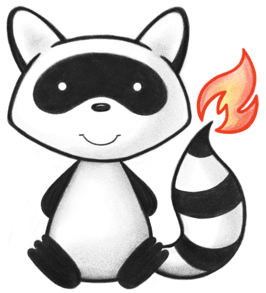
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The EvidenceVariable resource describes an element that knowledge (Evidence) is about. 052 */ 053@ResourceDef(name="EvidenceVariable", profile="http://hl7.org/fhir/StructureDefinition/EvidenceVariable") 054public class EvidenceVariable extends MetadataResource { 055 056 public enum CharacteristicCombination { 057 /** 058 * Combine characteristics with AND. 059 */ 060 ALLOF, 061 /** 062 * Combine characteristics with OR. 063 */ 064 ANYOF, 065 /** 066 * Meet at least the threshold number of characteristics for definition. 067 */ 068 ATLEAST, 069 /** 070 * Meet at most the threshold number of characteristics for definition. 071 */ 072 ATMOST, 073 /** 074 * Combine characteristics statistically. Use method to specify the statistical method. 075 */ 076 STATISTICAL, 077 /** 078 * Combine characteristics by addition of benefits and subtraction of harms. 079 */ 080 NETEFFECT, 081 /** 082 * Combine characteristics as a collection used as the dataset. 083 */ 084 DATASET, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static CharacteristicCombination fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("all-of".equals(codeString)) 093 return ALLOF; 094 if ("any-of".equals(codeString)) 095 return ANYOF; 096 if ("at-least".equals(codeString)) 097 return ATLEAST; 098 if ("at-most".equals(codeString)) 099 return ATMOST; 100 if ("statistical".equals(codeString)) 101 return STATISTICAL; 102 if ("net-effect".equals(codeString)) 103 return NETEFFECT; 104 if ("dataset".equals(codeString)) 105 return DATASET; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown CharacteristicCombination code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case ALLOF: return "all-of"; 114 case ANYOF: return "any-of"; 115 case ATLEAST: return "at-least"; 116 case ATMOST: return "at-most"; 117 case STATISTICAL: return "statistical"; 118 case NETEFFECT: return "net-effect"; 119 case DATASET: return "dataset"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case ALLOF: return "http://hl7.org/fhir/characteristic-combination"; 127 case ANYOF: return "http://hl7.org/fhir/characteristic-combination"; 128 case ATLEAST: return "http://hl7.org/fhir/characteristic-combination"; 129 case ATMOST: return "http://hl7.org/fhir/characteristic-combination"; 130 case STATISTICAL: return "http://hl7.org/fhir/characteristic-combination"; 131 case NETEFFECT: return "http://hl7.org/fhir/characteristic-combination"; 132 case DATASET: return "http://hl7.org/fhir/characteristic-combination"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case ALLOF: return "Combine characteristics with AND."; 140 case ANYOF: return "Combine characteristics with OR."; 141 case ATLEAST: return "Meet at least the threshold number of characteristics for definition."; 142 case ATMOST: return "Meet at most the threshold number of characteristics for definition."; 143 case STATISTICAL: return "Combine characteristics statistically. Use method to specify the statistical method."; 144 case NETEFFECT: return "Combine characteristics by addition of benefits and subtraction of harms."; 145 case DATASET: return "Combine characteristics as a collection used as the dataset."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case ALLOF: return "All of"; 153 case ANYOF: return "Any of"; 154 case ATLEAST: return "At least"; 155 case ATMOST: return "At most"; 156 case STATISTICAL: return "Statistical"; 157 case NETEFFECT: return "Net effect"; 158 case DATASET: return "Dataset"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class CharacteristicCombinationEnumFactory implements EnumFactory<CharacteristicCombination> { 166 public CharacteristicCombination fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("all-of".equals(codeString)) 171 return CharacteristicCombination.ALLOF; 172 if ("any-of".equals(codeString)) 173 return CharacteristicCombination.ANYOF; 174 if ("at-least".equals(codeString)) 175 return CharacteristicCombination.ATLEAST; 176 if ("at-most".equals(codeString)) 177 return CharacteristicCombination.ATMOST; 178 if ("statistical".equals(codeString)) 179 return CharacteristicCombination.STATISTICAL; 180 if ("net-effect".equals(codeString)) 181 return CharacteristicCombination.NETEFFECT; 182 if ("dataset".equals(codeString)) 183 return CharacteristicCombination.DATASET; 184 throw new IllegalArgumentException("Unknown CharacteristicCombination code '"+codeString+"'"); 185 } 186 public Enumeration<CharacteristicCombination> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.NULL, code); 194 if ("all-of".equals(codeString)) 195 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ALLOF, code); 196 if ("any-of".equals(codeString)) 197 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ANYOF, code); 198 if ("at-least".equals(codeString)) 199 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ATLEAST, code); 200 if ("at-most".equals(codeString)) 201 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ATMOST, code); 202 if ("statistical".equals(codeString)) 203 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.STATISTICAL, code); 204 if ("net-effect".equals(codeString)) 205 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.NETEFFECT, code); 206 if ("dataset".equals(codeString)) 207 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.DATASET, code); 208 throw new FHIRException("Unknown CharacteristicCombination code '"+codeString+"'"); 209 } 210 public String toCode(CharacteristicCombination code) { 211 if (code == CharacteristicCombination.ALLOF) 212 return "all-of"; 213 if (code == CharacteristicCombination.ANYOF) 214 return "any-of"; 215 if (code == CharacteristicCombination.ATLEAST) 216 return "at-least"; 217 if (code == CharacteristicCombination.ATMOST) 218 return "at-most"; 219 if (code == CharacteristicCombination.STATISTICAL) 220 return "statistical"; 221 if (code == CharacteristicCombination.NETEFFECT) 222 return "net-effect"; 223 if (code == CharacteristicCombination.DATASET) 224 return "dataset"; 225 return "?"; 226 } 227 public String toSystem(CharacteristicCombination code) { 228 return code.getSystem(); 229 } 230 } 231 232 @Block() 233 public static class EvidenceVariableCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 234 /** 235 * Label used for when a characteristic refers to another characteristic. 236 */ 237 @Child(name = "linkId", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=false) 238 @Description(shortDefinition="Label for internal linking", formalDefinition="Label used for when a characteristic refers to another characteristic." ) 239 protected IdType linkId; 240 241 /** 242 * A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user. 243 */ 244 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 245 @Description(shortDefinition="Natural language description of the characteristic", formalDefinition="A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user." ) 246 protected MarkdownType description; 247 248 /** 249 * A human-readable string to clarify or explain concepts about the characteristic. 250 */ 251 @Child(name = "note", type = {Annotation.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 252 @Description(shortDefinition="Used for footnotes or explanatory notes", formalDefinition="A human-readable string to clarify or explain concepts about the characteristic." ) 253 protected List<Annotation> note; 254 255 /** 256 * When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion. 257 */ 258 @Child(name = "exclude", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 259 @Description(shortDefinition="Whether the characteristic is an inclusion criterion or exclusion criterion", formalDefinition="When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion." ) 260 protected BooleanType exclude; 261 262 /** 263 * Defines the characteristic using a Reference. 264 */ 265 @Child(name = "definitionReference", type = {EvidenceVariable.class, Group.class, Evidence.class}, order=5, min=0, max=1, modifier=false, summary=true) 266 @Description(shortDefinition="Defines the characteristic (without using type and value) by a Reference", formalDefinition="Defines the characteristic using a Reference." ) 267 protected Reference definitionReference; 268 269 /** 270 * Defines the characteristic using Canonical. 271 */ 272 @Child(name = "definitionCanonical", type = {CanonicalType.class}, order=6, min=0, max=1, modifier=false, summary=true) 273 @Description(shortDefinition="Defines the characteristic (without using type and value) by a Canonical", formalDefinition="Defines the characteristic using Canonical." ) 274 protected CanonicalType definitionCanonical; 275 276 /** 277 * Defines the characteristic using CodeableConcept. 278 */ 279 @Child(name = "definitionCodeableConcept", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 280 @Description(shortDefinition="Defines the characteristic (without using type and value) by a CodeableConcept", formalDefinition="Defines the characteristic using CodeableConcept." ) 281 protected CodeableConcept definitionCodeableConcept; 282 283 /** 284 * Defines the characteristic using Expression. 285 */ 286 @Child(name = "definitionExpression", type = {Expression.class}, order=8, min=0, max=1, modifier=false, summary=true) 287 @Description(shortDefinition="Defines the characteristic (without using type and value) by an expression", formalDefinition="Defines the characteristic using Expression." ) 288 protected Expression definitionExpression; 289 290 /** 291 * Defines the characteristic using id. 292 */ 293 @Child(name = "definitionId", type = {IdType.class}, order=9, min=0, max=1, modifier=false, summary=true) 294 @Description(shortDefinition="Defines the characteristic (without using type and value) by an id", formalDefinition="Defines the characteristic using id." ) 295 protected IdType definitionId; 296 297 /** 298 * Defines the characteristic using both a type and value[x] elements. 299 */ 300 @Child(name = "definitionByTypeAndValue", type = {}, order=10, min=0, max=1, modifier=false, summary=true) 301 @Description(shortDefinition="Defines the characteristic using type and value", formalDefinition="Defines the characteristic using both a type and value[x] elements." ) 302 protected EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent definitionByTypeAndValue; 303 304 /** 305 * Defines the characteristic as a combination of two or more characteristics. 306 */ 307 @Child(name = "definitionByCombination", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 308 @Description(shortDefinition="Used to specify how two or more characteristics are combined", formalDefinition="Defines the characteristic as a combination of two or more characteristics." ) 309 protected EvidenceVariableCharacteristicDefinitionByCombinationComponent definitionByCombination; 310 311 /** 312 * Number of occurrences meeting the characteristic. 313 */ 314 @Child(name = "instances", type = {Quantity.class, Range.class}, order=12, min=0, max=1, modifier=false, summary=false) 315 @Description(shortDefinition="Number of occurrences meeting the characteristic", formalDefinition="Number of occurrences meeting the characteristic." ) 316 protected DataType instances; 317 318 /** 319 * Length of time in which the characteristic is met. 320 */ 321 @Child(name = "duration", type = {Quantity.class, Range.class}, order=13, min=0, max=1, modifier=false, summary=false) 322 @Description(shortDefinition="Length of time in which the characteristic is met", formalDefinition="Length of time in which the characteristic is met." ) 323 protected DataType duration; 324 325 /** 326 * Timing in which the characteristic is determined. 327 */ 328 @Child(name = "timeFromEvent", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 329 @Description(shortDefinition="Timing in which the characteristic is determined", formalDefinition="Timing in which the characteristic is determined." ) 330 protected List<EvidenceVariableCharacteristicTimeFromEventComponent> timeFromEvent; 331 332 private static final long serialVersionUID = 1658283810L; 333 334 /** 335 * Constructor 336 */ 337 public EvidenceVariableCharacteristicComponent() { 338 super(); 339 } 340 341 /** 342 * @return {@link #linkId} (Label used for when a characteristic refers to another characteristic.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 343 */ 344 public IdType getLinkIdElement() { 345 if (this.linkId == null) 346 if (Configuration.errorOnAutoCreate()) 347 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.linkId"); 348 else if (Configuration.doAutoCreate()) 349 this.linkId = new IdType(); // bb 350 return this.linkId; 351 } 352 353 public boolean hasLinkIdElement() { 354 return this.linkId != null && !this.linkId.isEmpty(); 355 } 356 357 public boolean hasLinkId() { 358 return this.linkId != null && !this.linkId.isEmpty(); 359 } 360 361 /** 362 * @param value {@link #linkId} (Label used for when a characteristic refers to another characteristic.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 363 */ 364 public EvidenceVariableCharacteristicComponent setLinkIdElement(IdType value) { 365 this.linkId = value; 366 return this; 367 } 368 369 /** 370 * @return Label used for when a characteristic refers to another characteristic. 371 */ 372 public String getLinkId() { 373 return this.linkId == null ? null : this.linkId.getValue(); 374 } 375 376 /** 377 * @param value Label used for when a characteristic refers to another characteristic. 378 */ 379 public EvidenceVariableCharacteristicComponent setLinkId(String value) { 380 if (Utilities.noString(value)) 381 this.linkId = null; 382 else { 383 if (this.linkId == null) 384 this.linkId = new IdType(); 385 this.linkId.setValue(value); 386 } 387 return this; 388 } 389 390 /** 391 * @return {@link #description} (A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 392 */ 393 public MarkdownType getDescriptionElement() { 394 if (this.description == null) 395 if (Configuration.errorOnAutoCreate()) 396 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.description"); 397 else if (Configuration.doAutoCreate()) 398 this.description = new MarkdownType(); // bb 399 return this.description; 400 } 401 402 public boolean hasDescriptionElement() { 403 return this.description != null && !this.description.isEmpty(); 404 } 405 406 public boolean hasDescription() { 407 return this.description != null && !this.description.isEmpty(); 408 } 409 410 /** 411 * @param value {@link #description} (A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 412 */ 413 public EvidenceVariableCharacteristicComponent setDescriptionElement(MarkdownType value) { 414 this.description = value; 415 return this; 416 } 417 418 /** 419 * @return A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user. 420 */ 421 public String getDescription() { 422 return this.description == null ? null : this.description.getValue(); 423 } 424 425 /** 426 * @param value A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user. 427 */ 428 public EvidenceVariableCharacteristicComponent setDescription(String value) { 429 if (Utilities.noString(value)) 430 this.description = null; 431 else { 432 if (this.description == null) 433 this.description = new MarkdownType(); 434 this.description.setValue(value); 435 } 436 return this; 437 } 438 439 /** 440 * @return {@link #note} (A human-readable string to clarify or explain concepts about the characteristic.) 441 */ 442 public List<Annotation> getNote() { 443 if (this.note == null) 444 this.note = new ArrayList<Annotation>(); 445 return this.note; 446 } 447 448 /** 449 * @return Returns a reference to <code>this</code> for easy method chaining 450 */ 451 public EvidenceVariableCharacteristicComponent setNote(List<Annotation> theNote) { 452 this.note = theNote; 453 return this; 454 } 455 456 public boolean hasNote() { 457 if (this.note == null) 458 return false; 459 for (Annotation item : this.note) 460 if (!item.isEmpty()) 461 return true; 462 return false; 463 } 464 465 public Annotation addNote() { //3 466 Annotation t = new Annotation(); 467 if (this.note == null) 468 this.note = new ArrayList<Annotation>(); 469 this.note.add(t); 470 return t; 471 } 472 473 public EvidenceVariableCharacteristicComponent addNote(Annotation t) { //3 474 if (t == null) 475 return this; 476 if (this.note == null) 477 this.note = new ArrayList<Annotation>(); 478 this.note.add(t); 479 return this; 480 } 481 482 /** 483 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 484 */ 485 public Annotation getNoteFirstRep() { 486 if (getNote().isEmpty()) { 487 addNote(); 488 } 489 return getNote().get(0); 490 } 491 492 /** 493 * @return {@link #exclude} (When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 494 */ 495 public BooleanType getExcludeElement() { 496 if (this.exclude == null) 497 if (Configuration.errorOnAutoCreate()) 498 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.exclude"); 499 else if (Configuration.doAutoCreate()) 500 this.exclude = new BooleanType(); // bb 501 return this.exclude; 502 } 503 504 public boolean hasExcludeElement() { 505 return this.exclude != null && !this.exclude.isEmpty(); 506 } 507 508 public boolean hasExclude() { 509 return this.exclude != null && !this.exclude.isEmpty(); 510 } 511 512 /** 513 * @param value {@link #exclude} (When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 514 */ 515 public EvidenceVariableCharacteristicComponent setExcludeElement(BooleanType value) { 516 this.exclude = value; 517 return this; 518 } 519 520 /** 521 * @return When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion. 522 */ 523 public boolean getExclude() { 524 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 525 } 526 527 /** 528 * @param value When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion. 529 */ 530 public EvidenceVariableCharacteristicComponent setExclude(boolean value) { 531 if (this.exclude == null) 532 this.exclude = new BooleanType(); 533 this.exclude.setValue(value); 534 return this; 535 } 536 537 /** 538 * @return {@link #definitionReference} (Defines the characteristic using a Reference.) 539 */ 540 public Reference getDefinitionReference() { 541 if (this.definitionReference == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionReference"); 544 else if (Configuration.doAutoCreate()) 545 this.definitionReference = new Reference(); // cc 546 return this.definitionReference; 547 } 548 549 public boolean hasDefinitionReference() { 550 return this.definitionReference != null && !this.definitionReference.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #definitionReference} (Defines the characteristic using a Reference.) 555 */ 556 public EvidenceVariableCharacteristicComponent setDefinitionReference(Reference value) { 557 this.definitionReference = value; 558 return this; 559 } 560 561 /** 562 * @return {@link #definitionCanonical} (Defines the characteristic using Canonical.). This is the underlying object with id, value and extensions. The accessor "getDefinitionCanonical" gives direct access to the value 563 */ 564 public CanonicalType getDefinitionCanonicalElement() { 565 if (this.definitionCanonical == null) 566 if (Configuration.errorOnAutoCreate()) 567 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionCanonical"); 568 else if (Configuration.doAutoCreate()) 569 this.definitionCanonical = new CanonicalType(); // bb 570 return this.definitionCanonical; 571 } 572 573 public boolean hasDefinitionCanonicalElement() { 574 return this.definitionCanonical != null && !this.definitionCanonical.isEmpty(); 575 } 576 577 public boolean hasDefinitionCanonical() { 578 return this.definitionCanonical != null && !this.definitionCanonical.isEmpty(); 579 } 580 581 /** 582 * @param value {@link #definitionCanonical} (Defines the characteristic using Canonical.). This is the underlying object with id, value and extensions. The accessor "getDefinitionCanonical" gives direct access to the value 583 */ 584 public EvidenceVariableCharacteristicComponent setDefinitionCanonicalElement(CanonicalType value) { 585 this.definitionCanonical = value; 586 return this; 587 } 588 589 /** 590 * @return Defines the characteristic using Canonical. 591 */ 592 public String getDefinitionCanonical() { 593 return this.definitionCanonical == null ? null : this.definitionCanonical.getValue(); 594 } 595 596 /** 597 * @param value Defines the characteristic using Canonical. 598 */ 599 public EvidenceVariableCharacteristicComponent setDefinitionCanonical(String value) { 600 if (Utilities.noString(value)) 601 this.definitionCanonical = null; 602 else { 603 if (this.definitionCanonical == null) 604 this.definitionCanonical = new CanonicalType(); 605 this.definitionCanonical.setValue(value); 606 } 607 return this; 608 } 609 610 /** 611 * @return {@link #definitionCodeableConcept} (Defines the characteristic using CodeableConcept.) 612 */ 613 public CodeableConcept getDefinitionCodeableConcept() { 614 if (this.definitionCodeableConcept == null) 615 if (Configuration.errorOnAutoCreate()) 616 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionCodeableConcept"); 617 else if (Configuration.doAutoCreate()) 618 this.definitionCodeableConcept = new CodeableConcept(); // cc 619 return this.definitionCodeableConcept; 620 } 621 622 public boolean hasDefinitionCodeableConcept() { 623 return this.definitionCodeableConcept != null && !this.definitionCodeableConcept.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #definitionCodeableConcept} (Defines the characteristic using CodeableConcept.) 628 */ 629 public EvidenceVariableCharacteristicComponent setDefinitionCodeableConcept(CodeableConcept value) { 630 this.definitionCodeableConcept = value; 631 return this; 632 } 633 634 /** 635 * @return {@link #definitionExpression} (Defines the characteristic using Expression.) 636 */ 637 public Expression getDefinitionExpression() { 638 if (this.definitionExpression == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionExpression"); 641 else if (Configuration.doAutoCreate()) 642 this.definitionExpression = new Expression(); // cc 643 return this.definitionExpression; 644 } 645 646 public boolean hasDefinitionExpression() { 647 return this.definitionExpression != null && !this.definitionExpression.isEmpty(); 648 } 649 650 /** 651 * @param value {@link #definitionExpression} (Defines the characteristic using Expression.) 652 */ 653 public EvidenceVariableCharacteristicComponent setDefinitionExpression(Expression value) { 654 this.definitionExpression = value; 655 return this; 656 } 657 658 /** 659 * @return {@link #definitionId} (Defines the characteristic using id.). This is the underlying object with id, value and extensions. The accessor "getDefinitionId" gives direct access to the value 660 */ 661 public IdType getDefinitionIdElement() { 662 if (this.definitionId == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionId"); 665 else if (Configuration.doAutoCreate()) 666 this.definitionId = new IdType(); // bb 667 return this.definitionId; 668 } 669 670 public boolean hasDefinitionIdElement() { 671 return this.definitionId != null && !this.definitionId.isEmpty(); 672 } 673 674 public boolean hasDefinitionId() { 675 return this.definitionId != null && !this.definitionId.isEmpty(); 676 } 677 678 /** 679 * @param value {@link #definitionId} (Defines the characteristic using id.). This is the underlying object with id, value and extensions. The accessor "getDefinitionId" gives direct access to the value 680 */ 681 public EvidenceVariableCharacteristicComponent setDefinitionIdElement(IdType value) { 682 this.definitionId = value; 683 return this; 684 } 685 686 /** 687 * @return Defines the characteristic using id. 688 */ 689 public String getDefinitionId() { 690 return this.definitionId == null ? null : this.definitionId.getValue(); 691 } 692 693 /** 694 * @param value Defines the characteristic using id. 695 */ 696 public EvidenceVariableCharacteristicComponent setDefinitionId(String value) { 697 if (Utilities.noString(value)) 698 this.definitionId = null; 699 else { 700 if (this.definitionId == null) 701 this.definitionId = new IdType(); 702 this.definitionId.setValue(value); 703 } 704 return this; 705 } 706 707 /** 708 * @return {@link #definitionByTypeAndValue} (Defines the characteristic using both a type and value[x] elements.) 709 */ 710 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent getDefinitionByTypeAndValue() { 711 if (this.definitionByTypeAndValue == null) 712 if (Configuration.errorOnAutoCreate()) 713 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionByTypeAndValue"); 714 else if (Configuration.doAutoCreate()) 715 this.definitionByTypeAndValue = new EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(); // cc 716 return this.definitionByTypeAndValue; 717 } 718 719 public boolean hasDefinitionByTypeAndValue() { 720 return this.definitionByTypeAndValue != null && !this.definitionByTypeAndValue.isEmpty(); 721 } 722 723 /** 724 * @param value {@link #definitionByTypeAndValue} (Defines the characteristic using both a type and value[x] elements.) 725 */ 726 public EvidenceVariableCharacteristicComponent setDefinitionByTypeAndValue(EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent value) { 727 this.definitionByTypeAndValue = value; 728 return this; 729 } 730 731 /** 732 * @return {@link #definitionByCombination} (Defines the characteristic as a combination of two or more characteristics.) 733 */ 734 public EvidenceVariableCharacteristicDefinitionByCombinationComponent getDefinitionByCombination() { 735 if (this.definitionByCombination == null) 736 if (Configuration.errorOnAutoCreate()) 737 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionByCombination"); 738 else if (Configuration.doAutoCreate()) 739 this.definitionByCombination = new EvidenceVariableCharacteristicDefinitionByCombinationComponent(); // cc 740 return this.definitionByCombination; 741 } 742 743 public boolean hasDefinitionByCombination() { 744 return this.definitionByCombination != null && !this.definitionByCombination.isEmpty(); 745 } 746 747 /** 748 * @param value {@link #definitionByCombination} (Defines the characteristic as a combination of two or more characteristics.) 749 */ 750 public EvidenceVariableCharacteristicComponent setDefinitionByCombination(EvidenceVariableCharacteristicDefinitionByCombinationComponent value) { 751 this.definitionByCombination = value; 752 return this; 753 } 754 755 /** 756 * @return {@link #instances} (Number of occurrences meeting the characteristic.) 757 */ 758 public DataType getInstances() { 759 return this.instances; 760 } 761 762 /** 763 * @return {@link #instances} (Number of occurrences meeting the characteristic.) 764 */ 765 public Quantity getInstancesQuantity() throws FHIRException { 766 if (this.instances == null) 767 this.instances = new Quantity(); 768 if (!(this.instances instanceof Quantity)) 769 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.instances.getClass().getName()+" was encountered"); 770 return (Quantity) this.instances; 771 } 772 773 public boolean hasInstancesQuantity() { 774 return this != null && this.instances instanceof Quantity; 775 } 776 777 /** 778 * @return {@link #instances} (Number of occurrences meeting the characteristic.) 779 */ 780 public Range getInstancesRange() throws FHIRException { 781 if (this.instances == null) 782 this.instances = new Range(); 783 if (!(this.instances instanceof Range)) 784 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.instances.getClass().getName()+" was encountered"); 785 return (Range) this.instances; 786 } 787 788 public boolean hasInstancesRange() { 789 return this != null && this.instances instanceof Range; 790 } 791 792 public boolean hasInstances() { 793 return this.instances != null && !this.instances.isEmpty(); 794 } 795 796 /** 797 * @param value {@link #instances} (Number of occurrences meeting the characteristic.) 798 */ 799 public EvidenceVariableCharacteristicComponent setInstances(DataType value) { 800 if (value != null && !(value instanceof Quantity || value instanceof Range)) 801 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.instances[x]: "+value.fhirType()); 802 this.instances = value; 803 return this; 804 } 805 806 /** 807 * @return {@link #duration} (Length of time in which the characteristic is met.) 808 */ 809 public DataType getDuration() { 810 return this.duration; 811 } 812 813 /** 814 * @return {@link #duration} (Length of time in which the characteristic is met.) 815 */ 816 public Quantity getDurationQuantity() throws FHIRException { 817 if (this.duration == null) 818 this.duration = new Quantity(); 819 if (!(this.duration instanceof Quantity)) 820 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.duration.getClass().getName()+" was encountered"); 821 return (Quantity) this.duration; 822 } 823 824 public boolean hasDurationQuantity() { 825 return this != null && this.duration instanceof Quantity; 826 } 827 828 /** 829 * @return {@link #duration} (Length of time in which the characteristic is met.) 830 */ 831 public Range getDurationRange() throws FHIRException { 832 if (this.duration == null) 833 this.duration = new Range(); 834 if (!(this.duration instanceof Range)) 835 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.duration.getClass().getName()+" was encountered"); 836 return (Range) this.duration; 837 } 838 839 public boolean hasDurationRange() { 840 return this != null && this.duration instanceof Range; 841 } 842 843 public boolean hasDuration() { 844 return this.duration != null && !this.duration.isEmpty(); 845 } 846 847 /** 848 * @param value {@link #duration} (Length of time in which the characteristic is met.) 849 */ 850 public EvidenceVariableCharacteristicComponent setDuration(DataType value) { 851 if (value != null && !(value instanceof Quantity || value instanceof Range)) 852 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.duration[x]: "+value.fhirType()); 853 this.duration = value; 854 return this; 855 } 856 857 /** 858 * @return {@link #timeFromEvent} (Timing in which the characteristic is determined.) 859 */ 860 public List<EvidenceVariableCharacteristicTimeFromEventComponent> getTimeFromEvent() { 861 if (this.timeFromEvent == null) 862 this.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 863 return this.timeFromEvent; 864 } 865 866 /** 867 * @return Returns a reference to <code>this</code> for easy method chaining 868 */ 869 public EvidenceVariableCharacteristicComponent setTimeFromEvent(List<EvidenceVariableCharacteristicTimeFromEventComponent> theTimeFromEvent) { 870 this.timeFromEvent = theTimeFromEvent; 871 return this; 872 } 873 874 public boolean hasTimeFromEvent() { 875 if (this.timeFromEvent == null) 876 return false; 877 for (EvidenceVariableCharacteristicTimeFromEventComponent item : this.timeFromEvent) 878 if (!item.isEmpty()) 879 return true; 880 return false; 881 } 882 883 public EvidenceVariableCharacteristicTimeFromEventComponent addTimeFromEvent() { //3 884 EvidenceVariableCharacteristicTimeFromEventComponent t = new EvidenceVariableCharacteristicTimeFromEventComponent(); 885 if (this.timeFromEvent == null) 886 this.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 887 this.timeFromEvent.add(t); 888 return t; 889 } 890 891 public EvidenceVariableCharacteristicComponent addTimeFromEvent(EvidenceVariableCharacteristicTimeFromEventComponent t) { //3 892 if (t == null) 893 return this; 894 if (this.timeFromEvent == null) 895 this.