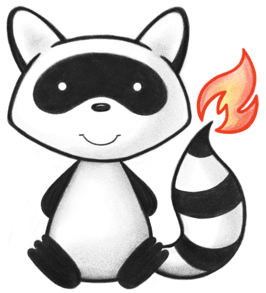
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * The Evidence Resource provides a machine-interpretable expression of an evidence concept including the evidence variables (e.g., population, exposures/interventions, comparators, outcomes, measured variables, confounding variables), the statistics, and the certainty of this evidence. 053 */ 054@ResourceDef(name="Evidence", profile="http://hl7.org/fhir/StructureDefinition/Evidence") 055public class Evidence extends MetadataResource { 056 057 @Block() 058 public static class EvidenceVariableDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * A text description or summary of the variable. 061 */ 062 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 063 @Description(shortDefinition="A text description or summary of the variable", formalDefinition="A text description or summary of the variable." ) 064 protected MarkdownType description; 065 066 /** 067 * Footnotes and/or explanatory notes. 068 */ 069 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 070 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Footnotes and/or explanatory notes." ) 071 protected List<Annotation> note; 072 073 /** 074 * population | subpopulation | exposure | referenceExposure | measuredVariable | confounder. 075 */ 076 @Child(name = "variableRole", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 077 @Description(shortDefinition="population | subpopulation | exposure | referenceExposure | measuredVariable | confounder", formalDefinition="population | subpopulation | exposure | referenceExposure | measuredVariable | confounder." ) 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/variable-role") 079 protected CodeableConcept variableRole; 080 081 /** 082 * Definition of the actual variable related to the statistic(s). 083 */ 084 @Child(name = "observed", type = {Group.class, EvidenceVariable.class}, order=4, min=0, max=1, modifier=false, summary=true) 085 @Description(shortDefinition="Definition of the actual variable related to the statistic(s)", formalDefinition="Definition of the actual variable related to the statistic(s)." ) 086 protected Reference observed; 087 088 /** 089 * Definition of the intended variable related to the Evidence. 090 */ 091 @Child(name = "intended", type = {Group.class, EvidenceVariable.class}, order=5, min=0, max=1, modifier=false, summary=false) 092 @Description(shortDefinition="Definition of the intended variable related to the Evidence", formalDefinition="Definition of the intended variable related to the Evidence." ) 093 protected Reference intended; 094 095 /** 096 * Indication of quality of match between intended variable to actual variable. 097 */ 098 @Child(name = "directnessMatch", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 099 @Description(shortDefinition="low | moderate | high | exact", formalDefinition="Indication of quality of match between intended variable to actual variable." ) 100 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/directness") 101 protected CodeableConcept directnessMatch; 102 103 private static final long serialVersionUID = -702346164L; 104 105 /** 106 * Constructor 107 */ 108 public EvidenceVariableDefinitionComponent() { 109 super(); 110 } 111 112 /** 113 * Constructor 114 */ 115 public EvidenceVariableDefinitionComponent(CodeableConcept variableRole) { 116 super(); 117 this.setVariableRole(variableRole); 118 } 119 120 /** 121 * @return {@link #description} (A text description or summary of the variable.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 122 */ 123 public MarkdownType getDescriptionElement() { 124 if (this.description == null) 125 if (Configuration.errorOnAutoCreate()) 126 throw new Error("Attempt to auto-create EvidenceVariableDefinitionComponent.description"); 127 else if (Configuration.doAutoCreate()) 128 this.description = new MarkdownType(); // bb 129 return this.description; 130 } 131 132 public boolean hasDescriptionElement() { 133 return this.description != null && !this.description.isEmpty(); 134 } 135 136 public boolean hasDescription() { 137 return this.description != null && !this.description.isEmpty(); 138 } 139 140 /** 141 * @param value {@link #description} (A text description or summary of the variable.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 142 */ 143 public EvidenceVariableDefinitionComponent setDescriptionElement(MarkdownType value) { 144 this.description = value; 145 return this; 146 } 147 148 /** 149 * @return A text description or summary of the variable. 150 */ 151 public String getDescription() { 152 return this.description == null ? null : this.description.getValue(); 153 } 154 155 /** 156 * @param value A text description or summary of the variable. 157 */ 158 public EvidenceVariableDefinitionComponent setDescription(String value) { 159 if (Utilities.noString(value)) 160 this.description = null; 161 else { 162 if (this.description == null) 163 this.description = new MarkdownType(); 164 this.description.setValue(value); 165 } 166 return this; 167 } 168 169 /** 170 * @return {@link #note} (Footnotes and/or explanatory notes.) 171 */ 172 public List<Annotation> getNote() { 173 if (this.note == null) 174 this.note = new ArrayList<Annotation>(); 175 return this.note; 176 } 177 178 /** 179 * @return Returns a reference to <code>this</code> for easy method chaining 180 */ 181 public EvidenceVariableDefinitionComponent setNote(List<Annotation> theNote) { 182 this.note = theNote; 183 return this; 184 } 185 186 public boolean hasNote() { 187 if (this.note == null) 188 return false; 189 for (Annotation item : this.note) 190 if (!item.isEmpty()) 191 return true; 192 return false; 193 } 194 195 public Annotation addNote() { //3 196 Annotation t = new Annotation(); 197 if (this.note == null) 198 this.note = new ArrayList<Annotation>(); 199 this.note.add(t); 200 return t; 201 } 202 203 public EvidenceVariableDefinitionComponent addNote(Annotation t) { //3 204 if (t == null) 205 return this; 206 if (this.note == null) 207 this.note = new ArrayList<Annotation>(); 208 this.note.add(t); 209 return this; 210 } 211 212 /** 213 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 214 */ 215 public Annotation getNoteFirstRep() { 216 if (getNote().isEmpty()) { 217 addNote(); 218 } 219 return getNote().get(0); 220 } 221 222 /** 223 * @return {@link #variableRole} (population | subpopulation | exposure | referenceExposure | measuredVariable | confounder.) 224 */ 225 public CodeableConcept getVariableRole() { 226 if (this.variableRole == null) 227 if (Configuration.errorOnAutoCreate()) 228 throw new Error("Attempt to auto-create EvidenceVariableDefinitionComponent.variableRole"); 229 else if (Configuration.doAutoCreate()) 230 this.variableRole = new CodeableConcept(); // cc 231 return this.variableRole; 232 } 233 234 public boolean hasVariableRole() { 235 return this.variableRole != null && !this.variableRole.isEmpty(); 236 } 237 238 /** 239 * @param value {@link #variableRole} (population | subpopulation | exposure | referenceExposure | measuredVariable | confounder.) 240 */ 241 public EvidenceVariableDefinitionComponent setVariableRole(CodeableConcept value) { 242 this.variableRole = value; 243 return this; 244 } 245 246 /** 247 * @return {@link #observed} (Definition of the actual variable related to the statistic(s).) 248 */ 249 public Reference getObserved() { 250 if (this.observed == null) 251 if (Configuration.errorOnAutoCreate()) 252 throw new Error("Attempt to auto-create EvidenceVariableDefinitionComponent.observed"); 253 else if (Configuration.doAutoCreate()) 254 this.observed = new Reference(); // cc 255 return this.observed; 256 } 257 258 public boolean hasObserved() { 259 return this.observed != null && !this.observed.isEmpty(); 260 } 261 262 /** 263 * @param value {@link #observed} (Definition of the actual variable related to the statistic(s).) 264 */ 265 public EvidenceVariableDefinitionComponent setObserved(Reference value) { 266 this.observed = value; 267 return this; 268 } 269 270 /** 271 * @return {@link #intended} (Definition of the intended variable related to the Evidence.) 272 */ 273 public Reference getIntended() { 274 if (this.intended == null) 275 if (Configuration.errorOnAutoCreate()) 276 throw new Error("Attempt to auto-create EvidenceVariableDefinitionComponent.intended"); 277 else if (Configuration.doAutoCreate()) 278 this.intended = new Reference(); // cc 279 return this.intended; 280 } 281 282 public boolean hasIntended() { 283 return this.intended != null && !this.intended.isEmpty(); 284 } 285 286 /** 287 * @param value {@link #intended} (Definition of the intended variable related to the Evidence.) 288 */ 289 public EvidenceVariableDefinitionComponent setIntended(Reference value) { 290 this.intended = value; 291 return this; 292 } 293 294 /** 295 * @return {@link #directnessMatch} (Indication of quality of match between intended variable to actual variable.) 296 */ 297 public CodeableConcept getDirectnessMatch() { 298 if (this.directnessMatch == null) 299 if (Configuration.errorOnAutoCreate()) 300 throw new Error("Attempt to auto-create EvidenceVariableDefinitionComponent.directnessMatch"); 301 else if (Configuration.doAutoCreate()) 302 this.directnessMatch = new CodeableConcept(); // cc 303 return this.directnessMatch; 304 } 305 306 public boolean hasDirectnessMatch() { 307 return this.directnessMatch != null && !this.directnessMatch.isEmpty(); 308 } 309 310 /** 311 * @param value {@link #directnessMatch} (Indication of quality of match between intended variable to actual variable.) 312 */ 313 public EvidenceVariableDefinitionComponent setDirectnessMatch(CodeableConcept value) { 314 this.directnessMatch = value; 315 return this; 316 } 317 318 protected void listChildren(List<Property> children) { 319 super.listChildren(children); 320 children.add(new Property("description", "markdown", "A text description or summary of the variable.", 0, 1, description)); 321 children.add(new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note)); 322 children.add(new Property("variableRole", "CodeableConcept", "population | subpopulation | exposure | referenceExposure | measuredVariable | confounder.", 0, 1, variableRole)); 323 children.add(new Property("observed", "Reference(Group|EvidenceVariable)", "Definition of the actual variable related to the statistic(s).", 0, 1, observed)); 324 children.add(new Property("intended", "Reference(Group|EvidenceVariable)", "Definition of the intended variable related to the Evidence.", 0, 1, intended)); 325 children.add(new Property("directnessMatch", "CodeableConcept", "Indication of quality of match between intended variable to actual variable.", 0, 1, directnessMatch)); 326 } 327 328 @Override 329 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 330 switch (_hash) { 331 case -1724546052: /*description*/ return new Property("description", "markdown", "A text description or summary of the variable.", 0, 1, description); 332 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note); 333 case -372889326: /*variableRole*/ return new Property("variableRole", "CodeableConcept", "population | subpopulation | exposure | referenceExposure | measuredVariable | confounder.", 0, 1, variableRole); 334 case 348607176: /*observed*/ return new Property("observed", "Reference(Group|EvidenceVariable)", "Definition of the actual variable related to the statistic(s).", 0, 1, observed); 335 case 570282027: /*intended*/ return new Property("intended", "Reference(Group|EvidenceVariable)", "Definition of the intended variable related to the Evidence.", 0, 1, intended); 336 case -2144864283: /*directnessMatch*/ return new Property("directnessMatch", "CodeableConcept", "Indication of quality of match between intended variable to actual variable.", 0, 1, directnessMatch); 337 default: return super.getNamedProperty(_hash, _name, _checkValid); 338 } 339 340 } 341 342 @Override 343 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 344 switch (hash) { 345 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 346 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 347 case -372889326: /*variableRole*/ return this.variableRole == null ? new Base[0] : new Base[] {this.variableRole}; // CodeableConcept 348 case 348607176: /*observed*/ return this.observed == null ? new Base[0] : new Base[] {this.observed}; // Reference 349 case 570282027: /*intended*/ return this.intended == null ? new Base[0] : new Base[] {this.intended}; // Reference 350 case -2144864283: /*directnessMatch*/ return this.directnessMatch == null ? new Base[0] : new Base[] {this.directnessMatch}; // CodeableConcept 351 default: return super.getProperty(hash, name, checkValid); 352 } 353 354 } 355 356 @Override 357 public Base setProperty(int hash, String name, Base value) throws FHIRException { 358 switch (hash) { 359 case -1724546052: // description 360 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 361 return value; 362 case 3387378: // note 363 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 364 return value; 365 case -372889326: // variableRole 366 this.variableRole = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 367 return value; 368 case 348607176: // observed 369 this.observed = TypeConvertor.castToReference(value); // Reference 370 return value; 371 case 570282027: // intended 372 this.intended = TypeConvertor.castToReference(value); // Reference 373 return value; 374 case -2144864283: // directnessMatch 375 this.directnessMatch = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 376 return value; 377 default: return super.setProperty(hash, name, value); 378 } 379 380 } 381 382 @Override 383 public Base setProperty(String name, Base value) throws FHIRException { 384 if (name.equals("description")) { 385 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 386 } else if (name.equals("note")) { 387 this.getNote().add(TypeConvertor.castToAnnotation(value)); 388 } else if (name.equals("variableRole")) { 389 this.variableRole = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 390 } else if (name.equals("observed")) { 391 this.observed = TypeConvertor.castToReference(value); // Reference 392 } else if (name.equals("intended")) { 393 this.intended = TypeConvertor.castToReference(value); // Reference 394 } else if (name.equals("directnessMatch")) { 395 this.directnessMatch = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 396 } else 397 return super.setProperty(name, value); 398 return value; 399 } 400 401 @Override 402 public void removeChild(String name, Base value) throws FHIRException { 403 if (name.equals("description")) { 404 this.description = null; 405 } else if (name.equals("note")) { 406 this.getNote().remove(value); 407 } else if (name.equals("variableRole")) { 408 this.variableRole = null; 409 } else if (name.equals("observed")) { 410 this.observed = null; 411 } else if (name.equals("intended")) { 412 this.intended = null; 413 } else if (name.equals("directnessMatch")) { 414 this.directnessMatch = null; 415 } else 416 super.removeChild(name, value); 417 418 } 419 420 @Override 421 public Base makeProperty(int hash, String name) throws FHIRException { 422 switch (hash) { 423 case -1724546052: return getDescriptionElement(); 424 case 3387378: return addNote(); 425 case -372889326: return getVariableRole(); 426 case 348607176: return getObserved(); 427 case 570282027: return getIntended(); 428 case -2144864283: return getDirectnessMatch(); 429 default: return super.makeProperty(hash, name); 430 } 431 432 } 433 434 @Override 435 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 436 switch (hash) { 437 case -1724546052: /*description*/ return new String[] {"markdown"}; 438 case 3387378: /*note*/ return new String[] {"Annotation"}; 439 case -372889326: /*variableRole*/ return new String[] {"CodeableConcept"}; 440 case 348607176: /*observed*/ return new String[] {"Reference"}; 441 case 570282027: /*intended*/ return new String[] {"Reference"}; 442 case -2144864283: /*directnessMatch*/ return new String[] {"CodeableConcept"}; 443 default: return super.getTypesForProperty(hash, name); 444 } 445 446 } 447 448 @Override 449 public Base addChild(String name) throws FHIRException { 450 if (name.equals("description")) { 451 throw new FHIRException("Cannot call addChild on a singleton property Evidence.variableDefinition.description"); 452 } 453 else if (name.equals("note")) { 454 return addNote(); 455 } 456 else if (name.equals("variableRole")) { 457 this.variableRole = new CodeableConcept(); 458 return this.variableRole; 459 } 460 else if (name.equals("observed")) { 461 this.observed = new Reference(); 462 return this.observed; 463 } 464 else if (name.equals("intended")) { 465 this.intended = new Reference(); 466 return this.intended; 467 } 468 else if (name.equals("directnessMatch")) { 469 this.directnessMatch = new CodeableConcept(); 470 return this.directnessMatch; 471 } 472 else 473 return super.addChild(name); 474 } 475 476 public EvidenceVariableDefinitionComponent copy() { 477 EvidenceVariableDefinitionComponent dst = new EvidenceVariableDefinitionComponent(); 478 copyValues(dst); 479 return dst; 480 } 481 482 public void copyValues(EvidenceVariableDefinitionComponent dst) { 483 super.copyValues(dst); 484 dst.description = description == null ? null : description.copy(); 485 if (note != null) { 486 dst.note = new ArrayList<Annotation>(); 487 for (Annotation i : note) 488 dst.note.add(i.copy()); 489 }; 490 dst.variableRole = variableRole == null ? null : variableRole.copy(); 491 dst.observed = observed == null ? null : observed.copy(); 492 dst.intended = intended == null ? null : intended.copy(); 493 dst.directnessMatch = directnessMatch == null ? null : directnessMatch.copy(); 494 } 495 496 @Override 497 public boolean equalsDeep(Base other_) { 498 if (!super.equalsDeep(other_)) 499 return false; 500 if (!(other_ instanceof EvidenceVariableDefinitionComponent)) 501 return false; 502 EvidenceVariableDefinitionComponent o = (EvidenceVariableDefinitionComponent) other_; 503 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(variableRole, o.variableRole, true) 504 && compareDeep(observed, o.observed, true) && compareDeep(intended, o.intended, true) && compareDeep(directnessMatch, o.directnessMatch, true) 505 ; 506 } 507 508 @Override 509 public boolean equalsShallow(Base other_) { 510 if (!super.equalsShallow(other_)) 511 return false; 512 if (!(other_ instanceof EvidenceVariableDefinitionComponent)) 513 return false; 514 EvidenceVariableDefinitionComponent o = (EvidenceVariableDefinitionComponent) other_; 515 return compareValues(description, o.description, true); 516 } 517 518 public boolean isEmpty() { 519 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, variableRole 520 , observed, intended, directnessMatch); 521 } 522 523 public String fhirType() { 524 return "Evidence.variableDefinition"; 525 526 } 527 528 } 529 530 @Block() 531 public static class EvidenceStatisticComponent extends BackboneElement implements IBaseBackboneElement { 532 /** 533 * A description of the content value of the statistic. 534 */ 535 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 536 @Description(shortDefinition="Description of content", formalDefinition="A description of the content value of the statistic." ) 537 protected MarkdownType description; 538 539 /** 540 * Footnotes and/or explanatory notes. 541 */ 542 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 543 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Footnotes and/or explanatory notes." ) 544 protected List<Annotation> note; 545 546 /** 547 * Type of statistic, e.g., relative risk. 548 */ 549 @Child(name = "statisticType", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 550 @Description(shortDefinition="Type of statistic, e.g., relative risk", formalDefinition="Type of statistic, e.g., relative risk." ) 551 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/statistic-type") 552 protected CodeableConcept statisticType; 553 554 /** 555 * When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting. 556 */ 557 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 558 @Description(shortDefinition="Associated category for categorical variable", formalDefinition="When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting." ) 559 protected CodeableConcept category; 560 561 /** 562 * Statistic value. 563 */ 564 @Child(name = "quantity", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 565 @Description(shortDefinition="Statistic value", formalDefinition="Statistic value." ) 566 protected Quantity quantity; 567 568 /** 569 * The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants. 570 */ 571 @Child(name = "numberOfEvents", type = {UnsignedIntType.class}, order=6, min=0, max=1, modifier=false, summary=false) 572 @Description(shortDefinition="The number of events associated with the statistic", formalDefinition="The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants." ) 573 protected UnsignedIntType numberOfEvents; 574 575 /** 576 * The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants. 577 */ 578 @Child(name = "numberAffected", type = {UnsignedIntType.class}, order=7, min=0, max=1, modifier=false, summary=false) 579 @Description(shortDefinition="The number of participants affected", formalDefinition="The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants." ) 580 protected UnsignedIntType numberAffected; 581 582 /** 583 * Number of samples in the statistic. 584 */ 585 @Child(name = "sampleSize", type = {}, order=8, min=0, max=1, modifier=false, summary=false) 586 @Description(shortDefinition="Number of samples in the statistic", formalDefinition="Number of samples in the statistic." ) 587 protected EvidenceStatisticSampleSizeComponent sampleSize; 588 589 /** 590 * A statistical attribute of the statistic such as a measure of heterogeneity. 591 */ 592 @Child(name = "attributeEstimate", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 593 @Description(shortDefinition="An attribute of the Statistic", formalDefinition="A statistical attribute of the statistic such as a measure of heterogeneity." ) 594 protected List<EvidenceStatisticAttributeEstimateComponent> attributeEstimate; 595 596 /** 597 * A component of the method to generate the statistic. 598 */ 599 @Child(name = "modelCharacteristic", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 600 @Description(shortDefinition="An aspect of the statistical model", formalDefinition="A component of the method to generate the statistic." ) 601 protected List<EvidenceStatisticModelCharacteristicComponent> modelCharacteristic; 602 603 private static final long serialVersionUID = 1798829622L; 604 605 /** 606 * Constructor 607 */ 608 public EvidenceStatisticComponent() { 609 super(); 610 } 611 612 /** 613 * @return {@link #description} (A description of the content value of the statistic.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 614 */ 615 public MarkdownType getDescriptionElement() { 616 if (this.description == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create EvidenceStatisticComponent.description"); 619 else if (Configuration.doAutoCreate()) 620 this.description = new MarkdownType(); // bb 621 return this.description; 622 } 623 624 public boolean hasDescriptionElement() { 625 return this.description != null && !this.description.isEmpty(); 626 } 627 628 public boolean hasDescription() { 629 return this.description != null && !this.description.isEmpty(); 630 } 631 632 /** 633 * @param value {@link #description} (A description of the content value of the statistic.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 634 */ 635 public EvidenceStatisticComponent setDescriptionElement(MarkdownType value) { 636 this.description = value; 637 return this; 638 } 639 640 /** 641 * @return A description of the content value of the statistic. 642 */ 643 public String getDescription() { 644 return this.description == null ? null : this.description.getValue(); 645 } 646 647 /** 648 * @param value A description of the content value of the statistic. 649 */ 650 public EvidenceStatisticComponent setDescription(String value) { 651 if (Utilities.noString(value)) 652 this.description = null; 653 else { 654 if (this.description == null) 655 this.description = new MarkdownType(); 656 this.description.setValue(value); 657 } 658 return this; 659 } 660 661 /** 662 * @return {@link #note} (Footnotes and/or explanatory notes.) 663 */ 664 public List<Annotation> getNote() { 665 if (this.note == null) 666 this.note = new ArrayList<Annotation>(); 667 return this.note; 668 } 669 670 /** 671 * @return Returns a reference to <code>this</code> for easy method chaining 672 */ 673 public EvidenceStatisticComponent setNote(List<Annotation> theNote) { 674 this.note = theNote; 675 return this; 676 } 677 678 public boolean hasNote() { 679 if (this.note == null) 680 return false; 681 for (Annotation item : this.note) 682 if (!item.isEmpty()) 683 return true; 684 return false; 685 } 686 687 public Annotation addNote() { //3 688 Annotation t = new Annotation(); 689 if (this.note == null) 690 this.note = new ArrayList<Annotation>(); 691 this.note.add(t); 692 return t; 693 } 694 695 public EvidenceStatisticComponent addNote(Annotation t) { //3 696 if (t == null) 697 return this; 698 if (this.note == null) 699 this.note = new ArrayList<Annotation>(); 700 this.note.add(t); 701 return this; 702 } 703 704 /** 705 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 706 */ 707 public Annotation getNoteFirstRep() { 708 if (getNote().isEmpty()) { 709 addNote(); 710 } 711 return getNote().get(0); 712 } 713 714 /** 715 * @return {@link #statisticType} (Type of statistic, e.g., relative risk.) 716 */ 717 public CodeableConcept getStatisticType() { 718 if (this.statisticType == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create EvidenceStatisticComponent.statisticType"); 721 else if (Configuration.doAutoCreate()) 722 this.statisticType = new CodeableConcept(); // cc 723 return this.statisticType; 724 } 725 726 public boolean hasStatisticType() { 727 return this.statisticType != null && !this.statisticType.isEmpty(); 728 } 729 730 /** 731 * @param value {@link #statisticType} (Type of statistic, e.g., relative risk.) 732 */ 733 public EvidenceStatisticComponent setStatisticType(CodeableConcept value) { 734 this.statisticType = value; 735 return this; 736 } 737 738 /** 739 * @return {@link #category} (When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.) 740 */ 741 public CodeableConcept getCategory() { 742 if (this.category == null) 743 if (Configuration.errorOnAutoCreate()) 744 throw new Error("Attempt to auto-create EvidenceStatisticComponent.category"); 745 else if (Configuration.doAutoCreate()) 746 this.category = new CodeableConcept(); // cc 747 return this.category; 748 } 749 750 public boolean hasCategory() { 751 return this.category != null && !this.category.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #category} (When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.) 756 */ 757 public EvidenceStatisticComponent setCategory(CodeableConcept value) { 758 this.category = value; 759 return this; 760 } 761 762 /** 763 * @return {@link #quantity} (Statistic value.) 764 */ 765 public Quantity getQuantity() { 766 if (this.quantity == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create EvidenceStatisticComponent.quantity"); 769 else if (Configuration.doAutoCreate()) 770 this.quantity = new Quantity(); // cc 771 return this.quantity; 772 } 773 774 public boolean hasQuantity() { 775 return this.quantity != null && !this.quantity.isEmpty(); 776 } 777 778 /** 779 * @param value {@link #quantity} (Statistic value.) 780 */ 781 public EvidenceStatisticComponent setQuantity(Quantity value) { 782 this.quantity = value; 783 return this; 784 } 785 786 /** 787 * @return {@link #numberOfEvents} (The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberOfEvents" gives direct access to the value 788 */ 789 public UnsignedIntType getNumberOfEventsElement() { 790 if (this.numberOfEvents == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create EvidenceStatisticComponent.numberOfEvents"); 793 else if (Configuration.doAutoCreate()) 794 this.numberOfEvents = new UnsignedIntType(); // bb 795 return this.numberOfEvents; 796 } 797 798 public boolean hasNumberOfEventsElement() { 799 return this.numberOfEvents != null && !this.numberOfEvents.isEmpty(); 800 } 801 802 public boolean hasNumberOfEvents() { 803 return this.numberOfEvents != null && !this.numberOfEvents.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #numberOfEvents} (The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberOfEvents" gives direct access to the value 808 */ 809 public EvidenceStatisticComponent setNumberOfEventsElement(UnsignedIntType value) { 810 this.numberOfEvents = value; 811 return this; 812 } 813 814 /** 815 * @return The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants. 816 */ 817 public int getNumberOfEvents() { 818 return this.numberOfEvents == null || this.numberOfEvents.isEmpty() ? 0 : this.numberOfEvents.getValue(); 819 } 820 821 /** 822 * @param value The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants. 823 */ 824 public EvidenceStatisticComponent setNumberOfEvents(int value) { 825 if (this.numberOfEvents == null) 826 this.numberOfEvents = new UnsignedIntType(); 827 this.numberOfEvents.setValue(value); 828 return this; 829 } 830 831 /** 832 * @return {@link #numberAffected} (The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberAffected" gives direct access to the value 833 */ 834 public UnsignedIntType getNumberAffectedElement() { 835 if (this.numberAffected == null) 836 if (Configuration.errorOnAutoCreate()) 837 throw new Error("Attempt to auto-create EvidenceStatisticComponent.numberAffected"); 838 else if (Configuration.doAutoCreate()) 839 this.numberAffected = new UnsignedIntType(); // bb 840 return this.numberAffected; 841 } 842 843 public boolean hasNumberAffectedElement() { 844 return this.numberAffected != null && !this.numberAffected.isEmpty(); 845 } 846 847 public boolean hasNumberAffected() { 848 return this.numberAffected != null && !this.numberAffected.isEmpty(); 849 } 850 851 /** 852 * @param value {@link #numberAffected} (The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberAffected" gives direct access to the value 853 */ 854 public EvidenceStatisticComponent setNumberAffectedElement(UnsignedIntType value) { 855 this.numberAffected = value; 856 return this; 857 } 858 859 /** 860 * @return The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants. 861 */ 862 public int getNumberAffected() { 863 return this.numberAffected == null || this.numberAffected.isEmpty() ? 0 : this.numberAffected.getValue(); 864 } 865 866 /** 867 * @param value The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants. 868 */ 869 public EvidenceStatisticComponent setNumberAffected(int value) { 870 if (this.numberAffected == null) 871 this.numberAffected = new UnsignedIntType(); 872 this.numberAffected.setValue(value); 873 return this; 874 } 875 876 /** 877 * @return {@link #sampleSize} (Number of samples in the statistic.) 878 */ 879 public EvidenceStatisticSampleSizeComponent getSampleSize() { 880 if (this.sampleSize == null) 881 if (Configuration.errorOnAutoCreate()) 882 throw new Error("Attempt to auto-create EvidenceStatisticComponent.sampleSize"); 883 else if (Configuration.doAutoCreate()) 884 this.sampleSize = new EvidenceStatisticSampleSizeComponent(); // cc 885 return this.sampleSize; 886 } 887 888 public boolean hasSampleSize() { 889 return this.sampleSize != null && !this.sampleSize.isEmpty(); 890 } 891 892 /** 893 * @param value {@link #sampleSize} (Number of samples in the statistic.) 894 */ 895 public EvidenceStatisticComponent setSampleSize(EvidenceStatisticSampleSizeComponent value) { 896 this.sampleSize = value; 897 return this; 898 } 899 900 /** 901 * @return {@link #attributeEstimate} (A statistical attribute of the statistic such as a measure of heterogeneity.) 902 */ 903 public List<EvidenceStatisticAttributeEstimateComponent> getAttributeEstimate() { 904 if (this.attributeEstimate == null) 905 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 906 return this.attributeEstimate; 907 } 908 909 /** 910 * @return Returns a reference to <code>this</code> for easy method chaining 911 */ 912 public EvidenceStatisticComponent setAttributeEstimate(List<EvidenceStatisticAttributeEstimateComponent> theAttributeEstimate) { 913 this.attributeEstimate = theAttributeEstimate; 914 return this; 915 } 916 917 public boolean hasAttributeEstimate() { 918 if (this.attributeEstimate == null) 919 return false; 920 for (EvidenceStatisticAttributeEstimateComponent item : this.attributeEstimate) 921 if (!item.isEmpty()) 922 return true; 923 return false; 924 } 925 926 public EvidenceStatisticAttributeEstimateComponent addAttributeEstimate() { //3 927 EvidenceStatisticAttributeEstimateComponent t = new EvidenceStatisticAttributeEstimateComponent(); 928 if (this.attributeEstimate == null) 929 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 930 this.attributeEstimate.add(t); 931 return t; 932 } 933 934 public EvidenceStatisticComponent addAttributeEstimate(EvidenceStatisticAttributeEstimateComponent t) { //3 935 if (t == null) 936 return this; 937 if (this.attributeEstimate == null) 938 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 939 this.attributeEstimate.add(t); 940 return this; 941 } 942 943 /** 944 * @return The first repetition of repeating field {@link #attributeEstimate}, creating it if it does not already exist {3} 945 */ 946 public EvidenceStatisticAttributeEstimateComponent getAttributeEstimateFirstRep() { 947 if (getAttributeEstimate().isEmpty()) { 948 addAttributeEstimate(); 949 } 950 return getAttributeEstimate().get(0); 951 } 952 953 /** 954 * @return {@link #modelCharacteristic} (A component of the method to generate the statistic.) 955 */ 956 public List<EvidenceStatisticModelCharacteristicComponent> getModelCharacteristic() { 957 if (this.modelCharacteristic == null) 958 this.modelCharacteristic = new ArrayList<EvidenceStatisticModelCharacteristicComponent>(); 959 return this.modelCharacteristic; 960 } 961 962 /** 963 * @return Returns a reference to <code>this</code> for easy method chaining 964 */ 965 public EvidenceStatisticComponent setModelCharacteristic(List<EvidenceStatisticModelCharacteristicComponent> theModelCharacteristic) { 966 this.modelCharacteristic = theModelCharacteristic; 967 return this; 968 } 969 970 public boolean hasModelCharacteristic() { 971 if (this.modelCharacteristic == null) 972 return false; 973 for (EvidenceStatisticModelCharacteristicComponent item : this.modelCharacteristic) 974 if (!item.isEmpty()) 975 return true; 976 return false; 977 } 978 979 public EvidenceStatisticModelCharacteristicComponent addModelCharacteristic() { //3 980 EvidenceStatisticModelCharacteristicComponent t = new EvidenceStatisticModelCharacteristicComponent(); 981 if (this.modelCharacteristic == null) 982 this.modelCharacteristic = new ArrayList<EvidenceStatisticModelCharacteristicComponent>(); 983 this.modelCharacteristic.add(t); 984 return t; 985 } 986 987 public EvidenceStatisticComponent addModelCharacteristic(EvidenceStatisticModelCharacteristicComponent t) { //3 988 if (t == null) 989 return this; 990 if (this.modelCharacteristic == null) 991 this.modelCharacteristic = new ArrayList<EvidenceStatisticModelCharacteristicComponent>(); 992 this.modelCharacteristic.add(t); 993 return this; 994 } 995 996 /** 997 * @return The first repetition of repeating field {@link #modelCharacteristic}, creating it if it does not already exist {3} 998 */ 999 public EvidenceStatisticModelCharacteristicComponent getModelCharacteristicFirstRep() { 1000 if (getModelCharacteristic().isEmpty()) { 1001 addModelCharacteristic(); 1002 } 1003 return getModelCharacteristic().get(0); 1004 } 1005 1006 protected void listChildren(List<Property> children) { 1007 super.listChildren(children); 1008 children.add(new Property("description", "markdown", "A description of the content value of the statistic.", 0, 1, description)); 1009 children.add(new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note)); 1010 children.add(new Property("statisticType", "CodeableConcept", "Type of statistic, e.g., relative risk.", 0, 1, statisticType)); 1011 children.add(new Property("category", "CodeableConcept", "When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.", 0, 1, category)); 1012 children.add(new Property("quantity", "Quantity", "Statistic value.", 0, 1, quantity)); 1013 children.add(new Property("numberOfEvents", "unsignedInt", "The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberOfEvents)); 1014 children.add(new Property("numberAffected", "unsignedInt", "The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberAffected)); 1015 children.add(new Property("sampleSize", "", "Number of samples in the statistic.", 0, 1, sampleSize)); 1016 children.add(new Property("attributeEstimate", "", "A statistical attribute of the statistic such as a measure of heterogeneity.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate)); 1017 children.add(new Property("modelCharacteristic", "", "A component of the method to generate the statistic.", 0, java.lang.Integer.MAX_VALUE, modelCharacteristic)); 1018 } 1019 1020 @Override 1021 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1022 switch (_hash) { 1023 case -1724546052: /*description*/ return new Property("description", "markdown", "A description of the content value of the statistic.", 0, 1, description); 1024 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note); 1025 case -392342358: /*statisticType*/ return new Property("statisticType", "CodeableConcept", "Type of statistic, e.g., relative risk.", 0, 1, statisticType); 1026 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.", 0, 1, category); 1027 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Statistic value.", 0, 1, quantity); 1028 case 1534510137: /*numberOfEvents*/ return new Property("numberOfEvents", "unsignedInt", "The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberOfEvents); 1029 case -460990243: /*numberAffected*/ return new Property("numberAffected", "unsignedInt", "The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberAffected); 1030 case 143123659: /*sampleSize*/ return new Property("sampleSize", "", "Number of samples in the statistic.", 0, 1, sampleSize); 1031 case -1539581980: /*attributeEstimate*/ return new Property("attributeEstimate", "", "A statistical attribute of the statistic such as a measure of heterogeneity.