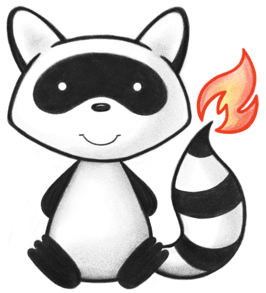
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.IOException; 035import java.io.ObjectInput; 036import java.io.ObjectOutput; 037import java.util.ArrayList; 038 039import org.hl7.fhir.instance.model.api.IBaseEnumeration; 040 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042 043/* 044Copyright (c) 2011+, HL7, Inc 045All rights reserved. 046 047Redistribution and use in source and binary forms, with or without modification, 048are permitted provided that the following conditions are met: 049 050 * Redistributions of source code must retain the above copyright notice, this 051 list of conditions and the following disclaimer. 052 * Redistributions in binary form must reproduce the above copyright notice, 053 this list of conditions and the following disclaimer in the documentation 054 and/or other materials provided with the distribution. 055 * Neither the name of HL7 nor the names of its contributors may be used to 056 endorse or promote products derived from this software without specific 057 prior written permission. 058 059THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 060ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 061WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 062IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 063INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 064NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 065PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 066WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 067ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 068POSSIBILITY OF SUCH DAMAGE. 069 070*/ 071 072/** 073 * Primitive type "code" in FHIR, where the code is tied to an enumerated list of possible values 074 * 075 */ 076@DatatypeDef(name = "code", isSpecialization = true) 077public class Enumeration<T extends Enum<?>> extends PrimitiveType<T> implements IBaseEnumeration<T>, ICoding { 078 079 private static final long serialVersionUID = 1L; 080 private EnumFactory<T> myEnumFactory; 081 082 /** 083 * Constructor 084 * 085 * @deprecated This no-arg constructor is provided for serialization only - Do not use 086 */ 087 @Deprecated 088 public Enumeration() { 089 // nothing 090 } 091 092 /** 093 * Constructor 094 */ 095 public Enumeration(EnumFactory<T> theEnumFactory) { 096 if (theEnumFactory == null) 097 throw new IllegalArgumentException("An enumeration factory must be provided"); 098 myEnumFactory = theEnumFactory; 099 } 100 101 /** 102 * Constructor 103 */ 104 public Enumeration(EnumFactory<T> theEnumFactory, String theValue) { 105 if (theEnumFactory == null) 106 throw new IllegalArgumentException("An enumeration factory must be provided"); 107 myEnumFactory = theEnumFactory; 108 setValueAsString(theValue); 109 } 110 111 /** 112 * Constructor 113 */ 114 public Enumeration(EnumFactory<T> theEnumFactory, T theValue) { 115 if (theEnumFactory == null) 116 throw new IllegalArgumentException("An enumeration factory must be provided"); 117 myEnumFactory = theEnumFactory; 118 setValue(theValue); 119 } 120 121 /** 122 * Constructor 123 */ 124 public Enumeration(EnumFactory<T> theEnumFactory, T theValue, Element source) { 125 if (theEnumFactory == null) 126 throw new IllegalArgumentException("An enumeration factory must be provided"); 127 myEnumFactory = theEnumFactory; 128 setValue(theValue); 129 setId(source.getId()); 130 getExtension().addAll(source.getExtension()); 131 } 132 133 /** 134 * Constructor 135 */ 136 public Enumeration(EnumFactory<T> theEnumFactory, CodeType source) { 137 if (theEnumFactory == null) 138 throw new IllegalArgumentException("An enumeration factory must be provided"); 139 myEnumFactory = theEnumFactory; 140 setValue(myEnumFactory.fromCode(source.getCode())); 141 setId(source.getId()); 142 getExtension().addAll(source.getExtension()); 143 } 144 145 @Override 146 public Enumeration<T> copy() { 147 Enumeration dst= new Enumeration(this.myEnumFactory, (Enum)this.getValue()); 148 //Copy the Extension 149 if (extension != null) { 150 dst.extension = new ArrayList<>(); 151 for (Extension i : extension) 152 dst.extension.add(i.copy()); 153 }; 154 return dst; 155 } 156 157 @Override 158 protected String encode(T theValue) { 159 return myEnumFactory.toCode(theValue); 160 } 161 162 public String fhirType() { 163 return "code"; 164 } 165 166 /** 167 * Provides the enum factory which binds this enumeration to a specific ValueSet 168 */ 169 public EnumFactory<T> getEnumFactory() { 170 return myEnumFactory; 171 } 172 173 @Override 174 protected T parse(String theValue) { 175 if (myEnumFactory != null) { 176 return myEnumFactory.fromCode(theValue); 177 } 178 return null; 179 } 180 181 @SuppressWarnings("unchecked") 182 @Override 183 public void readExternal(ObjectInput theIn) throws IOException, ClassNotFoundException { 184 myEnumFactory = (EnumFactory<T>) theIn.readObject(); 185 super.readExternal(theIn); 186 } 187 188 public String toSystem() { 189 return getEnumFactory().toSystem(getValue()); 190 } 191 192 @Override 193 public void writeExternal(ObjectOutput theOut) throws IOException { 194 theOut.writeObject(myEnumFactory); 195 super.writeExternal(theOut); 196 } 197 198 @Override 199 public String getSystem() { 200 return myEnumFactory.toSystem(myEnumFactory.fromCode(asStringValue())); 201 } 202 203 @Override 204 public boolean hasSystem() { 205 return myEnumFactory.toSystem(myEnumFactory.fromCode(asStringValue())) != null; 206 } 207 208 @Override 209 public String getVersion() { 210 return null; 211 } 212 213 @Override 214 public boolean hasVersion() { 215 return false; 216 } 217 218 @Override 219 public boolean supportsVersion() { 220 return false; 221 } 222 223 @Override 224 public String getCode() { 225 return asStringValue(); 226 } 227 228 @Override 229 public boolean hasCode() { 230 return asStringValue() != null; 231 } 232 233 @Override 234 public String getDisplay() { 235 return null; 236 } 237 238 @Override 239 public boolean hasDisplay() { 240 return false; 241 } 242 243 @Override 244 public boolean supportsDisplay() { 245 return false; 246 } 247 248 public CodeType getCodeType() { 249 CodeType ct = new CodeType(); 250 ct.setId(getId()); 251 ct.getExtension().addAll(getExtension()); 252 ct.setValue(asStringValue()); 253 return ct; 254 } 255}