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 896 this.timeFromEvent.add(t); 897 return this; 898 } 899 900 /** 901 * @return The first repetition of repeating field {@link #timeFromEvent}, creating it if it does not already exist {3} 902 */ 903 public EvidenceVariableCharacteristicTimeFromEventComponent getTimeFromEventFirstRep() { 904 if (getTimeFromEvent().isEmpty()) { 905 addTimeFromEvent(); 906 } 907 return getTimeFromEvent().get(0); 908 } 909 910 protected void listChildren(List<Property> children) { 911 super.listChildren(children); 912 children.add(new Property("linkId", "id", "Label used for when a characteristic refers to another characteristic.", 0, 1, linkId)); 913 children.add(new Property("description", "markdown", "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 0, 1, description)); 914 children.add(new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the characteristic.", 0, java.lang.Integer.MAX_VALUE, note)); 915 children.add(new Property("exclude", "boolean", "When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.", 0, 1, exclude)); 916 children.add(new Property("definitionReference", "Reference(EvidenceVariable|Group|Evidence)", "Defines the characteristic using a Reference.", 0, 1, definitionReference)); 917 children.add(new Property("definitionCanonical", "canonical(EvidenceVariable|Evidence)", "Defines the characteristic using Canonical.", 0, 1, definitionCanonical)); 918 children.add(new Property("definitionCodeableConcept", "CodeableConcept", "Defines the characteristic using CodeableConcept.", 0, 1, definitionCodeableConcept)); 919 children.add(new Property("definitionExpression", "Expression", "Defines the characteristic using Expression.", 0, 1, definitionExpression)); 920 children.add(new Property("definitionId", "id", "Defines the characteristic using id.", 0, 1, definitionId)); 921 children.add(new Property("definitionByTypeAndValue", "", "Defines the characteristic using both a type and value[x] elements.", 0, 1, definitionByTypeAndValue)); 922 children.add(new Property("definitionByCombination", "", "Defines the characteristic as a combination of two or more characteristics.", 0, 1, definitionByCombination)); 923 children.add(new Property("instances[x]", "Quantity|Range", "Number of occurrences meeting the characteristic.", 0, 1, instances)); 924 children.add(new Property("duration[x]", "Quantity|Range", "Length of time in which the characteristic is met.", 0, 1, duration)); 925 children.add(new Property("timeFromEvent", "", "Timing in which the characteristic is determined.", 0, java.lang.Integer.MAX_VALUE, timeFromEvent)); 926 } 927 928 @Override 929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 930 switch (_hash) { 931 case -1102667083: /*linkId*/ return new Property("linkId", "id", "Label used for when a characteristic refers to another characteristic.", 0, 1, linkId); 932 case -1724546052: /*description*/ return new Property("description", "markdown", "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 0, 1, description); 933 case 3387378: /*note*/ return new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the characteristic.", 0, java.lang.Integer.MAX_VALUE, note); 934 case -1321148966: /*exclude*/ return new Property("exclude", "boolean", "When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.", 0, 1, exclude); 935 case -820021448: /*definitionReference*/ return new Property("definitionReference", "Reference(EvidenceVariable|Group|Evidence)", "Defines the characteristic using a Reference.", 0, 1, definitionReference); 936 case 933485793: /*definitionCanonical*/ return new Property("definitionCanonical", "canonical(EvidenceVariable|Evidence)", "Defines the characteristic using Canonical.", 0, 1, definitionCanonical); 937 case -1446002226: /*definitionCodeableConcept*/ return new Property("definitionCodeableConcept", "CodeableConcept", "Defines the characteristic using CodeableConcept.", 0, 1, definitionCodeableConcept); 938 case 1463703627: /*definitionExpression*/ return new Property("definitionExpression", "Expression", "Defines the characteristic using Expression.", 0, 1, definitionExpression); 939 case 101791182: /*definitionId*/ return new Property("definitionId", "id", "Defines the characteristic using id.", 0, 1, definitionId); 940 case -164357794: /*definitionByTypeAndValue*/ return new Property("definitionByTypeAndValue", "", "Defines the characteristic using both a type and value[x] elements.", 0, 1, definitionByTypeAndValue); 941 case -2043280539: /*definitionByCombination*/ return new Property("definitionByCombination", "", "Defines the characteristic as a combination of two or more characteristics.", 0, 1, definitionByCombination); 942 case -736760510: /*instances[x]*/ return new Property("instances[x]", "Quantity|Range", "Number of occurrences meeting the characteristic.", 0, 1, instances); 943 case 29097598: /*instances*/ return new Property("instances[x]", "Quantity|Range", "Number of occurrences meeting the characteristic.", 0, 1, instances); 944 case -1378916055: /*instancesQuantity*/ return new Property("instances[x]", "Quantity", "Number of occurrences meeting the characteristic.", 0, 1, instances); 945 case 633776479: /*instancesRange*/ return new Property("instances[x]", "Range", "Number of occurrences meeting the characteristic.", 0, 1, instances); 946 case -478069140: /*duration[x]*/ return new Property("duration[x]", "Quantity|Range", "Length of time in which the characteristic is met.", 0, 1, duration); 947 case -1992012396: /*duration*/ return new Property("duration[x]", "Quantity|Range", "Length of time in which the characteristic is met.", 0, 1, duration); 948 case 159007295: /*durationQuantity*/ return new Property("duration[x]", "Quantity", "Length of time in which the characteristic is met.", 0, 1, duration); 949 case 128079881: /*durationRange*/ return new Property("duration[x]", "Range", "Length of time in which the characteristic is met.", 0, 1, duration); 950 case 2087274691: /*timeFromEvent*/ return new Property("timeFromEvent", "", "Timing in which the characteristic is determined.", 0, java.lang.Integer.MAX_VALUE, timeFromEvent); 951 default: return super.getNamedProperty(_hash, _name, _checkValid); 952 } 953 954 } 955 956 @Override 957 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 958 switch (hash) { 959 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // IdType 960 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 961 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 962 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // BooleanType 963 case -820021448: /*definitionReference*/ return this.definitionReference == null ? new Base[0] : new Base[] {this.definitionReference}; // Reference 964 case 933485793: /*definitionCanonical*/ return this.definitionCanonical == null ? new Base[0] : new Base[] {this.definitionCanonical}; // CanonicalType 965 case -1446002226: /*definitionCodeableConcept*/ return this.definitionCodeableConcept == null ? new Base[0] : new Base[] {this.definitionCodeableConcept}; // CodeableConcept 966 case 1463703627: /*definitionExpression*/ return this.definitionExpression == null ? new Base[0] : new Base[] {this.definitionExpression}; // Expression 967 case 101791182: /*definitionId*/ return this.definitionId == null ? new Base[0] : new Base[] {this.definitionId}; // IdType 968 case -164357794: /*definitionByTypeAndValue*/ return this.definitionByTypeAndValue == null ? new Base[0] : new Base[] {this.definitionByTypeAndValue}; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 969 case -2043280539: /*definitionByCombination*/ return this.definitionByCombination == null ? new Base[0] : new Base[] {this.definitionByCombination}; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 970 case 29097598: /*instances*/ return this.instances == null ? new Base[0] : new Base[] {this.instances}; // DataType 971 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // DataType 972 case 2087274691: /*timeFromEvent*/ return this.timeFromEvent == null ? new Base[0] : this.timeFromEvent.toArray(new Base[this.timeFromEvent.size()]); // EvidenceVariableCharacteristicTimeFromEventComponent 973 default: return super.getProperty(hash, name, checkValid); 974 } 975 976 } 977 978 @Override 979 public Base setProperty(int hash, String name, Base value) throws FHIRException { 980 switch (hash) { 981 case -1102667083: // linkId 982 this.linkId = TypeConvertor.castToId(value); // IdType 983 return value; 984 case -1724546052: // description 985 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 986 return value; 987 case 3387378: // note 988 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 989 return value; 990 case -1321148966: // exclude 991 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 992 return value; 993 case -820021448: // definitionReference 994 this.definitionReference = TypeConvertor.castToReference(value); // Reference 995 return value; 996 case 933485793: // definitionCanonical 997 this.definitionCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 998 return value; 999 case -1446002226: // definitionCodeableConcept 1000 this.definitionCodeableConcept = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1001 return value; 1002 case 1463703627: // definitionExpression 1003 this.definitionExpression = TypeConvertor.castToExpression(value); // Expression 1004 return value; 1005 case 101791182: // definitionId 1006 this.definitionId = TypeConvertor.castToId(value); // IdType 1007 return value; 1008 case -164357794: // definitionByTypeAndValue 1009 this.definitionByTypeAndValue = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) value; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 1010 return value; 1011 case -2043280539: // definitionByCombination 1012 this.definitionByCombination = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) value; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 1013 return value; 1014 case 29097598: // instances 1015 this.instances = TypeConvertor.castToType(value); // DataType 1016 return value; 1017 case -1992012396: // duration 1018 this.duration = TypeConvertor.castToType(value); // DataType 1019 return value; 1020 case 2087274691: // timeFromEvent 1021 this.getTimeFromEvent().add((EvidenceVariableCharacteristicTimeFromEventComponent) value); // EvidenceVariableCharacteristicTimeFromEventComponent 1022 return value; 1023 default: return super.setProperty(hash, name, value); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base setProperty(String name, Base value) throws FHIRException { 1030 if (name.equals("linkId")) { 1031 this.linkId = TypeConvertor.castToId(value); // IdType 1032 } else if (name.equals("description")) { 1033 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1034 } else if (name.equals("note")) { 1035 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1036 } else if (name.equals("exclude")) { 1037 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 1038 } else if (name.equals("definitionReference")) { 1039 this.definitionReference = TypeConvertor.castToReference(value); // Reference 1040 } else if (name.equals("definitionCanonical")) { 1041 this.definitionCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 1042 } else if (name.equals("definitionCodeableConcept")) { 1043 this.definitionCodeableConcept = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1044 } else if (name.equals("definitionExpression")) { 1045 this.definitionExpression = TypeConvertor.castToExpression(value); // Expression 1046 } else if (name.equals("definitionId")) { 1047 this.definitionId = TypeConvertor.castToId(value); // IdType 1048 } else if (name.equals("definitionByTypeAndValue")) { 1049 this.definitionByTypeAndValue = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) value; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 1050 } else if (name.equals("definitionByCombination")) { 1051 this.definitionByCombination = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) value; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 1052 } else if (name.equals("instances[x]")) { 1053 this.instances = TypeConvertor.castToType(value); // DataType 1054 } else if (name.equals("duration[x]")) { 1055 this.duration = TypeConvertor.castToType(value); // DataType 1056 } else if (name.equals("timeFromEvent")) { 1057 this.getTimeFromEvent().add((EvidenceVariableCharacteristicTimeFromEventComponent) value); 1058 } else 1059 return super.setProperty(name, value); 1060 return value; 1061 } 1062 1063 @Override 1064 public void removeChild(String name, Base value) throws FHIRException { 1065 if (name.equals("linkId")) { 1066 this.linkId = null; 1067 } else if (name.equals("description")) { 1068 this.description = null; 1069 } else if (name.equals("note")) { 1070 this.getNote().remove(value); 1071 } else if (name.equals("exclude")) { 1072 this.exclude = null; 1073 } else if (name.equals("definitionReference")) { 1074 this.definitionReference = null; 1075 } else if (name.equals("definitionCanonical")) { 1076 this.definitionCanonical = null; 1077 } else if (name.equals("definitionCodeableConcept")) { 1078 this.definitionCodeableConcept = null; 1079 } else if (name.equals("definitionExpression")) { 1080 this.definitionExpression = null; 1081 } else if (name.equals("definitionId")) { 1082 this.definitionId = null; 1083 } else if (name.equals("definitionByTypeAndValue")) { 1084 this.definitionByTypeAndValue = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) value; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 1085 } else if (name.equals("definitionByCombination")) { 1086 this.definitionByCombination = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) value; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 1087 } else if (name.equals("instances[x]")) { 1088 this.instances = null; 1089 } else if (name.equals("duration[x]")) { 1090 this.duration = null; 1091 } else if (name.equals("timeFromEvent")) { 1092 this.getTimeFromEvent().remove((EvidenceVariableCharacteristicTimeFromEventComponent) value); 1093 } else 1094 super.removeChild(name, value); 1095 1096 } 1097 1098 @Override 1099 public Base makeProperty(int hash, String name) throws FHIRException { 1100 switch (hash) { 1101 case -1102667083: return getLinkIdElement(); 1102 case -1724546052: return getDescriptionElement(); 1103 case 3387378: return addNote(); 1104 case -1321148966: return getExcludeElement(); 1105 case -820021448: return getDefinitionReference(); 1106 case 933485793: return getDefinitionCanonicalElement(); 1107 case -1446002226: return getDefinitionCodeableConcept(); 1108 case 1463703627: return getDefinitionExpression(); 1109 case 101791182: return getDefinitionIdElement(); 1110 case -164357794: return getDefinitionByTypeAndValue(); 1111 case -2043280539: return getDefinitionByCombination(); 1112 case -736760510: return getInstances(); 1113 case 29097598: return getInstances(); 1114 case -478069140: return getDuration(); 1115 case -1992012396: return getDuration(); 1116 case 2087274691: return addTimeFromEvent(); 1117 default: return super.makeProperty(hash, name); 1118 } 1119 1120 } 1121 1122 @Override 1123 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1124 switch (hash) { 1125 case -1102667083: /*linkId*/ return new String[] {"id"}; 1126 case -1724546052: /*description*/ return new String[] {"markdown"}; 1127 case 3387378: /*note*/ return new String[] {"Annotation"}; 1128 case -1321148966: /*exclude*/ return new String[] {"boolean"}; 1129 case -820021448: /*definitionReference*/ return new String[] {"Reference"}; 1130 case 933485793: /*definitionCanonical*/ return new String[] {"canonical"}; 1131 case -1446002226: /*definitionCodeableConcept*/ return new String[] {"CodeableConcept"}; 1132 case 1463703627: /*definitionExpression*/ return new String[] {"Expression"}; 1133 case 101791182: /*definitionId*/ return new String[] {"id"}; 1134 case -164357794: /*definitionByTypeAndValue*/ return new String[] {}; 1135 case -2043280539: /*definitionByCombination*/ return new String[] {}; 1136 case 29097598: /*instances*/ return new String[] {"Quantity", "Range"}; 1137 case -1992012396: /*duration*/ return new String[] {"Quantity", "Range"}; 1138 case 2087274691: /*timeFromEvent*/ return new String[] {}; 1139 default: return super.getTypesForProperty(hash, name); 1140 } 1141 1142 } 1143 1144 @Override 1145 public Base addChild(String name) throws FHIRException { 1146 if (name.equals("linkId")) { 1147 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.linkId"); 1148 } 1149 else if (name.equals("description")) { 1150 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.description"); 1151 } 1152 else if (name.equals("note")) { 1153 return addNote(); 1154 } 1155 else if (name.equals("exclude")) { 1156 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.exclude"); 1157 } 1158 else if (name.equals("definitionReference")) { 1159 this.definitionReference = new Reference(); 1160 return this.definitionReference; 1161 } 1162 else if (name.equals("definitionCanonical")) { 1163 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionCanonical"); 1164 } 1165 else if (name.equals("definitionCodeableConcept")) { 1166 this.definitionCodeableConcept = new CodeableConcept(); 1167 return this.definitionCodeableConcept; 1168 } 1169 else if (name.equals("definitionExpression")) { 1170 this.definitionExpression = new Expression(); 1171 return this.definitionExpression; 1172 } 1173 else if (name.equals("definitionId")) { 1174 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionId"); 1175 } 1176 else if (name.equals("definitionByTypeAndValue")) { 1177 this.definitionByTypeAndValue = new EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(); 1178 return this.definitionByTypeAndValue; 1179 } 1180 else if (name.equals("definitionByCombination")) { 1181 this.definitionByCombination = new EvidenceVariableCharacteristicDefinitionByCombinationComponent(); 1182 return this.definitionByCombination; 1183 } 1184 else if (name.equals("instancesQuantity")) { 1185 this.instances = new Quantity(); 1186 return this.instances; 1187 } 1188 else if (name.equals("instancesRange")) { 1189 this.instances = new Range(); 1190 return this.instances; 1191 } 1192 else if (name.equals("durationQuantity")) { 1193 this.duration = new Quantity(); 1194 return this.duration; 1195 } 1196 else if (name.equals("durationRange")) { 1197 this.duration = new Range(); 1198 return this.duration; 1199 } 1200 else if (name.equals("timeFromEvent")) { 1201 return addTimeFromEvent(); 1202 } 1203 else 1204 return super.addChild(name); 1205 } 1206 1207 public EvidenceVariableCharacteristicComponent copy() { 1208 EvidenceVariableCharacteristicComponent dst = new EvidenceVariableCharacteristicComponent(); 1209 copyValues(dst); 1210 return dst; 1211 } 1212 1213 public void copyValues(EvidenceVariableCharacteristicComponent dst) { 1214 super.copyValues(dst); 1215 dst.linkId = linkId == null ? null : linkId.copy(); 1216 dst.description = description == null ? null : description.copy(); 1217 if (note != null) { 1218 dst.note = new ArrayList<Annotation>(); 1219 for (Annotation i : note) 1220 dst.note.add(i.copy()); 1221 }; 1222 dst.exclude = exclude == null ? null : exclude.copy(); 1223 dst.definitionReference = definitionReference == null ? null : definitionReference.copy(); 1224 dst.definitionCanonical = definitionCanonical == null ? null : definitionCanonical.copy(); 1225 dst.definitionCodeableConcept = definitionCodeableConcept == null ? null : definitionCodeableConcept.copy(); 1226 dst.definitionExpression = definitionExpression == null ? null : definitionExpression.copy(); 1227 dst.definitionId = definitionId == null ? null : definitionId.copy(); 1228 dst.definitionByTypeAndValue = definitionByTypeAndValue == null ? null : definitionByTypeAndValue.copy(); 1229 dst.definitionByCombination = definitionByCombination == null ? null : definitionByCombination.copy(); 1230 dst.instances = instances == null ? null : instances.copy(); 1231 dst.duration = duration == null ? null : duration.copy(); 1232 if (timeFromEvent != null) { 1233 dst.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 1234 for (EvidenceVariableCharacteristicTimeFromEventComponent i : timeFromEvent) 1235 dst.timeFromEvent.add(i.copy()); 1236 }; 1237 } 1238 1239 @Override 1240 public boolean equalsDeep(Base other_) { 1241 if (!super.equalsDeep(other_)) 1242 return false; 1243 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1244 return false; 1245 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1246 return compareDeep(linkId, o.linkId, true) && compareDeep(description, o.description, true) && compareDeep(note, o.note, true) 1247 && compareDeep(exclude, o.exclude, true) && compareDeep(definitionReference, o.definitionReference, true) 1248 && compareDeep(definitionCanonical, o.definitionCanonical, true) && compareDeep(definitionCodeableConcept, o.definitionCodeableConcept, true) 1249 && compareDeep(definitionExpression, o.definitionExpression, true) && compareDeep(definitionId, o.definitionId, true) 1250 && compareDeep(definitionByTypeAndValue, o.definitionByTypeAndValue, true) && compareDeep(definitionByCombination, o.definitionByCombination, true) 1251 && compareDeep(instances, o.instances, true) && compareDeep(duration, o.duration, true) && compareDeep(timeFromEvent, o.timeFromEvent, true) 1252 ; 1253 } 1254 1255 @Override 1256 public boolean equalsShallow(Base other_) { 1257 if (!super.equalsShallow(other_)) 1258 return false; 1259 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1260 return false; 1261 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1262 return compareValues(linkId, o.linkId, true) && compareValues(description, o.description, true) && compareValues(exclude, o.exclude, true) 1263 && compareValues(definitionCanonical, o.definitionCanonical, true) && compareValues(definitionId, o.definitionId, true) 1264 ; 1265 } 1266 1267 public boolean isEmpty() { 1268 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, description, note 1269 , exclude, definitionReference, definitionCanonical, definitionCodeableConcept, definitionExpression 1270 , definitionId, definitionByTypeAndValue, definitionByCombination, instances, duration 1271 , timeFromEvent); 1272 } 1273 1274 public String fhirType() { 1275 return "EvidenceVariable.characteristic"; 1276 1277 } 1278 1279 } 1280 1281 @Block() 1282 public static class EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent extends BackboneElement implements IBaseBackboneElement { 1283 /** 1284 * Used to express the type of characteristic. 1285 */ 1286 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1287 @Description(shortDefinition="Expresses the type of characteristic", formalDefinition="Used to express the type of characteristic." ) 1288 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/usage-context-type") 1289 protected CodeableConcept type; 1290 1291 /** 1292 * Method for how the characteristic value was determined. 1293 */ 1294 @Child(name = "method", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1295 @Description(shortDefinition="Method for how the characteristic value was determined", formalDefinition="Method for how the characteristic value was determined." ) 1296 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-method") 1297 protected List<CodeableConcept> method; 1298 1299 /** 1300 * Device used for determining characteristic. 1301 */ 1302 @Child(name = "device", type = {Device.class, DeviceMetric.class}, order=3, min=0, max=1, modifier=false, summary=false) 1303 @Description(shortDefinition="Device used for determining characteristic", formalDefinition="Device used for determining characteristic." ) 1304 protected Reference device; 1305 1306 /** 1307 * Defines the characteristic when paired with characteristic.type. 1308 */ 1309 @Child(name = "value", type = {CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, Reference.class, IdType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1310 @Description(shortDefinition="Defines the characteristic when coupled with characteristic.type", formalDefinition="Defines the characteristic when paired with characteristic.type." ) 1311 protected DataType value; 1312 1313 /** 1314 * Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero. 1315 */ 1316 @Child(name = "offset", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1317 @Description(shortDefinition="Reference point for valueQuantity or valueRange", formalDefinition="Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero." ) 1318 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/characteristic-offset") 1319 protected CodeableConcept offset; 1320 1321 private static final long serialVersionUID = -498341653L; 1322 1323 /** 1324 * Constructor 1325 */ 1326 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent() { 1327 super(); 1328 } 1329 1330 /** 1331 * Constructor 1332 */ 1333 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(CodeableConcept type, DataType value) { 1334 super(); 1335 this.setType(type); 1336 this.setValue(value); 1337 } 1338 1339 /** 1340 * @return {@link #type} (Used to express the type of characteristic.) 1341 */ 1342 public CodeableConcept getType() { 1343 if (this.type == null) 1344 if (Configuration.errorOnAutoCreate()) 1345 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent.type"); 1346 else if (Configuration.doAutoCreate()) 1347 this.type = new CodeableConcept(); // cc 1348 return this.type; 1349 } 1350 1351 public boolean hasType() { 1352 return this.type != null && !this.type.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #type} (Used to express the type of characteristic.) 1357 */ 1358 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setType(CodeableConcept value) { 1359 this.type = value; 1360 return this; 1361 } 1362 1363 /** 1364 * @return {@link #method} (Method for how the characteristic value was determined.) 1365 */ 1366 public List<CodeableConcept> getMethod() { 1367 if (this.method == null) 1368 this.method = new ArrayList<CodeableConcept>(); 1369 return this.method; 1370 } 1371 1372 /** 1373 * @return Returns a reference to <code>this</code> for easy method chaining 1374 */ 1375 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setMethod(List<CodeableConcept> theMethod) { 1376 this.method = theMethod; 1377 return this; 1378 } 1379 1380 public boolean hasMethod() { 1381 if (this.method == null) 1382 return false; 1383 for (CodeableConcept item : this.method) 1384 if (!item.isEmpty()) 1385 return true; 1386 return false; 1387 } 1388 1389 public CodeableConcept addMethod() { //3 1390 CodeableConcept t = new CodeableConcept(); 1391 if (this.method == null) 1392 this.method = new ArrayList<CodeableConcept>(); 1393 this.method.add(t); 1394 return t; 1395 } 1396 1397 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent addMethod(CodeableConcept t) { //3 1398 if (t == null) 1399 return this; 1400 if (this.method == null) 1401 this.method = new ArrayList<CodeableConcept>(); 1402 this.method.add(t); 1403 return this; 1404 } 1405 1406 /** 1407 * @return The first repetition of repeating field {@link #method}, creating it if it does not already exist {3} 1408 */ 1409 public CodeableConcept getMethodFirstRep() { 1410 if (getMethod().isEmpty()) { 1411 addMethod(); 1412 } 1413 return getMethod().get(0); 1414 } 1415 1416 /** 1417 * @return {@link #device} (Device used for determining characteristic.) 1418 */ 1419 public Reference getDevice() { 1420 if (this.device == null) 1421 if (Configuration.errorOnAutoCreate()) 1422 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent.device"); 1423 else if (Configuration.doAutoCreate()) 1424 this.device = new Reference(); // cc 1425 return this.device; 1426 } 1427 1428 public boolean hasDevice() { 1429 return this.device != null && !this.device.isEmpty(); 1430 } 1431 1432 /** 1433 * @param value {@link #device} (Device used for determining characteristic.) 1434 */ 1435 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setDevice(Reference value) { 1436 this.device = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1442 */ 1443 public DataType getValue() { 1444 return this.value; 1445 } 1446 1447 /** 1448 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1449 */ 1450 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1451 if (this.value == null) 1452 this.value = new CodeableConcept(); 1453 if (!(this.value instanceof CodeableConcept)) 1454 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1455 return (CodeableConcept) this.value; 1456 } 1457 1458 public boolean hasValueCodeableConcept() { 1459 return this != null && this.value instanceof CodeableConcept; 1460 } 1461 1462 /** 1463 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1464 */ 1465 public BooleanType getValueBooleanType() throws FHIRException { 1466 if (this.value == null) 1467 this.value = new BooleanType(); 1468 if (!(this.value instanceof BooleanType)) 1469 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1470 return (BooleanType) this.value; 1471 } 1472 1473 public boolean hasValueBooleanType() { 1474 return this != null && this.value instanceof BooleanType; 1475 } 1476 1477 /** 1478 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1479 */ 1480 public Quantity getValueQuantity() throws FHIRException { 1481 if (this.value == null) 1482 this.value = new Quantity(); 1483 if (!(this.value instanceof Quantity)) 1484 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1485 return (Quantity) this.value; 1486 } 1487 1488 public boolean hasValueQuantity() { 1489 return this != null && this.value instanceof Quantity; 1490 } 1491 1492 /** 1493 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1494 */ 1495 public Range getValueRange() throws FHIRException { 1496 if (this.value == null) 1497 this.value = new Range(); 1498 if (!(this.value instanceof Range)) 1499 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1500 return (Range) this.value; 1501 } 1502 1503 public boolean hasValueRange() { 1504 return this != null && this.value instanceof Range; 1505 } 1506 1507 /** 1508 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1509 */ 1510 public Reference getValueReference() throws FHIRException { 1511 if (this.value == null) 1512 this.value = new Reference(); 1513 if (!(this.value instanceof Reference)) 1514 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1515 return (Reference) this.value; 1516 } 1517 1518 public boolean hasValueReference() { 1519 return this != null && this.value instanceof Reference; 1520 } 1521 1522 /** 1523 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1524 */ 1525 public IdType getValueIdType() throws FHIRException { 1526 if (this.value == null) 1527 this.value = new IdType(); 1528 if (!(this.value instanceof IdType)) 1529 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 1530 return (IdType) this.value; 1531 } 1532 1533 public boolean hasValueIdType() { 1534 return this != null && this.value instanceof IdType; 1535 } 1536 1537 public boolean hasValue() { 1538 return this.value != null && !this.value.isEmpty(); 1539 } 1540 1541 /** 1542 * @param value {@link #value} (Defines the characteristic when paired with characteristic.type.) 1543 */ 1544 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setValue(DataType value) { 1545 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range || value instanceof Reference || value instanceof IdType)) 1546 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.definitionByTypeAndValue.value[x]: "+value.fhirType()); 1547 this.value = value; 1548 return this; 1549 } 1550 1551 /** 1552 * @return {@link #offset} (Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.) 1553 */ 1554 public CodeableConcept getOffset() { 1555 if (this.offset == null) 1556 if (Configuration.errorOnAutoCreate()) 1557 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent.offset"); 1558 else if (Configuration.doAutoCreate()) 1559 this.offset = new CodeableConcept(); // cc 1560 return this.offset; 1561 } 1562 1563 public boolean hasOffset() { 1564 return this.offset != null && !this.offset.isEmpty(); 1565 } 1566 1567 /** 1568 * @param value {@link #offset} (Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.) 1569 */ 1570 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setOffset(CodeableConcept value) { 1571 this.offset = value; 1572 return this; 1573 } 1574 1575 protected void listChildren(List<Property> children) { 1576 super.listChildren(children); 1577 children.add(new Property("type", "CodeableConcept", "Used to express the type of characteristic.", 0, 1, type)); 1578 children.add(new Property("method", "CodeableConcept", "Method for how the characteristic value was determined.", 0, java.lang.Integer.MAX_VALUE, method)); 1579 children.add(new Property("device", "Reference(Device|DeviceMetric)", "Device used for determining characteristic.", 0, 1, device)); 1580 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference|id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value)); 1581 children.add(new Property("offset", "CodeableConcept", "Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.", 0, 1, offset)); 1582 } 1583 1584 @Override 1585 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1586 switch (_hash) { 1587 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Used to express the type of characteristic.", 0, 1, type); 1588 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Method for how the characteristic value was determined.", 0, java.lang.Integer.MAX_VALUE, method); 1589 case -1335157162: /*device*/ return new Property("device", "Reference(Device|DeviceMetric)", "Device used for determining characteristic.", 0, 1, device); 1590 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference|id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1591 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference|id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1592 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1593 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1594 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1595 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1596 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1597 case 231604844: /*valueId*/ return new Property("value[x]", "id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1598 case -1019779949: /*offset*/ return new Property("offset", "CodeableConcept", "Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.", 0, 1, offset); 1599 default: return super.getNamedProperty(_hash, _name, _checkValid); 1600 } 1601 1602 } 1603 1604 @Override 1605 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1606 switch (hash) { 1607 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1608 case -1077554975: /*method*/ return this.method == null ? new Base[0] : this.method.toArray(new Base[this.method.size()]); // CodeableConcept 1609 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 1610 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1611 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // CodeableConcept 1612 default: return super.getProperty(hash, name, checkValid); 1613 } 1614 1615 } 1616 1617 @Override 1618 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1619 switch (hash) { 1620 case 3575610: // type 1621 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1622 return value; 1623 case -1077554975: // method 1624 this.getMethod().