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate); 1032 case 274795812: /*modelCharacteristic*/ return new Property("modelCharacteristic", "", "A component of the method to generate the statistic.", 0, java.lang.Integer.MAX_VALUE, modelCharacteristic); 1033 default: return super.getNamedProperty(_hash, _name, _checkValid); 1034 } 1035 1036 } 1037 1038 @Override 1039 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1040 switch (hash) { 1041 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1042 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1043 case -392342358: /*statisticType*/ return this.statisticType == null ? new Base[0] : new Base[] {this.statisticType}; // CodeableConcept 1044 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1045 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1046 case 1534510137: /*numberOfEvents*/ return this.numberOfEvents == null ? new Base[0] : new Base[] {this.numberOfEvents}; // UnsignedIntType 1047 case -460990243: /*numberAffected*/ return this.numberAffected == null ? new Base[0] : new Base[] {this.numberAffected}; // UnsignedIntType 1048 case 143123659: /*sampleSize*/ return this.sampleSize == null ? new Base[0] : new Base[] {this.sampleSize}; // EvidenceStatisticSampleSizeComponent 1049 case -1539581980: /*attributeEstimate*/ return this.attributeEstimate == null ? new Base[0] : this.attributeEstimate.toArray(new Base[this.attributeEstimate.size()]); // EvidenceStatisticAttributeEstimateComponent 1050 case 274795812: /*modelCharacteristic*/ return this.modelCharacteristic == null ? new Base[0] : this.modelCharacteristic.toArray(new Base[this.modelCharacteristic.size()]); // EvidenceStatisticModelCharacteristicComponent 1051 default: return super.getProperty(hash, name, checkValid); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1058 switch (hash) { 1059 case -1724546052: // description 1060 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1061 return value; 1062 case 3387378: // note 1063 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1064 return value; 1065 case -392342358: // statisticType 1066 this.statisticType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1067 return value; 1068 case 50511102: // category 1069 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1070 return value; 1071 case -1285004149: // quantity 1072 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1073 return value; 1074 case 1534510137: // numberOfEvents 1075 this.numberOfEvents = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1076 return value; 1077 case -460990243: // numberAffected 1078 this.numberAffected = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1079 return value; 1080 case 143123659: // sampleSize 1081 this.sampleSize = (EvidenceStatisticSampleSizeComponent) value; // EvidenceStatisticSampleSizeComponent 1082 return value; 1083 case -1539581980: // attributeEstimate 1084 this.getAttributeEstimate().add((EvidenceStatisticAttributeEstimateComponent) value); // EvidenceStatisticAttributeEstimateComponent 1085 return value; 1086 case 274795812: // modelCharacteristic 1087 this.getModelCharacteristic().add((EvidenceStatisticModelCharacteristicComponent) value); // EvidenceStatisticModelCharacteristicComponent 1088 return value; 1089 default: return super.setProperty(hash, name, value); 1090 } 1091 1092 } 1093 1094 @Override 1095 public Base setProperty(String name, Base value) throws FHIRException { 1096 if (name.equals("description")) { 1097 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1098 } else if (name.equals("note")) { 1099 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1100 } else if (name.equals("statisticType")) { 1101 this.statisticType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1102 } else if (name.equals("category")) { 1103 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1104 } else if (name.equals("quantity")) { 1105 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1106 } else if (name.equals("numberOfEvents")) { 1107 this.numberOfEvents = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1108 } else if (name.equals("numberAffected")) { 1109 this.numberAffected = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1110 } else if (name.equals("sampleSize")) { 1111 this.sampleSize = (EvidenceStatisticSampleSizeComponent) value; // EvidenceStatisticSampleSizeComponent 1112 } else if (name.equals("attributeEstimate")) { 1113 this.getAttributeEstimate().add((EvidenceStatisticAttributeEstimateComponent) value); 1114 } else if (name.equals("modelCharacteristic")) { 1115 this.getModelCharacteristic().add((EvidenceStatisticModelCharacteristicComponent) value); 1116 } else 1117 return super.setProperty(name, value); 1118 return value; 1119 } 1120 1121 @Override 1122 public void removeChild(String name, Base value) throws FHIRException { 1123 if (name.equals("description")) { 1124 this.description = null; 1125 } else if (name.equals("note")) { 1126 this.getNote().remove(value); 1127 } else if (name.equals("statisticType")) { 1128 this.statisticType = null; 1129 } else if (name.equals("category")) { 1130 this.category = null; 1131 } else if (name.equals("quantity")) { 1132 this.quantity = null; 1133 } else if (name.equals("numberOfEvents")) { 1134 this.numberOfEvents = null; 1135 } else if (name.equals("numberAffected")) { 1136 this.numberAffected = null; 1137 } else if (name.equals("sampleSize")) { 1138 this.sampleSize = (EvidenceStatisticSampleSizeComponent) value; // EvidenceStatisticSampleSizeComponent 1139 } else if (name.equals("attributeEstimate")) { 1140 this.getAttributeEstimate().remove((EvidenceStatisticAttributeEstimateComponent) value); 1141 } else if (name.equals("modelCharacteristic")) { 1142 this.getModelCharacteristic().remove((EvidenceStatisticModelCharacteristicComponent) value); 1143 } else 1144 super.removeChild(name, value); 1145 1146 } 1147 1148 @Override 1149 public Base makeProperty(int hash, String name) throws FHIRException { 1150 switch (hash) { 1151 case -1724546052: return getDescriptionElement(); 1152 case 3387378: return addNote(); 1153 case -392342358: return getStatisticType(); 1154 case 50511102: return getCategory(); 1155 case -1285004149: return getQuantity(); 1156 case 1534510137: return getNumberOfEventsElement(); 1157 case -460990243: return getNumberAffectedElement(); 1158 case 143123659: return getSampleSize(); 1159 case -1539581980: return addAttributeEstimate(); 1160 case 274795812: return addModelCharacteristic(); 1161 default: return super.makeProperty(hash, name); 1162 } 1163 1164 } 1165 1166 @Override 1167 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1168 switch (hash) { 1169 case -1724546052: /*description*/ return new String[] {"markdown"}; 1170 case 3387378: /*note*/ return new String[] {"Annotation"}; 1171 case -392342358: /*statisticType*/ return new String[] {"CodeableConcept"}; 1172 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1173 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1174 case 1534510137: /*numberOfEvents*/ return new String[] {"unsignedInt"}; 1175 case -460990243: /*numberAffected*/ return new String[] {"unsignedInt"}; 1176 case 143123659: /*sampleSize*/ return new String[] {}; 1177 case -1539581980: /*attributeEstimate*/ return new String[] {}; 1178 case 274795812: /*modelCharacteristic*/ return new String[] {}; 1179 default: return super.getTypesForProperty(hash, name); 1180 } 1181 1182 } 1183 1184 @Override 1185 public Base addChild(String name) throws FHIRException { 1186 if (name.equals("description")) { 1187 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.description"); 1188 } 1189 else if (name.equals("note")) { 1190 return addNote(); 1191 } 1192 else if (name.equals("statisticType")) { 1193 this.statisticType = new CodeableConcept(); 1194 return this.statisticType; 1195 } 1196 else if (name.equals("category")) { 1197 this.category = new CodeableConcept(); 1198 return this.category; 1199 } 1200 else if (name.equals("quantity")) { 1201 this.quantity = new Quantity(); 1202 return this.quantity; 1203 } 1204 else if (name.equals("numberOfEvents")) { 1205 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.numberOfEvents"); 1206 } 1207 else if (name.equals("numberAffected")) { 1208 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.numberAffected"); 1209 } 1210 else if (name.equals("sampleSize")) { 1211 this.sampleSize = new EvidenceStatisticSampleSizeComponent(); 1212 return this.sampleSize; 1213 } 1214 else if (name.equals("attributeEstimate")) { 1215 return addAttributeEstimate(); 1216 } 1217 else if (name.equals("modelCharacteristic")) { 1218 return addModelCharacteristic(); 1219 } 1220 else 1221 return super.addChild(name); 1222 } 1223 1224 public EvidenceStatisticComponent copy() { 1225 EvidenceStatisticComponent dst = new EvidenceStatisticComponent(); 1226 copyValues(dst); 1227 return dst; 1228 } 1229 1230 public void copyValues(EvidenceStatisticComponent dst) { 1231 super.copyValues(dst); 1232 dst.description = description == null ? null : description.copy(); 1233 if (note != null) { 1234 dst.note = new ArrayList<Annotation>(); 1235 for (Annotation i : note) 1236 dst.note.add(i.copy()); 1237 }; 1238 dst.statisticType = statisticType == null ? null : statisticType.copy(); 1239 dst.category = category == null ? null : category.copy(); 1240 dst.quantity = quantity == null ? null : quantity.copy(); 1241 dst.numberOfEvents = numberOfEvents == null ? null : numberOfEvents.copy(); 1242 dst.numberAffected = numberAffected == null ? null : numberAffected.copy(); 1243 dst.sampleSize = sampleSize == null ? null : sampleSize.copy(); 1244 if (attributeEstimate != null) { 1245 dst.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 1246 for (EvidenceStatisticAttributeEstimateComponent i : attributeEstimate) 1247 dst.attributeEstimate.add(i.copy()); 1248 }; 1249 if (modelCharacteristic != null) { 1250 dst.modelCharacteristic = new ArrayList<EvidenceStatisticModelCharacteristicComponent>(); 1251 for (EvidenceStatisticModelCharacteristicComponent i : modelCharacteristic) 1252 dst.modelCharacteristic.add(i.copy()); 1253 }; 1254 } 1255 1256 @Override 1257 public boolean equalsDeep(Base other_) { 1258 if (!super.equalsDeep(other_)) 1259 return false; 1260 if (!(other_ instanceof EvidenceStatisticComponent)) 1261 return false; 1262 EvidenceStatisticComponent o = (EvidenceStatisticComponent) other_; 1263 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(statisticType, o.statisticType, true) 1264 && compareDeep(category, o.category, true) && compareDeep(quantity, o.quantity, true) && compareDeep(numberOfEvents, o.numberOfEvents, true) 1265 && compareDeep(numberAffected, o.numberAffected, true) && compareDeep(sampleSize, o.sampleSize, true) 1266 && compareDeep(attributeEstimate, o.attributeEstimate, true) && compareDeep(modelCharacteristic, o.modelCharacteristic, true) 1267 ; 1268 } 1269 1270 @Override 1271 public boolean equalsShallow(Base other_) { 1272 if (!super.equalsShallow(other_)) 1273 return false; 1274 if (!(other_ instanceof EvidenceStatisticComponent)) 1275 return false; 1276 EvidenceStatisticComponent o = (EvidenceStatisticComponent) other_; 1277 return compareValues(description, o.description, true) && compareValues(numberOfEvents, o.numberOfEvents, true) 1278 && compareValues(numberAffected, o.numberAffected, true); 1279 } 1280 1281 public boolean isEmpty() { 1282 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, statisticType 1283 , category, quantity, numberOfEvents, numberAffected, sampleSize, attributeEstimate 1284 , modelCharacteristic); 1285 } 1286 1287 public String fhirType() { 1288 return "Evidence.statistic"; 1289 1290 } 1291 1292 } 1293 1294 @Block() 1295 public static class EvidenceStatisticSampleSizeComponent extends BackboneElement implements IBaseBackboneElement { 1296 /** 1297 * Human-readable summary of population sample size. 1298 */ 1299 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1300 @Description(shortDefinition="Textual description of sample size for statistic", formalDefinition="Human-readable summary of population sample size." ) 1301 protected MarkdownType description; 1302 1303 /** 1304 * Footnote or explanatory note about the sample size. 1305 */ 1306 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1307 @Description(shortDefinition="Footnote or explanatory note about the sample size", formalDefinition="Footnote or explanatory note about the sample size." ) 1308 protected List<Annotation> note; 1309 1310 /** 1311 * Number of participants in the population. 1312 */ 1313 @Child(name = "numberOfStudies", type = {UnsignedIntType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1314 @Description(shortDefinition="Number of contributing studies", formalDefinition="Number of participants in the population." ) 1315 protected UnsignedIntType numberOfStudies; 1316 1317 /** 1318 * A human-readable string to clarify or explain concepts about the sample size. 1319 */ 1320 @Child(name = "numberOfParticipants", type = {UnsignedIntType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1321 @Description(shortDefinition="Cumulative number of participants", formalDefinition="A human-readable string to clarify or explain concepts about the sample size." ) 1322 protected UnsignedIntType numberOfParticipants; 1323 1324 /** 1325 * Number of participants with known results for measured variables. 1326 */ 1327 @Child(name = "knownDataCount", type = {UnsignedIntType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1328 @Description(shortDefinition="Number of participants with known results for measured variables", formalDefinition="Number of participants with known results for measured variables." ) 1329 protected UnsignedIntType knownDataCount; 1330 1331 private static final long serialVersionUID = -1710653289L; 1332 1333 /** 1334 * Constructor 1335 */ 1336 public EvidenceStatisticSampleSizeComponent() { 1337 super(); 1338 } 1339 1340 /** 1341 * @return {@link #description} (Human-readable summary of population sample size.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1342 */ 1343 public MarkdownType getDescriptionElement() { 1344 if (this.description == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error("Attempt to auto-create EvidenceStatisticSampleSizeComponent.description"); 1347 else if (Configuration.doAutoCreate()) 1348 this.description = new MarkdownType(); // bb 1349 return this.description; 1350 } 1351 1352 public boolean hasDescriptionElement() { 1353 return this.description != null && !this.description.isEmpty(); 1354 } 1355 1356 public boolean hasDescription() { 1357 return this.description != null && !this.description.isEmpty(); 1358 } 1359 1360 /** 1361 * @param value {@link #description} (Human-readable summary of population sample size.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1362 */ 1363 public EvidenceStatisticSampleSizeComponent setDescriptionElement(MarkdownType value) { 1364 this.description = value; 1365 return this; 1366 } 1367 1368 /** 1369 * @return Human-readable summary of population sample size. 1370 */ 1371 public String getDescription() { 1372 return this.description == null ? null : this.description.getValue(); 1373 } 1374 1375 /** 1376 * @param value Human-readable summary of population sample size. 1377 */ 1378 public EvidenceStatisticSampleSizeComponent setDescription(String value) { 1379 if (Utilities.noString(value)) 1380 this.description = null; 1381 else { 1382 if (this.description == null) 1383 this.description = new MarkdownType(); 1384 this.description.setValue(value); 1385 } 1386 return this; 1387 } 1388 1389 /** 1390 * @return {@link #note} (Footnote or explanatory note about the sample size.) 1391 */ 1392 public List<Annotation> getNote() { 1393 if (this.note == null) 1394 this.note = new ArrayList<Annotation>(); 1395 return this.note; 1396 } 1397 1398 /** 1399 * @return Returns a reference to <code>this</code> for easy method chaining 1400 */ 1401 public EvidenceStatisticSampleSizeComponent setNote(List<Annotation> theNote) { 1402 this.note = theNote; 1403 return this; 1404 } 1405 1406 public boolean hasNote() { 1407 if (this.note == null) 1408 return false; 1409 for (Annotation item : this.note) 1410 if (!item.isEmpty()) 1411 return true; 1412 return false; 1413 } 1414 1415 public Annotation addNote() { //3 1416 Annotation t = new Annotation(); 1417 if (this.note == null) 1418 this.note = new ArrayList<Annotation>(); 1419 this.note.add(t); 1420 return t; 1421 } 1422 1423 public EvidenceStatisticSampleSizeComponent addNote(Annotation t) { //3 1424 if (t == null) 1425 return this; 1426 if (this.note == null) 1427 this.note = new ArrayList<Annotation>(); 1428 this.note.add(t); 1429 return this; 1430 } 1431 1432 /** 1433 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1434 */ 1435 public Annotation getNoteFirstRep() { 1436 if (getNote().isEmpty()) { 1437 addNote(); 1438 } 1439 return getNote().get(0); 1440 } 1441 1442 /** 1443 * @return {@link #numberOfStudies} (Number of participants in the population.). This is the underlying object with id, value and extensions. The accessor "getNumberOfStudies" gives direct access to the value 1444 */ 1445 public UnsignedIntType getNumberOfStudiesElement() { 1446 if (this.numberOfStudies == null) 1447 if (Configuration.errorOnAutoCreate()) 1448 throw new Error("Attempt to auto-create EvidenceStatisticSampleSizeComponent.numberOfStudies"); 1449 else if (Configuration.doAutoCreate()) 1450 this.numberOfStudies = new UnsignedIntType(); // bb 1451 return this.numberOfStudies; 1452 } 1453 1454 public boolean hasNumberOfStudiesElement() { 1455 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 1456 } 1457 1458 public boolean hasNumberOfStudies() { 1459 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 1460 } 1461 1462 /** 1463 * @param value {@link #numberOfStudies} (Number of participants in the population.). This is the underlying object with id, value and extensions. The accessor "getNumberOfStudies" gives direct access to the value 1464 */ 1465 public EvidenceStatisticSampleSizeComponent setNumberOfStudiesElement(UnsignedIntType value) { 1466 this.numberOfStudies = value; 1467 return this; 1468 } 1469 1470 /** 1471 * @return Number of participants in the population. 1472 */ 1473 public int getNumberOfStudies() { 1474 return this.numberOfStudies == null || this.numberOfStudies.isEmpty() ? 0 : this.numberOfStudies.getValue(); 1475 } 1476 1477 /** 1478 * @param value Number of participants in the population. 1479 */ 1480 public EvidenceStatisticSampleSizeComponent setNumberOfStudies(int value) { 1481 if (this.numberOfStudies == null) 1482 this.numberOfStudies = new UnsignedIntType(); 1483 this.numberOfStudies.setValue(value); 1484 return this; 1485 } 1486 1487 /** 1488 * @return {@link #numberOfParticipants} (A human-readable string to clarify or explain concepts about the sample size.). This is the underlying object with id, value and extensions. The accessor "getNumberOfParticipants" gives direct access to the value 1489 */ 1490 public UnsignedIntType getNumberOfParticipantsElement() { 1491 if (this.numberOfParticipants == null) 1492 if (Configuration.errorOnAutoCreate()) 1493 throw new Error("Attempt to auto-create EvidenceStatisticSampleSizeComponent.numberOfParticipants"); 1494 else if (Configuration.doAutoCreate()) 1495 this.numberOfParticipants = new UnsignedIntType(); // bb 1496 return this.numberOfParticipants; 1497 } 1498 1499 public boolean hasNumberOfParticipantsElement() { 1500 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 1501 } 1502 1503 public boolean hasNumberOfParticipants() { 1504 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 1505 } 1506 1507 /** 1508 * @param value {@link #numberOfParticipants} (A human-readable string to clarify or explain concepts about the sample size.). This is the underlying object with id, value and extensions. The accessor "getNumberOfParticipants" gives direct access to the value 1509 */ 1510 public EvidenceStatisticSampleSizeComponent setNumberOfParticipantsElement(UnsignedIntType value) { 1511 this.numberOfParticipants = value; 1512 return this; 1513 } 1514 1515 /** 1516 * @return A human-readable string to clarify or explain concepts about the sample size. 1517 */ 1518 public int getNumberOfParticipants() { 1519 return this.numberOfParticipants == null || this.numberOfParticipants.isEmpty() ? 0 : this.numberOfParticipants.getValue(); 1520 } 1521 1522 /** 1523 * @param value A human-readable string to clarify or explain concepts about the sample size. 1524 */ 1525 public EvidenceStatisticSampleSizeComponent setNumberOfParticipants(int value) { 1526 if (this.numberOfParticipants == null) 1527 this.numberOfParticipants = new UnsignedIntType(); 1528 this.numberOfParticipants.setValue(value); 1529 return this; 1530 } 1531 1532 /** 1533 * @return {@link #knownDataCount} (Number of participants with known results for measured variables.). This is the underlying object with id, value and extensions. The accessor "getKnownDataCount" gives direct access to the value 1534 */ 1535 public UnsignedIntType getKnownDataCountElement() { 1536 if (this.knownDataCount == null) 1537 if (Configuration.errorOnAutoCreate()) 1538 throw new Error("Attempt to auto-create EvidenceStatisticSampleSizeComponent.knownDataCount"); 1539 else if (Configuration.doAutoCreate()) 1540 this.knownDataCount = new UnsignedIntType(); // bb 1541 return this.knownDataCount; 1542 } 1543 1544 public boolean hasKnownDataCountElement() { 1545 return this.knownDataCount != null && !this.knownDataCount.isEmpty(); 1546 } 1547 1548 public boolean hasKnownDataCount() { 1549 return this.knownDataCount != null && !this.knownDataCount.isEmpty(); 1550 } 1551 1552 /** 1553 * @param value {@link #knownDataCount} (Number of participants with known results for measured variables.). This is the underlying object with id, value and extensions. The accessor "getKnownDataCount" gives direct access to the value 1554 */ 1555 public EvidenceStatisticSampleSizeComponent setKnownDataCountElement(UnsignedIntType value) { 1556 this.knownDataCount = value; 1557 return this; 1558 } 1559 1560 /** 1561 * @return Number of participants with known results for measured variables. 1562 */ 1563 public int getKnownDataCount() { 1564 return this.knownDataCount == null || this.knownDataCount.isEmpty() ? 0 : this.knownDataCount.getValue(); 1565 } 1566 1567 /** 1568 * @param value Number of participants with known results for measured variables. 1569 */ 1570 public EvidenceStatisticSampleSizeComponent setKnownDataCount(int value) { 1571 if (this.knownDataCount == null) 1572 this.knownDataCount = new UnsignedIntType(); 1573 this.knownDataCount.setValue(value); 1574 return this; 1575 } 1576 1577 protected void listChildren(List<Property> children) { 1578 super.listChildren(children); 1579 children.add(new Property("description", "markdown", "Human-readable summary of population sample size.", 0, 1, description)); 1580 children.add(new Property("note", "Annotation", "Footnote or explanatory note about the sample size.", 0, java.lang.Integer.MAX_VALUE, note)); 1581 children.add(new Property("numberOfStudies", "unsignedInt", "Number of participants in the population.", 0, 1, numberOfStudies)); 1582 children.add(new Property("numberOfParticipants", "unsignedInt", "A human-readable string to clarify or explain concepts about the sample size.", 0, 1, numberOfParticipants)); 1583 children.add(new Property("knownDataCount", "unsignedInt", "Number of participants with known results for measured variables.", 0, 1, knownDataCount)); 1584 } 1585 1586 @Override 1587 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1588 switch (_hash) { 1589 case -1724546052: /*description*/ return new Property("description", "markdown", "Human-readable summary of population sample size.", 0, 1, description); 1590 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnote or explanatory note about the sample size.", 0, java.lang.Integer.MAX_VALUE, note); 1591 case -177467129: /*numberOfStudies*/ return new Property("numberOfStudies", "unsignedInt", "Number of participants in the population.", 0, 1, numberOfStudies); 1592 case 1799357120: /*numberOfParticipants*/ return new Property("numberOfParticipants", "unsignedInt", "A human-readable string to clarify or explain concepts about the sample size.", 0, 1, numberOfParticipants); 1593 case -937344126: /*knownDataCount*/ return new Property("knownDataCount", "unsignedInt", "Number of participants with known results for measured variables.", 0, 1, knownDataCount); 1594 default: return super.getNamedProperty(_hash, _name, _checkValid); 1595 } 1596 1597 } 1598 1599 @Override 1600 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1601 switch (hash) { 1602 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1603 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1604 case -177467129: /*numberOfStudies*/ return this.numberOfStudies == null ? new Base[0] : new Base[] {this.numberOfStudies}; // UnsignedIntType 1605 case 1799357120: /*numberOfParticipants*/ return this.numberOfParticipants == null ? new Base[0] : new Base[] {this.numberOfParticipants}; // UnsignedIntType 1606 case -937344126: /*knownDataCount*/ return this.knownDataCount == null ? new Base[0] : new Base[] {this.knownDataCount}; // UnsignedIntType 1607 default: return super.getProperty(hash, name, checkValid); 1608 } 1609 1610 } 1611 1612 @Override 1613 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1614 switch (hash) { 1615 case -1724546052: // description 1616 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1617 return value; 1618 case 3387378: // note 1619 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1620 return value; 1621 case -177467129: // numberOfStudies 1622 this.numberOfStudies = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1623 return value; 1624 case 1799357120: // numberOfParticipants 1625 this.numberOfParticipants = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1626 return value; 1627 case -937344126: // knownDataCount 1628 this.knownDataCount = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1629 return value; 1630 default: return super.setProperty(hash, name, value); 1631 } 1632 1633 } 1634 1635 @Override 1636 public Base setProperty(String name, Base value) throws FHIRException { 1637 if (name.equals("description")) { 1638 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1639 } else if (name.equals("note")) { 1640 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1641 } else if (name.equals("numberOfStudies")) { 1642 this.numberOfStudies = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1643 } else if (name.equals("numberOfParticipants")) { 1644 this.numberOfParticipants = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1645 } else if (name.equals("knownDataCount")) { 1646 this.knownDataCount = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1647 } else 1648 return super.setProperty(name, value); 1649 return value; 1650 } 1651 1652 @Override 1653 public void removeChild(String name, Base value) throws FHIRException { 1654 if (name.equals("description")) { 1655 this.description = null; 1656 } else if (name.equals("note")) { 1657 this.getNote().remove(value); 1658 } else if (name.equals("numberOfStudies")) { 1659 this.numberOfStudies = null; 1660 } else if (name.equals("numberOfParticipants")) { 1661 this.numberOfParticipants = null; 1662 } else if (name.equals("knownDataCount")) { 1663 this.knownDataCount = null; 1664 } else 1665 super.removeChild(name, value); 1666 1667 } 1668 1669 @Override 1670 public Base makeProperty(int hash, String name) throws FHIRException { 1671 switch (hash) { 1672 case -1724546052: return getDescriptionElement(); 1673 case 3387378: return addNote(); 1674 case -177467129: return getNumberOfStudiesElement(); 1675 case 1799357120: return getNumberOfParticipantsElement(); 1676 case -937344126: return getKnownDataCountElement(); 1677 default: return super.makeProperty(hash, name); 1678 } 1679 1680 } 1681 1682 @Override 1683 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1684 switch (hash) { 1685 case -1724546052: /*description*/ return new String[] {"markdown"}; 1686 case 3387378: /*note*/ return new String[] {"Annotation"}; 1687 case -177467129: /*numberOfStudies*/ return new String[] {"unsignedInt"}; 1688 case 1799357120: /*numberOfParticipants*/ return new String[] {"unsignedInt"}; 1689 case -937344126: /*knownDataCount*/ return new String[] {"unsignedInt"}; 1690 default: return super.getTypesForProperty(hash, name); 1691 } 1692 1693 } 1694 1695 @Override 1696 public Base addChild(String name) throws FHIRException { 1697 if (name.equals("description")) { 1698 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.sampleSize.description"); 1699 } 1700 else if (name.equals("note")) { 1701 return addNote(); 1702 } 1703 else if (name.equals("numberOfStudies")) { 1704 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.sampleSize.numberOfStudies"); 1705 } 1706 else if (name.equals("numberOfParticipants")) { 1707 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.sampleSize.numberOfParticipants"); 1708 } 1709 else if (name.equals("knownDataCount")) { 1710 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.sampleSize.knownDataCount"); 1711 } 1712 else 1713 return super.addChild(name); 1714 } 1715 1716 public EvidenceStatisticSampleSizeComponent copy() { 1717 EvidenceStatisticSampleSizeComponent dst = new EvidenceStatisticSampleSizeComponent(); 1718 copyValues(dst); 1719 return dst; 1720 } 1721 1722 public void copyValues(EvidenceStatisticSampleSizeComponent dst) { 1723 super.copyValues(dst); 1724 dst.description = description == null ? null : description.copy(); 1725 if (note != null) { 1726 dst.note = new ArrayList<Annotation>(); 1727 for (Annotation i : note) 1728 dst.note.add(i.copy()); 1729 }; 1730 dst.numberOfStudies = numberOfStudies == null ? null : numberOfStudies.copy(); 1731 dst.numberOfParticipants = numberOfParticipants == null ? null : numberOfParticipants.copy(); 1732 dst.knownDataCount = knownDataCount == null ? null : knownDataCount.copy(); 1733 } 1734 1735 @Override 1736 public boolean equalsDeep(Base other_) { 1737 if (!super.equalsDeep(other_)) 1738 return false; 1739 if (!(other_ instanceof EvidenceStatisticSampleSizeComponent)) 1740 return false; 1741 EvidenceStatisticSampleSizeComponent o = (EvidenceStatisticSampleSizeComponent) other_; 1742 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(numberOfStudies, o.numberOfStudies, true) 1743 && compareDeep(numberOfParticipants, o.numberOfParticipants, true) && compareDeep(knownDataCount, o.knownDataCount, true) 1744 ; 1745 } 1746 1747 @Override 1748 public boolean equalsShallow(Base other_) { 1749 if (!super.equalsShallow(other_)) 1750 return false; 1751 if (!(other_ instanceof EvidenceStatisticSampleSizeComponent)) 1752 return false; 1753 EvidenceStatisticSampleSizeComponent o = (EvidenceStatisticSampleSizeComponent) other_; 1754 return compareValues(description, o.description, true) && compareValues(numberOfStudies, o.numberOfStudies, true) 1755 && compareValues(numberOfParticipants, o.numberOfParticipants, true) && compareValues(knownDataCount, o.knownDataCount, true) 1756 ; 1757 } 1758 1759 public boolean isEmpty() { 1760 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, numberOfStudies 1761 , numberOfParticipants, knownDataCount); 1762 } 1763 1764 public String fhirType() { 1765 return "Evidence.statistic.sampleSize"; 1766 1767 } 1768 1769 } 1770 1771 @Block() 1772 public static class EvidenceStatisticAttributeEstimateComponent extends BackboneElement implements IBaseBackboneElement { 1773 /** 1774 * Human-readable summary of the estimate. 1775 */ 1776 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1777 @Description(shortDefinition="Textual description of the attribute estimate", formalDefinition="Human-readable summary of the estimate." ) 1778 protected MarkdownType description; 1779 1780 /** 1781 * Footnote or explanatory note about the estimate. 1782 */ 1783 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1784 @Description(shortDefinition="Footnote or explanatory note about the estimate", formalDefinition="Footnote or explanatory note about the estimate." ) 1785 protected List<Annotation> note; 1786 1787 /** 1788 * The type of attribute estimate, e.g., confidence interval or p value. 1789 */ 1790 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1791 @Description(shortDefinition="The type of attribute estimate, e.g., confidence interval or p value", formalDefinition="The type of attribute estimate, e.g., confidence interval or p value." ) 1792 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/attribute-estimate-type") 1793 protected CodeableConcept type; 1794 1795 /** 1796 * The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure. 1797 */ 1798 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 1799 @Description(shortDefinition="The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure", formalDefinition="The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure." ) 1800 protected Quantity quantity; 1801 1802 /** 1803 * Use 95 for a 95% confidence interval. 1804 */ 1805 @Child(name = "level", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1806 @Description(shortDefinition="Level of confidence interval, e.g., 0.95 for 95% confidence interval", formalDefinition="Use 95 for a 95% confidence interval." ) 1807 protected DecimalType level; 1808 1809 /** 1810 * Lower bound of confidence interval. 1811 */ 1812 @Child(name = "range", type = {Range.class}, order=6, min=0, max=1, modifier=false, summary=false) 1813 @Description(shortDefinition="Lower and upper bound values of the attribute estimate", formalDefinition="Lower bound of confidence interval." ) 1814 protected Range range; 1815 1816 /** 1817 * A nested attribute estimate; which is the attribute estimate of an attribute estimate. 1818 */ 1819 @Child(name = "attributeEstimate", type = {EvidenceStatisticAttributeEstimateComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1820 @Description(shortDefinition="A nested attribute estimate; which is the attribute estimate of an attribute estimate", formalDefinition="A nested attribute estimate; which is the attribute estimate of an attribute estimate." ) 1821 protected List<EvidenceStatisticAttributeEstimateComponent> attributeEstimate; 1822 1823 private static final long serialVersionUID = -1535970380L; 1824 1825 /** 1826 * Constructor 1827 */ 1828 public EvidenceStatisticAttributeEstimateComponent() { 1829 super(); 1830 } 1831 1832 /** 1833 * @return {@link #description} (Human-readable summary of the estimate.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1834 */ 1835 public MarkdownType getDescriptionElement() { 1836 if (this.description == null) 1837 if (Configuration.errorOnAutoCreate()) 1838 throw new Error("Attempt to auto-create EvidenceStatisticAttributeEstimateComponent.description"); 1839 else if (Configuration.doAutoCreate()) 1840 this.description = new MarkdownType(); // bb 1841 return this.description; 1842 } 1843 1844 public boolean hasDescriptionElement() { 1845 return this.description != null && !this.description.isEmpty(); 1846 } 1847 1848 public boolean hasDescription() { 1849 return this.description != null && !this.description.isEmpty(); 1850 } 1851 1852 /** 1853 * @param value {@link #description} (Human-readable summary of the estimate.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1854 */ 1855 public EvidenceStatisticAttributeEstimateComponent setDescriptionElement(MarkdownType value) { 1856 this.description = value; 1857 return this; 1858 } 1859 1860 /** 1861 * @return Human-readable summary of the estimate. 1862 */ 1863 public String getDescription() { 1864 return this.description == null ? null : this.description.getValue(); 1865 } 1866 1867 /** 1868 * @param value Human-readable summary of the estimate. 1869 */ 1870 public EvidenceStatisticAttributeEstimateComponent setDescription(String value) { 1871 if (Utilities.noString(value)) 1872 this.description = null; 1873 else { 1874 if (this.description == null) 1875 this.description = new MarkdownType(); 1876 this.description.setValue(value); 1877 } 1878 return this; 1879 } 1880 1881 /** 1882 * @return {@link #note} (Footnote or explanatory note about the estimate.) 1883 */ 1884 public List<Annotation> getNote() { 1885 if (this.note == null) 1886 this.note = new ArrayList<Annotation>(); 1887 return this.note; 1888 } 1889 1890 /** 1891 * @return Returns a reference to <code>this</code> for easy method chaining 1892 */ 1893 public EvidenceStatisticAttributeEstimateComponent setNote(List<Annotation> theNote) { 1894 this.note = theNote; 1895 return this; 1896 } 1897 1898 public boolean hasNote() { 1899 if (this.note == null) 1900 return false; 1901 for (Annotation item : this.note) 1902 if (!item.isEmpty()) 1903 return true; 1904 return false; 1905 } 1906 1907 public Annotation addNote() { //3 1908 Annotation t = new Annotation(); 1909 if (this.note == null) 1910 this.note = new ArrayList<Annotation>(); 1911 this.note.add(t); 1912 return t; 1913 } 1914 1915 public EvidenceStatisticAttributeEstimateComponent addNote(Annotation t) { //3 1916 if (t == null) 1917 return this; 1918 if (this.note == null) 1919 this.note = new ArrayList<Annotation>(); 1920 this.note.add(t); 1921 return this; 1922 } 1923 1924 /** 1925 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1926 */ 1927 public Annotation getNoteFirstRep() { 1928 if (getNote().isEmpty()) { 1929 addNote(); 1930 } 1931 return getNote().get(0); 1932 } 1933 1934 /** 1935 * @return {@link #type} (The type of attribute estimate, e.g., confidence interval or p value.) 