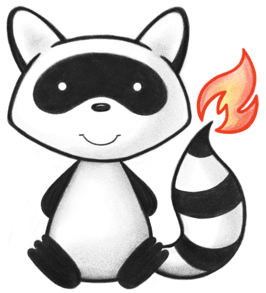
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The EventDefinition resource provides a reusable description of when a particular event can occur. 052 */ 053@ResourceDef(name="EventDefinition", profile="http://hl7.org/fhir/StructureDefinition/EventDefinition") 054public class EventDefinition extends MetadataResource { 055 056 /** 057 * An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers. 058 */ 059 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="Canonical identifier for this event definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers." ) 061 protected UriType url; 062 063 /** 064 * A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 065 */ 066 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 067 @Description(shortDefinition="Additional identifier for the event definition", formalDefinition="A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 068 protected List<Identifier> identifier; 069 070 /** 071 * The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 072 */ 073 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 074 @Description(shortDefinition="Business version of the event definition", formalDefinition="The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 075 protected StringType version; 076 077 /** 078 * Indicates the mechanism used to compare versions to determine which is more current. 079 */ 080 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 082 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 083 protected DataType versionAlgorithm; 084 085 /** 086 * A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 087 */ 088 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 089 @Description(shortDefinition="Name for this event definition (computer friendly)", formalDefinition="A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 090 protected StringType name; 091 092 /** 093 * A short, descriptive, user-friendly title for the event definition. 094 */ 095 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 096 @Description(shortDefinition="Name for this event definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the event definition." ) 097 protected StringType title; 098 099 /** 100 * An explanatory or alternate title for the event definition giving additional information about its content. 101 */ 102 @Child(name = "subtitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 103 @Description(shortDefinition="Subordinate title of the event definition", formalDefinition="An explanatory or alternate title for the event definition giving additional information about its content." ) 104 protected StringType subtitle; 105 106 /** 107 * The status of this event definition. Enables tracking the life-cycle of the content. 108 */ 109 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 110 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this event definition. Enables tracking the life-cycle of the content." ) 111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 112 protected Enumeration<PublicationStatus> status; 113 114 /** 115 * A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 116 */ 117 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 118 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 119 protected BooleanType experimental; 120 121 /** 122 * A code or group definition that describes the intended subject of the event definition. 123 */ 124 @Child(name = "subject", type = {CodeableConcept.class, Group.class}, order=9, min=0, max=1, modifier=false, summary=false) 125 @Description(shortDefinition="Type of individual the event definition is focused on", formalDefinition="A code or group definition that describes the intended subject of the event definition." ) 126 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 127 protected DataType subject; 128 129 /** 130 * The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes. 131 */ 132 @Child(name = "date", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 133 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes." ) 134 protected DateTimeType date; 135 136 /** 137 * The name of the organization or individual responsible for the release and ongoing maintenance of the event definition. 138 */ 139 @Child(name = "publisher", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 140 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the event definition." ) 141 protected StringType publisher; 142 143 /** 144 * Contact details to assist a user in finding and communicating with the publisher. 145 */ 146 @Child(name = "contact", type = {ContactDetail.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 147 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 148 protected List<ContactDetail> contact; 149 150 /** 151 * A free text natural language description of the event definition from a consumer's perspective. 152 */ 153 @Child(name = "description", type = {MarkdownType.class}, order=13, min=0, max=1, modifier=false, summary=false) 154 @Description(shortDefinition="Natural language description of the event definition", formalDefinition="A free text natural language description of the event definition from a consumer's perspective." ) 155 protected MarkdownType description; 156 157 /** 158 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate event definition instances. 159 */ 160 @Child(name = "useContext", type = {UsageContext.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 161 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate event definition instances." ) 162 protected List<UsageContext> useContext; 163 164 /** 165 * A legal or geographic region in which the event definition is intended to be used. 166 */ 167 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 168 @Description(shortDefinition="Intended jurisdiction for event definition (if applicable)", formalDefinition="A legal or geographic region in which the event definition is intended to be used." ) 169 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 170 protected List<CodeableConcept> jurisdiction; 171 172 /** 173 * Explanation of why this event definition is needed and why it has been designed as it has. 174 */ 175 @Child(name = "purpose", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="Why this event definition is defined", formalDefinition="Explanation of why this event definition is needed and why it has been designed as it has." ) 177 protected MarkdownType purpose; 178 179 /** 180 * A detailed description of how the event definition is used from a clinical perspective. 181 */ 182 @Child(name = "usage", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 183 @Description(shortDefinition="Describes the clinical usage of the event definition", formalDefinition="A detailed description of how the event definition is used from a clinical perspective." ) 184 protected MarkdownType usage; 185 186 /** 187 * A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition. 188 */ 189 @Child(name = "copyright", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition." ) 191 protected MarkdownType copyright; 192 193 /** 194 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 195 */ 196 @Child(name = "copyrightLabel", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 197 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 198 protected StringType copyrightLabel; 199 200 /** 201 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 202 */ 203 @Child(name = "approvalDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=true) 204 @Description(shortDefinition="When the event definition was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 205 protected DateType approvalDate; 206 207 /** 208 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 209 */ 210 @Child(name = "lastReviewDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=true) 211 @Description(shortDefinition="When the event definition was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 212 protected DateType lastReviewDate; 213 214 /** 215 * The period during which the event definition content was or is planned to be in active use. 216 */ 217 @Child(name = "effectivePeriod", type = {Period.class}, order=22, min=0, max=1, modifier=false, summary=true) 218 @Description(shortDefinition="When the event definition is expected to be used", formalDefinition="The period during which the event definition content was or is planned to be in active use." ) 219 protected Period effectivePeriod; 220 221 /** 222 * Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching. 223 */ 224 @Child(name = "topic", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 225 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching." ) 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 227 protected List<CodeableConcept> topic; 228 229 /** 230 * An individiual or organization primarily involved in the creation and maintenance of the content. 231 */ 232 @Child(name = "author", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 233 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the content." ) 234 protected List<ContactDetail> author; 235 236 /** 237 * An individual or organization primarily responsible for internal coherence of the content. 238 */ 239 @Child(name = "editor", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 240 @Description(shortDefinition="Who edited the content", formalDefinition="An individual or organization primarily responsible for internal coherence of the content." ) 241 protected List<ContactDetail> editor; 242 243 /** 244 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content. 245 */ 246 @Child(name = "reviewer", type = {ContactDetail.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 247 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content." ) 248 protected List<ContactDetail> reviewer; 249 250 /** 251 * An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting. 252 */ 253 @Child(name = "endorser", type = {ContactDetail.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 254 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting." ) 255 protected List<ContactDetail> endorser; 256 257 /** 258 * Related resources such as additional documentation, justification, or bibliographic references. 259 */ 260 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 261 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related resources such as additional documentation, justification, or bibliographic references." ) 262 protected List<RelatedArtifact> relatedArtifact; 263 264 /** 265 * The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met. 266 */ 267 @Child(name = "trigger", type = {TriggerDefinition.class}, order=29, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 268 @Description(shortDefinition="\"when\" the event occurs (multiple = 'or')", formalDefinition="The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met." ) 269 protected List<TriggerDefinition> trigger; 270 271 private static final long serialVersionUID = -1047563679L; 272 273 /** 274 * Constructor 275 */ 276 public EventDefinition() { 277 super(); 278 } 279 280 /** 281 * Constructor 282 */ 283 public EventDefinition(PublicationStatus status, TriggerDefinition trigger) { 284 super(); 285 this.setStatus(status); 286 this.addTrigger(trigger); 287 } 288 289 /** 290 * @return {@link #url} (An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 291 */ 292 public UriType getUrlElement() { 293 if (this.url == null) 294 if (Configuration.errorOnAutoCreate()) 295 throw new Error("Attempt to auto-create EventDefinition.url"); 296 else if (Configuration.doAutoCreate()) 297 this.url = new UriType(); // bb 298 return this.url; 299 } 300 301 public boolean hasUrlElement() { 302 return this.url != null && !this.url.isEmpty(); 303 } 304 305 public boolean hasUrl() { 306 return this.url != null && !this.url.isEmpty(); 307 } 308 309 /** 310 * @param value {@link #url} (An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 311 */ 312 public EventDefinition setUrlElement(UriType value) { 313 this.url = value; 314 return this; 315 } 316 317 /** 318 * @return An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers. 319 */ 320 public String getUrl() { 321 return this.url == null ? null : this.url.getValue(); 322 } 323 324 /** 325 * @param value An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers. 326 */ 327 public EventDefinition setUrl(String value) { 328 if (Utilities.noString(value)) 329 this.url = null; 330 else { 331 if (this.url == null) 332 this.url = new UriType(); 333 this.url.setValue(value); 334 } 335 return this; 336 } 337 338 /** 339 * @return {@link #identifier} (A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 340 */ 341 public List<Identifier> getIdentifier() { 342 if (this.identifier == null) 343 this.identifier = new ArrayList<Identifier>(); 344 return this.identifier; 345 } 346 347 /** 348 * @return Returns a reference to <code>this</code> for easy method chaining 349 */ 350 public EventDefinition setIdentifier(List<Identifier> theIdentifier) { 351 this.identifier = theIdentifier; 352 return this; 353 } 354 355 public boolean hasIdentifier() { 356 if (this.identifier == null) 357 return false; 358 for (Identifier item : this.identifier) 359 if (!item.isEmpty()) 360 return true; 361 return false; 362 } 363 364 public Identifier addIdentifier() { //3 365 Identifier t = new Identifier(); 366 if (this.identifier == null) 367 this.identifier = new ArrayList<Identifier>(); 368 this.identifier.add(t); 369 return t; 370 } 371 372 public EventDefinition addIdentifier(Identifier t) { //3 373 if (t == null) 374 return this; 375 if (this.identifier == null) 376 this.identifier = new ArrayList<Identifier>(); 377 this.identifier.add(t); 378 return this; 379 } 380 381 /** 382 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 383 */ 384 public Identifier getIdentifierFirstRep() { 385 if (getIdentifier().isEmpty()) { 386 addIdentifier(); 387 } 388 return getIdentifier().get(0); 389 } 390 391 /** 392 * @return {@link #version} (The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 393 */ 394 public StringType getVersionElement() { 395 if (this.version == null) 396 if (Configuration.errorOnAutoCreate()) 397 throw new Error("Attempt to auto-create EventDefinition.version"); 398 else if (Configuration.doAutoCreate()) 399 this.version = new StringType(); // bb 400 return this.version; 401 } 402 403 public boolean hasVersionElement() { 404 return this.version != null && !this.version.isEmpty(); 405 } 406 407 public boolean hasVersion() { 408 return this.version != null && !this.version.isEmpty(); 409 } 410 411 /** 412 * @param value {@link #version} (The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 413 */ 414 public EventDefinition setVersionElement(StringType value) { 415 this.version = value; 416 return this; 417 } 418 419 /** 420 * @return The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 421 */ 422 public String getVersion() { 423 return this.version == null ? null : this.version.getValue(); 424 } 425 426 /** 427 * @param value The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 428 */ 429 public EventDefinition setVersion(String value) { 430 if (Utilities.noString(value)) 431 this.version = null; 432 else { 433 if (this.version == null) 434 this.version = new StringType(); 435 this.version.setValue(value); 436 } 437 return this; 438 } 439 440 /** 441 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 442 */ 443 public DataType getVersionAlgorithm() { 444 return this.versionAlgorithm; 445 } 446 447 /** 448 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 449 */ 450 public StringType getVersionAlgorithmStringType() throws FHIRException { 451 if (this.versionAlgorithm == null) 452 this.versionAlgorithm = new StringType(); 453 if (!(this.versionAlgorithm instanceof StringType)) 454 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 455 return (StringType) this.versionAlgorithm; 456 } 457 458 public boolean hasVersionAlgorithmStringType() { 459 return this != null && this.versionAlgorithm instanceof StringType; 460 } 461 462 /** 463 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 464 */ 465 public Coding getVersionAlgorithmCoding() throws FHIRException { 466 if (this.versionAlgorithm == null) 467 this.versionAlgorithm = new Coding(); 468 if (!(this.versionAlgorithm instanceof Coding)) 469 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 470 return (Coding) this.versionAlgorithm; 471 } 472 473 public boolean hasVersionAlgorithmCoding() { 474 return this != null && this.versionAlgorithm instanceof Coding; 475 } 476 477 public boolean hasVersionAlgorithm() { 478 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 479 } 480 481 /** 482 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 483 */ 484 public EventDefinition setVersionAlgorithm(DataType value) { 485 if (value != null && !(value instanceof StringType || value instanceof Coding)) 486 throw new FHIRException("Not the right type for EventDefinition.versionAlgorithm[x]: "+value.fhirType()); 487 this.versionAlgorithm = value; 488 return this; 489 } 490 491 /** 492 * @return {@link #name} (A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 493 */ 494 public StringType getNameElement() { 495 if (this.name == null) 496 if (Configuration.errorOnAutoCreate()) 497 throw new Error("Attempt to auto-create EventDefinition.name"); 498 else if (Configuration.doAutoCreate()) 499 this.name = new StringType(); // bb 500 return this.name; 501 } 502 503 public boolean hasNameElement() { 504 return this.name != null && !this.name.isEmpty(); 505 } 506 507 public boolean hasName() { 508 return this.name != null && !this.name.isEmpty(); 509 } 510 511 /** 512 * @param value {@link #name} (A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 513 */ 514 public EventDefinition setNameElement(StringType value) { 515 this.name = value; 516 return this; 517 } 518 519 /** 520 * @return A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 521 */ 522 public String getName() { 523 return this.name == null ? null : this.name.getValue(); 524 } 525 526 /** 527 * @param value A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 528 */ 529 public EventDefinition setName(String value) { 530 if (Utilities.noString(value)) 531 this.name = null; 532 else { 533 if (this.name == null) 534 this.name = new StringType(); 535 this.name.setValue(value); 536 } 537 return this; 538 } 539 540 /** 541 * @return {@link #title} (A short, descriptive, user-friendly title for the event definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 542 */ 543 public StringType getTitleElement() { 544 if (this.title == null) 545 if (Configuration.errorOnAutoCreate()) 546 throw new Error("Attempt to auto-create EventDefinition.title"); 547 else if (Configuration.doAutoCreate()) 548 this.title = new StringType(); // bb 549 return this.title; 550 } 551 552 public boolean hasTitleElement() { 553 return this.title != null && !this.title.isEmpty(); 554 } 555 556 public boolean hasTitle() { 557 return this.title != null && !this.title.isEmpty(); 558 } 559 560 /** 561 * @param value {@link #title} (A short, descriptive, user-friendly title for the event definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 562 */ 563 public EventDefinition setTitleElement(StringType value) { 564 this.title = value; 565 return this; 566 } 567 568 /** 569 * @return A short, descriptive, user-friendly title for the event definition. 570 */ 571 public String getTitle() { 572 return this.title == null ? null : this.title.getValue(); 573 } 574 575 /** 576 * @param value A short, descriptive, user-friendly title for the event definition. 577 */ 578 public EventDefinition setTitle(String value) { 579 if (Utilities.noString(value)) 580 this.title = null; 581 else { 582 if (this.title == null) 583 this.title = new StringType(); 584 this.title.setValue(value); 585 } 586 return this; 587 } 588 589 /** 590 * @return {@link #subtitle} (An explanatory or alternate title for the event definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 591 */ 592 public StringType getSubtitleElement() { 593 if (this.subtitle == null) 594 if (Configuration.errorOnAutoCreate()) 595 throw new Error("Attempt to auto-create EventDefinition.subtitle"); 596 else if (Configuration.doAutoCreate()) 597 this.subtitle = new StringType(); // bb 598 return this.subtitle; 599 } 600 601 public boolean hasSubtitleElement() { 602 return this.subtitle != null && !this.subtitle.isEmpty(); 603 } 604 605 public boolean hasSubtitle() { 606 return this.subtitle != null && !this.subtitle.isEmpty(); 607 } 608 609 /** 610 * @param value {@link #subtitle} (An explanatory or alternate title for the event definition giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 611 */ 612 public EventDefinition setSubtitleElement(StringType value) { 613 this.subtitle = value; 614 return this; 615 } 616 617 /** 618 * @return An explanatory or alternate title for the event definition giving additional information about its content. 619 */ 620 public String getSubtitle() { 621 return this.subtitle == null ? null : this.subtitle.getValue(); 622 } 623 624 /** 625 * @param value An explanatory or alternate title for the event definition giving additional information about its content. 