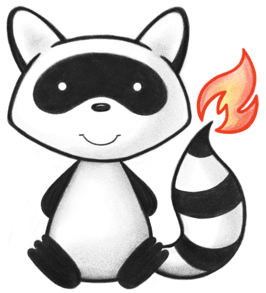
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The EvidenceVariable resource describes an element that knowledge (Evidence) is about. 052 */ 053@ResourceDef(name="EvidenceVariable", profile="http://hl7.org/fhir/StructureDefinition/EvidenceVariable") 054public class EvidenceVariable extends MetadataResource { 055 056 public enum CharacteristicCombination { 057 /** 058 * Combine characteristics with AND. 059 */ 060 ALLOF, 061 /** 062 * Combine characteristics with OR. 063 */ 064 ANYOF, 065 /** 066 * Meet at least the threshold number of characteristics for definition. 067 */ 068 ATLEAST, 069 /** 070 * Meet at most the threshold number of characteristics for definition. 071 */ 072 ATMOST, 073 /** 074 * Combine characteristics statistically. Use method to specify the statistical method. 075 */ 076 STATISTICAL, 077 /** 078 * Combine characteristics by addition of benefits and subtraction of harms. 079 */ 080 NETEFFECT, 081 /** 082 * Combine characteristics as a collection used as the dataset. 083 */ 084 DATASET, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static CharacteristicCombination fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("all-of".equals(codeString)) 093 return ALLOF; 094 if ("any-of".equals(codeString)) 095 return ANYOF; 096 if ("at-least".equals(codeString)) 097 return ATLEAST; 098 if ("at-most".equals(codeString)) 099 return ATMOST; 100 if ("statistical".equals(codeString)) 101 return STATISTICAL; 102 if ("net-effect".equals(codeString)) 103 return NETEFFECT; 104 if ("dataset".equals(codeString)) 105 return DATASET; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown CharacteristicCombination code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case ALLOF: return "all-of"; 114 case ANYOF: return "any-of"; 115 case ATLEAST: return "at-least"; 116 case ATMOST: return "at-most"; 117 case STATISTICAL: return "statistical"; 118 case NETEFFECT: return "net-effect"; 119 case DATASET: return "dataset"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case ALLOF: return "http://hl7.org/fhir/characteristic-combination"; 127 case ANYOF: return "http://hl7.org/fhir/characteristic-combination"; 128 case ATLEAST: return "http://hl7.org/fhir/characteristic-combination"; 129 case ATMOST: return "http://hl7.org/fhir/characteristic-combination"; 130 case STATISTICAL: return "http://hl7.org/fhir/characteristic-combination"; 131 case NETEFFECT: return "http://hl7.org/fhir/characteristic-combination"; 132 case DATASET: return "http://hl7.org/fhir/characteristic-combination"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case ALLOF: return "Combine characteristics with AND."; 140 case ANYOF: return "Combine characteristics with OR."; 141 case ATLEAST: return "Meet at least the threshold number of characteristics for definition."; 142 case ATMOST: return "Meet at most the threshold number of characteristics for definition."; 143 case STATISTICAL: return "Combine characteristics statistically. Use method to specify the statistical method."; 144 case NETEFFECT: return "Combine characteristics by addition of benefits and subtraction of harms."; 145 case DATASET: return "Combine characteristics as a collection used as the dataset."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case ALLOF: return "All of"; 153 case ANYOF: return "Any of"; 154 case ATLEAST: return "At least"; 155 case ATMOST: return "At most"; 156 case STATISTICAL: return "Statistical"; 157 case NETEFFECT: return "Net effect"; 158 case DATASET: return "Dataset"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class CharacteristicCombinationEnumFactory implements EnumFactory<CharacteristicCombination> { 166 public CharacteristicCombination fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("all-of".equals(codeString)) 171 return CharacteristicCombination.ALLOF; 172 if ("any-of".equals(codeString)) 173 return CharacteristicCombination.ANYOF; 174 if ("at-least".equals(codeString)) 175 return CharacteristicCombination.ATLEAST; 176 if ("at-most".equals(codeString)) 177 return CharacteristicCombination.ATMOST; 178 if ("statistical".equals(codeString)) 179 return CharacteristicCombination.STATISTICAL; 180 if ("net-effect".equals(codeString)) 181 return CharacteristicCombination.NETEFFECT; 182 if ("dataset".equals(codeString)) 183 return CharacteristicCombination.DATASET; 184 throw new IllegalArgumentException("Unknown CharacteristicCombination code '"+codeString+"'"); 185 } 186 public Enumeration<CharacteristicCombination> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.NULL, code); 194 if ("all-of".equals(codeString)) 195 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ALLOF, code); 196 if ("any-of".equals(codeString)) 197 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ANYOF, code); 198 if ("at-least".equals(codeString)) 199 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ATLEAST, code); 200 if ("at-most".equals(codeString)) 201 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.ATMOST, code); 202 if ("statistical".equals(codeString)) 203 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.STATISTICAL, code); 204 if ("net-effect".equals(codeString)) 205 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.NETEFFECT, code); 206 if ("dataset".equals(codeString)) 207 return new Enumeration<CharacteristicCombination>(this, CharacteristicCombination.DATASET, code); 208 throw new FHIRException("Unknown CharacteristicCombination code '"+codeString+"'"); 209 } 210 public String toCode(CharacteristicCombination code) { 211 if (code == CharacteristicCombination.NULL) 212 return null; 213 if (code == CharacteristicCombination.ALLOF) 214 return "all-of"; 215 if (code == CharacteristicCombination.ANYOF) 216 return "any-of"; 217 if (code == CharacteristicCombination.ATLEAST) 218 return "at-least"; 219 if (code == CharacteristicCombination.ATMOST) 220 return "at-most"; 221 if (code == CharacteristicCombination.STATISTICAL) 222 return "statistical"; 223 if (code == CharacteristicCombination.NETEFFECT) 224 return "net-effect"; 225 if (code == CharacteristicCombination.DATASET) 226 return "dataset"; 227 return "?"; 228 } 229 public String toSystem(CharacteristicCombination code) { 230 return code.getSystem(); 231 } 232 } 233 234 @Block() 235 public static class EvidenceVariableCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 236 /** 237 * Label used for when a characteristic refers to another characteristic. 238 */ 239 @Child(name = "linkId", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=false) 240 @Description(shortDefinition="Label for internal linking", formalDefinition="Label used for when a characteristic refers to another characteristic." ) 241 protected IdType linkId; 242 243 /** 244 * A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user. 245 */ 246 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 247 @Description(shortDefinition="Natural language description of the characteristic", formalDefinition="A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user." ) 248 protected MarkdownType description; 249 250 /** 251 * A human-readable string to clarify or explain concepts about the characteristic. 252 */ 253 @Child(name = "note", type = {Annotation.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 254 @Description(shortDefinition="Used for footnotes or explanatory notes", formalDefinition="A human-readable string to clarify or explain concepts about the characteristic." ) 255 protected List<Annotation> note; 256 257 /** 258 * When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion. 259 */ 260 @Child(name = "exclude", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 261 @Description(shortDefinition="Whether the characteristic is an inclusion criterion or exclusion criterion", formalDefinition="When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion." ) 262 protected BooleanType exclude; 263 264 /** 265 * Defines the characteristic using a Reference. 266 */ 267 @Child(name = "definitionReference", type = {EvidenceVariable.class, Group.class, Evidence.class}, order=5, min=0, max=1, modifier=false, summary=true) 268 @Description(shortDefinition="Defines the characteristic (without using type and value) by a Reference", formalDefinition="Defines the characteristic using a Reference." ) 269 protected Reference definitionReference; 270 271 /** 272 * Defines the characteristic using Canonical. 273 */ 274 @Child(name = "definitionCanonical", type = {CanonicalType.class}, order=6, min=0, max=1, modifier=false, summary=true) 275 @Description(shortDefinition="Defines the characteristic (without using type and value) by a Canonical", formalDefinition="Defines the characteristic using Canonical." ) 276 protected CanonicalType definitionCanonical; 277 278 /** 279 * Defines the characteristic using CodeableConcept. 280 */ 281 @Child(name = "definitionCodeableConcept", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 282 @Description(shortDefinition="Defines the characteristic (without using type and value) by a CodeableConcept", formalDefinition="Defines the characteristic using CodeableConcept." ) 283 protected CodeableConcept definitionCodeableConcept; 284 285 /** 286 * Defines the characteristic using Expression. 287 */ 288 @Child(name = "definitionExpression", type = {Expression.class}, order=8, min=0, max=1, modifier=false, summary=true) 289 @Description(shortDefinition="Defines the characteristic (without using type and value) by an expression", formalDefinition="Defines the characteristic using Expression." ) 290 protected Expression definitionExpression; 291 292 /** 293 * Defines the characteristic using id. 294 */ 295 @Child(name = "definitionId", type = {IdType.class}, order=9, min=0, max=1, modifier=false, summary=true) 296 @Description(shortDefinition="Defines the characteristic (without using type and value) by an id", formalDefinition="Defines the characteristic using id." ) 297 protected IdType definitionId; 298 299 /** 300 * Defines the characteristic using both a type and value[x] elements. 301 */ 302 @Child(name = "definitionByTypeAndValue", type = {}, order=10, min=0, max=1, modifier=false, summary=true) 303 @Description(shortDefinition="Defines the characteristic using type and value", formalDefinition="Defines the characteristic using both a type and value[x] elements." ) 304 protected EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent definitionByTypeAndValue; 305 306 /** 307 * Defines the characteristic as a combination of two or more characteristics. 308 */ 309 @Child(name = "definitionByCombination", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 310 @Description(shortDefinition="Used to specify how two or more characteristics are combined", formalDefinition="Defines the characteristic as a combination of two or more characteristics." ) 311 protected EvidenceVariableCharacteristicDefinitionByCombinationComponent definitionByCombination; 312 313 /** 314 * Number of occurrences meeting the characteristic. 315 */ 316 @Child(name = "instances", type = {Quantity.class, Range.class}, order=12, min=0, max=1, modifier=false, summary=false) 317 @Description(shortDefinition="Number of occurrences meeting the characteristic", formalDefinition="Number of occurrences meeting the characteristic." ) 318 protected DataType instances; 319 320 /** 321 * Length of time in which the characteristic is met. 322 */ 323 @Child(name = "duration", type = {Quantity.class, Range.class}, order=13, min=0, max=1, modifier=false, summary=false) 324 @Description(shortDefinition="Length of time in which the characteristic is met", formalDefinition="Length of time in which the characteristic is met." ) 325 protected DataType duration; 326 327 /** 328 * Timing in which the characteristic is determined. 329 */ 330 @Child(name = "timeFromEvent", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 331 @Description(shortDefinition="Timing in which the characteristic is determined", formalDefinition="Timing in which the characteristic is determined." ) 332 protected List<EvidenceVariableCharacteristicTimeFromEventComponent> timeFromEvent; 333 334 private static final long serialVersionUID = 1658283810L; 335 336 /** 337 * Constructor 338 */ 339 public EvidenceVariableCharacteristicComponent() { 340 super(); 341 } 342 343 /** 344 * @return {@link #linkId} (Label used for when a characteristic refers to another characteristic.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 345 */ 346 public IdType getLinkIdElement() { 347 if (this.linkId == null) 348 if (Configuration.errorOnAutoCreate()) 349 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.linkId"); 350 else if (Configuration.doAutoCreate()) 351 this.linkId = new IdType(); // bb 352 return this.linkId; 353 } 354 355 public boolean hasLinkIdElement() { 356 return this.linkId != null && !this.linkId.isEmpty(); 357 } 358 359 public boolean hasLinkId() { 360 return this.linkId != null && !this.linkId.isEmpty(); 361 } 362 363 /** 364 * @param value {@link #linkId} (Label used for when a characteristic refers to another characteristic.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 365 */ 366 public EvidenceVariableCharacteristicComponent setLinkIdElement(IdType value) { 367 this.linkId = value; 368 return this; 369 } 370 371 /** 372 * @return Label used for when a characteristic refers to another characteristic. 373 */ 374 public String getLinkId() { 375 return this.linkId == null ? null : this.linkId.getValue(); 376 } 377 378 /** 379 * @param value Label used for when a characteristic refers to another characteristic. 380 */ 381 public EvidenceVariableCharacteristicComponent setLinkId(String value) { 382 if (Utilities.noString(value)) 383 this.linkId = null; 384 else { 385 if (this.linkId == null) 386 this.linkId = new IdType(); 387 this.linkId.setValue(value); 388 } 389 return this; 390 } 391 392 /** 393 * @return {@link #description} (A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 394 */ 395 public MarkdownType getDescriptionElement() { 396 if (this.description == null) 397 if (Configuration.errorOnAutoCreate()) 398 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.description"); 399 else if (Configuration.doAutoCreate()) 400 this.description = new MarkdownType(); // bb 401 return this.description; 402 } 403 404 public boolean hasDescriptionElement() { 405 return this.description != null && !this.description.isEmpty(); 406 } 407 408 public boolean hasDescription() { 409 return this.description != null && !this.description.isEmpty(); 410 } 411 412 /** 413 * @param value {@link #description} (A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 414 */ 415 public EvidenceVariableCharacteristicComponent setDescriptionElement(MarkdownType value) { 416 this.description = value; 417 return this; 418 } 419 420 /** 421 * @return A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user. 422 */ 423 public String getDescription() { 424 return this.description == null ? null : this.description.getValue(); 425 } 426 427 /** 428 * @param value A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user. 429 */ 430 public EvidenceVariableCharacteristicComponent setDescription(String value) { 431 if (Utilities.noString(value)) 432 this.description = null; 433 else { 434 if (this.description == null) 435 this.description = new MarkdownType(); 436 this.description.setValue(value); 437 } 438 return this; 439 } 440 441 /** 442 * @return {@link #note} (A human-readable string to clarify or explain concepts about the characteristic.) 443 */ 444 public List<Annotation> getNote() { 445 if (this.note == null) 446 this.note = new ArrayList<Annotation>(); 447 return this.note; 448 } 449 450 /** 451 * @return Returns a reference to <code>this</code> for easy method chaining 452 */ 453 public EvidenceVariableCharacteristicComponent setNote(List<Annotation> theNote) { 454 this.note = theNote; 455 return this; 456 } 457 458 public boolean hasNote() { 459 if (this.note == null) 460 return false; 461 for (Annotation item : this.note) 462 if (!item.isEmpty()) 463 return true; 464 return false; 465 } 466 467 public Annotation addNote() { //3 468 Annotation t = new Annotation(); 469 if (this.note == null) 470 this.note = new ArrayList<Annotation>(); 471 this.note.add(t); 472 return t; 473 } 474 475 public EvidenceVariableCharacteristicComponent addNote(Annotation t) { //3 476 if (t == null) 477 return this; 478 if (this.note == null) 479 this.note = new ArrayList<Annotation>(); 480 this.note.add(t); 481 return this; 482 } 483 484 /** 485 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 486 */ 487 public Annotation getNoteFirstRep() { 488 if (getNote().isEmpty()) { 489 addNote(); 490 } 491 return getNote().get(0); 492 } 493 494 /** 495 * @return {@link #exclude} (When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 496 */ 497 public BooleanType getExcludeElement() { 498 if (this.exclude == null) 499 if (Configuration.errorOnAutoCreate()) 500 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.exclude"); 501 else if (Configuration.doAutoCreate()) 502 this.exclude = new BooleanType(); // bb 503 return this.exclude; 504 } 505 506 public boolean hasExcludeElement() { 507 return this.exclude != null && !this.exclude.isEmpty(); 508 } 509 510 public boolean hasExclude() { 511 return this.exclude != null && !this.exclude.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #exclude} (When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 516 */ 517 public EvidenceVariableCharacteristicComponent setExcludeElement(BooleanType value) { 518 this.exclude = value; 519 return this; 520 } 521 522 /** 523 * @return When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion. 524 */ 525 public boolean getExclude() { 526 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 527 } 528 529 /** 530 * @param value When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion. 531 */ 532 public EvidenceVariableCharacteristicComponent setExclude(boolean value) { 533 if (this.exclude == null) 534 this.exclude = new BooleanType(); 535 this.exclude.setValue(value); 536 return this; 537 } 538 539 /** 540 * @return {@link #definitionReference} (Defines the characteristic using a Reference.) 541 */ 542 public Reference getDefinitionReference() { 543 if (this.definitionReference == null) 544 if (Configuration.errorOnAutoCreate()) 545 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionReference"); 546 else if (Configuration.doAutoCreate()) 547 this.definitionReference = new Reference(); // cc 548 return this.definitionReference; 549 } 550 551 public boolean hasDefinitionReference() { 552 return this.definitionReference != null && !this.definitionReference.isEmpty(); 553 } 554 555 /** 556 * @param value {@link #definitionReference} (Defines the characteristic using a Reference.) 557 */ 558 public EvidenceVariableCharacteristicComponent setDefinitionReference(Reference value) { 559 this.definitionReference = value; 560 return this; 561 } 562 563 /** 564 * @return {@link #definitionCanonical} (Defines the characteristic using Canonical.). This is the underlying object with id, value and extensions. The accessor "getDefinitionCanonical" gives direct access to the value 565 */ 566 public CanonicalType getDefinitionCanonicalElement() { 567 if (this.definitionCanonical == null) 568 if (Configuration.errorOnAutoCreate()) 569 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionCanonical"); 570 else if (Configuration.doAutoCreate()) 571 this.definitionCanonical = new CanonicalType(); // bb 572 return this.definitionCanonical; 573 } 574 575 public boolean hasDefinitionCanonicalElement() { 576 return this.definitionCanonical != null && !this.definitionCanonical.isEmpty(); 577 } 578 579 public boolean hasDefinitionCanonical() { 580 return this.definitionCanonical != null && !this.definitionCanonical.isEmpty(); 581 } 582 583 /** 584 * @param value {@link #definitionCanonical} (Defines the characteristic using Canonical.). This is the underlying object with id, value and extensions. The accessor "getDefinitionCanonical" gives direct access to the value 585 */ 586 public EvidenceVariableCharacteristicComponent setDefinitionCanonicalElement(CanonicalType value) { 587 this.definitionCanonical = value; 588 return this; 589 } 590 591 /** 592 * @return Defines the characteristic using Canonical. 593 */ 594 public String getDefinitionCanonical() { 595 return this.definitionCanonical == null ? null : this.definitionCanonical.getValue(); 596 } 597 598 /** 599 * @param value Defines the characteristic using Canonical. 600 */ 601 public EvidenceVariableCharacteristicComponent setDefinitionCanonical(String value) { 602 if (Utilities.noString(value)) 603 this.definitionCanonical = null; 604 else { 605 if (this.definitionCanonical == null) 606 this.definitionCanonical = new CanonicalType(); 607 this.definitionCanonical.setValue(value); 608 } 609 return this; 610 } 611 612 /** 613 * @return {@link #definitionCodeableConcept} (Defines the characteristic using CodeableConcept.) 614 */ 615 public CodeableConcept getDefinitionCodeableConcept() { 616 if (this.definitionCodeableConcept == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionCodeableConcept"); 619 else if (Configuration.doAutoCreate()) 620 this.definitionCodeableConcept = new CodeableConcept(); // cc 621 return this.definitionCodeableConcept; 622 } 623 624 public boolean hasDefinitionCodeableConcept() { 625 return this.definitionCodeableConcept != null && !this.definitionCodeableConcept.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #definitionCodeableConcept} (Defines the characteristic using CodeableConcept.) 630 */ 631 public EvidenceVariableCharacteristicComponent setDefinitionCodeableConcept(CodeableConcept value) { 632 this.definitionCodeableConcept = value; 633 return this; 634 } 635 636 /** 637 * @return {@link #definitionExpression} (Defines the characteristic using Expression.) 638 */ 639 public Expression getDefinitionExpression() { 640 if (this.definitionExpression == null) 641 if (Configuration.errorOnAutoCreate()) 642 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionExpression"); 643 else if (Configuration.doAutoCreate()) 644 this.definitionExpression = new Expression(); // cc 645 return this.definitionExpression; 646 } 647 648 public boolean hasDefinitionExpression() { 649 return this.definitionExpression != null && !this.definitionExpression.isEmpty(); 650 } 651 652 /** 653 * @param value {@link #definitionExpression} (Defines the characteristic using Expression.) 654 */ 655 public EvidenceVariableCharacteristicComponent setDefinitionExpression(Expression value) { 656 this.definitionExpression = value; 657 return this; 658 } 659 660 /** 661 * @return {@link #definitionId} (Defines the characteristic using id.). This is the underlying object with id, value and extensions. The accessor "getDefinitionId" gives direct access to the value 662 */ 663 public IdType getDefinitionIdElement() { 664 if (this.definitionId == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionId"); 667 else if (Configuration.doAutoCreate()) 668 this.definitionId = new IdType(); // bb 669 return this.definitionId; 670 } 671 672 public boolean hasDefinitionIdElement() { 673 return this.definitionId != null && !this.definitionId.isEmpty(); 674 } 675 676 public boolean hasDefinitionId() { 677 return this.definitionId != null && !this.definitionId.isEmpty(); 678 } 679 680 /** 681 * @param value {@link #definitionId} (Defines the characteristic using id.). This is the underlying object with id, value and extensions. The accessor "getDefinitionId" gives direct access to the value 682 */ 683 public EvidenceVariableCharacteristicComponent setDefinitionIdElement(IdType value) { 684 this.definitionId = value; 685 return this; 686 } 687 688 /** 689 * @return Defines the characteristic using id. 690 */ 691 public String getDefinitionId() { 692 return this.definitionId == null ? null : this.definitionId.getValue(); 693 } 694 695 /** 696 * @param value Defines the characteristic using id. 697 */ 698 public EvidenceVariableCharacteristicComponent setDefinitionId(String value) { 699 if (Utilities.noString(value)) 700 this.definitionId = null; 701 else { 702 if (this.definitionId == null) 703 this.definitionId = new IdType(); 704 this.definitionId.setValue(value); 705 } 706 return this; 707 } 708 709 /** 710 * @return {@link #definitionByTypeAndValue} (Defines the characteristic using both a type and value[x] elements.) 711 */ 712 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent getDefinitionByTypeAndValue() { 713 if (this.definitionByTypeAndValue == null) 714 if (Configuration.errorOnAutoCreate()) 715 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionByTypeAndValue"); 716 else if (Configuration.doAutoCreate()) 717 this.definitionByTypeAndValue = new EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(); // cc 718 return this.definitionByTypeAndValue; 719 } 720 721 public boolean hasDefinitionByTypeAndValue() { 722 return this.definitionByTypeAndValue != null && !this.definitionByTypeAndValue.isEmpty(); 723 } 724 725 /** 726 * @param value {@link #definitionByTypeAndValue} (Defines the characteristic using both a type and value[x] elements.) 727 */ 728 public EvidenceVariableCharacteristicComponent setDefinitionByTypeAndValue(EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent value) { 729 this.definitionByTypeAndValue = value; 730 return this; 731 } 732 733 /** 734 * @return {@link #definitionByCombination} (Defines the characteristic as a combination of two or more characteristics.) 735 */ 736 public EvidenceVariableCharacteristicDefinitionByCombinationComponent getDefinitionByCombination() { 737 if (this.definitionByCombination == null) 738 if (Configuration.errorOnAutoCreate()) 739 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.definitionByCombination"); 740 else if (Configuration.doAutoCreate()) 741 this.definitionByCombination = new EvidenceVariableCharacteristicDefinitionByCombinationComponent(); // cc 742 return this.definitionByCombination; 743 } 744 745 public boolean hasDefinitionByCombination() { 746 return this.definitionByCombination != null && !this.definitionByCombination.isEmpty(); 747 } 748 749 /** 750 * @param value {@link #definitionByCombination} (Defines the characteristic as a combination of two or more characteristics.) 751 */ 752 public EvidenceVariableCharacteristicComponent setDefinitionByCombination(EvidenceVariableCharacteristicDefinitionByCombinationComponent value) { 753 this.definitionByCombination = value; 754 return this; 755 } 756 757 /** 758 * @return {@link #instances} (Number of occurrences meeting the characteristic.) 759 */ 760 public DataType getInstances() { 761 return this.instances; 762 } 763 764 /** 765 * @return {@link #instances} (Number of occurrences meeting the characteristic.) 766 */ 767 public Quantity getInstancesQuantity() throws FHIRException { 768 if (this.instances == null) 769 this.instances = new Quantity(); 770 if (!(this.instances instanceof Quantity)) 771 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.instances.getClass().getName()+" was encountered"); 772 return (Quantity) this.instances; 773 } 774 775 public boolean hasInstancesQuantity() { 776 return this.instances instanceof Quantity; 777 } 778 779 /** 780 * @return {@link #instances} (Number of occurrences meeting the characteristic.) 781 */ 782 public Range getInstancesRange() throws FHIRException { 783 if (this.instances == null) 784 this.instances = new Range(); 785 if (!(this.instances instanceof Range)) 786 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.instances.getClass().getName()+" was encountered"); 787 return (Range) this.instances; 788 } 789 790 public boolean hasInstancesRange() { 791 return this.instances instanceof Range; 792 } 793 794 public boolean hasInstances() { 795 return this.instances != null && !this.instances.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #instances} (Number of occurrences meeting the characteristic.) 800 */ 801 public EvidenceVariableCharacteristicComponent setInstances(DataType value) { 802 if (value != null && !(value instanceof Quantity || value instanceof Range)) 803 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.instances[x]: "+value.fhirType()); 804 this.instances = value; 805 return this; 806 } 807 808 /** 809 * @return {@link #duration} (Length of time in which the characteristic is met.) 810 */ 811 public DataType getDuration() { 812 return this.duration; 813 } 814 815 /** 816 * @return {@link #duration} (Length of time in which the characteristic is met.) 817 */ 818 public Quantity getDurationQuantity() throws FHIRException { 819 if (this.duration == null) 820 this.duration = new Quantity(); 821 if (!(this.duration instanceof Quantity)) 822 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.duration.getClass().getName()+" was encountered"); 823 return (Quantity) this.duration; 824 } 825 826 public boolean hasDurationQuantity() { 827 return this.duration instanceof Quantity; 828 } 829 830 /** 831 * @return {@link #duration} (Length of time in which the characteristic is met.) 832 */ 833 public Range getDurationRange() throws FHIRException { 834 if (this.duration == null) 835 this.duration = new Range(); 836 if (!(this.duration instanceof Range)) 837 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.duration.getClass().getName()+" was encountered"); 838 return (Range) this.duration; 839 } 840 841 public boolean hasDurationRange() { 842 return this.duration instanceof Range; 843 } 844 845 public boolean hasDuration() { 846 return this.duration != null && !this.duration.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #duration} (Length of time in which the characteristic is met.) 851 */ 852 public EvidenceVariableCharacteristicComponent setDuration(DataType value) { 853 if (value != null && !(value instanceof Quantity || value instanceof Range)) 854 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.duration[x]: "+value.fhirType()); 855 this.duration = value; 856 return this; 857 } 858 859 /** 860 * @return {@link #timeFromEvent} (Timing in which the characteristic is determined.) 861 */ 862 public List<EvidenceVariableCharacteristicTimeFromEventComponent> getTimeFromEvent() { 863 if (this.timeFromEvent == null) 864 this.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 865 return this.timeFromEvent; 866 } 867 868 /** 869 * @return Returns a reference to <code>this</code> for easy method chaining 870 */ 871 public EvidenceVariableCharacteristicComponent setTimeFromEvent(List<EvidenceVariableCharacteristicTimeFromEventComponent> theTimeFromEvent) { 872 this.timeFromEvent = theTimeFromEvent; 873 return this; 874 } 875 876 public boolean hasTimeFromEvent() { 877 if (this.timeFromEvent == null) 878 return false; 879 for (EvidenceVariableCharacteristicTimeFromEventComponent item : this.timeFromEvent) 880 if (!item.isEmpty()) 881 return true; 882 return false; 883 } 884 885 public EvidenceVariableCharacteristicTimeFromEventComponent addTimeFromEvent() { //3 886 EvidenceVariableCharacteristicTimeFromEventComponent t = new EvidenceVariableCharacteristicTimeFromEventComponent(); 887 if (this.timeFromEvent == null) 888 this.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 889 this.timeFromEvent.add(t); 890 return t; 891 } 892 893 public EvidenceVariableCharacteristicComponent addTimeFromEvent(EvidenceVariableCharacteristicTimeFromEventComponent t) { //3 894 if (t == null) 895 return this; 896 if (this.timeFromEvent == null) 897 this.