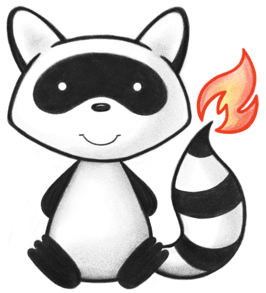
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 052 */ 053@ResourceDef(name="EpisodeOfCare", profile="http://hl7.org/fhir/StructureDefinition/EpisodeOfCare") 054public class EpisodeOfCare extends DomainResource { 055 056 public enum EpisodeOfCareStatus { 057 /** 058 * This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services. 059 */ 060 PLANNED, 061 /** 062 * This episode has been placed on a waitlist, pending the episode being made active (or cancelled). 063 */ 064 WAITLIST, 065 /** 066 * This episode of care is current. 067 */ 068 ACTIVE, 069 /** 070 * This episode of care is on hold; the organization has limited responsibility for the patient (such as while on respite). 071 */ 072 ONHOLD, 073 /** 074 * This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms. 075 */ 076 FINISHED, 077 /** 078 * The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow. 079 */ 080 CANCELLED, 081 /** 082 * This instance should not have been part of this patient's medical record. 083 */ 084 ENTEREDINERROR, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static EpisodeOfCareStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("planned".equals(codeString)) 093 return PLANNED; 094 if ("waitlist".equals(codeString)) 095 return WAITLIST; 096 if ("active".equals(codeString)) 097 return ACTIVE; 098 if ("onhold".equals(codeString)) 099 return ONHOLD; 100 if ("finished".equals(codeString)) 101 return FINISHED; 102 if ("cancelled".equals(codeString)) 103 return CANCELLED; 104 if ("entered-in-error".equals(codeString)) 105 return ENTEREDINERROR; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown EpisodeOfCareStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case PLANNED: return "planned"; 114 case WAITLIST: return "waitlist"; 115 case ACTIVE: return "active"; 116 case ONHOLD: return "onhold"; 117 case FINISHED: return "finished"; 118 case CANCELLED: return "cancelled"; 119 case ENTEREDINERROR: return "entered-in-error"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case PLANNED: return "http://hl7.org/fhir/episode-of-care-status"; 127 case WAITLIST: return "http://hl7.org/fhir/episode-of-care-status"; 128 case ACTIVE: return "http://hl7.org/fhir/episode-of-care-status"; 129 case ONHOLD: return "http://hl7.org/fhir/episode-of-care-status"; 130 case FINISHED: return "http://hl7.org/fhir/episode-of-care-status"; 131 case CANCELLED: return "http://hl7.org/fhir/episode-of-care-status"; 132 case ENTEREDINERROR: return "http://hl7.org/fhir/episode-of-care-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case PLANNED: return "This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services."; 140 case WAITLIST: return "This episode has been placed on a waitlist, pending the episode being made active (or cancelled)."; 141 case ACTIVE: return "This episode of care is current."; 142 case ONHOLD: return "This episode of care is on hold; the organization has limited responsibility for the patient (such as while on respite)."; 143 case FINISHED: return "This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms."; 144 case CANCELLED: return "The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow."; 145 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case PLANNED: return "Planned"; 153 case WAITLIST: return "Waitlist"; 154 case ACTIVE: return "Active"; 155 case ONHOLD: return "On Hold"; 156 case FINISHED: return "Finished"; 157 case CANCELLED: return "Cancelled"; 158 case ENTEREDINERROR: return "Entered in Error"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class EpisodeOfCareStatusEnumFactory implements EnumFactory<EpisodeOfCareStatus> { 166 public EpisodeOfCareStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("planned".equals(codeString)) 171 return EpisodeOfCareStatus.PLANNED; 172 if ("waitlist".equals(codeString)) 173 return EpisodeOfCareStatus.WAITLIST; 174 if ("active".equals(codeString)) 175 return EpisodeOfCareStatus.ACTIVE; 176 if ("onhold".equals(codeString)) 177 return EpisodeOfCareStatus.ONHOLD; 178 if ("finished".equals(codeString)) 179 return EpisodeOfCareStatus.FINISHED; 180 if ("cancelled".equals(codeString)) 181 return EpisodeOfCareStatus.CANCELLED; 182 if ("entered-in-error".equals(codeString)) 183 return EpisodeOfCareStatus.ENTEREDINERROR; 184 throw new IllegalArgumentException("Unknown EpisodeOfCareStatus code '"+codeString+"'"); 185 } 186 public Enumeration<EpisodeOfCareStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.NULL, code); 194 if ("planned".equals(codeString)) 195 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.PLANNED, code); 196 if ("waitlist".equals(codeString)) 197 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.WAITLIST, code); 198 if ("active".equals(codeString)) 199 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ACTIVE, code); 200 if ("onhold".equals(codeString)) 201 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ONHOLD, code); 202 if ("finished".equals(codeString)) 203 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.FINISHED, code); 204 if ("cancelled".equals(codeString)) 205 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.CANCELLED, code); 206 if ("entered-in-error".equals(codeString)) 207 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ENTEREDINERROR, code); 208 throw new FHIRException("Unknown EpisodeOfCareStatus code '"+codeString+"'"); 209 } 210 public String toCode(EpisodeOfCareStatus code) { 211 if (code == EpisodeOfCareStatus.NULL) 212 return null; 213 if (code == EpisodeOfCareStatus.PLANNED) 214 return "planned"; 215 if (code == EpisodeOfCareStatus.WAITLIST) 216 return "waitlist"; 217 if (code == EpisodeOfCareStatus.ACTIVE) 218 return "active"; 219 if (code == EpisodeOfCareStatus.ONHOLD) 220 return "onhold"; 221 if (code == EpisodeOfCareStatus.FINISHED) 222 return "finished"; 223 if (code == EpisodeOfCareStatus.CANCELLED) 224 return "cancelled"; 225 if (code == EpisodeOfCareStatus.ENTEREDINERROR) 226 return "entered-in-error"; 227 return "?"; 228 } 229 public String toSystem(EpisodeOfCareStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 @Block() 235 public static class EpisodeOfCareStatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 236 /** 237 * planned | waitlist | active | onhold | finished | cancelled. 238 */ 239 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 240 @Description(shortDefinition="planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition="planned | waitlist | active | onhold | finished | cancelled." ) 241 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/episode-of-care-status") 242 protected Enumeration<EpisodeOfCareStatus> status; 243 244 /** 245 * The period during this EpisodeOfCare that the specific status applied. 246 */ 247 @Child(name = "period", type = {Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 248 @Description(shortDefinition="Duration the EpisodeOfCare was in the specified status", formalDefinition="The period during this EpisodeOfCare that the specific status applied." ) 249 protected Period period; 250 251 private static final long serialVersionUID = -1192432864L; 252 253 /** 254 * Constructor 255 */ 256 public EpisodeOfCareStatusHistoryComponent() { 257 super(); 258 } 259 260 /** 261 * Constructor 262 */ 263 public EpisodeOfCareStatusHistoryComponent(EpisodeOfCareStatus status, Period period) { 264 super(); 265 this.setStatus(status); 266 this.setPeriod(period); 267 } 268 269 /** 270 * @return {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 271 */ 272 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 273 if (this.status == null) 274 if (Configuration.errorOnAutoCreate()) 275 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.status"); 276 else if (Configuration.doAutoCreate()) 277 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 278 return this.status; 279 } 280 281 public boolean hasStatusElement() { 282 return this.status != null && !this.status.isEmpty(); 283 } 284 285 public boolean hasStatus() { 286 return this.status != null && !this.status.isEmpty(); 287 } 288 289 /** 290 * @param value {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 291 */ 292 public EpisodeOfCareStatusHistoryComponent setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 293 this.status = value; 294 return this; 295 } 296 297 /** 298 * @return planned | waitlist | active | onhold | finished | cancelled. 299 */ 300 public EpisodeOfCareStatus getStatus() { 301 return this.status == null ? null : this.status.getValue(); 302 } 303 304 /** 305 * @param value planned | waitlist | active | onhold | finished | cancelled. 306 */ 307 public EpisodeOfCareStatusHistoryComponent setStatus(EpisodeOfCareStatus value) { 308 if (this.status == null) 309 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 310 this.status.setValue(value); 311 return this; 312 } 313 314 /** 315 * @return {@link #period} (The period during this EpisodeOfCare that the specific status applied.) 316 */ 317 public Period getPeriod() { 318 if (this.period == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.period"); 321 else if (Configuration.doAutoCreate()) 322 this.period = new Period(); // cc 323 return this.period; 324 } 325 326 public boolean hasPeriod() { 327 return this.period != null && !this.period.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #period} (The period during this EpisodeOfCare that the specific status applied.) 332 */ 333 public EpisodeOfCareStatusHistoryComponent setPeriod(Period value) { 334 this.period = value; 335 return this; 336 } 337 338 protected void listChildren(List<Property> children) { 339 super.listChildren(children); 340 children.add(new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 341 children.add(new Property("period", "Period", "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period)); 342 } 343 344 @Override 345 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 346 switch (_hash) { 347 case -892481550: /*status*/ return new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status); 348 case -991726143: /*period*/ return new Property("period", "Period", "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period); 349 default: return super.getNamedProperty(_hash, _name, _checkValid); 350 } 351 352 } 353 354 @Override 355 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 356 switch (hash) { 357 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EpisodeOfCareStatus> 358 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 359 default: return super.getProperty(hash, name, checkValid); 360 } 361 362 } 363 364 @Override 365 public Base setProperty(int hash, String name, Base value) throws FHIRException { 366 switch (hash) { 367 case -892481550: // status 368 value = new EpisodeOfCareStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 369 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 370 return value; 371 case -991726143: // period 372 this.period = TypeConvertor.castToPeriod(value); // Period 373 return value; 374 default: return super.setProperty(hash, name, value); 375 } 376 377 } 378 379 @Override 380 public Base setProperty(String name, Base value) throws FHIRException { 381 if (name.equals("status")) { 382 value = new EpisodeOfCareStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 383 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 384 } else if (name.equals("period")) { 385 this.period = TypeConvertor.castToPeriod(value); // Period 386 } else 387 return super.setProperty(name, value); 388 return value; 389 } 390 391 @Override 392 public void removeChild(String name, Base value) throws FHIRException { 393 if (name.equals("status")) { 394 value = new EpisodeOfCareStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 395 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 396 } else if (name.equals("period")) { 397 this.period = null; 398 } else 399 super.removeChild(name, value); 400 401 } 402 403 @Override 404 public Base makeProperty(int hash, String name) throws FHIRException { 405 switch (hash) { 406 case -892481550: return getStatusElement(); 407 case -991726143: return getPeriod(); 408 default: return super.makeProperty(hash, name); 409 } 410 411 } 412 413 @Override 414 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 415 switch (hash) { 416 case -892481550: /*status*/ return new String[] {"code"}; 417 case -991726143: /*period*/ return new String[] {"Period"}; 418 default: return super.getTypesForProperty(hash, name); 419 } 420 421 } 422 423 @Override 424 public Base addChild(String name) throws FHIRException { 425 if (name.equals("status")) { 426 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.statusHistory.status"); 427 } 428 else if (name.equals("period")) { 429 this.period = new Period(); 430 return this.period; 431 } 432 else 433 return super.addChild(name); 434 } 435 436 public EpisodeOfCareStatusHistoryComponent copy() { 437 EpisodeOfCareStatusHistoryComponent dst = new EpisodeOfCareStatusHistoryComponent(); 438 copyValues(dst); 439 return dst; 440 } 441 442 public void copyValues(EpisodeOfCareStatusHistoryComponent dst) { 443 super.copyValues(dst); 444 dst.status = status == null ? null : status.copy(); 445 dst.period = period == null ? null : period.copy(); 446 } 447 448 @Override 449 public boolean equalsDeep(Base other_) { 450 if (!super.equalsDeep(other_)) 451 return false; 452 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 453 return false; 454 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 455 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 456 } 457 458 @Override 459 public boolean equalsShallow(Base other_) { 460 if (!super.equalsShallow(other_)) 461 return false; 462 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 463 return false; 464 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 465 return compareValues(status, o.status, true); 466 } 467 468 public boolean isEmpty() { 469 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, period); 470 } 471 472 public String fhirType() { 473 return "EpisodeOfCare.statusHistory"; 474 475 } 476 477 } 478 479 @Block() 480 public static class ReasonComponent extends BackboneElement implements IBaseBackboneElement { 481 /** 482 * What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening). 