626 */ 627 public EventDefinition setSubtitle(String value) { 628 if (Utilities.noString(value)) 629 this.subtitle = null; 630 else { 631 if (this.subtitle == null) 632 this.subtitle = new StringType(); 633 this.subtitle.setValue(value); 634 } 635 return this; 636 } 637 638 /** 639 * @return {@link #status} (The status of this event definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 640 */ 641 public Enumeration<PublicationStatus> getStatusElement() { 642 if (this.status == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create EventDefinition.status"); 645 else if (Configuration.doAutoCreate()) 646 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 647 return this.status; 648 } 649 650 public boolean hasStatusElement() { 651 return this.status != null && !this.status.isEmpty(); 652 } 653 654 public boolean hasStatus() { 655 return this.status != null && !this.status.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #status} (The status of this event definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 660 */ 661 public EventDefinition setStatusElement(Enumeration<PublicationStatus> value) { 662 this.status = value; 663 return this; 664 } 665 666 /** 667 * @return The status of this event definition. Enables tracking the life-cycle of the content. 668 */ 669 public PublicationStatus getStatus() { 670 return this.status == null ? null : this.status.getValue(); 671 } 672 673 /** 674 * @param value The status of this event definition. Enables tracking the life-cycle of the content. 675 */ 676 public EventDefinition setStatus(PublicationStatus value) { 677 if (this.status == null) 678 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 679 this.status.setValue(value); 680 return this; 681 } 682 683 /** 684 * @return {@link #experimental} (A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 685 */ 686 public BooleanType getExperimentalElement() { 687 if (this.experimental == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create EventDefinition.experimental"); 690 else if (Configuration.doAutoCreate()) 691 this.experimental = new BooleanType(); // bb 692 return this.experimental; 693 } 694 695 public boolean hasExperimentalElement() { 696 return this.experimental != null && !this.experimental.isEmpty(); 697 } 698 699 public boolean hasExperimental() { 700 return this.experimental != null && !this.experimental.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #experimental} (A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 705 */ 706 public EventDefinition setExperimentalElement(BooleanType value) { 707 this.experimental = value; 708 return this; 709 } 710 711 /** 712 * @return A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 713 */ 714 public boolean getExperimental() { 715 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 716 } 717 718 /** 719 * @param value A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 720 */ 721 public EventDefinition setExperimental(boolean value) { 722 if (this.experimental == null) 723 this.experimental = new BooleanType(); 724 this.experimental.setValue(value); 725 return this; 726 } 727 728 /** 729 * @return {@link #subject} (A code or group definition that describes the intended subject of the event definition.) 730 */ 731 public DataType getSubject() { 732 return this.subject; 733 } 734 735 /** 736 * @return {@link #subject} (A code or group definition that describes the intended subject of the event definition.) 737 */ 738 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 739 if (this.subject == null) 740 this.subject = new CodeableConcept(); 741 if (!(this.subject instanceof CodeableConcept)) 742 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 743 return (CodeableConcept) this.subject; 744 } 745 746 public boolean hasSubjectCodeableConcept() { 747 return this != null && this.subject instanceof CodeableConcept; 748 } 749 750 /** 751 * @return {@link #subject} (A code or group definition that describes the intended subject of the event definition.) 752 */ 753 public Reference getSubjectReference() throws FHIRException { 754 if (this.subject == null) 755 this.subject = new Reference(); 756 if (!(this.subject instanceof Reference)) 757 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 758 return (Reference) this.subject; 759 } 760 761 public boolean hasSubjectReference() { 762 return this != null && this.subject instanceof Reference; 763 } 764 765 public boolean hasSubject() { 766 return this.subject != null && !this.subject.isEmpty(); 767 } 768 769 /** 770 * @param value {@link #subject} (A code or group definition that describes the intended subject of the event definition.) 771 */ 772 public EventDefinition setSubject(DataType value) { 773 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 774 throw new FHIRException("Not the right type for EventDefinition.subject[x]: "+value.fhirType()); 775 this.subject = value; 776 return this; 777 } 778 779 /** 780 * @return {@link #date} (The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 781 */ 782 public DateTimeType getDateElement() { 783 if (this.date == null) 784 if (Configuration.errorOnAutoCreate()) 785 throw new Error("Attempt to auto-create EventDefinition.date"); 786 else if (Configuration.doAutoCreate()) 787 this.date = new DateTimeType(); // bb 788 return this.date; 789 } 790 791 public boolean hasDateElement() { 792 return this.date != null && !this.date.isEmpty(); 793 } 794 795 public boolean hasDate() { 796 return this.date != null && !this.date.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #date} (The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 801 */ 802 public EventDefinition setDateElement(DateTimeType value) { 803 this.date = value; 804 return this; 805 } 806 807 /** 808 * @return The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes. 809 */ 810 public Date getDate() { 811 return this.date == null ? null : this.date.getValue(); 812 } 813 814 /** 815 * @param value The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes. 816 */ 817 public EventDefinition setDate(Date value) { 818 if (value == null) 819 this.date = null; 820 else { 821 if (this.date == null) 822 this.date = new DateTimeType(); 823 this.date.setValue(value); 824 } 825 return this; 826 } 827 828 /** 829 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the event definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 830 */ 831 public StringType getPublisherElement() { 832 if (this.publisher == null) 833 if (Configuration.errorOnAutoCreate()) 834 throw new Error("Attempt to auto-create EventDefinition.publisher"); 835 else if (Configuration.doAutoCreate()) 836 this.publisher = new StringType(); // bb 837 return this.publisher; 838 } 839 840 public boolean hasPublisherElement() { 841 return this.publisher != null && !this.publisher.isEmpty(); 842 } 843 844 public boolean hasPublisher() { 845 return this.publisher != null && !this.publisher.isEmpty(); 846 } 847 848 /** 849 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the event definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 850 */ 851 public EventDefinition setPublisherElement(StringType value) { 852 this.publisher = value; 853 return this; 854 } 855 856 /** 857 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the event definition. 858 */ 859 public String getPublisher() { 860 return this.publisher == null ? null : this.publisher.getValue(); 861 } 862 863 /** 864 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the event definition. 865 */ 866 public EventDefinition setPublisher(String value) { 867 if (Utilities.noString(value)) 868 this.publisher = null; 869 else { 870 if (this.publisher == null) 871 this.publisher = new StringType(); 872 this.publisher.setValue(value); 873 } 874 return this; 875 } 876 877 /** 878 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 879 */ 880 public List<ContactDetail> getContact() { 881 if (this.contact == null) 882 this.contact = new ArrayList<ContactDetail>(); 883 return this.contact; 884 } 885 886 /** 887 * @return Returns a reference to <code>this</code> for easy method chaining 888 */ 889 public EventDefinition setContact(List<ContactDetail> theContact) { 890 this.contact = theContact; 891 return this; 892 } 893 894 public boolean hasContact() { 895 if (this.contact == null) 896 return false; 897 for (ContactDetail item : this.contact) 898 if (!item.isEmpty()) 899 return true; 900 return false; 901 } 902 903 public ContactDetail addContact() { //3 904 ContactDetail t = new ContactDetail(); 905 if (this.contact == null) 906 this.contact = new ArrayList<ContactDetail>(); 907 this.contact.add(t); 908 return t; 909 } 910 911 public EventDefinition addContact(ContactDetail t) { //3 912 if (t == null) 913 return this; 914 if (this.contact == null) 915 this.contact = new ArrayList<ContactDetail>(); 916 this.contact.add(t); 917 return this; 918 } 919 920 /** 921 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 922 */ 923 public ContactDetail getContactFirstRep() { 924 if (getContact().isEmpty()) { 925 addContact(); 926 } 927 return getContact().get(0); 928 } 929 930 /** 931 * @return {@link #description} (A free text natural language description of the event definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 932 */ 933 public MarkdownType getDescriptionElement() { 934 if (this.description == null) 935 if (Configuration.errorOnAutoCreate()) 936 throw new Error("Attempt to auto-create EventDefinition.description"); 937 else if (Configuration.doAutoCreate()) 938 this.description = new MarkdownType(); // bb 939 return this.description; 940 } 941 942 public boolean hasDescriptionElement() { 943 return this.description != null && !this.description.isEmpty(); 944 } 945 946 public boolean hasDescription() { 947 return this.description != null && !this.description.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #description} (A free text natural language description of the event definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 952 */ 953 public EventDefinition setDescriptionElement(MarkdownType value) { 954 this.description = value; 955 return this; 956 } 957 958 /** 959 * @return A free text natural language description of the event definition from a consumer's perspective. 960 */ 961 public String getDescription() { 962 return this.description == null ? null : this.description.getValue(); 963 } 964 965 /** 966 * @param value A free text natural language description of the event definition from a consumer's perspective. 967 */ 968 public EventDefinition setDescription(String value) { 969 if (Utilities.noString(value)) 970 this.description = null; 971 else { 972 if (this.description == null) 973 this.description = new MarkdownType(); 974 this.description.setValue(value); 975 } 976 return this; 977 } 978 979 /** 980 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate event definition instances.) 981 */ 982 public List<UsageContext> getUseContext() { 983 if (this.useContext == null) 984 this.useContext = new ArrayList<UsageContext>(); 985 return this.useContext; 986 } 987 988 /** 989 * @return Returns a reference to <code>this</code> for easy method chaining 990 */ 991 public EventDefinition setUseContext(List<UsageContext> theUseContext) { 992 this.useContext = theUseContext; 993 return this; 994 } 995 996 public boolean hasUseContext() { 997 if (this.useContext == null) 998 return false; 999 for (UsageContext item : this.useContext) 1000 if (!item.isEmpty()) 1001 return true; 1002 return false; 1003 } 1004 1005 public UsageContext addUseContext() { //3 1006 UsageContext t = new UsageContext(); 1007 if (this.useContext == null) 1008 this.useContext = new ArrayList<UsageContext>(); 1009 this.useContext.add(t); 1010 return t; 1011 } 1012 1013 public EventDefinition addUseContext(UsageContext t) { //3 1014 if (t == null) 1015 return this; 1016 if (this.useContext == null) 1017 this.useContext = new ArrayList<UsageContext>(); 1018 this.useContext.add(t); 1019 return this; 1020 } 1021 1022 /** 1023 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1024 */ 1025 public UsageContext getUseContextFirstRep() { 1026 if (getUseContext().isEmpty()) { 1027 addUseContext(); 1028 } 1029 return getUseContext().get(0); 1030 } 1031 1032 /** 1033 * @return {@link #jurisdiction} (A legal or geographic region in which the event definition is intended to be used.) 1034 */ 1035 public List<CodeableConcept> getJurisdiction() { 1036 if (this.jurisdiction == null) 1037 this.jurisdiction = new ArrayList<CodeableConcept>(); 1038 return this.jurisdiction; 1039 } 1040 1041 /** 1042 * @return Returns a reference to <code>this</code> for easy method chaining 1043 */ 1044 public EventDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1045 this.jurisdiction = theJurisdiction; 1046 return this; 1047 } 1048 1049 public boolean hasJurisdiction() { 1050 if (this.jurisdiction == null) 1051 return false; 1052 for (CodeableConcept item : this.jurisdiction) 1053 if (!item.isEmpty()) 1054 return true; 1055 return false; 1056 } 1057 1058 public CodeableConcept addJurisdiction() { //3 1059 CodeableConcept t = new CodeableConcept(); 1060 if (this.jurisdiction == null) 1061 this.jurisdiction = new ArrayList<CodeableConcept>(); 1062 this.jurisdiction.add(t); 1063 return t; 1064 } 1065 1066 public EventDefinition addJurisdiction(CodeableConcept t) { //3 1067 if (t == null) 1068 return this; 1069 if (this.jurisdiction == null) 1070 this.jurisdiction = new ArrayList<CodeableConcept>(); 1071 this.jurisdiction.add(t); 1072 return this; 1073 } 1074 1075 /** 1076 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1077 */ 1078 public CodeableConcept getJurisdictionFirstRep() { 1079 if (getJurisdiction().isEmpty()) { 1080 addJurisdiction(); 1081 } 1082 return getJurisdiction().get(0); 1083 } 1084 1085 /** 1086 * @return {@link #purpose} (Explanation of why this event definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1087 */ 1088 public MarkdownType getPurposeElement() { 1089 if (this.purpose == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create EventDefinition.purpose"); 1092 else if (Configuration.doAutoCreate()) 1093 this.purpose = new MarkdownType(); // bb 1094 return this.purpose; 1095 } 1096 1097 public boolean hasPurposeElement() { 1098 return this.purpose != null && !this.purpose.isEmpty(); 1099 } 1100 1101 public boolean hasPurpose() { 1102 return this.purpose != null && !this.purpose.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #purpose} (Explanation of why this event definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1107 */ 1108 public EventDefinition setPurposeElement(MarkdownType value) { 1109 this.purpose = value; 1110 return this; 1111 } 1112 1113 /** 1114 * @return Explanation of why this event definition is needed and why it has been designed as it has. 1115 */ 1116 public String getPurpose() { 1117 return this.purpose == null ? null : this.purpose.getValue(); 1118 } 1119 1120 /** 1121 * @param value Explanation of why this event definition is needed and why it has been designed as it has. 1122 */ 1123 public EventDefinition setPurpose(String value) { 1124 if (Utilities.noString(value)) 1125 this.purpose = null; 1126 else { 1127 if (this.purpose == null) 1128 this.purpose = new MarkdownType(); 1129 this.purpose.setValue(value); 1130 } 1131 return this; 1132 } 1133 1134 /** 1135 * @return {@link #usage} (A detailed description of how the event definition is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 1136 */ 1137 public MarkdownType getUsageElement() { 1138 if (this.usage == null) 1139 if (Configuration.errorOnAutoCreate()) 1140 throw new Error("Attempt to auto-create EventDefinition.usage"); 1141 else if (Configuration.doAutoCreate()) 1142 this.usage = new MarkdownType(); // bb 1143 return this.usage; 1144 } 1145 1146 public boolean hasUsageElement() { 1147 return this.usage != null && !this.usage.isEmpty(); 1148 } 1149 1150 public boolean hasUsage() { 1151 return this.usage != null && !this.usage.isEmpty(); 1152 } 1153 1154 /** 1155 * @param value {@link #usage} (A detailed description of how the event definition is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 1156 */ 1157 public EventDefinition setUsageElement(MarkdownType value) { 1158 this.usage = value; 1159 return this; 1160 } 1161 1162 /** 1163 * @return A detailed description of how the event definition is used from a clinical perspective. 1164 */ 1165 public String getUsage() { 1166 return this.usage == null ? null : this.usage.getValue(); 1167 } 1168 1169 /** 1170 * @param value A detailed description of how the event definition is used from a clinical perspective. 1171 */ 1172 public EventDefinition setUsage(String value) { 1173 if (Utilities.noString(value)) 1174 this.usage = null; 1175 else { 1176 if (this.usage == null) 1177 this.usage = new MarkdownType(); 1178 this.usage.setValue(value); 1179 } 1180 return this; 1181 } 1182 1183 /** 1184 * @return {@link #copyright} (A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1185 */ 1186 public MarkdownType getCopyrightElement() { 1187 if (this.copyright == null) 1188 if (Configuration.errorOnAutoCreate()) 1189 throw new Error("Attempt to auto-create EventDefinition.copyright"); 1190 else if (Configuration.doAutoCreate()) 1191 this.copyright = new MarkdownType(); // bb 1192 return this.copyright; 1193 } 1194 1195 public boolean hasCopyrightElement() { 1196 return this.copyright != null && !this.copyright.isEmpty(); 1197 } 1198 1199 public boolean hasCopyright() { 1200 return this.copyright != null && !this.copyright.isEmpty(); 1201 } 1202 1203 /** 1204 * @param value {@link #copyright} (A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1205 */ 1206 public EventDefinition setCopyrightElement(MarkdownType value) { 1207 this.copyright = value; 1208 return this; 1209 } 1210 1211 /** 1212 * @return A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition. 1213 */ 1214 public String getCopyright() { 1215 return this.copyright == null ? null : this.copyright.getValue(); 1216 } 1217 1218 /** 1219 * @param value A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition. 1220 */ 1221 public EventDefinition setCopyright(String value) { 1222 if (Utilities.noString(value)) 1223 this.copyright = null; 1224 else { 1225 if (this.copyright == null) 1226 this.copyright = new MarkdownType(); 1227 this.copyright.setValue(value); 1228 } 1229 return this; 1230 } 1231 1232 /** 1233 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1234 */ 1235 public StringType getCopyrightLabelElement() { 1236 if (this.copyrightLabel == null) 1237 if (Configuration.errorOnAutoCreate()) 1238 throw new Error("Attempt to auto-create EventDefinition.copyrightLabel"); 1239 else if (Configuration.doAutoCreate()) 1240 this.copyrightLabel = new StringType(); // bb 1241 return this.copyrightLabel; 1242 } 1243 1244 public boolean hasCopyrightLabelElement() { 1245 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1246 } 1247 1248 public boolean hasCopyrightLabel() { 1249 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1250 } 1251 1252 /** 1253 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1254 */ 1255 public EventDefinition setCopyrightLabelElement(StringType value) { 1256 this.copyrightLabel = value; 1257 return this; 1258 } 1259 1260 /** 1261 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1262 */ 1263 public String getCopyrightLabel() { 1264 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 1265 } 1266 1267 /** 1268 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1269 */ 1270 public EventDefinition setCopyrightLabel(String value) { 1271 if (Utilities.noString(value)) 1272 this.copyrightLabel = null; 1273 else { 1274 if (this.copyrightLabel == null) 1275 this.copyrightLabel = new StringType(); 1276 this.copyrightLabel.setValue(value); 1277 } 1278 return this; 1279 } 1280 1281 /** 1282 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1283 */ 1284 public DateType getApprovalDateElement() { 1285 if (this.approvalDate == null) 1286 if (Configuration.errorOnAutoCreate()) 1287 throw new Error("Attempt to auto-create EventDefinition.approvalDate"); 1288 else if (Configuration.doAutoCreate()) 1289 this.approvalDate = new DateType(); // bb 1290 return this.approvalDate; 1291 } 1292 1293 public boolean hasApprovalDateElement() { 1294 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1295 } 1296 1297 public boolean hasApprovalDate() { 1298 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1299 } 1300 1301 /** 1302 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1303 */ 1304 public EventDefinition setApprovalDateElement(DateType value) { 1305 this.approvalDate = value; 1306 return this; 1307 } 1308 1309 /** 1310 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1311 */ 1312 public Date getApprovalDate() { 1313 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1314 } 1315 1316 /** 1317 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1318 */ 1319 public EventDefinition setApprovalDate(Date value) { 1320 if (value == null) 1321 this.approvalDate = null; 1322 else { 1323 if (this.approvalDate == null) 1324 this.approvalDate = new DateType(); 1325 this.approvalDate.setValue(value); 1326 } 1327 return this; 1328 } 1329 1330 /** 1331 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1332 */ 1333 public DateType getLastReviewDateElement() { 1334 if (this.lastReviewDate == null) 1335 if (Configuration.errorOnAutoCreate()) 1336 throw new Error("Attempt to auto-create EventDefinition.lastReviewDate"); 1337 else if (Configuration.doAutoCreate()) 1338 this.lastReviewDate = new DateType(); // bb 1339 return this.lastReviewDate; 1340 } 1341 1342 public boolean hasLastReviewDateElement() { 1343 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1344 } 1345 1346 public boolean hasLastReviewDate() { 1347 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1348 } 1349 1350 /** 1351 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1352 */ 1353 public EventDefinition setLastReviewDateElement(DateType value) { 1354 this.lastReviewDate = value; 1355 return this; 1356 } 1357 1358 /** 1359 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1360 */ 1361 public Date getLastReviewDate() { 1362 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1363 } 1364 1365 /** 1366 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1367 */ 1368 public EventDefinition setLastReviewDate(Date value) { 1369 if (value == null) 1370 this.lastReviewDate = null; 1371 else { 1372 if (this.lastReviewDate == null) 1373 this.lastReviewDate = new DateType(); 1374 this.lastReviewDate.setValue(value); 1375 } 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #effectivePeriod} (The period during which the event definition content was or is planned to be in active use.) 1381 */ 1382 public Period getEffectivePeriod() { 1383 if (this.effectivePeriod == null) 1384 if (Configuration.errorOnAutoCreate()) 1385 throw new Error("Attempt to auto-create EventDefinition.effectivePeriod"); 1386 else if (Configuration.doAutoCreate()) 1387 this.effectivePeriod = new Period(); // cc 1388 return this.effectivePeriod; 1389 } 1390 1391 public boolean hasEffectivePeriod() { 1392 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 1393 } 1394 1395 /** 1396 * @param value {@link #effectivePeriod} (The period during which the event definition content was or is planned to be in active use.) 1397 */ 1398 public EventDefinition setEffectivePeriod(Period value) { 1399 this.effectivePeriod = value; 1400 return this; 1401 } 1402 1403 /** 1404 * @return {@link #topic} (Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.) 