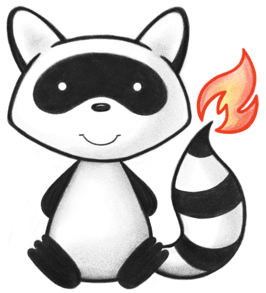
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The EvidenceReport Resource is a specialized container for a collection of resources and codeable concepts, adapted to support compositions of Evidence, EvidenceVariable, and Citation resources and related concepts. 052 */ 053@ResourceDef(name="EvidenceReport", profile="http://hl7.org/fhir/StructureDefinition/EvidenceReport") 054public class EvidenceReport extends MetadataResource { 055 056 public enum ReportRelationshipType { 057 /** 058 * This document replaces or supersedes the target document. 059 */ 060 REPLACES, 061 /** 062 * This document notes corrections or changes to replace or supersede parts of the target document. 063 */ 064 AMENDS, 065 /** 066 * This document adds additional information to the target document. 067 */ 068 APPENDS, 069 /** 070 * This document was generated by transforming the target document (eg format or language conversion). 071 */ 072 TRANSFORMS, 073 /** 074 * This document was. 075 */ 076 REPLACEDWITH, 077 /** 078 * This document was. 079 */ 080 AMENDEDWITH, 081 /** 082 * This document was. 083 */ 084 APPENDEDWITH, 085 /** 086 * This document was. 087 */ 088 TRANSFORMEDWITH, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 public static ReportRelationshipType fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("replaces".equals(codeString)) 097 return REPLACES; 098 if ("amends".equals(codeString)) 099 return AMENDS; 100 if ("appends".equals(codeString)) 101 return APPENDS; 102 if ("transforms".equals(codeString)) 103 return TRANSFORMS; 104 if ("replacedWith".equals(codeString)) 105 return REPLACEDWITH; 106 if ("amendedWith".equals(codeString)) 107 return AMENDEDWITH; 108 if ("appendedWith".equals(codeString)) 109 return APPENDEDWITH; 110 if ("transformedWith".equals(codeString)) 111 return TRANSFORMEDWITH; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown ReportRelationshipType code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case REPLACES: return "replaces"; 120 case AMENDS: return "amends"; 121 case APPENDS: return "appends"; 122 case TRANSFORMS: return "transforms"; 123 case REPLACEDWITH: return "replacedWith"; 124 case AMENDEDWITH: return "amendedWith"; 125 case APPENDEDWITH: return "appendedWith"; 126 case TRANSFORMEDWITH: return "transformedWith"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getSystem() { 132 switch (this) { 133 case REPLACES: return "http://hl7.org/fhir/report-relation-type"; 134 case AMENDS: return "http://hl7.org/fhir/report-relation-type"; 135 case APPENDS: return "http://hl7.org/fhir/report-relation-type"; 136 case TRANSFORMS: return "http://hl7.org/fhir/report-relation-type"; 137 case REPLACEDWITH: return "http://hl7.org/fhir/report-relation-type"; 138 case AMENDEDWITH: return "http://hl7.org/fhir/report-relation-type"; 139 case APPENDEDWITH: return "http://hl7.org/fhir/report-relation-type"; 140 case TRANSFORMEDWITH: return "http://hl7.org/fhir/report-relation-type"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getDefinition() { 146 switch (this) { 147 case REPLACES: return "This document replaces or supersedes the target document."; 148 case AMENDS: return "This document notes corrections or changes to replace or supersede parts of the target document."; 149 case APPENDS: return "This document adds additional information to the target document."; 150 case TRANSFORMS: return "This document was generated by transforming the target document (eg format or language conversion)."; 151 case REPLACEDWITH: return "This document was."; 152 case AMENDEDWITH: return "This document was."; 153 case APPENDEDWITH: return "This document was."; 154 case TRANSFORMEDWITH: return "This document was."; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 public String getDisplay() { 160 switch (this) { 161 case REPLACES: return "Replaces"; 162 case AMENDS: return "Amends"; 163 case APPENDS: return "Appends"; 164 case TRANSFORMS: return "Transforms"; 165 case REPLACEDWITH: return "Replaced With"; 166 case AMENDEDWITH: return "Amended With"; 167 case APPENDEDWITH: return "Appended With"; 168 case TRANSFORMEDWITH: return "Transformed With"; 169 case NULL: return null; 170 default: return "?"; 171 } 172 } 173 } 174 175 public static class ReportRelationshipTypeEnumFactory implements EnumFactory<ReportRelationshipType> { 176 public ReportRelationshipType fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("replaces".equals(codeString)) 181 return ReportRelationshipType.REPLACES; 182 if ("amends".equals(codeString)) 183 return ReportRelationshipType.AMENDS; 184 if ("appends".equals(codeString)) 185 return ReportRelationshipType.APPENDS; 186 if ("transforms".equals(codeString)) 187 return ReportRelationshipType.TRANSFORMS; 188 if ("replacedWith".equals(codeString)) 189 return ReportRelationshipType.REPLACEDWITH; 190 if ("amendedWith".equals(codeString)) 191 return ReportRelationshipType.AMENDEDWITH; 192 if ("appendedWith".equals(codeString)) 193 return ReportRelationshipType.APPENDEDWITH; 194 if ("transformedWith".equals(codeString)) 195 return ReportRelationshipType.TRANSFORMEDWITH; 196 throw new IllegalArgumentException("Unknown ReportRelationshipType code '"+codeString+"'"); 197 } 198 public Enumeration<ReportRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.NULL, code); 203 String codeString = ((PrimitiveType) code).asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.NULL, code); 206 if ("replaces".equals(codeString)) 207 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.REPLACES, code); 208 if ("amends".equals(codeString)) 209 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.AMENDS, code); 210 if ("appends".equals(codeString)) 211 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.APPENDS, code); 212 if ("transforms".equals(codeString)) 213 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.TRANSFORMS, code); 214 if ("replacedWith".equals(codeString)) 215 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.REPLACEDWITH, code); 216 if ("amendedWith".equals(codeString)) 217 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.AMENDEDWITH, code); 218 if ("appendedWith".equals(codeString)) 219 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.APPENDEDWITH, code); 220 if ("transformedWith".equals(codeString)) 221 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.TRANSFORMEDWITH, code); 222 throw new FHIRException("Unknown ReportRelationshipType code '"+codeString+"'"); 223 } 224 public String toCode(ReportRelationshipType code) { 225 if (code == ReportRelationshipType.REPLACES) 226 return "replaces"; 227 if (code == ReportRelationshipType.AMENDS) 228 return "amends"; 229 if (code == ReportRelationshipType.APPENDS) 230 return "appends"; 231 if (code == ReportRelationshipType.TRANSFORMS) 232 return "transforms"; 233 if (code == ReportRelationshipType.REPLACEDWITH) 234 return "replacedWith"; 235 if (code == ReportRelationshipType.AMENDEDWITH) 236 return "amendedWith"; 237 if (code == ReportRelationshipType.APPENDEDWITH) 238 return "appendedWith"; 239 if (code == ReportRelationshipType.TRANSFORMEDWITH) 240 return "transformedWith"; 241 return "?"; 242 } 243 public String toSystem(ReportRelationshipType code) { 244 return code.getSystem(); 245 } 246 } 247 248 @Block() 249 public static class EvidenceReportSubjectComponent extends BackboneElement implements IBaseBackboneElement { 250 /** 251 * Characteristic. 252 */ 253 @Child(name = "characteristic", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 254 @Description(shortDefinition="Characteristic", formalDefinition="Characteristic." ) 255 protected List<EvidenceReportSubjectCharacteristicComponent> characteristic; 256 257 /** 258 * Used for general notes and annotations not coded elsewhere. 259 */ 260 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 261 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Used for general notes and annotations not coded elsewhere." ) 262 protected List<Annotation> note; 263 264 private static final long serialVersionUID = -734040873L; 265 266 /** 267 * Constructor 268 */ 269 public EvidenceReportSubjectComponent() { 270 super(); 271 } 272 273 /** 274 * @return {@link #characteristic} (Characteristic.) 275 */ 276 public List<EvidenceReportSubjectCharacteristicComponent> getCharacteristic() { 277 if (this.characteristic == null) 278 this.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 279 return this.characteristic; 280 } 281 282 /** 283 * @return Returns a reference to <code>this</code> for easy method chaining 284 */ 285 public EvidenceReportSubjectComponent setCharacteristic(List<EvidenceReportSubjectCharacteristicComponent> theCharacteristic) { 286 this.characteristic = theCharacteristic; 287 return this; 288 } 289 290 public boolean hasCharacteristic() { 291 if (this.characteristic == null) 292 return false; 293 for (EvidenceReportSubjectCharacteristicComponent item : this.characteristic) 294 if (!item.isEmpty()) 295 return true; 296 return false; 297 } 298 299 public EvidenceReportSubjectCharacteristicComponent addCharacteristic() { //3 300 EvidenceReportSubjectCharacteristicComponent t = new EvidenceReportSubjectCharacteristicComponent(); 301 if (this.characteristic == null) 302 this.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 303 this.characteristic.add(t); 304 return t; 305 } 306 307 public EvidenceReportSubjectComponent addCharacteristic(EvidenceReportSubjectCharacteristicComponent t) { //3 308 if (t == null) 309 return this; 310 if (this.characteristic == null) 311 this.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 312 this.characteristic.add(t); 313 return this; 314 } 315 316 /** 317 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 318 */ 319 public EvidenceReportSubjectCharacteristicComponent getCharacteristicFirstRep() { 320 if (getCharacteristic().isEmpty()) { 321 addCharacteristic(); 322 } 323 return getCharacteristic().get(0); 324 } 325 326 /** 327 * @return {@link #note} (Used for general notes and annotations not coded elsewhere.) 328 */ 329 public List<Annotation> getNote() { 330 if (this.note == null) 331 this.note = new ArrayList<Annotation>(); 332 return this.note; 333 } 334 335 /** 336 * @return Returns a reference to <code>this</code> for easy method chaining 337 */ 338 public EvidenceReportSubjectComponent setNote(List<Annotation> theNote) { 339 this.note = theNote; 340 return this; 341 } 342 343 public boolean hasNote() { 344 if (this.note == null) 345 return false; 346 for (Annotation item : this.note) 347 if (!item.isEmpty()) 348 return true; 349 return false; 350 } 351 352 public Annotation addNote() { //3 353 Annotation t = new Annotation(); 354 if (this.note == null) 355 this.note = new ArrayList<Annotation>(); 356 this.note.add(t); 357 return t; 358 } 359 360 public EvidenceReportSubjectComponent addNote(Annotation t) { //3 361 if (t == null) 362 return this; 363 if (this.note == null) 364 this.note = new ArrayList<Annotation>(); 365 this.note.add(t); 366 return this; 367 } 368 369 /** 370 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 371 */ 372 public Annotation getNoteFirstRep() { 373 if (getNote().isEmpty()) { 374 addNote(); 375 } 376 return getNote().get(0); 377 } 378 379 protected void listChildren(List<Property> children) { 380 super.listChildren(children); 381 children.add(new Property("characteristic", "", "Characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 382 children.add(new Property("note", "Annotation", "Used for general notes and annotations not coded elsewhere.", 0, java.lang.Integer.MAX_VALUE, note)); 383 } 384 385 @Override 386 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 387 switch (_hash) { 388 case 366313883: /*characteristic*/ return new Property("characteristic", "", "Characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic); 389 case 3387378: /*note*/ return new Property("note", "Annotation", "Used for general notes and annotations not coded elsewhere.", 0, java.lang.Integer.MAX_VALUE, note); 390 default: return super.getNamedProperty(_hash, _name, _checkValid); 391 } 392 393 } 394 395 @Override 396 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 397 switch (hash) { 398 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceReportSubjectCharacteristicComponent 399 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 400 default: return super.getProperty(hash, name, checkValid); 401 } 402 403 } 404 405 @Override 406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 407 switch (hash) { 408 case 366313883: // characteristic 409 this.getCharacteristic().add((EvidenceReportSubjectCharacteristicComponent) value); // EvidenceReportSubjectCharacteristicComponent 410 return value; 411 case 3387378: // note 412 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 413 return value; 414 default: return super.setProperty(hash, name, value); 415 } 416 417 } 418 419 @Override 420 public Base setProperty(String name, Base value) throws FHIRException { 421 if (name.equals("characteristic")) { 422 this.getCharacteristic().add((EvidenceReportSubjectCharacteristicComponent) value); 423 } else if (name.equals("note")) { 424 this.getNote().add(TypeConvertor.castToAnnotation(value)); 425 } else 426 return super.setProperty(name, value); 427 return value; 428 } 429 430 @Override 431 public void removeChild(String name, Base value) throws FHIRException { 432 if (name.equals("characteristic")) { 433 this.getCharacteristic().remove((EvidenceReportSubjectCharacteristicComponent) value); 434 } else if (name.equals("note")) { 435 this.getNote().remove(value); 436 } else 437 super.removeChild(name, value); 438 439 } 440 441 @Override 442 public Base makeProperty(int hash, String name) throws FHIRException { 443 switch (hash) { 444 case 366313883: return addCharacteristic(); 445 case 3387378: return addNote(); 446 default: return super.makeProperty(hash, name); 447 } 448 449 } 450 451 @Override 452 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 453 switch (hash) { 454 case 366313883: /*characteristic*/ return new String[] {}; 455 case 3387378: /*note*/ return new String[] {"Annotation"}; 456 default: return super.getTypesForProperty(hash, name); 457 } 458 459 } 460 461 @Override 462 public Base addChild(String name) throws FHIRException { 463 if (name.equals("characteristic")) { 464 return addCharacteristic(); 465 } 466 else if (name.equals("note")) { 467 return addNote(); 468 } 469 else 470 return super.addChild(name); 471 } 472 473 public EvidenceReportSubjectComponent copy() { 474 EvidenceReportSubjectComponent dst = new EvidenceReportSubjectComponent(); 475 copyValues(dst); 476 return dst; 477 } 478 479 public void copyValues(EvidenceReportSubjectComponent dst) { 480 super.copyValues(dst); 481 if (characteristic != null) { 482 dst.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 483 for (EvidenceReportSubjectCharacteristicComponent i : characteristic) 484 dst.characteristic.add(i.copy()); 485 }; 486 if (note != null) { 487 dst.note = new ArrayList<Annotation>(); 488 for (Annotation i : note) 489 dst.note.add(i.copy()); 490 }; 491 } 492 493 @Override 494 public boolean equalsDeep(Base other_) { 495 if (!super.equalsDeep(other_)) 496 return false; 497 if (!(other_ instanceof EvidenceReportSubjectComponent)) 498 return false; 499 EvidenceReportSubjectComponent o = (EvidenceReportSubjectComponent) other_; 500 return compareDeep(characteristic, o.characteristic, true) && compareDeep(note, o.note, true); 501 } 502 503 @Override 504 public boolean equalsShallow(Base other_) { 505 if (!super.equalsShallow(other_)) 506 return false; 507 if (!(other_ instanceof EvidenceReportSubjectComponent)) 508 return false; 509 EvidenceReportSubjectComponent o = (EvidenceReportSubjectComponent) other_; 510 return true; 511 } 512 513 public boolean isEmpty() { 514 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(characteristic, note); 515 } 516 517 public String fhirType() { 518 return "EvidenceReport.subject"; 519 520 } 521 522 } 523 524 @Block() 525 public static class EvidenceReportSubjectCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 526 /** 527 * Characteristic code. 528 */ 529 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 530 @Description(shortDefinition="Characteristic code", formalDefinition="Characteristic code." ) 531 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/focus-characteristic-code") 532 protected CodeableConcept code; 533 534 /** 535 * Characteristic value. 536 */ 537 @Child(name = "value", type = {Reference.class, CodeableConcept.class, BooleanType.class, Quantity.class, Range.class}, order=2, min=1, max=1, modifier=false, summary=false) 538 @Description(shortDefinition="Characteristic value", formalDefinition="Characteristic value." ) 539 protected DataType value; 540 541 /** 542 * Is used to express not the characteristic. 543 */ 544 @Child(name = "exclude", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 545 @Description(shortDefinition="Is used to express not the characteristic", formalDefinition="Is used to express not the characteristic." ) 546 protected BooleanType exclude; 547 548 /** 549 * Timeframe for the characteristic. 550 */ 551 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 552 @Description(shortDefinition="Timeframe for the characteristic", formalDefinition="Timeframe for the characteristic." ) 553 protected Period period; 554 555 private static final long serialVersionUID = 279867823L; 556 557 /** 558 * Constructor 559 */ 560 public EvidenceReportSubjectCharacteristicComponent() { 561 super(); 562 } 563 564 /** 565 * Constructor 566 */ 567 public EvidenceReportSubjectCharacteristicComponent(CodeableConcept code, DataType value) { 568 super(); 569 this.setCode(code); 570 this.setValue(value); 571 } 572 573 /** 574 * @return {@link #code} (Characteristic code.) 575 */ 576 public CodeableConcept getCode() { 577 if (this.code == null) 578 if (Configuration.errorOnAutoCreate()) 579 throw new Error("Attempt to auto-create EvidenceReportSubjectCharacteristicComponent.code"); 580 else if (Configuration.doAutoCreate()) 581 this.code = new CodeableConcept(); // cc 582 return this.code; 583 } 584 585 public boolean hasCode() { 586 return this.code != null && !this.code.isEmpty(); 587 } 588 589 /** 590 * @param value {@link #code} (Characteristic code.) 591 */ 592 public EvidenceReportSubjectCharacteristicComponent setCode(CodeableConcept value) { 593 this.code = value; 594 return this; 595 } 596 597 /** 598 * @return {@link #value} (Characteristic value.) 599 */ 600 public DataType getValue() { 601 return this.value; 602 } 603 604 /** 605 * @return {@link #value} (Characteristic value.) 606 */ 607 public Reference getValueReference() throws FHIRException { 608 if (this.value == null) 609 this.value = new Reference(); 610 if (!(this.value instanceof Reference)) 611 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 612 return (Reference) this.value; 613 } 614 615 public boolean hasValueReference() { 616 return this != null && this.value instanceof Reference; 617 } 618 619 /** 620 * @return {@link #value} (Characteristic value.) 621 */ 622 public CodeableConcept getValueCodeableConcept() throws FHIRException { 623 if (this.value == null) 624 this.value = new CodeableConcept(); 625 if (!(this.value instanceof CodeableConcept)) 626 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 627 return (CodeableConcept) this.value; 628 } 629 630 public boolean hasValueCodeableConcept() { 631 return this != null && this.value instanceof CodeableConcept; 632 } 633 634 /** 635 * @return {@link #value} (Characteristic value.) 636 */ 637 public BooleanType getValueBooleanType() throws FHIRException { 638 if (this.value == null) 639 this.value = new BooleanType(); 640 if (!(this.value instanceof BooleanType)) 641 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 642 return (BooleanType) this.value; 643 } 644 645 public boolean hasValueBooleanType() { 646 return this != null && this.value instanceof BooleanType; 647 } 648 649 /** 650 * @return {@link #value} (Characteristic value.) 651 */ 652 public Quantity getValueQuantity() throws FHIRException { 653 if (this.value == null) 654 this.value = new Quantity(); 655 if (!(this.value instanceof Quantity)) 656 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 657 return (Quantity) this.value; 658 } 659 660 public boolean hasValueQuantity() { 661 return this != null && this.value instanceof Quantity; 662 } 663 664 /** 665 * @return {@link #value} (Characteristic value.) 666 */ 667 public Range getValueRange() throws FHIRException { 668 if (this.value == null) 669 this.value = new Range(); 670 if (!(this.value instanceof Range)) 671 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 672 return (Range) this.value; 673 } 674 675 public boolean hasValueRange() { 676 return this != null && this.value instanceof Range; 677 } 678 679 public boolean hasValue() { 680 return this.value != null && !this.value.isEmpty(); 681 } 682 683 /** 684 * @param value {@link #value} (Characteristic value.) 685 */ 686 public EvidenceReportSubjectCharacteristicComponent setValue(DataType value) { 687 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range)) 688 throw new FHIRException("Not the right type for EvidenceReport.subject.characteristic.value[x]: "+value.fhirType()); 689 this.value = value; 690 return this; 691 } 692 693 /** 694 * @return {@link #exclude} (Is used to express not the characteristic.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 695 */ 696 public BooleanType getExcludeElement() { 697 if (this.exclude == null) 698 if (Configuration.errorOnAutoCreate()) 699 throw new Error("Attempt to auto-create EvidenceReportSubjectCharacteristicComponent.exclude"); 700 else if (Configuration.doAutoCreate()) 701 this.exclude = new BooleanType(); // bb 702 return this.exclude; 703 } 704 705 public boolean hasExcludeElement() { 706 return this.exclude != null && !this.exclude.isEmpty(); 707 } 708 709 public boolean hasExclude() { 710 return this.exclude != null && !this.exclude.isEmpty(); 711 } 712 713 /** 714 * @param value {@link #exclude} (Is used to express not the characteristic.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 715 */ 716 public EvidenceReportSubjectCharacteristicComponent setExcludeElement(BooleanType value) { 717 this.exclude = value; 718 return this; 719 } 720 721 /** 722 * @return Is used to express not the characteristic. 723 */ 724 public boolean getExclude() { 725 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 726 } 727 728 /** 729 * @param value Is used to express not the characteristic. 730 */ 731 public EvidenceReportSubjectCharacteristicComponent setExclude(boolean value) { 732 if (this.exclude == null) 733 this.exclude = new BooleanType(); 734 this.exclude.setValue(value); 735 return this; 736 } 737 738 /** 739 * @return {@link #period} (Timeframe for the characteristic.) 740 */ 741 public Period getPeriod() { 742 if (this.period == null) 743 if (Configuration.errorOnAutoCreate()) 744 throw new Error("Attempt to auto-create EvidenceReportSubjectCharacteristicComponent.period"); 745 else if (Configuration.doAutoCreate()) 746 this.period = new Period(); // cc 747 return this.period; 748 } 749 750 public boolean hasPeriod() { 751 return this.period != null && !this.period.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #period} (Timeframe for the characteristic.) 