483 */ 484 @Child(name = "use", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 485 @Description(shortDefinition="What the reason value should be used for/as", formalDefinition="What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening)." ) 486 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-reason-use") 487 protected CodeableConcept use; 488 489 /** 490 * The medical reason that is expected to be addressed during the episode of care, expressed as a text, code or a reference to another resource. 491 */ 492 @Child(name = "value", type = {CodeableReference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 493 @Description(shortDefinition="Medical reason to be addressed", formalDefinition="The medical reason that is expected to be addressed during the episode of care, expressed as a text, code or a reference to another resource." ) 494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-reason") 495 protected List<CodeableReference> value; 496 497 private static final long serialVersionUID = 322767075L; 498 499 /** 500 * Constructor 501 */ 502 public ReasonComponent() { 503 super(); 504 } 505 506 /** 507 * @return {@link #use} (What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening).) 508 */ 509 public CodeableConcept getUse() { 510 if (this.use == null) 511 if (Configuration.errorOnAutoCreate()) 512 throw new Error("Attempt to auto-create ReasonComponent.use"); 513 else if (Configuration.doAutoCreate()) 514 this.use = new CodeableConcept(); // cc 515 return this.use; 516 } 517 518 public boolean hasUse() { 519 return this.use != null && !this.use.isEmpty(); 520 } 521 522 /** 523 * @param value {@link #use} (What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening).) 524 */ 525 public ReasonComponent setUse(CodeableConcept value) { 526 this.use = value; 527 return this; 528 } 529 530 /** 531 * @return {@link #value} (The medical reason that is expected to be addressed during the episode of care, expressed as a text, code or a reference to another resource.) 532 */ 533 public List<CodeableReference> getValue() { 534 if (this.value == null) 535 this.value = new ArrayList<CodeableReference>(); 536 return this.value; 537 } 538 539 /** 540 * @return Returns a reference to <code>this</code> for easy method chaining 541 */ 542 public ReasonComponent setValue(List<CodeableReference> theValue) { 543 this.value = theValue; 544 return this; 545 } 546 547 public boolean hasValue() { 548 if (this.value == null) 549 return false; 550 for (CodeableReference item : this.value) 551 if (!item.isEmpty()) 552 return true; 553 return false; 554 } 555 556 public CodeableReference addValue() { //3 557 CodeableReference t = new CodeableReference(); 558 if (this.value == null) 559 this.value = new ArrayList<CodeableReference>(); 560 this.value.add(t); 561 return t; 562 } 563 564 public ReasonComponent addValue(CodeableReference t) { //3 565 if (t == null) 566 return this; 567 if (this.value == null) 568 this.value = new ArrayList<CodeableReference>(); 569 this.value.add(t); 570 return this; 571 } 572 573 /** 574 * @return The first repetition of repeating field {@link #value}, creating it if it does not already exist {3} 575 */ 576 public CodeableReference getValueFirstRep() { 577 if (getValue().isEmpty()) { 578 addValue(); 579 } 580 return getValue().get(0); 581 } 582 583 protected void listChildren(List<Property> children) { 584 super.listChildren(children); 585 children.add(new Property("use", "CodeableConcept", "What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening).", 0, 1, use)); 586 children.add(new Property("value", "CodeableReference(Condition|Procedure|Observation|HealthcareService)", "The medical reason that is expected to be addressed during the episode of care, expressed as a text, code or a reference to another resource.", 0, java.lang.Integer.MAX_VALUE, value)); 587 } 588 589 @Override 590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 591 switch (_hash) { 592 case 116103: /*use*/ return new Property("use", "CodeableConcept", "What the reason value should be used as e.g. Chief Complaint, Health Concern, Health Maintenance (including screening).", 0, 1, use); 593 case 111972721: /*value*/ return new Property("value", "CodeableReference(Condition|Procedure|Observation|HealthcareService)", "The medical reason that is expected to be addressed during the episode of care, expressed as a text, code or a reference to another resource.", 0, java.lang.Integer.MAX_VALUE, value); 594 default: return super.getNamedProperty(_hash, _name, _checkValid); 595 } 596 597 } 598 599 @Override 600 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 601 switch (hash) { 602 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // CodeableConcept 603 case 111972721: /*value*/ return this.value == null ? new Base[0] : this.value.toArray(new Base[this.value.size()]); // CodeableReference 604 default: return super.getProperty(hash, name, checkValid); 605 } 606 607 } 608 609 @Override 610 public Base setProperty(int hash, String name, Base value) throws FHIRException { 611 switch (hash) { 612 case 116103: // use 613 this.use = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 614 return value; 615 case 111972721: // value 616 this.getValue().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 617 return value; 618 default: return super.setProperty(hash, name, value); 619 } 620 621 } 622 623 @Override 624 public Base setProperty(String name, Base value) throws FHIRException { 625 if (name.equals("use")) { 626 this.use = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 627 } else if (name.equals("value")) { 628 this.getValue().add(TypeConvertor.castToCodeableReference(value)); 629 } else 630 return super.setProperty(name, value); 631 return value; 632 } 633 634 @Override 635 public void removeChild(String name, Base value) throws FHIRException { 636 if (name.equals("use")) { 637 this.use = null; 638 } else if (name.equals("value")) { 639 this.getValue().remove(value); 640 } else 641 super.removeChild(name, value); 642 643 } 644 645 @Override 646 public Base makeProperty(int hash, String name) throws FHIRException { 647 switch (hash) { 648 case 116103: return getUse(); 649 case 111972721: return addValue(); 650 default: return super.makeProperty(hash, name); 651 } 652 653 } 654 655 @Override 656 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 657 switch (hash) { 658 case 116103: /*use*/ return new String[] {"CodeableConcept"}; 659 case 111972721: /*value*/ return new String[] {"CodeableReference"}; 660 default: return super.getTypesForProperty(hash, name); 661 } 662 663 } 664 665 @Override 666 public Base addChild(String name) throws FHIRException { 667 if (name.equals("use")) { 668 this.use = new CodeableConcept(); 669 return this.use; 670 } 671 else if (name.equals("value")) { 672 return addValue(); 673 } 674 else 675 return super.addChild(name); 676 } 677 678 public ReasonComponent copy() { 679 ReasonComponent dst = new ReasonComponent(); 680 copyValues(dst); 681 return dst; 682 } 683 684 public void copyValues(ReasonComponent dst) { 685 super.copyValues(dst); 686 dst.use = use == null ? null : use.copy(); 687 if (value != null) { 688 dst.value = new ArrayList<CodeableReference>(); 689 for (CodeableReference i : value) 690 dst.value.add(i.copy()); 691 }; 692 } 693 694 @Override 695 public boolean equalsDeep(Base other_) { 696 if (!super.equalsDeep(other_)) 697 return false; 698 if (!(other_ instanceof ReasonComponent)) 699 return false; 700 ReasonComponent o = (ReasonComponent) other_; 701 return compareDeep(use, o.use, true) && compareDeep(value, o.value, true); 702 } 703 704 @Override 705 public boolean equalsShallow(Base other_) { 706 if (!super.equalsShallow(other_)) 707 return false; 708 if (!(other_ instanceof ReasonComponent)) 709 return false; 710 ReasonComponent o = (ReasonComponent) other_; 711 return true; 712 } 713 714 public boolean isEmpty() { 715 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, value); 716 } 717 718 public String fhirType() { 719 return "EpisodeOfCare.reason"; 720 721 } 722 723 } 724 725 @Block() 726 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 727 /** 728 * The medical condition that was addressed during the episode of care, expressed as a text, code or a reference to another resource. 729 */ 730 @Child(name = "condition", type = {CodeableReference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 731 @Description(shortDefinition="The medical condition that was addressed during the episode of care", formalDefinition="The medical condition that was addressed during the episode of care, expressed as a text, code or a reference to another resource." ) 732 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 733 protected List<CodeableReference> condition; 734 735 /** 736 * Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?). 737 */ 738 @Child(name = "use", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 739 @Description(shortDefinition="Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?)", formalDefinition="Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?)." ) 740 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-diagnosis-use") 741 protected CodeableConcept use; 742 743 private static final long serialVersionUID = -1997962887L; 744 745 /** 746 * Constructor 747 */ 748 public DiagnosisComponent() { 749 super(); 750 } 751 752 /** 753 * @return {@link #condition} (The medical condition that was addressed during the episode of care, expressed as a text, code or a reference to another resource.) 754 */ 755 public List<CodeableReference> getCondition() { 756 if (this.condition == null) 757 this.condition = new ArrayList<CodeableReference>(); 758 return this.condition; 759 } 760 761 /** 762 * @return Returns a reference to <code>this</code> for easy method chaining 763 */ 764 public DiagnosisComponent setCondition(List<CodeableReference> theCondition) { 765 this.condition = theCondition; 766 return this; 767 } 768 769 public boolean hasCondition() { 770 if (this.condition == null) 771 return false; 772 for (CodeableReference item : this.condition) 773 if (!item.isEmpty()) 774 return true; 775 return false; 776 } 777 778 public CodeableReference addCondition() { //3 779 CodeableReference t = new CodeableReference(); 780 if (this.condition == null) 781 this.condition = new ArrayList<CodeableReference>(); 782 this.condition.add(t); 783 return t; 784 } 785 786 public DiagnosisComponent addCondition(CodeableReference t) { //3 787 if (t == null) 788 return this; 789 if (this.condition == null) 790 this.condition = new ArrayList<CodeableReference>(); 791 this.condition.add(t); 792 return this; 793 } 794 795 /** 796 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 797 */ 798 public CodeableReference getConditionFirstRep() { 799 if (getCondition().isEmpty()) { 800 addCondition(); 801 } 802 return getCondition().get(0); 803 } 804 805 /** 806 * @return {@link #use} (Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).) 807 */ 808 public CodeableConcept getUse() { 809 if (this.use == null) 810 if (Configuration.errorOnAutoCreate()) 811 throw new Error("Attempt to auto-create DiagnosisComponent.use"); 812 else if (Configuration.doAutoCreate()) 813 this.use = new CodeableConcept(); // cc 814 return this.use; 815 } 816 817 public boolean hasUse() { 818 return this.use != null && !this.use.isEmpty(); 819 } 820 821 /** 822 * @param value {@link #use} (Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).) 