1936 */ 1937 public CodeableConcept getType() { 1938 if (this.type == null) 1939 if (Configuration.errorOnAutoCreate()) 1940 throw new Error("Attempt to auto-create EvidenceStatisticAttributeEstimateComponent.type"); 1941 else if (Configuration.doAutoCreate()) 1942 this.type = new CodeableConcept(); // cc 1943 return this.type; 1944 } 1945 1946 public boolean hasType() { 1947 return this.type != null && !this.type.isEmpty(); 1948 } 1949 1950 /** 1951 * @param value {@link #type} (The type of attribute estimate, e.g., confidence interval or p value.) 1952 */ 1953 public EvidenceStatisticAttributeEstimateComponent setType(CodeableConcept value) { 1954 this.type = value; 1955 return this; 1956 } 1957 1958 /** 1959 * @return {@link #quantity} (The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.) 1960 */ 1961 public Quantity getQuantity() { 1962 if (this.quantity == null) 1963 if (Configuration.errorOnAutoCreate()) 1964 throw new Error("Attempt to auto-create EvidenceStatisticAttributeEstimateComponent.quantity"); 1965 else if (Configuration.doAutoCreate()) 1966 this.quantity = new Quantity(); // cc 1967 return this.quantity; 1968 } 1969 1970 public boolean hasQuantity() { 1971 return this.quantity != null && !this.quantity.isEmpty(); 1972 } 1973 1974 /** 1975 * @param value {@link #quantity} (The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.) 1976 */ 1977 public EvidenceStatisticAttributeEstimateComponent setQuantity(Quantity value) { 1978 this.quantity = value; 1979 return this; 1980 } 1981 1982 /** 1983 * @return {@link #level} (Use 95 for a 95% confidence interval.). This is the underlying object with id, value and extensions. The accessor "getLevel" gives direct access to the value 1984 */ 1985 public DecimalType getLevelElement() { 1986 if (this.level == null) 1987 if (Configuration.errorOnAutoCreate()) 1988 throw new Error("Attempt to auto-create EvidenceStatisticAttributeEstimateComponent.level"); 1989 else if (Configuration.doAutoCreate()) 1990 this.level = new DecimalType(); // bb 1991 return this.level; 1992 } 1993 1994 public boolean hasLevelElement() { 1995 return this.level != null && !this.level.isEmpty(); 1996 } 1997 1998 public boolean hasLevel() { 1999 return this.level != null && !this.level.isEmpty(); 2000 } 2001 2002 /** 2003 * @param value {@link #level} (Use 95 for a 95% confidence interval.). This is the underlying object with id, value and extensions. The accessor "getLevel" gives direct access to the value 2004 */ 2005 public EvidenceStatisticAttributeEstimateComponent setLevelElement(DecimalType value) { 2006 this.level = value; 2007 return this; 2008 } 2009 2010 /** 2011 * @return Use 95 for a 95% confidence interval. 2012 */ 2013 public BigDecimal getLevel() { 2014 return this.level == null ? null : this.level.getValue(); 2015 } 2016 2017 /** 2018 * @param value Use 95 for a 95% confidence interval. 2019 */ 2020 public EvidenceStatisticAttributeEstimateComponent setLevel(BigDecimal value) { 2021 if (value == null) 2022 this.level = null; 2023 else { 2024 if (this.level == null) 2025 this.level = new DecimalType(); 2026 this.level.setValue(value); 2027 } 2028 return this; 2029 } 2030 2031 /** 2032 * @param value Use 95 for a 95% confidence interval. 2033 */ 2034 public EvidenceStatisticAttributeEstimateComponent setLevel(long value) { 2035 this.level = new DecimalType(); 2036 this.level.setValue(value); 2037 return this; 2038 } 2039 2040 /** 2041 * @param value Use 95 for a 95% confidence interval. 2042 */ 2043 public EvidenceStatisticAttributeEstimateComponent setLevel(double value) { 2044 this.level = new DecimalType(); 2045 this.level.setValue(value); 2046 return this; 2047 } 2048 2049 /** 2050 * @return {@link #range} (Lower bound of confidence interval.) 2051 */ 2052 public Range getRange() { 2053 if (this.range == null) 2054 if (Configuration.errorOnAutoCreate()) 2055 throw new Error("Attempt to auto-create EvidenceStatisticAttributeEstimateComponent.range"); 2056 else if (Configuration.doAutoCreate()) 2057 this.range = new Range(); // cc 2058 return this.range; 2059 } 2060 2061 public boolean hasRange() { 2062 return this.range != null && !this.range.isEmpty(); 2063 } 2064 2065 /** 2066 * @param value {@link #range} (Lower bound of confidence interval.) 2067 */ 2068 public EvidenceStatisticAttributeEstimateComponent setRange(Range value) { 2069 this.range = value; 2070 return this; 2071 } 2072 2073 /** 2074 * @return {@link #attributeEstimate} (A nested attribute estimate; which is the attribute estimate of an attribute estimate.) 2075 */ 2076 public List<EvidenceStatisticAttributeEstimateComponent> getAttributeEstimate() { 2077 if (this.attributeEstimate == null) 2078 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2079 return this.attributeEstimate; 2080 } 2081 2082 /** 2083 * @return Returns a reference to <code>this</code> for easy method chaining 2084 */ 2085 public EvidenceStatisticAttributeEstimateComponent setAttributeEstimate(List<EvidenceStatisticAttributeEstimateComponent> theAttributeEstimate) { 2086 this.attributeEstimate = theAttributeEstimate; 2087 return this; 2088 } 2089 2090 public boolean hasAttributeEstimate() { 2091 if (this.attributeEstimate == null) 2092 return false; 2093 for (EvidenceStatisticAttributeEstimateComponent item : this.attributeEstimate) 2094 if (!item.isEmpty()) 2095 return true; 2096 return false; 2097 } 2098 2099 public EvidenceStatisticAttributeEstimateComponent addAttributeEstimate() { //3 2100 EvidenceStatisticAttributeEstimateComponent t = new EvidenceStatisticAttributeEstimateComponent(); 2101 if (this.attributeEstimate == null) 2102 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2103 this.attributeEstimate.add(t); 2104 return t; 2105 } 2106 2107 public EvidenceStatisticAttributeEstimateComponent addAttributeEstimate(EvidenceStatisticAttributeEstimateComponent t) { //3 2108 if (t == null) 2109 return this; 2110 if (this.attributeEstimate == null) 2111 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2112 this.attributeEstimate.add(t); 2113 return this; 2114 } 2115 2116 /** 2117 * @return The first repetition of repeating field {@link #attributeEstimate}, creating it if it does not already exist {3} 2118 */ 2119 public EvidenceStatisticAttributeEstimateComponent getAttributeEstimateFirstRep() { 2120 if (getAttributeEstimate().isEmpty()) { 2121 addAttributeEstimate(); 2122 } 2123 return getAttributeEstimate().get(0); 2124 } 2125 2126 protected void listChildren(List<Property> children) { 2127 super.listChildren(children); 2128 children.add(new Property("description", "markdown", "Human-readable summary of the estimate.", 0, 1, description)); 2129 children.add(new Property("note", "Annotation", "Footnote or explanatory note about the estimate.", 0, java.lang.Integer.MAX_VALUE, note)); 2130 children.add(new Property("type", "CodeableConcept", "The type of attribute estimate, e.g., confidence interval or p value.", 0, 1, type)); 2131 children.add(new Property("quantity", "Quantity", "The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.", 0, 1, quantity)); 2132 children.add(new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level)); 2133 children.add(new Property("range", "Range", "Lower bound of confidence interval.", 0, 1, range)); 2134 children.add(new Property("attributeEstimate", "@Evidence.statistic.attributeEstimate", "A nested attribute estimate; which is the attribute estimate of an attribute estimate.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate)); 2135 } 2136 2137 @Override 2138 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2139 switch (_hash) { 2140 case -1724546052: /*description*/ return new Property("description", "markdown", "Human-readable summary of the estimate.", 0, 1, description); 2141 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnote or explanatory note about the estimate.", 0, java.lang.Integer.MAX_VALUE, note); 2142 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of attribute estimate, e.g., confidence interval or p value.", 0, 1, type); 2143 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.", 0, 1, quantity); 2144 case 102865796: /*level*/ return new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level); 2145 case 108280125: /*range*/ return new Property("range", "Range", "Lower bound of confidence interval.", 0, 1, range); 2146 case -1539581980: /*attributeEstimate*/ return new Property("attributeEstimate", "@Evidence.statistic.attributeEstimate", "A nested attribute estimate; which is the attribute estimate of an attribute estimate.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate); 2147 default: return super.getNamedProperty(_hash, _name, _checkValid); 2148 } 2149 2150 } 2151 2152 @Override 2153 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2154 switch (hash) { 2155 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2156 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2157 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2158 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 2159 case 102865796: /*level*/ return this.level == null ? new Base[0] : new Base[] {this.level}; // DecimalType 2160 case 108280125: /*range*/ return this.range == null ? new Base[0] : new Base[] {this.range}; // Range 2161 case -1539581980: /*attributeEstimate*/ return this.attributeEstimate == null ? new Base[0] : this.attributeEstimate.toArray(new Base[this.attributeEstimate.size()]); // EvidenceStatisticAttributeEstimateComponent 2162 default: return super.getProperty(hash, name, checkValid); 2163 } 2164 2165 } 2166 2167 @Override 2168 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2169 switch (hash) { 2170 case -1724546052: // description 2171 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2172 return value; 2173 case 3387378: // note 2174 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2175 return value; 2176 case 3575610: // type 2177 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2178 return value; 2179 case -1285004149: // quantity 2180 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2181 return value; 2182 case 102865796: // level 2183 this.level = TypeConvertor.castToDecimal(value); // DecimalType 2184 return value; 2185 case 108280125: // range 2186 this.range = TypeConvertor.castToRange(value); // Range 2187 return value; 2188 case -1539581980: // attributeEstimate 2189 this.getAttributeEstimate().add((EvidenceStatisticAttributeEstimateComponent) value); // EvidenceStatisticAttributeEstimateComponent 2190 return value; 2191 default: return super.setProperty(hash, name, value); 2192 } 2193 2194 } 2195 2196 @Override 2197 public Base setProperty(String name, Base value) throws FHIRException { 2198 if (name.equals("description")) { 2199 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2200 } else if (name.equals("note")) { 2201 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2202 } else if (name.equals("type")) { 2203 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2204 } else if (name.equals("quantity")) { 2205 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2206 } else if (name.equals("level")) { 2207 this.level = TypeConvertor.castToDecimal(value); // DecimalType 2208 } else if (name.equals("range")) { 2209 this.range = TypeConvertor.castToRange(value); // Range 2210 } else if (name.equals("attributeEstimate")) { 2211 this.getAttributeEstimate().add((EvidenceStatisticAttributeEstimateComponent) value); 2212 } else 2213 return super.setProperty(name, value); 2214 return value; 2215 } 2216 2217 @Override 2218 public void removeChild(String name, Base value) throws FHIRException { 2219 if (name.equals("description")) { 2220 this.description = null; 2221 } else if (name.equals("note")) { 2222 this.getNote().remove(value); 2223 } else if (name.equals("type")) { 2224 this.type = null; 2225 } else if (name.equals("quantity")) { 2226 this.quantity = null; 2227 } else if (name.equals("level")) { 2228 this.level = null; 2229 } else if (name.equals("range")) { 2230 this.range = null; 2231 } else if (name.equals("attributeEstimate")) { 2232 this.getAttributeEstimate().remove((EvidenceStatisticAttributeEstimateComponent) value); 2233 } else 2234 super.removeChild(name, value); 2235 2236 } 2237 2238 @Override 2239 public Base makeProperty(int hash, String name) throws FHIRException { 2240 switch (hash) { 2241 case -1724546052: return getDescriptionElement(); 2242 case 3387378: return addNote(); 2243 case 3575610: return getType(); 2244 case -1285004149: return getQuantity(); 2245 case 102865796: return getLevelElement(); 2246 case 108280125: return getRange(); 2247 case -1539581980: return addAttributeEstimate(); 2248 default: return super.makeProperty(hash, name); 2249 } 2250 2251 } 2252 2253 @Override 2254 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2255 switch (hash) { 2256 case -1724546052: /*description*/ return new String[] {"markdown"}; 2257 case 3387378: /*note*/ return new String[] {"Annotation"}; 2258 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2259 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 2260 case 102865796: /*level*/ return new String[] {"decimal"}; 2261 case 108280125: /*range*/ return new String[] {"Range"}; 2262 case -1539581980: /*attributeEstimate*/ return new String[] {"@Evidence.statistic.attributeEstimate"}; 2263 default: return super.getTypesForProperty(hash, name); 2264 } 2265 2266 } 2267 2268 @Override 2269 public Base addChild(String name) throws FHIRException { 2270 if (name.equals("description")) { 2271 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.attributeEstimate.description"); 2272 } 2273 else if (name.equals("note")) { 2274 return addNote(); 2275 } 2276 else if (name.equals("type")) { 2277 this.type = new CodeableConcept(); 2278 return this.type; 2279 } 2280 else if (name.equals("quantity")) { 2281 this.quantity = new Quantity(); 2282 return this.quantity; 2283 } 2284 else if (name.equals("level")) { 2285 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.attributeEstimate.level"); 2286 } 2287 else if (name.equals("range")) { 2288 this.range = new Range(); 2289 return this.range; 2290 } 2291 else if (name.equals("attributeEstimate")) { 2292 return addAttributeEstimate(); 2293 } 2294 else 2295 return super.addChild(name); 2296 } 2297 2298 public EvidenceStatisticAttributeEstimateComponent copy() { 2299 EvidenceStatisticAttributeEstimateComponent dst = new EvidenceStatisticAttributeEstimateComponent(); 2300 copyValues(dst); 2301 return dst; 2302 } 2303 2304 public void copyValues(EvidenceStatisticAttributeEstimateComponent dst) { 2305 super.copyValues(dst); 2306 dst.description = description == null ? null : description.copy(); 2307 if (note != null) { 2308 dst.note = new ArrayList<Annotation>(); 2309 for (Annotation i : note) 2310 dst.note.add(i.copy()); 2311 }; 2312 dst.type = type == null ? null : type.copy(); 2313 dst.quantity = quantity == null ? null : quantity.copy(); 2314 dst.level = level == null ? null : level.copy(); 2315 dst.range = range == null ? null : range.copy(); 2316 if (attributeEstimate != null) { 2317 dst.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2318 for (EvidenceStatisticAttributeEstimateComponent i : attributeEstimate) 2319 dst.attributeEstimate.add(i.copy()); 2320 }; 2321 } 2322 2323 @Override 2324 public boolean equalsDeep(Base other_) { 2325 if (!super.equalsDeep(other_)) 2326 return false; 2327 if (!(other_ instanceof EvidenceStatisticAttributeEstimateComponent)) 2328 return false; 2329 EvidenceStatisticAttributeEstimateComponent o = (EvidenceStatisticAttributeEstimateComponent) other_; 2330 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(type, o.type, true) 2331 && compareDeep(quantity, o.quantity, true) && compareDeep(level, o.level, true) && compareDeep(range, o.range, true) 2332 && compareDeep(attributeEstimate, o.attributeEstimate, true); 2333 } 2334 2335 @Override 2336 public boolean equalsShallow(Base other_) { 2337 if (!super.equalsShallow(other_)) 2338 return false; 2339 if (!(other_ instanceof EvidenceStatisticAttributeEstimateComponent)) 2340 return false; 2341 EvidenceStatisticAttributeEstimateComponent o = (EvidenceStatisticAttributeEstimateComponent) other_; 2342 return compareValues(description, o.description, true) && compareValues(level, o.level, true); 2343 } 2344 2345 public boolean isEmpty() { 2346 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, type 2347 , quantity, level, range, attributeEstimate); 2348 } 2349 2350 public String fhirType() { 2351 return "Evidence.statistic.attributeEstimate"; 2352 2353 } 2354 2355 } 2356 2357 @Block() 2358 public static class EvidenceStatisticModelCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 2359 /** 2360 * Description of a component of the method to generate the statistic. 2361 */ 2362 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2363 @Description(shortDefinition="Model specification", formalDefinition="Description of a component of the method to generate the statistic." ) 2364 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/statistic-model-code") 2365 protected CodeableConcept code; 2366 2367 /** 2368 * Further specification of the quantified value of the component of the method to generate the statistic. 2369 */ 2370 @Child(name = "value", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 2371 @Description(shortDefinition="Numerical value to complete model specification", formalDefinition="Further specification of the quantified value of the component of the method to generate the statistic." ) 2372 protected Quantity value; 2373 2374 /** 2375 * A variable adjusted for in the adjusted analysis. 2376 */ 2377 @Child(name = "variable", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2378 @Description(shortDefinition="A variable adjusted for in the adjusted analysis", formalDefinition="A variable adjusted for in the adjusted analysis." ) 2379 protected List<EvidenceStatisticModelCharacteristicVariableComponent> variable; 2380 2381 /** 2382 * An attribute of the statistic used as a model characteristic. 2383 */ 2384 @Child(name = "attributeEstimate", type = {EvidenceStatisticAttributeEstimateComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2385 @Description(shortDefinition="An attribute of the statistic used as a model characteristic", formalDefinition="An attribute of the statistic used as a model characteristic." ) 2386 protected List<EvidenceStatisticAttributeEstimateComponent> attributeEstimate; 2387 2388 private static final long serialVersionUID = 1787793468L; 2389 2390 /** 2391 * Constructor 2392 */ 2393 public EvidenceStatisticModelCharacteristicComponent() { 2394 super(); 2395 } 2396 2397 /** 2398 * Constructor 2399 */ 2400 public EvidenceStatisticModelCharacteristicComponent(CodeableConcept code) { 2401 super(); 2402 this.setCode(code); 2403 } 2404 2405 /** 2406 * @return {@link #code} (Description of a component of the method to generate the statistic.) 2407 */ 2408 public CodeableConcept getCode() { 2409 if (this.code == null) 2410 if (Configuration.errorOnAutoCreate()) 2411 throw new Error("Attempt to auto-create EvidenceStatisticModelCharacteristicComponent.code"); 2412 else if (Configuration.doAutoCreate()) 2413 this.code = new CodeableConcept(); // cc 2414 return this.code; 2415 } 2416 2417 public boolean hasCode() { 2418 return this.code != null && !this.code.isEmpty(); 2419 } 2420 2421 /** 2422 * @param value {@link #code} (Description of a component of the method to generate the statistic.) 2423 */ 2424 public EvidenceStatisticModelCharacteristicComponent setCode(CodeableConcept value) { 2425 this.code = value; 2426 return this; 2427 } 2428 2429 /** 2430 * @return {@link #value} (Further specification of the quantified value of the component of the method to generate the statistic.) 2431 */ 2432 public Quantity getValue() { 2433 if (this.value == null) 2434 if (Configuration.errorOnAutoCreate()) 2435 throw new Error("Attempt to auto-create EvidenceStatisticModelCharacteristicComponent.value"); 2436 else if (Configuration.doAutoCreate()) 2437 this.value = new Quantity(); // cc 2438 return this.value; 2439 } 2440 2441 public boolean hasValue() { 2442 return this.value != null && !this.value.isEmpty(); 2443 } 2444 2445 /** 2446 * @param value {@link #value} (Further specification of the quantified value of the component of the method to generate the statistic.) 2447 */ 2448 public EvidenceStatisticModelCharacteristicComponent setValue(Quantity value) { 2449 this.value = value; 2450 return this; 2451 } 2452 2453 /** 2454 * @return {@link #variable} (A variable adjusted for in the adjusted analysis.) 2455 */ 2456 public List<EvidenceStatisticModelCharacteristicVariableComponent> getVariable() { 2457 if (this.variable == null) 2458 this.variable = new ArrayList<EvidenceStatisticModelCharacteristicVariableComponent>(); 2459 return this.variable; 2460 } 2461 2462 /** 2463 * @return Returns a reference to <code>this</code> for easy method chaining 2464 */ 2465 public EvidenceStatisticModelCharacteristicComponent setVariable(List<EvidenceStatisticModelCharacteristicVariableComponent> theVariable) { 2466 this.variable = theVariable; 2467 return this; 2468 } 2469 2470 public boolean hasVariable() { 2471 if (this.variable == null) 2472 return false; 2473 for (EvidenceStatisticModelCharacteristicVariableComponent item : this.variable) 2474 if (!item.isEmpty()) 2475 return true; 2476 return false; 2477 } 2478 2479 public EvidenceStatisticModelCharacteristicVariableComponent addVariable() { //3 2480 EvidenceStatisticModelCharacteristicVariableComponent t = new EvidenceStatisticModelCharacteristicVariableComponent(); 2481 if (this.variable == null) 2482 this.variable = new ArrayList<EvidenceStatisticModelCharacteristicVariableComponent>(); 2483 this.variable.add(t); 2484 return t; 2485 } 2486 2487 public EvidenceStatisticModelCharacteristicComponent addVariable(EvidenceStatisticModelCharacteristicVariableComponent t) { //3 2488 if (t == null) 2489 return this; 2490 if (this.variable == null) 2491 this.variable = new ArrayList<EvidenceStatisticModelCharacteristicVariableComponent>(); 2492 this.variable.add(t); 2493 return this; 2494 } 2495 2496 /** 2497 * @return The first repetition of repeating field {@link #variable}, creating it if it does not already exist {3} 2498 */ 2499 public EvidenceStatisticModelCharacteristicVariableComponent getVariableFirstRep() { 2500 if (getVariable().isEmpty()) { 2501 addVariable(); 2502 } 2503 return getVariable().get(0); 2504 } 2505 2506 /** 2507 * @return {@link #attributeEstimate} (An attribute of the statistic used as a model characteristic.) 2508 */ 2509 public List<EvidenceStatisticAttributeEstimateComponent> getAttributeEstimate() { 2510 if (this.attributeEstimate == null) 2511 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2512 return this.attributeEstimate; 2513 } 2514 2515 /** 2516 * @return Returns a reference to <code>this</code> for easy method chaining 2517 */ 2518 public EvidenceStatisticModelCharacteristicComponent setAttributeEstimate(List<EvidenceStatisticAttributeEstimateComponent> theAttributeEstimate) { 2519 this.attributeEstimate = theAttributeEstimate; 2520 return this; 2521 } 2522 2523 public boolean hasAttributeEstimate() { 2524 if (this.attributeEstimate == null) 2525 return false; 2526 for (EvidenceStatisticAttributeEstimateComponent item : this.attributeEstimate) 2527 if (!item.isEmpty()) 2528 return true; 2529 return false; 2530 } 2531 2532 public EvidenceStatisticAttributeEstimateComponent addAttributeEstimate() { //3 2533 EvidenceStatisticAttributeEstimateComponent t = new EvidenceStatisticAttributeEstimateComponent(); 2534 if (this.attributeEstimate == null) 2535 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2536 this.attributeEstimate.add(t); 2537 return t; 2538 } 2539 2540 public EvidenceStatisticModelCharacteristicComponent addAttributeEstimate(EvidenceStatisticAttributeEstimateComponent t) { //3 2541 if (t == null) 2542 return this; 2543 if (this.attributeEstimate == null) 2544 this.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2545 this.attributeEstimate.add(t); 2546 return this; 2547 } 2548 2549 /** 2550 * @return The first repetition of repeating field {@link #attributeEstimate}, creating it if it does not already exist {3} 2551 */ 2552 public EvidenceStatisticAttributeEstimateComponent getAttributeEstimateFirstRep() { 2553 if (getAttributeEstimate().isEmpty()) { 2554 addAttributeEstimate(); 2555 } 2556 return getAttributeEstimate().get(0); 2557 } 2558 2559 protected void listChildren(List<Property> children) { 2560 super.listChildren(children); 2561 children.add(new Property("code", "CodeableConcept", "Description of a component of the method to generate the statistic.", 0, 1, code)); 2562 children.add(new Property("value", "Quantity", "Further specification of the quantified value of the component of the method to generate the statistic.", 0, 1, value)); 2563 children.add(new Property("variable", "", "A variable adjusted for in the adjusted analysis.", 0, java.lang.Integer.MAX_VALUE, variable)); 2564 children.add(new Property("attributeEstimate", "@Evidence.statistic.attributeEstimate", "An attribute of the statistic used as a model characteristic.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate)); 2565 } 2566 2567 @Override 2568 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2569 switch (_hash) { 2570 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Description of a component of the method to generate the statistic.", 0, 1, code); 2571 case 111972721: /*value*/ return new Property("value", "Quantity", "Further specification of the quantified value of the component of the method to generate the statistic.", 0, 1, value); 2572 case -1249586564: /*variable*/ return new Property("variable", "", "A variable adjusted for in the adjusted analysis.", 0, java.lang.Integer.MAX_VALUE, variable); 2573 case -1539581980: /*attributeEstimate*/ return new Property("attributeEstimate", "@Evidence.statistic.attributeEstimate", "An attribute of the statistic used as a model characteristic.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate); 2574 default: return super.getNamedProperty(_hash, _name, _checkValid); 2575 } 2576 2577 } 2578 2579 @Override 2580 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2581 switch (hash) { 2582 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2583 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Quantity 2584 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : this.variable.toArray(new Base[this.variable.size()]); // EvidenceStatisticModelCharacteristicVariableComponent 2585 case -1539581980: /*attributeEstimate*/ return this.attributeEstimate == null ? new Base[0] : this.attributeEstimate.toArray(new Base[this.attributeEstimate.size()]); // EvidenceStatisticAttributeEstimateComponent 2586 default: return super.getProperty(hash, name, checkValid); 2587 } 2588 2589 } 2590 2591 @Override 2592 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2593 switch (hash) { 2594 case 3059181: // code 2595 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2596 return value; 2597 case 111972721: // value 2598 this.value = TypeConvertor.castToQuantity(value); // Quantity 2599 return value; 2600 case -1249586564: // variable 2601 this.getVariable().add((EvidenceStatisticModelCharacteristicVariableComponent) value); // EvidenceStatisticModelCharacteristicVariableComponent 2602 return value; 2603 case -1539581980: // attributeEstimate 2604 this.getAttributeEstimate().add((EvidenceStatisticAttributeEstimateComponent) value); // EvidenceStatisticAttributeEstimateComponent 2605 return value; 2606 default: return super.setProperty(hash, name, value); 2607 } 2608 2609 } 2610 2611 @Override 2612 public Base setProperty(String name, Base value) throws FHIRException { 2613 if (name.equals("code")) { 2614 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2615 } else if (name.equals("value")) { 2616 this.value = TypeConvertor.castToQuantity(value); // Quantity 2617 } else if (name.equals("variable")) { 2618 this.getVariable().add((EvidenceStatisticModelCharacteristicVariableComponent) value); 2619 } else if (name.equals("attributeEstimate")) { 2620 this.getAttributeEstimate().add((EvidenceStatisticAttributeEstimateComponent) value); 2621 } else 2622 return super.setProperty(name, value); 2623 return value; 2624 } 2625 2626 @Override 2627 public void removeChild(String name, Base value) throws FHIRException { 2628 if (name.equals("code")) { 2629 this.code = null; 2630 } else if (name.equals("value")) { 2631 this.value = null; 2632 } else if (name.equals("variable")) { 2633 this.getVariable().remove((EvidenceStatisticModelCharacteristicVariableComponent) value); 2634 } else if (name.equals("attributeEstimate")) { 2635 this.getAttributeEstimate().remove((EvidenceStatisticAttributeEstimateComponent) value); 2636 } else 2637 super.removeChild(name, value); 2638 2639 } 2640 2641 @Override 2642 public Base makeProperty(int hash, String name) throws FHIRException { 2643 switch (hash) { 2644 case 3059181: return getCode(); 2645 case 111972721: return getValue(); 2646 case -1249586564: return addVariable(); 2647 case -1539581980: return addAttributeEstimate(); 2648 default: return super.makeProperty(hash, name); 2649 } 2650 2651 } 2652 2653 @Override 2654 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2655 switch (hash) { 2656 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2657 case 111972721: /*value*/ return new String[] {"Quantity"}; 2658 case -1249586564: /*variable*/ return new String[] {}; 2659 case -1539581980: /*attributeEstimate*/ return new String[] {"@Evidence.statistic.attributeEstimate"}; 2660 default: return super.getTypesForProperty(hash, name); 2661 } 2662 2663 } 2664 2665 @Override 2666 public Base addChild(String name) throws FHIRException { 2667 if (name.equals("code")) { 2668 this.code = new CodeableConcept(); 2669 return this.code; 2670 } 2671 else if (name.equals("value")) { 2672 this.value = new Quantity(); 2673 return this.value; 2674 } 2675 else if (name.equals("variable")) { 2676 return addVariable(); 2677 } 2678 else if (name.equals("attributeEstimate")) { 2679 return addAttributeEstimate(); 2680 } 2681 else 2682 return super.addChild(name); 2683 } 2684 2685 public EvidenceStatisticModelCharacteristicComponent copy() { 2686 EvidenceStatisticModelCharacteristicComponent dst = new EvidenceStatisticModelCharacteristicComponent(); 2687 copyValues(dst); 2688 return dst; 2689 } 2690 2691 public void copyValues(EvidenceStatisticModelCharacteristicComponent dst) { 2692 super.copyValues(dst); 2693 dst.code = code == null ? null : code.copy(); 2694 dst.value = value == null ? null : value.copy(); 2695 if (variable != null) { 2696 dst.variable = new ArrayList<EvidenceStatisticModelCharacteristicVariableComponent>(); 2697 for (EvidenceStatisticModelCharacteristicVariableComponent i : variable) 2698 dst.variable.add(i.copy()); 2699 }; 2700 if (attributeEstimate != null) { 2701 dst.attributeEstimate = new ArrayList<EvidenceStatisticAttributeEstimateComponent>(); 2702 for (EvidenceStatisticAttributeEstimateComponent i : attributeEstimate) 2703 dst.attributeEstimate.add(i.copy()); 2704 }; 2705 } 2706 2707 @Override 2708 public boolean equalsDeep(Base other_) { 2709 if (!super.equalsDeep(other_)) 2710 return false; 2711 if (!(other_ instanceof EvidenceStatisticModelCharacteristicComponent)) 2712 return false; 2713 EvidenceStatisticModelCharacteristicComponent o = (EvidenceStatisticModelCharacteristicComponent) other_; 2714 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(variable, o.variable, true) 2715 && compareDeep(attributeEstimate, o.attributeEstimate, true); 2716 } 2717 2718 @Override 2719 public boolean equalsShallow(Base other_) { 2720 if (!super.equalsShallow(other_)) 2721 return false; 2722 if (!(other_ instanceof EvidenceStatisticModelCharacteristicComponent)) 2723 return false; 2724 EvidenceStatisticModelCharacteristicComponent o = (EvidenceStatisticModelCharacteristicComponent) other_; 2725 return true; 2726 } 2727 2728 public boolean isEmpty() { 2729 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, variable, attributeEstimate 2730 ); 2731 } 2732 2733 public String fhirType() { 2734 return "Evidence.statistic.modelCharacteristic"; 2735 2736 } 2737 2738 } 2739 2740 @Block() 2741 public static class EvidenceStatisticModelCharacteristicVariableComponent extends BackboneElement implements IBaseBackboneElement { 2742 /** 2743 * Description of the variable. 2744 */ 2745 @Child(name = "variableDefinition", type = {Group.class, EvidenceVariable.class}, order=1, min=1, max=1, modifier=false, summary=false) 2746 @Description(shortDefinition="Description of the variable", formalDefinition="Description of the variable." ) 2747 protected Reference variableDefinition; 2748 2749 /** 2750 * How the variable is classified for use in adjusted analysis. 2751 */ 2752 @Child(name = "handling", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2753 @Description(shortDefinition="continuous | dichotomous | ordinal | polychotomous", formalDefinition="How the variable is classified for use in adjusted analysis." ) 2754 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/variable-handling") 2755 protected Enumeration<EvidenceVariableHandling> handling; 2756 2757 /** 2758 * Description for grouping of ordinal or polychotomous variables. 2759 */ 2760 @Child(name = "valueCategory", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2761 @Description(shortDefinition="Description for grouping of ordinal or polychotomous variables", formalDefinition="Description for grouping of ordinal or polychotomous variables." ) 2762 protected List<CodeableConcept> valueCategory; 2763 2764 /** 2765 * Discrete value for grouping of ordinal or polychotomous variables. 2766 */ 2767 @Child(name = "valueQuantity", type = {Quantity.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2768 @Description(shortDefinition="Discrete value for grouping of ordinal or polychotomous variables", formalDefinition="Discrete value for grouping of ordinal or polychotomous variables." ) 2769 protected List<Quantity> valueQuantity; 2770 2771 /** 2772 * Range of values for grouping of ordinal or polychotomous variables. 2773 */ 2774 @Child(name = "valueRange", type = {Range.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2775 @Description(shortDefinition="Range of values for grouping of ordinal or polychotomous variables", formalDefinition="Range of values for grouping of ordinal or polychotomous variables." ) 2776 protected List<Range> valueRange; 2777 2778 private static final long serialVersionUID = 1516174900L; 2779 2780 /** 2781 * Constructor 2782 */ 2783 public EvidenceStatisticModelCharacteristicVariableComponent() { 2784 super(); 2785 } 2786 2787 /** 2788 * Constructor 2789 */ 2790 public EvidenceStatisticModelCharacteristicVariableComponent(Reference variableDefinition) { 2791 super(); 2792 this.setVariableDefinition(variableDefinition); 2793 } 2794 2795 /** 2796 * @return {@link #variableDefinition} (Description of the variable.) 2797 */ 2798 public Reference getVariableDefinition() { 2799 if (this.variableDefinition == null) 2800 if (Configuration.errorOnAutoCreate()) 2801 throw new Error("Attempt to auto-create EvidenceStatisticModelCharacteristicVariableComponent.variableDefinition"); 2802 else if (Configuration.doAutoCreate()) 2803 this.variableDefinition = new Reference(); // cc 2804 return this.variableDefinition; 2805 } 2806 2807 public boolean hasVariableDefinition() { 2808 return this.variableDefinition != null && !this.variableDefinition.isEmpty(); 2809 } 2810 2811 /** 2812 * @param value {@link #variableDefinition} (Description of the variable.) 2813 */ 2814 public EvidenceStatisticModelCharacteristicVariableComponent setVariableDefinition(Reference value) { 2815 this.variableDefinition = value; 2816 return this; 2817 } 2818 2819 /** 2820 * @return {@link #handling} (How the variable is classified for use in adjusted analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 2821 */ 2822 public Enumeration<EvidenceVariableHandling> getHandlingElement() { 2823 if (this.handling == null) 2824 if (Configuration.errorOnAutoCreate()) 2825 throw new Error("Attempt to auto-create EvidenceStatisticModelCharacteristicVariableComponent.handling"); 2826 else if (Configuration.doAutoCreate()) 2827 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); // bb 2828 return this.handling; 2829 } 2830 2831 public boolean hasHandlingElement() { 2832 return this.handling != null && !this.handling.isEmpty(); 2833 } 2834 2835 public boolean hasHandling() { 2836 return this.handling != null && !this.handling.isEmpty(); 2837 } 2838 2839 /** 2840 * @param value {@link #handling} (How the variable is classified for use in adjusted analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 2841 */ 2842 public EvidenceStatisticModelCharacteristicVariableComponent setHandlingElement(Enumeration<EvidenceVariableHandling> value) { 2843 this.handling = value; 2844 return this; 2845 } 2846 2847 /** 2848 * @return How the variable is classified for use in adjusted analysis. 2849 */ 2850 public EvidenceVariableHandling getHandling() { 2851 return this.handling == null ? null : this.handling.getValue(); 2852 } 2853 2854 /** 2855 * @param value How the variable is classified for use in adjusted analysis. 2856 */ 2857 public EvidenceStatisticModelCharacteristicVariableComponent setHandling(EvidenceVariableHandling value) { 2858 if (value == null) 2859 this.handling = null; 2860 else { 2861 if (this.handling == null) 2862 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); 2863 this.handling.setValue(value); 2864 } 2865 return this; 2866 } 2867 2868 /** 2869 * @return {@link #valueCategory} (Description for grouping of ordinal or polychotomous variables.) 