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1625 return value; 1626 case -1335157162: // device 1627 this.device = TypeConvertor.castToReference(value); // Reference 1628 return value; 1629 case 111972721: // value 1630 this.value = TypeConvertor.castToType(value); // DataType 1631 return value; 1632 case -1019779949: // offset 1633 this.offset = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1634 return value; 1635 default: return super.setProperty(hash, name, value); 1636 } 1637 1638 } 1639 1640 @Override 1641 public Base setProperty(String name, Base value) throws FHIRException { 1642 if (name.equals("type")) { 1643 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1644 } else if (name.equals("method")) { 1645 this.getMethod().add(TypeConvertor.castToCodeableConcept(value)); 1646 } else if (name.equals("device")) { 1647 this.device = TypeConvertor.castToReference(value); // Reference 1648 } else if (name.equals("value[x]")) { 1649 this.value = TypeConvertor.castToType(value); // DataType 1650 } else if (name.equals("offset")) { 1651 this.offset = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1652 } else 1653 return super.setProperty(name, value); 1654 return value; 1655 } 1656 1657 @Override 1658 public void removeChild(String name, Base value) throws FHIRException { 1659 if (name.equals("type")) { 1660 this.type = null; 1661 } else if (name.equals("method")) { 1662 this.getMethod().remove(value); 1663 } else if (name.equals("device")) { 1664 this.device = null; 1665 } else if (name.equals("value[x]")) { 1666 this.value = null; 1667 } else if (name.equals("offset")) { 1668 this.offset = null; 1669 } else 1670 super.removeChild(name, value); 1671 1672 } 1673 1674 @Override 1675 public Base makeProperty(int hash, String name) throws FHIRException { 1676 switch (hash) { 1677 case 3575610: return getType(); 1678 case -1077554975: return addMethod(); 1679 case -1335157162: return getDevice(); 1680 case -1410166417: return getValue(); 1681 case 111972721: return getValue(); 1682 case -1019779949: return getOffset(); 1683 default: return super.makeProperty(hash, name); 1684 } 1685 1686 } 1687 1688 @Override 1689 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1690 switch (hash) { 1691 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1692 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1693 case -1335157162: /*device*/ return new String[] {"Reference"}; 1694 case 111972721: /*value*/ return new String[] {"CodeableConcept", "boolean", "Quantity", "Range", "Reference", "id"}; 1695 case -1019779949: /*offset*/ return new String[] {"CodeableConcept"}; 1696 default: return super.getTypesForProperty(hash, name); 1697 } 1698 1699 } 1700 1701 @Override 1702 public Base addChild(String name) throws FHIRException { 1703 if (name.equals("type")) { 1704 this.type = new CodeableConcept(); 1705 return this.type; 1706 } 1707 else if (name.equals("method")) { 1708 return addMethod(); 1709 } 1710 else if (name.equals("device")) { 1711 this.device = new Reference(); 1712 return this.device; 1713 } 1714 else if (name.equals("valueCodeableConcept")) { 1715 this.value = new CodeableConcept(); 1716 return this.value; 1717 } 1718 else if (name.equals("valueBoolean")) { 1719 this.value = new BooleanType(); 1720 return this.value; 1721 } 1722 else if (name.equals("valueQuantity")) { 1723 this.value = new Quantity(); 1724 return this.value; 1725 } 1726 else if (name.equals("valueRange")) { 1727 this.value = new Range(); 1728 return this.value; 1729 } 1730 else if (name.equals("valueReference")) { 1731 this.value = new Reference(); 1732 return this.value; 1733 } 1734 else if (name.equals("valueId")) { 1735 this.value = new IdType(); 1736 return this.value; 1737 } 1738 else if (name.equals("offset")) { 1739 this.offset = new CodeableConcept(); 1740 return this.offset; 1741 } 1742 else 1743 return super.addChild(name); 1744 } 1745 1746 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent copy() { 1747 EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent dst = new EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(); 1748 copyValues(dst); 1749 return dst; 1750 } 1751 1752 public void copyValues(EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent dst) { 1753 super.copyValues(dst); 1754 dst.type = type == null ? null : type.copy(); 1755 if (method != null) { 1756 dst.method = new ArrayList<CodeableConcept>(); 1757 for (CodeableConcept i : method) 1758 dst.method.add(i.copy()); 1759 }; 1760 dst.device = device == null ? null : device.copy(); 1761 dst.value = value == null ? null : value.copy(); 1762 dst.offset = offset == null ? null : offset.copy(); 1763 } 1764 1765 @Override 1766 public boolean equalsDeep(Base other_) { 1767 if (!super.equalsDeep(other_)) 1768 return false; 1769 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent)) 1770 return false; 1771 EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent o = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) other_; 1772 return compareDeep(type, o.type, true) && compareDeep(method, o.method, true) && compareDeep(device, o.device, true) 1773 && compareDeep(value, o.value, true) && compareDeep(offset, o.offset, true); 1774 } 1775 1776 @Override 1777 public boolean equalsShallow(Base other_) { 1778 if (!super.equalsShallow(other_)) 1779 return false; 1780 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent)) 1781 return false; 1782 EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent o = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) other_; 1783 return true; 1784 } 1785 1786 public boolean isEmpty() { 1787 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, method, device, value 1788 , offset); 1789 } 1790 1791 public String fhirType() { 1792 return "EvidenceVariable.characteristic.definitionByTypeAndValue"; 1793 1794 } 1795 1796 } 1797 1798 @Block() 1799 public static class EvidenceVariableCharacteristicDefinitionByCombinationComponent extends BackboneElement implements IBaseBackboneElement { 1800 /** 1801 * Used to specify if two or more characteristics are combined with OR or AND. 1802 */ 1803 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1804 @Description(shortDefinition="all-of | any-of | at-least | at-most | statistical | net-effect | dataset", formalDefinition="Used to specify if two or more characteristics are combined with OR or AND." ) 1805 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/characteristic-combination") 1806 protected Enumeration<CharacteristicCombination> code; 1807 1808 /** 1809 * Provides the value of "n" when "at-least" or "at-most" codes are used. 1810 */ 1811 @Child(name = "threshold", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1812 @Description(shortDefinition="Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used", formalDefinition="Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used." ) 1813 protected PositiveIntType threshold; 1814 1815 /** 1816 * A defining factor of the characteristic. 1817 */ 1818 @Child(name = "characteristic", type = {EvidenceVariableCharacteristicComponent.class}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1819 @Description(shortDefinition="A defining factor of the characteristic", formalDefinition="A defining factor of the characteristic." ) 1820 protected List<EvidenceVariableCharacteristicComponent> characteristic; 1821 1822 private static final long serialVersionUID = -2053118515L; 1823 1824 /** 1825 * Constructor 1826 */ 1827 public EvidenceVariableCharacteristicDefinitionByCombinationComponent() { 1828 super(); 1829 } 1830 1831 /** 1832 * Constructor 1833 */ 1834 public EvidenceVariableCharacteristicDefinitionByCombinationComponent(CharacteristicCombination code, EvidenceVariableCharacteristicComponent characteristic) { 1835 super(); 1836 this.setCode(code); 1837 this.addCharacteristic(characteristic); 1838 } 1839 1840 /** 1841 * @return {@link #code} (Used to specify if two or more characteristics are combined with OR or AND.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1842 */ 1843 public Enumeration<CharacteristicCombination> getCodeElement() { 1844 if (this.code == null) 1845 if (Configuration.errorOnAutoCreate()) 1846 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByCombinationComponent.code"); 1847 else if (Configuration.doAutoCreate()) 1848 this.code = new Enumeration<CharacteristicCombination>(new CharacteristicCombinationEnumFactory()); // bb 1849 return this.code; 1850 } 1851 1852 public boolean hasCodeElement() { 1853 return this.code != null && !this.code.isEmpty(); 1854 } 1855 1856 public boolean hasCode() { 1857 return this.code != null && !this.code.isEmpty(); 1858 } 1859 1860 /** 1861 * @param value {@link #code} (Used to specify if two or more characteristics are combined with OR or AND.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1862 */ 1863 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setCodeElement(Enumeration<CharacteristicCombination> value) { 1864 this.code = value; 1865 return this; 1866 } 1867 1868 /** 1869 * @return Used to specify if two or more characteristics are combined with OR or AND. 1870 */ 1871 public CharacteristicCombination getCode() { 1872 return this.code == null ? null : this.code.getValue(); 1873 } 1874 1875 /** 1876 * @param value Used to specify if two or more characteristics are combined with OR or AND. 1877 */ 1878 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setCode(CharacteristicCombination value) { 1879 if (this.code == null) 1880 this.code = new Enumeration<CharacteristicCombination>(new CharacteristicCombinationEnumFactory()); 1881 this.code.setValue(value); 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #threshold} (Provides the value of "n" when "at-least" or "at-most" codes are used.). This is the underlying object with id, value and extensions. The accessor "getThreshold" gives direct access to the value 1887 */ 1888 public PositiveIntType getThresholdElement() { 1889 if (this.threshold == null) 1890 if (Configuration.errorOnAutoCreate()) 1891 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByCombinationComponent.threshold"); 1892 else if (Configuration.doAutoCreate()) 1893 this.threshold = new PositiveIntType(); // bb 1894 return this.threshold; 1895 } 1896 1897 public boolean hasThresholdElement() { 1898 return this.threshold != null && !this.threshold.isEmpty(); 1899 } 1900 1901 public boolean hasThreshold() { 1902 return this.threshold != null && !this.threshold.isEmpty(); 1903 } 1904 1905 /** 1906 * @param value {@link #threshold} (Provides the value of "n" when "at-least" or "at-most" codes are used.). This is the underlying object with id, value and extensions. The accessor "getThreshold" gives direct access to the value 1907 */ 1908 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setThresholdElement(PositiveIntType value) { 1909 this.threshold = value; 1910 return this; 1911 } 1912 1913 /** 1914 * @return Provides the value of "n" when "at-least" or "at-most" codes are used. 1915 */ 1916 public int getThreshold() { 1917 return this.threshold == null || this.threshold.isEmpty() ? 0 : this.threshold.getValue(); 1918 } 1919 1920 /** 1921 * @param value Provides the value of "n" when "at-least" or "at-most" codes are used. 1922 */ 1923 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setThreshold(int value) { 1924 if (this.threshold == null) 1925 this.threshold = new PositiveIntType(); 1926 this.threshold.setValue(value); 1927 return this; 1928 } 1929 1930 /** 1931 * @return {@link #characteristic} (A defining factor of the characteristic.) 1932 */ 1933 public List<EvidenceVariableCharacteristicComponent> getCharacteristic() { 1934 if (this.characteristic == null) 1935 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 1936 return this.characteristic; 1937 } 1938 1939 /** 1940 * @return Returns a reference to <code>this</code> for easy method chaining 1941 */ 1942 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setCharacteristic(List<EvidenceVariableCharacteristicComponent> theCharacteristic) { 1943 this.characteristic = theCharacteristic; 1944 return this; 1945 } 1946 1947 public boolean hasCharacteristic() { 1948 if (this.characteristic == null) 1949 return false; 1950 for (EvidenceVariableCharacteristicComponent item : this.characteristic) 1951 if (!item.isEmpty()) 1952 return true; 1953 return false; 1954 } 1955 1956 public EvidenceVariableCharacteristicComponent addCharacteristic() { //3 1957 EvidenceVariableCharacteristicComponent t = new EvidenceVariableCharacteristicComponent(); 1958 if (this.characteristic == null) 1959 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 1960 this.characteristic.add(t); 1961 return t; 1962 } 1963 1964 public EvidenceVariableCharacteristicDefinitionByCombinationComponent addCharacteristic(EvidenceVariableCharacteristicComponent t) { //3 1965 if (t == null) 1966 return this; 1967 if (this.characteristic == null) 1968 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 1969 this.characteristic.add(t); 1970 return this; 1971 } 1972 1973 /** 1974 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 1975 */ 1976 public EvidenceVariableCharacteristicComponent getCharacteristicFirstRep() { 1977 if (getCharacteristic().isEmpty()) { 1978 addCharacteristic(); 1979 } 1980 return getCharacteristic().get(0); 1981 } 1982 1983 protected void listChildren(List<Property> children) { 1984 super.listChildren(children); 1985 children.add(new Property("code", "code", "Used to specify if two or more characteristics are combined with OR or AND.", 0, 1, code)); 1986 children.add(new Property("threshold", "positiveInt", "Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used.", 0, 1, threshold)); 1987 children.add(new Property("characteristic", "@EvidenceVariable.characteristic", "A defining factor of the characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 1988 } 1989 1990 @Override 1991 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1992 switch (_hash) { 1993 case 3059181: /*code*/ return new Property("code", "code", "Used to specify if two or more characteristics are combined with OR or AND.", 0, 1, code); 1994 case -1545477013: /*threshold*/ return new Property("threshold", "positiveInt", "Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used.", 0, 1, threshold); 1995 case 366313883: /*characteristic*/ return new Property("characteristic", "@EvidenceVariable.characteristic", "A defining factor of the characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic); 1996 default: return super.getNamedProperty(_hash, _name, _checkValid); 1997 } 1998 1999 } 2000 2001 @Override 2002 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2003 switch (hash) { 2004 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<CharacteristicCombination> 2005 case -1545477013: /*threshold*/ return this.threshold == null ? new Base[0] : new Base[] {this.threshold}; // PositiveIntType 2006 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceVariableCharacteristicComponent 2007 default: return super.getProperty(hash, name, checkValid); 2008 } 2009 2010 } 2011 2012 @Override 2013 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2014 switch (hash) { 2015 case 3059181: // code 2016 value = new CharacteristicCombinationEnumFactory().fromType(TypeConvertor.castToCode(value)); 2017 this.code = (Enumeration) value; // Enumeration<CharacteristicCombination> 2018 return value; 2019 case -1545477013: // threshold 2020 this.threshold = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2021 return value; 2022 case 366313883: // characteristic 2023 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); // EvidenceVariableCharacteristicComponent 2024 return value; 2025 default: return super.setProperty(hash, name, value); 2026 } 2027 2028 } 2029 2030 @Override 2031 public Base setProperty(String name, Base value) throws FHIRException { 2032 if (name.equals("code")) { 2033 value = new CharacteristicCombinationEnumFactory().fromType(TypeConvertor.castToCode(value)); 2034 this.code = (Enumeration) value; // Enumeration<CharacteristicCombination> 2035 } else if (name.equals("threshold")) { 2036 this.threshold = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2037 } else if (name.equals("characteristic")) { 2038 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); 2039 } else 2040 return super.setProperty(name, value); 2041 return value; 2042 } 2043 2044 @Override 2045 public void removeChild(String name, Base value) throws FHIRException { 2046 if (name.equals("code")) { 2047 value = new CharacteristicCombinationEnumFactory().fromType(TypeConvertor.castToCode(value)); 2048 this.code = (Enumeration) value; // Enumeration<CharacteristicCombination> 2049 } else if (name.equals("threshold")) { 2050 this.threshold = null; 2051 } else if (name.equals("characteristic")) { 2052 this.getCharacteristic().remove((EvidenceVariableCharacteristicComponent) value); 2053 } else 2054 super.removeChild(name, value); 2055 2056 } 2057 2058 @Override 2059 public Base makeProperty(int hash, String name) throws FHIRException { 2060 switch (hash) { 2061 case 3059181: return getCodeElement(); 2062 case -1545477013: return getThresholdElement(); 2063 case 366313883: return addCharacteristic(); 2064 default: return super.makeProperty(hash, name); 2065 } 2066 2067 } 2068 2069 @Override 2070 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2071 switch (hash) { 2072 case 3059181: /*code*/ return new String[] {"code"}; 2073 case -1545477013: /*threshold*/ return new String[] {"positiveInt"}; 2074 case 366313883: /*characteristic*/ return new String[] {"@EvidenceVariable.characteristic"}; 2075 default: return super.getTypesForProperty(hash, name); 2076 } 2077 2078 } 2079 2080 @Override 2081 public Base addChild(String name) throws FHIRException { 2082 if (name.equals("code")) { 2083 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionByCombination.code"); 2084 } 2085 else if (name.equals("threshold")) { 2086 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionByCombination.threshold"); 2087 } 2088 else if (name.equals("characteristic")) { 2089 return addCharacteristic(); 2090 } 2091 else 2092 return super.addChild(name); 2093 } 2094 2095 public EvidenceVariableCharacteristicDefinitionByCombinationComponent copy() { 2096 EvidenceVariableCharacteristicDefinitionByCombinationComponent dst = new EvidenceVariableCharacteristicDefinitionByCombinationComponent(); 2097 copyValues(dst); 2098 return dst; 2099 } 2100 2101 public void copyValues(EvidenceVariableCharacteristicDefinitionByCombinationComponent dst) { 2102 super.copyValues(dst); 2103 dst.code = code == null ? null : code.copy(); 2104 dst.threshold = threshold == null ? null : threshold.copy(); 2105 if (characteristic != null) { 2106 dst.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 2107 for (EvidenceVariableCharacteristicComponent i : characteristic) 2108 dst.characteristic.add(i.copy()); 2109 }; 2110 } 2111 2112 @Override 2113 public boolean equalsDeep(Base other_) { 2114 if (!super.equalsDeep(other_)) 2115 return false; 2116 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByCombinationComponent)) 2117 return false; 2118 EvidenceVariableCharacteristicDefinitionByCombinationComponent o = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) other_; 2119 return compareDeep(code, o.code, true) && compareDeep(threshold, o.threshold, true) && compareDeep(characteristic, o.characteristic, true) 2120 ; 2121 } 2122 2123 @Override 2124 public boolean equalsShallow(Base other_) { 2125 if (!super.equalsShallow(other_)) 2126 return false; 2127 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByCombinationComponent)) 2128 return false; 2129 EvidenceVariableCharacteristicDefinitionByCombinationComponent o = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) other_; 2130 return compareValues(code, o.code, true) && compareValues(threshold, o.threshold, true); 2131 } 2132 2133 public boolean isEmpty() { 2134 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, threshold, characteristic 2135 ); 2136 } 2137 2138 public String fhirType() { 2139 return "EvidenceVariable.characteristic.definitionByCombination"; 2140 2141 } 2142 2143 } 2144 2145 @Block() 2146 public static class EvidenceVariableCharacteristicTimeFromEventComponent extends BackboneElement implements IBaseBackboneElement { 2147 /** 2148 * Human readable description. 2149 */ 2150 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2151 @Description(shortDefinition="Human readable description", formalDefinition="Human readable description." ) 2152 protected MarkdownType description; 2153 2154 /** 2155 * A human-readable string to clarify or explain concepts about the timeFromEvent. 2156 */ 2157 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2158 @Description(shortDefinition="Used for footnotes or explanatory notes", formalDefinition="A human-readable string to clarify or explain concepts about the timeFromEvent." ) 2159 protected List<Annotation> note; 2160 2161 /** 2162 * The event used as a base point (reference point) in time. 2163 */ 2164 @Child(name = "event", type = {CodeableConcept.class, Reference.class, DateTimeType.class, IdType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2165 @Description(shortDefinition="The event used as a base point (reference point) in time", formalDefinition="The event used as a base point (reference point) in time." ) 2166 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-variable-event") 2167 protected DataType event; 2168 2169 /** 2170 * Used to express the observation at a defined amount of time before or after the event. 2171 */ 2172 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 2173 @Description(shortDefinition="Used to express the observation at a defined amount of time before or after the event", formalDefinition="Used to express the observation at a defined amount of time before or after the event." ) 2174 protected Quantity quantity; 2175 2176 /** 2177 * Used to express the observation within a period before and/or after the event. 2178 */ 2179 @Child(name = "range", type = {Range.class}, order=5, min=0, max=1, modifier=false, summary=false) 2180 @Description(shortDefinition="Used to express the observation within a period before and/or after the event", formalDefinition="Used to express the observation within a period before and/or after the event." ) 2181 protected Range range; 2182 2183 private static final long serialVersionUID = -1593501640L; 2184 2185 /** 2186 * Constructor 2187 */ 2188 public EvidenceVariableCharacteristicTimeFromEventComponent() { 2189 super(); 2190 } 2191 2192 /** 2193 * @return {@link #description} (Human readable description.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2194 */ 2195 public MarkdownType getDescriptionElement() { 2196 if (this.description == null) 2197 if (Configuration.errorOnAutoCreate()) 2198 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicTimeFromEventComponent.description"); 2199 else if (Configuration.doAutoCreate()) 2200 this.description = new MarkdownType(); // bb 2201 return this.description; 2202 } 2203 2204 public boolean hasDescriptionElement() { 2205 return this.description != null && !this.description.isEmpty(); 2206 } 2207 2208 public boolean hasDescription() { 2209 return this.description != null && !this.description.isEmpty(); 2210 } 2211 2212 /** 2213 * @param value {@link #description} (Human readable description.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2214 */ 2215 public EvidenceVariableCharacteristicTimeFromEventComponent setDescriptionElement(MarkdownType value) { 2216 this.description = value; 2217 return this; 2218 } 2219 2220 /** 2221 * @return Human readable description. 2222 */ 2223 public String getDescription() { 2224 return this.description == null ? null : this.description.getValue(); 2225 } 2226 2227 /** 2228 * @param value Human readable description. 2229 */ 2230 public EvidenceVariableCharacteristicTimeFromEventComponent setDescription(String value) { 2231 if (Utilities.noString(value)) 2232 this.description = null; 2233 else { 2234 if (this.description == null) 2235 this.description = new MarkdownType(); 2236 this.description.setValue(value); 2237 } 2238 return this; 2239 } 2240 2241 /** 2242 * @return {@link #note} (A human-readable string to clarify or explain concepts about the timeFromEvent.) 2243 */ 2244 public List<Annotation> getNote() { 2245 if (this.note == null) 2246 this.note = new ArrayList<Annotation>(); 2247 return this.note; 2248 } 2249 2250 /** 2251 * @return Returns a reference to <code>this</code> for easy method chaining 2252 */ 2253 public EvidenceVariableCharacteristicTimeFromEventComponent setNote(List<Annotation> theNote) { 2254 this.note = theNote; 2255 return this; 2256 } 2257 2258 public boolean hasNote() { 2259 if (this.note == null) 2260 return false; 2261 for (Annotation item : this.note) 2262 if (!item.isEmpty()) 2263 return true; 2264 return false; 2265 } 2266 2267 public Annotation addNote() { //3 2268 Annotation t = new Annotation(); 2269 if (this.note == null) 2270 this.note = new ArrayList<Annotation>(); 2271 this.note.add(t); 2272 return t; 2273 } 2274 2275 public EvidenceVariableCharacteristicTimeFromEventComponent addNote(Annotation t) { //3 2276 if (t == null) 2277 return this; 2278 if (this.note == null) 2279 this.note = new ArrayList<Annotation>(); 2280 this.note.add(t); 2281 return this; 2282 } 2283 2284 /** 2285 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2286 */ 2287 public Annotation getNoteFirstRep() { 2288 if (getNote().isEmpty()) { 2289 addNote(); 2290 } 2291 return getNote().get(0); 2292 } 2293 2294 /** 2295 * @return {@link #event} (The event used as a base point (reference point) in time.) 2296 */ 2297 public DataType getEvent() { 2298 return this.event; 2299 } 2300 2301 /** 2302 * @return {@link #event} (The event used as a base point (reference point) in time.) 2303 */ 2304 public CodeableConcept getEventCodeableConcept() throws FHIRException { 2305 if (this.event == null) 2306 this.event = new CodeableConcept(); 2307 if (!(this.event instanceof CodeableConcept)) 2308 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.event.getClass().getName()+" was encountered"); 2309 return (CodeableConcept) this.event; 2310 } 2311 2312 public boolean hasEventCodeableConcept() { 2313 return this != null && this.event instanceof CodeableConcept; 2314 } 2315 2316 /** 2317 * @return {@link #event} (The event used as a base point (reference point) in time.) 2318 */ 2319 public Reference getEventReference() throws FHIRException { 2320 if (this.event == null) 2321 this.event = new Reference(); 2322 if (!(this.event instanceof Reference)) 2323 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.event.getClass().getName()+" was encountered"); 2324 return (Reference) this.event; 2325 } 2326 2327 public boolean hasEventReference() { 2328 return this != null && this.event instanceof Reference; 2329 } 2330 2331 /** 2332 * @return {@link #event} (The event used as a base point (reference point) in time.) 2333 */ 2334 public DateTimeType getEventDateTimeType() throws FHIRException { 2335 if (this.event == null) 2336 this.event = new DateTimeType(); 2337 if (!(this.event instanceof DateTimeType)) 2338 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.event.getClass().getName()+" was encountered"); 2339 return (DateTimeType) this.event; 2340 } 2341 2342 public boolean hasEventDateTimeType() { 2343 return this != null && this.event instanceof DateTimeType; 2344 } 2345 2346 /** 2347 * @return {@link #event} (The event used as a base point (reference point) in time.) 2348 */ 2349 public IdType getEventIdType() throws FHIRException { 2350 if (this.event == null) 2351 this.event = new IdType(); 2352 if (!(this.event instanceof IdType)) 2353 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.event.getClass().getName()+" was encountered"); 2354 return (IdType) this.event; 2355 } 2356 2357 public boolean hasEventIdType() { 2358 return this != null && this.event instanceof IdType; 2359 } 2360 2361 public boolean hasEvent() { 2362 return this.event != null && !this.event.isEmpty(); 2363 } 2364 2365 /** 2366 * @param value {@link #event} (The event used as a base point (reference point) in time.) 2367 */ 2368 public EvidenceVariableCharacteristicTimeFromEventComponent setEvent(DataType value) { 2369 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference || value instanceof DateTimeType || value instanceof IdType)) 2370 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.timeFromEvent.event[x]: "+value.fhirType()); 2371 this.event = value; 2372 return this; 2373 } 2374 2375 /** 2376 * @return {@link #quantity} (Used to express the observation at a defined amount of time before or after the event.) 2377 */ 2378 public Quantity getQuantity() { 2379 if (this.quantity == null) 2380 if (Configuration.errorOnAutoCreate()) 2381 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicTimeFromEventComponent.quantity"); 2382 else if (Configuration.doAutoCreate()) 2383 this.quantity = new Quantity(); // cc 2384 return this.quantity; 2385 } 2386 2387 public boolean hasQuantity() { 2388 return this.quantity != null && !this.quantity.isEmpty(); 2389 } 2390 2391 /** 2392 * @param value {@link #quantity} (Used to express the observation at a defined amount of time before or after the event.) 2393 */ 2394 public EvidenceVariableCharacteristicTimeFromEventComponent setQuantity(Quantity value) { 2395 this.quantity = value; 2396 return this; 2397 } 2398 2399 /** 2400 * @return {@link #range} (Used to express the observation within a period before and/or after the event.) 2401 */ 2402 public Range getRange() { 2403 if (this.range == null) 2404 if (Configuration.errorOnAutoCreate()) 2405 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicTimeFromEventComponent.range"); 2406 else if (Configuration.doAutoCreate()) 2407 this.range = new Range(); // cc 2408 return this.range; 2409 } 2410 2411 public boolean hasRange() { 2412 return this.range != null && !this.range.isEmpty(); 2413 } 2414 2415 /** 2416 * @param value {@link #range} (Used to express the observation within a period before and/or after the event.) 2417 */ 2418 public EvidenceVariableCharacteristicTimeFromEventComponent setRange(Range value) { 2419 this.range = value; 2420 return this; 2421 } 2422 2423 protected void listChildren(List<Property> children) { 2424 super.listChildren(children); 2425 children.add(new Property("description", "markdown", "Human readable description.", 0, 1, description)); 2426 children.add(new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the timeFromEvent.", 0, java.lang.Integer.MAX_VALUE, note)); 2427 children.add(new Property("event[x]", "CodeableConcept|Reference|dateTime|id", "The event used as a base point (reference point) in time.", 0, 1, event)); 2428 children.add(new Property("quantity", "Quantity", "Used to express the observation at a defined amount of time before or after the event.", 0, 1, quantity)); 2429 children.add(new Property("range", "Range", "Used to express the observation within a period before and/or after the event.", 0, 1, range)); 2430 } 2431 2432 @Override 2433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2434 switch (_hash) { 2435 case -1724546052: /*description*/ return new Property("description", "markdown", "Human readable description.", 0, 1, description); 2436 case 3387378: /*note*/ return new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the timeFromEvent.", 0, java.lang.Integer.MAX_VALUE, note); 2437 case 278115238: /*event[x]*/ return new Property("event[x]", "CodeableConcept|Reference|dateTime|id", "The event used as a base point (reference point) in time.", 0, 1, event); 2438 case 96891546: /*event*/ return new Property("event[x]", "CodeableConcept|Reference|dateTime|id", "The event used as a base point (reference point) in time.", 0, 1, event); 2439 case 1464167847: /*eventCodeableConcept*/ return new Property("event[x]", "CodeableConcept", "The event used as a base point (reference point) in time.", 0, 1, event); 2440 case 30751185: /*eventReference*/ return new Property("event[x]", "Reference", "The event used as a base point (reference point) in time.", 0, 1, event); 2441 case -116077483: /*eventDateTime*/ return new Property("event[x]", "dateTime", "The event used as a base point (reference point) in time.", 0, 1, event); 2442 case -1376502443: /*eventId*/ return new Property("event[x]", "id", "The event used as a base point (reference point) in time.", 0, 1, event); 2443 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Used to express the observation at a defined amount of time before or after the event.", 0, 1, quantity); 2444 case 108280125: /*range*/ return new Property("range", "Range", "Used to express the observation within a period before and/or after the event.", 0, 1, range); 2445 default: return super.getNamedProperty(_hash, _name, _checkValid); 2446 } 2447 2448 } 2449 2450 @Override 2451 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2452 switch (hash) { 2453 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2454 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2455 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // DataType 2456 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 2457 case 108280125: /*range*/ return this.range == null ? new Base[0] : new Base[] {this.range}; // Range 2458 default: return super.getProperty(hash, name, checkValid); 2459 } 2460 2461 } 2462 2463 @Override 2464 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2465 switch (hash) { 2466 case -1724546052: // description 2467 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2468 return value; 2469 case 3387378: // note 2470 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2471 return value; 2472 case 96891546: // event 2473 this.event = TypeConvertor.castToType(value); // DataType 2474 return value; 2475 case -1285004149: // quantity 2476 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2477 return value; 2478 case 108280125: // range 2479 this.range = TypeConvertor.castToRange(value); // Range 2480 return value; 2481 default: return super.setProperty(hash, name, value); 2482 } 2483 2484 } 2485 2486 @Override 2487 public Base setProperty(String name, Base value) throws FHIRException { 2488 if (name.equals("description")) { 2489 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2490 } else if (name.equals("note")) { 2491 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2492 } else if (name.equals("event[x]")) { 2493 this.event = TypeConvertor.castToType(value); // DataType 2494 } else if (name.equals("quantity")) { 2495 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2496 } else if (name.equals("range")) { 2497 this.range = TypeConvertor.castToRange(value); // Range 2498 } else 2499 return super.setProperty(name, value); 2500 return value; 2501 } 2502 2503 @Override 2504 public void removeChild(String name, Base value) throws FHIRException { 2505 if (name.equals("description")) { 2506 this.description = null; 2507 } else if (name.equals("note")) { 2508 this.getNote().remove(value); 2509 } else if (name.equals("event[x]")) { 2510 this.event = null; 2511 } else if (name.equals("quantity")) { 2512 this.quantity = null; 2513 } else if (name.equals("range")) { 2514 this.range = null; 2515 } else 2516 super.removeChild(name, value); 2517 2518 } 2519 2520 @Override 2521 public Base makeProperty(int hash, String name) throws FHIRException { 2522 switch (hash) { 2523 case -1724546052: return getDescriptionElement(); 2524 case 3387378: return addNote(); 2525 case 278115238: return getEvent(); 2526 case 96891546: return getEvent(); 2527 case -1285004149: return getQuantity(); 2528 case 108280125: return getRange(); 2529 default: return super.makeProperty(hash, name); 2530 } 2531 2532 } 2533 2534 @Override 2535 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2536 switch (hash) { 2537 case -1724546052: /*description*/ return new String[] {"markdown"}; 2538 case 3387378: /*note*/ return new String[] {"Annotation"}; 2539 case 96891546: /*event*/ return new String[] {"CodeableConcept", "Reference", "dateTime", "id"}; 2540 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 2541 case 108280125: /*range*/ return new String[] {"Range"}; 2542 default: return super.getTypesForProperty(hash, name); 2543 } 2544 2545 } 2546 2547 @Override 2548 public Base addChild(String name) throws FHIRException { 2549 if (name.equals("description")) { 2550 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.timeFromEvent.description"); 2551 } 2552 else if (name.equals("note")) { 2553 return addNote(); 2554 } 2555 else if (name.equals("eventCodeableConcept")) { 2556 this.event = new CodeableConcept(); 2557 return this.event; 2558 } 2559 else if (name.equals("eventReference")) { 2560 this.event = new Reference(); 2561 return this.event; 2562 } 2563 else if (name.equals("eventDateTime")) { 2564 this.event = new DateTimeType(); 2565 return this.event; 2566 } 2567 else if (name.equals("eventId")) { 2568 this.event = new IdType(); 2569 return this.event; 2570 } 2571 else if (name.equals("quantity")) { 2572 this.quantity = new Quantity(); 2573 return this.quantity; 2574 } 2575 else if (name.equals("range")) { 2576 this.range = new Range(); 2577 return this.range; 2578 } 2579 else 2580 return super.addChild(name); 2581 } 2582 2583 public EvidenceVariableCharacteristicTimeFromEventComponent copy() { 2584 EvidenceVariableCharacteristicTimeFromEventComponent dst = new EvidenceVariableCharacteristicTimeFromEventComponent(); 2585 copyValues(dst); 2586 return dst; 2587 } 2588 2589 public void copyValues(EvidenceVariableCharacteristicTimeFromEventComponent dst) { 2590 super.copyValues(dst); 2591 dst.description = description == null ? null : description.copy(); 2592 if (note != null) { 2593 dst.note = new ArrayList<Annotation>(); 2594 for (Annotation i : note) 2595 dst.note.add(i.copy()); 2596 }; 2597 dst.event = event == null ? null : event.copy(); 2598 dst.quantity = quantity == null ? null : quantity.copy(); 2599 dst.range = range == null ? null : range.copy(); 2600 } 2601 2602 @Override 2603 public boolean equalsDeep(Base other_) { 2604 if (!super.equalsDeep(other_)) 2605 return false; 2606 if (!(other_ instanceof EvidenceVariableCharacteristicTimeFromEventComponent)) 2607 return false; 2608 EvidenceVariableCharacteristicTimeFromEventComponent o = (EvidenceVariableCharacteristicTimeFromEventComponent) other_; 2609 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(event, o.event, true) 2610 && compareDeep(quantity, o.quantity, true) && compareDeep(range, o.range, true); 2611 } 2612 2613 @Override 2614 public boolean equalsShallow(Base other_) { 2615 if (!super.equalsShallow(other_)) 2616 return false; 2617 if (!