756 */ 757 public EvidenceReportSubjectCharacteristicComponent setPeriod(Period value) { 758 this.period = value; 759 return this; 760 } 761 762 protected void listChildren(List<Property> children) { 763 super.listChildren(children); 764 children.add(new Property("code", "CodeableConcept", "Characteristic code.", 0, 1, code)); 765 children.add(new Property("value[x]", "Reference(Any)|CodeableConcept|boolean|Quantity|Range", "Characteristic value.", 0, 1, value)); 766 children.add(new Property("exclude", "boolean", "Is used to express not the characteristic.", 0, 1, exclude)); 767 children.add(new Property("period", "Period", "Timeframe for the characteristic.", 0, 1, period)); 768 } 769 770 @Override 771 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 772 switch (_hash) { 773 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Characteristic code.", 0, 1, code); 774 case -1410166417: /*value[x]*/ return new Property("value[x]", "Reference(Any)|CodeableConcept|boolean|Quantity|Range", "Characteristic value.", 0, 1, value); 775 case 111972721: /*value*/ return new Property("value[x]", "Reference(Any)|CodeableConcept|boolean|Quantity|Range", "Characteristic value.", 0, 1, value); 776 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "Characteristic value.", 0, 1, value); 777 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Characteristic value.", 0, 1, value); 778 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Characteristic value.", 0, 1, value); 779 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Characteristic value.", 0, 1, value); 780 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Characteristic value.", 0, 1, value); 781 case -1321148966: /*exclude*/ return new Property("exclude", "boolean", "Is used to express not the characteristic.", 0, 1, exclude); 782 case -991726143: /*period*/ return new Property("period", "Period", "Timeframe for the characteristic.", 0, 1, period); 783 default: return super.getNamedProperty(_hash, _name, _checkValid); 784 } 785 786 } 787 788 @Override 789 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 790 switch (hash) { 791 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 792 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 793 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // BooleanType 794 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 795 default: return super.getProperty(hash, name, checkValid); 796 } 797 798 } 799 800 @Override 801 public Base setProperty(int hash, String name, Base value) throws FHIRException { 802 switch (hash) { 803 case 3059181: // code 804 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 805 return value; 806 case 111972721: // value 807 this.value = TypeConvertor.castToType(value); // DataType 808 return value; 809 case -1321148966: // exclude 810 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 811 return value; 812 case -991726143: // period 813 this.period = TypeConvertor.castToPeriod(value); // Period 814 return value; 815 default: return super.setProperty(hash, name, value); 816 } 817 818 } 819 820 @Override 821 public Base setProperty(String name, Base value) throws FHIRException { 822 if (name.equals("code")) { 823 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 824 } else if (name.equals("value[x]")) { 825 this.value = TypeConvertor.castToType(value); // DataType 826 } else if (name.equals("exclude")) { 827 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 828 } else if (name.equals("period")) { 829 this.period = TypeConvertor.castToPeriod(value); // Period 830 } else 831 return super.setProperty(name, value); 832 return value; 833 } 834 835 @Override 836 public void removeChild(String name, Base value) throws FHIRException { 837 if (name.equals("code")) { 838 this.code = null; 839 } else if (name.equals("value[x]")) { 840 this.value = null; 841 } else if (name.equals("exclude")) { 842 this.exclude = null; 843 } else if (name.equals("period")) { 844 this.period = null; 845 } else 846 super.removeChild(name, value); 847 848 } 849 850 @Override 851 public Base makeProperty(int hash, String name) throws FHIRException { 852 switch (hash) { 853 case 3059181: return getCode(); 854 case -1410166417: return getValue(); 855 case 111972721: return getValue(); 856 case -1321148966: return getExcludeElement(); 857 case -991726143: return getPeriod(); 858 default: return super.makeProperty(hash, name); 859 } 860 861 } 862 863 @Override 864 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 865 switch (hash) { 866 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 867 case 111972721: /*value*/ return new String[] {"Reference", "CodeableConcept", "boolean", "Quantity", "Range"}; 868 case -1321148966: /*exclude*/ return new String[] {"boolean"}; 869 case -991726143: /*period*/ return new String[] {"Period"}; 870 default: return super.getTypesForProperty(hash, name); 871 } 872 873 } 874 875 @Override 876 public Base addChild(String name) throws FHIRException { 877 if (name.equals("code")) { 878 this.code = new CodeableConcept(); 879 return this.code; 880 } 881 else if (name.equals("valueReference")) { 882 this.value = new Reference(); 883 return this.value; 884 } 885 else if (name.equals("valueCodeableConcept")) { 886 this.value = new CodeableConcept(); 887 return this.value; 888 } 889 else if (name.equals("valueBoolean")) { 890 this.value = new BooleanType(); 891 return this.value; 892 } 893 else if (name.equals("valueQuantity")) { 894 this.value = new Quantity(); 895 return this.value; 896 } 897 else if (name.equals("valueRange")) { 898 this.value = new Range(); 899 return this.value; 900 } 901 else if (name.equals("exclude")) { 902 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.subject.characteristic.exclude"); 903 } 904 else if (name.equals("period")) { 905 this.period = new Period(); 906 return this.period; 907 } 908 else 909 return super.addChild(name); 910 } 911 912 public EvidenceReportSubjectCharacteristicComponent copy() { 913 EvidenceReportSubjectCharacteristicComponent dst = new EvidenceReportSubjectCharacteristicComponent(); 914 copyValues(dst); 915 return dst; 916 } 917 918 public void copyValues(EvidenceReportSubjectCharacteristicComponent dst) { 919 super.copyValues(dst); 920 dst.code = code == null ? null : code.copy(); 921 dst.value = value == null ? null : value.copy(); 922 dst.exclude = exclude == null ? null : exclude.copy(); 923 dst.period = period == null ? null : period.copy(); 924 } 925 926 @Override 927 public boolean equalsDeep(Base other_) { 928 if (!super.equalsDeep(other_)) 929 return false; 930 if (!(other_ instanceof EvidenceReportSubjectCharacteristicComponent)) 931 return false; 932 EvidenceReportSubjectCharacteristicComponent o = (EvidenceReportSubjectCharacteristicComponent) other_; 933 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(exclude, o.exclude, true) 934 && compareDeep(period, o.period, true); 935 } 936 937 @Override 938 public boolean equalsShallow(Base other_) { 939 if (!super.equalsShallow(other_)) 940 return false; 941 if (!(other_ instanceof EvidenceReportSubjectCharacteristicComponent)) 942 return false; 943 EvidenceReportSubjectCharacteristicComponent o = (EvidenceReportSubjectCharacteristicComponent) other_; 944 return compareValues(exclude, o.exclude, true); 945 } 946 947 public boolean isEmpty() { 948 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, exclude, period 949 ); 950 } 951 952 public String fhirType() { 953 return "EvidenceReport.subject.characteristic"; 954 955 } 956 957 } 958 959 @Block() 960 public static class EvidenceReportRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 961 /** 962 * The type of relationship that this composition has with anther composition or document. 963 */ 964 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 965 @Description(shortDefinition="replaces | amends | appends | transforms | replacedWith | amendedWith | appendedWith | transformedWith", formalDefinition="The type of relationship that this composition has with anther composition or document." ) 966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-relation-type") 967 protected Enumeration<ReportRelationshipType> code; 968 969 /** 970 * The target composition/document of this relationship. 971 */ 972 @Child(name = "target", type = {}, order=2, min=1, max=1, modifier=false, summary=false) 973 @Description(shortDefinition="Target of the relationship", formalDefinition="The target composition/document of this relationship." ) 974 protected EvidenceReportRelatesToTargetComponent target; 975 976 private static final long serialVersionUID = -1716908969L; 977 978 /** 979 * Constructor 980 */ 981 public EvidenceReportRelatesToComponent() { 982 super(); 983 } 984 985 /** 986 * Constructor 987 */ 988 public EvidenceReportRelatesToComponent(ReportRelationshipType code, EvidenceReportRelatesToTargetComponent target) { 989 super(); 990 this.setCode(code); 991 this.setTarget(target); 992 } 993 994 /** 995 * @return {@link #code} (The type of relationship that this composition has with anther composition or document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 996 */ 997 public Enumeration<ReportRelationshipType> getCodeElement() { 998 if (this.code == null) 999 if (Configuration.errorOnAutoCreate()) 1000 throw new Error("Attempt to auto-create EvidenceReportRelatesToComponent.code"); 1001 else if (Configuration.doAutoCreate()) 1002 this.code = new Enumeration<ReportRelationshipType>(new ReportRelationshipTypeEnumFactory()); // bb 1003 return this.code; 1004 } 1005 1006 public boolean hasCodeElement() { 1007 return this.code != null && !this.code.isEmpty(); 1008 } 1009 1010 public boolean hasCode() { 1011 return this.code != null && !this.code.isEmpty(); 1012 } 1013 1014 /** 1015 * @param value {@link #code} (The type of relationship that this composition has with anther composition or document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1016 */ 1017 public EvidenceReportRelatesToComponent setCodeElement(Enumeration<ReportRelationshipType> value) { 1018 this.code = value; 1019 return this; 1020 } 1021 1022 /** 1023 * @return The type of relationship that this composition has with anther composition or document. 1024 */ 1025 public ReportRelationshipType getCode() { 1026 return this.code == null ? null : this.code.getValue(); 1027 } 1028 1029 /** 1030 * @param value The type of relationship that this composition has with anther composition or document. 1031 */ 1032 public EvidenceReportRelatesToComponent setCode(ReportRelationshipType value) { 1033 if (this.code == null) 1034 this.code = new Enumeration<ReportRelationshipType>(new ReportRelationshipTypeEnumFactory()); 1035 this.code.setValue(value); 1036 return this; 1037 } 1038 1039 /** 1040 * @return {@link #target} (The target composition/document of this relationship.) 1041 */ 1042 public EvidenceReportRelatesToTargetComponent getTarget() { 1043 if (this.target == null) 1044 if (Configuration.errorOnAutoCreate()) 1045 throw new Error("Attempt to auto-create EvidenceReportRelatesToComponent.target"); 1046 else if (Configuration.doAutoCreate()) 1047 this.target = new EvidenceReportRelatesToTargetComponent(); // cc 1048 return this.target; 1049 } 1050 1051 public boolean hasTarget() { 1052 return this.target != null && !this.target.isEmpty(); 1053 } 1054 1055 /** 1056 * @param value {@link #target} (The target composition/document of this relationship.) 1057 */ 1058 public EvidenceReportRelatesToComponent setTarget(EvidenceReportRelatesToTargetComponent value) { 1059 this.target = value; 1060 return this; 1061 } 1062 1063 protected void listChildren(List<Property> children) { 1064 super.listChildren(children); 1065 children.add(new Property("code", "code", "The type of relationship that this composition has with anther composition or document.", 0, 1, code)); 1066 children.add(new Property("target", "", "The target composition/document of this relationship.", 0, 1, target)); 1067 } 1068 1069 @Override 1070 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1071 switch (_hash) { 1072 case 3059181: /*code*/ return new Property("code", "code", "The type of relationship that this composition has with anther composition or document.", 0, 1, code); 1073 case -880905839: /*target*/ return new Property("target", "", "The target composition/document of this relationship.", 0, 1, target); 1074 default: return super.getNamedProperty(_hash, _name, _checkValid); 1075 } 1076 1077 } 1078 1079 @Override 1080 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1081 switch (hash) { 1082 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<ReportRelationshipType> 1083 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // EvidenceReportRelatesToTargetComponent 1084 default: return super.getProperty(hash, name, checkValid); 1085 } 1086 1087 } 1088 1089 @Override 1090 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1091 switch (hash) { 1092 case 3059181: // code 1093 value = new ReportRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1094 this.code = (Enumeration) value; // Enumeration<ReportRelationshipType> 1095 return value; 1096 case -880905839: // target 1097 this.target = (EvidenceReportRelatesToTargetComponent) value; // EvidenceReportRelatesToTargetComponent 1098 return value; 1099 default: return super.setProperty(hash, name, value); 1100 } 1101 1102 } 1103 1104 @Override 1105 public Base setProperty(String name, Base value) throws FHIRException { 1106 if (name.equals("code")) { 1107 value = new ReportRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1108 this.code = (Enumeration) value; // Enumeration<ReportRelationshipType> 1109 } else if (name.equals("target")) { 1110 this.target = (EvidenceReportRelatesToTargetComponent) value; // EvidenceReportRelatesToTargetComponent 1111 } else 1112 return super.setProperty(name, value); 1113 return value; 1114 } 1115 1116 @Override 1117 public void removeChild(String name, Base value) throws FHIRException { 1118 if (name.equals("code")) { 1119 value = new ReportRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1120 this.code = (Enumeration) value; // Enumeration<ReportRelationshipType> 1121 } else if (name.equals("target")) { 1122 this.target = (EvidenceReportRelatesToTargetComponent) value; // EvidenceReportRelatesToTargetComponent 1123 } else 1124 super.removeChild(name, value); 1125 1126 } 1127 1128 @Override 1129 public Base makeProperty(int hash, String name) throws FHIRException { 1130 switch (hash) { 1131 case 3059181: return getCodeElement(); 1132 case -880905839: return getTarget(); 1133 default: return super.makeProperty(hash, name); 1134 } 1135 1136 } 1137 1138 @Override 1139 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1140 switch (hash) { 1141 case 3059181: /*code*/ return new String[] {"code"}; 1142 case -880905839: /*target*/ return new String[] {}; 1143 default: return super.getTypesForProperty(hash, name); 1144 } 1145 1146 } 1147 1148 @Override 1149 public Base addChild(String name) throws FHIRException { 1150 if (name.equals("code")) { 1151 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.relatesTo.code"); 1152 } 1153 else if (name.equals("target")) { 1154 this.target = new EvidenceReportRelatesToTargetComponent(); 1155 return this.target; 1156 } 1157 else 1158 return super.addChild(name); 1159 } 1160 1161 public EvidenceReportRelatesToComponent copy() { 1162 EvidenceReportRelatesToComponent dst = new EvidenceReportRelatesToComponent(); 1163 copyValues(dst); 1164 return dst; 1165 } 1166 1167 public void copyValues(EvidenceReportRelatesToComponent dst) { 1168 super.copyValues(dst); 1169 dst.code = code == null ? null : code.copy(); 1170 dst.target = target == null ? null : target.copy(); 1171 } 1172 1173 @Override 1174 public boolean equalsDeep(Base other_) { 1175 if (!super.equalsDeep(other_)) 1176 return false; 1177 if (!(other_ instanceof EvidenceReportRelatesToComponent)) 1178 return false; 1179 EvidenceReportRelatesToComponent o = (EvidenceReportRelatesToComponent) other_; 1180 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 1181 } 1182 1183 @Override 1184 public boolean equalsShallow(Base other_) { 1185 if (!super.equalsShallow(other_)) 1186 return false; 1187 if (!(other_ instanceof EvidenceReportRelatesToComponent)) 1188 return false; 1189 EvidenceReportRelatesToComponent o = (EvidenceReportRelatesToComponent) other_; 1190 return compareValues(code, o.code, true); 1191 } 1192 1193 public boolean isEmpty() { 1194 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 1195 } 1196 1197 public String fhirType() { 1198 return "EvidenceReport.relatesTo"; 1199 1200 } 1201 1202 } 1203 1204 @Block() 1205 public static class EvidenceReportRelatesToTargetComponent extends BackboneElement implements IBaseBackboneElement { 1206 /** 1207 * Target of the relationship URL. 1208 */ 1209 @Child(name = "url", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1210 @Description(shortDefinition="Target of the relationship URL", formalDefinition="Target of the relationship URL." ) 1211 protected UriType url; 1212 1213 /** 1214 * Target of the relationship Identifier. 1215 */ 1216 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 1217 @Description(shortDefinition="Target of the relationship Identifier", formalDefinition="Target of the relationship Identifier." ) 1218 protected Identifier identifier; 1219 1220 /** 1221 * Target of the relationship Display. 1222 */ 1223 @Child(name = "display", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1224 @Description(shortDefinition="Target of the relationship Display", formalDefinition="Target of the relationship Display." ) 1225 protected MarkdownType display; 1226 1227 /** 1228 * Target of the relationship Resource reference. 1229 */ 1230 @Child(name = "resource", type = {Reference.class}, order=4, min=0, max=1, modifier=false, summary=false) 1231 @Description(shortDefinition="Target of the relationship Resource reference", formalDefinition="Target of the relationship Resource reference." ) 1232 protected Reference resource; 1233 1234 private static final long serialVersionUID = -804526425L; 1235 1236 /** 1237 * Constructor 1238 */ 1239 public EvidenceReportRelatesToTargetComponent() { 1240 super(); 1241 } 1242 1243 /** 1244 * @return {@link #url} (Target of the relationship URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1245 */ 1246 public UriType getUrlElement() { 1247 if (this.url == null) 1248 if (Configuration.errorOnAutoCreate()) 1249 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.url"); 1250 else if (Configuration.doAutoCreate()) 1251 this.url = new UriType(); // bb 1252 return this.url; 1253 } 1254 1255 public boolean hasUrlElement() { 1256 return this.url != null && !this.url.isEmpty(); 1257 } 1258 1259 public boolean hasUrl() { 1260 return this.url != null && !this.url.isEmpty(); 1261 } 1262 1263 /** 1264 * @param value {@link #url} (Target of the relationship URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1265 */ 1266 public EvidenceReportRelatesToTargetComponent setUrlElement(UriType value) { 1267 this.url = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return Target of the relationship URL. 1273 */ 1274 public String getUrl() { 1275 return this.url == null ? null : this.url.getValue(); 1276 } 1277 1278 /** 1279 * @param value Target of the relationship URL. 1280 */ 1281 public EvidenceReportRelatesToTargetComponent setUrl(String value) { 1282 if (Utilities.noString(value)) 1283 this.url = null; 1284 else { 1285 if (this.url == null) 1286 this.url = new UriType(); 1287 this.url.setValue(value); 1288 } 1289 return this; 1290 } 1291 1292 /** 1293 * @return {@link #identifier} (Target of the relationship Identifier.) 1294 */ 1295 public Identifier getIdentifier() { 1296 if (this.identifier == null) 1297 if (Configuration.errorOnAutoCreate()) 1298 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.identifier"); 1299 else if (Configuration.doAutoCreate()) 1300 this.identifier = new Identifier(); // cc 1301 return this.identifier; 1302 } 1303 1304 public boolean hasIdentifier() { 1305 return this.identifier != null && !this.identifier.isEmpty(); 1306 } 1307 1308 /** 1309 * @param value {@link #identifier} (Target of the relationship Identifier.) 1310 */ 1311 public EvidenceReportRelatesToTargetComponent setIdentifier(Identifier value) { 1312 this.identifier = value; 1313 return this; 1314 } 1315 1316 /** 1317 * @return {@link #display} (Target of the relationship Display.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1318 */ 1319 public MarkdownType getDisplayElement() { 1320 if (this.display == null) 1321 if (Configuration.errorOnAutoCreate()) 1322 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.display"); 1323 else if (Configuration.doAutoCreate()) 1324 this.display = new MarkdownType(); // bb 1325 return this.display; 1326 } 1327 1328 public boolean hasDisplayElement() { 1329 return this.display != null && !this.display.isEmpty(); 1330 } 1331 1332 public boolean hasDisplay() { 1333 return this.display != null && !this.display.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #display} (Target of the relationship Display.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1338 */ 1339 public EvidenceReportRelatesToTargetComponent setDisplayElement(MarkdownType value) { 1340 this.display = value; 1341 return this; 1342 } 1343 1344 /** 1345 * @return Target of the relationship Display. 1346 */ 1347 public String getDisplay() { 1348 return this.display == null ? null : this.display.getValue(); 1349 } 1350 1351 /** 1352 * @param value Target of the relationship Display. 1353 */ 1354 public EvidenceReportRelatesToTargetComponent setDisplay(String value) { 1355 if (Utilities.noString(value)) 1356 this.display = null; 1357 else { 1358 if (this.display == null) 1359 this.display = new MarkdownType(); 1360 this.display.setValue(value); 1361 } 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #resource} (Target of the relationship Resource reference.) 1367 */ 1368 public Reference getResource() { 1369 if (this.resource == null) 1370 if (Configuration.errorOnAutoCreate()) 1371 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.resource"); 1372 else if (Configuration.doAutoCreate()) 1373 this.resource = new Reference(); // cc 1374 return this.resource; 1375 } 1376 1377 public boolean hasResource() { 1378 return this.resource != null && !this.resource.isEmpty(); 1379 } 1380 1381 /** 1382 * @param value {@link #resource} (Target of the relationship Resource reference.) 1383 */ 1384 public EvidenceReportRelatesToTargetComponent setResource(Reference value) { 1385 this.resource = value; 1386 return this; 1387 } 1388 1389 protected void listChildren(List<Property> children) { 1390 super.listChildren(children); 1391 children.add(new Property("url", "uri", "Target of the relationship URL.", 0, 1, url)); 1392 children.add(new Property("identifier", "Identifier", "Target of the relationship Identifier.", 0, 1, identifier)); 1393 children.add(new Property("display", "markdown", "Target of the relationship Display.", 0, 1, display)); 1394 children.add(new Property("resource", "Reference(Any)", "Target of the relationship Resource reference.", 0, 1, resource)); 1395 } 1396 1397 @Override 1398 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1399 switch (_hash) { 1400 case 116079: /*url*/ return new Property("url", "uri", "Target of the relationship URL.", 0, 1, url); 1401 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Target of the relationship Identifier.", 0, 1, identifier); 1402 case 1671764162: /*display*/ return new Property("display", "markdown", "Target of the relationship Display.", 0, 1, display); 1403 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "Target of the relationship Resource reference.", 0, 1, resource); 1404 default: return super.getNamedProperty(_hash, _name, _checkValid); 1405 } 1406 1407 } 1408 1409 @Override 1410 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1411 switch (hash) { 1412 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1413 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1414 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // MarkdownType 1415 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 1416 default: return super.getProperty(hash, name, checkValid); 1417 } 1418 1419 } 1420 1421 @Override 1422 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1423 switch (hash) { 1424 case 116079: // url 1425 this.url = TypeConvertor.castToUri(value); // UriType 1426 return value; 1427 case -1618432855: // identifier 1428 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1429 return value; 1430 case 1671764162: // display 1431 this.display = TypeConvertor.castToMarkdown(value); // MarkdownType 1432 return value; 1433 case -341064690: // resource 1434 this.resource = TypeConvertor.castToReference(value); // Reference 1435 return value; 1436 default: return super.setProperty(hash, name, value); 1437 } 1438 1439 } 1440 1441 @Override 1442 public Base setProperty(String name, Base value) throws FHIRException { 1443 if (name.equals("url")) { 1444 this.url = TypeConvertor.castToUri(value); // UriType 1445 } else if (name.equals("identifier")) { 1446 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1447 } else if (name.equals("display")) { 1448 this.display = TypeConvertor.castToMarkdown(value); // MarkdownType 1449 } else if (name.equals("resource")) { 1450 this.resource = TypeConvertor.castToReference(value); // Reference 1451 } else 1452 return super.setProperty(name, value); 1453 return value; 1454 } 1455 1456 @Override 1457 public void removeChild(String name, Base value) throws FHIRException { 1458 if (name.equals("url")) { 1459 this.url = null; 1460 } else if (name.equals("identifier")) { 1461 this.identifier = null; 1462 } else if (name.equals("display")) { 1463 this.display = null; 1464 } else if (name.equals("resource")) { 1465 this.resource = null; 1466 } else 1467 super.removeChild(name, value); 1468 1469 } 1470 1471 @Override 1472 public Base makeProperty(int hash, String name) throws FHIRException { 1473 switch (hash) { 1474 case 116079: return getUrlElement(); 1475 case -1618432855: return getIdentifier(); 1476 case 1671764162: return getDisplayElement(); 1477 case -341064690: return getResource(); 1478 default: return super.makeProperty(hash, name); 1479 } 1480 1481 } 1482 1483 @Override 1484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1485 switch (hash) { 1486 case 116079: /*url*/ return new String[] {"uri"}; 1487 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1488 case 1671764162: /*display*/ return new String[] {"markdown"}; 1489 case -341064690: /*resource*/ return new String[] {"Reference"}; 1490 default: return super.getTypesForProperty(hash, name); 1491 } 1492 1493 } 1494 1495 @Override 1496 public Base addChild(String name) throws FHIRException { 1497 if (name.equals("url")) { 1498 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.relatesTo.target.url"); 1499 } 1500 else if (name.equals("identifier")) { 1501 this.identifier = new Identifier(); 1502 return this.identifier; 1503 } 1504 else if (name.equals("display")) { 1505 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.relatesTo.target.display"); 1506 } 1507 else if (name.equals("resource")) { 1508 this.resource = new Reference(); 1509 return this.resource; 1510 } 1511 else 1512 return super.addChild(name); 1513 } 1514 1515 public EvidenceReportRelatesToTargetComponent copy() { 1516 EvidenceReportRelatesToTargetComponent dst = new EvidenceReportRelatesToTargetComponent(); 1517 copyValues(dst); 1518 return dst; 1519 } 1520 1521 public void copyValues(EvidenceReportRelatesToTargetComponent dst) { 1522 super.copyValues(dst); 1523 dst.url = url == null ? null : url.copy(); 1524 dst.identifier = identifier == null ? null : identifier.copy(); 1525 dst.display = display == null ? null : display.copy(); 1526 dst.resource = resource == null ? null : resource.copy(); 1527 } 1528 1529 @Override 1530 public boolean equalsDeep(Base other_) { 1531 if (!super.equalsDeep(other_)) 1532 return false; 1533 if (!