2870 */ 2871 public List<CodeableConcept> getValueCategory() { 2872 if (this.valueCategory == null) 2873 this.valueCategory = new ArrayList<CodeableConcept>(); 2874 return this.valueCategory; 2875 } 2876 2877 /** 2878 * @return Returns a reference to <code>this</code> for easy method chaining 2879 */ 2880 public EvidenceStatisticModelCharacteristicVariableComponent setValueCategory(List<CodeableConcept> theValueCategory) { 2881 this.valueCategory = theValueCategory; 2882 return this; 2883 } 2884 2885 public boolean hasValueCategory() { 2886 if (this.valueCategory == null) 2887 return false; 2888 for (CodeableConcept item : this.valueCategory) 2889 if (!item.isEmpty()) 2890 return true; 2891 return false; 2892 } 2893 2894 public CodeableConcept addValueCategory() { //3 2895 CodeableConcept t = new CodeableConcept(); 2896 if (this.valueCategory == null) 2897 this.valueCategory = new ArrayList<CodeableConcept>(); 2898 this.valueCategory.add(t); 2899 return t; 2900 } 2901 2902 public EvidenceStatisticModelCharacteristicVariableComponent addValueCategory(CodeableConcept t) { //3 2903 if (t == null) 2904 return this; 2905 if (this.valueCategory == null) 2906 this.valueCategory = new ArrayList<CodeableConcept>(); 2907 this.valueCategory.add(t); 2908 return this; 2909 } 2910 2911 /** 2912 * @return The first repetition of repeating field {@link #valueCategory}, creating it if it does not already exist {3} 2913 */ 2914 public CodeableConcept getValueCategoryFirstRep() { 2915 if (getValueCategory().isEmpty()) { 2916 addValueCategory(); 2917 } 2918 return getValueCategory().get(0); 2919 } 2920 2921 /** 2922 * @return {@link #valueQuantity} (Discrete value for grouping of ordinal or polychotomous variables.) 2923 */ 2924 public List<Quantity> getValueQuantity() { 2925 if (this.valueQuantity == null) 2926 this.valueQuantity = new ArrayList<Quantity>(); 2927 return this.valueQuantity; 2928 } 2929 2930 /** 2931 * @return Returns a reference to <code>this</code> for easy method chaining 2932 */ 2933 public EvidenceStatisticModelCharacteristicVariableComponent setValueQuantity(List<Quantity> theValueQuantity) { 2934 this.valueQuantity = theValueQuantity; 2935 return this; 2936 } 2937 2938 public boolean hasValueQuantity() { 2939 if (this.valueQuantity == null) 2940 return false; 2941 for (Quantity item : this.valueQuantity) 2942 if (!item.isEmpty()) 2943 return true; 2944 return false; 2945 } 2946 2947 public Quantity addValueQuantity() { //3 2948 Quantity t = new Quantity(); 2949 if (this.valueQuantity == null) 2950 this.valueQuantity = new ArrayList<Quantity>(); 2951 this.valueQuantity.add(t); 2952 return t; 2953 } 2954 2955 public EvidenceStatisticModelCharacteristicVariableComponent addValueQuantity(Quantity t) { //3 2956 if (t == null) 2957 return this; 2958 if (this.valueQuantity == null) 2959 this.valueQuantity = new ArrayList<Quantity>(); 2960 this.valueQuantity.add(t); 2961 return this; 2962 } 2963 2964 /** 2965 * @return The first repetition of repeating field {@link #valueQuantity}, creating it if it does not already exist {3} 2966 */ 2967 public Quantity getValueQuantityFirstRep() { 2968 if (getValueQuantity().isEmpty()) { 2969 addValueQuantity(); 2970 } 2971 return getValueQuantity().get(0); 2972 } 2973 2974 /** 2975 * @return {@link #valueRange} (Range of values for grouping of ordinal or polychotomous variables.) 2976 */ 2977 public List<Range> getValueRange() { 2978 if (this.valueRange == null) 2979 this.valueRange = new ArrayList<Range>(); 2980 return this.valueRange; 2981 } 2982 2983 /** 2984 * @return Returns a reference to <code>this</code> for easy method chaining 2985 */ 2986 public EvidenceStatisticModelCharacteristicVariableComponent setValueRange(List<Range> theValueRange) { 2987 this.valueRange = theValueRange; 2988 return this; 2989 } 2990 2991 public boolean hasValueRange() { 2992 if (this.valueRange == null) 2993 return false; 2994 for (Range item : this.valueRange) 2995 if (!item.isEmpty()) 2996 return true; 2997 return false; 2998 } 2999 3000 public Range addValueRange() { //3 3001 Range t = new Range(); 3002 if (this.valueRange == null) 3003 this.valueRange = new ArrayList<Range>(); 3004 this.valueRange.add(t); 3005 return t; 3006 } 3007 3008 public EvidenceStatisticModelCharacteristicVariableComponent addValueRange(Range t) { //3 3009 if (t == null) 3010 return this; 3011 if (this.valueRange == null) 3012 this.valueRange = new ArrayList<Range>(); 3013 this.valueRange.add(t); 3014 return this; 3015 } 3016 3017 /** 3018 * @return The first repetition of repeating field {@link #valueRange}, creating it if it does not already exist {3} 3019 */ 3020 public Range getValueRangeFirstRep() { 3021 if (getValueRange().isEmpty()) { 3022 addValueRange(); 3023 } 3024 return getValueRange().get(0); 3025 } 3026 3027 protected void listChildren(List<Property> children) { 3028 super.listChildren(children); 3029 children.add(new Property("variableDefinition", "Reference(Group|EvidenceVariable)", "Description of the variable.", 0, 1, variableDefinition)); 3030 children.add(new Property("handling", "code", "How the variable is classified for use in adjusted analysis.", 0, 1, handling)); 3031 children.add(new Property("valueCategory", "CodeableConcept", "Description for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueCategory)); 3032 children.add(new Property("valueQuantity", "Quantity", "Discrete value for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueQuantity)); 3033 children.add(new Property("valueRange", "Range", "Range of values for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueRange)); 3034 } 3035 3036 @Override 3037 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3038 switch (_hash) { 3039 case -1807222545: /*variableDefinition*/ return new Property("variableDefinition", "Reference(Group|EvidenceVariable)", "Description of the variable.", 0, 1, variableDefinition); 3040 case 2072805: /*handling*/ return new Property("handling", "code", "How the variable is classified for use in adjusted analysis.", 0, 1, handling); 3041 case -694308465: /*valueCategory*/ return new Property("valueCategory", "CodeableConcept", "Description for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueCategory); 3042 case -2029823716: /*valueQuantity*/ return new Property("valueQuantity", "Quantity", "Discrete value for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueQuantity); 3043 case 2030761548: /*valueRange*/ return new Property("valueRange", "Range", "Range of values for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueRange); 3044 default: return super.getNamedProperty(_hash, _name, _checkValid); 3045 } 3046 3047 } 3048 3049 @Override 3050 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3051 switch (hash) { 3052 case -1807222545: /*variableDefinition*/ return this.variableDefinition == null ? new Base[0] : new Base[] {this.variableDefinition}; // Reference 3053 case 2072805: /*handling*/ return this.handling == null ? new Base[0] : new Base[] {this.handling}; // Enumeration<EvidenceVariableHandling> 3054 case -694308465: /*valueCategory*/ return this.valueCategory == null ? new Base[0] : this.valueCategory.toArray(new Base[this.valueCategory.size()]); // CodeableConcept 3055 case -2029823716: /*valueQuantity*/ return this.valueQuantity == null ? new Base[0] : this.valueQuantity.toArray(new Base[this.valueQuantity.size()]); // Quantity 3056 case 2030761548: /*valueRange*/ return this.valueRange == null ? new Base[0] : this.valueRange.toArray(new Base[this.valueRange.size()]); // Range 3057 default: return super.getProperty(hash, name, checkValid); 3058 } 3059 3060 } 3061 3062 @Override 3063 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3064 switch (hash) { 3065 case -1807222545: // variableDefinition 3066 this.variableDefinition = TypeConvertor.castToReference(value); // Reference 3067 return value; 3068 case 2072805: // handling 3069 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 3070 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 3071 return value; 3072 case -694308465: // valueCategory 3073 this.getValueCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3074 return value; 3075 case -2029823716: // valueQuantity 3076 this.getValueQuantity().add(TypeConvertor.castToQuantity(value)); // Quantity 3077 return value; 3078 case 2030761548: // valueRange 3079 this.getValueRange().add(TypeConvertor.castToRange(value)); // Range 3080 return value; 3081 default: return super.setProperty(hash, name, value); 3082 } 3083 3084 } 3085 3086 @Override 3087 public Base setProperty(String name, Base value) throws FHIRException { 3088 if (name.equals("variableDefinition")) { 3089 this.variableDefinition = TypeConvertor.castToReference(value); // Reference 3090 } else if (name.equals("handling")) { 3091 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 3092 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 3093 } else if (name.equals("valueCategory")) { 3094 this.getValueCategory().add(TypeConvertor.castToCodeableConcept(value)); 3095 } else if (name.equals("valueQuantity")) { 3096 this.getValueQuantity().add(TypeConvertor.castToQuantity(value)); 3097 } else if (name.equals("valueRange")) { 3098 this.getValueRange().add(TypeConvertor.castToRange(value)); 3099 } else 3100 return super.setProperty(name, value); 3101 return value; 3102 } 3103 3104 @Override 3105 public void removeChild(String name, Base value) throws FHIRException { 3106 if (name.equals("variableDefinition")) { 3107 this.variableDefinition = null; 3108 } else if (name.equals("handling")) { 3109 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 3110 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 3111 } else if (name.equals("valueCategory")) { 3112 this.getValueCategory().remove(value); 3113 } else if (name.equals("valueQuantity")) { 3114 this.getValueQuantity().remove(value); 3115 } else if (name.equals("valueRange")) { 3116 this.getValueRange().remove(value); 3117 } else 3118 super.removeChild(name, value); 3119 3120 } 3121 3122 @Override 3123 public Base makeProperty(int hash, String name) throws FHIRException { 3124 switch (hash) { 3125 case -1807222545: return getVariableDefinition(); 3126 case 2072805: return getHandlingElement(); 3127 case -694308465: return addValueCategory(); 3128 case -2029823716: return addValueQuantity(); 3129 case 2030761548: return addValueRange(); 3130 default: return super.makeProperty(hash, name); 3131 } 3132 3133 } 3134 3135 @Override 3136 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3137 switch (hash) { 3138 case -1807222545: /*variableDefinition*/ return new String[] {"Reference"}; 3139 case 2072805: /*handling*/ return new String[] {"code"}; 3140 case -694308465: /*valueCategory*/ return new String[] {"CodeableConcept"}; 3141 case -2029823716: /*valueQuantity*/ return new String[] {"Quantity"}; 3142 case 2030761548: /*valueRange*/ return new String[] {"Range"}; 3143 default: return super.getTypesForProperty(hash, name); 3144 } 3145 3146 } 3147 3148 @Override 3149 public Base addChild(String name) throws FHIRException { 3150 if (name.equals("variableDefinition")) { 3151 this.variableDefinition = new Reference(); 3152 return this.variableDefinition; 3153 } 3154 else if (name.equals("handling")) { 3155 throw new FHIRException("Cannot call addChild on a singleton property Evidence.statistic.modelCharacteristic.variable.handling"); 3156 } 3157 else if (name.equals("valueCategory")) { 3158 return addValueCategory(); 3159 } 3160 else if (name.equals("valueQuantity")) { 3161 return addValueQuantity(); 3162 } 3163 else if (name.equals("valueRange")) { 3164 return addValueRange(); 3165 } 3166 else 3167 return super.addChild(name); 3168 } 3169 3170 public EvidenceStatisticModelCharacteristicVariableComponent copy() { 3171 EvidenceStatisticModelCharacteristicVariableComponent dst = new EvidenceStatisticModelCharacteristicVariableComponent(); 3172 copyValues(dst); 3173 return dst; 3174 } 3175 3176 public void copyValues(EvidenceStatisticModelCharacteristicVariableComponent dst) { 3177 super.copyValues(dst); 3178 dst.variableDefinition = variableDefinition == null ? null : variableDefinition.copy(); 3179 dst.handling = handling == null ? null : handling.copy(); 3180 if (valueCategory != null) { 3181 dst.valueCategory = new ArrayList<CodeableConcept>(); 3182 for (CodeableConcept i : valueCategory) 3183 dst.valueCategory.add(i.copy()); 3184 }; 3185 if (valueQuantity != null) { 3186 dst.valueQuantity = new ArrayList<Quantity>(); 3187 for (Quantity i : valueQuantity) 3188 dst.valueQuantity.add(i.copy()); 3189 }; 3190 if (valueRange != null) { 3191 dst.valueRange = new ArrayList<Range>(); 3192 for (Range i : valueRange) 3193 dst.valueRange.add(i.copy()); 3194 }; 3195 } 3196 3197 @Override 3198 public boolean equalsDeep(Base other_) { 3199 if (!super.equalsDeep(other_)) 3200 return false; 3201 if (!(other_ instanceof EvidenceStatisticModelCharacteristicVariableComponent)) 3202 return false; 3203 EvidenceStatisticModelCharacteristicVariableComponent o = (EvidenceStatisticModelCharacteristicVariableComponent) other_; 3204 return compareDeep(variableDefinition, o.variableDefinition, true) && compareDeep(handling, o.handling, true) 3205 && compareDeep(valueCategory, o.valueCategory, true) && compareDeep(valueQuantity, o.valueQuantity, true) 3206 && compareDeep(valueRange, o.valueRange, true); 3207 } 3208 3209 @Override 3210 public boolean equalsShallow(Base other_) { 3211 if (!super.equalsShallow(other_)) 3212 return false; 3213 if (!(other_ instanceof EvidenceStatisticModelCharacteristicVariableComponent)) 3214 return false; 3215 EvidenceStatisticModelCharacteristicVariableComponent o = (EvidenceStatisticModelCharacteristicVariableComponent) other_; 3216 return compareValues(handling, o.handling, true); 3217 } 3218 3219 public boolean isEmpty() { 3220 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(variableDefinition, handling 3221 , valueCategory, valueQuantity, valueRange); 3222 } 3223 3224 public String fhirType() { 3225 return "Evidence.statistic.modelCharacteristic.variable"; 3226 3227 } 3228 3229 } 3230 3231 @Block() 3232 public static class EvidenceCertaintyComponent extends BackboneElement implements IBaseBackboneElement { 3233 /** 3234 * Textual description of certainty. 3235 */ 3236 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3237 @Description(shortDefinition="Textual description of certainty", formalDefinition="Textual description of certainty." ) 3238 protected MarkdownType description; 3239 3240 /** 3241 * Footnotes and/or explanatory notes. 3242 */ 3243 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3244 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Footnotes and/or explanatory notes." ) 3245 protected List<Annotation> note; 3246 3247 /** 3248 * Aspect of certainty being rated. 3249 */ 3250 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 3251 @Description(shortDefinition="Aspect of certainty being rated", formalDefinition="Aspect of certainty being rated." ) 3252 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/certainty-type") 3253 protected CodeableConcept type; 3254 3255 /** 3256 * Assessment or judgement of the aspect. 3257 */ 3258 @Child(name = "rating", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 3259 @Description(shortDefinition="Assessment or judgement of the aspect", formalDefinition="Assessment or judgement of the aspect." ) 3260 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/certainty-rating") 3261 protected CodeableConcept rating; 3262 3263 /** 3264 * Individual or group who did the rating. 3265 */ 3266 @Child(name = "rater", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 3267 @Description(shortDefinition="Individual or group who did the rating", formalDefinition="Individual or group who did the rating." ) 3268 protected StringType rater; 3269 3270 /** 3271 * A domain or subdomain of certainty. 3272 */ 3273 @Child(name = "subcomponent", type = {EvidenceCertaintyComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3274 @Description(shortDefinition="A domain or subdomain of certainty", formalDefinition="A domain or subdomain of certainty." ) 3275 protected List<EvidenceCertaintyComponent> subcomponent; 3276 3277 private static final long serialVersionUID = -139300414L; 3278 3279 /** 3280 * Constructor 3281 */ 3282 public EvidenceCertaintyComponent() { 3283 super(); 3284 } 3285 3286 /** 3287 * @return {@link #description} (Textual description of certainty.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3288 */ 3289 public MarkdownType getDescriptionElement() { 3290 if (this.description == null) 3291 if (Configuration.errorOnAutoCreate()) 3292 throw new Error("Attempt to auto-create EvidenceCertaintyComponent.description"); 3293 else if (Configuration.doAutoCreate()) 3294 this.description = new MarkdownType(); // bb 3295 return this.description; 3296 } 3297 3298 public boolean hasDescriptionElement() { 3299 return this.description != null && !this.description.isEmpty(); 3300 } 3301 3302 public boolean hasDescription() { 3303 return this.description != null && !this.description.isEmpty(); 3304 } 3305 3306 /** 3307 * @param value {@link #description} (Textual description of certainty.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3308 */ 3309 public EvidenceCertaintyComponent setDescriptionElement(MarkdownType value) { 3310 this.description = value; 3311 return this; 3312 } 3313 3314 /** 3315 * @return Textual description of certainty. 3316 */ 3317 public String getDescription() { 3318 return this.description == null ? null : this.description.getValue(); 3319 } 3320 3321 /** 3322 * @param value Textual description of certainty. 3323 */ 3324 public EvidenceCertaintyComponent setDescription(String value) { 3325 if (Utilities.noString(value)) 3326 this.description = null; 3327 else { 3328 if (this.description == null) 3329 this.description = new MarkdownType(); 3330 this.description.setValue(value); 3331 } 3332 return this; 3333 } 3334 3335 /** 3336 * @return {@link #note} (Footnotes and/or explanatory notes.) 3337 */ 3338 public List<Annotation> getNote() { 3339 if (this.note == null) 3340 this.note = new ArrayList<Annotation>(); 3341 return this.note; 3342 } 3343 3344 /** 3345 * @return Returns a reference to <code>this</code> for easy method chaining 3346 */ 3347 public EvidenceCertaintyComponent setNote(List<Annotation> theNote) { 3348 this.note = theNote; 3349 return this; 3350 } 3351 3352 public boolean hasNote() { 3353 if (this.note == null) 3354 return false; 3355 for (Annotation item : this.note) 3356 if (!item.isEmpty()) 3357 return true; 3358 return false; 3359 } 3360 3361 public Annotation addNote() { //3 3362 Annotation t = new Annotation(); 3363 if (this.note == null) 3364 this.note = new ArrayList<Annotation>(); 3365 this.note.add(t); 3366 return t; 3367 } 3368 3369 public EvidenceCertaintyComponent addNote(Annotation t) { //3 3370 if (t == null) 3371 return this; 3372 if (this.note == null) 3373 this.note = new ArrayList<Annotation>(); 3374 this.note.add(t); 3375 return this; 3376 } 3377 3378 /** 3379 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3380 */ 3381 public Annotation getNoteFirstRep() { 3382 if (getNote().isEmpty()) { 3383 addNote(); 3384 } 3385 return getNote().get(0); 3386 } 3387 3388 /** 3389 * @return {@link #type} (Aspect of certainty being rated.) 3390 */ 3391 public CodeableConcept getType() { 3392 if (this.type == null) 3393 if (Configuration.errorOnAutoCreate()) 3394 throw new Error("Attempt to auto-create EvidenceCertaintyComponent.type"); 3395 else if (Configuration.doAutoCreate()) 3396 this.type = new CodeableConcept(); // cc 3397 return this.type; 3398 } 3399 3400 public boolean hasType() { 3401 return this.type != null && !this.type.isEmpty(); 3402 } 3403 3404 /** 3405 * @param value {@link #type} (Aspect of certainty being rated.) 3406 */ 3407 public EvidenceCertaintyComponent setType(CodeableConcept value) { 3408 this.type = value; 3409 return this; 3410 } 3411 3412 /** 3413 * @return {@link #rating} (Assessment or judgement of the aspect.) 3414 */ 3415 public CodeableConcept getRating() { 3416 if (this.rating == null) 3417 if (Configuration.errorOnAutoCreate()) 3418 throw new Error("Attempt to auto-create EvidenceCertaintyComponent.rating"); 3419 else if (Configuration.doAutoCreate()) 3420 this.rating = new CodeableConcept(); // cc 3421 return this.rating; 3422 } 3423 3424 public boolean hasRating() { 3425 return this.rating != null && !this.rating.isEmpty(); 3426 } 3427 3428 /** 3429 * @param value {@link #rating} (Assessment or judgement of the aspect.) 3430 */ 3431 public EvidenceCertaintyComponent setRating(CodeableConcept value) { 3432 this.rating = value; 3433 return this; 3434 } 3435 3436 /** 3437 * @return {@link #rater} (Individual or group who did the rating.). This is the underlying object with id, value and extensions. The accessor "getRater" gives direct access to the value 3438 */ 3439 public StringType getRaterElement() { 3440 if (this.rater == null) 3441 if (Configuration.errorOnAutoCreate()) 3442 throw new Error("Attempt to auto-create EvidenceCertaintyComponent.rater"); 3443 else if (Configuration.doAutoCreate()) 3444 this.rater = new StringType(); // bb 3445 return this.rater; 3446 } 3447 3448 public boolean hasRaterElement() { 3449 return this.rater != null && !this.rater.isEmpty(); 3450 } 3451 3452 public boolean hasRater() { 3453 return this.rater != null && !this.rater.isEmpty(); 3454 } 3455 3456 /** 3457 * @param value {@link #rater} (Individual or group who did the rating.). This is the underlying object with id, value and extensions. The accessor "getRater" gives direct access to the value 3458 */ 3459 public EvidenceCertaintyComponent setRaterElement(StringType value) { 3460 this.rater = value; 3461 return this; 3462 } 3463 3464 /** 3465 * @return Individual or group who did the rating. 3466 */ 3467 public String getRater() { 3468 return this.rater == null ? null : this.rater.getValue(); 3469 } 3470 3471 /** 3472 * @param value Individual or group who did the rating. 3473 */ 3474 public EvidenceCertaintyComponent setRater(String value) { 3475 if (Utilities.noString(value)) 3476 this.rater = null; 3477 else { 3478 if (this.rater == null) 3479 this.rater = new StringType(); 3480 this.rater.setValue(value); 3481 } 3482 return this; 3483 } 3484 3485 /** 3486 * @return {@link #subcomponent} (A domain or subdomain of certainty.) 3487 */ 3488 public List<EvidenceCertaintyComponent> getSubcomponent() { 3489 if (this.subcomponent == null) 3490 this.subcomponent = new ArrayList<EvidenceCertaintyComponent>(); 3491 return this.subcomponent; 3492 } 3493 3494 /** 3495 * @return Returns a reference to <code>this</code> for easy method chaining 3496 */ 3497 public EvidenceCertaintyComponent setSubcomponent(List<EvidenceCertaintyComponent> theSubcomponent) { 3498 this.subcomponent = theSubcomponent; 3499 return this; 3500 } 3501 3502 public boolean hasSubcomponent() { 3503 if (this.subcomponent == null) 3504 return false; 3505 for (EvidenceCertaintyComponent item : this.subcomponent) 3506 if (!item.isEmpty()) 3507 return true; 3508 return false; 3509 } 3510 3511 public EvidenceCertaintyComponent addSubcomponent() { //3 3512 EvidenceCertaintyComponent t = new EvidenceCertaintyComponent(); 3513 if (this.subcomponent == null) 3514 this.subcomponent = new ArrayList<EvidenceCertaintyComponent>(); 3515 this.subcomponent.add(t); 3516 return t; 3517 } 3518 3519 public EvidenceCertaintyComponent addSubcomponent(EvidenceCertaintyComponent t) { //3 3520 if (t == null) 3521 return this; 3522 if (this.subcomponent == null) 3523 this.subcomponent = new ArrayList<EvidenceCertaintyComponent>(); 3524 this.subcomponent.add(t); 3525 return this; 3526 } 3527 3528 /** 3529 * @return The first repetition of repeating field {@link #subcomponent}, creating it if it does not already exist {3} 3530 */ 3531 public EvidenceCertaintyComponent getSubcomponentFirstRep() { 3532 if (getSubcomponent().isEmpty()) { 3533 addSubcomponent(); 3534 } 3535 return getSubcomponent().get(0); 3536 } 3537 3538 protected void listChildren(List<Property> children) { 3539 super.listChildren(children); 3540 children.add(new Property("description", "markdown", "Textual description of certainty.", 0, 1, description)); 3541 children.add(new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note)); 3542 children.add(new Property("type", "CodeableConcept", "Aspect of certainty being rated.", 0, 1, type)); 3543 children.add(new Property("rating", "CodeableConcept", "Assessment or judgement of the aspect.", 0, 1, rating)); 3544 children.add(new Property("rater", "string", "Individual or group who did the rating.", 0, 1, rater)); 3545 children.add(new Property("subcomponent", "@Evidence.certainty", "A domain or subdomain of certainty.", 0, java.lang.Integer.MAX_VALUE, subcomponent)); 3546 } 3547 3548 @Override 3549 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3550 switch (_hash) { 3551 case -1724546052: /*description*/ return new Property("description", "markdown", "Textual description of certainty.", 0, 1, description); 3552 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note); 3553 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Aspect of certainty being rated.", 0, 1, type); 3554 case -938102371: /*rating*/ return new Property("rating", "CodeableConcept", "Assessment or judgement of the aspect.", 0, 1, rating); 3555 case 108285842: /*rater*/ return new Property("rater", "string", "Individual or group who did the rating.", 0, 1, rater); 3556 case -1308662083: /*subcomponent*/ return new Property("subcomponent", "@Evidence.certainty", "A domain or subdomain of certainty.", 0, java.lang.Integer.MAX_VALUE, subcomponent); 3557 default: return super.getNamedProperty(_hash, _name, _checkValid); 3558 } 3559 3560 } 3561 3562 @Override 3563 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3564 switch (hash) { 3565 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3566 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3567 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3568 case -938102371: /*rating*/ return this.rating == null ? new Base[0] : new Base[] {this.rating}; // CodeableConcept 3569 case 108285842: /*rater*/ return this.rater == null ? new Base[0] : new Base[] {this.rater}; // StringType 3570 case -1308662083: /*subcomponent*/ return this.subcomponent == null ? new Base[0] : this.subcomponent.toArray(new Base[this.subcomponent.size()]); // EvidenceCertaintyComponent 3571 default: return super.getProperty(hash, name, checkValid); 3572 } 3573 3574 } 3575 3576 @Override 3577 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3578 switch (hash) { 3579 case -1724546052: // description 3580 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3581 return value; 3582 case 3387378: // note 3583 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3584 return value; 3585 case 3575610: // type 3586 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3587 return value; 3588 case -938102371: // rating 3589 this.rating = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3590 return value; 3591 case 108285842: // rater 3592 this.rater = TypeConvertor.castToString(value); // StringType 3593 return value; 3594 case -1308662083: // subcomponent 3595 this.getSubcomponent().add((EvidenceCertaintyComponent) value); // EvidenceCertaintyComponent 3596 return value; 3597 default: return super.setProperty(hash, name, value); 3598 } 3599 3600 } 3601 3602 @Override 3603 public Base setProperty(String name, Base value) throws FHIRException { 3604 if (name.equals("description")) { 3605 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3606 } else if (name.equals("note")) { 3607 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3608 } else if (name.equals("type")) { 3609 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3610 } else if (name.equals("rating")) { 3611 this.rating = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3612 } else if (name.equals("rater")) { 3613 this.rater = TypeConvertor.castToString(value); // StringType 3614 } else if (name.equals("subcomponent")) { 3615 this.getSubcomponent().add((EvidenceCertaintyComponent) value); 3616 } else 3617 return super.setProperty(name, value); 3618 return value; 3619 } 3620 3621 @Override 3622 public void removeChild(String name, Base value) throws FHIRException { 3623 if (name.equals("description")) { 3624 this.description = null; 3625 } else if (name.equals("note")) { 3626 this.getNote().remove(value); 3627 } else if (name.equals("type")) { 3628 this.type = null; 3629 } else if (name.equals("rating")) { 3630 this.rating = null; 3631 } else if (name.equals("rater")) { 3632 this.rater = null; 3633 } else if (name.equals("subcomponent")) { 3634 this.getSubcomponent().remove((EvidenceCertaintyComponent) value); 3635 } else 3636 super.removeChild(name, value); 3637 3638 } 3639 3640 @Override 3641 public Base makeProperty(int hash, String name) throws FHIRException { 3642 switch (hash) { 3643 case -1724546052: return getDescriptionElement(); 3644 case 3387378: return addNote(); 3645 case 3575610: return getType(); 3646 case -938102371: return getRating(); 3647 case 108285842: return getRaterElement(); 3648 case -1308662083: return addSubcomponent(); 3649 default: return super.makeProperty(hash, name); 3650 } 3651 3652 } 3653 3654 @Override 3655 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3656 switch (hash) { 3657 case -1724546052: /*description*/ return new String[] {"markdown"}; 3658 case 3387378: /*note*/ return new String[] {"Annotation"}; 3659 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3660 case -938102371: /*rating*/ return new String[] {"CodeableConcept"}; 3661 case 108285842: /*rater*/ return new String[] {"string"}; 3662 case -1308662083: /*subcomponent*/ return new String[] {"@Evidence.certainty"}; 3663 default: return super.getTypesForProperty(hash, name); 3664 } 3665 3666 } 3667 3668 @Override 3669 public Base addChild(String name) throws FHIRException { 3670 if (name.equals("description")) { 3671 throw new FHIRException("Cannot call addChild on a singleton property Evidence.certainty.description"); 3672 } 3673 else if (name.equals("note")) { 3674 return addNote(); 3675 } 3676 else if (name.equals("type")) { 3677 this.type = new CodeableConcept(); 3678 return this.type; 3679 } 3680 else if (name.equals("rating")) { 3681 this.rating = new CodeableConcept(); 3682 return this.rating; 3683 } 3684 else if (name.equals("rater")) { 3685 throw new FHIRException("Cannot call addChild on a singleton property Evidence.certainty.rater"); 3686 } 3687 else if (name.equals("subcomponent")) { 3688 return addSubcomponent(); 3689 } 3690 else 3691 return super.addChild(name); 3692 } 3693 3694 public EvidenceCertaintyComponent copy() { 3695 EvidenceCertaintyComponent dst = new EvidenceCertaintyComponent(); 3696 copyValues(dst); 3697 return dst; 3698 } 3699 3700 public void copyValues(EvidenceCertaintyComponent dst) { 3701 super.copyValues(dst); 3702 dst.description = description == null ? null : description.copy(); 3703 if (note != null) { 3704 dst.note = new ArrayList<Annotation>(); 3705 for (Annotation i : note) 3706 dst.note.add(i.copy()); 3707 }; 3708 dst.type = type == null ? null : type.copy(); 3709 dst.rating = rating == null ? null : rating.copy(); 3710 dst.rater = rater == null ? null : rater.copy(); 3711 if (subcomponent != null) { 3712 dst.subcomponent = new ArrayList<EvidenceCertaintyComponent>(); 3713 for (EvidenceCertaintyComponent i : subcomponent) 3714 dst.subcomponent.add(i.copy()); 3715 }; 3716 } 3717 3718 @Override 3719 public boolean equalsDeep(Base other_) { 3720 if (!super.equalsDeep(other_)) 3721 return false; 3722 if (!(other_ instanceof EvidenceCertaintyComponent)) 3723 return false; 3724 EvidenceCertaintyComponent o = (EvidenceCertaintyComponent) other_; 3725 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(type, o.type, true) 3726 && compareDeep(rating, o.rating, true) && compareDeep(rater, o.rater, true) && compareDeep(subcomponent, o.subcomponent, true) 3727 ; 3728 } 3729 3730 @Override 3731 public boolean equalsShallow(Base other_) { 3732 if (!super.equalsShallow(other_)) 3733 return false; 3734 if (!(other_ instanceof EvidenceCertaintyComponent)) 3735 return false; 3736 EvidenceCertaintyComponent o = (EvidenceCertaintyComponent) other_; 3737 return compareValues(description, o.description, true) && compareValues(rater, o.rater, true); 3738 } 3739 3740 public boolean isEmpty() { 3741 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, type 3742 , rating, rater, subcomponent); 3743 } 3744 3745 public String fhirType() { 3746 return "Evidence.certainty"; 3747 3748 } 3749 3750 } 3751 3752 /** 3753 * An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 3754 */ 3755 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 3756 @Description(shortDefinition="Canonical identifier for this evidence, represented as a globally unique URI", formalDefinition="An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers." ) 3757 protected UriType url; 3758 3759 /** 3760 * A formal identifier that is used to identify this summary when it is represented in other formats, or referenced in a specification, model, design or an instance. 3761 */ 3762 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3763 @Description(shortDefinition="Additional identifier for the summary", formalDefinition="A formal identifier that is used to identify this summary when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 3764 protected List<Identifier> identifier; 3765 3766 /** 3767 * The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3768 */ 3769 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3770 @Description(shortDefinition="Business version of this summary", formalDefinition="The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 3771 protected StringType version; 3772 3773 /** 3774 * Indicates the mechanism used to compare versions to determine which is more current. 3775 */ 3776 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 3777 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 3778 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 3779 protected DataType versionAlgorithm; 3780 3781 /** 3782 * A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3783 */ 3784 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3785 @Description(shortDefinition="Name for this summary (machine friendly)", formalDefinition="A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 3786 protected StringType name; 3787 3788 /** 3789 * A short, descriptive, user-friendly title for the summary. 3790 */ 3791 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3792 @Description(shortDefinition="Name for this summary (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the summary." ) 3793 protected StringType title; 3794 3795 /** 3796 * Citation Resource or display of suggested citation for this evidence. 3797 */ 3798 @Child(name = "citeAs", type = {Citation.class, MarkdownType.class}, order=6, min=0, max=1, modifier=false, summary=false) 3799 @Description(shortDefinition="Citation for this evidence", formalDefinition="Citation Resource or display of suggested citation for this evidence." ) 3800 protected DataType citeAs; 3801 3802 /** 3803 * The status of this summary. Enables tracking the life-cycle of the content. 3804 */ 3805 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 3806 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this summary. Enables tracking the life-cycle of the content." ) 3807 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 3808 protected Enumeration<PublicationStatus> status; 3809 3810 /** 3811 * A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3812 */ 3813 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=false) 3814 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 3815 protected BooleanType experimental; 3816 3817 /** 3818 * The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes. 3819 */ 3820 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 3821 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes." ) 3822 protected DateTimeType date; 3823 3824 /** 3825 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3826 */ 3827 @Child(name = "approvalDate", type = {DateType.class}, order=10, min=0, max=1, modifier=false, summary=false) 3828 @Description(shortDefinition="When the summary was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 3829 protected DateType approvalDate; 3830 3831 /** 3832 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3833 */ 3834 @Child(name = "lastReviewDate", type = {DateType.class}, order=11, min=0, max=1, modifier=false, summary=false) 3835 @Description(shortDefinition="When the summary was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 3836 protected DateType lastReviewDate; 3837 3838 /** 3839 * The name of the organization or individual responsible for the release and ongoing maintenance of the evidence. 3840 */ 3841 @Child(name = "publisher", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 3842 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the evidence." ) 3843 protected StringType publisher; 3844 3845 /** 3846 * Contact details to assist a user in finding and communicating with the publisher. 3847 */ 3848 @Child(name = "contact", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3849 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 3850 protected List<ContactDetail> contact; 3851 3852 /** 3853 * An individiual, organization, or device primarily involved in the creation and maintenance of the content. 3854 */ 3855 @Child(name = "author", type = {ContactDetail.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3856 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual, organization, or device primarily involved in the creation and maintenance of the content." ) 3857 protected List<ContactDetail> author; 3858 3859 /** 3860 * An individiual, organization, or device primarily responsible for internal coherence of the content. 3861 */ 3862 @Child(name = "editor", type = {ContactDetail.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3863 @Description(shortDefinition="Who edited the content", formalDefinition="An individiual, organization, or device primarily responsible for internal coherence of the content." ) 3864 protected List<ContactDetail> editor; 3865 3866 /** 3867 * An individiual, organization, or device primarily responsible for review of some aspect of the content. 3868 */ 3869 @Child(name = "reviewer", type = {ContactDetail.