1405 */ 1406 public List<CodeableConcept> getTopic() { 1407 if (this.topic == null) 1408 this.topic = new ArrayList<CodeableConcept>(); 1409 return this.topic; 1410 } 1411 1412 /** 1413 * @return Returns a reference to <code>this</code> for easy method chaining 1414 */ 1415 public EventDefinition setTopic(List<CodeableConcept> theTopic) { 1416 this.topic = theTopic; 1417 return this; 1418 } 1419 1420 public boolean hasTopic() { 1421 if (this.topic == null) 1422 return false; 1423 for (CodeableConcept item : this.topic) 1424 if (!item.isEmpty()) 1425 return true; 1426 return false; 1427 } 1428 1429 public CodeableConcept addTopic() { //3 1430 CodeableConcept t = new CodeableConcept(); 1431 if (this.topic == null) 1432 this.topic = new ArrayList<CodeableConcept>(); 1433 this.topic.add(t); 1434 return t; 1435 } 1436 1437 public EventDefinition addTopic(CodeableConcept t) { //3 1438 if (t == null) 1439 return this; 1440 if (this.topic == null) 1441 this.topic = new ArrayList<CodeableConcept>(); 1442 this.topic.add(t); 1443 return this; 1444 } 1445 1446 /** 1447 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 1448 */ 1449 public CodeableConcept getTopicFirstRep() { 1450 if (getTopic().isEmpty()) { 1451 addTopic(); 1452 } 1453 return getTopic().get(0); 1454 } 1455 1456 /** 1457 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the content.) 1458 */ 1459 public List<ContactDetail> getAuthor() { 1460 if (this.author == null) 1461 this.author = new ArrayList<ContactDetail>(); 1462 return this.author; 1463 } 1464 1465 /** 1466 * @return Returns a reference to <code>this</code> for easy method chaining 1467 */ 1468 public EventDefinition setAuthor(List<ContactDetail> theAuthor) { 1469 this.author = theAuthor; 1470 return this; 1471 } 1472 1473 public boolean hasAuthor() { 1474 if (this.author == null) 1475 return false; 1476 for (ContactDetail item : this.author) 1477 if (!item.isEmpty()) 1478 return true; 1479 return false; 1480 } 1481 1482 public ContactDetail addAuthor() { //3 1483 ContactDetail t = new ContactDetail(); 1484 if (this.author == null) 1485 this.author = new ArrayList<ContactDetail>(); 1486 this.author.add(t); 1487 return t; 1488 } 1489 1490 public EventDefinition addAuthor(ContactDetail t) { //3 1491 if (t == null) 1492 return this; 1493 if (this.author == null) 1494 this.author = new ArrayList<ContactDetail>(); 1495 this.author.add(t); 1496 return this; 1497 } 1498 1499 /** 1500 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 1501 */ 1502 public ContactDetail getAuthorFirstRep() { 1503 if (getAuthor().isEmpty()) { 1504 addAuthor(); 1505 } 1506 return getAuthor().get(0); 1507 } 1508 1509 /** 1510 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the content.) 1511 */ 1512 public List<ContactDetail> getEditor() { 1513 if (this.editor == null) 1514 this.editor = new ArrayList<ContactDetail>(); 1515 return this.editor; 1516 } 1517 1518 /** 1519 * @return Returns a reference to <code>this</code> for easy method chaining 1520 */ 1521 public EventDefinition setEditor(List<ContactDetail> theEditor) { 1522 this.editor = theEditor; 1523 return this; 1524 } 1525 1526 public boolean hasEditor() { 1527 if (this.editor == null) 1528 return false; 1529 for (ContactDetail item : this.editor) 1530 if (!item.isEmpty()) 1531 return true; 1532 return false; 1533 } 1534 1535 public ContactDetail addEditor() { //3 1536 ContactDetail t = new ContactDetail(); 1537 if (this.editor == null) 1538 this.editor = new ArrayList<ContactDetail>(); 1539 this.editor.add(t); 1540 return t; 1541 } 1542 1543 public EventDefinition addEditor(ContactDetail t) { //3 1544 if (t == null) 1545 return this; 1546 if (this.editor == null) 1547 this.editor = new ArrayList<ContactDetail>(); 1548 this.editor.add(t); 1549 return this; 1550 } 1551 1552 /** 1553 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 1554 */ 1555 public ContactDetail getEditorFirstRep() { 1556 if (getEditor().isEmpty()) { 1557 addEditor(); 1558 } 1559 return getEditor().get(0); 1560 } 1561 1562 /** 1563 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.) 1564 */ 1565 public List<ContactDetail> getReviewer() { 1566 if (this.reviewer == null) 1567 this.reviewer = new ArrayList<ContactDetail>(); 1568 return this.reviewer; 1569 } 1570 1571 /** 1572 * @return Returns a reference to <code>this</code> for easy method chaining 1573 */ 1574 public EventDefinition setReviewer(List<ContactDetail> theReviewer) { 1575 this.reviewer = theReviewer; 1576 return this; 1577 } 1578 1579 public boolean hasReviewer() { 1580 if (this.reviewer == null) 1581 return false; 1582 for (ContactDetail item : this.reviewer) 1583 if (!item.isEmpty()) 1584 return true; 1585 return false; 1586 } 1587 1588 public ContactDetail addReviewer() { //3 1589 ContactDetail t = new ContactDetail(); 1590 if (this.reviewer == null) 1591 this.reviewer = new ArrayList<ContactDetail>(); 1592 this.reviewer.add(t); 1593 return t; 1594 } 1595 1596 public EventDefinition addReviewer(ContactDetail t) { //3 1597 if (t == null) 1598 return this; 1599 if (this.reviewer == null) 1600 this.reviewer = new ArrayList<ContactDetail>(); 1601 this.reviewer.add(t); 1602 return this; 1603 } 1604 1605 /** 1606 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 1607 */ 1608 public ContactDetail getReviewerFirstRep() { 1609 if (getReviewer().isEmpty()) { 1610 addReviewer(); 1611 } 1612 return getReviewer().get(0); 1613 } 1614 1615 /** 1616 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.) 1617 */ 1618 public List<ContactDetail> getEndorser() { 1619 if (this.endorser == null) 1620 this.endorser = new ArrayList<ContactDetail>(); 1621 return this.endorser; 1622 } 1623 1624 /** 1625 * @return Returns a reference to <code>this</code> for easy method chaining 1626 */ 1627 public EventDefinition setEndorser(List<ContactDetail> theEndorser) { 1628 this.endorser = theEndorser; 1629 return this; 1630 } 1631 1632 public boolean hasEndorser() { 1633 if (this.endorser == null) 1634 return false; 1635 for (ContactDetail item : this.endorser) 1636 if (!item.isEmpty()) 1637 return true; 1638 return false; 1639 } 1640 1641 public ContactDetail addEndorser() { //3 1642 ContactDetail t = new ContactDetail(); 1643 if (this.endorser == null) 1644 this.endorser = new ArrayList<ContactDetail>(); 1645 this.endorser.add(t); 1646 return t; 1647 } 1648 1649 public EventDefinition addEndorser(ContactDetail t) { //3 1650 if (t == null) 1651 return this; 1652 if (this.endorser == null) 1653 this.endorser = new ArrayList<ContactDetail>(); 1654 this.endorser.add(t); 1655 return this; 1656 } 1657 1658 /** 1659 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 1660 */ 1661 public ContactDetail getEndorserFirstRep() { 1662 if (getEndorser().isEmpty()) { 1663 addEndorser(); 1664 } 1665 return getEndorser().get(0); 1666 } 1667 1668 /** 1669 * @return {@link #relatedArtifact} (Related resources such as additional documentation, justification, or bibliographic references.) 1670 */ 1671 public List<RelatedArtifact> getRelatedArtifact() { 1672 if (this.relatedArtifact == null) 1673 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1674 return this.relatedArtifact; 1675 } 1676 1677 /** 1678 * @return Returns a reference to <code>this</code> for easy method chaining 1679 */ 1680 public EventDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1681 this.relatedArtifact = theRelatedArtifact; 1682 return this; 1683 } 1684 1685 public boolean hasRelatedArtifact() { 1686 if (this.relatedArtifact == null) 1687 return false; 1688 for (RelatedArtifact item : this.relatedArtifact) 1689 if (!item.isEmpty()) 1690 return true; 1691 return false; 1692 } 1693 1694 public RelatedArtifact addRelatedArtifact() { //3 1695 RelatedArtifact t = new RelatedArtifact(); 1696 if (this.relatedArtifact == null) 1697 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1698 this.relatedArtifact.add(t); 1699 return t; 1700 } 1701 1702 public EventDefinition addRelatedArtifact(RelatedArtifact t) { //3 1703 if (t == null) 1704 return this; 1705 if (this.relatedArtifact == null) 1706 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1707 this.relatedArtifact.add(t); 1708 return this; 1709 } 1710 1711 /** 1712 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 1713 */ 1714 public RelatedArtifact getRelatedArtifactFirstRep() { 1715 if (getRelatedArtifact().isEmpty()) { 1716 addRelatedArtifact(); 1717 } 1718 return getRelatedArtifact().get(0); 1719 } 1720 1721 /** 1722 * @return {@link #trigger} (The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met.) 1723 */ 1724 public List<TriggerDefinition> getTrigger() { 1725 if (this.trigger == null) 1726 this.trigger = new ArrayList<TriggerDefinition>(); 1727 return this.trigger; 1728 } 1729 1730 /** 1731 * @return Returns a reference to <code>this</code> for easy method chaining 1732 */ 1733 public EventDefinition setTrigger(List<TriggerDefinition> theTrigger) { 1734 this.trigger = theTrigger; 1735 return this; 1736 } 1737 1738 public boolean hasTrigger() { 1739 if (this.trigger == null) 1740 return false; 1741 for (TriggerDefinition item : this.trigger) 1742 if (!item.isEmpty()) 1743 return true; 1744 return false; 1745 } 1746 1747 public TriggerDefinition addTrigger() { //3 1748 TriggerDefinition t = new TriggerDefinition(); 1749 if (this.trigger == null) 1750 this.trigger = new ArrayList<TriggerDefinition>(); 1751 this.trigger.add(t); 1752 return t; 1753 } 1754 1755 public EventDefinition addTrigger(TriggerDefinition t) { //3 1756 if (t == null) 1757 return this; 1758 if (this.trigger == null) 1759 this.trigger = new ArrayList<TriggerDefinition>(); 1760 this.trigger.add(t); 1761 return this; 1762 } 1763 1764 /** 1765 * @return The first repetition of repeating field {@link #trigger}, creating it if it does not already exist {3} 1766 */ 1767 public TriggerDefinition getTriggerFirstRep() { 1768 if (getTrigger().isEmpty()) { 1769 addTrigger(); 1770 } 1771 return getTrigger().get(0); 1772 } 1773 1774 protected void listChildren(List<Property> children) { 1775 super.listChildren(children); 1776 children.add(new Property("url", "uri", "An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers.", 0, 1, url)); 1777 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1778 children.add(new Property("version", "string", "The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 1779 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 1780 children.add(new Property("name", "string", "A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1781 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the event definition.", 0, 1, title)); 1782 children.add(new Property("subtitle", "string", "An explanatory or alternate title for the event definition giving additional information about its content.", 0, 1, subtitle)); 1783 children.add(new Property("status", "code", "The status of this event definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1784 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 1785 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject)); 1786 children.add(new Property("date", "dateTime", "The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes.", 0, 1, date)); 1787 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the event definition.", 0, 1, publisher)); 1788 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1789 children.add(new Property("description", "markdown", "A free text natural language description of the event definition from a consumer's perspective.", 0, 1, description)); 1790 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate event definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1791 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the event definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1792 children.add(new Property("purpose", "markdown", "Explanation of why this event definition is needed and why it has been designed as it has.", 0, 1, purpose)); 1793 children.add(new Property("usage", "markdown", "A detailed description of how the event definition is used from a clinical perspective.", 0, 1, usage)); 1794 children.add(new Property("copyright", "markdown", "A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition.", 0, 1, copyright)); 1795 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 1796 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 1797 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 1798 children.add(new Property("effectivePeriod", "Period", "The period during which the event definition content was or is planned to be in active use.", 0, 1, effectivePeriod)); 1799 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 1800 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 1801 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 1802 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 1803 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 1804 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related resources such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 1805 children.add(new Property("trigger", "TriggerDefinition", "The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met.", 0, java.lang.Integer.MAX_VALUE, trigger)); 1806 } 1807 1808 @Override 1809 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1810 switch (_hash) { 1811 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this event definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this event definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the event definition is stored on different servers.", 0, 1, url); 1812 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this event definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 1813 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the event definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the event definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 1814 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1815 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1816 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1817 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1818 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the event definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1819 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the event definition.", 0, 1, title); 1820 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "An explanatory or alternate title for the event definition giving additional information about its content.", 0, 1, subtitle); 1821 case -892481550: /*status*/ return new Property("status", "code", "The status of this event definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1822 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this event definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 1823 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1824 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1825 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1826 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group)", "A code or group definition that describes the intended subject of the event definition.", 0, 1, subject); 1827 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the event definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the event definition changes.", 0, 1, date); 1828 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the event definition.", 0, 1, publisher); 1829 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1830 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the event definition from a consumer's perspective.", 0, 1, description); 1831 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate event definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1832 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the event definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1833 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this event definition is needed and why it has been designed as it has.", 0, 1, purpose); 1834 case 111574433: /*usage*/ return new Property("usage", "markdown", "A detailed description of how the event definition is used from a clinical perspective.", 0, 1, usage); 1835 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the event definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the event definition.", 0, 1, copyright); 1836 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 1837 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 1838 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 1839 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the event definition content was or is planned to be in active use.", 0, 1, effectivePeriod); 1840 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the module. Topics provide a high-level categorization of the module that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 1841 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 1842 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 1843 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 1844 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 1845 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related resources such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 1846 case -1059891784: /*trigger*/ return new Property("trigger", "TriggerDefinition", "The trigger element defines when the event occurs. If more than one trigger condition is specified, the event fires whenever any one of the trigger conditions is met.", 0, java.lang.Integer.MAX_VALUE, trigger); 1847 default: return super.getNamedProperty(_hash, _name, _checkValid); 1848 } 1849 1850 } 1851 1852 @Override 1853 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1854 switch (hash) { 1855 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1856 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1857 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1858 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 1859 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1860 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1861 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 1862 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1863 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 1864 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 1865 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1866 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1867 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1868 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1869 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1870 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1871 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 1872 