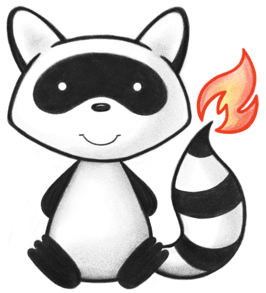
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource. 052 */ 053@ResourceDef(name="EnrollmentResponse", profile="http://hl7.org/fhir/StructureDefinition/EnrollmentResponse") 054public class EnrollmentResponse extends DomainResource { 055 056 public enum EnrollmentOutcome { 057 /** 058 * The Claim/Pre-authorization/Pre-determination has been received but processing has not begun. 059 */ 060 QUEUED, 061 /** 062 * The processing has completed without errors 063 */ 064 COMPLETE, 065 /** 066 * One or more errors have been detected in the Claim 067 */ 068 ERROR, 069 /** 070 * No errors have been detected in the Claim and some of the adjudication has been performed. 071 */ 072 PARTIAL, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static EnrollmentOutcome fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("queued".equals(codeString)) 081 return QUEUED; 082 if ("complete".equals(codeString)) 083 return COMPLETE; 084 if ("error".equals(codeString)) 085 return ERROR; 086 if ("partial".equals(codeString)) 087 return PARTIAL; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown EnrollmentOutcome code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case QUEUED: return "queued"; 096 case COMPLETE: return "complete"; 097 case ERROR: return "error"; 098 case PARTIAL: return "partial"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case QUEUED: return "http://hl7.org/fhir/enrollment-outcome"; 106 case COMPLETE: return "http://hl7.org/fhir/enrollment-outcome"; 107 case ERROR: return "http://hl7.org/fhir/enrollment-outcome"; 108 case PARTIAL: return "http://hl7.org/fhir/enrollment-outcome"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case QUEUED: return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 116 case COMPLETE: return "The processing has completed without errors"; 117 case ERROR: return "One or more errors have been detected in the Claim"; 118 case PARTIAL: return "No errors have been detected in the Claim and some of the adjudication has been performed."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case QUEUED: return "Queued"; 126 case COMPLETE: return "Processing Complete"; 127 case ERROR: return "Error"; 128 case PARTIAL: return "Partial Processing"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class EnrollmentOutcomeEnumFactory implements EnumFactory<EnrollmentOutcome> { 136 public EnrollmentOutcome fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("queued".equals(codeString)) 141 return EnrollmentOutcome.QUEUED; 142 if ("complete".equals(codeString)) 143 return EnrollmentOutcome.COMPLETE; 144 if ("error".equals(codeString)) 145 return EnrollmentOutcome.ERROR; 146 if ("partial".equals(codeString)) 147 return EnrollmentOutcome.PARTIAL; 148 throw new IllegalArgumentException("Unknown EnrollmentOutcome code '"+codeString+"'"); 149 } 150 public Enumeration<EnrollmentOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<EnrollmentOutcome>(this, EnrollmentOutcome.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<EnrollmentOutcome>(this, EnrollmentOutcome.NULL, code); 158 if ("queued".equals(codeString)) 159 return new Enumeration<EnrollmentOutcome>(this, EnrollmentOutcome.QUEUED, code); 160 if ("complete".equals(codeString)) 161 return new Enumeration<EnrollmentOutcome>(this, EnrollmentOutcome.COMPLETE, code); 162 if ("error".equals(codeString)) 163 return new Enumeration<EnrollmentOutcome>(this, EnrollmentOutcome.ERROR, code); 164 if ("partial".equals(codeString)) 165 return new Enumeration<EnrollmentOutcome>(this, EnrollmentOutcome.PARTIAL, code); 166 throw new FHIRException("Unknown EnrollmentOutcome code '"+codeString+"'"); 167 } 168 public String toCode(EnrollmentOutcome code) { 169 if (code == EnrollmentOutcome.NULL) 170 return null; 171 if (code == EnrollmentOutcome.QUEUED) 172 return "queued"; 173 if (code == EnrollmentOutcome.COMPLETE) 174 return "complete"; 175 if (code == EnrollmentOutcome.ERROR) 176 return "error"; 177 if (code == EnrollmentOutcome.PARTIAL) 178 return "partial"; 179 return "?"; 180 } 181 public String toSystem(EnrollmentOutcome code) { 182 return code.getSystem(); 183 } 184 } 185 186 /** 187 * The Response business identifier. 188 */ 189 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 190 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 191 protected List<Identifier> identifier; 192 193 /** 194 * The status of the resource instance. 195 */ 196 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 197 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 198 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 199 protected Enumeration<FinancialResourceStatusCodes> status; 200 201 /** 202 * Original request resource reference. 