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3870 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individiual, organization, or device primarily responsible for review of some aspect of the content." ) 3871 protected List<ContactDetail> reviewer; 3872 3873 /** 3874 * An individiual, organization, or device responsible for officially endorsing the content for use in some setting. 3875 */ 3876 @Child(name = "endorser", type = {ContactDetail.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3877 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individiual, organization, or device responsible for officially endorsing the content for use in some setting." ) 3878 protected List<ContactDetail> endorser; 3879 3880 /** 3881 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence instances. 3882 */ 3883 @Child(name = "useContext", type = {UsageContext.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3884 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence instances." ) 3885 protected List<UsageContext> useContext; 3886 3887 /** 3888 * Explanation of why this Evidence is needed and why it has been designed as it has. 3889 */ 3890 @Child(name = "purpose", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3891 @Description(shortDefinition="Why this Evidence is defined", formalDefinition="Explanation of why this Evidence is needed and why it has been designed as it has." ) 3892 protected MarkdownType purpose; 3893 3894 /** 3895 * A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence. 3896 */ 3897 @Child(name = "copyright", type = {MarkdownType.class}, order=20, min=0, max=1, modifier=false, summary=false) 3898 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence." ) 3899 protected MarkdownType copyright; 3900 3901 /** 3902 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3903 */ 3904 @Child(name = "copyrightLabel", type = {StringType.class}, order=21, min=0, max=1, modifier=false, summary=false) 3905 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 3906 protected StringType copyrightLabel; 3907 3908 /** 3909 * Link or citation to artifact associated with the summary. 3910 */ 3911 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3912 @Description(shortDefinition="Link or citation to artifact associated with the summary", formalDefinition="Link or citation to artifact associated with the summary." ) 3913 protected List<RelatedArtifact> relatedArtifact; 3914 3915 /** 3916 * A free text natural language description of the evidence from a consumer's perspective. 3917 */ 3918 @Child(name = "description", type = {MarkdownType.class}, order=23, min=0, max=1, modifier=false, summary=false) 3919 @Description(shortDefinition="Description of the particular summary", formalDefinition="A free text natural language description of the evidence from a consumer's perspective." ) 3920 protected MarkdownType description; 3921 3922 /** 3923 * Declarative description of the Evidence. 3924 */ 3925 @Child(name = "assertion", type = {MarkdownType.class}, order=24, min=0, max=1, modifier=false, summary=false) 3926 @Description(shortDefinition="Declarative description of the Evidence", formalDefinition="Declarative description of the Evidence." ) 3927 protected MarkdownType assertion; 3928 3929 /** 3930 * Footnotes and/or explanatory notes. 3931 */ 3932 @Child(name = "note", type = {Annotation.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3933 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Footnotes and/or explanatory notes." ) 3934 protected List<Annotation> note; 3935 3936 /** 3937 * Evidence variable such as population, exposure, or outcome. 3938 */ 3939 @Child(name = "variableDefinition", type = {}, order=26, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3940 @Description(shortDefinition="Evidence variable such as population, exposure, or outcome", formalDefinition="Evidence variable such as population, exposure, or outcome." ) 3941 protected List<EvidenceVariableDefinitionComponent> variableDefinition; 3942 3943 /** 3944 * The method to combine studies. 3945 */ 3946 @Child(name = "synthesisType", type = {CodeableConcept.class}, order=27, min=0, max=1, modifier=false, summary=false) 3947 @Description(shortDefinition="The method to combine studies", formalDefinition="The method to combine studies." ) 3948 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/synthesis-type") 3949 protected CodeableConcept synthesisType; 3950 3951 /** 3952 * The design of the study that produced this evidence. The design is described with any number of study design characteristics. 3953 */ 3954 @Child(name = "studyDesign", type = {CodeableConcept.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3955 @Description(shortDefinition="The design of the study that produced this evidence", formalDefinition="The design of the study that produced this evidence. The design is described with any number of study design characteristics." ) 3956 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/study-design") 3957 protected List<CodeableConcept> studyDesign; 3958 3959 /** 3960 * Values and parameters for a single statistic. 3961 */ 3962 @Child(name = "statistic", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3963 @Description(shortDefinition="Values and parameters for a single statistic", formalDefinition="Values and parameters for a single statistic." ) 3964 protected List<EvidenceStatisticComponent> statistic; 3965 3966 /** 3967 * Assessment of certainty, confidence in the estimates, or quality of the evidence. 3968 */ 3969 @Child(name = "certainty", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3970 @Description(shortDefinition="Certainty or quality of the evidence", formalDefinition="Assessment of certainty, confidence in the estimates, or quality of the evidence." ) 3971 protected List<EvidenceCertaintyComponent> certainty; 3972 3973 private static final long serialVersionUID = 1427750968L; 3974 3975 /** 3976 * Constructor 3977 */ 3978 public Evidence() { 3979 super(); 3980 } 3981 3982 /** 3983 * Constructor 3984 */ 3985 public Evidence(PublicationStatus status, EvidenceVariableDefinitionComponent variableDefinition) { 3986 super(); 3987 this.setStatus(status); 3988 this.addVariableDefinition(variableDefinition); 3989 } 3990 3991 /** 3992 * @return {@link #url} (An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3993 */ 3994 public UriType getUrlElement() { 3995 if (this.url == null) 3996 if (Configuration.errorOnAutoCreate()) 3997 throw new Error("Attempt to auto-create Evidence.url"); 3998 else if (Configuration.doAutoCreate()) 3999 this.url = new UriType(); // bb 4000 return this.url; 4001 } 4002 4003 public boolean hasUrlElement() { 4004 return this.url != null && !this.url.isEmpty(); 4005 } 4006 4007 public boolean hasUrl() { 4008 return this.url != null && !this.url.isEmpty(); 4009 } 4010 4011 /** 4012 * @param value {@link #url} (An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 4013 */ 4014 public Evidence setUrlElement(UriType value) { 4015 this.url = value; 4016 return this; 4017 } 4018 4019 /** 4020 * @return An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 4021 */ 4022 public String getUrl() { 4023 return this.url == null ? null : this.url.getValue(); 4024 } 4025 4026 /** 4027 * @param value An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 4028 */ 4029 public Evidence setUrl(String value) { 4030 if (Utilities.noString(value)) 4031 this.url = null; 4032 else { 4033 if (this.url == null) 4034 this.url = new UriType(); 4035 this.url.setValue(value); 4036 } 4037 return this; 4038 } 4039 4040 /** 4041 * @return {@link #identifier} (A formal identifier that is used to identify this summary when it is represented in other formats, or referenced in a specification, model, design or an instance.) 4042 */ 4043 public List<Identifier> getIdentifier() { 4044 if (this.identifier == null) 4045 this.identifier = new ArrayList<Identifier>(); 4046 return this.identifier; 4047 } 4048 4049 /** 4050 * @return Returns a reference to <code>this</code> for easy method chaining 4051 */ 4052 public Evidence setIdentifier(List<Identifier> theIdentifier) { 4053 this.identifier = theIdentifier; 4054 return this; 4055 } 4056 4057 public boolean hasIdentifier() { 4058 if (this.identifier == null) 4059 return false; 4060 for (Identifier item : this.identifier) 4061 if (!item.isEmpty()) 4062 return true; 4063 return false; 4064 } 4065 4066 public Identifier addIdentifier() { //3 4067 Identifier t = new Identifier(); 4068 if (this.identifier == null) 4069 this.identifier = new ArrayList<Identifier>(); 4070 this.identifier.add(t); 4071 return t; 4072 } 4073 4074 public Evidence addIdentifier(Identifier t) { //3 4075 if (t == null) 4076 return this; 4077 if (this.identifier == null) 4078 this.identifier = new ArrayList<Identifier>(); 4079 this.identifier.add(t); 4080 return this; 4081 } 4082 4083 /** 4084 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 4085 */ 4086 public Identifier getIdentifierFirstRep() { 4087 if (getIdentifier().isEmpty()) { 4088 addIdentifier(); 4089 } 4090 return getIdentifier().get(0); 4091 } 4092 4093 /** 4094 * @return {@link #version} (The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 4095 */ 4096 public StringType getVersionElement() { 4097 if (this.version == null) 4098 if (Configuration.errorOnAutoCreate()) 4099 throw new Error("Attempt to auto-create Evidence.version"); 4100 else if (Configuration.doAutoCreate()) 4101 this.version = new StringType(); // bb 4102 return this.version; 4103 } 4104 4105 public boolean hasVersionElement() { 4106 return this.version != null && !this.version.isEmpty(); 4107 } 4108 4109 public boolean hasVersion() { 4110 return this.version != null && !this.version.isEmpty(); 4111 } 4112 4113 /** 4114 * @param value {@link #version} (The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 4115 */ 4116 public Evidence setVersionElement(StringType value) { 4117 this.version = value; 4118 return this; 4119 } 4120 4121 /** 4122 * @return The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 4123 */ 4124 public String getVersion() { 4125 return this.version == null ? null : this.version.getValue(); 4126 } 4127 4128 /** 4129 * @param value The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 4130 */ 4131 public Evidence setVersion(String value) { 4132 if (Utilities.noString(value)) 4133 this.version = null; 4134 else { 4135 if (this.version == null) 4136 this.version = new StringType(); 4137 this.version.setValue(value); 4138 } 4139 return this; 4140 } 4141 4142 /** 4143 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4144 */ 4145 public DataType getVersionAlgorithm() { 4146 return this.versionAlgorithm; 4147 } 4148 4149 /** 4150 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4151 */ 4152 public StringType getVersionAlgorithmStringType() throws FHIRException { 4153 if (this.versionAlgorithm == null) 4154 this.versionAlgorithm = new StringType(); 4155 if (!(this.versionAlgorithm instanceof StringType)) 4156 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4157 return (StringType) this.versionAlgorithm; 4158 } 4159 4160 public boolean hasVersionAlgorithmStringType() { 4161 return this != null && this.versionAlgorithm instanceof StringType; 4162 } 4163 4164 /** 4165 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4166 */ 4167 public Coding getVersionAlgorithmCoding() throws FHIRException { 4168 if (this.versionAlgorithm == null) 4169 this.versionAlgorithm = new Coding(); 4170 if (!(this.versionAlgorithm instanceof Coding)) 4171 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4172 return (Coding) this.versionAlgorithm; 4173 } 4174 4175 public boolean hasVersionAlgorithmCoding() { 4176 return this != null && this.versionAlgorithm instanceof Coding; 4177 } 4178 4179 public boolean hasVersionAlgorithm() { 4180 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 4181 } 4182 4183 /** 4184 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4185 */ 4186 public Evidence setVersionAlgorithm(DataType value) { 4187 if (value != null && !(value instanceof StringType || value instanceof Coding)) 4188 throw new FHIRException("Not the right type for Evidence.versionAlgorithm[x]: "+value.fhirType()); 4189 this.versionAlgorithm = value; 4190 return this; 4191 } 4192 4193 /** 4194 * @return {@link #name} (A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4195 */ 4196 public StringType getNameElement() { 4197 if (this.name == null) 4198 if (Configuration.errorOnAutoCreate()) 4199 throw new Error("Attempt to auto-create Evidence.name"); 4200 else if (Configuration.doAutoCreate()) 4201 this.name = new StringType(); // bb 4202 return this.name; 4203 } 4204 4205 public boolean hasNameElement() { 4206 return this.name != null && !this.name.isEmpty(); 4207 } 4208 4209 public boolean hasName() { 4210 return this.name != null && !this.name.isEmpty(); 4211 } 4212 4213 /** 4214 * @param value {@link #name} (A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4215 */ 4216 public Evidence setNameElement(StringType value) { 4217 this.name = value; 4218 return this; 4219 } 4220 4221 /** 4222 * @return A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation. 4223 */ 4224 public String getName() { 4225 return this.name == null ? null : this.name.getValue(); 4226 } 4227 4228 /** 4229 * @param value A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation. 4230 */ 4231 public Evidence setName(String value) { 4232 if (Utilities.noString(value)) 4233 this.name = null; 4234 else { 4235 if (this.name == null) 4236 this.name = new StringType(); 4237 this.name.setValue(value); 4238 } 4239 return this; 4240 } 4241 4242 /** 4243 * @return {@link #title} (A short, descriptive, user-friendly title for the summary.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4244 */ 4245 public StringType getTitleElement() { 4246 if (this.title == null) 4247 if (Configuration.errorOnAutoCreate()) 4248 throw new Error("Attempt to auto-create Evidence.title"); 4249 else if (Configuration.doAutoCreate()) 4250 this.title = new StringType(); // bb 4251 return this.title; 4252 } 4253 4254 public boolean hasTitleElement() { 4255 return this.title != null && !this.title.isEmpty(); 4256 } 4257 4258 public boolean hasTitle() { 4259 return this.title != null && !this.title.isEmpty(); 4260 } 4261 4262 /** 4263 * @param value {@link #title} (A short, descriptive, user-friendly title for the summary.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4264 */ 4265 public Evidence setTitleElement(StringType value) { 4266 this.title = value; 4267 return this; 4268 } 4269 4270 /** 4271 * @return A short, descriptive, user-friendly title for the summary. 4272 */ 4273 public String getTitle() { 4274 return this.title == null ? null : this.title.getValue(); 4275 } 4276 4277 /** 4278 * @param value A short, descriptive, user-friendly title for the summary. 4279 */ 4280 public Evidence setTitle(String value) { 4281 if (Utilities.noString(value)) 4282 this.title = null; 4283 else { 4284 if (this.title == null) 4285 this.title = new StringType(); 4286 this.title.setValue(value); 4287 } 4288 return this; 4289 } 4290 4291 /** 4292 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this evidence.) 4293 */ 4294 public DataType getCiteAs() { 4295 return this.citeAs; 4296 } 4297 4298 /** 4299 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this evidence.) 4300 */ 4301 public Reference getCiteAsReference() throws FHIRException { 4302 if (this.citeAs == null) 4303 this.citeAs = new Reference(); 4304 if (!(this.citeAs instanceof Reference)) 4305 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 4306 return (Reference) this.citeAs; 4307 } 4308 4309 public boolean hasCiteAsReference() { 4310 return this != null && this.citeAs instanceof Reference; 4311 } 4312 4313 /** 4314 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this evidence.) 4315 */ 4316 public MarkdownType getCiteAsMarkdownType() throws FHIRException { 4317 if (this.citeAs == null) 4318 this.citeAs = new MarkdownType(); 4319 if (!(this.citeAs instanceof MarkdownType)) 4320 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 4321 return (MarkdownType) this.citeAs; 4322 } 4323 4324 public boolean hasCiteAsMarkdownType() { 4325 return this != null && this.citeAs instanceof MarkdownType; 4326 } 4327 4328 public boolean hasCiteAs() { 4329 return this.citeAs != null && !this.citeAs.isEmpty(); 4330 } 4331 4332 /** 4333 * @param value {@link #citeAs} (Citation Resource or display of suggested citation for this evidence.) 4334 */ 4335 public Evidence setCiteAs(DataType value) { 4336 if (value != null && !(value instanceof Reference || value instanceof MarkdownType)) 4337 throw new FHIRException("Not the right type for Evidence.citeAs[x]: "+value.fhirType()); 4338 this.citeAs = value; 4339 return this; 4340 } 4341 4342 /** 4343 * @return {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4344 */ 4345 public Enumeration<PublicationStatus> getStatusElement() { 4346 if (this.status == null) 4347 if (Configuration.errorOnAutoCreate()) 4348 throw new Error("Attempt to auto-create Evidence.status"); 4349 else if (Configuration.doAutoCreate()) 4350 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 4351 return this.status; 4352 } 4353 4354 public boolean hasStatusElement() { 4355 return this.status != null && !this.status.isEmpty(); 4356 } 4357 4358 public boolean hasStatus() { 4359 return this.status != null && !this.status.isEmpty(); 4360 } 4361 4362 /** 4363 * @param value {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4364 */ 4365 public Evidence setStatusElement(Enumeration<PublicationStatus> value) { 4366 this.status = value; 4367 return this; 4368 } 4369 4370 /** 4371 * @return The status of this summary. Enables tracking the life-cycle of the content. 4372 */ 4373 public PublicationStatus getStatus() { 4374 return this.status == null ? null : this.status.getValue(); 4375 } 4376 4377 /** 4378 * @param value The status of this summary. Enables tracking the life-cycle of the content. 4379 */ 4380 public Evidence setStatus(PublicationStatus value) { 4381 if (this.status == null) 4382 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 4383 this.status.setValue(value); 4384 return this; 4385 } 4386 4387 /** 4388 * @return {@link #experimental} (A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4389 */ 4390 public BooleanType getExperimentalElement() { 4391 if (this.experimental == null) 4392 if (Configuration.errorOnAutoCreate()) 4393 throw new Error("Attempt to auto-create Evidence.experimental"); 4394 else if (Configuration.doAutoCreate()) 4395 this.experimental = new BooleanType(); // bb 4396 return this.experimental; 4397 } 4398 4399 public boolean hasExperimentalElement() { 4400 return this.experimental != null && !this.experimental.isEmpty(); 4401 } 4402 4403 public boolean hasExperimental() { 4404 return this.experimental != null && !this.experimental.isEmpty(); 4405 } 4406 4407 /** 4408 * @param value {@link #experimental} (A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4409 */ 4410 public Evidence setExperimentalElement(BooleanType value) { 4411 this.experimental = value; 4412 return this; 4413 } 4414 4415 /** 4416 * @return A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 4417 */ 4418 public boolean getExperimental() { 4419 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4420 } 4421 4422 /** 4423 * @param value A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 4424 */ 4425 public Evidence setExperimental(boolean value) { 4426 if (this.experimental == null) 4427 this.experimental = new BooleanType(); 4428 this.experimental.setValue(value); 4429 return this; 4430 } 4431 4432 /** 4433 * @return {@link #date} (The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4434 */ 4435 public DateTimeType getDateElement() { 4436 if (this.date == null) 4437 if (Configuration.errorOnAutoCreate()) 4438 throw new Error("Attempt to auto-create Evidence.date"); 4439 else if (Configuration.doAutoCreate()) 4440 this.date = new DateTimeType(); // bb 4441 return this.date; 4442 } 4443 4444 public boolean hasDateElement() { 4445 return this.date != null && !this.date.isEmpty(); 4446 } 4447 4448 public boolean hasDate() { 4449 return this.date != null && !this.date.isEmpty(); 4450 } 4451 4452 /** 4453 * @param value {@link #date} (The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4454 */ 4455 public Evidence setDateElement(DateTimeType value) { 4456 this.date = value; 4457 return this; 4458 } 4459 4460 /** 4461 * @return The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes. 4462 */ 4463 public Date getDate() { 4464 return this.date == null ? null : this.date.getValue(); 4465 } 4466 4467 /** 4468 * @param value The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes. 4469 */ 4470 public Evidence setDate(Date value) { 4471 if (value == null) 4472 this.date = null; 4473 else { 4474 if (this.date == null) 4475 this.date = new DateTimeType(); 4476 this.date.setValue(value); 4477 } 4478 return this; 4479 } 4480 4481 /** 4482 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4483 */ 4484 public DateType getApprovalDateElement() { 4485 if (this.approvalDate == null) 4486 if (Configuration.errorOnAutoCreate()) 4487 throw new Error("Attempt to auto-create Evidence.approvalDate"); 4488 else if (Configuration.doAutoCreate()) 4489 this.approvalDate = new DateType(); // bb 4490 return this.approvalDate; 4491 } 4492 4493 public boolean hasApprovalDateElement() { 4494 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4495 } 4496 4497 public boolean hasApprovalDate() { 4498 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4499 } 4500 4501 /** 4502 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4503 */ 4504 public Evidence setApprovalDateElement(DateType value) { 4505 this.approvalDate = value; 4506 return this; 4507 } 4508 4509 /** 4510 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4511 */ 4512 public Date getApprovalDate() { 4513 return this.approvalDate == null ? null : this.approvalDate.getValue(); 4514 } 4515 4516 /** 4517 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4518 */ 4519 public Evidence setApprovalDate(Date value) { 4520 if (value == null) 4521 this.approvalDate = null; 4522 else { 4523 if (this.approvalDate == null) 4524 this.approvalDate = new DateType(); 4525 this.approvalDate.setValue(value); 4526 } 4527 return this; 4528 } 4529 4530 /** 4531 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4532 */ 4533 public DateType getLastReviewDateElement() { 4534 if (this.lastReviewDate == null) 4535 if (Configuration.errorOnAutoCreate()) 4536 throw new Error("Attempt to auto-create Evidence.lastReviewDate"); 4537 else if (Configuration.doAutoCreate()) 4538 this.lastReviewDate = new DateType(); // bb 4539 return this.lastReviewDate; 4540 } 4541 4542 public boolean hasLastReviewDateElement() { 4543 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4544 } 4545 4546 public boolean hasLastReviewDate() { 4547 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4548 } 4549 4550 /** 4551 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4552 */ 4553 public Evidence setLastReviewDateElement(DateType value) { 4554 this.lastReviewDate = value; 4555 return this; 4556 } 4557 4558 /** 4559 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4560 */ 4561 public Date getLastReviewDate() { 4562 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 4563 } 4564 4565 /** 4566 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4567 */ 4568 public Evidence setLastReviewDate(Date value) { 4569 if (value == null) 4570 this.lastReviewDate = null; 4571 else { 4572 if (this.lastReviewDate == null) 4573 this.lastReviewDate = new DateType(); 4574 this.lastReviewDate.setValue(value); 4575 } 4576 return this; 4577 } 4578 4579 /** 4580 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4581 */ 4582 public StringType getPublisherElement() { 4583 if (this.publisher == null) 4584 if (Configuration.errorOnAutoCreate()) 4585 throw new Error("Attempt to auto-create Evidence.publisher"); 4586 else if (Configuration.doAutoCreate()) 4587 this.publisher = new StringType(); // bb 4588 return this.publisher; 4589 } 4590 4591 public boolean hasPublisherElement() { 4592 return this.publisher != null && !this.publisher.isEmpty(); 4593 } 4594 4595 public boolean hasPublisher() { 4596 return this.publisher != null && !this.publisher.isEmpty(); 4597 } 4598 4599 /** 4600 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4601 */ 4602 public Evidence setPublisherElement(StringType value) { 4603 this.publisher = value; 4604 return this; 4605 } 4606 4607 /** 4608 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the evidence. 4609 */ 4610 public String getPublisher() { 4611 return this.publisher == null ? null : this.publisher.getValue(); 4612 } 4613 4614 /** 4615 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the evidence. 4616 */ 4617 public Evidence setPublisher(String value) { 4618 if (Utilities.noString(value)) 4619 this.publisher = null; 4620 else { 4621 if (this.publisher == null) 4622 this.publisher = new StringType(); 4623 this.publisher.setValue(value); 4624 } 4625 return this; 4626 } 4627 4628 /** 4629 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 4630 */ 4631 public List<ContactDetail> getContact() { 4632 if (this.contact == null) 4633 this.contact = new ArrayList<ContactDetail>(); 4634 return this.contact; 4635 } 4636 4637 /** 4638 * @return Returns a reference to <code>this</code> for easy method chaining 4639 */ 4640 public Evidence setContact(List<ContactDetail> theContact) { 4641 this.contact = theContact; 4642 return this; 4643 } 4644 4645 public boolean hasContact() { 4646 if (this.contact == null) 4647 return false; 4648 for (ContactDetail item : this.contact) 4649 if (!item.isEmpty()) 4650 return true; 4651 return false; 4652 } 4653 4654 public ContactDetail addContact() { //3 4655 ContactDetail t = new ContactDetail(); 4656 if (this.contact == null) 4657 this.contact = new ArrayList<ContactDetail>(); 4658 this.contact.add(t); 4659 return t; 4660 } 4661 4662 public Evidence addContact(ContactDetail t) { //3 4663 if (t == null) 4664 return this; 4665 if (this.contact == null) 4666 this.contact = new ArrayList<ContactDetail>(); 4667 this.contact.add(t); 4668 return this; 4669 } 4670 4671 /** 4672 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 4673 */ 4674 public ContactDetail getContactFirstRep() { 4675 if (getContact().isEmpty()) { 4676 addContact(); 4677 } 4678 return getContact().get(0); 4679 } 4680 4681 /** 4682 * @return {@link #author} (An individiual, organization, or device primarily involved in the creation and maintenance of the content.) 4683 */ 4684 public List<ContactDetail> getAuthor() { 4685 if (this.author == null) 4686 this.author = new ArrayList<ContactDetail>(); 4687 return this.author; 4688 } 4689 4690 /** 4691 * @return Returns a reference to <code>this</code> for easy method chaining 4692 */ 4693 public Evidence setAuthor(List<ContactDetail> theAuthor) { 4694 this.author = theAuthor; 4695 return this; 4696 } 4697 4698 public boolean hasAuthor() { 4699 if (this.author == null) 4700 return false; 4701 for (ContactDetail item : this.author) 4702 if (!item.isEmpty()) 4703 return true; 4704 return false; 4705 } 4706 4707 public ContactDetail addAuthor() { //3 4708 ContactDetail t = new ContactDetail(); 4709 if (this.author == null) 4710 this.author = new ArrayList<ContactDetail>(); 4711 this.author.add(t); 4712 return t; 4713 } 4714 4715 public Evidence addAuthor(ContactDetail t) { //3 4716 if (t == null) 4717 return this; 4718 if (this.author == null) 4719 this.author = new ArrayList<ContactDetail>(); 4720 this.author.add(t); 4721 return this; 4722 } 4723 4724 /** 4725 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 4726 */ 4727 public ContactDetail getAuthorFirstRep() { 4728 if (getAuthor().isEmpty()) { 4729 addAuthor(); 4730 } 4731 return getAuthor().get(0); 4732 } 4733 4734 /** 4735 * @return {@link #editor} (An individiual, organization, or device primarily responsible for internal coherence of the content.) 4736 */ 4737 public List<ContactDetail> getEditor() { 4738 if (this.editor == null) 4739 this.editor = new ArrayList<ContactDetail>(); 4740 return this.editor; 4741 } 4742 4743 /** 4744 * @return Returns a reference to <code>this</code> for easy method chaining 4745 */ 4746 public Evidence setEditor(List<ContactDetail> theEditor) { 4747 this.editor = theEditor; 4748 return this; 4749 } 4750 4751 public boolean hasEditor() { 4752 if (this.editor == null) 4753 return false; 4754 for (ContactDetail item : this.editor) 4755 if (!item.isEmpty()) 4756 return true; 4757 return false; 4758 } 4759 4760 public ContactDetail addEditor() { //3 4761 ContactDetail t = new ContactDetail(); 4762 if (this.editor == null) 4763 this.editor = new ArrayList<ContactDetail>(); 4764 this.editor.add(t); 4765 return t; 4766 } 4767 4768 public Evidence addEditor(ContactDetail t) { //3 4769 if (t == null) 4770 return this; 4771 if (this.editor == null) 4772 this.editor = new ArrayList<ContactDetail>(); 4773 this.editor.add(t); 4774 return this; 4775 } 4776 4777 /** 4778 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 4779 */ 4780 public ContactDetail getEditorFirstRep() { 4781 if (getEditor().isEmpty()) { 4782 addEditor(); 4783 } 4784 return getEditor().get(0); 4785 } 4786 4787 /** 4788 * @return {@link #reviewer} (An individiual, organization, or device primarily responsible for review of some aspect of the content.) 4789 */ 4790 public List<ContactDetail> getReviewer() { 4791 if (this.reviewer == null) 4792 this.reviewer = new ArrayList<ContactDetail>(); 4793 return this.reviewer; 4794 } 4795 4796 /** 4797 * @return Returns a reference to <code>this</code> for easy method chaining 4798 */ 4799 public Evidence setReviewer(List<ContactDetail> theReviewer) { 4800 this.reviewer = theReviewer; 4801 return this; 4802 } 4803 4804 public boolean hasReviewer() { 4805 if (this.reviewer == null) 4806 return false; 4807 for (ContactDetail item : this.reviewer) 4808 if (!item.isEmpty()) 4809 return true; 4810 return false; 4811 } 4812 4813 public ContactDetail addReviewer() { //3 4814 ContactDetail t = new ContactDetail(); 4815 if (this.reviewer == null) 4816 this.reviewer = new ArrayList<ContactDetail>(); 4817 this.reviewer.add(t); 4818 return t; 4819 } 4820 4821 public Evidence addReviewer(ContactDetail t) { //3 4822 if (t == null) 4823 return this; 4824 if (this.reviewer == null) 4825 this.reviewer = new ArrayList<ContactDetail>(); 4826 this.reviewer.add(t); 4827 return this; 4828 } 4829 4830 /** 4831 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 4832 */ 4833 public ContactDetail getReviewerFirstRep() { 4834 if (getReviewer().isEmpty()) { 4835 addReviewer(); 4836 } 4837 return getReviewer().get(0); 4838 } 4839 4840 /** 4841 * @return {@link #endorser} (An individiual, organization, or device responsible for officially endorsing the content for use in some setting.) 4842 */ 4843 public List<ContactDetail> getEndorser() { 4844 if (this.endorser == null) 4845 this.endorser = new ArrayList<ContactDetail>(); 4846 return this.endorser; 4847 } 4848 4849 /** 4850 * @return Returns a reference to <code>this</code> for easy method chaining 4851 */ 4852 public Evidence setEndorser(List<ContactDetail> theEndorser) { 4853 this.endorser = theEndorser; 4854 return this; 4855 } 4856 4857 public boolean hasEndorser() { 4858 if (this.endorser == null) 4859 return false; 4860 for (ContactDetail item : this.endorser) 4861 if (!item.isEmpty()) 4862 return true; 4863 return false; 4864 } 4865 4866 public ContactDetail addEndorser() { //3 4867 ContactDetail t = new ContactDetail(); 4868 if (this.endorser == null) 4869 this.endorser = new ArrayList<ContactDetail>(); 4870 this.endorser.add(t); 4871 return t; 4872 } 4873 4874 public Evidence addEndorser(ContactDetail t) { //3 4875 if (t == null) 4876 return this; 4877 if (this.endorser == null) 4878 this.endorser = new ArrayList<ContactDetail>(); 4879 this.endorser.add(t); 4880 return this; 4881 } 4882 4883 /** 4884 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 4885 */ 4886 public ContactDetail getEndorserFirstRep() { 4887 if (getEndorser().isEmpty()) { 4888 addEndorser(); 4889 } 4890 return getEndorser().get(0); 4891 } 4892 4893 /** 4894 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence instances.) 4895 */ 4896 public List<UsageContext> getUseContext() { 4897 if (this.useContext == null) 4898 this.useContext = new ArrayList<UsageContext>(); 4899 return this.useContext; 4900 } 4901 4902 /** 4903 * @return Returns a reference to <code>this</code> for easy method chaining 4904 */ 4905 public Evidence setUseContext(List<UsageContext> theUseContext) { 4906 this.useContext = theUseContext; 4907 return this; 4908 } 4909 4910 public boolean hasUseContext() { 4911 if (this.useContext == null) 4912 return false; 4913 for (UsageContext item : this.useContext) 4914 if (!item.isEmpty()) 4915 return true; 4916 return false; 4917 } 4918 4919 public UsageContext addUseContext() { //3 4920 UsageContext t = new UsageContext(); 4921 if (this.useContext == null) 4922 this.useContext = new ArrayList<UsageContext>(); 4923 this.useContext.add(t); 4924 return t; 4925 } 4926 4927 public Evidence addUseContext(UsageContext t) { //3 4928 if (t == null) 4929 return this; 4930 if (this.useContext == null) 4931 this.useContext = new ArrayList<UsageContext>(); 4932 this.useContext.add(t); 4933 return this; 4934 } 4935 4936 /** 4937 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 4938 */ 4939 public UsageContext getUseContextFirstRep() { 4940 if (getUseContext().isEmpty()) { 4941 addUseContext(); 4942 } 4943 return getUseContext().get(0); 4944 } 4945 4946 /** 4947 * @return {@link #purpose} (Explanation of why this Evidence is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4948 */ 4949 public MarkdownType getPurposeElement() { 4950 if (this.purpose == null) 4951 if (Configuration.errorOnAutoCreate()) 4952 throw new Error("Attempt to auto-create Evidence.purpose"); 4953 else if (Configuration.doAutoCreate()) 4954 this.purpose = new MarkdownType(); // bb 4955 return this.purpose; 4956 } 4957 4958 public boolean hasPurposeElement() { 4959 return this.purpose != null && !this.purpose.isEmpty(); 4960 } 4961 4962 public boolean hasPurpose() { 4963 return this.purpose != null && !this.purpose.isEmpty(); 4964 } 4965 4966 /** 4967 * @param value {@link #purpose} (Explanation of why this Evidence is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4968 */ 4969 public Evidence setPurposeElement(MarkdownType value) { 4970 this.purpose = value; 4971 return this; 4972 } 4973 4974 /** 4975 * @return Explanation of why this Evidence is needed and why it has been designed as it has. 4976 */ 4977 public String getPurpose() { 4978 return this.purpose == null ? null : this.purpose.getValue(); 4979 } 4980 4981 /** 4982 * @param value Explanation of why this Evidence is needed and why it has been designed as it has. 4983 */ 4984 public Evidence setPurpose(String value) { 4985 if (Utilities.noString(value)) 4986 this.purpose = null; 4987 else { 4988 if (this.purpose == null) 4989 this.purpose = new MarkdownType(); 4990 this.purpose.setValue(value); 4991 } 4992 return this; 4993 } 4994 4995 /** 4996 * @return {@link #copyright} (A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4997 */ 4998 public MarkdownType getCopyrightElement() { 4999 if (this.copyright == null) 5000 if (Configuration.errorOnAutoCreate()) 5001 throw new Error("Attempt to auto-create Evidence.copyright"); 5002 else if (Configuration.doAutoCreate()) 5003 this.copyright = new MarkdownType(); // bb 5004 return this.copyright; 5005 } 5006 5007 public boolean hasCopyrightElement() { 5008 return this.copyright != null && !this.copyright.isEmpty(); 5009 } 5010 5011 public boolean hasCopyright() { 5012 return this.copyright != null && !this.copyright.isEmpty(); 5013 } 5014 5015 /** 5016 * @param value {@link #copyright} (A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 5017 */ 5018 public Evidence setCopyrightElement(MarkdownType value) { 5019 this.copyright = value; 5020 return this; 5021 } 5022 5023 /** 5024 * @return A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence. 5025 */ 5026 public String getCopyright() { 5027 return this.copyright == null ? null : this.copyright.getValue(); 5028 } 5029 5030 /** 5031 * @param value A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence. 5032 */ 5033 public Evidence setCopyright(String value) { 5034 if (Utilities.noString(value)) 5035 this.copyright = null; 5036 else { 5037 if (this.copyright == null) 5038 this.copyright = new MarkdownType(); 5039 this.copyright.setValue(value); 5040 } 5041 return this; 5042 } 5043 5044 /** 5045 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 5046 */ 5047 public StringType getCopyrightLabelElement() { 5048 if (this.copyrightLabel == null) 5049 if (Configuration.errorOnAutoCreate()) 5050 throw new Error("Attempt to auto-create Evidence.copyrightLabel"); 5051 else if (Configuration.doAutoCreate()) 5052 this.copyrightLabel = new StringType(); // bb 5053 return this.copyrightLabel; 5054 } 5055 5056 public boolean hasCopyrightLabelElement() { 5057 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 5058 } 5059 5060 public boolean hasCopyrightLabel() { 5061 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 5062 } 5063 5064 /** 5065 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 5066 */ 5067 public Evidence setCopyrightLabelElement(StringType value) { 5068 this.copyrightLabel = value; 5069 return this; 5070 } 5071 5072 /** 5073 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 5074 */ 5075 public String getCopyrightLabel() { 5076 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 5077 } 5078 5079 /** 5080 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 5081 */ 5082 public Evidence setCopyrightLabel(String value) { 5083 if (Utilities.noString(value)) 5084 this.copyrightLabel = null; 5085 else { 5086 if (this.copyrightLabel == null) 5087 this.copyrightLabel = new StringType(); 5088 this.copyrightLabel.setValue(value); 5089 } 5090 return this; 5091 } 5092 5093 /** 5094 * @return {@link #relatedArtifact} (Link or citation to artifact associated with the summary.) 