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // MarkdownType 1873 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 1874 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 1875 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 1876 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 1877 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 1878 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 1879 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 1880 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 1881 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 1882 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 1883 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 1884 case -1059891784: /*trigger*/ return this.trigger == null ? new Base[0] : this.trigger.toArray(new Base[this.trigger.size()]); // TriggerDefinition 1885 default: return super.getProperty(hash, name, checkValid); 1886 } 1887 1888 } 1889 1890 @Override 1891 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1892 switch (hash) { 1893 case 116079: // url 1894 this.url = TypeConvertor.castToUri(value); // UriType 1895 return value; 1896 case -1618432855: // identifier 1897 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1898 return value; 1899 case 351608024: // version 1900 this.version = TypeConvertor.castToString(value); // StringType 1901 return value; 1902 case 1508158071: // versionAlgorithm 1903 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 1904 return value; 1905 case 3373707: // name 1906 this.name = TypeConvertor.castToString(value); // StringType 1907 return value; 1908 case 110371416: // title 1909 this.title = TypeConvertor.castToString(value); // StringType 1910 return value; 1911 case -2060497896: // subtitle 1912 this.subtitle = TypeConvertor.castToString(value); // StringType 1913 return value; 1914 case -892481550: // status 1915 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1916 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1917 return value; 1918 case -404562712: // experimental 1919 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 1920 return value; 1921 case -1867885268: // subject 1922 this.subject = TypeConvertor.castToType(value); // DataType 1923 return value; 1924 case 3076014: // date 1925 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1926 return value; 1927 case 1447404028: // publisher 1928 this.publisher = TypeConvertor.castToString(value); // StringType 1929 return value; 1930 case 951526432: // contact 1931 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 1932 return value; 1933 case -1724546052: // description 1934 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1935 return value; 1936 case -669707736: // useContext 1937 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 1938 return value; 1939 case -507075711: // jurisdiction 1940 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1941 return value; 1942 case -220463842: // purpose 1943 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 1944 return value; 1945 case 111574433: // usage 1946 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 1947 return value; 1948 case 1522889671: // copyright 1949 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 1950 return value; 1951 case 765157229: // copyrightLabel 1952 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 1953 return value; 1954 case 223539345: // approvalDate 1955 this.approvalDate = TypeConvertor.castToDate(value); // DateType 1956 return value; 1957 case -1687512484: // lastReviewDate 1958 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 1959 return value; 1960 case -403934648: // effectivePeriod 1961 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 1962 return value; 1963 case 110546223: // topic 1964 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1965 return value; 1966 case -1406328437: // author 1967 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 1968 return value; 1969 case -1307827859: // editor 1970 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 1971 return value; 1972 case -261190139: // reviewer 1973 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 1974 return value; 1975 case 1740277666: // endorser 1976 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 1977 return value; 1978 case 666807069: // relatedArtifact 1979 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 1980 return value; 1981 case -1059891784: // trigger 1982 this.getTrigger().add(TypeConvertor.castToTriggerDefinition(value)); // TriggerDefinition 1983 return value; 1984 default: return super.setProperty(hash, name, value); 1985 } 1986 1987 } 1988 1989 @Override 1990 public Base setProperty(String name, Base value) throws FHIRException { 1991 if (name.equals("url")) { 1992 this.url = TypeConvertor.castToUri(value); // UriType 1993 } else if (name.equals("identifier")) { 1994 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1995 } else if (name.equals("version")) { 1996 this.version = TypeConvertor.castToString(value); // StringType 1997 } else if (name.equals("versionAlgorithm[x]")) { 1998 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 1999 } else if (name.equals("name")) { 2000 this.name = TypeConvertor.castToString(value); // StringType 2001 } else if (name.equals("title")) { 2002 this.title = TypeConvertor.castToString(value); // StringType 2003 } else if (name.equals("subtitle")) { 2004 this.subtitle = TypeConvertor.castToString(value); // StringType 2005 } else if (name.equals("status")) { 2006 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2007 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2008 } else if (name.equals("experimental")) { 2009 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2010 } else if (name.equals("subject[x]")) { 2011 this.subject = TypeConvertor.castToType(value); // DataType 2012 } else if (name.equals("date")) { 2013 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2014 } else if (name.equals("publisher")) { 2015 this.publisher = TypeConvertor.castToString(value); // StringType 2016 } else if (name.equals("contact")) { 2017 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2018 } else if (name.equals("description")) { 2019 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2020 } else if (name.equals("useContext")) { 2021 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2022 } else if (name.equals("jurisdiction")) { 2023 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2024 } else if (name.equals("purpose")) { 2025 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2026 } else if (name.equals("usage")) { 2027 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 2028 } else if (name.equals("copyright")) { 2029 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2030 } else if (name.equals("copyrightLabel")) { 2031 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2032 } else if (name.equals("approvalDate")) { 2033 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2034 } else if (name.equals("lastReviewDate")) { 2035 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2036 } else if (name.equals("effectivePeriod")) { 2037 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 2038 } else if (name.equals("topic")) { 2039 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 2040 } else if (name.equals("author")) { 2041 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 2042 } else if (name.equals("editor")) { 2043 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 2044 } else if (name.equals("reviewer")) { 2045 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 2046 } else if (name.equals("endorser")) { 2047 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 2048 } else if (name.equals("relatedArtifact")) { 2049 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 2050 } else if (name.equals("trigger")) { 2051 this.getTrigger().add(TypeConvertor.castToTriggerDefinition(value)); 2052 } else 2053 return super.setProperty(name, value); 2054 return value; 2055 } 2056 2057 @Override 2058 public void removeChild(String name, Base value) throws FHIRException { 2059 if (name.equals("url")) { 2060 this.url = null; 2061 } else if (name.equals("identifier")) { 2062 this.getIdentifier().remove(value); 2063 } else if (name.equals("version")) { 2064 this.version = null; 2065 } else if (name.equals("versionAlgorithm[x]")) { 2066 this.versionAlgorithm = null; 2067 } else if (name.equals("name")) { 2068 this.name = null; 2069 } else if (name.equals("title")) { 2070 this.title = null; 2071 } else if (name.equals("subtitle")) { 2072 this.subtitle = null; 2073 } else if (name.equals("status")) { 2074 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2075 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2076 } else if (name.equals("experimental")) { 2077 this.experimental = null; 2078 } else if (name.equals("subject[x]")) { 2079 this.subject = null; 2080 } else if (name.equals("date")) { 2081 this.date = null; 2082 } else if (name.equals("publisher")) { 2083 this.publisher = null; 2084 } else if (name.equals("contact")) { 2085 this.getContact().remove(value); 2086 } else if (name.equals("description")) { 2087 this.description = null; 2088 } else if (name.equals("useContext")) { 2089 this.getUseContext().remove(value); 2090 } else if (name.equals("jurisdiction")) { 2091 this.getJurisdiction().remove(value); 2092 } else if (name.equals("purpose")) { 2093 this.purpose = null; 2094 } else if (name.equals("usage")) { 2095 this.usage = null; 2096 } else if (name.equals("copyright")) { 2097 this.copyright = null; 2098 } else if (name.equals("copyrightLabel")) { 2099 this.copyrightLabel = null; 2100 } else if (name.equals("approvalDate")) { 2101 this.approvalDate = null; 2102 } else if (name.equals("lastReviewDate")) { 2103 this.lastReviewDate = null; 2104 } else if (name.equals("effectivePeriod")) { 2105 this.effectivePeriod = null; 2106 } else if (name.equals("topic")) { 2107 this.getTopic().remove(value); 2108 } else if (name.equals("author")) { 2109 this.getAuthor().remove(value); 2110 } else if (name.equals("editor")) { 2111 this.getEditor().remove(value); 2112 } else if (name.equals("reviewer")) { 2113 this.getReviewer().remove(value); 2114 } else if (name.equals("endorser")) { 2115 this.getEndorser().remove(value); 2116 } else if (name.equals("relatedArtifact")) { 2117 this.getRelatedArtifact().remove(value); 2118 } else if (name.equals("trigger")) { 2119 this.getTrigger().remove(value); 2120 } else 2121 super.removeChild(name, value); 2122 2123 } 2124 2125 @Override 2126 public Base makeProperty(int hash, String name) throws FHIRException { 2127 switch (hash) { 2128 case 116079: return getUrlElement(); 2129 case -1618432855: return addIdentifier(); 2130 case 351608024: return getVersionElement(); 2131 case -115699031: return getVersionAlgorithm(); 2132 case 1508158071: return getVersionAlgorithm(); 2133 case 3373707: return getNameElement(); 2134 case 110371416: return getTitleElement(); 2135 case -2060497896: return getSubtitleElement(); 2136 case -892481550: return getStatusElement(); 2137 case -404562712: return getExperimentalElement(); 2138 case -573640748: return getSubject(); 2139 case -1867885268: return getSubject(); 2140 case 3076014: return getDateElement(); 2141 case 1447404028: return getPublisherElement(); 2142 case 951526432: return addContact(); 2143 case -1724546052: return getDescriptionElement(); 2144 case -669707736: return addUseContext(); 2145 case -507075711: return addJurisdiction(); 2146 case -220463842: return getPurposeElement(); 2147 case 111574433: return getUsageElement(); 2148 case 1522889671: return getCopyrightElement(); 2149 case 765157229: return getCopyrightLabelElement(); 2150 case 223539345: return getApprovalDateElement(); 2151 case -1687512484: return getLastReviewDateElement(); 2152 case -403934648: return getEffectivePeriod(); 2153 case 110546223: return addTopic(); 2154 case -1406328437: return addAuthor(); 2155 case -1307827859: return addEditor(); 2156 case -261190139: return addReviewer(); 2157 case 1740277666: return addEndorser(); 2158 case 666807069: return addRelatedArtifact(); 2159 case -1059891784: return addTrigger(); 2160 default: return super.makeProperty(hash, name); 2161 } 2162 2163 } 2164 2165 @Override 2166 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2167 switch (hash) { 2168 case 116079: /*url*/ return new String[] {"uri"}; 2169 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2170 case 351608024: /*version*/ return new String[] {"string"}; 2171 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2172 case 3373707: /*name*/ return new String[] {"string"}; 2173 case 110371416: /*title*/ return new String[] {"string"}; 2174 case -2060497896: /*subtitle*/ return new String[] {"string"}; 2175 case -892481550: /*status*/ return new String[] {"code"}; 2176 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2177 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference"}; 2178 case 3076014: /*date*/ return new String[] {"dateTime"}; 2179 case 1447404028: /*publisher*/ return new String[] {"string"}; 2180 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2181 case -1724546052: /*description*/ return new String[] {"markdown"}; 2182 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2183 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2184 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2185 case 111574433: /*usage*/ return new String[] {"markdown"}; 2186 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2187 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2188 case 223539345: /*approvalDate*/ return new String[] {"date"}; 2189 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 2190 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 2191 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 2192 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 2193 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 2194 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 2195 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 2196 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 2197 case -1059891784: /*trigger*/ return new String[] {"TriggerDefinition"}; 2198 default: return super.getTypesForProperty(hash, name); 2199 } 2200 2201 } 2202 2203 @Override 2204 public Base addChild(String name) throws FHIRException { 2205 if (name.equals("url")) { 2206 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.url"); 2207 } 2208 else if (name.equals("identifier")) { 2209 return addIdentifier(); 2210 } 2211 else if (name.equals("version")) { 2212 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.version"); 2213 } 2214 else if (name.equals("versionAlgorithmString")) { 2215 this.versionAlgorithm = new StringType(); 2216 return this.versionAlgorithm; 2217 } 2218 else if (name.equals("versionAlgorithmCoding")) { 2219 this.versionAlgorithm = new Coding(); 2220 return this.versionAlgorithm; 2221 } 2222 else if (name.equals("name")) { 2223 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.name"); 2224 } 2225 else if (name.equals("title")) { 2226 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.title"); 2227 } 2228 else if (name.equals("subtitle")) { 2229 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.subtitle"); 2230 } 2231 else if (name.equals("status")) { 2232 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.status"); 2233 } 2234 else if (name.equals("experimental")) { 2235 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.experimental"); 2236 } 2237 else if (name.equals("subjectCodeableConcept")) { 2238 this.subject = new CodeableConcept(); 2239 return this.subject; 2240 } 2241 else if (name.equals("subjectReference")) { 2242 this.subject = new Reference(); 2243 return this.subject; 2244 } 2245 else if (name.equals("date")) { 2246 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.date"); 2247 } 2248 else if (name.equals("publisher")) { 2249 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.publisher"); 2250 } 2251 else if (name.equals("contact")) { 2252 return addContact(); 2253 } 2254 else if (name.equals("description")) { 2255 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.description"); 2256 } 2257 else if (name.equals("useContext")) { 2258 return addUseContext(); 2259 } 2260 else if (name.equals("jurisdiction")) { 2261 return addJurisdiction(); 2262 } 2263 else if (name.equals("purpose")) { 2264 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.purpose"); 2265 } 2266 else if (name.equals("usage")) { 2267 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.usage"); 2268 } 2269 else if (name.equals("copyright")) { 2270 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.copyright"); 2271 } 2272 else if (name.equals("copyrightLabel")) { 2273 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.copyrightLabel"); 2274 } 2275 else if (name.equals("approvalDate")) { 2276 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.approvalDate"); 2277 } 2278 else if (name.equals("lastReviewDate")) { 2279 throw new FHIRException("Cannot call addChild on a singleton property EventDefinition.lastReviewDate"); 2280 } 2281 else if (name.equals("effectivePeriod")) { 2282 this.effectivePeriod = new Period(); 2283 return this.effectivePeriod; 2284 } 2285 else if (name.equals("topic")) { 2286 return addTopic(); 2287 } 2288 else if (name.equals("author")) { 2289 return addAuthor(); 2290 } 2291 else if (name.equals("editor")) { 2292 return addEditor(); 2293 } 2294 else if (name.equals("reviewer")) { 2295 return addReviewer(); 2296 } 2297 else if (name.equals("endorser")) { 2298 return addEndorser(); 2299 } 2300 else if (name.equals("relatedArtifact")) { 2301 return addRelatedArtifact(); 2302 } 2303 else if (name.equals("trigger")) { 2304 return addTrigger(); 2305 } 2306 else 2307 return super.addChild(name); 2308 } 2309 2310 public String fhirType() { 2311 return "EventDefinition"; 2312 2313 } 2314 2315 public EventDefinition copy() { 2316 EventDefinition dst = new EventDefinition(); 2317 copyValues(dst); 2318 return dst; 2319 } 2320 2321 public void copyValues(EventDefinition dst) { 2322 super.copyValues(dst); 2323 dst.url = url == null ? null : url.copy(); 2324 if (identifier != null) { 2325 dst.identifier = new ArrayList<Identifier>(); 2326 for (Identifier i : identifier) 2327 dst.identifier.add(i.copy()); 2328 }; 2329 dst.version = version == null ? null : version.copy(); 2330 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2331 dst.name = name == null ? null : name.copy(); 2332 dst.title = title == null ? null : title.copy(); 2333 dst.subtitle = subtitle == null ? null : subtitle.copy(); 2334 dst.status = status == null ? null : status.copy(); 2335 dst.experimental = experimental == null ? null : experimental.copy(); 2336 dst.subject = subject == null ? null : subject.copy(); 2337 dst.date = date == null ? null : date.copy(); 2338 dst.publisher = publisher == null ? null : publisher.copy(); 2339 if (contact != null) { 2340 dst.contact = new ArrayList<ContactDetail>(); 2341 for (ContactDetail i : contact) 2342 dst.contact.add(i.copy()); 2343 }; 2344 dst.description = description == null ? null : description.copy(); 2345 if (useContext != null) { 2346 dst.useContext = new ArrayList<UsageContext>(); 2347 for (UsageContext i : useContext) 2348 dst.useContext.add(i.copy()); 2349 }; 2350 if (jurisdiction != null) { 2351 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2352 for (CodeableConcept i : jurisdiction) 2353 dst.jurisdiction.add(i.copy()); 2354 }; 2355 dst.purpose = purpose == null ? null : purpose.copy(); 2356 dst.usage = usage == null ? null : usage.copy(); 2357 dst.copyright = copyright == null ? null : copyright.copy(); 2358 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2359 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2360 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2361 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 2362 if (topic != null) { 2363 dst.topic = new ArrayList<CodeableConcept>(); 2364 for (CodeableConcept i : topic) 2365 dst.topic.add(i.copy()); 2366 }; 2367 if (author != null) { 2368 dst.author = new ArrayList<ContactDetail>(); 2369 for (ContactDetail i : author) 2370 dst.author.add(i.copy()); 2371 }; 2372 if (editor != null) { 2373 dst.editor = new ArrayList<ContactDetail>(); 2374 for (ContactDetail i : editor) 2375 dst.editor.add(i.copy()); 2376 }; 2377 if (reviewer != null) { 2378 dst.reviewer = new ArrayList<ContactDetail>(); 2379 for (ContactDetail i : reviewer) 2380 dst.reviewer.add(i.copy()); 2381 }; 2382 if (endorser != null) { 2383 dst.endorser = new ArrayList<ContactDetail>(); 2384 for (ContactDetail i : endorser) 2385 dst.endorser.add(i.copy()); 2386 }; 2387 if (relatedArtifact != null) { 2388 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 2389 for (RelatedArtifact i : relatedArtifact) 2390 dst.relatedArtifact.add(i.copy()); 2391 }; 2392 if (trigger != null) { 2393 dst.trigger = new ArrayList<TriggerDefinition>(); 2394 for (TriggerDefinition i : trigger) 2395 dst.trigger.add(i.copy()); 2396 }; 2397 } 2398 2399 protected EventDefinition typedCopy() { 2400 return copy(); 2401 } 2402 2403 @Override 2404 public boolean equalsDeep(Base other_) { 2405 if (!super.equalsDeep(other_)) 2406 return false; 2407 if (!