(other_ instanceof EvidenceReportRelatesToTargetComponent)) 1534 return false; 1535 EvidenceReportRelatesToTargetComponent o = (EvidenceReportRelatesToTargetComponent) other_; 1536 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(display, o.display, true) 1537 && compareDeep(resource, o.resource, true); 1538 } 1539 1540 @Override 1541 public boolean equalsShallow(Base other_) { 1542 if (!super.equalsShallow(other_)) 1543 return false; 1544 if (!(other_ instanceof EvidenceReportRelatesToTargetComponent)) 1545 return false; 1546 EvidenceReportRelatesToTargetComponent o = (EvidenceReportRelatesToTargetComponent) other_; 1547 return compareValues(url, o.url, true) && compareValues(display, o.display, true); 1548 } 1549 1550 public boolean isEmpty() { 1551 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, display 1552 , resource); 1553 } 1554 1555 public String fhirType() { 1556 return "EvidenceReport.relatesTo.target"; 1557 1558 } 1559 1560 } 1561 1562 @Block() 1563 public static class SectionComponent extends BackboneElement implements IBaseBackboneElement { 1564 /** 1565 * The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1566 */ 1567 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1568 @Description(shortDefinition="Label for section (e.g. for ToC)", formalDefinition="The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents." ) 1569 protected StringType title; 1570 1571 /** 1572 * A code identifying the kind of content contained within the section. This should be consistent with the section title. 1573 */ 1574 @Child(name = "focus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1575 @Description(shortDefinition="Classification of section (recommended)", formalDefinition="A code identifying the kind of content contained within the section. This should be consistent with the section title." ) 1576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-report-section") 1577 protected CodeableConcept focus; 1578 1579 /** 1580 * A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title. 1581 */ 1582 @Child(name = "focusReference", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 1583 @Description(shortDefinition="Classification of section by Resource", formalDefinition="A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title." ) 1584 protected Reference focusReference; 1585 1586 /** 1587 * Identifies who is responsible for the information in this section, not necessarily who typed it in. 1588 */ 1589 @Child(name = "author", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Device.class, Group.class, Organization.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1590 @Description(shortDefinition="Who and/or what authored the section", formalDefinition="Identifies who is responsible for the information in this section, not necessarily who typed it in." ) 1591 protected List<Reference> author; 1592 1593 /** 1594 * A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative. 1595 */ 1596 @Child(name = "text", type = {Narrative.class}, order=5, min=0, max=1, modifier=false, summary=false) 1597 @Description(shortDefinition="Text summary of the section, for human interpretation", formalDefinition="A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative." ) 1598 protected Narrative text; 1599 1600 /** 1601 * How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1602 */ 1603 @Child(name = "mode", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1604 @Description(shortDefinition="working | snapshot | changes", formalDefinition="How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted." ) 1605 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-mode") 1606 protected Enumeration<ListMode> mode; 1607 1608 /** 1609 * Specifies the order applied to the items in the section entries. 1610 */ 1611 @Child(name = "orderedBy", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1612 @Description(shortDefinition="Order of section entries", formalDefinition="Specifies the order applied to the items in the section entries." ) 1613 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-order") 1614 protected CodeableConcept orderedBy; 1615 1616 /** 1617 * Specifies any type of classification of the evidence report. 1618 */ 1619 @Child(name = "entryClassifier", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1620 @Description(shortDefinition="Extensible classifiers as content", formalDefinition="Specifies any type of classification of the evidence report." ) 1621 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-classifier-code") 1622 protected List<CodeableConcept> entryClassifier; 1623 1624 /** 1625 * A reference to the actual resource from which the narrative in the section is derived. 1626 */ 1627 @Child(name = "entryReference", type = {Reference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1628 @Description(shortDefinition="Reference to resources as content", formalDefinition="A reference to the actual resource from which the narrative in the section is derived." ) 1629 protected List<Reference> entryReference; 1630 1631 /** 1632 * Quantity as content. 1633 */ 1634 @Child(name = "entryQuantity", type = {Quantity.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1635 @Description(shortDefinition="Quantity as content", formalDefinition="Quantity as content." ) 1636 protected List<Quantity> entryQuantity; 1637 1638 /** 1639 * If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason. 1640 */ 1641 @Child(name = "emptyReason", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=false) 1642 @Description(shortDefinition="Why the section is empty", formalDefinition="If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason." ) 1643 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-empty-reason") 1644 protected CodeableConcept emptyReason; 1645 1646 /** 1647 * A nested sub-section within this section. 1648 */ 1649 @Child(name = "section", type = {SectionComponent.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1650 @Description(shortDefinition="Nested Section", formalDefinition="A nested sub-section within this section." ) 1651 protected List<SectionComponent> section; 1652 1653 private static final long serialVersionUID = -324854168L; 1654 1655 /** 1656 * Constructor 1657 */ 1658 public SectionComponent() { 1659 super(); 1660 } 1661 1662 /** 1663 * @return {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1664 */ 1665 public StringType getTitleElement() { 1666 if (this.title == null) 1667 if (Configuration.errorOnAutoCreate()) 1668 throw new Error("Attempt to auto-create SectionComponent.title"); 1669 else if (Configuration.doAutoCreate()) 1670 this.title = new StringType(); // bb 1671 return this.title; 1672 } 1673 1674 public boolean hasTitleElement() { 1675 return this.title != null && !this.title.isEmpty(); 1676 } 1677 1678 public boolean hasTitle() { 1679 return this.title != null && !this.title.isEmpty(); 1680 } 1681 1682 /** 1683 * @param value {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1684 */ 1685 public SectionComponent setTitleElement(StringType value) { 1686 this.title = value; 1687 return this; 1688 } 1689 1690 /** 1691 * @return The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1692 */ 1693 public String getTitle() { 1694 return this.title == null ? null : this.title.getValue(); 1695 } 1696 1697 /** 1698 * @param value The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1699 */ 1700 public SectionComponent setTitle(String value) { 1701 if (Utilities.noString(value)) 1702 this.title = null; 1703 else { 1704 if (this.title == null) 1705 this.title = new StringType(); 1706 this.title.setValue(value); 1707 } 1708 return this; 1709 } 1710 1711 /** 1712 * @return {@link #focus} (A code identifying the kind of content contained within the section. This should be consistent with the section title.) 1713 */ 1714 public CodeableConcept getFocus() { 1715 if (this.focus == null) 1716 if (Configuration.errorOnAutoCreate()) 1717 throw new Error("Attempt to auto-create SectionComponent.focus"); 1718 else if (Configuration.doAutoCreate()) 1719 this.focus = new CodeableConcept(); // cc 1720 return this.focus; 1721 } 1722 1723 public boolean hasFocus() { 1724 return this.focus != null && !this.focus.isEmpty(); 1725 } 1726 1727 /** 1728 * @param value {@link #focus} (A code identifying the kind of content contained within the section. This should be consistent with the section title.) 1729 */ 1730 public SectionComponent setFocus(CodeableConcept value) { 1731 this.focus = value; 1732 return this; 1733 } 1734 1735 /** 1736 * @return {@link #focusReference} (A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.) 1737 */ 1738 public Reference getFocusReference() { 1739 if (this.focusReference == null) 1740 if (Configuration.errorOnAutoCreate()) 1741 throw new Error("Attempt to auto-create SectionComponent.focusReference"); 1742 else if (Configuration.doAutoCreate()) 1743 this.focusReference = new Reference(); // cc 1744 return this.focusReference; 1745 } 1746 1747 public boolean hasFocusReference() { 1748 return this.focusReference != null && !this.focusReference.isEmpty(); 1749 } 1750 1751 /** 1752 * @param value {@link #focusReference} (A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.) 1753 */ 1754 public SectionComponent setFocusReference(Reference value) { 1755 this.focusReference = value; 1756 return this; 1757 } 1758 1759 /** 1760 * @return {@link #author} (Identifies who is responsible for the information in this section, not necessarily who typed it in.) 1761 */ 1762 public List<Reference> getAuthor() { 1763 if (this.author == null) 1764 this.author = new ArrayList<Reference>(); 1765 return this.author; 1766 } 1767 1768 /** 1769 * @return Returns a reference to <code>this</code> for easy method chaining 1770 */ 1771 public SectionComponent setAuthor(List<Reference> theAuthor) { 1772 this.author = theAuthor; 1773 return this; 1774 } 1775 1776 public boolean hasAuthor() { 1777 if (this.author == null) 1778 return false; 1779 for (Reference item : this.author) 1780 if (!item.isEmpty()) 1781 return true; 1782 return false; 1783 } 1784 1785 public Reference addAuthor() { //3 1786 Reference t = new Reference(); 1787 if (this.author == null) 1788 this.author = new ArrayList<Reference>(); 1789 this.author.add(t); 1790 return t; 1791 } 1792 1793 public SectionComponent addAuthor(Reference t) { //3 1794 if (t == null) 1795 return this; 1796 if (this.author == null) 1797 this.author = new ArrayList<Reference>(); 1798 this.author.add(t); 1799 return this; 1800 } 1801 1802 /** 1803 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 1804 */ 1805 public Reference getAuthorFirstRep() { 1806 if (getAuthor().isEmpty()) { 1807 addAuthor(); 1808 } 1809 return getAuthor().get(0); 1810 } 1811 1812 /** 1813 * @return {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.) 1814 */ 1815 public Narrative getText() { 1816 if (this.text == null) 1817 if (Configuration.errorOnAutoCreate()) 1818 throw new Error("Attempt to auto-create SectionComponent.text"); 1819 else if (Configuration.doAutoCreate()) 1820 this.text = new Narrative(); // cc 1821 return this.text; 1822 } 1823 1824 public boolean hasText() { 1825 return this.text != null && !this.text.isEmpty(); 1826 } 1827 1828 /** 1829 * @param value {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.) 1830 */ 1831 public SectionComponent setText(Narrative value) { 1832 this.text = value; 1833 return this; 1834 } 1835 1836 /** 1837 * @return {@link #mode} (How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1838 */ 1839 public Enumeration<ListMode> getModeElement() { 1840 if (this.mode == null) 1841 if (Configuration.errorOnAutoCreate()) 1842 throw new Error("Attempt to auto-create SectionComponent.mode"); 1843 else if (Configuration.doAutoCreate()) 1844 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); // bb 1845 return this.mode; 1846 } 1847 1848 public boolean hasModeElement() { 1849 return this.mode != null && !this.mode.isEmpty(); 1850 } 1851 1852 public boolean hasMode() { 1853 return this.mode != null && !this.mode.isEmpty(); 1854 } 1855 1856 /** 1857 * @param value {@link #mode} (How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1858 */ 1859 public SectionComponent setModeElement(Enumeration<ListMode> value) { 1860 this.mode = value; 1861 return this; 1862 } 1863 1864 /** 1865 * @return How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1866 */ 1867 public ListMode getMode() { 1868 return this.mode == null ? null : this.mode.getValue(); 1869 } 1870 1871 /** 1872 * @param value How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1873 */ 1874 public SectionComponent setMode(ListMode value) { 1875 if (value == null) 1876 this.mode = null; 1877 else { 1878 if (this.mode == null) 1879 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); 1880 this.mode.setValue(value); 1881 } 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 1887 */ 1888 public CodeableConcept getOrderedBy() { 1889 if (this.orderedBy == null) 1890 if (Configuration.errorOnAutoCreate()) 1891 throw new Error("Attempt to auto-create SectionComponent.orderedBy"); 1892 else if (Configuration.doAutoCreate()) 1893 this.orderedBy = new CodeableConcept(); // cc 1894 return this.orderedBy; 1895 } 1896 1897 public boolean hasOrderedBy() { 1898 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1899 } 1900 1901 /** 1902 * @param value {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 1903 */ 1904 public SectionComponent setOrderedBy(CodeableConcept value) { 1905 this.orderedBy = value; 1906 return this; 1907 } 1908 1909 /** 1910 * @return {@link #entryClassifier} (Specifies any type of classification of the evidence report.) 1911 */ 1912 public List<CodeableConcept> getEntryClassifier() { 1913 if (this.entryClassifier == null) 1914 this.entryClassifier = new ArrayList<CodeableConcept>(); 1915 return this.entryClassifier; 1916 } 1917 1918 /** 1919 * @return Returns a reference to <code>this</code> for easy method chaining 1920 */ 1921 public SectionComponent setEntryClassifier(List<CodeableConcept> theEntryClassifier) { 1922 this.entryClassifier = theEntryClassifier; 1923 return this; 1924 } 1925 1926 public boolean hasEntryClassifier() { 1927 if (this.entryClassifier == null) 1928 return false; 1929 for (CodeableConcept item : this.entryClassifier) 1930 if (!item.isEmpty()) 1931 return true; 1932 return false; 1933 } 1934 1935 public CodeableConcept addEntryClassifier() { //3 1936 CodeableConcept t = new CodeableConcept(); 1937 if (this.entryClassifier == null) 1938 this.entryClassifier = new ArrayList<CodeableConcept>(); 1939 this.entryClassifier.add(t); 1940 return t; 1941 } 1942 1943 public SectionComponent addEntryClassifier(CodeableConcept t) { //3 1944 if (t == null) 1945 return this; 1946 if (this.entryClassifier == null) 1947 this.entryClassifier = new ArrayList<CodeableConcept>(); 1948 this.entryClassifier.add(t); 1949 return this; 1950 } 1951 1952 /** 1953 * @return The first repetition of repeating field {@link #entryClassifier}, creating it if it does not already exist {3} 1954 */ 1955 public CodeableConcept getEntryClassifierFirstRep() { 1956 if (getEntryClassifier().isEmpty()) { 1957 addEntryClassifier(); 1958 } 1959 return getEntryClassifier().get(0); 1960 } 1961 1962 /** 1963 * @return {@link #entryReference} (A reference to the actual resource from which the narrative in the section is derived.) 1964 */ 1965 public List<Reference> getEntryReference() { 1966 if (this.entryReference == null) 1967 this.entryReference = new ArrayList<Reference>(); 1968 return this.entryReference; 1969 } 1970 1971 /** 1972 * @return Returns a reference to <code>this</code> for easy method chaining 1973 */ 1974 public SectionComponent setEntryReference(List<Reference> theEntryReference) { 1975 this.entryReference = theEntryReference; 1976 return this; 1977 } 1978 1979 public boolean hasEntryReference() { 1980 if (this.entryReference == null) 1981 return false; 1982 for (Reference item : this.entryReference) 1983 if (!item.isEmpty()) 1984 return true; 1985 return false; 1986 } 1987 1988 public Reference addEntryReference() { //3 1989 Reference t = new Reference(); 1990 if (this.entryReference == null) 1991 this.entryReference = new ArrayList<Reference>(); 1992 this.entryReference.add(t); 1993 return t; 1994 } 1995 1996 public SectionComponent addEntryReference(Reference t) { //3 1997 if (t == null) 1998 return this; 1999 if (this.entryReference == null) 2000 this.entryReference = new ArrayList<Reference>(); 2001 this.entryReference.add(t); 2002 return this; 2003 } 2004 2005 /** 2006 * @return The first repetition of repeating field {@link #entryReference}, creating it if it does not already exist {3} 2007 */ 2008 public Reference getEntryReferenceFirstRep() { 2009 if (getEntryReference().isEmpty()) { 2010 addEntryReference(); 2011 } 2012 return getEntryReference().get(0); 2013 } 2014 2015 /** 2016 * @return {@link #entryQuantity} (Quantity as content.) 2017 */ 2018 public List<Quantity> getEntryQuantity() { 2019 if (this.entryQuantity == null) 2020 this.entryQuantity = new ArrayList<Quantity>(); 2021 return this.entryQuantity; 2022 } 2023 2024 /** 2025 * @return Returns a reference to <code>this</code> for easy method chaining 2026 */ 2027 public SectionComponent setEntryQuantity(List<Quantity> theEntryQuantity) { 2028 this.entryQuantity = theEntryQuantity; 2029 return this; 2030 } 2031 2032 public boolean hasEntryQuantity() { 2033 if (this.entryQuantity == null) 2034 return false; 2035 for (Quantity item : this.entryQuantity) 2036 if (!item.isEmpty()) 2037 return true; 2038 return false; 2039 } 2040 2041 public Quantity addEntryQuantity() { //3 2042 Quantity t = new Quantity(); 2043 if (this.entryQuantity == null) 2044 this.entryQuantity = new ArrayList<Quantity>(); 2045 this.entryQuantity.add(t); 2046 return t; 2047 } 2048 2049 public SectionComponent addEntryQuantity(Quantity t) { //3 2050 if (t == null) 2051 return this; 2052 if (this.entryQuantity == null) 2053 this.entryQuantity = new ArrayList<Quantity>(); 2054 this.entryQuantity.add(t); 2055 return this; 2056 } 2057 2058 /** 2059 * @return The first repetition of repeating field {@link #entryQuantity}, creating it if it does not already exist {3} 2060 */ 2061 public Quantity getEntryQuantityFirstRep() { 2062 if (getEntryQuantity().isEmpty()) { 2063 addEntryQuantity(); 2064 } 2065 return getEntryQuantity().get(0); 2066 } 2067 2068 /** 2069 * @return {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 2070 */ 2071 public CodeableConcept getEmptyReason() { 2072 if (this.emptyReason == null) 2073 if (Configuration.errorOnAutoCreate()) 2074 throw new Error("Attempt to auto-create SectionComponent.emptyReason"); 2075 else if (Configuration.doAutoCreate()) 2076 this.emptyReason = new CodeableConcept(); // cc 2077 return this.emptyReason; 2078 } 2079 2080 public boolean hasEmptyReason() { 2081 return this.emptyReason != null && !this.emptyReason.isEmpty(); 2082 } 2083 2084 /** 2085 * @param value {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 2086 */ 2087 public SectionComponent setEmptyReason(CodeableConcept value) { 2088 this.emptyReason = value; 2089 return this; 2090 } 2091 2092 /** 2093 * @return {@link #section} (A nested sub-section within this section.) 2094 */ 2095 public List<SectionComponent> getSection() { 2096 if (this.section == null) 2097 this.section = new ArrayList<SectionComponent>(); 2098 return this.section; 2099 } 2100 2101 /** 2102 * @return Returns a reference to <code>this</code> for easy method chaining 2103 */ 2104 public SectionComponent setSection(List<SectionComponent> theSection) { 2105 this.section = theSection; 2106 return this; 2107 } 2108 2109 public boolean hasSection() { 2110 if (this.section == null) 2111 return false; 2112 for (SectionComponent item : this.section) 2113 if (!item.isEmpty()) 2114 return true; 2115 return false; 2116 } 2117 2118 public SectionComponent addSection() { //3 2119 SectionComponent t = new SectionComponent(); 2120 if (this.section == null) 2121 this.section = new ArrayList<SectionComponent>(); 2122 this.section.add(t); 2123 return t; 2124 } 2125 2126 public SectionComponent addSection(SectionComponent t) { //3 2127 if (t == null) 2128 return this; 2129 if (this.section == null) 2130 this.section = new ArrayList<SectionComponent>(); 2131 this.section.add(t); 2132 return this; 2133 } 2134 2135 /** 2136 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist {3} 2137 */ 2138 public SectionComponent getSectionFirstRep() { 2139 if (getSection().isEmpty()) { 2140 addSection(); 2141 } 2142 return getSection().get(0); 2143 } 2144 2145 protected void listChildren(List<Property> children) { 2146 super.listChildren(children); 2147 children.add(new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title)); 2148 children.add(new Property("focus", "CodeableConcept", "A code identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focus)); 2149 children.add(new Property("focusReference", "Reference(Any)", "A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focusReference)); 2150 children.add(new Property("author", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|Group|Organization)", "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author)); 2151 children.add(new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.", 0, 1, text)); 2152 children.add(new Property("mode", "code", "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode)); 2153 children.add(new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy)); 2154 children.add(new Property("entryClassifier", "CodeableConcept", "Specifies any type of classification of the evidence report.", 0, java.lang.Integer.MAX_VALUE, entryClassifier)); 2155 children.add(new Property("entryReference", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entryReference)); 2156 children.add(new Property("entryQuantity", "Quantity", "Quantity as content.", 0, java.lang.Integer.MAX_VALUE, entryQuantity)); 2157 children.add(new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason)); 2158 children.add(new Property("section", "@EvidenceReport.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section)); 2159 } 2160 2161 @Override 2162 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2163 switch (_hash) { 2164 case 110371416: /*title*/ return new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title); 2165 case 97604824: /*focus*/ return new Property("focus", "CodeableConcept", "A code identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focus); 2166 case 1823604051: /*focusReference*/ return new Property("focusReference", "Reference(Any)", "A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focusReference); 2167 case -1406328437: /*author*/ return new Property("author", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|Group|Organization)", "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author); 2168 case 3556653: /*text*/ return new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.", 0, 1, text); 2169 case 3357091: /*mode*/ return new Property("mode", "code", "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode); 2170 case -391079516: /*orderedBy*/ return new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy); 2171 case -948201421: /*entryClassifier*/ return new Property("entryClassifier", "CodeableConcept", "Specifies any type of classification of the evidence report.", 0, java.lang.Integer.MAX_VALUE, entryClassifier); 2172 case 438810361: /*entryReference*/ return new Property("entryReference", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entryReference); 2173 case 1945583389: /*entryQuantity*/ return new Property("entryQuantity", "Quantity", "Quantity as content.", 0, java.lang.Integer.MAX_VALUE, entryQuantity); 2174 case 1140135409: /*emptyReason*/ return new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason); 2175 case 1970241253: /*section*/ return new Property("section", "@EvidenceReport.