5095 */ 5096 public List<RelatedArtifact> getRelatedArtifact() { 5097 if (this.relatedArtifact == null) 5098 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 5099 return this.relatedArtifact; 5100 } 5101 5102 /** 5103 * @return Returns a reference to <code>this</code> for easy method chaining 5104 */ 5105 public Evidence setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 5106 this.relatedArtifact = theRelatedArtifact; 5107 return this; 5108 } 5109 5110 public boolean hasRelatedArtifact() { 5111 if (this.relatedArtifact == null) 5112 return false; 5113 for (RelatedArtifact item : this.relatedArtifact) 5114 if (!item.isEmpty()) 5115 return true; 5116 return false; 5117 } 5118 5119 public RelatedArtifact addRelatedArtifact() { //3 5120 RelatedArtifact t = new RelatedArtifact(); 5121 if (this.relatedArtifact == null) 5122 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 5123 this.relatedArtifact.add(t); 5124 return t; 5125 } 5126 5127 public Evidence addRelatedArtifact(RelatedArtifact t) { //3 5128 if (t == null) 5129 return this; 5130 if (this.relatedArtifact == null) 5131 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 5132 this.relatedArtifact.add(t); 5133 return this; 5134 } 5135 5136 /** 5137 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 5138 */ 5139 public RelatedArtifact getRelatedArtifactFirstRep() { 5140 if (getRelatedArtifact().isEmpty()) { 5141 addRelatedArtifact(); 5142 } 5143 return getRelatedArtifact().get(0); 5144 } 5145 5146 /** 5147 * @return {@link #description} (A free text natural language description of the evidence from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5148 */ 5149 public MarkdownType getDescriptionElement() { 5150 if (this.description == null) 5151 if (Configuration.errorOnAutoCreate()) 5152 throw new Error("Attempt to auto-create Evidence.description"); 5153 else if (Configuration.doAutoCreate()) 5154 this.description = new MarkdownType(); // bb 5155 return this.description; 5156 } 5157 5158 public boolean hasDescriptionElement() { 5159 return this.description != null && !this.description.isEmpty(); 5160 } 5161 5162 public boolean hasDescription() { 5163 return this.description != null && !this.description.isEmpty(); 5164 } 5165 5166 /** 5167 * @param value {@link #description} (A free text natural language description of the evidence from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5168 */ 5169 public Evidence setDescriptionElement(MarkdownType value) { 5170 this.description = value; 5171 return this; 5172 } 5173 5174 /** 5175 * @return A free text natural language description of the evidence from a consumer's perspective. 5176 */ 5177 public String getDescription() { 5178 return this.description == null ? null : this.description.getValue(); 5179 } 5180 5181 /** 5182 * @param value A free text natural language description of the evidence from a consumer's perspective. 5183 */ 5184 public Evidence setDescription(String value) { 5185 if (Utilities.noString(value)) 5186 this.description = null; 5187 else { 5188 if (this.description == null) 5189 this.description = new MarkdownType(); 5190 this.description.setValue(value); 5191 } 5192 return this; 5193 } 5194 5195 /** 5196 * @return {@link #assertion} (Declarative description of the Evidence.). This is the underlying object with id, value and extensions. The accessor "getAssertion" gives direct access to the value 5197 */ 5198 public MarkdownType getAssertionElement() { 5199 if (this.assertion == null) 5200 if (Configuration.errorOnAutoCreate()) 5201 throw new Error("Attempt to auto-create Evidence.assertion"); 5202 else if (Configuration.doAutoCreate()) 5203 this.assertion = new MarkdownType(); // bb 5204 return this.assertion; 5205 } 5206 5207 public boolean hasAssertionElement() { 5208 return this.assertion != null && !this.assertion.isEmpty(); 5209 } 5210 5211 public boolean hasAssertion() { 5212 return this.assertion != null && !this.assertion.isEmpty(); 5213 } 5214 5215 /** 5216 * @param value {@link #assertion} (Declarative description of the Evidence.). This is the underlying object with id, value and extensions. The accessor "getAssertion" gives direct access to the value 5217 */ 5218 public Evidence setAssertionElement(MarkdownType value) { 5219 this.assertion = value; 5220 return this; 5221 } 5222 5223 /** 5224 * @return Declarative description of the Evidence. 5225 */ 5226 public String getAssertion() { 5227 return this.assertion == null ? null : this.assertion.getValue(); 5228 } 5229 5230 /** 5231 * @param value Declarative description of the Evidence. 5232 */ 5233 public Evidence setAssertion(String value) { 5234 if (Utilities.noString(value)) 5235 this.assertion = null; 5236 else { 5237 if (this.assertion == null) 5238 this.assertion = new MarkdownType(); 5239 this.assertion.setValue(value); 5240 } 5241 return this; 5242 } 5243 5244 /** 5245 * @return {@link #note} (Footnotes and/or explanatory notes.) 5246 */ 5247 public List<Annotation> getNote() { 5248 if (this.note == null) 5249 this.note = new ArrayList<Annotation>(); 5250 return this.note; 5251 } 5252 5253 /** 5254 * @return Returns a reference to <code>this</code> for easy method chaining 5255 */ 5256 public Evidence setNote(List<Annotation> theNote) { 5257 this.note = theNote; 5258 return this; 5259 } 5260 5261 public boolean hasNote() { 5262 if (this.note == null) 5263 return false; 5264 for (Annotation item : this.note) 5265 if (!item.isEmpty()) 5266 return true; 5267 return false; 5268 } 5269 5270 public Annotation addNote() { //3 5271 Annotation t = new Annotation(); 5272 if (this.note == null) 5273 this.note = new ArrayList<Annotation>(); 5274 this.note.add(t); 5275 return t; 5276 } 5277 5278 public Evidence addNote(Annotation t) { //3 5279 if (t == null) 5280 return this; 5281 if (this.note == null) 5282 this.note = new ArrayList<Annotation>(); 5283 this.note.add(t); 5284 return this; 5285 } 5286 5287 /** 5288 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 5289 */ 5290 public Annotation getNoteFirstRep() { 5291 if (getNote().isEmpty()) { 5292 addNote(); 5293 } 5294 return getNote().get(0); 5295 } 5296 5297 /** 5298 * @return {@link #variableDefinition} (Evidence variable such as population, exposure, or outcome.) 5299 */ 5300 public List<EvidenceVariableDefinitionComponent> getVariableDefinition() { 5301 if (this.variableDefinition == null) 5302 this.variableDefinition = new ArrayList<EvidenceVariableDefinitionComponent>(); 5303 return this.variableDefinition; 5304 } 5305 5306 /** 5307 * @return Returns a reference to <code>this</code> for easy method chaining 5308 */ 5309 public Evidence setVariableDefinition(List<EvidenceVariableDefinitionComponent> theVariableDefinition) { 5310 this.variableDefinition = theVariableDefinition; 5311 return this; 5312 } 5313 5314 public boolean hasVariableDefinition() { 5315 if (this.variableDefinition == null) 5316 return false; 5317 for (EvidenceVariableDefinitionComponent item : this.variableDefinition) 5318 if (!item.isEmpty()) 5319 return true; 5320 return false; 5321 } 5322 5323 public EvidenceVariableDefinitionComponent addVariableDefinition() { //3 5324 EvidenceVariableDefinitionComponent t = new EvidenceVariableDefinitionComponent(); 5325 if (this.variableDefinition == null) 5326 this.variableDefinition = new ArrayList<EvidenceVariableDefinitionComponent>(); 5327 this.variableDefinition.add(t); 5328 return t; 5329 } 5330 5331 public Evidence addVariableDefinition(EvidenceVariableDefinitionComponent t) { //3 5332 if (t == null) 5333 return this; 5334 if (this.variableDefinition == null) 5335 this.variableDefinition = new ArrayList<EvidenceVariableDefinitionComponent>(); 5336 this.variableDefinition.add(t); 5337 return this; 5338 } 5339 5340 /** 5341 * @return The first repetition of repeating field {@link #variableDefinition}, creating it if it does not already exist {3} 5342 */ 5343 public EvidenceVariableDefinitionComponent getVariableDefinitionFirstRep() { 5344 if (getVariableDefinition().isEmpty()) { 5345 addVariableDefinition(); 5346 } 5347 return getVariableDefinition().get(0); 5348 } 5349 5350 /** 5351 * @return {@link #synthesisType} (The method to combine studies.) 5352 */ 5353 public CodeableConcept getSynthesisType() { 5354 if (this.synthesisType == null) 5355 if (Configuration.errorOnAutoCreate()) 5356 throw new Error("Attempt to auto-create Evidence.synthesisType"); 5357 else if (Configuration.doAutoCreate()) 5358 this.synthesisType = new CodeableConcept(); // cc 5359 return this.synthesisType; 5360 } 5361 5362 public boolean hasSynthesisType() { 5363 return this.synthesisType != null && !this.synthesisType.isEmpty(); 5364 } 5365 5366 /** 5367 * @param value {@link #synthesisType} (The method to combine studies.) 5368 */ 5369 public Evidence setSynthesisType(CodeableConcept value) { 5370 this.synthesisType = value; 5371 return this; 5372 } 5373 5374 /** 5375 * @return {@link #studyDesign} (The design of the study that produced this evidence. The design is described with any number of study design characteristics.) 5376 */ 5377 public List<CodeableConcept> getStudyDesign() { 5378 if (this.studyDesign == null) 5379 this.studyDesign = new ArrayList<CodeableConcept>(); 5380 return this.studyDesign; 5381 } 5382 5383 /** 5384 * @return Returns a reference to <code>this</code> for easy method chaining 5385 */ 5386 public Evidence setStudyDesign(List<CodeableConcept> theStudyDesign) { 5387 this.studyDesign = theStudyDesign; 5388 return this; 5389 } 5390 5391 public boolean hasStudyDesign() { 5392 if (this.studyDesign == null) 5393 return false; 5394 for (CodeableConcept item : this.studyDesign) 5395 if (!item.isEmpty()) 5396 return true; 5397 return false; 5398 } 5399 5400 public CodeableConcept addStudyDesign() { //3 5401 CodeableConcept t = new CodeableConcept(); 5402 if (this.studyDesign == null) 5403 this.studyDesign = new ArrayList<CodeableConcept>(); 5404 this.studyDesign.add(t); 5405 return t; 5406 } 5407 5408 public Evidence addStudyDesign(CodeableConcept t) { //3 5409 if (t == null) 5410 return this; 5411 if (this.studyDesign == null) 5412 this.studyDesign = new ArrayList<CodeableConcept>(); 5413 this.studyDesign.add(t); 5414 return this; 5415 } 5416 5417 /** 5418 * @return The first repetition of repeating field {@link #studyDesign}, creating it if it does not already exist {3} 5419 */ 5420 public CodeableConcept getStudyDesignFirstRep() { 5421 if (getStudyDesign().isEmpty()) { 5422 addStudyDesign(); 5423 } 5424 return getStudyDesign().get(0); 5425 } 5426 5427 /** 5428 * @return {@link #statistic} (Values and parameters for a single statistic.) 5429 */ 5430 public List<EvidenceStatisticComponent> getStatistic() { 5431 if (this.statistic == null) 5432 this.statistic = new ArrayList<EvidenceStatisticComponent>(); 5433 return this.statistic; 5434 } 5435 5436 /** 5437 * @return Returns a reference to <code>this</code> for easy method chaining 5438 */ 5439 public Evidence setStatistic(List<EvidenceStatisticComponent> theStatistic) { 5440 this.statistic = theStatistic; 5441 return this; 5442 } 5443 5444 public boolean hasStatistic() { 5445 if (this.statistic == null) 5446 return false; 5447 for (EvidenceStatisticComponent item : this.statistic) 5448 if (!item.isEmpty()) 5449 return true; 5450 return false; 5451 } 5452 5453 public EvidenceStatisticComponent addStatistic() { //3 5454 EvidenceStatisticComponent t = new EvidenceStatisticComponent(); 5455 if (this.statistic == null) 5456 this.statistic = new ArrayList<EvidenceStatisticComponent>(); 5457 this.statistic.add(t); 5458 return t; 5459 } 5460 5461 public Evidence addStatistic(EvidenceStatisticComponent t) { //3 5462 if (t == null) 5463 return this; 5464 if (this.statistic == null) 5465 this.statistic = new ArrayList<EvidenceStatisticComponent>(); 5466 this.statistic.add(t); 5467 return this; 5468 } 5469 5470 /** 5471 * @return The first repetition of repeating field {@link #statistic}, creating it if it does not already exist {3} 5472 */ 5473 public EvidenceStatisticComponent getStatisticFirstRep() { 5474 if (getStatistic().isEmpty()) { 5475 addStatistic(); 5476 } 5477 return getStatistic().get(0); 5478 } 5479 5480 /** 5481 * @return {@link #certainty} (Assessment of certainty, confidence in the estimates, or quality of the evidence.) 5482 */ 5483 public List<EvidenceCertaintyComponent> getCertainty() { 5484 if (this.certainty == null) 5485 this.certainty = new ArrayList<EvidenceCertaintyComponent>(); 5486 return this.certainty; 5487 } 5488 5489 /** 5490 * @return Returns a reference to <code>this</code> for easy method chaining 5491 */ 5492 public Evidence setCertainty(List<EvidenceCertaintyComponent> theCertainty) { 5493 this.certainty = theCertainty; 5494 return this; 5495 } 5496 5497 public boolean hasCertainty() { 5498 if (this.certainty == null) 5499 return false; 5500 for (EvidenceCertaintyComponent item : this.certainty) 5501 if (!item.isEmpty()) 5502 return true; 5503 return false; 5504 } 5505 5506 public EvidenceCertaintyComponent addCertainty() { //3 5507 EvidenceCertaintyComponent t = new EvidenceCertaintyComponent(); 5508 if (this.certainty == null) 5509 this.certainty = new ArrayList<EvidenceCertaintyComponent>(); 5510 this.certainty.add(t); 5511 return t; 5512 } 5513 5514 public Evidence addCertainty(EvidenceCertaintyComponent t) { //3 5515 if (t == null) 5516 return this; 5517 if (this.certainty == null) 5518 this.certainty = new ArrayList<EvidenceCertaintyComponent>(); 5519 this.certainty.add(t); 5520 return this; 5521 } 5522 5523 /** 5524 * @return The first repetition of repeating field {@link #certainty}, creating it if it does not already exist {3} 5525 */ 5526 public EvidenceCertaintyComponent getCertaintyFirstRep() { 5527 if (getCertainty().isEmpty()) { 5528 addCertainty(); 5529 } 5530 return getCertainty().get(0); 5531 } 5532 5533 /** 5534 * not supported on this implementation 5535 */ 5536 @Override 5537 public int getJurisdictionMax() { 5538 return 0; 5539 } 5540 /** 5541 * @return {@link #jurisdiction} (A legal or geographic region in which the evidence is intended to be used.) 5542 */ 5543 public List<CodeableConcept> getJurisdiction() { 5544 return new ArrayList<>(); 5545 } 5546 /** 5547 * @return Returns a reference to <code>this</code> for easy method chaining 5548 */ 5549 public Evidence setJurisdiction(List<CodeableConcept> theJurisdiction) { 5550 throw new Error("The resource type \"Evidence\" does not implement the property \"jurisdiction\""); 5551 } 5552 public boolean hasJurisdiction() { 5553 return false; 5554 } 5555 5556 public CodeableConcept addJurisdiction() { //3 5557 throw new Error("The resource type \"Evidence\" does not implement the property \"jurisdiction\""); 5558 } 5559 public Evidence addJurisdiction(CodeableConcept t) { //3 5560 throw new Error("The resource type \"Evidence\" does not implement the property \"jurisdiction\""); 5561 } 5562 /** 5563 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {2} 5564 */ 5565 public CodeableConcept getJurisdictionFirstRep() { 5566 throw new Error("The resource type \"Evidence\" does not implement the property \"jurisdiction\""); 5567 } 5568 /** 5569 * not supported on this implementation 5570 */ 5571 @Override 5572 public int getEffectivePeriodMax() { 5573 return 0; 5574 } 5575 /** 5576 * @return {@link #effectivePeriod} (The period during which the evidence content was or is planned to be in active use.) 5577 */ 5578 public Period getEffectivePeriod() { 5579 throw new Error("The resource type \"Evidence\" does not implement the property \"effectivePeriod\""); 5580 } 5581 public boolean hasEffectivePeriod() { 5582 return false; 5583 } 5584 /** 5585 * @param value {@link #effectivePeriod} (The period during which the evidence content was or is planned to be in active use.) 5586 */ 5587 public Evidence setEffectivePeriod(Period value) { 5588 throw new Error("The resource type \"Evidence\" does not implement the property \"effectivePeriod\""); 5589 } 5590 5591 /** 5592 * not supported on this implementation 5593 */ 5594 @Override 5595 public int getTopicMax() { 5596 return 0; 5597 } 5598 /** 5599 * @return {@link #topic} (Descriptive topics related to the content of the evidence. Topics provide a high-level categorization as well as keywords for the evidence that can be useful for filtering and searching.) 5600 */ 5601 public List<CodeableConcept> getTopic() { 5602 return new ArrayList<>(); 5603 } 5604 /** 5605 * @return Returns a reference to <code>this</code> for easy method chaining 5606 */ 5607 public Evidence setTopic(List<CodeableConcept> theTopic) { 5608 throw new Error("The resource type \"Evidence\" does not implement the property \"topic\""); 5609 } 5610 public boolean hasTopic() { 5611 return false; 5612 } 5613 5614 public CodeableConcept addTopic() { //3 5615 throw new Error("The resource type \"Evidence\" does not implement the property \"topic\""); 5616 } 5617 public Evidence addTopic(CodeableConcept t) { //3 5618 throw new Error("The resource type \"Evidence\" does not implement the property \"topic\""); 5619 } 5620 /** 5621 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 5622 */ 5623 public CodeableConcept getTopicFirstRep() { 5624 throw new Error("The resource type \"Evidence\" does not implement the property \"topic\""); 5625 } 5626 protected void listChildren(List<Property> children) { 5627 super.listChildren(children); 5628 children.add(new Property("url", "uri", "An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url)); 5629 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this summary when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 5630 children.add(new Property("version", "string", "The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 5631 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 5632 children.add(new Property("name", "string", "A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 5633 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the summary.", 0, 1, title)); 5634 children.add(new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this evidence.", 0, 1, citeAs)); 5635 children.add(new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status)); 5636 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 5637 children.add(new Property("date", "dateTime", "The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes.", 0, 1, date)); 5638 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 5639 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 5640 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence.", 0, 1, publisher)); 5641 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 5642 children.add(new Property("author", "ContactDetail", "An individiual, organization, or device primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 5643 children.add(new Property("editor", "ContactDetail", "An individiual, organization, or device primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 5644 children.add(new Property("reviewer", "ContactDetail", "An individiual, organization, or device primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 5645 children.add(new Property("endorser", "ContactDetail", "An individiual, organization, or device responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 5646 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 5647 children.add(new Property("purpose", "markdown", "Explanation of why this Evidence is needed and why it has been designed as it has.", 0, 1, purpose)); 5648 children.add(new Property("copyright", "markdown", "A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence.", 0, 1, copyright)); 5649 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 5650 children.add(new Property("relatedArtifact", "RelatedArtifact", "Link or citation to artifact associated with the summary.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 5651 children.add(new Property("description", "markdown", "A free text natural language description of the evidence from a consumer's perspective.", 0, 1, description)); 5652 children.add(new Property("assertion", "markdown", "Declarative description of the Evidence.", 0, 1, assertion)); 5653 children.add(new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note)); 5654 children.add(new Property("variableDefinition", "", "Evidence variable such as population, exposure, or outcome.", 0, java.lang.Integer.MAX_VALUE, variableDefinition)); 5655 children.add(new Property("synthesisType", "CodeableConcept", "The method to combine studies.", 0, 1, synthesisType)); 5656 children.add(new Property("studyDesign", "CodeableConcept", "The design of the study that produced this evidence. The design is described with any number of study design characteristics.", 0, java.lang.Integer.MAX_VALUE, studyDesign)); 5657 children.add(new Property("statistic", "", "Values and parameters for a single statistic.", 0, java.lang.Integer.MAX_VALUE, statistic)); 5658 children.add(new Property("certainty", "", "Assessment of certainty, confidence in the estimates, or quality of the evidence.", 0, java.lang.Integer.MAX_VALUE, certainty)); 5659 } 5660 5661 @Override 5662 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5663 switch (_hash) { 5664 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url); 5665 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this summary when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 5666 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the summary when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the summary author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 5667 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5668 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5669 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5670 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5671 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 5672 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the summary.", 0, 1, title); 5673 case -1706539017: /*citeAs[x]*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this evidence.", 0, 1, citeAs); 5674 case -1360156695: /*citeAs*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this evidence.", 0, 1, citeAs); 5675 case 1269009762: /*citeAsReference*/ return new Property("citeAs[x]", "Reference(Citation)", "Citation Resource or display of suggested citation for this evidence.", 0, 1, citeAs); 5676 case 456265720: /*citeAsMarkdown*/ return new Property("citeAs[x]", "markdown", "Citation Resource or display of suggested citation for this evidence.", 0, 1, citeAs); 5677 case -892481550: /*status*/ return new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status); 5678 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 5679 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the summary was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the summary changes.", 0, 1, date); 5680 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 5681 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 5682 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence.", 0, 1, publisher); 5683 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 5684 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual, organization, or device primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 5685 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individiual, organization, or device primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 5686 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individiual, organization, or device primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 5687 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individiual, organization, or device responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 5688 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 5689 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this Evidence is needed and why it has been designed as it has.", 0, 1, purpose); 5690 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the Evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Evidence.", 0, 1, copyright); 5691 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 5692 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Link or citation to artifact associated with the summary.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 5693 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the evidence from a consumer's perspective.", 0, 1, description); 5694 case 1314395906: /*assertion*/ return new Property("assertion", "markdown", "Declarative description of the Evidence.", 0, 1, assertion); 5695 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note); 5696 case -1807222545: /*variableDefinition*/ return new Property("variableDefinition", "", "Evidence variable such as population, exposure, or outcome.", 0, java.lang.Integer.MAX_VALUE, variableDefinition); 5697 case 672726254: /*synthesisType*/ return new Property("synthesisType", "CodeableConcept", "The method to combine studies.", 0, 1, synthesisType); 5698 case 1709211879: /*studyDesign*/ return new Property("studyDesign", "CodeableConcept", "The design of the study that produced this evidence. The design is described with any number of study design characteristics.", 0, java.lang.Integer.MAX_VALUE, studyDesign); 5699 case -2081261232: /*statistic*/ return new Property("statistic", "", "Values and parameters for a single statistic.", 0, java.lang.Integer.MAX_VALUE, statistic); 5700 case -1404142937: /*certainty*/ return new Property("certainty", "", "Assessment of certainty, confidence in the estimates, or quality of the evidence.", 0, java.lang.Integer.MAX_VALUE, certainty); 5701 default: return super.getNamedProperty(_hash, _name, _checkValid); 5702 } 5703 5704 } 5705 5706 @Override 5707 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5708 switch (hash) { 5709 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 5710 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5711 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 5712 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 5713 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 5714 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 5715 case -1360156695: /*citeAs*/ return this.citeAs == null ? new Base[0] : new Base[] {this.citeAs}; // DataType 5716 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 5717 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 5718 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 5719 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 5720 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 5721 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 5722 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5723 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 5724 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 5725 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 5726 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 5727 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5728 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 5729 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 5730 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 5731 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5732 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 5733 case 1314395906: /*assertion*/ return this.assertion == null ? new Base[0] : new Base[] {this.assertion}; // MarkdownType 5734 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5735 case -1807222545: /*variableDefinition*/ return this.variableDefinition == null ? new Base[0] : this.variableDefinition.toArray(new Base[this.variableDefinition.size()]); // EvidenceVariableDefinitionComponent 5736 case 672726254: /*synthesisType*/ return this.synthesisType == null ? new Base[0] : new Base[] {this.synthesisType}; // CodeableConcept 5737 case 1709211879: /*studyDesign*/ return this.studyDesign == null ? new Base[0] : this.studyDesign.toArray(new Base[this.studyDesign.size()]); // CodeableConcept 5738 case -2081261232: /*statistic*/ return this.statistic == null ? new Base[0] : this.statistic.toArray(new Base[this.statistic.size()]); // EvidenceStatisticComponent 5739 case -1404142937: /*certainty*/ return this.certainty == null ? new Base[0] : this.certainty.toArray(new Base[this.certainty.size()]); // EvidenceCertaintyComponent 5740 default: return super.getProperty(hash, name, checkValid); 5741 } 5742 5743 } 5744 5745 @Override 5746 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5747 switch (hash) { 5748 case 116079: // url 5749 this.url = TypeConvertor.castToUri(value); // UriType 5750 return value; 5751 case -1618432855: // identifier 5752 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 5753 return value; 5754 case 351608024: // version 5755 this.version = TypeConvertor.castToString(value); // StringType 5756 return value; 5757 case 1508158071: // versionAlgorithm 5758 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5759 return value; 5760 case 3373707: // name 5761 this.name = TypeConvertor.castToString(value); // StringType 5762 return value; 5763 case 110371416: // title 5764 this.title = TypeConvertor.castToString(value); // StringType 5765 return value; 5766 case -1360156695: // citeAs 5767 this.citeAs = TypeConvertor.castToType(value); // DataType 5768 return value; 5769 case -892481550: // status 5770 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5771 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5772 return value; 5773 case -404562712: // experimental 5774 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 5775 return value; 5776 case 3076014: // date 5777 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 5778 return value; 5779 case 223539345: // approvalDate 5780 this.approvalDate = TypeConvertor.castToDate(value); // DateType 5781 return value; 5782 case -1687512484: // lastReviewDate 5783 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 5784 return value; 5785 case 1447404028: // publisher 5786 this.publisher = TypeConvertor.castToString(value); // StringType 5787 return value; 5788 case 951526432: // contact 5789 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5790 return value; 5791 case -1406328437: // author 5792 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5793 return value; 5794 case -1307827859: // editor 5795 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5796 return value; 5797 case -261190139: // reviewer 5798 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5799 return value; 5800 case 1740277666: // endorser 5801 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5802 return value; 5803 case -669707736: // useContext 5804 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5805 return value; 5806 case -220463842: // purpose 5807 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 5808 return value; 5809 case 1522889671: // copyright 5810 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5811 return value; 5812 case 765157229: // copyrightLabel 5813 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 5814 return value; 5815 case 666807069: // relatedArtifact 5816 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 5817 return value; 5818 case -1724546052: // description 5819 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 5820 return value; 5821 case 1314395906: // assertion 5822 this.assertion = TypeConvertor.castToMarkdown(value); // MarkdownType 5823 return value; 5824 case 3387378: // note 5825 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 5826 return value; 5827 case -1807222545: // variableDefinition 5828 this.getVariableDefinition().add((EvidenceVariableDefinitionComponent) value); // EvidenceVariableDefinitionComponent 5829 return value; 5830 case 672726254: // synthesisType 5831 this.synthesisType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5832 return value; 5833 case 1709211879: // studyDesign 5834 this.getStudyDesign().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5835 return value; 5836 case -2081261232: // statistic 5837 this.getStatistic().add((EvidenceStatisticComponent) value); // EvidenceStatisticComponent 5838 return value; 5839 case -1404142937: // certainty 5840 this.getCertainty().add((EvidenceCertaintyComponent) value); // EvidenceCertaintyComponent 5841 return value; 5842 default: return super.setProperty(hash, name, value); 5843 } 5844 5845 } 5846 5847 @Override 5848 public Base setProperty(String name, Base value) throws FHIRException { 5849 if (name.equals("url")) { 5850 this.url = TypeConvertor.castToUri(value); // UriType 5851 } else if (name.equals("identifier")) { 5852 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5853 } else if (name.equals("version")) { 5854 this.version = TypeConvertor.castToString(value); // StringType 5855 } else if (name.equals("versionAlgorithm[x]")) { 5856 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5857 } else if (name.equals("name")) { 5858 this.name = TypeConvertor.castToString(value); // StringType 5859 } else if (name.equals("title")) { 5860 this.title = TypeConvertor.castToString(value); // StringType 5861 } else if (name.equals("citeAs[x]")) { 5862 this.citeAs = TypeConvertor.castToType(value); // DataType 5863 } else if (name.equals("status")) { 5864 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5865 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5866 } else if (name.equals("experimental")) { 5867 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 5868 } else if (name.equals("date")) { 5869 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 5870 } else if (name.equals("approvalDate")) { 5871 this.approvalDate = TypeConvertor.castToDate(value); // DateType 5872 } else if (name.equals("lastReviewDate")) { 5873 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 5874 } else if (name.equals("publisher")) { 5875 this.publisher = TypeConvertor.castToString(value); // StringType 5876 } else if (name.equals("contact")) { 5877 this.getContact().add(TypeConvertor.castToContactDetail(value)); 5878 } else if (name.equals("author")) { 5879 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 5880 } else if (name.equals("editor")) { 5881 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 5882 } else if (name.equals("reviewer")) { 5883 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 5884 } else if (name.equals("endorser")) { 5885 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 5886 } else if (name.equals("useContext")) { 5887 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 5888 } else if (name.equals("purpose")) { 5889 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 5890 } else if (name.equals("copyright")) { 5891 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5892 } else if (name.equals("copyrightLabel")) { 5893 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 5894 } else if (name.equals("relatedArtifact")) { 5895 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 5896 } else if (name.equals("description")) { 5897 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 5898 } else if (name.equals("assertion")) { 5899 this.assertion = TypeConvertor.castToMarkdown(value); // MarkdownType 5900 } else if (name.equals("note")) { 5901 this.getNote().add(TypeConvertor.castToAnnotation(value)); 5902 } else if (name.equals("variableDefinition")) { 5903 this.getVariableDefinition().add((EvidenceVariableDefinitionComponent) value); 5904 } else if (name.equals("synthesisType")) { 5905 this.synthesisType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5906 } else if (name.equals("studyDesign")) { 5907 this.getStudyDesign().add(TypeConvertor.castToCodeableConcept(value)); 5908 } else if (name.equals("statistic")) { 5909 this.getStatistic().add((EvidenceStatisticComponent) value); 5910 } else if (name.equals("certainty")) { 5911 this.getCertainty().add((EvidenceCertaintyComponent) value); 5912 } else 5913 return super.setProperty(name, value); 5914 return value; 5915 } 5916 5917 @Override 5918 public void removeChild(String name, Base value) throws FHIRException { 5919 if (name.equals("url")) { 5920 this.url = null; 5921 } else if (name.equals("identifier")) { 5922 this.getIdentifier().remove(value); 5923 } else if (name.equals("version")) { 5924 this.version = null; 5925 } else if (name.equals("versionAlgorithm[x]")) { 5926 this.versionAlgorithm = null; 5927 } else if (name.equals("name")) { 5928 this.name = null; 5929 } else if (name.equals("title")) { 5930 this.title = null; 5931 } else if (name.equals("citeAs[x]")) { 5932 this.citeAs = null; 5933 } else if (name.equals("status")) { 5934 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5935 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5936 } else if (name.equals("experimental")) { 5937 this.experimental = null; 5938 } else if (name.equals("date")) { 5939 this.date = null; 5940 } else if (name.equals("approvalDate")) { 5941 this.approvalDate = null; 5942 } else if (name.equals("lastReviewDate")) { 5943 this.lastReviewDate = null; 5944 } else if (name.equals("publisher")) { 5945 this.publisher = null; 5946 } else if (name.equals("contact")) { 5947 this.getContact().remove(value); 5948 } else if (name.equals("author")) { 5949 this.getAuthor().remove(value); 5950 } else if (name.equals("editor")) { 5951 this.getEditor().remove(value); 5952 } else if (name.equals("reviewer")) { 5953 this.getReviewer().remove(value); 5954 } else if (name.equals("endorser")) { 5955 this.getEndorser().remove(value); 5956 } else if (name.equals("useContext")) { 5957 this.getUseContext().remove(value); 5958 } else if (name.equals("purpose")) { 5959 this.purpose = null; 5960 } else if (name.equals("copyright")) { 5961 this.copyright = null; 5962 } else if (name.equals("copyrightLabel")) { 5963 this.copyrightLabel = null; 5964 } else if (name.equals("relatedArtifact")) { 5965 this.getRelatedArtifact().remove(value); 5966 } else if (name.equals("description")) { 5967 this.description = null; 5968 } else if (name.equals("assertion")) { 5969 this.assertion = null; 5970 } else if (name.equals("note")) { 5971 this.getNote().remove(value); 5972 } else if (name.equals("variableDefinition")) { 5973 