(other_ instanceof EventDefinition)) 2408 return false; 2409 EventDefinition o = (EventDefinition) other_; 2410 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2411 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2412 && compareDeep(subtitle, o.subtitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 2413 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) 2414 && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 2415 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 2416 && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 2417 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 2418 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 2419 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 2420 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(trigger, o.trigger, true) 2421 ; 2422 } 2423 2424 @Override 2425 public boolean equalsShallow(Base other_) { 2426 if (!super.equalsShallow(other_)) 2427 return false; 2428 if (!(other_ instanceof EventDefinition)) 2429 return false; 2430 EventDefinition o = (EventDefinition) other_; 2431 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2432 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) && compareValues(status, o.status, true) 2433 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 2434 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) 2435 && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 2436 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 2437 ; 2438 } 2439 2440 public boolean isEmpty() { 2441 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2442 , versionAlgorithm, name, title, subtitle, status, experimental, subject, date 2443 , publisher, contact, description, useContext, jurisdiction, purpose, usage, copyright 2444 , copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor 2445 , reviewer, endorser, relatedArtifact, trigger); 2446 } 2447 2448 @Override 2449 public ResourceType getResourceType() { 2450 return ResourceType.EventDefinition; 2451 } 2452 2453 /** 2454 * Search parameter: <b>context-quantity</b> 2455 * <p> 2456 * Description: <b>Multiple Resources: 2457 2458* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2459* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2460* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2461* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2462* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2463* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2464* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2465* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2466* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2467* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2468* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2469* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2470* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2471* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2472* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2473* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2474* [Library](library.html): A quantity- or range-valued use context assigned to the library 2475* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2476* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2477* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2478* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2479* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2480* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2481* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2482* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2483* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2484* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2485* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2486* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2487* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2488</b><br> 2489 * Type: <b>quantity</b><br> 2490 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2491 * </p> 2492 */ 2493 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2494 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2495 /** 2496 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2497 * <p> 2498 * Description: <b>Multiple Resources: 2499 2500* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2501* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2502* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2503* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2504* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2505* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2506* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2507* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2508* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2509* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2510* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2511* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2512* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2513* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2514* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2515* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2516* [Library](library.html): A quantity- or range-valued use context assigned to the library 2517* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2518* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2519* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2520* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2521* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2522* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2523* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2524* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2525* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2526* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2527* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2528* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2529* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2530</b><br> 2531 * Type: <b>quantity</b><br> 2532 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2533 * </p> 2534 */ 2535 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 2536 2537 /** 2538 * Search parameter: <b>context-type-quantity</b> 2539 * <p> 2540 * Description: <b>Multiple Resources: 2541 2542* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2543* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2544* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2545* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2546* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2547* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2548* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2549* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2550* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2551* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2552* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2553* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2554* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2555* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2556* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2557* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2558* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2559* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2560* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2561* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2562* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2563* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2564* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2565* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2566* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2567* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2568* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2569* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2570* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2571* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2572</b><br> 2573 * Type: <b>composite</b><br> 2574 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2575 * </p> 2576 */ 2577 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2578 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2579 /** 2580 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2581 * <p> 2582 * Description: <b>Multiple Resources: 2583 2584* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2585* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2586* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2587* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2588* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2589* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2590* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2591* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2592* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2593* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2594* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2595* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2596* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2597* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2598* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2599* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2600* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2601* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2602* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2603* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2604* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2605* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2606* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2607* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2608* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2609* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2610* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2611* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2612* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2613* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2614</b><br> 2615 * Type: <b>composite</b><br> 2616 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2617 * </p> 2618 */ 2619 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 2620 2621 /** 2622 * Search parameter: <b>context-type-value</b> 2623 * <p> 2624 * Description: <b>Multiple Resources: 2625 2626* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2627* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2628* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2629* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2630* [Citation](citation.html): A use context type and value assigned to the citation 2631* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2632* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2633* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2634* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2635* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2636* [Evidence](evidence.html): A use context type and value assigned to the evidence 2637* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2638* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2639* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2640* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2641* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2642* [Library](library.html): A use context type and value assigned to the library 2643* [Measure](measure.html): A use context type and value assigned to the measure 2644* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2645* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2646* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2647* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2648* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2649* [Requirements](requirements.html): A use context type and value assigned to the requirements 2650* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2651* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2652* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2653* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2654* [TestScript](testscript.html): A use context type and value assigned to the test script 2655* [ValueSet](valueset.html): A use context type and value assigned to the value set 2656</b><br> 2657 * Type: <b>composite</b><br> 2658 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2659 * </p> 2660 */ 2661 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 2662 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2663 /** 2664 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2665 * <p> 2666 * Description: <b>Multiple Resources: 2667 2668* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2669* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2670* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2671* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2672* [Citation](citation.html): A use context type and value assigned to the citation 2673* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2674* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2675* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2676* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2677* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2678* [Evidence](evidence.html): A use context type and value assigned to the evidence 2679* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2680* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2681* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2682* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2683* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2684* [Library](library.html): A use context type and value assigned to the library 2685* [Measure](measure.html): A use context type and value assigned to the measure 2686* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2687* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2688* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2689* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2690* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2691* [Requirements](requirements.html): A use context type and value assigned to the requirements 2692* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2693* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2694* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2695* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2696* [TestScript](testscript.html): A use context type and value assigned to the test script 2697* [ValueSet](valueset.html): A use context type and value assigned to the value set 2698</b><br> 2699 * Type: <b>composite</b><br> 2700 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2701 * </p> 2702 */ 2703 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 2704 2705 /** 2706 * Search parameter: <b>context-type</b> 2707 * <p> 2708 * Description: <b>Multiple Resources: 2709 2710* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2711* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2712* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2713* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2714* [Citation](citation.html): A type of use context assigned to the citation 2715* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2716* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2717* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2718* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2719* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2720* [Evidence](evidence.html): A type of use context assigned to the evidence 2721* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2722* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2723* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2724* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2725* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2726* [Library](library.html): A type of use context assigned to the library 2727* [Measure](measure.html): A type of use context assigned to the measure 2728* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2729* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2730* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2731* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2732* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2733* [Requirements](requirements.html): A type of use context assigned to the requirements 2734* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2735* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2736* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2737* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2738* [TestScript](testscript.html): A type of use context assigned to the test script 2739* [ValueSet](valueset.html): A type of use context assigned to the value set 2740</b><br> 2741 * Type: <b>token</b><br> 2742 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2743 * </p> 2744 */ 2745 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 2746 public static final String SP_CONTEXT_TYPE = "context-type"; 2747 /** 2748 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2749 * <p> 2750 * Description: <b>Multiple Resources: 2751 2752* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2753* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2754* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2755* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2756* [Citation](citation.html): A type of use context assigned to the citation 2757* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2758* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2759* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2760* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2761* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2762* [Evidence](evidence.html): A type of use context assigned to the evidence 2763* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2764* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2765* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2766* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2767* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2768* [Library](library.html): A type of use context assigned to the library 2769* [Measure](measure.html): A type of use context assigned to the measure 2770* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2771* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2772* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2773* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2774* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2775* [Requirements](requirements.html): A type of use context assigned to the requirements 2776* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2777* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2778* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2779* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2780* [TestScript](testscript.html): A type of use context assigned to the test script 2781* [ValueSet](valueset.html): A type of use context assigned to the value set 2782</b><br> 2783 * Type: <b>token</b><br> 2784 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2785 * </p> 2786 */ 2787 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 2788 2789 /** 2790 * Search parameter: <b>context</b> 2791 * <p> 2792 * Description: <b>Multiple Resources: 2793 2794* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 2795* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 2796* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 2797* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 2798* [Citation](citation.html): A use context assigned to the citation 2799* [CodeSystem](codesystem.html): A use context assigned to the code system 2800* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 2801* [ConceptMap](conceptmap.html): A use context assigned to the concept map 2802* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 2803* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 2804* [Evidence](evidence.html): A use context assigned to the evidence 2805* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 2806* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 2807* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 2808* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 2809* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 2810* [Library](library.html): A use context assigned to the library 2811* [Measure](measure.html): A use context assigned to the measure 2812* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 2813* [NamingSystem](namingsystem.html): A use context assigned to the naming system 2814* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 2815* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 2816* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 2817* [Requirements](requirements.html): A use context assigned to the requirements 