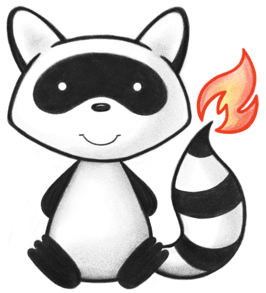
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The EvidenceReport Resource is a specialized container for a collection of resources and codeable concepts, adapted to support compositions of Evidence, EvidenceVariable, and Citation resources and related concepts. 052 */ 053@ResourceDef(name="EvidenceReport", profile="http://hl7.org/fhir/StructureDefinition/EvidenceReport") 054public class EvidenceReport extends MetadataResource { 055 056 public enum ReportRelationshipType { 057 /** 058 * This document replaces or supersedes the target document. 059 */ 060 REPLACES, 061 /** 062 * This document notes corrections or changes to replace or supersede parts of the target document. 063 */ 064 AMENDS, 065 /** 066 * This document adds additional information to the target document. 067 */ 068 APPENDS, 069 /** 070 * This document was generated by transforming the target document (eg format or language conversion). 071 */ 072 TRANSFORMS, 073 /** 074 * This document was. 075 */ 076 REPLACEDWITH, 077 /** 078 * This document was. 079 */ 080 AMENDEDWITH, 081 /** 082 * This document was. 083 */ 084 APPENDEDWITH, 085 /** 086 * This document was. 087 */ 088 TRANSFORMEDWITH, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 public static ReportRelationshipType fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("replaces".equals(codeString)) 097 return REPLACES; 098 if ("amends".equals(codeString)) 099 return AMENDS; 100 if ("appends".equals(codeString)) 101 return APPENDS; 102 if ("transforms".equals(codeString)) 103 return TRANSFORMS; 104 if ("replacedWith".equals(codeString)) 105 return REPLACEDWITH; 106 if ("amendedWith".equals(codeString)) 107 return AMENDEDWITH; 108 if ("appendedWith".equals(codeString)) 109 return APPENDEDWITH; 110 if ("transformedWith".equals(codeString)) 111 return TRANSFORMEDWITH; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown ReportRelationshipType code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case REPLACES: return "replaces"; 120 case AMENDS: return "amends"; 121 case APPENDS: return "appends"; 122 case TRANSFORMS: return "transforms"; 123 case REPLACEDWITH: return "replacedWith"; 124 case AMENDEDWITH: return "amendedWith"; 125 case APPENDEDWITH: return "appendedWith"; 126 case TRANSFORMEDWITH: return "transformedWith"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 public String getSystem() { 132 switch (this) { 133 case REPLACES: return "http://hl7.org/fhir/report-relation-type"; 134 case AMENDS: return "http://hl7.org/fhir/report-relation-type"; 135 case APPENDS: return "http://hl7.org/fhir/report-relation-type"; 136 case TRANSFORMS: return "http://hl7.org/fhir/report-relation-type"; 137 case REPLACEDWITH: return "http://hl7.org/fhir/report-relation-type"; 138 case AMENDEDWITH: return "http://hl7.org/fhir/report-relation-type"; 139 case APPENDEDWITH: return "http://hl7.org/fhir/report-relation-type"; 140 case TRANSFORMEDWITH: return "http://hl7.org/fhir/report-relation-type"; 141 case NULL: return null; 142 default: return "?"; 143 } 144 } 145 public String getDefinition() { 146 switch (this) { 147 case REPLACES: return "This document replaces or supersedes the target document."; 148 case AMENDS: return "This document notes corrections or changes to replace or supersede parts of the target document."; 149 case APPENDS: return "This document adds additional information to the target document."; 150 case TRANSFORMS: return "This document was generated by transforming the target document (eg format or language conversion)."; 151 case REPLACEDWITH: return "This document was."; 152 case AMENDEDWITH: return "This document was."; 153 case APPENDEDWITH: return "This document was."; 154 case TRANSFORMEDWITH: return "This document was."; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 public String getDisplay() { 160 switch (this) { 161 case REPLACES: return "Replaces"; 162 case AMENDS: return "Amends"; 163 case APPENDS: return "Appends"; 164 case TRANSFORMS: return "Transforms"; 165 case REPLACEDWITH: return "Replaced With"; 166 case AMENDEDWITH: return "Amended With"; 167 case APPENDEDWITH: return "Appended With"; 168 case TRANSFORMEDWITH: return "Transformed With"; 169 case NULL: return null; 170 default: return "?"; 171 } 172 } 173 } 174 175 public static class ReportRelationshipTypeEnumFactory implements EnumFactory<ReportRelationshipType> { 176 public ReportRelationshipType fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("replaces".equals(codeString)) 181 return ReportRelationshipType.REPLACES; 182 if ("amends".equals(codeString)) 183 return ReportRelationshipType.AMENDS; 184 if ("appends".equals(codeString)) 185 return ReportRelationshipType.APPENDS; 186 if ("transforms".equals(codeString)) 187 return ReportRelationshipType.TRANSFORMS; 188 if ("replacedWith".equals(codeString)) 189 return ReportRelationshipType.REPLACEDWITH; 190 if ("amendedWith".equals(codeString)) 191 return ReportRelationshipType.AMENDEDWITH; 192 if ("appendedWith".equals(codeString)) 193 return ReportRelationshipType.APPENDEDWITH; 194 if ("transformedWith".equals(codeString)) 195 return ReportRelationshipType.TRANSFORMEDWITH; 196 throw new IllegalArgumentException("Unknown ReportRelationshipType code '"+codeString+"'"); 197 } 198 public Enumeration<ReportRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.NULL, code); 203 String codeString = ((PrimitiveType) code).asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.NULL, code); 206 if ("replaces".equals(codeString)) 207 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.REPLACES, code); 208 if ("amends".equals(codeString)) 209 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.AMENDS, code); 210 if ("appends".equals(codeString)) 211 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.APPENDS, code); 212 if ("transforms".equals(codeString)) 213 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.TRANSFORMS, code); 214 if ("replacedWith".equals(codeString)) 215 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.REPLACEDWITH, code); 216 if ("amendedWith".equals(codeString)) 217 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.AMENDEDWITH, code); 218 if ("appendedWith".equals(codeString)) 219 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.APPENDEDWITH, code); 220 if ("transformedWith".equals(codeString)) 221 return new Enumeration<ReportRelationshipType>(this, ReportRelationshipType.TRANSFORMEDWITH, code); 222 throw new FHIRException("Unknown ReportRelationshipType code '"+codeString+"'"); 223 } 224 public String toCode(ReportRelationshipType code) { 225 if (code == ReportRelationshipType.NULL) 226 return null; 227 if (code == ReportRelationshipType.REPLACES) 228 return "replaces"; 229 if (code == ReportRelationshipType.AMENDS) 230 return "amends"; 231 if (code == ReportRelationshipType.APPENDS) 232 return "appends"; 233 if (code == ReportRelationshipType.TRANSFORMS) 234 return "transforms"; 235 if (code == ReportRelationshipType.REPLACEDWITH) 236 return "replacedWith"; 237 if (code == ReportRelationshipType.AMENDEDWITH) 238 return "amendedWith"; 239 if (code == ReportRelationshipType.APPENDEDWITH) 240 return "appendedWith"; 241 if (code == ReportRelationshipType.TRANSFORMEDWITH) 242 return "transformedWith"; 243 return "?"; 244 } 245 public String toSystem(ReportRelationshipType code) { 246 return code.getSystem(); 247 } 248 } 249 250 @Block() 251 public static class EvidenceReportSubjectComponent extends BackboneElement implements IBaseBackboneElement { 252 /** 253 * Characteristic. 254 */ 255 @Child(name = "characteristic", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 256 @Description(shortDefinition="Characteristic", formalDefinition="Characteristic." ) 257 protected List<EvidenceReportSubjectCharacteristicComponent> characteristic; 258 259 /** 260 * Used for general notes and annotations not coded elsewhere. 261 */ 262 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 263 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Used for general notes and annotations not coded elsewhere." ) 264 protected List<Annotation> note; 265 266 private static final long serialVersionUID = -734040873L; 267 268 /** 269 * Constructor 270 */ 271 public EvidenceReportSubjectComponent() { 272 super(); 273 } 274 275 /** 276 * @return {@link #characteristic} (Characteristic.) 277 */ 278 public List<EvidenceReportSubjectCharacteristicComponent> getCharacteristic() { 279 if (this.characteristic == null) 280 this.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 281 return this.characteristic; 282 } 283 284 /** 285 * @return Returns a reference to <code>this</code> for easy method chaining 286 */ 287 public EvidenceReportSubjectComponent setCharacteristic(List<EvidenceReportSubjectCharacteristicComponent> theCharacteristic) { 288 this.characteristic = theCharacteristic; 289 return this; 290 } 291 292 public boolean hasCharacteristic() { 293 if (this.characteristic == null) 294 return false; 295 for (EvidenceReportSubjectCharacteristicComponent item : this.characteristic) 296 if (!item.isEmpty()) 297 return true; 298 return false; 299 } 300 301 public EvidenceReportSubjectCharacteristicComponent addCharacteristic() { //3 302 EvidenceReportSubjectCharacteristicComponent t = new EvidenceReportSubjectCharacteristicComponent(); 303 if (this.characteristic == null) 304 this.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 305 this.characteristic.add(t); 306 return t; 307 } 308 309 public EvidenceReportSubjectComponent addCharacteristic(EvidenceReportSubjectCharacteristicComponent t) { //3 310 if (t == null) 311 return this; 312 if (this.characteristic == null) 313 this.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 314 this.characteristic.add(t); 315 return this; 316 } 317 318 /** 319 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 320 */ 321 public EvidenceReportSubjectCharacteristicComponent getCharacteristicFirstRep() { 322 if (getCharacteristic().isEmpty()) { 323 addCharacteristic(); 324 } 325 return getCharacteristic().get(0); 326 } 327 328 /** 329 * @return {@link #note} (Used for general notes and annotations not coded elsewhere.) 330 */ 331 public List<Annotation> getNote() { 332 if (this.note == null) 333 this.note = new ArrayList<Annotation>(); 334 return this.note; 335 } 336 337 /** 338 * @return Returns a reference to <code>this</code> for easy method chaining 339 */ 340 public EvidenceReportSubjectComponent setNote(List<Annotation> theNote) { 341 this.note = theNote; 342 return this; 343 } 344 345 public boolean hasNote() { 346 if (this.note == null) 347 return false; 348 for (Annotation item : this.note) 349 if (!item.isEmpty()) 350 return true; 351 return false; 352 } 353 354 public Annotation addNote() { //3 355 Annotation t = new Annotation(); 356 if (this.note == null) 357 this.note = new ArrayList<Annotation>(); 358 this.note.add(t); 359 return t; 360 } 361 362 public EvidenceReportSubjectComponent addNote(Annotation t) { //3 363 if (t == null) 364 return this; 365 if (this.note == null) 366 this.note = new ArrayList<Annotation>(); 367 this.note.add(t); 368 return this; 369 } 370 371 /** 372 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 373 */ 374 public Annotation getNoteFirstRep() { 375 if (getNote().isEmpty()) { 376 addNote(); 377 } 378 return getNote().get(0); 379 } 380 381 protected void listChildren(List<Property> children) { 382 super.listChildren(children); 383 children.add(new Property("characteristic", "", "Characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 384 children.add(new Property("note", "Annotation", "Used for general notes and annotations not coded elsewhere.", 0, java.lang.Integer.MAX_VALUE, note)); 385 } 386 387 @Override 388 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 389 switch (_hash) { 390 case 366313883: /*characteristic*/ return new Property("characteristic", "", "Characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic); 391 case 3387378: /*note*/ return new Property("note", "Annotation", "Used for general notes and annotations not coded elsewhere.", 0, java.lang.Integer.MAX_VALUE, note); 392 default: return super.getNamedProperty(_hash, _name, _checkValid); 393 } 394 395 } 396 397 @Override 398 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 399 switch (hash) { 400 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceReportSubjectCharacteristicComponent 401 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 402 default: return super.getProperty(hash, name, checkValid); 403 } 404 405 } 406 407 @Override 408 public Base setProperty(int hash, String name, Base value) throws FHIRException { 409 switch (hash) { 410 case 366313883: // characteristic 411 this.getCharacteristic().add((EvidenceReportSubjectCharacteristicComponent) value); // EvidenceReportSubjectCharacteristicComponent 412 return value; 413 case 3387378: // note 414 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 415 return value; 416 default: return super.setProperty(hash, name, value); 417 } 418 419 } 420 421 @Override 422 public Base setProperty(String name, Base value) throws FHIRException { 423 if (name.equals("characteristic")) { 424 this.getCharacteristic().add((EvidenceReportSubjectCharacteristicComponent) value); 425 } else if (name.equals("note")) { 426 this.getNote().add(TypeConvertor.castToAnnotation(value)); 427 } else 428 return super.setProperty(name, value); 429 return value; 430 } 431 432 @Override 433 public void removeChild(String name, Base value) throws FHIRException { 434 if (name.equals("characteristic")) { 435 this.getCharacteristic().remove((EvidenceReportSubjectCharacteristicComponent) value); 436 } else if (name.equals("note")) { 437 this.getNote().remove(value); 438 } else 439 super.removeChild(name, value); 440 441 } 442 443 @Override 444 public Base makeProperty(int hash, String name) throws FHIRException { 445 switch (hash) { 446 case 366313883: return addCharacteristic(); 447 case 3387378: return addNote(); 448 default: return super.makeProperty(hash, name); 449 } 450 451 } 452 453 @Override 454 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 455 switch (hash) { 456 case 366313883: /*characteristic*/ return new String[] {}; 457 case 3387378: /*note*/ return new String[] {"Annotation"}; 458 default: return super.getTypesForProperty(hash, name); 459 } 460 461 } 462 463 @Override 464 public Base addChild(String name) throws FHIRException { 465 if (name.equals("characteristic")) { 466 return addCharacteristic(); 467 } 468 else if (name.equals("note")) { 469 return addNote(); 470 } 471 else 472 return super.addChild(name); 473 } 474 475 public EvidenceReportSubjectComponent copy() { 476 EvidenceReportSubjectComponent dst = new EvidenceReportSubjectComponent(); 477 copyValues(dst); 478 return dst; 479 } 480 481 public void copyValues(EvidenceReportSubjectComponent dst) { 482 super.copyValues(dst); 483 if (characteristic != null) { 484 dst.characteristic = new ArrayList<EvidenceReportSubjectCharacteristicComponent>(); 485 for (EvidenceReportSubjectCharacteristicComponent i : characteristic) 486 dst.characteristic.add(i.copy()); 487 }; 488 if (note != null) { 489 dst.note = new ArrayList<Annotation>(); 490 for (Annotation i : note) 491 dst.note.add(i.copy()); 492 }; 493 } 494 495 @Override 496 public boolean equalsDeep(Base other_) { 497 if (!super.equalsDeep(other_)) 498 return false; 499 if (!(other_ instanceof EvidenceReportSubjectComponent)) 500 return false; 501 EvidenceReportSubjectComponent o = (EvidenceReportSubjectComponent) other_; 502 return compareDeep(characteristic, o.characteristic, true) && compareDeep(note, o.note, true); 503 } 504 505 @Override 506 public boolean equalsShallow(Base other_) { 507 if (!super.equalsShallow(other_)) 508 return false; 509 if (!(other_ instanceof EvidenceReportSubjectComponent)) 510 return false; 511 EvidenceReportSubjectComponent o = (EvidenceReportSubjectComponent) other_; 512 return true; 513 } 514 515 public boolean isEmpty() { 516 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(characteristic, note); 517 } 518 519 public String fhirType() { 520 return "EvidenceReport.subject"; 521 522 } 523 524 } 525 526 @Block() 527 public static class EvidenceReportSubjectCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 528 /** 529 * Characteristic code. 530 */ 531 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 532 @Description(shortDefinition="Characteristic code", formalDefinition="Characteristic code." ) 533 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/focus-characteristic-code") 534 protected CodeableConcept code; 535 536 /** 537 * Characteristic value. 538 */ 539 @Child(name = "value", type = {Reference.class, CodeableConcept.class, BooleanType.class, Quantity.class, Range.class}, order=2, min=1, max=1, modifier=false, summary=false) 540 @Description(shortDefinition="Characteristic value", formalDefinition="Characteristic value." ) 541 protected DataType value; 542 543 /** 544 * Is used to express not the characteristic. 545 */ 546 @Child(name = "exclude", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 547 @Description(shortDefinition="Is used to express not the characteristic", formalDefinition="Is used to express not the characteristic." ) 548 protected BooleanType exclude; 549 550 /** 551 * Timeframe for the characteristic. 552 */ 553 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 554 @Description(shortDefinition="Timeframe for the characteristic", formalDefinition="Timeframe for the characteristic." ) 555 protected Period period; 556 557 private static final long serialVersionUID = 279867823L; 558 559 /** 560 * Constructor 561 */ 562 public EvidenceReportSubjectCharacteristicComponent() { 563 super(); 564 } 565 566 /** 567 * Constructor 568 */ 569 public EvidenceReportSubjectCharacteristicComponent(CodeableConcept code, DataType value) { 570 super(); 571 this.setCode(code); 572 this.setValue(value); 573 } 574 575 /** 576 * @return {@link #code} (Characteristic code.) 577 */ 578 public CodeableConcept getCode() { 579 if (this.code == null) 580 if (Configuration.errorOnAutoCreate()) 581 throw new Error("Attempt to auto-create EvidenceReportSubjectCharacteristicComponent.code"); 582 else if (Configuration.doAutoCreate()) 583 this.code = new CodeableConcept(); // cc 584 return this.code; 585 } 586 587 public boolean hasCode() { 588 return this.code != null && !this.code.isEmpty(); 589 } 590 591 /** 592 * @param value {@link #code} (Characteristic code.) 593 */ 594 public EvidenceReportSubjectCharacteristicComponent setCode(CodeableConcept value) { 595 this.code = value; 596 return this; 597 } 598 599 /** 600 * @return {@link #value} (Characteristic value.) 601 */ 602 public DataType getValue() { 603 return this.value; 604 } 605 606 /** 607 * @return {@link #value} (Characteristic value.) 608 */ 609 public Reference getValueReference() throws FHIRException { 610 if (this.value == null) 611 this.value = new Reference(); 612 if (!(this.value instanceof Reference)) 613 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 614 return (Reference) this.value; 615 } 616 617 public boolean hasValueReference() { 618 return this.value instanceof Reference; 619 } 620 621 /** 622 * @return {@link #value} (Characteristic value.) 623 */ 624 public CodeableConcept getValueCodeableConcept() throws FHIRException { 625 if (this.value == null) 626 this.value = new CodeableConcept(); 627 if (!(this.value instanceof CodeableConcept)) 628 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 629 return (CodeableConcept) this.value; 630 } 631 632 public boolean hasValueCodeableConcept() { 633 return this.value instanceof CodeableConcept; 634 } 635 636 /** 637 * @return {@link #value} (Characteristic value.) 638 */ 639 public BooleanType getValueBooleanType() throws FHIRException { 640 if (this.value == null) 641 this.value = new BooleanType(); 642 if (!(this.value instanceof BooleanType)) 643 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 644 return (BooleanType) this.value; 645 } 646 647 public boolean hasValueBooleanType() { 648 return this.value instanceof BooleanType; 649 } 650 651 /** 652 * @return {@link #value} (Characteristic value.) 653 */ 654 public Quantity getValueQuantity() throws FHIRException { 655 if (this.value == null) 656 this.value = new Quantity(); 657 if (!(this.value instanceof Quantity)) 658 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 659 return (Quantity) this.value; 660 } 661 662 public boolean hasValueQuantity() { 663 return this.value instanceof Quantity; 664 } 665 666 /** 667 * @return {@link #value} (Characteristic value.) 668 */ 669 public Range getValueRange() throws FHIRException { 670 if (this.value == null) 671 this.value = new Range(); 672 if (!(this.value instanceof Range)) 673 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 674 return (Range) this.value; 675 } 676 677 public boolean hasValueRange() { 678 return this.value instanceof Range; 679 } 680 681 public boolean hasValue() { 682 return this.value != null && !this.value.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #value} (Characteristic value.) 687 */ 688 public EvidenceReportSubjectCharacteristicComponent setValue(DataType value) { 689 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range)) 690 throw new FHIRException("Not the right type for EvidenceReport.subject.characteristic.value[x]: "+value.fhirType()); 691 this.value = value; 692 return this; 693 } 694 695 /** 696 * @return {@link #exclude} (Is used to express not the characteristic.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 697 */ 698 public BooleanType getExcludeElement() { 699 if (this.exclude == null) 700 if (Configuration.errorOnAutoCreate()) 701 throw new Error("Attempt to auto-create EvidenceReportSubjectCharacteristicComponent.exclude"); 702 else if (Configuration.doAutoCreate()) 703 this.exclude = new BooleanType(); // bb 704 return this.exclude; 705 } 706 707 public boolean hasExcludeElement() { 708 return this.exclude != null && !this.exclude.isEmpty(); 709 } 710 711 public boolean hasExclude() { 712 return this.exclude != null && !this.exclude.isEmpty(); 713 } 714 715 /** 716 * @param value {@link #exclude} (Is used to express not the characteristic.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 717 */ 718 public EvidenceReportSubjectCharacteristicComponent setExcludeElement(BooleanType value) { 719 this.exclude = value; 720 return this; 721 } 722 723 /** 724 * @return Is used to express not the characteristic. 725 */ 726 public boolean getExclude() { 727 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 728 } 729 730 /** 731 * @param value Is used to express not the characteristic. 732 */ 733 public EvidenceReportSubjectCharacteristicComponent setExclude(boolean value) { 734 if (this.exclude == null) 735 this.exclude = new BooleanType(); 736 this.exclude.setValue(value); 737 return this; 738 } 739 740 /** 741 * @return {@link #period} (Timeframe for the characteristic.) 742 */ 743 public Period getPeriod() { 744 if (this.period == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create EvidenceReportSubjectCharacteristicComponent.period"); 747 else if (Configuration.doAutoCreate()) 748 this.period = new Period(); // cc 749 return this.period; 750 } 751 752 public boolean hasPeriod() { 753 return this.period != null && !this.period.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #period} (Timeframe for the characteristic.) 