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 898 this.timeFromEvent.add(t); 899 return this; 900 } 901 902 /** 903 * @return The first repetition of repeating field {@link #timeFromEvent}, creating it if it does not already exist {3} 904 */ 905 public EvidenceVariableCharacteristicTimeFromEventComponent getTimeFromEventFirstRep() { 906 if (getTimeFromEvent().isEmpty()) { 907 addTimeFromEvent(); 908 } 909 return getTimeFromEvent().get(0); 910 } 911 912 protected void listChildren(List<Property> children) { 913 super.listChildren(children); 914 children.add(new Property("linkId", "id", "Label used for when a characteristic refers to another characteristic.", 0, 1, linkId)); 915 children.add(new Property("description", "markdown", "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 0, 1, description)); 916 children.add(new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the characteristic.", 0, java.lang.Integer.MAX_VALUE, note)); 917 children.add(new Property("exclude", "boolean", "When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.", 0, 1, exclude)); 918 children.add(new Property("definitionReference", "Reference(EvidenceVariable|Group|Evidence)", "Defines the characteristic using a Reference.", 0, 1, definitionReference)); 919 children.add(new Property("definitionCanonical", "canonical(EvidenceVariable|Evidence)", "Defines the characteristic using Canonical.", 0, 1, definitionCanonical)); 920 children.add(new Property("definitionCodeableConcept", "CodeableConcept", "Defines the characteristic using CodeableConcept.", 0, 1, definitionCodeableConcept)); 921 children.add(new Property("definitionExpression", "Expression", "Defines the characteristic using Expression.", 0, 1, definitionExpression)); 922 children.add(new Property("definitionId", "id", "Defines the characteristic using id.", 0, 1, definitionId)); 923 children.add(new Property("definitionByTypeAndValue", "", "Defines the characteristic using both a type and value[x] elements.", 0, 1, definitionByTypeAndValue)); 924 children.add(new Property("definitionByCombination", "", "Defines the characteristic as a combination of two or more characteristics.", 0, 1, definitionByCombination)); 925 children.add(new Property("instances[x]", "Quantity|Range", "Number of occurrences meeting the characteristic.", 0, 1, instances)); 926 children.add(new Property("duration[x]", "Quantity|Range", "Length of time in which the characteristic is met.", 0, 1, duration)); 927 children.add(new Property("timeFromEvent", "", "Timing in which the characteristic is determined.", 0, java.lang.Integer.MAX_VALUE, timeFromEvent)); 928 } 929 930 @Override 931 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 932 switch (_hash) { 933 case -1102667083: /*linkId*/ return new Property("linkId", "id", "Label used for when a characteristic refers to another characteristic.", 0, 1, linkId); 934 case -1724546052: /*description*/ return new Property("description", "markdown", "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 0, 1, description); 935 case 3387378: /*note*/ return new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the characteristic.", 0, java.lang.Integer.MAX_VALUE, note); 936 case -1321148966: /*exclude*/ return new Property("exclude", "boolean", "When true, this characteristic is an exclusion criterion. In other words, not matching this characteristic definition is equivalent to meeting this criterion.", 0, 1, exclude); 937 case -820021448: /*definitionReference*/ return new Property("definitionReference", "Reference(EvidenceVariable|Group|Evidence)", "Defines the characteristic using a Reference.", 0, 1, definitionReference); 938 case 933485793: /*definitionCanonical*/ return new Property("definitionCanonical", "canonical(EvidenceVariable|Evidence)", "Defines the characteristic using Canonical.", 0, 1, definitionCanonical); 939 case -1446002226: /*definitionCodeableConcept*/ return new Property("definitionCodeableConcept", "CodeableConcept", "Defines the characteristic using CodeableConcept.", 0, 1, definitionCodeableConcept); 940 case 1463703627: /*definitionExpression*/ return new Property("definitionExpression", "Expression", "Defines the characteristic using Expression.", 0, 1, definitionExpression); 941 case 101791182: /*definitionId*/ return new Property("definitionId", "id", "Defines the characteristic using id.", 0, 1, definitionId); 942 case -164357794: /*definitionByTypeAndValue*/ return new Property("definitionByTypeAndValue", "", "Defines the characteristic using both a type and value[x] elements.", 0, 1, definitionByTypeAndValue); 943 case -2043280539: /*definitionByCombination*/ return new Property("definitionByCombination", "", "Defines the characteristic as a combination of two or more characteristics.", 0, 1, definitionByCombination); 944 case -736760510: /*instances[x]*/ return new Property("instances[x]", "Quantity|Range", "Number of occurrences meeting the characteristic.", 0, 1, instances); 945 case 29097598: /*instances*/ return new Property("instances[x]", "Quantity|Range", "Number of occurrences meeting the characteristic.", 0, 1, instances); 946 case -1378916055: /*instancesQuantity*/ return new Property("instances[x]", "Quantity", "Number of occurrences meeting the characteristic.", 0, 1, instances); 947 case 633776479: /*instancesRange*/ return new Property("instances[x]", "Range", "Number of occurrences meeting the characteristic.", 0, 1, instances); 948 case -478069140: /*duration[x]*/ return new Property("duration[x]", "Quantity|Range", "Length of time in which the characteristic is met.", 0, 1, duration); 949 case -1992012396: /*duration*/ return new Property("duration[x]", "Quantity|Range", "Length of time in which the characteristic is met.", 0, 1, duration); 950 case 159007295: /*durationQuantity*/ return new Property("duration[x]", "Quantity", "Length of time in which the characteristic is met.", 0, 1, duration); 951 case 128079881: /*durationRange*/ return new Property("duration[x]", "Range", "Length of time in which the characteristic is met.", 0, 1, duration); 952 case 2087274691: /*timeFromEvent*/ return new Property("timeFromEvent", "", "Timing in which the characteristic is determined.", 0, java.lang.Integer.MAX_VALUE, timeFromEvent); 953 default: return super.getNamedProperty(_hash, _name, _checkValid); 954 } 955 956 } 957 958 @Override 959 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 960 switch (hash) { 961 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // IdType 962 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 963 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 964 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // BooleanType 965 case -820021448: /*definitionReference*/ return this.definitionReference == null ? new Base[0] : new Base[] {this.definitionReference}; // Reference 966 case 933485793: /*definitionCanonical*/ return this.definitionCanonical == null ? new Base[0] : new Base[] {this.definitionCanonical}; // CanonicalType 967 case -1446002226: /*definitionCodeableConcept*/ return this.definitionCodeableConcept == null ? new Base[0] : new Base[] {this.definitionCodeableConcept}; // CodeableConcept 968 case 1463703627: /*definitionExpression*/ return this.definitionExpression == null ? new Base[0] : new Base[] {this.definitionExpression}; // Expression 969 case 101791182: /*definitionId*/ return this.definitionId == null ? new Base[0] : new Base[] {this.definitionId}; // IdType 970 case -164357794: /*definitionByTypeAndValue*/ return this.definitionByTypeAndValue == null ? new Base[0] : new Base[] {this.definitionByTypeAndValue}; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 971 case -2043280539: /*definitionByCombination*/ return this.definitionByCombination == null ? new Base[0] : new Base[] {this.definitionByCombination}; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 972 case 29097598: /*instances*/ return this.instances == null ? new Base[0] : new Base[] {this.instances}; // DataType 973 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // DataType 974 case 2087274691: /*timeFromEvent*/ return this.timeFromEvent == null ? new Base[0] : this.timeFromEvent.toArray(new Base[this.timeFromEvent.size()]); // EvidenceVariableCharacteristicTimeFromEventComponent 975 default: return super.getProperty(hash, name, checkValid); 976 } 977 978 } 979 980 @Override 981 public Base setProperty(int hash, String name, Base value) throws FHIRException { 982 switch (hash) { 983 case -1102667083: // linkId 984 this.linkId = TypeConvertor.castToId(value); // IdType 985 return value; 986 case -1724546052: // description 987 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 988 return value; 989 case 3387378: // note 990 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 991 return value; 992 case -1321148966: // exclude 993 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 994 return value; 995 case -820021448: // definitionReference 996 this.definitionReference = TypeConvertor.castToReference(value); // Reference 997 return value; 998 case 933485793: // definitionCanonical 999 this.definitionCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 1000 return value; 1001 case -1446002226: // definitionCodeableConcept 1002 this.definitionCodeableConcept = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1003 return value; 1004 case 1463703627: // definitionExpression 1005 this.definitionExpression = TypeConvertor.castToExpression(value); // Expression 1006 return value; 1007 case 101791182: // definitionId 1008 this.definitionId = TypeConvertor.castToId(value); // IdType 1009 return value; 1010 case -164357794: // definitionByTypeAndValue 1011 this.definitionByTypeAndValue = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) value; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 1012 return value; 1013 case -2043280539: // definitionByCombination 1014 this.definitionByCombination = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) value; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 1015 return value; 1016 case 29097598: // instances 1017 this.instances = TypeConvertor.castToType(value); // DataType 1018 return value; 1019 case -1992012396: // duration 1020 this.duration = TypeConvertor.castToType(value); // DataType 1021 return value; 1022 case 2087274691: // timeFromEvent 1023 this.getTimeFromEvent().add((EvidenceVariableCharacteristicTimeFromEventComponent) value); // EvidenceVariableCharacteristicTimeFromEventComponent 1024 return value; 1025 default: return super.setProperty(hash, name, value); 1026 } 1027 1028 } 1029 1030 @Override 1031 public Base setProperty(String name, Base value) throws FHIRException { 1032 if (name.equals("linkId")) { 1033 this.linkId = TypeConvertor.castToId(value); // IdType 1034 } else if (name.equals("description")) { 1035 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1036 } else if (name.equals("note")) { 1037 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1038 } else if (name.equals("exclude")) { 1039 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 1040 } else if (name.equals("definitionReference")) { 1041 this.definitionReference = TypeConvertor.castToReference(value); // Reference 1042 } else if (name.equals("definitionCanonical")) { 1043 this.definitionCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 1044 } else if (name.equals("definitionCodeableConcept")) { 1045 this.definitionCodeableConcept = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1046 } else if (name.equals("definitionExpression")) { 1047 this.definitionExpression = TypeConvertor.castToExpression(value); // Expression 1048 } else if (name.equals("definitionId")) { 1049 this.definitionId = TypeConvertor.castToId(value); // IdType 1050 } else if (name.equals("definitionByTypeAndValue")) { 1051 this.definitionByTypeAndValue = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) value; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 1052 } else if (name.equals("definitionByCombination")) { 1053 this.definitionByCombination = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) value; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 1054 } else if (name.equals("instances[x]")) { 1055 this.instances = TypeConvertor.castToType(value); // DataType 1056 } else if (name.equals("duration[x]")) { 1057 this.duration = TypeConvertor.castToType(value); // DataType 1058 } else if (name.equals("timeFromEvent")) { 1059 this.getTimeFromEvent().add((EvidenceVariableCharacteristicTimeFromEventComponent) value); 1060 } else 1061 return super.setProperty(name, value); 1062 return value; 1063 } 1064 1065 @Override 1066 public void removeChild(String name, Base value) throws FHIRException { 1067 if (name.equals("linkId")) { 1068 this.linkId = null; 1069 } else if (name.equals("description")) { 1070 this.description = null; 1071 } else if (name.equals("note")) { 1072 this.getNote().remove(value); 1073 } else if (name.equals("exclude")) { 1074 this.exclude = null; 1075 } else if (name.equals("definitionReference")) { 1076 this.definitionReference = null; 1077 } else if (name.equals("definitionCanonical")) { 1078 this.definitionCanonical = null; 1079 } else if (name.equals("definitionCodeableConcept")) { 1080 this.definitionCodeableConcept = null; 1081 } else if (name.equals("definitionExpression")) { 1082 this.definitionExpression = null; 1083 } else if (name.equals("definitionId")) { 1084 this.definitionId = null; 1085 } else if (name.equals("definitionByTypeAndValue")) { 1086 this.definitionByTypeAndValue = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) value; // EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent 1087 } else if (name.equals("definitionByCombination")) { 1088 this.definitionByCombination = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) value; // EvidenceVariableCharacteristicDefinitionByCombinationComponent 1089 } else if (name.equals("instances[x]")) { 1090 this.instances = null; 1091 } else if (name.equals("duration[x]")) { 1092 this.duration = null; 1093 } else if (name.equals("timeFromEvent")) { 1094 this.getTimeFromEvent().remove((EvidenceVariableCharacteristicTimeFromEventComponent) value); 1095 } else 1096 super.removeChild(name, value); 1097 1098 } 1099 1100 @Override 1101 public Base makeProperty(int hash, String name) throws FHIRException { 1102 switch (hash) { 1103 case -1102667083: return getLinkIdElement(); 1104 case -1724546052: return getDescriptionElement(); 1105 case 3387378: return addNote(); 1106 case -1321148966: return getExcludeElement(); 1107 case -820021448: return getDefinitionReference(); 1108 case 933485793: return getDefinitionCanonicalElement(); 1109 case -1446002226: return getDefinitionCodeableConcept(); 1110 case 1463703627: return getDefinitionExpression(); 1111 case 101791182: return getDefinitionIdElement(); 1112 case -164357794: return getDefinitionByTypeAndValue(); 1113 case -2043280539: return getDefinitionByCombination(); 1114 case -736760510: return getInstances(); 1115 case 29097598: return getInstances(); 1116 case -478069140: return getDuration(); 1117 case -1992012396: return getDuration(); 1118 case 2087274691: return addTimeFromEvent(); 1119 default: return super.makeProperty(hash, name); 1120 } 1121 1122 } 1123 1124 @Override 1125 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1126 switch (hash) { 1127 case -1102667083: /*linkId*/ return new String[] {"id"}; 1128 case -1724546052: /*description*/ return new String[] {"markdown"}; 1129 case 3387378: /*note*/ return new String[] {"Annotation"}; 1130 case -1321148966: /*exclude*/ return new String[] {"boolean"}; 1131 case -820021448: /*definitionReference*/ return new String[] {"Reference"}; 1132 case 933485793: /*definitionCanonical*/ return new String[] {"canonical"}; 1133 case -1446002226: /*definitionCodeableConcept*/ return new String[] {"CodeableConcept"}; 1134 case 1463703627: /*definitionExpression*/ return new String[] {"Expression"}; 1135 case 101791182: /*definitionId*/ return new String[] {"id"}; 1136 case -164357794: /*definitionByTypeAndValue*/ return new String[] {}; 1137 case -2043280539: /*definitionByCombination*/ return new String[] {}; 1138 case 29097598: /*instances*/ return new String[] {"Quantity", "Range"}; 1139 case -1992012396: /*duration*/ return new String[] {"Quantity", "Range"}; 1140 case 2087274691: /*timeFromEvent*/ return new String[] {}; 1141 default: return super.getTypesForProperty(hash, name); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base addChild(String name) throws FHIRException { 1148 if (name.equals("linkId")) { 1149 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.linkId"); 1150 } 1151 else if (name.equals("description")) { 1152 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.description"); 1153 } 1154 else if (name.equals("note")) { 1155 return addNote(); 1156 } 1157 else if (name.equals("exclude")) { 1158 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.exclude"); 1159 } 1160 else if (name.equals("definitionReference")) { 1161 this.definitionReference = new Reference(); 1162 return this.definitionReference; 1163 } 1164 else if (name.equals("definitionCanonical")) { 1165 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionCanonical"); 1166 } 1167 else if (name.equals("definitionCodeableConcept")) { 1168 this.definitionCodeableConcept = new CodeableConcept(); 1169 return this.definitionCodeableConcept; 1170 } 1171 else if (name.equals("definitionExpression")) { 1172 this.definitionExpression = new Expression(); 1173 return this.definitionExpression; 1174 } 1175 else if (name.equals("definitionId")) { 1176 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionId"); 1177 } 1178 else if (name.equals("definitionByTypeAndValue")) { 1179 this.definitionByTypeAndValue = new EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(); 1180 return this.definitionByTypeAndValue; 1181 } 1182 else if (name.equals("definitionByCombination")) { 1183 this.definitionByCombination = new EvidenceVariableCharacteristicDefinitionByCombinationComponent(); 1184 return this.definitionByCombination; 1185 } 1186 else if (name.equals("instancesQuantity")) { 1187 this.instances = new Quantity(); 1188 return this.instances; 1189 } 1190 else if (name.equals("instancesRange")) { 1191 this.instances = new Range(); 1192 return this.instances; 1193 } 1194 else if (name.equals("durationQuantity")) { 1195 this.duration = new Quantity(); 1196 return this.duration; 1197 } 1198 else if (name.equals("durationRange")) { 1199 this.duration = new Range(); 1200 return this.duration; 1201 } 1202 else if (name.equals("timeFromEvent")) { 1203 return addTimeFromEvent(); 1204 } 1205 else 1206 return super.addChild(name); 1207 } 1208 1209 public EvidenceVariableCharacteristicComponent copy() { 1210 EvidenceVariableCharacteristicComponent dst = new EvidenceVariableCharacteristicComponent(); 1211 copyValues(dst); 1212 return dst; 1213 } 1214 1215 public void copyValues(EvidenceVariableCharacteristicComponent dst) { 1216 super.copyValues(dst); 1217 dst.linkId = linkId == null ? null : linkId.copy(); 1218 dst.description = description == null ? null : description.copy(); 1219 if (note != null) { 1220 dst.note = new ArrayList<Annotation>(); 1221 for (Annotation i : note) 1222 dst.note.add(i.copy()); 1223 }; 1224 dst.exclude = exclude == null ? null : exclude.copy(); 1225 dst.definitionReference = definitionReference == null ? null : definitionReference.copy(); 1226 dst.definitionCanonical = definitionCanonical == null ? null : definitionCanonical.copy(); 1227 dst.definitionCodeableConcept = definitionCodeableConcept == null ? null : definitionCodeableConcept.copy(); 1228 dst.definitionExpression = definitionExpression == null ? null : definitionExpression.copy(); 1229 dst.definitionId = definitionId == null ? null : definitionId.copy(); 1230 dst.definitionByTypeAndValue = definitionByTypeAndValue == null ? null : definitionByTypeAndValue.copy(); 1231 dst.definitionByCombination = definitionByCombination == null ? null : definitionByCombination.copy(); 1232 dst.instances = instances == null ? null : instances.copy(); 1233 dst.duration = duration == null ? null : duration.copy(); 1234 if (timeFromEvent != null) { 1235 dst.timeFromEvent = new ArrayList<EvidenceVariableCharacteristicTimeFromEventComponent>(); 1236 for (EvidenceVariableCharacteristicTimeFromEventComponent i : timeFromEvent) 1237 dst.timeFromEvent.add(i.copy()); 1238 }; 1239 } 1240 1241 @Override 1242 public boolean equalsDeep(Base other_) { 1243 if (!super.equalsDeep(other_)) 1244 return false; 1245 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1246 return false; 1247 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1248 return compareDeep(linkId, o.linkId, true) && compareDeep(description, o.description, true) && compareDeep(note, o.note, true) 1249 && compareDeep(exclude, o.exclude, true) && compareDeep(definitionReference, o.definitionReference, true) 1250 && compareDeep(definitionCanonical, o.definitionCanonical, true) && compareDeep(definitionCodeableConcept, o.definitionCodeableConcept, true) 1251 && compareDeep(definitionExpression, o.definitionExpression, true) && compareDeep(definitionId, o.definitionId, true) 1252 && compareDeep(definitionByTypeAndValue, o.definitionByTypeAndValue, true) && compareDeep(definitionByCombination, o.definitionByCombination, true) 1253 && compareDeep(instances, o.instances, true) && compareDeep(duration, o.duration, true) && compareDeep(timeFromEvent, o.timeFromEvent, true) 1254 ; 1255 } 1256 1257 @Override 1258 public boolean equalsShallow(Base other_) { 1259 if (!super.equalsShallow(other_)) 1260 return false; 1261 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1262 return false; 1263 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1264 return compareValues(linkId, o.linkId, true) && compareValues(description, o.description, true) && compareValues(exclude, o.exclude, true) 1265 && compareValues(definitionCanonical, o.definitionCanonical, true) && compareValues(definitionId, o.definitionId, true) 1266 ; 1267 } 1268 1269 public boolean isEmpty() { 1270 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, description, note 1271 , exclude, definitionReference, definitionCanonical, definitionCodeableConcept, definitionExpression 1272 , definitionId, definitionByTypeAndValue, definitionByCombination, instances, duration 1273 , timeFromEvent); 1274 } 1275 1276 public String fhirType() { 1277 return "EvidenceVariable.characteristic"; 1278 1279 } 1280 1281 } 1282 1283 @Block() 1284 public static class EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent extends BackboneElement implements IBaseBackboneElement { 1285 /** 1286 * Used to express the type of characteristic. 1287 */ 1288 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1289 @Description(shortDefinition="Expresses the type of characteristic", formalDefinition="Used to express the type of characteristic." ) 1290 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/usage-context-type") 1291 protected CodeableConcept type; 1292 1293 /** 1294 * Method for how the characteristic value was determined. 1295 */ 1296 @Child(name = "method", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1297 @Description(shortDefinition="Method for how the characteristic value was determined", formalDefinition="Method for how the characteristic value was determined." ) 1298 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-method") 1299 protected List<CodeableConcept> method; 1300 1301 /** 1302 * Device used for determining characteristic. 1303 */ 1304 @Child(name = "device", type = {Device.class, DeviceMetric.class}, order=3, min=0, max=1, modifier=false, summary=false) 1305 @Description(shortDefinition="Device used for determining characteristic", formalDefinition="Device used for determining characteristic." ) 1306 protected Reference device; 1307 1308 /** 1309 * Defines the characteristic when paired with characteristic.type. 1310 */ 1311 @Child(name = "value", type = {CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, Reference.class, IdType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1312 @Description(shortDefinition="Defines the characteristic when coupled with characteristic.type", formalDefinition="Defines the characteristic when paired with characteristic.type." ) 1313 protected DataType value; 1314 1315 /** 1316 * Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero. 1317 */ 1318 @Child(name = "offset", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1319 @Description(shortDefinition="Reference point for valueQuantity or valueRange", formalDefinition="Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero." ) 1320 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/characteristic-offset") 1321 protected CodeableConcept offset; 1322 1323 private static final long serialVersionUID = -498341653L; 1324 1325 /** 1326 * Constructor 1327 */ 1328 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent() { 1329 super(); 1330 } 1331 1332 /** 1333 * Constructor 1334 */ 1335 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(CodeableConcept type, DataType value) { 1336 super(); 1337 this.setType(type); 1338 this.setValue(value); 1339 } 1340 1341 /** 1342 * @return {@link #type} (Used to express the type of characteristic.) 1343 */ 1344 public CodeableConcept getType() { 1345 if (this.type == null) 1346 if (Configuration.errorOnAutoCreate()) 1347 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent.type"); 1348 else if (Configuration.doAutoCreate()) 1349 this.type = new CodeableConcept(); // cc 1350 return this.type; 1351 } 1352 1353 public boolean hasType() { 1354 return this.type != null && !this.type.isEmpty(); 1355 } 1356 1357 /** 1358 * @param value {@link #type} (Used to express the type of characteristic.) 1359 */ 1360 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setType(CodeableConcept value) { 1361 this.type = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #method} (Method for how the characteristic value was determined.) 1367 */ 1368 public List<CodeableConcept> getMethod() { 1369 if (this.method == null) 1370 this.method = new ArrayList<CodeableConcept>(); 1371 return this.method; 1372 } 1373 1374 /** 1375 * @return Returns a reference to <code>this</code> for easy method chaining 1376 */ 1377 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setMethod(List<CodeableConcept> theMethod) { 1378 this.method = theMethod; 1379 return this; 1380 } 1381 1382 public boolean hasMethod() { 1383 if (this.method == null) 1384 return false; 1385 for (CodeableConcept item : this.method) 1386 if (!item.isEmpty()) 1387 return true; 1388 return false; 1389 } 1390 1391 public CodeableConcept addMethod() { //3 1392 CodeableConcept t = new CodeableConcept(); 1393 if (this.method == null) 1394 this.method = new ArrayList<CodeableConcept>(); 1395 this.method.add(t); 1396 return t; 1397 } 1398 1399 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent addMethod(CodeableConcept t) { //3 1400 if (t == null) 1401 return this; 1402 if (this.method == null) 1403 this.method = new ArrayList<CodeableConcept>(); 1404 this.method.add(t); 1405 return this; 1406 } 1407 1408 /** 1409 * @return The first repetition of repeating field {@link #method}, creating it if it does not already exist {3} 1410 */ 1411 public CodeableConcept getMethodFirstRep() { 1412 if (getMethod().isEmpty()) { 1413 addMethod(); 1414 } 1415 return getMethod().get(0); 1416 } 1417 1418 /** 1419 * @return {@link #device} (Device used for determining characteristic.) 1420 */ 1421 public Reference getDevice() { 1422 if (this.device == null) 1423 if (Configuration.errorOnAutoCreate()) 1424 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent.device"); 1425 else if (Configuration.doAutoCreate()) 1426 this.device = new Reference(); // cc 1427 return this.device; 1428 } 1429 1430 public boolean hasDevice() { 1431 return this.device != null && !this.device.isEmpty(); 1432 } 1433 1434 /** 1435 * @param value {@link #device} (Device used for determining characteristic.) 1436 */ 1437 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setDevice(Reference value) { 1438 this.device = value; 1439 return this; 1440 } 1441 1442 /** 1443 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1444 */ 1445 public DataType getValue() { 1446 return this.value; 1447 } 1448 1449 /** 1450 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1451 */ 1452 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1453 if (this.value == null) 1454 this.value = new CodeableConcept(); 1455 if (!(this.value instanceof CodeableConcept)) 1456 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1457 return (CodeableConcept) this.value; 1458 } 1459 1460 public boolean hasValueCodeableConcept() { 1461 return this.value instanceof CodeableConcept; 1462 } 1463 1464 /** 1465 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1466 */ 1467 public BooleanType getValueBooleanType() throws FHIRException { 1468 if (this.value == null) 1469 this.value = new BooleanType(); 1470 if (!(this.value instanceof BooleanType)) 1471 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1472 return (BooleanType) this.value; 1473 } 1474 1475 public boolean hasValueBooleanType() { 1476 return this.value instanceof BooleanType; 1477 } 1478 1479 /** 1480 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1481 */ 1482 public Quantity getValueQuantity() throws FHIRException { 1483 if (this.value == null) 1484 this.value = new Quantity(); 1485 if (!(this.value instanceof Quantity)) 1486 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1487 return (Quantity) this.value; 1488 } 1489 1490 public boolean hasValueQuantity() { 1491 return this.value instanceof Quantity; 1492 } 1493 1494 /** 1495 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1496 */ 1497 public Range getValueRange() throws FHIRException { 1498 if (this.value == null) 1499 this.value = new Range(); 1500 if (!(this.value instanceof Range)) 1501 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1502 return (Range) this.value; 1503 } 1504 1505 public boolean hasValueRange() { 1506 return this.value instanceof Range; 1507 } 1508 1509 /** 1510 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1511 */ 1512 public Reference getValueReference() throws FHIRException { 1513 if (this.value == null) 1514 this.value = new Reference(); 1515 if (!(this.value instanceof Reference)) 1516 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1517 return (Reference) this.value; 1518 } 1519 1520 public boolean hasValueReference() { 1521 return this.value instanceof Reference; 1522 } 1523 1524 /** 1525 * @return {@link #value} (Defines the characteristic when paired with characteristic.type.) 1526 */ 1527 public IdType getValueIdType() throws FHIRException { 1528 if (this.value == null) 1529 this.value = new IdType(); 1530 if (!(this.value instanceof IdType)) 1531 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 1532 return (IdType) this.value; 1533 } 1534 1535 public boolean hasValueIdType() { 1536 return this.value instanceof IdType; 1537 } 1538 1539 public boolean hasValue() { 1540 return this.value != null && !this.value.isEmpty(); 1541 } 1542 1543 /** 1544 * @param value {@link #value} (Defines the characteristic when paired with characteristic.type.) 1545 */ 1546 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setValue(DataType value) { 1547 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range || value instanceof Reference || value instanceof IdType)) 1548 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.definitionByTypeAndValue.value[x]: "+value.fhirType()); 1549 this.value = value; 1550 return this; 1551 } 1552 1553 /** 1554 * @return {@link #offset} (Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.) 1555 */ 1556 public CodeableConcept getOffset() { 1557 if (this.offset == null) 1558 if (Configuration.errorOnAutoCreate()) 1559 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent.offset"); 1560 else if (Configuration.doAutoCreate()) 1561 this.offset = new CodeableConcept(); // cc 1562 return this.offset; 1563 } 1564 1565 public boolean hasOffset() { 1566 return this.offset != null && !this.offset.isEmpty(); 1567 } 1568 1569 /** 1570 * @param value {@link #offset} (Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.) 1571 */ 1572 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent setOffset(CodeableConcept value) { 1573 this.offset = value; 1574 return this; 1575 } 1576 1577 protected void listChildren(List<Property> children) { 1578 super.listChildren(children); 1579 children.add(new Property("type", "CodeableConcept", "Used to express the type of characteristic.", 0, 1, type)); 1580 children.add(new Property("method", "CodeableConcept", "Method for how the characteristic value was determined.", 0, java.lang.Integer.MAX_VALUE, method)); 1581 children.add(new Property("device", "Reference(Device|DeviceMetric)", "Device used for determining characteristic.", 0, 1, device)); 1582 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference|id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value)); 1583 children.add(new Property("offset", "CodeableConcept", "Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.", 0, 1, offset)); 1584 } 1585 1586 @Override 1587 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1588 switch (_hash) { 1589 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Used to express the type of characteristic.", 0, 1, type); 1590 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Method for how the characteristic value was determined.", 0, java.lang.Integer.MAX_VALUE, method); 1591 case -1335157162: /*device*/ return new Property("device", "Reference(Device|DeviceMetric)", "Device used for determining characteristic.", 0, 1, device); 1592 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference|id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1593 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference|id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1594 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1595 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1596 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1597 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1598 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1599 case 231604844: /*valueId*/ return new Property("value[x]", "id", "Defines the characteristic when paired with characteristic.type.", 0, 1, value); 1600 case -1019779949: /*offset*/ return new Property("offset", "CodeableConcept", "Defines the reference point for comparison when valueQuantity or valueRange is not compared to zero.", 0, 1, offset); 1601 default: return super.getNamedProperty(_hash, _name, _checkValid); 1602 } 1603 1604 } 1605 1606 @Override 1607 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1608 switch (hash) { 1609 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1610 case -1077554975: /*method*/ return this.method == null ? new Base[0] : this.method.toArray(new Base[this.method.size()]); // CodeableConcept 1611 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 1612 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1613 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // CodeableConcept 1614 default: return super.getProperty(hash, name, checkValid); 1615 } 1616 1617 } 1618 1619 @Override 1620 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1621 switch (hash) { 1622 case 3575610: // type 1623 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1624 return value; 1625 case -1077554975: // method 1626 this.getMethod().