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section); 2176 default: return super.getNamedProperty(_hash, _name, _checkValid); 2177 } 2178 2179 } 2180 2181 @Override 2182 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2183 switch (hash) { 2184 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2185 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // CodeableConcept 2186 case 1823604051: /*focusReference*/ return this.focusReference == null ? new Base[0] : new Base[] {this.focusReference}; // Reference 2187 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2188 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // Narrative 2189 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<ListMode> 2190 case -391079516: /*orderedBy*/ return this.orderedBy == null ? new Base[0] : new Base[] {this.orderedBy}; // CodeableConcept 2191 case -948201421: /*entryClassifier*/ return this.entryClassifier == null ? new Base[0] : this.entryClassifier.toArray(new Base[this.entryClassifier.size()]); // CodeableConcept 2192 case 438810361: /*entryReference*/ return this.entryReference == null ? new Base[0] : this.entryReference.toArray(new Base[this.entryReference.size()]); // Reference 2193 case 1945583389: /*entryQuantity*/ return this.entryQuantity == null ? new Base[0] : this.entryQuantity.toArray(new Base[this.entryQuantity.size()]); // Quantity 2194 case 1140135409: /*emptyReason*/ return this.emptyReason == null ? new Base[0] : new Base[] {this.emptyReason}; // CodeableConcept 2195 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 2196 default: return super.getProperty(hash, name, checkValid); 2197 } 2198 2199 } 2200 2201 @Override 2202 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2203 switch (hash) { 2204 case 110371416: // title 2205 this.title = TypeConvertor.castToString(value); // StringType 2206 return value; 2207 case 97604824: // focus 2208 this.focus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2209 return value; 2210 case 1823604051: // focusReference 2211 this.focusReference = TypeConvertor.castToReference(value); // Reference 2212 return value; 2213 case -1406328437: // author 2214 this.getAuthor().add(TypeConvertor.castToReference(value)); // Reference 2215 return value; 2216 case 3556653: // text 2217 this.text = TypeConvertor.castToNarrative(value); // Narrative 2218 return value; 2219 case 3357091: // mode 2220 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2221 this.mode = (Enumeration) value; // Enumeration<ListMode> 2222 return value; 2223 case -391079516: // orderedBy 2224 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2225 return value; 2226 case -948201421: // entryClassifier 2227 this.getEntryClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2228 return value; 2229 case 438810361: // entryReference 2230 this.getEntryReference().add(TypeConvertor.castToReference(value)); // Reference 2231 return value; 2232 case 1945583389: // entryQuantity 2233 this.getEntryQuantity().add(TypeConvertor.castToQuantity(value)); // Quantity 2234 return value; 2235 case 1140135409: // emptyReason 2236 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2237 return value; 2238 case 1970241253: // section 2239 this.getSection().add((SectionComponent) value); // SectionComponent 2240 return value; 2241 default: return super.setProperty(hash, name, value); 2242 } 2243 2244 } 2245 2246 @Override 2247 public Base setProperty(String name, Base value) throws FHIRException { 2248 if (name.equals("title")) { 2249 this.title = TypeConvertor.castToString(value); // StringType 2250 } else if (name.equals("focus")) { 2251 this.focus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2252 } else if (name.equals("focusReference")) { 2253 this.focusReference = TypeConvertor.castToReference(value); // Reference 2254 } else if (name.equals("author")) { 2255 this.getAuthor().add(TypeConvertor.castToReference(value)); 2256 } else if (name.equals("text")) { 2257 this.text = TypeConvertor.castToNarrative(value); // Narrative 2258 } else if (name.equals("mode")) { 2259 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2260 this.mode = (Enumeration) value; // Enumeration<ListMode> 2261 } else if (name.equals("orderedBy")) { 2262 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2263 } else if (name.equals("entryClassifier")) { 2264 this.getEntryClassifier().add(TypeConvertor.castToCodeableConcept(value)); 2265 } else if (name.equals("entryReference")) { 2266 this.getEntryReference().add(TypeConvertor.castToReference(value)); 2267 } else if (name.equals("entryQuantity")) { 2268 this.getEntryQuantity().add(TypeConvertor.castToQuantity(value)); 2269 } else if (name.equals("emptyReason")) { 2270 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2271 } else if (name.equals("section")) { 2272 this.getSection().add((SectionComponent) value); 2273 } else 2274 return super.setProperty(name, value); 2275 return value; 2276 } 2277 2278 @Override 2279 public void removeChild(String name, Base value) throws FHIRException { 2280 if (name.equals("title")) { 2281 this.title = null; 2282 } else if (name.equals("focus")) { 2283 this.focus = null; 2284 } else if (name.equals("focusReference")) { 2285 this.focusReference = null; 2286 } else if (name.equals("author")) { 2287 this.getAuthor().remove(value); 2288 } else if (name.equals("text")) { 2289 this.text = null; 2290 } else if (name.equals("mode")) { 2291 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2292 this.mode = (Enumeration) value; // Enumeration<ListMode> 2293 } else if (name.equals("orderedBy")) { 2294 this.orderedBy = null; 2295 } else if (name.equals("entryClassifier")) { 2296 this.getEntryClassifier().remove(value); 2297 } else if (name.equals("entryReference")) { 2298 this.getEntryReference().remove(value); 2299 } else if (name.equals("entryQuantity")) { 2300 this.getEntryQuantity().remove(value); 2301 } else if (name.equals("emptyReason")) { 2302 this.emptyReason = null; 2303 } else if (name.equals("section")) { 2304 this.getSection().remove((SectionComponent) value); 2305 } else 2306 super.removeChild(name, value); 2307 2308 } 2309 2310 @Override 2311 public Base makeProperty(int hash, String name) throws FHIRException { 2312 switch (hash) { 2313 case 110371416: return getTitleElement(); 2314 case 97604824: return getFocus(); 2315 case 1823604051: return getFocusReference(); 2316 case -1406328437: return addAuthor(); 2317 case 3556653: return getText(); 2318 case 3357091: return getModeElement(); 2319 case -391079516: return getOrderedBy(); 2320 case -948201421: return addEntryClassifier(); 2321 case 438810361: return addEntryReference(); 2322 case 1945583389: return addEntryQuantity(); 2323 case 1140135409: return getEmptyReason(); 2324 case 1970241253: return addSection(); 2325 default: return super.makeProperty(hash, name); 2326 } 2327 2328 } 2329 2330 @Override 2331 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2332 switch (hash) { 2333 case 110371416: /*title*/ return new String[] {"string"}; 2334 case 97604824: /*focus*/ return new String[] {"CodeableConcept"}; 2335 case 1823604051: /*focusReference*/ return new String[] {"Reference"}; 2336 case -1406328437: /*author*/ return new String[] {"Reference"}; 2337 case 3556653: /*text*/ return new String[] {"Narrative"}; 2338 case 3357091: /*mode*/ return new String[] {"code"}; 2339 case -391079516: /*orderedBy*/ return new String[] {"CodeableConcept"}; 2340 case -948201421: /*entryClassifier*/ return new String[] {"CodeableConcept"}; 2341 case 438810361: /*entryReference*/ return new String[] {"Reference"}; 2342 case 1945583389: /*entryQuantity*/ return new String[] {"Quantity"}; 2343 case 1140135409: /*emptyReason*/ return new String[] {"CodeableConcept"}; 2344 case 1970241253: /*section*/ return new String[] {"@EvidenceReport.section"}; 2345 default: return super.getTypesForProperty(hash, name); 2346 } 2347 2348 } 2349 2350 @Override 2351 public Base addChild(String name) throws FHIRException { 2352 if (name.equals("title")) { 2353 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.section.title"); 2354 } 2355 else if (name.equals("focus")) { 2356 this.focus = new CodeableConcept(); 2357 return this.focus; 2358 } 2359 else if (name.equals("focusReference")) { 2360 this.focusReference = new Reference(); 2361 return this.focusReference; 2362 } 2363 else if (name.equals("author")) { 2364 return addAuthor(); 2365 } 2366 else if (name.equals("text")) { 2367 this.text = new Narrative(); 2368 return this.text; 2369 } 2370 else if (name.equals("mode")) { 2371 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.section.mode"); 2372 } 2373 else if (name.equals("orderedBy")) { 2374 this.orderedBy = new CodeableConcept(); 2375 return this.orderedBy; 2376 } 2377 else if (name.equals("entryClassifier")) { 2378 return addEntryClassifier(); 2379 } 2380 else if (name.equals("entryReference")) { 2381 return addEntryReference(); 2382 } 2383 else if (name.equals("entryQuantity")) { 2384 return addEntryQuantity(); 2385 } 2386 else if (name.equals("emptyReason")) { 2387 this.emptyReason = new CodeableConcept(); 2388 return this.emptyReason; 2389 } 2390 else if (name.equals("section")) { 2391 return addSection(); 2392 } 2393 else 2394 return super.addChild(name); 2395 } 2396 2397 public SectionComponent copy() { 2398 SectionComponent dst = new SectionComponent(); 2399 copyValues(dst); 2400 return dst; 2401 } 2402 2403 public void copyValues(SectionComponent dst) { 2404 super.copyValues(dst); 2405 dst.title = title == null ? null : title.copy(); 2406 dst.focus = focus == null ? null : focus.copy(); 2407 dst.focusReference = focusReference == null ? null : focusReference.copy(); 2408 if (author != null) { 2409 dst.author = new ArrayList<Reference>(); 2410 for (Reference i : author) 2411 dst.author.add(i.copy()); 2412 }; 2413 dst.text = text == null ? null : text.copy(); 2414 dst.mode = mode == null ? null : mode.copy(); 2415 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 2416 if (entryClassifier != null) { 2417 dst.entryClassifier = new ArrayList<CodeableConcept>(); 2418 for (CodeableConcept i : entryClassifier) 2419 dst.entryClassifier.add(i.copy()); 2420 }; 2421 if (entryReference != null) { 2422 dst.entryReference = new ArrayList<Reference>(); 2423 for (Reference i : entryReference) 2424 dst.entryReference.add(i.copy()); 2425 }; 2426 if (entryQuantity != null) { 2427 dst.entryQuantity = new ArrayList<Quantity>(); 2428 for (Quantity i : entryQuantity) 2429 dst.entryQuantity.add(i.copy()); 2430 }; 2431 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 2432 if (section != null) { 2433 dst.section = new ArrayList<SectionComponent>(); 2434 for (SectionComponent i : section) 2435 dst.section.add(i.copy()); 2436 }; 2437 } 2438 2439 @Override 2440 public boolean equalsDeep(Base other_) { 2441 if (!super.equalsDeep(other_)) 2442 return false; 2443 if (!(other_ instanceof SectionComponent)) 2444 return false; 2445 SectionComponent o = (SectionComponent) other_; 2446 return compareDeep(title, o.title, true) && compareDeep(focus, o.focus, true) && compareDeep(focusReference, o.focusReference, true) 2447 && compareDeep(author, o.author, true) && compareDeep(text, o.text, true) && compareDeep(mode, o.mode, true) 2448 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(entryClassifier, o.entryClassifier, true) 2449 && compareDeep(entryReference, o.entryReference, true) && compareDeep(entryQuantity, o.entryQuantity, true) 2450 && compareDeep(emptyReason, o.emptyReason, true) && compareDeep(section, o.section, true); 2451 } 2452 2453 @Override 2454 public boolean equalsShallow(Base other_) { 2455 if (!super.equalsShallow(other_)) 2456 return false; 2457 if (!(other_ instanceof SectionComponent)) 2458 return false; 2459 SectionComponent o = (SectionComponent) other_; 2460 return compareValues(title, o.title, true) && compareValues(mode, o.mode, true); 2461 } 2462 2463 public boolean isEmpty() { 2464 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, focus, focusReference 2465 , author, text, mode, orderedBy, entryClassifier, entryReference, entryQuantity 2466 , emptyReason, section); 2467 } 2468 2469 public String fhirType() { 2470 return "EvidenceReport.section"; 2471 2472 } 2473 2474 } 2475 2476 /** 2477 * An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 2478 */ 2479 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2480 @Description(shortDefinition="Canonical identifier for this EvidenceReport, represented as a globally unique URI", formalDefinition="An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers." ) 2481 protected UriType url; 2482 2483 /** 2484 * The status of this summary. Enables tracking the life-cycle of the content. 2485 */ 2486 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2487 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this summary. Enables tracking the life-cycle of the content." ) 2488 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2489 protected Enumeration<PublicationStatus> status; 2490 2491 /** 2492 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances. 2493 */ 2494 @Child(name = "useContext", type = {UsageContext.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2495 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances." ) 2496 protected List<UsageContext> useContext; 2497 2498 /** 2499 * A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance. 2500 */ 2501 @Child(name = "identifier", type = {Identifier.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2502 @Description(shortDefinition="Unique identifier for the evidence report", formalDefinition="A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2503 protected List<Identifier> identifier; 2504 2505 /** 2506 * A formal identifier that is used to identify things closely related to this EvidenceReport. 2507 */ 2508 @Child(name = "relatedIdentifier", type = {Identifier.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2509 @Description(shortDefinition="Identifiers for articles that may relate to more than one evidence report", formalDefinition="A formal identifier that is used to identify things closely related to this EvidenceReport." ) 2510 protected List<Identifier> relatedIdentifier; 2511 2512 /** 2513 * Citation Resource or display of suggested citation for this report. 2514 */ 2515 @Child(name = "citeAs", type = {Citation.class, MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2516 @Description(shortDefinition="Citation for this report", formalDefinition="Citation Resource or display of suggested citation for this report." ) 2517 protected DataType citeAs; 2518 2519 /** 2520 * Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression. 2521 */ 2522 @Child(name = "type", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 2523 @Description(shortDefinition="Kind of report", formalDefinition="Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression." ) 2524 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-report-type") 2525 protected CodeableConcept type; 2526 2527 /** 2528 * Used for footnotes and annotations. 2529 */ 2530 @Child(name = "note", type = {Annotation.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2531 @Description(shortDefinition="Used for footnotes and annotations", formalDefinition="Used for footnotes and annotations." ) 2532 protected List<Annotation> note; 2533 2534 /** 2535 * Link, description or reference to artifact associated with the report. 2536 */ 2537 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2538 @Description(shortDefinition="Link, description or reference to artifact associated with the report", formalDefinition="Link, description or reference to artifact associated with the report." ) 2539 protected List<RelatedArtifact> relatedArtifact; 2540 2541 /** 2542 * Specifies the subject or focus of the report. Answers "What is this report about?". 2543 */ 2544 @Child(name = "subject", type = {}, order=9, min=1, max=1, modifier=false, summary=true) 2545 @Description(shortDefinition="Focus of the report", formalDefinition="Specifies the subject or focus of the report. Answers \"What is this report about?\"." ) 2546 protected EvidenceReportSubjectComponent subject; 2547 2548 /** 2549 * The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report. 2550 */ 2551 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 2552 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report." ) 2553 protected StringType publisher; 2554 2555 /** 2556 * Contact details to assist a user in finding and communicating with the publisher. 2557 */ 2558 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2559 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2560 protected List<ContactDetail> contact; 2561 2562 /** 2563 * An individiual, organization, or device primarily involved in the creation and maintenance of the content. 2564 */ 2565 @Child(name = "author", type = {ContactDetail.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2566 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual, organization, or device primarily involved in the creation and maintenance of the content." ) 2567 protected List<ContactDetail> author; 2568 2569 /** 2570 * An individiual, organization, or device primarily responsible for internal coherence of the content. 2571 */ 2572 @Child(name = "editor", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2573 @Description(shortDefinition="Who edited the content", formalDefinition="An individiual, organization, or device primarily responsible for internal coherence of the content." ) 2574 protected List<ContactDetail> editor; 2575 2576 /** 2577 * An individiual, organization, or device primarily responsible for review of some aspect of the content. 2578 */ 2579 @Child(name = "reviewer", type = {ContactDetail.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2580 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individiual, organization, or device primarily responsible for review of some aspect of the content." ) 2581 protected List<ContactDetail> reviewer; 2582 2583 /** 2584 * An individiual, organization, or device responsible for officially endorsing the content for use in some setting. 2585 */ 2586 @Child(name = "endorser", type = {ContactDetail.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2587 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individiual, organization, or device responsible for officially endorsing the content for use in some setting." ) 2588 protected List<ContactDetail> endorser; 2589 2590 /** 2591 * Relationships that this composition has with other compositions or documents that already exist. 2592 */ 2593 @Child(name = "relatesTo", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2594 @Description(shortDefinition="Relationships to other compositions/documents", formalDefinition="Relationships that this composition has with other compositions or documents that already exist." ) 2595 protected List<EvidenceReportRelatesToComponent> relatesTo; 2596 2597 /** 2598 * The root of the sections that make up the composition. 2599 */ 2600 @Child(name = "section", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2601 @Description(shortDefinition="Composition is broken into sections", formalDefinition="The root of the sections that make up the composition." ) 2602 protected List<SectionComponent> section; 2603 2604 private static final long serialVersionUID = -1087028792L; 2605 2606 /** 2607 * Constructor 2608 */ 2609 public EvidenceReport() { 2610 super(); 2611 } 2612 2613 /** 2614 * Constructor 2615 */ 2616 public EvidenceReport(PublicationStatus status, EvidenceReportSubjectComponent subject) { 2617 super(); 2618 this.setStatus(status); 2619 this.setSubject(subject); 2620 } 2621 2622 /** 2623 * @return {@link #url} (An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2624 */ 2625 public UriType getUrlElement() { 2626 if (this.url == null) 2627 if (Configuration.errorOnAutoCreate()) 2628 throw new Error("Attempt to auto-create EvidenceReport.url"); 2629 else if (Configuration.doAutoCreate()) 2630 this.url = new UriType(); // bb 2631 return this.url; 2632 } 2633 2634 public boolean hasUrlElement() { 2635 return this.url != null && !this.url.isEmpty(); 2636 } 2637 2638 public boolean hasUrl() { 2639 return this.url != null && !this.url.isEmpty(); 2640 } 2641 2642 /** 2643 * @param value {@link #url} (An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2644 */ 2645 public EvidenceReport setUrlElement(UriType value) { 2646 this.url = value; 2647 return this; 2648 } 2649 2650 /** 2651 * @return An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 2652 */ 2653 public String getUrl() { 2654 return this.url == null ? null : this.url.getValue(); 2655 } 2656 2657 /** 2658 * @param value An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 2659 */ 2660 public EvidenceReport setUrl(String value) { 2661 if (Utilities.noString(value)) 2662 this.url = null; 2663 else { 2664 if (this.url == null) 2665 this.url = new UriType(); 2666 this.url.setValue(value); 2667 } 2668 return this; 2669 } 2670 2671 /** 2672 * @return {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2673 */ 2674 public Enumeration<PublicationStatus> getStatusElement() { 2675 if (this.status == null) 2676 if (Configuration.errorOnAutoCreate()) 2677 throw new Error("Attempt to auto-create EvidenceReport.status"); 2678 else if (Configuration.doAutoCreate()) 2679 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2680 return this.status; 2681 } 2682 2683 public boolean hasStatusElement() { 2684 return this.status != null && !this.status.isEmpty(); 2685 } 2686 2687 public boolean hasStatus() { 2688 return this.status != null && !this.status.isEmpty(); 2689 } 2690 2691 /** 2692 * @param value {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2693 */ 2694 public EvidenceReport setStatusElement(Enumeration<PublicationStatus> value) { 2695 this.status = value; 2696 return this; 2697 } 2698 2699 /** 2700 * @return The status of this summary. Enables tracking the life-cycle of the content. 2701 */ 2702 public PublicationStatus getStatus() { 2703 return this.status == null ? null : this.status.getValue(); 2704 } 2705 2706 /** 2707 * @param value The status of this summary. Enables tracking the life-cycle of the content. 2708 */ 2709 public EvidenceReport setStatus(PublicationStatus value) { 2710 if (this.status == null) 2711 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2712 this.status.setValue(value); 2713 return this; 2714 } 2715 2716 /** 2717 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances.) 2718 */ 2719 public List<UsageContext> getUseContext() { 2720 if (this.useContext == null) 2721 this.useContext = new ArrayList<UsageContext>(); 2722 return this.useContext; 2723 } 2724 2725 /** 2726 * @return Returns a reference to <code>this</code> for easy method chaining 2727 */ 2728 public EvidenceReport setUseContext(List<UsageContext> theUseContext) { 2729 this.useContext = theUseContext; 2730 return this; 2731 } 2732 2733 public boolean hasUseContext() { 2734 if (this.useContext == null) 2735 return false; 2736 for (UsageContext item : this.useContext) 2737 if (!item.isEmpty()) 2738 return true; 2739 return false; 2740 } 2741 2742 public UsageContext addUseContext() { //3 2743 UsageContext t = new UsageContext(); 2744 if (this.useContext == null) 2745 this.useContext = new ArrayList<UsageContext>(); 2746 this.useContext.add(t); 2747 return t; 2748 } 2749 2750 public EvidenceReport addUseContext(UsageContext t) { //3 2751 if (t == null) 2752 return this; 2753 if (this.useContext == null) 2754 this.useContext = new ArrayList<UsageContext>(); 2755 this.useContext.add(t); 2756 return this; 2757 } 2758 2759 /** 2760 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2761 */ 2762 public UsageContext getUseContextFirstRep() { 2763 if (getUseContext().isEmpty()) { 2764 addUseContext(); 2765 } 2766 return getUseContext().get(0); 2767 } 2768 2769 /** 2770 * @return {@link #identifier} (A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2771 */ 2772 public List<Identifier> getIdentifier() { 2773 if (this.identifier == null) 2774 this.identifier = new ArrayList<Identifier>(); 2775 return this.identifier; 2776 } 2777 2778 /** 2779 * @return Returns a reference to <code>this</code> for easy method chaining 2780 */ 2781 public EvidenceReport setIdentifier(List<Identifier> theIdentifier) { 2782 this.identifier = theIdentifier; 2783 return this; 2784 } 2785 2786 public boolean hasIdentifier() { 2787 if (this.identifier == null) 2788 return false; 2789 for (Identifier item : this.identifier) 2790 if (!item.isEmpty()) 2791 return true; 2792 return false; 2793 } 2794 2795 public Identifier addIdentifier() { //3 2796 Identifier t = new Identifier(); 2797 if (this.identifier == null) 2798 this.identifier = new ArrayList<Identifier>(); 2799 this.identifier.add(t); 2800 return t; 2801 } 2802 2803 public EvidenceReport addIdentifier(Identifier t) { //3 2804 if (t == null) 2805 return this; 2806 if (this.identifier == null) 2807 this.identifier = new ArrayList<Identifier>(); 2808 this.identifier.add(t); 2809 return this; 2810 } 2811 2812 /** 2813 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2814 */ 2815 public Identifier getIdentifierFirstRep() { 2816 if (getIdentifier().isEmpty()) { 2817 addIdentifier(); 2818 } 2819 return getIdentifier().get(0); 2820 } 2821 2822 /** 2823 * @return {@link #relatedIdentifier} (A formal identifier that is used to identify things closely related to this EvidenceReport.) 