(other_ instanceof EvidenceVariableCharacteristicTimeFromEventComponent)) 2618 return false; 2619 EvidenceVariableCharacteristicTimeFromEventComponent o = (EvidenceVariableCharacteristicTimeFromEventComponent) other_; 2620 return compareValues(description, o.description, true); 2621 } 2622 2623 public boolean isEmpty() { 2624 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, event 2625 , quantity, range); 2626 } 2627 2628 public String fhirType() { 2629 return "EvidenceVariable.characteristic.timeFromEvent"; 2630 2631 } 2632 2633 } 2634 2635 @Block() 2636 public static class EvidenceVariableCategoryComponent extends BackboneElement implements IBaseBackboneElement { 2637 /** 2638 * Description of the grouping. 2639 */ 2640 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2641 @Description(shortDefinition="Description of the grouping", formalDefinition="Description of the grouping." ) 2642 protected StringType name; 2643 2644 /** 2645 * Definition of the grouping. 2646 */ 2647 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, Range.class}, order=2, min=0, max=1, modifier=false, summary=false) 2648 @Description(shortDefinition="Definition of the grouping", formalDefinition="Definition of the grouping." ) 2649 protected DataType value; 2650 2651 private static final long serialVersionUID = 1839679495L; 2652 2653 /** 2654 * Constructor 2655 */ 2656 public EvidenceVariableCategoryComponent() { 2657 super(); 2658 } 2659 2660 /** 2661 * @return {@link #name} (Description of the grouping.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2662 */ 2663 public StringType getNameElement() { 2664 if (this.name == null) 2665 if (Configuration.errorOnAutoCreate()) 2666 throw new Error("Attempt to auto-create EvidenceVariableCategoryComponent.name"); 2667 else if (Configuration.doAutoCreate()) 2668 this.name = new StringType(); // bb 2669 return this.name; 2670 } 2671 2672 public boolean hasNameElement() { 2673 return this.name != null && !this.name.isEmpty(); 2674 } 2675 2676 public boolean hasName() { 2677 return this.name != null && !this.name.isEmpty(); 2678 } 2679 2680 /** 2681 * @param value {@link #name} (Description of the grouping.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2682 */ 2683 public EvidenceVariableCategoryComponent setNameElement(StringType value) { 2684 this.name = value; 2685 return this; 2686 } 2687 2688 /** 2689 * @return Description of the grouping. 2690 */ 2691 public String getName() { 2692 return this.name == null ? null : this.name.getValue(); 2693 } 2694 2695 /** 2696 * @param value Description of the grouping. 2697 */ 2698 public EvidenceVariableCategoryComponent setName(String value) { 2699 if (Utilities.noString(value)) 2700 this.name = null; 2701 else { 2702 if (this.name == null) 2703 this.name = new StringType(); 2704 this.name.setValue(value); 2705 } 2706 return this; 2707 } 2708 2709 /** 2710 * @return {@link #value} (Definition of the grouping.) 2711 */ 2712 public DataType getValue() { 2713 return this.value; 2714 } 2715 2716 /** 2717 * @return {@link #value} (Definition of the grouping.) 2718 */ 2719 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2720 if (this.value == null) 2721 this.value = new CodeableConcept(); 2722 if (!(this.value instanceof CodeableConcept)) 2723 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2724 return (CodeableConcept) this.value; 2725 } 2726 2727 public boolean hasValueCodeableConcept() { 2728 return this != null && this.value instanceof CodeableConcept; 2729 } 2730 2731 /** 2732 * @return {@link #value} (Definition of the grouping.) 2733 */ 2734 public Quantity getValueQuantity() throws FHIRException { 2735 if (this.value == null) 2736 this.value = new Quantity(); 2737 if (!(this.value instanceof Quantity)) 2738 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2739 return (Quantity) this.value; 2740 } 2741 2742 public boolean hasValueQuantity() { 2743 return this != null && this.value instanceof Quantity; 2744 } 2745 2746 /** 2747 * @return {@link #value} (Definition of the grouping.) 2748 */ 2749 public Range getValueRange() throws FHIRException { 2750 if (this.value == null) 2751 this.value = new Range(); 2752 if (!(this.value instanceof Range)) 2753 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2754 return (Range) this.value; 2755 } 2756 2757 public boolean hasValueRange() { 2758 return this != null && this.value instanceof Range; 2759 } 2760 2761 public boolean hasValue() { 2762 return this.value != null && !this.value.isEmpty(); 2763 } 2764 2765 /** 2766 * @param value {@link #value} (Definition of the grouping.) 2767 */ 2768 public EvidenceVariableCategoryComponent setValue(DataType value) { 2769 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range)) 2770 throw new FHIRException("Not the right type for EvidenceVariable.category.value[x]: "+value.fhirType()); 2771 this.value = value; 2772 return this; 2773 } 2774 2775 protected void listChildren(List<Property> children) { 2776 super.listChildren(children); 2777 children.add(new Property("name", "string", "Description of the grouping.", 0, 1, name)); 2778 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range", "Definition of the grouping.", 0, 1, value)); 2779 } 2780 2781 @Override 2782 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2783 switch (_hash) { 2784 case 3373707: /*name*/ return new Property("name", "string", "Description of the grouping.", 0, 1, name); 2785 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "Definition of the grouping.", 0, 1, value); 2786 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "Definition of the grouping.", 0, 1, value); 2787 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Definition of the grouping.", 0, 1, value); 2788 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Definition of the grouping.", 0, 1, value); 2789 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Definition of the grouping.", 0, 1, value); 2790 default: return super.getNamedProperty(_hash, _name, _checkValid); 2791 } 2792 2793 } 2794 2795 @Override 2796 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2797 switch (hash) { 2798 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2799 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2800 default: return super.getProperty(hash, name, checkValid); 2801 } 2802 2803 } 2804 2805 @Override 2806 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2807 switch (hash) { 2808 case 3373707: // name 2809 this.name = TypeConvertor.castToString(value); // StringType 2810 return value; 2811 case 111972721: // value 2812 this.value = TypeConvertor.castToType(value); // DataType 2813 return value; 2814 default: return super.setProperty(hash, name, value); 2815 } 2816 2817 } 2818 2819 @Override 2820 public Base setProperty(String name, Base value) throws FHIRException { 2821 if (name.equals("name")) { 2822 this.name = TypeConvertor.castToString(value); // StringType 2823 } else if (name.equals("value[x]")) { 2824 this.value = TypeConvertor.castToType(value); // DataType 2825 } else 2826 return super.setProperty(name, value); 2827 return value; 2828 } 2829 2830 @Override 2831 public void removeChild(String name, Base value) throws FHIRException { 2832 if (name.equals("name")) { 2833 this.name = null; 2834 } else if (name.equals("value[x]")) { 2835 this.value = null; 2836 } else 2837 super.removeChild(name, value); 2838 2839 } 2840 2841 @Override 2842 public Base makeProperty(int hash, String name) throws FHIRException { 2843 switch (hash) { 2844 case 3373707: return getNameElement(); 2845 case -1410166417: return getValue(); 2846 case 111972721: return getValue(); 2847 default: return super.makeProperty(hash, name); 2848 } 2849 2850 } 2851 2852 @Override 2853 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2854 switch (hash) { 2855 case 3373707: /*name*/ return new String[] {"string"}; 2856 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "Range"}; 2857 default: return super.getTypesForProperty(hash, name); 2858 } 2859 2860 } 2861 2862 @Override 2863 public Base addChild(String name) throws FHIRException { 2864 if (name.equals("name")) { 2865 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.category.name"); 2866 } 2867 else if (name.equals("valueCodeableConcept")) { 2868 this.value = new CodeableConcept(); 2869 return this.value; 2870 } 2871 else if (name.equals("valueQuantity")) { 2872 this.value = new Quantity(); 2873 return this.value; 2874 } 2875 else if (name.equals("valueRange")) { 2876 this.value = new Range(); 2877 return this.value; 2878 } 2879 else 2880 return super.addChild(name); 2881 } 2882 2883 public EvidenceVariableCategoryComponent copy() { 2884 EvidenceVariableCategoryComponent dst = new EvidenceVariableCategoryComponent(); 2885 copyValues(dst); 2886 return dst; 2887 } 2888 2889 public void copyValues(EvidenceVariableCategoryComponent dst) { 2890 super.copyValues(dst); 2891 dst.name = name == null ? null : name.copy(); 2892 dst.value = value == null ? null : value.copy(); 2893 } 2894 2895 @Override 2896 public boolean equalsDeep(Base other_) { 2897 if (!super.equalsDeep(other_)) 2898 return false; 2899 if (!(other_ instanceof EvidenceVariableCategoryComponent)) 2900 return false; 2901 EvidenceVariableCategoryComponent o = (EvidenceVariableCategoryComponent) other_; 2902 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 2903 } 2904 2905 @Override 2906 public boolean equalsShallow(Base other_) { 2907 if (!super.equalsShallow(other_)) 2908 return false; 2909 if (!(other_ instanceof EvidenceVariableCategoryComponent)) 2910 return false; 2911 EvidenceVariableCategoryComponent o = (EvidenceVariableCategoryComponent) other_; 2912 return compareValues(name, o.name, true); 2913 } 2914 2915 public boolean isEmpty() { 2916 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 2917 } 2918 2919 public String fhirType() { 2920 return "EvidenceVariable.category"; 2921 2922 } 2923 2924 } 2925 2926 /** 2927 * An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers. 2928 */ 2929 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2930 @Description(shortDefinition="Canonical identifier for this evidence variable, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers." ) 2931 protected UriType url; 2932 2933 /** 2934 * A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance. 2935 */ 2936 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2937 @Description(shortDefinition="Additional identifier for the evidence variable", formalDefinition="A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2938 protected List<Identifier> identifier; 2939 2940 /** 2941 * The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 2942 */ 2943 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2944 @Description(shortDefinition="Business version of the evidence variable", formalDefinition="The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts." ) 2945 protected StringType version; 2946 2947 /** 2948 * Indicates the mechanism used to compare versions to determine which is more current. 2949 */ 2950 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2951 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 2952 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2953 protected DataType versionAlgorithm; 2954 2955 /** 2956 * A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2957 */ 2958 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2959 @Description(shortDefinition="Name for this evidence variable (computer friendly)", formalDefinition="A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2960 protected StringType name; 2961 2962 /** 2963 * A short, descriptive, user-friendly title for the evidence variable. 2964 */ 2965 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2966 @Description(shortDefinition="Name for this evidence variable (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the evidence variable." ) 2967 protected StringType title; 2968 2969 /** 2970 * The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary. 2971 */ 2972 @Child(name = "shortTitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2973 @Description(shortDefinition="Title for use in informal contexts", formalDefinition="The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary." ) 2974 protected StringType shortTitle; 2975 2976 /** 2977 * The status of this evidence variable. Enables tracking the life-cycle of the content. 2978 */ 2979 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 2980 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this evidence variable. Enables tracking the life-cycle of the content." ) 2981 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2982 protected Enumeration<PublicationStatus> status; 2983 2984 /** 2985 * A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2986 */ 2987 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2988 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 2989 protected BooleanType experimental; 2990 2991 /** 2992 * The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes. 2993 */ 2994 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2995 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes." ) 2996 protected DateTimeType date; 2997 2998 /** 2999 * The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable. 3000 */ 3001 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3002 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable." ) 3003 protected StringType publisher; 3004 3005 /** 3006 * Contact details to assist a user in finding and communicating with the publisher. 3007 */ 3008 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3009 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 3010 protected List<ContactDetail> contact; 3011 3012 /** 3013 * A free text natural language description of the evidence variable from a consumer's perspective. 3014 */ 3015 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=true) 3016 @Description(shortDefinition="Natural language description of the evidence variable", formalDefinition="A free text natural language description of the evidence variable from a consumer's perspective." ) 3017 protected MarkdownType description; 3018 3019 /** 3020 * A human-readable string to clarify or explain concepts about the resource. 3021 */ 3022 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3023 @Description(shortDefinition="Used for footnotes or explanatory notes", formalDefinition="A human-readable string to clarify or explain concepts about the resource." ) 3024 protected List<Annotation> note; 3025 3026 /** 3027 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances. 3028 */ 3029 @Child(name = "useContext", type = {UsageContext.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3030 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances." ) 3031 protected List<UsageContext> useContext; 3032 3033 /** 3034 * Explanation of why this EvidenceVariable is needed and why it has been designed as it has. 3035 */ 3036 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 3037 @Description(shortDefinition="Why this EvidenceVariable is defined", formalDefinition="Explanation of why this EvidenceVariable is needed and why it has been designed as it has." ) 3038 protected MarkdownType purpose; 3039 3040 /** 3041 * A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource. 3042 */ 3043 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 3044 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource." ) 3045 protected MarkdownType copyright; 3046 3047 /** 3048 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3049 */ 3050 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 3051 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 3052 protected StringType copyrightLabel; 3053 3054 /** 3055 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3056 3057See guidance around (not) making local changes to elements [here](canonicalresource.html#localization). 3058 */ 3059 @Child(name = "approvalDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 3060 @Description(shortDefinition="When the resource was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.\n\nSee guidance around (not) making local changes to elements [here](canonicalresource.html#localization)." ) 3061 protected DateType approvalDate; 3062 3063 /** 3064 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3065 */ 3066 @Child(name = "lastReviewDate", type = {DateType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3067 @Description(shortDefinition="When the resource was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 3068 protected DateType lastReviewDate; 3069 3070 /** 3071 * The period during which the resource content was or is planned to be in active use. 3072 */ 3073 @Child(name = "effectivePeriod", type = {Period.class}, order=20, min=0, max=1, modifier=false, summary=false) 3074 @Description(shortDefinition="When the resource is expected to be used", formalDefinition="The period during which the resource content was or is planned to be in active use." ) 3075 protected Period effectivePeriod; 3076 3077 /** 3078 * An individiual or organization primarily involved in the creation and maintenance of the content. 3079 */ 3080 @Child(name = "author", type = {ContactDetail.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3081 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the content." ) 3082 protected List<ContactDetail> author; 3083 3084 /** 3085 * An individual or organization primarily responsible for internal coherence of the content. 3086 */ 3087 @Child(name = "editor", type = {ContactDetail.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3088 @Description(shortDefinition="Who edited the content", formalDefinition="An individual or organization primarily responsible for internal coherence of the content." ) 3089 protected List<ContactDetail> editor; 3090 3091 /** 3092 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content. 3093 */ 3094 @Child(name = "reviewer", type = {ContactDetail.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3095 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content." ) 3096 protected List<ContactDetail> reviewer; 3097 3098 /** 3099 * An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting. 3100 */ 3101 @Child(name = "endorser", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3102 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting." ) 3103 protected List<ContactDetail> endorser; 3104 3105 /** 3106 * Related artifacts such as additional documentation, justification, or bibliographic references. 3107 */ 3108 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3109 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 3110 protected List<RelatedArtifact> relatedArtifact; 3111 3112 /** 3113 * True if the actual variable measured, false if a conceptual representation of the intended variable. 3114 */ 3115 @Child(name = "actual", type = {BooleanType.class}, order=26, min=0, max=1, modifier=false, summary=false) 3116 @Description(shortDefinition="Actual or conceptual", formalDefinition="True if the actual variable measured, false if a conceptual representation of the intended variable." ) 3117 protected BooleanType actual; 3118 3119 /** 3120 * A defining factor of the EvidenceVariable. Multiple characteristics are applied with "and" semantics. 3121 */ 3122 @Child(name = "characteristic", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3123 @Description(shortDefinition="A defining factor of the EvidenceVariable", formalDefinition="A defining factor of the EvidenceVariable. Multiple characteristics are applied with \"and\" semantics." ) 3124 protected List<EvidenceVariableCharacteristicComponent> characteristic; 3125 3126 /** 3127 * The method of handling in statistical analysis. 3128 */ 3129 @Child(name = "handling", type = {CodeType.class}, order=28, min=0, max=1, modifier=false, summary=false) 3130 @Description(shortDefinition="continuous | dichotomous | ordinal | polychotomous", formalDefinition="The method of handling in statistical analysis." ) 3131 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/variable-handling") 3132 protected Enumeration<EvidenceVariableHandling> handling; 3133 3134 /** 3135 * A grouping for ordinal or polychotomous variables. 3136 */ 3137 @Child(name = "category", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3138 @Description(shortDefinition="A grouping for ordinal or polychotomous variables", formalDefinition="A grouping for ordinal or polychotomous variables." ) 3139 protected List<EvidenceVariableCategoryComponent> category; 3140 3141 private static final long serialVersionUID = -1626354085L; 3142 3143 /** 3144 * Constructor 3145 */ 3146 public EvidenceVariable() { 3147 super(); 3148 } 3149 3150 /** 3151 * Constructor 3152 */ 3153 public EvidenceVariable(PublicationStatus status) { 3154 super(); 3155 this.setStatus(status); 3156 } 3157 3158 /** 3159 * @return {@link #url} (An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3160 */ 3161 public UriType getUrlElement() { 3162 if (this.url == null) 3163 if (Configuration.errorOnAutoCreate()) 3164 throw new Error("Attempt to auto-create EvidenceVariable.url"); 3165 else if (Configuration.doAutoCreate()) 3166 this.url = new UriType(); // bb 3167 return this.url; 3168 } 3169 3170 public boolean hasUrlElement() { 3171 return this.url != null && !this.url.isEmpty(); 3172 } 3173 3174 public boolean hasUrl() { 3175 return this.url != null && !this.url.isEmpty(); 3176 } 3177 3178 /** 3179 * @param value {@link #url} (An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3180 */ 3181 public EvidenceVariable setUrlElement(UriType value) { 3182 this.url = value; 3183 return this; 3184 } 3185 3186 /** 3187 * @return An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers. 3188 */ 3189 public String getUrl() { 3190 return this.url == null ? null : this.url.getValue(); 3191 } 3192 3193 /** 3194 * @param value An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers. 3195 */ 3196 public EvidenceVariable setUrl(String value) { 3197 if (Utilities.noString(value)) 3198 this.url = null; 3199 else { 3200 if (this.url == null) 3201 this.url = new UriType(); 3202 this.url.setValue(value); 3203 } 3204 return this; 3205 } 3206 3207 /** 3208 * @return {@link #identifier} (A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.) 3209 */ 3210 public List<Identifier> getIdentifier() { 3211 if (this.identifier == null) 3212 this.identifier = new ArrayList<Identifier>(); 3213 return this.identifier; 3214 } 3215 3216 /** 3217 * @return Returns a reference to <code>this</code> for easy method chaining 3218 */ 3219 public EvidenceVariable setIdentifier(List<Identifier> theIdentifier) { 3220 this.identifier = theIdentifier; 3221 return this; 3222 } 3223 3224 public boolean hasIdentifier() { 3225 if (this.identifier == null) 3226 return false; 3227 for (Identifier item : this.identifier) 3228 if (!item.isEmpty()) 3229 return true; 3230 return false; 3231 } 3232 3233 public Identifier addIdentifier() { //3 3234 Identifier t = new Identifier(); 3235 if (this.identifier == null) 3236 this.identifier = new ArrayList<Identifier>(); 3237 this.identifier.add(t); 3238 return t; 3239 } 3240 3241 public EvidenceVariable addIdentifier(Identifier t) { //3 3242 if (t == null) 3243 return this; 3244 if (this.identifier == null) 3245 this.identifier = new ArrayList<Identifier>(); 3246 this.identifier.add(t); 3247 return this; 3248 } 3249 3250 /** 3251 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3252 */ 3253 public Identifier getIdentifierFirstRep() { 3254 if (getIdentifier().isEmpty()) { 3255 addIdentifier(); 3256 } 3257 return getIdentifier().get(0); 3258 } 3259 3260 /** 3261 * @return {@link #version} (The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3262 */ 3263 public StringType getVersionElement() { 3264 if (this.version == null) 3265 if (Configuration.errorOnAutoCreate()) 3266 throw new Error("Attempt to auto-create EvidenceVariable.version"); 3267 else if (Configuration.doAutoCreate()) 3268 this.version = new StringType(); // bb 3269 return this.version; 3270 } 3271 3272 public boolean hasVersionElement() { 3273 return this.version != null && !this.version.isEmpty(); 3274 } 3275 3276 public boolean hasVersion() { 3277 return this.version != null && !this.version.isEmpty(); 3278 } 3279 3280 /** 3281 * @param value {@link #version} (The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3282 */ 3283 public EvidenceVariable setVersionElement(StringType value) { 3284 this.version = value; 3285 return this; 3286 } 3287 3288 /** 3289 * @return The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 3290 */ 3291 public String getVersion() { 3292 return this.version == null ? null : this.version.getValue(); 3293 } 3294 3295 /** 3296 * @param value The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 3297 */ 3298 public EvidenceVariable setVersion(String value) { 3299 if (Utilities.noString(value)) 3300 this.version = null; 3301 else { 3302 if (this.version == null) 3303 this.version = new StringType(); 3304 this.version.setValue(value); 3305 } 3306 return this; 3307 } 3308 3309 /** 3310 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3311 */ 3312 public DataType getVersionAlgorithm() { 3313 return this.versionAlgorithm; 3314 } 3315 3316 /** 3317 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3318 */ 3319 public StringType getVersionAlgorithmStringType() throws FHIRException { 3320 if (this.versionAlgorithm == null) 3321 this.versionAlgorithm = new StringType(); 3322 if (!(this.versionAlgorithm instanceof StringType)) 3323 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3324 return (StringType) this.versionAlgorithm; 3325 } 3326 3327 public boolean hasVersionAlgorithmStringType() { 3328 return this != null && this.versionAlgorithm instanceof StringType; 3329 } 3330 3331 /** 3332 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3333 */ 3334 public Coding getVersionAlgorithmCoding() throws FHIRException { 3335 if (this.versionAlgorithm == null) 3336 this.versionAlgorithm = new Coding(); 3337 if (!(this.versionAlgorithm instanceof Coding)) 3338 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3339 return (Coding) this.versionAlgorithm; 3340 } 3341 3342 public boolean hasVersionAlgorithmCoding() { 3343 return this != null && this.versionAlgorithm instanceof Coding; 3344 } 3345 3346 public boolean hasVersionAlgorithm() { 3347 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 3348 } 3349 3350 /** 3351 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3352 */ 3353 public EvidenceVariable setVersionAlgorithm(DataType value) { 3354 if (value != null && !(value instanceof StringType || value instanceof Coding)) 3355 throw new FHIRException("Not the right type for EvidenceVariable.versionAlgorithm[x]: "+value.fhirType()); 3356 this.versionAlgorithm = value; 3357 return this; 3358 } 3359 3360 /** 3361 * @return {@link #name} (A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3362 */ 3363 public StringType getNameElement() { 3364 if (this.name == null) 3365 if (Configuration.errorOnAutoCreate()) 3366 throw new Error("Attempt to auto-create EvidenceVariable.name"); 3367 else if (Configuration.doAutoCreate()) 3368 this.name = new StringType(); // bb 3369 return this.name; 3370 } 3371 3372 public boolean hasNameElement() { 3373 return this.name != null && !this.name.isEmpty(); 3374 } 3375 3376 public boolean hasName() { 3377 return this.name != null && !this.name.isEmpty(); 3378 } 3379 3380 /** 3381 * @param value {@link #name} (A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3382 */ 3383 public EvidenceVariable setNameElement(StringType value) { 3384 this.name = value; 3385 return this; 3386 } 3387 3388 /** 3389 * @return A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3390 */ 3391 public String getName() { 3392 return this.name == null ? null : this.name.getValue(); 3393 } 3394 3395 /** 3396 * @param value A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3397 */ 3398 public EvidenceVariable setName(String value) { 3399 if (Utilities.noString(value)) 3400 this.name = null; 3401 else { 3402 if (this.name == null) 3403 this.name = new StringType(); 3404 this.name.setValue(value); 3405 } 3406 return this; 3407 } 3408 3409 /** 3410 * @return {@link #title} (A short, descriptive, user-friendly title for the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3411 */ 3412 public StringType getTitleElement() { 3413 if (this.title == null) 3414 if (Configuration.errorOnAutoCreate()) 3415 throw new Error("Attempt to auto-create EvidenceVariable.title"); 3416 else if (Configuration.doAutoCreate()) 3417 this.title = new StringType(); // bb 3418 return this.title; 3419 } 3420 3421 public boolean hasTitleElement() { 3422 return this.title != null && !this.title.isEmpty(); 3423 } 3424 3425 public boolean hasTitle() { 3426 return this.title != null && !this.title.isEmpty(); 3427 } 3428 3429 /** 3430 * @param value {@link #title} (A short, descriptive, user-friendly title for the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3431 */ 3432 public EvidenceVariable setTitleElement(StringType value) { 3433 this.title = value; 3434 return this; 3435 } 3436 3437 /** 3438 * @return A short, descriptive, user-friendly title for the evidence variable. 3439 */ 3440 public String getTitle() { 3441 return this.title == null ? null : this.title.getValue(); 3442 } 3443 3444 /** 3445 * @param value A short, descriptive, user-friendly title for the evidence variable. 3446 */ 3447 public EvidenceVariable setTitle(String value) { 3448 if (Utilities.noString(value)) 3449 this.title = null; 3450 else { 3451 if (this.title == null) 3452 this.title = new StringType(); 3453 this.title.setValue(value); 3454 } 3455 return this; 3456 } 3457 3458 /** 3459 * @return {@link #shortTitle} (The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.). This is the underlying object with id, value and extensions. The accessor "getShortTitle" gives direct access to the value 3460 */ 3461 public StringType getShortTitleElement() { 3462 if (this.shortTitle == null) 3463 if (Configuration.errorOnAutoCreate()) 3464 throw new Error("Attempt to auto-create EvidenceVariable.shortTitle"); 3465 else if (Configuration.doAutoCreate()) 3466 this.shortTitle = new StringType(); // bb 3467 return this.shortTitle; 3468 } 3469 3470 public boolean hasShortTitleElement() { 3471 return this.shortTitle != null && !this.shortTitle.isEmpty(); 3472 } 3473 3474 public boolean hasShortTitle() { 3475 return this.shortTitle != null && !this.shortTitle.isEmpty(); 3476 } 3477 3478 /** 3479 * @param value {@link #shortTitle} (The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.). This is the underlying object with id, value and extensions. The accessor "getShortTitle" gives direct access to the value 3480 */ 3481 public EvidenceVariable setShortTitleElement(StringType value) { 3482 this.shortTitle = value; 3483 return this; 3484 } 3485 3486 /** 3487 * @return The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary. 3488 */ 3489 public String getShortTitle() { 3490 return this.shortTitle == null ? null : this.shortTitle.getValue(); 3491 } 3492 3493 /** 3494 * @param value The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary. 3495 */ 3496 public EvidenceVariable setShortTitle(String value) { 3497 if (Utilities.noString(value)) 3498 this.shortTitle = null; 3499 else { 3500 if (this.shortTitle == null) 3501 this.shortTitle = new StringType(); 3502 this.shortTitle.setValue(value); 3503 } 3504 return this; 3505 } 3506 3507 /** 3508 * @return {@link #status} (The status of this evidence variable. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3509 */ 3510 public Enumeration<PublicationStatus> getStatusElement() { 3511 if (this.status == null) 3512 if (Configuration.errorOnAutoCreate()) 3513 throw new Error("Attempt to auto-create EvidenceVariable.status"); 3514 else if (Configuration.doAutoCreate()) 3515 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3516 return this.status; 3517 } 3518 3519 public boolean hasStatusElement() { 3520 return this.status != null && !this.status.isEmpty(); 3521 } 3522 3523 public boolean hasStatus() { 3524 return this.status != null && !this.status.isEmpty(); 3525 } 3526 3527 /** 3528 * @param value {@link #status} (The status of this evidence variable. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3529 */ 3530 public EvidenceVariable setStatusElement(Enumeration<PublicationStatus> value) { 3531 this.status = value; 3532 return this; 3533 } 3534 3535 /** 3536 * @return The status of this evidence variable. Enables tracking the life-cycle of the content. 3537 */ 3538 public PublicationStatus getStatus() { 3539 return this.status == null ? null : this.status.getValue(); 3540 } 3541 3542 /** 3543 * @param value The status of this evidence variable. Enables tracking the life-cycle of the content. 