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1627 return value; 1628 case -1335157162: // device 1629 this.device = TypeConvertor.castToReference(value); // Reference 1630 return value; 1631 case 111972721: // value 1632 this.value = TypeConvertor.castToType(value); // DataType 1633 return value; 1634 case -1019779949: // offset 1635 this.offset = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1636 return value; 1637 default: return super.setProperty(hash, name, value); 1638 } 1639 1640 } 1641 1642 @Override 1643 public Base setProperty(String name, Base value) throws FHIRException { 1644 if (name.equals("type")) { 1645 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1646 } else if (name.equals("method")) { 1647 this.getMethod().add(TypeConvertor.castToCodeableConcept(value)); 1648 } else if (name.equals("device")) { 1649 this.device = TypeConvertor.castToReference(value); // Reference 1650 } else if (name.equals("value[x]")) { 1651 this.value = TypeConvertor.castToType(value); // DataType 1652 } else if (name.equals("offset")) { 1653 this.offset = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1654 } else 1655 return super.setProperty(name, value); 1656 return value; 1657 } 1658 1659 @Override 1660 public void removeChild(String name, Base value) throws FHIRException { 1661 if (name.equals("type")) { 1662 this.type = null; 1663 } else if (name.equals("method")) { 1664 this.getMethod().remove(value); 1665 } else if (name.equals("device")) { 1666 this.device = null; 1667 } else if (name.equals("value[x]")) { 1668 this.value = null; 1669 } else if (name.equals("offset")) { 1670 this.offset = null; 1671 } else 1672 super.removeChild(name, value); 1673 1674 } 1675 1676 @Override 1677 public Base makeProperty(int hash, String name) throws FHIRException { 1678 switch (hash) { 1679 case 3575610: return getType(); 1680 case -1077554975: return addMethod(); 1681 case -1335157162: return getDevice(); 1682 case -1410166417: return getValue(); 1683 case 111972721: return getValue(); 1684 case -1019779949: return getOffset(); 1685 default: return super.makeProperty(hash, name); 1686 } 1687 1688 } 1689 1690 @Override 1691 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1692 switch (hash) { 1693 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1694 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1695 case -1335157162: /*device*/ return new String[] {"Reference"}; 1696 case 111972721: /*value*/ return new String[] {"CodeableConcept", "boolean", "Quantity", "Range", "Reference", "id"}; 1697 case -1019779949: /*offset*/ return new String[] {"CodeableConcept"}; 1698 default: return super.getTypesForProperty(hash, name); 1699 } 1700 1701 } 1702 1703 @Override 1704 public Base addChild(String name) throws FHIRException { 1705 if (name.equals("type")) { 1706 this.type = new CodeableConcept(); 1707 return this.type; 1708 } 1709 else if (name.equals("method")) { 1710 return addMethod(); 1711 } 1712 else if (name.equals("device")) { 1713 this.device = new Reference(); 1714 return this.device; 1715 } 1716 else if (name.equals("valueCodeableConcept")) { 1717 this.value = new CodeableConcept(); 1718 return this.value; 1719 } 1720 else if (name.equals("valueBoolean")) { 1721 this.value = new BooleanType(); 1722 return this.value; 1723 } 1724 else if (name.equals("valueQuantity")) { 1725 this.value = new Quantity(); 1726 return this.value; 1727 } 1728 else if (name.equals("valueRange")) { 1729 this.value = new Range(); 1730 return this.value; 1731 } 1732 else if (name.equals("valueReference")) { 1733 this.value = new Reference(); 1734 return this.value; 1735 } 1736 else if (name.equals("valueId")) { 1737 this.value = new IdType(); 1738 return this.value; 1739 } 1740 else if (name.equals("offset")) { 1741 this.offset = new CodeableConcept(); 1742 return this.offset; 1743 } 1744 else 1745 return super.addChild(name); 1746 } 1747 1748 public EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent copy() { 1749 EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent dst = new EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent(); 1750 copyValues(dst); 1751 return dst; 1752 } 1753 1754 public void copyValues(EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent dst) { 1755 super.copyValues(dst); 1756 dst.type = type == null ? null : type.copy(); 1757 if (method != null) { 1758 dst.method = new ArrayList<CodeableConcept>(); 1759 for (CodeableConcept i : method) 1760 dst.method.add(i.copy()); 1761 }; 1762 dst.device = device == null ? null : device.copy(); 1763 dst.value = value == null ? null : value.copy(); 1764 dst.offset = offset == null ? null : offset.copy(); 1765 } 1766 1767 @Override 1768 public boolean equalsDeep(Base other_) { 1769 if (!super.equalsDeep(other_)) 1770 return false; 1771 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent)) 1772 return false; 1773 EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent o = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) other_; 1774 return compareDeep(type, o.type, true) && compareDeep(method, o.method, true) && compareDeep(device, o.device, true) 1775 && compareDeep(value, o.value, true) && compareDeep(offset, o.offset, true); 1776 } 1777 1778 @Override 1779 public boolean equalsShallow(Base other_) { 1780 if (!super.equalsShallow(other_)) 1781 return false; 1782 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent)) 1783 return false; 1784 EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent o = (EvidenceVariableCharacteristicDefinitionByTypeAndValueComponent) other_; 1785 return true; 1786 } 1787 1788 public boolean isEmpty() { 1789 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, method, device, value 1790 , offset); 1791 } 1792 1793 public String fhirType() { 1794 return "EvidenceVariable.characteristic.definitionByTypeAndValue"; 1795 1796 } 1797 1798 } 1799 1800 @Block() 1801 public static class EvidenceVariableCharacteristicDefinitionByCombinationComponent extends BackboneElement implements IBaseBackboneElement { 1802 /** 1803 * Used to specify if two or more characteristics are combined with OR or AND. 1804 */ 1805 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1806 @Description(shortDefinition="all-of | any-of | at-least | at-most | statistical | net-effect | dataset", formalDefinition="Used to specify if two or more characteristics are combined with OR or AND." ) 1807 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/characteristic-combination") 1808 protected Enumeration<CharacteristicCombination> code; 1809 1810 /** 1811 * Provides the value of "n" when "at-least" or "at-most" codes are used. 1812 */ 1813 @Child(name = "threshold", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1814 @Description(shortDefinition="Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used", formalDefinition="Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used." ) 1815 protected PositiveIntType threshold; 1816 1817 /** 1818 * A defining factor of the characteristic. 1819 */ 1820 @Child(name = "characteristic", type = {EvidenceVariableCharacteristicComponent.class}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1821 @Description(shortDefinition="A defining factor of the characteristic", formalDefinition="A defining factor of the characteristic." ) 1822 protected List<EvidenceVariableCharacteristicComponent> characteristic; 1823 1824 private static final long serialVersionUID = -2053118515L; 1825 1826 /** 1827 * Constructor 1828 */ 1829 public EvidenceVariableCharacteristicDefinitionByCombinationComponent() { 1830 super(); 1831 } 1832 1833 /** 1834 * Constructor 1835 */ 1836 public EvidenceVariableCharacteristicDefinitionByCombinationComponent(CharacteristicCombination code, EvidenceVariableCharacteristicComponent characteristic) { 1837 super(); 1838 this.setCode(code); 1839 this.addCharacteristic(characteristic); 1840 } 1841 1842 /** 1843 * @return {@link #code} (Used to specify if two or more characteristics are combined with OR or AND.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1844 */ 1845 public Enumeration<CharacteristicCombination> getCodeElement() { 1846 if (this.code == null) 1847 if (Configuration.errorOnAutoCreate()) 1848 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByCombinationComponent.code"); 1849 else if (Configuration.doAutoCreate()) 1850 this.code = new Enumeration<CharacteristicCombination>(new CharacteristicCombinationEnumFactory()); // bb 1851 return this.code; 1852 } 1853 1854 public boolean hasCodeElement() { 1855 return this.code != null && !this.code.isEmpty(); 1856 } 1857 1858 public boolean hasCode() { 1859 return this.code != null && !this.code.isEmpty(); 1860 } 1861 1862 /** 1863 * @param value {@link #code} (Used to specify if two or more characteristics are combined with OR or AND.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1864 */ 1865 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setCodeElement(Enumeration<CharacteristicCombination> value) { 1866 this.code = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return Used to specify if two or more characteristics are combined with OR or AND. 1872 */ 1873 public CharacteristicCombination getCode() { 1874 return this.code == null ? null : this.code.getValue(); 1875 } 1876 1877 /** 1878 * @param value Used to specify if two or more characteristics are combined with OR or AND. 1879 */ 1880 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setCode(CharacteristicCombination value) { 1881 if (this.code == null) 1882 this.code = new Enumeration<CharacteristicCombination>(new CharacteristicCombinationEnumFactory()); 1883 this.code.setValue(value); 1884 return this; 1885 } 1886 1887 /** 1888 * @return {@link #threshold} (Provides the value of "n" when "at-least" or "at-most" codes are used.). This is the underlying object with id, value and extensions. The accessor "getThreshold" gives direct access to the value 1889 */ 1890 public PositiveIntType getThresholdElement() { 1891 if (this.threshold == null) 1892 if (Configuration.errorOnAutoCreate()) 1893 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicDefinitionByCombinationComponent.threshold"); 1894 else if (Configuration.doAutoCreate()) 1895 this.threshold = new PositiveIntType(); // bb 1896 return this.threshold; 1897 } 1898 1899 public boolean hasThresholdElement() { 1900 return this.threshold != null && !this.threshold.isEmpty(); 1901 } 1902 1903 public boolean hasThreshold() { 1904 return this.threshold != null && !this.threshold.isEmpty(); 1905 } 1906 1907 /** 1908 * @param value {@link #threshold} (Provides the value of "n" when "at-least" or "at-most" codes are used.). This is the underlying object with id, value and extensions. The accessor "getThreshold" gives direct access to the value 1909 */ 1910 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setThresholdElement(PositiveIntType value) { 1911 this.threshold = value; 1912 return this; 1913 } 1914 1915 /** 1916 * @return Provides the value of "n" when "at-least" or "at-most" codes are used. 1917 */ 1918 public int getThreshold() { 1919 return this.threshold == null || this.threshold.isEmpty() ? 0 : this.threshold.getValue(); 1920 } 1921 1922 /** 1923 * @param value Provides the value of "n" when "at-least" or "at-most" codes are used. 1924 */ 1925 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setThreshold(int value) { 1926 if (this.threshold == null) 1927 this.threshold = new PositiveIntType(); 1928 this.threshold.setValue(value); 1929 return this; 1930 } 1931 1932 /** 1933 * @return {@link #characteristic} (A defining factor of the characteristic.) 1934 */ 1935 public List<EvidenceVariableCharacteristicComponent> getCharacteristic() { 1936 if (this.characteristic == null) 1937 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 1938 return this.characteristic; 1939 } 1940 1941 /** 1942 * @return Returns a reference to <code>this</code> for easy method chaining 1943 */ 1944 public EvidenceVariableCharacteristicDefinitionByCombinationComponent setCharacteristic(List<EvidenceVariableCharacteristicComponent> theCharacteristic) { 1945 this.characteristic = theCharacteristic; 1946 return this; 1947 } 1948 1949 public boolean hasCharacteristic() { 1950 if (this.characteristic == null) 1951 return false; 1952 for (EvidenceVariableCharacteristicComponent item : this.characteristic) 1953 if (!item.isEmpty()) 1954 return true; 1955 return false; 1956 } 1957 1958 public EvidenceVariableCharacteristicComponent addCharacteristic() { //3 1959 EvidenceVariableCharacteristicComponent t = new EvidenceVariableCharacteristicComponent(); 1960 if (this.characteristic == null) 1961 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 1962 this.characteristic.add(t); 1963 return t; 1964 } 1965 1966 public EvidenceVariableCharacteristicDefinitionByCombinationComponent addCharacteristic(EvidenceVariableCharacteristicComponent t) { //3 1967 if (t == null) 1968 return this; 1969 if (this.characteristic == null) 1970 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 1971 this.characteristic.add(t); 1972 return this; 1973 } 1974 1975 /** 1976 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 1977 */ 1978 public EvidenceVariableCharacteristicComponent getCharacteristicFirstRep() { 1979 if (getCharacteristic().isEmpty()) { 1980 addCharacteristic(); 1981 } 1982 return getCharacteristic().get(0); 1983 } 1984 1985 protected void listChildren(List<Property> children) { 1986 super.listChildren(children); 1987 children.add(new Property("code", "code", "Used to specify if two or more characteristics are combined with OR or AND.", 0, 1, code)); 1988 children.add(new Property("threshold", "positiveInt", "Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used.", 0, 1, threshold)); 1989 children.add(new Property("characteristic", "@EvidenceVariable.characteristic", "A defining factor of the characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 1990 } 1991 1992 @Override 1993 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1994 switch (_hash) { 1995 case 3059181: /*code*/ return new Property("code", "code", "Used to specify if two or more characteristics are combined with OR or AND.", 0, 1, code); 1996 case -1545477013: /*threshold*/ return new Property("threshold", "positiveInt", "Provides the value of \"n\" when \"at-least\" or \"at-most\" codes are used.", 0, 1, threshold); 1997 case 366313883: /*characteristic*/ return new Property("characteristic", "@EvidenceVariable.characteristic", "A defining factor of the characteristic.", 0, java.lang.Integer.MAX_VALUE, characteristic); 1998 default: return super.getNamedProperty(_hash, _name, _checkValid); 1999 } 2000 2001 } 2002 2003 @Override 2004 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2005 switch (hash) { 2006 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<CharacteristicCombination> 2007 case -1545477013: /*threshold*/ return this.threshold == null ? new Base[0] : new Base[] {this.threshold}; // PositiveIntType 2008 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceVariableCharacteristicComponent 2009 default: return super.getProperty(hash, name, checkValid); 2010 } 2011 2012 } 2013 2014 @Override 2015 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2016 switch (hash) { 2017 case 3059181: // code 2018 value = new CharacteristicCombinationEnumFactory().fromType(TypeConvertor.castToCode(value)); 2019 this.code = (Enumeration) value; // Enumeration<CharacteristicCombination> 2020 return value; 2021 case -1545477013: // threshold 2022 this.threshold = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2023 return value; 2024 case 366313883: // characteristic 2025 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); // EvidenceVariableCharacteristicComponent 2026 return value; 2027 default: return super.setProperty(hash, name, value); 2028 } 2029 2030 } 2031 2032 @Override 2033 public Base setProperty(String name, Base value) throws FHIRException { 2034 if (name.equals("code")) { 2035 value = new CharacteristicCombinationEnumFactory().fromType(TypeConvertor.castToCode(value)); 2036 this.code = (Enumeration) value; // Enumeration<CharacteristicCombination> 2037 } else if (name.equals("threshold")) { 2038 this.threshold = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2039 } else if (name.equals("characteristic")) { 2040 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); 2041 } else 2042 return super.setProperty(name, value); 2043 return value; 2044 } 2045 2046 @Override 2047 public void removeChild(String name, Base value) throws FHIRException { 2048 if (name.equals("code")) { 2049 value = new CharacteristicCombinationEnumFactory().fromType(TypeConvertor.castToCode(value)); 2050 this.code = (Enumeration) value; // Enumeration<CharacteristicCombination> 2051 } else if (name.equals("threshold")) { 2052 this.threshold = null; 2053 } else if (name.equals("characteristic")) { 2054 this.getCharacteristic().remove((EvidenceVariableCharacteristicComponent) value); 2055 } else 2056 super.removeChild(name, value); 2057 2058 } 2059 2060 @Override 2061 public Base makeProperty(int hash, String name) throws FHIRException { 2062 switch (hash) { 2063 case 3059181: return getCodeElement(); 2064 case -1545477013: return getThresholdElement(); 2065 case 366313883: return addCharacteristic(); 2066 default: return super.makeProperty(hash, name); 2067 } 2068 2069 } 2070 2071 @Override 2072 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2073 switch (hash) { 2074 case 3059181: /*code*/ return new String[] {"code"}; 2075 case -1545477013: /*threshold*/ return new String[] {"positiveInt"}; 2076 case 366313883: /*characteristic*/ return new String[] {"@EvidenceVariable.characteristic"}; 2077 default: return super.getTypesForProperty(hash, name); 2078 } 2079 2080 } 2081 2082 @Override 2083 public Base addChild(String name) throws FHIRException { 2084 if (name.equals("code")) { 2085 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionByCombination.code"); 2086 } 2087 else if (name.equals("threshold")) { 2088 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.definitionByCombination.threshold"); 2089 } 2090 else if (name.equals("characteristic")) { 2091 return addCharacteristic(); 2092 } 2093 else 2094 return super.addChild(name); 2095 } 2096 2097 public EvidenceVariableCharacteristicDefinitionByCombinationComponent copy() { 2098 EvidenceVariableCharacteristicDefinitionByCombinationComponent dst = new EvidenceVariableCharacteristicDefinitionByCombinationComponent(); 2099 copyValues(dst); 2100 return dst; 2101 } 2102 2103 public void copyValues(EvidenceVariableCharacteristicDefinitionByCombinationComponent dst) { 2104 super.copyValues(dst); 2105 dst.code = code == null ? null : code.copy(); 2106 dst.threshold = threshold == null ? null : threshold.copy(); 2107 if (characteristic != null) { 2108 dst.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 2109 for (EvidenceVariableCharacteristicComponent i : characteristic) 2110 dst.characteristic.add(i.copy()); 2111 }; 2112 } 2113 2114 @Override 2115 public boolean equalsDeep(Base other_) { 2116 if (!super.equalsDeep(other_)) 2117 return false; 2118 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByCombinationComponent)) 2119 return false; 2120 EvidenceVariableCharacteristicDefinitionByCombinationComponent o = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) other_; 2121 return compareDeep(code, o.code, true) && compareDeep(threshold, o.threshold, true) && compareDeep(characteristic, o.characteristic, true) 2122 ; 2123 } 2124 2125 @Override 2126 public boolean equalsShallow(Base other_) { 2127 if (!super.equalsShallow(other_)) 2128 return false; 2129 if (!(other_ instanceof EvidenceVariableCharacteristicDefinitionByCombinationComponent)) 2130 return false; 2131 EvidenceVariableCharacteristicDefinitionByCombinationComponent o = (EvidenceVariableCharacteristicDefinitionByCombinationComponent) other_; 2132 return compareValues(code, o.code, true) && compareValues(threshold, o.threshold, true); 2133 } 2134 2135 public boolean isEmpty() { 2136 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, threshold, characteristic 2137 ); 2138 } 2139 2140 public String fhirType() { 2141 return "EvidenceVariable.characteristic.definitionByCombination"; 2142 2143 } 2144 2145 } 2146 2147 @Block() 2148 public static class EvidenceVariableCharacteristicTimeFromEventComponent extends BackboneElement implements IBaseBackboneElement { 2149 /** 2150 * Human readable description. 2151 */ 2152 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2153 @Description(shortDefinition="Human readable description", formalDefinition="Human readable description." ) 2154 protected MarkdownType description; 2155 2156 /** 2157 * A human-readable string to clarify or explain concepts about the timeFromEvent. 2158 */ 2159 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2160 @Description(shortDefinition="Used for footnotes or explanatory notes", formalDefinition="A human-readable string to clarify or explain concepts about the timeFromEvent." ) 2161 protected List<Annotation> note; 2162 2163 /** 2164 * The event used as a base point (reference point) in time. 2165 */ 2166 @Child(name = "event", type = {CodeableConcept.class, Reference.class, DateTimeType.class, IdType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2167 @Description(shortDefinition="The event used as a base point (reference point) in time", formalDefinition="The event used as a base point (reference point) in time." ) 2168 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/evidence-variable-event") 2169 protected DataType event; 2170 2171 /** 2172 * Used to express the observation at a defined amount of time before or after the event. 2173 */ 2174 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 2175 @Description(shortDefinition="Used to express the observation at a defined amount of time before or after the event", formalDefinition="Used to express the observation at a defined amount of time before or after the event." ) 2176 protected Quantity quantity; 2177 2178 /** 2179 * Used to express the observation within a period before and/or after the event. 2180 */ 2181 @Child(name = "range", type = {Range.class}, order=5, min=0, max=1, modifier=false, summary=false) 2182 @Description(shortDefinition="Used to express the observation within a period before and/or after the event", formalDefinition="Used to express the observation within a period before and/or after the event." ) 2183 protected Range range; 2184 2185 private static final long serialVersionUID = -1593501640L; 2186 2187 /** 2188 * Constructor 2189 */ 2190 public EvidenceVariableCharacteristicTimeFromEventComponent() { 2191 super(); 2192 } 2193 2194 /** 2195 * @return {@link #description} (Human readable description.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2196 */ 2197 public MarkdownType getDescriptionElement() { 2198 if (this.description == null) 2199 if (Configuration.errorOnAutoCreate()) 2200 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicTimeFromEventComponent.description"); 2201 else if (Configuration.doAutoCreate()) 2202 this.description = new MarkdownType(); // bb 2203 return this.description; 2204 } 2205 2206 public boolean hasDescriptionElement() { 2207 return this.description != null && !this.description.isEmpty(); 2208 } 2209 2210 public boolean hasDescription() { 2211 return this.description != null && !this.description.isEmpty(); 2212 } 2213 2214 /** 2215 * @param value {@link #description} (Human readable description.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2216 */ 2217 public EvidenceVariableCharacteristicTimeFromEventComponent setDescriptionElement(MarkdownType value) { 2218 this.description = value; 2219 return this; 2220 } 2221 2222 /** 2223 * @return Human readable description. 2224 */ 2225 public String getDescription() { 2226 return this.description == null ? null : this.description.getValue(); 2227 } 2228 2229 /** 2230 * @param value Human readable description. 2231 */ 2232 public EvidenceVariableCharacteristicTimeFromEventComponent setDescription(String value) { 2233 if (Utilities.noString(value)) 2234 this.description = null; 2235 else { 2236 if (this.description == null) 2237 this.description = new MarkdownType(); 2238 this.description.setValue(value); 2239 } 2240 return this; 2241 } 2242 2243 /** 2244 * @return {@link #note} (A human-readable string to clarify or explain concepts about the timeFromEvent.) 2245 */ 2246 public List<Annotation> getNote() { 2247 if (this.note == null) 2248 this.note = new ArrayList<Annotation>(); 2249 return this.note; 2250 } 2251 2252 /** 2253 * @return Returns a reference to <code>this</code> for easy method chaining 2254 */ 2255 public EvidenceVariableCharacteristicTimeFromEventComponent setNote(List<Annotation> theNote) { 2256 this.note = theNote; 2257 return this; 2258 } 2259 2260 public boolean hasNote() { 2261 if (this.note == null) 2262 return false; 2263 for (Annotation item : this.note) 2264 if (!item.isEmpty()) 2265 return true; 2266 return false; 2267 } 2268 2269 public Annotation addNote() { //3 2270 Annotation t = new Annotation(); 2271 if (this.note == null) 2272 this.note = new ArrayList<Annotation>(); 2273 this.note.add(t); 2274 return t; 2275 } 2276 2277 public EvidenceVariableCharacteristicTimeFromEventComponent addNote(Annotation t) { //3 2278 if (t == null) 2279 return this; 2280 if (this.note == null) 2281 this.note = new ArrayList<Annotation>(); 2282 this.note.add(t); 2283 return this; 2284 } 2285 2286 /** 2287 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2288 */ 2289 public Annotation getNoteFirstRep() { 2290 if (getNote().isEmpty()) { 2291 addNote(); 2292 } 2293 return getNote().get(0); 2294 } 2295 2296 /** 2297 * @return {@link #event} (The event used as a base point (reference point) in time.) 2298 */ 2299 public DataType getEvent() { 2300 return this.event; 2301 } 2302 2303 /** 2304 * @return {@link #event} (The event used as a base point (reference point) in time.) 2305 */ 2306 public CodeableConcept getEventCodeableConcept() throws FHIRException { 2307 if (this.event == null) 2308 this.event = new CodeableConcept(); 2309 if (!(this.event instanceof CodeableConcept)) 2310 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.event.getClass().getName()+" was encountered"); 2311 return (CodeableConcept) this.event; 2312 } 2313 2314 public boolean hasEventCodeableConcept() { 2315 return this.event instanceof CodeableConcept; 2316 } 2317 2318 /** 2319 * @return {@link #event} (The event used as a base point (reference point) in time.) 2320 */ 2321 public Reference getEventReference() throws FHIRException { 2322 if (this.event == null) 2323 this.event = new Reference(); 2324 if (!(this.event instanceof Reference)) 2325 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.event.getClass().getName()+" was encountered"); 2326 return (Reference) this.event; 2327 } 2328 2329 public boolean hasEventReference() { 2330 return this.event instanceof Reference; 2331 } 2332 2333 /** 2334 * @return {@link #event} (The event used as a base point (reference point) in time.) 2335 */ 2336 public DateTimeType getEventDateTimeType() throws FHIRException { 2337 if (this.event == null) 2338 this.event = new DateTimeType(); 2339 if (!(this.event instanceof DateTimeType)) 2340 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.event.getClass().getName()+" was encountered"); 2341 return (DateTimeType) this.event; 2342 } 2343 2344 public boolean hasEventDateTimeType() { 2345 return this.event instanceof DateTimeType; 2346 } 2347 2348 /** 2349 * @return {@link #event} (The event used as a base point (reference point) in time.) 2350 */ 2351 public IdType getEventIdType() throws FHIRException { 2352 if (this.event == null) 2353 this.event = new IdType(); 2354 if (!(this.event instanceof IdType)) 2355 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.event.getClass().getName()+" was encountered"); 2356 return (IdType) this.event; 2357 } 2358 2359 public boolean hasEventIdType() { 2360 return this.event instanceof IdType; 2361 } 2362 2363 public boolean hasEvent() { 2364 return this.event != null && !this.event.isEmpty(); 2365 } 2366 2367 /** 2368 * @param value {@link #event} (The event used as a base point (reference point) in time.) 2369 */ 2370 public EvidenceVariableCharacteristicTimeFromEventComponent setEvent(DataType value) { 2371 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference || value instanceof DateTimeType || value instanceof IdType)) 2372 throw new FHIRException("Not the right type for EvidenceVariable.characteristic.timeFromEvent.event[x]: "+value.fhirType()); 2373 this.event = value; 2374 return this; 2375 } 2376 2377 /** 2378 * @return {@link #quantity} (Used to express the observation at a defined amount of time before or after the event.) 2379 */ 2380 public Quantity getQuantity() { 2381 if (this.quantity == null) 2382 if (Configuration.errorOnAutoCreate()) 2383 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicTimeFromEventComponent.quantity"); 2384 else if (Configuration.doAutoCreate()) 2385 this.quantity = new Quantity(); // cc 2386 return this.quantity; 2387 } 2388 2389 public boolean hasQuantity() { 2390 return this.quantity != null && !this.quantity.isEmpty(); 2391 } 2392 2393 /** 2394 * @param value {@link #quantity} (Used to express the observation at a defined amount of time before or after the event.) 2395 */ 2396 public EvidenceVariableCharacteristicTimeFromEventComponent setQuantity(Quantity value) { 2397 this.quantity = value; 2398 return this; 2399 } 2400 2401 /** 2402 * @return {@link #range} (Used to express the observation within a period before and/or after the event.) 2403 */ 2404 public Range getRange() { 2405 if (this.range == null) 2406 if (Configuration.errorOnAutoCreate()) 2407 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicTimeFromEventComponent.range"); 2408 else if (Configuration.doAutoCreate()) 2409 this.range = new Range(); // cc 2410 return this.range; 2411 } 2412 2413 public boolean hasRange() { 2414 return this.range != null && !this.range.isEmpty(); 2415 } 2416 2417 /** 2418 * @param value {@link #range} (Used to express the observation within a period before and/or after the event.) 2419 */ 2420 public EvidenceVariableCharacteristicTimeFromEventComponent setRange(Range value) { 2421 this.range = value; 2422 return this; 2423 } 2424 2425 protected void listChildren(List<Property> children) { 2426 super.listChildren(children); 2427 children.add(new Property("description", "markdown", "Human readable description.", 0, 1, description)); 2428 children.add(new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the timeFromEvent.", 0, java.lang.Integer.MAX_VALUE, note)); 2429 children.add(new Property("event[x]", "CodeableConcept|Reference|dateTime|id", "The event used as a base point (reference point) in time.", 0, 1, event)); 2430 children.add(new Property("quantity", "Quantity", "Used to express the observation at a defined amount of time before or after the event.", 0, 1, quantity)); 2431 children.add(new Property("range", "Range", "Used to express the observation within a period before and/or after the event.", 0, 1, range)); 2432 } 2433 2434 @Override 2435 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2436 switch (_hash) { 2437 case -1724546052: /*description*/ return new Property("description", "markdown", "Human readable description.", 0, 1, description); 2438 case 3387378: /*note*/ return new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the timeFromEvent.", 0, java.lang.Integer.MAX_VALUE, note); 2439 case 278115238: /*event[x]*/ return new Property("event[x]", "CodeableConcept|Reference|dateTime|id", "The event used as a base point (reference point) in time.", 0, 1, event); 2440 case 96891546: /*event*/ return new Property("event[x]", "CodeableConcept|Reference|dateTime|id", "The event used as a base point (reference point) in time.", 0, 1, event); 2441 case 1464167847: /*eventCodeableConcept*/ return new Property("event[x]", "CodeableConcept", "The event used as a base point (reference point) in time.", 0, 1, event); 2442 case 30751185: /*eventReference*/ return new Property("event[x]", "Reference", "The event used as a base point (reference point) in time.", 0, 1, event); 2443 case -116077483: /*eventDateTime*/ return new Property("event[x]", "dateTime", "The event used as a base point (reference point) in time.", 0, 1, event); 2444 case -1376502443: /*eventId*/ return new Property("event[x]", "id", "The event used as a base point (reference point) in time.", 0, 1, event); 2445 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Used to express the observation at a defined amount of time before or after the event.", 0, 1, quantity); 2446 case 108280125: /*range*/ return new Property("range", "Range", "Used to express the observation within a period before and/or after the event.", 0, 1, range); 2447 default: return super.getNamedProperty(_hash, _name, _checkValid); 2448 } 2449 2450 } 2451 2452 @Override 2453 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2454 switch (hash) { 2455 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2456 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2457 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // DataType 2458 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 2459 case 108280125: /*range*/ return this.range == null ? new Base[0] : new Base[] {this.range}; // Range 2460 default: return super.getProperty(hash, name, checkValid); 2461 } 2462 2463 } 2464 2465 @Override 2466 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2467 switch (hash) { 2468 case -1724546052: // description 2469 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2470 return value; 2471 case 3387378: // note 2472 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2473 return value; 2474 case 96891546: // event 2475 this.event = TypeConvertor.castToType(value); // DataType 2476 return value; 2477 case -1285004149: // quantity 2478 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2479 return value; 2480 case 108280125: // range 2481 this.range = TypeConvertor.castToRange(value); // Range 2482 return value; 2483 default: return super.setProperty(hash, name, value); 2484 } 2485 2486 } 2487 2488 @Override 2489 public Base setProperty(String name, Base value) throws FHIRException { 2490 if (name.equals("description")) { 2491 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2492 } else if (name.equals("note")) { 2493 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2494 } else if (name.equals("event[x]")) { 2495 this.event = TypeConvertor.castToType(value); // DataType 2496 } else if (name.equals("quantity")) { 2497 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2498 } else if (name.equals("range")) { 2499 this.range = TypeConvertor.castToRange(value); // Range 2500 } else 2501 return super.setProperty(name, value); 2502 return value; 2503 } 2504 2505 @Override 2506 public void removeChild(String name, Base value) throws FHIRException { 2507 if (name.equals("description")) { 2508 this.description = null; 2509 } else if (name.equals("note")) { 2510 this.getNote().remove(value); 2511 } else if (name.equals("event[x]")) { 2512 this.event = null; 2513 } else if (name.equals("quantity")) { 2514 this.quantity = null; 2515 } else if (name.equals("range")) { 2516 this.range = null; 2517 } else 2518 super.removeChild(name, value); 2519 2520 } 2521 2522 @Override 2523 public Base makeProperty(int hash, String name) throws FHIRException { 2524 switch (hash) { 2525 case -1724546052: return getDescriptionElement(); 2526 case 3387378: return addNote(); 2527 case 278115238: return getEvent(); 2528 case 96891546: return getEvent(); 2529 case -1285004149: return getQuantity(); 2530 case 108280125: return getRange(); 2531 default: return super.makeProperty(hash, name); 2532 } 2533 2534 } 2535 2536 @Override 2537 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2538 switch (hash) { 2539 case -1724546052: /*description*/ return new String[] {"markdown"}; 2540 case 3387378: /*note*/ return new String[] {"Annotation"}; 2541 case 96891546: /*event*/ return new String[] {"CodeableConcept", "Reference", "dateTime", "id"}; 2542 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 2543 case 108280125: /*range*/ return new String[] {"Range"}; 2544 default: return super.getTypesForProperty(hash, name); 2545 } 2546 2547 } 2548 2549 @Override 2550 public Base addChild(String name) throws FHIRException { 2551 if (name.equals("description")) { 2552 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.characteristic.timeFromEvent.description"); 2553 } 2554 else if (name.equals("note")) { 2555 return addNote(); 2556 } 2557 else if (name.equals("eventCodeableConcept")) { 2558 this.event = new CodeableConcept(); 2559 return this.event; 2560 } 2561 else if (name.equals("eventReference")) { 2562 this.event = new Reference(); 2563 return this.event; 2564 } 2565 else if (name.equals("eventDateTime")) { 2566 this.event = new DateTimeType(); 2567 return this.event; 2568 } 2569 else if (name.equals("eventId")) { 2570 this.event = new IdType(); 2571 return this.event; 2572 } 2573 else if (name.equals("quantity")) { 2574 this.quantity = new Quantity(); 2575 return this.quantity; 2576 } 2577 else if (name.equals("range")) { 2578 this.range = new Range(); 2579 return this.range; 2580 } 2581 else 2582 return super.addChild(name); 2583 } 2584 2585 public EvidenceVariableCharacteristicTimeFromEventComponent copy() { 2586 EvidenceVariableCharacteristicTimeFromEventComponent dst = new EvidenceVariableCharacteristicTimeFromEventComponent(); 2587 copyValues(dst); 2588 return dst; 2589 } 2590 2591 public void copyValues(EvidenceVariableCharacteristicTimeFromEventComponent dst) { 2592 super.copyValues(dst); 2593 dst.description = description == null ? null : description.copy(); 2594 if (note != null) { 2595 dst.note = new ArrayList<Annotation>(); 2596 for (Annotation i : note) 2597 dst.note.add(i.copy()); 2598 }; 2599 dst.event = event == null ? null : event.copy(); 2600 dst.quantity = quantity == null ? null : quantity.copy(); 2601 dst.range = range == null ? null : range.copy(); 2602 } 2603 2604 @Override 2605 public boolean equalsDeep(Base other_) { 2606 if (!super.equalsDeep(other_)) 2607 return false; 2608 if (!(other_ instanceof EvidenceVariableCharacteristicTimeFromEventComponent)) 2609 return false; 2610 EvidenceVariableCharacteristicTimeFromEventComponent o = (EvidenceVariableCharacteristicTimeFromEventComponent) other_; 2611 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(event, o.event, true) 2612 && compareDeep(quantity, o.quantity, true) && compareDeep(range, o.range, true); 2613 } 2614 2615 @Override 2616 public boolean equalsShallow(Base other_) { 2617 if (!super.equalsShallow(other_)) 2618 return false; 2619 if (!