2818* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 2819* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 2820* [StructureMap](structuremap.html): A use context assigned to the structure map 2821* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 2822* [TestScript](testscript.html): A use context assigned to the test script 2823* [ValueSet](valueset.html): A use context assigned to the value set 2824</b><br> 2825 * Type: <b>token</b><br> 2826 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 2827 * </p> 2828 */ 2829 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 2830 public static final String SP_CONTEXT = "context"; 2831 /** 2832 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2833 * <p> 2834 * Description: <b>Multiple Resources: 2835 2836* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 2837* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 2838* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 2839* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 2840* [Citation](citation.html): A use context assigned to the citation 2841* [CodeSystem](codesystem.html): A use context assigned to the code system 2842* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 2843* [ConceptMap](conceptmap.html): A use context assigned to the concept map 2844* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 2845* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 2846* [Evidence](evidence.html): A use context assigned to the evidence 2847* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 2848* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 2849* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 2850* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 2851* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 2852* [Library](library.html): A use context assigned to the library 2853* [Measure](measure.html): A use context assigned to the measure 2854* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 2855* [NamingSystem](namingsystem.html): A use context assigned to the naming system 2856* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 2857* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 2858* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 2859* [Requirements](requirements.html): A use context assigned to the requirements 2860* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 2861* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 2862* [StructureMap](structuremap.html): A use context assigned to the structure map 2863* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 2864* [TestScript](testscript.html): A use context assigned to the test script 2865* [ValueSet](valueset.html): A use context assigned to the value set 2866</b><br> 2867 * Type: <b>token</b><br> 2868 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 2869 * </p> 2870 */ 2871 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 2872 2873 /** 2874 * Search parameter: <b>date</b> 2875 * <p> 2876 * Description: <b>Multiple Resources: 2877 2878* [ActivityDefinition](activitydefinition.html): The activity definition publication date 2879* [ActorDefinition](actordefinition.html): The Actor Definition publication date 2880* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 2881* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 2882* [Citation](citation.html): The citation publication date 2883* [CodeSystem](codesystem.html): The code system publication date 2884* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 2885* [ConceptMap](conceptmap.html): The concept map publication date 2886* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 2887* [EventDefinition](eventdefinition.html): The event definition publication date 2888* [Evidence](evidence.html): The evidence publication date 2889* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 2890* [ExampleScenario](examplescenario.html): The example scenario publication date 2891* [GraphDefinition](graphdefinition.html): The graph definition publication date 2892* [ImplementationGuide](implementationguide.html): The implementation guide publication date 2893* [Library](library.html): The library publication date 2894* [Measure](measure.html): The measure publication date 2895* [MessageDefinition](messagedefinition.html): The message definition publication date 2896* [NamingSystem](namingsystem.html): The naming system publication date 2897* [OperationDefinition](operationdefinition.html): The operation definition publication date 2898* [PlanDefinition](plandefinition.html): The plan definition publication date 2899* [Questionnaire](questionnaire.html): The questionnaire publication date 2900* [Requirements](requirements.html): The requirements publication date 2901* [SearchParameter](searchparameter.html): The search parameter publication date 2902* [StructureDefinition](structuredefinition.html): The structure definition publication date 2903* [StructureMap](structuremap.html): The structure map publication date 2904* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 2905* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 2906* [TestScript](testscript.html): The test script publication date 2907* [ValueSet](valueset.html): The value set publication date 2908</b><br> 2909 * Type: <b>date</b><br> 2910 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 2911 * </p> 2912 */ 2913 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 2914 public static final String SP_DATE = "date"; 2915 /** 2916 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2917 * <p> 2918 * Description: <b>Multiple Resources: 2919 2920* [ActivityDefinition](activitydefinition.html): The activity definition publication date 2921* [ActorDefinition](actordefinition.html): The Actor Definition publication date 2922* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 2923* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 2924* [Citation](citation.html): The citation publication date 2925* [CodeSystem](codesystem.html): The code system publication date 2926* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 2927* [ConceptMap](conceptmap.html): The concept map publication date 2928* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 2929* [EventDefinition](eventdefinition.html): The event definition publication date 2930* [Evidence](evidence.html): The evidence publication date 2931* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 2932* [ExampleScenario](examplescenario.html): The example scenario publication date 2933* [GraphDefinition](graphdefinition.html): The graph definition publication date 2934* [ImplementationGuide](implementationguide.html): The implementation guide publication date 2935* [Library](library.html): The library publication date 2936* [Measure](measure.html): The measure publication date 2937* [MessageDefinition](messagedefinition.html): The message definition publication date 2938* [NamingSystem](namingsystem.html): The naming system publication date 2939* [OperationDefinition](operationdefinition.html): The operation definition publication date 2940* [PlanDefinition](plandefinition.html): The plan definition publication date 2941* [Questionnaire](questionnaire.html): The questionnaire publication date 2942* [Requirements](requirements.html): The requirements publication date 2943* [SearchParameter](searchparameter.html): The search parameter publication date 2944* [StructureDefinition](structuredefinition.html): The structure definition publication date 2945* [StructureMap](structuremap.html): The structure map publication date 2946* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 2947* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 2948* [TestScript](testscript.html): The test script publication date 2949* [ValueSet](valueset.html): The value set publication date 2950</b><br> 2951 * Type: <b>date</b><br> 2952 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 2953 * </p> 2954 */ 2955 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2956 2957 /** 2958 * Search parameter: <b>description</b> 2959 * <p> 2960 * Description: <b>Multiple Resources: 2961 2962* [ActivityDefinition](activitydefinition.html): The description of the activity definition 2963* [ActorDefinition](actordefinition.html): The description of the Actor Definition 2964* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 2965* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 2966* [Citation](citation.html): The description of the citation 2967* [CodeSystem](codesystem.html): The description of the code system 2968* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 2969* [ConceptMap](conceptmap.html): The description of the concept map 2970* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 2971* [EventDefinition](eventdefinition.html): The description of the event definition 2972* [Evidence](evidence.html): The description of the evidence 2973* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 2974* [GraphDefinition](graphdefinition.html): The description of the graph definition 2975* [ImplementationGuide](implementationguide.html): The description of the implementation guide 2976* [Library](library.html): The description of the library 2977* [Measure](measure.html): The description of the measure 2978* [MessageDefinition](messagedefinition.html): The description of the message definition 2979* [NamingSystem](namingsystem.html): The description of the naming system 2980* [OperationDefinition](operationdefinition.html): The description of the operation definition 2981* [PlanDefinition](plandefinition.html): The description of the plan definition 2982* [Questionnaire](questionnaire.html): The description of the questionnaire 2983* [Requirements](requirements.html): The description of the requirements 2984* [SearchParameter](searchparameter.html): The description of the search parameter 2985* [StructureDefinition](structuredefinition.html): The description of the structure definition 2986* [StructureMap](structuremap.html): The description of the structure map 2987* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 2988* [TestScript](testscript.html): The description of the test script 2989* [ValueSet](valueset.html): The description of the value set 2990</b><br> 2991 * Type: <b>string</b><br> 2992 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 2993 * </p> 2994 */ 2995 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 2996 public static final String SP_DESCRIPTION = "description"; 2997 /** 2998 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2999 * <p> 3000 * Description: <b>Multiple Resources: 3001 3002* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3003* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3004* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3005* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3006* [Citation](citation.html): The description of the citation 3007* [CodeSystem](codesystem.html): The description of the code system 3008* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3009* [ConceptMap](conceptmap.html): The description of the concept map 3010* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3011* [EventDefinition](eventdefinition.html): The description of the event definition 3012* [Evidence](evidence.html): The description of the evidence 3013* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3014* [GraphDefinition](graphdefinition.html): The description of the graph definition 3015* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3016* [Library](library.html): The description of the library 3017* [Measure](measure.html): The description of the measure 3018* [MessageDefinition](messagedefinition.html): The description of the message definition 3019* [NamingSystem](namingsystem.html): The description of the naming system 3020* [OperationDefinition](operationdefinition.html): The description of the operation definition 3021* [PlanDefinition](plandefinition.html): The description of the plan definition 3022* [Questionnaire](questionnaire.html): The description of the questionnaire 3023* [Requirements](requirements.html): The description of the requirements 3024* [SearchParameter](searchparameter.html): The description of the search parameter 3025* [StructureDefinition](structuredefinition.html): The description of the structure definition 3026* [StructureMap](structuremap.html): The description of the structure map 3027* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3028* [TestScript](testscript.html): The description of the test script 3029* [ValueSet](valueset.html): The description of the value set 3030</b><br> 3031 * Type: <b>string</b><br> 3032 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3033 * </p> 3034 */ 3035 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3036 3037 /** 3038 * Search parameter: <b>identifier</b> 3039 * <p> 3040 * Description: <b>Multiple Resources: 3041 3042* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3043* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3044* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3045* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3046* [Citation](citation.html): External identifier for the citation 3047* [CodeSystem](codesystem.html): External identifier for the code system 3048* [ConceptMap](conceptmap.html): External identifier for the concept map 3049* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3050* [EventDefinition](eventdefinition.html): External identifier for the event definition 3051* [Evidence](evidence.html): External identifier for the evidence 3052* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3053* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3054* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3055* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3056* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3057* [Library](library.html): External identifier for the library 3058* [Measure](measure.html): External identifier for the measure 3059* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3060* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3061* [NamingSystem](namingsystem.html): External identifier for the naming system 3062* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3063* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3064* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3065* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3066* [Requirements](requirements.html): External identifier for the requirements 3067* [SearchParameter](searchparameter.html): External identifier for the search parameter 3068* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3069* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3070* [StructureMap](structuremap.html): External identifier for the structure map 3071* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3072* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3073* [TestPlan](testplan.html): An identifier for the test plan 3074* [TestScript](testscript.html): External identifier for the test script 3075* [ValueSet](valueset.html): External identifier for the value set 3076</b><br> 3077 * Type: <b>token</b><br> 3078 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3079 * </p> 3080 */ 3081 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3082 public static final String SP_IDENTIFIER = "identifier"; 3083 /** 3084 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3085 * <p> 3086 * Description: <b>Multiple Resources: 3087 3088* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3089* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3090* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3091* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3092* [Citation](citation.html): External identifier for the citation 3093* [CodeSystem](codesystem.html): External identifier for the code system 3094* [ConceptMap](conceptmap.html): External identifier for the concept map 3095* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3096* [EventDefinition](eventdefinition.html): External identifier for the event definition 3097* [Evidence](evidence.html): External identifier for the evidence 3098* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3099* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3100* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3101* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3102* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3103* [Library](library.html): External identifier for the library 3104* [Measure](measure.html): External identifier for the measure 3105* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3106* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3107* [NamingSystem](namingsystem.html): External identifier for the naming system 3108* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3109* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3110* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3111* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3112* [Requirements](requirements.html): External identifier for the requirements 3113* [SearchParameter](searchparameter.html): External identifier for the search parameter 3114* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3115* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3116* [StructureMap](structuremap.html): External identifier for the structure map 3117* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3118* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3119* [TestPlan](testplan.html): An identifier for the test plan 3120* [TestScript](testscript.html): External identifier for the test script 3121* [ValueSet](valueset.html): External identifier for the value set 3122</b><br> 3123 * Type: <b>token</b><br> 3124 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3125 * </p> 3126 */ 3127 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3128 3129 /** 3130 * Search parameter: <b>jurisdiction</b> 3131 * <p> 3132 * Description: <b>Multiple Resources: 3133 3134* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3135* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3136* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3137* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3138* [Citation](citation.html): Intended jurisdiction for the citation 3139* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3140* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3141* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3142* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3143* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3144* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3145* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3146* [Library](library.html): Intended jurisdiction for the library 3147* [Measure](measure.html): Intended jurisdiction for the measure 3148* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3149* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3150* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3151* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3152* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3153* [Requirements](requirements.html): Intended jurisdiction for the requirements 3154* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3155* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3156* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3157* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3158* [TestScript](testscript.html): Intended jurisdiction for the test script 3159* [ValueSet](valueset.html): Intended jurisdiction for the value set 3160</b><br> 3161 * Type: <b>token</b><br> 3162 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3163 * </p> 3164 */ 3165 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3166 public static final String SP_JURISDICTION = "jurisdiction"; 3167 /** 3168 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3169 * <p> 3170 * Description: <b>Multiple Resources: 3171 3172* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3173* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3174* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3175* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3176* [Citation](citation.html): Intended jurisdiction for the citation 3177* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3178* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3179* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3180* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3181* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3182* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3183* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3184* [Library](library.html): Intended jurisdiction for the library 3185* [Measure](measure.html): Intended jurisdiction for the measure 3186* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3187* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3188* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3189* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3190* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3191* [Requirements](requirements.html): Intended jurisdiction for the