this.getVariableDefinition().remove((EvidenceVariableDefinitionComponent) value); 5974 } else if (name.equals("synthesisType")) { 5975 this.synthesisType = null; 5976 } else if (name.equals("studyDesign")) { 5977 this.getStudyDesign().remove(value); 5978 } else if (name.equals("statistic")) { 5979 this.getStatistic().remove((EvidenceStatisticComponent) value); 5980 } else if (name.equals("certainty")) { 5981 this.getCertainty().remove((EvidenceCertaintyComponent) value); 5982 } else 5983 super.removeChild(name, value); 5984 5985 } 5986 5987 @Override 5988 public Base makeProperty(int hash, String name) throws FHIRException { 5989 switch (hash) { 5990 case 116079: return getUrlElement(); 5991 case -1618432855: return addIdentifier(); 5992 case 351608024: return getVersionElement(); 5993 case -115699031: return getVersionAlgorithm(); 5994 case 1508158071: return getVersionAlgorithm(); 5995 case 3373707: return getNameElement(); 5996 case 110371416: return getTitleElement(); 5997 case -1706539017: return getCiteAs(); 5998 case -1360156695: return getCiteAs(); 5999 case -892481550: return getStatusElement(); 6000 case -404562712: return getExperimentalElement(); 6001 case 3076014: return getDateElement(); 6002 case 223539345: return getApprovalDateElement(); 6003 case -1687512484: return getLastReviewDateElement(); 6004 case 1447404028: return getPublisherElement(); 6005 case 951526432: return addContact(); 6006 case -1406328437: return addAuthor(); 6007 case -1307827859: return addEditor(); 6008 case -261190139: return addReviewer(); 6009 case 1740277666: return addEndorser(); 6010 case -669707736: return addUseContext(); 6011 case -220463842: return getPurposeElement(); 6012 case 1522889671: return getCopyrightElement(); 6013 case 765157229: return getCopyrightLabelElement(); 6014 case 666807069: return addRelatedArtifact(); 6015 case -1724546052: return getDescriptionElement(); 6016 case 1314395906: return getAssertionElement(); 6017 case 3387378: return addNote(); 6018 case -1807222545: return addVariableDefinition(); 6019 case 672726254: return getSynthesisType(); 6020 case 1709211879: return addStudyDesign(); 6021 case -2081261232: return addStatistic(); 6022 case -1404142937: return addCertainty(); 6023 default: return super.makeProperty(hash, name); 6024 } 6025 6026 } 6027 6028 @Override 6029 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6030 switch (hash) { 6031 case 116079: /*url*/ return new String[] {"uri"}; 6032 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6033 case 351608024: /*version*/ return new String[] {"string"}; 6034 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 6035 case 3373707: /*name*/ return new String[] {"string"}; 6036 case 110371416: /*title*/ return new String[] {"string"}; 6037 case -1360156695: /*citeAs*/ return new String[] {"Reference", "markdown"}; 6038 case -892481550: /*status*/ return new String[] {"code"}; 6039 case -404562712: /*experimental*/ return new String[] {"boolean"}; 6040 case 3076014: /*date*/ return new String[] {"dateTime"}; 6041 case 223539345: /*approvalDate*/ return new String[] {"date"}; 6042 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 6043 case 1447404028: /*publisher*/ return new String[] {"string"}; 6044 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 6045 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 6046 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 6047 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 6048 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 6049 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 6050 case -220463842: /*purpose*/ return new String[] {"markdown"}; 6051 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 6052 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 6053 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 6054 case -1724546052: /*description*/ return new String[] {"markdown"}; 6055 case 1314395906: /*assertion*/ return new String[] {"markdown"}; 6056 case 3387378: /*note*/ return new String[] {"Annotation"}; 6057 case -1807222545: /*variableDefinition*/ return new String[] {}; 6058 case 672726254: /*synthesisType*/ return new String[] {"CodeableConcept"}; 6059 case 1709211879: /*studyDesign*/ return new String[] {"CodeableConcept"}; 6060 case -2081261232: /*statistic*/ return new String[] {}; 6061 case -1404142937: /*certainty*/ return new String[] {}; 6062 default: return super.getTypesForProperty(hash, name); 6063 } 6064 6065 } 6066 6067 @Override 6068 public Base addChild(String name) throws FHIRException { 6069 if (name.equals("url")) { 6070 throw new FHIRException("Cannot call addChild on a singleton property Evidence.url"); 6071 } 6072 else if (name.equals("identifier")) { 6073 return addIdentifier(); 6074 } 6075 else if (name.equals("version")) { 6076 throw new FHIRException("Cannot call addChild on a singleton property Evidence.version"); 6077 } 6078 else if (name.equals("versionAlgorithmString")) { 6079 this.versionAlgorithm = new StringType(); 6080 return this.versionAlgorithm; 6081 } 6082 else if (name.equals("versionAlgorithmCoding")) { 6083 this.versionAlgorithm = new Coding(); 6084 return this.versionAlgorithm; 6085 } 6086 else if (name.equals("name")) { 6087 throw new FHIRException("Cannot call addChild on a singleton property Evidence.name"); 6088 } 6089 else if (name.equals("title")) { 6090 throw new FHIRException("Cannot call addChild on a singleton property Evidence.title"); 6091 } 6092 else if (name.equals("citeAsReference")) { 6093 this.citeAs = new Reference(); 6094 return this.citeAs; 6095 } 6096 else if (name.equals("citeAsMarkdown")) { 6097 this.citeAs = new MarkdownType(); 6098 return this.citeAs; 6099 } 6100 else if (name.equals("status")) { 6101 throw new FHIRException("Cannot call addChild on a singleton property Evidence.status"); 6102 } 6103 else if (name.equals("experimental")) { 6104 throw new FHIRException("Cannot call addChild on a singleton property Evidence.experimental"); 6105 } 6106 else if (name.equals("date")) { 6107 throw new FHIRException("Cannot call addChild on a singleton property Evidence.date"); 6108 } 6109 else if (name.equals("approvalDate")) { 6110 throw new FHIRException("Cannot call addChild on a singleton property Evidence.approvalDate"); 6111 } 6112 else if (name.equals("lastReviewDate")) { 6113 throw new FHIRException("Cannot call addChild on a singleton property Evidence.lastReviewDate"); 6114 } 6115 else if (name.equals("publisher")) { 6116 throw new FHIRException("Cannot call addChild on a singleton property Evidence.publisher"); 6117 } 6118 else if (name.equals("contact")) { 6119 return addContact(); 6120 } 6121 else if (name.equals("author")) { 6122 return addAuthor(); 6123 } 6124 else if (name.equals("editor")) { 6125 return addEditor(); 6126 } 6127 else if (name.equals("reviewer")) { 6128 return addReviewer(); 6129 } 6130 else if (name.equals("endorser")) { 6131 return addEndorser(); 6132 } 6133 else if (name.equals("useContext")) { 6134 return addUseContext(); 6135 } 6136 else if (name.equals("purpose")) { 6137 throw new FHIRException("Cannot call addChild on a singleton property Evidence.purpose"); 6138 } 6139 else if (name.equals("copyright")) { 6140 throw new FHIRException("Cannot call addChild on a singleton property Evidence.copyright"); 6141 } 6142 else if (name.equals("copyrightLabel")) { 6143 throw new FHIRException("Cannot call addChild on a singleton property Evidence.copyrightLabel"); 6144 } 6145 else if (name.equals("relatedArtifact")) { 6146 return addRelatedArtifact(); 6147 } 6148 else if (name.equals("description")) { 6149 throw new FHIRException("Cannot call addChild on a singleton property Evidence.description"); 6150 } 6151 else if (name.equals("assertion")) { 6152 throw new FHIRException("Cannot call addChild on a singleton property Evidence.assertion"); 6153 } 6154 else if (name.equals("note")) { 6155 return addNote(); 6156 } 6157 else if (name.equals("variableDefinition")) { 6158 return addVariableDefinition(); 6159 } 6160 else if (name.equals("synthesisType")) { 6161 this.synthesisType = new CodeableConcept(); 6162 return this.synthesisType; 6163 } 6164 else if (name.equals("studyDesign")) { 6165 return addStudyDesign(); 6166 } 6167 else if (name.equals("statistic")) { 6168 return addStatistic(); 6169 } 6170 else if (name.equals("certainty")) { 6171 return addCertainty(); 6172 } 6173 else 6174 return super.addChild(name); 6175 } 6176 6177 public String fhirType() { 6178 return "Evidence"; 6179 6180 } 6181 6182 public Evidence copy() { 6183 Evidence dst = new Evidence(); 6184 copyValues(dst); 6185 return dst; 6186 } 6187 6188 public void copyValues(Evidence dst) { 6189 super.copyValues(dst); 6190 dst.url = url == null ? null : url.copy(); 6191 if (identifier != null) { 6192 dst.identifier = new ArrayList<Identifier>(); 6193 for (Identifier i : identifier) 6194 dst.identifier.add(i.copy()); 6195 }; 6196 dst.version = version == null ? null : version.copy(); 6197 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 6198 dst.name = name == null ? null : name.copy(); 6199 dst.title = title == null ? null : title.copy(); 6200 dst.citeAs = citeAs == null ? null : citeAs.copy(); 6201 dst.status = status == null ? null : status.copy(); 6202 dst.experimental = experimental == null ? null : experimental.copy(); 6203 dst.date = date == null ? null : date.copy(); 6204 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 6205 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 6206 dst.publisher = publisher == null ? null : publisher.copy(); 6207 if (contact != null) { 6208 dst.contact = new ArrayList<ContactDetail>(); 6209 for (ContactDetail i : contact) 6210 dst.contact.add(i.copy()); 6211 }; 6212 if (author != null) { 6213 dst.author = new ArrayList<ContactDetail>(); 6214 for (ContactDetail i : author) 6215 dst.author.add(i.copy()); 6216 }; 6217 if (editor != null) { 6218 dst.editor = new ArrayList<ContactDetail>(); 6219 for (ContactDetail i : editor) 6220 dst.editor.add(i.copy()); 6221 }; 6222 if (reviewer != null) { 6223 dst.reviewer = new ArrayList<ContactDetail>(); 6224 for (ContactDetail i : reviewer) 6225 dst.reviewer.add(i.copy()); 6226 }; 6227 if (endorser != null) { 6228 dst.endorser = new ArrayList<ContactDetail>(); 6229 for (ContactDetail i : endorser) 6230 dst.endorser.add(i.copy()); 6231 }; 6232 if (useContext != null) { 6233 dst.useContext = new ArrayList<UsageContext>(); 6234 for (UsageContext i : useContext) 6235 dst.useContext.add(i.copy()); 6236 }; 6237 dst.purpose = purpose == null ? null : purpose.copy(); 6238 dst.copyright = copyright == null ? null : copyright.copy(); 6239 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 6240 if (relatedArtifact != null) { 6241 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 6242 for (RelatedArtifact i : relatedArtifact) 6243 dst.relatedArtifact.add(i.copy()); 6244 }; 6245 dst.description = description == null ? null : description.copy(); 6246 dst.assertion = assertion == null ? null : assertion.copy(); 6247 if (note != null) { 6248 dst.note = new ArrayList<Annotation>(); 6249 for (Annotation i : note) 6250 dst.note.add(i.copy()); 6251 }; 6252 if (variableDefinition != null) { 6253 dst.variableDefinition = new ArrayList<EvidenceVariableDefinitionComponent>(); 6254 for (EvidenceVariableDefinitionComponent i : variableDefinition) 6255 dst.variableDefinition.add(i.copy()); 6256 }; 6257 dst.synthesisType = synthesisType == null ? null : synthesisType.copy(); 6258 if (studyDesign != null) { 6259 dst.studyDesign = new ArrayList<CodeableConcept>(); 6260 for (CodeableConcept i : studyDesign) 6261 dst.studyDesign.add(i.copy()); 6262 }; 6263 if (statistic != null) { 6264 dst.statistic = new ArrayList<EvidenceStatisticComponent>(); 6265 for (EvidenceStatisticComponent i : statistic) 6266 dst.statistic.add(i.copy()); 6267 }; 6268 if (certainty != null) { 6269 dst.certainty = new ArrayList<EvidenceCertaintyComponent>(); 6270 for (EvidenceCertaintyComponent i : certainty) 6271 dst.certainty.add(i.copy()); 6272 }; 6273 } 6274 6275 protected Evidence typedCopy() { 6276 return copy(); 6277 } 6278 6279 @Override 6280 public boolean equalsDeep(Base other_) { 6281 if (!super.equalsDeep(other_)) 6282 return false; 6283 if (!(other_ instanceof Evidence)) 6284 return false; 6285 Evidence o = (Evidence) other_; 6286 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 6287 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 6288 && compareDeep(citeAs, o.citeAs, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 6289 && compareDeep(date, o.date, true) && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 6290 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(author, o.author, true) 6291 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 6292 && compareDeep(useContext, o.useContext, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 6293 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 6294 && compareDeep(description, o.description, true) && compareDeep(assertion, o.assertion, true) && compareDeep(note, o.note, true) 6295 && compareDeep(variableDefinition, o.variableDefinition, true) && compareDeep(synthesisType, o.synthesisType, true) 6296 && compareDeep(studyDesign, o.studyDesign, true) && compareDeep(statistic, o.statistic, true) && compareDeep(certainty, o.certainty, true) 6297 ; 6298 } 6299 6300 @Override 6301 public boolean equalsShallow(Base other_) { 6302 if (!super.equalsShallow(other_)) 6303 return false; 6304 if (!(other_ instanceof Evidence)) 6305 return false; 6306 Evidence o = (Evidence) other_; 6307 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 6308 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 6309 && compareValues(date, o.date, true) && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 6310 && compareValues(publisher, o.publisher, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 6311 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(description, o.description, true) 6312 && compareValues(assertion, o.assertion, true); 6313 } 6314 6315 public boolean isEmpty() { 6316 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 6317 , versionAlgorithm, name, title, citeAs, status, experimental, date, approvalDate 6318 , lastReviewDate, publisher, contact, author, editor, reviewer, endorser, useContext 6319 , purpose, copyright, copyrightLabel, relatedArtifact, description, assertion, note 6320 , variableDefinition, synthesisType, studyDesign, statistic, certainty); 6321 } 6322 6323 @Override 6324 public ResourceType getResourceType() { 6325 return ResourceType.Evidence; 6326 } 6327 6328 /** 6329 * Search parameter: <b>context-quantity</b> 6330 * <p> 6331 * Description: <b>Multiple Resources: 6332 6333* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 6334* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 6335* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 6336* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 6337* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 6338* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 6339* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 6340* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 6341* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 6342* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 6343* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 6344* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 6345* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 6346* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 6347* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 6348* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 6349* [Library](library.html): A quantity- or range-valued use context assigned to the library 6350* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 6351* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 6352* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 6353* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 6354* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 6355* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 6356* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 6357* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 6358* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 6359* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 6360* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 6361* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 6362* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 6363</b><br> 6364 * Type: <b>quantity</b><br> 6365 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 6366 * </p> 6367 */ 6368 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 6369 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6370 /** 6371 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6372 * <p> 6373 * Description: <b>Multiple Resources: 6374 6375* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 6376* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 6377* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 6378* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 6379* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 6380* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 6381* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 6382* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 6383* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 6384* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 6385* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 6386* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 6387* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 6388* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 6389* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 6390* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 6391* [Library](library.html): A quantity- or range-valued use context assigned to the library 6392* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 6393* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 6394* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 6395* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 6396* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 6397* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 6398* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 6399* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 6400* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 6401* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 6402* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 6403* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 6404* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 6405</b><br> 6406 * Type: <b>quantity</b><br> 6407 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 6408 * </p> 6409 */ 6410 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 6411 6412 /** 6413 * Search parameter: <b>context-type-quantity</b> 6414 * <p> 6415 * Description: <b>Multiple Resources: 6416 6417* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 6418* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 6419* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 6420* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 6421* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 6422* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 6423* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 6424* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 6425* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 6426* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 6427* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 6428* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 6429* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 6430* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 6431* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 6432* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 6433* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 6434* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 6435* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 6436* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 6437* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 6438* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 6439* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 6440* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 6441* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 6442* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 6443* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 6444* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 6445* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 6446* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 6447</b><br> 6448 * Type: <b>composite</b><br> 6449 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6450 * </p> 6451 */ 6452 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 6453 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6454 /** 6455 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 6456 * <p> 6457 * Description: <b>Multiple Resources: 6458 6459* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 6460* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 6461* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 6462* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 6463* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 6464* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 6465* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 6466* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 6467* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 6468* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 6469* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 6470* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 6471* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 6472* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 6473* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 6474* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 6475* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 6476* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 6477* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 6478* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 6479* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 6480* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 6481* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 6482* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 6483* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 6484* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 6485* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 6486* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 6487* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 6488* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 6489</b><br> 6490 * Type: <b>composite</b><br> 6491 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6492 * </p> 6493 */ 6494 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 6495 6496 /** 6497 * Search parameter: <b>context-type-value</b> 6498 * <p> 6499 * Description: <b>Multiple Resources: 6500 6501* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 6502* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 6503* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 6504* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 6505* [Citation](citation.html): A use context type and value assigned to the citation 6506* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 6507* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 6508* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 6509* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 6510* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 6511* [Evidence](evidence.html): A use context type and value assigned to the evidence 6512* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 6513* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 6514* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 6515* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 6516* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 6517* [Library](library.html): A use context type and value assigned to the library 6518* [Measure](measure.html): A use context type and value assigned to the measure 6519* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 6520* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 6521* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 6522* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 6523* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 6524* [Requirements](requirements.html): A use context type and value assigned to the requirements 6525* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 6526* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 6527* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 6528* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 6529* [TestScript](testscript.html): A use context type and value assigned to the test script 6530* [ValueSet](valueset.html): A use context type and value assigned to the value set 6531</b><br> 6532 * Type: <b>composite</b><br> 6533 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6534 * </p> 6535 */ 6536 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 6537 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 6538 /** 6539 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 6540 * <p> 6541 * Description: <b>Multiple Resources: 6542 6543* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 6544* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 6545* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 6546* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 6547* [Citation](citation.html): A use context type and value assigned to the citation 6548* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 6549* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 6550* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 6551* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 6552* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 6553* [Evidence](evidence.html): A use context type and value assigned to the evidence 6554* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 6555* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 6556* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 6557* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 6558* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 6559* [Library](library.html): A use context type and value assigned to the library 6560* [Measure](measure.html): A use context type and value assigned to the measure 6561* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 6562* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 6563* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 6564* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 6565* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 6566* [Requirements](requirements.html): A use context type and value assigned to the requirements 6567* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 6568* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 6569* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 6570* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 6571* [TestScript](testscript.html): A use context type and value assigned to the test script 6572* [ValueSet](valueset.html): A use context type and value assigned to the value set 6573</b><br> 6574 * Type: <b>composite</b><br> 6575 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6576 * </p> 6577 */ 6578 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 6579 6580 /** 6581 * Search parameter: <b>context-type</b> 6582 * <p> 6583 * Description: <b>Multiple Resources: 6584 6585* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 6586* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 6587* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 6588* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 6589* [Citation](citation.html): A type of use context assigned to the citation 6590* [CodeSystem](codesystem.html): A type of use context assigned to the code system 6591* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 6592* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 6593* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 6594* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 6595* [Evidence](evidence.html): A type of use context assigned to the evidence 6596* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 6597* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 6598* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 6599* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 6600* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 6601* [Library](library.html): A type of use context assigned to the library 6602* [Measure](measure.html): A type of use context assigned to the measure 6603* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 6604* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 6605* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 6606* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 6607* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 6608* [Requirements](requirements.html): A type of use context assigned to the requirements 6609* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 6610* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 6611* [StructureMap](structuremap.html): A type of use context assigned to the structure map 6612* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 6613* [TestScript](testscript.html): A type of use context assigned to the test script 6614* [ValueSet](valueset.html): A type of use context assigned to the value set 6615</b><br> 6616 * Type: <b>token</b><br> 6617 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 6618 * </p> 6619 */ 6620 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 6621 public static final String SP_CONTEXT_TYPE = "context-type"; 6622 /** 6623 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6624 * <p> 6625 * Description: <b>Multiple Resources: 6626 6627* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 6628* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 6629* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 6630* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 6631* [Citation](citation.html): A type of use context assigned to the citation 6632* [CodeSystem](codesystem.html): A type of use context assigned to the code system 6633* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 6634* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 6635* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 6636* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 6637* [Evidence](evidence.html): A type of use context assigned to the evidence 6638* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 6639* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 6640* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 6641* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 6642* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 6643* [Library](library.html): A type of use context assigned to the library 6644* [Measure](measure.html): A type of use context assigned to the measure 6645* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 6646* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 6647* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 6648* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 6649* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 6650* [Requirements](requirements.html): A type of use context assigned to the requirements 6651* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 6652* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 6653* [StructureMap](structuremap.html): A type of use context assigned to the structure map 6654* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 6655* [TestScript](testscript.html): A type of use context assigned to the test script 6656* [ValueSet](valueset.html): A type of use context assigned to the value set 6657</b><br> 6658 * Type: <b>token</b><br> 6659 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 6660 * </p> 6661 */ 6662 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 6663 6664 /** 6665 * Search parameter: <b>context</b> 6666 * <p> 6667 * Description: <b>Multiple Resources: 6668 6669* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 6670* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 6671* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 6672* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 6673* [Citation](citation.html): A use context assigned to the citation 6674* [CodeSystem](codesystem.html): A use context assigned to the code system 6675* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 6676* [ConceptMap](conceptmap.html): A use context assigned to the concept map 6677* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 6678* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 6679* [Evidence](evidence.html): A use context assigned to the evidence 6680* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 6681* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 6682* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 6683* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 6684* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 6685* [Library](library.html): A use context assigned to the library 6686* [Measure](measure.html): A use context assigned to the measure 6687* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 6688* [NamingSystem](namingsystem.html): A use context assigned to the naming system 6689* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 6690* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 6691* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 6692* [Requirements](requirements.html): A use context assigned to the requirements 6693* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 6694* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 6695* [StructureMap](structuremap.html): A use context assigned to the structure map 6696* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 6697* [TestScript](testscript.html): A use context assigned to the test script 6698* [ValueSet](valueset.html): A use context assigned to the value set 6699</b><br> 6700 * Type: <b>token</b><br> 6701 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 6702 * </p> 6703 */ 6704 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 6705 public static final String SP_CONTEXT = "context"; 6706 /** 6707 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6708 * <p> 6709 * Description: <b>Multiple Resources: 6710 6711* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 6712* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 6713* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 6714* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 6715* [Citation](citation.html): A use context assigned to the citation 6716* [CodeSystem](codesystem.html): A use context assigned to the code system 6717* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 6718* [ConceptMap](conceptmap.html): A use context assigned to the concept map 6719* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 6720* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 6721* [Evidence](evidence.html): A use context assigned to the evidence 6722* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 6723* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 6724* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 6725* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 6726* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 6727* [Library](library.html): A use context assigned to the library 6728* [Measure](measure.html): A use context assigned to the measure 6729* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 6730* [NamingSystem](namingsystem.html): A use context assigned to the naming system 6731* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 6732* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 6733* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 6734* [Requirements](requirements.html): A use context assigned to the requirements 6735* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 6736* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 6737* [StructureMap](structuremap.html): A use context assigned to the structure map 6738* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 6739* [TestScript](testscript.html): A use context assigned to the test script 6740* [ValueSet](valueset.html): A use context assigned to the value set 6741</b><br> 6742 * Type: <b>token</b><br> 6743 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 6744 * </p> 6745 */ 6746 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 6747 6748 /** 6749 * Search parameter: <b>date</b> 6750 * <p> 6751 * Description: <b>Multiple Resources: 6752 6753* [ActivityDefinition](activitydefinition.html): The activity definition publication date 6754* [ActorDefinition](actordefinition.html): The Actor Definition publication date 6755* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 6756* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 6757* [Citation](citation.html): The citation publication date 6758* [CodeSystem](codesystem.html): The code system publication date 6759* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 6760* [ConceptMap](conceptmap.html): The concept map publication date 6761* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 6762* [EventDefinition](eventdefinition.html): The event definition publication date 6763* [Evidence](evidence.html): The evidence publication date 6764* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 6765* [ExampleScenario](examplescenario.html): The example scenario publication date 6766* [GraphDefinition](graphdefinition.html): The graph definition publication date 6767* [ImplementationGuide](implementationguide.html): The implementation guide publication date 6768* [Library](library.html): The library publication date 6769* [Measure](measure.html): The