(other_ instanceof EvidenceVariableCharacteristicTimeFromEventComponent)) 2620 return false; 2621 EvidenceVariableCharacteristicTimeFromEventComponent o = (EvidenceVariableCharacteristicTimeFromEventComponent) other_; 2622 return compareValues(description, o.description, true); 2623 } 2624 2625 public boolean isEmpty() { 2626 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, event 2627 , quantity, range); 2628 } 2629 2630 public String fhirType() { 2631 return "EvidenceVariable.characteristic.timeFromEvent"; 2632 2633 } 2634 2635 } 2636 2637 @Block() 2638 public static class EvidenceVariableCategoryComponent extends BackboneElement implements IBaseBackboneElement { 2639 /** 2640 * Description of the grouping. 2641 */ 2642 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2643 @Description(shortDefinition="Description of the grouping", formalDefinition="Description of the grouping." ) 2644 protected StringType name; 2645 2646 /** 2647 * Definition of the grouping. 2648 */ 2649 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, Range.class}, order=2, min=0, max=1, modifier=false, summary=false) 2650 @Description(shortDefinition="Definition of the grouping", formalDefinition="Definition of the grouping." ) 2651 protected DataType value; 2652 2653 private static final long serialVersionUID = 1839679495L; 2654 2655 /** 2656 * Constructor 2657 */ 2658 public EvidenceVariableCategoryComponent() { 2659 super(); 2660 } 2661 2662 /** 2663 * @return {@link #name} (Description of the grouping.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2664 */ 2665 public StringType getNameElement() { 2666 if (this.name == null) 2667 if (Configuration.errorOnAutoCreate()) 2668 throw new Error("Attempt to auto-create EvidenceVariableCategoryComponent.name"); 2669 else if (Configuration.doAutoCreate()) 2670 this.name = new StringType(); // bb 2671 return this.name; 2672 } 2673 2674 public boolean hasNameElement() { 2675 return this.name != null && !this.name.isEmpty(); 2676 } 2677 2678 public boolean hasName() { 2679 return this.name != null && !this.name.isEmpty(); 2680 } 2681 2682 /** 2683 * @param value {@link #name} (Description of the grouping.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2684 */ 2685 public EvidenceVariableCategoryComponent setNameElement(StringType value) { 2686 this.name = value; 2687 return this; 2688 } 2689 2690 /** 2691 * @return Description of the grouping. 2692 */ 2693 public String getName() { 2694 return this.name == null ? null : this.name.getValue(); 2695 } 2696 2697 /** 2698 * @param value Description of the grouping. 2699 */ 2700 public EvidenceVariableCategoryComponent setName(String value) { 2701 if (Utilities.noString(value)) 2702 this.name = null; 2703 else { 2704 if (this.name == null) 2705 this.name = new StringType(); 2706 this.name.setValue(value); 2707 } 2708 return this; 2709 } 2710 2711 /** 2712 * @return {@link #value} (Definition of the grouping.) 2713 */ 2714 public DataType getValue() { 2715 return this.value; 2716 } 2717 2718 /** 2719 * @return {@link #value} (Definition of the grouping.) 2720 */ 2721 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2722 if (this.value == null) 2723 this.value = new CodeableConcept(); 2724 if (!(this.value instanceof CodeableConcept)) 2725 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2726 return (CodeableConcept) this.value; 2727 } 2728 2729 public boolean hasValueCodeableConcept() { 2730 return this.value instanceof CodeableConcept; 2731 } 2732 2733 /** 2734 * @return {@link #value} (Definition of the grouping.) 2735 */ 2736 public Quantity getValueQuantity() throws FHIRException { 2737 if (this.value == null) 2738 this.value = new Quantity(); 2739 if (!(this.value instanceof Quantity)) 2740 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2741 return (Quantity) this.value; 2742 } 2743 2744 public boolean hasValueQuantity() { 2745 return this.value instanceof Quantity; 2746 } 2747 2748 /** 2749 * @return {@link #value} (Definition of the grouping.) 2750 */ 2751 public Range getValueRange() throws FHIRException { 2752 if (this.value == null) 2753 this.value = new Range(); 2754 if (!(this.value instanceof Range)) 2755 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2756 return (Range) this.value; 2757 } 2758 2759 public boolean hasValueRange() { 2760 return this.value instanceof Range; 2761 } 2762 2763 public boolean hasValue() { 2764 return this.value != null && !this.value.isEmpty(); 2765 } 2766 2767 /** 2768 * @param value {@link #value} (Definition of the grouping.) 2769 */ 2770 public EvidenceVariableCategoryComponent setValue(DataType value) { 2771 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range)) 2772 throw new FHIRException("Not the right type for EvidenceVariable.category.value[x]: "+value.fhirType()); 2773 this.value = value; 2774 return this; 2775 } 2776 2777 protected void listChildren(List<Property> children) { 2778 super.listChildren(children); 2779 children.add(new Property("name", "string", "Description of the grouping.", 0, 1, name)); 2780 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range", "Definition of the grouping.", 0, 1, value)); 2781 } 2782 2783 @Override 2784 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2785 switch (_hash) { 2786 case 3373707: /*name*/ return new Property("name", "string", "Description of the grouping.", 0, 1, name); 2787 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "Definition of the grouping.", 0, 1, value); 2788 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "Definition of the grouping.", 0, 1, value); 2789 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Definition of the grouping.", 0, 1, value); 2790 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Definition of the grouping.", 0, 1, value); 2791 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Definition of the grouping.", 0, 1, value); 2792 default: return super.getNamedProperty(_hash, _name, _checkValid); 2793 } 2794 2795 } 2796 2797 @Override 2798 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2799 switch (hash) { 2800 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2801 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2802 default: return super.getProperty(hash, name, checkValid); 2803 } 2804 2805 } 2806 2807 @Override 2808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2809 switch (hash) { 2810 case 3373707: // name 2811 this.name = TypeConvertor.castToString(value); // StringType 2812 return value; 2813 case 111972721: // value 2814 this.value = TypeConvertor.castToType(value); // DataType 2815 return value; 2816 default: return super.setProperty(hash, name, value); 2817 } 2818 2819 } 2820 2821 @Override 2822 public Base setProperty(String name, Base value) throws FHIRException { 2823 if (name.equals("name")) { 2824 this.name = TypeConvertor.castToString(value); // StringType 2825 } else if (name.equals("value[x]")) { 2826 this.value = TypeConvertor.castToType(value); // DataType 2827 } else 2828 return super.setProperty(name, value); 2829 return value; 2830 } 2831 2832 @Override 2833 public void removeChild(String name, Base value) throws FHIRException { 2834 if (name.equals("name")) { 2835 this.name = null; 2836 } else if (name.equals("value[x]")) { 2837 this.value = null; 2838 } else 2839 super.removeChild(name, value); 2840 2841 } 2842 2843 @Override 2844 public Base makeProperty(int hash, String name) throws FHIRException { 2845 switch (hash) { 2846 case 3373707: return getNameElement(); 2847 case -1410166417: return getValue(); 2848 case 111972721: return getValue(); 2849 default: return super.makeProperty(hash, name); 2850 } 2851 2852 } 2853 2854 @Override 2855 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2856 switch (hash) { 2857 case 3373707: /*name*/ return new String[] {"string"}; 2858 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "Range"}; 2859 default: return super.getTypesForProperty(hash, name); 2860 } 2861 2862 } 2863 2864 @Override 2865 public Base addChild(String name) throws FHIRException { 2866 if (name.equals("name")) { 2867 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.category.name"); 2868 } 2869 else if (name.equals("valueCodeableConcept")) { 2870 this.value = new CodeableConcept(); 2871 return this.value; 2872 } 2873 else if (name.equals("valueQuantity")) { 2874 this.value = new Quantity(); 2875 return this.value; 2876 } 2877 else if (name.equals("valueRange")) { 2878 this.value = new Range(); 2879 return this.value; 2880 } 2881 else 2882 return super.addChild(name); 2883 } 2884 2885 public EvidenceVariableCategoryComponent copy() { 2886 EvidenceVariableCategoryComponent dst = new EvidenceVariableCategoryComponent(); 2887 copyValues(dst); 2888 return dst; 2889 } 2890 2891 public void copyValues(EvidenceVariableCategoryComponent dst) { 2892 super.copyValues(dst); 2893 dst.name = name == null ? null : name.copy(); 2894 dst.value = value == null ? null : value.copy(); 2895 } 2896 2897 @Override 2898 public boolean equalsDeep(Base other_) { 2899 if (!super.equalsDeep(other_)) 2900 return false; 2901 if (!(other_ instanceof EvidenceVariableCategoryComponent)) 2902 return false; 2903 EvidenceVariableCategoryComponent o = (EvidenceVariableCategoryComponent) other_; 2904 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 2905 } 2906 2907 @Override 2908 public boolean equalsShallow(Base other_) { 2909 if (!super.equalsShallow(other_)) 2910 return false; 2911 if (!(other_ instanceof EvidenceVariableCategoryComponent)) 2912 return false; 2913 EvidenceVariableCategoryComponent o = (EvidenceVariableCategoryComponent) other_; 2914 return compareValues(name, o.name, true); 2915 } 2916 2917 public boolean isEmpty() { 2918 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 2919 } 2920 2921 public String fhirType() { 2922 return "EvidenceVariable.category"; 2923 2924 } 2925 2926 } 2927 2928 /** 2929 * An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers. 2930 */ 2931 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2932 @Description(shortDefinition="Canonical identifier for this evidence variable, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers." ) 2933 protected UriType url; 2934 2935 /** 2936 * A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance. 2937 */ 2938 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2939 @Description(shortDefinition="Additional identifier for the evidence variable", formalDefinition="A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2940 protected List<Identifier> identifier; 2941 2942 /** 2943 * The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 2944 */ 2945 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2946 @Description(shortDefinition="Business version of the evidence variable", formalDefinition="The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts." ) 2947 protected StringType version; 2948 2949 /** 2950 * Indicates the mechanism used to compare versions to determine which is more current. 2951 */ 2952 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2953 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 2954 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2955 protected DataType versionAlgorithm; 2956 2957 /** 2958 * A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2959 */ 2960 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2961 @Description(shortDefinition="Name for this evidence variable (computer friendly)", formalDefinition="A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2962 protected StringType name; 2963 2964 /** 2965 * A short, descriptive, user-friendly title for the evidence variable. 2966 */ 2967 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2968 @Description(shortDefinition="Name for this evidence variable (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the evidence variable." ) 2969 protected StringType title; 2970 2971 /** 2972 * The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary. 2973 */ 2974 @Child(name = "shortTitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2975 @Description(shortDefinition="Title for use in informal contexts", formalDefinition="The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary." ) 2976 protected StringType shortTitle; 2977 2978 /** 2979 * The status of this evidence variable. Enables tracking the life-cycle of the content. 2980 */ 2981 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 2982 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this evidence variable. Enables tracking the life-cycle of the content." ) 2983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2984 protected Enumeration<PublicationStatus> status; 2985 2986 /** 2987 * A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2988 */ 2989 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2990 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 2991 protected BooleanType experimental; 2992 2993 /** 2994 * The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes. 2995 */ 2996 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2997 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes." ) 2998 protected DateTimeType date; 2999 3000 /** 3001 * The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable. 3002 */ 3003 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3004 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable." ) 3005 protected StringType publisher; 3006 3007 /** 3008 * Contact details to assist a user in finding and communicating with the publisher. 3009 */ 3010 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3011 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 3012 protected List<ContactDetail> contact; 3013 3014 /** 3015 * A free text natural language description of the evidence variable from a consumer's perspective. 3016 */ 3017 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=true) 3018 @Description(shortDefinition="Natural language description of the evidence variable", formalDefinition="A free text natural language description of the evidence variable from a consumer's perspective." ) 3019 protected MarkdownType description; 3020 3021 /** 3022 * A human-readable string to clarify or explain concepts about the resource. 3023 */ 3024 @Child(name = "note", type = {Annotation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3025 @Description(shortDefinition="Used for footnotes or explanatory notes", formalDefinition="A human-readable string to clarify or explain concepts about the resource." ) 3026 protected List<Annotation> note; 3027 3028 /** 3029 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances. 3030 */ 3031 @Child(name = "useContext", type = {UsageContext.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3032 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances." ) 3033 protected List<UsageContext> useContext; 3034 3035 /** 3036 * Explanation of why this EvidenceVariable is needed and why it has been designed as it has. 3037 */ 3038 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 3039 @Description(shortDefinition="Why this EvidenceVariable is defined", formalDefinition="Explanation of why this EvidenceVariable is needed and why it has been designed as it has." ) 3040 protected MarkdownType purpose; 3041 3042 /** 3043 * A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource. 3044 */ 3045 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 3046 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource." ) 3047 protected MarkdownType copyright; 3048 3049 /** 3050 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3051 */ 3052 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 3053 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 3054 protected StringType copyrightLabel; 3055 3056 /** 3057 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3058 3059See guidance around (not) making local changes to elements [here](canonicalresource.html#localization). 3060 */ 3061 @Child(name = "approvalDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 3062 @Description(shortDefinition="When the resource was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.\n\nSee guidance around (not) making local changes to elements [here](canonicalresource.html#localization)." ) 3063 protected DateType approvalDate; 3064 3065 /** 3066 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3067 */ 3068 @Child(name = "lastReviewDate", type = {DateType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3069 @Description(shortDefinition="When the resource was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 3070 protected DateType lastReviewDate; 3071 3072 /** 3073 * The period during which the resource content was or is planned to be in active use. 3074 */ 3075 @Child(name = "effectivePeriod", type = {Period.class}, order=20, min=0, max=1, modifier=false, summary=false) 3076 @Description(shortDefinition="When the resource is expected to be used", formalDefinition="The period during which the resource content was or is planned to be in active use." ) 3077 protected Period effectivePeriod; 3078 3079 /** 3080 * An individiual or organization primarily involved in the creation and maintenance of the content. 3081 */ 3082 @Child(name = "author", type = {ContactDetail.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3083 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the content." ) 3084 protected List<ContactDetail> author; 3085 3086 /** 3087 * An individual or organization primarily responsible for internal coherence of the content. 3088 */ 3089 @Child(name = "editor", type = {ContactDetail.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3090 @Description(shortDefinition="Who edited the content", formalDefinition="An individual or organization primarily responsible for internal coherence of the content." ) 3091 protected List<ContactDetail> editor; 3092 3093 /** 3094 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content. 3095 */ 3096 @Child(name = "reviewer", type = {ContactDetail.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3097 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content." ) 3098 protected List<ContactDetail> reviewer; 3099 3100 /** 3101 * An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting. 3102 */ 3103 @Child(name = "endorser", type = {ContactDetail.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3104 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting." ) 3105 protected List<ContactDetail> endorser; 3106 3107 /** 3108 * Related artifacts such as additional documentation, justification, or bibliographic references. 3109 */ 3110 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3111 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 3112 protected List<RelatedArtifact> relatedArtifact; 3113 3114 /** 3115 * True if the actual variable measured, false if a conceptual representation of the intended variable. 3116 */ 3117 @Child(name = "actual", type = {BooleanType.class}, order=26, min=0, max=1, modifier=false, summary=false) 3118 @Description(shortDefinition="Actual or conceptual", formalDefinition="True if the actual variable measured, false if a conceptual representation of the intended variable." ) 3119 protected BooleanType actual; 3120 3121 /** 3122 * A defining factor of the EvidenceVariable. Multiple characteristics are applied with "and" semantics. 3123 */ 3124 @Child(name = "characteristic", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3125 @Description(shortDefinition="A defining factor of the EvidenceVariable", formalDefinition="A defining factor of the EvidenceVariable. Multiple characteristics are applied with \"and\" semantics." ) 3126 protected List<EvidenceVariableCharacteristicComponent> characteristic; 3127 3128 /** 3129 * The method of handling in statistical analysis. 3130 */ 3131 @Child(name = "handling", type = {CodeType.class}, order=28, min=0, max=1, modifier=false, summary=false) 3132 @Description(shortDefinition="continuous | dichotomous | ordinal | polychotomous", formalDefinition="The method of handling in statistical analysis." ) 3133 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/variable-handling") 3134 protected Enumeration<EvidenceVariableHandling> handling; 3135 3136 /** 3137 * A grouping for ordinal or polychotomous variables. 3138 */ 3139 @Child(name = "category", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3140 @Description(shortDefinition="A grouping for ordinal or polychotomous variables", formalDefinition="A grouping for ordinal or polychotomous variables." ) 3141 protected List<EvidenceVariableCategoryComponent> category; 3142 3143 private static final long serialVersionUID = -1626354085L; 3144 3145 /** 3146 * Constructor 3147 */ 3148 public EvidenceVariable() { 3149 super(); 3150 } 3151 3152 /** 3153 * Constructor 3154 */ 3155 public EvidenceVariable(PublicationStatus status) { 3156 super(); 3157 this.setStatus(status); 3158 } 3159 3160 /** 3161 * @return {@link #url} (An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3162 */ 3163 public UriType getUrlElement() { 3164 if (this.url == null) 3165 if (Configuration.errorOnAutoCreate()) 3166 throw new Error("Attempt to auto-create EvidenceVariable.url"); 3167 else if (Configuration.doAutoCreate()) 3168 this.url = new UriType(); // bb 3169 return this.url; 3170 } 3171 3172 public boolean hasUrlElement() { 3173 return this.url != null && !this.url.isEmpty(); 3174 } 3175 3176 public boolean hasUrl() { 3177 return this.url != null && !this.url.isEmpty(); 3178 } 3179 3180 /** 3181 * @param value {@link #url} (An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3182 */ 3183 public EvidenceVariable setUrlElement(UriType value) { 3184 this.url = value; 3185 return this; 3186 } 3187 3188 /** 3189 * @return An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers. 3190 */ 3191 public String getUrl() { 3192 return this.url == null ? null : this.url.getValue(); 3193 } 3194 3195 /** 3196 * @param value An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers. 3197 */ 3198 public EvidenceVariable setUrl(String value) { 3199 if (Utilities.noString(value)) 3200 this.url = null; 3201 else { 3202 if (this.url == null) 3203 this.url = new UriType(); 3204 this.url.setValue(value); 3205 } 3206 return this; 3207 } 3208 3209 /** 3210 * @return {@link #identifier} (A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.) 3211 */ 3212 public List<Identifier> getIdentifier() { 3213 if (this.identifier == null) 3214 this.identifier = new ArrayList<Identifier>(); 3215 return this.identifier; 3216 } 3217 3218 /** 3219 * @return Returns a reference to <code>this</code> for easy method chaining 3220 */ 3221 public EvidenceVariable setIdentifier(List<Identifier> theIdentifier) { 3222 this.identifier = theIdentifier; 3223 return this; 3224 } 3225 3226 public boolean hasIdentifier() { 3227 if (this.identifier == null) 3228 return false; 3229 for (Identifier item : this.identifier) 3230 if (!item.isEmpty()) 3231 return true; 3232 return false; 3233 } 3234 3235 public Identifier addIdentifier() { //3 3236 Identifier t = new Identifier(); 3237 if (this.identifier == null) 3238 this.identifier = new ArrayList<Identifier>(); 3239 this.identifier.add(t); 3240 return t; 3241 } 3242 3243 public EvidenceVariable addIdentifier(Identifier t) { //3 3244 if (t == null) 3245 return this; 3246 if (this.identifier == null) 3247 this.identifier = new ArrayList<Identifier>(); 3248 this.identifier.add(t); 3249 return this; 3250 } 3251 3252 /** 3253 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3254 */ 3255 public Identifier getIdentifierFirstRep() { 3256 if (getIdentifier().isEmpty()) { 3257 addIdentifier(); 3258 } 3259 return getIdentifier().get(0); 3260 } 3261 3262 /** 3263 * @return {@link #version} (The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3264 */ 3265 public StringType getVersionElement() { 3266 if (this.version == null) 3267 if (Configuration.errorOnAutoCreate()) 3268 throw new Error("Attempt to auto-create EvidenceVariable.version"); 3269 else if (Configuration.doAutoCreate()) 3270 this.version = new StringType(); // bb 3271 return this.version; 3272 } 3273 3274 public boolean hasVersionElement() { 3275 return this.version != null && !this.version.isEmpty(); 3276 } 3277 3278 public boolean hasVersion() { 3279 return this.version != null && !this.version.isEmpty(); 3280 } 3281 3282 /** 3283 * @param value {@link #version} (The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3284 */ 3285 public EvidenceVariable setVersionElement(StringType value) { 3286 this.version = value; 3287 return this; 3288 } 3289 3290 /** 3291 * @return The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 3292 */ 3293 public String getVersion() { 3294 return this.version == null ? null : this.version.getValue(); 3295 } 3296 3297 /** 3298 * @param value The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 3299 */ 3300 public EvidenceVariable setVersion(String value) { 3301 if (Utilities.noString(value)) 3302 this.version = null; 3303 else { 3304 if (this.version == null) 3305 this.version = new StringType(); 3306 this.version.setValue(value); 3307 } 3308 return this; 3309 } 3310 3311 /** 3312 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3313 */ 3314 public DataType getVersionAlgorithm() { 3315 return this.versionAlgorithm; 3316 } 3317 3318 /** 3319 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3320 */ 3321 public StringType getVersionAlgorithmStringType() throws FHIRException { 3322 if (this.versionAlgorithm == null) 3323 this.versionAlgorithm = new StringType(); 3324 if (!(this.versionAlgorithm instanceof StringType)) 3325 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3326 return (StringType) this.versionAlgorithm; 3327 } 3328 3329 public boolean hasVersionAlgorithmStringType() { 3330 return this.versionAlgorithm instanceof StringType; 3331 } 3332 3333 /** 3334 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3335 */ 3336 public Coding getVersionAlgorithmCoding() throws FHIRException { 3337 if (this.versionAlgorithm == null) 3338 this.versionAlgorithm = new Coding(); 3339 if (!(this.versionAlgorithm instanceof Coding)) 3340 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3341 return (Coding) this.versionAlgorithm; 3342 } 3343 3344 public boolean hasVersionAlgorithmCoding() { 3345 return this.versionAlgorithm instanceof Coding; 3346 } 3347 3348 public boolean hasVersionAlgorithm() { 3349 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 3350 } 3351 3352 /** 3353 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3354 */ 3355 public EvidenceVariable setVersionAlgorithm(DataType value) { 3356 if (value != null && !(value instanceof StringType || value instanceof Coding)) 3357 throw new FHIRException("Not the right type for EvidenceVariable.versionAlgorithm[x]: "+value.fhirType()); 3358 this.versionAlgorithm = value; 3359 return this; 3360 } 3361 3362 /** 3363 * @return {@link #name} (A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3364 */ 3365 public StringType getNameElement() { 3366 if (this.name == null) 3367 if (Configuration.errorOnAutoCreate()) 3368 throw new Error("Attempt to auto-create EvidenceVariable.name"); 3369 else if (Configuration.doAutoCreate()) 3370 this.name = new StringType(); // bb 3371 return this.name; 3372 } 3373 3374 public boolean hasNameElement() { 3375 return this.name != null && !this.name.isEmpty(); 3376 } 3377 3378 public boolean hasName() { 3379 return this.name != null && !this.name.isEmpty(); 3380 } 3381 3382 /** 3383 * @param value {@link #name} (A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3384 */ 3385 public EvidenceVariable setNameElement(StringType value) { 3386 this.name = value; 3387 return this; 3388 } 3389 3390 /** 3391 * @return A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3392 */ 3393 public String getName() { 3394 return this.name == null ? null : this.name.getValue(); 3395 } 3396 3397 /** 3398 * @param value A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3399 */ 3400 public EvidenceVariable setName(String value) { 3401 if (Utilities.noString(value)) 3402 this.name = null; 3403 else { 3404 if (this.name == null) 3405 this.name = new StringType(); 3406 this.name.setValue(value); 3407 } 3408 return this; 3409 } 3410 3411 /** 3412 * @return {@link #title} (A short, descriptive, user-friendly title for the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3413 */ 3414 public StringType getTitleElement() { 3415 if (this.title == null) 3416 if (Configuration.errorOnAutoCreate()) 3417 throw new Error("Attempt to auto-create EvidenceVariable.title"); 3418 else if (Configuration.doAutoCreate()) 3419 this.title = new StringType(); // bb 3420 return this.title; 3421 } 3422 3423 public boolean hasTitleElement() { 3424 return this.title != null && !this.title.isEmpty(); 3425 } 3426 3427 public boolean hasTitle() { 3428 return this.title != null && !this.title.isEmpty(); 3429 } 3430 3431 /** 3432 * @param value {@link #title} (A short, descriptive, user-friendly title for the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3433 */ 3434 public EvidenceVariable setTitleElement(StringType value) { 3435 this.title = value; 3436 return this; 3437 } 3438 3439 /** 3440 * @return A short, descriptive, user-friendly title for the evidence variable. 3441 */ 3442 public String getTitle() { 3443 return this.title == null ? null : this.title.getValue(); 3444 } 3445 3446 /** 3447 * @param value A short, descriptive, user-friendly title for the evidence variable. 3448 */ 3449 public EvidenceVariable setTitle(String value) { 3450 if (Utilities.noString(value)) 3451 this.title = null; 3452 else { 3453 if (this.title == null) 3454 this.title = new StringType(); 3455 this.title.setValue(value); 3456 } 3457 return this; 3458 } 3459 3460 /** 3461 * @return {@link #shortTitle} (The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.). This is the underlying object with id, value and extensions. The accessor "getShortTitle" gives direct access to the value 3462 */ 3463 public StringType getShortTitleElement() { 3464 if (this.shortTitle == null) 3465 if (Configuration.errorOnAutoCreate()) 3466 throw new Error("Attempt to auto-create EvidenceVariable.shortTitle"); 3467 else if (Configuration.doAutoCreate()) 3468 this.shortTitle = new StringType(); // bb 3469 return this.shortTitle; 3470 } 3471 3472 public boolean hasShortTitleElement() { 3473 return this.shortTitle != null && !this.shortTitle.isEmpty(); 3474 } 3475 3476 public boolean hasShortTitle() { 3477 return this.shortTitle != null && !this.shortTitle.isEmpty(); 3478 } 3479 3480 /** 3481 * @param value {@link #shortTitle} (The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.). This is the underlying object with id, value and extensions. The accessor "getShortTitle" gives direct access to the value 3482 */ 3483 public EvidenceVariable setShortTitleElement(StringType value) { 3484 this.shortTitle = value; 3485 return this; 3486 } 3487 3488 /** 3489 * @return The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary. 3490 */ 3491 public String getShortTitle() { 3492 return this.shortTitle == null ? null : this.shortTitle.getValue(); 3493 } 3494 3495 /** 3496 * @param value The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary. 3497 */ 3498 public EvidenceVariable setShortTitle(String value) { 3499 if (Utilities.noString(value)) 3500 this.shortTitle = null; 3501 else { 3502 if (this.shortTitle == null) 3503 this.shortTitle = new StringType(); 3504 this.shortTitle.setValue(value); 3505 } 3506 return this; 3507 } 3508 3509 /** 3510 * @return {@link #status} (The status of this evidence variable. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3511 */ 3512 public Enumeration<PublicationStatus> getStatusElement() { 3513 if (this.status == null) 3514 if (Configuration.errorOnAutoCreate()) 3515 throw new Error("Attempt to auto-create EvidenceVariable.status"); 3516 else if (Configuration.doAutoCreate()) 3517 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3518 return this.status; 3519 } 3520 3521 public boolean hasStatusElement() { 3522 return this.status != null && !this.status.isEmpty(); 3523 } 3524 3525 public boolean hasStatus() { 3526 return this.status != null && !this.status.isEmpty(); 3527 } 3528 3529 /** 3530 * @param value {@link #status} (The status of this evidence variable. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3531 */ 3532 public EvidenceVariable setStatusElement(Enumeration<PublicationStatus> value) { 3533 this.status = value; 3534 return this; 3535 } 3536 3537 /** 3538 * @return The status of this evidence variable. Enables tracking the life-cycle of the content. 3539 */ 3540 public PublicationStatus getStatus() { 3541 return this.status == null ? null : this.status.getValue(); 3542 } 3543 3544 /** 3545 * @param value The status of this evidence variable. Enables tracking the life-cycle of the content. 