3544 */ 3545 public EvidenceVariable setStatus(PublicationStatus value) { 3546 if (this.status == null) 3547 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3548 this.status.setValue(value); 3549 return this; 3550 } 3551 3552 /** 3553 * @return {@link #experimental} (A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3554 */ 3555 public BooleanType getExperimentalElement() { 3556 if (this.experimental == null) 3557 if (Configuration.errorOnAutoCreate()) 3558 throw new Error("Attempt to auto-create EvidenceVariable.experimental"); 3559 else if (Configuration.doAutoCreate()) 3560 this.experimental = new BooleanType(); // bb 3561 return this.experimental; 3562 } 3563 3564 public boolean hasExperimentalElement() { 3565 return this.experimental != null && !this.experimental.isEmpty(); 3566 } 3567 3568 public boolean hasExperimental() { 3569 return this.experimental != null && !this.experimental.isEmpty(); 3570 } 3571 3572 /** 3573 * @param value {@link #experimental} (A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3574 */ 3575 public EvidenceVariable setExperimentalElement(BooleanType value) { 3576 this.experimental = value; 3577 return this; 3578 } 3579 3580 /** 3581 * @return A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3582 */ 3583 public boolean getExperimental() { 3584 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3585 } 3586 3587 /** 3588 * @param value A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3589 */ 3590 public EvidenceVariable setExperimental(boolean value) { 3591 if (this.experimental == null) 3592 this.experimental = new BooleanType(); 3593 this.experimental.setValue(value); 3594 return this; 3595 } 3596 3597 /** 3598 * @return {@link #date} (The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3599 */ 3600 public DateTimeType getDateElement() { 3601 if (this.date == null) 3602 if (Configuration.errorOnAutoCreate()) 3603 throw new Error("Attempt to auto-create EvidenceVariable.date"); 3604 else if (Configuration.doAutoCreate()) 3605 this.date = new DateTimeType(); // bb 3606 return this.date; 3607 } 3608 3609 public boolean hasDateElement() { 3610 return this.date != null && !this.date.isEmpty(); 3611 } 3612 3613 public boolean hasDate() { 3614 return this.date != null && !this.date.isEmpty(); 3615 } 3616 3617 /** 3618 * @param value {@link #date} (The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3619 */ 3620 public EvidenceVariable setDateElement(DateTimeType value) { 3621 this.date = value; 3622 return this; 3623 } 3624 3625 /** 3626 * @return The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes. 3627 */ 3628 public Date getDate() { 3629 return this.date == null ? null : this.date.getValue(); 3630 } 3631 3632 /** 3633 * @param value The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes. 3634 */ 3635 public EvidenceVariable setDate(Date value) { 3636 if (value == null) 3637 this.date = null; 3638 else { 3639 if (this.date == null) 3640 this.date = new DateTimeType(); 3641 this.date.setValue(value); 3642 } 3643 return this; 3644 } 3645 3646 /** 3647 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3648 */ 3649 public StringType getPublisherElement() { 3650 if (this.publisher == null) 3651 if (Configuration.errorOnAutoCreate()) 3652 throw new Error("Attempt to auto-create EvidenceVariable.publisher"); 3653 else if (Configuration.doAutoCreate()) 3654 this.publisher = new StringType(); // bb 3655 return this.publisher; 3656 } 3657 3658 public boolean hasPublisherElement() { 3659 return this.publisher != null && !this.publisher.isEmpty(); 3660 } 3661 3662 public boolean hasPublisher() { 3663 return this.publisher != null && !this.publisher.isEmpty(); 3664 } 3665 3666 /** 3667 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3668 */ 3669 public EvidenceVariable setPublisherElement(StringType value) { 3670 this.publisher = value; 3671 return this; 3672 } 3673 3674 /** 3675 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable. 3676 */ 3677 public String getPublisher() { 3678 return this.publisher == null ? null : this.publisher.getValue(); 3679 } 3680 3681 /** 3682 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable. 3683 */ 3684 public EvidenceVariable setPublisher(String value) { 3685 if (Utilities.noString(value)) 3686 this.publisher = null; 3687 else { 3688 if (this.publisher == null) 3689 this.publisher = new StringType(); 3690 this.publisher.setValue(value); 3691 } 3692 return this; 3693 } 3694 3695 /** 3696 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3697 */ 3698 public List<ContactDetail> getContact() { 3699 if (this.contact == null) 3700 this.contact = new ArrayList<ContactDetail>(); 3701 return this.contact; 3702 } 3703 3704 /** 3705 * @return Returns a reference to <code>this</code> for easy method chaining 3706 */ 3707 public EvidenceVariable setContact(List<ContactDetail> theContact) { 3708 this.contact = theContact; 3709 return this; 3710 } 3711 3712 public boolean hasContact() { 3713 if (this.contact == null) 3714 return false; 3715 for (ContactDetail item : this.contact) 3716 if (!item.isEmpty()) 3717 return true; 3718 return false; 3719 } 3720 3721 public ContactDetail addContact() { //3 3722 ContactDetail t = new ContactDetail(); 3723 if (this.contact == null) 3724 this.contact = new ArrayList<ContactDetail>(); 3725 this.contact.add(t); 3726 return t; 3727 } 3728 3729 public EvidenceVariable addContact(ContactDetail t) { //3 3730 if (t == null) 3731 return this; 3732 if (this.contact == null) 3733 this.contact = new ArrayList<ContactDetail>(); 3734 this.contact.add(t); 3735 return this; 3736 } 3737 3738 /** 3739 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3740 */ 3741 public ContactDetail getContactFirstRep() { 3742 if (getContact().isEmpty()) { 3743 addContact(); 3744 } 3745 return getContact().get(0); 3746 } 3747 3748 /** 3749 * @return {@link #description} (A free text natural language description of the evidence variable from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3750 */ 3751 public MarkdownType getDescriptionElement() { 3752 if (this.description == null) 3753 if (Configuration.errorOnAutoCreate()) 3754 throw new Error("Attempt to auto-create EvidenceVariable.description"); 3755 else if (Configuration.doAutoCreate()) 3756 this.description = new MarkdownType(); // bb 3757 return this.description; 3758 } 3759 3760 public boolean hasDescriptionElement() { 3761 return this.description != null && !this.description.isEmpty(); 3762 } 3763 3764 public boolean hasDescription() { 3765 return this.description != null && !this.description.isEmpty(); 3766 } 3767 3768 /** 3769 * @param value {@link #description} (A free text natural language description of the evidence variable from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3770 */ 3771 public EvidenceVariable setDescriptionElement(MarkdownType value) { 3772 this.description = value; 3773 return this; 3774 } 3775 3776 /** 3777 * @return A free text natural language description of the evidence variable from a consumer's perspective. 3778 */ 3779 public String getDescription() { 3780 return this.description == null ? null : this.description.getValue(); 3781 } 3782 3783 /** 3784 * @param value A free text natural language description of the evidence variable from a consumer's perspective. 3785 */ 3786 public EvidenceVariable setDescription(String value) { 3787 if (Utilities.noString(value)) 3788 this.description = null; 3789 else { 3790 if (this.description == null) 3791 this.description = new MarkdownType(); 3792 this.description.setValue(value); 3793 } 3794 return this; 3795 } 3796 3797 /** 3798 * @return {@link #note} (A human-readable string to clarify or explain concepts about the resource.) 3799 */ 3800 public List<Annotation> getNote() { 3801 if (this.note == null) 3802 this.note = new ArrayList<Annotation>(); 3803 return this.note; 3804 } 3805 3806 /** 3807 * @return Returns a reference to <code>this</code> for easy method chaining 3808 */ 3809 public EvidenceVariable setNote(List<Annotation> theNote) { 3810 this.note = theNote; 3811 return this; 3812 } 3813 3814 public boolean hasNote() { 3815 if (this.note == null) 3816 return false; 3817 for (Annotation item : this.note) 3818 if (!item.isEmpty()) 3819 return true; 3820 return false; 3821 } 3822 3823 public Annotation addNote() { //3 3824 Annotation t = new Annotation(); 3825 if (this.note == null) 3826 this.note = new ArrayList<Annotation>(); 3827 this.note.add(t); 3828 return t; 3829 } 3830 3831 public EvidenceVariable addNote(Annotation t) { //3 3832 if (t == null) 3833 return this; 3834 if (this.note == null) 3835 this.note = new ArrayList<Annotation>(); 3836 this.note.add(t); 3837 return this; 3838 } 3839 3840 /** 3841 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3842 */ 3843 public Annotation getNoteFirstRep() { 3844 if (getNote().isEmpty()) { 3845 addNote(); 3846 } 3847 return getNote().get(0); 3848 } 3849 3850 /** 3851 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.) 3852 */ 3853 public List<UsageContext> getUseContext() { 3854 if (this.useContext == null) 3855 this.useContext = new ArrayList<UsageContext>(); 3856 return this.useContext; 3857 } 3858 3859 /** 3860 * @return Returns a reference to <code>this</code> for easy method chaining 3861 */ 3862 public EvidenceVariable setUseContext(List<UsageContext> theUseContext) { 3863 this.useContext = theUseContext; 3864 return this; 3865 } 3866 3867 public boolean hasUseContext() { 3868 if (this.useContext == null) 3869 return false; 3870 for (UsageContext item : this.useContext) 3871 if (!item.isEmpty()) 3872 return true; 3873 return false; 3874 } 3875 3876 public UsageContext addUseContext() { //3 3877 UsageContext t = new UsageContext(); 3878 if (this.useContext == null) 3879 this.useContext = new ArrayList<UsageContext>(); 3880 this.useContext.add(t); 3881 return t; 3882 } 3883 3884 public EvidenceVariable addUseContext(UsageContext t) { //3 3885 if (t == null) 3886 return this; 3887 if (this.useContext == null) 3888 this.useContext = new ArrayList<UsageContext>(); 3889 this.useContext.add(t); 3890 return this; 3891 } 3892 3893 /** 3894 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3895 */ 3896 public UsageContext getUseContextFirstRep() { 3897 if (getUseContext().isEmpty()) { 3898 addUseContext(); 3899 } 3900 return getUseContext().get(0); 3901 } 3902 3903 /** 3904 * @return {@link #purpose} (Explanation of why this EvidenceVariable is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3905 */ 3906 public MarkdownType getPurposeElement() { 3907 if (this.purpose == null) 3908 if (Configuration.errorOnAutoCreate()) 3909 throw new Error("Attempt to auto-create EvidenceVariable.purpose"); 3910 else if (Configuration.doAutoCreate()) 3911 this.purpose = new MarkdownType(); // bb 3912 return this.purpose; 3913 } 3914 3915 public boolean hasPurposeElement() { 3916 return this.purpose != null && !this.purpose.isEmpty(); 3917 } 3918 3919 public boolean hasPurpose() { 3920 return this.purpose != null && !this.purpose.isEmpty(); 3921 } 3922 3923 /** 3924 * @param value {@link #purpose} (Explanation of why this EvidenceVariable is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3925 */ 3926 public EvidenceVariable setPurposeElement(MarkdownType value) { 3927 this.purpose = value; 3928 return this; 3929 } 3930 3931 /** 3932 * @return Explanation of why this EvidenceVariable is needed and why it has been designed as it has. 3933 */ 3934 public String getPurpose() { 3935 return this.purpose == null ? null : this.purpose.getValue(); 3936 } 3937 3938 /** 3939 * @param value Explanation of why this EvidenceVariable is needed and why it has been designed as it has. 3940 */ 3941 public EvidenceVariable setPurpose(String value) { 3942 if (Utilities.noString(value)) 3943 this.purpose = null; 3944 else { 3945 if (this.purpose == null) 3946 this.purpose = new MarkdownType(); 3947 this.purpose.setValue(value); 3948 } 3949 return this; 3950 } 3951 3952 /** 3953 * @return {@link #copyright} (A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3954 */ 3955 public MarkdownType getCopyrightElement() { 3956 if (this.copyright == null) 3957 if (Configuration.errorOnAutoCreate()) 3958 throw new Error("Attempt to auto-create EvidenceVariable.copyright"); 3959 else if (Configuration.doAutoCreate()) 3960 this.copyright = new MarkdownType(); // bb 3961 return this.copyright; 3962 } 3963 3964 public boolean hasCopyrightElement() { 3965 return this.copyright != null && !this.copyright.isEmpty(); 3966 } 3967 3968 public boolean hasCopyright() { 3969 return this.copyright != null && !this.copyright.isEmpty(); 3970 } 3971 3972 /** 3973 * @param value {@link #copyright} (A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3974 */ 3975 public EvidenceVariable setCopyrightElement(MarkdownType value) { 3976 this.copyright = value; 3977 return this; 3978 } 3979 3980 /** 3981 * @return A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource. 3982 */ 3983 public String getCopyright() { 3984 return this.copyright == null ? null : this.copyright.getValue(); 3985 } 3986 3987 /** 3988 * @param value A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource. 3989 */ 3990 public EvidenceVariable setCopyright(String value) { 3991 if (Utilities.noString(value)) 3992 this.copyright = null; 3993 else { 3994 if (this.copyright == null) 3995 this.copyright = new MarkdownType(); 3996 this.copyright.setValue(value); 3997 } 3998 return this; 3999 } 4000 4001 /** 4002 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4003 */ 4004 public StringType getCopyrightLabelElement() { 4005 if (this.copyrightLabel == null) 4006 if (Configuration.errorOnAutoCreate()) 4007 throw new Error("Attempt to auto-create EvidenceVariable.copyrightLabel"); 4008 else if (Configuration.doAutoCreate()) 4009 this.copyrightLabel = new StringType(); // bb 4010 return this.copyrightLabel; 4011 } 4012 4013 public boolean hasCopyrightLabelElement() { 4014 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4015 } 4016 4017 public boolean hasCopyrightLabel() { 4018 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4019 } 4020 4021 /** 4022 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4023 */ 4024 public EvidenceVariable setCopyrightLabelElement(StringType value) { 4025 this.copyrightLabel = value; 4026 return this; 4027 } 4028 4029 /** 4030 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4031 */ 4032 public String getCopyrightLabel() { 4033 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 4034 } 4035 4036 /** 4037 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4038 */ 4039 public EvidenceVariable setCopyrightLabel(String value) { 4040 if (Utilities.noString(value)) 4041 this.copyrightLabel = null; 4042 else { 4043 if (this.copyrightLabel == null) 4044 this.copyrightLabel = new StringType(); 4045 this.copyrightLabel.setValue(value); 4046 } 4047 return this; 4048 } 4049 4050 /** 4051 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4052 4053See guidance around (not) making local changes to elements [here](canonicalresource.html#localization).). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4054 */ 4055 public DateType getApprovalDateElement() { 4056 if (this.approvalDate == null) 4057 if (Configuration.errorOnAutoCreate()) 4058 throw new Error("Attempt to auto-create EvidenceVariable.approvalDate"); 4059 else if (Configuration.doAutoCreate()) 4060 this.approvalDate = new DateType(); // bb 4061 return this.approvalDate; 4062 } 4063 4064 public boolean hasApprovalDateElement() { 4065 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4066 } 4067 4068 public boolean hasApprovalDate() { 4069 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4070 } 4071 4072 /** 4073 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4074 4075See guidance around (not) making local changes to elements [here](canonicalresource.html#localization).). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4076 */ 4077 public EvidenceVariable setApprovalDateElement(DateType value) { 4078 this.approvalDate = value; 4079 return this; 4080 } 4081 4082 /** 4083 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4084 4085See guidance around (not) making local changes to elements [here](canonicalresource.html#localization). 4086 */ 4087 public Date getApprovalDate() { 4088 return this.approvalDate == null ? null : this.approvalDate.getValue(); 4089 } 4090 4091 /** 4092 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4093 4094See guidance around (not) making local changes to elements [here](canonicalresource.html#localization). 4095 */ 4096 public EvidenceVariable setApprovalDate(Date value) { 4097 if (value == null) 4098 this.approvalDate = null; 4099 else { 4100 if (this.approvalDate == null) 4101 this.approvalDate = new DateType(); 4102 this.approvalDate.setValue(value); 4103 } 4104 return this; 4105 } 4106 4107 /** 4108 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4109 */ 4110 public DateType getLastReviewDateElement() { 4111 if (this.lastReviewDate == null) 4112 if (Configuration.errorOnAutoCreate()) 4113 throw new Error("Attempt to auto-create EvidenceVariable.lastReviewDate"); 4114 else if (Configuration.doAutoCreate()) 4115 this.lastReviewDate = new DateType(); // bb 4116 return this.lastReviewDate; 4117 } 4118 4119 public boolean hasLastReviewDateElement() { 4120 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4121 } 4122 4123 public boolean hasLastReviewDate() { 4124 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4125 } 4126 4127 /** 4128 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4129 */ 4130 public EvidenceVariable setLastReviewDateElement(DateType value) { 4131 this.lastReviewDate = value; 4132 return this; 4133 } 4134 4135 /** 4136 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4137 */ 4138 public Date getLastReviewDate() { 4139 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 4140 } 4141 4142 /** 4143 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4144 */ 4145 public EvidenceVariable setLastReviewDate(Date value) { 4146 if (value == null) 4147 this.lastReviewDate = null; 4148 else { 4149 if (this.lastReviewDate == null) 4150 this.lastReviewDate = new DateType(); 4151 this.lastReviewDate.setValue(value); 4152 } 4153 return this; 4154 } 4155 4156 /** 4157 * @return {@link #effectivePeriod} (The period during which the resource content was or is planned to be in active use.) 4158 */ 4159 public Period getEffectivePeriod() { 4160 if (this.effectivePeriod == null) 4161 if (Configuration.errorOnAutoCreate()) 4162 throw new Error("Attempt to auto-create EvidenceVariable.effectivePeriod"); 4163 else if (Configuration.doAutoCreate()) 4164 this.effectivePeriod = new Period(); // cc 4165 return this.effectivePeriod; 4166 } 4167 4168 public boolean hasEffectivePeriod() { 4169 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 4170 } 4171 4172 /** 4173 * @param value {@link #effectivePeriod} (The period during which the resource content was or is planned to be in active use.) 4174 */ 4175 public EvidenceVariable setEffectivePeriod(Period value) { 4176 this.effectivePeriod = value; 4177 return this; 4178 } 4179 4180 /** 4181 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the content.) 4182 */ 4183 public List<ContactDetail> getAuthor() { 4184 if (this.author == null) 4185 this.author = new ArrayList<ContactDetail>(); 4186 return this.author; 4187 } 4188 4189 /** 4190 * @return Returns a reference to <code>this</code> for easy method chaining 4191 */ 4192 public EvidenceVariable setAuthor(List<ContactDetail> theAuthor) { 4193 this.author = theAuthor; 4194 return this; 4195 } 4196 4197 public boolean hasAuthor() { 4198 if (this.author == null) 4199 return false; 4200 for (ContactDetail item : this.author) 4201 if (!item.isEmpty()) 4202 return true; 4203 return false; 4204 } 4205 4206 public ContactDetail addAuthor() { //3 4207 ContactDetail t = new ContactDetail(); 4208 if (this.author == null) 4209 this.author = new ArrayList<ContactDetail>(); 4210 this.author.add(t); 4211 return t; 4212 } 4213 4214 public EvidenceVariable addAuthor(ContactDetail t) { //3 4215 if (t == null) 4216 return this; 4217 if (this.author == null) 4218 this.author = new ArrayList<ContactDetail>(); 4219 this.author.add(t); 4220 return this; 4221 } 4222 4223 /** 4224 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 4225 */ 4226 public ContactDetail getAuthorFirstRep() { 4227 if (getAuthor().isEmpty()) { 4228 addAuthor(); 4229 } 4230 return getAuthor().get(0); 4231 } 4232 4233 /** 4234 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the content.) 4235 */ 4236 public List<ContactDetail> getEditor() { 4237 if (this.editor == null) 4238 this.editor = new ArrayList<ContactDetail>(); 4239 return this.editor; 4240 } 4241 4242 /** 4243 * @return Returns a reference to <code>this</code> for easy method chaining 4244 */ 4245 public EvidenceVariable setEditor(List<ContactDetail> theEditor) { 4246 this.editor = theEditor; 4247 return this; 4248 } 4249 4250 public boolean hasEditor() { 4251 if (this.editor == null) 4252 return false; 4253 for (ContactDetail item : this.editor) 4254 if (!item.isEmpty()) 4255 return true; 4256 return false; 4257 } 4258 4259 public ContactDetail addEditor() { //3 4260 ContactDetail t = new ContactDetail(); 4261 if (this.editor == null) 4262 this.editor = new ArrayList<ContactDetail>(); 4263 this.editor.add(t); 4264 return t; 4265 } 4266 4267 public EvidenceVariable addEditor(ContactDetail t) { //3 4268 if (t == null) 4269 return this; 4270 if (this.editor == null) 4271 this.editor = new ArrayList<ContactDetail>(); 4272 this.editor.add(t); 4273 return this; 4274 } 4275 4276 /** 4277 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 4278 */ 4279 public ContactDetail getEditorFirstRep() { 4280 if (getEditor().isEmpty()) { 4281 addEditor(); 4282 } 4283 return getEditor().get(0); 4284 } 4285 4286 /** 4287 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.) 4288 */ 4289 public List<ContactDetail> getReviewer() { 4290 if (this.reviewer == null) 4291 this.reviewer = new ArrayList<ContactDetail>(); 4292 return this.reviewer; 4293 } 4294 4295 /** 4296 * @return Returns a reference to <code>this</code> for easy method chaining 4297 */ 4298 public EvidenceVariable setReviewer(List<ContactDetail> theReviewer) { 4299 this.reviewer = theReviewer; 4300 return this; 4301 } 4302 4303 public boolean hasReviewer() { 4304 if (this.reviewer == null) 4305 return false; 4306 for (ContactDetail item : this.reviewer) 4307 if (!item.isEmpty()) 4308 return true; 4309 return false; 4310 } 4311 4312 public ContactDetail addReviewer() { //3 4313 ContactDetail t = new ContactDetail(); 4314 if (this.reviewer == null) 4315 this.reviewer = new ArrayList<ContactDetail>(); 4316 this.reviewer.add(t); 4317 return t; 4318 } 4319 4320 public EvidenceVariable addReviewer(ContactDetail t) { //3 4321 if (t == null) 4322 return this; 4323 if (this.reviewer == null) 4324 this.reviewer = new ArrayList<ContactDetail>(); 4325 this.reviewer.add(t); 4326 return this; 4327 } 4328 4329 /** 4330 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 4331 */ 4332 public ContactDetail getReviewerFirstRep() { 4333 if (getReviewer().isEmpty()) { 4334 addReviewer(); 4335 } 4336 return getReviewer().get(0); 4337 } 4338 4339 /** 4340 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.) 4341 */ 4342 public List<ContactDetail> getEndorser() { 4343 if (this.endorser == null) 4344 this.endorser = new ArrayList<ContactDetail>(); 4345 return this.endorser; 4346 } 4347 4348 /** 4349 * @return Returns a reference to <code>this</code> for easy method chaining 4350 */ 4351 public EvidenceVariable setEndorser(List<ContactDetail> theEndorser) { 4352 this.endorser = theEndorser; 4353 return this; 4354 } 4355 4356 public boolean hasEndorser() { 4357 if (this.endorser == null) 4358 return false; 4359 for (ContactDetail item : this.endorser) 4360 if (!item.isEmpty()) 4361 return true; 4362 return false; 4363 } 4364 4365 public ContactDetail addEndorser() { //3 4366 ContactDetail t = new ContactDetail(); 4367 if (this.endorser == null) 4368 this.endorser = new ArrayList<ContactDetail>(); 4369 this.endorser.add(t); 4370 return t; 4371 } 4372 4373 public EvidenceVariable addEndorser(ContactDetail t) { //3 4374 if (t == null) 4375 return this; 4376 if (this.endorser == null) 4377 this.endorser = new ArrayList<ContactDetail>(); 4378 this.endorser.add(t); 4379 return this; 4380 } 4381 4382 /** 4383 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 4384 */ 4385 public ContactDetail getEndorserFirstRep() { 4386 if (getEndorser().isEmpty()) { 4387 addEndorser(); 4388 } 4389 return getEndorser().get(0); 4390 } 4391 4392 /** 4393 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 4394 */ 4395 public List<RelatedArtifact> getRelatedArtifact() { 4396 if (this.relatedArtifact == null) 4397 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4398 return this.relatedArtifact; 4399 } 4400 4401 /** 4402 * @return Returns a reference to <code>this</code> for easy method chaining 4403 */ 4404 public EvidenceVariable setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 4405 this.relatedArtifact = theRelatedArtifact; 4406 return this; 4407 } 4408 4409 public boolean hasRelatedArtifact() { 4410 if (this.relatedArtifact == null) 4411 return false; 4412 for (RelatedArtifact item : this.relatedArtifact) 4413 if (!item.isEmpty()) 4414 return true; 4415 return false; 4416 } 4417 4418 public RelatedArtifact addRelatedArtifact() { //3 4419 RelatedArtifact t = new RelatedArtifact(); 4420 if (this.relatedArtifact == null) 4421 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4422 this.relatedArtifact.add(t); 4423 return t; 4424 } 4425 4426 public EvidenceVariable addRelatedArtifact(RelatedArtifact t) { //3 4427 if (t == null) 4428 return this; 4429 if (this.relatedArtifact == null) 4430 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4431 this.relatedArtifact.add(t); 4432 return this; 4433 } 4434 4435 /** 4436 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 4437 */ 4438 public RelatedArtifact getRelatedArtifactFirstRep() { 4439 if (getRelatedArtifact().isEmpty()) { 4440 addRelatedArtifact(); 4441 } 4442 return getRelatedArtifact().get(0); 4443 } 4444 4445 /** 4446 * @return {@link #actual} (True if the actual variable measured, false if a conceptual representation of the intended variable.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 4447 */ 4448 public BooleanType getActualElement() { 4449 if (this.actual == null) 4450 if (Configuration.errorOnAutoCreate()) 4451 throw new Error("Attempt to auto-create EvidenceVariable.actual"); 4452 else if (Configuration.doAutoCreate()) 4453 this.actual = new BooleanType(); // bb 4454 return this.actual; 4455 } 4456 4457 public boolean hasActualElement() { 4458 return this.actual != null && !this.actual.isEmpty(); 4459 } 4460 4461 public boolean hasActual() { 4462 return this.actual != null && !this.actual.isEmpty(); 4463 } 4464 4465 /** 4466 * @param value {@link #actual} (True if the actual variable measured, false if a conceptual representation of the intended variable.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 4467 */ 4468 public EvidenceVariable setActualElement(BooleanType value) { 4469 this.actual = value; 4470 return this; 4471 } 4472 4473 /** 4474 * @return True if the actual variable measured, false if a conceptual representation of the intended variable. 4475 */ 4476 public boolean getActual() { 4477 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 4478 } 4479 4480 /** 4481 * @param value True if the actual variable measured, false if a conceptual representation of the intended variable. 4482 */ 4483 public EvidenceVariable setActual(boolean value) { 4484 if (this.actual == null) 4485 this.actual = new BooleanType(); 4486 this.actual.setValue(value); 4487 return this; 4488 } 4489 4490 /** 4491 * @return {@link #characteristic} (A defining factor of the EvidenceVariable. Multiple characteristics are applied with "and" semantics.) 4492 */ 4493 public List<EvidenceVariableCharacteristicComponent> getCharacteristic() { 4494 if (this.characteristic == null) 4495 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 4496 return this.characteristic; 4497 } 4498 4499 /** 4500 * @return Returns a reference to <code>this</code> for easy method chaining 4501 */ 4502 public EvidenceVariable setCharacteristic(List<EvidenceVariableCharacteristicComponent> theCharacteristic) { 4503 this.characteristic = theCharacteristic; 4504 return this; 4505 } 4506 4507 public boolean hasCharacteristic() { 4508 if (this.characteristic == null) 4509 return false; 4510 for (EvidenceVariableCharacteristicComponent item : this.characteristic) 4511 if (!item.isEmpty()) 4512 return true; 4513 return false; 4514 } 4515 4516 public EvidenceVariableCharacteristicComponent addCharacteristic() { //3 4517 EvidenceVariableCharacteristicComponent t = new EvidenceVariableCharacteristicComponent(); 4518 if (this.characteristic == null) 4519 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 4520 this.characteristic.add(t); 4521 return t; 4522 } 4523 4524 public EvidenceVariable addCharacteristic(EvidenceVariableCharacteristicComponent t) { //3 4525 if (t == null) 4526 return this; 4527 if (this.characteristic == null) 4528 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 4529 this.characteristic.add(t); 4530 return this; 4531 } 4532 4533 /** 4534 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 4535 */ 4536 public EvidenceVariableCharacteristicComponent getCharacteristicFirstRep() { 4537 if (getCharacteristic().isEmpty()) { 4538 addCharacteristic(); 4539 } 4540 return getCharacteristic().get(0); 4541 } 4542 4543 /** 4544 * @return {@link #handling} (The method of handling in statistical analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 4545 */ 4546 public Enumeration<EvidenceVariableHandling> getHandlingElement() { 4547 if (this.handling == null) 4548 if (Configuration.errorOnAutoCreate()) 4549 throw new Error("Attempt to auto-create EvidenceVariable.handling"); 4550 else if (Configuration.doAutoCreate()) 4551 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); // bb 4552 return this.handling; 4553 } 4554 4555 public boolean hasHandlingElement() { 4556 return this.handling != null && !this.handling.isEmpty(); 4557 } 4558 4559 public boolean hasHandling() { 4560 return this.handling != null && !this.handling.isEmpty(); 4561 } 4562 4563 /** 4564 * @param value {@link #handling} (The method of handling in statistical analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 4565 */ 4566 public EvidenceVariable setHandlingElement(Enumeration<EvidenceVariableHandling> value) { 4567 this.handling = value; 4568 return this; 4569 } 4570 4571 /** 4572 * @return The method of handling in statistical analysis. 4573 */ 4574 public EvidenceVariableHandling getHandling() { 4575 return this.handling == null ? null : this.handling.getValue(); 4576 } 4577 4578 /** 4579 * @param value The method of handling in statistical analysis. 4580 */ 4581 public EvidenceVariable setHandling(EvidenceVariableHandling value) { 4582 if (value == null) 4583 this.handling = null; 4584 else { 4585 if (this.handling == null) 4586 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); 4587 this.handling.setValue(value); 4588 } 4589 return this; 4590 } 4591 4592 /** 4593 * @return {@link #category} (A grouping for ordinal or polychotomous variables.) 4594 */ 4595 public List<EvidenceVariableCategoryComponent> getCategory() { 4596 if (this.category == null) 4597 this.category = new ArrayList<EvidenceVariableCategoryComponent>(); 4598 return this.category; 4599 } 4600 4601 /** 4602 * @return Returns a reference to <code>this</code> for easy method chaining 4603 */ 4604 public EvidenceVariable setCategory(List<EvidenceVariableCategoryComponent> theCategory) { 4605 this.category = theCategory; 4606 return this; 4607 } 4608 4609 public boolean hasCategory() { 4610 if (this.category == null) 4611 return false; 4612 for (EvidenceVariableCategoryComponent item : this.category) 4613 if (!item.isEmpty()) 4614 return true; 4615 return false; 4616 } 4617 4618 public EvidenceVariableCategoryComponent addCategory() { //3 4619 EvidenceVariableCategoryComponent t = new EvidenceVariableCategoryComponent(); 4620 if (this.category == null) 4621 this.category = new ArrayList<EvidenceVariableCategoryComponent>(); 4622 this.category.add(t); 4623 return t; 4624 } 4625 4626 public EvidenceVariable addCategory(EvidenceVariableCategoryComponent t) { //3 4627 if (t == null) 4628 return this; 4629 if (this.category == null) 4630 this.category = new ArrayList<EvidenceVariableCategoryComponent>(); 4631 this.category.add(t); 4632 return this; 4633 } 4634 4635 /** 4636 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 4637 */ 4638 public EvidenceVariableCategoryComponent getCategoryFirstRep() { 4639 if (getCategory().isEmpty()) { 4640 addCategory(); 4641 } 4642 return getCategory().get(0); 4643 } 4644 4645 /** 4646 * not supported on this implementation 4647 */ 4648 @Override 4649 public int getJurisdictionMax() { 4650 return 0; 4651 } 4652 /** 4653 * @return {@link #jurisdiction} (A legal or geographic region in which the evidence variable is intended to be used.) 4654 */ 4655 public List<CodeableConcept> getJurisdiction() { 4656 return new ArrayList<>(); 4657 } 4658 /** 4659 * @return Returns a reference to <code>this</code> for easy method chaining 4660 */ 4661 public EvidenceVariable setJurisdiction(List<CodeableConcept> theJurisdiction) { 4662 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4663 } 4664 public boolean hasJurisdiction() { 4665 return false; 4666 } 4667 4668 public CodeableConcept addJurisdiction() { //3 4669 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4670 } 4671 public EvidenceVariable addJurisdiction(CodeableConcept t) { //3 4672 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4673 } 4674 /** 4675 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {2} 4676 */ 4677 public CodeableConcept getJurisdictionFirstRep() { 4678 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4679 } 4680 /** 4681 * not supported on this implementation 4682 */ 4683 @Override 4684 public int getTopicMax() { 4685 return 0; 4686 } 4687 /** 4688 * @return {@link #topic} (Descriptive topics related to the content of the evidence variable. Topics provide a high-level categorization as well as keywords for the evidence variable that can be useful for filtering and searching.) 4689 */ 4690 public List<CodeableConcept> getTopic() { 4691 return new ArrayList<>(); 4692 } 4693 /** 4694 * @return Returns a reference to <code>this</code> for easy method chaining 4695 */ 4696 public EvidenceVariable setTopic(List<CodeableConcept> theTopic) { 4697 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4698 } 4699 public boolean hasTopic() { 4700 return false; 4701 } 4702 4703 public CodeableConcept addTopic() { //3 4704 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4705 } 4706 public EvidenceVariable addTopic(CodeableConcept t) { //3 4707 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4708 } 4709 /** 4710 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 4711 */ 4712 public CodeableConcept getTopicFirstRep() { 4713 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4714 } 4715 protected void listChildren(List<Property> children) { 4716 super.listChildren(children); 4717 children.add(new Property("url", "uri", "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 0, 1, url)); 4718 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4719 children.add(new Property("version", "string", "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 4720 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 4721 children.add(new Property("name", "string", "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 4722 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the evidence variable.", 0, 1, title)); 4723 children.add(new Property("shortTitle", "string", "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 0, 1, shortTitle)); 4724 children.add(new Property("status", "code", "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status)); 4725 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 4726 children.add(new Property("date", "dateTime", "The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 0, 1, date)); 4727 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.", 0, 1, publisher)); 4728 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4729 children.add(new Property("description", "markdown", "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, description)); 4730 children.add(new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, note)); 4731 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4732 children.add(new Property("purpose", "markdown", "Explanation of why this EvidenceVariable is needed and why it has been designed as it has.", 0, 1, purpose)); 4733 children.add(new Property("copyright", "markdown", "A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.", 0, 1, copyright)); 4734 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 4735 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.