758 */ 759 public EvidenceReportSubjectCharacteristicComponent setPeriod(Period value) { 760 this.period = value; 761 return this; 762 } 763 764 protected void listChildren(List<Property> children) { 765 super.listChildren(children); 766 children.add(new Property("code", "CodeableConcept", "Characteristic code.", 0, 1, code)); 767 children.add(new Property("value[x]", "Reference(Any)|CodeableConcept|boolean|Quantity|Range", "Characteristic value.", 0, 1, value)); 768 children.add(new Property("exclude", "boolean", "Is used to express not the characteristic.", 0, 1, exclude)); 769 children.add(new Property("period", "Period", "Timeframe for the characteristic.", 0, 1, period)); 770 } 771 772 @Override 773 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 774 switch (_hash) { 775 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Characteristic code.", 0, 1, code); 776 case -1410166417: /*value[x]*/ return new Property("value[x]", "Reference(Any)|CodeableConcept|boolean|Quantity|Range", "Characteristic value.", 0, 1, value); 777 case 111972721: /*value*/ return new Property("value[x]", "Reference(Any)|CodeableConcept|boolean|Quantity|Range", "Characteristic value.", 0, 1, value); 778 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "Characteristic value.", 0, 1, value); 779 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Characteristic value.", 0, 1, value); 780 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Characteristic value.", 0, 1, value); 781 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Characteristic value.", 0, 1, value); 782 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Characteristic value.", 0, 1, value); 783 case -1321148966: /*exclude*/ return new Property("exclude", "boolean", "Is used to express not the characteristic.", 0, 1, exclude); 784 case -991726143: /*period*/ return new Property("period", "Period", "Timeframe for the characteristic.", 0, 1, period); 785 default: return super.getNamedProperty(_hash, _name, _checkValid); 786 } 787 788 } 789 790 @Override 791 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 792 switch (hash) { 793 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 794 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 795 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // BooleanType 796 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 797 default: return super.getProperty(hash, name, checkValid); 798 } 799 800 } 801 802 @Override 803 public Base setProperty(int hash, String name, Base value) throws FHIRException { 804 switch (hash) { 805 case 3059181: // code 806 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 807 return value; 808 case 111972721: // value 809 this.value = TypeConvertor.castToType(value); // DataType 810 return value; 811 case -1321148966: // exclude 812 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 813 return value; 814 case -991726143: // period 815 this.period = TypeConvertor.castToPeriod(value); // Period 816 return value; 817 default: return super.setProperty(hash, name, value); 818 } 819 820 } 821 822 @Override 823 public Base setProperty(String name, Base value) throws FHIRException { 824 if (name.equals("code")) { 825 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 826 } else if (name.equals("value[x]")) { 827 this.value = TypeConvertor.castToType(value); // DataType 828 } else if (name.equals("exclude")) { 829 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 830 } else if (name.equals("period")) { 831 this.period = TypeConvertor.castToPeriod(value); // Period 832 } else 833 return super.setProperty(name, value); 834 return value; 835 } 836 837 @Override 838 public void removeChild(String name, Base value) throws FHIRException { 839 if (name.equals("code")) { 840 this.code = null; 841 } else if (name.equals("value[x]")) { 842 this.value = null; 843 } else if (name.equals("exclude")) { 844 this.exclude = null; 845 } else if (name.equals("period")) { 846 this.period = null; 847 } else 848 super.removeChild(name, value); 849 850 } 851 852 @Override 853 public Base makeProperty(int hash, String name) throws FHIRException { 854 switch (hash) { 855 case 3059181: return getCode(); 856 case -1410166417: return getValue(); 857 case 111972721: return getValue(); 858 case -1321148966: return getExcludeElement(); 859 case -991726143: return getPeriod(); 860 default: return super.makeProperty(hash, name); 861 } 862 863 } 864 865 @Override 866 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 867 switch (hash) { 868 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 869 case 111972721: /*value*/ return new String[] {"Reference", "CodeableConcept", "boolean", "Quantity", "Range"}; 870 case -1321148966: /*exclude*/ return new String[] {"boolean"}; 871 case -991726143: /*period*/ return new String[] {"Period"}; 872 default: return super.getTypesForProperty(hash, name); 873 } 874 875 } 876 877 @Override 878 public Base addChild(String name) throws FHIRException { 879 if (name.equals("code")) { 880 this.code = new CodeableConcept(); 881 return this.code; 882 } 883 else if (name.equals("valueReference")) { 884 this.value = new Reference(); 885 return this.value; 886 } 887 else if (name.equals("valueCodeableConcept")) { 888 this.value = new CodeableConcept(); 889 return this.value; 890 } 891 else if (name.equals("valueBoolean")) { 892 this.value = new BooleanType(); 893 return this.value; 894 } 895 else if (name.equals("valueQuantity")) { 896 this.value = new Quantity(); 897 return this.value; 898 } 899 else if (name.equals("valueRange")) { 900 this.value = new Range(); 901 return this.value; 902 } 903 else if (name.equals("exclude")) { 904 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.subject.characteristic.exclude"); 905 } 906 else if (name.equals("period")) { 907 this.period = new Period(); 908 return this.period; 909 } 910 else 911 return super.addChild(name); 912 } 913 914 public EvidenceReportSubjectCharacteristicComponent copy() { 915 EvidenceReportSubjectCharacteristicComponent dst = new EvidenceReportSubjectCharacteristicComponent(); 916 copyValues(dst); 917 return dst; 918 } 919 920 public void copyValues(EvidenceReportSubjectCharacteristicComponent dst) { 921 super.copyValues(dst); 922 dst.code = code == null ? null : code.copy(); 923 dst.value = value == null ? null : value.copy(); 924 dst.exclude = exclude == null ? null : exclude.copy(); 925 dst.period = period == null ? null : period.copy(); 926 } 927 928 @Override 929 public boolean equalsDeep(Base other_) { 930 if (!super.equalsDeep(other_)) 931 return false; 932 if (!(other_ instanceof EvidenceReportSubjectCharacteristicComponent)) 933 return false; 934 EvidenceReportSubjectCharacteristicComponent o = (EvidenceReportSubjectCharacteristicComponent) other_; 935 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(exclude, o.exclude, true) 936 && compareDeep(period, o.period, true); 937 } 938 939 @Override 940 public boolean equalsShallow(Base other_) { 941 if (!super.equalsShallow(other_)) 942 return false; 943 if (!(other_ instanceof EvidenceReportSubjectCharacteristicComponent)) 944 return false; 945 EvidenceReportSubjectCharacteristicComponent o = (EvidenceReportSubjectCharacteristicComponent) other_; 946 return compareValues(exclude, o.exclude, true); 947 } 948 949 public boolean isEmpty() { 950 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, exclude, period 951 ); 952 } 953 954 public String fhirType() { 955 return "EvidenceReport.subject.characteristic"; 956 957 } 958 959 } 960 961 @Block() 962 public static class EvidenceReportRelatesToComponent extends BackboneElement implements IBaseBackboneElement { 963 /** 964 * The type of relationship that this composition has with anther composition or document. 965 */ 966 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 967 @Description(shortDefinition="replaces | amends | appends | transforms | replacedWith | amendedWith | appendedWith | transformedWith", formalDefinition="The type of relationship that this composition has with anther composition or document." ) 968 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-relation-type") 969 protected Enumeration<ReportRelationshipType> code; 970 971 /** 972 * The target composition/document of this relationship. 973 */ 974 @Child(name = "target", type = {}, order=2, min=1, max=1, modifier=false, summary=false) 975 @Description(shortDefinition="Target of the relationship", formalDefinition="The target composition/document of this relationship." ) 976 protected EvidenceReportRelatesToTargetComponent target; 977 978 private static final long serialVersionUID = -1716908969L; 979 980 /** 981 * Constructor 982 */ 983 public EvidenceReportRelatesToComponent() { 984 super(); 985 } 986 987 /** 988 * Constructor 989 */ 990 public EvidenceReportRelatesToComponent(ReportRelationshipType code, EvidenceReportRelatesToTargetComponent target) { 991 super(); 992 this.setCode(code); 993 this.setTarget(target); 994 } 995 996 /** 997 * @return {@link #code} (The type of relationship that this composition has with anther composition or document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 998 */ 999 public Enumeration<ReportRelationshipType> getCodeElement() { 1000 if (this.code == null) 1001 if (Configuration.errorOnAutoCreate()) 1002 throw new Error("Attempt to auto-create EvidenceReportRelatesToComponent.code"); 1003 else if (Configuration.doAutoCreate()) 1004 this.code = new Enumeration<ReportRelationshipType>(new ReportRelationshipTypeEnumFactory()); // bb 1005 return this.code; 1006 } 1007 1008 public boolean hasCodeElement() { 1009 return this.code != null && !this.code.isEmpty(); 1010 } 1011 1012 public boolean hasCode() { 1013 return this.code != null && !this.code.isEmpty(); 1014 } 1015 1016 /** 1017 * @param value {@link #code} (The type of relationship that this composition has with anther composition or document.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1018 */ 1019 public EvidenceReportRelatesToComponent setCodeElement(Enumeration<ReportRelationshipType> value) { 1020 this.code = value; 1021 return this; 1022 } 1023 1024 /** 1025 * @return The type of relationship that this composition has with anther composition or document. 1026 */ 1027 public ReportRelationshipType getCode() { 1028 return this.code == null ? null : this.code.getValue(); 1029 } 1030 1031 /** 1032 * @param value The type of relationship that this composition has with anther composition or document. 1033 */ 1034 public EvidenceReportRelatesToComponent setCode(ReportRelationshipType value) { 1035 if (this.code == null) 1036 this.code = new Enumeration<ReportRelationshipType>(new ReportRelationshipTypeEnumFactory()); 1037 this.code.setValue(value); 1038 return this; 1039 } 1040 1041 /** 1042 * @return {@link #target} (The target composition/document of this relationship.) 1043 */ 1044 public EvidenceReportRelatesToTargetComponent getTarget() { 1045 if (this.target == null) 1046 if (Configuration.errorOnAutoCreate()) 1047 throw new Error("Attempt to auto-create EvidenceReportRelatesToComponent.target"); 1048 else if (Configuration.doAutoCreate()) 1049 this.target = new EvidenceReportRelatesToTargetComponent(); // cc 1050 return this.target; 1051 } 1052 1053 public boolean hasTarget() { 1054 return this.target != null && !this.target.isEmpty(); 1055 } 1056 1057 /** 1058 * @param value {@link #target} (The target composition/document of this relationship.) 1059 */ 1060 public EvidenceReportRelatesToComponent setTarget(EvidenceReportRelatesToTargetComponent value) { 1061 this.target = value; 1062 return this; 1063 } 1064 1065 protected void listChildren(List<Property> children) { 1066 super.listChildren(children); 1067 children.add(new Property("code", "code", "The type of relationship that this composition has with anther composition or document.", 0, 1, code)); 1068 children.add(new Property("target", "", "The target composition/document of this relationship.", 0, 1, target)); 1069 } 1070 1071 @Override 1072 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1073 switch (_hash) { 1074 case 3059181: /*code*/ return new Property("code", "code", "The type of relationship that this composition has with anther composition or document.", 0, 1, code); 1075 case -880905839: /*target*/ return new Property("target", "", "The target composition/document of this relationship.", 0, 1, target); 1076 default: return super.getNamedProperty(_hash, _name, _checkValid); 1077 } 1078 1079 } 1080 1081 @Override 1082 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1083 switch (hash) { 1084 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<ReportRelationshipType> 1085 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // EvidenceReportRelatesToTargetComponent 1086 default: return super.getProperty(hash, name, checkValid); 1087 } 1088 1089 } 1090 1091 @Override 1092 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1093 switch (hash) { 1094 case 3059181: // code 1095 value = new ReportRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1096 this.code = (Enumeration) value; // Enumeration<ReportRelationshipType> 1097 return value; 1098 case -880905839: // target 1099 this.target = (EvidenceReportRelatesToTargetComponent) value; // EvidenceReportRelatesToTargetComponent 1100 return value; 1101 default: return super.setProperty(hash, name, value); 1102 } 1103 1104 } 1105 1106 @Override 1107 public Base setProperty(String name, Base value) throws FHIRException { 1108 if (name.equals("code")) { 1109 value = new ReportRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1110 this.code = (Enumeration) value; // Enumeration<ReportRelationshipType> 1111 } else if (name.equals("target")) { 1112 this.target = (EvidenceReportRelatesToTargetComponent) value; // EvidenceReportRelatesToTargetComponent 1113 } else 1114 return super.setProperty(name, value); 1115 return value; 1116 } 1117 1118 @Override 1119 public void removeChild(String name, Base value) throws FHIRException { 1120 if (name.equals("code")) { 1121 value = new ReportRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1122 this.code = (Enumeration) value; // Enumeration<ReportRelationshipType> 1123 } else if (name.equals("target")) { 1124 this.target = (EvidenceReportRelatesToTargetComponent) value; // EvidenceReportRelatesToTargetComponent 1125 } else 1126 super.removeChild(name, value); 1127 1128 } 1129 1130 @Override 1131 public Base makeProperty(int hash, String name) throws FHIRException { 1132 switch (hash) { 1133 case 3059181: return getCodeElement(); 1134 case -880905839: return getTarget(); 1135 default: return super.makeProperty(hash, name); 1136 } 1137 1138 } 1139 1140 @Override 1141 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1142 switch (hash) { 1143 case 3059181: /*code*/ return new String[] {"code"}; 1144 case -880905839: /*target*/ return new String[] {}; 1145 default: return super.getTypesForProperty(hash, name); 1146 } 1147 1148 } 1149 1150 @Override 1151 public Base addChild(String name) throws FHIRException { 1152 if (name.equals("code")) { 1153 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.relatesTo.code"); 1154 } 1155 else if (name.equals("target")) { 1156 this.target = new EvidenceReportRelatesToTargetComponent(); 1157 return this.target; 1158 } 1159 else 1160 return super.addChild(name); 1161 } 1162 1163 public EvidenceReportRelatesToComponent copy() { 1164 EvidenceReportRelatesToComponent dst = new EvidenceReportRelatesToComponent(); 1165 copyValues(dst); 1166 return dst; 1167 } 1168 1169 public void copyValues(EvidenceReportRelatesToComponent dst) { 1170 super.copyValues(dst); 1171 dst.code = code == null ? null : code.copy(); 1172 dst.target = target == null ? null : target.copy(); 1173 } 1174 1175 @Override 1176 public boolean equalsDeep(Base other_) { 1177 if (!super.equalsDeep(other_)) 1178 return false; 1179 if (!(other_ instanceof EvidenceReportRelatesToComponent)) 1180 return false; 1181 EvidenceReportRelatesToComponent o = (EvidenceReportRelatesToComponent) other_; 1182 return compareDeep(code, o.code, true) && compareDeep(target, o.target, true); 1183 } 1184 1185 @Override 1186 public boolean equalsShallow(Base other_) { 1187 if (!super.equalsShallow(other_)) 1188 return false; 1189 if (!(other_ instanceof EvidenceReportRelatesToComponent)) 1190 return false; 1191 EvidenceReportRelatesToComponent o = (EvidenceReportRelatesToComponent) other_; 1192 return compareValues(code, o.code, true); 1193 } 1194 1195 public boolean isEmpty() { 1196 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, target); 1197 } 1198 1199 public String fhirType() { 1200 return "EvidenceReport.relatesTo"; 1201 1202 } 1203 1204 } 1205 1206 @Block() 1207 public static class EvidenceReportRelatesToTargetComponent extends BackboneElement implements IBaseBackboneElement { 1208 /** 1209 * Target of the relationship URL. 1210 */ 1211 @Child(name = "url", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1212 @Description(shortDefinition="Target of the relationship URL", formalDefinition="Target of the relationship URL." ) 1213 protected UriType url; 1214 1215 /** 1216 * Target of the relationship Identifier. 1217 */ 1218 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=false) 1219 @Description(shortDefinition="Target of the relationship Identifier", formalDefinition="Target of the relationship Identifier." ) 1220 protected Identifier identifier; 1221 1222 /** 1223 * Target of the relationship Display. 1224 */ 1225 @Child(name = "display", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1226 @Description(shortDefinition="Target of the relationship Display", formalDefinition="Target of the relationship Display." ) 1227 protected MarkdownType display; 1228 1229 /** 1230 * Target of the relationship Resource reference. 1231 */ 1232 @Child(name = "resource", type = {Reference.class}, order=4, min=0, max=1, modifier=false, summary=false) 1233 @Description(shortDefinition="Target of the relationship Resource reference", formalDefinition="Target of the relationship Resource reference." ) 1234 protected Reference resource; 1235 1236 private static final long serialVersionUID = -804526425L; 1237 1238 /** 1239 * Constructor 1240 */ 1241 public EvidenceReportRelatesToTargetComponent() { 1242 super(); 1243 } 1244 1245 /** 1246 * @return {@link #url} (Target of the relationship URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1247 */ 1248 public UriType getUrlElement() { 1249 if (this.url == null) 1250 if (Configuration.errorOnAutoCreate()) 1251 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.url"); 1252 else if (Configuration.doAutoCreate()) 1253 this.url = new UriType(); // bb 1254 return this.url; 1255 } 1256 1257 public boolean hasUrlElement() { 1258 return this.url != null && !this.url.isEmpty(); 1259 } 1260 1261 public boolean hasUrl() { 1262 return this.url != null && !this.url.isEmpty(); 1263 } 1264 1265 /** 1266 * @param value {@link #url} (Target of the relationship URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1267 */ 1268 public EvidenceReportRelatesToTargetComponent setUrlElement(UriType value) { 1269 this.url = value; 1270 return this; 1271 } 1272 1273 /** 1274 * @return Target of the relationship URL. 1275 */ 1276 public String getUrl() { 1277 return this.url == null ? null : this.url.getValue(); 1278 } 1279 1280 /** 1281 * @param value Target of the relationship URL. 1282 */ 1283 public EvidenceReportRelatesToTargetComponent setUrl(String value) { 1284 if (Utilities.noString(value)) 1285 this.url = null; 1286 else { 1287 if (this.url == null) 1288 this.url = new UriType(); 1289 this.url.setValue(value); 1290 } 1291 return this; 1292 } 1293 1294 /** 1295 * @return {@link #identifier} (Target of the relationship Identifier.) 1296 */ 1297 public Identifier getIdentifier() { 1298 if (this.identifier == null) 1299 if (Configuration.errorOnAutoCreate()) 1300 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.identifier"); 1301 else if (Configuration.doAutoCreate()) 1302 this.identifier = new Identifier(); // cc 1303 return this.identifier; 1304 } 1305 1306 public boolean hasIdentifier() { 1307 return this.identifier != null && !this.identifier.isEmpty(); 1308 } 1309 1310 /** 1311 * @param value {@link #identifier} (Target of the relationship Identifier.) 1312 */ 1313 public EvidenceReportRelatesToTargetComponent setIdentifier(Identifier value) { 1314 this.identifier = value; 1315 return this; 1316 } 1317 1318 /** 1319 * @return {@link #display} (Target of the relationship Display.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1320 */ 1321 public MarkdownType getDisplayElement() { 1322 if (this.display == null) 1323 if (Configuration.errorOnAutoCreate()) 1324 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.display"); 1325 else if (Configuration.doAutoCreate()) 1326 this.display = new MarkdownType(); // bb 1327 return this.display; 1328 } 1329 1330 public boolean hasDisplayElement() { 1331 return this.display != null && !this.display.isEmpty(); 1332 } 1333 1334 public boolean hasDisplay() { 1335 return this.display != null && !this.display.isEmpty(); 1336 } 1337 1338 /** 1339 * @param value {@link #display} (Target of the relationship Display.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1340 */ 1341 public EvidenceReportRelatesToTargetComponent setDisplayElement(MarkdownType value) { 1342 this.display = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return Target of the relationship Display. 1348 */ 1349 public String getDisplay() { 1350 return this.display == null ? null : this.display.getValue(); 1351 } 1352 1353 /** 1354 * @param value Target of the relationship Display. 1355 */ 1356 public EvidenceReportRelatesToTargetComponent setDisplay(String value) { 1357 if (Utilities.noString(value)) 1358 this.display = null; 1359 else { 1360 if (this.display == null) 1361 this.display = new MarkdownType(); 1362 this.display.setValue(value); 1363 } 1364 return this; 1365 } 1366 1367 /** 1368 * @return {@link #resource} (Target of the relationship Resource reference.) 1369 */ 1370 public Reference getResource() { 1371 if (this.resource == null) 1372 if (Configuration.errorOnAutoCreate()) 1373 throw new Error("Attempt to auto-create EvidenceReportRelatesToTargetComponent.resource"); 1374 else if (Configuration.doAutoCreate()) 1375 this.resource = new Reference(); // cc 1376 return this.resource; 1377 } 1378 1379 public boolean hasResource() { 1380 return this.resource != null && !this.resource.isEmpty(); 1381 } 1382 1383 /** 1384 * @param value {@link #resource} (Target of the relationship Resource reference.) 1385 */ 1386 public EvidenceReportRelatesToTargetComponent setResource(Reference value) { 1387 this.resource = value; 1388 return this; 1389 } 1390 1391 protected void listChildren(List<Property> children) { 1392 super.listChildren(children); 1393 children.add(new Property("url", "uri", "Target of the relationship URL.", 0, 1, url)); 1394 children.add(new Property("identifier", "Identifier", "Target of the relationship Identifier.", 0, 1, identifier)); 1395 children.add(new Property("display", "markdown", "Target of the relationship Display.", 0, 1, display)); 1396 children.add(new Property("resource", "Reference(Any)", "Target of the relationship Resource reference.", 0, 1, resource)); 1397 } 1398 1399 @Override 1400 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1401 switch (_hash) { 1402 case 116079: /*url*/ return new Property("url", "uri", "Target of the relationship URL.", 0, 1, url); 1403 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Target of the relationship Identifier.", 0, 1, identifier); 1404 case 1671764162: /*display*/ return new Property("display", "markdown", "Target of the relationship Display.", 0, 1, display); 1405 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "Target of the relationship Resource reference.", 0, 1, resource); 1406 default: return super.getNamedProperty(_hash, _name, _checkValid); 1407 } 1408 1409 } 1410 1411 @Override 1412 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1413 switch (hash) { 1414 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1415 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1416 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // MarkdownType 1417 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 1418 default: return super.getProperty(hash, name, checkValid); 1419 } 1420 1421 } 1422 1423 @Override 1424 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1425 switch (hash) { 1426 case 116079: // url 1427 this.url = TypeConvertor.castToUri(value); // UriType 1428 return value; 1429 case -1618432855: // identifier 1430 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1431 return value; 1432 case 1671764162: // display 1433 this.display = TypeConvertor.castToMarkdown(value); // MarkdownType 1434 return value; 1435 case -341064690: // resource 1436 this.resource = TypeConvertor.castToReference(value); // Reference 1437 return value; 1438 default: return super.setProperty(hash, name, value); 1439 } 1440 1441 } 1442 1443 @Override 1444 public Base setProperty(String name, Base value) throws FHIRException { 1445 if (name.equals("url")) { 1446 this.url = TypeConvertor.castToUri(value); // UriType 1447 } else if (name.equals("identifier")) { 1448 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1449 } else if (name.equals("display")) { 1450 this.display = TypeConvertor.castToMarkdown(value); // MarkdownType 1451 } else if (name.equals("resource")) { 1452 this.resource = TypeConvertor.castToReference(value); // Reference 1453 } else 1454 return super.setProperty(name, value); 1455 return value; 1456 } 1457 1458 @Override 1459 public void removeChild(String name, Base value) throws FHIRException { 1460 if (name.equals("url")) { 1461 this.url = null; 1462 } else if (name.equals("identifier")) { 1463 this.identifier = null; 1464 } else if (name.equals("display")) { 1465 this.display = null; 1466 } else if (name.equals("resource")) { 1467 this.resource = null; 1468 } else 1469 super.removeChild(name, value); 1470 1471 } 1472 1473 @Override 1474 public Base makeProperty(int hash, String name) throws FHIRException { 1475 switch (hash) { 1476 case 116079: return getUrlElement(); 1477 case -1618432855: return getIdentifier(); 1478 case 1671764162: return getDisplayElement(); 1479 case -341064690: return getResource(); 1480 default: return super.makeProperty(hash, name); 1481 } 1482 1483 } 1484 1485 @Override 1486 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1487 switch (hash) { 1488 case 116079: /*url*/ return new String[] {"uri"}; 1489 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1490 case 1671764162: /*display*/ return new String[] {"markdown"}; 1491 case -341064690: /*resource*/ return new String[] {"Reference"}; 1492 default: return super.getTypesForProperty(hash, name); 1493 } 1494 1495 } 1496 1497 @Override 1498 public Base addChild(String name) throws FHIRException { 1499 if (name.equals("url")) { 1500 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.relatesTo.target.url"); 1501 } 1502 else if (name.equals("identifier")) { 1503 this.identifier = new Identifier(); 1504 return this.identifier; 1505 } 1506 else if (name.equals("display")) { 1507 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.relatesTo.target.display"); 1508 } 1509 else if (name.equals("resource")) { 1510 this.resource = new Reference(); 1511 return this.resource; 1512 } 1513 else 1514 return super.addChild(name); 1515 } 1516 1517 public EvidenceReportRelatesToTargetComponent copy() { 1518 EvidenceReportRelatesToTargetComponent dst = new EvidenceReportRelatesToTargetComponent(); 1519 copyValues(dst); 1520 return dst; 1521 } 1522 1523 public void copyValues(EvidenceReportRelatesToTargetComponent dst) { 1524 super.copyValues(dst); 1525 dst.url = url == null ? null : url.copy(); 1526 dst.identifier = identifier == null ? null : identifier.copy(); 1527 dst.display = display == null ? null : display.copy(); 1528 dst.resource = resource == null ? null : resource.copy(); 1529 } 1530 1531 @Override 1532 public boolean equalsDeep(Base other_) { 1533 if (!super.equalsDeep(other_)) 1534 return false; 1535 if (!