203 */ 204 @Child(name = "request", type = {EnrollmentRequest.class}, order=2, min=0, max=1, modifier=false, summary=false) 205 @Description(shortDefinition="Claim reference", formalDefinition="Original request resource reference." ) 206 protected Reference request; 207 208 /** 209 * Processing status: error, complete. 210 */ 211 @Child(name = "outcome", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 212 @Description(shortDefinition="queued | complete | error | partial", formalDefinition="Processing status: error, complete." ) 213 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/enrollment-outcome") 214 protected Enumeration<EnrollmentOutcome> outcome; 215 216 /** 217 * A description of the status of the adjudication. 218 */ 219 @Child(name = "disposition", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 220 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication." ) 221 protected StringType disposition; 222 223 /** 224 * The date when the enclosed suite of services were performed or completed. 225 */ 226 @Child(name = "created", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 227 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 228 protected DateTimeType created; 229 230 /** 231 * The Insurer who produced this adjudicated response. 232 */ 233 @Child(name = "organization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 234 @Description(shortDefinition="Insurer", formalDefinition="The Insurer who produced this adjudicated response." ) 235 protected Reference organization; 236 237 /** 238 * The practitioner who is responsible for the services rendered to the patient. 239 */ 240 @Child(name = "requestProvider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=7, min=0, max=1, modifier=false, summary=false) 241 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 242 protected Reference requestProvider; 243 244 private static final long serialVersionUID = -1503790623L; 245 246 /** 247 * Constructor 248 */ 249 public EnrollmentResponse() { 250 super(); 251 } 252 253 /** 254 * @return {@link #identifier} (The Response business identifier.) 255 */ 256 public List<Identifier> getIdentifier() { 257 if (this.identifier == null) 258 this.identifier = new ArrayList<Identifier>(); 259 return this.identifier; 260 } 261 262 /** 263 * @return Returns a reference to <code>this</code> for easy method chaining 264 */ 265 public EnrollmentResponse setIdentifier(List<Identifier> theIdentifier) { 266 this.identifier = theIdentifier; 267 return this; 268 } 269 270 public boolean hasIdentifier() { 271 if (this.identifier == null) 272 return false; 273 for (Identifier item : this.identifier) 274 if (!item.isEmpty()) 275 return true; 276 return false; 277 } 278 279 public Identifier addIdentifier() { //3 280 Identifier t = new Identifier(); 281 if (this.identifier == null) 282 this.identifier = new ArrayList<Identifier>(); 283 this.identifier.add(t); 284 return t; 285 } 286 287 public EnrollmentResponse addIdentifier(Identifier t) { //3 288 if (t == null) 289 return this; 290 if (this.identifier == null) 291 this.identifier = new ArrayList<Identifier>(); 292 this.identifier.add(t); 293 return this; 294 } 295 296 /** 297 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 298 */ 299 public Identifier getIdentifierFirstRep() { 300 if (getIdentifier().isEmpty()) { 301 addIdentifier(); 302 } 303 return getIdentifier().get(0); 304 } 305 306 /** 307 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 308 */ 309 public Enumeration<FinancialResourceStatusCodes> getStatusElement() { 310 if (this.status == null) 311 if (Configuration.errorOnAutoCreate()) 312 throw new Error("Attempt to auto-create EnrollmentResponse.status"); 313 else if (Configuration.doAutoCreate()) 314 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); // bb 315 return this.status; 316 } 317 318 public boolean hasStatusElement() { 319 return this.status != null && !this.status.isEmpty(); 320 } 321 322 public boolean hasStatus() { 323 return this.status != null && !this.status.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 328 */ 329 public EnrollmentResponse setStatusElement(Enumeration<FinancialResourceStatusCodes> value) { 330 this.status = value; 331 return this; 332 } 333 334 /** 335 * @return The status of the resource instance. 336 */ 337 public FinancialResourceStatusCodes getStatus() { 338 return this.status == null ? null : this.status.getValue(); 339 } 340 341 /** 342 * @param value The status of the resource instance. 343 */ 344 public EnrollmentResponse setStatus(FinancialResourceStatusCodes value) { 345 if (value == null) 346 this.status = null; 347 else { 348 if (this.status == null) 349 this.status = new Enumeration<FinancialResourceStatusCodes>(new FinancialResourceStatusCodesEnumFactory()); 350 this.status.setValue(value); 351 } 352 return this; 353 } 354 355 /** 356 * @return {@link #request} (Original request resource reference.) 