\n\nSee guidance around (not) making local changes to elements [here](canonicalresource.html#localization).", 0, 1, approvalDate)); 4736 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 4737 children.add(new Property("effectivePeriod", "Period", "The period during which the resource content was or is planned to be in active use.", 0, 1, effectivePeriod)); 4738 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 4739 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 4740 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 4741 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 4742 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4743 children.add(new Property("actual", "boolean", "True if the actual variable measured, false if a conceptual representation of the intended variable.", 0, 1, actual)); 4744 children.add(new Property("characteristic", "", "A defining factor of the EvidenceVariable. Multiple characteristics are applied with \"and\" semantics.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 4745 children.add(new Property("handling", "code", "The method of handling in statistical analysis.", 0, 1, handling)); 4746 children.add(new Property("category", "", "A grouping for ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, category)); 4747 } 4748 4749 @Override 4750 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4751 switch (_hash) { 4752 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 0, 1, url); 4753 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4754 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 4755 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4756 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4757 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4758 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4759 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 4760 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the evidence variable.", 0, 1, title); 4761 case 1555503932: /*shortTitle*/ return new Property("shortTitle", "string", "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 0, 1, shortTitle); 4762 case -892481550: /*status*/ return new Property("status", "code", "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status); 4763 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 4764 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 0, 1, date); 4765 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.", 0, 1, publisher); 4766 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4767 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, description); 4768 case 3387378: /*note*/ return new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, note); 4769 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4770 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this EvidenceVariable is needed and why it has been designed as it has.", 0, 1, purpose); 4771 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.", 0, 1, copyright); 4772 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 4773 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.\n\nSee guidance around (not) making local changes to elements [here](canonicalresource.html#localization).", 0, 1, approvalDate); 4774 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 4775 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the resource content was or is planned to be in active use.", 0, 1, effectivePeriod); 4776 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 4777 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 4778 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 4779 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 4780 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4781 case -1422939762: /*actual*/ return new Property("actual", "boolean", "True if the actual variable measured, false if a conceptual representation of the intended variable.", 0, 1, actual); 4782 case 366313883: /*characteristic*/ return new Property("characteristic", "", "A defining factor of the EvidenceVariable. Multiple characteristics are applied with \"and\" semantics.", 0, java.lang.Integer.MAX_VALUE, characteristic); 4783 case 2072805: /*handling*/ return new Property("handling", "code", "The method of handling in statistical analysis.", 0, 1, handling); 4784 case 50511102: /*category*/ return new Property("category", "", "A grouping for ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, category); 4785 default: return super.getNamedProperty(_hash, _name, _checkValid); 4786 } 4787 4788 } 4789 4790 @Override 4791 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4792 switch (hash) { 4793 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4794 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4795 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4796 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 4797 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4798 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4799 case 1555503932: /*shortTitle*/ return this.shortTitle == null ? new Base[0] : new Base[] {this.shortTitle}; // StringType 4800 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4801 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4802 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4803 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4804 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4805 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4806 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4807 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4808 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4809 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4810 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 4811 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 4812 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 4813 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 4814 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4815 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4816 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4817 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4818 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4819 case -1422939762: /*actual*/ return this.actual == null ? new Base[0] : new Base[] {this.actual}; // BooleanType 4820 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceVariableCharacteristicComponent 4821 case 2072805: /*handling*/ return this.handling == null ? new Base[0] : new Base[] {this.handling}; // Enumeration<EvidenceVariableHandling> 4822 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // EvidenceVariableCategoryComponent 4823 default: return super.getProperty(hash, name, checkValid); 4824 } 4825 4826 } 4827 4828 @Override 4829 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4830 switch (hash) { 4831 case 116079: // url 4832 this.url = TypeConvertor.castToUri(value); // UriType 4833 return value; 4834 case -1618432855: // identifier 4835 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4836 return value; 4837 case 351608024: // version 4838 this.version = TypeConvertor.castToString(value); // StringType 4839 return value; 4840 case 1508158071: // versionAlgorithm 4841 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4842 return value; 4843 case 3373707: // name 4844 this.name = TypeConvertor.castToString(value); // StringType 4845 return value; 4846 case 110371416: // title 4847 this.title = TypeConvertor.castToString(value); // StringType 4848 return value; 4849 case 1555503932: // shortTitle 4850 this.shortTitle = TypeConvertor.castToString(value); // StringType 4851 return value; 4852 case -892481550: // status 4853 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4854 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4855 return value; 4856 case -404562712: // experimental 4857 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4858 return value; 4859 case 3076014: // date 4860 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4861 return value; 4862 case 1447404028: // publisher 4863 this.publisher = TypeConvertor.castToString(value); // StringType 4864 return value; 4865 case 951526432: // contact 4866 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4867 return value; 4868 case -1724546052: // description 4869 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4870 return value; 4871 case 3387378: // note 4872 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4873 return value; 4874 case -669707736: // useContext 4875 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4876 return value; 4877 case -220463842: // purpose 4878 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4879 return value; 4880 case 1522889671: // copyright 4881 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4882 return value; 4883 case 765157229: // copyrightLabel 4884 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4885 return value; 4886 case 223539345: // approvalDate 4887 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4888 return value; 4889 case -1687512484: // lastReviewDate 4890 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4891 return value; 4892 case -403934648: // effectivePeriod 4893 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4894 return value; 4895 case -1406328437: // author 4896 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4897 return value; 4898 case -1307827859: // editor 4899 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4900 return value; 4901 case -261190139: // reviewer 4902 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4903 return value; 4904 case 1740277666: // endorser 4905 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4906 return value; 4907 case 666807069: // relatedArtifact 4908 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4909 return value; 4910 case -1422939762: // actual 4911 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 4912 return value; 4913 case 366313883: // characteristic 4914 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); // EvidenceVariableCharacteristicComponent 4915 return value; 4916 case 2072805: // handling 4917 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 4918 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 4919 return value; 4920 case 50511102: // category 4921 this.getCategory().add((EvidenceVariableCategoryComponent) value); // EvidenceVariableCategoryComponent 4922 return value; 4923 default: return super.setProperty(hash, name, value); 4924 } 4925 4926 } 4927 4928 @Override 4929 public Base setProperty(String name, Base value) throws FHIRException { 4930 if (name.equals("url")) { 4931 this.url = TypeConvertor.castToUri(value); // UriType 4932 } else if (name.equals("identifier")) { 4933 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4934 } else if (name.equals("version")) { 4935 this.version = TypeConvertor.castToString(value); // StringType 4936 } else if (name.equals("versionAlgorithm[x]")) { 4937 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4938 } else if (name.equals("name")) { 4939 this.name = TypeConvertor.castToString(value); // StringType 4940 } else if (name.equals("title")) { 4941 this.title = TypeConvertor.castToString(value); // StringType 4942 } else if (name.equals("shortTitle")) { 4943 this.shortTitle = TypeConvertor.castToString(value); // StringType 4944 } else if (name.equals("status")) { 4945 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4946 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4947 } else if (name.equals("experimental")) { 4948 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4949 } else if (name.equals("date")) { 4950 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4951 } else if (name.equals("publisher")) { 4952 this.publisher = TypeConvertor.castToString(value); // StringType 4953 } else if (name.equals("contact")) { 4954 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4955 } else if (name.equals("description")) { 4956 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4957 } else if (name.equals("note")) { 4958 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4959 } else if (name.equals("useContext")) { 4960 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4961 } else if (name.equals("purpose")) { 4962 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4963 } else if (name.equals("copyright")) { 4964 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4965 } else if (name.equals("copyrightLabel")) { 4966 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4967 } else if (name.equals("approvalDate")) { 4968 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4969 } else if (name.equals("lastReviewDate")) { 4970 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4971 } else if (name.equals("effectivePeriod")) { 4972 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4973 } else if (name.equals("author")) { 4974 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 4975 } else if (name.equals("editor")) { 4976 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 4977 } else if (name.equals("reviewer")) { 4978 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 4979 } else if (name.equals("endorser")) { 4980 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 4981 } else if (name.equals("relatedArtifact")) { 4982 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4983 } else if (name.equals("actual")) { 4984 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 4985 } else if (name.equals("characteristic")) { 4986 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); 4987 } else if (name.equals("handling")) { 4988 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 4989 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 4990 } else if (name.equals("category")) { 4991 this.getCategory().add((EvidenceVariableCategoryComponent) value); 4992 } else 4993 return super.setProperty(name, value); 4994 return value; 4995 } 4996 4997 @Override 4998 public void removeChild(String name, Base value) throws FHIRException { 4999 if (name.equals("url")) { 5000 this.url = null; 5001 } else if (name.equals("identifier")) { 5002 this.getIdentifier().remove(value); 5003 } else if (name.equals("version")) { 5004 this.version = null; 5005 } else if (name.equals("versionAlgorithm[x]")) { 5006 this.versionAlgorithm = null; 5007 } else if (name.equals("name")) { 5008 this.name = null; 5009 } else if (name.equals("title")) { 5010 this.title = null; 5011 } else if (name.equals("shortTitle")) { 5012 this.shortTitle = null; 5013 } else if (name.equals("status")) { 5014 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5015 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5016 } else if (name.equals("experimental")) { 5017 this.experimental = null; 5018 } else if (name.equals("date")) { 5019 this.date = null; 5020 } else if (name.equals("publisher")) { 5021 this.publisher = null; 5022 } else if (name.equals("contact")) { 5023 this.getContact().remove(value); 5024 } else if (name.equals("description")) { 5025 this.description = null; 5026 } else if (name.equals("note")) { 5027 this.getNote().remove(value); 5028 } else if (name.equals("useContext")) { 5029 this.getUseContext().remove(value); 5030 } else if (name.equals("purpose")) { 5031 this.purpose = null; 5032 } else if (name.equals("copyright")) { 5033 this.copyright = null; 5034 } else if (name.equals("copyrightLabel")) { 5035 this.copyrightLabel = null; 5036 } else if (name.equals("approvalDate")) { 5037 this.approvalDate = null; 5038 } else if (name.equals("lastReviewDate")) { 5039 this.lastReviewDate = null; 5040 } else if (name.equals("effectivePeriod")) { 5041 this.effectivePeriod = null; 5042 } else if (name.equals("author")) { 5043 this.getAuthor().remove(value); 5044 } else if (name.equals("editor")) { 5045 this.getEditor().remove(value); 5046 } else if (name.equals("reviewer")) { 5047 this.getReviewer().remove(value); 5048 } else if (name.equals("endorser")) { 5049 this.getEndorser().remove(value); 5050 } else if (name.equals("relatedArtifact")) { 5051 this.getRelatedArtifact().remove(value); 5052 } else if (name.equals("actual")) { 5053 this.actual = null; 5054 } else if (name.equals("characteristic")) { 5055 this.getCharacteristic().remove((EvidenceVariableCharacteristicComponent) value); 5056 } else if (name.equals("handling")) { 5057 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 5058 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 5059 } else if (name.equals("category")) { 5060 this.getCategory().remove((EvidenceVariableCategoryComponent) value); 5061 } else 5062 super.removeChild(name, value); 5063 5064 } 5065 5066 @Override 5067 public Base makeProperty(int hash, String name) throws FHIRException { 5068 switch (hash) { 5069 case 116079: return getUrlElement(); 5070 case -1618432855: return addIdentifier(); 5071 case 351608024: return getVersionElement(); 5072 case -115699031: return getVersionAlgorithm(); 5073 case 1508158071: return getVersionAlgorithm(); 5074 case 3373707: return getNameElement(); 5075 case 110371416: return getTitleElement(); 5076 case 1555503932: return getShortTitleElement(); 5077 case -892481550: return getStatusElement(); 5078 case -404562712: return getExperimentalElement(); 5079 case 3076014: return getDateElement(); 5080 case 1447404028: return getPublisherElement(); 5081 case 951526432: return addContact(); 5082 case -1724546052: return getDescriptionElement(); 5083 case 3387378: return addNote(); 5084 case -669707736: return addUseContext(); 5085 case -220463842: return getPurposeElement(); 5086 case 1522889671: return getCopyrightElement(); 5087 case 765157229: return getCopyrightLabelElement(); 5088 case 223539345: return getApprovalDateElement(); 5089 case -1687512484: return getLastReviewDateElement(); 5090 case -403934648: return getEffectivePeriod(); 5091 case -1406328437: return addAuthor(); 5092 case -1307827859: return addEditor(); 5093 case -261190139: return addReviewer(); 5094 case 1740277666: return addEndorser(); 5095 case 666807069: return addRelatedArtifact(); 5096 case -1422939762: return getActualElement(); 5097 case 366313883: return addCharacteristic(); 5098 case 2072805: return getHandlingElement(); 5099 case 50511102: return addCategory(); 5100 default: return super.makeProperty(hash, name); 5101 } 5102 5103 } 5104 5105 @Override 5106 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5107 switch (hash) { 5108 case 116079: /*url*/ return new String[] {"uri"}; 5109 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5110 case 351608024: /*version*/ return new String[] {"string"}; 5111 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 5112 case 3373707: /*name*/ return new String[] {"string"}; 5113 case 110371416: /*title*/ return new String[] {"string"}; 5114 case 1555503932: /*shortTitle*/ return new String[] {"string"}; 5115 case -892481550: /*status*/ return new String[] {"code"}; 5116 case -404562712: /*experimental*/ return new String[] {"boolean"}; 5117 case 3076014: /*date*/ return new String[] {"dateTime"}; 5118 case 1447404028: /*publisher*/ return new String[] {"string"}; 5119 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 5120 case -1724546052: /*description*/ return new String[] {"markdown"}; 5121 case 3387378: /*note*/ return new String[] {"Annotation"}; 5122 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5123 case -220463842: /*purpose*/ return new String[] {"markdown"}; 5124 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5125 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 5126 case 223539345: /*approvalDate*/ return new String[] {"date"}; 5127 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 5128 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 5129 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 5130 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 5131 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 5132 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 5133 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 5134 case -1422939762: /*actual*/ return new String[] {"boolean"}; 5135 case 366313883: /*characteristic*/ return new String[] {}; 5136 case 2072805: /*handling*/ return new String[] {"code"}; 5137 case 50511102: /*category*/ return new String[] {}; 5138 default: return super.getTypesForProperty(hash, name); 5139 } 5140 5141 } 5142 5143 @Override 5144 public Base addChild(String name) throws FHIRException { 5145 if (name.equals("url")) { 5146 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.url"); 5147 } 5148 else if (name.equals("identifier")) { 5149 return addIdentifier(); 5150 } 5151 else if (name.equals("version")) { 5152 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.version"); 5153 } 5154 else if (name.equals("versionAlgorithmString")) { 5155 this.versionAlgorithm = new StringType(); 5156 return this.versionAlgorithm; 5157 } 5158 else if (name.equals("versionAlgorithmCoding")) { 5159 this.versionAlgorithm = new Coding(); 5160 return this.versionAlgorithm; 5161 } 5162 else if (name.equals("name")) { 5163 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.name"); 5164 } 5165 else if (name.equals("title")) { 5166 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.title"); 5167 } 5168 else if (name.equals("shortTitle")) { 5169 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.shortTitle"); 5170 } 5171 else if (name.equals("status")) { 5172 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.status"); 5173 } 5174 else if (name.equals("experimental")) { 5175 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.experimental"); 5176 } 5177 else if (name.equals("date")) { 5178 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.date"); 5179 } 5180 else if (name.equals("publisher")) { 5181 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.publisher"); 5182 } 5183 else if (name.equals("contact")) { 5184 return addContact(); 5185 } 5186 else if (name.equals("description")) { 5187 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.description"); 5188 } 5189 else if (name.equals("note")) { 5190 return addNote(); 5191 } 5192 else if (name.equals("useContext")) { 5193 return addUseContext(); 5194 } 5195 else if (name.equals("purpose")) { 5196 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.purpose"); 5197 } 5198 else if (name.equals("copyright")) { 5199 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.copyright"); 5200 } 5201 else if (name.equals("copyrightLabel")) { 5202 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.copyrightLabel"); 5203 } 5204 else if (name.equals("approvalDate")) { 5205 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.approvalDate"); 5206 } 5207 else if (name.equals("lastReviewDate")) { 5208 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.lastReviewDate"); 5209 } 5210 else if (name.equals("effectivePeriod")) { 5211 this.effectivePeriod = new Period(); 5212 return this.effectivePeriod; 5213 } 5214 else if (name.equals("author")) { 5215 return addAuthor(); 5216 } 5217 else if (name.equals("editor")) { 5218 return addEditor(); 5219 } 5220 else if (name.equals("reviewer")) { 5221 return addReviewer(); 5222 } 5223 else if (name.equals("endorser")) { 5224 return addEndorser(); 5225 } 5226 else if (name.equals("relatedArtifact")) { 5227 return addRelatedArtifact(); 5228 } 5229 else if (name.equals("actual")) { 5230 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.actual"); 5231 } 5232 else if (name.equals("characteristic")) { 5233 return addCharacteristic(); 5234 } 5235 else if (name.equals("handling")) { 5236 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.handling"); 5237 } 5238 else if (name.equals("category")) { 5239 return addCategory(); 5240 } 5241 else 5242 return super.addChild(name); 5243 } 5244 5245 public String fhirType() { 5246 return "EvidenceVariable"; 5247 5248 } 5249 5250 public EvidenceVariable copy() { 5251 EvidenceVariable dst = new EvidenceVariable(); 5252 copyValues(dst); 5253 return dst; 5254 } 5255 5256 public void copyValues(EvidenceVariable dst) { 5257 super.copyValues(dst); 5258 dst.url = url == null ? null : url.copy(); 5259 if (identifier != null) { 5260 dst.identifier = new ArrayList<Identifier>(); 5261 for (Identifier i : identifier) 5262 dst.identifier.add(i.copy()); 5263 }; 5264 dst.version = version == null ? null : version.copy(); 5265 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 5266 dst.name = name == null ? null : name.copy(); 5267 dst.title = title == null ? null : title.copy(); 5268 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 5269 dst.status = status == null ? null : status.copy(); 5270 dst.experimental = experimental == null ? null : experimental.copy(); 5271 dst.date = date == null ? null : date.copy(); 5272 dst.publisher = publisher == null ? null : publisher.copy(); 5273 if (contact != null) { 5274 dst.contact = new ArrayList<ContactDetail>(); 5275 for (ContactDetail i : contact) 5276 dst.contact.add(i.copy()); 5277 }; 5278 dst.description = description == null ? null : description.copy(); 5279 if (note != null) { 5280 dst.note = new ArrayList<Annotation>(); 5281 for (Annotation i : note) 5282 dst.note.add(i.copy()); 5283 }; 5284 if (useContext != null) { 5285 dst.useContext = new ArrayList<UsageContext>(); 5286 for (UsageContext i : useContext) 5287 dst.useContext.add(i.copy()); 5288 }; 5289 dst.purpose = purpose == null ? null : purpose.copy(); 5290 dst.copyright = copyright == null ? null : copyright.copy(); 5291 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 5292 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5293 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5294 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5295 if (author != null) { 5296 dst.author = new ArrayList<ContactDetail>(); 5297 for (ContactDetail i : author) 5298 dst.author.add(i.copy()); 5299 }; 5300 if (editor != null) { 5301 dst.editor = new ArrayList<ContactDetail>(); 5302 for (ContactDetail i : editor) 5303 dst.editor.add(i.copy()); 5304 }; 5305 if (reviewer != null) { 5306 dst.reviewer = new ArrayList<ContactDetail>(); 5307 for (ContactDetail i : reviewer) 5308 dst.reviewer.add(i.copy()); 5309 }; 5310 if (endorser != null) { 5311 dst.endorser = new ArrayList<ContactDetail>(); 5312 for (ContactDetail i : endorser) 5313 dst.endorser.add(i.copy()); 5314 }; 5315 if (relatedArtifact != null) { 5316 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5317 for (RelatedArtifact i : relatedArtifact) 5318 dst.relatedArtifact.add(i.copy()); 5319 }; 5320 dst.actual = actual == null ? null : actual.copy(); 5321 if (characteristic != null) { 5322 dst.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 5323 for (EvidenceVariableCharacteristicComponent i : characteristic) 5324 dst.characteristic.add(i.copy()); 5325 }; 5326 dst.handling = handling == null ? null : handling.copy(); 5327 if (category != null) { 5328 dst.category = new ArrayList<EvidenceVariableCategoryComponent>(); 5329 for (EvidenceVariableCategoryComponent i : category) 5330 dst.category.add(i.copy()); 5331 }; 5332 } 5333 5334 protected EvidenceVariable typedCopy() { 5335 return copy(); 5336 } 5337 5338 @Override 5339 public boolean equalsDeep(Base other_) { 5340 if (!super.equalsDeep(other_)) 5341 return false; 5342 if (!(other_ instanceof EvidenceVariable)) 5343 return false; 5344 EvidenceVariable o = (EvidenceVariable) other_; 5345 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 5346 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 5347 && compareDeep(shortTitle, o.shortTitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 5348 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 5349 && compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(useContext, o.useContext, true) 5350 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 5351 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5352 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(author, o.author, true) 5353 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 5354 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(actual, o.actual, true) 5355 && compareDeep(characteristic, o.characteristic, true) && compareDeep(handling, o.handling, true) 5356 && compareDeep(category, o.category, true); 5357 } 5358 5359 @Override 5360 public boolean equalsShallow(Base other_) { 5361 if (!super.equalsShallow(other_)) 5362 return false; 5363 if (!(other_ instanceof EvidenceVariable)) 5364 return false; 5365 EvidenceVariable o = (EvidenceVariable) other_; 5366 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 5367 && compareValues(title, o.title, true) && compareValues(shortTitle, o.shortTitle, true) && compareValues(status, o.status, true) 5368 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 5369 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 5370 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(approvalDate, o.approvalDate, true) 5371 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(actual, o.actual, true) && compareValues(handling, o.handling, true) 5372 ; 5373 } 5374 5375 public boolean isEmpty() { 5376 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 5377 , versionAlgorithm, name, title, shortTitle, status, experimental, date, publisher 5378 , contact, description, note, useContext, purpose, copyright, copyrightLabel, approvalDate 5379 , lastReviewDate, effectivePeriod, author, editor, reviewer, endorser, relatedArtifact 5380 , actual, characteristic, handling, category); 5381 } 5382 5383 @Override 5384 public ResourceType getResourceType() { 5385 return ResourceType.EvidenceVariable; 5386 } 5387 5388 /** 5389 * Search parameter: <b>context-quantity</b> 5390 * <p> 5391 * Description: <b>Multiple Resources: 5392 5393* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5394* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5395* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5396* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5397* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5398* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5399* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5400* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5401* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5402* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5403* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5404* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5405* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5406* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5407* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5408* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5409* [Library](library.html): A quantity- or range-valued use context assigned to the library 5410* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5411* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5412* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5413* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5414* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5415* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5416* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5417* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5418* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5419* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5420* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5421* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5422* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5423</b><br> 5424 * Type: <b>quantity</b><br> 5425 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5426 * </p> 5427 */ 5428 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 5429 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5430 /** 5431 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5432 * <p> 5433 * Description: <b>Multiple Resources: 5434 5435* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5436* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5437* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5438* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5439* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5440* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5441* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5442* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5443* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5444* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5445* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5446* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5447* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5448* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5449* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5450* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5451* [Library](library.html): A quantity- or range-valued use context assigned to the library 5452* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5453* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5454* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5455* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5456* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5457* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5458* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5459* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5460* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5461* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5462* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5463* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5464* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5465</b><br> 5466 * Type: <b>quantity</b><br> 5467 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5468 * </p> 5469 */ 5470 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 5471 5472 /** 5473 * Search parameter: <b>context-type-quantity</b> 5474 * <p> 5475 * Description: <b>Multiple Resources: 5476 5477* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5478* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5479* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5480* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5481* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5482* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5483* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5484* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5485* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5486* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5487* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5488* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5489* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5490* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5491* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5492* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5493* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5494* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5495* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5496* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5497* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5498* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5499* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5500* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5501* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5502* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5503* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5504* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5505* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5506* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5507</b><br> 