3546 */ 3547 public EvidenceVariable setStatus(PublicationStatus value) { 3548 if (this.status == null) 3549 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3550 this.status.setValue(value); 3551 return this; 3552 } 3553 3554 /** 3555 * @return {@link #experimental} (A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3556 */ 3557 public BooleanType getExperimentalElement() { 3558 if (this.experimental == null) 3559 if (Configuration.errorOnAutoCreate()) 3560 throw new Error("Attempt to auto-create EvidenceVariable.experimental"); 3561 else if (Configuration.doAutoCreate()) 3562 this.experimental = new BooleanType(); // bb 3563 return this.experimental; 3564 } 3565 3566 public boolean hasExperimentalElement() { 3567 return this.experimental != null && !this.experimental.isEmpty(); 3568 } 3569 3570 public boolean hasExperimental() { 3571 return this.experimental != null && !this.experimental.isEmpty(); 3572 } 3573 3574 /** 3575 * @param value {@link #experimental} (A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3576 */ 3577 public EvidenceVariable setExperimentalElement(BooleanType value) { 3578 this.experimental = value; 3579 return this; 3580 } 3581 3582 /** 3583 * @return A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3584 */ 3585 public boolean getExperimental() { 3586 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3587 } 3588 3589 /** 3590 * @param value A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3591 */ 3592 public EvidenceVariable setExperimental(boolean value) { 3593 if (this.experimental == null) 3594 this.experimental = new BooleanType(); 3595 this.experimental.setValue(value); 3596 return this; 3597 } 3598 3599 /** 3600 * @return {@link #date} (The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3601 */ 3602 public DateTimeType getDateElement() { 3603 if (this.date == null) 3604 if (Configuration.errorOnAutoCreate()) 3605 throw new Error("Attempt to auto-create EvidenceVariable.date"); 3606 else if (Configuration.doAutoCreate()) 3607 this.date = new DateTimeType(); // bb 3608 return this.date; 3609 } 3610 3611 public boolean hasDateElement() { 3612 return this.date != null && !this.date.isEmpty(); 3613 } 3614 3615 public boolean hasDate() { 3616 return this.date != null && !this.date.isEmpty(); 3617 } 3618 3619 /** 3620 * @param value {@link #date} (The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3621 */ 3622 public EvidenceVariable setDateElement(DateTimeType value) { 3623 this.date = value; 3624 return this; 3625 } 3626 3627 /** 3628 * @return The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes. 3629 */ 3630 public Date getDate() { 3631 return this.date == null ? null : this.date.getValue(); 3632 } 3633 3634 /** 3635 * @param value The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes. 3636 */ 3637 public EvidenceVariable setDate(Date value) { 3638 if (value == null) 3639 this.date = null; 3640 else { 3641 if (this.date == null) 3642 this.date = new DateTimeType(); 3643 this.date.setValue(value); 3644 } 3645 return this; 3646 } 3647 3648 /** 3649 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3650 */ 3651 public StringType getPublisherElement() { 3652 if (this.publisher == null) 3653 if (Configuration.errorOnAutoCreate()) 3654 throw new Error("Attempt to auto-create EvidenceVariable.publisher"); 3655 else if (Configuration.doAutoCreate()) 3656 this.publisher = new StringType(); // bb 3657 return this.publisher; 3658 } 3659 3660 public boolean hasPublisherElement() { 3661 return this.publisher != null && !this.publisher.isEmpty(); 3662 } 3663 3664 public boolean hasPublisher() { 3665 return this.publisher != null && !this.publisher.isEmpty(); 3666 } 3667 3668 /** 3669 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3670 */ 3671 public EvidenceVariable setPublisherElement(StringType value) { 3672 this.publisher = value; 3673 return this; 3674 } 3675 3676 /** 3677 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable. 3678 */ 3679 public String getPublisher() { 3680 return this.publisher == null ? null : this.publisher.getValue(); 3681 } 3682 3683 /** 3684 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable. 3685 */ 3686 public EvidenceVariable setPublisher(String value) { 3687 if (Utilities.noString(value)) 3688 this.publisher = null; 3689 else { 3690 if (this.publisher == null) 3691 this.publisher = new StringType(); 3692 this.publisher.setValue(value); 3693 } 3694 return this; 3695 } 3696 3697 /** 3698 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3699 */ 3700 public List<ContactDetail> getContact() { 3701 if (this.contact == null) 3702 this.contact = new ArrayList<ContactDetail>(); 3703 return this.contact; 3704 } 3705 3706 /** 3707 * @return Returns a reference to <code>this</code> for easy method chaining 3708 */ 3709 public EvidenceVariable setContact(List<ContactDetail> theContact) { 3710 this.contact = theContact; 3711 return this; 3712 } 3713 3714 public boolean hasContact() { 3715 if (this.contact == null) 3716 return false; 3717 for (ContactDetail item : this.contact) 3718 if (!item.isEmpty()) 3719 return true; 3720 return false; 3721 } 3722 3723 public ContactDetail addContact() { //3 3724 ContactDetail t = new ContactDetail(); 3725 if (this.contact == null) 3726 this.contact = new ArrayList<ContactDetail>(); 3727 this.contact.add(t); 3728 return t; 3729 } 3730 3731 public EvidenceVariable addContact(ContactDetail t) { //3 3732 if (t == null) 3733 return this; 3734 if (this.contact == null) 3735 this.contact = new ArrayList<ContactDetail>(); 3736 this.contact.add(t); 3737 return this; 3738 } 3739 3740 /** 3741 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3742 */ 3743 public ContactDetail getContactFirstRep() { 3744 if (getContact().isEmpty()) { 3745 addContact(); 3746 } 3747 return getContact().get(0); 3748 } 3749 3750 /** 3751 * @return {@link #description} (A free text natural language description of the evidence variable from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3752 */ 3753 public MarkdownType getDescriptionElement() { 3754 if (this.description == null) 3755 if (Configuration.errorOnAutoCreate()) 3756 throw new Error("Attempt to auto-create EvidenceVariable.description"); 3757 else if (Configuration.doAutoCreate()) 3758 this.description = new MarkdownType(); // bb 3759 return this.description; 3760 } 3761 3762 public boolean hasDescriptionElement() { 3763 return this.description != null && !this.description.isEmpty(); 3764 } 3765 3766 public boolean hasDescription() { 3767 return this.description != null && !this.description.isEmpty(); 3768 } 3769 3770 /** 3771 * @param value {@link #description} (A free text natural language description of the evidence variable from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3772 */ 3773 public EvidenceVariable setDescriptionElement(MarkdownType value) { 3774 this.description = value; 3775 return this; 3776 } 3777 3778 /** 3779 * @return A free text natural language description of the evidence variable from a consumer's perspective. 3780 */ 3781 public String getDescription() { 3782 return this.description == null ? null : this.description.getValue(); 3783 } 3784 3785 /** 3786 * @param value A free text natural language description of the evidence variable from a consumer's perspective. 3787 */ 3788 public EvidenceVariable setDescription(String value) { 3789 if (Utilities.noString(value)) 3790 this.description = null; 3791 else { 3792 if (this.description == null) 3793 this.description = new MarkdownType(); 3794 this.description.setValue(value); 3795 } 3796 return this; 3797 } 3798 3799 /** 3800 * @return {@link #note} (A human-readable string to clarify or explain concepts about the resource.) 3801 */ 3802 public List<Annotation> getNote() { 3803 if (this.note == null) 3804 this.note = new ArrayList<Annotation>(); 3805 return this.note; 3806 } 3807 3808 /** 3809 * @return Returns a reference to <code>this</code> for easy method chaining 3810 */ 3811 public EvidenceVariable setNote(List<Annotation> theNote) { 3812 this.note = theNote; 3813 return this; 3814 } 3815 3816 public boolean hasNote() { 3817 if (this.note == null) 3818 return false; 3819 for (Annotation item : this.note) 3820 if (!item.isEmpty()) 3821 return true; 3822 return false; 3823 } 3824 3825 public Annotation addNote() { //3 3826 Annotation t = new Annotation(); 3827 if (this.note == null) 3828 this.note = new ArrayList<Annotation>(); 3829 this.note.add(t); 3830 return t; 3831 } 3832 3833 public EvidenceVariable addNote(Annotation t) { //3 3834 if (t == null) 3835 return this; 3836 if (this.note == null) 3837 this.note = new ArrayList<Annotation>(); 3838 this.note.add(t); 3839 return this; 3840 } 3841 3842 /** 3843 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3844 */ 3845 public Annotation getNoteFirstRep() { 3846 if (getNote().isEmpty()) { 3847 addNote(); 3848 } 3849 return getNote().get(0); 3850 } 3851 3852 /** 3853 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.) 3854 */ 3855 public List<UsageContext> getUseContext() { 3856 if (this.useContext == null) 3857 this.useContext = new ArrayList<UsageContext>(); 3858 return this.useContext; 3859 } 3860 3861 /** 3862 * @return Returns a reference to <code>this</code> for easy method chaining 3863 */ 3864 public EvidenceVariable setUseContext(List<UsageContext> theUseContext) { 3865 this.useContext = theUseContext; 3866 return this; 3867 } 3868 3869 public boolean hasUseContext() { 3870 if (this.useContext == null) 3871 return false; 3872 for (UsageContext item : this.useContext) 3873 if (!item.isEmpty()) 3874 return true; 3875 return false; 3876 } 3877 3878 public UsageContext addUseContext() { //3 3879 UsageContext t = new UsageContext(); 3880 if (this.useContext == null) 3881 this.useContext = new ArrayList<UsageContext>(); 3882 this.useContext.add(t); 3883 return t; 3884 } 3885 3886 public EvidenceVariable addUseContext(UsageContext t) { //3 3887 if (t == null) 3888 return this; 3889 if (this.useContext == null) 3890 this.useContext = new ArrayList<UsageContext>(); 3891 this.useContext.add(t); 3892 return this; 3893 } 3894 3895 /** 3896 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3897 */ 3898 public UsageContext getUseContextFirstRep() { 3899 if (getUseContext().isEmpty()) { 3900 addUseContext(); 3901 } 3902 return getUseContext().get(0); 3903 } 3904 3905 /** 3906 * @return {@link #purpose} (Explanation of why this EvidenceVariable is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3907 */ 3908 public MarkdownType getPurposeElement() { 3909 if (this.purpose == null) 3910 if (Configuration.errorOnAutoCreate()) 3911 throw new Error("Attempt to auto-create EvidenceVariable.purpose"); 3912 else if (Configuration.doAutoCreate()) 3913 this.purpose = new MarkdownType(); // bb 3914 return this.purpose; 3915 } 3916 3917 public boolean hasPurposeElement() { 3918 return this.purpose != null && !this.purpose.isEmpty(); 3919 } 3920 3921 public boolean hasPurpose() { 3922 return this.purpose != null && !this.purpose.isEmpty(); 3923 } 3924 3925 /** 3926 * @param value {@link #purpose} (Explanation of why this EvidenceVariable is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3927 */ 3928 public EvidenceVariable setPurposeElement(MarkdownType value) { 3929 this.purpose = value; 3930 return this; 3931 } 3932 3933 /** 3934 * @return Explanation of why this EvidenceVariable is needed and why it has been designed as it has. 3935 */ 3936 public String getPurpose() { 3937 return this.purpose == null ? null : this.purpose.getValue(); 3938 } 3939 3940 /** 3941 * @param value Explanation of why this EvidenceVariable is needed and why it has been designed as it has. 3942 */ 3943 public EvidenceVariable setPurpose(String value) { 3944 if (Utilities.noString(value)) 3945 this.purpose = null; 3946 else { 3947 if (this.purpose == null) 3948 this.purpose = new MarkdownType(); 3949 this.purpose.setValue(value); 3950 } 3951 return this; 3952 } 3953 3954 /** 3955 * @return {@link #copyright} (A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3956 */ 3957 public MarkdownType getCopyrightElement() { 3958 if (this.copyright == null) 3959 if (Configuration.errorOnAutoCreate()) 3960 throw new Error("Attempt to auto-create EvidenceVariable.copyright"); 3961 else if (Configuration.doAutoCreate()) 3962 this.copyright = new MarkdownType(); // bb 3963 return this.copyright; 3964 } 3965 3966 public boolean hasCopyrightElement() { 3967 return this.copyright != null && !this.copyright.isEmpty(); 3968 } 3969 3970 public boolean hasCopyright() { 3971 return this.copyright != null && !this.copyright.isEmpty(); 3972 } 3973 3974 /** 3975 * @param value {@link #copyright} (A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3976 */ 3977 public EvidenceVariable setCopyrightElement(MarkdownType value) { 3978 this.copyright = value; 3979 return this; 3980 } 3981 3982 /** 3983 * @return A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource. 3984 */ 3985 public String getCopyright() { 3986 return this.copyright == null ? null : this.copyright.getValue(); 3987 } 3988 3989 /** 3990 * @param value A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource. 3991 */ 3992 public EvidenceVariable setCopyright(String value) { 3993 if (Utilities.noString(value)) 3994 this.copyright = null; 3995 else { 3996 if (this.copyright == null) 3997 this.copyright = new MarkdownType(); 3998 this.copyright.setValue(value); 3999 } 4000 return this; 4001 } 4002 4003 /** 4004 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4005 */ 4006 public StringType getCopyrightLabelElement() { 4007 if (this.copyrightLabel == null) 4008 if (Configuration.errorOnAutoCreate()) 4009 throw new Error("Attempt to auto-create EvidenceVariable.copyrightLabel"); 4010 else if (Configuration.doAutoCreate()) 4011 this.copyrightLabel = new StringType(); // bb 4012 return this.copyrightLabel; 4013 } 4014 4015 public boolean hasCopyrightLabelElement() { 4016 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4017 } 4018 4019 public boolean hasCopyrightLabel() { 4020 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4021 } 4022 4023 /** 4024 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4025 */ 4026 public EvidenceVariable setCopyrightLabelElement(StringType value) { 4027 this.copyrightLabel = value; 4028 return this; 4029 } 4030 4031 /** 4032 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4033 */ 4034 public String getCopyrightLabel() { 4035 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 4036 } 4037 4038 /** 4039 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4040 */ 4041 public EvidenceVariable setCopyrightLabel(String value) { 4042 if (Utilities.noString(value)) 4043 this.copyrightLabel = null; 4044 else { 4045 if (this.copyrightLabel == null) 4046 this.copyrightLabel = new StringType(); 4047 this.copyrightLabel.setValue(value); 4048 } 4049 return this; 4050 } 4051 4052 /** 4053 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4054 4055See guidance around (not) making local changes to elements [here](canonicalresource.html#localization).). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4056 */ 4057 public DateType getApprovalDateElement() { 4058 if (this.approvalDate == null) 4059 if (Configuration.errorOnAutoCreate()) 4060 throw new Error("Attempt to auto-create EvidenceVariable.approvalDate"); 4061 else if (Configuration.doAutoCreate()) 4062 this.approvalDate = new DateType(); // bb 4063 return this.approvalDate; 4064 } 4065 4066 public boolean hasApprovalDateElement() { 4067 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4068 } 4069 4070 public boolean hasApprovalDate() { 4071 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4072 } 4073 4074 /** 4075 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4076 4077See guidance around (not) making local changes to elements [here](canonicalresource.html#localization).). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4078 */ 4079 public EvidenceVariable setApprovalDateElement(DateType value) { 4080 this.approvalDate = value; 4081 return this; 4082 } 4083 4084 /** 4085 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4086 4087See guidance around (not) making local changes to elements [here](canonicalresource.html#localization). 4088 */ 4089 public Date getApprovalDate() { 4090 return this.approvalDate == null ? null : this.approvalDate.getValue(); 4091 } 4092 4093 /** 4094 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4095 4096See guidance around (not) making local changes to elements [here](canonicalresource.html#localization). 4097 */ 4098 public EvidenceVariable setApprovalDate(Date value) { 4099 if (value == null) 4100 this.approvalDate = null; 4101 else { 4102 if (this.approvalDate == null) 4103 this.approvalDate = new DateType(); 4104 this.approvalDate.setValue(value); 4105 } 4106 return this; 4107 } 4108 4109 /** 4110 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4111 */ 4112 public DateType getLastReviewDateElement() { 4113 if (this.lastReviewDate == null) 4114 if (Configuration.errorOnAutoCreate()) 4115 throw new Error("Attempt to auto-create EvidenceVariable.lastReviewDate"); 4116 else if (Configuration.doAutoCreate()) 4117 this.lastReviewDate = new DateType(); // bb 4118 return this.lastReviewDate; 4119 } 4120 4121 public boolean hasLastReviewDateElement() { 4122 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4123 } 4124 4125 public boolean hasLastReviewDate() { 4126 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4127 } 4128 4129 /** 4130 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4131 */ 4132 public EvidenceVariable setLastReviewDateElement(DateType value) { 4133 this.lastReviewDate = value; 4134 return this; 4135 } 4136 4137 /** 4138 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4139 */ 4140 public Date getLastReviewDate() { 4141 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 4142 } 4143 4144 /** 4145 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4146 */ 4147 public EvidenceVariable setLastReviewDate(Date value) { 4148 if (value == null) 4149 this.lastReviewDate = null; 4150 else { 4151 if (this.lastReviewDate == null) 4152 this.lastReviewDate = new DateType(); 4153 this.lastReviewDate.setValue(value); 4154 } 4155 return this; 4156 } 4157 4158 /** 4159 * @return {@link #effectivePeriod} (The period during which the resource content was or is planned to be in active use.) 4160 */ 4161 public Period getEffectivePeriod() { 4162 if (this.effectivePeriod == null) 4163 if (Configuration.errorOnAutoCreate()) 4164 throw new Error("Attempt to auto-create EvidenceVariable.effectivePeriod"); 4165 else if (Configuration.doAutoCreate()) 4166 this.effectivePeriod = new Period(); // cc 4167 return this.effectivePeriod; 4168 } 4169 4170 public boolean hasEffectivePeriod() { 4171 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 4172 } 4173 4174 /** 4175 * @param value {@link #effectivePeriod} (The period during which the resource content was or is planned to be in active use.) 4176 */ 4177 public EvidenceVariable setEffectivePeriod(Period value) { 4178 this.effectivePeriod = value; 4179 return this; 4180 } 4181 4182 /** 4183 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the content.) 4184 */ 4185 public List<ContactDetail> getAuthor() { 4186 if (this.author == null) 4187 this.author = new ArrayList<ContactDetail>(); 4188 return this.author; 4189 } 4190 4191 /** 4192 * @return Returns a reference to <code>this</code> for easy method chaining 4193 */ 4194 public EvidenceVariable setAuthor(List<ContactDetail> theAuthor) { 4195 this.author = theAuthor; 4196 return this; 4197 } 4198 4199 public boolean hasAuthor() { 4200 if (this.author == null) 4201 return false; 4202 for (ContactDetail item : this.author) 4203 if (!item.isEmpty()) 4204 return true; 4205 return false; 4206 } 4207 4208 public ContactDetail addAuthor() { //3 4209 ContactDetail t = new ContactDetail(); 4210 if (this.author == null) 4211 this.author = new ArrayList<ContactDetail>(); 4212 this.author.add(t); 4213 return t; 4214 } 4215 4216 public EvidenceVariable addAuthor(ContactDetail t) { //3 4217 if (t == null) 4218 return this; 4219 if (this.author == null) 4220 this.author = new ArrayList<ContactDetail>(); 4221 this.author.add(t); 4222 return this; 4223 } 4224 4225 /** 4226 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 4227 */ 4228 public ContactDetail getAuthorFirstRep() { 4229 if (getAuthor().isEmpty()) { 4230 addAuthor(); 4231 } 4232 return getAuthor().get(0); 4233 } 4234 4235 /** 4236 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the content.) 4237 */ 4238 public List<ContactDetail> getEditor() { 4239 if (this.editor == null) 4240 this.editor = new ArrayList<ContactDetail>(); 4241 return this.editor; 4242 } 4243 4244 /** 4245 * @return Returns a reference to <code>this</code> for easy method chaining 4246 */ 4247 public EvidenceVariable setEditor(List<ContactDetail> theEditor) { 4248 this.editor = theEditor; 4249 return this; 4250 } 4251 4252 public boolean hasEditor() { 4253 if (this.editor == null) 4254 return false; 4255 for (ContactDetail item : this.editor) 4256 if (!item.isEmpty()) 4257 return true; 4258 return false; 4259 } 4260 4261 public ContactDetail addEditor() { //3 4262 ContactDetail t = new ContactDetail(); 4263 if (this.editor == null) 4264 this.editor = new ArrayList<ContactDetail>(); 4265 this.editor.add(t); 4266 return t; 4267 } 4268 4269 public EvidenceVariable addEditor(ContactDetail t) { //3 4270 if (t == null) 4271 return this; 4272 if (this.editor == null) 4273 this.editor = new ArrayList<ContactDetail>(); 4274 this.editor.add(t); 4275 return this; 4276 } 4277 4278 /** 4279 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 4280 */ 4281 public ContactDetail getEditorFirstRep() { 4282 if (getEditor().isEmpty()) { 4283 addEditor(); 4284 } 4285 return getEditor().get(0); 4286 } 4287 4288 /** 4289 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.) 4290 */ 4291 public List<ContactDetail> getReviewer() { 4292 if (this.reviewer == null) 4293 this.reviewer = new ArrayList<ContactDetail>(); 4294 return this.reviewer; 4295 } 4296 4297 /** 4298 * @return Returns a reference to <code>this</code> for easy method chaining 4299 */ 4300 public EvidenceVariable setReviewer(List<ContactDetail> theReviewer) { 4301 this.reviewer = theReviewer; 4302 return this; 4303 } 4304 4305 public boolean hasReviewer() { 4306 if (this.reviewer == null) 4307 return false; 4308 for (ContactDetail item : this.reviewer) 4309 if (!item.isEmpty()) 4310 return true; 4311 return false; 4312 } 4313 4314 public ContactDetail addReviewer() { //3 4315 ContactDetail t = new ContactDetail(); 4316 if (this.reviewer == null) 4317 this.reviewer = new ArrayList<ContactDetail>(); 4318 this.reviewer.add(t); 4319 return t; 4320 } 4321 4322 public EvidenceVariable addReviewer(ContactDetail t) { //3 4323 if (t == null) 4324 return this; 4325 if (this.reviewer == null) 4326 this.reviewer = new ArrayList<ContactDetail>(); 4327 this.reviewer.add(t); 4328 return this; 4329 } 4330 4331 /** 4332 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 4333 */ 4334 public ContactDetail getReviewerFirstRep() { 4335 if (getReviewer().isEmpty()) { 4336 addReviewer(); 4337 } 4338 return getReviewer().get(0); 4339 } 4340 4341 /** 4342 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.) 4343 */ 4344 public List<ContactDetail> getEndorser() { 4345 if (this.endorser == null) 4346 this.endorser = new ArrayList<ContactDetail>(); 4347 return this.endorser; 4348 } 4349 4350 /** 4351 * @return Returns a reference to <code>this</code> for easy method chaining 4352 */ 4353 public EvidenceVariable setEndorser(List<ContactDetail> theEndorser) { 4354 this.endorser = theEndorser; 4355 return this; 4356 } 4357 4358 public boolean hasEndorser() { 4359 if (this.endorser == null) 4360 return false; 4361 for (ContactDetail item : this.endorser) 4362 if (!item.isEmpty()) 4363 return true; 4364 return false; 4365 } 4366 4367 public ContactDetail addEndorser() { //3 4368 ContactDetail t = new ContactDetail(); 4369 if (this.endorser == null) 4370 this.endorser = new ArrayList<ContactDetail>(); 4371 this.endorser.add(t); 4372 return t; 4373 } 4374 4375 public EvidenceVariable addEndorser(ContactDetail t) { //3 4376 if (t == null) 4377 return this; 4378 if (this.endorser == null) 4379 this.endorser = new ArrayList<ContactDetail>(); 4380 this.endorser.add(t); 4381 return this; 4382 } 4383 4384 /** 4385 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 4386 */ 4387 public ContactDetail getEndorserFirstRep() { 4388 if (getEndorser().isEmpty()) { 4389 addEndorser(); 4390 } 4391 return getEndorser().get(0); 4392 } 4393 4394 /** 4395 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 4396 */ 4397 public List<RelatedArtifact> getRelatedArtifact() { 4398 if (this.relatedArtifact == null) 4399 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4400 return this.relatedArtifact; 4401 } 4402 4403 /** 4404 * @return Returns a reference to <code>this</code> for easy method chaining 4405 */ 4406 public EvidenceVariable setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 4407 this.relatedArtifact = theRelatedArtifact; 4408 return this; 4409 } 4410 4411 public boolean hasRelatedArtifact() { 4412 if (this.relatedArtifact == null) 4413 return false; 4414 for (RelatedArtifact item : this.relatedArtifact) 4415 if (!item.isEmpty()) 4416 return true; 4417 return false; 4418 } 4419 4420 public RelatedArtifact addRelatedArtifact() { //3 4421 RelatedArtifact t = new RelatedArtifact(); 4422 if (this.relatedArtifact == null) 4423 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4424 this.relatedArtifact.add(t); 4425 return t; 4426 } 4427 4428 public EvidenceVariable addRelatedArtifact(RelatedArtifact t) { //3 4429 if (t == null) 4430 return this; 4431 if (this.relatedArtifact == null) 4432 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4433 this.relatedArtifact.add(t); 4434 return this; 4435 } 4436 4437 /** 4438 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 4439 */ 4440 public RelatedArtifact getRelatedArtifactFirstRep() { 4441 if (getRelatedArtifact().isEmpty()) { 4442 addRelatedArtifact(); 4443 } 4444 return getRelatedArtifact().get(0); 4445 } 4446 4447 /** 4448 * @return {@link #actual} (True if the actual variable measured, false if a conceptual representation of the intended variable.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 4449 */ 4450 public BooleanType getActualElement() { 4451 if (this.actual == null) 4452 if (Configuration.errorOnAutoCreate()) 4453 throw new Error("Attempt to auto-create EvidenceVariable.actual"); 4454 else if (Configuration.doAutoCreate()) 4455 this.actual = new BooleanType(); // bb 4456 return this.actual; 4457 } 4458 4459 public boolean hasActualElement() { 4460 return this.actual != null && !this.actual.isEmpty(); 4461 } 4462 4463 public boolean hasActual() { 4464 return this.actual != null && !this.actual.isEmpty(); 4465 } 4466 4467 /** 4468 * @param value {@link #actual} (True if the actual variable measured, false if a conceptual representation of the intended variable.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 4469 */ 4470 public EvidenceVariable setActualElement(BooleanType value) { 4471 this.actual = value; 4472 return this; 4473 } 4474 4475 /** 4476 * @return True if the actual variable measured, false if a conceptual representation of the intended variable. 4477 */ 4478 public boolean getActual() { 4479 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 4480 } 4481 4482 /** 4483 * @param value True if the actual variable measured, false if a conceptual representation of the intended variable. 4484 */ 4485 public EvidenceVariable setActual(boolean value) { 4486 if (this.actual == null) 4487 this.actual = new BooleanType(); 4488 this.actual.setValue(value); 4489 return this; 4490 } 4491 4492 /** 4493 * @return {@link #characteristic} (A defining factor of the EvidenceVariable. Multiple characteristics are applied with "and" semantics.) 4494 */ 4495 public List<EvidenceVariableCharacteristicComponent> getCharacteristic() { 4496 if (this.characteristic == null) 4497 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 4498 return this.characteristic; 4499 } 4500 4501 /** 4502 * @return Returns a reference to <code>this</code> for easy method chaining 4503 */ 4504 public EvidenceVariable setCharacteristic(List<EvidenceVariableCharacteristicComponent> theCharacteristic) { 4505 this.characteristic = theCharacteristic; 4506 return this; 4507 } 4508 4509 public boolean hasCharacteristic() { 4510 if (this.characteristic == null) 4511 return false; 4512 for (EvidenceVariableCharacteristicComponent item : this.characteristic) 4513 if (!item.isEmpty()) 4514 return true; 4515 return false; 4516 } 4517 4518 public EvidenceVariableCharacteristicComponent addCharacteristic() { //3 4519 EvidenceVariableCharacteristicComponent t = new EvidenceVariableCharacteristicComponent(); 4520 if (this.characteristic == null) 4521 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 4522 this.characteristic.add(t); 4523 return t; 4524 } 4525 4526 public EvidenceVariable addCharacteristic(EvidenceVariableCharacteristicComponent t) { //3 4527 if (t == null) 4528 return this; 4529 if (this.characteristic == null) 4530 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 4531 this.characteristic.add(t); 4532 return this; 4533 } 4534 4535 /** 4536 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 4537 */ 4538 public EvidenceVariableCharacteristicComponent getCharacteristicFirstRep() { 4539 if (getCharacteristic().isEmpty()) { 4540 addCharacteristic(); 4541 } 4542 return getCharacteristic().get(0); 4543 } 4544 4545 /** 4546 * @return {@link #handling} (The method of handling in statistical analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 4547 */ 4548 public Enumeration<EvidenceVariableHandling> getHandlingElement() { 4549 if (this.handling == null) 4550 if (Configuration.errorOnAutoCreate()) 4551 throw new Error("Attempt to auto-create EvidenceVariable.handling"); 4552 else if (Configuration.doAutoCreate()) 4553 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); // bb 4554 return this.handling; 4555 } 4556 4557 public boolean hasHandlingElement() { 4558 return this.handling != null && !this.handling.isEmpty(); 4559 } 4560 4561 public boolean hasHandling() { 4562 return this.handling != null && !this.handling.isEmpty(); 4563 } 4564 4565 /** 4566 * @param value {@link #handling} (The method of handling in statistical analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 4567 */ 4568 public EvidenceVariable setHandlingElement(Enumeration<EvidenceVariableHandling> value) { 4569 this.handling = value; 4570 return this; 4571 } 4572 4573 /** 4574 * @return The method of handling in statistical analysis. 4575 */ 4576 public EvidenceVariableHandling getHandling() { 4577 return this.handling == null ? null : this.handling.getValue(); 4578 } 4579 4580 /** 4581 * @param value The method of handling in statistical analysis. 4582 */ 4583 public EvidenceVariable setHandling(EvidenceVariableHandling value) { 4584 if (value == null) 4585 this.handling = null; 4586 else { 4587 if (this.handling == null) 4588 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); 4589 this.handling.setValue(value); 4590 } 4591 return this; 4592 } 4593 4594 /** 4595 * @return {@link #category} (A grouping for ordinal or polychotomous variables.) 4596 */ 4597 public List<EvidenceVariableCategoryComponent> getCategory() { 4598 if (this.category == null) 4599 this.category = new ArrayList<EvidenceVariableCategoryComponent>(); 4600 return this.category; 4601 } 4602 4603 /** 4604 * @return Returns a reference to <code>this</code> for easy method chaining 4605 */ 4606 public EvidenceVariable setCategory(List<EvidenceVariableCategoryComponent> theCategory) { 4607 this.category = theCategory; 4608 return this; 4609 } 4610 4611 public boolean hasCategory() { 4612 if (this.category == null) 4613 return false; 4614 for (EvidenceVariableCategoryComponent item : this.category) 4615 if (!item.isEmpty()) 4616 return true; 4617 return false; 4618 } 4619 4620 public EvidenceVariableCategoryComponent addCategory() { //3 4621 EvidenceVariableCategoryComponent t = new EvidenceVariableCategoryComponent(); 4622 if (this.category == null) 4623 this.category = new ArrayList<EvidenceVariableCategoryComponent>(); 4624 this.category.add(t); 4625 return t; 4626 } 4627 4628 public EvidenceVariable addCategory(EvidenceVariableCategoryComponent t) { //3 4629 if (t == null) 4630 return this; 4631 if (this.category == null) 4632 this.category = new ArrayList<EvidenceVariableCategoryComponent>(); 4633 this.category.add(t); 4634 return this; 4635 } 4636 4637 /** 4638 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 4639 */ 4640 public EvidenceVariableCategoryComponent getCategoryFirstRep() { 4641 if (getCategory().isEmpty()) { 4642 addCategory(); 4643 } 4644 return getCategory().get(0); 4645 } 4646 4647 /** 4648 * not supported on this implementation 4649 */ 4650 @Override 4651 public int getJurisdictionMax() { 4652 return 0; 4653 } 4654 /** 4655 * @return {@link #jurisdiction} (A legal or geographic region in which the evidence variable is intended to be used.) 4656 */ 4657 public List<CodeableConcept> getJurisdiction() { 4658 return new ArrayList<>(); 4659 } 4660 /** 4661 * @return Returns a reference to <code>this</code> for easy method chaining 4662 */ 4663 public EvidenceVariable setJurisdiction(List<CodeableConcept> theJurisdiction) { 4664 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4665 } 4666 public boolean hasJurisdiction() { 4667 return false; 4668 } 4669 4670 public CodeableConcept addJurisdiction() { //3 4671 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4672 } 4673 public EvidenceVariable addJurisdiction(CodeableConcept t) { //3 4674 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4675 } 4676 /** 4677 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {2} 4678 */ 4679 public CodeableConcept getJurisdictionFirstRep() { 4680 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"jurisdiction\""); 4681 } 4682 /** 4683 * not supported on this implementation 4684 */ 4685 @Override 4686 public int getTopicMax() { 4687 return 0; 4688 } 4689 /** 4690 * @return {@link #topic} (Descriptive topics related to the content of the evidence variable. Topics provide a high-level categorization as well as keywords for the evidence variable that can be useful for filtering and searching.) 4691 */ 4692 public List<CodeableConcept> getTopic() { 4693 return new ArrayList<>(); 4694 } 4695 /** 4696 * @return Returns a reference to <code>this</code> for easy method chaining 4697 */ 4698 public EvidenceVariable setTopic(List<CodeableConcept> theTopic) { 4699 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4700 } 4701 public boolean hasTopic() { 4702 return false; 4703 } 4704 4705 public CodeableConcept addTopic() { //3 4706 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4707 } 4708 public EvidenceVariable addTopic(CodeableConcept t) { //3 4709 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4710 } 4711 /** 4712 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {2} 4713 */ 4714 public CodeableConcept getTopicFirstRep() { 4715 throw new Error("The resource type \"EvidenceVariable\" does not implement the property \"topic\""); 4716 } 4717 protected void listChildren(List<Property> children) { 4718 super.listChildren(children); 4719 children.add(new Property("url", "uri", "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 0, 1, url)); 4720 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4721 children.add(new Property("version", "string", "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 4722 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 4723 children.add(new Property("name", "string", "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 4724 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the evidence variable.", 0, 1, title)); 4725 children.add(new Property("shortTitle", "string", "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 0, 1, shortTitle)); 4726 children.add(new Property("status", "code", "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status)); 4727 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 4728 children.add(new Property("date", "dateTime", "The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 0, 1, date)); 4729 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.", 0, 1, publisher)); 4730 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4731 children.add(new Property("description", "markdown", "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, description)); 4732 children.add(new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, note)); 4733 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4734 children.add(new Property("purpose", "markdown", "Explanation of why this EvidenceVariable is needed and why it has been designed as it has.", 0, 1, purpose)); 4735 children.add(new Property("copyright", "markdown", "A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.", 0, 1, copyright)); 4736 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 4737 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.