357 */ 358 public Reference getRequest() { 359 if (this.request == null) 360 if (Configuration.errorOnAutoCreate()) 361 throw new Error("Attempt to auto-create EnrollmentResponse.request"); 362 else if (Configuration.doAutoCreate()) 363 this.request = new Reference(); // cc 364 return this.request; 365 } 366 367 public boolean hasRequest() { 368 return this.request != null && !this.request.isEmpty(); 369 } 370 371 /** 372 * @param value {@link #request} (Original request resource reference.) 373 */ 374 public EnrollmentResponse setRequest(Reference value) { 375 this.request = value; 376 return this; 377 } 378 379 /** 380 * @return {@link #outcome} (Processing status: error, complete.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 381 */ 382 public Enumeration<EnrollmentOutcome> getOutcomeElement() { 383 if (this.outcome == null) 384 if (Configuration.errorOnAutoCreate()) 385 throw new Error("Attempt to auto-create EnrollmentResponse.outcome"); 386 else if (Configuration.doAutoCreate()) 387 this.outcome = new Enumeration<EnrollmentOutcome>(new EnrollmentOutcomeEnumFactory()); // bb 388 return this.outcome; 389 } 390 391 public boolean hasOutcomeElement() { 392 return this.outcome != null && !this.outcome.isEmpty(); 393 } 394 395 public boolean hasOutcome() { 396 return this.outcome != null && !this.outcome.isEmpty(); 397 } 398 399 /** 400 * @param value {@link #outcome} (Processing status: error, complete.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 401 */ 402 public EnrollmentResponse setOutcomeElement(Enumeration<EnrollmentOutcome> value) { 403 this.outcome = value; 404 return this; 405 } 406 407 /** 408 * @return Processing status: error, complete. 409 */ 410 public EnrollmentOutcome getOutcome() { 411 return this.outcome == null ? null : this.outcome.getValue(); 412 } 413 414 /** 415 * @param value Processing status: error, complete. 416 */ 417 public EnrollmentResponse setOutcome(EnrollmentOutcome value) { 418 if (value == null) 419 this.outcome = null; 420 else { 421 if (this.outcome == null) 422 this.outcome = new Enumeration<EnrollmentOutcome>(new EnrollmentOutcomeEnumFactory()); 423 this.outcome.setValue(value); 424 } 425 return this; 426 } 427 428 /** 429 * @return {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 430 */ 431 public StringType getDispositionElement() { 432 if (this.disposition == null) 433 if (Configuration.errorOnAutoCreate()) 434 throw new Error("Attempt to auto-create EnrollmentResponse.disposition"); 435 else if (Configuration.doAutoCreate()) 436 this.disposition = new StringType(); // bb 437 return this.disposition; 438 } 439 440 public boolean hasDispositionElement() { 441 return this.disposition != null && !this.disposition.isEmpty(); 442 } 443 444 public boolean hasDisposition() { 445 return this.disposition != null && !this.disposition.isEmpty(); 446 } 447 448 /** 449 * @param value {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 450 */ 451 public EnrollmentResponse setDispositionElement(StringType value) { 452 this.disposition = value; 453 return this; 454 } 455 456 /** 457 * @return A description of the status of the adjudication. 458 */ 459 public String getDisposition() { 460 return this.disposition == null ? null : this.disposition.getValue(); 461 } 462 463 /** 464 * @param value A description of the status of the adjudication. 465 */ 466 public EnrollmentResponse setDisposition(String value) { 467 if (Utilities.noString(value)) 468 this.disposition = null; 469 else { 470 if (this.disposition == null) 471 this.disposition = new StringType(); 472 this.disposition.setValue(value); 473 } 474 return this; 475 } 476 477 /** 478 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 479 */ 480 public DateTimeType getCreatedElement() { 481 if (this.created == null) 482 if (Configuration.errorOnAutoCreate()) 483 throw new Error("Attempt to auto-create EnrollmentResponse.created"); 484 else if (Configuration.doAutoCreate()) 485 this.created = new DateTimeType(); // bb 486 return this.created; 487 } 488 489 public boolean hasCreatedElement() { 490 return this.created != null && !this.created.isEmpty(); 491 } 492 493 public boolean hasCreated() { 494 return this.created != null && !this.created.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 499 */ 500 public EnrollmentResponse setCreatedElement(DateTimeType value) { 501 this.created = value; 502 return this; 503 } 504 505 /** 506 * @return The date when the enclosed suite of services were performed or completed. 507 */ 508 public Date getCreated() { 509 return this.created == null ? null : this.created.getValue(); 510 } 511 512 /** 513 * @param value The date when the enclosed suite of services were performed or completed. 514 */ 515 public EnrollmentResponse setCreated(Date value) { 516 if (value == null) 517 this.created = null; 518 else { 519 if (this.created == null) 520 this.created = new DateTimeType(); 521 this.created.setValue(value); 522 } 523 return this; 524 } 525 526 /** 527 * @return {@link #organization} (The Insurer who produced this adjudicated response.) 