(other_ instanceof EvidenceReportRelatesToTargetComponent)) 1536 return false; 1537 EvidenceReportRelatesToTargetComponent o = (EvidenceReportRelatesToTargetComponent) other_; 1538 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(display, o.display, true) 1539 && compareDeep(resource, o.resource, true); 1540 } 1541 1542 @Override 1543 public boolean equalsShallow(Base other_) { 1544 if (!super.equalsShallow(other_)) 1545 return false; 1546 if (!(other_ instanceof EvidenceReportRelatesToTargetComponent)) 1547 return false; 1548 EvidenceReportRelatesToTargetComponent o = (EvidenceReportRelatesToTargetComponent) other_; 1549 return compareValues(url, o.url, true) && compareValues(display, o.display, true); 1550 } 1551 1552 public boolean isEmpty() { 1553 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, display 1554 , resource); 1555 } 1556 1557 public String fhirType() { 1558 return "EvidenceReport.relatesTo.target"; 1559 1560 } 1561 1562 } 1563 1564 @Block() 1565 public static class SectionComponent extends BackboneElement implements IBaseBackboneElement { 1566 /** 1567 * The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1568 */ 1569 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1570 @Description(shortDefinition="Label for section (e.g. for ToC)", formalDefinition="The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents." ) 1571 protected StringType title; 1572 1573 /** 1574 * A code identifying the kind of content contained within the section. This should be consistent with the section title. 1575 */ 1576 @Child(name = "focus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1577 @Description(shortDefinition="Classification of section (recommended)", formalDefinition="A code identifying the kind of content contained within the section. This should be consistent with the section title." ) 1578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-report-section") 1579 protected CodeableConcept focus; 1580 1581 /** 1582 * A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title. 1583 */ 1584 @Child(name = "focusReference", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 1585 @Description(shortDefinition="Classification of section by Resource", formalDefinition="A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title." ) 1586 protected Reference focusReference; 1587 1588 /** 1589 * Identifies who is responsible for the information in this section, not necessarily who typed it in. 1590 */ 1591 @Child(name = "author", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Device.class, Group.class, Organization.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1592 @Description(shortDefinition="Who and/or what authored the section", formalDefinition="Identifies who is responsible for the information in this section, not necessarily who typed it in." ) 1593 protected List<Reference> author; 1594 1595 /** 1596 * A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative. 1597 */ 1598 @Child(name = "text", type = {Narrative.class}, order=5, min=0, max=1, modifier=false, summary=false) 1599 @Description(shortDefinition="Text summary of the section, for human interpretation", formalDefinition="A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative." ) 1600 protected Narrative text; 1601 1602 /** 1603 * How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1604 */ 1605 @Child(name = "mode", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1606 @Description(shortDefinition="working | snapshot | changes", formalDefinition="How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted." ) 1607 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-mode") 1608 protected Enumeration<ListMode> mode; 1609 1610 /** 1611 * Specifies the order applied to the items in the section entries. 1612 */ 1613 @Child(name = "orderedBy", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1614 @Description(shortDefinition="Order of section entries", formalDefinition="Specifies the order applied to the items in the section entries." ) 1615 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-order") 1616 protected CodeableConcept orderedBy; 1617 1618 /** 1619 * Specifies any type of classification of the evidence report. 1620 */ 1621 @Child(name = "entryClassifier", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1622 @Description(shortDefinition="Extensible classifiers as content", formalDefinition="Specifies any type of classification of the evidence report." ) 1623 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-classifier-code") 1624 protected List<CodeableConcept> entryClassifier; 1625 1626 /** 1627 * A reference to the actual resource from which the narrative in the section is derived. 1628 */ 1629 @Child(name = "entryReference", type = {Reference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1630 @Description(shortDefinition="Reference to resources as content", formalDefinition="A reference to the actual resource from which the narrative in the section is derived." ) 1631 protected List<Reference> entryReference; 1632 1633 /** 1634 * Quantity as content. 1635 */ 1636 @Child(name = "entryQuantity", type = {Quantity.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1637 @Description(shortDefinition="Quantity as content", formalDefinition="Quantity as content." ) 1638 protected List<Quantity> entryQuantity; 1639 1640 /** 1641 * If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason. 1642 */ 1643 @Child(name = "emptyReason", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=false) 1644 @Description(shortDefinition="Why the section is empty", formalDefinition="If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason." ) 1645 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-empty-reason") 1646 protected CodeableConcept emptyReason; 1647 1648 /** 1649 * A nested sub-section within this section. 1650 */ 1651 @Child(name = "section", type = {SectionComponent.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1652 @Description(shortDefinition="Nested Section", formalDefinition="A nested sub-section within this section." ) 1653 protected List<SectionComponent> section; 1654 1655 private static final long serialVersionUID = -324854168L; 1656 1657 /** 1658 * Constructor 1659 */ 1660 public SectionComponent() { 1661 super(); 1662 } 1663 1664 /** 1665 * @return {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1666 */ 1667 public StringType getTitleElement() { 1668 if (this.title == null) 1669 if (Configuration.errorOnAutoCreate()) 1670 throw new Error("Attempt to auto-create SectionComponent.title"); 1671 else if (Configuration.doAutoCreate()) 1672 this.title = new StringType(); // bb 1673 return this.title; 1674 } 1675 1676 public boolean hasTitleElement() { 1677 return this.title != null && !this.title.isEmpty(); 1678 } 1679 1680 public boolean hasTitle() { 1681 return this.title != null && !this.title.isEmpty(); 1682 } 1683 1684 /** 1685 * @param value {@link #title} (The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1686 */ 1687 public SectionComponent setTitleElement(StringType value) { 1688 this.title = value; 1689 return this; 1690 } 1691 1692 /** 1693 * @return The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1694 */ 1695 public String getTitle() { 1696 return this.title == null ? null : this.title.getValue(); 1697 } 1698 1699 /** 1700 * @param value The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents. 1701 */ 1702 public SectionComponent setTitle(String value) { 1703 if (Utilities.noString(value)) 1704 this.title = null; 1705 else { 1706 if (this.title == null) 1707 this.title = new StringType(); 1708 this.title.setValue(value); 1709 } 1710 return this; 1711 } 1712 1713 /** 1714 * @return {@link #focus} (A code identifying the kind of content contained within the section. This should be consistent with the section title.) 1715 */ 1716 public CodeableConcept getFocus() { 1717 if (this.focus == null) 1718 if (Configuration.errorOnAutoCreate()) 1719 throw new Error("Attempt to auto-create SectionComponent.focus"); 1720 else if (Configuration.doAutoCreate()) 1721 this.focus = new CodeableConcept(); // cc 1722 return this.focus; 1723 } 1724 1725 public boolean hasFocus() { 1726 return this.focus != null && !this.focus.isEmpty(); 1727 } 1728 1729 /** 1730 * @param value {@link #focus} (A code identifying the kind of content contained within the section. This should be consistent with the section title.) 1731 */ 1732 public SectionComponent setFocus(CodeableConcept value) { 1733 this.focus = value; 1734 return this; 1735 } 1736 1737 /** 1738 * @return {@link #focusReference} (A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.) 1739 */ 1740 public Reference getFocusReference() { 1741 if (this.focusReference == null) 1742 if (Configuration.errorOnAutoCreate()) 1743 throw new Error("Attempt to auto-create SectionComponent.focusReference"); 1744 else if (Configuration.doAutoCreate()) 1745 this.focusReference = new Reference(); // cc 1746 return this.focusReference; 1747 } 1748 1749 public boolean hasFocusReference() { 1750 return this.focusReference != null && !this.focusReference.isEmpty(); 1751 } 1752 1753 /** 1754 * @param value {@link #focusReference} (A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.) 1755 */ 1756 public SectionComponent setFocusReference(Reference value) { 1757 this.focusReference = value; 1758 return this; 1759 } 1760 1761 /** 1762 * @return {@link #author} (Identifies who is responsible for the information in this section, not necessarily who typed it in.) 1763 */ 1764 public List<Reference> getAuthor() { 1765 if (this.author == null) 1766 this.author = new ArrayList<Reference>(); 1767 return this.author; 1768 } 1769 1770 /** 1771 * @return Returns a reference to <code>this</code> for easy method chaining 1772 */ 1773 public SectionComponent setAuthor(List<Reference> theAuthor) { 1774 this.author = theAuthor; 1775 return this; 1776 } 1777 1778 public boolean hasAuthor() { 1779 if (this.author == null) 1780 return false; 1781 for (Reference item : this.author) 1782 if (!item.isEmpty()) 1783 return true; 1784 return false; 1785 } 1786 1787 public Reference addAuthor() { //3 1788 Reference t = new Reference(); 1789 if (this.author == null) 1790 this.author = new ArrayList<Reference>(); 1791 this.author.add(t); 1792 return t; 1793 } 1794 1795 public SectionComponent addAuthor(Reference t) { //3 1796 if (t == null) 1797 return this; 1798 if (this.author == null) 1799 this.author = new ArrayList<Reference>(); 1800 this.author.add(t); 1801 return this; 1802 } 1803 1804 /** 1805 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 1806 */ 1807 public Reference getAuthorFirstRep() { 1808 if (getAuthor().isEmpty()) { 1809 addAuthor(); 1810 } 1811 return getAuthor().get(0); 1812 } 1813 1814 /** 1815 * @return {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.) 1816 */ 1817 public Narrative getText() { 1818 if (this.text == null) 1819 if (Configuration.errorOnAutoCreate()) 1820 throw new Error("Attempt to auto-create SectionComponent.text"); 1821 else if (Configuration.doAutoCreate()) 1822 this.text = new Narrative(); // cc 1823 return this.text; 1824 } 1825 1826 public boolean hasText() { 1827 return this.text != null && !this.text.isEmpty(); 1828 } 1829 1830 /** 1831 * @param value {@link #text} (A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.) 1832 */ 1833 public SectionComponent setText(Narrative value) { 1834 this.text = value; 1835 return this; 1836 } 1837 1838 /** 1839 * @return {@link #mode} (How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1840 */ 1841 public Enumeration<ListMode> getModeElement() { 1842 if (this.mode == null) 1843 if (Configuration.errorOnAutoCreate()) 1844 throw new Error("Attempt to auto-create SectionComponent.mode"); 1845 else if (Configuration.doAutoCreate()) 1846 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); // bb 1847 return this.mode; 1848 } 1849 1850 public boolean hasModeElement() { 1851 return this.mode != null && !this.mode.isEmpty(); 1852 } 1853 1854 public boolean hasMode() { 1855 return this.mode != null && !this.mode.isEmpty(); 1856 } 1857 1858 /** 1859 * @param value {@link #mode} (How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1860 */ 1861 public SectionComponent setModeElement(Enumeration<ListMode> value) { 1862 this.mode = value; 1863 return this; 1864 } 1865 1866 /** 1867 * @return How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1868 */ 1869 public ListMode getMode() { 1870 return this.mode == null ? null : this.mode.getValue(); 1871 } 1872 1873 /** 1874 * @param value How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 1875 */ 1876 public SectionComponent setMode(ListMode value) { 1877 if (value == null) 1878 this.mode = null; 1879 else { 1880 if (this.mode == null) 1881 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); 1882 this.mode.setValue(value); 1883 } 1884 return this; 1885 } 1886 1887 /** 1888 * @return {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 1889 */ 1890 public CodeableConcept getOrderedBy() { 1891 if (this.orderedBy == null) 1892 if (Configuration.errorOnAutoCreate()) 1893 throw new Error("Attempt to auto-create SectionComponent.orderedBy"); 1894 else if (Configuration.doAutoCreate()) 1895 this.orderedBy = new CodeableConcept(); // cc 1896 return this.orderedBy; 1897 } 1898 1899 public boolean hasOrderedBy() { 1900 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1901 } 1902 1903 /** 1904 * @param value {@link #orderedBy} (Specifies the order applied to the items in the section entries.) 1905 */ 1906 public SectionComponent setOrderedBy(CodeableConcept value) { 1907 this.orderedBy = value; 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #entryClassifier} (Specifies any type of classification of the evidence report.) 1913 */ 1914 public List<CodeableConcept> getEntryClassifier() { 1915 if (this.entryClassifier == null) 1916 this.entryClassifier = new ArrayList<CodeableConcept>(); 1917 return this.entryClassifier; 1918 } 1919 1920 /** 1921 * @return Returns a reference to <code>this</code> for easy method chaining 1922 */ 1923 public SectionComponent setEntryClassifier(List<CodeableConcept> theEntryClassifier) { 1924 this.entryClassifier = theEntryClassifier; 1925 return this; 1926 } 1927 1928 public boolean hasEntryClassifier() { 1929 if (this.entryClassifier == null) 1930 return false; 1931 for (CodeableConcept item : this.entryClassifier) 1932 if (!item.isEmpty()) 1933 return true; 1934 return false; 1935 } 1936 1937 public CodeableConcept addEntryClassifier() { //3 1938 CodeableConcept t = new CodeableConcept(); 1939 if (this.entryClassifier == null) 1940 this.entryClassifier = new ArrayList<CodeableConcept>(); 1941 this.entryClassifier.add(t); 1942 return t; 1943 } 1944 1945 public SectionComponent addEntryClassifier(CodeableConcept t) { //3 1946 if (t == null) 1947 return this; 1948 if (this.entryClassifier == null) 1949 this.entryClassifier = new ArrayList<CodeableConcept>(); 1950 this.entryClassifier.add(t); 1951 return this; 1952 } 1953 1954 /** 1955 * @return The first repetition of repeating field {@link #entryClassifier}, creating it if it does not already exist {3} 1956 */ 1957 public CodeableConcept getEntryClassifierFirstRep() { 1958 if (getEntryClassifier().isEmpty()) { 1959 addEntryClassifier(); 1960 } 1961 return getEntryClassifier().get(0); 1962 } 1963 1964 /** 1965 * @return {@link #entryReference} (A reference to the actual resource from which the narrative in the section is derived.) 1966 */ 1967 public List<Reference> getEntryReference() { 1968 if (this.entryReference == null) 1969 this.entryReference = new ArrayList<Reference>(); 1970 return this.entryReference; 1971 } 1972 1973 /** 1974 * @return Returns a reference to <code>this</code> for easy method chaining 1975 */ 1976 public SectionComponent setEntryReference(List<Reference> theEntryReference) { 1977 this.entryReference = theEntryReference; 1978 return this; 1979 } 1980 1981 public boolean hasEntryReference() { 1982 if (this.entryReference == null) 1983 return false; 1984 for (Reference item : this.entryReference) 1985 if (!item.isEmpty()) 1986 return true; 1987 return false; 1988 } 1989 1990 public Reference addEntryReference() { //3 1991 Reference t = new Reference(); 1992 if (this.entryReference == null) 1993 this.entryReference = new ArrayList<Reference>(); 1994 this.entryReference.add(t); 1995 return t; 1996 } 1997 1998 public SectionComponent addEntryReference(Reference t) { //3 1999 if (t == null) 2000 return this; 2001 if (this.entryReference == null) 2002 this.entryReference = new ArrayList<Reference>(); 2003 this.entryReference.add(t); 2004 return this; 2005 } 2006 2007 /** 2008 * @return The first repetition of repeating field {@link #entryReference}, creating it if it does not already exist {3} 2009 */ 2010 public Reference getEntryReferenceFirstRep() { 2011 if (getEntryReference().isEmpty()) { 2012 addEntryReference(); 2013 } 2014 return getEntryReference().get(0); 2015 } 2016 2017 /** 2018 * @return {@link #entryQuantity} (Quantity as content.) 2019 */ 2020 public List<Quantity> getEntryQuantity() { 2021 if (this.entryQuantity == null) 2022 this.entryQuantity = new ArrayList<Quantity>(); 2023 return this.entryQuantity; 2024 } 2025 2026 /** 2027 * @return Returns a reference to <code>this</code> for easy method chaining 2028 */ 2029 public SectionComponent setEntryQuantity(List<Quantity> theEntryQuantity) { 2030 this.entryQuantity = theEntryQuantity; 2031 return this; 2032 } 2033 2034 public boolean hasEntryQuantity() { 2035 if (this.entryQuantity == null) 2036 return false; 2037 for (Quantity item : this.entryQuantity) 2038 if (!item.isEmpty()) 2039 return true; 2040 return false; 2041 } 2042 2043 public Quantity addEntryQuantity() { //3 2044 Quantity t = new Quantity(); 2045 if (this.entryQuantity == null) 2046 this.entryQuantity = new ArrayList<Quantity>(); 2047 this.entryQuantity.add(t); 2048 return t; 2049 } 2050 2051 public SectionComponent addEntryQuantity(Quantity t) { //3 2052 if (t == null) 2053 return this; 2054 if (this.entryQuantity == null) 2055 this.entryQuantity = new ArrayList<Quantity>(); 2056 this.entryQuantity.add(t); 2057 return this; 2058 } 2059 2060 /** 2061 * @return The first repetition of repeating field {@link #entryQuantity}, creating it if it does not already exist {3} 2062 */ 2063 public Quantity getEntryQuantityFirstRep() { 2064 if (getEntryQuantity().isEmpty()) { 2065 addEntryQuantity(); 2066 } 2067 return getEntryQuantity().get(0); 2068 } 2069 2070 /** 2071 * @return {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 2072 */ 2073 public CodeableConcept getEmptyReason() { 2074 if (this.emptyReason == null) 2075 if (Configuration.errorOnAutoCreate()) 2076 throw new Error("Attempt to auto-create SectionComponent.emptyReason"); 2077 else if (Configuration.doAutoCreate()) 2078 this.emptyReason = new CodeableConcept(); // cc 2079 return this.emptyReason; 2080 } 2081 2082 public boolean hasEmptyReason() { 2083 return this.emptyReason != null && !this.emptyReason.isEmpty(); 2084 } 2085 2086 /** 2087 * @param value {@link #emptyReason} (If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.) 2088 */ 2089 public SectionComponent setEmptyReason(CodeableConcept value) { 2090 this.emptyReason = value; 2091 return this; 2092 } 2093 2094 /** 2095 * @return {@link #section} (A nested sub-section within this section.) 2096 */ 2097 public List<SectionComponent> getSection() { 2098 if (this.section == null) 2099 this.section = new ArrayList<SectionComponent>(); 2100 return this.section; 2101 } 2102 2103 /** 2104 * @return Returns a reference to <code>this</code> for easy method chaining 2105 */ 2106 public SectionComponent setSection(List<SectionComponent> theSection) { 2107 this.section = theSection; 2108 return this; 2109 } 2110 2111 public boolean hasSection() { 2112 if (this.section == null) 2113 return false; 2114 for (SectionComponent item : this.section) 2115 if (!item.isEmpty()) 2116 return true; 2117 return false; 2118 } 2119 2120 public SectionComponent addSection() { //3 2121 SectionComponent t = new SectionComponent(); 2122 if (this.section == null) 2123 this.section = new ArrayList<SectionComponent>(); 2124 this.section.add(t); 2125 return t; 2126 } 2127 2128 public SectionComponent addSection(SectionComponent t) { //3 2129 if (t == null) 2130 return this; 2131 if (this.section == null) 2132 this.section = new ArrayList<SectionComponent>(); 2133 this.section.add(t); 2134 return this; 2135 } 2136 2137 /** 2138 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist {3} 2139 */ 2140 public SectionComponent getSectionFirstRep() { 2141 if (getSection().isEmpty()) { 2142 addSection(); 2143 } 2144 return getSection().get(0); 2145 } 2146 2147 protected void listChildren(List<Property> children) { 2148 super.listChildren(children); 2149 children.add(new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title)); 2150 children.add(new Property("focus", "CodeableConcept", "A code identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focus)); 2151 children.add(new Property("focusReference", "Reference(Any)", "A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focusReference)); 2152 children.add(new Property("author", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|Group|Organization)", "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author)); 2153 children.add(new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.", 0, 1, text)); 2154 children.add(new Property("mode", "code", "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode)); 2155 children.add(new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy)); 2156 children.add(new Property("entryClassifier", "CodeableConcept", "Specifies any type of classification of the evidence report.", 0, java.lang.Integer.MAX_VALUE, entryClassifier)); 2157 children.add(new Property("entryReference", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entryReference)); 2158 children.add(new Property("entryQuantity", "Quantity", "Quantity as content.", 0, java.lang.Integer.MAX_VALUE, entryQuantity)); 2159 children.add(new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason)); 2160 children.add(new Property("section", "@EvidenceReport.