823 */ 824 public DiagnosisComponent setUse(CodeableConcept value) { 825 this.use = value; 826 return this; 827 } 828 829 protected void listChildren(List<Property> children) { 830 super.listChildren(children); 831 children.add(new Property("condition", "CodeableReference(Condition)", "The medical condition that was addressed during the episode of care, expressed as a text, code or a reference to another resource.", 0, java.lang.Integer.MAX_VALUE, condition)); 832 children.add(new Property("use", "CodeableConcept", "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, use)); 833 } 834 835 @Override 836 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 837 switch (_hash) { 838 case -861311717: /*condition*/ return new Property("condition", "CodeableReference(Condition)", "The medical condition that was addressed during the episode of care, expressed as a text, code or a reference to another resource.", 0, java.lang.Integer.MAX_VALUE, condition); 839 case 116103: /*use*/ return new Property("use", "CodeableConcept", "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, use); 840 default: return super.getNamedProperty(_hash, _name, _checkValid); 841 } 842 843 } 844 845 @Override 846 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 847 switch (hash) { 848 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // CodeableReference 849 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // CodeableConcept 850 default: return super.getProperty(hash, name, checkValid); 851 } 852 853 } 854 855 @Override 856 public Base setProperty(int hash, String name, Base value) throws FHIRException { 857 switch (hash) { 858 case -861311717: // condition 859 this.getCondition().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 860 return value; 861 case 116103: // use 862 this.use = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 863 return value; 864 default: return super.setProperty(hash, name, value); 865 } 866 867 } 868 869 @Override 870 public Base setProperty(String name, Base value) throws FHIRException { 871 if (name.equals("condition")) { 872 this.getCondition().add(TypeConvertor.castToCodeableReference(value)); 873 } else if (name.equals("use")) { 874 this.use = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 875 } else 876 return super.setProperty(name, value); 877 return value; 878 } 879 880 @Override 881 public void removeChild(String name, Base value) throws FHIRException { 882 if (name.equals("condition")) { 883 this.getCondition().remove(value); 884 } else if (name.equals("use")) { 885 this.use = null; 886 } else 887 super.removeChild(name, value); 888 889 } 890 891 @Override 892 public Base makeProperty(int hash, String name) throws FHIRException { 893 switch (hash) { 894 case -861311717: return addCondition(); 895 case 116103: return getUse(); 896 default: return super.makeProperty(hash, name); 897 } 898 899 } 900 901 @Override 902 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 903 switch (hash) { 904 case -861311717: /*condition*/ return new String[] {"CodeableReference"}; 905 case 116103: /*use*/ return new String[] {"CodeableConcept"}; 906 default: return super.getTypesForProperty(hash, name); 907 } 908 909 } 910 911 @Override 912 public Base addChild(String name) throws FHIRException { 913 if (name.equals("condition")) { 914 return addCondition(); 915 } 916 else if (name.equals("use")) { 917 this.use = new CodeableConcept(); 918 return this.use; 919 } 920 else 921 return super.addChild(name); 922 } 923 924 public DiagnosisComponent copy() { 925 DiagnosisComponent dst = new DiagnosisComponent(); 926 copyValues(dst); 927 return dst; 928 } 929 930 public void copyValues(DiagnosisComponent dst) { 931 super.copyValues(dst); 932 if (condition != null) { 933 dst.condition = new ArrayList<CodeableReference>(); 934 for (CodeableReference i : condition) 935 dst.condition.add(i.copy()); 936 }; 937 dst.use = use == null ? null : use.copy(); 938 } 939 940 @Override 941 public boolean equalsDeep(Base other_) { 942 if (!super.equalsDeep(other_)) 943 return false; 944 if (!(other_ instanceof DiagnosisComponent)) 945 return false; 946 DiagnosisComponent o = (DiagnosisComponent) other_; 947 return compareDeep(condition, o.condition, true) && compareDeep(use, o.use, true); 948 } 949 950 @Override 951 public boolean equalsShallow(Base other_) { 952 if (!super.equalsShallow(other_)) 953 return false; 954 if (!(other_ instanceof DiagnosisComponent)) 955 return false; 956 DiagnosisComponent o = (DiagnosisComponent) other_; 957 return true; 958 } 959 960 public boolean isEmpty() { 961 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, use); 962 } 963 964 public String fhirType() { 965 return "EpisodeOfCare.diagnosis"; 966 967 } 968 969 } 970 971 /** 972 * The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes. 973 */ 974 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 975 @Description(shortDefinition="Business Identifier(s) relevant for this EpisodeOfCare", formalDefinition="The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes." ) 976 protected List<Identifier> identifier; 977 978 /** 979 * planned | waitlist | active | onhold | finished | cancelled. 980 */ 981 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 982 @Description(shortDefinition="planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition="planned | waitlist | active | onhold | finished | cancelled." ) 983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/episode-of-care-status") 984 protected Enumeration<EpisodeOfCareStatus> status; 985 986 /** 987 * The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource). 988 */ 989 @Child(name = "statusHistory", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 990 @Description(shortDefinition="Past list of status codes (the current status may be included to cover the start date of the status)", formalDefinition="The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource)." ) 991 protected List<EpisodeOfCareStatusHistoryComponent> statusHistory; 992 993 /** 994 * A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care. 995 */ 996 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 997 @Description(shortDefinition="Type/class - e.g. specialist referral, disease management", formalDefinition="A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care." ) 998 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/episodeofcare-type") 999 protected List<CodeableConcept> type; 1000 1001 /** 1002 * The list of medical reasons that are expected to be addressed during the episode of care. 1003 */ 1004 @Child(name = "reason", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1005 @Description(shortDefinition="The list of medical reasons that are expected to be addressed during the episode of care", formalDefinition="The list of medical reasons that are expected to be addressed during the episode of care." ) 1006 protected List<ReasonComponent> reason; 1007 1008 /** 1009 * The list of medical conditions that were addressed during the episode of care. 1010 */ 1011 @Child(name = "diagnosis", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1012 @Description(shortDefinition="The list of medical conditions that were addressed during the episode of care", formalDefinition="The list of medical conditions that were addressed during the episode of care." ) 1013 protected List<DiagnosisComponent> diagnosis; 1014 1015 /** 1016 * The patient who is the focus of this episode of care. 1017 */ 1018 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 1019 @Description(shortDefinition="The patient who is the focus of this episode of care", formalDefinition="The patient who is the focus of this episode of care." ) 1020 protected Reference patient; 1021 1022 /** 1023 * The organization that has assumed the specific responsibilities for care coordination, care delivery, or other services for the specified duration. 1024 */ 1025 @Child(name = "managingOrganization", type = {Organization.class}, order=7, min=0, max=1, modifier=false, summary=true) 1026 @Description(shortDefinition="Organization that assumes responsibility for care coordination", formalDefinition="The organization that has assumed the specific responsibilities for care coordination, care delivery, or other services for the specified duration." ) 1027 protected Reference managingOrganization; 1028 1029 /** 1030 * The interval during which the managing organization assumes the defined responsibility. 1031 */ 1032 @Child(name = "period", type = {Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 1033 @Description(shortDefinition="Interval during responsibility is assumed", formalDefinition="The interval during which the managing organization assumes the defined responsibility." ) 1034 protected Period period; 1035 1036 /** 1037 * Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals. 1038 */ 1039 @Child(name = "referralRequest", type = {ServiceRequest.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1040 @Description(shortDefinition="Originating Referral Request(s)", formalDefinition="Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals." ) 1041 protected List<Reference> referralRequest; 1042 1043 /** 1044 * The practitioner that is the care manager/care coordinator for this patient. 1045 */ 1046 @Child(name = "careManager", type = {Practitioner.class, PractitionerRole.class}, order=10, min=0, max=1, modifier=false, summary=false) 1047 @Description(shortDefinition="Care manager/care coordinator for the patient", formalDefinition="The practitioner that is the care manager/care coordinator for this patient." ) 1048 protected Reference careManager; 1049 1050 /** 1051 * The list of practitioners that may be facilitating this episode of care for specific purposes. 1052 */ 1053 @Child(name = "careTeam", type = {CareTeam.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1054 @Description(shortDefinition="Other practitioners facilitating this episode of care", formalDefinition="The list of practitioners that may be facilitating this episode of care for specific purposes." ) 1055 protected List<Reference> careTeam; 1056 1057 /** 1058 * The set of accounts that may be used for billing for this EpisodeOfCare. 1059 */ 1060 @Child(name = "account", type = {Account.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1061 @Description(shortDefinition="The set of accounts that may be used for billing for this EpisodeOfCare", formalDefinition="The set of accounts that may be used for billing for this EpisodeOfCare." ) 1062 protected List<Reference> account; 1063 1064 private static final long serialVersionUID = 678200746L; 1065 1066 /** 1067 * Constructor 1068 */ 1069 public EpisodeOfCare() { 1070 super(); 1071 } 1072 1073 /** 1074 * Constructor 1075 */ 1076 public EpisodeOfCare(EpisodeOfCareStatus status, Reference patient) { 1077 super(); 1078 this.setStatus(status); 1079 this.setPatient(patient); 1080 } 1081 1082 /** 1083 * @return {@link #identifier} (The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.) 1084 */ 1085 public List<Identifier> getIdentifier() { 1086 if (this.identifier == null) 1087 this.identifier = new ArrayList<Identifier>(); 1088 return this.identifier; 1089 } 1090 1091 /** 1092 * @return Returns a reference to <code>this</code> for easy method chaining 1093 */ 1094 public EpisodeOfCare setIdentifier(List<Identifier> theIdentifier) { 1095 this.identifier = theIdentifier; 1096 return this; 1097 } 1098 1099 public boolean hasIdentifier() { 1100 if (this.identifier == null) 1101 return false; 1102 for (Identifier item : this.identifier) 1103 if (!item.isEmpty()) 1104 return true; 1105 return false; 1106 } 1107 1108 public Identifier addIdentifier() { //3 1109 Identifier t = new Identifier(); 1110 if (this.identifier == null) 1111 this.identifier = new ArrayList<Identifier>(); 1112 this.identifier.add(t); 1113 return t; 1114 } 1115 1116 public EpisodeOfCare addIdentifier(Identifier t) { //3 1117 if (t == null) 1118 return this; 1119 if (this.identifier == null) 1120 this.identifier = new ArrayList<Identifier>(); 1121 this.identifier.add(t); 1122 return this; 1123 } 1124 1125 /** 1126 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1127 */ 1128 public Identifier getIdentifierFirstRep() { 1129 if (getIdentifier().isEmpty()) { 1130 addIdentifier(); 1131 } 1132 return getIdentifier().get(0); 1133 } 1134 1135 /** 1136 * @return {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1137 */ 1138 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 1139 if (this.status == null) 1140 if (Configuration.errorOnAutoCreate()) 1141 throw new Error("Attempt to auto-create EpisodeOfCare.status"); 1142 else if (Configuration.doAutoCreate()) 1143 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 1144 return this.status; 1145 } 1146 1147 public boolean hasStatusElement() { 1148 return this.status != null && !this.status.isEmpty(); 1149 } 1150 1151 public boolean hasStatus() { 1152 return this.status != null && !this.status.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1157 */ 1158 public EpisodeOfCare setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 1159 this.status = value; 1160 return this; 1161 } 1162 1163 /** 1164 * @return planned | waitlist | active | onhold | finished | cancelled. 