528 */ 529 public Reference getOrganization() { 530 if (this.organization == null) 531 if (Configuration.errorOnAutoCreate()) 532 throw new Error("Attempt to auto-create EnrollmentResponse.organization"); 533 else if (Configuration.doAutoCreate()) 534 this.organization = new Reference(); // cc 535 return this.organization; 536 } 537 538 public boolean hasOrganization() { 539 return this.organization != null && !this.organization.isEmpty(); 540 } 541 542 /** 543 * @param value {@link #organization} (The Insurer who produced this adjudicated response.) 544 */ 545 public EnrollmentResponse setOrganization(Reference value) { 546 this.organization = value; 547 return this; 548 } 549 550 /** 551 * @return {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 552 */ 553 public Reference getRequestProvider() { 554 if (this.requestProvider == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create EnrollmentResponse.requestProvider"); 557 else if (Configuration.doAutoCreate()) 558 this.requestProvider = new Reference(); // cc 559 return this.requestProvider; 560 } 561 562 public boolean hasRequestProvider() { 563 return this.requestProvider != null && !this.requestProvider.isEmpty(); 564 } 565 566 /** 567 * @param value {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 568 */ 569 public EnrollmentResponse setRequestProvider(Reference value) { 570 this.requestProvider = value; 571 return this; 572 } 573 574 protected void listChildren(List<Property> children) { 575 super.listChildren(children); 576 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 577 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 578 children.add(new Property("request", "Reference(EnrollmentRequest)", "Original request resource reference.", 0, 1, request)); 579 children.add(new Property("outcome", "code", "Processing status: error, complete.", 0, 1, outcome)); 580 children.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 581 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 582 children.add(new Property("organization", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, organization)); 583 children.add(new Property("requestProvider", "Reference(Practitioner|PractitionerRole|Organization)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider)); 584 } 585 586 @Override 587 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 588 switch (_hash) { 589 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 590 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 591 case 1095692943: /*request*/ return new Property("request", "Reference(EnrollmentRequest)", "Original request resource reference.", 0, 1, request); 592 case -1106507950: /*outcome*/ return new Property("outcome", "code", "Processing status: error, complete.", 0, 1, outcome); 593 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition); 594 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 595 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, organization); 596 case 1601527200: /*requestProvider*/ return new Property("requestProvider", "Reference(Practitioner|PractitionerRole|Organization)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider); 597 default: return super.getNamedProperty(_hash, _name, _checkValid); 598 } 599 600 } 601 602 @Override 603 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 604 switch (hash) { 605 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 606 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FinancialResourceStatusCodes> 607 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 608 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Enumeration<EnrollmentOutcome> 609 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 610 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 611 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 612 case 1601527200: /*requestProvider*/ return this.requestProvider == null ? new Base[0] : new Base[] {this.requestProvider}; // Reference 613 default: return super.getProperty(hash, name, checkValid); 614 } 615 616 } 617 618 @Override 619 public Base setProperty(int hash, String name, Base value) throws FHIRException { 620 switch (hash) { 621 case -1618432855: // identifier 622 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 623 return value; 624 case -892481550: // status 625 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 626 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 627 return value; 628 case 1095692943: // request 629 this.request = TypeConvertor.castToReference(value); // Reference 630 return value; 631 case -1106507950: // outcome 632 value = new EnrollmentOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 633 this.outcome = (Enumeration) value; // Enumeration<EnrollmentOutcome> 634 return value; 635 case 583380919: // disposition 636 this.disposition = TypeConvertor.castToString(value); // StringType 637 return value; 638 case 1028554472: // created 639 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 640 return value; 641 case 1178922291: // organization 642 this.organization = TypeConvertor.castToReference(value); // Reference 643 return value; 644 case 1601527200: // requestProvider 645 this.requestProvider = TypeConvertor.castToReference(value); // Reference 646 return value; 647 default: return super.setProperty(hash, name, value); 648 } 649 650 } 651 652 @Override 653 public Base setProperty(String name, Base value) throws FHIRException { 654 if (name.equals("identifier")) { 655 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 656 } else if (name.equals("status")) { 657 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 658 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 659 } else if (name.equals("request")) { 660 this.request = TypeConvertor.castToReference(value); // Reference 661 } else if (name.equals("outcome")) { 662 value = new EnrollmentOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 663 this.outcome = (Enumeration) value; // Enumeration<EnrollmentOutcome> 664 } else if (name.equals("disposition")) { 665 this.disposition = TypeConvertor.castToString(value); // StringType 666 } else if (name.equals("created")) { 667 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 668 } else if (name.equals("organization")) { 669 this.organization = TypeConvertor.castToReference(value); // Reference 670 } else if (name.equals("requestProvider")) { 671 this.requestProvider = TypeConvertor.castToReference(value); // Reference 672 } else 673 return super.setProperty(name, value); 674 return value; 675 } 676 677 @Override 678 public void removeChild(String name, Base value) throws FHIRException { 679 if (name.equals("identifier")) { 680 this.getIdentifier().remove(value); 681 } else if (name.equals("status")) { 682 value = new FinancialResourceStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 683 this.status = (Enumeration) value; // Enumeration<FinancialResourceStatusCodes> 684 } else if (name.equals("request")) { 685 this.request = null; 686 } else if (name.equals("outcome")) { 687 value = new EnrollmentOutcomeEnumFactory().fromType(TypeConvertor.castToCode(value)); 688 this.outcome = (Enumeration) value; // Enumeration<EnrollmentOutcome> 689 } else if (name.equals("disposition")) { 690 this.disposition = null; 691 } else if (name.equals("created")) { 692 this.created = null; 693 } else if (name.equals("organization")) { 694 this.organization = null; 695 } else if (name.equals("requestProvider")) { 696 this.requestProvider = null; 697 } else 698 super.removeChild(name, value); 699 700 } 701 702 @Override 703 public Base makeProperty(int hash, String name) throws FHIRException { 704 switch (hash) { 705 case -1618432855: return addIdentifier(); 706 case -892481550: return getStatusElement(); 707 case 1095692943: return getRequest(); 708 case -1106507950: return getOutcomeElement(); 709 case 583380919: return getDispositionElement(); 710 case 1028554472: return getCreatedElement(); 711 case 1178922291: return getOrganization(); 712 case 1601527200: return getRequestProvider(); 713 default: return super.makeProperty(hash, name); 714 } 715 716 } 717 718 @Override 719 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 720 switch (hash) { 721 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 722 case -892481550: /*status*/ return new String[] {"code"}; 723 case 1095692943: /*request*/ return new String[] {"Reference"}; 724 case -1106507950: /*outcome*/ return new String[] {"code"}; 725 case 583380919: /*disposition*/ return new String[] {"string"}; 726 case 1028554472: /*created*/ return new String[] {"dateTime"}; 727 case 1178922291: /*organization*/ return new String[] {"Reference"}; 728 case 1601527200: /*requestProvider*/ return new String[] {"Reference"}; 729 default: return super.getTypesForProperty(hash, name); 730 } 731 732 } 733 734 @Override 735 public Base addChild(String name) throws FHIRException { 736 if (name.equals("identifier")) { 737 return addIdentifier(); 738 } 739 else if (name.equals("status")) { 740 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.status"); 741 } 742 else if (name.equals("request")) { 743 this.request = new Reference(); 744 return this.request; 745 } 746 else if (name.equals("outcome")) { 747 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.outcome"); 748 } 749 else if (name.equals("disposition")) { 750 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.disposition"); 751 } 752 else if (name.equals("created")) { 753 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.created"); 754 } 755 else if (name.equals("organization")) { 756 this.organization = new Reference(); 757 return this.organization; 758 } 759 else if (name.equals("requestProvider")) { 760 