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section)); 2161 } 2162 2163 @Override 2164 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2165 switch (_hash) { 2166 case 110371416: /*title*/ return new Property("title", "string", "The label for this particular section. This will be part of the rendered content for the document, and is often used to build a table of contents.", 0, 1, title); 2167 case 97604824: /*focus*/ return new Property("focus", "CodeableConcept", "A code identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focus); 2168 case 1823604051: /*focusReference*/ return new Property("focusReference", "Reference(Any)", "A definitional Resource identifying the kind of content contained within the section. This should be consistent with the section title.", 0, 1, focusReference); 2169 case -1406328437: /*author*/ return new Property("author", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|Group|Organization)", "Identifies who is responsible for the information in this section, not necessarily who typed it in.", 0, java.lang.Integer.MAX_VALUE, author); 2170 case 3556653: /*text*/ return new Property("text", "Narrative", "A human-readable narrative that contains the attested content of the section, used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is peferred to contain sufficient detail to make it acceptable for a human to just read the narrative.", 0, 1, text); 2171 case 3357091: /*mode*/ return new Property("mode", "code", "How the entry list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode); 2172 case -391079516: /*orderedBy*/ return new Property("orderedBy", "CodeableConcept", "Specifies the order applied to the items in the section entries.", 0, 1, orderedBy); 2173 case -948201421: /*entryClassifier*/ return new Property("entryClassifier", "CodeableConcept", "Specifies any type of classification of the evidence report.", 0, java.lang.Integer.MAX_VALUE, entryClassifier); 2174 case 438810361: /*entryReference*/ return new Property("entryReference", "Reference(Any)", "A reference to the actual resource from which the narrative in the section is derived.", 0, java.lang.Integer.MAX_VALUE, entryReference); 2175 case 1945583389: /*entryQuantity*/ return new Property("entryQuantity", "Quantity", "Quantity as content.", 0, java.lang.Integer.MAX_VALUE, entryQuantity); 2176 case 1140135409: /*emptyReason*/ return new Property("emptyReason", "CodeableConcept", "If the section is empty, why the list is empty. An empty section typically has some text explaining the empty reason.", 0, 1, emptyReason); 2177 case 1970241253: /*section*/ return new Property("section", "@EvidenceReport.section", "A nested sub-section within this section.", 0, java.lang.Integer.MAX_VALUE, section); 2178 default: return super.getNamedProperty(_hash, _name, _checkValid); 2179 } 2180 2181 } 2182 2183 @Override 2184 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2185 switch (hash) { 2186 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2187 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // CodeableConcept 2188 case 1823604051: /*focusReference*/ return this.focusReference == null ? new Base[0] : new Base[] {this.focusReference}; // Reference 2189 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 2190 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // Narrative 2191 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<ListMode> 2192 case -391079516: /*orderedBy*/ return this.orderedBy == null ? new Base[0] : new Base[] {this.orderedBy}; // CodeableConcept 2193 case -948201421: /*entryClassifier*/ return this.entryClassifier == null ? new Base[0] : this.entryClassifier.toArray(new Base[this.entryClassifier.size()]); // CodeableConcept 2194 case 438810361: /*entryReference*/ return this.entryReference == null ? new Base[0] : this.entryReference.toArray(new Base[this.entryReference.size()]); // Reference 2195 case 1945583389: /*entryQuantity*/ return this.entryQuantity == null ? new Base[0] : this.entryQuantity.toArray(new Base[this.entryQuantity.size()]); // Quantity 2196 case 1140135409: /*emptyReason*/ return this.emptyReason == null ? new Base[0] : new Base[] {this.emptyReason}; // CodeableConcept 2197 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 2198 default: return super.getProperty(hash, name, checkValid); 2199 } 2200 2201 } 2202 2203 @Override 2204 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2205 switch (hash) { 2206 case 110371416: // title 2207 this.title = TypeConvertor.castToString(value); // StringType 2208 return value; 2209 case 97604824: // focus 2210 this.focus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2211 return value; 2212 case 1823604051: // focusReference 2213 this.focusReference = TypeConvertor.castToReference(value); // Reference 2214 return value; 2215 case -1406328437: // author 2216 this.getAuthor().add(TypeConvertor.castToReference(value)); // Reference 2217 return value; 2218 case 3556653: // text 2219 this.text = TypeConvertor.castToNarrative(value); // Narrative 2220 return value; 2221 case 3357091: // mode 2222 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2223 this.mode = (Enumeration) value; // Enumeration<ListMode> 2224 return value; 2225 case -391079516: // orderedBy 2226 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2227 return value; 2228 case -948201421: // entryClassifier 2229 this.getEntryClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2230 return value; 2231 case 438810361: // entryReference 2232 this.getEntryReference().add(TypeConvertor.castToReference(value)); // Reference 2233 return value; 2234 case 1945583389: // entryQuantity 2235 this.getEntryQuantity().add(TypeConvertor.castToQuantity(value)); // Quantity 2236 return value; 2237 case 1140135409: // emptyReason 2238 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2239 return value; 2240 case 1970241253: // section 2241 this.getSection().add((SectionComponent) value); // SectionComponent 2242 return value; 2243 default: return super.setProperty(hash, name, value); 2244 } 2245 2246 } 2247 2248 @Override 2249 public Base setProperty(String name, Base value) throws FHIRException { 2250 if (name.equals("title")) { 2251 this.title = TypeConvertor.castToString(value); // StringType 2252 } else if (name.equals("focus")) { 2253 this.focus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2254 } else if (name.equals("focusReference")) { 2255 this.focusReference = TypeConvertor.castToReference(value); // Reference 2256 } else if (name.equals("author")) { 2257 this.getAuthor().add(TypeConvertor.castToReference(value)); 2258 } else if (name.equals("text")) { 2259 this.text = TypeConvertor.castToNarrative(value); // Narrative 2260 } else if (name.equals("mode")) { 2261 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2262 this.mode = (Enumeration) value; // Enumeration<ListMode> 2263 } else if (name.equals("orderedBy")) { 2264 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2265 } else if (name.equals("entryClassifier")) { 2266 this.getEntryClassifier().add(TypeConvertor.castToCodeableConcept(value)); 2267 } else if (name.equals("entryReference")) { 2268 this.getEntryReference().add(TypeConvertor.castToReference(value)); 2269 } else if (name.equals("entryQuantity")) { 2270 this.getEntryQuantity().add(TypeConvertor.castToQuantity(value)); 2271 } else if (name.equals("emptyReason")) { 2272 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2273 } else if (name.equals("section")) { 2274 this.getSection().add((SectionComponent) value); 2275 } else 2276 return super.setProperty(name, value); 2277 return value; 2278 } 2279 2280 @Override 2281 public void removeChild(String name, Base value) throws FHIRException { 2282 if (name.equals("title")) { 2283 this.title = null; 2284 } else if (name.equals("focus")) { 2285 this.focus = null; 2286 } else if (name.equals("focusReference")) { 2287 this.focusReference = null; 2288 } else if (name.equals("author")) { 2289 this.getAuthor().remove(value); 2290 } else if (name.equals("text")) { 2291 this.text = null; 2292 } else if (name.equals("mode")) { 2293 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2294 this.mode = (Enumeration) value; // Enumeration<ListMode> 2295 } else if (name.equals("orderedBy")) { 2296 this.orderedBy = null; 2297 } else if (name.equals("entryClassifier")) { 2298 this.getEntryClassifier().remove(value); 2299 } else if (name.equals("entryReference")) { 2300 this.getEntryReference().remove(value); 2301 } else if (name.equals("entryQuantity")) { 2302 this.getEntryQuantity().remove(value); 2303 } else if (name.equals("emptyReason")) { 2304 this.emptyReason = null; 2305 } else if (name.equals("section")) { 2306 this.getSection().remove((SectionComponent) value); 2307 } else 2308 super.removeChild(name, value); 2309 2310 } 2311 2312 @Override 2313 public Base makeProperty(int hash, String name) throws FHIRException { 2314 switch (hash) { 2315 case 110371416: return getTitleElement(); 2316 case 97604824: return getFocus(); 2317 case 1823604051: return getFocusReference(); 2318 case -1406328437: return addAuthor(); 2319 case 3556653: return getText(); 2320 case 3357091: return getModeElement(); 2321 case -391079516: return getOrderedBy(); 2322 case -948201421: return addEntryClassifier(); 2323 case 438810361: return addEntryReference(); 2324 case 1945583389: return addEntryQuantity(); 2325 case 1140135409: return getEmptyReason(); 2326 case 1970241253: return addSection(); 2327 default: return super.makeProperty(hash, name); 2328 } 2329 2330 } 2331 2332 @Override 2333 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2334 switch (hash) { 2335 case 110371416: /*title*/ return new String[] {"string"}; 2336 case 97604824: /*focus*/ return new String[] {"CodeableConcept"}; 2337 case 1823604051: /*focusReference*/ return new String[] {"Reference"}; 2338 case -1406328437: /*author*/ return new String[] {"Reference"}; 2339 case 3556653: /*text*/ return new String[] {"Narrative"}; 2340 case 3357091: /*mode*/ return new String[] {"code"}; 2341 case -391079516: /*orderedBy*/ return new String[] {"CodeableConcept"}; 2342 case -948201421: /*entryClassifier*/ return new String[] {"CodeableConcept"}; 2343 case 438810361: /*entryReference*/ return new String[] {"Reference"}; 2344 case 1945583389: /*entryQuantity*/ return new String[] {"Quantity"}; 2345 case 1140135409: /*emptyReason*/ return new String[] {"CodeableConcept"}; 2346 case 1970241253: /*section*/ return new String[] {"@EvidenceReport.section"}; 2347 default: return super.getTypesForProperty(hash, name); 2348 } 2349 2350 } 2351 2352 @Override 2353 public Base addChild(String name) throws FHIRException { 2354 if (name.equals("title")) { 2355 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.section.title"); 2356 } 2357 else if (name.equals("focus")) { 2358 this.focus = new CodeableConcept(); 2359 return this.focus; 2360 } 2361 else if (name.equals("focusReference")) { 2362 this.focusReference = new Reference(); 2363 return this.focusReference; 2364 } 2365 else if (name.equals("author")) { 2366 return addAuthor(); 2367 } 2368 else if (name.equals("text")) { 2369 this.text = new Narrative(); 2370 return this.text; 2371 } 2372 else if (name.equals("mode")) { 2373 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.section.mode"); 2374 } 2375 else if (name.equals("orderedBy")) { 2376 this.orderedBy = new CodeableConcept(); 2377 return this.orderedBy; 2378 } 2379 else if (name.equals("entryClassifier")) { 2380 return addEntryClassifier(); 2381 } 2382 else if (name.equals("entryReference")) { 2383 return addEntryReference(); 2384 } 2385 else if (name.equals("entryQuantity")) { 2386 return addEntryQuantity(); 2387 } 2388 else if (name.equals("emptyReason")) { 2389 this.emptyReason = new CodeableConcept(); 2390 return this.emptyReason; 2391 } 2392 else if (name.equals("section")) { 2393 return addSection(); 2394 } 2395 else 2396 return super.addChild(name); 2397 } 2398 2399 public SectionComponent copy() { 2400 SectionComponent dst = new SectionComponent(); 2401 copyValues(dst); 2402 return dst; 2403 } 2404 2405 public void copyValues(SectionComponent dst) { 2406 super.copyValues(dst); 2407 dst.title = title == null ? null : title.copy(); 2408 dst.focus = focus == null ? null : focus.copy(); 2409 dst.focusReference = focusReference == null ? null : focusReference.copy(); 2410 if (author != null) { 2411 dst.author = new ArrayList<Reference>(); 2412 for (Reference i : author) 2413 dst.author.add(i.copy()); 2414 }; 2415 dst.text = text == null ? null : text.copy(); 2416 dst.mode = mode == null ? null : mode.copy(); 2417 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 2418 if (entryClassifier != null) { 2419 dst.entryClassifier = new ArrayList<CodeableConcept>(); 2420 for (CodeableConcept i : entryClassifier) 2421 dst.entryClassifier.add(i.copy()); 2422 }; 2423 if (entryReference != null) { 2424 dst.entryReference = new ArrayList<Reference>(); 2425 for (Reference i : entryReference) 2426 dst.entryReference.add(i.copy()); 2427 }; 2428 if (entryQuantity != null) { 2429 dst.entryQuantity = new ArrayList<Quantity>(); 2430 for (Quantity i : entryQuantity) 2431 dst.entryQuantity.add(i.copy()); 2432 }; 2433 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 2434 if (section != null) { 2435 dst.section = new ArrayList<SectionComponent>(); 2436 for (SectionComponent i : section) 2437 dst.section.add(i.copy()); 2438 }; 2439 } 2440 2441 @Override 2442 public boolean equalsDeep(Base other_) { 2443 if (!super.equalsDeep(other_)) 2444 return false; 2445 if (!(other_ instanceof SectionComponent)) 2446 return false; 2447 SectionComponent o = (SectionComponent) other_; 2448 return compareDeep(title, o.title, true) && compareDeep(focus, o.focus, true) && compareDeep(focusReference, o.focusReference, true) 2449 && compareDeep(author, o.author, true) && compareDeep(text, o.text, true) && compareDeep(mode, o.mode, true) 2450 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(entryClassifier, o.entryClassifier, true) 2451 && compareDeep(entryReference, o.entryReference, true) && compareDeep(entryQuantity, o.entryQuantity, true) 2452 && compareDeep(emptyReason, o.emptyReason, true) && compareDeep(section, o.section, true); 2453 } 2454 2455 @Override 2456 public boolean equalsShallow(Base other_) { 2457 if (!super.equalsShallow(other_)) 2458 return false; 2459 if (!(other_ instanceof SectionComponent)) 2460 return false; 2461 SectionComponent o = (SectionComponent) other_; 2462 return compareValues(title, o.title, true) && compareValues(mode, o.mode, true); 2463 } 2464 2465 public boolean isEmpty() { 2466 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, focus, focusReference 2467 , author, text, mode, orderedBy, entryClassifier, entryReference, entryQuantity 2468 , emptyReason, section); 2469 } 2470 2471 public String fhirType() { 2472 return "EvidenceReport.section"; 2473 2474 } 2475 2476 } 2477 2478 /** 2479 * An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 2480 */ 2481 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2482 @Description(shortDefinition="Canonical identifier for this EvidenceReport, represented as a globally unique URI", formalDefinition="An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers." ) 2483 protected UriType url; 2484 2485 /** 2486 * The status of this summary. Enables tracking the life-cycle of the content. 2487 */ 2488 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2489 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this summary. Enables tracking the life-cycle of the content." ) 2490 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2491 protected Enumeration<PublicationStatus> status; 2492 2493 /** 2494 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances. 2495 */ 2496 @Child(name = "useContext", type = {UsageContext.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2497 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances." ) 2498 protected List<UsageContext> useContext; 2499 2500 /** 2501 * A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance. 2502 */ 2503 @Child(name = "identifier", type = {Identifier.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2504 @Description(shortDefinition="Unique identifier for the evidence report", formalDefinition="A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2505 protected List<Identifier> identifier; 2506 2507 /** 2508 * A formal identifier that is used to identify things closely related to this EvidenceReport. 2509 */ 2510 @Child(name = "relatedIdentifier", type = {Identifier.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2511 @Description(shortDefinition="Identifiers for articles that may relate to more than one evidence report", formalDefinition="A formal identifier that is used to identify things closely related to this EvidenceReport." ) 2512 protected List<Identifier> relatedIdentifier; 2513 2514 /** 2515 * Citation Resource or display of suggested citation for this report. 2516 */ 2517 @Child(name = "citeAs", type = {Citation.class, MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2518 @Description(shortDefinition="Citation for this report", formalDefinition="Citation Resource or display of suggested citation for this report." ) 2519 protected DataType citeAs; 2520 2521 /** 2522 * Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression. 2523 */ 2524 @Child(name = "type", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 2525 @Description(shortDefinition="Kind of report", formalDefinition="Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression." ) 2526 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-report-type") 2527 protected CodeableConcept type; 2528 2529 /** 2530 * Used for footnotes and annotations. 2531 */ 2532 @Child(name = "note", type = {Annotation.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2533 @Description(shortDefinition="Used for footnotes and annotations", formalDefinition="Used for footnotes and annotations." ) 2534 protected List<Annotation> note; 2535 2536 /** 2537 * Link, description or reference to artifact associated with the report. 2538 */ 2539 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2540 @Description(shortDefinition="Link, description or reference to artifact associated with the report", formalDefinition="Link, description or reference to artifact associated with the report." ) 2541 protected List<RelatedArtifact> relatedArtifact; 2542 2543 /** 2544 * Specifies the subject or focus of the report. Answers "What is this report about?". 2545 */ 2546 @Child(name = "subject", type = {}, order=9, min=1, max=1, modifier=false, summary=true) 2547 @Description(shortDefinition="Focus of the report", formalDefinition="Specifies the subject or focus of the report. Answers \"What is this report about?\"." ) 2548 protected EvidenceReportSubjectComponent subject; 2549 2550 /** 2551 * The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report. 2552 */ 2553 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 2554 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report." ) 2555 protected StringType publisher; 2556 2557 /** 2558 * Contact details to assist a user in finding and communicating with the publisher. 2559 */ 2560 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2561 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2562 protected List<ContactDetail> contact; 2563 2564 /** 2565 * An individiual, organization, or device primarily involved in the creation and maintenance of the content. 2566 */ 2567 @Child(name = "author", type = {ContactDetail.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2568 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual, organization, or device primarily involved in the creation and maintenance of the content." ) 2569 protected List<ContactDetail> author; 2570 2571 /** 2572 * An individiual, organization, or device primarily responsible for internal coherence of the content. 2573 */ 2574 @Child(name = "editor", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2575 @Description(shortDefinition="Who edited the content", formalDefinition="An individiual, organization, or device primarily responsible for internal coherence of the content." ) 2576 protected List<ContactDetail> editor; 2577 2578 /** 2579 * An individiual, organization, or device primarily responsible for review of some aspect of the content. 2580 */ 2581 @Child(name = "reviewer", type = {ContactDetail.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2582 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individiual, organization, or device primarily responsible for review of some aspect of the content." ) 2583 protected List<ContactDetail> reviewer; 2584 2585 /** 2586 * An individiual, organization, or device responsible for officially endorsing the content for use in some setting. 2587 */ 2588 @Child(name = "endorser", type = {ContactDetail.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2589 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individiual, organization, or device responsible for officially endorsing the content for use in some setting." ) 2590 protected List<ContactDetail> endorser; 2591 2592 /** 2593 * Relationships that this composition has with other compositions or documents that already exist. 2594 */ 2595 @Child(name = "relatesTo", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2596 @Description(shortDefinition="Relationships to other compositions/documents", formalDefinition="Relationships that this composition has with other compositions or documents that already exist." ) 2597 protected List<EvidenceReportRelatesToComponent> relatesTo; 2598 2599 /** 2600 * The root of the sections that make up the composition. 2601 */ 2602 @Child(name = "section", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2603 @Description(shortDefinition="Composition is broken into sections", formalDefinition="The root of the sections that make up the composition." ) 2604 protected List<SectionComponent> section; 2605 2606 private static final long serialVersionUID = -1087028792L; 2607 2608 /** 2609 * Constructor 2610 */ 2611 public EvidenceReport() { 2612 super(); 2613 } 2614 2615 /** 2616 * Constructor 2617 */ 2618 public EvidenceReport(PublicationStatus status, EvidenceReportSubjectComponent subject) { 2619 super(); 2620 this.setStatus(status); 2621 this.setSubject(subject); 2622 } 2623 2624 /** 2625 * @return {@link #url} (An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2626 */ 2627 public UriType getUrlElement() { 2628 if (this.url == null) 2629 if (Configuration.errorOnAutoCreate()) 2630 throw new Error("Attempt to auto-create EvidenceReport.url"); 2631 else if (Configuration.doAutoCreate()) 2632 this.url = new UriType(); // bb 2633 return this.url; 2634 } 2635 2636 public boolean hasUrlElement() { 2637 return this.url != null && !this.url.isEmpty(); 2638 } 2639 2640 public boolean hasUrl() { 2641 return this.url != null && !this.url.isEmpty(); 2642 } 2643 2644 /** 2645 * @param value {@link #url} (An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2646 */ 2647 public EvidenceReport setUrlElement(UriType value) { 2648 this.url = value; 2649 return this; 2650 } 2651 2652 /** 2653 * @return An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 2654 */ 2655 public String getUrl() { 2656 return this.url == null ? null : this.url.getValue(); 2657 } 2658 2659 /** 2660 * @param value An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers. 2661 */ 2662 public EvidenceReport setUrl(String value) { 2663 if (Utilities.noString(value)) 2664 this.url = null; 2665 else { 2666 if (this.url == null) 2667 this.url = new UriType(); 2668 this.url.setValue(value); 2669 } 2670 return this; 2671 } 2672 2673 /** 2674 * @return {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2675 */ 2676 public Enumeration<PublicationStatus> getStatusElement() { 2677 if (this.status == null) 2678 if (Configuration.errorOnAutoCreate()) 2679 throw new Error("Attempt to auto-create EvidenceReport.status"); 2680 else if (Configuration.doAutoCreate()) 2681 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2682 return this.status; 2683 } 2684 2685 public boolean hasStatusElement() { 2686 return this.status != null && !this.status.isEmpty(); 2687 } 2688 2689 public boolean hasStatus() { 2690 return this.status != null && !this.status.isEmpty(); 2691 } 2692 2693 /** 2694 * @param value {@link #status} (The status of this summary. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2695 */ 2696 public EvidenceReport setStatusElement(Enumeration<PublicationStatus> value) { 2697 this.status = value; 2698 return this; 2699 } 2700 2701 /** 2702 * @return The status of this summary. Enables tracking the life-cycle of the content. 2703 */ 2704 public PublicationStatus getStatus() { 2705 return this.status == null ? null : this.status.getValue(); 2706 } 2707 2708 /** 2709 * @param value The status of this summary. Enables tracking the life-cycle of the content. 2710 */ 2711 public EvidenceReport setStatus(PublicationStatus value) { 2712 if (this.status == null) 2713 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2714 this.status.setValue(value); 2715 return this; 2716 } 2717 2718 /** 2719 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances.) 2720 */ 2721 public List<UsageContext> getUseContext() { 2722 if (this.useContext == null) 2723 this.useContext = new ArrayList<UsageContext>(); 2724 return this.useContext; 2725 } 2726 2727 /** 2728 * @return Returns a reference to <code>this</code> for easy method chaining 2729 */ 2730 public EvidenceReport setUseContext(List<UsageContext> theUseContext) { 2731 this.useContext = theUseContext; 2732 return this; 2733 } 2734 2735 public boolean hasUseContext() { 2736 if (this.useContext == null) 2737 return false; 2738 for (UsageContext item : this.useContext) 2739 if (!item.isEmpty()) 2740 return true; 2741 return false; 2742 } 2743 2744 public UsageContext addUseContext() { //3 2745 UsageContext t = new UsageContext(); 2746 if (this.useContext == null) 2747 this.useContext = new ArrayList<UsageContext>(); 2748 this.useContext.add(t); 2749 return t; 2750 } 2751 2752 public EvidenceReport addUseContext(UsageContext t) { //3 2753 if (t == null) 2754 return this; 2755 if (this.useContext == null) 2756 this.useContext = new ArrayList<UsageContext>(); 2757 this.useContext.add(t); 2758 return this; 2759 } 2760 2761 /** 2762 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2763 */ 2764 public UsageContext getUseContextFirstRep() { 2765 if (getUseContext().isEmpty()) { 2766 addUseContext(); 2767 } 2768 return getUseContext().get(0); 2769 } 2770 2771 /** 2772 * @return {@link #identifier} (A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2773 */ 2774 public List<Identifier> getIdentifier() { 2775 if (this.identifier == null) 2776 this.identifier = new ArrayList<Identifier>(); 2777 return this.identifier; 2778 } 2779 2780 /** 2781 * @return Returns a reference to <code>this</code> for easy method chaining 2782 */ 2783 public EvidenceReport setIdentifier(List<Identifier> theIdentifier) { 2784 this.identifier = theIdentifier; 2785 return this; 2786 } 2787 2788 public boolean hasIdentifier() { 2789 if (this.identifier == null) 2790 return false; 2791 for (Identifier item : this.identifier) 2792 if (!item.isEmpty()) 2793 return true; 2794 return false; 2795 } 2796 2797 public Identifier addIdentifier() { //3 2798 Identifier t = new Identifier(); 2799 if (this.identifier == null) 2800 this.identifier = new ArrayList<Identifier>(); 2801 this.identifier.add(t); 2802 return t; 2803 } 2804 2805 public EvidenceReport addIdentifier(Identifier t) { //3 2806 if (t == null) 2807 return this; 2808 if (this.identifier == null) 2809 this.identifier = new ArrayList<Identifier>(); 2810 this.identifier.add(t); 2811 return this; 2812 } 2813 2814 /** 2815 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2816 */ 2817 public Identifier getIdentifierFirstRep() { 2818 if (getIdentifier().isEmpty()) { 2819 addIdentifier(); 2820 } 2821 return getIdentifier().get(0); 2822 } 2823 2824 /** 2825 * @return {@link #relatedIdentifier} (A formal identifier that is used to identify things closely related to this EvidenceReport.) 