1165 */ 1166 public EpisodeOfCareStatus getStatus() { 1167 return this.status == null ? null : this.status.getValue(); 1168 } 1169 1170 /** 1171 * @param value planned | waitlist | active | onhold | finished | cancelled. 1172 */ 1173 public EpisodeOfCare setStatus(EpisodeOfCareStatus value) { 1174 if (this.status == null) 1175 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 1176 this.status.setValue(value); 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #statusHistory} (The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).) 1182 */ 1183 public List<EpisodeOfCareStatusHistoryComponent> getStatusHistory() { 1184 if (this.statusHistory == null) 1185 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1186 return this.statusHistory; 1187 } 1188 1189 /** 1190 * @return Returns a reference to <code>this</code> for easy method chaining 1191 */ 1192 public EpisodeOfCare setStatusHistory(List<EpisodeOfCareStatusHistoryComponent> theStatusHistory) { 1193 this.statusHistory = theStatusHistory; 1194 return this; 1195 } 1196 1197 public boolean hasStatusHistory() { 1198 if (this.statusHistory == null) 1199 return false; 1200 for (EpisodeOfCareStatusHistoryComponent item : this.statusHistory) 1201 if (!item.isEmpty()) 1202 return true; 1203 return false; 1204 } 1205 1206 public EpisodeOfCareStatusHistoryComponent addStatusHistory() { //3 1207 EpisodeOfCareStatusHistoryComponent t = new EpisodeOfCareStatusHistoryComponent(); 1208 if (this.statusHistory == null) 1209 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1210 this.statusHistory.add(t); 1211 return t; 1212 } 1213 1214 public EpisodeOfCare addStatusHistory(EpisodeOfCareStatusHistoryComponent t) { //3 1215 if (t == null) 1216 return this; 1217 if (this.statusHistory == null) 1218 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1219 this.statusHistory.add(t); 1220 return this; 1221 } 1222 1223 /** 1224 * @return The first repetition of repeating field {@link #statusHistory}, creating it if it does not already exist {3} 1225 */ 1226 public EpisodeOfCareStatusHistoryComponent getStatusHistoryFirstRep() { 1227 if (getStatusHistory().isEmpty()) { 1228 addStatusHistory(); 1229 } 1230 return getStatusHistory().get(0); 1231 } 1232 1233 /** 1234 * @return {@link #type} (A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.) 1235 */ 1236 public List<CodeableConcept> getType() { 1237 if (this.type == null) 1238 this.type = new ArrayList<CodeableConcept>(); 1239 return this.type; 1240 } 1241 1242 /** 1243 * @return Returns a reference to <code>this</code> for easy method chaining 1244 */ 1245 public EpisodeOfCare setType(List<CodeableConcept> theType) { 1246 this.type = theType; 1247 return this; 1248 } 1249 1250 public boolean hasType() { 1251 if (this.type == null) 1252 return false; 1253 for (CodeableConcept item : this.type) 1254 if (!item.isEmpty()) 1255 return true; 1256 return false; 1257 } 1258 1259 public CodeableConcept addType() { //3 1260 CodeableConcept t = new CodeableConcept(); 1261 if (this.type == null) 1262 this.type = new ArrayList<CodeableConcept>(); 1263 this.type.add(t); 1264 return t; 1265 } 1266 1267 public EpisodeOfCare addType(CodeableConcept t) { //3 1268 if (t == null) 1269 return this; 1270 if (this.type == null) 1271 this.type = new ArrayList<CodeableConcept>(); 1272 this.type.add(t); 1273 return this; 1274 } 1275 1276 /** 1277 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 1278 */ 1279 public CodeableConcept getTypeFirstRep() { 1280 if (getType().isEmpty()) { 1281 addType(); 1282 } 1283 return getType().get(0); 1284 } 1285 1286 /** 1287 * @return {@link #reason} (The list of medical reasons that are expected to be addressed during the episode of care.) 1288 */ 1289 public List<ReasonComponent> getReason() { 1290 if (this.reason == null) 1291 this.reason = new ArrayList<ReasonComponent>(); 1292 return this.reason; 1293 } 1294 1295 /** 1296 * @return Returns a reference to <code>this</code> for easy method chaining 1297 */ 1298 public EpisodeOfCare setReason(List<ReasonComponent> theReason) { 1299 this.reason = theReason; 1300 return this; 1301 } 1302 1303 public boolean hasReason() { 1304 if (this.reason == null) 1305 return false; 1306 for (ReasonComponent item : this.reason) 1307 if (!item.isEmpty()) 1308 return true; 1309 return false; 1310 } 1311 1312 public ReasonComponent addReason() { //3 1313 ReasonComponent t = new ReasonComponent(); 1314 if (this.reason == null) 1315 this.reason = new ArrayList<ReasonComponent>(); 1316 this.reason.add(t); 1317 return t; 1318 } 1319 1320 public EpisodeOfCare addReason(ReasonComponent t) { //3 1321 if (t == null) 1322 return this; 1323 if (this.reason == null) 1324 this.reason = new ArrayList<ReasonComponent>(); 1325 this.reason.add(t); 1326 return this; 1327 } 1328 1329 /** 1330 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1331 */ 1332 public ReasonComponent getReasonFirstRep() { 1333 if (getReason().isEmpty()) { 1334 addReason(); 1335 } 1336 return getReason().get(0); 1337 } 1338 1339 /** 1340 * @return {@link #diagnosis} (The list of medical conditions that were addressed during the episode of care.) 1341 */ 1342 public List<DiagnosisComponent> getDiagnosis() { 1343 if (this.diagnosis == null) 1344 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1345 return this.diagnosis; 1346 } 1347 1348 /** 1349 * @return Returns a reference to <code>this</code> for easy method chaining 1350 */ 1351 public EpisodeOfCare setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 1352 this.diagnosis = theDiagnosis; 1353 return this; 1354 } 1355 1356 public boolean hasDiagnosis() { 1357 if (this.diagnosis == null) 1358 return false; 1359 for (DiagnosisComponent item : this.diagnosis) 1360 if (!item.isEmpty()) 1361 return true; 1362 return false; 1363 } 1364 1365 public DiagnosisComponent addDiagnosis() { //3 1366 DiagnosisComponent t = new DiagnosisComponent(); 1367 if (this.diagnosis == null) 1368 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1369 this.diagnosis.add(t); 1370 return t; 1371 } 1372 1373 public EpisodeOfCare addDiagnosis(DiagnosisComponent t) { //3 1374 if (t == null) 1375 return this; 1376 if (this.diagnosis == null) 1377 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1378 this.diagnosis.add(t); 1379 return this; 1380 } 1381 1382 /** 1383 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 1384 */ 1385 public DiagnosisComponent getDiagnosisFirstRep() { 1386 if (getDiagnosis().isEmpty()) { 1387 addDiagnosis(); 1388 } 1389 return getDiagnosis().get(0); 1390 } 1391 1392 /** 1393 * @return {@link #patient} (The patient who is the focus of this episode of care.) 1394 */ 1395 public Reference getPatient() { 1396 if (this.patient == null) 1397 if (Configuration.errorOnAutoCreate()) 1398 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1399 else if (Configuration.doAutoCreate()) 1400 this.patient = new Reference(); // cc 1401 return this.patient; 1402 } 1403 1404 public boolean hasPatient() { 1405 return this.patient != null && !this.patient.isEmpty(); 1406 } 1407 1408 /** 1409 * @param value {@link #patient} (The patient who is the focus of this episode of care.) 1410 */ 1411 public EpisodeOfCare setPatient(Reference value) { 1412 this.patient = value; 1413 return this; 1414 } 1415 1416 /** 1417 * @return {@link #managingOrganization} (The organization that has assumed the specific responsibilities for care coordination, care delivery, or other services for the specified duration.) 1418 */ 1419 public Reference getManagingOrganization() { 1420 if (this.managingOrganization == null) 1421 if (Configuration.errorOnAutoCreate()) 1422 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1423 else if (Configuration.doAutoCreate()) 1424 this.managingOrganization = new Reference(); // cc 1425 return this.managingOrganization; 1426 } 1427 1428 public boolean hasManagingOrganization() { 1429 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1430 } 1431 1432 /** 1433 * @param value {@link #managingOrganization} (The organization that has assumed the specific responsibilities for care coordination, care delivery, or other services for the specified duration.) 1434 */ 1435 public EpisodeOfCare setManagingOrganization(Reference value) { 1436 this.managingOrganization = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #period} (The interval during which the managing organization assumes the defined responsibility.) 1442 */ 1443 public Period getPeriod() { 1444 if (this.period == null) 1445 if (Configuration.errorOnAutoCreate()) 1446 throw new Error("Attempt to auto-create EpisodeOfCare.period"); 1447 else if (Configuration.doAutoCreate()) 1448 this.period = new Period(); // cc 1449 return this.period; 1450 } 1451 1452 public boolean hasPeriod() { 1453 return this.period != null && !this.period.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #period} (The interval during which the managing organization assumes the defined responsibility.) 1458 */ 1459 public EpisodeOfCare setPeriod(Period value) { 1460 this.period = value; 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #referralRequest} (Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.) 1466 */ 1467 public List<Reference> getReferralRequest() { 1468 if (this.referralRequest == null) 1469 this.referralRequest = new ArrayList<Reference>(); 1470 return this.referralRequest; 1471 } 1472 1473 /** 1474 * @return Returns a reference to <code>this</code> for easy method chaining 1475 */ 1476 public EpisodeOfCare setReferralRequest(List<Reference> theReferralRequest) { 1477 this.referralRequest = theReferralRequest; 1478 return this; 1479 } 1480 1481 public boolean hasReferralRequest() { 1482 if (this.referralRequest == null) 1483 return false; 1484 for (Reference item : this.referralRequest) 1485 if (!item.isEmpty()) 1486 return true; 1487 return false; 1488 } 1489 1490 public Reference addReferralRequest() { //3 1491 Reference t = new Reference(); 1492 if (this.referralRequest == null) 1493 this.referralRequest = new ArrayList<Reference>(); 1494 this.referralRequest.add(t); 1495 return t; 1496 } 1497 1498 public EpisodeOfCare addReferralRequest(Reference t) { //3 1499 if (t == null) 1500 return this; 1501 if (this.referralRequest == null) 1502 this.referralRequest = new ArrayList<Reference>(); 1503 this.referralRequest.add(t); 1504 return this; 1505 } 1506 1507 /** 1508 * @return The first repetition of repeating field {@link #referralRequest}, creating it if it does not already exist {3} 1509 */ 1510 public Reference getReferralRequestFirstRep() { 1511 if (getReferralRequest().isEmpty()) { 1512 addReferralRequest(); 1513 } 1514 return getReferralRequest().get(0); 1515 } 1516 1517 /** 1518 * @return {@link #careManager} (The practitioner that is the care manager/care coordinator for this patient.) 1519 */ 1520 public Reference getCareManager() { 1521 if (this.careManager == null) 1522 if (Configuration.errorOnAutoCreate()) 1523 throw new Error("Attempt to auto-create EpisodeOfCare.careManager"); 1524 else if (Configuration.doAutoCreate()) 1525 this.careManager = new Reference(); // cc 1526 return this.careManager; 1527 } 1528 1529 public boolean hasCareManager() { 1530 return this.careManager != null && !this.careManager.isEmpty(); 1531 } 1532 1533 /** 1534 * @param value {@link #careManager} (The practitioner that is the care manager/care coordinator for this patient.) 1535 */ 1536 public EpisodeOfCare setCareManager(Reference value) { 1537 this.careManager = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return {@link #careTeam} (The list of practitioners that may be facilitating this episode of care for specific purposes.) 1543 */ 1544 public List<Reference> getCareTeam() { 1545 if (this.careTeam == null) 1546 this.careTeam = new ArrayList<Reference>(); 1547 return this.careTeam; 1548 } 1549 1550 /** 1551 * @return Returns a reference to <code>this</code> for easy method chaining 1552 */ 1553 public EpisodeOfCare setCareTeam(List<Reference> theCareTeam) { 1554 this.careTeam = theCareTeam; 1555 return this; 1556 } 1557 1558 public boolean hasCareTeam() { 1559 if (this.careTeam == null) 1560 return false; 1561 for (Reference item : this.careTeam) 1562 if (!item.isEmpty()) 1563 return true; 1564 return false; 1565 } 1566 1567 public Reference addCareTeam() { //3 1568 Reference t = new Reference(); 1569 if (this.careTeam == null) 1570 this.careTeam = new ArrayList<Reference>(); 1571 this.careTeam.add(t); 1572 return t; 1573 } 1574 1575 public EpisodeOfCare addCareTeam(Reference t) { //3 1576 if (t == null) 1577 return this; 1578 if (this.careTeam == null) 1579 this.careTeam = new ArrayList<Reference>(); 1580 this.careTeam.add(t); 1581 return this; 1582 } 1583 1584 /** 1585 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist {3} 1586 */ 1587 public Reference getCareTeamFirstRep() { 1588 if (getCareTeam().isEmpty()) { 1589 addCareTeam(); 1590 } 1591 return getCareTeam().get(0); 1592 } 1593 1594 /** 1595 * @return {@link #account} (The set of accounts that may be used for billing for this EpisodeOfCare.) 