen](specimen.html): The patient the specimen comes from 845* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 846* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 847* [Task](task.html): Search by patient 848* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 849</b><br> 850 * Type: <b>reference</b><br> 851 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 852 * </p> 853 */ 854 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 855 public static final String SP_PATIENT = "patient"; 856 /** 857 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 858 * <p> 859 * Description: <b>Multiple Resources: 860 861* [Account](account.html): The entity that caused the expenses 862* [AdverseEvent](adverseevent.html): Subject impacted by event 863* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 864* [Appointment](appointment.html): One of the individuals of the appointment is this patient 865* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 866* [AuditEvent](auditevent.html): Where the activity involved patient data 867* [Basic](basic.html): Identifies the focus of this resource 868* [BodyStructure](bodystructure.html): Who this is about 869* [CarePlan](careplan.html): Who the care plan is for 870* [CareTeam](careteam.html): Who care team is for 871* [ChargeItem](chargeitem.html): Individual service was done for/to 872* [Claim](claim.html): Patient receiving the products or services 873* [ClaimResponse](claimresponse.html): The subject of care 874* [ClinicalImpression](clinicalimpression.html): Patient assessed 875* [Communication](communication.html): Focus of message 876* [CommunicationRequest](communicationrequest.html): Focus of message 877* [Composition](composition.html): Who and/or what the composition is about 878* [Condition](condition.html): Who has the condition? 879* [Consent](consent.html): Who the consent applies to 880* [Contract](contract.html): The identity of the subject of the contract (if a patient) 881* [Coverage](coverage.html): Retrieve coverages for a patient 882* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 883* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 884* [DetectedIssue](detectedissue.html): Associated patient 885* [DeviceRequest](devicerequest.html): Individual the service is ordered for 886* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 887* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 888* [DocumentReference](documentreference.html): Who/what is the subject of the document 889* [Encounter](encounter.html): The patient present at the encounter 890* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 891* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 892* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 893* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 894* [Flag](flag.html): The identity of a subject to list flags for 895* [Goal](goal.html): Who this goal is intended for 896* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 897* [ImagingSelection](imagingselection.html): Who the study is about 898* [ImagingStudy](imagingstudy.html): Who the study is about 899* [Immunization](immunization.html): The patient for the vaccination record 900* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 901* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 902* [Invoice](invoice.html): Recipient(s) of goods and services 903* [List](list.html): If all resources have the same subject 904* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 905* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 906* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 907* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 908* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 909* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 910* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 911* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 912* [Observation](observation.html): The subject that the observation is about (if patient) 913* [Person](person.html): The Person links to this Patient 914* [Procedure](procedure.html): Search by subject - a patient 915* [Provenance](provenance.html): Where the activity involved patient data 916* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 917* [RelatedPerson](relatedperson.html): The patient this related person is related to 918* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 919* [ResearchSubject](researchsubject.html): Who or what is part of study 920* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 921* [ServiceRequest](servicerequest.html): Search by subject - a patient 922* [Specimen](specimen.html): The patient the specimen comes from 923* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 924* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 925* [Task](task.html): Search by patient 926* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 927</b><br> 928 * Type: <b>reference</b><br> 929 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 930 * </p> 931 */ 932 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 933 934/** 935 * Constant for fluent queries to be used to add include statements. Specifies 936 * the path value of "<b>EnrollmentRequest:patient</b>". 937 */ 938 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("EnrollmentRequest:patient").toLocked(); 939 940 941} 942