2824 */ 2825 public List<Identifier> getRelatedIdentifier() { 2826 if (this.relatedIdentifier == null) 2827 this.relatedIdentifier = new ArrayList<Identifier>(); 2828 return this.relatedIdentifier; 2829 } 2830 2831 /** 2832 * @return Returns a reference to <code>this</code> for easy method chaining 2833 */ 2834 public EvidenceReport setRelatedIdentifier(List<Identifier> theRelatedIdentifier) { 2835 this.relatedIdentifier = theRelatedIdentifier; 2836 return this; 2837 } 2838 2839 public boolean hasRelatedIdentifier() { 2840 if (this.relatedIdentifier == null) 2841 return false; 2842 for (Identifier item : this.relatedIdentifier) 2843 if (!item.isEmpty()) 2844 return true; 2845 return false; 2846 } 2847 2848 public Identifier addRelatedIdentifier() { //3 2849 Identifier t = new Identifier(); 2850 if (this.relatedIdentifier == null) 2851 this.relatedIdentifier = new ArrayList<Identifier>(); 2852 this.relatedIdentifier.add(t); 2853 return t; 2854 } 2855 2856 public EvidenceReport addRelatedIdentifier(Identifier t) { //3 2857 if (t == null) 2858 return this; 2859 if (this.relatedIdentifier == null) 2860 this.relatedIdentifier = new ArrayList<Identifier>(); 2861 this.relatedIdentifier.add(t); 2862 return this; 2863 } 2864 2865 /** 2866 * @return The first repetition of repeating field {@link #relatedIdentifier}, creating it if it does not already exist {3} 2867 */ 2868 public Identifier getRelatedIdentifierFirstRep() { 2869 if (getRelatedIdentifier().isEmpty()) { 2870 addRelatedIdentifier(); 2871 } 2872 return getRelatedIdentifier().get(0); 2873 } 2874 2875 /** 2876 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2877 */ 2878 public DataType getCiteAs() { 2879 return this.citeAs; 2880 } 2881 2882 /** 2883 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2884 */ 2885 public Reference getCiteAsReference() throws FHIRException { 2886 if (this.citeAs == null) 2887 this.citeAs = new Reference(); 2888 if (!(this.citeAs instanceof Reference)) 2889 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 2890 return (Reference) this.citeAs; 2891 } 2892 2893 public boolean hasCiteAsReference() { 2894 return this != null && this.citeAs instanceof Reference; 2895 } 2896 2897 /** 2898 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2899 */ 2900 public MarkdownType getCiteAsMarkdownType() throws FHIRException { 2901 if (this.citeAs == null) 2902 this.citeAs = new MarkdownType(); 2903 if (!(this.citeAs instanceof MarkdownType)) 2904 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 2905 return (MarkdownType) this.citeAs; 2906 } 2907 2908 public boolean hasCiteAsMarkdownType() { 2909 return this != null && this.citeAs instanceof MarkdownType; 2910 } 2911 2912 public boolean hasCiteAs() { 2913 return this.citeAs != null && !this.citeAs.isEmpty(); 2914 } 2915 2916 /** 2917 * @param value {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2918 */ 2919 public EvidenceReport setCiteAs(DataType value) { 2920 if (value != null && !(value instanceof Reference || value instanceof MarkdownType)) 2921 throw new FHIRException("Not the right type for EvidenceReport.citeAs[x]: "+value.fhirType()); 2922 this.citeAs = value; 2923 return this; 2924 } 2925 2926 /** 2927 * @return {@link #type} (Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.) 2928 */ 2929 public CodeableConcept getType() { 2930 if (this.type == null) 2931 if (Configuration.errorOnAutoCreate()) 2932 throw new Error("Attempt to auto-create EvidenceReport.type"); 2933 else if (Configuration.doAutoCreate()) 2934 this.type = new CodeableConcept(); // cc 2935 return this.type; 2936 } 2937 2938 public boolean hasType() { 2939 return this.type != null && !this.type.isEmpty(); 2940 } 2941 2942 /** 2943 * @param value {@link #type} (Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.) 2944 */ 2945 public EvidenceReport setType(CodeableConcept value) { 2946 this.type = value; 2947 return this; 2948 } 2949 2950 /** 2951 * @return {@link #note} (Used for footnotes and annotations.) 2952 */ 2953 public List<Annotation> getNote() { 2954 if (this.note == null) 2955 this.note = new ArrayList<Annotation>(); 2956 return this.note; 2957 } 2958 2959 /** 2960 * @return Returns a reference to <code>this</code> for easy method chaining 2961 */ 2962 public EvidenceReport setNote(List<Annotation> theNote) { 2963 this.note = theNote; 2964 return this; 2965 } 2966 2967 public boolean hasNote() { 2968 if (this.note == null) 2969 return false; 2970 for (Annotation item : this.note) 2971 if (!item.isEmpty()) 2972 return true; 2973 return false; 2974 } 2975 2976 public Annotation addNote() { //3 2977 Annotation t = new Annotation(); 2978 if (this.note == null) 2979 this.note = new ArrayList<Annotation>(); 2980 this.note.add(t); 2981 return t; 2982 } 2983 2984 public EvidenceReport addNote(Annotation t) { //3 2985 if (t == null) 2986 return this; 2987 if (this.note == null) 2988 this.note = new ArrayList<Annotation>(); 2989 this.note.add(t); 2990 return this; 2991 } 2992 2993 /** 2994 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2995 */ 2996 public Annotation getNoteFirstRep() { 2997 if (getNote().isEmpty()) { 2998 addNote(); 2999 } 3000 return getNote().get(0); 3001 } 3002 3003 /** 3004 * @return {@link #relatedArtifact} (Link, description or reference to artifact associated with the report.) 3005 */ 3006 public List<RelatedArtifact> getRelatedArtifact() { 3007 if (this.relatedArtifact == null) 3008 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3009 return this.relatedArtifact; 3010 } 3011 3012 /** 3013 * @return Returns a reference to <code>this</code> for easy method chaining 3014 */ 3015 public EvidenceReport setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3016 this.relatedArtifact = theRelatedArtifact; 3017 return this; 3018 } 3019 3020 public boolean hasRelatedArtifact() { 3021 if (this.relatedArtifact == null) 3022 return false; 3023 for (RelatedArtifact item : this.relatedArtifact) 3024 if (!item.isEmpty()) 3025 return true; 3026 return false; 3027 } 3028 3029 public RelatedArtifact addRelatedArtifact() { //3 3030 RelatedArtifact t = new RelatedArtifact(); 3031 if (this.relatedArtifact == null) 3032 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3033 this.relatedArtifact.add(t); 3034 return t; 3035 } 3036 3037 public EvidenceReport addRelatedArtifact(RelatedArtifact t) { //3 3038 if (t == null) 3039 return this; 3040 if (this.relatedArtifact == null) 3041 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3042 this.relatedArtifact.add(t); 3043 return this; 3044 } 3045 3046 /** 3047 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 3048 */ 3049 public RelatedArtifact getRelatedArtifactFirstRep() { 3050 if (getRelatedArtifact().isEmpty()) { 3051 addRelatedArtifact(); 3052 } 3053 return getRelatedArtifact().get(0); 3054 } 3055 3056 /** 3057 * @return {@link #subject} (Specifies the subject or focus of the report. Answers "What is this report about?".) 3058 */ 3059 public EvidenceReportSubjectComponent getSubject() { 3060 if (this.subject == null) 3061 if (Configuration.errorOnAutoCreate()) 3062 throw new Error("Attempt to auto-create EvidenceReport.subject"); 3063 else if (Configuration.doAutoCreate()) 3064 this.subject = new EvidenceReportSubjectComponent(); // cc 3065 return this.subject; 3066 } 3067 3068 public boolean hasSubject() { 3069 return this.subject != null && !this.subject.isEmpty(); 3070 } 3071 3072 /** 3073 * @param value {@link #subject} (Specifies the subject or focus of the report. Answers "What is this report about?".) 3074 */ 3075 public EvidenceReport setSubject(EvidenceReportSubjectComponent value) { 3076 this.subject = value; 3077 return this; 3078 } 3079 3080 /** 3081 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3082 */ 3083 public StringType getPublisherElement() { 3084 if (this.publisher == null) 3085 if (Configuration.errorOnAutoCreate()) 3086 throw new Error("Attempt to auto-create EvidenceReport.publisher"); 3087 else if (Configuration.doAutoCreate()) 3088 this.publisher = new StringType(); // bb 3089 return this.publisher; 3090 } 3091 3092 public boolean hasPublisherElement() { 3093 return this.publisher != null && !this.publisher.isEmpty(); 3094 } 3095 3096 public boolean hasPublisher() { 3097 return this.publisher != null && !this.publisher.isEmpty(); 3098 } 3099 3100 /** 3101 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3102 */ 3103 public EvidenceReport setPublisherElement(StringType value) { 3104 this.publisher = value; 3105 return this; 3106 } 3107 3108 /** 3109 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report. 3110 */ 3111 public String getPublisher() { 3112 return this.publisher == null ? null : this.publisher.getValue(); 3113 } 3114 3115 /** 3116 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report. 3117 */ 3118 public EvidenceReport setPublisher(String value) { 3119 if (Utilities.noString(value)) 3120 this.publisher = null; 3121 else { 3122 if (this.publisher == null) 3123 this.publisher = new StringType(); 3124 this.publisher.setValue(value); 3125 } 3126 return this; 3127 } 3128 3129 /** 3130 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3131 */ 3132 public List<ContactDetail> getContact() { 3133 if (this.contact == null) 3134 this.contact = new ArrayList<ContactDetail>(); 3135 return this.contact; 3136 } 3137 3138 /** 3139 * @return Returns a reference to <code>this</code> for easy method chaining 3140 */ 3141 public EvidenceReport setContact(List<ContactDetail> theContact) { 3142 this.contact = theContact; 3143 return this; 3144 } 3145 3146 public boolean hasContact() { 3147 if (this.contact == null) 3148 return false; 3149 for (ContactDetail item : this.contact) 3150 if (!item.isEmpty()) 3151 return true; 3152 return false; 3153 } 3154 3155 public ContactDetail addContact() { //3 3156 ContactDetail t = new ContactDetail(); 3157 if (this.contact == null) 3158 this.contact = new ArrayList<ContactDetail>(); 3159 this.contact.add(t); 3160 return t; 3161 } 3162 3163 public EvidenceReport addContact(ContactDetail t) { //3 3164 if (t == null) 3165 return this; 3166 if (this.contact == null) 3167 this.contact = new ArrayList<ContactDetail>(); 3168 this.contact.add(t); 3169 return this; 3170 } 3171 3172 /** 3173 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3174 */ 3175 public ContactDetail getContactFirstRep() { 3176 if (getContact().isEmpty()) { 3177 addContact(); 3178 } 3179 return getContact().get(0); 3180 } 3181 3182 /** 3183 * @return {@link #author} (An individiual, organization, or device primarily involved in the creation and maintenance of the content.) 3184 */ 3185 public List<ContactDetail> getAuthor() { 3186 if (this.author == null) 3187 this.author = new ArrayList<ContactDetail>(); 3188 return this.author; 3189 } 3190 3191 /** 3192 * @return Returns a reference to <code>this</code> for easy method chaining 3193 */ 3194 public EvidenceReport setAuthor(List<ContactDetail> theAuthor) { 3195 this.author = theAuthor; 3196 return this; 3197 } 3198 3199 public boolean hasAuthor() { 3200 if (this.author == null) 3201 return false; 3202 for (ContactDetail item : this.author) 3203 if (!item.isEmpty()) 3204 return true; 3205 return false; 3206 } 3207 3208 public ContactDetail addAuthor() { //3 3209 ContactDetail t = new ContactDetail(); 3210 if (this.author == null) 3211 this.author = new ArrayList<ContactDetail>(); 3212 this.author.add(t); 3213 return t; 3214 } 3215 3216 public EvidenceReport addAuthor(ContactDetail t) { //3 3217 if (t == null) 3218 return this; 3219 if (this.author == null) 3220 this.author = new ArrayList<ContactDetail>(); 3221 this.author.add(t); 3222 return this; 3223 } 3224 3225 /** 3226 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 3227 */ 3228 public ContactDetail getAuthorFirstRep() { 3229 if (getAuthor().isEmpty()) { 3230 addAuthor(); 3231 } 3232 return getAuthor().get(0); 3233 } 3234 3235 /** 3236 * @return {@link #editor} (An individiual, organization, or device primarily responsible for internal coherence of the content.) 3237 */ 3238 public List<ContactDetail> getEditor() { 3239 if (this.editor == null) 3240 this.editor = new ArrayList<ContactDetail>(); 3241 return this.editor; 3242 } 3243 3244 /** 3245 * @return Returns a reference to <code>this</code> for easy method chaining 3246 */ 3247 public EvidenceReport setEditor(List<ContactDetail> theEditor) { 3248 this.editor = theEditor; 3249 return this; 3250 } 3251 3252 public boolean hasEditor() { 3253 if (this.editor == null) 3254 return false; 3255 for (ContactDetail item : this.editor) 3256 if (!item.isEmpty()) 3257 return true; 3258 return false; 3259 } 3260 3261 public ContactDetail addEditor() { //3 3262 ContactDetail t = new ContactDetail(); 3263 if (this.editor == null) 3264 this.editor = new ArrayList<ContactDetail>(); 3265 this.editor.add(t); 3266 return t; 3267 } 3268 3269 public EvidenceReport addEditor(ContactDetail t) { //3 3270 if (t == null) 3271 return this; 3272 if (this.editor == null) 3273 this.editor = new ArrayList<ContactDetail>(); 3274 this.editor.add(t); 3275 return this; 3276 } 3277 3278 /** 3279 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 3280 */ 3281 public ContactDetail getEditorFirstRep() { 3282 if (getEditor().isEmpty()) { 3283 addEditor(); 3284 } 3285 return getEditor().get(0); 3286 } 3287 3288 /** 3289 * @return {@link #reviewer} (An individiual, organization, or device primarily responsible for review of some aspect of the content.) 3290 */ 3291 public List<ContactDetail> getReviewer() { 3292 if (this.reviewer == null) 3293 this.reviewer = new ArrayList<ContactDetail>(); 3294 return this.reviewer; 3295 } 3296 3297 /** 3298 * @return Returns a reference to <code>this</code> for easy method chaining 3299 */ 3300 public EvidenceReport setReviewer(List<ContactDetail> theReviewer) { 3301 this.reviewer = theReviewer; 3302 return this; 3303 } 3304 3305 public boolean hasReviewer() { 3306 if (this.reviewer == null) 3307 return false; 3308 for (ContactDetail item : this.reviewer) 3309 if (!item.isEmpty()) 3310 return true; 3311 return false; 3312 } 3313 3314 public ContactDetail addReviewer() { //3 3315 ContactDetail t = new ContactDetail(); 3316 if (this.reviewer == null) 3317 this.reviewer = new ArrayList<ContactDetail>(); 3318 this.reviewer.add(t); 3319 return t; 3320 } 3321 3322 public EvidenceReport addReviewer(ContactDetail t) { //3 3323 if (t == null) 3324 return this; 3325 if (this.reviewer == null) 3326 this.reviewer = new ArrayList<ContactDetail>(); 3327 this.reviewer.add(t); 3328 return this; 3329 } 3330 3331 /** 3332 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 3333 */ 3334 public ContactDetail getReviewerFirstRep() { 3335 if (getReviewer().isEmpty()) { 3336 addReviewer(); 3337 } 3338 return getReviewer().get(0); 3339 } 3340 3341 /** 3342 * @return {@link #endorser} (An individiual, organization, or device responsible for officially endorsing the content for use in some setting.) 3343 */ 3344 public List<ContactDetail> getEndorser() { 3345 if (this.endorser == null) 3346 this.endorser = new ArrayList<ContactDetail>(); 3347 return this.endorser; 3348 } 3349 3350 /** 3351 * @return Returns a reference to <code>this</code> for easy method chaining 3352 */ 3353 public EvidenceReport setEndorser(List<ContactDetail> theEndorser) { 3354 this.endorser = theEndorser; 3355 return this; 3356 } 3357 3358 public boolean hasEndorser() { 3359 if (this.endorser == null) 3360 return false; 3361 for (ContactDetail item : this.endorser) 3362 if (!item.isEmpty()) 3363 return true; 3364 return false; 3365 } 3366 3367 public ContactDetail addEndorser() { //3 3368 ContactDetail t = new ContactDetail(); 3369 if (this.endorser == null) 3370 this.endorser = new ArrayList<ContactDetail>(); 3371 this.endorser.add(t); 3372 return t; 3373 } 3374 3375 public EvidenceReport addEndorser(ContactDetail t) { //3 3376 if (t == null) 3377 return this; 3378 if (this.endorser == null) 3379 this.endorser = new ArrayList<ContactDetail>(); 3380 this.endorser.add(t); 3381 return this; 3382 } 3383 3384 /** 3385 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 3386 */ 3387 public ContactDetail getEndorserFirstRep() { 3388 if (getEndorser().isEmpty()) { 3389 addEndorser(); 3390 } 3391 return getEndorser().get(0); 3392 } 3393 3394 /** 3395 * @return {@link #relatesTo} (Relationships that this composition has with other compositions or documents that already exist.) 3396 */ 3397 public List<EvidenceReportRelatesToComponent> getRelatesTo() { 3398 if (this.relatesTo == null) 3399 this.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 3400 return this.relatesTo; 3401 } 3402 3403 /** 3404 * @return Returns a reference to <code>this</code> for easy method chaining 3405 */ 3406 public EvidenceReport setRelatesTo(List<EvidenceReportRelatesToComponent> theRelatesTo) { 3407 this.relatesTo = theRelatesTo; 3408 return this; 3409 } 3410 3411 public boolean hasRelatesTo() { 3412 if (this.relatesTo == null) 3413 return false; 3414 for (EvidenceReportRelatesToComponent item : this.relatesTo) 3415 if (!item.isEmpty()) 3416 return true; 3417 return false; 3418 } 3419 3420 public EvidenceReportRelatesToComponent addRelatesTo() { //3 3421 EvidenceReportRelatesToComponent t = new EvidenceReportRelatesToComponent(); 3422 if (this.relatesTo == null) 3423 this.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 3424 this.relatesTo.add(t); 3425 return t; 3426 } 3427 3428 public EvidenceReport addRelatesTo(EvidenceReportRelatesToComponent t) { //3 3429 if (t == null) 3430 return this; 3431 if (this.relatesTo == null) 3432 this.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 3433 this.relatesTo.add(t); 3434 return this; 3435 } 3436 3437 /** 3438 * @return The first repetition of repeating field {@link #relatesTo}, creating it if it does not already exist {3} 3439 */ 3440 public EvidenceReportRelatesToComponent getRelatesToFirstRep() { 3441 if (getRelatesTo().isEmpty()) { 3442 addRelatesTo(); 3443 } 3444 return getRelatesTo().get(0); 3445 } 3446 3447 /** 3448 * @return {@link #section} (The root of the sections that make up the composition.) 3449 */ 3450 public List<SectionComponent> getSection() { 3451 if (this.section == null) 3452 this.section = new ArrayList<SectionComponent>(); 3453 return this.section; 3454 } 3455 3456 /** 3457 * @return Returns a reference to <code>this</code> for easy method chaining 3458 */ 3459 public EvidenceReport setSection(List<SectionComponent> theSection) { 3460 this.section = theSection; 3461 return this; 3462 } 3463 3464 public boolean hasSection() { 3465 if (this.section == null) 3466 return false; 3467 for (SectionComponent item : this.section) 3468 if (!item.isEmpty()) 3469 return true; 3470 return false; 3471 } 3472 3473 public SectionComponent addSection() { //3 3474 SectionComponent t = new SectionComponent(); 3475 if (this.section == null) 3476 this.section = new ArrayList<SectionComponent>(); 3477 this.section.add(t); 3478 return t; 3479 } 3480 3481 public EvidenceReport addSection(SectionComponent t) { //3 3482 if (t == null) 3483 return this; 3484 if (this.section == null) 3485 this.section = new ArrayList<SectionComponent>(); 3486 this.section.add(t); 3487 return this; 3488 } 3489 3490 /** 3491 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist {3} 3492 */ 3493 public SectionComponent getSectionFirstRep() { 3494 if (getSection().isEmpty()) { 3495 addSection(); 3496 } 3497 return getSection().get(0); 3498 } 3499 3500 /** 3501 * not supported on this implementation 3502 */ 3503 @Override 3504 public int getVersionMax() { 3505 return 0; 3506 } 3507 /** 3508 * @return {@link #version} (The identifier that is used to identify this version of the evidence report when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence report author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.)). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3509 */ 3510 public StringType getVersionElement() { 3511 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3512 } 3513 3514 public boolean hasVersionElement() { 3515 return false; 3516 } 3517 public boolean hasVersion() { 3518 return false; 3519 } 3520 3521 /** 3522 * @param value {@link #version} (The identifier that is used to identify this version of the evidence report when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence report author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.)). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3523 */ 3524 public EvidenceReport setVersionElement(StringType value) { 3525 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3526 } 3527 public String getVersion() { 3528 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3529 } 3530 /** 3531 * @param value The identifier that is used to identify this version of the evidence report when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence report author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.) 3532 */ 3533 public EvidenceReport setVersion(String value) { 3534 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3535 } 3536 /** 3537 * not supported on this implementation 3538 */ 3539 @Override 3540 public int getVersionAlgorithmMax() { 3541 return 0; 3542 } 3543 /** 3544 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3545 */ 3546 public DataType getVersionAlgorithm() { 3547 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3548 } 3549 /** 3550 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3551 */ 3552 public StringType getVersionAlgorithmStringType() { 3553 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3554 } 3555 public boolean hasVersionAlgorithmStringType() { 3556 return false;////K 3557 } 3558 /** 3559 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3560 */ 3561 public Coding getVersionAlgorithmCoding() { 3562 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3563 } 3564 public boolean hasVersionAlgorithmCoding() { 3565 return false;////K 3566 } 3567 public boolean hasVersionAlgorithm() { 3568 return false; 3569 } 3570 /** 3571 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3572 */ 3573 public EvidenceReport setVersionAlgorithm(DataType value) { 3574 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3575 } 3576 3577 /** 3578 * not supported on this implementation 3579 */ 3580 @Override 3581 public int getNameMax() { 3582 return 0; 3583 } 3584 /** 3585 * @return {@link #name} (A natural language name identifying the evidence report. This name should be usable as an identifier for the resource by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3586 */ 3587 public StringType getNameElement() { 3588 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3589 } 3590 3591 public boolean hasNameElement() { 3592 return false; 3593 } 3594 public boolean hasName() { 3595 return false; 3596 } 3597 3598 /** 3599 * @param value {@link #name} (A natural language name identifying the evidence report. This name should be usable as an identifier for the resource by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3600 */ 3601 public EvidenceReport setNameElement(StringType value) { 3602 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3603 } 3604 public String getName() { 3605 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3606 } 3607 /** 3608 * @param value A natural language name identifying the evidence report. This name should be usable as an identifier for the resource by machine processing applications such as code generation. 3609 */ 3610 public EvidenceReport setName(String value) { 3611 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3612 } 3613 /** 3614 * not supported on this implementation 3615 */ 3616 @Override 3617 public int getTitleMax() { 3618 return 0; 3619 } 3620 /** 3621 * @return {@link #title} (A short, descriptive, user-friendly title for the evidence report.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3622 */ 3623 public StringType getTitleElement() { 3624 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3625 } 3626 3627 public boolean hasTitleElement() { 3628 return false; 3629 } 3630 public boolean hasTitle() { 3631 return false; 3632 } 3633 3634 /** 3635 * @param value {@link #title} (A short, descriptive, user-friendly title for the evidence report.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3636 */ 3637 public EvidenceReport setTitleElement(StringType value) { 3638 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3639 } 3640 public String getTitle() { 3641 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3642 } 3643 /** 3644 * @param value A short, descriptive, user-friendly title for the evidence report. 