1596 */ 1597 public List<Reference> getAccount() { 1598 if (this.account == null) 1599 this.account = new ArrayList<Reference>(); 1600 return this.account; 1601 } 1602 1603 /** 1604 * @return Returns a reference to <code>this</code> for easy method chaining 1605 */ 1606 public EpisodeOfCare setAccount(List<Reference> theAccount) { 1607 this.account = theAccount; 1608 return this; 1609 } 1610 1611 public boolean hasAccount() { 1612 if (this.account == null) 1613 return false; 1614 for (Reference item : this.account) 1615 if (!item.isEmpty()) 1616 return true; 1617 return false; 1618 } 1619 1620 public Reference addAccount() { //3 1621 Reference t = new Reference(); 1622 if (this.account == null) 1623 this.account = new ArrayList<Reference>(); 1624 this.account.add(t); 1625 return t; 1626 } 1627 1628 public EpisodeOfCare addAccount(Reference t) { //3 1629 if (t == null) 1630 return this; 1631 if (this.account == null) 1632 this.account = new ArrayList<Reference>(); 1633 this.account.add(t); 1634 return this; 1635 } 1636 1637 /** 1638 * @return The first repetition of repeating field {@link #account}, creating it if it does not already exist {3} 1639 */ 1640 public Reference getAccountFirstRep() { 1641 if (getAccount().isEmpty()) { 1642 addAccount(); 1643 } 1644 return getAccount().get(0); 1645 } 1646 1647 protected void listChildren(List<Property> children) { 1648 super.listChildren(children); 1649 children.add(new Property("identifier", "Identifier", "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1650 children.add(new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 1651 children.add(new Property("statusHistory", "", "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 0, java.lang.Integer.MAX_VALUE, statusHistory)); 1652 children.add(new Property("type", "CodeableConcept", "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 0, java.lang.Integer.MAX_VALUE, type)); 1653 children.add(new Property("reason", "", "The list of medical reasons that are expected to be addressed during the episode of care.", 0, java.lang.Integer.MAX_VALUE, reason)); 1654 children.add(new Property("diagnosis", "", "The list of medical conditions that were addressed during the episode of care.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 1655 children.add(new Property("patient", "Reference(Patient)", "The patient who is the focus of this episode of care.", 0, 1, patient)); 1656 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization that has assumed the specific responsibilities for care coordination, care delivery, or other services for the specified duration.", 0, 1, managingOrganization)); 1657 children.add(new Property("period", "Period", "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period)); 1658 children.add(new Property("referralRequest", "Reference(ServiceRequest)", "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, java.lang.Integer.MAX_VALUE, referralRequest)); 1659 children.add(new Property("careManager", "Reference(Practitioner|PractitionerRole)", "The practitioner that is the care manager/care coordinator for this patient.", 0, 1, careManager)); 1660 children.add(new Property("careTeam", "Reference(CareTeam)", "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 1661 children.add(new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, account)); 1662 } 1663 1664 @Override 1665 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1666 switch (_hash) { 1667 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 0, java.lang.Integer.MAX_VALUE, identifier); 1668 case -892481550: /*status*/ return new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status); 1669 case -986695614: /*statusHistory*/ return new Property("statusHistory", "", "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 0, java.lang.Integer.MAX_VALUE, statusHistory); 1670 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 0, java.lang.Integer.MAX_VALUE, type); 1671 case -934964668: /*reason*/ return new Property("reason", "", "The list of medical reasons that are expected to be addressed during the episode of care.", 0, java.lang.Integer.MAX_VALUE, reason); 1672 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "The list of medical conditions that were addressed during the episode of care.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 1673 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who is the focus of this episode of care.", 0, 1, patient); 1674 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization that has assumed the specific responsibilities for care coordination, care delivery, or other services for the specified duration.", 0, 1, managingOrganization); 1675 case -991726143: /*period*/ return new Property("period", "Period", "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period); 1676 case -310299598: /*referralRequest*/ return new Property("referralRequest", "Reference(ServiceRequest)", "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, java.lang.Integer.MAX_VALUE, referralRequest); 1677 case -1147746468: /*careManager*/ return new Property("careManager", "Reference(Practitioner|PractitionerRole)", "The practitioner that is the care manager/care coordinator for this patient.", 0, 1, careManager); 1678 case -7323378: /*careTeam*/ return new Property("careTeam", "Reference(CareTeam)", "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, java.lang.Integer.MAX_VALUE, careTeam); 1679 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, account); 1680 default: return super.getNamedProperty(_hash, _name, _checkValid); 1681 } 1682 1683 } 1684 1685 @Override 1686 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1687 switch (hash) { 1688 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1689 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EpisodeOfCareStatus> 1690 case -986695614: /*statusHistory*/ return this.statusHistory == null ? new Base[0] : this.statusHistory.toArray(new Base[this.statusHistory.size()]); // EpisodeOfCareStatusHistoryComponent 1691 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1692 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // ReasonComponent 1693 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 1694 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1695 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 1696 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1697 case -310299598: /*referralRequest*/ return this.referralRequest == null ? new Base[0] : this.referralRequest.toArray(new Base[this.referralRequest.size()]); // Reference 1698 case -1147746468: /*careManager*/ return this.careManager == null ? new Base[0] : new Base[] {this.careManager}; // Reference 1699 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // Reference 1700 case -1177318867: /*account*/ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 1701 default: return super.getProperty(hash, name, checkValid); 1702 } 1703 1704 } 1705 1706 @Override 1707 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1708 switch (hash) { 1709 case -1618432855: // identifier 1710 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1711 return value; 1712 case -892481550: // status 1713 value = new EpisodeOfCareStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1714 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1715 return value; 1716 case -986695614: // statusHistory 1717 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); // EpisodeOfCareStatusHistoryComponent 1718 return value; 1719 case 3575610: // type 1720 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1721 return value; 1722 case -934964668: // reason 1723 this.getReason().add((ReasonComponent) value); // ReasonComponent 1724 return value; 1725 case 1196993265: // diagnosis 1726 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 1727 return value; 1728 case -791418107: // patient 1729 this.patient = TypeConvertor.castToReference(value); // Reference 1730 return value; 1731 case -2058947787: // managingOrganization 1732 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1733 return value; 1734 case -991726143: // period 1735 this.period = TypeConvertor.castToPeriod(value); // Period 1736 return value; 1737 case -310299598: // referralRequest 1738 this.getReferralRequest().add(TypeConvertor.castToReference(value)); // Reference 1739 return value; 1740 case -1147746468: // careManager 1741 this.careManager = TypeConvertor.castToReference(value); // Reference 1742 return value; 1743 case -7323378: // careTeam 1744 this.getCareTeam().add(TypeConvertor.castToReference(value)); // Reference 1745 return value; 1746 case -1177318867: // account 1747 this.getAccount().add(TypeConvertor.castToReference(value)); // Reference 1748 return value; 1749 default: return super.setProperty(hash, name, value); 1750 } 1751 1752 } 1753 1754 @Override 1755 public Base setProperty(String name, Base value) throws FHIRException { 1756 if (name.equals("identifier")) { 1757 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1758 } else if (name.equals("status")) { 1759 value = new EpisodeOfCareStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1760 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1761 } else if (name.equals("statusHistory")) { 1762 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); 1763 } else if (name.equals("type")) { 1764 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1765 } else if (name.equals("reason")) { 1766 this.getReason().add((ReasonComponent) value); 1767 } else if (name.equals("diagnosis")) { 1768 this.getDiagnosis().add((DiagnosisComponent) value); 1769 } else if (name.equals("patient")) { 1770 this.patient = TypeConvertor.castToReference(value); // Reference 1771 } else if (name.equals("managingOrganization")) { 1772 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1773 } else if (name.equals("period")) { 1774 this.period = TypeConvertor.castToPeriod(value); // Period 1775 } else if (name.equals("referralRequest")) { 1776 this.getReferralRequest().add(TypeConvertor.castToReference(value)); 1777 } else if (name.equals("careManager")) { 1778 this.careManager = TypeConvertor.castToReference(value); // Reference 1779 } else if (name.equals("careTeam")) { 1780 this.getCareTeam().add(TypeConvertor.castToReference(value)); 1781 } else if (name.equals("account")) { 1782 this.getAccount().add(TypeConvertor.castToReference(value)); 1783 } else 1784 return super.setProperty(name, value); 1785 return value; 1786 } 1787 1788 @Override 1789 public void removeChild(String name, Base value) throws FHIRException { 1790 if (name.equals("identifier")) { 1791 this.getIdentifier().remove(value); 1792 } else if (name.equals("status")) { 1793 value = new EpisodeOfCareStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1794 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1795 } else if (name.equals("statusHistory")) { 1796 this.getStatusHistory().remove((EpisodeOfCareStatusHistoryComponent) value); 1797 } else if (name.equals("type")) { 1798 this.getType().remove(value); 1799 } else if (name.equals("reason")) { 1800 this.getReason().remove((ReasonComponent) value); 1801 } else if (name.equals("diagnosis")) { 1802 this.getDiagnosis().remove((DiagnosisComponent) value); 1803 } else if (name.equals("patient")) { 1804 this.patient = null; 1805 } else if (name.equals("managingOrganization")) { 1806 this.managingOrganization = null; 1807 } else if (name.equals("period")) { 1808 this.period = null; 1809 } else if (name.equals("referralRequest")) { 1810 this.getReferralRequest().remove(value); 1811 } else if (name.equals("careManager")) { 1812 this.careManager = null; 1813 } else if (name.equals("careTeam")) { 1814 this.getCareTeam().remove(value); 1815 } else if (name.equals("account")) { 1816 this.getAccount().remove(value); 1817 } else 1818 super.removeChild(name, value); 1819 1820 } 1821 1822 @Override 1823 public Base makeProperty(int hash, String name) throws FHIRException { 1824 switch (hash) { 1825 case -1618432855: return addIdentifier(); 1826 case -892481550: return getStatusElement(); 1827 case -986695614: return addStatusHistory(); 1828 case 3575610: return addType(); 1829 case -934964668: return addReason(); 1830 case 1196993265: return addDiagnosis(); 1831 case -791418107: return getPatient(); 1832 case -2058947787: return getManagingOrganization(); 1833 case -991726143: return getPeriod(); 1834 case -310299598: return addReferralRequest(); 1835 case -1147746468: return getCareManager(); 1836 case -7323378: return addCareTeam(); 1837 case -1177318867: return addAccount(); 1838 default: return super.makeProperty(hash, name); 1839 } 1840 1841 } 1842 1843 @Override 1844 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1845 switch (hash) { 1846 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1847 case -892481550: /*status*/ return new String[] {"code"}; 1848 case -986695614: /*statusHistory*/ return new String[] {}; 1849 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1850 case -934964668: /*reason*/ return new String[] {}; 1851 case 1196993265: /*diagnosis*/ return new String[] {}; 1852 case -791418107: /*patient*/ return new String[] {"Reference"}; 1853 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1854 case -991726143: /*period*/ return new String[] {"Period"}; 1855 case -310299598: /*referralRequest*/ return new String[] {"Reference"}; 1856 case -1147746468: /*careManager*/ return new String[] {"Reference"}; 1857 case -7323378: /*careTeam*/ return new String[] {"Reference"}; 1858 case -1177318867: /*account*/ return new String[] {"Reference"}; 1859 default: return super.getTypesForProperty(hash, name); 1860 } 1861 1862 } 1863 1864 @Override 1865 public Base addChild(String name) throws FHIRException { 1866 if (name.equals("identifier")) { 1867 return addIdentifier(); 1868 } 1869 else if (name.equals("status")) { 1870 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 1871 } 1872 else if (name.equals("statusHistory")) { 1873 return addStatusHistory(); 1874 } 1875 else if (name.equals("type")) { 1876 return addType(); 1877 } 1878 else if (name.equals("reason")) { 1879 return addReason(); 1880 } 1881 else if (name.equals("diagnosis")) { 1882 return addDiagnosis(); 1883 } 1884 else if (name.equals("patient")) { 1885 this.patient = new Reference(); 1886 return this.patient; 1887 } 1888 else if (name.equals("managingOrganization")) { 1889 this.managingOrganization = new Reference(); 1890 return this.managingOrganization; 1891 } 1892 else if (name.equals("period")) { 1893 this.period = new Period(); 1894 return this.period; 1895 } 1896 else if (name.equals("referralRequest")) { 1897 return addReferralRequest(); 1898 } 1899 else if (name.equals("careManager")) { 1900 this.careManager = new Reference(); 1901 return this.careManager; 1902 } 1903 else if (name.equals("careTeam")) { 1904 return addCareTeam(); 1905 } 1906 else if (name.equals("account")) { 1907 return addAccount(); 1908 } 1909 else 1910 return super.addChild(name); 1911 } 1912 1913 public String fhirType() { 1914 return "EpisodeOfCare"; 1915 1916 } 1917 1918 public EpisodeOfCare copy() { 1919 EpisodeOfCare dst = new EpisodeOfCare(); 1920 copyValues(dst); 1921 return dst; 1922 } 1923 1924 public void copyValues(EpisodeOfCare dst) { 1925 super.copyValues(dst); 1926 if (identifier != null) { 1927 dst.identifier = new ArrayList<Identifier>(); 1928 for (Identifier i : identifier) 1929 dst.identifier.add(i.copy()); 1930 }; 1931 dst.status = status == null ? null : status.copy(); 1932 if (statusHistory != null) { 1933 dst.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1934 for (EpisodeOfCareStatusHistoryComponent i : statusHistory) 1935 dst.statusHistory.add(i.copy()); 1936 }; 1937 if (type != null) { 1938 dst.type = new ArrayList<CodeableConcept>(); 1939 for (CodeableConcept i : type) 1940 dst.type.add(i.copy()); 1941 }; 1942 if (reason != null) { 1943 dst.reason = new ArrayList<ReasonComponent>(); 1944 for (ReasonComponent i : reason) 1945 dst.reason.add(i.copy()); 1946 }; 1947 if (diagnosis != null) { 1948 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 1949 for (DiagnosisComponent i : diagnosis) 1950 dst.diagnosis.add(i.copy()); 1951 }; 1952 dst.patient = patient == null ? null : patient.copy(); 1953 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1954 dst.period = period == null ? null : period.copy(); 1955 if (referralRequest != null) { 1956 dst.referralRequest = new ArrayList<Reference>(); 1957 for (Reference i : referralRequest) 1958 dst.referralRequest.add(i.copy()); 1959 }; 1960 dst.careManager = careManager == null ? null : careManager.copy(); 1961 if (careTeam != null) { 1962 dst.careTeam = new ArrayList<Reference>(); 1963 for (Reference i : careTeam) 1964 dst.careTeam.add(i.copy()); 1965 }; 1966 if (account != null) { 1967 dst.account = new ArrayList<Reference>(); 1968 for (Reference i : account) 1969 dst.account.add(i.copy()); 1970 }; 1971 } 1972 1973 protected EpisodeOfCare typedCopy() { 1974 return copy(); 1975 } 1976 1977 @Override 1978 public boolean equalsDeep(Base other_) { 1979 if (!super.equalsDeep(other_)) 1980 return false; 1981 if (!(other_ instanceof EpisodeOfCare)) 1982 return false; 1983 EpisodeOfCare o = (EpisodeOfCare) other_; 1984 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(statusHistory, o.statusHistory, true) 1985 && compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true) && compareDeep(diagnosis, o.diagnosis, true) 1986 && compareDeep(patient, o.patient, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1987 && compareDeep(period, o.period, true) && compareDeep(referralRequest, o.referralRequest, true) 1988 && compareDeep(careManager, o.careManager, true) && compareDeep(careTeam, o.careTeam, true) && compareDeep(account, o.account, true) 1989 ; 1990 } 1991 1992 @Override 1993 public boolean equalsShallow(Base other_) { 1994 if (!super.equalsShallow(other_)) 1995 return false; 1996 if (!(other_ instanceof EpisodeOfCare)) 1997 return false; 1998 EpisodeOfCare o = (EpisodeOfCare) other_; 1999 return compareValues(status, o.status, true); 2000 } 2001 2002 public boolean isEmpty() { 2003 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusHistory 2004 , type, reason, diagnosis, patient, managingOrganization, period, referralRequest 2005 , careManager, careTeam, account); 2006 } 2007 2008 @Override 2009 public ResourceType getResourceType() { 2010 return ResourceType.EpisodeOfCare; 2011 } 2012 2013 /** 2014 * Search parameter: <b>care-manager</b> 2015 * <p> 2016 * Description: <b>Care manager/care coordinator for the patient</b><br> 2017 * Type: <b>reference</b><br> 2018 * Path: <b>EpisodeOfCare.careManager.where(resolve() is Practitioner)</b><br> 2019 * </p> 2020 */ 2021 @SearchParamDefinition(name="care-manager", path="EpisodeOfCare.careManager.where(resolve() is Practitioner)", description="Care manager/care coordinator for the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Practitioner.class } ) 2022 public static final String SP_CARE_MANAGER = "care-manager"; 2023 /** 2024 * <b>Fluent Client</b> search parameter constant for <b>care-manager</b> 2025 * <p> 2026 * Description: <b>Care manager/care coordinator for the patient</b><br> 2027 * Type: <b>reference</b><br> 2028 * Path: <b>EpisodeOfCare.careManager.where(resolve() is Practitioner)</b><br> 2029 * </p> 2030 */ 2031 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_MANAGER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_MANAGER); 2032 2033/** 2034 * Constant for fluent queries to be used to add include statements. Specifies 2035 * the path value of "<b>EpisodeOfCare:care-manager</b>". 2036 */ 2037 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_MANAGER = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:care-manager").toLocked(); 2038 2039 /** 2040 * Search parameter: <b>diagnosis-code</b> 2041 * <p> 2042 * Description: <b>Conditions/problems/diagnoses this episode of care is for (coded)</b><br> 2043 * Type: <b>token</b><br> 2044 * Path: <b>EpisodeOfCare.diagnosis.condition.concept</b><br> 2045 * </p> 2046 */ 2047 @SearchParamDefinition(name="diagnosis-code", path="EpisodeOfCare.diagnosis.condition.concept", description="Conditions/problems/diagnoses this episode of care is for (coded)", type="token" ) 2048 public static final String SP_DIAGNOSIS_CODE = "diagnosis-code"; 2049 /** 2050 * <b>Fluent Client</b> search parameter constant for <b>diagnosis-code</b> 2051 * <p> 2052 * Description: <b>Conditions/problems/diagnoses this episode of care is for (coded)</b><br> 2053 * Type: <b>token</b><br> 2054 * Path: <b>EpisodeOfCare.diagnosis.condition.concept</b><br> 2055 * </p> 2056 */ 2057 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DIAGNOSIS_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DIAGNOSIS_CODE); 2058 2059 /** 2060 * Search parameter: <b>diagnosis-reference</b> 2061 * <p> 2062 * Description: <b>Conditions/problems/diagnoses this episode of care is for (resource reference)</b><br> 2063 * Type: <b>reference</b><br> 2064 * Path: <b>EpisodeOfCare.diagnosis.condition.reference</b><br> 2065 * </p> 2066 */ 2067 @SearchParamDefinition(name="diagnosis-reference", path="EpisodeOfCare.diagnosis.condition.reference", description="Conditions/problems/diagnoses this episode of care is for (resource reference)", type="reference", target={Condition.class } ) 2068 public static final String SP_DIAGNOSIS_REFERENCE = "diagnosis-reference"; 2069 /** 2070 * <b>Fluent Client</b> search parameter constant for <b>diagnosis-reference</b> 2071 * <p> 2072 * Description: <b>Conditions/problems/diagnoses this episode of care is for (resource reference)</b><br> 2073 * Type: <b>reference</b><br> 2074 * Path: <b>EpisodeOfCare.diagnosis.condition.reference</b><br> 2075 * </p> 2076 */ 2077 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DIAGNOSIS_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DIAGNOSIS_REFERENCE); 2078 2079/** 2080 * Constant for fluent queries to be used to add include statements. Specifies 2081 * the path value of "<b>EpisodeOfCare:diagnosis-reference</b>". 2082 */ 2083 public static final ca.uhn.fhir.model.api.Include INCLUDE_DIAGNOSIS_REFERENCE = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:diagnosis-reference").toLocked(); 2084 2085 /** 2086 * Search parameter: <b>incoming-referral</b> 2087 * <p> 2088 * Description: <b>Incoming Referral Request</b><br> 2089 * Type: <b>reference</b><br> 2090 * Path: <b>EpisodeOfCare.referralRequest</b><br> 2091 * </p> 2092 */ 2093 @SearchParamDefinition(name="incoming-referral", path="EpisodeOfCare.referralRequest", description="Incoming Referral Request", type="reference", target={ServiceRequest.class } ) 2094 public static final String SP_INCOMING_REFERRAL = "incoming-referral"; 2095 /** 2096 * <b>Fluent Client</b> search parameter constant for <b>incoming-referral</b> 2097 * <p> 2098 * Description: <b>Incoming Referral Request</b><br> 2099 * Type: <b>reference</b><br> 2100 * Path: <b>EpisodeOfCare.referralRequest</b><br> 2101 * </p> 2102 */ 2103 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INCOMING_REFERRAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INCOMING_REFERRAL); 2104 2105/** 2106 * Constant for fluent queries to be used to add include statements. Specifies 2107 * the path value of "<b>EpisodeOfCare:incoming-referral</b>". 2108 */ 2109 public static final ca.uhn.fhir.model.api.Include INCLUDE_INCOMING_REFERRAL = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:incoming-referral").toLocked(); 2110 2111 /** 2112 * Search parameter: <b>organization</b> 2113 * <p> 2114 * Description: <b>The organization that has assumed the specific responsibilities of this EpisodeOfCare</b><br> 2115 * Type: <b>reference</b><br> 2116 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 2117 * </p> 2118 */ 2119 @SearchParamDefinition(name="organization", path="EpisodeOfCare.managingOrganization", description="The organization that has assumed the specific responsibilities of this EpisodeOfCare", type="reference", target={Organization.class } ) 2120 public static final String SP_ORGANIZATION = "organization"; 2121 /** 2122 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2123 * <p> 2124 * Description: <b>The organization that has assumed the specific responsibilities of this EpisodeOfCare</b><br> 2125 * Type: <b>reference</b><br> 2126 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 2127 * </p> 2128 */ 2129 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2130 2131/** 2132 * Constant for fluent queries to be used to add include statements. Specifies 2133 * the path value of "<b>EpisodeOfCare:organization</b>". 2134 */ 2135 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:organization").toLocked(); 2136 2137 /** 2138 * Search parameter: <b>reason-code</b> 2139 * <p> 2140 * Description: <b>Reference to a concept (coded)</b><br> 2141 * Type: <b>token</b><br> 2142 * Path: <b>EpisodeOfCare.reason.value.concept</b><br> 2143 * </p> 2144 */ 2145 @SearchParamDefinition(name="reason-code", path="EpisodeOfCare.reason.value.concept", description="Reference to a concept (coded)", type="token" ) 2146 public static final String SP_REASON_CODE = "reason-code"; 2147 /** 2148 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 2149 * <p> 2150 * Description: <b>Reference to a concept (coded)</b><br> 2151 * Type: <b>token</b><br> 2152 * Path: <b>EpisodeOfCare.reason.value.concept</b><br> 2153 * </p> 2154 */ 2155 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_CODE); 2156 2157 /** 2158 * Search parameter: <b>reason-reference</b> 2159 * <p> 2160 * Description: <b>Reference to a resource (resource reference)</b><br> 2161 * Type: <b>reference</b><br> 2162 * Path: <b>EpisodeOfCare.reason.value.reference</b><br> 2163 * </p> 2164 */ 2165 @SearchParamDefinition(name="reason-reference", path="EpisodeOfCare.reason.value.reference", description="Reference to a resource (resource reference)", type="reference", target={Condition.class, HealthcareService.class, Observation.class, Procedure.class } ) 2166 public static final String SP_REASON_REFERENCE = "reason-reference"; 2167 /** 2168 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 2169 * <p> 2170 * Description: <b>Reference to a resource (resource reference)</b><br> 2171 * Type: <b>reference</b><br> 2172 * Path: <b>EpisodeOfCare.reason.value.reference</b><br> 2173 * </p> 2174 */ 2175 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REASON_REFERENCE); 2176 2177/** 2178 * Constant for fluent queries to be used to add include statements. Specifies 2179 * the path value of "<b>EpisodeOfCare:reason-reference</b>". 