\n\nSee guidance around (not) making local changes to elements [here](canonicalresource.html#localization).", 0, 1, approvalDate)); 4738 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 4739 children.add(new Property("effectivePeriod", "Period", "The period during which the resource content was or is planned to be in active use.", 0, 1, effectivePeriod)); 4740 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 4741 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 4742 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 4743 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 4744 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4745 children.add(new Property("actual", "boolean", "True if the actual variable measured, false if a conceptual representation of the intended variable.", 0, 1, actual)); 4746 children.add(new Property("characteristic", "", "A defining factor of the EvidenceVariable. Multiple characteristics are applied with \"and\" semantics.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 4747 children.add(new Property("handling", "code", "The method of handling in statistical analysis.", 0, 1, handling)); 4748 children.add(new Property("category", "", "A grouping for ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, category)); 4749 } 4750 4751 @Override 4752 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4753 switch (_hash) { 4754 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 0, 1, url); 4755 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4756 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 4757 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4758 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4759 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4760 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4761 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 4762 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the evidence variable.", 0, 1, title); 4763 case 1555503932: /*shortTitle*/ return new Property("shortTitle", "string", "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 0, 1, shortTitle); 4764 case -892481550: /*status*/ return new Property("status", "code", "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status); 4765 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 4766 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the evidence variable was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 0, 1, date); 4767 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the evidence variable.", 0, 1, publisher); 4768 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4769 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, description); 4770 case 3387378: /*note*/ return new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, note); 4771 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4772 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this EvidenceVariable is needed and why it has been designed as it has.", 0, 1, purpose); 4773 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the resource and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the resource.", 0, 1, copyright); 4774 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 4775 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.\n\nSee guidance around (not) making local changes to elements [here](canonicalresource.html#localization).", 0, 1, approvalDate); 4776 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 4777 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the resource content was or is planned to be in active use.", 0, 1, effectivePeriod); 4778 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 4779 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 4780 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 4781 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 4782 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4783 case -1422939762: /*actual*/ return new Property("actual", "boolean", "True if the actual variable measured, false if a conceptual representation of the intended variable.", 0, 1, actual); 4784 case 366313883: /*characteristic*/ return new Property("characteristic", "", "A defining factor of the EvidenceVariable. Multiple characteristics are applied with \"and\" semantics.", 0, java.lang.Integer.MAX_VALUE, characteristic); 4785 case 2072805: /*handling*/ return new Property("handling", "code", "The method of handling in statistical analysis.", 0, 1, handling); 4786 case 50511102: /*category*/ return new Property("category", "", "A grouping for ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, category); 4787 default: return super.getNamedProperty(_hash, _name, _checkValid); 4788 } 4789 4790 } 4791 4792 @Override 4793 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4794 switch (hash) { 4795 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4796 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4797 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4798 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 4799 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4800 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4801 case 1555503932: /*shortTitle*/ return this.shortTitle == null ? new Base[0] : new Base[] {this.shortTitle}; // StringType 4802 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4803 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4804 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4805 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4806 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4807 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4808 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4809 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4810 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4811 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4812 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 4813 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 4814 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 4815 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 4816 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4817 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4818 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4819 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4820 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4821 case -1422939762: /*actual*/ return this.actual == null ? new Base[0] : new Base[] {this.actual}; // BooleanType 4822 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceVariableCharacteristicComponent 4823 case 2072805: /*handling*/ return this.handling == null ? new Base[0] : new Base[] {this.handling}; // Enumeration<EvidenceVariableHandling> 4824 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // EvidenceVariableCategoryComponent 4825 default: return super.getProperty(hash, name, checkValid); 4826 } 4827 4828 } 4829 4830 @Override 4831 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4832 switch (hash) { 4833 case 116079: // url 4834 this.url = TypeConvertor.castToUri(value); // UriType 4835 return value; 4836 case -1618432855: // identifier 4837 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4838 return value; 4839 case 351608024: // version 4840 this.version = TypeConvertor.castToString(value); // StringType 4841 return value; 4842 case 1508158071: // versionAlgorithm 4843 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4844 return value; 4845 case 3373707: // name 4846 this.name = TypeConvertor.castToString(value); // StringType 4847 return value; 4848 case 110371416: // title 4849 this.title = TypeConvertor.castToString(value); // StringType 4850 return value; 4851 case 1555503932: // shortTitle 4852 this.shortTitle = TypeConvertor.castToString(value); // StringType 4853 return value; 4854 case -892481550: // status 4855 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4856 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4857 return value; 4858 case -404562712: // experimental 4859 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4860 return value; 4861 case 3076014: // date 4862 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4863 return value; 4864 case 1447404028: // publisher 4865 this.publisher = TypeConvertor.castToString(value); // StringType 4866 return value; 4867 case 951526432: // contact 4868 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4869 return value; 4870 case -1724546052: // description 4871 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4872 return value; 4873 case 3387378: // note 4874 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4875 return value; 4876 case -669707736: // useContext 4877 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4878 return value; 4879 case -220463842: // purpose 4880 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4881 return value; 4882 case 1522889671: // copyright 4883 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4884 return value; 4885 case 765157229: // copyrightLabel 4886 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4887 return value; 4888 case 223539345: // approvalDate 4889 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4890 return value; 4891 case -1687512484: // lastReviewDate 4892 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4893 return value; 4894 case -403934648: // effectivePeriod 4895 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4896 return value; 4897 case -1406328437: // author 4898 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4899 return value; 4900 case -1307827859: // editor 4901 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4902 return value; 4903 case -261190139: // reviewer 4904 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4905 return value; 4906 case 1740277666: // endorser 4907 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4908 return value; 4909 case 666807069: // relatedArtifact 4910 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4911 return value; 4912 case -1422939762: // actual 4913 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 4914 return value; 4915 case 366313883: // characteristic 4916 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); // EvidenceVariableCharacteristicComponent 4917 return value; 4918 case 2072805: // handling 4919 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 4920 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 4921 return value; 4922 case 50511102: // category 4923 this.getCategory().add((EvidenceVariableCategoryComponent) value); // EvidenceVariableCategoryComponent 4924 return value; 4925 default: return super.setProperty(hash, name, value); 4926 } 4927 4928 } 4929 4930 @Override 4931 public Base setProperty(String name, Base value) throws FHIRException { 4932 if (name.equals("url")) { 4933 this.url = TypeConvertor.castToUri(value); // UriType 4934 } else if (name.equals("identifier")) { 4935 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4936 } else if (name.equals("version")) { 4937 this.version = TypeConvertor.castToString(value); // StringType 4938 } else if (name.equals("versionAlgorithm[x]")) { 4939 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4940 } else if (name.equals("name")) { 4941 this.name = TypeConvertor.castToString(value); // StringType 4942 } else if (name.equals("title")) { 4943 this.title = TypeConvertor.castToString(value); // StringType 4944 } else if (name.equals("shortTitle")) { 4945 this.shortTitle = TypeConvertor.castToString(value); // StringType 4946 } else if (name.equals("status")) { 4947 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4948 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4949 } else if (name.equals("experimental")) { 4950 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4951 } else if (name.equals("date")) { 4952 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4953 } else if (name.equals("publisher")) { 4954 this.publisher = TypeConvertor.castToString(value); // StringType 4955 } else if (name.equals("contact")) { 4956 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4957 } else if (name.equals("description")) { 4958 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4959 } else if (name.equals("note")) { 4960 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4961 } else if (name.equals("useContext")) { 4962 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4963 } else if (name.equals("purpose")) { 4964 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4965 } else if (name.equals("copyright")) { 4966 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4967 } else if (name.equals("copyrightLabel")) { 4968 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4969 } else if (name.equals("approvalDate")) { 4970 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4971 } else if (name.equals("lastReviewDate")) { 4972 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4973 } else if (name.equals("effectivePeriod")) { 4974 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4975 } else if (name.equals("author")) { 4976 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 4977 } else if (name.equals("editor")) { 4978 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 4979 } else if (name.equals("reviewer")) { 4980 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 4981 } else if (name.equals("endorser")) { 4982 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 4983 } else if (name.equals("relatedArtifact")) { 4984 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4985 } else if (name.equals("actual")) { 4986 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 4987 } else if (name.equals("characteristic")) { 4988 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); 4989 } else if (name.equals("handling")) { 4990 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 4991 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 4992 } else if (name.equals("category")) { 4993 this.getCategory().add((EvidenceVariableCategoryComponent) value); 4994 } else 4995 return super.setProperty(name, value); 4996 return value; 4997 } 4998 4999 @Override 5000 public void removeChild(String name, Base value) throws FHIRException { 5001 if (name.equals("url")) { 5002 this.url = null; 5003 } else if (name.equals("identifier")) { 5004 this.getIdentifier().remove(value); 5005 } else if (name.equals("version")) { 5006 this.version = null; 5007 } else if (name.equals("versionAlgorithm[x]")) { 5008 this.versionAlgorithm = null; 5009 } else if (name.equals("name")) { 5010 this.name = null; 5011 } else if (name.equals("title")) { 5012 this.title = null; 5013 } else if (name.equals("shortTitle")) { 5014 this.shortTitle = null; 5015 } else if (name.equals("status")) { 5016 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5017 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5018 } else if (name.equals("experimental")) { 5019 this.experimental = null; 5020 } else if (name.equals("date")) { 5021 this.date = null; 5022 } else if (name.equals("publisher")) { 5023 this.publisher = null; 5024 } else if (name.equals("contact")) { 5025 this.getContact().remove(value); 5026 } else if (name.equals("description")) { 5027 this.description = null; 5028 } else if (name.equals("note")) { 5029 this.getNote().remove(value); 5030 } else if (name.equals("useContext")) { 5031 this.getUseContext().remove(value); 5032 } else if (name.equals("purpose")) { 5033 this.purpose = null; 5034 } else if (name.equals("copyright")) { 5035 this.copyright = null; 5036 } else if (name.equals("copyrightLabel")) { 5037 this.copyrightLabel = null; 5038 } else if (name.equals("approvalDate")) { 5039 this.approvalDate = null; 5040 } else if (name.equals("lastReviewDate")) { 5041 this.lastReviewDate = null; 5042 } else if (name.equals("effectivePeriod")) { 5043 this.effectivePeriod = null; 5044 } else if (name.equals("author")) { 5045 this.getAuthor().remove(value); 5046 } else if (name.equals("editor")) { 5047 this.getEditor().remove(value); 5048 } else if (name.equals("reviewer")) { 5049 this.getReviewer().remove(value); 5050 } else if (name.equals("endorser")) { 5051 this.getEndorser().remove(value); 5052 } else if (name.equals("relatedArtifact")) { 5053 this.getRelatedArtifact().remove(value); 5054 } else if (name.equals("actual")) { 5055 this.actual = null; 5056 } else if (name.equals("characteristic")) { 5057 this.getCharacteristic().remove((EvidenceVariableCharacteristicComponent) value); 5058 } else if (name.equals("handling")) { 5059 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 5060 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 5061 } else if (name.equals("category")) { 5062 this.getCategory().remove((EvidenceVariableCategoryComponent) value); 5063 } else 5064 super.removeChild(name, value); 5065 5066 } 5067 5068 @Override 5069 public Base makeProperty(int hash, String name) throws FHIRException { 5070 switch (hash) { 5071 case 116079: return getUrlElement(); 5072 case -1618432855: return addIdentifier(); 5073 case 351608024: return getVersionElement(); 5074 case -115699031: return getVersionAlgorithm(); 5075 case 1508158071: return getVersionAlgorithm(); 5076 case 3373707: return getNameElement(); 5077 case 110371416: return getTitleElement(); 5078 case 1555503932: return getShortTitleElement(); 5079 case -892481550: return getStatusElement(); 5080 case -404562712: return getExperimentalElement(); 5081 case 3076014: return getDateElement(); 5082 case 1447404028: return getPublisherElement(); 5083 case 951526432: return addContact(); 5084 case -1724546052: return getDescriptionElement(); 5085 case 3387378: return addNote(); 5086 case -669707736: return addUseContext(); 5087 case -220463842: return getPurposeElement(); 5088 case 1522889671: return getCopyrightElement(); 5089 case 765157229: return getCopyrightLabelElement(); 5090 case 223539345: return getApprovalDateElement(); 5091 case -1687512484: return getLastReviewDateElement(); 5092 case -403934648: return getEffectivePeriod(); 5093 case -1406328437: return addAuthor(); 5094 case -1307827859: return addEditor(); 5095 case -261190139: return addReviewer(); 5096 case 1740277666: return addEndorser(); 5097 case 666807069: return addRelatedArtifact(); 5098 case -1422939762: return getActualElement(); 5099 case 366313883: return addCharacteristic(); 5100 case 2072805: return getHandlingElement(); 5101 case 50511102: return addCategory(); 5102 default: return super.makeProperty(hash, name); 5103 } 5104 5105 } 5106 5107 @Override 5108 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5109 switch (hash) { 5110 case 116079: /*url*/ return new String[] {"uri"}; 5111 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5112 case 351608024: /*version*/ return new String[] {"string"}; 5113 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 5114 case 3373707: /*name*/ return new String[] {"string"}; 5115 case 110371416: /*title*/ return new String[] {"string"}; 5116 case 1555503932: /*shortTitle*/ return new String[] {"string"}; 5117 case -892481550: /*status*/ return new String[] {"code"}; 5118 case -404562712: /*experimental*/ return new String[] {"boolean"}; 5119 case 3076014: /*date*/ return new String[] {"dateTime"}; 5120 case 1447404028: /*publisher*/ return new String[] {"string"}; 5121 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 5122 case -1724546052: /*description*/ return new String[] {"markdown"}; 5123 case 3387378: /*note*/ return new String[] {"Annotation"}; 5124 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5125 case -220463842: /*purpose*/ return new String[] {"markdown"}; 5126 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5127 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 5128 case 223539345: /*approvalDate*/ return new String[] {"date"}; 5129 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 5130 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 5131 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 5132 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 5133 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 5134 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 5135 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 5136 case -1422939762: /*actual*/ return new String[] {"boolean"}; 5137 case 366313883: /*characteristic*/ return new String[] {}; 5138 case 2072805: /*handling*/ return new String[] {"code"}; 5139 case 50511102: /*category*/ return new String[] {}; 5140 default: return super.getTypesForProperty(hash, name); 5141 } 5142 5143 } 5144 5145 @Override 5146 public Base addChild(String name) throws FHIRException { 5147 if (name.equals("url")) { 5148 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.url"); 5149 } 5150 else if (name.equals("identifier")) { 5151 return addIdentifier(); 5152 } 5153 else if (name.equals("version")) { 5154 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.version"); 5155 } 5156 else if (name.equals("versionAlgorithmString")) { 5157 this.versionAlgorithm = new StringType(); 5158 return this.versionAlgorithm; 5159 } 5160 else if (name.equals("versionAlgorithmCoding")) { 5161 this.versionAlgorithm = new Coding(); 5162 return this.versionAlgorithm; 5163 } 5164 else if (name.equals("name")) { 5165 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.name"); 5166 } 5167 else if (name.equals("title")) { 5168 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.title"); 5169 } 5170 else if (name.equals("shortTitle")) { 5171 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.shortTitle"); 5172 } 5173 else if (name.equals("status")) { 5174 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.status"); 5175 } 5176 else if (name.equals("experimental")) { 5177 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.experimental"); 5178 } 5179 else if (name.equals("date")) { 5180 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.date"); 5181 } 5182 else if (name.equals("publisher")) { 5183 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.publisher"); 5184 } 5185 else if (name.equals("contact")) { 5186 return addContact(); 5187 } 5188 else if (name.equals("description")) { 5189 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.description"); 5190 } 5191 else if (name.equals("note")) { 5192 return addNote(); 5193 } 5194 else if (name.equals("useContext")) { 5195 return addUseContext(); 5196 } 5197 else if (name.equals("purpose")) { 5198 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.purpose"); 5199 } 5200 else if (name.equals("copyright")) { 5201 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.copyright"); 5202 } 5203 else if (name.equals("copyrightLabel")) { 5204 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.copyrightLabel"); 5205 } 5206 else if (name.equals("approvalDate")) { 5207 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.approvalDate"); 5208 } 5209 else if (name.equals("lastReviewDate")) { 5210 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.lastReviewDate"); 5211 } 5212 else if (name.equals("effectivePeriod")) { 5213 this.effectivePeriod = new Period(); 5214 return this.effectivePeriod; 5215 } 5216 else if (name.equals("author")) { 5217 return addAuthor(); 5218 } 5219 else if (name.equals("editor")) { 5220 return addEditor(); 5221 } 5222 else if (name.equals("reviewer")) { 5223 return addReviewer(); 5224 } 5225 else if (name.equals("endorser")) { 5226 return addEndorser(); 5227 } 5228 else if (name.equals("relatedArtifact")) { 5229 return addRelatedArtifact(); 5230 } 5231 else if (name.equals("actual")) { 5232 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.actual"); 5233 } 5234 else if (name.equals("characteristic")) { 5235 return addCharacteristic(); 5236 } 5237 else if (name.equals("handling")) { 5238 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.handling"); 5239 } 5240 else if (name.equals("category")) { 5241 return addCategory(); 5242 } 5243 else 5244 return super.addChild(name); 5245 } 5246 5247 public String fhirType() { 5248 return "EvidenceVariable"; 5249 5250 } 5251 5252 public EvidenceVariable copy() { 5253 EvidenceVariable dst = new EvidenceVariable(); 5254 copyValues(dst); 5255 return dst; 5256 } 5257 5258 public void copyValues(EvidenceVariable dst) { 5259 super.copyValues(dst); 5260 dst.url = url == null ? null : url.copy(); 5261 if (identifier != null) { 5262 dst.identifier = new ArrayList<Identifier>(); 5263 for (Identifier i : identifier) 5264 dst.identifier.add(i.copy()); 5265 }; 5266 dst.version = version == null ? null : version.copy(); 5267 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 5268 dst.name = name == null ? null : name.copy(); 5269 dst.title = title == null ? null : title.copy(); 5270 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 5271 dst.status = status == null ? null : status.copy(); 5272 dst.experimental = experimental == null ? null : experimental.copy(); 5273 dst.date = date == null ? null : date.copy(); 5274 dst.publisher = publisher == null ? null : publisher.copy(); 5275 if (contact != null) { 5276 dst.contact = new ArrayList<ContactDetail>(); 5277 for (ContactDetail i : contact) 5278 dst.contact.add(i.copy()); 5279 }; 5280 dst.description = description == null ? null : description.copy(); 5281 if (note != null) { 5282 dst.note = new ArrayList<Annotation>(); 5283 for (Annotation i : note) 5284 dst.note.add(i.copy()); 5285 }; 5286 if (useContext != null) { 5287 dst.useContext = new ArrayList<UsageContext>(); 5288 for (UsageContext i : useContext) 5289 dst.useContext.add(i.copy()); 5290 }; 5291 dst.purpose = purpose == null ? null : purpose.copy(); 5292 dst.copyright = copyright == null ? null : copyright.copy(); 5293 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 5294 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5295 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5296 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5297 if (author != null) { 5298 dst.author = new ArrayList<ContactDetail>(); 5299 for (ContactDetail i : author) 5300 dst.author.add(i.copy()); 5301 }; 5302 if (editor != null) { 5303 dst.editor = new ArrayList<ContactDetail>(); 5304 for (ContactDetail i : editor) 5305 dst.editor.add(i.copy()); 5306 }; 5307 if (reviewer != null) { 5308 dst.reviewer = new ArrayList<ContactDetail>(); 5309 for (ContactDetail i : reviewer) 5310 dst.reviewer.add(i.copy()); 5311 }; 5312 if (endorser != null) { 5313 dst.endorser = new ArrayList<ContactDetail>(); 5314 for (ContactDetail i : endorser) 5315 dst.endorser.add(i.copy()); 5316 }; 5317 if (relatedArtifact != null) { 5318 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5319 for (RelatedArtifact i : relatedArtifact) 5320 dst.relatedArtifact.add(i.copy()); 5321 }; 5322 dst.actual = actual == null ? null : actual.copy(); 5323 if (characteristic != null) { 5324 dst.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 5325 for (EvidenceVariableCharacteristicComponent i : characteristic) 5326 dst.characteristic.add(i.copy()); 5327 }; 5328 dst.handling = handling == null ? null : handling.copy(); 5329 if (category != null) { 5330 dst.category = new ArrayList<EvidenceVariableCategoryComponent>(); 5331 for (EvidenceVariableCategoryComponent i : category) 5332 dst.category.add(i.copy()); 5333 }; 5334 } 5335 5336 protected EvidenceVariable typedCopy() { 5337 return copy(); 5338 } 5339 5340 @Override 5341 public boolean equalsDeep(Base other_) { 5342 if (!super.equalsDeep(other_)) 5343 return false; 5344 if (!(other_ instanceof EvidenceVariable)) 5345 return false; 5346 EvidenceVariable o = (EvidenceVariable) other_; 5347 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 5348 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 5349 && compareDeep(shortTitle, o.shortTitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 5350 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 5351 && compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(useContext, o.useContext, true) 5352 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 5353 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5354 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(author, o.author, true) 5355 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 5356 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(actual, o.actual, true) 5357 && compareDeep(characteristic, o.characteristic, true) && compareDeep(handling, o.handling, true) 5358 && compareDeep(category, o.category, true); 5359 } 5360 5361 @Override 5362 public boolean equalsShallow(Base other_) { 5363 if (!super.equalsShallow(other_)) 5364 return false; 5365 if (!(other_ instanceof EvidenceVariable)) 5366 return false; 5367 EvidenceVariable o = (EvidenceVariable) other_; 5368 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 5369 && compareValues(title, o.title, true) && compareValues(shortTitle, o.shortTitle, true) && compareValues(status, o.status, true) 5370 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 5371 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 5372 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(approvalDate, o.approvalDate, true) 5373 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(actual, o.actual, true) && compareValues(handling, o.handling, true) 5374 ; 5375 } 5376 5377 public boolean isEmpty() { 5378 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 5379 , versionAlgorithm, name, title, shortTitle, status, experimental, date, publisher 5380 , contact, description, note, useContext, purpose, copyright, copyrightLabel, approvalDate 5381 , lastReviewDate, effectivePeriod, author, editor, reviewer, endorser, relatedArtifact 5382 , actual, characteristic, handling, category); 5383 } 5384 5385 @Override 5386 public ResourceType getResourceType() { 5387 return ResourceType.EvidenceVariable; 5388 } 5389 5390 /** 5391 * Search parameter: <b>context-quantity</b> 5392 * <p> 5393 * Description: <b>Multiple Resources: 5394 5395* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5396* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5397* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5398* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5399* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5400* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5401* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5402* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5403* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5404* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5405* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5406* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5407* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5408* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5409* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5410* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5411* [Library](library.html): A quantity- or range-valued use context assigned to the library 5412* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5413* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5414* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5415* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5416* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5417* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5418* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5419* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5420* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5421* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5422* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5423* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5424* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5425</b><br> 5426 * Type: <b>quantity</b><br> 5427 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5428 * </p> 5429 */ 5430 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 5431 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5432 /** 5433 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5434 * <p> 5435 * Description: <b>Multiple Resources: 5436 5437* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5438* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5439* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5440* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5441* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5442* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5443* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5444* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5445* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5446* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5447* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5448* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5449* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5450* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5451* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5452* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5453* [Library](library.html): A quantity- or range-valued use context assigned to the library 5454* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5455* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5456* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5457* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5458* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5459* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5460* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5461* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5462* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5463* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5464* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5465* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5466* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5467</b><br> 5468 * Type: <b>quantity</b><br> 5469 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5470 * </p> 5471 */ 5472 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 5473 5474 /** 5475 * Search parameter: <b>context-type-quantity</b> 5476 * <p> 5477 * Description: <b>Multiple Resources: 5478 5479* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5480* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5481* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5482* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5483* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5484* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5485* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5486* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5487* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5488* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5489* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5490* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5491* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5492* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5493* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5494* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5495* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5496* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5497* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5498* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5499* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5500* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5501* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5502* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5503* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5504* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5505* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5506* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5507* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5508* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5509</b><br> 