requirements 3192* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3193* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3194* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3195* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3196* [TestScript](testscript.html): Intended jurisdiction for the test script 3197* [ValueSet](valueset.html): Intended jurisdiction for the value set 3198</b><br> 3199 * Type: <b>token</b><br> 3200 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3201 * </p> 3202 */ 3203 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3204 3205 /** 3206 * Search parameter: <b>name</b> 3207 * <p> 3208 * Description: <b>Multiple Resources: 3209 3210* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3211* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3212* [Citation](citation.html): Computationally friendly name of the citation 3213* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3214* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3215* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3216* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3217* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3218* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3219* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3220* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3221* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3222* [Library](library.html): Computationally friendly name of the library 3223* [Measure](measure.html): Computationally friendly name of the measure 3224* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3225* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3226* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3227* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3228* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3229* [Requirements](requirements.html): Computationally friendly name of the requirements 3230* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3231* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3232* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3233* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3234* [TestScript](testscript.html): Computationally friendly name of the test script 3235* [ValueSet](valueset.html): Computationally friendly name of the value set 3236</b><br> 3237 * Type: <b>string</b><br> 3238 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3239 * </p> 3240 */ 3241 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3242 public static final String SP_NAME = "name"; 3243 /** 3244 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3245 * <p> 3246 * Description: <b>Multiple Resources: 3247 3248* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3249* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3250* [Citation](citation.html): Computationally friendly name of the citation 3251* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3252* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3253* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3254* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3255* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3256* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3257* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3258* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3259* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3260* [Library](library.html): Computationally friendly name of the library 3261* [Measure](measure.html): Computationally friendly name of the measure 3262* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3263* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3264* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3265* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3266* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3267* [Requirements](requirements.html): Computationally friendly name of the requirements 3268* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3269* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3270* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3271* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3272* [TestScript](testscript.html): Computationally friendly name of the test script 3273* [ValueSet](valueset.html): Computationally friendly name of the value set 3274</b><br> 3275 * Type: <b>string</b><br> 3276 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3277 * </p> 3278 */ 3279 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3280 3281 /** 3282 * Search parameter: <b>publisher</b> 3283 * <p> 3284 * Description: <b>Multiple Resources: 3285 3286* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3287* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3288* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3289* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3290* [Citation](citation.html): Name of the publisher of the citation 3291* [CodeSystem](codesystem.html): Name of the publisher of the code system 3292* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3293* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3294* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3295* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3296* [Evidence](evidence.html): Name of the publisher of the evidence 3297* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3298* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3299* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3300* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3301* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3302* [Library](library.html): Name of the publisher of the library 3303* [Measure](measure.html): Name of the publisher of the measure 3304* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3305* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3306* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3307* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3308* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3309* [Requirements](requirements.html): Name of the publisher of the requirements 3310* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3311* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3312* [StructureMap](structuremap.html): Name of the publisher of the structure map 3313* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3314* [TestScript](testscript.html): Name of the publisher of the test script 3315* [ValueSet](valueset.html): Name of the publisher of the value set 3316</b><br> 3317 * Type: <b>string</b><br> 3318 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3319 * </p> 3320 */ 3321 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3322 public static final String SP_PUBLISHER = "publisher"; 3323 /** 3324 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3325 * <p> 3326 * Description: <b>Multiple Resources: 3327 3328* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3329* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3330* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3331* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3332* [Citation](citation.html): Name of the publisher of the citation 3333* [CodeSystem](codesystem.html): Name of the publisher of the code system 3334* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3335* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3336* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3337* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3338* [Evidence](evidence.html): Name of the publisher of the evidence 3339* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3340* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3341* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3342* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3343* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3344* [Library](library.html): Name of the publisher of the library 3345* [Measure](measure.html): Name of the publisher of the measure 3346* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3347* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3348* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3349* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3350* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3351* [Requirements](requirements.html): Name of the publisher of the requirements 3352* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3353* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3354* [StructureMap](structuremap.html): Name of the publisher of the structure map 3355* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3356* [TestScript](testscript.html): Name of the publisher of the test script 3357* [ValueSet](valueset.html): Name of the publisher of the value set 3358</b><br> 3359 * Type: <b>string</b><br> 3360 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3361 * </p> 3362 */ 3363 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3364 3365 /** 3366 * Search parameter: <b>status</b> 3367 * <p> 3368 * Description: <b>Multiple Resources: 3369 3370* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3371* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3372* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3373* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3374* [Citation](citation.html): The current status of the citation 3375* [CodeSystem](codesystem.html): The current status of the code system 3376* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3377* [ConceptMap](conceptmap.html): The current status of the concept map 3378* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3379* [EventDefinition](eventdefinition.html): The current status of the event definition 3380* [Evidence](evidence.html): The current status of the evidence 3381* [EvidenceReport](evidencereport.html): The current status of the evidence report 3382* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3383* [ExampleScenario](examplescenario.html): The current status of the example scenario 3384* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3385* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3386* [Library](library.html): The current status of the library 3387* [Measure](measure.html): The current status of the measure 3388* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3389* [MessageDefinition](messagedefinition.html): The current status of the message definition 3390* [NamingSystem](namingsystem.html): The current status of the naming system 3391* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3392* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3393* [PlanDefinition](plandefinition.html): The current status of the plan definition 3394* [Questionnaire](questionnaire.html): The current status of the questionnaire 3395* [Requirements](requirements.html): The current status of the requirements 3396* [SearchParameter](searchparameter.html): The current status of the search parameter 3397* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3398* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3399* [StructureMap](structuremap.html): The current status of the structure map 3400* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3401* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3402* [TestPlan](testplan.html): The current status of the test plan 3403* [TestScript](testscript.html): The current status of the test script 3404* [ValueSet](valueset.html): The current status of the value set 3405</b><br> 3406 * Type: <b>token</b><br> 3407 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3408 * </p> 3409 */ 3410 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3411 public static final String SP_STATUS = "status"; 3412 /** 3413 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3414 * <p> 3415 * Description: <b>Multiple Resources: 3416 3417* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3418* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3419* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3420* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3421* [Citation](citation.html): The current status of the citation 3422* [CodeSystem](codesystem.html): The current status of the code system 3423* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3424* [ConceptMap](conceptmap.html): The current status of the concept map 3425* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3426* [EventDefinition](eventdefinition.html): The current status of the event definition 3427* [Evidence](evidence.html): The current status of the evidence 3428* [EvidenceReport](evidencereport.html): The current status of the evidence report 3429* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3430* [ExampleScenario](examplescenario.html): The current status of the example scenario 3431* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3432* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3433* [Library](library.html): The current status of the library 3434* [Measure](measure.html): The current status of the measure 3435* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3436* [MessageDefinition](messagedefinition.html): The current status of the message definition 3437* [NamingSystem](namingsystem.html): The current status of the naming system 3438* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3439* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3440* [PlanDefinition](plandefinition.html): The current status of the plan definition 3441* [Questionnaire](questionnaire.html): The current status of the questionnaire 3442* [Requirements](requirements.html): The current status of the requirements 3443* [SearchParameter](searchparameter.html): The current status of the search parameter 3444* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3445* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3446* [StructureMap](structuremap.html): The current status of the structure map 3447* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3448* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3449* [TestPlan](testplan.html): The current status of the test plan 3450* [TestScript](testscript.html): The current status of the test script 3451* [ValueSet](valueset.html): The current status of the value set 3452</b><br> 3453 * Type: <b>token</b><br> 3454 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3455 * </p> 3456 */ 3457 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3458 3459 /** 3460 * Search parameter: <b>title</b> 3461 * <p> 3462 * Description: <b>Multiple Resources: 3463 3464* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3465* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3466* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3467* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3468* [Citation](citation.html): The human-friendly name of the citation 3469* [CodeSystem](codesystem.html): The human-friendly name of the code system 3470* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3471* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3472* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3473* [Evidence](evidence.html): The human-friendly name of the evidence 3474* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3475* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3476* [Library](library.html): The human-friendly name of the library 3477* [Measure](measure.html): The human-friendly name of the measure 3478* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3479* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3480* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3481* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3482* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3483* [Requirements](requirements.html): The human-friendly name of the requirements 3484* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3485* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3486* [StructureMap](structuremap.html): The human-friendly name of the structure map 3487* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3488* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3489* [TestScript](testscript.html): The human-friendly name of the test script 3490* [ValueSet](valueset.html): The human-friendly name of the value set 3491</b><br> 3492 * Type: <b>string</b><br> 3493 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3494 * </p> 3495 */ 3496 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 3497 public static final String SP_TITLE = "title"; 3498 /** 3499 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3500 * <p> 3501 * Description: <b>Multiple Resources: 3502 3503* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3504* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3505* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3506* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3507* [Citation](citation.html): The human-friendly name of the citation 3508* [CodeSystem](codesystem.html): The human-friendly name of the code system 3509* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3510* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3511* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3512* [Evidence](evidence.html): The human-friendly name of the evidence 3513* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3514* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3515* [Library](library.html): The human-friendly name of the library 3516* [Measure](measure.html): The human-friendly name of the measure 3517* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3518* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3519* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3520* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3521* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3522* [Requirements](requirements.html): The human-friendly name of the requirements 3523* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3524* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3525* [StructureMap](structuremap.html): The human-friendly name of the structure map 3526* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3527* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3528* [TestScript](testscript.html): The human-friendly name of the test script 3529* [ValueSet](valueset.html): The human-friendly name of the value set 3530</b><br> 3531 * Type: <b>string</b><br> 3532 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3533 * </p> 3534 */ 3535 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3536 3537 /** 3538 * Search parameter: <b>url</b> 3539 * <p> 3540 * Description: <b>Multiple Resources: 3541 3542* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3543* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3544* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3545* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3546* [Citation](citation.html): The uri that identifies the citation 3547* [CodeSystem](codesystem.html): The uri that identifies the code system 3548* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3549* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3550* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3551* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3552* [Evidence](evidence.html): The uri that identifies the evidence 3553* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3554* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3555* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3556* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3557* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3558* [Library](library.html): The uri that identifies the library 3559* [Measure](measure.html): The uri that identifies the measure 3560* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3561* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3562* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3563* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3564* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3565* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3566* [Requirements](requirements.html): The uri that identifies the requirements 3567* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3568* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3569* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3570* [StructureMap](structuremap.html): The uri that identifies the structure map 3571* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3572* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3573* [TestPlan](testplan.html): The uri that identifies the test plan 3574* [TestScript](testscript.html): The uri that identifies the test script 3575* [ValueSet](valueset.html): The uri that identifies the value set 3576</b><br> 3577 * Type: <b>uri</b><br> 3578 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3579 * </p> 3580 */ 3581 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3582 public static final String SP_URL = "url"; 3583 /** 3584 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3585 * <p> 3586 * Description: <b>Multiple Resources: 3587 