2180 */ 2181 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:reason-reference").toLocked(); 2182 2183 /** 2184 * Search parameter: <b>status</b> 2185 * <p> 2186 * Description: <b>The current status of the Episode of Care as provided (does not check the status history collection)</b><br> 2187 * Type: <b>token</b><br> 2188 * Path: <b>EpisodeOfCare.status</b><br> 2189 * </p> 2190 */ 2191 @SearchParamDefinition(name="status", path="EpisodeOfCare.status", description="The current status of the Episode of Care as provided (does not check the status history collection)", type="token" ) 2192 public static final String SP_STATUS = "status"; 2193 /** 2194 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2195 * <p> 2196 * Description: <b>The current status of the Episode of Care as provided (does not check the status history collection)</b><br> 2197 * Type: <b>token</b><br> 2198 * Path: <b>EpisodeOfCare.status</b><br> 2199 * </p> 2200 */ 2201 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2202 2203 /** 2204 * Search parameter: <b>date</b> 2205 * <p> 2206 * Description: <b>Multiple Resources: 2207 2208* [AdverseEvent](adverseevent.html): When the event occurred 2209* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2210* [Appointment](appointment.html): Appointment date/time. 2211* [AuditEvent](auditevent.html): Time when the event was recorded 2212* [CarePlan](careplan.html): Time period plan covers 2213* [CareTeam](careteam.html): A date within the coverage time period. 2214* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2215* [Composition](composition.html): Composition editing time 2216* [Consent](consent.html): When consent was agreed to 2217* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2218* [DocumentReference](documentreference.html): When this document reference was created 2219* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2220* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2221* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2222* [Flag](flag.html): Time period when flag is active 2223* [Immunization](immunization.html): Vaccination (non)-Administration Date 2224* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2225* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2226* [Invoice](invoice.html): Invoice date / posting date 2227* [List](list.html): When the list was prepared 2228* [MeasureReport](measurereport.html): The date of the measure report 2229* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2230* [Observation](observation.html): Clinically relevant time/time-period for observation 2231* [Procedure](procedure.html): When the procedure occurred or is occurring 2232* [ResearchSubject](researchsubject.html): Start and end of participation 2233* [RiskAssessment](riskassessment.html): When was assessment made? 2234* [SupplyRequest](supplyrequest.html): When the request was made 2235</b><br> 2236 * Type: <b>date</b><br> 2237 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2238 * </p> 2239 */ 2240 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 2241 public static final String SP_DATE = "date"; 2242 /** 2243 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2244 * <p> 2245 * Description: <b>Multiple Resources: 2246 2247* [AdverseEvent](adverseevent.html): When the event occurred 2248* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2249* [Appointment](appointment.html): Appointment date/time. 2250* [AuditEvent](auditevent.html): Time when the event was recorded 2251* [CarePlan](careplan.html): Time period plan covers 2252* [CareTeam](careteam.html): A date within the coverage time period. 2253* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2254* [Composition](composition.html): Composition editing time 2255* [Consent](consent.html): When consent was agreed to 2256* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2257* [DocumentReference](documentreference.html): When this document reference was created 2258* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2259* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2260* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2261* [Flag](flag.html): Time period when flag is active 2262* [Immunization](immunization.html): Vaccination (non)-Administration Date 2263* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2264* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2265* [Invoice](invoice.html): Invoice date / posting date 2266* [List](list.html): When the list was prepared 2267* [MeasureReport](measurereport.html): The date of the measure report 2268* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2269* [Observation](observation.html): Clinically relevant time/time-period for observation 2270* [Procedure](procedure.html): When the procedure occurred or is occurring 2271* [ResearchSubject](researchsubject.html): Start and end of participation 2272* [RiskAssessment](riskassessment.html): When was assessment made? 2273* [SupplyRequest](supplyrequest.html): When the request was made 2274</b><br> 2275 * Type: <b>date</b><br> 2276 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2277 * </p> 2278 */ 2279 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2280 2281 /** 2282 * Search parameter: <b>identifier</b> 2283 * <p> 2284 * Description: <b>Multiple Resources: 2285 2286* [Account](account.html): Account number 2287* [AdverseEvent](adverseevent.html): Business identifier for the event 2288* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2289* [Appointment](appointment.html): An Identifier of the Appointment 2290* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2291* [Basic](basic.html): Business identifier 2292* [BodyStructure](bodystructure.html): Bodystructure identifier 2293* [CarePlan](careplan.html): External Ids for this plan 2294* [CareTeam](careteam.html): External Ids for this team 2295* [ChargeItem](chargeitem.html): Business Identifier for item 2296* [Claim](claim.html): The primary identifier of the financial resource 2297* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2298* [ClinicalImpression](clinicalimpression.html): Business identifier 2299* [Communication](communication.html): Unique identifier 2300* [CommunicationRequest](communicationrequest.html): Unique identifier 2301* [Composition](composition.html): Version-independent identifier for the Composition 2302* [Condition](condition.html): A unique identifier of the condition record 2303* [Consent](consent.html): Identifier for this record (external references) 2304* [Contract](contract.html): The identity of the contract 2305* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2306* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2307* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2308* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2309* [DeviceRequest](devicerequest.html): Business identifier for request/order 2310* [DeviceUsage](deviceusage.html): Search by identifier 2311* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2312* [DocumentReference](documentreference.html): Identifier of the attachment binary 2313* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2314* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2315* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2316* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2317* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2318* [Flag](flag.html): Business identifier 2319* [Goal](goal.html): External Ids for this goal 2320* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2321* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2322* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2323* [Immunization](immunization.html): Business identifier 2324* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2325* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2326* [Invoice](invoice.html): Business Identifier for item 2327* [List](list.html): Business identifier 2328* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2329* [Medication](medication.html): Returns medications with this external identifier 2330* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2331* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2332* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2333* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2334* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2335* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2336* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2337* [Observation](observation.html): The unique id for a particular observation 2338* [Person](person.html): A person Identifier 2339* [Procedure](procedure.html): A unique identifier for a procedure 2340* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2341* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2342* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2343* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2344* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2345* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2346* [Specimen](specimen.html): The unique identifier associated with the specimen 2347* [SupplyDelivery](supplydelivery.html): External identifier 2348* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2349* [Task](task.html): Search for a task instance by its business identifier 2350* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2351</b><br> 2352 * Type: <b>token</b><br> 2353 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2354 * </p> 2355 */ 2356 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2357 public static final String SP_IDENTIFIER = "identifier"; 2358 /** 2359 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2360 * <p> 2361 * Description: <b>Multiple Resources: 2362 2363* [Account](account.html): Account number 2364* [AdverseEvent](adverseevent.html): Business identifier for the event 2365* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2366* [Appointment](appointment.html): An Identifier of the Appointment 2367* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2368* [Basic](basic.html): Business identifier 2369* [BodyStructure](bodystructure.html): Bodystructure identifier 2370* [CarePlan](careplan.html): External Ids for this plan 2371* [CareTeam](careteam.html): External Ids for this team 2372* [ChargeItem](chargeitem.html): Business Identifier for item 2373* [Claim](claim.html): The primary identifier of the financial resource 2374* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2375* [ClinicalImpression](clinicalimpression.html): Business identifier 2376* [Communication](communication.html): Unique identifier 2377* [CommunicationRequest](communicationrequest.html): Unique identifier 2378* [Composition](composition.html): Version-independent identifier for the Composition 2379* [Condition](condition.html): A unique identifier of the condition record 2380* [Consent](consent.html): Identifier for this record (external references) 2381* [Contract](contract.html): The identity of the contract 2382* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2383* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2384* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2385* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2386* [DeviceRequest](devicerequest.html): Business identifier for request/order 2387* [DeviceUsage](deviceusage.html): Search by identifier 2388* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2389* [DocumentReference](documentreference.html): Identifier of the attachment binary 2390* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2391* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2392* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2393* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2394* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2395* [Flag](flag.html): Business identifier 2396* [Goal](goal.html): External Ids for this goal 2397* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2398* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2399* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2400* [Immunization](immunization.html): Business identifier 2401* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2402* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2403* [Invoice](invoice.html): Business