this.requestProvider = new Reference(); 761 return this.requestProvider; 762 } 763 else 764 return super.addChild(name); 765 } 766 767 public String fhirType() { 768 return "EnrollmentResponse"; 769 770 } 771 772 public EnrollmentResponse copy() { 773 EnrollmentResponse dst = new EnrollmentResponse(); 774 copyValues(dst); 775 return dst; 776 } 777 778 public void copyValues(EnrollmentResponse dst) { 779 super.copyValues(dst); 780 if (identifier != null) { 781 dst.identifier = new ArrayList<Identifier>(); 782 for (Identifier i : identifier) 783 dst.identifier.add(i.copy()); 784 }; 785 dst.status = status == null ? null : status.copy(); 786 dst.request = request == null ? null : request.copy(); 787 dst.outcome = outcome == null ? null : outcome.copy(); 788 dst.disposition = disposition == null ? null : disposition.copy(); 789 dst.created = created == null ? null : created.copy(); 790 dst.organization = organization == null ? null : organization.copy(); 791 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 792 } 793 794 protected EnrollmentResponse typedCopy() { 795 return copy(); 796 } 797 798 @Override 799 public boolean equalsDeep(Base other_) { 800 if (!super.equalsDeep(other_)) 801 return false; 802 if (!(other_ instanceof EnrollmentResponse)) 803 return false; 804 EnrollmentResponse o = (EnrollmentResponse) other_; 805 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(request, o.request, true) 806 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) && compareDeep(created, o.created, true) 807 && compareDeep(organization, o.organization, true) && compareDeep(requestProvider, o.requestProvider, true) 808 ; 809 } 810 811 @Override 812 public boolean equalsShallow(Base other_) { 813 if (!super.equalsShallow(other_)) 814 return false; 815 if (!(other_ instanceof EnrollmentResponse)) 816 return false; 817 EnrollmentResponse o = (EnrollmentResponse) other_; 818 return compareValues(status, o.status, true) && compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) 819 && compareValues(created, o.created, true); 820 } 821 822 public boolean isEmpty() { 823 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, request 824 , outcome, disposition, created, organization, requestProvider); 825 } 826 827 @Override 828 public ResourceType getResourceType() { 829 return ResourceType.EnrollmentResponse; 830 } 831 832 /** 833 * Search parameter: <b>identifier</b> 834 * <p> 835 * Description: <b>The business identifier of the EnrollmentResponse</b><br> 836 * Type: <b>token</b><br> 837 * Path: <b>EnrollmentResponse.identifier</b><br> 838 * </p> 839 */ 840 @SearchParamDefinition(name="identifier", path="EnrollmentResponse.identifier", description="The business identifier of the EnrollmentResponse", type="token" ) 841 public static final String SP_IDENTIFIER = "identifier"; 842 /** 843 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 844 * <p> 845 * Description: <b>The business identifier of the EnrollmentResponse</b><br> 846 * Type: <b>token</b><br> 847 * Path: <b>EnrollmentResponse.identifier</b><br> 848 * </p> 849 */ 850 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 851 852 /** 853 * Search parameter: <b>request</b> 854 * <p> 855 * Description: <b>The reference to the claim</b><br> 856 * Type: <b>reference</b><br> 857 * Path: <b>EnrollmentResponse.request</b><br> 858 * </p> 859 */ 860 @SearchParamDefinition(name="request", path="EnrollmentResponse.request", description="The reference to the claim", type="reference", target={EnrollmentRequest.class } ) 861 public static final String SP_REQUEST = "request"; 862 /** 863 * <b>Fluent Client</b> search parameter constant for <b>request</b> 864 * <p> 865 * Description: <b>The reference to the claim</b><br> 866 * Type: <b>reference</b><br> 867 * Path: <b>EnrollmentResponse.request</b><br> 868 * </p> 869 */ 870 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 871 872/** 873 * Constant for fluent queries to be used to add include statements. Specifies 874 * the path value of "<b>EnrollmentResponse:request</b>". 875 */ 876 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("EnrollmentResponse:request").toLocked(); 877 878 /** 879 * Search parameter: <b>status</b> 880 * <p> 881 * Description: <b>The status of the enrollment response</b><br> 882 * Type: <b>token</b><br> 883 * Path: <b>EnrollmentResponse.status</b><br> 884 * </p> 885 */ 886 @SearchParamDefinition(name="status", path="EnrollmentResponse.status", description="The status of the enrollment response", type="token" ) 887 public static final String SP_STATUS = "status"; 888 /** 889 * <b>Fluent Client</b> search parameter constant for <b>status</b> 890 * <p> 891 * Description: <b>The status of the enrollment response</b><br> 892 * Type: <b>token</b><br> 893 * Path: <b>EnrollmentResponse.status</b><br> 894 * </p> 895 */ 896 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 897 898 899} 900