2826 */ 2827 public List<Identifier> getRelatedIdentifier() { 2828 if (this.relatedIdentifier == null) 2829 this.relatedIdentifier = new ArrayList<Identifier>(); 2830 return this.relatedIdentifier; 2831 } 2832 2833 /** 2834 * @return Returns a reference to <code>this</code> for easy method chaining 2835 */ 2836 public EvidenceReport setRelatedIdentifier(List<Identifier> theRelatedIdentifier) { 2837 this.relatedIdentifier = theRelatedIdentifier; 2838 return this; 2839 } 2840 2841 public boolean hasRelatedIdentifier() { 2842 if (this.relatedIdentifier == null) 2843 return false; 2844 for (Identifier item : this.relatedIdentifier) 2845 if (!item.isEmpty()) 2846 return true; 2847 return false; 2848 } 2849 2850 public Identifier addRelatedIdentifier() { //3 2851 Identifier t = new Identifier(); 2852 if (this.relatedIdentifier == null) 2853 this.relatedIdentifier = new ArrayList<Identifier>(); 2854 this.relatedIdentifier.add(t); 2855 return t; 2856 } 2857 2858 public EvidenceReport addRelatedIdentifier(Identifier t) { //3 2859 if (t == null) 2860 return this; 2861 if (this.relatedIdentifier == null) 2862 this.relatedIdentifier = new ArrayList<Identifier>(); 2863 this.relatedIdentifier.add(t); 2864 return this; 2865 } 2866 2867 /** 2868 * @return The first repetition of repeating field {@link #relatedIdentifier}, creating it if it does not already exist {3} 2869 */ 2870 public Identifier getRelatedIdentifierFirstRep() { 2871 if (getRelatedIdentifier().isEmpty()) { 2872 addRelatedIdentifier(); 2873 } 2874 return getRelatedIdentifier().get(0); 2875 } 2876 2877 /** 2878 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2879 */ 2880 public DataType getCiteAs() { 2881 return this.citeAs; 2882 } 2883 2884 /** 2885 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2886 */ 2887 public Reference getCiteAsReference() throws FHIRException { 2888 if (this.citeAs == null) 2889 this.citeAs = new Reference(); 2890 if (!(this.citeAs instanceof Reference)) 2891 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 2892 return (Reference) this.citeAs; 2893 } 2894 2895 public boolean hasCiteAsReference() { 2896 return this.citeAs instanceof Reference; 2897 } 2898 2899 /** 2900 * @return {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2901 */ 2902 public MarkdownType getCiteAsMarkdownType() throws FHIRException { 2903 if (this.citeAs == null) 2904 this.citeAs = new MarkdownType(); 2905 if (!(this.citeAs instanceof MarkdownType)) 2906 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.citeAs.getClass().getName()+" was encountered"); 2907 return (MarkdownType) this.citeAs; 2908 } 2909 2910 public boolean hasCiteAsMarkdownType() { 2911 return this.citeAs instanceof MarkdownType; 2912 } 2913 2914 public boolean hasCiteAs() { 2915 return this.citeAs != null && !this.citeAs.isEmpty(); 2916 } 2917 2918 /** 2919 * @param value {@link #citeAs} (Citation Resource or display of suggested citation for this report.) 2920 */ 2921 public EvidenceReport setCiteAs(DataType value) { 2922 if (value != null && !(value instanceof Reference || value instanceof MarkdownType)) 2923 throw new FHIRException("Not the right type for EvidenceReport.citeAs[x]: "+value.fhirType()); 2924 this.citeAs = value; 2925 return this; 2926 } 2927 2928 /** 2929 * @return {@link #type} (Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.) 2930 */ 2931 public CodeableConcept getType() { 2932 if (this.type == null) 2933 if (Configuration.errorOnAutoCreate()) 2934 throw new Error("Attempt to auto-create EvidenceReport.type"); 2935 else if (Configuration.doAutoCreate()) 2936 this.type = new CodeableConcept(); // cc 2937 return this.type; 2938 } 2939 2940 public boolean hasType() { 2941 return this.type != null && !this.type.isEmpty(); 2942 } 2943 2944 /** 2945 * @param value {@link #type} (Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.) 2946 */ 2947 public EvidenceReport setType(CodeableConcept value) { 2948 this.type = value; 2949 return this; 2950 } 2951 2952 /** 2953 * @return {@link #note} (Used for footnotes and annotations.) 2954 */ 2955 public List<Annotation> getNote() { 2956 if (this.note == null) 2957 this.note = new ArrayList<Annotation>(); 2958 return this.note; 2959 } 2960 2961 /** 2962 * @return Returns a reference to <code>this</code> for easy method chaining 2963 */ 2964 public EvidenceReport setNote(List<Annotation> theNote) { 2965 this.note = theNote; 2966 return this; 2967 } 2968 2969 public boolean hasNote() { 2970 if (this.note == null) 2971 return false; 2972 for (Annotation item : this.note) 2973 if (!item.isEmpty()) 2974 return true; 2975 return false; 2976 } 2977 2978 public Annotation addNote() { //3 2979 Annotation t = new Annotation(); 2980 if (this.note == null) 2981 this.note = new ArrayList<Annotation>(); 2982 this.note.add(t); 2983 return t; 2984 } 2985 2986 public EvidenceReport addNote(Annotation t) { //3 2987 if (t == null) 2988 return this; 2989 if (this.note == null) 2990 this.note = new ArrayList<Annotation>(); 2991 this.note.add(t); 2992 return this; 2993 } 2994 2995 /** 2996 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2997 */ 2998 public Annotation getNoteFirstRep() { 2999 if (getNote().isEmpty()) { 3000 addNote(); 3001 } 3002 return getNote().get(0); 3003 } 3004 3005 /** 3006 * @return {@link #relatedArtifact} (Link, description or reference to artifact associated with the report.) 3007 */ 3008 public List<RelatedArtifact> getRelatedArtifact() { 3009 if (this.relatedArtifact == null) 3010 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3011 return this.relatedArtifact; 3012 } 3013 3014 /** 3015 * @return Returns a reference to <code>this</code> for easy method chaining 3016 */ 3017 public EvidenceReport setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3018 this.relatedArtifact = theRelatedArtifact; 3019 return this; 3020 } 3021 3022 public boolean hasRelatedArtifact() { 3023 if (this.relatedArtifact == null) 3024 return false; 3025 for (RelatedArtifact item : this.relatedArtifact) 3026 if (!item.isEmpty()) 3027 return true; 3028 return false; 3029 } 3030 3031 public RelatedArtifact addRelatedArtifact() { //3 3032 RelatedArtifact t = new RelatedArtifact(); 3033 if (this.relatedArtifact == null) 3034 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3035 this.relatedArtifact.add(t); 3036 return t; 3037 } 3038 3039 public EvidenceReport addRelatedArtifact(RelatedArtifact t) { //3 3040 if (t == null) 3041 return this; 3042 if (this.relatedArtifact == null) 3043 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3044 this.relatedArtifact.add(t); 3045 return this; 3046 } 3047 3048 /** 3049 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 3050 */ 3051 public RelatedArtifact getRelatedArtifactFirstRep() { 3052 if (getRelatedArtifact().isEmpty()) { 3053 addRelatedArtifact(); 3054 } 3055 return getRelatedArtifact().get(0); 3056 } 3057 3058 /** 3059 * @return {@link #subject} (Specifies the subject or focus of the report. Answers "What is this report about?".) 3060 */ 3061 public EvidenceReportSubjectComponent getSubject() { 3062 if (this.subject == null) 3063 if (Configuration.errorOnAutoCreate()) 3064 throw new Error("Attempt to auto-create EvidenceReport.subject"); 3065 else if (Configuration.doAutoCreate()) 3066 this.subject = new EvidenceReportSubjectComponent(); // cc 3067 return this.subject; 3068 } 3069 3070 public boolean hasSubject() { 3071 return this.subject != null && !this.subject.isEmpty(); 3072 } 3073 3074 /** 3075 * @param value {@link #subject} (Specifies the subject or focus of the report. Answers "What is this report about?".) 3076 */ 3077 public EvidenceReport setSubject(EvidenceReportSubjectComponent value) { 3078 this.subject = value; 3079 return this; 3080 } 3081 3082 /** 3083 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3084 */ 3085 public StringType getPublisherElement() { 3086 if (this.publisher == null) 3087 if (Configuration.errorOnAutoCreate()) 3088 throw new Error("Attempt to auto-create EvidenceReport.publisher"); 3089 else if (Configuration.doAutoCreate()) 3090 this.publisher = new StringType(); // bb 3091 return this.publisher; 3092 } 3093 3094 public boolean hasPublisherElement() { 3095 return this.publisher != null && !this.publisher.isEmpty(); 3096 } 3097 3098 public boolean hasPublisher() { 3099 return this.publisher != null && !this.publisher.isEmpty(); 3100 } 3101 3102 /** 3103 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3104 */ 3105 public EvidenceReport setPublisherElement(StringType value) { 3106 this.publisher = value; 3107 return this; 3108 } 3109 3110 /** 3111 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report. 3112 */ 3113 public String getPublisher() { 3114 return this.publisher == null ? null : this.publisher.getValue(); 3115 } 3116 3117 /** 3118 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report. 3119 */ 3120 public EvidenceReport setPublisher(String value) { 3121 if (Utilities.noString(value)) 3122 this.publisher = null; 3123 else { 3124 if (this.publisher == null) 3125 this.publisher = new StringType(); 3126 this.publisher.setValue(value); 3127 } 3128 return this; 3129 } 3130 3131 /** 3132 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3133 */ 3134 public List<ContactDetail> getContact() { 3135 if (this.contact == null) 3136 this.contact = new ArrayList<ContactDetail>(); 3137 return this.contact; 3138 } 3139 3140 /** 3141 * @return Returns a reference to <code>this</code> for easy method chaining 3142 */ 3143 public EvidenceReport setContact(List<ContactDetail> theContact) { 3144 this.contact = theContact; 3145 return this; 3146 } 3147 3148 public boolean hasContact() { 3149 if (this.contact == null) 3150 return false; 3151 for (ContactDetail item : this.contact) 3152 if (!item.isEmpty()) 3153 return true; 3154 return false; 3155 } 3156 3157 public ContactDetail addContact() { //3 3158 ContactDetail t = new ContactDetail(); 3159 if (this.contact == null) 3160 this.contact = new ArrayList<ContactDetail>(); 3161 this.contact.add(t); 3162 return t; 3163 } 3164 3165 public EvidenceReport addContact(ContactDetail t) { //3 3166 if (t == null) 3167 return this; 3168 if (this.contact == null) 3169 this.contact = new ArrayList<ContactDetail>(); 3170 this.contact.add(t); 3171 return this; 3172 } 3173 3174 /** 3175 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3176 */ 3177 public ContactDetail getContactFirstRep() { 3178 if (getContact().isEmpty()) { 3179 addContact(); 3180 } 3181 return getContact().get(0); 3182 } 3183 3184 /** 3185 * @return {@link #author} (An individiual, organization, or device primarily involved in the creation and maintenance of the content.) 3186 */ 3187 public List<ContactDetail> getAuthor() { 3188 if (this.author == null) 3189 this.author = new ArrayList<ContactDetail>(); 3190 return this.author; 3191 } 3192 3193 /** 3194 * @return Returns a reference to <code>this</code> for easy method chaining 3195 */ 3196 public EvidenceReport setAuthor(List<ContactDetail> theAuthor) { 3197 this.author = theAuthor; 3198 return this; 3199 } 3200 3201 public boolean hasAuthor() { 3202 if (this.author == null) 3203 return false; 3204 for (ContactDetail item : this.author) 3205 if (!item.isEmpty()) 3206 return true; 3207 return false; 3208 } 3209 3210 public ContactDetail addAuthor() { //3 3211 ContactDetail t = new ContactDetail(); 3212 if (this.author == null) 3213 this.author = new ArrayList<ContactDetail>(); 3214 this.author.add(t); 3215 return t; 3216 } 3217 3218 public EvidenceReport addAuthor(ContactDetail t) { //3 3219 if (t == null) 3220 return this; 3221 if (this.author == null) 3222 this.author = new ArrayList<ContactDetail>(); 3223 this.author.add(t); 3224 return this; 3225 } 3226 3227 /** 3228 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 3229 */ 3230 public ContactDetail getAuthorFirstRep() { 3231 if (getAuthor().isEmpty()) { 3232 addAuthor(); 3233 } 3234 return getAuthor().get(0); 3235 } 3236 3237 /** 3238 * @return {@link #editor} (An individiual, organization, or device primarily responsible for internal coherence of the content.) 3239 */ 3240 public List<ContactDetail> getEditor() { 3241 if (this.editor == null) 3242 this.editor = new ArrayList<ContactDetail>(); 3243 return this.editor; 3244 } 3245 3246 /** 3247 * @return Returns a reference to <code>this</code> for easy method chaining 3248 */ 3249 public EvidenceReport setEditor(List<ContactDetail> theEditor) { 3250 this.editor = theEditor; 3251 return this; 3252 } 3253 3254 public boolean hasEditor() { 3255 if (this.editor == null) 3256 return false; 3257 for (ContactDetail item : this.editor) 3258 if (!item.isEmpty()) 3259 return true; 3260 return false; 3261 } 3262 3263 public ContactDetail addEditor() { //3 3264 ContactDetail t = new ContactDetail(); 3265 if (this.editor == null) 3266 this.editor = new ArrayList<ContactDetail>(); 3267 this.editor.add(t); 3268 return t; 3269 } 3270 3271 public EvidenceReport addEditor(ContactDetail t) { //3 3272 if (t == null) 3273 return this; 3274 if (this.editor == null) 3275 this.editor = new ArrayList<ContactDetail>(); 3276 this.editor.add(t); 3277 return this; 3278 } 3279 3280 /** 3281 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 3282 */ 3283 public ContactDetail getEditorFirstRep() { 3284 if (getEditor().isEmpty()) { 3285 addEditor(); 3286 } 3287 return getEditor().get(0); 3288 } 3289 3290 /** 3291 * @return {@link #reviewer} (An individiual, organization, or device primarily responsible for review of some aspect of the content.) 3292 */ 3293 public List<ContactDetail> getReviewer() { 3294 if (this.reviewer == null) 3295 this.reviewer = new ArrayList<ContactDetail>(); 3296 return this.reviewer; 3297 } 3298 3299 /** 3300 * @return Returns a reference to <code>this</code> for easy method chaining 3301 */ 3302 public EvidenceReport setReviewer(List<ContactDetail> theReviewer) { 3303 this.reviewer = theReviewer; 3304 return this; 3305 } 3306 3307 public boolean hasReviewer() { 3308 if (this.reviewer == null) 3309 return false; 3310 for (ContactDetail item : this.reviewer) 3311 if (!item.isEmpty()) 3312 return true; 3313 return false; 3314 } 3315 3316 public ContactDetail addReviewer() { //3 3317 ContactDetail t = new ContactDetail(); 3318 if (this.reviewer == null) 3319 this.reviewer = new ArrayList<ContactDetail>(); 3320 this.reviewer.add(t); 3321 return t; 3322 } 3323 3324 public EvidenceReport addReviewer(ContactDetail t) { //3 3325 if (t == null) 3326 return this; 3327 if (this.reviewer == null) 3328 this.reviewer = new ArrayList<ContactDetail>(); 3329 this.reviewer.add(t); 3330 return this; 3331 } 3332 3333 /** 3334 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 3335 */ 3336 public ContactDetail getReviewerFirstRep() { 3337 if (getReviewer().isEmpty()) { 3338 addReviewer(); 3339 } 3340 return getReviewer().get(0); 3341 } 3342 3343 /** 3344 * @return {@link #endorser} (An individiual, organization, or device responsible for officially endorsing the content for use in some setting.) 3345 */ 3346 public List<ContactDetail> getEndorser() { 3347 if (this.endorser == null) 3348 this.endorser = new ArrayList<ContactDetail>(); 3349 return this.endorser; 3350 } 3351 3352 /** 3353 * @return Returns a reference to <code>this</code> for easy method chaining 3354 */ 3355 public EvidenceReport setEndorser(List<ContactDetail> theEndorser) { 3356 this.endorser = theEndorser; 3357 return this; 3358 } 3359 3360 public boolean hasEndorser() { 3361 if (this.endorser == null) 3362 return false; 3363 for (ContactDetail item : this.endorser) 3364 if (!item.isEmpty()) 3365 return true; 3366 return false; 3367 } 3368 3369 public ContactDetail addEndorser() { //3 3370 ContactDetail t = new ContactDetail(); 3371 if (this.endorser == null) 3372 this.endorser = new ArrayList<ContactDetail>(); 3373 this.endorser.add(t); 3374 return t; 3375 } 3376 3377 public EvidenceReport addEndorser(ContactDetail t) { //3 3378 if (t == null) 3379 return this; 3380 if (this.endorser == null) 3381 this.endorser = new ArrayList<ContactDetail>(); 3382 this.endorser.add(t); 3383 return this; 3384 } 3385 3386 /** 3387 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 3388 */ 3389 public ContactDetail getEndorserFirstRep() { 3390 if (getEndorser().isEmpty()) { 3391 addEndorser(); 3392 } 3393 return getEndorser().get(0); 3394 } 3395 3396 /** 3397 * @return {@link #relatesTo} (Relationships that this composition has with other compositions or documents that already exist.) 3398 */ 3399 public List<EvidenceReportRelatesToComponent> getRelatesTo() { 3400 if (this.relatesTo == null) 3401 this.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 3402 return this.relatesTo; 3403 } 3404 3405 /** 3406 * @return Returns a reference to <code>this</code> for easy method chaining 3407 */ 3408 public EvidenceReport setRelatesTo(List<EvidenceReportRelatesToComponent> theRelatesTo) { 3409 this.relatesTo = theRelatesTo; 3410 return this; 3411 } 3412 3413 public boolean hasRelatesTo() { 3414 if (this.relatesTo == null) 3415 return false; 3416 for (EvidenceReportRelatesToComponent item : this.relatesTo) 3417 if (!item.isEmpty()) 3418 return true; 3419 return false; 3420 } 3421 3422 public EvidenceReportRelatesToComponent addRelatesTo() { //3 3423 EvidenceReportRelatesToComponent t = new EvidenceReportRelatesToComponent(); 3424 if (this.relatesTo == null) 3425 this.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 3426 this.relatesTo.add(t); 3427 return t; 3428 } 3429 3430 public EvidenceReport addRelatesTo(EvidenceReportRelatesToComponent t) { //3 3431 if (t == null) 3432 return this; 3433 if (this.relatesTo == null) 3434 this.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 3435 this.relatesTo.add(t); 3436 return this; 3437 } 3438 3439 /** 3440 * @return The first repetition of repeating field {@link #relatesTo}, creating it if it does not already exist {3} 3441 */ 3442 public EvidenceReportRelatesToComponent getRelatesToFirstRep() { 3443 if (getRelatesTo().isEmpty()) { 3444 addRelatesTo(); 3445 } 3446 return getRelatesTo().get(0); 3447 } 3448 3449 /** 3450 * @return {@link #section} (The root of the sections that make up the composition.) 3451 */ 3452 public List<SectionComponent> getSection() { 3453 if (this.section == null) 3454 this.section = new ArrayList<SectionComponent>(); 3455 return this.section; 3456 } 3457 3458 /** 3459 * @return Returns a reference to <code>this</code> for easy method chaining 3460 */ 3461 public EvidenceReport setSection(List<SectionComponent> theSection) { 3462 this.section = theSection; 3463 return this; 3464 } 3465 3466 public boolean hasSection() { 3467 if (this.section == null) 3468 return false; 3469 for (SectionComponent item : this.section) 3470 if (!item.isEmpty()) 3471 return true; 3472 return false; 3473 } 3474 3475 public SectionComponent addSection() { //3 3476 SectionComponent t = new SectionComponent(); 3477 if (this.section == null) 3478 this.section = new ArrayList<SectionComponent>(); 3479 this.section.add(t); 3480 return t; 3481 } 3482 3483 public EvidenceReport addSection(SectionComponent t) { //3 3484 if (t == null) 3485 return this; 3486 if (this.section == null) 3487 this.section = new ArrayList<SectionComponent>(); 3488 this.section.add(t); 3489 return this; 3490 } 3491 3492 /** 3493 * @return The first repetition of repeating field {@link #section}, creating it if it does not already exist {3} 3494 */ 3495 public SectionComponent getSectionFirstRep() { 3496 if (getSection().isEmpty()) { 3497 addSection(); 3498 } 3499 return getSection().get(0); 3500 } 3501 3502 /** 3503 * not supported on this implementation 3504 */ 3505 @Override 3506 public int getVersionMax() { 3507 return 0; 3508 } 3509 /** 3510 * @return {@link #version} (The identifier that is used to identify this version of the evidence report when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence report author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.)). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3511 */ 3512 public StringType getVersionElement() { 3513 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3514 } 3515 3516 public boolean hasVersionElement() { 3517 return false; 3518 } 3519 public boolean hasVersion() { 3520 return false; 3521 } 3522 3523 /** 3524 * @param value {@link #version} (The identifier that is used to identify this version of the evidence report when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence report author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.)). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3525 */ 3526 public EvidenceReport setVersionElement(StringType value) { 3527 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3528 } 3529 public String getVersion() { 3530 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3531 } 3532 /** 3533 * @param value The identifier that is used to identify this version of the evidence report when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence report author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence without additional knowledge. (See the versionAlgorithm element.) 3534 */ 3535 public EvidenceReport setVersion(String value) { 3536 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"version\""); 3537 } 3538 /** 3539 * not supported on this implementation 3540 */ 3541 @Override 3542 public int getVersionAlgorithmMax() { 3543 return 0; 3544 } 3545 /** 3546 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3547 */ 3548 public DataType getVersionAlgorithm() { 3549 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3550 } 3551 /** 3552 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3553 */ 3554 public StringType getVersionAlgorithmStringType() { 3555 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3556 } 3557 public boolean hasVersionAlgorithmStringType() { 3558 return false;////K 3559 } 3560 /** 3561 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3562 */ 3563 public Coding getVersionAlgorithmCoding() { 3564 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3565 } 3566 public boolean hasVersionAlgorithmCoding() { 3567 return false;////K 3568 } 3569 public boolean hasVersionAlgorithm() { 3570 return false; 3571 } 3572 /** 3573 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3574 */ 3575 public EvidenceReport setVersionAlgorithm(DataType value) { 3576 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"versionAlgorithm[x]\""); 3577 } 3578 3579 /** 3580 * not supported on this implementation 3581 */ 3582 @Override 3583 public int getNameMax() { 3584 return 0; 3585 } 3586 /** 3587 * @return {@link #name} (A natural language name identifying the evidence report. This name should be usable as an identifier for the resource by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3588 */ 3589 public StringType getNameElement() { 3590 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3591 } 3592 3593 public boolean hasNameElement() { 3594 return false; 3595 } 3596 public boolean hasName() { 3597 return false; 3598 } 3599 3600 /** 3601 * @param value {@link #name} (A natural language name identifying the evidence report. This name should be usable as an identifier for the resource by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3602 */ 3603 public EvidenceReport setNameElement(StringType value) { 3604 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3605 } 3606 public String getName() { 3607 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3608 } 3609 /** 3610 * @param value A natural language name identifying the evidence report. This name should be usable as an identifier for the resource by machine processing applications such as code generation. 3611 */ 3612 public EvidenceReport setName(String value) { 3613 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"name\""); 3614 } 3615 /** 3616 * not supported on this implementation 3617 */ 3618 @Override 3619 public int getTitleMax() { 3620 return 0; 3621 } 3622 /** 3623 * @return {@link #title} (A short, descriptive, user-friendly title for the evidence report.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3624 */ 3625 public StringType getTitleElement() { 3626 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3627 } 3628 3629 public boolean hasTitleElement() { 3630 return false; 3631 } 3632 public boolean hasTitle() { 3633 return false; 3634 } 3635 3636 /** 3637 * @param value {@link #title} (A short, descriptive, user-friendly title for the evidence report.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3638 */ 3639 public EvidenceReport setTitleElement(StringType value) { 3640 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3641 } 3642 public String getTitle() { 3643 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3644 } 3645 /** 3646 * @param value A short, descriptive, user-friendly title for the evidence report. 