Identifier for item 2404* [List](list.html): Business identifier 2405* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2406* [Medication](medication.html): Returns medications with this external identifier 2407* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2408* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2409* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2410* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2411* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2412* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2413* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2414* [Observation](observation.html): The unique id for a particular observation 2415* [Person](person.html): A person Identifier 2416* [Procedure](procedure.html): A unique identifier for a procedure 2417* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2418* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2419* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2420* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2421* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2422* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2423* [Specimen](specimen.html): The unique identifier associated with the specimen 2424* [SupplyDelivery](supplydelivery.html): External identifier 2425* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2426* [Task](task.html): Search for a task instance by its business identifier 2427* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2428</b><br> 2429 * Type: <b>token</b><br> 2430 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2431 * </p> 2432 */ 2433 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2434 2435 /** 2436 * Search parameter: <b>patient</b> 2437 * <p> 2438 * Description: <b>Multiple Resources: 2439 2440* [Account](account.html): The entity that caused the expenses 2441* [AdverseEvent](adverseevent.html): Subject impacted by event 2442* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2443* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2444* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2445* [AuditEvent](auditevent.html): Where the activity involved patient data 2446* [Basic](basic.html): Identifies the focus of this resource 2447* [BodyStructure](bodystructure.html): Who this is about 2448* [CarePlan](careplan.html): Who the care plan is for 2449* [CareTeam](careteam.html): Who care team is for 2450* [ChargeItem](chargeitem.html): Individual service was done for/to 2451* [Claim](claim.html): Patient receiving the products or services 2452* [ClaimResponse](claimresponse.html): The subject of care 2453* [ClinicalImpression](clinicalimpression.html): Patient assessed 2454* [Communication](communication.html): Focus of message 2455* [CommunicationRequest](communicationrequest.html): Focus of message 2456* [Composition](composition.html): Who and/or what the composition is about 2457* [Condition](condition.html): Who has the condition? 2458* [Consent](consent.html): Who the consent applies to 2459* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2460* [Coverage](coverage.html): Retrieve coverages for a patient 2461* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2462* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2463* [DetectedIssue](detectedissue.html): Associated patient 2464* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2465* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2466* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2467* [DocumentReference](documentreference.html): Who/what is the subject of the document 2468* [Encounter](encounter.html): The patient present at the encounter 2469* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2470* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2471* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2472* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2473* [Flag](flag.html): The identity of a subject to list flags for 2474* [Goal](goal.html): Who this goal is intended for 2475* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2476* [ImagingSelection](imagingselection.html): Who the study is about 2477* [ImagingStudy](imagingstudy.html): Who the study is about 2478* [Immunization](immunization.html): The patient for the vaccination record 2479* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2480* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2481* [Invoice](invoice.html): Recipient(s) of goods and services 2482* [List](list.html): If all resources have the same subject 2483* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2484* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2485* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2486* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2487* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2488* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2489* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2490* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2491* [Observation](observation.html): The subject that the observation is about (if patient) 2492* [Person](person.html): The Person links to this Patient 2493* [Procedure](procedure.html): Search by subject - a patient 2494* [Provenance](provenance.html): Where the activity involved patient data 2495* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2496* [RelatedPerson](relatedperson.html): The patient this related person is related to 2497* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2498* [ResearchSubject](researchsubject.html): Who or what is part of study 2499* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2500* [ServiceRequest](servicerequest.html): Search by subject - a patient 2501* [Specimen](specimen.html): The patient the specimen comes from 2502* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2503* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2504* [Task](task.html): Search by patient 2505* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2506</b><br> 2507 * Type: <b>reference</b><br> 2508 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2509 * </p> 2510 */ 2511 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2512 public static final String SP_PATIENT = "patient"; 2513 /** 2514 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2515 * <p> 2516 * Description: <b>Multiple Resources: 2517 2518* [Account](account.html): The entity that caused the expenses 2519* [AdverseEvent](adverseevent.html): Subject impacted by event 2520* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2521* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2522* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2523* [AuditEvent](auditevent.html): Where the activity involved patient data 2524* [Basic](basic.html): Identifies the focus of this resource 2525* [BodyStructure](bodystructure.html): Who this is about 2526* [CarePlan](careplan.html): Who the care plan is for 2527* [CareTeam](careteam.html): Who care team is for 2528* [ChargeItem](chargeitem.html): Individual service was done for/to 2529* [Claim](claim.html): Patient receiving the products or services 2530* [ClaimResponse](claimresponse.html): The subject of care 2531* [ClinicalImpression](clinicalimpression.html): Patient assessed 2532* [Communication](communication.html): Focus of message 2533* [CommunicationRequest](communicationrequest.html): Focus of message 2534* [Composition](composition.html): Who and/or what the composition is about 2535* [Condition](condition.html): Who has the condition? 2536* [Consent](consent.html): Who the consent applies to 2537* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2538* [Coverage](coverage.html): Retrieve coverages for a patient 2539* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2540* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2541* [DetectedIssue](detectedissue.html): Associated patient 2542* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2543* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2544* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2545* [DocumentReference](documentreference.html): Who/what is the subject of the document 2546* [Encounter](encounter.html): The patient present at the encounter 2547* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2548* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2549* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2550* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2551* [Flag](flag.html): The identity of a subject to list flags for 2552* [Goal](goal.html): Who this goal is intended for 2553* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2554* [ImagingSelection](imagingselection.html): Who the study is about 2555* [ImagingStudy](imagingstudy.html): Who the study is about 2556* [Immunization](immunization.html): The patient for the vaccination record 2557* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2558* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2559* [Invoice](invoice.html): Recipient(s) of goods and services 2560* [List](list.html): If all resources have the same subject 2561* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2562* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2563* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2564* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2565* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2566* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2567* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2568* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2569* [Observation](observation.html): The subject that the observation is about (if patient) 2570* [Person](person.html): The Person links to this Patient 2571* [Procedure](procedure.html): Search by subject - a patient 2572* [Provenance](provenance.html): Where the activity involved patient data 2573* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2574* [RelatedPerson](relatedperson.html): The patient this related person is related to 2575* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2576* [ResearchSubject](researchsubject.html): Who or what is part of study 2577* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2578* [ServiceRequest](servicerequest.html): Search by subject - a patient 2579* [Specimen](specimen.html): The patient the specimen comes from 2580* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2581* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2582* [Task](task.html): Search by patient 2583* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2584</b><br> 2585 * Type: <b>reference</b><br> 2586 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2587 * </p> 2588 */ 2589 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2590 2591/** 2592 * Constant for fluent queries to be used to add include statements. Specifies 2593 * the path value of "<b>EpisodeOfCare:patient</b>". 2594 */ 2595 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:patient").toLocked(); 2596 2597 /** 2598 * Search parameter: <b>type</b> 2599 * <p> 2600 * Description: <b>Multiple Resources: 2601 2602* [Account](account.html): E.g. patient, expense, depreciation 2603* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2604* [Composition](composition.html): Kind of composition (LOINC if possible) 2605* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2606* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2607* [Encounter](encounter.html): Specific type of encounter 2608* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2609* [Invoice](invoice.html): Type of Invoice 2610* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2611* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2612* [Specimen](specimen.html): The specimen type 2613</b><br> 2614 * Type: <b>token</b><br> 2615 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2616 * </p> 2617 */ 2618 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 2619 public static final String SP_TYPE = "type"; 2620 /** 2621 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2622 * <p> 2623 * Description: <b>Multiple Resources: 2624 2625* [Account](account.html): E.g. patient, expense, depreciation 2626* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2627* [Composition](composition.html): Kind of composition (LOINC if possible) 2628* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2629* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2630* [Encounter](encounter.html): Specific type of encounter 2631* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2632* [Invoice](invoice.html): Type of Invoice 2633* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2634* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2635* [Specimen](specimen.html): The specimen type 2636</b><br> 2637 * Type: <b>token</b><br> 2638 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2639 * </p> 2640 */ 2641 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2642 2643 2644} 2645