5508 * Type: <b>composite</b><br> 5509 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5510 * </p> 5511 */ 5512 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 5513 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5514 /** 5515 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 5516 * <p> 5517 * Description: <b>Multiple Resources: 5518 5519* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5520* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5521* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5522* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5523* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5524* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5525* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5526* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5527* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5528* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5529* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5530* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5531* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5532* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5533* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5534* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5535* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5536* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5537* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5538* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5539* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5540* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5541* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5542* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5543* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5544* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5545* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5546* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5547* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5548* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5549</b><br> 5550 * Type: <b>composite</b><br> 5551 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5552 * </p> 5553 */ 5554 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 5555 5556 /** 5557 * Search parameter: <b>context-type-value</b> 5558 * <p> 5559 * Description: <b>Multiple Resources: 5560 5561* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5562* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5563* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5564* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5565* [Citation](citation.html): A use context type and value assigned to the citation 5566* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5567* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5568* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5569* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5570* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5571* [Evidence](evidence.html): A use context type and value assigned to the evidence 5572* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5573* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5574* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5575* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5576* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5577* [Library](library.html): A use context type and value assigned to the library 5578* [Measure](measure.html): A use context type and value assigned to the measure 5579* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5580* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5581* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5582* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5583* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5584* [Requirements](requirements.html): A use context type and value assigned to the requirements 5585* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5586* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5587* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5588* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5589* [TestScript](testscript.html): A use context type and value assigned to the test script 5590* [ValueSet](valueset.html): A use context type and value assigned to the value set 5591</b><br> 5592 * Type: <b>composite</b><br> 5593 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5594 * </p> 5595 */ 5596 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 5597 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5598 /** 5599 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5600 * <p> 5601 * Description: <b>Multiple Resources: 5602 5603* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5604* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5605* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5606* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5607* [Citation](citation.html): A use context type and value assigned to the citation 5608* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5609* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5610* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5611* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5612* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5613* [Evidence](evidence.html): A use context type and value assigned to the evidence 5614* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5615* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5616* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5617* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5618* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5619* [Library](library.html): A use context type and value assigned to the library 5620* [Measure](measure.html): A use context type and value assigned to the measure 5621* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5622* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5623* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5624* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5625* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5626* [Requirements](requirements.html): A use context type and value assigned to the requirements 5627* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5628* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5629* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5630* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5631* [TestScript](testscript.html): A use context type and value assigned to the test script 5632* [ValueSet](valueset.html): A use context type and value assigned to the value set 5633</b><br> 5634 * Type: <b>composite</b><br> 5635 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5636 * </p> 5637 */ 5638 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 5639 5640 /** 5641 * Search parameter: <b>context-type</b> 5642 * <p> 5643 * Description: <b>Multiple Resources: 5644 5645* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5646* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5647* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5648* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5649* [Citation](citation.html): A type of use context assigned to the citation 5650* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5651* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5652* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5653* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5654* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5655* [Evidence](evidence.html): A type of use context assigned to the evidence 5656* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5657* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5658* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5659* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5660* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5661* [Library](library.html): A type of use context assigned to the library 5662* [Measure](measure.html): A type of use context assigned to the measure 5663* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5664* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5665* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5666* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5667* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5668* [Requirements](requirements.html): A type of use context assigned to the requirements 5669* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5670* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5671* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5672* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5673* [TestScript](testscript.html): A type of use context assigned to the test script 5674* [ValueSet](valueset.html): A type of use context assigned to the value set 5675</b><br> 5676 * Type: <b>token</b><br> 5677 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5678 * </p> 5679 */ 5680 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 5681 public static final String SP_CONTEXT_TYPE = "context-type"; 5682 /** 5683 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5684 * <p> 5685 * Description: <b>Multiple Resources: 5686 5687* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5688* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5689* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5690* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5691* [Citation](citation.html): A type of use context assigned to the citation 5692* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5693* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5694* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5695* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5696* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5697* [Evidence](evidence.html): A type of use context assigned to the evidence 5698* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5699* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5700* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5701* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5702* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5703* [Library](library.html): A type of use context assigned to the library 5704* [Measure](measure.html): A type of use context assigned to the measure 5705* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5706* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5707* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5708* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5709* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5710* [Requirements](requirements.html): A type of use context assigned to the requirements 5711* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5712* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5713* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5714* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5715* [TestScript](testscript.html): A type of use context assigned to the test script 5716* [ValueSet](valueset.html): A type of use context assigned to the value set 5717</b><br> 5718 * Type: <b>token</b><br> 5719 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5720 * </p> 5721 */ 5722 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 5723 5724 /** 5725 * Search parameter: <b>context</b> 5726 * <p> 5727 * Description: <b>Multiple Resources: 5728 5729* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5730* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5731* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5732* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5733* [Citation](citation.html): A use context assigned to the citation 5734* [CodeSystem](codesystem.html): A use context assigned to the code system 5735* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5736* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5737* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5738* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5739* [Evidence](evidence.html): A use context assigned to the evidence 5740* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5741* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5742* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5743* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5744* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5745* [Library](library.html): A use context assigned to the library 5746* [Measure](measure.html): A use context assigned to the measure 5747* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5748* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5749* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5750* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5751* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5752* [Requirements](requirements.html): A use context assigned to the requirements 5753* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5754* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5755* [StructureMap](structuremap.html): A use context assigned to the structure map 5756* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5757* [TestScript](testscript.html): A use context assigned to the test script 5758* [ValueSet](valueset.html): A use context assigned to the value set 5759</b><br> 5760 * Type: <b>token</b><br> 5761 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5762 * </p> 5763 */ 5764 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 5765 public static final String SP_CONTEXT = "context"; 5766 /** 5767 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5768 * <p> 5769 * Description: <b>Multiple Resources: 5770 5771* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5772* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5773* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5774* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5775* [Citation](citation.html): A use context assigned to the citation 5776* [CodeSystem](codesystem.html): A use context assigned to the code system 5777* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5778* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5779* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5780* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5781* [Evidence](evidence.html): A use context assigned to the evidence 5782* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5783* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5784* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5785* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5786* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5787* [Library](library.html): A use context assigned to the library 5788* [Measure](measure.html): A use context assigned to the measure 5789* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5790* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5791* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5792* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5793* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5794* [Requirements](requirements.html): A use context assigned to the requirements 5795* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5796* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5797* [StructureMap](structuremap.html): A use context assigned to the structure map 5798* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5799* [TestScript](testscript.html): A use context assigned to the test script 5800* [ValueSet](valueset.html): A use context assigned to the value set 5801</b><br> 5802 * Type: <b>token</b><br> 5803 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5804 * </p> 5805 */ 5806 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 5807 5808 /** 5809 * Search parameter: <b>date</b> 5810 * <p> 5811 * Description: <b>Multiple Resources: 5812 5813* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5814* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5815* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5816* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5817* [Citation](citation.html): The citation publication date 5818* [CodeSystem](codesystem.html): The code system publication date 5819* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5820* [ConceptMap](conceptmap.html): The concept map publication date 5821* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5822* [EventDefinition](eventdefinition.html): The event definition publication date 5823* [Evidence](evidence.html): The evidence publication date 5824* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5825* [ExampleScenario](examplescenario.html): The example scenario publication date 5826* [GraphDefinition](graphdefinition.html): The graph definition publication date 5827* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5828* [Library](library.html): The library publication date 5829* [Measure](measure.html): The measure publication date 5830* [MessageDefinition](messagedefinition.html): The message definition publication date 5831* [NamingSystem](namingsystem.html): The naming system publication date 5832* [OperationDefinition](operationdefinition.html): The operation definition publication date 5833* [PlanDefinition](plandefinition.html): The plan definition publication date 5834* [Questionnaire](questionnaire.html): The questionnaire publication date 5835* [Requirements](requirements.html): The requirements publication date 5836* [SearchParameter](searchparameter.html): The search parameter publication date 5837* [StructureDefinition](structuredefinition.html): The structure definition publication date 5838* [StructureMap](structuremap.html): The structure map publication date 5839* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5840* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5841* [TestScript](testscript.html): The test script publication date 5842* [ValueSet](valueset.html): The value set publication date 5843</b><br> 5844 * Type: <b>date</b><br> 5845 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5846 * </p> 5847 */ 5848 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 5849 public static final String SP_DATE = "date"; 5850 /** 5851 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5852 * <p> 5853 * Description: <b>Multiple Resources: 5854 5855* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5856* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5857* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5858* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5859* [Citation](citation.html): The citation publication date 5860* [CodeSystem](codesystem.html): The code system publication date 5861* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5862* [ConceptMap](conceptmap.html): The concept map publication date 5863* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5864* [EventDefinition](eventdefinition.html): The event definition publication date 5865* [Evidence](evidence.html): The evidence publication date 5866* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5867* [ExampleScenario](examplescenario.html): The example scenario publication date 5868* [GraphDefinition](graphdefinition.html): The graph definition publication date 5869* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5870* [Library](library.html): The library publication date 5871* [Measure](measure.html): The measure publication date 5872* [MessageDefinition](messagedefinition.html): The message definition publication date 5873* [NamingSystem](namingsystem.html): The naming system publication date 5874* [OperationDefinition](operationdefinition.html): The operation definition publication date 5875* [PlanDefinition](plandefinition.html): The plan definition publication date 5876* [Questionnaire](questionnaire.html): The questionnaire publication date 5877* [Requirements](requirements.html): The requirements publication date 5878* [SearchParameter](searchparameter.html): The search parameter publication date 5879* [StructureDefinition](structuredefinition.html): The structure definition publication date 5880* [StructureMap](structuremap.html): The structure map publication date 5881* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5882* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5883* [TestScript](testscript.html): The test script publication date 5884* [ValueSet](valueset.html): The value set publication date 5885</b><br> 5886 * Type: <b>date</b><br> 5887 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5888 * </p> 5889 */ 5890 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5891 5892 /** 5893 * Search parameter: <b>description</b> 5894 * <p> 5895 * Description: <b>Multiple Resources: 5896 5897* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5898* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5899* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5900* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5901* [Citation](citation.html): The description of the citation 5902* [CodeSystem](codesystem.html): The description of the code system 5903* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5904* [ConceptMap](conceptmap.html): The description of the concept map 5905* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5906* [EventDefinition](eventdefinition.html): The description of the event definition 5907* [Evidence](evidence.html): The description of the evidence 5908* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5909* [GraphDefinition](graphdefinition.html): The description of the graph definition 5910* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5911* [Library](library.html): The description of the library 5912* [Measure](measure.html): The description of the measure 5913* [MessageDefinition](messagedefinition.html): The description of the message definition 5914* [NamingSystem](namingsystem.html): The description of the naming system 5915* [OperationDefinition](operationdefinition.html): The description of the operation definition 5916* [PlanDefinition](plandefinition.html): The description of the plan definition 5917* [Questionnaire](questionnaire.html): The description of the questionnaire 5918* [Requirements](requirements.html): The description of the requirements 5919* [SearchParameter](searchparameter.html): The description of the search parameter 5920* [StructureDefinition](structuredefinition.html): The description of the structure definition 5921* [StructureMap](structuremap.html): The description of the structure map 5922* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5923* [TestScript](testscript.html): The description of the test script 5924* [ValueSet](valueset.html): The description of the value set 5925</b><br> 5926 * Type: <b>string</b><br> 5927 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5928 * </p> 5929 */ 5930 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 5931 public static final String SP_DESCRIPTION = "description"; 5932 /** 5933 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5934 * <p> 5935 * Description: <b>Multiple Resources: 5936 5937* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5938* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5939* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5940* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5941* [Citation](citation.html): The description of the citation 5942* [CodeSystem](codesystem.html): The description of the code system 5943* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5944* [ConceptMap](conceptmap.html): The description of the concept map 5945* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5946* [EventDefinition](eventdefinition.html): The description of the event definition 5947* [Evidence](evidence.html): The description of the evidence 5948* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5949* [GraphDefinition](graphdefinition.html): The description of the graph definition 5950* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5951* [Library](library.html): The description of the library 5952* [Measure](measure.html): The description of the measure 5953* [MessageDefinition](messagedefinition.html): The description of the message definition 5954* [NamingSystem](namingsystem.html): The description of the naming system 5955* [OperationDefinition](operationdefinition.html): The description of the operation definition 5956* [PlanDefinition](plandefinition.html): The description of the plan definition 5957* [Questionnaire](questionnaire.html): The description of the questionnaire 5958* [Requirements](requirements.html): The description of the requirements 5959* [SearchParameter](searchparameter.html): The description of the search parameter 5960* [StructureDefinition](structuredefinition.html): The description of the structure definition 5961* [StructureMap](structuremap.html): The description of the structure map 5962* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5963* [TestScript](testscript.html): The description of the test script 5964* [ValueSet](valueset.html): The description of the value set 5965</b><br> 5966 * Type: <b>string</b><br> 5967 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5968 * </p> 5969 */ 5970 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5971 5972 /** 5973 * Search parameter: <b>identifier</b> 5974 * <p> 5975 * Description: <b>Multiple Resources: 5976 5977* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5978* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5979* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5980* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5981* [Citation](citation.html): External identifier for the citation 5982* [CodeSystem](codesystem.html): External identifier for the code system 5983* [ConceptMap](conceptmap.html): External identifier for the concept map 5984* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5985* [EventDefinition](eventdefinition.html): External identifier for the event definition 5986* [Evidence](evidence.html): External identifier for the evidence 5987* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5988* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5989* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5990* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5991* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5992* [Library](library.html): External identifier for the library 5993* [Measure](measure.html): External identifier for the measure 5994* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5995* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5996* [NamingSystem](namingsystem.html): External identifier for the naming system 5997* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 5998* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 5999* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6000* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6001* [Requirements](requirements.html): External identifier for the requirements 6002* [SearchParameter](searchparameter.html): External identifier for the search parameter 6003* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6004* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6005* [StructureMap](structuremap.html): External identifier for the structure map 6006* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6007* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6008* [TestPlan](testplan.html): An identifier for the test plan 6009* [TestScript](testscript.html): External identifier for the test script 6010* [ValueSet](valueset.html): External identifier for the value set 6011</b><br> 6012 * Type: <b>token</b><br> 6013 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6014 * </p> 6015 */ 6016 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 6017 public static final String SP_IDENTIFIER = "identifier"; 6018 /** 6019 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6020 * <p> 6021 * Description: <b>Multiple Resources: 6022 6023* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6024* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6025* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6026* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6027* [Citation](citation.html): External identifier for the citation 6028* [CodeSystem](codesystem.html): External identifier for the code system 6029* [ConceptMap](conceptmap.html): External identifier for the concept map 6030* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6031* [EventDefinition](eventdefinition.html): External identifier for the event definition 6032* [Evidence](evidence.html): External identifier for the evidence 6033* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6034* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6035* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6036* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6037* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6038* [Library](library.html): External identifier for the library 6039* [Measure](measure.html): External identifier for the measure 6040* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6041* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6042* [NamingSystem](namingsystem.html): External identifier for the naming system 6043* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6044* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6045* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6046* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6047* [Requirements](requirements.html): External identifier for the requirements 6048* [SearchParameter](searchparameter.html): External identifier for the search parameter 6049* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6050* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6051* [StructureMap](structuremap.html): External identifier for the structure map 6052* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6053* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6054* [TestPlan](testplan.html): An identifier for the test plan 6055* [TestScript](testscript.html): External identifier for the test script 6056* [ValueSet](valueset.html): External identifier for the value set 6057</b><br> 6058 * Type: <b>token</b><br> 6059 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6060 * </p> 6061 */ 6062 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6063 6064 /** 6065 * Search parameter: <b>name</b> 6066 * <p> 6067 * Description: <b>Multiple Resources: 6068 6069* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6070* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6071* [Citation](citation.html): Computationally friendly name of the citation 6072* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6073* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6074* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6075* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6076* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6077* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6078* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6079* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6080* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6081* [Library](library.html): Computationally friendly name of the library 6082* [Measure](measure.html): Computationally friendly name of the measure 6083* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6084* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6085* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6086* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6087* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6088* [Requirements](requirements.html): Computationally friendly name of the requirements 6089* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6090* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6091* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6092* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6093* [TestScript](testscript.html): Computationally friendly name of the test script 6094* [ValueSet](valueset.html): Computationally friendly name of the value set 6095</b><br> 6096 * Type: <b>string</b><br> 6097 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6098 * </p> 6099 */ 6100 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 6101 public static final String SP_NAME = "name"; 6102 /** 6103 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6104 * <p> 6105 * Description: <b>Multiple Resources: 6106 6107* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6108* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6109* [Citation](citation.html): Computationally friendly name of the citation 6110* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6111* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6112* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6113* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6114* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6115* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6116* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6117* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6118* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6119* [Library](library.html): Computationally friendly name of the library 6120* [Measure](measure.html): Computationally friendly name of the measure 6121* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6122* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6123* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6124* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6125* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6126* [Requirements](requirements.html): Computationally friendly name of the requirements 6127* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6128* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6129* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6130* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6131* [TestScript](testscript.html): Computationally friendly name of the test script 6132* [ValueSet](valueset.html): Computationally friendly name of the value set 6133</b><br> 6134 * Type: <b>string</b><br> 6135 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6136 * </p> 6137 */ 6138 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 6139 6140 /** 6141 * Search parameter: <b>publisher</b> 6142 * <p> 6143 * Description: <b>Multiple Resources: 6144 6145* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6146* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6147* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6148* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6149* [Citation](citation.html): Name of the