3588* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3589* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3590* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3591* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3592* [Citation](citation.html): The uri that identifies the citation 3593* [CodeSystem](codesystem.html): The uri that identifies the code system 3594* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3595* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3596* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3597* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3598* [Evidence](evidence.html): The uri that identifies the evidence 3599* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3600* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3601* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3602* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3603* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3604* [Library](library.html): The uri that identifies the library 3605* [Measure](measure.html): The uri that identifies the measure 3606* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3607* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3608* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3609* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3610* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3611* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3612* [Requirements](requirements.html): The uri that identifies the requirements 3613* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3614* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3615* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3616* [StructureMap](structuremap.html): The uri that identifies the structure map 3617* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3618* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3619* [TestPlan](testplan.html): The uri that identifies the test plan 3620* [TestScript](testscript.html): The uri that identifies the test script 3621* [ValueSet](valueset.html): The uri that identifies the value set 3622</b><br> 3623 * Type: <b>uri</b><br> 3624 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3625 * </p> 3626 */ 3627 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3628 3629 /** 3630 * Search parameter: <b>version</b> 3631 * <p> 3632 * Description: <b>Multiple Resources: 3633 3634* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3635* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3636* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3637* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3638* [Citation](citation.html): The business version of the citation 3639* [CodeSystem](codesystem.html): The business version of the code system 3640* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3641* [ConceptMap](conceptmap.html): The business version of the concept map 3642* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3643* [EventDefinition](eventdefinition.html): The business version of the event definition 3644* [Evidence](evidence.html): The business version of the evidence 3645* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3646* [ExampleScenario](examplescenario.html): The business version of the example scenario 3647* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3648* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3649* [Library](library.html): The business version of the library 3650* [Measure](measure.html): The business version of the measure 3651* [MessageDefinition](messagedefinition.html): The business version of the message definition 3652* [NamingSystem](namingsystem.html): The business version of the naming system 3653* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3654* [PlanDefinition](plandefinition.html): The business version of the plan definition 3655* [Questionnaire](questionnaire.html): The business version of the questionnaire 3656* [Requirements](requirements.html): The business version of the requirements 3657* [SearchParameter](searchparameter.html): The business version of the search parameter 3658* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3659* [StructureMap](structuremap.html): The business version of the structure map 3660* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3661* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3662* [TestScript](testscript.html): The business version of the test script 3663* [ValueSet](valueset.html): The business version of the value set 3664</b><br> 3665 * Type: <b>token</b><br> 3666 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3667 * </p> 3668 */ 3669 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 3670 public static final String SP_VERSION = "version"; 3671 /** 3672 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3673 * <p> 3674 * Description: <b>Multiple Resources: 3675 3676* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3677* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3678* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3679* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3680* [Citation](citation.html): The business version of the citation 3681* [CodeSystem](codesystem.html): The business version of the code system 3682* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3683* [ConceptMap](conceptmap.html): The business version of the concept map 3684* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3685* [EventDefinition](eventdefinition.html): The business version of the event definition 3686* [Evidence](evidence.html): The business version of the evidence 3687* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3688* [ExampleScenario](examplescenario.html): The business version of the example scenario 3689* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3690* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3691* [Library](library.html): The business version of the library 3692* [Measure](measure.html): The business version of the measure 3693* [MessageDefinition](messagedefinition.html): The business version of the message definition 3694* [NamingSystem](namingsystem.html): The business version of the naming system 3695* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3696* [PlanDefinition](plandefinition.html): The business version of the plan definition 3697* [Questionnaire](questionnaire.html): The business version of the questionnaire 3698* [Requirements](requirements.html): The business version of the requirements 3699* [SearchParameter](searchparameter.html): The business version of the search parameter 3700* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3701* [StructureMap](structuremap.html): The business version of the structure map 3702* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3703* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3704* [TestScript](testscript.html): The business version of the test script 3705* [ValueSet](valueset.html): The business version of the value set 3706</b><br> 3707 * Type: <b>token</b><br> 3708 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3709 * </p> 3710 */ 3711 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3712 3713 /** 3714 * Search parameter: <b>composed-of</b> 3715 * <p> 3716 * Description: <b>Multiple Resources: 3717 3718* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3719* [EventDefinition](eventdefinition.html): What resource is being referenced 3720* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3721* [Library](library.html): What resource is being referenced 3722* [Measure](measure.html): What resource is being referenced 3723* [PlanDefinition](plandefinition.html): What resource is being referenced 3724</b><br> 3725 * Type: <b>reference</b><br> 3726 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 3727 * </p> 3728 */ 3729 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3730 public static final String SP_COMPOSED_OF = "composed-of"; 3731 /** 3732 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 3733 * <p> 3734 * Description: <b>Multiple Resources: 3735 3736* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3737* [EventDefinition](eventdefinition.html): What resource is being referenced 3738* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3739* [Library](library.html): What resource is being referenced 3740* [Measure](measure.html): What resource is being referenced 3741* [PlanDefinition](plandefinition.html): What resource is being referenced 3742</b><br> 3743 * Type: <b>reference</b><br> 3744 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 3745 * </p> 3746 */ 3747 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 3748 3749/** 3750 * Constant for fluent queries to be used to add include statements. Specifies 3751 * the path value of "<b>EventDefinition:composed-of</b>". 3752 */ 3753 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("EventDefinition:composed-of").toLocked(); 3754 3755 /** 3756 * Search parameter: <b>depends-on</b> 3757 * <p> 3758 * Description: <b>Multiple Resources: 3759 3760* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3761* [EventDefinition](eventdefinition.html): What resource is being referenced 3762* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3763* [Library](library.html): What resource is being referenced 3764* [Measure](measure.html): What resource is being referenced 3765* [PlanDefinition](plandefinition.html): What resource is being referenced 3766</b><br> 3767 * Type: <b>reference</b><br> 3768 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 3769 * </p> 3770 */ 3771 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3772 public static final String SP_DEPENDS_ON = "depends-on"; 3773 /** 3774 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 3775 * <p> 3776 * Description: <b>Multiple Resources: 3777 3778* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3779* [EventDefinition](eventdefinition.html): What resource is being referenced 3780* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3781* [Library](library.html): What resource is being referenced 3782* [Measure](measure.html): What resource is being referenced 3783* [PlanDefinition](plandefinition.html): What resource is being referenced 3784</b><br> 3785 * Type: <b>reference</b><br> 3786 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 3787 * </p> 3788 */ 3789 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 3790 3791/** 3792 * Constant for fluent queries to be used to add include statements. Specifies 3793 * the path value of "<b>EventDefinition:depends-on</b>". 3794 */ 3795 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("EventDefinition:depends-on").toLocked(); 3796 3797 /** 3798 * Search parameter: <b>derived-from</b> 3799 * <p> 3800 * Description: <b>Multiple Resources: 3801 3802* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3803* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 3804* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 3805* [EventDefinition](eventdefinition.html): What resource is being referenced 3806* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3807* [Library](library.html): What resource is being referenced 3808* [Measure](measure.html): What resource is being referenced 3809* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 3810* [PlanDefinition](plandefinition.html): What resource is being referenced 3811* [ValueSet](valueset.html): A resource that the ValueSet is derived from 3812</b><br> 3813 * Type: <b>reference</b><br> 3814 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 3815 * </p> 3816 */ 3817 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3818 public static final String SP_DERIVED_FROM = "derived-from"; 3819 /** 3820 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 3821 * <p> 3822 * Description: <b>Multiple Resources: 3823 3824* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3825* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 3826* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 3827* [EventDefinition](eventdefinition.html): What resource is being referenced 3828* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3829* [Library](library.html): What resource is being referenced 3830* [Measure](measure.html): What resource is being referenced 3831* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 3832* [PlanDefinition](plandefinition.html): What resource is being referenced 3833* [ValueSet](valueset.html): A resource that the ValueSet is derived from 3834</b><br> 3835 * Type: <b>reference</b><br> 3836 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 3837 * </p> 3838 */ 3839 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 3840 3841/** 3842 * Constant for fluent queries to be used to add include statements. Specifies 3843 * the path value of "<b>EventDefinition:derived-from</b>". 3844 */ 3845 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("EventDefinition:derived-from").toLocked(); 3846 3847 /** 3848 * Search parameter: <b>effective</b> 3849 * <p> 3850 * Description: <b>Multiple Resources: 3851 3852* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 3853* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 3854* [Citation](citation.html): The time during which the citation is intended to be in use 3855* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 3856* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 3857* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 3858* [Library](library.html): The time during which the library is intended to be in use 3859* [Measure](measure.html): The time during which the measure is intended to be in use 3860* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 3861* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 3862* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 3863* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 3864</b><br> 3865 * Type: <b>date</b><br> 3866 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 3867 * </p> 3868 */ 3869 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 3870 public static final String SP_EFFECTIVE = "effective"; 3871 /** 3872 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 3873 * <p> 3874 * Description: <b>Multiple Resources: 3875 3876* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 3877* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 3878* [Citation](citation.html): The time during which the citation is intended to be in use 3879* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 3880* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 3881* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 3882* [Library](library.html): The time during which the library is intended to be in use 3883* [Measure](measure.html): The time during which the measure is intended to be in use 3884* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 3885* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 3886* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 3887* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 3888</b><br> 3889 * Type: <b>date</b><br> 3890 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 3891 * </p> 3892 */ 3893 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 3894 3895 /** 3896 * Search parameter: <b>predecessor</b> 3897 * <p> 3898 * Description: <b>Multiple Resources: 3899 3900* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3901* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 3902* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 3903* [EventDefinition](eventdefinition.html): What resource is being referenced 3904* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3905* [Library](library.html): What resource is being referenced 3906* [Measure](measure.html): What resource is being referenced 3907* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 3908* [PlanDefinition](plandefinition.html): What resource is being referenced 3909* [ValueSet](valueset.html): The predecessor of the ValueSet 3910</b><br> 3911 * Type: <b>reference</b><br> 3912 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 3913 * </p> 3914 */ 3915 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3916 public static final String SP_PREDECESSOR = "predecessor"; 3917 /** 3918 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 3919 * <p> 3920 * Description: <b>Multiple Resources: 3921 3922* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3923* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 3924* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 3925* [EventDefinition](eventdefinition.html): What resource is being referenced 3926* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3927* [Library](library.html): What resource is being referenced 3928* [Measure](measure.html): What resource is being referenced 3929* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 3930* [PlanDefinition](plandefinition.html): What resource is being referenced 3931* [ValueSet](valueset.html): The predecessor of the ValueSet 3932</b><br> 3933 * Type: <b>reference</b><br> 3934 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 3935 * </p> 3936 */ 3937 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 3938 3939/** 3940 * Constant for fluent queries to be used to add include statements. Specifies 3941 * the path value of "<b>EventDefinition:predecessor</b>". 3942 */ 3943 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("EventDefinition:predecessor").toLocked(); 3944 3945 /** 3946 * Search parameter: <b>successor</b> 3947 * <p> 3948 * Description: <b>Multiple Resources: 3949 3950* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3951* [EventDefinition](eventdefinition.html): What resource is being referenced 3952* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3953* [Library](library.html): What resource is being referenced 3954* [Measure](measure.html): What resource is being referenced 3955* [PlanDefinition](plandefinition.html): What resource is being referenced 3956</b><br> 3957 * Type: <b>reference</b><br> 3958 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 3959 * </p> 3960 */ 3961 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3962 public static final String SP_SUCCESSOR = "successor"; 3963 /** 3964 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 3965 * <p> 3966 * Description: <b>Multiple Resources: 3967 3968* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3969* [EventDefinition](eventdefinition.html): What resource is being referenced 3970* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3971* [Library](library.html): What resource is being referenced 3972* [Measure](measure.html): What resource is being referenced 3973* [PlanDefinition](plandefinition.html): What resource is being referenced 3974</b><br> 3975 * Type: <b>reference</b><br> 3976 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 3977 * </p> 3978 */ 3979 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 3980 3981/** 3982 * Constant for fluent queries to be used to add include statements. Specifies 3983 * the path value of "<b>EventDefinition:successor</b>". 3984 */ 3985 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("EventDefinition:successor").toLocked(); 3986 3987 /** 3988 * Search parameter: <b>topic</b> 3989 * <p> 3990 * Description: <b>Multiple Resources: 3991 3992* [ActivityDefinition](activitydefinition.html): Topics associated with the module 3993* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 3994* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 3995* [EventDefinition](eventdefinition.html): Topics associated with the module 3996* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 3997* [Library](library.html): Topics associated with the module 3998* [Measure](measure.html): Topics associated with the measure 3999* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4000* [PlanDefinition](plandefinition.html): Topics associated with the module 4001* [ValueSet](valueset.html): Topics associated with the ValueSet 4002</b><br> 4003 * Type: <b>token</b><br> 4004 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4005 * </p> 4006 */ 4007 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 4008 public static final String SP_TOPIC = "topic"; 4009 /** 4010 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 4011 * <p> 4012 * Description: <b>Multiple Resources: 4013 4014* [ActivityDefinition](activitydefinition.html): Topics associated with the module 4015* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 4016* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 4017* [EventDefinition](eventdefinition.html): Topics associated with the module 4018* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 4019* [Library](library.html): Topics associated with the module 4020* [Measure](measure.html): Topics associated with the measure 4021* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4022* [PlanDefinition](plandefinition.html): Topics associated with the module 4023* [ValueSet](valueset.html): Topics associated with the ValueSet 4024</b><br> 4025 * Type: <b>token</b><br> 4026 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4027 * </p> 4028 */ 4029 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 4030 4031 4032} 4033