5510 * Type: <b>composite</b><br> 5511 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5512 * </p> 5513 */ 5514 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 5515 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5516 /** 5517 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 5518 * <p> 5519 * Description: <b>Multiple Resources: 5520 5521* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5522* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5523* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5524* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5525* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5526* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5527* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5528* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5529* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5530* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5531* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5532* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5533* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5534* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5535* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5536* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5537* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5538* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5539* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5540* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5541* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5542* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5543* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5544* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5545* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5546* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5547* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5548* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5549* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5550* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5551</b><br> 5552 * Type: <b>composite</b><br> 5553 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5554 * </p> 5555 */ 5556 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 5557 5558 /** 5559 * Search parameter: <b>context-type-value</b> 5560 * <p> 5561 * Description: <b>Multiple Resources: 5562 5563* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5564* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5565* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5566* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5567* [Citation](citation.html): A use context type and value assigned to the citation 5568* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5569* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5570* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5571* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5572* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5573* [Evidence](evidence.html): A use context type and value assigned to the evidence 5574* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5575* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5576* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5577* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5578* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5579* [Library](library.html): A use context type and value assigned to the library 5580* [Measure](measure.html): A use context type and value assigned to the measure 5581* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5582* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5583* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5584* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5585* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5586* [Requirements](requirements.html): A use context type and value assigned to the requirements 5587* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5588* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5589* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5590* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5591* [TestScript](testscript.html): A use context type and value assigned to the test script 5592* [ValueSet](valueset.html): A use context type and value assigned to the value set 5593</b><br> 5594 * Type: <b>composite</b><br> 5595 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5596 * </p> 5597 */ 5598 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 5599 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5600 /** 5601 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5602 * <p> 5603 * Description: <b>Multiple Resources: 5604 5605* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5606* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5607* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5608* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5609* [Citation](citation.html): A use context type and value assigned to the citation 5610* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5611* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5612* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5613* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5614* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5615* [Evidence](evidence.html): A use context type and value assigned to the evidence 5616* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5617* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5618* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5619* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5620* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5621* [Library](library.html): A use context type and value assigned to the library 5622* [Measure](measure.html): A use context type and value assigned to the measure 5623* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5624* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5625* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5626* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5627* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5628* [Requirements](requirements.html): A use context type and value assigned to the requirements 5629* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5630* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5631* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5632* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5633* [TestScript](testscript.html): A use context type and value assigned to the test script 5634* [ValueSet](valueset.html): A use context type and value assigned to the value set 5635</b><br> 5636 * Type: <b>composite</b><br> 5637 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5638 * </p> 5639 */ 5640 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 5641 5642 /** 5643 * Search parameter: <b>context-type</b> 5644 * <p> 5645 * Description: <b>Multiple Resources: 5646 5647* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5648* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5649* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5650* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5651* [Citation](citation.html): A type of use context assigned to the citation 5652* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5653* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5654* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5655* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5656* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5657* [Evidence](evidence.html): A type of use context assigned to the evidence 5658* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5659* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5660* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5661* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5662* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5663* [Library](library.html): A type of use context assigned to the library 5664* [Measure](measure.html): A type of use context assigned to the measure 5665* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5666* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5667* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5668* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5669* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5670* [Requirements](requirements.html): A type of use context assigned to the requirements 5671* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5672* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5673* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5674* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5675* [TestScript](testscript.html): A type of use context assigned to the test script 5676* [ValueSet](valueset.html): A type of use context assigned to the value set 5677</b><br> 5678 * Type: <b>token</b><br> 5679 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5680 * </p> 5681 */ 5682 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 5683 public static final String SP_CONTEXT_TYPE = "context-type"; 5684 /** 5685 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5686 * <p> 5687 * Description: <b>Multiple Resources: 5688 5689* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5690* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5691* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5692* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5693* [Citation](citation.html): A type of use context assigned to the citation 5694* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5695* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5696* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5697* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5698* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5699* [Evidence](evidence.html): A type of use context assigned to the evidence 5700* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5701* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5702* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5703* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5704* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5705* [Library](library.html): A type of use context assigned to the library 5706* [Measure](measure.html): A type of use context assigned to the measure 5707* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5708* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5709* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5710* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5711* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5712* [Requirements](requirements.html): A type of use context assigned to the requirements 5713* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5714* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5715* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5716* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5717* [TestScript](testscript.html): A type of use context assigned to the test script 5718* [ValueSet](valueset.html): A type of use context assigned to the value set 5719</b><br> 5720 * Type: <b>token</b><br> 5721 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5722 * </p> 5723 */ 5724 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 5725 5726 /** 5727 * Search parameter: <b>context</b> 5728 * <p> 5729 * Description: <b>Multiple Resources: 5730 5731* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5732* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5733* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5734* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5735* [Citation](citation.html): A use context assigned to the citation 5736* [CodeSystem](codesystem.html): A use context assigned to the code system 5737* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5738* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5739* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5740* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5741* [Evidence](evidence.html): A use context assigned to the evidence 5742* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5743* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5744* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5745* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5746* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5747* [Library](library.html): A use context assigned to the library 5748* [Measure](measure.html): A use context assigned to the measure 5749* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5750* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5751* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5752* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5753* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5754* [Requirements](requirements.html): A use context assigned to the requirements 5755* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5756* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5757* [StructureMap](structuremap.html): A use context assigned to the structure map 5758* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5759* [TestScript](testscript.html): A use context assigned to the test script 5760* [ValueSet](valueset.html): A use context assigned to the value set 5761</b><br> 5762 * Type: <b>token</b><br> 5763 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5764 * </p> 5765 */ 5766 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 5767 public static final String SP_CONTEXT = "context"; 5768 /** 5769 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5770 * <p> 5771 * Description: <b>Multiple Resources: 5772 5773* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5774* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5775* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5776* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5777* [Citation](citation.html): A use context assigned to the citation 5778* [CodeSystem](codesystem.html): A use context assigned to the code system 5779* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5780* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5781* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5782* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5783* [Evidence](evidence.html): A use context assigned to the evidence 5784* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5785* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5786* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5787* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5788* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5789* [Library](library.html): A use context assigned to the library 5790* [Measure](measure.html): A use context assigned to the measure 5791* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5792* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5793* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5794* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5795* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5796* [Requirements](requirements.html): A use context assigned to the requirements 5797* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5798* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5799* [StructureMap](structuremap.html): A use context assigned to the structure map 5800* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5801* [TestScript](testscript.html): A use context assigned to the test script 5802* [ValueSet](valueset.html): A use context assigned to the value set 5803</b><br> 5804 * Type: <b>token</b><br> 5805 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5806 * </p> 5807 */ 5808 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 5809 5810 /** 5811 * Search parameter: <b>date</b> 5812 * <p> 5813 * Description: <b>Multiple Resources: 5814 5815* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5816* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5817* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5818* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5819* [Citation](citation.html): The citation publication date 5820* [CodeSystem](codesystem.html): The code system publication date 5821* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5822* [ConceptMap](conceptmap.html): The concept map publication date 5823* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5824* [EventDefinition](eventdefinition.html): The event definition publication date 5825* [Evidence](evidence.html): The evidence publication date 5826* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5827* [ExampleScenario](examplescenario.html): The example scenario publication date 5828* [GraphDefinition](graphdefinition.html): The graph definition publication date 5829* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5830* [Library](library.html): The library publication date 5831* [Measure](measure.html): The measure publication date 5832* [MessageDefinition](messagedefinition.html): The message definition publication date 5833* [NamingSystem](namingsystem.html): The naming system publication date 5834* [OperationDefinition](operationdefinition.html): The operation definition publication date 5835* [PlanDefinition](plandefinition.html): The plan definition publication date 5836* [Questionnaire](questionnaire.html): The questionnaire publication date 5837* [Requirements](requirements.html): The requirements publication date 5838* [SearchParameter](searchparameter.html): The search parameter publication date 5839* [StructureDefinition](structuredefinition.html): The structure definition publication date 5840* [StructureMap](structuremap.html): The structure map publication date 5841* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5842* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5843* [TestScript](testscript.html): The test script publication date 5844* [ValueSet](valueset.html): The value set publication date 5845</b><br> 5846 * Type: <b>date</b><br> 5847 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5848 * </p> 5849 */ 5850 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 5851 public static final String SP_DATE = "date"; 5852 /** 5853 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5854 * <p> 5855 * Description: <b>Multiple Resources: 5856 5857* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5858* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5859* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5860* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5861* [Citation](citation.html): The citation publication date 5862* [CodeSystem](codesystem.html): The code system publication date 5863* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5864* [ConceptMap](conceptmap.html): The concept map publication date 5865* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5866* [EventDefinition](eventdefinition.html): The event definition publication date 5867* [Evidence](evidence.html): The evidence publication date 5868* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5869* [ExampleScenario](examplescenario.html): The example scenario publication date 5870* [GraphDefinition](graphdefinition.html): The graph definition publication date 5871* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5872* [Library](library.html): The library publication date 5873* [Measure](measure.html): The measure publication date 5874* [MessageDefinition](messagedefinition.html): The message definition publication date 5875* [NamingSystem](namingsystem.html): The naming system publication date 5876* [OperationDefinition](operationdefinition.html): The operation definition publication date 5877* [PlanDefinition](plandefinition.html): The plan definition publication date 5878* [Questionnaire](questionnaire.html): The questionnaire publication date 5879* [Requirements](requirements.html): The requirements publication date 5880* [SearchParameter](searchparameter.html): The search parameter publication date 5881* [StructureDefinition](structuredefinition.html): The structure definition publication date 5882* [StructureMap](structuremap.html): The structure map publication date 5883* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5884* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5885* [TestScript](testscript.html): The test script publication date 5886* [ValueSet](valueset.html): The value set publication date 5887</b><br> 5888 * Type: <b>date</b><br> 5889 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5890 * </p> 5891 */ 5892 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5893 5894 /** 5895 * Search parameter: <b>description</b> 5896 * <p> 5897 * Description: <b>Multiple Resources: 5898 5899* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5900* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5901* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5902* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5903* [Citation](citation.html): The description of the citation 5904* [CodeSystem](codesystem.html): The description of the code system 5905* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5906* [ConceptMap](conceptmap.html): The description of the concept map 5907* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5908* [EventDefinition](eventdefinition.html): The description of the event definition 5909* [Evidence](evidence.html): The description of the evidence 5910* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5911* [GraphDefinition](graphdefinition.html): The description of the graph definition 5912* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5913* [Library](library.html): The description of the library 5914* [Measure](measure.html): The description of the measure 5915* [MessageDefinition](messagedefinition.html): The description of the message definition 5916* [NamingSystem](namingsystem.html): The description of the naming system 5917* [OperationDefinition](operationdefinition.html): The description of the operation definition 5918* [PlanDefinition](plandefinition.html): The description of the plan definition 5919* [Questionnaire](questionnaire.html): The description of the questionnaire 5920* [Requirements](requirements.html): The description of the requirements 5921* [SearchParameter](searchparameter.html): The description of the search parameter 5922* [StructureDefinition](structuredefinition.html): The description of the structure definition 5923* [StructureMap](structuremap.html): The description of the structure map 5924* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5925* [TestScript](testscript.html): The description of the test script 5926* [ValueSet](valueset.html): The description of the value set 5927</b><br> 5928 * Type: <b>string</b><br> 5929 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5930 * </p> 5931 */ 5932 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 5933 public static final String SP_DESCRIPTION = "description"; 5934 /** 5935 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5936 * <p> 5937 * Description: <b>Multiple Resources: 5938 5939* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5940* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5941* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5942* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5943* [Citation](citation.html): The description of the citation 5944* [CodeSystem](codesystem.html): The description of the code system 5945* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5946* [ConceptMap](conceptmap.html): The description of the concept map 5947* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5948* [EventDefinition](eventdefinition.html): The description of the event definition 5949* [Evidence](evidence.html): The description of the evidence 5950* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5951* [GraphDefinition](graphdefinition.html): The description of the graph definition 5952* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5953* [Library](library.html): The description of the library 5954* [Measure](measure.html): The description of the measure 5955* [MessageDefinition](messagedefinition.html): The description of the message definition 5956* [NamingSystem](namingsystem.html): The description of the naming system 5957* [OperationDefinition](operationdefinition.html): The description of the operation definition 5958* [PlanDefinition](plandefinition.html): The description of the plan definition 5959* [Questionnaire](questionnaire.html): The description of the questionnaire 5960* [Requirements](requirements.html): The description of the requirements 5961* [SearchParameter](searchparameter.html): The description of the search parameter 5962* [StructureDefinition](structuredefinition.html): The description of the structure definition 5963* [StructureMap](structuremap.html): The description of the structure map 5964* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5965* [TestScript](testscript.html): The description of the test script 5966* [ValueSet](valueset.html): The description of the value set 5967</b><br> 5968 * Type: <b>string</b><br> 5969 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5970 * </p> 5971 */ 5972 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5973 5974 /** 5975 * Search parameter: <b>identifier</b> 5976 * <p> 5977 * Description: <b>Multiple Resources: 5978 5979* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5980* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5981* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5982* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5983* [Citation](citation.html): External identifier for the citation 5984* [CodeSystem](codesystem.html): External identifier for the code system 5985* [ConceptMap](conceptmap.html): External identifier for the concept map 5986* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5987* [EventDefinition](eventdefinition.html): External identifier for the event definition 5988* [Evidence](evidence.html): External identifier for the evidence 5989* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5990* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5991* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5992* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5993* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5994* [Library](library.html): External identifier for the library 5995* [Measure](measure.html): External identifier for the measure 5996* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5997* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5998* [NamingSystem](namingsystem.html): External identifier for the naming system 5999* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6000* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6001* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6002* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6003* [Requirements](requirements.html): External identifier for the requirements 6004* [SearchParameter](searchparameter.html): External identifier for the search parameter 6005* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6006* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6007* [StructureMap](structuremap.html): External identifier for the structure map 6008* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6009* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6010* [TestPlan](testplan.html): An identifier for the test plan 6011* [TestScript](testscript.html): External identifier for the test script 6012* [ValueSet](valueset.html): External identifier for the value set 6013</b><br> 6014 * Type: <b>token</b><br> 6015 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6016 * </p> 6017 */ 6018 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 6019 public static final String SP_IDENTIFIER = "identifier"; 6020 /** 6021 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6022 * <p> 6023 * Description: <b>Multiple Resources: 6024 6025* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6026* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6027* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6028* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6029* [Citation](citation.html): External identifier for the citation 6030* [CodeSystem](codesystem.html): External identifier for the code system 6031* [ConceptMap](conceptmap.html): External identifier for the concept map 6032* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6033* [EventDefinition](eventdefinition.html): External identifier for the event definition 6034* [Evidence](evidence.html): External identifier for the evidence 6035* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6036* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6037* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6038* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6039* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6040* [Library](library.html): External identifier for the library 6041* [Measure](measure.html): External identifier for the measure 6042* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6043* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6044* [NamingSystem](namingsystem.html): External identifier for the naming system 6045* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6046* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6047* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6048* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6049* [Requirements](requirements.html): External identifier for the requirements 6050* [SearchParameter](searchparameter.html): External identifier for the search parameter 6051* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6052* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6053* [StructureMap](structuremap.html): External identifier for the structure map 6054* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6055* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6056* [TestPlan](testplan.html): An identifier for the test plan 6057* [TestScript](testscript.html): External identifier for the test script 6058* [ValueSet](valueset.html): External identifier for the value set 6059</b><br> 6060 * Type: <b>token</b><br> 6061 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6062 * </p> 6063 */ 6064 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6065 6066 /** 6067 * Search parameter: <b>name</b> 6068 * <p> 6069 * Description: <b>Multiple Resources: 6070 6071* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6072* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6073* [Citation](citation.html): Computationally friendly name of the citation 6074* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6075* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6076* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6077* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6078* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6079* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6080* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6081* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6082* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6083* [Library](library.html): Computationally friendly name of the library 6084* [Measure](measure.html): Computationally friendly name of the measure 6085* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6086* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6087* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6088* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6089* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6090* [Requirements](requirements.html): Computationally friendly name of the requirements 6091* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6092* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6093* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6094* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6095* [TestScript](testscript.html): Computationally friendly name of the test script 6096* [ValueSet](valueset.html): Computationally friendly name of the value set 6097</b><br> 6098 * Type: <b>string</b><br> 6099 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6100 * </p> 6101 */ 6102 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 6103 public static final String SP_NAME = "name"; 6104 /** 6105 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6106 * <p> 6107 * Description: <b>Multiple Resources: 6108 6109* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6110* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6111* [Citation](citation.html): Computationally friendly name of the citation 6112* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6113* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6114* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6115* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6116* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6117* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6118* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6119* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6120* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6121* [Library](library.html): Computationally friendly name of the library 6122* [Measure](measure.html): Computationally friendly name of the measure 6123* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6124* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6125* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6126* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6127* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6128* [Requirements](requirements.html): Computationally friendly name of the requirements 6129* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6130* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6131* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6132* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6133* [TestScript](testscript.html): Computationally friendly name of the test script 6134* [ValueSet](valueset.html): Computationally friendly name of the value set 6135</b><br> 6136 * Type: <b>string</b><br> 6137 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6138 * </p> 6139 */ 6140 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 6141 6142 /** 6143 * Search parameter: <b>publisher</b> 6144 * <p> 6145 * Description: <b>Multiple Resources: 6146 6147* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6148* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6149* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6150* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6151* [Citation](citation.html): Name