3647 */ 3648 public EvidenceReport setTitle(String value) { 3649 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"title\""); 3650 } 3651 /** 3652 * not supported on this implementation 3653 */ 3654 @Override 3655 public int getExperimentalMax() { 3656 return 0; 3657 } 3658 /** 3659 * @return {@link #experimental} (A Boolean value to indicate that this evidence report is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3660 */ 3661 public BooleanType getExperimentalElement() { 3662 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3663 } 3664 3665 public boolean hasExperimentalElement() { 3666 return false; 3667 } 3668 public boolean hasExperimental() { 3669 return false; 3670 } 3671 3672 /** 3673 * @param value {@link #experimental} (A Boolean value to indicate that this evidence report is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3674 */ 3675 public EvidenceReport setExperimentalElement(BooleanType value) { 3676 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3677 } 3678 public boolean getExperimental() { 3679 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3680 } 3681 /** 3682 * @param value A Boolean value to indicate that this evidence report is authored for testing purposes (or education/evaluation/marketing) and is not intended for genuine usage. 3683 */ 3684 public EvidenceReport setExperimental(boolean value) { 3685 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"experimental\""); 3686 } 3687 /** 3688 * not supported on this implementation 3689 */ 3690 @Override 3691 public int getDateMax() { 3692 return 0; 3693 } 3694 /** 3695 * @return {@link #date} (The date (and optionally time) when the evidence report was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence report changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3696 */ 3697 public DateTimeType getDateElement() { 3698 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3699 } 3700 3701 public boolean hasDateElement() { 3702 return false; 3703 } 3704 public boolean hasDate() { 3705 return false; 3706 } 3707 3708 /** 3709 * @param value {@link #date} (The date (and optionally time) when the evidence report was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence report changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3710 */ 3711 public EvidenceReport setDateElement(DateTimeType value) { 3712 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3713 } 3714 public Date getDate() { 3715 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3716 } 3717 /** 3718 * @param value The date (and optionally time) when the evidence report was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence report changes. 3719 */ 3720 public EvidenceReport setDate(Date value) { 3721 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"date\""); 3722 } 3723 /** 3724 * not supported on this implementation 3725 */ 3726 @Override 3727 public int getDescriptionMax() { 3728 return 0; 3729 } 3730 /** 3731 * @return {@link #description} (A free text natural language description of the evidence report from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3732 */ 3733 public MarkdownType getDescriptionElement() { 3734 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3735 } 3736 3737 public boolean hasDescriptionElement() { 3738 return false; 3739 } 3740 public boolean hasDescription() { 3741 return false; 3742 } 3743 3744 /** 3745 * @param value {@link #description} (A free text natural language description of the evidence report from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3746 */ 3747 public EvidenceReport setDescriptionElement(MarkdownType value) { 3748 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3749 } 3750 public String getDescription() { 3751 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3752 } 3753 /** 3754 * @param value A free text natural language description of the evidence report from a consumer's perspective. 3755 */ 3756 public EvidenceReport setDescription(String value) { 3757 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"description\""); 3758 } 3759 /** 3760 * not supported on this implementation 3761 */ 3762 @Override 3763 public int getJurisdictionMax() { 3764 return 0; 3765 } 3766 /** 3767 * @return {@link #jurisdiction} (A legal or geographic region in which the evidence report is intended to be used.) 3768 */ 3769 public List<CodeableConcept> getJurisdiction() { 3770 return new ArrayList<>(); 3771 } 3772 /** 3773 * @return Returns a reference to <code>this</code> for easy method chaining 3774 */ 3775 public EvidenceReport setJurisdiction(List<CodeableConcept> theJurisdiction) { 3776 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3777 } 3778 public boolean hasJurisdiction() { 3779 return false; 3780 } 3781 3782 public CodeableConcept addJurisdiction() { //3 3783 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3784 } 3785 public EvidenceReport addJurisdiction(CodeableConcept t) { //3 3786 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3787 } 3788 /** 3789 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {2} 3790 */ 3791 public CodeableConcept getJurisdictionFirstRep() { 3792 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"jurisdiction\""); 3793 } 3794 /** 3795 * not supported on this implementation 3796 */ 3797 @Override 3798 public int getPurposeMax() { 3799 return 0; 3800 } 3801 /** 3802 * @return {@link #purpose} (Explanation of why this evidence report is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3803 */ 3804 public MarkdownType getPurposeElement() { 3805 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3806 } 3807 3808 public boolean hasPurposeElement() { 3809 return false; 3810 } 3811 public boolean hasPurpose() { 3812 return false; 3813 } 3814 3815 /** 3816 * @param value {@link #purpose} (Explanation of why this evidence report is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3817 */ 3818 public EvidenceReport setPurposeElement(MarkdownType value) { 3819 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3820 } 3821 public String getPurpose() { 3822 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3823 } 3824 /** 3825 * @param value Explanation of why this evidence report is needed and why it has been designed as it has. 3826 */ 3827 public EvidenceReport setPurpose(String value) { 3828 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"purpose\""); 3829 } 3830 /** 3831 * not supported on this implementation 3832 */ 3833 @Override 3834 public int getCopyrightMax() { 3835 return 0; 3836 } 3837 /** 3838 * @return {@link #copyright} (A copyright statement relating to the evidence report and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3839 */ 3840 public MarkdownType getCopyrightElement() { 3841 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3842 } 3843 3844 public boolean hasCopyrightElement() { 3845 return false; 3846 } 3847 public boolean hasCopyright() { 3848 return false; 3849 } 3850 3851 /** 3852 * @param value {@link #copyright} (A copyright statement relating to the evidence report and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence report.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3853 */ 3854 public EvidenceReport setCopyrightElement(MarkdownType value) { 3855 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3856 } 3857 public String getCopyright() { 3858 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3859 } 3860 /** 3861 * @param value A copyright statement relating to the evidence report and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence report. 3862 */ 3863 public EvidenceReport setCopyright(String value) { 3864 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyright\""); 3865 } 3866 /** 3867 * not supported on this implementation 3868 */ 3869 @Override 3870 public int getCopyrightLabelMax() { 3871 return 0; 3872 } 3873 /** 3874 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3875 */ 3876 public StringType getCopyrightLabelElement() { 3877 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3878 } 3879 3880 public boolean hasCopyrightLabelElement() { 3881 return false; 3882 } 3883 public boolean hasCopyrightLabel() { 3884 return false; 3885 } 3886 3887 /** 3888 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3889 */ 3890 public EvidenceReport setCopyrightLabelElement(StringType value) { 3891 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3892 } 3893 public String getCopyrightLabel() { 3894 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3895 } 3896 /** 3897 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3898 */ 3899 public EvidenceReport setCopyrightLabel(String value) { 3900 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"copyrightLabel\""); 3901 } 3902 /** 3903 * not supported on this implementation 3904 */ 3905 @Override 3906 public int getApprovalDateMax() { 3907 return 0; 3908 } 3909 /** 3910 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3911 */ 3912 public DateType getApprovalDateElement() { 3913 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3914 } 3915 3916 public boolean hasApprovalDateElement() { 3917 return false; 3918 } 3919 public boolean hasApprovalDate() { 3920 return false; 3921 } 3922 3923 /** 3924 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3925 */ 3926 public EvidenceReport setApprovalDateElement(DateType value) { 3927 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3928 } 3929 public Date getApprovalDate() { 3930 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3931 } 3932 /** 3933 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3934 */ 3935 public EvidenceReport setApprovalDate(Date value) { 3936 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"approvalDate\""); 3937 } 3938 /** 3939 * not supported on this implementation 3940 */ 3941 @Override 3942 public int getLastReviewDateMax() { 3943 return 0; 3944 } 3945 /** 3946 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3947 */ 3948 public DateType getLastReviewDateElement() { 3949 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3950 } 3951 3952 public boolean hasLastReviewDateElement() { 3953 return false; 3954 } 3955 public boolean hasLastReviewDate() { 3956 return false; 3957 } 3958 3959 /** 3960 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3961 */ 3962 public EvidenceReport setLastReviewDateElement(DateType value) { 3963 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3964 } 3965 public Date getLastReviewDate() { 3966 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3967 } 3968 /** 3969 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3970 */ 3971 public EvidenceReport setLastReviewDate(Date value) { 3972 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"lastReviewDate\""); 3973 } 3974 /** 3975 * not supported on this implementation 3976 */ 3977 @Override 3978 public int getEffectivePeriodMax() { 3979 return 0; 3980 } 3981 /** 3982 * @return {@link #effectivePeriod} (The period during which the evidence report content was or is planned to be in active use.) 3983 */ 3984 public Period getEffectivePeriod() { 3985 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"effectivePeriod\""); 3986 } 3987 public boolean hasEffectivePeriod() { 3988 return false; 3989 } 3990 /** 3991 * @param value {@link #effectivePeriod} (The period during which the evidence report content was or is planned to be in active use.) 3992 */ 3993 public EvidenceReport setEffectivePeriod(Period value) { 3994 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"effectivePeriod\""); 3995 } 3996 3997 /** 3998 * not supported on this implementation 3999 */ 4000 @Override 4001 public int getTopicMax() { 4002 return 0; 4003 } 4004 /** 4005 * @return {@link #topic} (Descriptive topics related to the content of the evidence report. Topics provide a high-level categorization as well as keywords for the evidence report that can be useful for filtering and searching.) 4006 */ 4007 public List<CodeableConcept> getTopic() { 4008 return new ArrayList<>(); 4009 } 4010 /** 4011 * @return Returns a reference to <code>this</code> for easy method chaining 4012 */ 4013 public EvidenceReport setTopic(List<CodeableConcept> theTopic) { 4014 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4015 } 4016 public boolean hasTopic() { 4017 return false; 4018 } 4019 4020 public CodeableConcept addTopic() { //3 4021 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4022 } 4023 public EvidenceReport addTopic(CodeableConcept t) { //3 4024 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4025 } 4026 /** 4027 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 4028 */ 4029 public CodeableConcept getTopicFirstRep() { 4030 throw new Error("The resource type \"EvidenceReport\" does not implement the property \"topic\""); 4031 } 4032 protected void listChildren(List<Property> children) { 4033 super.listChildren(children); 4034 children.add(new Property("url", "uri", "An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url)); 4035 children.add(new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status)); 4036 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4037 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4038 children.add(new Property("relatedIdentifier", "Identifier", "A formal identifier that is used to identify things closely related to this EvidenceReport.", 0, java.lang.Integer.MAX_VALUE, relatedIdentifier)); 4039 children.add(new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs)); 4040 children.add(new Property("type", "CodeableConcept", "Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.", 0, 1, type)); 4041 children.add(new Property("note", "Annotation", "Used for footnotes and annotations.", 0, java.lang.Integer.MAX_VALUE, note)); 4042 children.add(new Property("relatedArtifact", "RelatedArtifact", "Link, description or reference to artifact associated with the report.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4043 children.add(new Property("subject", "", "Specifies the subject or focus of the report. Answers \"What is this report about?\".", 0, 1, subject)); 4044 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.", 0, 1, publisher)); 4045 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4046 children.add(new Property("author", "ContactDetail", "An individiual, organization, or device primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 4047 children.add(new Property("editor", "ContactDetail", "An individiual, organization, or device primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 4048 children.add(new Property("reviewer", "ContactDetail", "An individiual, organization, or device primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 4049 children.add(new Property("endorser", "ContactDetail", "An individiual, organization, or device responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 4050 children.add(new Property("relatesTo", "", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo)); 4051 children.add(new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section)); 4052 } 4053 4054 @Override 4055 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4056 switch (_hash) { 4057 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this EvidenceReport when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this summary is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the summary is stored on different servers.", 0, 1, url); 4058 case -892481550: /*status*/ return new Property("status", "code", "The status of this summary. Enables tracking the life-cycle of the content.", 0, 1, status); 4059 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence report instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4060 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this EvidenceReport when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4061 case -1007604940: /*relatedIdentifier*/ return new Property("relatedIdentifier", "Identifier", "A formal identifier that is used to identify things closely related to this EvidenceReport.", 0, java.lang.Integer.MAX_VALUE, relatedIdentifier); 4062 case -1706539017: /*citeAs[x]*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4063 case -1360156695: /*citeAs*/ return new Property("citeAs[x]", "Reference(Citation)|markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4064 case 1269009762: /*citeAsReference*/ return new Property("citeAs[x]", "Reference(Citation)", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4065 case 456265720: /*citeAsMarkdown*/ return new Property("citeAs[x]", "markdown", "Citation Resource or display of suggested citation for this report.", 0, 1, citeAs); 4066 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the kind of report, such as grouping of classifiers, search results, or human-compiled expression.", 0, 1, type); 4067 case 3387378: /*note*/ return new Property("note", "Annotation", "Used for footnotes and annotations.", 0, java.lang.Integer.MAX_VALUE, note); 4068 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Link, description or reference to artifact associated with the report.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4069 case -1867885268: /*subject*/ return new Property("subject", "", "Specifies the subject or focus of the report. Answers \"What is this report about?\".", 0, 1, subject); 4070 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence report.", 0, 1, publisher); 4071 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4072 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual, organization, or device primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 4073 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individiual, organization, or device primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 4074 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individiual, organization, or device primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 4075 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individiual, organization, or device responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 4076 case -7765931: /*relatesTo*/ return new Property("relatesTo", "", "Relationships that this composition has with other compositions or documents that already exist.", 0, java.lang.Integer.MAX_VALUE, relatesTo); 4077 case 1970241253: /*section*/ return new Property("section", "", "The root of the sections that make up the composition.", 0, java.lang.Integer.MAX_VALUE, section); 4078 default: return super.getNamedProperty(_hash, _name, _checkValid); 4079 } 4080 4081 } 4082 4083 @Override 4084 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4085 switch (hash) { 4086 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4087 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4088 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4089 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4090 case -1007604940: /*relatedIdentifier*/ return this.relatedIdentifier == null ? new Base[0] : this.relatedIdentifier.toArray(new Base[this.relatedIdentifier.size()]); // Identifier 4091 case -1360156695: /*citeAs*/ return this.citeAs == null ? new Base[0] : new Base[] {this.citeAs}; // DataType 4092 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 4093 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4094 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4095 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // EvidenceReportSubjectComponent 4096 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4097 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4098 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4099 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4100 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4101 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4102 case -7765931: /*relatesTo*/ return this.relatesTo == null ? new Base[0] : this.relatesTo.toArray(new Base[this.relatesTo.size()]); // EvidenceReportRelatesToComponent 4103 case 1970241253: /*section*/ return this.section == null ? new Base[0] : this.section.toArray(new Base[this.section.size()]); // SectionComponent 4104 default: return super.getProperty(hash, name, checkValid); 4105 } 4106 4107 } 4108 4109 @Override 4110 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4111 switch (hash) { 4112 case 116079: // url 4113 this.url = TypeConvertor.castToUri(value); // UriType 4114 return value; 4115 case -892481550: // status 4116 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4117 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4118 return value; 4119 case -669707736: // useContext 4120 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4121 return value; 4122 case -1618432855: // identifier 4123 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4124 return value; 4125 case -1007604940: // relatedIdentifier 4126 this.getRelatedIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4127 return value; 4128 case -1360156695: // citeAs 4129 this.citeAs = TypeConvertor.castToType(value); // DataType 4130 return value; 4131 case 3575610: // type 4132 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4133 return value; 4134 case 3387378: // note 4135 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4136 return value; 4137 case 666807069: // relatedArtifact 4138 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4139 return value; 4140 case -1867885268: // subject 4141 this.subject = (EvidenceReportSubjectComponent) value; // EvidenceReportSubjectComponent 4142 return value; 4143 case 1447404028: // publisher 4144 this.publisher = TypeConvertor.castToString(value); // StringType 4145 return value; 4146 case 951526432: // contact 4147 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4148 return value; 4149 case -1406328437: // author 4150 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4151 return value; 4152 case -1307827859: // editor 4153 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4154 return value; 4155 case -261190139: // reviewer 4156 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4157 return value; 4158 case 1740277666: // endorser 4159 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4160 return value; 4161 case -7765931: // relatesTo 4162 this.getRelatesTo().add((EvidenceReportRelatesToComponent) value); // EvidenceReportRelatesToComponent 4163 return value; 4164 case 1970241253: // section 4165 this.getSection().add((SectionComponent) value); // SectionComponent 4166 return value; 4167 default: return super.setProperty(hash, name, value); 4168 } 4169 4170 } 4171 4172 @Override 4173 public Base setProperty(String name, Base value) throws FHIRException { 4174 if (name.equals("url")) { 4175 this.url = TypeConvertor.castToUri(value); // UriType 4176 } else if (name.equals("status")) { 4177 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4178 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4179 } else if (name.equals("useContext")) { 4180 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4181 } else if (name.equals("identifier")) { 4182 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4183 } else if (name.equals("relatedIdentifier")) { 4184 this.getRelatedIdentifier().add(TypeConvertor.castToIdentifier(value)); 4185 } else if (name.equals("citeAs[x]")) { 4186 this.citeAs = TypeConvertor.castToType(value); // DataType 4187 } else if (name.equals("type")) { 4188 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4189 } else if (name.equals("note")) { 4190 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4191 } else if (name.equals("relatedArtifact")) { 4192 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4193 } else if (name.equals("subject")) { 4194 this.subject = (EvidenceReportSubjectComponent) value; // EvidenceReportSubjectComponent 4195 } else if (name.equals("publisher")) { 4196 this.publisher = TypeConvertor.castToString(value); // StringType 4197 } else if (name.equals("contact")) { 4198 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4199 } else if (name.equals("author")) { 4200 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 4201 } else if (name.equals("editor")) { 4202 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 4203 } else if (name.equals("reviewer")) { 4204 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 4205 } else if (name.equals("endorser")) { 4206 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 4207 } else if (name.equals("relatesTo")) { 4208 this.getRelatesTo().add((EvidenceReportRelatesToComponent) value); 4209 } else if (name.equals("section")) { 4210 this.getSection().add((SectionComponent) value); 4211 } else 4212 return super.setProperty(name, value); 4213 return value; 4214 } 4215 4216 @Override 4217 public void removeChild(String name, Base value) throws FHIRException { 4218 if (name.equals("url")) { 4219 this.url = null; 4220 } else if (name.equals("status")) { 4221 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4222 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4223 } else if (name.equals("useContext")) { 4224 this.getUseContext().remove(value); 4225 } else if (name.equals("identifier")) { 4226 this.getIdentifier().remove(value); 4227 } else if (name.equals("relatedIdentifier")) { 4228 this.getRelatedIdentifier().remove(value); 4229 } else if (name.equals("citeAs[x]")) { 4230 this.citeAs = null; 4231 } else if (name.equals("type")) { 4232 this.type = null; 4233 } else if (name.equals("note")) { 4234 this.getNote().remove(value); 4235 } else if (name.equals("relatedArtifact")) { 4236 this.getRelatedArtifact().remove(value); 4237 } else if (name.equals("subject")) { 4238 this.subject = (EvidenceReportSubjectComponent) value; // EvidenceReportSubjectComponent 4239 } else if (name.equals("publisher")) { 4240 this.publisher = null; 4241 } else if (name.equals("contact")) { 4242 this.getContact().remove(value); 4243 } else if (name.equals("author")) { 4244 this.getAuthor().remove(value); 4245 } else if (name.equals("editor")) { 4246 this.getEditor().remove(value); 4247 } else if (name.equals("reviewer")) { 4248 this.getReviewer().remove(value); 4249 } else if (name.equals("endorser")) { 4250 this.getEndorser().remove(value); 