measure publication date 6770* [MessageDefinition](messagedefinition.html): The message definition publication date 6771* [NamingSystem](namingsystem.html): The naming system publication date 6772* [OperationDefinition](operationdefinition.html): The operation definition publication date 6773* [PlanDefinition](plandefinition.html): The plan definition publication date 6774* [Questionnaire](questionnaire.html): The questionnaire publication date 6775* [Requirements](requirements.html): The requirements publication date 6776* [SearchParameter](searchparameter.html): The search parameter publication date 6777* [StructureDefinition](structuredefinition.html): The structure definition publication date 6778* [StructureMap](structuremap.html): The structure map publication date 6779* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 6780* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 6781* [TestScript](testscript.html): The test script publication date 6782* [ValueSet](valueset.html): The value set publication date 6783</b><br> 6784 * Type: <b>date</b><br> 6785 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 6786 * </p> 6787 */ 6788 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 6789 public static final String SP_DATE = "date"; 6790 /** 6791 * <b>Fluent Client</b> search parameter constant for <b>date</b> 6792 * <p> 6793 * Description: <b>Multiple Resources: 6794 6795* [ActivityDefinition](activitydefinition.html): The activity definition publication date 6796* [ActorDefinition](actordefinition.html): The Actor Definition publication date 6797* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 6798* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 6799* [Citation](citation.html): The citation publication date 6800* [CodeSystem](codesystem.html): The code system publication date 6801* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 6802* [ConceptMap](conceptmap.html): The concept map publication date 6803* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 6804* [EventDefinition](eventdefinition.html): The event definition publication date 6805* [Evidence](evidence.html): The evidence publication date 6806* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 6807* [ExampleScenario](examplescenario.html): The example scenario publication date 6808* [GraphDefinition](graphdefinition.html): The graph definition publication date 6809* [ImplementationGuide](implementationguide.html): The implementation guide publication date 6810* [Library](library.html): The library publication date 6811* [Measure](measure.html): The measure publication date 6812* [MessageDefinition](messagedefinition.html): The message definition publication date 6813* [NamingSystem](namingsystem.html): The naming system publication date 6814* [OperationDefinition](operationdefinition.html): The operation definition publication date 6815* [PlanDefinition](plandefinition.html): The plan definition publication date 6816* [Questionnaire](questionnaire.html): The questionnaire publication date 6817* [Requirements](requirements.html): The requirements publication date 6818* [SearchParameter](searchparameter.html): The search parameter publication date 6819* [StructureDefinition](structuredefinition.html): The structure definition publication date 6820* [StructureMap](structuremap.html): The structure map publication date 6821* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 6822* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 6823* [TestScript](testscript.html): The test script publication date 6824* [ValueSet](valueset.html): The value set publication date 6825</b><br> 6826 * Type: <b>date</b><br> 6827 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 6828 * </p> 6829 */ 6830 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 6831 6832 /** 6833 * Search parameter: <b>description</b> 6834 * <p> 6835 * Description: <b>Multiple Resources: 6836 6837* [ActivityDefinition](activitydefinition.html): The description of the activity definition 6838* [ActorDefinition](actordefinition.html): The description of the Actor Definition 6839* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 6840* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 6841* [Citation](citation.html): The description of the citation 6842* [CodeSystem](codesystem.html): The description of the code system 6843* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 6844* [ConceptMap](conceptmap.html): The description of the concept map 6845* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 6846* [EventDefinition](eventdefinition.html): The description of the event definition 6847* [Evidence](evidence.html): The description of the evidence 6848* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 6849* [GraphDefinition](graphdefinition.html): The description of the graph definition 6850* [ImplementationGuide](implementationguide.html): The description of the implementation guide 6851* [Library](library.html): The description of the library 6852* [Measure](measure.html): The description of the measure 6853* [MessageDefinition](messagedefinition.html): The description of the message definition 6854* [NamingSystem](namingsystem.html): The description of the naming system 6855* [OperationDefinition](operationdefinition.html): The description of the operation definition 6856* [PlanDefinition](plandefinition.html): The description of the plan definition 6857* [Questionnaire](questionnaire.html): The description of the questionnaire 6858* [Requirements](requirements.html): The description of the requirements 6859* [SearchParameter](searchparameter.html): The description of the search parameter 6860* [StructureDefinition](structuredefinition.html): The description of the structure definition 6861* [StructureMap](structuremap.html): The description of the structure map 6862* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 6863* [TestScript](testscript.html): The description of the test script 6864* [ValueSet](valueset.html): The description of the value set 6865</b><br> 6866 * Type: <b>string</b><br> 6867 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 6868 * </p> 6869 */ 6870 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 6871 public static final String SP_DESCRIPTION = "description"; 6872 /** 6873 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6874 * <p> 6875 * Description: <b>Multiple Resources: 6876 6877* [ActivityDefinition](activitydefinition.html): The description of the activity definition 6878* [ActorDefinition](actordefinition.html): The description of the Actor Definition 6879* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 6880* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 6881* [Citation](citation.html): The description of the citation 6882* [CodeSystem](codesystem.html): The description of the code system 6883* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 6884* [ConceptMap](conceptmap.html): The description of the concept map 6885* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 6886* [EventDefinition](eventdefinition.html): The description of the event definition 6887* [Evidence](evidence.html): The description of the evidence 6888* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 6889* [GraphDefinition](graphdefinition.html): The description of the graph definition 6890* [ImplementationGuide](implementationguide.html): The description of the implementation guide 6891* [Library](library.html): The description of the library 6892* [Measure](measure.html): The description of the measure 6893* [MessageDefinition](messagedefinition.html): The description of the message definition 6894* [NamingSystem](namingsystem.html): The description of the naming system 6895* [OperationDefinition](operationdefinition.html): The description of the operation definition 6896* [PlanDefinition](plandefinition.html): The description of the plan definition 6897* [Questionnaire](questionnaire.html): The description of the questionnaire 6898* [Requirements](requirements.html): The description of the requirements 6899* [SearchParameter](searchparameter.html): The description of the search parameter 6900* [StructureDefinition](structuredefinition.html): The description of the structure definition 6901* [StructureMap](structuremap.html): The description of the structure map 6902* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 6903* [TestScript](testscript.html): The description of the test script 6904* [ValueSet](valueset.html): The description of the value set 6905</b><br> 6906 * Type: <b>string</b><br> 6907 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 6908 * </p> 6909 */ 6910 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 6911 6912 /** 6913 * Search parameter: <b>identifier</b> 6914 * <p> 6915 * Description: <b>Multiple Resources: 6916 6917* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6918* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6919* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6920* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6921* [Citation](citation.html): External identifier for the citation 6922* [CodeSystem](codesystem.html): External identifier for the code system 6923* [ConceptMap](conceptmap.html): External identifier for the concept map 6924* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6925* [EventDefinition](eventdefinition.html): External identifier for the event definition 6926* [Evidence](evidence.html): External identifier for the evidence 6927* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6928* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6929* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6930* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6931* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6932* [Library](library.html): External identifier for the library 6933* [Measure](measure.html): External identifier for the measure 6934* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6935* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6936* [NamingSystem](namingsystem.html): External identifier for the naming system 6937* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6938* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6939* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6940* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6941* [Requirements](requirements.html): External identifier for the requirements 6942* [SearchParameter](searchparameter.html): External identifier for the search parameter 6943* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6944* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6945* [StructureMap](structuremap.html): External identifier for the structure map 6946* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6947* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6948* [TestPlan](testplan.html): An identifier for the test plan 6949* [TestScript](testscript.html): External identifier for the test script 6950* [ValueSet](valueset.html): External identifier for the value set 6951</b><br> 6952 * Type: <b>token</b><br> 6953 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6954 * </p> 6955 */ 6956 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 6957 public static final String SP_IDENTIFIER = "identifier"; 6958 /** 6959 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6960 * <p> 6961 * Description: <b>Multiple Resources: 6962 6963* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6964* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6965* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6966* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6967* [Citation](citation.html): External identifier for the citation 6968* [CodeSystem](codesystem.html): External identifier for the code system 6969* [ConceptMap](conceptmap.html): External identifier for the concept map 6970* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6971* [EventDefinition](eventdefinition.html): External identifier for the event definition 6972* [Evidence](evidence.html): External identifier for the evidence 6973* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6974* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6975* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6976* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6977* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6978* [Library](library.html): External identifier for the library 6979* [Measure](measure.html): External identifier for the measure 6980* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6981* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6982* [NamingSystem](namingsystem.html): External identifier for the naming system 6983* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6984* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6985* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6986* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6987* [Requirements](requirements.html): External identifier for the requirements 6988* [SearchParameter](searchparameter.html): External identifier for the search parameter 6989* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6990* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6991* [StructureMap](structuremap.html): External identifier for the structure map 6992* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6993* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6994* [TestPlan](testplan.html): An identifier for the test plan 6995* [TestScript](testscript.html): External identifier for the test script 6996* [ValueSet](valueset.html): External identifier for the value set 6997</b><br> 6998 * Type: <b>token</b><br> 6999 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7000 * </p> 7001 */ 7002 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7003 7004 /** 7005 * Search parameter: <b>publisher</b> 7006 * <p> 7007 * Description: <b>Multiple Resources: 7008 7009* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 7010* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 7011* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 7012* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 7013* [Citation](citation.html): Name of the publisher of the citation 7014* [CodeSystem](codesystem.html): Name of the publisher of the code system 7015* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 7016* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 7017* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 7018* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 7019* [Evidence](evidence.html): Name of the publisher of the evidence 7020* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 7021* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 7022* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 7023* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 7024* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 7025* [Library](library.html): Name of the publisher of the library 7026* [Measure](measure.html): Name of the publisher of the measure 7027* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 7028* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 7029* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 7030* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 7031* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 7032* [Requirements](requirements.html): Name of the publisher of the requirements 7033* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 7034* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 7035* [StructureMap](structuremap.html): Name of the publisher of the structure map 7036* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 7037* [TestScript](testscript.html): Name of the publisher of the test script 7038* [ValueSet](valueset.html): Name of the publisher of the value set 7039</b><br> 7040 * Type: <b>string</b><br> 7041 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 7042 * </p> 7043 */ 7044 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 7045 public static final String SP_PUBLISHER = "publisher"; 7046 /** 7047 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 7048 * <p> 7049 * Description: <b>Multiple Resources: 7050 7051* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 7052* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 7053* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 7054* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 7055* [Citation](citation.html): Name of the publisher of the citation 7056* [CodeSystem](codesystem.html): Name of the publisher of the code system 7057* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 7058* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 7059* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 7060* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 7061* [Evidence](evidence.html): Name of the publisher of the evidence 7062* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 7063* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 7064* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 7065* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 7066* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 7067* [Library](library.html): Name of the publisher of the library 7068* [Measure](measure.html): Name of the publisher of the measure 7069* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 7070* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 7071* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 7072* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 7073* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 7074* [Requirements](requirements.html): Name of the publisher of the requirements 7075* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 7076* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 7077* [StructureMap](structuremap.html): Name of the publisher of the structure map 7078* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 7079* [TestScript](testscript.html): Name of the publisher of the test script 7080* [ValueSet](valueset.html): Name of the publisher of the value set 7081</b><br> 7082 * Type: <b>string</b><br> 7083 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 7084 * </p> 7085 */ 7086 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 7087 7088 /** 7089 * Search parameter: <b>status</b> 7090 * <p> 7091 * Description: <b>Multiple Resources: 7092 7093* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7094* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7095* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7096* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7097* [Citation](citation.html): The current status of the citation 7098* [CodeSystem](codesystem.html): The current status of the code system 7099* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7100* [ConceptMap](conceptmap.html): The current status of the concept map 7101* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7102* [EventDefinition](eventdefinition.html): The current status of the event definition 7103* [Evidence](evidence.html): The current status of the evidence 7104* [EvidenceReport](evidencereport.html): The current status of the evidence report 7105* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7106* [ExampleScenario](examplescenario.html): The current status of the example scenario 7107* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7108* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7109* [Library](library.html): The current status of the library 7110* [Measure](measure.html): The current status of the measure 7111* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7112* [MessageDefinition](messagedefinition.html): The current status of the message definition 7113* [NamingSystem](namingsystem.html): The current status of the naming system 7114* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7115* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7116* [PlanDefinition](plandefinition.html): The current status of the plan definition 7117* [Questionnaire](questionnaire.html): The current status of the questionnaire 7118* [Requirements](requirements.html): The current status of the requirements 7119* [SearchParameter](searchparameter.html): The current status of the search parameter 7120* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7121* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7122* [StructureMap](structuremap.html): The current status of the structure map 7123* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7124* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7125* [TestPlan](testplan.html): The current status of the test plan 7126* [TestScript](testscript.html): The current status of the test script 7127* [ValueSet](valueset.html): The current status of the value set 7128</b><br> 7129 * Type: <b>token</b><br> 7130 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7131 * </p> 7132 */ 7133 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 7134 public static final String SP_STATUS = "status"; 7135 /** 7136 * <b>Fluent Client</b> search parameter constant for <b>status</b> 7137 * <p> 7138 * Description: <b>Multiple Resources: 7139 7140* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7141* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7142* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7143* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7144* [Citation](citation.html): The current status of the citation 7145* [CodeSystem](codesystem.html): The current status of the code system 7146* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7147* [ConceptMap](conceptmap.html): The current status of the concept map 7148* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7149* [EventDefinition](eventdefinition.html): The current status of the event definition 7150* [Evidence](evidence.html): The current status of the evidence 7151* [EvidenceReport](evidencereport.html): The current status of the evidence report 7152* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7153* [ExampleScenario](examplescenario.html): The current status of the example scenario 7154* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7155* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7156* [Library](library.html): The current status of the library 7157* [Measure](measure.html): The current status of the measure 7158* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7159* [MessageDefinition](messagedefinition.html): The current status of the message definition 7160* [NamingSystem](namingsystem.html): The current status of the naming system 7161* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7162* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7163* [PlanDefinition](plandefinition.html): The current status of the plan definition 7164* [Questionnaire](questionnaire.html): The current status of the questionnaire 7165* [Requirements](requirements.html): The current status of the requirements 7166* [SearchParameter](searchparameter.html): The current status of the search parameter 7167* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7168* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7169* [StructureMap](structuremap.html): The current status of the structure map 7170* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7171* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7172* [TestPlan](testplan.html): The current status of the test plan 7173* [TestScript](testscript.html): The current status of the test script 7174* [ValueSet](valueset.html): The current status of the value set 7175</b><br> 7176 * Type: <b>token</b><br> 7177 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7178 * </p> 7179 */ 7180 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 7181 7182 /** 7183 * Search parameter: <b>title</b> 7184 * <p> 7185 * Description: <b>Multiple Resources: 7186 7187* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 7188* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 7189* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 7190* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 7191* [Citation](citation.html): The human-friendly name of the citation 7192* [CodeSystem](codesystem.html): The human-friendly name of the code system 7193* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 7194* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 7195* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 7196* [Evidence](evidence.html): The human-friendly name of the evidence 7197* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 7198* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 7199* [Library](library.html): The human-friendly name of the library 7200* [Measure](measure.html): The human-friendly name of the measure 7201* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 7202* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 7203* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 7204* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 7205* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 7206* [Requirements](requirements.html): The human-friendly name of the requirements 7207* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 7208* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 7209* [StructureMap](structuremap.html): The human-friendly name of the structure map 7210* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 7211* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 7212* [TestScript](testscript.html): The human-friendly name of the test script 7213* [ValueSet](valueset.html): The human-friendly name of the value set 7214</b><br> 7215 * Type: <b>string</b><br> 7216 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 7217 * </p> 7218 */ 7219 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 7220 public static final String SP_TITLE = "title"; 7221 /** 7222 * <b>Fluent Client</b> search parameter constant for <b>title</b> 7223 * <p> 7224 * Description: <b>Multiple Resources: 7225 7226* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 7227* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 7228* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 7229* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 7230* [Citation](citation.html): The human-friendly name of the citation 7231* [CodeSystem](codesystem.html): The human-friendly name of the code system 7232* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 7233* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 7234* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 7235* [Evidence](evidence.html): The human-friendly name of the evidence 7236* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 7237* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 7238* [Library](library.html): The human-friendly name of the library 7239* [Measure](measure.html): The human-friendly name of the measure 7240* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 7241* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 7242* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 7243* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 7244* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 7245* [Requirements](requirements.html): The human-friendly name of the requirements 7246* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 7247* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 7248* [StructureMap](structuremap.html): The human-friendly name of the structure map 7249* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 7250* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 7251* [TestScript](testscript.html): The human-friendly name of the test script 7252* [ValueSet](valueset.html): The human-friendly name of the value set 7253</b><br> 7254 * Type: <b>string</b><br> 7255 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 7256 * </p> 7257 */ 7258 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 7259 7260 /** 7261 * Search parameter: <b>url</b> 7262 * <p> 7263 * Description: <b>Multiple Resources: 7264 7265* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 7266* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 7267* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 7268* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 7269* [Citation](citation.html): The uri that identifies the citation 7270* [CodeSystem](codesystem.html): The uri that identifies the code system 7271* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 7272* [ConceptMap](conceptmap.html): The URI that identifies the concept map 7273* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 7274* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 7275* [Evidence](evidence.html): The uri that identifies the evidence 7276* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 7277* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 7278* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 7279* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 7280* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 7281* [Library](library.html): The uri that identifies the library 7282* [Measure](measure.html): The uri that identifies the measure 7283* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 7284* [NamingSystem](namingsystem.html): The uri that identifies the naming system 7285* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 7286* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 7287* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 7288* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 7289* [Requirements](requirements.html): The uri that identifies the requirements 7290* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 7291* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 7292* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 7293* [StructureMap](structuremap.html): The uri that identifies the structure map 7294* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 7295* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 7296* [TestPlan](testplan.html): The uri that identifies the test plan 7297* [TestScript](testscript.html): The uri that identifies the test script 7298* [ValueSet](valueset.html): The uri that identifies the value set 7299</b><br> 7300 * Type: <b>uri</b><br> 7301 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 7302 * </p> 7303 */ 7304 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 7305 public static final String SP_URL = "url"; 7306 /** 7307 * <b>Fluent Client</b> search parameter constant for <b>url</b> 7308 * <p> 7309 * Description: <b>Multiple Resources: 7310 7311* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 7312* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 7313* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 7314* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 7315* [Citation](citation.html): The uri that identifies the citation 7316* [CodeSystem](codesystem.html): The uri that identifies the code system 7317* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 7318* [ConceptMap](conceptmap.html): The URI that identifies the concept map 7319* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 7320* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 7321* [Evidence](evidence.html): The uri that identifies the evidence 7322* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 7323* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 7324* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 7325* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 7326* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 7327* [Library](library.html): The uri that identifies the library 7328* [Measure](measure.html): The uri that identifies the measure 7329* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 7330* [NamingSystem](namingsystem.html): The uri that identifies the naming system 7331* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 7332* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 7333* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 7334* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 7335* [Requirements](requirements.html): The uri that identifies the requirements 7336* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 7337* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 7338* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 7339* [StructureMap](structuremap.html): The uri that identifies the structure map 7340* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 7341* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 7342* [TestPlan](testplan.html): The uri that identifies the test plan 7343* [TestScript](testscript.html): The uri that identifies the test script 7344* [ValueSet](valueset.html): The uri that identifies the value set 7345</b><br> 7346 * Type: <b>uri</b><br> 7347 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 7348 * </p> 7349 */ 7350 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 7351 7352 /** 7353 * Search parameter: <b>version</b> 7354 * <p> 7355 * Description: <b>Multiple Resources: 7356 7357* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 7358* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 7359* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 7360* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 7361* [Citation](citation.html): The business version of the citation 7362* [CodeSystem](codesystem.html): The business version of the code system 7363* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 7364* [ConceptMap](conceptmap.html): The business version of the concept map 7365* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 7366* [EventDefinition](eventdefinition.html): The business version of the event definition 7367* [Evidence](evidence.html): The business version of the evidence 7368* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 7369* [ExampleScenario](examplescenario.html): The business version of the example scenario 7370* [GraphDefinition](graphdefinition.html): The business version of the graph definition 7371* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 7372* [Library](library.html): The business version of the library 7373* [Measure](measure.html): The business version of the measure 7374* [MessageDefinition](messagedefinition.html): The business version of the message definition 7375* [NamingSystem](namingsystem.html): The business version of the naming system 7376* [OperationDefinition](operationdefinition.html): The business version of the operation definition 7377* [PlanDefinition](plandefinition.html): The business version of the plan definition 7378* [Questionnaire](questionnaire.html): The business version of the questionnaire 7379* [Requirements](requirements.html): The business version of the requirements 7380* [SearchParameter](searchparameter.html): The business version of the search parameter 7381* [StructureDefinition](structuredefinition.html): The business version of the structure definition 7382* [StructureMap](structuremap.html): The business version of the structure map 7383* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 7384* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 7385* [TestScript](testscript.html): The business version of the test script 7386* [ValueSet](valueset.html): The business version of the value set 7387</b><br> 7388 * Type: <b>token</b><br> 7389 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 7390 * </p> 7391 */ 7392 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 7393 public static final String SP_VERSION = "version"; 7394 /** 7395 * <b>Fluent Client</b> search parameter constant for <b>version</b> 7396 * <p> 7397 * Description: <b>Multiple Resources: 7398 7399* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 7400* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 7401* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 7402* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 7403* [Citation](citation.html): The business version of the citation 7404* [CodeSystem](codesystem.html): The business version of the code system 7405* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 7406* [ConceptMap](conceptmap.html): The business version of the concept map 7407* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 7408* [EventDefinition](eventdefinition.html): The business version of the event definition 7409* [Evidence](evidence.html): The business version of the evidence 7410* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 7411* [ExampleScenario](examplescenario.html): The business version of the example scenario 7412* [GraphDefinition](graphdefinition.html): The business version of the graph definition 7413* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 7414* [Library](library.html): The business version of the library 7415* [Measure](measure.html): The business version of the measure 7416* [MessageDefinition](messagedefinition.html): The business version of the message definition 7417* [NamingSystem](namingsystem.html): The business version of the naming system 7418* [OperationDefinition](operationdefinition.html): The business version of the operation definition 7419* [PlanDefinition](plandefinition.html): The business version of the plan definition 7420* [Questionnaire](questionnaire.html): The business version of the questionnaire 7421* [Requirements](requirements.html): The business version of the requirements 7422* [SearchParameter](searchparameter.html): The business version of the search parameter 7423* [StructureDefinition](structuredefinition.html): The business version of the structure definition 7424* [StructureMap](structuremap.html): The business version of the structure map 7425* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 7426* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 7427* [TestScript](testscript.html): The business version of the test script 7428* [ValueSet](valueset.html): The business version of the value set 7429</b><br> 7430 * Type: <b>token</b><br> 7431 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 7432 * </p> 7433 */ 7434 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 7435 7436 7437} 7438