3645 */ 3646 public EvidenceReport setTitle(String value) { 3647 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3648 } 3649 /** 3650 * not supported on this implementation 3651 */ 3652 @Override 3653 public int getExperimentalMax() { 3654 return 0; 3655 } 3656 /** 3657 * @return {@link #experimental} (A Boolean value to indicate that this evidence report is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3658 */ 3659 public BooleanType getExperimentalElement() { 3660 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3661 } 3662 3663 public boolean hasExperimentalElement() { 3664 return false; 3665 } 3666 public boolean hasExperimental() { 3667 return false; 3668 } 3669 3670 /** 3671 * @param value {@link #experimental} (A Boolean value to indicate that this evidence report is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3672 */ 3673 public EvidenceReport setExperimentalElement(BooleanType value) { 3674 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3675 } 3676 public boolean getExperimental() { 3677 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3678 } 3679 /** 3680 * @param value A Boolean value to indicate that this evidence report is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 3681 */ 3682 public EvidenceReport setExperimental(boolean value) { 3683 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3684 } 3685 /** 3686 * not supported on this implementation 3687 */ 3688 @Override 3689 public int getDateMax() { 3690 return 0; 3691 } 3692 /** 3693 * @return {@link #date} (The date (and optionally time) when the evidence report was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence report changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3694 */ 3695 public DateTimeType getDateElement() { 3696 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3697 } 3698 3699 public boolean hasDateElement() { 3700 return false; 3701 } 3702 public boolean hasDate() { 3703 return false; 3704 } 3705 3706 /** 3707 * @param value {@link #date} (The date (and optionally time) when the evidence report was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence report changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3708 */ 3709 public EvidenceReport setDateElement(DateTimeType value) { 3710 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3711 } 3712 public Date getDate() { 3713 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3714 } 3715 /** 3716 * @param value The date (and optionally time) when the evidence report was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence report changes. 3717 */ 3718 public EvidenceReport setDate(Date value) { 3719 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3720 } 3721 /** 3722 * not supported on this implementation 3723 */ 3724 @Override 3725 public int getDescriptionMax() { 3726 return 0; 3727 } 3728 /** 3729 * @return {@link #description} (A free text natural language description of the evidence report from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3730 */ 3731 public MarkdownType getDescriptionElement() { 3732 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3733 } 3734 3735 public boolean hasDescriptionElement() { 3736 return false; 3737 } 3738 public boolean hasDescription() { 3739 return false; 3740 } 3741 3742 /** 3743 * @param value {@link #description} (A free text natural language description of the evidence report from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3744 */ 3745 public EvidenceReport setDescriptionElement(MarkdownType value) { 3746 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3747 } 3748 public String getDescription() { 3749 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3750 } 3751 /** 3752 * @param value A free text natural language description of the evidence report from a consumer's perspective. 3753 */ 3754 public EvidenceReport setDescription(String value) { 3755 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3756 } 3757 /** 3758 * not supported on this implementation 3759 */ 3760 @Override 3761 public int getJurisdictionMax() { 3762 return 0; 3763 } 3764 /** 3765 * @return {@link #jurisdiction} (A legal or geographic region in which the evidence report is intended to be used.) 3766 */ 3767 public List<CodeableConcept> getJurisdiction() { 3768 return new ArrayList<>(); 3769 } 3770 /** 3771 * @return Returns a reference to <code>this</code> for easy method chaining 3772 */ 3773 public EvidenceReport setJurisdiction(List<CodeableConcept> theJurisdiction) { 3774 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3775 } 3776 public boolean hasJurisdiction() { 3777 return false; 3778 } 3779 3780 public CodeableConcept addJurisdiction() { //3 3781 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3782 } 3783 public EvidenceReport addJurisdiction(CodeableConcept t) { //3 3784 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3785 } 3786 /** 3787 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {2} 3788 */ 3789 public CodeableConcept getJurisdictionFirstRep() { 3790 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3791 } 3792 /** 3793 * not supported on this implementation 3794 */ 3795 @Override 3796 public int getPurposeMax() { 3797 return 0; 3798 } 3799 /** 3800 * @return {@link #purpose} (Explanation of why this evidence report is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3801 */ 3802 public MarkdownType getPurposeElement() { 3803 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3804 } 3805 3806 public boolean hasPurposeElement() { 3807 return false; 3808 } 3809 public boolean hasPurpose() { 3810 return false; 3811 } 3812 3813 /** 3814 * @param value {@link #purpose} (Explanation of why this evidence report is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3815 */ 3816 public EvidenceReport setPurposeElement(MarkdownType value) { 3817 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3818 } 3819 public String getPurpose() { 3820 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3821 } 3822 /** 3823 * @param value Explanation of why this evidence report is needed and why it has been designed as it has. 3824 */ 3825 public EvidenceReport setPurpose(String value) { 3826 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3827 } 3828 /** 3829 * not supported on this implementation 3830 */ 3831 @Override 3832 public int getCopyrightMax() { 3833 return 0; 3834 } 3835 /** 3836 * @return {@link #copyright} (A copyright statement relating to the evidence report and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3837 */ 3838 public MarkdownType getCopyrightElement() { 3839 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3840 } 3841 3842 public boolean hasCopyrightElement() { 3843 return false; 3844 } 3845 public boolean hasCopyright() { 3846 return false; 3847 } 3848 3849 /** 3850 * @param value {@link #copyright} (A copyright statement relating to the evidence report and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3851 */ 3852 public EvidenceReport setCopyrightElement(MarkdownType value) { 3853 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3854 } 3855 public String getCopyright() { 3856 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3857 } 3858 /** 3859 * @param value A copyright statement relating to the evidence report and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence report. 3860 */ 3861 public EvidenceReport setCopyright(String value) { 3862 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3863 } 3864 /** 3865 * not supported on this implementation 3866 */ 3867 @Override 3868 public int getCopyrightLabelMax() { 3869 return 0; 3870 } 3871 /** 3872 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3873 */ 3874 public StringType getCopyrightLabelElement() { 3875 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3876 } 3877 3878 public boolean hasCopyrightLabelElement() { 3879 return false; 3880 } 3881 public boolean hasCopyrightLabel() { 3882 return false; 3883 } 3884 3885 /** 3886 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3887 */ 3888 public EvidenceReport setCopyrightLabelElement(StringType value) { 3889 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3890 } 3891 public String getCopyrightLabel() { 3892 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3893 } 3894 /** 3895 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3896 */ 3897 public EvidenceReport setCopyrightLabel(String value) { 3898 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3899 } 3900 /** 3901 * not supported on this implementation 3902 */ 3903 @Override 3904 public int getApprovalDateMax() { 3905 return 0; 3906 } 3907 /** 3908 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3909 */ 3910 public DateType getApprovalDateElement() { 3911 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3912 } 3913 3914 public boolean hasApprovalDateElement() { 3915 return false; 3916 } 3917 public boolean hasApprovalDate() { 3918 return false; 3919 } 3920 3921 /** 3922 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3923 */ 3924 public EvidenceReport setApprovalDateElement(DateType value) { 3925 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3926 } 3927 public Date getApprovalDate() { 3928 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3929 } 3930 /** 3931 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3932 */ 3933 public EvidenceReport setApprovalDate(Date value) { 3934 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3935 } 3936 /** 3937 * not supported on this implementation 3938 */ 3939 @Override 3940 public int getLastReviewDateMax() { 3941 return 0; 3942 } 3943 /** 3944 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3945 */ 3946 public DateType getLastReviewDateElement() { 3947 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3948 } 3949 3950 public boolean hasLastReviewDateElement() { 3951 return false; 3952 } 3953 public boolean hasLastReviewDate() { 3954 return false; 3955 } 3956 3957 /** 3958 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3959 */ 3960 public EvidenceReport setLastReviewDateElement(DateType value) { 3961 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3962 } 3963 public Date getLastReviewDate() { 3964 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3965 } 3966 /** 3967 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3968 */ 3969 public EvidenceReport setLastReviewDate(Date value) { 3970 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3971 } 3972 /** 3973 * not supported on this implementation 3974 */ 3975 @Override 3976 public int getEffectivePeriodMax() { 3977 return 0; 3978 } 3979 /** 3980 * @return {@link #effectivePeriod} (The period during which the evidence report content was or is planned to be in active use.) 3981 */ 3982 public Period getEffectivePeriod() { 3983 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"effectivePeriod\""); 3984 } 3985 public boolean hasEffectivePeriod() { 3986 return false; 3987 } 3988 /** 3989 * @param value {@link #effectivePeriod} (The period during which the evidence report content was or is planned to be in active use.) 3990 */ 3991 public EvidenceReport setEffectivePeriod(Period value) { 3992 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"effectivePeriod\""); 3993 } 3994 3995 /** 3996 * not supported on this implementation 3997 */ 3998 @Override 3999 public int getTopicMax() { 4000 return 0; 4001 } 4002 /** 4003 * @return {@link #topic} (Descriptive topics related to the content of the evidence report. Topics provide a high-level categorization as well as keywords for the evidence report that can be useful for filtering and searching.) 4004 */ 4005 public List<CodeableConcept> getTopic() { 4006 return new ArrayList<>(); 4007 } 4008 /** 4009 * @return Returns a reference to <code>this</code> for easy method chaining 4010 */ 4011 public EvidenceReport setTopic(List<CodeableConcept> theTopic) { 4012 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4013 } 4014 public boolean hasTopic() { 4015 return false; 4016 } 4017 4018 public CodeableConcept addTopic() { //3 4019 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4020 } 4021 public EvidenceReport addTopic(CodeableConcept t) { //3 4022 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4023 } 4024 /** 4025 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 4026 */ 4027 public CodeableConcept getTopicFirstRep() { 4028 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4029 } 4030 protected void listChildren(List<Property> children) { 4031 super.listChildren(children); 4032 children.add(new Property("url", "uri", "An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url)); 4033 children.add(new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status)); 4034 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4035 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4036 children.add(new Property("relatedIdentifier", "Identifier", "A formal identifier that is used to identify things closely related to this EvidenceReport.", 0, java.lang.Integer.MAX_VALUE, relatedIdentifier)); 4037 children.add(new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs)); 4038 children.add(new Property("type", "CodeableConcept", "Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.", 0, 1, type)); 4039 children.add(new Property("note", "Annotation", "Used for footnotes and annotations.", 0, java.lang.Integer.MAX_VALUE, note)); 4040 children.add(new Property("relatedArtifact", "RelatedArtifact", "Link, description or reference to artifact associated with the report.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4041 children.add(new Property("subject", "", "Specifies the subject or focus of the report. Answers \"What is this report about?\".", 0, 1, subject)); 4042 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.", 0, 1, publisher)); 4043 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4044 children.add(new Property("author", "ContactDetail", "An individiual, organization, or device primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 4045 children.add(new Property("editor", "ContactDetail", "An individiual, organization, or device primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 4046 children.add(new Property("reviewer", "ContactDetail", "An individiual, organization, or device primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 4047 children.add(new Property("endorser", "ContactDetail", "An individiual, organization, or device responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 4048 children.add(new Property("relatesTo", "", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo)); 4049 children.add(new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section)); 4050 } 4051 4052 @Override 4053 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4054 switch (_hash) { 4055 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url); 4056 case -892481550: /*status*/ return new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status); 4057 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4058 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4059 case -1007604940: /*relatedIdentifier*/ return new Property("relatedIdentifier", "Identifier", "A formal identifier that is used to identify things closely related to this EvidenceReport.", 0, java.lang.Integer.MAX_VALUE, relatedIdentifier); 4060 case -1706539017: /*citeAs[x]*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4061 case -1360156695: /*citeAs*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4062 case 1269009762: /*citeAsReference*/ return new Property("citeAs[x]", "Reference(Citation)", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4063 case 456265720: /*citeAsMarkdown*/ return new Property("citeAs[x]", "markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4064 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.", 0, 1, type); 4065 case 3387378: /*note*/ return new Property("note", "Annotation", "Used for footnotes and annotations.", 0, java.lang.Integer.MAX_VALUE, note); 4066 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Link, description or reference to artifact associated with the report.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4067 case -1867885268: /*subject*/ return new Property("subject", "", "Specifies the subject or focus of the report. Answers \"What is this report about?\".", 0, 1, subject); 4068 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.", 0, 1, publisher); 4069 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4070 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual, organization, or device primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 4071 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individiual, organization, or device primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 4072 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individiual, organization, or device primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 4073 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individiual, organization, or device responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 4074 case -7765931: /*relatesTo*/ return new Property("relatesTo", "", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo); 4075 case 1970241253: /*section*/ return new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section); 4076 default: return super.getNamedProperty(_hash, _name, _checkValid); 4077 } 4078 4079 } 4080 4081 @Override 4082 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4083 switch (hash) { 4084 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4085 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4086 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4087 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4088 case -1007604940: /*relatedIdentifier*/ return this.relatedIdentifier == null ? new Base[0] : this.relatedIdentifier.toArray(new Base[this.relatedIdentifier.size()]); // Identifier 4089 case -1360156695: /*citeAs*/ return this.citeAs == null ? new Base[0] : new Base[] {this.citeAs}; // DataType 4090 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 4091 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4092 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4093 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // EvidenceReportSubjectComponent 4094 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4095 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4096 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4097 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4098 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4099 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4100 case -7765931: /*relatesTo*/ return this.relatesTo == null ? new Base[0] : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // EvidenceReportRelatesToComponent 4101 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 4102 default: return super.getProperty(hash, name, checkValid); 4103 } 4104 4105 } 4106 4107 @Override 4108 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4109 switch (hash) { 4110 case 116079: // url 4111 this.url = TypeConvertor.castToUri(value); // UriType 4112 return value; 4113 case -892481550: // status 4114 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4115 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4116 return value; 4117 case -669707736: // useContext 4118 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4119 return value; 4120 case -1618432855: // identifier 4121 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4122 return value; 4123 case -1007604940: // relatedIdentifier 4124 this.getRelatedIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4125 return value; 4126 case -1360156695: // citeAs 4127 this.citeAs = TypeConvertor.castToType(value); // DataType 4128 return value; 4129 case 3575610: // type 4130 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4131 return value; 4132 case 3387378: // note 4133 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4134 return value; 4135 case 666807069: // relatedArtifact 4136 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4137 return value; 4138 case -1867885268: // subject 4139 this.subject = (EvidenceReportSubjectComponent) value; // EvidenceReportSubjectComponent 4140 return value; 4141 case 1447404028: // publisher 4142 this.publisher = TypeConvertor.castToString(value); // StringType 4143 return value; 4144 case 951526432: // contact 4145 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4146 return value; 4147 case -1406328437: // author 4148 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4149 return value; 4150 case -1307827859: // editor 4151 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4152 return value; 4153 case -261190139: // reviewer 4154 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4155 return value; 4156 case 1740277666: // endorser 4157 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4158 return value; 4159 case -7765931: // relatesTo 4160 this.getRelatesTo().add((EvidenceReportRelatesToComponent) value); // EvidenceReportRelatesToComponent 4161 return value; 4162 case 1970241253: // section 4163 this.getSection().add((SectionComponent) value); // SectionComponent 4164 return value; 4165 default: return super.setProperty(hash, name, value); 4166 } 4167 4168 } 4169 4170 @Override 4171 public Base setProperty(String name, Base value) throws FHIRException { 4172 if (name.equals("url")) { 4173 this.url = TypeConvertor.castToUri(value); // UriType 4174 } else if (name.equals("status")) { 4175 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4176 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4177 } else if (name.equals("useContext")) { 4178 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4179 } else if (name.equals("identifier")) { 4180 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4181 } else if (name.equals("relatedIdentifier")) { 4182 this.getRelatedIdentifier().add(TypeConvertor.castToIdentifier(value)); 4183 } else if (name.equals("citeAs[x]")) { 4184 this.citeAs = TypeConvertor.castToType(value); // DataType 4185 } else if (name.equals("type")) { 4186 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4187 } else if (name.equals("note")) { 4188 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4189 } else if (name.equals("relatedArtifact")) { 4190 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4191 } else if (name.equals("subject")) { 4192 this.subject = (EvidenceReportSubjectComponent) value; // EvidenceReportSubjectComponent 4193 } else if (name.equals("publisher")) { 4194 this.publisher = TypeConvertor.castToString(value); // StringType 4195 } else if (name.equals("contact")) { 4196 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4197 } else if (name.equals("author")) { 4198 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 4199 } else if (name.equals("editor")) { 4200 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 4201 } else if (name.equals("reviewer")) { 4202 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 4203 } else if (name.equals("endorser")) { 4204 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 4205 } else if (name.equals("relatesTo")) { 4206 this.getRelatesTo().add((EvidenceReportRelatesToComponent) value); 4207 } else if (name.equals("section")) { 4208 this.getSection().add((SectionComponent) value); 4209 } else 4210 return super.setProperty(name, value); 4211 return value; 4212 } 4213 4214 @Override 4215 public void removeChild(String name, Base value) throws FHIRException { 4216 if (name.equals("url")) { 4217 this.url = null; 4218 } else if (name.equals("status")) { 4219 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4220 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4221 } else if (name.equals("useContext")) { 4222 this.getUseContext().remove(value); 4223 } else if (name.equals("identifier")) { 4224 this.getIdentifier().remove(value); 4225 } else if (name.equals("relatedIdentifier")) { 4226 this.getRelatedIdentifier().remove(value); 4227 } else if (name.equals("citeAs[x]")) { 4228 this.citeAs = null; 4229 } else if (name.equals("type")) { 4230 this.type = null; 4231 } else if (name.equals("note")) { 4232 this.getNote().remove(value); 4233 } else if (name.equals("relatedArtifact")) { 4234 this.getRelatedArtifact().remove(value); 4235 } else if (name.equals("subject")) { 4236 this.subject = (EvidenceReportSubjectComponent) value; // EvidenceReportSubjectComponent 4237 } else if (name.equals("publisher")) { 4238 this.publisher = null; 4239 } else if (name.equals("contact")) { 4240 this.getContact().remove(value); 4241 } else if (name.equals("author")) { 4242 this.getAuthor().remove(value); 4243 } else if (name.equals("editor")) { 4244 this.getEditor().remove(value); 4245 } else if (name.equals("reviewer")) { 4246 this.getReviewer().remove(value); 4247 } else if (name.equals("endorser")) { 4248 this.getEndorser().remove(value); 4249 } else if (name.equals("relatesTo")) { 4250 this.getRelatesTo().remove((EvidenceReportRelatesToComponent) value); 4251 } else if (name.equals("section")) { 4252 this.getSection().remove((SectionComponent) value); 4253 } else 4254 super.removeChild(name, value); 4255 4256 } 4257 4258 @Override 4259 public Base makeProperty(int hash, String name) throws FHIRException { 4260 switch (hash) { 4261 case 