of the publisher of the citation 6152* [CodeSystem](codesystem.html): Name of the publisher of the code system 6153* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6154* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6155* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6156* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6157* [Evidence](evidence.html): Name of the publisher of the evidence 6158* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6159* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6160* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6161* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6162* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6163* [Library](library.html): Name of the publisher of the library 6164* [Measure](measure.html): Name of the publisher of the measure 6165* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6166* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6167* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6168* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6169* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6170* [Requirements](requirements.html): Name of the publisher of the requirements 6171* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6172* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6173* [StructureMap](structuremap.html): Name of the publisher of the structure map 6174* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6175* [TestScript](testscript.html): Name of the publisher of the test script 6176* [ValueSet](valueset.html): Name of the publisher of the value set 6177</b><br> 6178 * Type: <b>string</b><br> 6179 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6180 * </p> 6181 */ 6182 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 6183 public static final String SP_PUBLISHER = "publisher"; 6184 /** 6185 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6186 * <p> 6187 * Description: <b>Multiple Resources: 6188 6189* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6190* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6191* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6192* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6193* [Citation](citation.html): Name of the publisher of the citation 6194* [CodeSystem](codesystem.html): Name of the publisher of the code system 6195* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6196* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6197* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6198* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6199* [Evidence](evidence.html): Name of the publisher of the evidence 6200* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6201* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6202* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6203* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6204* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6205* [Library](library.html): Name of the publisher of the library 6206* [Measure](measure.html): Name of the publisher of the measure 6207* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6208* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6209* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6210* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6211* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6212* [Requirements](requirements.html): Name of the publisher of the requirements 6213* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6214* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6215* [StructureMap](structuremap.html): Name of the publisher of the structure map 6216* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6217* [TestScript](testscript.html): Name of the publisher of the test script 6218* [ValueSet](valueset.html): Name of the publisher of the value set 6219</b><br> 6220 * Type: <b>string</b><br> 6221 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6222 * </p> 6223 */ 6224 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 6225 6226 /** 6227 * Search parameter: <b>status</b> 6228 * <p> 6229 * Description: <b>Multiple Resources: 6230 6231* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6232* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6233* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6234* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6235* [Citation](citation.html): The current status of the citation 6236* [CodeSystem](codesystem.html): The current status of the code system 6237* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6238* [ConceptMap](conceptmap.html): The current status of the concept map 6239* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6240* [EventDefinition](eventdefinition.html): The current status of the event definition 6241* [Evidence](evidence.html): The current status of the evidence 6242* [EvidenceReport](evidencereport.html): The current status of the evidence report 6243* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6244* [ExampleScenario](examplescenario.html): The current status of the example scenario 6245* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6246* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6247* [Library](library.html): The current status of the library 6248* [Measure](measure.html): The current status of the measure 6249* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6250* [MessageDefinition](messagedefinition.html): The current status of the message definition 6251* [NamingSystem](namingsystem.html): The current status of the naming system 6252* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6253* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6254* [PlanDefinition](plandefinition.html): The current status of the plan definition 6255* [Questionnaire](questionnaire.html): The current status of the questionnaire 6256* [Requirements](requirements.html): The current status of the requirements 6257* [SearchParameter](searchparameter.html): The current status of the search parameter 6258* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6259* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6260* [StructureMap](structuremap.html): The current status of the structure map 6261* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6262* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6263* [TestPlan](testplan.html): The current status of the test plan 6264* [TestScript](testscript.html): The current status of the test script 6265* [ValueSet](valueset.html): The current status of the value set 6266</b><br> 6267 * Type: <b>token</b><br> 6268 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6269 * </p> 6270 */ 6271 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 6272 public static final String SP_STATUS = "status"; 6273 /** 6274 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6275 * <p> 6276 * Description: <b>Multiple Resources: 6277 6278* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6279* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6280* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6281* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6282* [Citation](citation.html): The current status of the citation 6283* [CodeSystem](codesystem.html): The current status of the code system 6284* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6285* [ConceptMap](conceptmap.html): The current status of the concept map 6286* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6287* [EventDefinition](eventdefinition.html): The current status of the event definition 6288* [Evidence](evidence.html): The current status of the evidence 6289* [EvidenceReport](evidencereport.html): The current status of the evidence report 6290* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6291* [ExampleScenario](examplescenario.html): The current status of the example scenario 6292* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6293* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6294* [Library](library.html): The current status of the library 6295* [Measure](measure.html): The current status of the measure 6296* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6297* [MessageDefinition](messagedefinition.html): The current status of the message definition 6298* [NamingSystem](namingsystem.html): The current status of the naming system 6299* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6300* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6301* [PlanDefinition](plandefinition.html): The current status of the plan definition 6302* [Questionnaire](questionnaire.html): The current status of the questionnaire 6303* [Requirements](requirements.html): The current status of the requirements 6304* [SearchParameter](searchparameter.html): The current status of the search parameter 6305* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6306* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6307* [StructureMap](structuremap.html): The current status of the structure map 6308* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6309* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6310* [TestPlan](testplan.html): The current status of the test plan 6311* [TestScript](testscript.html): The current status of the test script 6312* [ValueSet](valueset.html): The current status of the value set 6313</b><br> 6314 * Type: <b>token</b><br> 6315 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6316 * </p> 6317 */ 6318 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6319 6320 /** 6321 * Search parameter: <b>title</b> 6322 * <p> 6323 * Description: <b>Multiple Resources: 6324 6325* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6326* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6327* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6328* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6329* [Citation](citation.html): The human-friendly name of the citation 6330* [CodeSystem](codesystem.html): The human-friendly name of the code system 6331* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6332* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6333* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6334* [Evidence](evidence.html): The human-friendly name of the evidence 6335* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6336* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6337* [Library](library.html): The human-friendly name of the library 6338* [Measure](measure.html): The human-friendly name of the measure 6339* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6340* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6341* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6342* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6343* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6344* [Requirements](requirements.html): The human-friendly name of the requirements 6345* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6346* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6347* [StructureMap](structuremap.html): The human-friendly name of the structure map 6348* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6349* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6350* [TestScript](testscript.html): The human-friendly name of the test script 6351* [ValueSet](valueset.html): The human-friendly name of the value set 6352</b><br> 6353 * Type: <b>string</b><br> 6354 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6355 * </p> 6356 */ 6357 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 6358 public static final String SP_TITLE = "title"; 6359 /** 6360 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6361 * <p> 6362 * Description: <b>Multiple Resources: 6363 6364* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6365* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6366* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6367* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6368* [Citation](citation.html): The human-friendly name of the citation 6369* [CodeSystem](codesystem.html): The human-friendly name of the code system 6370* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6371* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6372* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6373* [Evidence](evidence.html): The human-friendly name of the evidence 6374* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6375* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6376* [Library](library.html): The human-friendly name of the library 6377* [Measure](measure.html): The human-friendly name of the measure 6378* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6379* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6380* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6381* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6382* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6383* [Requirements](requirements.html): The human-friendly name of the requirements 6384* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6385* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6386* [StructureMap](structuremap.html): The human-friendly name of the structure map 6387* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6388* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6389* [TestScript](testscript.html): The human-friendly name of the test script 6390* [ValueSet](valueset.html): The human-friendly name of the value set 6391</b><br> 6392 * Type: <b>string</b><br> 6393 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6394 * </p> 6395 */ 6396 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 6397 6398 /** 6399 * Search parameter: <b>url</b> 6400 * <p> 6401 * Description: <b>Multiple Resources: 6402 6403* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6404* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6405* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6406* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6407* [Citation](citation.html): The uri that identifies the citation 6408* [CodeSystem](codesystem.html): The uri that identifies the code system 6409* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6410* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6411* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6412* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6413* [Evidence](evidence.html): The uri that identifies the evidence 6414* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6415* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6416* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6417* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6418* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6419* [Library](library.html): The uri that identifies the library 6420* [Measure](measure.html): The uri that identifies the measure 6421* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6422* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6423* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6424* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6425* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6426* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6427* [Requirements](requirements.html): The uri that identifies the requirements 6428* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6429* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6430* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6431* [StructureMap](structuremap.html): The uri that identifies the structure map 6432* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6433* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6434* [TestPlan](testplan.html): The uri that identifies the test plan 6435* [TestScript](testscript.html): The uri that identifies the test script 6436* [ValueSet](valueset.html): The uri that identifies the value set 6437</b><br> 6438 * Type: <b>uri</b><br> 6439 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6440 * </p> 6441 */ 6442 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 6443 public static final String SP_URL = "url"; 6444 /** 6445 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6446 * <p> 6447 * Description: <b>Multiple Resources: 6448 6449* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6450* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6451* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6452* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6453* [Citation](citation.html): The uri that identifies the citation 6454* [CodeSystem](codesystem.html): The uri that identifies the code system 6455* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6456* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6457* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6458* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6459* [Evidence](evidence.html): The uri that identifies the evidence 6460* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6461* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6462* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6463* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6464* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6465* [Library](library.html): The uri that identifies the library 6466* [Measure](measure.html): The uri that identifies the measure 6467* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6468* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6469* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6470* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6471* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6472* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6473* [Requirements](requirements.html): The uri that identifies the requirements 6474* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6475* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6476* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6477* [StructureMap](structuremap.html): The uri that identifies the structure map 6478* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6479* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6480* [TestPlan](testplan.html): The uri that identifies the test plan 6481* [TestScript](testscript.html): The uri that identifies the test script 6482* [ValueSet](valueset.html): The uri that identifies the value set 6483</b><br> 6484 * Type: <b>uri</b><br> 6485 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6486 * </p> 6487 */ 6488 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6489 6490 /** 6491 * Search parameter: <b>version</b> 6492 * <p> 6493 * Description: <b>Multiple Resources: 6494 6495* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6496* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6497* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6498* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6499* [Citation](citation.html): The business version of the citation 6500* [CodeSystem](codesystem.html): The business version of the code system 6501* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6502* [ConceptMap](conceptmap.html): The business version of the concept map 6503* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6504* [EventDefinition](eventdefinition.html): The business version of the event definition 6505* [Evidence](evidence.html): The business version of the evidence 6506* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6507* [ExampleScenario](examplescenario.html): The business version of the example scenario 6508* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6509* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6510* [Library](library.html): The business version of the library 6511* [Measure](measure.html): The business version of the measure 6512* [MessageDefinition](messagedefinition.html): The business version of the message definition 6513* [NamingSystem](namingsystem.html): The business version of the naming system 6514* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6515* [PlanDefinition](plandefinition.html): The business version of the plan definition 6516* [Questionnaire](questionnaire.html): The business version of the questionnaire 6517* [Requirements](requirements.html): The business version of the requirements 6518* [SearchParameter](searchparameter.html): The business version of the search parameter 6519* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6520* [StructureMap](structuremap.html): The business version of the structure map 6521* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6522* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6523* [TestScript](testscript.html): The business version of the test script 6524* [ValueSet](valueset.html): The business version of the value set 6525</b><br> 6526 * Type: <b>token</b><br> 6527 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6528 * </p> 6529 */ 6530 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 6531 public static final String SP_VERSION = "version"; 6532 /** 6533 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6534 * <p> 6535 * Description: <b>Multiple Resources: 6536 6537* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6538* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6539* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6540* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6541* [Citation](citation.html): The business version of the citation 6542* [CodeSystem](codesystem.html): The business version of the code system 6543* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6544* [ConceptMap](conceptmap.html): The business version of the concept map 6545* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6546* [EventDefinition](eventdefinition.html): The business version of the event definition 6547* [Evidence](evidence.html): The business version of the evidence 6548* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6549* [ExampleScenario](examplescenario.html): The business version of the example scenario 6550* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6551* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6552* [Library](library.html): The business version of the library 6553* [Measure](measure.html): The business version of the measure 6554* [MessageDefinition](messagedefinition.html): The business version of the message definition 6555* [NamingSystem](namingsystem.html): The business version of the naming system 6556* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6557* [PlanDefinition](plandefinition.html): The business version of the plan definition 6558* [Questionnaire](questionnaire.html): The business version of the questionnaire 6559* [Requirements](requirements.html): The business version of the requirements 6560* [SearchParameter](searchparameter.html): The business version of the search parameter 6561* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6562* [StructureMap](structuremap.html): The business version of the structure map 6563* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6564* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6565* [TestScript](testscript.html): The business version of the test script 6566* [ValueSet](valueset.html): The business version of the value set 6567</b><br> 6568 * Type: <b>token</b><br> 6569 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6570 * </p> 6571 */ 6572 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 6573 6574 /** 6575 * Search parameter: <b>composed-of</b> 6576 * <p> 6577 * Description: <b>Multiple Resources: 6578 6579* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6580* [EventDefinition](eventdefinition.html): What resource is being referenced 6581* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6582* [Library](library.html): What resource is being referenced 6583* [Measure](measure.html): What resource is being referenced 6584* [PlanDefinition](plandefinition.html): What resource is being referenced 6585</b><br> 6586 * Type: <b>reference</b><br> 6587 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 6588 * </p> 6589 */ 6590 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6591 public static final String SP_COMPOSED_OF = "composed-of"; 6592 /** 6593 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 6594 * <p> 6595 * Description: <b>Multiple Resources: 6596 6597* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6598* [EventDefinition](eventdefinition.html): What resource is being referenced 6599* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6600* [Library](library.html): What resource is being referenced 6601* [Measure](measure.html): What resource is being referenced 6602* [PlanDefinition](plandefinition.html): What resource is being referenced 6603</b><br> 6604 * Type: <b>reference</b><br> 6605 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 6606 * </p> 6607 */ 6608 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 6609 6610/** 6611 * Constant for fluent queries to be used to add include statements. Specifies 6612 * the path value of "<b>EvidenceVariable:composed-of</b>". 6613 */ 6614 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("EvidenceVariable:composed-of").toLocked(); 6615 6616 /** 6617 * Search parameter: <b>depends-on</b> 6618 * <p> 6619 * Description: <b>Multiple Resources: 6620 6621* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6622* [EventDefinition](eventdefinition.html): What resource is being referenced 6623* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6624* [Library](library.html): What resource is being referenced 6625* [Measure](measure.html): What resource is being referenced 6626* [PlanDefinition](plandefinition.html): What resource is being referenced 6627</b><br> 6628 * Type: <b>reference</b><br> 6629 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 6630 * </p> 6631 */ 6632 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6633 public static final String SP_DEPENDS_ON = "depends-on"; 6634 /** 6635 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 6636 * <p> 6637 * Description: <b>Multiple Resources: 6638 6639* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6640* [EventDefinition](eventdefinition.html): What resource is being referenced 6641* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6642* [Library](library.html): What resource is being referenced 6643* [Measure](measure.html): What resource is being referenced 6644* [PlanDefinition](plandefinition.html): What resource is being referenced 6645</b><br> 6646 * Type: <b>reference</b><br> 6647 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 6648 * </p> 6649 */ 6650 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 6651 6652/** 6653 * Constant for fluent queries to be used to add include statements. Specifies 6654 * the path value of "<b>EvidenceVariable:depends-on</b>". 6655 */ 6656 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("EvidenceVariable:depends-on").toLocked(); 6657 6658 /** 6659 * Search parameter: <b>derived-from</b> 6660 * <p> 6661 * Description: <b>Multiple Resources: 6662 6663* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6664* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6665* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6666* [EventDefinition](eventdefinition.html): What resource is being referenced 6667* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6668* [Library](library.html): What resource is being referenced 6669* [Measure](measure.html): What resource is being referenced 6670* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6671* [PlanDefinition](plandefinition.html): What resource is being referenced 6672* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6673</b><br> 6674 * Type: <b>reference</b><br> 6675 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6676 * </p> 6677 */ 6678 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6679 public static final String SP_DERIVED_FROM = "derived-from"; 6680 /** 6681 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 6682 * <p> 6683 * Description: <b>Multiple Resources: 6684 6685* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6686* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 6687* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 6688* [EventDefinition](eventdefinition.html): What resource is being referenced 6689* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6690* [Library](library.html): What resource is being referenced 6691* [Measure](measure.html): What resource is being referenced 6692* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 6693* [PlanDefinition](plandefinition.html): What resource is being referenced 6694* [ValueSet](valueset.html): A resource that the ValueSet is derived from 6695</b><br> 6696 * Type: <b>reference</b><br> 6697 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 6698 * </p> 6699 */ 6700 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 6701 6702/** 6703 * Constant for fluent queries to be used to add include statements. Specifies 6704 * the path value of "<b>EvidenceVariable:derived-from</b>". 6705 */ 6706 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("EvidenceVariable:derived-from").toLocked(); 6707 6708 /** 6709 * Search parameter: <b>predecessor</b> 6710 * <p> 6711 * Description: <b>Multiple Resources: 6712 6713* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6714* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6715* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6716* [EventDefinition](eventdefinition.html): What resource is being referenced 6717* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6718* [Library](library.html): What resource is being referenced 6719* [Measure](measure.html): What resource is being referenced 6720* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6721* [PlanDefinition](plandefinition.html): What resource is being referenced 6722* [ValueSet](valueset.html): The predecessor of the ValueSet 6723</b><br> 6724 * Type: <b>reference</b><br> 6725 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6726 * </p> 6727 */ 6728 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6729 public static final String SP_PREDECESSOR = "predecessor"; 6730 /** 6731 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 6732 * <p> 6733 * Description: <b>Multiple Resources: 6734 6735* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6736* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 6737* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 6738* [EventDefinition](eventdefinition.html): What resource is being referenced 6739* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6740* [Library](library.html): What resource is being referenced 6741* [Measure](measure.html): What resource is being referenced 6742* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 6743* [PlanDefinition](plandefinition.html): What resource is being referenced 6744* [ValueSet](valueset.html): The predecessor of the ValueSet 6745</b><br> 6746 * Type: <b>reference</b><br> 6747 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 6748 * </p> 6749 */ 6750 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 6751 6752/** 6753 * Constant for fluent queries to be used to add include statements. Specifies 6754 * the path value of "<b>EvidenceVariable:predecessor</b>". 6755 */ 6756 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("EvidenceVariable:predecessor").toLocked(); 6757 6758 /** 6759 * Search parameter: <b>successor</b> 6760 * <p> 6761 * Description: <b>Multiple Resources: 6762 6763* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6764* [EventDefinition](eventdefinition.html): What resource is being referenced 6765* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6766* [Library](library.html): What resource is being referenced 6767* [Measure](measure.html): What resource is being referenced 6768* [PlanDefinition](plandefinition.html): What resource is being referenced 6769</b><br> 6770 * Type: <b>reference</b><br> 6771 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 6772 * </p> 6773 */ 6774 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6775 public static final String SP_SUCCESSOR = "successor"; 6776 /** 6777 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 6778 * <p> 6779 * Description: <b>Multiple Resources: 6780 6781* [ActivityDefinition](activitydefinition.html): What resource is being referenced 6782* [EventDefinition](eventdefinition.html): What resource is being referenced 6783* [EvidenceVariable](evidencevariable.html): What resource is being referenced 6784* [Library](library.html): What resource is being referenced 6785* [Measure](measure.html): What resource is being referenced 6786* [PlanDefinition](plandefinition.html): What resource is being referenced 6787</b><br> 6788 * Type: <b>reference</b><br> 6789 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 6790 * </p> 6791 */ 6792 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 6793 6794/** 6795 * Constant for fluent queries to be used to add include statements. Specifies 6796 * the path value of "<b>EvidenceVariable:successor</b>". 6797 */ 6798 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("EvidenceVariable:successor").toLocked(); 6799 6800 /** 6801 * Search parameter: <b>topic</b> 6802 * <p> 6803 * Description: <b>Multiple Resources: 6804 6805* [ActivityDefinition](activitydefinition.html): Topics associated with the module 6806* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 6807* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 6808* [EventDefinition](eventdefinition.html): Topics associated with the module 6809* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 6810* [Library](library.html): Topics associated with the module 6811* [Measure](measure.html): Topics associated with the measure 6812* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 6813* [PlanDefinition](plandefinition.html): Topics associated with the module 6814* [ValueSet](valueset.html): Topics associated with the ValueSet 6815</b><br> 6816 * Type: <b>token</b><br> 6817 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 6818 * </p> 6819 */ 6820 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 6821 public static final String SP_TOPIC = "topic"; 6822 /** 6823 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 6824 * <p> 6825 * Description: <b>Multiple Resources: 6826 6827* [ActivityDefinition](activitydefinition.html): Topics associated with the module 6828* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 6829* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 6830* [EventDefinition](eventdefinition.html): Topics associated with the module 6831* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 6832* [Library](library.html): Topics associated with the module 6833* [Measure](measure.html): Topics associated with the measure 6834* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 6835* [PlanDefinition](plandefinition.html): Topics associated with the module 6836* [ValueSet](valueset.html): Topics associated with the ValueSet 6837</b><br> 6838 * Type: <b>token</b><br> 6839 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 6840 * </p> 6841 */ 6842 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 6843 6844 6845} 6846