publisher of the citation 6150* [CodeSystem](codesystem.html): Name of the publisher of the code system 6151* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6152* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6153* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6154* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6155* [Evidence](evidence.html): Name of the publisher of the evidence 6156* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6157* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6158* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6159* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6160* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6161* [Library](library.html): Name of the publisher of the library 6162* [Measure](measure.html): Name of the publisher of the measure 6163* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6164* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6165* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6166* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6167* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6168* [Requirements](requirements.html): Name of the publisher of the requirements 6169* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6170* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6171* [StructureMap](structuremap.html): Name of the publisher of the structure map 6172* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6173* [TestScript](testscript.html): Name of the publisher of the test script 6174* [ValueSet](valueset.html): Name of the publisher of the value set 6175</b><br> 6176 * Type: <b>string</b><br> 6177 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6178 * </p> 6179 */ 6180 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 6181 public static final String SP_PUBLISHER = "publisher"; 6182 /** 6183 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6184 * <p> 6185 * Description: <b>Multiple Resources: 6186 6187* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6188* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6189* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6190* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6191* [Citation](citation.html): Name of the publisher of the citation 6192* [CodeSystem](codesystem.html): Name of the publisher of the code system 6193* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6194* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6195* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6196* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6197* [Evidence](evidence.html): Name of the publisher of the evidence 6198* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6199* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6200* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6201* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6202* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6203* [Library](library.html): Name of the publisher of the library 6204* [Measure](measure.html): Name of the publisher of the measure 6205* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6206* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6207* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6208* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6209* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6210* [Requirements](requirements.html): Name of the publisher of the requirements 6211* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6212* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6213* [StructureMap](structuremap.html): Name of the publisher of the structure map 6214* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6215* [TestScript](testscript.html): Name of the publisher of the test script 6216* [ValueSet](valueset.html): Name of the publisher of the value set 6217</b><br> 6218 * Type: <b>string</b><br> 6219 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6220 * </p> 6221 */ 6222 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 6223 6224 /** 6225 * Search parameter: <b>status</b> 6226 * <p> 6227 * Description: <b>Multiple Resources: 6228 6229* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6230* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6231* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6232* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6233* [Citation](citation.html): The current status of the citation 6234* [CodeSystem](codesystem.html): The current status of the code system 6235* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6236* [ConceptMap](conceptmap.html): The current status of the concept map 6237* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6238* [EventDefinition](eventdefinition.html): The current status of the event definition 6239* [Evidence](evidence.html): The current status of the evidence 6240* [EvidenceReport](evidencereport.html): The current status of the evidence report 6241* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6242* [ExampleScenario](examplescenario.html): The current status of the example scenario 6243* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6244* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6245* [Library](library.html): The current status of the library 6246* [Measure](measure.html): The current status of the measure 6247* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6248* [MessageDefinition](messagedefinition.html): The current status of the message definition 6249* [NamingSystem](namingsystem.html): The current status of the naming system 6250* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6251* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6252* [PlanDefinition](plandefinition.html): The current status of the plan definition 6253* [Questionnaire](questionnaire.html): The current status of the questionnaire 6254* [Requirements](requirements.html): The current status of the requirements 6255* [SearchParameter](searchparameter.html): The current status of the search parameter 6256* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6257* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6258* [StructureMap](structuremap.html): The current status of the structure map 6259* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6260* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6261* [TestPlan](testplan.html): The current status of the test plan 6262* [TestScript](testscript.html): The current status of the test script 6263* [ValueSet](valueset.html): The current status of the value set 6264</b><br> 6265 * Type: <b>token</b><br> 6266 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6267 * </p> 6268 */ 6269 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 6270 public static final String SP_STATUS = "status"; 6271 /** 6272 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6273 * <p> 6274 * Description: <b>Multiple Resources: 6275 6276* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6277* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6278* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6279* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6280* [Citation](citation.html): The current status of the citation 6281* [CodeSystem](codesystem.html): The current status of the code system 6282* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6283* [ConceptMap](conceptmap.html): The current status of the concept map 6284* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6285* [EventDefinition](eventdefinition.html): The current status of the event definition 6286* [Evidence](evidence.html): The current status of the evidence 6287* [EvidenceReport](evidencereport.html): The current status of the evidence report 6288* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6289* [ExampleScenario](examplescenario.html): The current status of the example scenario 6290* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6291* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6292* [Library](library.html): The current status of the library 6293* [Measure](measure.html): The current status of the measure 6294* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6295* [MessageDefinition](messagedefinition.html): The current status of the message definition 6296* [NamingSystem](namingsystem.html): The current status of the naming system 6297* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6298* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6299* [PlanDefinition](plandefinition.html): The current status of the plan definition 6300* [Questionnaire](questionnaire.html): The current status of the questionnaire 6301* [Requirements](requirements.html): The current status of the requirements 6302* [SearchParameter](searchparameter.html): The current status of the search parameter 6303* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6304* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6305* [StructureMap](structuremap.html): The current status of the structure map 6306* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6307* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6308* [TestPlan](testplan.html): The current status of the test plan 6309* [TestScript](testscript.html): The current status of the test script 6310* [ValueSet](valueset.html): The current status of the value set 6311</b><br> 6312 * Type: <b>token</b><br> 6313 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6314 * </p> 6315 */ 6316 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6317 6318 /** 6319 * Search parameter: <b>title</b> 6320 * <p> 6321 * Description: <b>Multiple Resources: 6322 6323* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6324* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6325* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6326* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6327* [Citation](citation.html): The human-friendly name of the citation 6328* [CodeSystem](codesystem.html): The human-friendly name of the code system 6329* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6330* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6331* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6332* [Evidence](evidence.html): The human-friendly name of the evidence 6333* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6334* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6335* [Library](library.html): The human-friendly name of the library 6336* [Measure](measure.html): The human-friendly name of the measure 6337* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6338* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6339* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6340* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6341* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6342* [Requirements](requirements.html): The human-friendly name of the requirements 6343* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6344* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6345* [StructureMap](structuremap.html): The human-friendly name of the structure map 6346* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6347* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6348* [TestScript](testscript.html): The human-friendly name of the test script 6349* [ValueSet](valueset.html): The human-friendly name of the value set 6350</b><br> 6351 * Type: <b>string</b><br> 6352 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6353 * </p> 6354 */ 6355 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 6356 public static final String SP_TITLE = "title"; 6357 /** 6358 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6359 * <p> 6360 * Description: <b>Multiple Resources: 6361 6362* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6363* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6364* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6365* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6366* [Citation](citation.html): The human-friendly name of the citation 6367* [CodeSystem](codesystem.html): The human-friendly name of the code system 6368* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6369* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6370* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6371* [Evidence](evidence.html): The human-friendly name of the evidence 6372* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6373* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6374* [Library](library.html): The human-friendly name of the library 6375* [Measure](measure.html): The human-friendly name of the measure 6376* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6377* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6378* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6379* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6380* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6381* [Requirements](requirements.html): The human-friendly name of the requirements 6382* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6383* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6384* [StructureMap](structuremap.html): The human-friendly name of the structure map 6385* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6386* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6387* [TestScript](testscript.html): The human-friendly name of the test script 6388* [ValueSet](valueset.html): The human-friendly name of the value set 6389</b><br> 6390 * Type: <b>string</b><br> 6391 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6392 * </p> 6393 */ 6394 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 6395 6396 /** 6397 * Search parameter: <b>url</b> 6398 * <p> 6399 * Description: <b>Multiple Resources: 6400 6401* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6402* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6403* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6404* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6405* [Citation](citation.html): The uri that identifies the citation 6406* [CodeSystem](codesystem.html): The uri that identifies the code system 6407* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6408* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6409* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6410* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6411* [Evidence](evidence.html): The uri that identifies the evidence 6412* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6413* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6414* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6415* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6416* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6417* [Library](library.html): The uri that identifies the library 6418* [Measure](measure.html): The uri that identifies the measure 6419* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6420* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6421* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6422* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6423* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6424* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6425* [Requirements](requirements.html): The uri that identifies the requirements 6426* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6427* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6428* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6429* [StructureMap](structuremap.html): The uri that identifies the structure map 6430* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6431* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6432* [TestPlan](testplan.html): The uri that identifies the test plan 6433* [TestScript](testscript.html): The uri that identifies the test script 6434* [ValueSet](valueset.html): The uri that identifies the value set 6435</b><br> 6436 * Type: <b>uri</b><br> 6437 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6438 * </p> 6439 */ 6440 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 6441 public static final String SP_URL = "url"; 6442 /** 6443 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6444 * <p> 6445 * Description: <b>Multiple Resources: 6446 6447* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6448* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6449* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6450* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6451* [Citation](citation.html): The uri that identifies the citation 6452* [CodeSystem](codesystem.html): The uri that identifies the code system 6453* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6454* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6455* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6456* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6457* [Evidence](evidence.html): The uri that identifies the evidence 6458* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6459* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6460* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6461* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6462* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6463* [Library](library.html): The uri that identifies the library 6464* [Measure](measure.html): The uri that identifies the measure 6465* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6466* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6467* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6468* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6469* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6470* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6471* [Requirements](requirements.html): The uri that identifies the requirements 6472* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6473* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6474* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6475* [StructureMap](structuremap.html): The uri that identifies the structure map 6476* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6477* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6478* [TestPlan](testplan.html): The uri that identifies the test plan 6479* [TestScript](testscript.html): The uri that identifies the test script 6480* [ValueSet](valueset.html): The uri that identifies the value set 6481</b><br> 6482 * Type: <b>uri</b><br> 6483 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6484 * </p> 6485 */ 6486 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6487 6488 /** 6489 * Search parameter: <b>version</b> 6490 * <p> 6491 * Description: <b>Multiple Resources: 6492 6493* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6494* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6495* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6496* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6497* [Citation](citation.html): The business version of the citation 6498* [CodeSystem](codesystem.html): The business version of the code system 6499* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6500* [ConceptMap](conceptmap.html): The business version of the concept map 6501* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6502* [EventDefinition](eventdefinition.html): The business version of the event definition 6503* [Evidence](evidence.html): The business version of the evidence 6504* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6505* [ExampleScenario](examplescenario.html): The business version of the example scenario 6506* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6507* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6508* [Library](library.html): The business version of the library 6509* [Measure](measure.html): The business version of the measure 6510* [MessageDefinition](messagedefinition.html): The business version of the message definition 6511* [NamingSystem](namingsystem.html): The business version of the naming system 6512* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6513* [PlanDefinition](plandefinition.html): The business version of the plan definition 6514* [Questionnaire](questionnaire.html): The business version of the questionnaire 6515* [Requirements](requirements.html): The business version of the requirements 6516* [SearchParameter](searchparameter.html): The business version of the search parameter 6517* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6518* [StructureMap](structuremap.html): The business version of the structure map 6519* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6520* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6521* [TestScript](testscript.html): The business version of the test script 6522* [ValueSet](valueset.html): The business version of the value set 6523</b><br> 6524 * Type: <b>token</b><br> 6525 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6526 * </p> 6527 */ 6528 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 6529 public static final String SP_VERSION = "version"; 6530 /** 6531 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6532 * <p> 6533 * Description: <b>Multiple Resources: 6534 6535* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6536* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6537* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6538* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6539* [Citation](citation.html): The business version of the citation 6540* [CodeSystem](codesystem.html): The business version of the code system 6541* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6542* [ConceptMap](conceptmap.html): The business version of the concept map 6543* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6544* [EventDefinition](eventdefinition.html): The business version of the event definition 6545* [Evidence](evidence.html): The business version of the evidence 6546* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6547* [ExampleScenario](examplescenario.html): The business version of the example scenario 6548* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6549* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6550* [Library](library.html): The business version of the library 6551* [Measure](measure.html): The business version of the measure 6552* [MessageDefinition](messagedefinition.html): The business version of the message definition 6553* [NamingSystem](namingsystem.html): The business version of the naming system 6554* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6555* [PlanDefinition](plandefinition.html): The business version of the plan definition 6556* [Questionnaire](questionnaire.html): The business version of the questionnaire 6557* [Requirements](requirements.html): The business version of the requirements 6558* [SearchParameter](searchparameter.html): The business version of the search parameter 6559* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6560* [StructureMap](structuremap.html): The business version of the structure map 6561* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6562* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6563* [TestScript](testscript.html): The business version of the test script 6564* [ValueSet](valueset.html): The business version of the value set 6565</b><br> 6566 * Type: <b>token</b><br> 6567 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6568 * </p> 6569 */ 6570 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 6571 6572 /** 6573 * Search parameter: <b>composed-of</b> 6574 * <p> 6575 * Description: <b>Multiple Resources: 6576 6577* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6578* [EventDefinition](eventdefinition.html): What resource is being referenced 6579* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6580* [Library](library.html): What resource is being referenced 6581* [Measure](measure.html): What resource is being referenced 6582* [PlanDefinition](plandefinition.html): What resource is being referenced 6583</b><br> 6584 * Type: <b>reference</b><br> 6585 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 6586 * </p> 6587 */ 6588 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6589 public static final String SP_COMPOSED_OF = "composed-of"; 6590 /** 6591 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 6592 * <p> 6593 * Description: <b>Multiple Resources: 6594 6595* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6596* [EventDefinition](eventdefinition.html): What resource is being referenced 6597* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6598* [Library](library.html): What resource is being referenced 6599* [Measure](measure.html): What resource is being referenced 6600* [PlanDefinition](plandefinition.html): What resource is being referenced 6601</b><br> 6602 * Type: <b>reference</b><br> 6603 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 6604 * </p> 6605 */ 6606 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 6607 6608/** 6609 * Constant for fluent queries to be used to add include statements. Specifies 6610 * the path value of "<b>EvidenceVariable:composed-of</b>". 6611 */ 6612 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("EvidenceVariable:composed-of").toLocked(); 6613 6614 /** 6615 * Search parameter: <b>depends-on</b> 6616 * <p> 6617 * Description: <b>Multiple Resources: 6618 6619* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6620* [EventDefinition](eventdefinition.html): What resource is being referenced 6621* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6622* [Library](library.html): What resource is being referenced 6623* [Measure](measure.html): What resource is being referenced 6624* [PlanDefinition](plandefinition.html): What resource is being referenced 6625</b><br> 6626 * Type: <b>reference</b><br> 6627 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 6628 * </p> 6629 */ 6630 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6631 public static final String SP_DEPENDS_ON = "depends-on"; 6632 /** 6633 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 6634 * <p> 6635 * Description: <b>Multiple Resources: 6636 6637* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6638* [EventDefinition](eventdefinition.html): What resource is being referenced 6639* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6640* [Library](library.html): What resource is being referenced 6641* [Measure](measure.html): What resource is being referenced 6642* [PlanDefinition](plandefinition.html): What resource is being referenced 6643</b><br> 6644 * Type: <b>reference</b><br> 6645 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 6646 * </p> 6647 */ 6648 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 6649 6650/** 6651 * Constant for fluent queries to be used to add include statements. Specifies 6652 * the path value of "<b>EvidenceVariable:depends-on</b>". 6653 */ 6654 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("EvidenceVariable:depends-on").toLocked(); 6655 6656 /** 6657 * Search parameter: <b>derived-from</b> 6658 * <p> 6659 * Description: <b>Multiple Resources: 6660 6661* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6662* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6663* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6664* [EventDefinition](eventdefinition.html): What resource is being referenced 6665* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6666* [Library](library.html): What resource is being referenced 6667* [Measure](measure.html): What resource is being referenced 6668* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6669* [PlanDefinition](plandefinition.html): What resource is being referenced 6670* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6671</b><br> 6672 * Type: <b>reference</b><br> 6673 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6674 * </p> 6675 */ 6676 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6677 public static final String SP_DERIVED_FROM = "derived-from"; 6678 /** 6679 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 6680 * <p> 6681 * Description: <b>Multiple Resources: 6682 6683* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6684* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6685* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6686* [EventDefinition](eventdefinition.html): What resource is being referenced 6687* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6688* [Library](library.html): What resource is being referenced 6689* [Measure](measure.html): What resource is being referenced 6690* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6691* [PlanDefinition](plandefinition.html): What resource is being referenced 6692* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6693</b><br> 6694 * Type: <b>reference</b><br> 6695 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6696 * </p> 6697 */ 6698 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 6699 6700/** 6701 * Constant for fluent queries to be used to add include statements. Specifies 6702 * the path value of "<b>EvidenceVariable:derived-from</b>". 6703 */ 6704 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("EvidenceVariable:derived-from").toLocked(); 6705 6706 /** 6707 * Search parameter: <b>predecessor</b> 6708 * <p> 6709 * Description: <b>Multiple Resources: 6710 6711* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6712* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6713* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6714* [EventDefinition](eventdefinition.html): What resource is being referenced 6715* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6716* [Library](library.html): What resource is being referenced 6717* [Measure](measure.html): What resource is being referenced 6718* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6719* [PlanDefinition](plandefinition.html): What resource is being referenced 6720* [ValueSet](valueset.html): The predecessor of the ValueSet 6721</b><br> 6722 * Type: <b>reference</b><br> 6723 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6724 * </p> 6725 */ 6726 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6727 public static final String SP_PREDECESSOR = "predecessor"; 6728 /** 6729 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 6730 * <p> 6731 * Description: <b>Multiple Resources: 6732 6733* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6734* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6735* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6736* [EventDefinition](eventdefinition.html): What resource is being referenced 6737* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6738* [Library](library.html): What resource is being referenced 6739* [Measure](measure.html): What resource is being referenced 6740* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6741* [PlanDefinition](plandefinition.html): What resource is being referenced 6742* [ValueSet](valueset.html): The predecessor of the ValueSet 6743</b><br> 6744 * Type: <b>reference</b><br> 6745 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6746 * </p> 6747 */ 6748 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 6749 6750/** 6751 * Constant for fluent queries to be used to add include statements. Specifies 6752 * the path value of "<b>EvidenceVariable:predecessor</b>". 6753 */ 6754 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("EvidenceVariable:predecessor").toLocked(); 6755 6756 /** 6757 * Search parameter: <b>successor</b> 6758 * <p> 6759 * Description: <b>Multiple Resources: 6760 6761* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6762* [EventDefinition](eventdefinition.html): What resource is being referenced 6763* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6764* [Library](library.html): What resource is being referenced 6765* [Measure](measure.html): What resource is being referenced 6766* [PlanDefinition](plandefinition.html): What resource is being referenced 6767</b><br> 6768 * Type: <b>reference</b><br> 6769 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 6770 * </p> 6771 */ 6772 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6773 public static final String SP_SUCCESSOR = "successor"; 6774 /** 6775 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 6776 * <p> 6777 * Description: <b>Multiple Resources: 6778 6779* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6780* [EventDefinition](eventdefinition.html): What resource is being referenced 6781* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6782* [Library](library.html): What resource is being referenced 6783* [Measure](measure.html): What resource is being referenced 6784* [PlanDefinition](plandefinition.html): What resource is being referenced 6785</b><br> 6786 * Type: <b>reference</b><br> 6787 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 6788 * </p> 6789 */ 6790 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 6791 6792/** 6793 * Constant for fluent queries to be used to add include statements. Specifies 6794 * the path value of "<b>EvidenceVariable:successor</b>". 6795 */ 6796 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("EvidenceVariable:successor").toLocked(); 6797 6798 /** 6799 * Search parameter: <b>topic</b> 6800 * <p> 6801 * Description: <b>Multiple Resources: 6802 6803* [ActivityDefinition](activitydefinition.html): Topics associated with the module 6804* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 6805* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 6806* [EventDefinition](eventdefinition.html): Topics associated with the module 6807* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 6808* [Library](library.html): Topics associated with the module 6809* [Measure](measure.html): Topics associated with the measure 6810* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 6811* [PlanDefinition](plandefinition.html): Topics associated with the module 6812* [ValueSet](valueset.html): Topics associated with the ValueSet 6813</b><br> 6814 * Type: <b>token</b><br> 6815 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 6816 * </p> 6817 */ 6818 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 6819 public static final String SP_TOPIC = "topic"; 6820 /** 6821 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 6822 * <p> 6823 * Description: <b>Multiple Resources: 6824 6825* [ActivityDefinition](activitydefinition.html): Topics associated with the module 6826* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 6827* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 6828* [EventDefinition](eventdefinition.html): Topics associated with the module 6829* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 6830* [Library](library.html): Topics associated with the module 6831* [Measure](measure.html): Topics associated with the measure 6832* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 6833* [PlanDefinition](plandefinition.html): Topics associated with the module 6834* [ValueSet](valueset.html): Topics associated with the ValueSet 6835</b><br> 6836 * Type: <b>token</b><br> 6837 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 6838 * </p> 6839 */ 6840 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 6841 6842 6843} 6844