116079: return getUrlElement(); 4262 case -892481550: return getStatusElement(); 4263 case -669707736: return addUseContext(); 4264 case -1618432855: return addIdentifier(); 4265 case -1007604940: return addRelatedIdentifier(); 4266 case -1706539017: return getCiteAs(); 4267 case -1360156695: return getCiteAs(); 4268 case 3575610: return getType(); 4269 case 3387378: return addNote(); 4270 case 666807069: return addRelatedArtifact(); 4271 case -1867885268: return getSubject(); 4272 case 1447404028: return getPublisherElement(); 4273 case 951526432: return addContact(); 4274 case -1406328437: return addAuthor(); 4275 case -1307827859: return addEditor(); 4276 case -261190139: return addReviewer(); 4277 case 1740277666: return addEndorser(); 4278 case -7765931: return addRelatesTo(); 4279 case 1970241253: return addSection(); 4280 default: return super.makeProperty(hash, name); 4281 } 4282 4283 } 4284 4285 @Override 4286 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4287 switch (hash) { 4288 case 116079: /*url*/ return new String[] {"uri"}; 4289 case -892481550: /*status*/ return new String[] {"code"}; 4290 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4291 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4292 case -1007604940: /*relatedIdentifier*/ return new String[] {"Identifier"}; 4293 case -1360156695: /*citeAs*/ return new String[] {"Reference", "markdown"}; 4294 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4295 case 3387378: /*note*/ return new String[] {"Annotation"}; 4296 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 4297 case -1867885268: /*subject*/ return new String[] {}; 4298 case 1447404028: /*publisher*/ return new String[] {"string"}; 4299 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4300 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 4301 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 4302 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 4303 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 4304 case -7765931: /*relatesTo*/ return new String[] {}; 4305 case 1970241253: /*section*/ return new String[] {}; 4306 default: return super.getTypesForProperty(hash, name); 4307 } 4308 4309 } 4310 4311 @Override 4312 public Base addChild(String name) throws FHIRException { 4313 if (name.equals("url")) { 4314 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.url"); 4315 } 4316 else if (name.equals("status")) { 4317 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.status"); 4318 } 4319 else if (name.equals("useContext")) { 4320 return addUseContext(); 4321 } 4322 else if (name.equals("identifier")) { 4323 return addIdentifier(); 4324 } 4325 else if (name.equals("relatedIdentifier")) { 4326 return addRelatedIdentifier(); 4327 } 4328 else if (name.equals("citeAsReference")) { 4329 this.citeAs = new Reference(); 4330 return this.citeAs; 4331 } 4332 else if (name.equals("citeAsMarkdown")) { 4333 this.citeAs = new MarkdownType(); 4334 return this.citeAs; 4335 } 4336 else if (name.equals("type")) { 4337 this.type = new CodeableConcept(); 4338 return this.type; 4339 } 4340 else if (name.equals("note")) { 4341 return addNote(); 4342 } 4343 else if (name.equals("relatedArtifact")) { 4344 return addRelatedArtifact(); 4345 } 4346 else if (name.equals("subject")) { 4347 this.subject = new EvidenceReportSubjectComponent(); 4348 return this.subject; 4349 } 4350 else if (name.equals("publisher")) { 4351 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.publisher"); 4352 } 4353 else if (name.equals("contact")) { 4354 return addContact(); 4355 } 4356 else if (name.equals("author")) { 4357 return addAuthor(); 4358 } 4359 else if (name.equals("editor")) { 4360 return addEditor(); 4361 } 4362 else if (name.equals("reviewer")) { 4363 return addReviewer(); 4364 } 4365 else if (name.equals("endorser")) { 4366 return addEndorser(); 4367 } 4368 else if (name.equals("relatesTo")) { 4369 return addRelatesTo(); 4370 } 4371 else if (name.equals("section")) { 4372 return addSection(); 4373 } 4374 else 4375 return super.addChild(name); 4376 } 4377 4378 public String fhirType() { 4379 return "EvidenceReport"; 4380 4381 } 4382 4383 public EvidenceReport copy() { 4384 EvidenceReport dst = new EvidenceReport(); 4385 copyValues(dst); 4386 return dst; 4387 } 4388 4389 public void copyValues(EvidenceReport dst) { 4390 super.copyValues(dst); 4391 dst.url = url == null ? null : url.copy(); 4392 dst.status = status == null ? null : status.copy(); 4393 if (useContext != null) { 4394 dst.useContext = new ArrayList<UsageContext>(); 4395 for (UsageContext i : useContext) 4396 dst.useContext.add(i.copy()); 4397 }; 4398 if (identifier != null) { 4399 dst.identifier = new ArrayList<Identifier>(); 4400 for (Identifier i : identifier) 4401 dst.identifier.add(i.copy()); 4402 }; 4403 if (relatedIdentifier != null) { 4404 dst.relatedIdentifier = new ArrayList<Identifier>(); 4405 for (Identifier i : relatedIdentifier) 4406 dst.relatedIdentifier.add(i.copy()); 4407 }; 4408 dst.citeAs = citeAs == null ? null : citeAs.copy(); 4409 dst.type = type == null ? null : type.copy(); 4410 if (note != null) { 4411 dst.note = new ArrayList<Annotation>(); 4412 for (Annotation i : note) 4413 dst.note.add(i.copy()); 4414 }; 4415 if (relatedArtifact != null) { 4416 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4417 for (RelatedArtifact i : relatedArtifact) 4418 dst.relatedArtifact.add(i.copy()); 4419 }; 4420 dst.subject = subject == null ? null : subject.copy(); 4421 dst.publisher = publisher == null ? null : publisher.copy(); 4422 if (contact != null) { 4423 dst.contact = new ArrayList<ContactDetail>(); 4424 for (ContactDetail i : contact) 4425 dst.contact.add(i.copy()); 4426 }; 4427 if (author != null) { 4428 dst.author = new ArrayList<ContactDetail>(); 4429 for (ContactDetail i : author) 4430 dst.author.add(i.copy()); 4431 }; 4432 if (editor != null) { 4433 dst.editor = new ArrayList<ContactDetail>(); 4434 for (ContactDetail i : editor) 4435 dst.editor.add(i.copy()); 4436 }; 4437 if (reviewer != null) { 4438 dst.reviewer = new ArrayList<ContactDetail>(); 4439 for (ContactDetail i : reviewer) 4440 dst.reviewer.add(i.copy()); 4441 }; 4442 if (endorser != null) { 4443 dst.endorser = new ArrayList<ContactDetail>(); 4444 for (ContactDetail i : endorser) 4445 dst.endorser.add(i.copy()); 4446 }; 4447 if (relatesTo != null) { 4448 dst.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 4449 for (EvidenceReportRelatesToComponent i : relatesTo) 4450 dst.relatesTo.add(i.copy()); 4451 }; 4452 if (section != null) { 4453 dst.section = new ArrayList<SectionComponent>(); 4454 for (SectionComponent i : section) 4455 dst.section.add(i.copy()); 4456 }; 4457 } 4458 4459 protected EvidenceReport typedCopy() { 4460 return copy(); 4461 } 4462 4463 @Override 4464 public boolean equalsDeep(Base other_) { 4465 if (!super.equalsDeep(other_)) 4466 return false; 4467 if (!(other_ instanceof EvidenceReport)) 4468 return false; 4469 EvidenceReport o = (EvidenceReport) other_; 4470 return compareDeep(url, o.url, true) && compareDeep(status, o.status, true) && compareDeep(useContext, o.useContext, true) 4471 && compareDeep(identifier, o.identifier, true) && compareDeep(relatedIdentifier, o.relatedIdentifier, true) 4472 && compareDeep(citeAs, o.citeAs, true) && compareDeep(type, o.type, true) && compareDeep(note, o.note, true) 4473 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(subject, o.subject, true) 4474 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(author, o.author, true) 4475 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 4476 && compareDeep(relatesTo, o.relatesTo, true) && compareDeep(section, o.section, true); 4477 } 4478 4479 @Override 4480 public boolean equalsShallow(Base other_) { 4481 if (!super.equalsShallow(other_)) 4482 return false; 4483 if (!(other_ instanceof EvidenceReport)) 4484 return false; 4485 EvidenceReport o = (EvidenceReport) other_; 4486 return compareValues(url, o.url, true) && compareValues(status, o.status, true) && compareValues(publisher, o.publisher, true) 4487 ; 4488 } 4489 4490 public boolean isEmpty() { 4491 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, status, useContext 4492 , identifier, relatedIdentifier, citeAs, type, note, relatedArtifact, subject 4493 , publisher, contact, author, editor, reviewer, endorser, relatesTo, section 4494 ); 4495 } 4496 4497 @Override 4498 public ResourceType getResourceType() { 4499 return ResourceType.EvidenceReport; 4500 } 4501 4502 /** 4503 * Search parameter: <b>context-quantity</b> 4504 * <p> 4505 * Description: <b>Multiple Resources: 4506 4507* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4508* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4509* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4510* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4511* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4512* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4513* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4514* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4515* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4516* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4517* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4518* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4519* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4520* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4521* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4522* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4523* [Library](library.html): A quantity- or range-valued use context assigned to the library 4524* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4525* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4526* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4527* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4528* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4529* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4530* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4531* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4532* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4533* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4534* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4535* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4536* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4537</b><br> 4538 * Type: <b>quantity</b><br> 4539 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4540 * </p> 4541 */ 4542 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 4543 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4544 /** 4545 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4546 * <p> 4547 * Description: <b>Multiple Resources: 4548 4549* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4550* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4551* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4552* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4553* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4554* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4555* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4556* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4557* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4558* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4559* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4560* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4561* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4562* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4563* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4564* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4565* [Library](library.html): A quantity- or range-valued use context assigned to the library 4566* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4567* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4568* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4569* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4570* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4571* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4572* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4573* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4574* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4575* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4576* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4577* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4578* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4579</b><br> 4580 * Type: <b>quantity</b><br> 4581 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4582 * </p> 4583 */ 4584 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 4585 4586 /** 4587 * Search parameter: <b>context-type-quantity</b> 4588 * <p> 4589 * Description: <b>Multiple Resources: 4590 4591* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4592* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4593* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4594* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4595* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4596* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4597* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4598* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4599* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4600* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4601* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4602* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4603* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4604* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4605* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4606* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4607* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4608* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4609* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4610* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4611* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4612* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4613* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4614* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4615* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4616* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4617* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4618* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4619* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4620* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4621</b><br> 4622 * Type: <b>composite</b><br> 4623 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4624 * </p> 4625 */ 4626 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 4627 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4628 /** 4629 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 4630 * <p> 4631 * Description: <b>Multiple Resources: 4632 4633* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4634* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4635* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4636* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4637* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4638* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4639* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4640* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4641* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4642* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4643* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4644* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4645* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4646* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4647* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4648* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4649* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4650* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4651* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4652* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4653* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4654* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4655* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4656* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4657* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4658* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4659* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4660* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4661* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4662* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4663</b><br> 4664 * Type: <b>composite</b><br> 4665 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4666 * </p> 4667 */ 4668 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 4669 4670 /** 4671 * Search parameter: <b>context-type-value</b> 4672 * <p> 4673 * Description: <b>Multiple Resources: 4674 4675* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4676* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4677* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4678* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4679* [Citation](citation.html): A use context type and value assigned to the citation 4680* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4681* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4682* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4683* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4684* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4685* [Evidence](evidence.html): A use context type and value assigned to the evidence 4686* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4687* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4688* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4689* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4690* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4691* [Library](library.html): A use context type and value assigned to the library 4692* [Measure](measure.html): A use context type and value assigned to the measure 4693* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4694* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4695* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4696* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4697* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4698* [Requirements](requirements.html): A use context type and value assigned to the requirements 4699* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4700* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4701* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4702* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4703* [TestScript](testscript.html): A use context type and value assigned to the test script 4704* [ValueSet](valueset.html): A use context type and value assigned to the value set 4705</b><br> 4706 * Type: <b>composite</b><br> 4707 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4708 * </p> 4709 */ 4710 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 4711 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4712 /** 4713 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4714 * <p> 4715 * Description: <b>Multiple Resources: 4716 4717* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4718* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4719* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4720* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4721* [Citation](citation.html): A use context type and value assigned to the citation 4722* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4723* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4724* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4725* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4726* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4727* [Evidence](evidence.html): A use context type and value assigned to the evidence 4728* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4729* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4730* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4731* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4732* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4733* [Library](library.html): A use context type and value assigned to the library 4734* [Measure](measure.html): A use context type and value assigned to the measure 4735* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4736* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4737* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4738* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4739* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4740* [Requirements](requirements.html): A use context type and value assigned to the requirements 4741* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4742* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4743* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4744* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4745* [TestScript](testscript.html): A use context type and value assigned to the test script 4746* [ValueSet](valueset.html): A use context type and value assigned to the value set 4747</b><br> 4748 * Type: <b>composite</b><br> 4749 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4750 * </p> 4751 */ 4752 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 4753 4754 /** 4755 * Search parameter: <b>context-type</b> 4756 * <p> 4757 * Description: <b>Multiple Resources: 4758 4759* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4760* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4761* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4762* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4763* [Citation](citation.html): A type of use context assigned to the citation 4764* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4765* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4766* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4767* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4768* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4769* [Evidence](evidence.html): A type of use context assigned to the evidence 4770* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4771* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4772* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4773* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4774* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4775* [Library](library.html): A type of use context assigned to the library 4776* [Measure](measure.html): A type of use context assigned to the measure 4777* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4778* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4779* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4780* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4781* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4782* [Requirements](requirements.html): A type of use context assigned to the requirements 4783* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4784* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4785* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4786* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4787* [TestScript](testscript.html): A type of use context assigned to the test script 4788* [ValueSet](valueset.html): A type of use context assigned to the value set 4789</b><br> 4790 * Type: <b>token</b><br> 4791 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4792 * </p> 4793 */ 4794 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 4795 public static final String SP_CONTEXT_TYPE = "context-type"; 4796 /** 4797 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4798 * <p> 4799 * Description: <b>Multiple Resources: 4800 4801* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4802* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4803* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4804* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4805* [Citation](citation.html): A type of use context assigned to the citation 4806* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4807* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4808* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4809* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4810* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4811* [Evidence](evidence.html): A type of use context assigned to the evidence 4812* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4813* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4814* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4815* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4816* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4817* [Library](library.html): A type of use context assigned to the library 4818* [Measure](measure.html): A type of use context assigned to the measure 4819* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4820* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4821* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4822* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4823* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4824* [Requirements](requirements.html): A