4251 } else if (name.equals("relatesTo")) { 4252 this.getRelatesTo().remove((EvidenceReportRelatesToComponent) value); 4253 } else if (name.equals("section")) { 4254 this.getSection().remove((SectionComponent) value); 4255 } else 4256 super.removeChild(name, value); 4257 4258 } 4259 4260 @Override 4261 public Base makeProperty(int hash, String name) throws FHIRException { 4262 switch (hash) { 4263 case 116079: return getUrlElement(); 4264 case -892481550: return getStatusElement(); 4265 case -669707736: return addUseContext(); 4266 case -1618432855: return addIdentifier(); 4267 case -1007604940: return addRelatedIdentifier(); 4268 case -1706539017: return getCiteAs(); 4269 case -1360156695: return getCiteAs(); 4270 case 3575610: return getType(); 4271 case 3387378: return addNote(); 4272 case 666807069: return addRelatedArtifact(); 4273 case -1867885268: return getSubject(); 4274 case 1447404028: return getPublisherElement(); 4275 case 951526432: return addContact(); 4276 case -1406328437: return addAuthor(); 4277 case -1307827859: return addEditor(); 4278 case -261190139: return addReviewer(); 4279 case 1740277666: return addEndorser(); 4280 case -7765931: return addRelatesTo(); 4281 case 1970241253: return addSection(); 4282 default: return super.makeProperty(hash, name); 4283 } 4284 4285 } 4286 4287 @Override 4288 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4289 switch (hash) { 4290 case 116079: /*url*/ return new String[] {"uri"}; 4291 case -892481550: /*status*/ return new String[] {"code"}; 4292 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4293 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4294 case -1007604940: /*relatedIdentifier*/ return new String[] {"Identifier"}; 4295 case -1360156695: /*citeAs*/ return new String[] {"Reference", "markdown"}; 4296 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4297 case 3387378: /*note*/ return new String[] {"Annotation"}; 4298 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 4299 case -1867885268: /*subject*/ return new String[] {}; 4300 case 1447404028: /*publisher*/ return new String[] {"string"}; 4301 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4302 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 4303 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 4304 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 4305 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 4306 case -7765931: /*relatesTo*/ return new String[] {}; 4307 case 1970241253: /*section*/ return new String[] {}; 4308 default: return super.getTypesForProperty(hash, name); 4309 } 4310 4311 } 4312 4313 @Override 4314 public Base addChild(String name) throws FHIRException { 4315 if (name.equals("url")) { 4316 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.url"); 4317 } 4318 else if (name.equals("status")) { 4319 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.status"); 4320 } 4321 else if (name.equals("useContext")) { 4322 return addUseContext(); 4323 } 4324 else if (name.equals("identifier")) { 4325 return addIdentifier(); 4326 } 4327 else if (name.equals("relatedIdentifier")) { 4328 return addRelatedIdentifier(); 4329 } 4330 else if (name.equals("citeAsReference")) { 4331 this.citeAs = new Reference(); 4332 return this.citeAs; 4333 } 4334 else if (name.equals("citeAsMarkdown")) { 4335 this.citeAs = new MarkdownType(); 4336 return this.citeAs; 4337 } 4338 else if (name.equals("type")) { 4339 this.type = new CodeableConcept(); 4340 return this.type; 4341 } 4342 else if (name.equals("note")) { 4343 return addNote(); 4344 } 4345 else if (name.equals("relatedArtifact")) { 4346 return addRelatedArtifact(); 4347 } 4348 else if (name.equals("subject")) { 4349 this.subject = new EvidenceReportSubjectComponent(); 4350 return this.subject; 4351 } 4352 else if (name.equals("publisher")) { 4353 throw new FHIRException("Cannot call addChild on a singleton property EvidenceReport.publisher"); 4354 } 4355 else if (name.equals("contact")) { 4356 return addContact(); 4357 } 4358 else if (name.equals("author")) { 4359 return addAuthor(); 4360 } 4361 else if (name.equals("editor")) { 4362 return addEditor(); 4363 } 4364 else if (name.equals("reviewer")) { 4365 return addReviewer(); 4366 } 4367 else if (name.equals("endorser")) { 4368 return addEndorser(); 4369 } 4370 else if (name.equals("relatesTo")) { 4371 return addRelatesTo(); 4372 } 4373 else if (name.equals("section")) { 4374 return addSection(); 4375 } 4376 else 4377 return super.addChild(name); 4378 } 4379 4380 public String fhirType() { 4381 return "EvidenceReport"; 4382 4383 } 4384 4385 public EvidenceReport copy() { 4386 EvidenceReport dst = new EvidenceReport(); 4387 copyValues(dst); 4388 return dst; 4389 } 4390 4391 public void copyValues(EvidenceReport dst) { 4392 super.copyValues(dst); 4393 dst.url = url == null ? null : url.copy(); 4394 dst.status = status == null ? null : status.copy(); 4395 if (useContext != null) { 4396 dst.useContext = new ArrayList<UsageContext>(); 4397 for (UsageContext i : useContext) 4398 dst.useContext.add(i.copy()); 4399 }; 4400 if (identifier != null) { 4401 dst.identifier = new ArrayList<Identifier>(); 4402 for (Identifier i : identifier) 4403 dst.identifier.add(i.copy()); 4404 }; 4405 if (relatedIdentifier != null) { 4406 dst.relatedIdentifier = new ArrayList<Identifier>(); 4407 for (Identifier i : relatedIdentifier) 4408 dst.relatedIdentifier.add(i.copy()); 4409 }; 4410 dst.citeAs = citeAs == null ? null : citeAs.copy(); 4411 dst.type = type == null ? null : type.copy(); 4412 if (note != null) { 4413 dst.note = new ArrayList<Annotation>(); 4414 for (Annotation i : note) 4415 dst.note.add(i.copy()); 4416 }; 4417 if (relatedArtifact != null) { 4418 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4419 for (RelatedArtifact i : relatedArtifact) 4420 dst.relatedArtifact.add(i.copy()); 4421 }; 4422 dst.subject = subject == null ? null : subject.copy(); 4423 dst.publisher = publisher == null ? null : publisher.copy(); 4424 if (contact != null) { 4425 dst.contact = new ArrayList<ContactDetail>(); 4426 for (ContactDetail i : contact) 4427 dst.contact.add(i.copy()); 4428 }; 4429 if (author != null) { 4430 dst.author = new ArrayList<ContactDetail>(); 4431 for (ContactDetail i : author) 4432 dst.author.add(i.copy()); 4433 }; 4434 if (editor != null) { 4435 dst.editor = new ArrayList<ContactDetail>(); 4436 for (ContactDetail i : editor) 4437 dst.editor.add(i.copy()); 4438 }; 4439 if (reviewer != null) { 4440 dst.reviewer = new ArrayList<ContactDetail>(); 4441 for (ContactDetail i : reviewer) 4442 dst.reviewer.add(i.copy()); 4443 }; 4444 if (endorser != null) { 4445 dst.endorser = new ArrayList<ContactDetail>(); 4446 for (ContactDetail i : endorser) 4447 dst.endorser.add(i.copy()); 4448 }; 4449 if (relatesTo != null) { 4450 dst.relatesTo = new ArrayList<EvidenceReportRelatesToComponent>(); 4451 for (EvidenceReportRelatesToComponent i : relatesTo) 4452 dst.relatesTo.add(i.copy()); 4453 }; 4454 if (section != null) { 4455 dst.section = new ArrayList<SectionComponent>(); 4456 for (SectionComponent i : section) 4457 dst.section.add(i.copy()); 4458 }; 4459 } 4460 4461 protected EvidenceReport typedCopy() { 4462 return copy(); 4463 } 4464 4465 @Override 4466 public boolean equalsDeep(Base other_) { 4467 if (!super.equalsDeep(other_)) 4468 return false; 4469 if (!(other_ instanceof EvidenceReport)) 4470 return false; 4471 EvidenceReport o = (EvidenceReport) other_; 4472 return compareDeep(url, o.url, true) && compareDeep(status, o.status, true) && compareDeep(useContext, o.useContext, true) 4473 && compareDeep(identifier, o.identifier, true) && compareDeep(relatedIdentifier, o.relatedIdentifier, true) 4474 && compareDeep(citeAs, o.citeAs, true) && compareDeep(type, o.type, true) && compareDeep(note, o.note, true) 4475 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(subject, o.subject, true) 4476 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(author, o.author, true) 4477 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 4478 && compareDeep(relatesTo, o.relatesTo, true) && compareDeep(section, o.section, true); 4479 } 4480 4481 @Override 4482 public boolean equalsShallow(Base other_) { 4483 if (!super.equalsShallow(other_)) 4484 return false; 4485 if (!(other_ instanceof EvidenceReport)) 4486 return false; 4487 EvidenceReport o = (EvidenceReport) other_; 4488 return compareValues(url, o.url, true) && compareValues(status, o.status, true) && compareValues(publisher, o.publisher, true) 4489 ; 4490 } 4491 4492 public boolean isEmpty() { 4493 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, status, useContext 4494 , identifier, relatedIdentifier, citeAs, type, note, relatedArtifact, subject 4495 , publisher, contact, author, editor, reviewer, endorser, relatesTo, section 4496 ); 4497 } 4498 4499 @Override 4500 public ResourceType getResourceType() { 4501 return ResourceType.EvidenceReport; 4502 } 4503 4504 /** 4505 * Search parameter: <b>context-quantity</b> 4506 * <p> 4507 * Description: <b>Multiple Resources: 4508 4509* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4510* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4511* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4512* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4513* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4514* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4515* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4516* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4517* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4518* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4519* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4520* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4521* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4522* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4523* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4524* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4525* [Library](library.html): A quantity- or range-valued use context assigned to the library 4526* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4527* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4528* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4529* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4530* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4531* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4532* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4533* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4534* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4535* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4536* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4537* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4538* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4539</b><br> 4540 * Type: <b>quantity</b><br> 4541 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4542 * </p> 4543 */ 4544 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 4545 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4546 /** 4547 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4548 * <p> 4549 * Description: <b>Multiple Resources: 4550 4551* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 4552* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 4553* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 4554* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 4555* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 4556* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 4557* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 4558* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 4559* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 4560* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 4561* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 4562* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 4563* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 4564* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 4565* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 4566* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 4567* [Library](library.html): A quantity- or range-valued use context assigned to the library 4568* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 4569* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 4570* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 4571* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 4572* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 4573* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 4574* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 4575* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 4576* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 4577* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 4578* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 4579* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 4580* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 4581</b><br> 4582 * Type: <b>quantity</b><br> 4583 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 4584 * </p> 4585 */ 4586 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 4587 4588 /** 4589 * Search parameter: <b>context-type-quantity</b> 4590 * <p> 4591 * Description: <b>Multiple Resources: 4592 4593* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4594* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4595* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4596* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4597* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4598* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4599* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4600* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4601* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4602* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4603* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4604* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4605* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4606* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4607* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4608* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4609* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4610* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4611* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4612* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4613* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4614* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4615* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4616* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4617* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4618* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4619* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4620* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4621* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4622* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4623</b><br> 4624 * Type: <b>composite</b><br> 4625 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4626 * </p> 4627 */ 4628 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 4629 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4630 /** 4631 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 4632 * <p> 4633 * Description: <b>Multiple Resources: 4634 4635* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 4636* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 4637* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 4638* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 4639* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 4640* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 4641* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 4642* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 4643* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 4644* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 4645* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 4646* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 4647* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 4648* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 4649* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 4650* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 4651* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 4652* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 4653* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 4654* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 4655* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 4656* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 4657* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 4658* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 4659* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 4660* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 4661* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 4662* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 4663* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 4664* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 4665</b><br> 4666 * Type: <b>composite</b><br> 4667 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4668 * </p> 4669 */ 4670 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 4671 4672 /** 4673 * Search parameter: <b>context-type-value</b> 4674 * <p> 4675 * Description: <b>Multiple Resources: 4676 4677* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4678* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4679* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4680* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4681* [Citation](citation.html): A use context type and value assigned to the citation 4682* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4683* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4684* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4685* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4686* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4687* [Evidence](evidence.html): A use context type and value assigned to the evidence 4688* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4689* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4690* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4691* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4692* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4693* [Library](library.html): A use context type and value assigned to the library 4694* [Measure](measure.html): A use context type and value assigned to the measure 4695* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4696* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4697* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4698* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4699* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4700* [Requirements](requirements.html): A use context type and value assigned to the requirements 4701* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4702* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4703* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4704* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4705* [TestScript](testscript.html): A use context type and value assigned to the test script 4706* [ValueSet](valueset.html): A use context type and value assigned to the value set 4707</b><br> 4708 * Type: <b>composite</b><br> 4709 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4710 * </p> 4711 */ 4712 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 4713 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4714 /** 4715 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4716 * <p> 4717 * Description: <b>Multiple Resources: 4718 4719* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4720* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4721* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4722* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4723* [Citation](citation.html): A use context type and value assigned to the citation 4724* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4725* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4726* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4727* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4728* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4729* [Evidence](evidence.html): A use context type and value assigned to the evidence 4730* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4731* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4732* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4733* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4734* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4735* [Library](library.html): A use context type and value assigned to the library 4736* [Measure](measure.html): A use context type and value assigned to the measure 4737* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4738* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4739* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4740* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4741* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4742* [Requirements](requirements.html): A use context type and value assigned to the requirements 4743* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4744* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4745* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4746* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4747* [TestScript](testscript.html): A use context type and value assigned to the test script 4748* [ValueSet](valueset.html): A use context type and value assigned to the value set 4749</b><br> 4750 * Type: <b>composite</b><br> 4751 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4752 * </p> 4753 */ 4754 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 4755 4756 /** 4757 * Search parameter: <b>context-type</b> 4758 * <p> 4759 * Description: <b>Multiple Resources: 4760 4761* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4762* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4763* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4764* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4765* [Citation](citation.html): A type of use context assigned to the citation 4766* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4767* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4768* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4769* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4770* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4771* [Evidence](evidence.html): A type of use context assigned to the evidence 4772* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4773* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4774* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4775* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4776* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4777* [Library](library.html): A type of use context assigned to the library 4778* [Measure](measure.html): A type of use context assigned to the measure 4779* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4780* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4781* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4782* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4783* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4784* [Requirements](requirements.html): A type of use context assigned to the requirements 4785* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4786* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4787* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4788* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4789* [TestScript](testscript.html): A type of use context assigned to the test script 4790* [ValueSet](valueset.html): A type of use context assigned to the value set 4791</b><br> 4792 * Type: <b>token</b><br> 4793 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4794 * </p> 4795 */ 4796 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 4797 public static final String SP_CONTEXT_TYPE = "context-type"; 4798 /** 4799 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4800 * <p> 4801 * Description: <b>Multiple Resources: 4802 4803* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4804* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4805* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4806* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4807* [Citation](citation.html): A type of use context assigned to the citation 4808* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4809* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4810* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4811* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4812* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4813* [Evidence](evidence.html): A type of use context assigned to the evidence 4814* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4815* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4816* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4817* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4818* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4819* [Library](library.html): A type of use context assigned to the library 4820* [Measure](measure.html): A type of use context assigned to the measure 4821* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4822* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4823* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4824* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4825* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4826* [Requirements](requirements.html): A type of use context assigned to the requirements 4827* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4828* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4829* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4830* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4831* [TestScript](testscript.html): A type of use context assigned to the test script 4832* [ValueSet](valueset.html): A type of use context assigned to the value set 4833</b><br> 4834 * Type: <b>token</b><br> 4835 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4836 * </p> 4837 */ 4838 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 4839 4840 /** 4841 * Search parameter: <b>context</b> 4842 * <p> 4843 * Description: <b>Multiple Resources: 4844 4845* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4846* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4847* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4848* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4849* [Citation](citation.html): A use context assigned to the citation 4850* [CodeSystem](codesystem.html): A use context assigned to the code system 4851* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4852* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4853* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4854* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4855* [Evidence](evidence.html): A use context assigned to the evidence 4856* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4857* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4858* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4859* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4860* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4861* [Library](library.html): A use context assigned to the library 4862* [Measure](measure.html): A use context assigned to the measure 4863* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4864* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4865* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4866* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4867* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4868* [Requirements](requirements.html): A use context assigned to the requirements 4869* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4870* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4871* [StructureMap](structuremap.html): A use context assigned to the structure map 4872* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4873* [TestScript](testscript.html): A use context assigned to the test script 4874* [ValueSet](valueset.html): A use context assigned to the value set 4875</b><br> 4876 * Type: <b>token</b><br> 4877 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4878 * </p> 4879 */ 4880 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 4881 public static final String SP_CONTEXT = "context"; 4882 /** 4883 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4884 * <p> 4885 * Description: <b>Multiple Resources: 4886 4887* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4888* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4889* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4890* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4891* [Citation](citation.html): A use context assigned to the citation 4892* [CodeSystem](codesystem.html): A use context assigned to the code system 4893* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4894* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4895* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4896* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4897* [Evidence](evidence.html): A use context assigned to the evidence 4898* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4899* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4900* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4901* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4902* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4903* [Library](library.html): A use context assigned to the library 4904* [Measure](measure.html): A use context assigned to the measure 4905* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4906* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4907* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4908* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4909* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4910* [Requirements](requirements.html): A use context assigned to the requirements 4911* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4912* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4913* [StructureMap](structuremap.html): A use context assigned to the structure map 4914* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4915* [TestScript](testscript.html): A use context assigned to the test script 4916* [ValueSet](valueset.html): A use context assigned to the value set 4917</b><br> 4918 * Type: <b>token</b><br> 4919 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4920 * </p> 4921 */ 4922 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4923 4924 /** 4925 * Search parameter: <b>identifier</b> 4926 * <p> 4927 * Description: <b>Multiple Resources: 4928 4929* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4930* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4931* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4932* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4933* [Citation](citation.html): External identifier for the citation 4934* [CodeSystem](codesystem.html): External identifier for the code system 4935* [ConceptMap](conceptmap.html): External identifier for the concept map 4936* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4937* [EventDefinition](eventdefinition.html): External identifier for the event definition 4938* [Evidence](evidence.html): External identifier for the evidence 4939* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4940* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4941* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4942* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4943* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4944* [Library](library.html): External identifier for the library 4945* [Measure](measure.html): External identifier for the measure 4946* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4947* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4948* [NamingSystem](namingsystem.html): External identifier for the naming system 4949* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4950* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4951* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4952* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4953* [Requirements](requirements.html): External identifier for the requirements 4954* [SearchParameter](searchparameter.html): External identifier for the search parameter 4955* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4956* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4957* [StructureMap](structuremap.html): External identifier for the structure map 4958* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4959* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4960* [TestPlan](testplan.html): An identifier for the test plan 4961* [TestScript](testscript.html): External identifier for the test script 4962* [ValueSet](valueset.html): External identifier for the value set 4963</b><br> 4964 * Type: <b>token</b><br> 4965 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4966 * </p> 4967 */ 4968 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4969 public static final String SP_IDENTIFIER = "identifier"; 4970 /** 4971 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4972 * <p> 4973 * Description: <b>Multiple Resources: 4974 4975* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4976* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4977* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4978* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4979* [Citation](citation.html): External identifier for the citation 4980* [CodeSystem](codesystem.html): External identifier for the code system 4981* [ConceptMap](conceptmap.html): External identifier for the concept map 4982* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4983* [EventDefinition](eventdefinition.html): External identifier for the event definition 4984* [Evidence](evidence.html): External identifier for the evidence 4985* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4986* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4987* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4988* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4989* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4990* [Library](library.html): External identifier for the library 4991* [Measure](measure.html): External identifier for the measure 4992* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4993* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4994* [NamingSystem](namingsystem.html): External identifier for the naming system 4995* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4996* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4997* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4998* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4999* [Requirements](requirements.html): External identifier for the requirements 5000* [SearchParameter](searchparameter.html): External identifier for the search parameter 5001* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5002* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5003* [StructureMap](structuremap.html): External identifier for the structure map 5004* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5005* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5006* [TestPlan](testplan.html): An identifier for the test plan 5007* [TestScript](testscript.html): External identifier for the test script 5008* [ValueSet](valueset.html): External identifier for the value set 5009</b><br> 5010 * Type: <b>token</b><br> 5011 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5012 * </p> 5013 */ 5014 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5015 5016 /** 5017 * Search parameter: <b>publisher</b> 5018 * <p> 5019 * Description: <b>Multiple Resources: 5020 5021* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5022* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5023* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5024* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5025* [Citation](citation.html): Name of the publisher of the citation 5026* [CodeSystem](codesystem.html): Name of the publisher of the code system 5027* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5028* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5029* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5030* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5031* [Evidence](evidence.html): Name of the publisher of the evidence 5032* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5033* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5034* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5035* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5036* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5037* [Library](library.html): Name of the publisher of the library 5038* [Measure](measure.html): Name of the publisher of the measure 5039* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5040* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5041* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5042* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5043* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5044* [Requirements](requirements.html): Name of the publisher of the requirements 5045* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5046* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5047* [StructureMap](structuremap.html): Name of the publisher of the structure map 5048* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5049* [TestScript](testscript.html): Name of the publisher of the test script 5050* [ValueSet](valueset.html): Name of the publisher of the value set 5051</b><br> 5052 * Type: <b>string</b><br> 5053 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5054 * </p> 5055 */ 5056 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 5057 public static final String SP_PUBLISHER = "publisher"; 5058 /** 5059 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5060 * <p> 5061 * Description: <b>Multiple Resources: 5062 5063* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 5064* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 5065* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 5066* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 5067* [Citation](citation.html): Name of the publisher of the citation 5068* [CodeSystem](codesystem.html): Name of the publisher of the code system 5069* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 5070* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 5071* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 5072* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 5073* [Evidence](evidence.html): Name of the publisher of the evidence 5074* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 5075* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 5076* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 5077* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 5078* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 5079* [Library](library.html): Name of the publisher of the library 5080* [Measure](measure.html): Name of the publisher of the measure 5081* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 5082* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 5083* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 5084* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 5085* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 5086* [Requirements](requirements.html): Name of the publisher of the requirements 5087* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 5088* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 5089* [StructureMap](structuremap.html): Name of the publisher of the structure map 5090* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 5091* [TestScript](testscript.html): Name of the publisher of the test script 5092* [ValueSet](valueset.html): Name of the publisher of the value set 5093</b><br> 5094 * Type: <b>string</b><br> 5095 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 5096 * </p> 5097 */ 5098 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5099 5100 /** 5101 * Search parameter: <b>status</b> 5102 * <p> 5103 * Description: <b>Multiple Resources: 5104 5105* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5106* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5107* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5108* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5109* [Citation](citation.html): The current status of the citation 5110* [CodeSystem](codesystem.html): The current status of the code system 5111* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5112* [ConceptMap](conceptmap.html): The current status of the concept map 5113* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5114* [EventDefinition](eventdefinition.html): The current status of the event definition 5115* [Evidence](evidence.html): The current status of the evidence 5116* [EvidenceReport](evidencereport.html): The current status of the evidence report 5117* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5118* [ExampleScenario](examplescenario.html): The current status of the example scenario 5119* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5120* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5121* [Library](library.html): The current status of the library 5122* [Measure](measure.html): The current status of the measure 5123* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5124* [MessageDefinition](messagedefinition.html): The current status of the message definition 5125* [NamingSystem](namingsystem.html): The current status of the naming system 5126* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5127* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5128* [PlanDefinition](plandefinition.html): The current status of the plan definition 5129* [Questionnaire](questionnaire.html): The current status of the questionnaire 5130* [Requirements](requirements.html): The current status of the requirements 5131* [SearchParameter](searchparameter.html): The current status of the search parameter 5132* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5133* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5134* [StructureMap](structuremap.html): The current status of the structure map 5135* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5136* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5137* [TestPlan](testplan.html): The current status of the test plan 5138* [TestScript](testscript.html): The current status of the test script 5139* [ValueSet](valueset.html): The current status of the value set 5140</b><br> 5141 * Type: <b>token</b><br> 5142 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5143 * </p> 5144 */ 5145 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 5146 public static final String SP_STATUS = "status"; 5147 /** 5148 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5149 * <p> 5150 * Description: <b>Multiple Resources: 5151 5152* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 5153* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 5154* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 5155* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 5156* [Citation](citation.html): The current status of the citation 5157* [CodeSystem](codesystem.html): The current status of the code system 5158* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 5159* [ConceptMap](conceptmap.html): The current status of the concept map 5160* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 5161* [EventDefinition](eventdefinition.html): The current status of the event definition 5162* [Evidence](evidence.html): The current status of the evidence 5163* [EvidenceReport](evidencereport.html): The current status of the evidence report 5164* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 5165* [ExampleScenario](examplescenario.html): The current status of the example scenario 5166* [GraphDefinition](graphdefinition.html): The current status of the graph definition 5167* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 5168* [Library](library.html): The current status of the library 5169* [Measure](measure.html): The current status of the measure 5170* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 5171* [MessageDefinition](messagedefinition.html): The current status of the message definition 5172* [NamingSystem](namingsystem.html): The current status of the naming system 5173* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 5174* [OperationDefinition](operationdefinition.html): The current status of the operation definition 5175* [PlanDefinition](plandefinition.html): The current status of the plan definition 5176* [Questionnaire](questionnaire.html): The current status of the questionnaire 5177* [Requirements](requirements.html): The current status of the requirements 5178* [SearchParameter](searchparameter.html): The current status of the search parameter 5179* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 5180* [StructureDefinition](structuredefinition.html): The current status of the structure definition 5181* [StructureMap](structuremap.html): The current status of the structure map 5182* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 5183* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 5184* [TestPlan](testplan.html): The current status of the test plan 5185* [TestScript](testscript.html): The current status of the test script 5186* [ValueSet](valueset.html): The current status of the value set 5187</b><br> 5188 * Type: <b>token</b><br> 5189 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 5190 * </p> 5191 */ 5192 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5193 5194 /** 5195 * Search parameter: <b>url</b> 5196 * <p> 5197 * Description: <b>Multiple Resources: 5198 5199* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5200* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5201* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5202* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5203* [Citation](citation.html): The uri that identifies the citation 5204* [CodeSystem](codesystem.html): The uri that identifies the code system 5205* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5206* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5207* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5208* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5209* [Evidence](evidence.html): The uri that identifies the evidence 5210* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5211* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5212* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5213* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5214* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5215* [Library](library.html): The uri that identifies the library 5216* [Measure](measure.html): The uri that identifies the measure 5217* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5218* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5219* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5220* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5221* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5222* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5223* [Requirements](requirements.html): The uri that identifies the requirements 5224* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5225* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5226* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5227* [StructureMap](structuremap.html): The uri that identifies the structure map 5228* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5229* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5230* [TestPlan](testplan.html): The uri that identifies the test plan 5231* [TestScript](testscript.html): The uri that identifies the test script 5232* [ValueSet](valueset.html): The uri that identifies the value set 5233</b><br> 5234 * Type: <b>uri</b><br> 5235 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5236 * </p> 5237 */ 5238 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 5239 public static final String SP_URL = "url"; 5240 /** 5241 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5242 * <p> 5243 * Description: <b>Multiple Resources: 5244 5245* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 5246* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 5247* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 5248* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 5249* [Citation](citation.html): The uri that identifies the citation 5250* [CodeSystem](codesystem.html): The uri that identifies the code system 5251* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 5252* [ConceptMap](conceptmap.html): The URI that identifies the concept map 5253* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 5254* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 5255* [Evidence](evidence.html): The uri that identifies the evidence 5256* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 5257* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 5258* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 5259* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 5260* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 5261* [Library](library.html): The uri that identifies the library 5262* [Measure](measure.html): The uri that identifies the measure 5263* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 5264* [NamingSystem](namingsystem.html): The uri that identifies the naming system 5265* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 5266* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 5267* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 5268* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 5269* [Requirements](requirements.html): The uri that identifies the requirements 5270* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 5271* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 5272* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 5273* [StructureMap](structuremap.html): The uri that identifies the structure map 5274* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 5275* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 5276* [TestPlan](testplan.html): The uri that identifies the test plan 5277* [TestScript](testscript.html): The uri that identifies the test script 5278* [ValueSet](valueset.html): The uri that identifies the value set 5279</b><br> 5280 * Type: <b>uri</b><br> 5281 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 5282 * </p> 5283 */ 5284 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5285 5286 5287} 5288