type of use context assigned to the requirements 4825* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4826* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4827* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4828* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4829* [TestScript](testscript.html): A type of use context assigned to the test script 4830* [ValueSet](valueset.html): A type of use context assigned to the value set 4831</b><br> 4832 * Type: <b>token</b><br> 4833 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4834 * </p> 4835 */ 4836 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 4837 4838 /** 4839 * Search parameter: <b>context</b> 4840 * <p> 4841 * Description: <b>Multiple Resources: 4842 4843* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4844* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4845* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4846* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4847* [Citation](citation.html): A use context assigned to the citation 4848* [CodeSystem](codesystem.html): A use context assigned to the code system 4849* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4850* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4851* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4852* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4853* [Evidence](evidence.html): A use context assigned to the evidence 4854* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4855* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4856* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4857* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4858* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4859* [Library](library.html): A use context assigned to the library 4860* [Measure](measure.html): A use context assigned to the measure 4861* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4862* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4863* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4864* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4865* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4866* [Requirements](requirements.html): A use context assigned to the requirements 4867* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4868* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4869* [StructureMap](structuremap.html): A use context assigned to the structure map 4870* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4871* [TestScript](testscript.html): A use context assigned to the test script 4872* [ValueSet](valueset.html): A use context assigned to the value set 4873</b><br> 4874 * Type: <b>token</b><br> 4875 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4876 * </p> 4877 */ 4878 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 4879 public static final String SP_CONTEXT = "context"; 4880 /** 4881 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4882 * <p> 4883 * Description: <b>Multiple Resources: 4884 4885* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4886* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4887* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4888* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4889* [Citation](citation.html): A use context assigned to the citation 4890* [CodeSystem](codesystem.html): A use context assigned to the code system 4891* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4892* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4893* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4894* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4895* [Evidence](evidence.html): A use context assigned to the evidence 4896* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4897* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4898* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4899* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4900* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4901* [Library](library.html): A use context assigned to the library 4902* [Measure](measure.html): A use context assigned to the measure 4903* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4904* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4905* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4906* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4907* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4908* [Requirements](requirements.html): A use context assigned to the requirements 4909* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4910* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4911* [StructureMap](structuremap.html): A use context assigned to the structure map 4912* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4913* [TestScript](testscript.html): A use context assigned to the test script 4914* [ValueSet](valueset.html): A use context assigned to the value set 4915</b><br> 4916 * Type: <b>token</b><br> 4917 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4918 * </p> 4919 */ 4920 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4921 4922 /** 4923 * Search parameter: <b>identifier</b> 4924 * <p> 4925 * Description: <b>Multiple Resources: 4926 4927* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4928* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4929* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4930* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4931* [Citation](citation.html): External identifier for the citation 4932* [CodeSystem](codesystem.html): External identifier for the code system 4933* [ConceptMap](conceptmap.html): External identifier for the concept map 4934* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4935* [EventDefinition](eventdefinition.html): External identifier for the event definition 4936* [Evidence](evidence.html): External identifier for the evidence 4937* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4938* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4939* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4940* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4941* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4942* [Library](library.html): External identifier for the library 4943* [Measure](measure.html): External identifier for the measure 4944* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4945* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4946* [NamingSystem](namingsystem.html): External identifier for the naming system 4947* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4948* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4949* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4950* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4951* [Requirements](requirements.html): External identifier for the requirements 4952* [SearchParameter](searchparameter.html): External identifier for the search parameter 4953* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4954* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4955* [StructureMap](structuremap.html): External identifier for the structure map 4956* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4957* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4958* [TestPlan](testplan.html): An identifier for the test plan 4959* [TestScript](testscript.html): External identifier for the test script 4960* [ValueSet](valueset.html): External identifier for the value set 4961</b><br> 4962 * Type: <b>token</b><br> 4963 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4964 * </p> 4965 */ 4966 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4967 public static final String SP_IDENTIFIER = "identifier"; 4968 /** 4969 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4970 * <p> 4971 * Description: <b>Multiple Resources: 4972 4973* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4974* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4975* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4976* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4977* [Citation](citation.html): External identifier for the citation 4978* [CodeSystem](codesystem.html): External identifier for the code system 4979* [ConceptMap](conceptmap.html): External identifier for the concept map 4980* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4981* [EventDefinition](eventdefinition.html): External identifier for the event definition 4982* [Evidence](evidence.html): External identifier for the evidence 4983* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4984* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4985* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4986* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4987* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4988* [Library](library.html): External identifier for the library 4989* [Measure](measure.html): External identifier for the measure 4990* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4991* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4992* [NamingSystem](namingsystem.html): External identifier for the naming system 4993* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4994* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4995* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4996* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4997* [Requirements](requirements.html): External identifier for the requirements 4998* [SearchParameter](searchparameter.html): External identifier for the search parameter 4999* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5000* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5001* [StructureMap](structuremap.html): External identifier for the structure map 5002* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5003* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5004* [TestPlan](testplan.html): An identifier for the test plan 5005* [TestScript](testscript.html): External identifier for the test script 5006* [ValueSet](valueset.html): External identifier for the value set 5007</b><br> 5008 * Type: <b>token</b><br> 5009 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5010 * </p> 5011 */ 5012 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5013 5014 /** 5015 * Search parameter: <b>publisher</b> 5016 * <p> 5017 * Description: <b>Multiple Resources: 5018 5019* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5020* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5021* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5022* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5023* [Citation](citation.html): Name of the publisher of the citation 5024* [CodeSystem](codesystem.html): Name of the publisher of the code system 5025* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5026* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5027* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5028* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5029* [Evidence](evidence.html): Name of the publisher of the evidence 5030* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5031* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5032* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5033* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5034* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5035* [Library](library.html): Name of the publisher of the library 5036* [Measure](measure.html): Name of the publisher of the measure 5037* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5038* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5039* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5040* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5041* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5042* [Requirements](requirements.html): Name of the publisher of the requirements 5043* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5044* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5045* [StructureMap](structuremap.html): Name of the publisher of the structure map 5046* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5047* [TestScript](testscript.html): Name of the publisher of the test script 5048* [ValueSet](valueset.html): Name of the publisher of the value set 5049</b><br> 5050 * Type: <b>string</b><br> 5051 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5052 * </p> 5053 */ 5054 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 5055 public static final String SP_PUBLISHER = "publisher"; 5056 /** 5057 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5058 * <p> 5059 * Description: <b>Multiple Resources: 5060 5061* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5062* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5063* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5064* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5065* [Citation](citation.html): Name of the publisher of the citation 5066* [CodeSystem](codesystem.html): Name of the publisher of the code system 5067* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5068* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5069* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5070* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5071* [Evidence](evidence.html): Name of the publisher of the evidence 5072* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5073* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5074* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5075* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5076* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5077* [Library](library.html): Name of the publisher of the library 5078* [Measure](measure.html): Name of the publisher of the measure 5079* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5080* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5081* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5082* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5083* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5084* [Requirements](requirements.html): Name of the publisher of the requirements 5085* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5086* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5087* [StructureMap](structuremap.html): Name of the publisher of the structure map 5088* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5089* [TestScript](testscript.html): Name of the publisher of the test script 5090* [ValueSet](valueset.html): Name of the publisher of the value set 5091</b><br> 5092 * Type: <b>string</b><br> 5093 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5094 * </p> 5095 */ 5096 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5097 5098 /** 5099 * Search parameter: <b>status</b> 5100 * <p> 5101 * Description: <b>Multiple Resources: 5102 5103* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5104* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5105* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5106* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5107* [Citation](citation.html): The current status of the citation 5108* [CodeSystem](codesystem.html): The current status of the code system 5109* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5110* [ConceptMap](conceptmap.html): The current status of the concept map 5111* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5112* [EventDefinition](eventdefinition.html): The current status of the event definition 5113* [Evidence](evidence.html): The current status of the evidence 5114* [EvidenceReport](evidencereport.html): The current status of the evidence report 5115* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5116* [ExampleScenario](examplescenario.html): The current status of the example scenario 5117* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5118* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5119* [Library](library.html): The current status of the library 5120* [Measure](measure.html): The current status of the measure 5121* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5122* [MessageDefinition](messagedefinition.html): The current status of the message definition 5123* [NamingSystem](namingsystem.html): The current status of the naming system 5124* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5125* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5126* [PlanDefinition](plandefinition.html): The current status of the plan definition 5127* [Questionnaire](questionnaire.html): The current status of the questionnaire 5128* [Requirements](requirements.html): The current status of the requirements 5129* [SearchParameter](searchparameter.html): The current status of the search parameter 5130* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5131* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5132* [StructureMap](structuremap.html): The current status of the structure map 5133* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5134* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5135* [TestPlan](testplan.html): The current status of the test plan 5136* [TestScript](testscript.html): The current status of the test script 5137* [ValueSet](valueset.html): The current status of the value set 5138</b><br> 5139 * Type: <b>token</b><br> 5140 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5141 * </p> 5142 */ 5143 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 5144 public static final String SP_STATUS = "status"; 5145 /** 5146 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5147 * <p> 5148 * Description: <b>Multiple Resources: 5149 5150* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5151* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5152* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5153* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5154* [Citation](citation.html): The current status of the citation 5155* [CodeSystem](codesystem.html): The current status of the code system 5156* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5157* [ConceptMap](conceptmap.html): The current status of the concept map 5158* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5159* [EventDefinition](eventdefinition.html): The current status of the event definition 5160* [Evidence](evidence.html): The current status of the evidence 5161* [EvidenceReport](evidencereport.html): The current status of the evidence report 5162* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5163* [ExampleScenario](examplescenario.html): The current status of the example scenario 5164* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5165* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5166* [Library](library.html): The current status of the library 5167* [Measure](measure.html): The current status of the measure 5168* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5169* [MessageDefinition](messagedefinition.html): The current status of the message definition 5170* [NamingSystem](namingsystem.html): The current status of the naming system 5171* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5172* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5173* [PlanDefinition](plandefinition.html): The current status of the plan definition 5174* [Questionnaire](questionnaire.html): The current status of the questionnaire 5175* [Requirements](requirements.html): The current status of the requirements 5176* [SearchParameter](searchparameter.html): The current status of the search parameter 5177* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5178* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5179* [StructureMap](structuremap.html): The current status of the structure map 5180* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5181* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5182* [TestPlan](testplan.html): The current status of the test plan 5183* [TestScript](testscript.html): The current status of the test script 5184* [ValueSet](valueset.html): The current status of the value set 5185</b><br> 5186 * Type: <b>token</b><br> 5187 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5188 * </p> 5189 */ 5190 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5191 5192 /** 5193 * Search parameter: <b>url</b> 5194 * <p> 5195 * Description: <b>Multiple Resources: 5196 5197* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5198* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5199* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5200* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5201* [Citation](citation.html): The uri that identifies the citation 5202* [CodeSystem](codesystem.html): The uri that identifies the code system 5203* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5204* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5205* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5206* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5207* [Evidence](evidence.html): The uri that identifies the evidence 5208* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5209* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5210* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5211* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5212* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5213* [Library](library.html): The uri that identifies the library 5214* [Measure](measure.html): The uri that identifies the measure 5215* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5216* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5217* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5218* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5219* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5220* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5221* [Requirements](requirements.html): The uri that identifies the requirements 5222* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5223* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5224* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5225* [StructureMap](structuremap.html): The uri that identifies the structure map 5226* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5227* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5228* [TestPlan](testplan.html): The uri that identifies the test plan 5229* [TestScript](testscript.html): The uri that identifies the test script 5230* [ValueSet](valueset.html): The uri that identifies the value set 5231</b><br> 5232 * Type: <b>uri</b><br> 5233 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5234 * </p> 5235 */ 5236 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 5237 public static final String SP_URL = "url"; 5238 /** 5239 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5240 * <p> 5241 * Description: <b>Multiple Resources: 5242 5243* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5244* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5245* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5246* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5247* [Citation](citation.html): The uri that identifies the citation 5248* [CodeSystem](codesystem.html): The uri that identifies the code system 5249* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5250* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5251* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5252* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5253* [Evidence](evidence.html): The uri that identifies the evidence 5254* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5255* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5256* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5257* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5258* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5259* [Library](library.html): The uri that identifies the library 5260* [Measure](measure.html): The uri that identifies the measure 5261* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5262* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5263* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5264* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5265* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5266* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5267* [Requirements](requirements.html): The uri that identifies the requirements 5268* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5269* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5270* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5271* [StructureMap](structuremap.html): The uri that identifies the structure map 5272* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5273* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5274* [TestPlan](testplan.html): The uri that identifies the test plan 5275* [TestScript](testscript.html): The uri that identifies the test script 5276* [ValueSet](valueset.html): The uri that identifies the value set 5277</b><br> 5278 * Type: <b>uri</b><br> 5279 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5280 * </p> 5281 */ 5282 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5283 5284 5285} 5286